diff --git a/notes/Bear Notes/Capturing Stereo Audio from Built-In Microphones.md b/notes/Bear Notes/Capturing Stereo Audio from Built-In Microphones.md new file mode 100644 index 00000000..62e057e8 --- /dev/null +++ b/notes/Bear Notes/Capturing Stereo Audio from Built-In Microphones.md @@ -0,0 +1,102 @@ +# Capturing Stereo Audio from Built-In Microphones +Configure an iOS device’s built-in microphones to add stereo recording capabilities to your app. + +## Overview +Stereo audio uses two channels to create the illusion of multidirectional sound, adding greater depth and dimension to your audio and resulting in an immersive listening experience. iOS provides a number of ways to record audio from the built-in microphones, but until now it's been limited to mono audio only. Starting in iOS 14 and iPadOS 14, you can now capture stereo audio using the built-in microphones on supported devices. + +Because a user can hold an iOS device in a variety of ways, you need to specify the orientation of the right and left channels in the stereo field. Set the built-in microphone’s directionality by configuring: +* Polar pattern. The system represents the individual device microphones, and beamformers that use multiple microphones, as data sources. Select the front or back data source and set its polar pattern to stereo. +* Input orientation. When recording video, set the input orientation to match the video orientation. When recording audio only, set the input orientation to match the user interface orientation. In both cases, don’t change the orientation during recording. + +This sample app shows how to configure your app to record stereo audio, and helps you visualize changes to the input orientation and data-source selection. + +* Note: You must run the sample on a supported physical device running iOS 14, or later. To determine whether a device supports stereo recording, query the audio session’s selected data source to see if its [supportedPolarPatterns](https://developer.apple.com/documentation/avfoundation/avaudiosessiondatasourcedescription/1616450-supportedpolarpatterns) array contains the [stereo](https://developer.apple.com/documentation/avfoundation/avaudiosession/polarpattern/3551726-stereo) polar pattern. + +## Configure the Audio Session Category +Recording stereo audio requires the app’s audio session to use either the [record](https://developer.apple.com/documentation/avfoundation/avaudiosession/category/1616451-record) or [playAndRecord](https://developer.apple.com/documentation/avfoundation/avaudiosession/category/1616568-playandrecord) category. The sample uses the `playAndRecord` category so it can do both. It also passes the `defaultToSpeaker` and `allowBluetooth` options to route the audio to the speaker instead of the receiver, and to Bluetooth headphones. + +```swift +func setupAudioSession() { + do { + let session = AVAudioSession.sharedInstance() + try session.setCategory(.playAndRecord, options: [.defaultToSpeaker, .allowBluetooth]) + try session.setActive(true) + } catch { + fatalError("Failed to configure and activate session.") + } +} +``` +[View in Source](x-source-tag://SetupAudioSession) + +## Select and Configure a Built-In Microphone +An iOS device’s built-in microphone input consists of an array of physical microphones and beamformers, each represented as an instance of `AVAudioSessionDataSourceDescription`. The sample app finds the built-in microphone input by querying the available inputs for the one where the port type equals the built-in microphone, and sets it as the preferred input. + +```swift +private func enableBuiltInMic() { + // Get the shared audio session. + let session = AVAudioSession.sharedInstance() + + // Find the built-in microphone input. + guard let availableInputs = session.availableInputs, + let builtInMicInput = availableInputs.first(where: { $0.portType == .builtInMic }) else { + print("The device must have a built-in microphone.") + return + } + + // Make the built-in microphone input the preferred input. + do { + try session.setPreferredInput(builtInMicInput) + } catch { + print("Unable to set the built-in mic as the preferred input.") + } +} +``` +[View in Source](x-source-tag://EnableBuiltInMic) + +## Configure the Microphone Input’s Directionality +To configure the microphone input’s directionality, the sample sets its data source’s preferred polar pattern and the session’s input orientation. It performs this configuration in its `selectRecordingOption(_:orientation)` method, which it calls whenever the user rotates the device or changes the recording option selection. + +```swift +func selectRecordingOption(_ option: RecordingOption, orientation: Orientation, completion: (StereoLayout) -> Void) { + + // Get the shared audio session. + let session = AVAudioSession.sharedInstance() + + // Find the built-in microphone input's data sources, + // and select the one that matches the specified name. + guard let preferredInput = session.preferredInput, + let dataSources = preferredInput.dataSources, + let newDataSource = dataSources.first(where: { $0.dataSourceName == option.dataSourceName }), + let supportedPolarPatterns = newDataSource.supportedPolarPatterns else { + completion(.none) + return + } + + do { + isStereoSupported = supportedPolarPatterns.contains(.stereo) + // If the data source supports stereo, set it as the preferred polar pattern. + if isStereoSupported { + // Set the preferred polar pattern to stereo. + try newDataSource.setPreferredPolarPattern(.stereo) + } + + // Set the preferred data source and polar pattern. + try preferredInput.setPreferredDataSource(newDataSource) + + // Update the input orientation to match the current user interface orientation. + try session.setPreferredInputOrientation(orientation.inputOrientation) + + } catch { + fatalError("Unable to select the \(option.dataSourceName) data source.") + } + + // Call the completion handler with the updated stereo layout. + completion(StereoLayout(orientation: newDataSource.orientation!, + stereoOrientation: session.inputOrientation)) +} +``` +[View in Source](x-source-tag://SelectDataSource) + +This method finds the data source with the selected name, sets its preferred polar pattern to stereo, and then sets it as the input’s preferred data source. Finally, it sets the preferred input orientation to match the device’s user interface orientation. +#documentation +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/Form.md b/notes/Bear Notes/Form.md new file mode 100644 index 00000000..059a1a74 --- /dev/null +++ b/notes/Bear Notes/Form.md @@ -0,0 +1,6 @@ +# Form +Leave it to [Jon Rettinger](https://youtu.be/eRxq9IyZ4Cc) to back me up on the size hype thing... Yes, the 12 Pro Max is *bigger*- and definitely prompts a conversation on how big we’re actually going to let smartphones get before we... idk.. call them something else(?) - but it’s far from *alarmingly huge* or anything, as [Nilay Patel’s review](https://www.theverge.com/21555901/iphone-12-pro-max-review) seems to suggest. It’s very possible that I say this out of Big Hand Privilege, though - auto journalist Matt Farrah (whom I trust completely) noted that he chose the 12 Mini because he can use it one-handed. It occurred to me, hearing this, that my own usership hasn’t really ever included this aspect. Nilay felt so strongly about the 12 Pro Max’s size that he recommends you find a way to experience its size in person before ordering one. My concern - as much as I love the idea of the 12 Mini - centers around the fact that I am already starting to struggle with the diminishing size of the buttons in the UX of popular apps like [TikTok](https://vm.tiktok.com/ZMe17ocYh/), and I’d rather not make my eyes struggle with text size any more than absolutely necessary. Handily enough, one can now access iOS’ text-enlarging slider from within the Control Center. + +For myself and others, the flat sides of the design prompt a reflection on how this may be the first genuinely beautiful iPhone since the iPhone 4. + +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/Galaxy S21 Ultra vs. iPhone 12 Pro Max Which takes better night photos?.md b/notes/Bear Notes/Galaxy S21 Ultra vs. iPhone 12 Pro Max Which takes better night photos?.md new file mode 100644 index 00000000..7421a27d --- /dev/null +++ b/notes/Bear Notes/Galaxy S21 Ultra vs. iPhone 12 Pro Max Which takes better night photos?.md @@ -0,0 +1,7 @@ +# Galaxy S21 Ultra vs. iPhone 12 Pro Max: Which takes better night photos? + + +This isn't a review of the Galaxy S21 Ultra or its regular camera capabilities. [You can read our in-depth review here](https://www.inputmag.com/reviews/galaxy-s21-ultra-review-the-iphone-12-pro-max-gets-put-to-shame). This is a head-to-head showdown between the S21 Ultra and iPhone 12 Pro Max cameras for low-light and night photography. + +[Galaxy S21 Ultra vs. iPhone 12 Pro Max: Which takes better night photos?](https://www.inputmag.com/reviews/galaxy-s21-ultra-vs-iphone-12-pro-max-which-camera-phone-takes-better-night-photos) +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/Galaxy S21 Ultra vs. iPhone 12 Pro Max Which takes better night photos?/545b927b-387d-491a-aaf6-2ccaa44881d2-galaxy-s21-ultra-review-08.jpg b/notes/Bear Notes/Galaxy S21 Ultra vs. iPhone 12 Pro Max Which takes better night photos?/545b927b-387d-491a-aaf6-2ccaa44881d2-galaxy-s21-ultra-review-08.jpg new file mode 100644 index 00000000..3969a45e Binary files /dev/null and b/notes/Bear Notes/Galaxy S21 Ultra vs. iPhone 12 Pro Max Which takes better night photos?/545b927b-387d-491a-aaf6-2ccaa44881d2-galaxy-s21-ultra-review-08.jpg differ diff --git a/notes/Bear Notes/Hardware Notes.md b/notes/Bear Notes/Hardware Notes.md new file mode 100644 index 00000000..f79af9a7 --- /dev/null +++ b/notes/Bear Notes/Hardware Notes.md @@ -0,0 +1,20 @@ +# Hardware Notes +- [ ] The **Crud** issue... +[GSMArena’s Video Review](https://youtu.be/TfRtC5cQCpA) also shows crud around the lenses. +## Display +> OLED can be seen as a replacement for LCD, or liquid crystal displays. You might be reading this article on an LCD screen right now, given how ubiquitous the technology has been since its inception in the early 1960s. For reference, every iPhone before the iPhone X used LCD screens. Even the regular iPhone 11 last year used it, with the more expensive 11 Pro moving to OLED. For reference, the iPhone X and XS used OLED, too. +> The most important functional difference between OLED and LCD that you need to know is the presence of a backlight. An OLED display uses that organic film we mentioned earlier to emit light when an electrical current runs through it. In other words, OLED can function all on its own without the need for a backlight. LCD has served humanity well for decades, but it needs a backlight to display anything. +[Everything you need to know about the OLED display rumored to be on the iPhone 12](https://mashable.com/article/oled-explained-iphone-apple/) +It’s difficult to remark with much insight on the OLED display vs. the LCD unit on my iPhone 8 Plus without the latter device in my posession to physically compare, but the contrast (literally, hehe) is especially obvious when a given app’s “high contrast dark mode” is selected. While my iPhone 8 Plus review began with a note on how insignificant True Tone appeared to be, it’s worth noting that it’s certainly a significant component of the experience, now - how much the upgraded display technology factors into this change, I know not. +The Big Issue among tech media is a refresh rate complaint... the iPhone 12 Pro line’s display is limited to 60Hz, while the rest of the market’s flagship lineup pushes to 120Hz and beyond. This is not something I am equipped to comment on, but I will say that 60 frames per second has always seemed like plenty to me. + +## Camera +> Front-facing 4K, 60fps capture is impressive, but useless — vloggers all have GoPros or DSLRs, these days, and sharing through Snapchat and Instagram will always be ultra-compressed. +I am not - nor have I ever been - one of these self-described *Camera Nerds*, but it strikes ~~ me that “real” camera nerds own one or more DSLRs and would surely prefer them over their handset’s camera in nearly every situation. Obviously, though, I am 100% out of touch when it comes to The Camera Issue. Two years later, and tech media appears to be unable to stop talking about smartphone cameras. The industry, predictably, seems to have gone right along with it. Now, I have in my posession an $1100 telephone designed around its camera system, which has sent me on a similar, completely bonkers camera-testing escapaade. In my defense, the thought *I should’ve just bought a DSLR* was [stuck in my mind](https://twitter.com/neoyokel/status/1344559416968966144?s=21) throughout the lot of it. +[Camera Test Embeds] +In short, I think we’re expecting far too much out of our smartphones and would end up gaining much in the end if we stopped. +- [ ] I have been shooting RAW photos on iPhone for a while now... thanks to Halide, which may or may not be completely worthless, now. + +## Battery and Magnets +I am genuinely worried about the future we continue to set ourselves up for, full of spent batteries and no way to responsibly despose of them. +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/Jorts Battery Log.md b/notes/Bear Notes/Jorts Battery Log.md new file mode 100644 index 00000000..549ee800 --- /dev/null +++ b/notes/Bear Notes/Jorts Battery Log.md @@ -0,0 +1,52 @@ +# Jorts Battery Log +- - - - +**TOE**: December 12, 2020 at 08:34:41 CST +**Location**: 38.93397363372048,-92.38790472046693 +**Battery Level**: 80% +Outside Temp/Humidity: 39.2°F/88% +- - - - +**TOE**: December 12, 2020 at 03:37:59 CST +**Location**: 38.93400410193851,-92.3879305367287 +**Battery Level**: 99% +Outside Temp/Humidity: 41°F/84% +- - - - +**TOE**: December 11, 2020 at 21:23:20 CST +**Location**: 38.93397572919627,-92.38797244624456 +**Battery Level**: 87% +Outside Temp/Humidity: 48.2°F/90% +- - - - +**TOE**: December 11, 2020 at 18:26:38 CST +**Location**: 38.93407166007807,-92.38806623974105 +**Battery Level**: 92% +Outside Temp/Humidity: 53.6°F/87% +- - - - +**TOE**: December 11, 2020 at 17:03:36 CST +**Location**: 38.93370335235362,-92.38772160263653 +**Battery Level**: 66% +Outside Temp/Humidity: 51.8°F/94% +- - - - +**TOE**: December 11, 2020 at 16:27:34 CST +**Location**: 38.9340088377138,-92.38798317508062 +**Battery Level**: 69% +Outside Temp/Humidity: 51.8°F/93% +- - - - +**TOE**: December 11, 2020 at 16:01:22 CST +**Location**: 38.93418427094718,-92.38783624031802 +**Battery Level**: 56% +Outside Temp/Humidity: 51.8°F/94% +- - - - +**TOE**: December 11, 2020 at 12:51:16 CST +**Location**: 38.93400766424735,-92.38797487699648 +**Battery Level**: 99% +Outside Temp/Humidity: 48.2°F/91% +- - - - +**TOE**: December 11, 2020 at 12:49:42 