forked from gniziemazity/2d-vectors
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
8 changed files
with
727 additions
and
168 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,11 @@ | ||
# 2d-vectors | ||
2D vectors explained using JavaScript | ||
# About the project | ||
|
||
My little version of Radu's [Learn 2D Vectors with JavaScript](https://github.com/gniziemazity/2d-vectors) explanation: | ||
|
||
[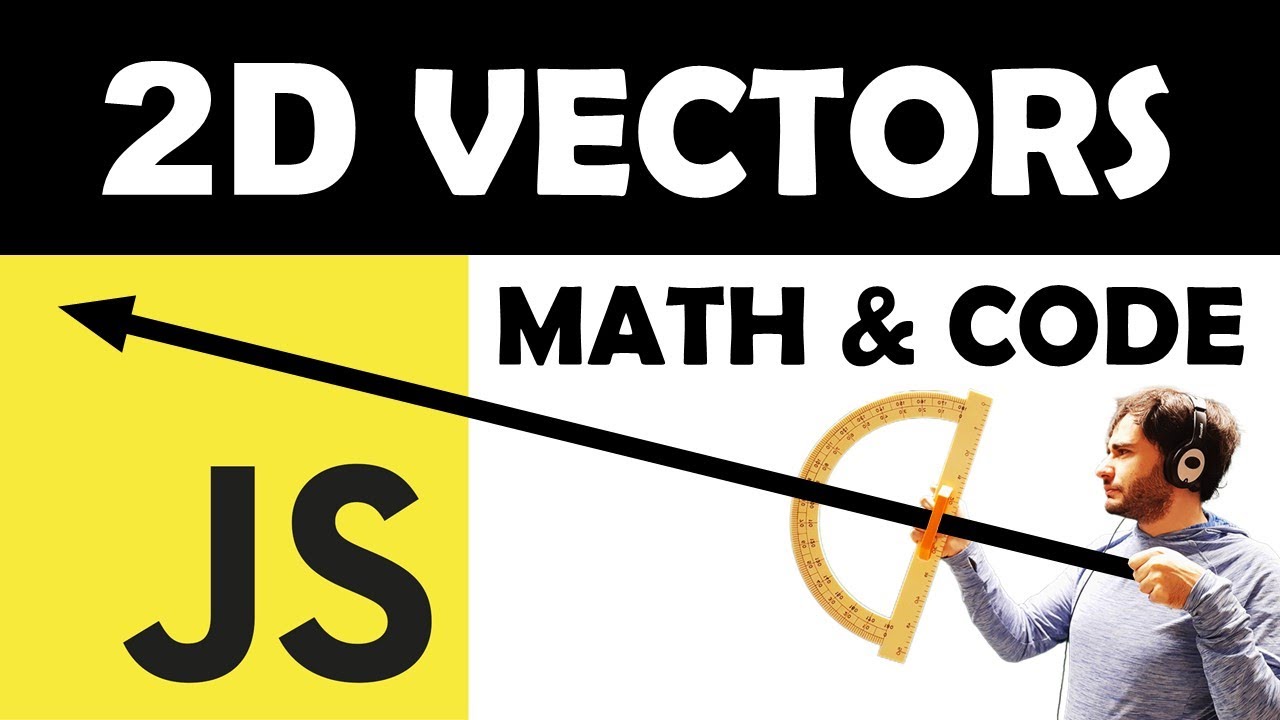](https://www.youtube.com/watch?v=nzyOCd9FcCA&ab_channel=RaduMariescu-Istodor) | ||
|
||
It was a great way to have fun and reinforce my knowledge of vanilla JavaScript and its [module](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) implementation and [OOP](https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Classes_in_JavaScript) abstractions. Also, it was an extraordinary opportunity to be able to work with the [Canvas API](https://developer.mozilla.org/en-US/docs/Web/API/Canvas_API) and initial concepts of linear algebra and vectors. | ||
|
||
## Author | ||
|
||
- [LinkedIn](https://www.linkedin.com/in/cristian-marcelo-de-picciotto/) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,294 @@ | ||
/** | ||
* | ||
* @description Bootstrap | ||
* | ||
* @author C. M. de Picciotto <[email protected]> | ||
* | ||
* {@link https://www.youtube.com/watch?v=nzyOCd9FcCA&ab_channel=RaduMariescu-Istodor} | ||
* | ||
*/ | ||
import GraphicVectorRenderer from "./canvas/coordinate-system/renderer/graphic-vector-renderer.js"; | ||
import CoordinateSystem from "./canvas/coordinate-system.js"; | ||
|
||
export default class Bootstrap { | ||
/** | ||
* | ||
* @type {HTMLCanvasElement} | ||
* | ||
*/ | ||
#canvas; | ||
|
||
/** | ||
* | ||
* @type {GraphicVector} | ||
* | ||
*/ | ||
#point; | ||
|
||
/** | ||
* | ||
* @type {GraphicVector} | ||
* | ||
*/ | ||
#G; | ||
|
||
/** | ||
* | ||
* @type {CanvasRenderingContext2D} | ||
* | ||
*/ | ||
#context; | ||
|
||
/** | ||
* | ||
* @type {Object} | ||
* | ||
*/ | ||
#offset; | ||
|
||
/** | ||
* | ||
* @type {CoordinateSystem} | ||
* | ||
*/ | ||
#coordinateSystem; | ||
|
||
/** | ||
* | ||
* @type {GraphicVector[]} | ||
* | ||
*/ | ||
#vectors; | ||
|
||
/** | ||
* | ||
* Constructor | ||
* | ||
* @param {HTMLCanvasElement} canvas | ||
* @param {GraphicVector} point | ||
* @param {GraphicVector} G | ||
* | ||
*/ | ||
constructor(canvas, point, G) { | ||
this.#canvas = canvas; | ||
this.#point = point; | ||
this.#G = G; | ||
this.#initOffset(); | ||
this.#initContext(); | ||
this.#initCoordinateSystem(); | ||
this.#initEvents(); | ||
} | ||
|
||
/** | ||
* | ||
* Run | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
run() { | ||
/** | ||
* | ||
* @note Init vectors to render | ||
* | ||
*/ | ||
this.#vectors = []; | ||
|
||
/** | ||
* | ||
* @note Add vectors | ||
* | ||
*/ | ||
const resultAdd = this.#add() | ||
|
||
/** | ||
* | ||
* @note Subtract vectors | ||
* | ||
*/ | ||
this.#subtract() | ||
|
||
/** | ||
* | ||
* @note Subtract vectors. Move result to subtrahend tail | ||
* | ||
*/ | ||
this.#subtract().moveToTail(this.#G); | ||
|
||
/** | ||
* | ||
* @note Scale vector | ||
* | ||
*/ | ||
