fix(core): snap the text pos of cloneNode to grid(#1545) #1550
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
fix #1545
fix #1440 => related: transform相关问题汇总
问题发生的原因
在
menu
鼠标右键点击复制时,会触发最终执行逻辑也就是
this.graphModel.cloneNode(nodeId)
从上面代码可以知道,复制
node
时会增加30
左右的偏移量,然后触发this.addNode()
进行克隆节点的复制从上面
this.addNode()
方法可以知道,会对坐标进行一定的修正,也就是下面代码所示因此问题就出在,当
this.gridSize
不为1时,node.x
和node.y
会根据this.gridSize
进行一定的修正,但是node.text.x
和node.text.y
却没有进行根据this.gridSize
进行一定的修正,导致了位置错误而在
GraphModel.ts
的其它方法中,我们可以发现一些相似的代码,如下面代码所示在执行
snapToGrid()
之后,是有进行node.text.x -= getGridOffset(nodeX, this.gridSize)
,因此#1545
的问题就在于,缺失了node.text.x
和node.text.y
根据this.gridSize
进行一定坐标修正的逻辑解决方法
node.text
进行gridSize
的处理将
graphDataToModel()
对node.text.x
和node.text.y
的处理补充到addNode()
优化1
我们可以发现
graphDataToModel()
和addNode()
中创建new Model(nodeOriginData, this)
的代码一模一样,因此可以抽离出一个公共方法优化2
我们发现一个问题,当初始化参数
grid=true
,此时this.gridSize=20
nodeX=29
时,snapToGrid(29, 20)=20
,getGridOffset(29, 20)=9
,node
坐标从29->20
,减少了9
,node.text
坐标也减少了9,这样结果是正确的nodeX=31
时,snapToGrid(31, 20)=40
,getGridOffset(31, 20)=11
,node
坐标从31->40
,增加了9
,node.text
坐标由于是node.text.x -= getGridOffset(nodeX, this.gridSize)
,因此减少了11,这样的结果是错的因此使用
Math.round()
这种四舍五入的做法,我们无法判断到底该node.text.x -= getGridOffset(nodeX, this.gridSize)
还是node.text.x += getGridOffset(nodeX, this.gridSize)
单测
1545-spec.test.ts
简单模拟了下buggraphmodel.test.ts
对新增方法getModelAfterSnapToGrid()
进行测试未解决的问题
虽然单元测试能够
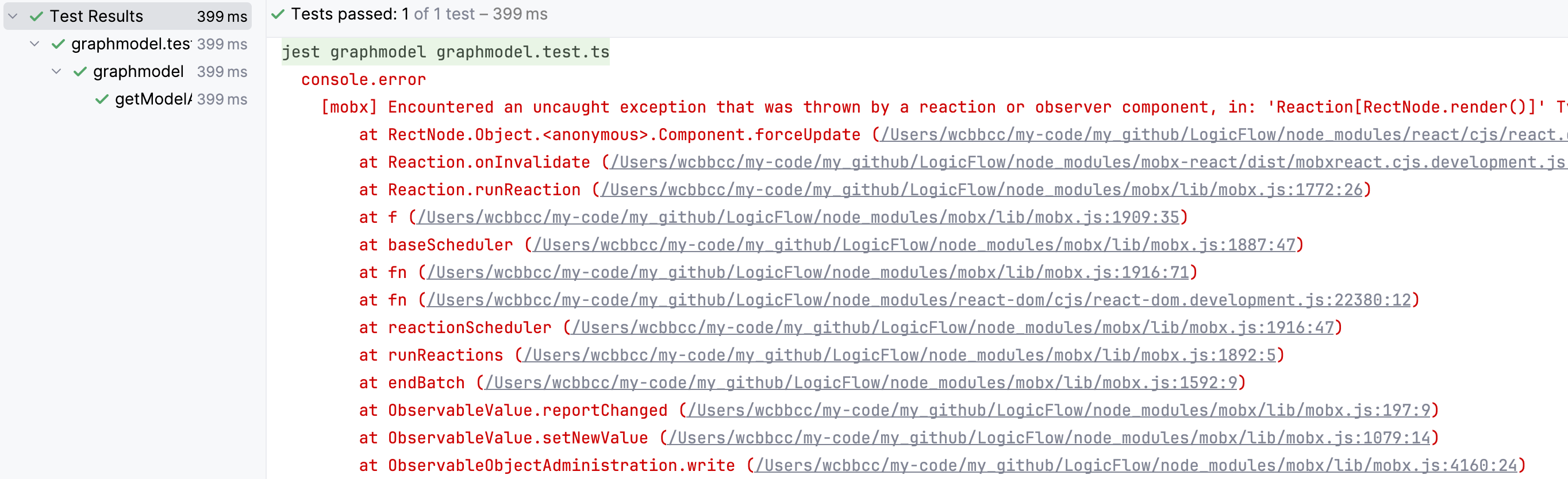
Pass
,但是最后会报错测试了其它已经存在的单元测试,比如
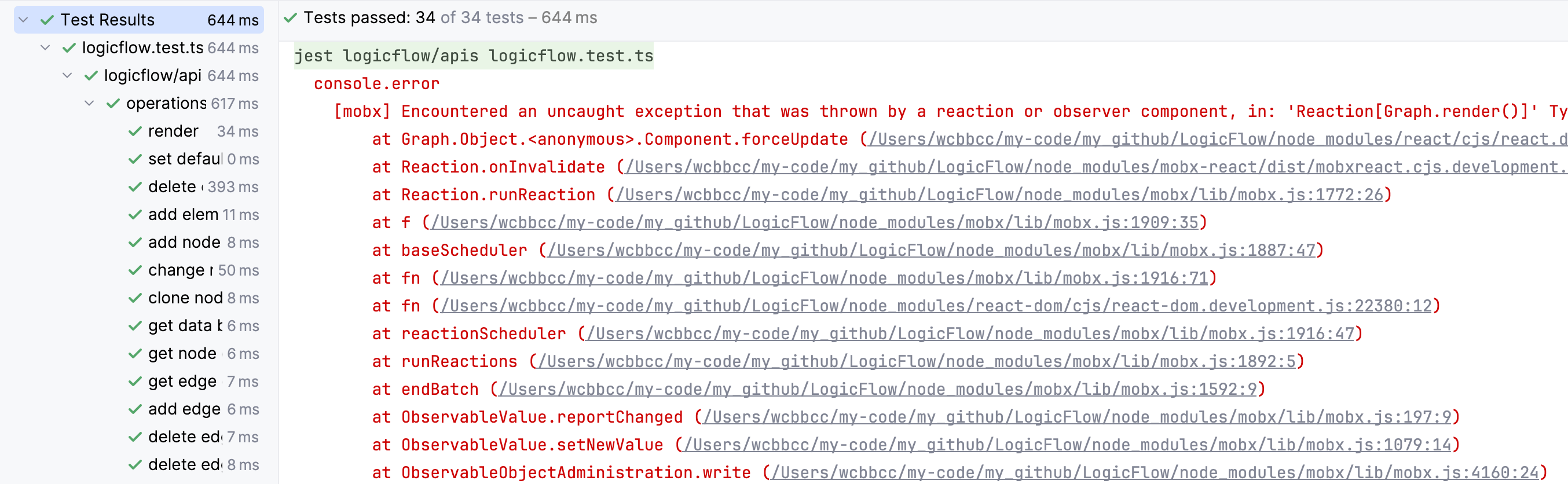
packages/core/__tests__/logicflow.test.ts
,也存在这个问题暂时还没找到解决方法,后面有空的时候可能要集中处理下