You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
As a side note: `belongs-to-*` definitions will create a new column in that table, that is used to save the id of the counterpart model (foreign key field).
400
400
Whereas `has-*` definitions are just virtual fields which are going to query the linked models by their own id (the inverse way). This leads us to the following configuration schema:
@@ -567,7 +567,7 @@ In case you want to bind a many-to-many relation to itself, meaning that you'd l
567
567
</tr>
568
568
</table>
569
569
570
-
A common scenario is where a `User` has friends and that relation target is also `User`. So in you would bind the relation to itself:
570
+
A common scenario is where a `User` has friends and that relation target is also `User`. So it would bind the relation to itself:
571
571
572
572
```php
573
573
namespace Model;
@@ -580,7 +580,7 @@ class User extends \DB\Cortex {
580
580
}
581
581
```
582
582
583
-
Because this is also a many to many relation, a pivor table is needed too. It's name is generated based on the table and fields name, but can also be defined as 3rd array parameter, i.e. `['\Model\User','friends','user_friends']`.
583
+
Because this is also a many to many relation, a pivot table is needed too. Its name is generated based on the table and fields name, but can also be defined as 3rd array parameter, i.e. `['\Model\User','friends','user_friends']`.
584
584
585
585

586
586
@@ -755,6 +755,13 @@ Especially for value comparison, it's **highly recommended** to use placeholders
755
755
* The `IN` operator usually needs multiple placeholders in raw PDO (like `foo IN (?,?,?)`). In Cortex queries you simply use an array for this, the QueryParser does the rest.
756
756
757
757
`array('foo IN ?', array(1,2,3))`
758
+
759
+
You can also use a CortexCollection as bind parameter. In that case, the primary keys are automatically used for matching:
This method is useful to compare the current collection with another collection or a list of values that is checked for existence in the collection records.
1640
+
1641
+
In example you got a relation collection that is about to be updated and you want to know which records are going to be removed or would be new in the collection:
1642
+
1643
+
```php
1644
+
$res = $user->friends->compare($newFriendIds);
1645
+
if (isset($res['old'])) {
1646
+
// removed friends
1647
+
}
1648
+
if (isset($res['new'])) {
1649
+
// added friends
1650
+
foreach($res['new'] as $userId) {
1651
+
// do something with $userId
1652
+
}
1653
+
}
1654
+
```
1655
+
1656
+
The compare result `$res` is an array that can contain the array keys `old` and `new`, which both represent an array of `$cpm_key` values.
1657
+
1658
+
NB: This is just a comparison - it actually does not update any of the collections. Add a simple `$user->friends = $newFriendIds;` after comparison to update the collection.
1659
+
1660
+
1661
+
### contains
1662
+
**check if the collection contains a record with the given key-val set**
This method can come handy to check if a collections contains a given record, or has a record with a given value:
1669
+
1670
+
```php
1671
+
if ($user->friends && $user->friends->contains($newFriend)) {
1672
+
$f3->error(400,'This user is already your friend');
1673
+
return;
1674
+
}
1675
+
```
1676
+
1677
+
With custom compare key:
1678
+
1679
+
```php
1680
+
if ($user->blocked_users->contains($currentUserId,'target')) {
1681
+
// this user has blocked you
1682
+
}
1683
+
```
1684
+
1685
+
1617
1686
### expose
1618
1687
**return internal array representation**
1619
1688
@@ -1742,7 +1811,7 @@ Cortex currently only reflects to the most common use cases. If you need more ex
1742
1811
1743
1812
Anyways, I hope you find this useful. If you like this plugin, why not make a donation?
1744
1813
1745
-
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=44UHPNUCVP7QG)
1814
+
[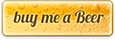](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=44UHPNUCVP7QG)
1746
1815
1747
1816
If you like to see Cortex in action, have a look at [fabulog](https://github.com/ikkez/fabulog"the new fabulous blog-ware").
0 commit comments