Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Check for conflicting accesses in
assert_is_system
(#8154)
# Objective The function `assert_is_system` is used in documentation tests to ensure that example code actually produces valid systems. Currently, `assert_is_system` just checks that each function parameter implements `SystemParam`. To further check the validity of the system, we should initialize the passed system so that it will be checked for conflicting accesses. Not only does this enforce the validity of our examples, but it provides a convenient way to demonstrate conflicting accesses via a `should_panic` example, which is nicely rendered by rustdoc: 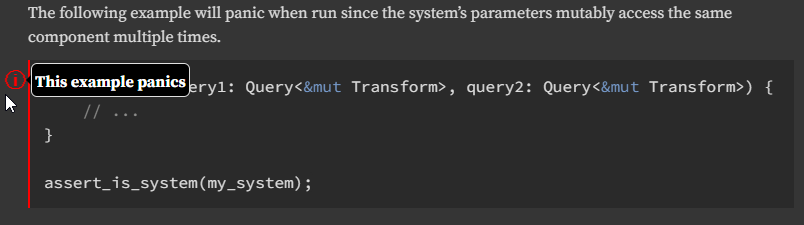 ## Solution Initialize the system with an empty world to trigger its internal access conflict checks. --- ## Changelog The function `bevy::ecs::system::assert_is_system` now panics when passed a system with conflicting world accesses, as does `assert_is_read_only_system`. ## Migration Guide The functions `assert_is_system` and `assert_is_read_only_system` (in `bevy_ecs::system`) now panic if the passed system has invalid world accesses. Any tests that called this function on a system with invalid accesses will now fail. Either fix the system's conflicting accesses, or specify that the test is meant to fail: 1. For regular tests (that is, functions annotated with `#[test]`), add the `#[should_panic]` attribute to the function. 2. For documentation tests, add `should_panic` to the start of the code block: ` ```should_panic`
- Loading branch information