-
Notifications
You must be signed in to change notification settings - Fork 36
List
Eonist edited this page Mar 20, 2017
·
2 revisions
Case study of the List element
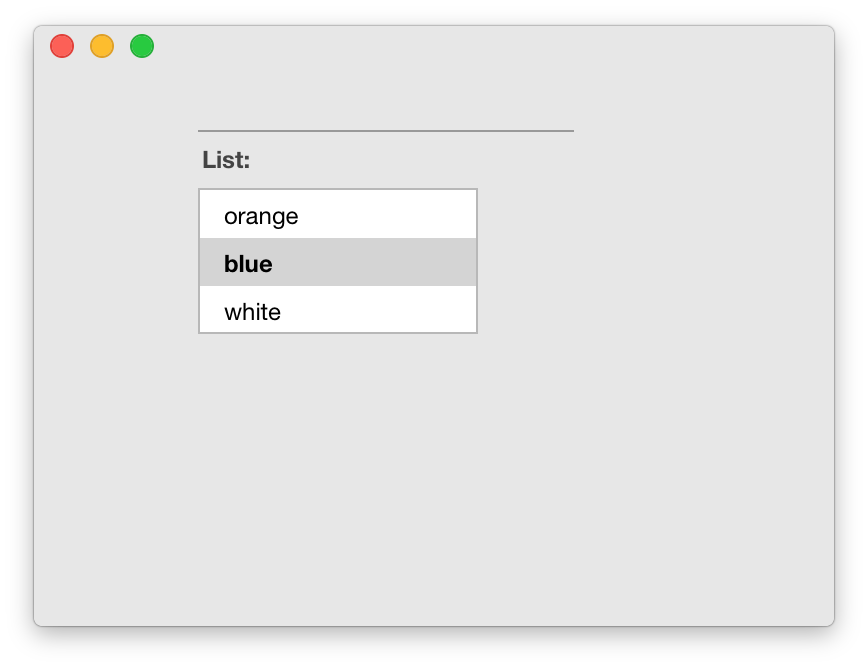
swift:
var listSection:Section = stage.addSubView(Section(CGFloat.NaN, CGFloat.NaN, "List: ", self, "listSection")) as! Section
let dp = DataProvider(FileParser.xml("~/Desktop/test.xml"))/*Loads xml from a xml file on the desktop*/
var list:List = listSection.addSubView(List(140, 72, CGFloat.NaN, dp,listSection)) as! List
ListModifier.selectAt(list, 1)/*Selects the second item list*/
list.dataProvider.addItemAt(["title":"brown"], 0)/*adds a new item at index 0*/
list.dataProvider.addItem(["title":"pink"])/*adds a new item to the end of the list*/
list.dataProvider.addItems([["title":"purple"], ["title":"turquoise"]])/*adds 2 items to the end of the list*/
list.dataProvider.removeItem(list.dataProvider.getItem("brown")!)/*remove the item with title brown*/
list.dataProvider.removeItemAt(0)/*remove the first item in the list*/
Swift.print("Selected: " + "\(ListParser.selected(list))")/*print the selected item*/
Swift.print("Selected index: " + "\(ListParser.selectedIndex(list))")/*print the index of the selected item*/
Swift.print("Selected title: " + "\(ListParser.selectedTitle(list))")/*print the title of the selected item*/
list.dataProvider.removeAll()/*removes all the items in the list*/
Alternate way of adding data to the dataProvider:
let orange:Dictionary<String,String> = ["title":"orange", "property":"fruit"]
let blue = ["title":"blue", "property":"0x00FF00"]
let red = ["title":"white", "property":"0xFFFFFF"]
let dp:DataProvider = DataProvider([orange,blue,red])
XML Structure for adding data to the dataProvider:
<items>
<item title="orange" property="harry"/>
<item title="blue" property="na"/>
<item title="white" property="spring"/>
<item title="purple" property="dark"/>
<item title="turquoise" property="1"/>
</items>
CSS:
List{
float:left;
clear:left;
fill:white;
line:white9;
line-alpha:1;
line-offset-type:outside;
line-thickness:1px;
drop-shadow:<InsetShadow>;
}
List Container{
float:left;
width:100%;
}
List Container SelectTextButton{
float:left;
clear:left;
width:100%;
height:24px;
fill:white;
fill-alpha:0;
line:white9;
line-alpha:1;
line-thickness:1px;
line-offset-type:outside;
padding-bottom:-1px;
padding-left,padding-right:-1px;
margin-bottom:-1px;
drop-shadow:none;
}
List Container SelectTextButton:selected{
fill:#B9B9B9;
}
List Container SelectTextButton Text{
margin-left:12px;
margin-top:2;
width:100%;
height:24px;
autoSize:left;
color:grey5;
bold:false;
selectable,mouseEnabled:false;
}
List Container SelectTextButton:selected Text{
bold:true;
}
- Start testing
- implement the event system to the list component
- ==Implement an extension that has fileURL init methods and other useful inits for the DataProvider==
- Use a foreground background skin scheme to get the right look when using the inset shadow
- complete the list utility methods, add test for these
- when you click outside an item the item should be deselected? This functionality can be added through subclassing
- Select the first item on init? This functionality can be added through subclassing
- ==implement the sorting algorithm for views==
- Add the slider component to the SliderList class
- do research into the scroll wheel gesture (delta etc)
- recap how the legacy code did the scroll stuff.
- get the scrollbar working
- get a fake view being scrolled working
- get SliderList working
- Look into rubber-band animation for the scrollbar
- implement sortOn