CST +**Location**: 38.93397644165804,-92.38805383452436 +**Battery Level**: 99% +Outside Temp/Humidity: 48.2°F/91% +- - - - +**TOE**: December 11, 2020 at 12:47:10 CST +**Location**: 38.93404781356355,-92.38795911901852 +**Battery Level**: 99% +Outside Temp/Humidity: 48.2°F/91% +#documentation #iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/Jorts Data.md b/notes/Bear Notes/Jorts Data.md new file mode 100644 index 00000000..9b8283a9 --- /dev/null +++ b/notes/Bear Notes/Jorts Data.md @@ -0,0 +1,114 @@ +# Jorts Data +### Operating System +- System: iOS 14.2.1 +- System Build: 18B121 +- Kernel: Darwin 20.1.0 + +### Device Information +- Device: iPhone +- Device ID: iPhone13,4 +- Model: D54pAP +- Name: Jorts +- Hostname: Jorts + +### CPU Information +- CPU Model: N/A +- GPU Model: N/A +- Motion Coprocessor: N/A +- Core Number: 6 +- CPU Architecture: 64 +- CPU Frequency: N/A +- TB Frequency: 24 +- L1 Cache Size: 128 +- L1D Cache Size: 64 +- L2 Cache Size: 4096 +- Byteorder: 1234 +- Cacheline: 128 + +### Hardware Features +- Display Resolution: 2688 x 1242 +- Pixel Density: N/A +- Battery Voltage: 3.8 V +- Battery Capacity: N/A +- Rear Camera: No +- Front Camera: No +- Touchscreen: Yes +- Microphone: Yes +- Speaker: Yes +- Wi-Fi: Yes +- Bluetooth: Yes +- NFC: Yes +- Accelerometer: Yes +- Gyroscopic Sensor: Yes +- Ambient Light Sensor: Yes +- Proximity Sensor: Yes +- Fingerprint Sensor: Yes +- Magnetometer: Yes +- Barometer: Yes +- Phone: Yes +- GPS: Yes + +Battery Status +- Battery Percentage: 78% +- Battery State: Charging + +Storage Usage +- Used: 90.9 GB (35.5 %) +- Free: 164.9 GB (64.5 %) +- Total Storage: 255.9 GB + +CPU Usage +- Usage: 3.8 % +- Idle: 96.2 % +- Avg Load (1, 5, 15 min): 1.31, 1.60, 1.90 + +Memory +- Wired: 861.2 MB +- Active: 1654.3 MB +- Inactive: 1621.7 MB +- Other: 1359.6 MB +- Free: 214.4 MB +- Total Memory: 5711.2 MB + +System Uptime +- Boot Time: 12/11/20, 16:55 +- Uptime: 21h 4m + +Connection +- Default Gateway IP: 192.168.0.1 +- DNS Server IP: 192.168.0.1, 108.166.149.2 +- External IP: 104.166.200.131 +- External IP Hostname: 104-166-200-131.client.mchsi.com +- External IP AS Number: AS30036 +- External IP AS Name: MEDIACOM-ENTERPRISE-BUSINESS +- Default Gateway IPv6: fe80::1eb0:44ff:feb2:cca3 +- DNS Server IPv6: fe80::1eb0:44ff:feb2:cca3 +- External IPv6: 2604:2d80:4d02:4e00:31fd:134b:a717:4b25 +- External IPv6 Hostname: N/A +- External IPv6 AS Number: AS30036 +- External IPv6 AS Name: MEDIACOM-ENTERPRISE-BUSINESS +- HTTP Proxy: N/A + +Wi-Fi Information +- Network Connected: Yes +- SSID: long1950-5G +- BSSID: 1c:b0:44:b2:cc:a7 +- IP Address: 192.168.0.83 +- Subnet Mask: 255.255.255.0 +- IPv6 Addresses: fe80::428:33d5:606e:19ef / 64, 2604:2d80:4d02:4e00:10a0:c529:f10c:f4b4 / 64, 2604:2d80:4d02:4e00:31fd:134b:a717:4b25 / 64, 2604:2d80:4d02:4e00::1004 / 64 +- Received Since Boot: 448.67 MB +- Sent Since Boot: 1.17 GB + +Cell Information +- Network Connected: Yes +- Network Type: N/A +- IP Address: 10.41.19.40 +- IPv6 Addresses: fe80::cfe:2ae8:2d5:101a / 64, 2600:381:1129:c4d:1871:f625:78cd:d4fd / 64, 2600:381:1129:c4d:bc11:175c:7450:f7bc / 64 +- Carrier Name: AT&T +- Country Code: us +- MCC / MNC: 310 / 280 +- VOIP Support: Yes +- Received Since Boot: 757.71 MB +- Sent Since Boot: 95.04 MB +#documentation +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/Native Software (iOS 14).md b/notes/Bear Notes/Native Software (iOS 14).md new file mode 100644 index 00000000..ea0ed00e --- /dev/null +++ b/notes/Bear Notes/Native Software (iOS 14).md @@ -0,0 +1,8 @@ +# Native Software (iOS 14) +[[iOS 14 Review Notes]] +## Siri, Discreet Idiot +Siri Shortcuts are essentially associated with Apple’s personal assistent only in name, but she does still have a particular value you may or may not have considered: if you’re the sort to worry about your “privacy” in 2021, Siri is [the most discreet voice assistent available](https://www.theverge.com/2019/8/29/20837077/apple-siri-privacy-opt-out-voice-human-grading-review), by far. Of course, this also makes her the definitive *worst* in functional terms, yet she remains the only one which has added (albeit diminutive) value to my own life. The “disable all alarms” command - while it should probably exist on its own in the native Clocks app - is a particularly useful function. +- - - - +- [ ] Fonts? + +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/On iOS Excess.md b/notes/Bear Notes/On iOS Excess.md new file mode 100644 index 00000000..6fd5e2c9 --- /dev/null +++ b/notes/Bear Notes/On iOS Excess.md @@ -0,0 +1,9 @@ +# On iOS Excess +A Symbol of Excess. A less-walled garden?.m4a +> Well I have now lived with the flag ship phone the iPhone 12 pro max for seven whole days and it's it's been insane which is ridiculous thing to say about having a new phone but it's what I mean makes clear Wow what should I get should be obvious I guess I am in the in the literature in a litter litter history of the iPhone him This promax It's just access it's over it's him gluttony Yeah I've been without jailbreaking keep in mind I am which is something I have to investigate again can you please jailbreaking still think but I am I have set up like a clone get repositories in my fucking phone I am I've downloaded Torrance arm I have played far too much of what's basically a full consul game I've seen myself playing that game in HD Arm And I've set up a bunch of automated stuff Yeah I've taken some ridiculous photos This thing is just No it's it's I guess compared to the 8+ I have before I am Through this feeling of limitless power I am And I also to second revelation I want to discuss in his voice now is that I don't well Apple is not the wall garden That it that people still seem to think it is I am I thought about that when Siri shortcuts came out and they've been neutered in a big way I am at seems like all the shortcut library's have been going to There's still people messing around that There's really not that much she can do that with Shortcuts there isn't more elegantly automated another way but that's another subject I guess I am I really can't imagine what the regular iPhone user I think some shortcuts I am there just a toy they've been made a toy another official Shortcuts a really useful I don't think I'd like to now on Yeah this is this devices So much more Then I guess even my original like my Like grandest vision for the iPhone as someone is using iPhones those with my whole adult life more arm I feel like this is has exceeded it And I don't know what that means other than I think it means that it's a very wasteful thing I am and it's very tempting distracting for family distracting thing I am I don't I don't know at least for my own life if we define a useful device as one That rolls of who's fault it is as a net positive affect on my productivity Well so far It's not it but that's my fault I guess I just can't imagine What is four Who is for This professional who Just want to carry around in the SLR Because you can't you can't replace a DSLR with one of these phones he just can't is good like I don't understand much about the tire for you but like I am The addition in this one the super white angle there are useless for that sure but like If you actually take those photographs No it's too much is too wide and I mean I think it's certainly smart of them to do that and to invest a lot and making and they did make mechanical engineers I wish I haven't seen if I can hear it moving yet I need to do that a Yeah cause I don't think it's reasonable to try to make a highly telescopic lens either what exactly are we trying to do here you know I think you if you been observing my past few days well with my past my life I think you are always saying that over and over again but especially my use of this phone +## Apps +* [iSH](https://apps.apple.com/us/app/ish-shell/id1436902243) +* [Copied](https://apps.apple.com/us/app/copied-touch/id1015767349) +* [Drafts](https://apps.apple.com/us/app/drafts/id1236254471) +* [Toolbox Pro](https://apps.apple.com/us/app/toolbox-pro-for-shortcuts/id1476205977) (As an addendum to the power of Siri Shortcuts.) +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/On iOS Excess/A Symbol of Excess. A less-walled garden?.m4a b/notes/Bear Notes/On iOS Excess/A Symbol of Excess. A less-walled garden?.m4a new file mode 100644 index 00000000..37f0ee7e Binary files /dev/null and b/notes/Bear Notes/On iOS Excess/A Symbol of Excess. A less-walled garden?.m4a differ diff --git a/notes/Bear Notes/Reflecting on Apple Arcade and the Blistering Evolution of Mobile Gaming.md b/notes/Bear Notes/Reflecting on Apple Arcade and the Blistering Evolution of Mobile Gaming.md new file mode 100644 index 00000000..8ec8e49b --- /dev/null +++ b/notes/Bear Notes/Reflecting on Apple Arcade and the Blistering Evolution of Mobile Gaming.md @@ -0,0 +1,12 @@ +# Reflecting on Apple Arcade and the Blistering Evolution of Mobile Gaming +## The world’s most valuable company takes on gaming distribution, as told from a non-gaemer’s perspective. +I’ve now spent 56 days with Apple’s current flagship handset (more on that coming soon) and the experience has been even more profound than I expected, for a variety of reasons. One of them has been an enlightening returning exploration of “Mobile Gaming,” thanks in large part to the free 3 month trial of Apple Arcade included with the purchase of the device. This space - now probably best described as this *industry* - has expanded exponentially since my last visit. One of the first apps I remember downloading when the App Store launched in the Summer of 2008 was a brutally simple *Pong* ripoff, which had zero options, zero multiplayer or connected functions whatsoever, yet managed to be buggy as fuck. + +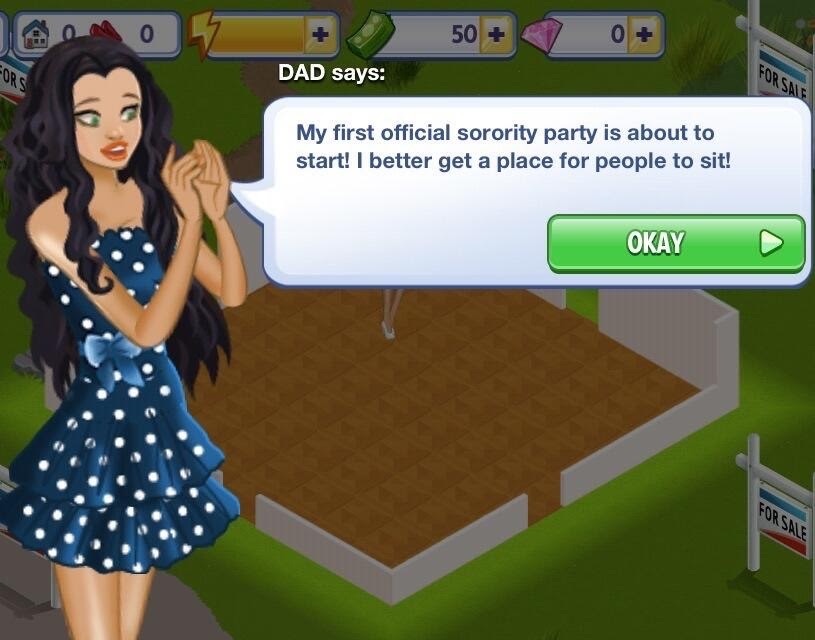 +- - - - +- [ ] [Real Racing Tweet](https://twitter.com/neoyokel/status/115875263518216193?s=21) +- [ ] [Real Racing 2 Menu Music](https://youtu.be/RBL5zyR1XN8) +- [ ] [Pocket Planes Tweet](https://twitter.com/neoyokel/status/358328255163535362?s=21) + +#ios #iP12PM +[iOS 14.5 will support PS5 DualSense and Xbox Series X controllers - The Verge](https://www.theverge.com/2021/2/1/22260671/ios-14-5-ps5-dualsense-xbox-series-x-controller-support) \ No newline at end of file diff --git a/notes/Bear Notes/Reflecting on Apple Arcade and the Blistering Evolution of Mobile Gaming/Photo Jan 30, 2021 at 131422.jpg b/notes/Bear Notes/Reflecting on Apple Arcade and the Blistering Evolution of Mobile Gaming/Photo Jan 30, 2021 at 131422.jpg new file mode 100644 index 00000000..9faa9f46 Binary files /dev/null and b/notes/Bear Notes/Reflecting on Apple Arcade and the Blistering Evolution of Mobile Gaming/Photo Jan 30, 2021 at 131422.jpg differ diff --git a/notes/Bear Notes/Siri Voice Commands Documentation.md b/notes/Bear Notes/Siri Voice Commands Documentation.md new file mode 100644 index 00000000..55bf9a64 --- /dev/null +++ b/notes/Bear Notes/Siri Voice Commands Documentation.md @@ -0,0 +1,589 @@ +# Siri Voice Commands Documentation +## Table of Contents + +* [Wallet Siri Commands](#wallet-siri-commands) +* [Web and YouTube Search Siri Commands](#web-and-youtube-search-siri-commands) +* [More Phone and Notifications Siri Commands](#more-phone-and-notifications-siri-commands) +* [Contacts Siri Commands](#contacts-siri-commands) +* [Affective (Fun) Siri Commands](#affective--fun--siri-commands) +* [Notes Siri Commands](#notes-siri-commands) +* [Translation Siri Commands](#translation-siri-commands) +* [Dictionary, Reference and Trivia Siri Commands](#dictionary--reference-and-trivia-siri-commands) +* [Science Reference Siri Commands](#science-reference-siri-commands) +* [Geography Siri Commands](#geography-siri-commands) +* [Clock App Siri Commands](#clock-app-siri-commands) + * [Clock Time Siri Commands](#clock-time-siri-commands) + * [Timer Siri Commands](#timer-siri-commands) + * [Stopwatch Siri Commands](#stopwatch-siri-commands) + * [Alarm Siri Commands](#alarm-siri-commands) +* [Podcast Siri Commands](#podcast-siri-commands) +* [Calendar Siri Commands](#calendar-siri-commands) +* [Maps and Geolocation Siri Commands](#maps-and-geolocation-siri-commands) +* [Find My Siri Commands](#find-my-siri-commands) +* [Reminder Siri Commands](#reminder-siri-commands) +* [Weather Siri Commands](#weather-siri-commands) +* [Live Radio Streaming Siri Commands](#live-radio-streaming-siri-commands) +* [Music and Music Streaming Siri Commands](#music-and-music-streaming-siri-commands) +* [Hourly News Update Siri Commands](#hourly-news-update-siri-commands) +* [Finance Siri Commands](#finance-siri-commands) +* [Useful Math Siri Commands](#useful-math-siri-commands) +* [General Math Siri Commands](#general-math-siri-commands) +* [Money Math Siri Commands](#money-math-siri-commands) +* [Local and Navigtion Siri Commands](#local-and-navigtion-siri-commands) +* [Flashlight Siri Commands](#flashlight-siri-commands) +* [Audio Volume Settings Siri Commands](#audio-volume-settings-siri-commands) +* [Screen Brightness Settings Siri Commands](#screen-brightness-settings-siri-commands) +* [General Siri Commands](#general-siri-commands) +* [Sports Siri Commands](#sports-siri-commands) + +## Wallet Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Show my wallet.** | Opens Wallet App and displays payment cards and/or transit passes. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Web and YouTube Search Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Web Search dogs.** | Returns a response card showing Google web searches. | +| **YouTube Search cats.** | Returns a response card showing YouTube video searches. | +| **Bing search bicycles.** | Opens web page with Bing search results. | +| **Bing search dog food.** | Opens web page with Bing search results. | +| **Search Yahoo for silly straws.** | Opens web page with Yahoo search results. | +| **Search Duck Duck Go for running shoes.** | Opens web page with Duck Duck Go search results. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## More Phone and Notifications Siri Commands + +> **NOTE:** Silent Mode must be switched off for audio responses. +| **Siri Command** | Response | +| :--- | :--- | +| **Read all notifications.** | Prompts user regarding notifications. | +| **Do I have missed calls?** | Checks for missed calls and returns the total number. | +| **Check voicemail.** | Checks and reads voicemails and saved messages. | +| **Read voicemails.** | Checks and reads voicemails and saved messages. | +| **Play voicemails.** | Checks and reads voicemails and saved messages. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Contacts Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Abby is my sister.** | Prompts the user for confirmation before adding the label "sister" to Abby's contact information. | +| **Bobby is my brother.