-
Notifications
You must be signed in to change notification settings - Fork 3.2k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Incorrect results returned when joining a key-less view to an entity #21607
Comments
Also, we setup the data as the following: var blog1 = new Blog {Url = "a"};
var blog2 = new Blog {Url = "b"};
var blog3 = new Blog {Url = "c"};
var blog4 = new Blog {Url = "d"};
var blog5 = new Blog {Url = "e"};
await context.AddAsync(blog1);
await context.AddAsync(blog2);
await context.AddAsync(blog3);
await context.AddAsync(blog4);
await context.AddAsync(blog5);
await context.SaveChangesAsync();
var blog2post1 = new Post {PostId = 1, BlogId = blog2.BlogId};
await context.AddAsync(blog2post1);
var blog3post1 = new Post { PostId = 1, BlogId = blog3.BlogId };
await context.AddAsync(blog3post1);
var blog5post1 = new Post { PostId = 1, BlogId = blog5.BlogId };
await context.AddAsync(blog5post1);
await context.SaveChangesAsync(); and execute the following query: var blogs = context.BlogView.Select(blog => new
{
BlogId = blog.BlogId,
BlogUrl = blog.Url,
Titles = context.Posts
.Where(post => post.BlogId == blog.BlogId)
.Select(post => post.PostId)
.ToArray(),
})
.OrderBy(x => x.BlogId)
.ToArray(); This returns incorrect lists for the root objects |
@smitpatel to follow up. |
We should block the scenario. |
@ajcvickers / @smitpatel Thanks for a fast update on the issue 👍. |
poaching |
…ew to an entity Disabling the scenario when we try to add collection join to keyless entity parent, since we don't have identifying columns to properly bucket the results. Fixes #21607
…ew to an entity Disabling the scenario when we try to add collection join to keyless entity parent, since we don't have identifying columns to properly bucket the results. Fixes #21607
…ew to an entity Disabling the scenario when we try to add collection join to keyless entity parent, since we don't have identifying columns to properly bucket the results. Fixes #21607
…ew to an entity Disabling the scenario when we try to add collection join to keyless entity parent, since we don't have identifying columns to properly bucket the results. Fixes #21607
…ew to an entity Disabling the scenario when we try to add collection join to keyless entity parent, since we don't have identifying columns to properly bucket the results. Fixes #21607
When trying to join a key-less view to an entity the incorrect results are returned and sometimes the join returns another row's results.
Steps to reproduce
Executing the following code:
Returns the following results.
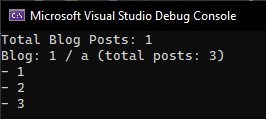
Profiling SQL Server we get the following executed:
This seems all fine and executing it, it returns the correct results as expected.
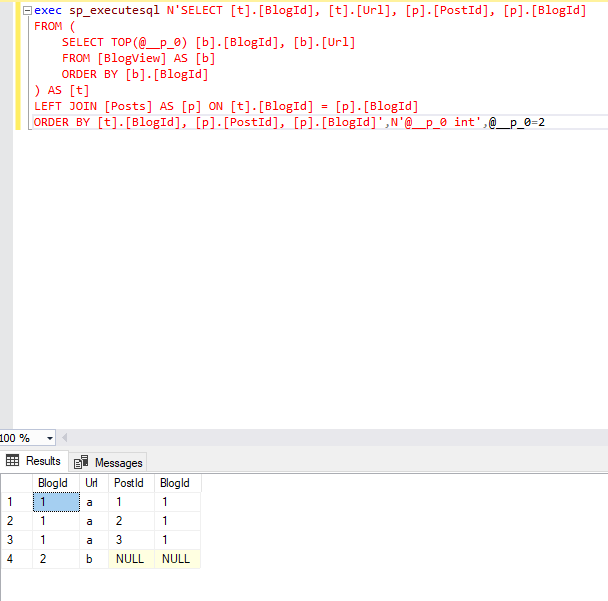
If we change the view to be a keyed entity, by configuring the model builder as the following
This generates exactly the same SQL.
However, the output from the console app is the following:
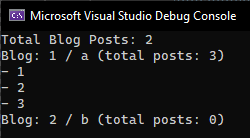
Further technical details
EF Core version: 3.1.5
Database provider: Microsoft.EntityFrameworkCore.SqlServer
Target framework: .NET Core 3.1
Operating system: Windows 10
The text was updated successfully, but these errors were encountered: