@@ -90,46 +42,21 @@ export const MyComponent = ({ title }) => (
);
```
-
- MDX does not compile inside the body of an arrow function. Stick to HTML
- syntax when you can or use a default export if you need to use MDX.
-
-
-2. Import the snippet into your destination file and pass in the props
-
-```typescript destination-file.mdx
----
-title: My title
-description: My Description
----
-
-import { MyComponent } from '/snippets/custom-component.mdx';
-
-Lorem ipsum dolor sit amet.
-
-
-```
+
+ MDX doesn't compile inside arrow functions. Use HTML syntax or default exports for MDX content.
+
### Client-Side Content
-By default, Mintlify employs server-side rendering, generating content
-at build time. For client-side content loading, ensure to verify the
-`document` object's availability before initiating the rendering process.
-
+For client-side rendering, check for `document` availability:
```typescript snippets/client-component.mdx
-{/* `setTimeout` simulates a React.useEffect, which is called after the component is mounted. */}
export const ClientComponent = () => {
- if (typeof document === "undefined") {
- return null;
- } else {
- setTimeout(() => {
- const clientComponent = document.getElementById("client-component");
- if (clientComponent) {
- clientComponent.innerHTML = "Hello, client-side component!";
- }
- }, 1);
-
- return
- }
-}
-```
+ if (typeof document === "undefined") return null;
+
+ setTimeout(() => {
+ const element = document.getElementById("client-component");
+ if (element) element.innerHTML = "Hello, client-side component!";
+ }, 1);
+
+ return
;
+}
\ No newline at end of file
diff --git a/settings/add-members.mdx b/settings/add-members.mdx
index 3db06c9..3fd0b87 100644
--- a/settings/add-members.mdx
+++ b/settings/add-members.mdx
@@ -4,14 +4,14 @@ description: 'Allow more members of your team to update your docs'
icon: 'user-group-simple'
---
-The team member who created your initial docs will have update access to your docs, as long as they push to your documentation repo with the same GitHub account that was used while signing up for Mintlify.
+The initial docs creator has update access when using the same GitHub account used during Mintlify signup.
-If another editor attempts to update the docs while on the free plan, you will see a warning in your git commit check.
+Free plan users will see a warning in their git commit check when other editors attempt to update the docs.

-In the details of the git check warning, you'll find the link to upgrade your plan. You can also upgrade your plan on the [dashboard](https://dashboard.mintlify.com) to enable unlimited editors to update your docs. Once you upgrade your plan, trigger a manual update or push another change to deploy your updates.
+To enable unlimited editors, upgrade your plan via the git check warning link or the [dashboard](https://dashboard.mintlify.com). After upgrading, trigger a manual update or push a change to deploy updates.
-Learn more about our pricing [here](https://mintlify.com/pricing).
+For pricing details, visit our [pricing page](https://mintlify.com/pricing).
\ No newline at end of file
diff --git a/settings/authentication.mdx b/settings/authentication.mdx
index 38de095..1f2ac7e 100644
--- a/settings/authentication.mdx
+++ b/settings/authentication.mdx
@@ -4,11 +4,10 @@ description: "Customize how your team can login to Mintlify"
icon: 'user-unlock'
---
-Mintlify supports single sign-on to your dashboard via SAML and OIDC. If you use Okta or Google Workspace, we have provider-specific documentation for setting up SSO, but if you use another provider, please contact us!
+Mintlify supports SSO via SAML and OIDC for enterprise customers. We offer streamlined setup for Okta and Google Workspace.
- SSO functionality is available on our Enterprise plan. [Contact
- us](https://mintlify.com/enterprise) to learn more!
+ SSO is available on our Enterprise plan. [Contact us](https://mintlify.com/enterprise) to learn more!
## Okta
@@ -16,38 +15,35 @@ Mintlify supports single sign-on to your dashboard via SAML and OIDC. If you use
-
- Under `Applications`, click to create a new app integration using SAML 2.0.
+
+ Create a new app integration using SAML 2.0 under `Applications`.
-
- Enter the following:
- * Single sign-on URL (provided by Mintlify)
- * Audience URI (provided by Mintlify)
+
+ Configure with:
+ * Single sign-on URL (from Mintlify)
+ * Audience URI (from Mintlify)
* Name ID Format: `EmailAddress`
- * Attribute Statements:
- | Name | Name format | Value
- | ---- | ----------- | -----
+ * Attributes:
+ | Name | Format | Value |
+ | ---- | ------ | ----- |
| `firstName` | Basic | `user.firstName` |
| `lastName` | Basic | `user.lastName` |
-
- Once the application is set up, navigate to the sign-on tab and send us the metadata URL.
- We'll enable the connection from our side using this information.
+
+ Send us your metadata URL from the sign-on tab for connection enablement.
-
- Under `Applications`, click to create a new app integration using OIDC.
- You should choose the `Web Application` application type.
+
+ Create a new OIDC `Web Application` under `Applications`.
-
- Select the authorization code grant type and enter the Redirect URI provided by Mintlify.
+
+ Set authorization code grant type and Mintlify-provided Redirect URI.
-
- Once the application is set up, navigate to the General tab and locate the client ID & client secret.
- Please securely provide us with these, along with your Okta instance URL (e.g. `.okta.com`). You can send these via a service like 1Password or SendSafely.
+
+ Securely share your client ID, client secret, and Okta instance URL (`.okta.com`).
@@ -58,38 +54,27 @@ Mintlify supports single sign-on to your dashboard via SAML and OIDC. If you use
-
- Under `Web and mobile apps`, select `Add custom SAML app` from the `Add app` dropdown.
-
- 
-
+
+ Select `Add custom SAML app` under `Web and mobile apps`.
-
- Copy the provided SSO URL, Entity ID, and x509 certificate and send it to the Mintlify team.
-
- 
-
+
+ Provide us the SSO URL, Entity ID, and x509 certificate.
-
- On the Service provider details page, enter the following:
- * ACS URL (provided by Mintlify)
- * Entity ID (provided by Mintlify)
+
+ Enter service provider details:
+ * ACS URL (from Mintlify)
+ * Entity ID (from Mintlify)
* Name ID format: `EMAIL`
* Name ID: `Basic Information > Primary email`
-
- 
-
-
- On the next page, enter the following attribute statements:
- | Google Directory Attribute | App Attribute |
- | -------------------------- | ------------- |
- | `First name` | `firstName` |
+ Add attributes:
+ | Google Directory | App Attribute |
+ | ---------------- | ------------- |
+ | `First name` | `firstName` |
| `Last name` | `lastName` |
- Once this step is complete and users are assigned to the application, let our team know and we'll enable SSO for your account!
+ After user assignment, contact us to enable SSO.
-
-
+
\ No newline at end of file
diff --git a/settings/custom-domain.mdx b/settings/custom-domain.mdx
index 015e845..726670e 100644
--- a/settings/custom-domain.mdx
+++ b/settings/custom-domain.mdx
@@ -4,41 +4,15 @@ description: "Host the documentation at your website's custom subdomain"
icon: 'globe'
---
-To set up your documentation on a custom subdomain, you'll need to set your desired custom subdomain in your Mintlify settings and configure your DNS settings on your domain provider.
-
- Looking to set up a custom subdirectory domain like mintlify.com/docs? Find
- instructions [here](/advanced/subpath/cloudflare).
+ For custom subdirectory domains (e.g., mintlify.com/docs), see instructions [here](/advanced/subpath/cloudflare).
-## Dashboard Settings
-
-1. Head over to your [dashboard project](https://dashboard.mintlify.com)
-2. Click on the "Add custom subdomain".
-3. Enter your desired custom subdomain. For example, `docs.mintlify.com`.
-
-
-

-

-
-
-## Verification with Vercel
-
-If Vercel happens to be your domain provider, you will have to add a verification `TXT` record. This information will show on your dashboard after submitting your custom subdomain, as well as be emailed to you.
-
-## Configuring your DNS
-
-1. Proceed to your domain's DNS settings on your domain provider.
-2. Create a new DNS entry, inputting the following values:
+## Setup Steps
+1. Go to your [dashboard project](https://dashboard.mintlify.com)
+2. Click "Add custom subdomain" and enter your desired subdomain (e.g., `docs.mintlify.com`)
+3. Add the following DNS record at your domain provider:
```
CNAME | docs | cname.vercel-dns.com.
```
@@ -47,6 +21,8 @@ CNAME | docs | cname.vercel-dns.com.
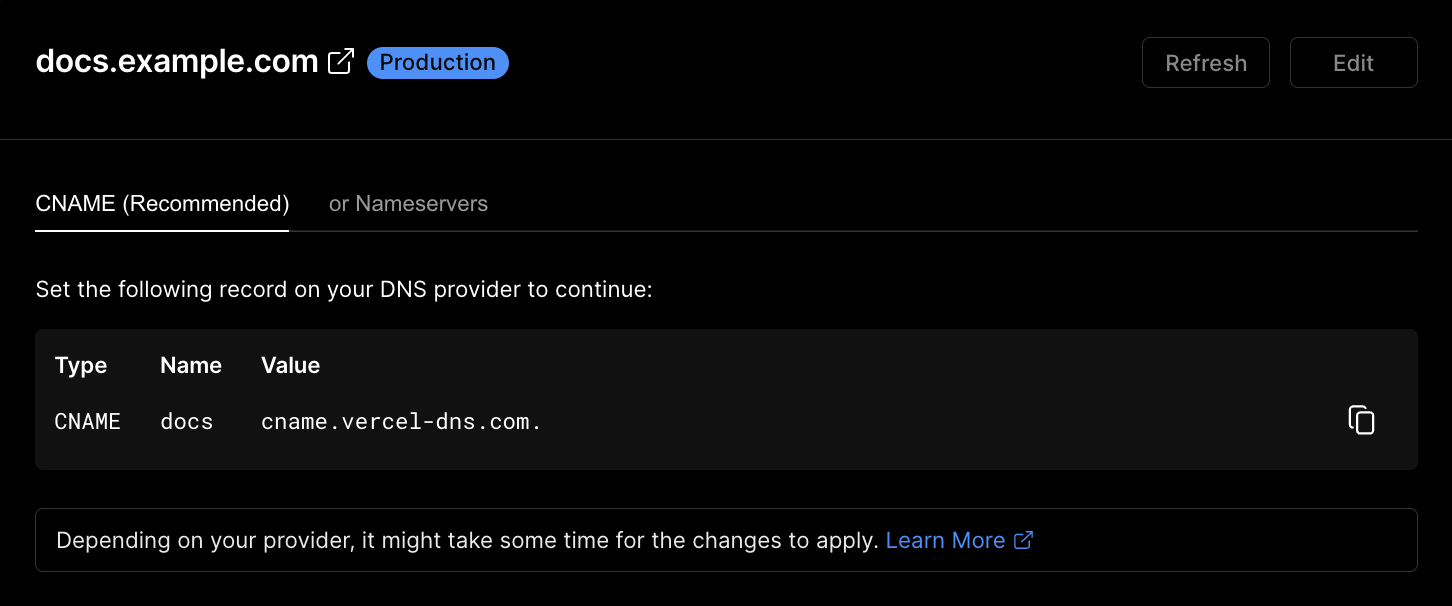
-If you are using Cloudflare for your DNS provider, you'll need to have the “full strict” security option enabled for the https setting.
+**Note:**
+- Vercel users need to add a verification `TXT` record (details provided in dashboard)
+- Cloudflare users must enable "full strict" security for https settings
-Please [contact support](mailto:sales@mintlify.com) if you don't see the custom subdomain set up after the above configuration.
+Need help? [Contact support](mailto:sales@mintlify.com)
\ No newline at end of file
diff --git a/settings/github.mdx b/settings/github.mdx
index 310499c..53abb0e 100644
--- a/settings/github.mdx
+++ b/settings/github.mdx
@@ -4,23 +4,13 @@ description: "Sync your docs with a GitHub repo"
icon: 'github'
---
-Mintlify integrates with the GitHub API, enabling synchronization between your
-docs and your GitHub repository. This integration is made possible through the
-utilization of
-[GitHub Apps](https://docs.github.com/en/developers/apps/getting-started-with-apps/about-apps#about-github-apps).
+Mintlify integrates with GitHub through a [GitHub App](https://docs.github.com/en/developers/apps/getting-started-with-apps/about-apps#about-github-apps) to sync your documentation with your repository.
## Installation
-To begin, you need to install the Mintlify GitHub App into the GitHub account
-where your docs repo resides. Installing a GitHub App requires either
-organization ownership or admin permissions in a repository. If you lack the
-necessary permissions, the repository owner will need to approve the request.
-You can access the installation page by logging into the
-[Mintlify dashboard](https://dashboard.mintlify.com).
-
-For GitHub Apps, you can choose to only give permissions to a single repository.
-We highly recommend you do so as we only need access to the repository where
-your docs are hosted.
+1. Install the Mintlify GitHub App through the [Mintlify dashboard](https://dashboard.mintlify.com)
+2. Select the repository where your docs are hosted
+3. Grant the required permissions

@@ -28,35 +18,16 @@ your docs are hosted.
## Permissions
-During the installation of our GitHub app, you will be prompted to grant certain
-permissions:
-
-- Read and write access to `checks`, `contents`, `deployments`, `pull requests`,
- and `workflows`
-
-These permissions are leveraged to provide a seamless experience when managing
-your docs.
+The GitHub App requires the following permissions:
+- Read and write access to `checks`, `contents`, `deployments`, `pull requests`, and `workflows`
-When you make a commit to the branch you configured as your docs deployment
-branch, we fetch the contents of the files changed to update your docs. To
-accomplish this we need read access to your `contents`.
-
-When pull requests are created we create a check and preview deployment which is
-why we need write access to `checks` and `deployments`.
-
-Inside the Mintlify web editor, Mintlify works on your behalf to create branches
-and pull requests which is why we need write access to `pull requests` and
-`workflows`.
+These permissions enable:
+- Fetching and updating doc content
+- Creating preview deployments for pull requests
+- Managing branches and pull requests through the Mintlify web editor
- If you are concerned about the write permissions, the GitHub App will only
- have access to the repos you give it access to _and_ if you have branch
- protections on it _cannot_ push directly to your branches - it abides by your
- branch protection rules.
+ The GitHub App only has access to repositories you explicitly grant it access to and follows your branch protection rules.
-## Repositories Installations
-
-When installing our GitHub app, you will be prompted to select all repositories
-or a subset of them. This selection can be changed at any time by going to the
-[GitHub app settings](https://github.com/apps/mintlify/installations/new).
+You can modify repository access anytime in your [GitHub app settings](https://github.com/apps/mintlify/installations/new).
\ No newline at end of file
diff --git a/settings/gitlab.mdx b/settings/gitlab.mdx
index 576ba17..1c070a6 100644
--- a/settings/gitlab.mdx
+++ b/settings/gitlab.mdx
@@ -9,90 +9,33 @@ icon: "gitlab"
plan](https://mintlify.com/pricing).
-We use a combination of Access tokens and Webhooks to authenticate and sync
-changes between GitLab and Mintlify.
+Mintlify uses Access tokens for pulling information from GitLab and Webhooks for receiving notifications about repository changes.
-- We use Access tokens to pull information from GitLab.
-- We use Webhooks so GitLab can notify Mintlify when changes are made.
- - This allows Mintlify to create preview deployments when a MR is created.
-
-## Set up the connection
+## Setup Guide
-
- Within your GitLab project, navigate to `Settings` > `General` and find the `Project ID`.
-
-
-
-
-
- a. Navigate to `Settings` > `Access Tokens`.
-
- b. Select `Add new token`.
- 1. Name the token "Mintlify".
- 2. If you have a private repo, you must set the role as `Maintainer`.
- 3. Choose `api` and `read_api` for the scopes.
-
- c. Finally click `Create project access token` and copy the token.
-
-
-
-
-
-
-
- Within the [Mintlify dashboard](https://dashboard.mintlify.com/mintlify/mintlify/settings/deployment/git-settings), add the project ID and access token from the previous steps alongside the other configurations. Click "Save Changes" when you're done.
-
-
-
-
-
-
-## Create the webhook
-
-Webhooks allow us to receive events when changes are made so that we can
-automatically trigger deployments.
-
-
-
-
-
-
-
-
- In the "URL" field, enter the endpoint `https://leaves.mintlify.com/gitlab-webhook` and name the webhook "Mintlify".
-
-
-
- Paste the Webhook token generated after setting up the connection.
-
-
-
-
-
- Select the events you want to trigger the webhook:
+
+ 1. Find your Project ID under `Settings` > `General`
+ 2. Generate an access token:
+ - Go to `Settings` > `Access Tokens`
+ - Create a token named "Mintlify"
+ - For private repos: Set role as `Maintainer`
+ - Select `api` and `read_api` scopes
+
+
+ Add your project ID and access token in the [Mintlify dashboard](https://dashboard.mintlify.com/mintlify/mintlify/settings/deployment/git-settings).
+
+
+ 1. Go to `Settings` > `Webhooks`
+ 2. Add new webhook with URL: `https://leaves.mintlify.com/gitlab-webhook`
+ 3. Enter the Webhook token from Mintlify dashboard
+ 4. Enable:
- Push events (All branches)
- Merge requests events
-
- When you're done it should look like this:
-
-
-
-
-
- After creating the Webhook, click the "Test" dropdown and select "Push events" to send a sample payload to ensure it's configured correctly. It'll say "Hook executed successfully: HTTP 200" if configured correctly.
-
- This will help you verify that everything is working correctly and that your documentation will sync properly with your GitLab repository.
-
-
-
-
+ 5. Test the webhook using "Push events" test
- Reach out to the Mintlify team if you need help. Contact us
- [here](https://mintlify.com/enterprise).
-
-
-[git-settings]: https://dashboard.mintlify.com/settings/deployment/git-settings
+ Need help? Contact us [here](https://mintlify.com/enterprise).
+
\ No newline at end of file
diff --git a/settings/versioning.mdx b/settings/versioning.mdx
index dbafd45..63a4164 100644
--- a/settings/versioning.mdx
+++ b/settings/versioning.mdx
@@ -4,22 +4,15 @@ description: 'Build separate versions'
icon: 'square-chevron-down'
---
-These guides will assume `v1` pages are in a folder named `v1`, `v2` pages are in a folder named `v2`, and so on. While this method of structuring your files isn't strictly necessary, it's a great way to keep your files organized.
-
## Setup
-Add `"versions": ["v2", "v1"]` to your `mint.json` file where `v1` and `v2` are the names of your versions. You can put any number of versions in this array. The first version from the array serves as the default version.
-
-
- The versions dropdown will show your versions in the order you include them in
- `mint.json`.
-
+1. Structure your docs with version folders (e.g., `v1`, `v2`)
+2. Add versions to `mint.json`: `"versions": ["v2", "v1"]` (first version is default)
-## Versioning Groups and Pages
+## Implementation
-The best way to specify page versions is by adding a version value to a group in the navigation. When you specify the version of a group, that version is applied to all pages within that group.
-
-You can also specify the version of a single page in the page metadata. Versions on individual pages always take precedence.
+### Groups and Pages
+Add versions to groups in navigation or individual pages:
```json Groups (mint.json)
@@ -40,75 +33,33 @@ version: 'v2'
```
-
- While it is possible to nest versioned groups within versioned groups, it is not recommended. If you do take this approach, the more deeply-nested version takes precedence.
-
-
-### Versioning Tabs and Anchors
-
-You can hide a tab or anchor based on a version. This is useful if you have links that are only relevant in one version. Importantly, this does **not** apply the version to the pages within that anchor.
+### Tabs and Anchors
+Control version visibility in navigation:
-In `mint.json`, simply add `version` to your tab or anchor. Tabs and anchors without a specified version are shown in every version.
-
-
-```json Tabs
+```json mint.json
"tabs": [
{
"name": "API Reference V1",
"url": "v1/api-reference",
"version": "v1"
- },
- {
- "name": "API Reference V2",
- "url": "v2/api-reference",
- "version": "v2"
- },
- {
- "name": "Migrating from V1 to V2",
- "url": "https://mintlify.com/changelog/v2"
- },
-],
-```
-
-```json Anchors
-"anchors": [
- {
- "name": "API Reference V1",
- "url": "v1/api-reference",
- "version": "v1"
- },
- {
- "name": "API Reference V2",
- "url": "v2/api-reference",
- "version": "v2"
- },
- {
- "name": "Migrating from V1 to V2",
- "url": "https://mintlify.com/changelog/v2",
- "version": "v2"
- },
-],
+ }
+]
```
-
-### Sharing Between Versions
-
-Not _all_ content has to be hidden though! Any content without a specified version appears in every version so you don't have to duplicate content!
+
+- Content without a version appears in all versions
+- Page versions override group versions
+- Avoid nesting versioned groups
+
## Troubleshooting
-Common errors and how to fix them
-
- You likely nested a version inside of another. For example, your group had version "v1" but your page had version "v2".
-
- We do not recommend nesting versions inside of each other because it's hard to maintain your docs later.
+ Check for conflicting nested versions between groups and pages.
- If you add versions to your docs and the pages disappeared from your
- navigation, make sure you spelled the version the same as in your `versions`
- array in `mint.json`.
+ Verify version names match exactly with those in `mint.json`.
-
+
\ No newline at end of file
diff --git a/table.mdx b/table.mdx
index 1ccea1e..f1715c9 100644
--- a/table.mdx
+++ b/table.mdx
@@ -3,28 +3,13 @@ title: "Tables"
description: "Display an arrangement of data in rows and columns"
---
-| Property | Description |
-| -------- | ------------------------------------- |
-| Name | Full name of user |
-| Age | Reported age |
-| Joined | Whether the user joined the community |
-
-## Table
-
-### Creating a table
-
-
-
-The Table component follows the official [markdown syntax](https://www.markdownguide.org/extended-syntax/#tables).