** | Prompts the user for confirmation before adding the label "brother" to Bobby's contact information. | +| **Cammy is my lawyer.** | Prompts the user for confirmation before adding the label "lawyer" to Cammy's contact information. | +| **Danny is my plumber.** | Prompts the user for confirmation before adding the label "plumber" to Danny's contact information. | +| **Eddy is my broker.** | Prompts the user for confirmation before adding the label "broker" to Eddy's contact information. | +| **Freddy is my chef.** | Prompts the user for confirmation before adding the label "chef" to Freddy's contact information. | +| **My nickname is Goose.** | Prompts the user for confirmation before changing the personal name that Siri uses in social graphs and query discourse. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Affective (Fun) Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Hi.** | Siri replies. | +| **Hello.** | Siri replies. | +| **Hello world.** | Siri replies. | +| **Good morning.** | Siri replies. | +| **Good afternoon.** | Siri replies. | +| **Good evening.** | Siri replies. | +| **Good night.** | Siri replies. | +| **Good luck.** | Siri replies. | +| **Tell me a joke.** | Tells a joke. | +| **I am tired.** | Siri replies. | +| **I am drunk.** | Siri replies with recommendation for designated driver. | +| **Rock, paper, scissors.** | Replies to the challenge. | +| **I am feeling peckish.** | Replies with nearby restaurants. | +| **I am hungry.** | Replies with nearby restaurants. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Notes Siri Commands + +> **NOTE:** Silent Mode must be switched off for audio responses. +| **Siri Command** | Response | +| :--- | :--- | +| **Make a note.** | Prompts user for details about the new note. | +| **Note that the ocean is deep.** | Creates a new note "the ocean is deep". | +| **Note that the sky is blue.** | Creates a new note "the sky is blue". | +| **Make a note that the grass is green.** | Creates a new note "grass is green". | +| **Show notes.** | Display most recent notes. | +| **Show my notes.** | Display most recent notes. | +| **Read my notes.** | Display most recent notes, read the most recent note aloud, and prompts user for further action. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Translation Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **How do you say hello in German?** | Returns translation. | +| **How do you say wonderful in French?** | Returns translation. | +| **How do you say Germany in German?** | Returns translation. | +| **How do you say twenty-five in Greek?** | Returns translation. | +| **How do you say Happy Birthday in Chinese?** | Returns translation. | +| **How do you say Happy Birthday in Cantonese?** | Siri cannot translate into Cantonese yet. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Dictionary, Reference and Trivia Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Define geometry.** | Returns definition of the word geometry. | +| **Spell Mississippi.** | Returns the audio spelling of Mississippi. Requires Silent Mode off. | +| **Words that start with the letter G.** | Returns response card with partial list of words starting with G. | +| **Words that start with Q and end with R.** | Returns response card with partial list of words start with Q and end with R. | +| **Words that begin with A and end with S.** | Returns response card with partial list of words start with A and end with S. | +| **Words that begin with S and end with T.** | Returns response card with partial list of words start with S and end with T. | +| **Words that begin with the letter Q.** | Returns response card with partial list of words starting with Q. | +| **Words that begin with the letter Q.** | Returns response card with partial list of words starting with Q. | +| **Fruits that start with the letter A.** | Returns response card and (optionally) provides audio response listing the items. Requires Silent Mode off for audio response. | +| **Fruits that start with the letter T.** | Returns response card and (optionally) provides audio response listing the items. Requires Silent Mode off for audio response. | +| **Vegetables that start with the letter T.** | Returns response card and (optionally) provides audio response listing the items. Requires Silent Mode off for audio response. | +| **Words that rhyme with grace.** | Returns list of words that rhyme. | +| **Words that rhyme with hat.** | Returns list of words that rhyme. | +| **How many letters in Mississippi?** | Returns the total number of characters in Mississippi. | +| **How many characters in Mississippi?** | Returns the total number of characters in Mississippi. | +| **How many syllables in Mississippi?** | Returns the total number of syllables in Mississippi. | +| **What song is this?** | Prompts user to provide music for audio scan and search. | +| **World's oldest person.** | Returns information about the oldest person in the world. | +| **World's tallest person.