generated from jeffrafter/honk-action
-
Notifications
You must be signed in to change notification settings - Fork 0
/
honk.ts
49 lines (42 loc) · 1.62 KB
/
honk.ts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
import * as core from '@actions/core'
import * as github from '@actions/github'
const run = async (): Promise<void> => {
try {
const token = process.env['HONK_USER_TOKEN'] || process.env['GITHUB_TOKEN'] || core.getInput('token')
if (!token || token === '') return
// Create the octokit client
const octokit: github.GitHub = new github.GitHub(token)
const nwo = process.env['GITHUB_REPOSITORY'] || '/'
const [owner, repo] = nwo.split('/')
const issue = github.context.payload['issue']
if (!issue) return
const comment = github.context.payload.comment
const commentBody = comment.body
if (commentBody.match(/honk/i)) return
// Delete the comment
// https://octokit.github.io/rest.js/#octokit-routes-issues-delete-comment
const deleteCommentResponse = await octokit.issues.deleteComment({
owner,
repo,
comment_id: comment.id,
})
console.log(`Deleted comment! ${JSON.stringify(deleteCommentResponse.status)}`)
// Add a new comment that says honk
// https://octokit.github.io/rest.js/#octokit-routes-issues-create-comment
const issueCommentResponse = await octokit.issues.createComment({
owner,
repo,
issue_number: issue.number,
body: '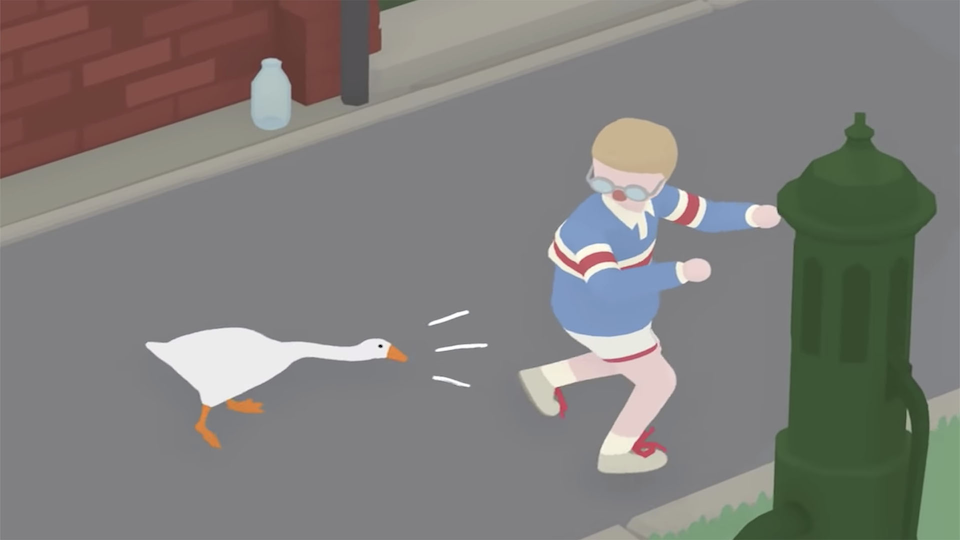',
})
console.log(`Honk! ${issueCommentResponse.data.url}`)
} catch (error) {
console.error(error.message)
core.setFailed(`Honk-action failure: ${error}`)
}
}
// Don't auto-execute in the test environment
if (process.env['NODE_ENV'] !== 'test') {
run()
}
export default run