-
-
+## Creating a Table
-To add a table, use three or more hyphens (`---`) to create each column's header, and use pipes (`|`) to separate each column. For compatibility, you should also add a pipe on either end of the row.
+Tables follow standard [markdown syntax](https://www.markdownguide.org/extended-syntax/#tables). Create them using pipes (`|`) to separate columns and three or more hyphens (`---`) for headers.
-```md
+Example:
| Property | Description |
| -------- | ------------------------------------- |
| Name | Full name of user |
| Age | Reported age |
-| Joined | Whether the user joined the community |
-```
+| Joined | Whether the user joined the community |
\ No newline at end of file
diff --git a/text.mdx b/text.mdx
index 45cec91..2e6f6dc 100644
--- a/text.mdx
+++ b/text.mdx
@@ -5,7 +5,6 @@ icon: 'heading'
---
## Titles
-
Best used for section headers.
```md
@@ -13,7 +12,6 @@ Best used for section headers.
```
### Subtitles
-
Best used for subsection headers.
```md
@@ -21,14 +19,11 @@ Best used for subsection headers.
```
-
-Each **title** and **subtitle** creates an anchor and also shows up on the table of contents on the right.
-
+Each **title** and **subtitle** creates an anchor and appears in the table of contents.
## Text Formatting
-
-We support most markdown formatting. Simply add `**`, `_`, or `~` around text to format it.
+Common markdown formatting uses `**`, `_`, or `~` around text:
| Style | How to write it | Result |
| ------------- | ----------------- | --------------- |
@@ -36,9 +31,9 @@ We support most markdown formatting. Simply add `**`, `_`, or `~` around text to
| Italic | `_italic_` | _italic_ |
| Strikethrough | `~strikethrough~` | ~strikethrough~ |
-You can combine these. For example, write `**_bold and italic_**` to get **_bold and italic_** text.
+Combinations like `**_bold and italic_**` create **_bold and italic_** text.
-You need to use HTML to write superscript and subscript text. That is, add `
` or `` around your text.
+For superscript and subscript, use HTML tags:
| Text Size | How to write it | Result |
| ----------- | ------------------------ | ---------------------- |
@@ -46,61 +41,33 @@ You need to use HTML to write superscript and subscript text. That is, add `subscript` | subscript |
## Linking to Pages
+Create links with `[]()`: `[link to google](https://google.com)` shows as [link to google](https://google.com).
-You can add a link by wrapping text in `[]()`. You would write `[link to google](https://google.com)` to [link to google](https://google.com).
-
-Links to pages in your docs need to be root-relative. Basically, you should include the entire folder path. For example, `[link to text](/content/text)` links to the page "Text" in our components section.
+For internal docs, use root-relative paths: `[link to text](/content/text)`. Avoid relative links like `[link to text](../text)`.
-Relative links like `[link to text](../text)` will open slower because we cannot optimize them as easily.
-
-You can validate broken links in your docs with [our CLI](/development).
+You can validate links using [our CLI](/development).
## Blockquotes
-
-### Singleline
-
-To create a blockquote, add a `>` in front of a paragraph.
-
-> Dorothy followed her through many of the beautiful rooms in her castle.
-
-```md
-> Dorothy followed her through many of the beautiful rooms in her castle.
-```
-
-### Multiline
+Add `>` for blockquotes:
> Dorothy followed her through many of the beautiful rooms in her castle.
->
-> The Witch bade her clean the pots and kettles and sweep the floor and keep the fire fed with wood.
-```md
+For multiline quotes, use multiple `>`:
> Dorothy followed her through many of the beautiful rooms in her castle.
>
> The Witch bade her clean the pots and kettles and sweep the floor and keep the fire fed with wood.
-```
-
-### LaTeX
-Mintlify supports in-line [LaTeX](https://www.latex-project.org) by surrounding your LaTeX code with dollar signs (\$). For example, `$(a^2 + b^2 = c^2)$` will render as $(a^2 + b^2 = c^2)$.
-
-Equations on their own line can be created with double dollar signs (\$\$):
+## LaTeX
+Use \$ for inline LaTeX: `$(a^2 + b^2 = c^2)$` renders as $(a^2 + b^2 = c^2)$.
+Use \$\$ for equation blocks:
$$\exists \, x \notin [0,1]$$
-```md
-$$\exists \, x \notin [0,1]$$
-```
-
-### Line Breaks
-
-Markdown syntax also recognizes a double enter in your MDX as a linebreak.
-
-```html
-
-```
+## Line Breaks
+Use double enter or `
` for line breaks:
```md
Paragraph 1
Paragraph 2
-```
+```
\ No newline at end of file
diff --git a/web-editor.mdx b/web-editor.mdx
index 25de127..67f0d1c 100644
--- a/web-editor.mdx
+++ b/web-editor.mdx
@@ -4,289 +4,46 @@ description: 'Edit your docs directly from the dashboard with live previews.'
---
- Mintlify Web Editor is currently in beta. We are in the process of adding additional
- features and fixing bugs. We'd love to get your feedback on what we can improve at [support@mintlify.com](mailto:support@mintlify.com).
+ Mintlify Web Editor is currently in beta. Contact [support@mintlify.com](mailto:support@mintlify.com) for feedback.
-Web Editor is the preferred way to edit docs directly without having to open your IDE or run `mintlify dev`.
+Web Editor allows you to edit docs directly without using an IDE or running `mintlify dev`. It includes git workflow features and a fully editable experience with custom components.
-The editor includes a few key features to integrate directly into your existing git workflow,
-like creating branches, pull requests, commits, and diffs for your current changes.
+## Git Workflow
-It also includes a fully editable experience for changing and adding content directly,
-even with custom components.
-
-If you understand git workflows and our integrations already, you can skip to [here](#editing-content).
-
-## Git and update workflows
-
-### Git basics
-
-While Web Editor means you don’t need to go to GitHub or your command line to make
-changes, it’s still helpful to know the basics of git.
-
-Git terminology:
-
-- **Repository**: The folder in which your code lives. It can be local (on your computer) or remote (like GitHub).
-
-- **Commit**: A snapshot of changes made to files in the repository.
-
-- **Branch**: A separate line of development. It's a working copy of the code that allows you to work on changes without affecting the main version.
-
-- **Pull request:** A request to merge changes from a working branch into the main branch. This is used for reviewing content before making changes live.
-
-### Making updates
-
-In order to make updates to your docs, we include a few buttons specifically to
-integrate with your git workflow.
+1. **Create a Branch**: Click the branches modal on the top left to create or switch branches.
+2. **Make Changes**: Edit your content using the editor.
+3. **Commit Changes**:
+ - On main branch: Click "Publish"
+ - On other branches: Click "Save Changes" or "Publish Pull Request"
- If you haven't done so already, please install the Mintlify GitHub app to your GitHub account.
- You can find [documentation here](#1-deploying-your-docs-repository), or you can install
- the app in the [GitHub App page](https://dashboard.mintlify.com/settings/organization/github-app)
- page of the dashboard.
+ Install the Mintlify GitHub app from the [GitHub App page](https://dashboard.mintlify.com/settings/organization/github-app) before making changes.
-
-
- In order to make changes to your docs, you might want to change from the main branch
- to avoid publishing directly to your main docs site.
-
- To do this, you can open the branches modal on the top left of the editor.
-
-
-
-
-
-
- From here, you can either switch to a different git branch than `main`, or you can
- create a new branch by clicking the **"New Branch"** button.
-
-
-
-
-
-
- After you create a new branch, you'll automatically be switched, and all changes
- you make will be made to this new branch until you change branches again or reload the page.
-
- By default, when you load the page again, you'll default to the main branch.
-
-
- As a best practice, you should always create a new branch to make changes so you can submit a pull request for review by other teammates. You also may not have permissions to make changes to the main branch, in which case we'll try to open a pull request for you.
-
-
-
-
- In order to make a commit, you have two options, both of which appear on the top
- right of the editor:
-
-
-
- If you're on the main branch of your docs repository, you can push a commit
- directly to the repo by clicking the **"Publish"** button. You'll see your changes
- reflect in your git branch the next time you run `git pull`.
-
-
-
-
-
-
-
-
- If you're not on the main branch, you can push a commit to your branch by clicking
- on the **"Save Changes"** button. If you're ready to publish a pull request to put your branch
- up for review, you can click the **"Publish Pull Request"** button.
-
-
-
-
-
-
-
-
- This will create the pull request for you on GitHub using the branch you selected onto
- your main branch.
