-
Notifications
You must be signed in to change notification settings - Fork 4.9k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
ios: update demos to display errors (#192)
Update the Swift and Objective-C demos to follow the UI logic implemented by envoyproxy/envoy-mobile#166. Resolves envoyproxy/envoy-mobile#168. 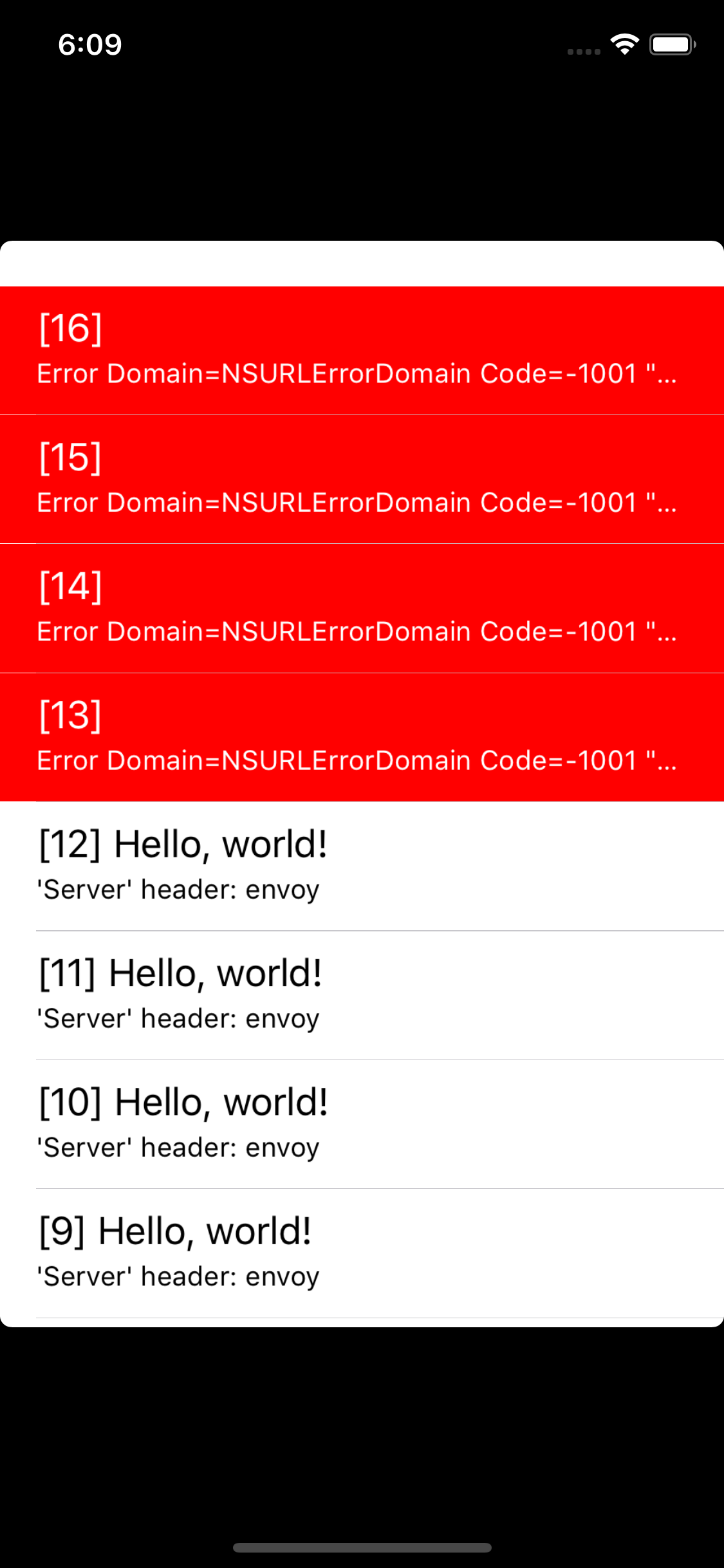 Signed-off-by: Michael Rebello <[email protected]> Signed-off-by: JP Simard <[email protected]>
- Loading branch information
Showing
5 changed files
with
168 additions
and
76 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file was deleted.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,12 @@ | ||
/// Represents a response from the server. | ||
struct Response { | ||
let id: Int | ||
let body: String | ||
let serverHeader: String | ||
} | ||
|
||
/// Error that was encountered when executing a request. | ||
struct RequestError: Error { | ||
let id: Int | ||
let message: String | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters