Commit 98078c1
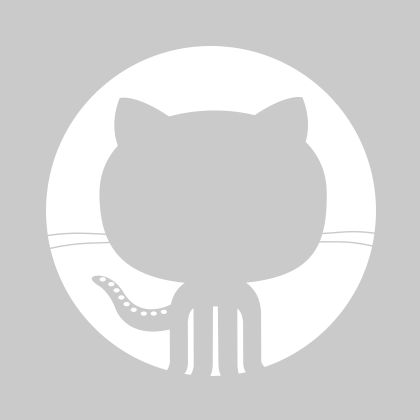
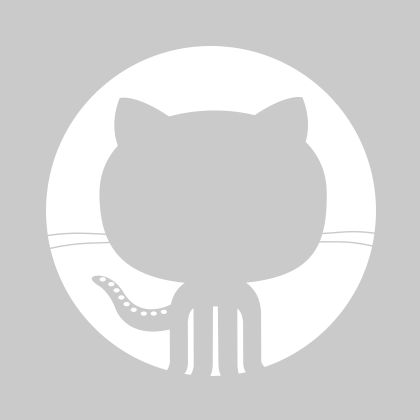
Michael Nieß
Michael Nieß
1 parent 658248e commit 98078c1
File tree
9 files changed
+154
-280
lines changed- main
- core
- avr
- sim
- util
9 files changed
+154
-280
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
| |
6 |
| - | |
7 |
| - | |
8 |
| - | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
24 | 24 |
| |
25 | 25 |
| |
26 | 26 |
| |
27 |
| - | |
| 27 | + | |
28 | 28 |
| |
29 | 29 |
| |
30 | 30 |
| |
| |||
40 | 40 |
| |
41 | 41 |
| |
42 | 42 |
| |
43 |
| - | |
| 43 | + | |
44 | 44 |
| |
45 | 45 |
| |
46 | 46 |
| |
|
+49-115
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| |||
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
| 30 | + | |
38 | 31 |
| |
| 32 | + | |
39 | 33 |
| |
40 | 34 |
| |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
77 | 46 |
| |
78 | 47 |
| |
79 | 48 |
| |
80 | 49 |
| |
81 |
| - | |
82 |
| - | |
83 |
| - | |
84 |
| - | |
85 |
| - | |
86 |
| - | |
| 50 | + | |
| 51 | + | |
87 | 52 |
| |
88 | 53 |
| |
89 |
| - | |
90 | 54 |
| |
91 |
| - | |
92 |
| - | |
| 55 | + | |
93 | 56 |
| |
94 | 57 |
| |
95 | 58 |
| |
96 |
| - | |
97 |
| - | |
98 |
| - | |
99 |
| - | |
100 |
| - | |
101 |
| - | |
| 59 | + | |
| 60 | + | |
102 | 61 |
| |
103 |
| - | |
| 62 | + | |
104 | 63 |
| |
105 | 64 |
| |
106 | 65 |
| |
107 | 66 |
| |
108 | 67 |
| |
109 |
| - | |
| 68 | + | |
110 | 69 |
| |
111 | 70 |
| |
112 |
| - | |
113 |
| - | |
114 |
| - | |
115 |
| - | |
116 |
| - | |
117 |
| - | |
118 |
| - | |
119 |
| - | |
120 |
| - | |
121 |
| - | |
122 |
| - | |
123 |
| - | |
124 |
| - | |
125 |
| - | |
126 |
| - | |
127 |
| - | |
128 |
| - | |
129 |
| - | |
130 |
| - | |
131 |
| - | |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 |
| - | |
136 |
| - | |
137 |
| - | |
138 |
| - | |
139 |
| - | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
140 | 87 |
| |
141 |
| - | |
142 |
| - | |
143 |
| - | |
144 |
| - | |
145 |
| - | |
146 |
| - | |
147 |
| - | |
148 |
| - | |
149 |
| - | |
150 |
| - | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
151 | 94 |
| |
152 |
| - | |
153 |
| - | |
154 |
| - | |
155 |
| - | |
156 |
| - | |
| 95 | + | |
| 96 | + | |
157 | 97 |
| |
158 | 98 |
| |
159 | 99 |
| |
160 | 100 |
| |
161 | 101 |
| |
162 | 102 |
| |
163 |
| - | |
164 |
| - | |
165 |
| - | |
166 |
| - | |
167 |
| - | |
168 |
| - | |
169 |
| - | |
170 |
| - | |
171 |
| - | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
172 | 106 |
| |
173 |
| - | |
| 107 | + | |
174 | 108 |
| |
175 | 109 |
| |
176 | 110 |
| |
|
+3-3
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
26 | 26 |
| |
27 | 27 |
| |
28 | 28 |
| |
29 |
| - | |
30 |
| - | |
31 |
| - | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
32 | 32 |
| |
33 | 33 |
| |
34 | 34 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| |||
34 | 34 |
| |
35 | 35 |
| |
36 | 36 |
| |
37 |
| - | |
| 37 | + | |
| 38 | + | |
38 | 39 |
| |
39 | 40 |
| |
40 |
| - | |
41 |
| - | |
42 | 41 |
| |
43 |
| - | |
| 42 | + | |
44 | 43 |
| |
45 | 44 |
| |
46 | 45 |
| |
47 | 46 |
| |
48 | 47 |
| |
49 |
| - | |
50 |
| - | |
| 48 | + | |
| 49 | + | |
51 | 50 |
| |
52 | 51 |
| |
53 | 52 |
| |
54 | 53 |
| |
55 | 54 |
| |
56 | 55 |
| |
57 |
| - | |
| 56 | + | |
58 | 57 |
| |
59 | 58 |
| |
60 | 59 |
| |
| |||
90 | 89 |
| |
91 | 90 |
| |
92 | 91 |
| |
93 |
| - | |
| 92 | + | |
94 | 93 |
| |
95 | 94 |
| |
96 | 95 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + |
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
| 2 | + | |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
| |||
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
22 |
| - | |
23 |
| - | |
| 22 | + | |
| 23 | + | |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
27 | 26 |
| |
| 27 | + | |
28 | 28 |
| |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
| 29 | + | |
36 | 30 |
| |
| 31 | + | |
37 | 32 |
| |
38 | 33 |
|
0 commit comments