this.#scale(); | ||
|
||
/** | ||
* | ||
* @note Add init vectors | ||
* | ||
*/ | ||
this.#vectors.push(this.#point); | ||
this.#vectors.push(this.#G); | ||
|
||
/** | ||
* | ||
* @note Draw coordinate system | ||
* | ||
*/ | ||
this.#drawCoordinateSystem(); | ||
|
||
/** | ||
* | ||
* @note Draw parallelogram rule | ||
* | ||
*/ | ||
this.#drawParallelogram(resultAdd); | ||
} | ||
|
||
/** | ||
* | ||
* Add | ||
* | ||
* @returns {GraphicVector} | ||
* | ||
*/ | ||
#add() { | ||
const resultAdd = this.#point.add(this.#G); | ||
this.#addVector(resultAdd); | ||
return resultAdd; | ||
} | ||
|
||
/** | ||
* | ||
* Subtract | ||
* | ||
* @returns {GraphicVector} | ||
* | ||
*/ | ||
#subtract() { | ||
/** | ||
* | ||
* @note Subtract vectors | ||
* | ||
*/ | ||
const resultSub = this.#point.subtract(this.#G); | ||
this.#addVector(resultSub); | ||
return resultSub; | ||
} | ||
|
||
/** | ||
* | ||
* Scale | ||
* | ||
* @returns {GraphicVector} | ||
* | ||
*/ | ||
#scale() { | ||
const resultSub = this.#subtract(); | ||
const scaledSub = resultSub.normalize().scale(50); | ||
this.#addVector(scaledSub); | ||
return scaledSub; | ||
} | ||
|
||
/** | ||
* | ||
* Draw coordinate system | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
#drawCoordinateSystem() { | ||
this.#coordinateSystem.addVectors(this.#vectors); | ||
this.#coordinateSystem.draw(); | ||
} | ||
|
||
/** | ||
* | ||
* Draw parallelogram rule | ||
* | ||
* @param {GraphicVector} resultAdd | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
#drawParallelogram(resultAdd) { | ||
this.#context.beginPath(); | ||
this.#context.setLineDash([3,3]); | ||
this.#context.moveTo(this.#G.x, this.#G.y); | ||
this.#context.lineTo(resultAdd.x,resultAdd.y); | ||
this.#context.lineTo(this.#point.x, this.#point.y); | ||
this.#context.stroke(); | ||
this.#context.setLineDash([]); | ||
} | ||
|
||
/** | ||
* | ||
* Init offset | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
#initOffset() { | ||
this.#offset = { | ||
x: this.#canvas.width/2, | ||
y: this.#canvas.height/2 | ||
} | ||
} | ||
|
||
/** | ||
* | ||
* Init context | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
#initContext() { | ||
this.#context = this.#canvas.getContext("2d"); | ||
} | ||
|
||
/** | ||
* | ||
* Init coordinate system | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
#initCoordinateSystem() { | ||
/** | ||
* | ||
* @note Init coordinate system | ||
* | ||
*/ | ||
const vectorRenderer = new GraphicVectorRenderer(this.#context); | ||
this.#coordinateSystem = new CoordinateSystem(this.#context, vectorRenderer, this.#offset.x, this.#offset.y); | ||
} | ||
|
||
/** | ||
* | ||
* Init events | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
#initEvents() { | ||
document.onmousemove = (event) => { | ||
this.#point.x = event.x - this.#offset.x; | ||
this.#point.y = event.y - this.#offset.y; | ||
this.run(); | ||
} | ||
} | ||
|
||
/** | ||
* | ||
* Add vector | ||
* | ||
* @param {GraphicVector} vector | ||
* | ||
* @returns {void} | ||
* | ||
*/ | ||
#addVector(vector) { | ||
vector.color = 'red'; | ||
this.#vectors.push(vector); | ||
} | ||
} |
Oops, something went wrong.