forked from rust-lang/rust
-
Notifications
You must be signed in to change notification settings - Fork 7
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Auto merge of rust-lang#11557 - bruno-ortiz:rust-dependencies, r=brun…
…o-ortiz Creating rust dependencies tree explorer Hello! I tried to implement a tree view that shows the dependencies of a project. It allows to see all dependencies to the project and it uses `cargo tree` for it. Also it allows to click and open the files, the viewtree tries its best to follow the openned file in the editor. Here is an example: 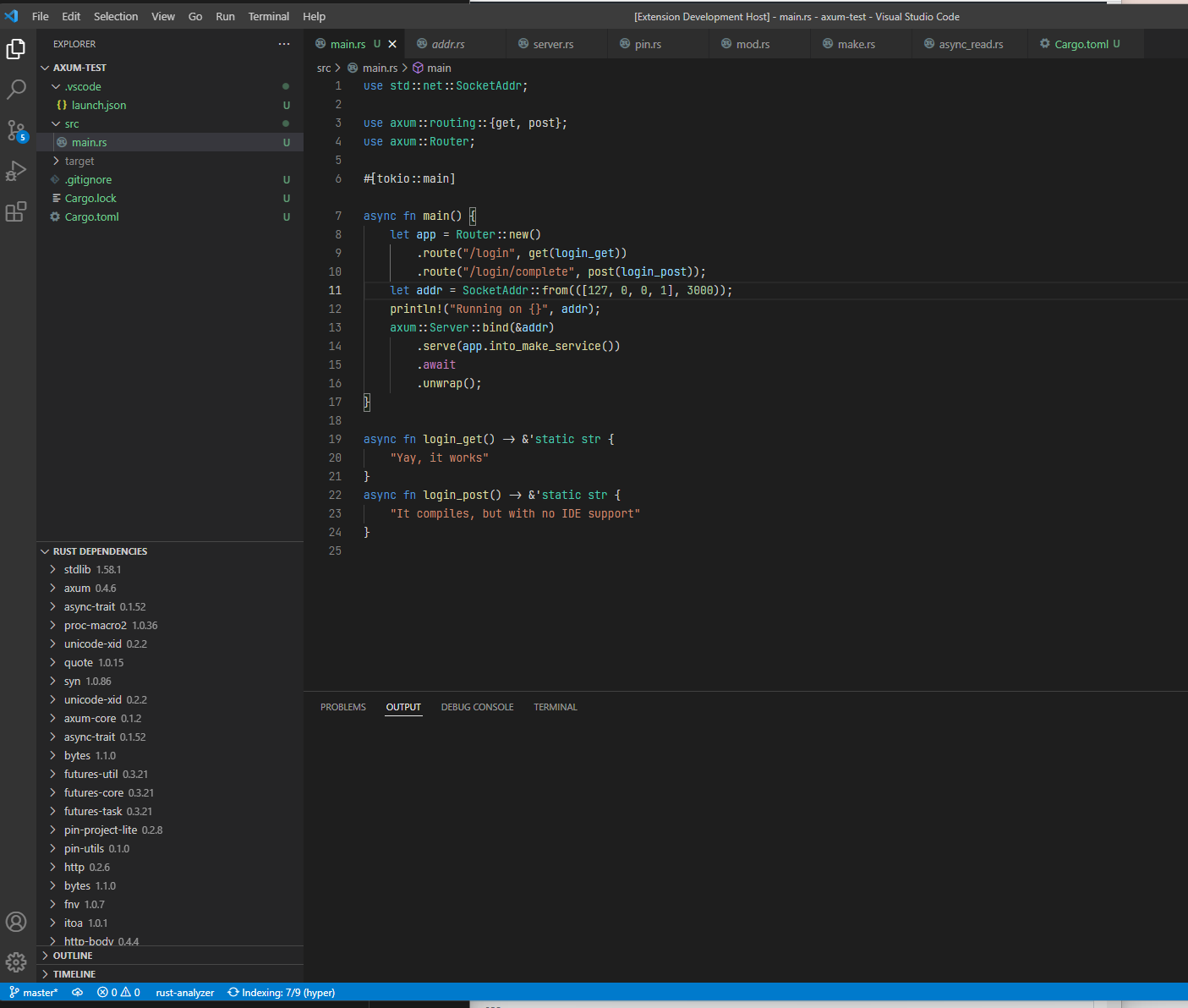 Any feedback is welcome since i have basically no professional experience with TS.
- Loading branch information
Showing
17 changed files
with
522 additions
and
13 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
use ide_db::{ | ||
base_db::{CrateOrigin, FileId, SourceDatabase}, | ||
FxIndexSet, RootDatabase, | ||
}; | ||
|
||
#[derive(Debug, PartialEq, Eq, PartialOrd, Ord, Hash)] | ||
pub struct CrateInfo { | ||
pub name: Option<String>, | ||
pub version: Option<String>, | ||
pub root_file_id: FileId, | ||
} | ||
|
||
// Feature: Show Dependency Tree | ||
// | ||
// Shows a view tree with all the dependencies of this project | ||
// | ||
// |=== | ||
// image::https://user-images.githubusercontent.com/5748995/229394139-2625beab-f4c9-484b-84ed-ad5dee0b1e1a.png[] | ||
pub(crate) fn fetch_crates(db: &RootDatabase) -> FxIndexSet<CrateInfo> { | ||
let crate_graph = db.crate_graph(); | ||
crate_graph | ||
.iter() | ||
.map(|crate_id| &crate_graph[crate_id]) | ||
.filter(|&data| !matches!(data.origin, CrateOrigin::Local { .. })) | ||
.map(|data| crate_info(data)) | ||
.collect() | ||
} | ||
|
||
fn crate_info(data: &ide_db::base_db::CrateData) -> CrateInfo { | ||
let crate_name = crate_name(data); | ||
let version = data.version.clone(); | ||
CrateInfo { name: crate_name, version, root_file_id: data.root_file_id } | ||
} | ||
|
||
fn crate_name(data: &ide_db::base_db::CrateData) -> Option<String> { | ||
data.display_name.as_ref().map(|it| it.canonical_name().to_owned()) | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.