-
-
-
- If you do put your pull request up for review, you can edit the title and description
- of the PR, but a default Mintlify title will be provided for you if you leave it
- empty.
-
-
-
-
-
-
-
-
-## Editing content
-
-### Slash commands
-
-The easiest way to add content in the editor is by using **"Slash commands"**, which are
-commands you have access to after typing `/` in the **"Visual Editor"** mode:
-
-
-
-
-
-
-As you type, you'll see more options pop up:
-
-
-
-
-
-
-#### Content blocks
-
-Here are the types of content blocks available to add in the **"Visual Editor"**:
-
-
-
-
- Paragraph
- Headings
- Bullet List
- Numbered List
- Table
- Image
- Blockquote
-
-
-
-
-
- Callouts
- Accordions
- Accordion Group
- Cards
- Card Group
- Code Block
- Code Group
- Tabs
- Steps
- Frames
- Update
-
-
-
-
-### Adding images
-
-You can add images to your page by typing `/image` and either clicking on the **"Image"**
-option or hitting ↓ + Enter on the **"Image"** option.
-
-This will open up the image modal where you have the option to either upload a new
-image or use an existing image from the repo.
-
-
-
-
-
-
-Uploading an image can be done through the modal:
-
-
-
-
-
-
-And you can preview an existing image before you add it.
-
-
-
-
-
-
-### Editing images
-
-In order to edit images, you just have to hover over the image to see the **"Delete"**
-and **"Edit"** buttons on the top right of the image.
-
-
-
-
-
-
-Clicking the **"Edit"** button lets you edit the attributes of the image.
-
-
-
-
-
-
-If you want to update the source of an image to be a new image that you haven't yet
-uploaded, you have to first delete the image you're changing, and then add a new one
-using the [instructions above](#adding-images).
-
-## Editor modes
-
-In order to offer the most flexibility, the editor has three modes:
-
-
-
-
-
-
-### Visual Editor
-
-The **"Visual Editor"** is the typical WYSIWYG mode you'd see in something like Notion.
-It offers you a view into your docs in a fully editable way that reflects what the final
-page would look like on your main docs site.
-
-### Source Editor
-
-The **"Source Editor"** offers you a way to edit your MDX files in code, the same way
-you'd do in your IDE. This offers less flexibility, in that our components aren't available
-for auto-complete, but it does have two unique advantages.
-
-1. It allows you to edit props of components (see our [limitations below](#current-limitations))
-which is currently not available in **"Visual Editor"** mode yet.
-
-2. It allows you to correct syntax errors which might've appeared in your repo that
-might not be compatible with **"Visual Editor"** mode until you've fixed them.
-
-### Diff View
-
-The **"Diff View"** is a way to view the changes made to a specific document before
-committing it to your repository.
-
-This will help you see changes you've made along with formatting changes made by
-the editor.
+## Editing Content
-## Current limitations
+### Using Slash Commands
-We do have a few current limitations in the editor that we're working to resolve.
+Type `/` in Visual Editor mode to access commands for adding:
+- Standard blocks (paragraphs, headings, lists, tables, images, blockquotes)
+- Mintlify components (callouts, accordions, cards, code blocks, tabs, steps, frames)
-
-
- You currently cannot live-update your navigation based on added/edited files. You
- still have to manually edit renamed, added, and deleted files in your `mint.json`
-
+### Managing Images
-
- We currently don't show any previews for custom snippets. This is on our roadmap to support
- as fully editable components.
-
+- Add images: Type `/image` to upload or select existing images
+- Edit images: Hover over an image to see edit and delete options
-
- We currently don't show any previews for OpenAPI specs. This is on our roadmap to support
- as a read-only preview.
-
+## Editor Modes
-
- There are currently some bugs around keyboard navigation that we're working to resolve.
- This includes things like going back/forward and up/down between Mintlify components and normal
- markdown blocks, or deleting a line vs. a Mintlify component.
-
-
+1. **Visual Editor**: WYSIWYG editing with live preview
+2. **Source Editor**: Direct MDX code editing
+3. **Diff View**: Review changes before committing
-## Feedback
+## Current Limitations
-If you have any bug reports, feature requests, or other general feedback, we'd love to hear
-where we can improve.
+- Navigation tree cannot be live-updated
+- No preview for custom snippets or OpenAPI specs
+- Some keyboard navigation issues
-Email us at [support@mintlify.com](mailto:support@mintlify.com)
+For feedback or support, contact [support@mintlify.com](mailto:support@mintlify.com)
\ No newline at end of file