The k6 documentation is a Gatsby application using React components and markdown files for the content of the different pages.
There are two types of pages: Welcome Pages and Documentation articles.
Welcome Pages are the pages shown on the header menu: Guides
, Javascript API
, Cloud Docs
, Integration
, and Examples
. They are made as separate React Components for maximum customisation.
Documentation articles are markdown files structured under the src/data/markdown/docs
folder.
Root folders represent main categories at the top of the page.
Use numbers in front of the folder name to set the order.
Pattern: {number}{space}{page name}
Example: 02 Hello world
The same pattern used to define orders not only root folderы but also pages(md files) inside the category.
Use typical ## markdown definition to format headers.
## Making HTTP requests
Make sure you are using '##' which stands for a h2 tag - h1 is reserved for the title of a page, that gets parsed from the frontmatter. Also the navigation box that is being dynamically created on each page based on h2 tags.
Use h2 and only h2 as landmarks. h3 tag is designed to be used in your blockquote heading, like that:
And h4,h5,h6 have no specified styles, therefore will be rendered by default very similar to h1, so you probably do not want to use them, but if there are use cases, please, let us know.
Default image syntax for markdown files

You have to store images in src/images folder and access them from md files by using relative path. This way allows image-sharp-plugin process your image: compressing, converting and lazy loading.
If you really have to put there some remote picture, write it like that:
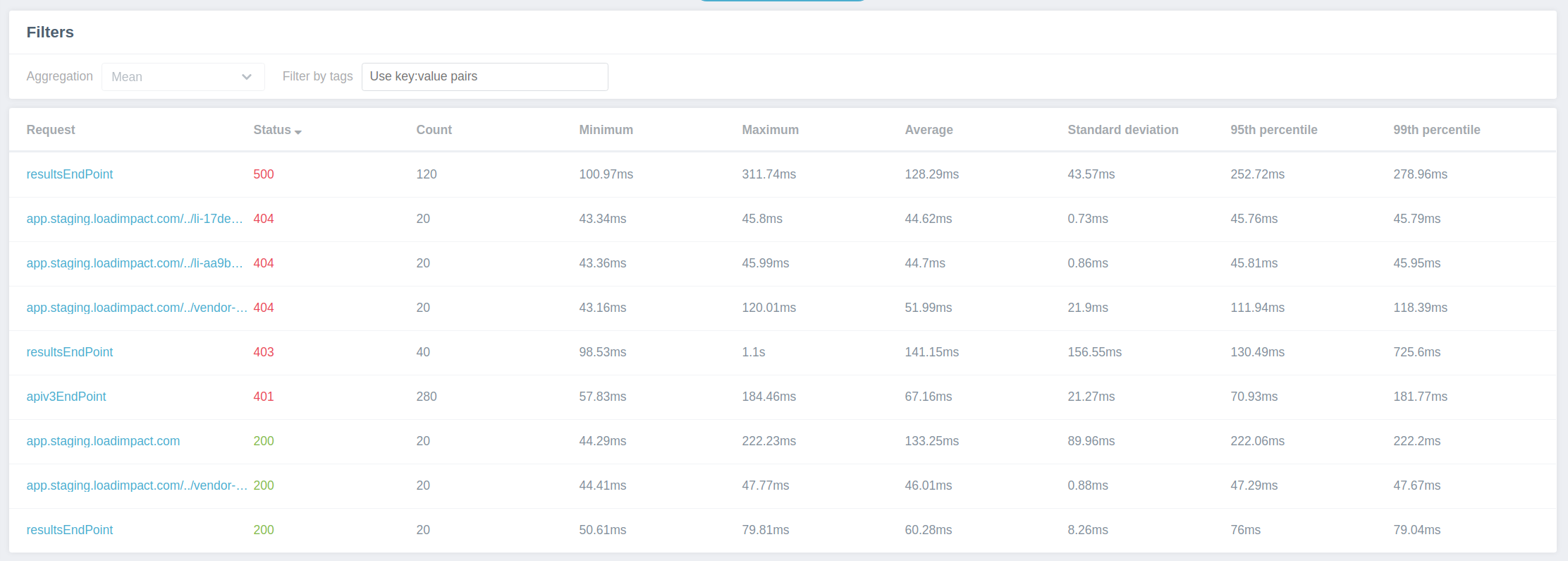
But keep in mind the size of an image on the other side of a link to prevent page overweighting.
Here things are getting a bit hairy. It is still default md, so it is perfectly fine to write blockquotes like:
> ### Docker syntax
>
> When using the `k6` docker image, you can't just give the script name since
> the script file will not be available to the container as it runs. Instead
> you must tell k6 to read `stdin` by passing the file name as `-`. Then you
> pipe the actual file into the container with `<` or equivalent. This will
> cause the file to be redirected into the container and be read by k6.
>
> **Note**: If your script imports other files (JS modules), piping like this
> will not work since the extra files will not be visible inside the container.
> To use modules you need to first mount your host/local directory into the
> Docker container, see [Modules with Docker](https://docs.k6.io/v1.0/docs/modules#section-using-local-modules-with-docker)."
And you'll get a fine quote block:
And, in case of a 'warning' mod for a blockquote, you just add an
> ### ⚠️ Docker syntax
>
> When using the `k6` docker image, you can't just give the script name since
> the script file will not be available to the container as it runs. Instead
> you must tell k6 to read `stdin` by passing the file name as `-`. Then you
> pipe the actual file into the container with `<` or equivalent. This will
> cause the file to be redirected into the container and be read by k6.
>
> **Note**: If your script imports other files (JS modules), piping like this
> will not work since the extra files will not be visible inside the container.
> To use modules you need to first mount your host/local directory into the
> Docker container, see [Modules with Docker](https://docs.k6.io/v1.0/docs/modules#section-using-local-modules-with-docker)."
Pay attention to those empty lines between md block and a wrapper, they are required to correctly parsing.
And our default blockquote will take a form of:
At the moment there are only two mods available, default (no wrapper needed) and warning. If you need more, let us know.
So, there are basically three types of code blocks, small ones, headerless ones and headerfull ones,
that last two have one possible modification - line numbers.
No hardwork required, just wrap your small stuff like keywords in backticks:
`API_VARIABLE` should be stored under a pillow
and you are good to go
We are going to write them a bit differently, half-native md, just like blockquotes:
<div class="code-group" data-props='{"labels": []}'>
```javascript
for (var id = 1; id <= 100; id++) {
http.get(http.url`http://example.com/posts/${id}`)
}
// tags.name="http://example.com/posts/${}",
// tags.name="http://example.com/posts/${}"
```
</div>
And, as a result:
If we want line numbers to be rendered, we shall adjust our data-props to:
<div class="code-group" data-props='{"labels": [], "lineNumbers": [true]}'>
```javascript
for (var id = 1; id <= 100; id++) {
http.get(http.url`http://example.com/posts/${id}`)
}
// tags.name="http://example.com/posts/${}",
// tags.name="http://example.com/posts/${}"
```
</div>
Here you go:
Pretty much the same routine as with headerless ones, but one difference in data-props, labels field, but I bet, you already got that:
<div class="code-group" data-props='{"labels": ["Nice code!"], "lineNumbers": [true]}'>
```javascript
for (var id = 1; id <= 100; id++) {
http.get(http.url`http://example.com/posts/${id}`)
}
// tags.name="http://example.com/posts/${}",
// tags.name="http://example.com/posts/${}"
```
</div>
To be able to switch between different code tabs, we have to repeat the headerfull routine, but extend labels and md code blocks:
<div class="code-group" data-props='{"labels": ["Nice code!", "This one is better", "Oh my.."], "lineNumbers": [true, true, true]}'>
```javascript
for (var id = 1; id <= 100; id++) {
http.get(http.url`http://example.com/posts/${id}`)
}
// tags.name="http://example.com/posts/${}",
// tags.name="http://example.com/posts/${}"
```
```javascript
for (var id = 1; id <= 100; id++) {
http.get(http.url`http://example.com/posts/${id}`)
}
// tags.name="http://example.com/posts/${}",
// tags.name="http://example.com/posts/${}"
```
```javascript
for (var id = 1; id <= 100; id++) {
http.get(http.url`http://example.com/posts/${id}`)
}
// tags.name="http://example.com/posts/${}",
// tags.name="http://example.com/posts/${}"
```
</div>
'{"labels": ["Nice code!", "This one is better", "Oh my.."], "lineNumbers": [true, true, true]}'
Line numbers are optional not for the whole code block, but for each tab. That is why here we have an array of bool.
In md file it should look like this to be formatted as a table. You could use online markdown tables generator to simplify the process – https://www.tablesgenerator.com/text_tables
| | |
|-------------------------------------|-------------------------------------|
|[batch()](https://docs.k6.io/docs/batch-requests) | Issue multiple HTTP requests in parallel (like e.g. browsers tend to do) |
|[del()](https://docs.k6.io/docs/del-url-body-params) | Issue an HTTP DELETE request. |
|[get()](https://docs.k6.io/docs/get-url-body-params) | Issue an HTTP GET request. |
|[options()](https://docs.k6.io/docs/options-url-body-params) | Issue an HTTP OPTIONS request. |
|[patch()](https://docs.k6.io/docs/patch-url-body-params) | Issue an HTTP PATCH request. |
|[post()](https://docs.k6.io/docs/post-url-body-params) | Issue an HTTP POST request. |
|[put()](https://docs.k6.io/docs/put-url-body-params) | Issue an HTTP PUT request. |
|[request()](https://docs.k6.io/docs/request-method-url-body-params) | Issue any type of HTTP request. |
Result:
The rest of elements you could write as you would in native md. It includes p, ul, ol, em, strong etc.
Check out the project Wiki for additional information