** | Returns information about the tallest person in the world. | +| **World's shortest person.** | Returns information about the shortest person in the world. | +| **Airport code for Heathrow.** | Returns IATA Code for an airport or aerodrome. | +| **Show planes above me.** | Returns sky map with civial aviation data. | +| **What is the ceiling for a SR-71.** | Returns the ceiling for the aircraft. | +| **What is the ceiling for a Piper Cherokee.** | Returns the ceiling for the aircraft. | +| **Where can I buy some headphones?** | Suggests a visit to Apple.com. | +| **Tell me about the iPod touch.** | Suggests a visit to Apple.com. | +| **Tell me about Apple TV.** | Suggests a visit to Apple.com. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Science Reference Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Ask Wolfram Alpha about copper sulfate.** | Displays response card about molecular compound from Wolfram Alpha. | +| **Ask Wikipedia about copper sulfate.** | Displays response card about molecular compound from Wikipedia. | +| **Show structure of benzene.** | Displays stick stucture of molecular compound. | +| **Show structure of acetylcholine.** | Displays stick stucture of molecular compound. | +| **Chemical structure of nicotine.** | Returns the stick structure of molecular compound. | +| **Chemical formula for glucose.** | Returns the chemical formula of molecular compound. | +| **Chemical formula for hydrogen peroxide.** | Returns the chemical formula of molecular compound. | +| **What is the density of aluminum?** | Returns the density of aluminum. | +| **What is the atomic number of calcium?** | Returns the atomic number of calcium. | +| **What is the melting point of potassium?** | Returns the melting point of potassium. | +| **What is the boiling point of water?** | Returns the boiling point of water. | +| **Tungsten melting point.** | Returns the melting point of tungsten. | +| **Symbol for samarium.** | Returns the periodic table symbol for samarium. | +| **Atomic weight of platinum.** | Returns the atomic weight of platinum. | +| **Distance between Earth and Mars.** | Returns the distance between the two planets. May show telemetry graph. +| **Distance between Earth and the Sun.** | Returns the distance between two celestial objects. May show telemetry graph.| + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Geography Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **What is the capital of Georgia?** | Returns the capital of Georgia. | +| **What is the capital of Italy?** | Returns the capital of Italy. | +| **What is the population of Brazil?** | Returns the population of Brazil. | +| **Show me the flag of Bulgaria.** | Returns the flag of Bulgaria from a web search. | +| **What are the official languages of Canada?** | Returns the official languages of Canada. | +| **List the official languages of Canada.** | Returns the official languages of Canada. | +| **How tall is Mount Everest?** | Returns height of Mount Everest. | +| **Where is the highest feature on Earth?** | Returns latitude and longitude. May show details. | +| **Where is the lowest feature on Earth?** | Returns latitude and longitude. May show details. | +| **Where is the lowest feature on Europe?** | Returns the place name in Europe. May show details. | +| **Where is the highest feature on South America?** | Returns the place name in South America. May show details. | +| **Distance from Salesforce Tower to the Space Needle.** | Returns the distance by car and by bird. | +| **Distance from Tulsa to Chicago.** | Returns the distance by car and by bird. | +| **Distance from Dulles to Heathrow.** | Returns the distance by bird. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Clock App Siri Commands + +### Clock Time Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Time.** | Returns the current time. | +| **Current time.** | Returns the current time. | +| **Time in London.** | Returns the current time in London. | +| **Daylight saving time.** | Provides the calendar start and end dates for DST. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +### Timer Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Show timer.** | Displays timer. | +| **Set timer for 20 minutes.** | Begins countdown from 20 minutes to zero. | +| **Stop timer.** | Pauses countdown. | +| **Pause timer.** | Pauses countdown. | +| **Resume timer.** | Resumes countdown. | +| **Reset timer.** | Pauses countdown and resets to start time. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +### Stopwatch Siri Commands + +* Works on iOS or watchOS. + +| **Siri Command** | Response | +| :--- | :--- | +| **Show stopwatch.** | Displays stopwatch. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +### Alarm Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Delete all alarms.** | Prompts user to confirm deletion of all alarms. | +| **Create new alarm.** | Creates a new alarm, then prompts for more details. | +| **Set alarm for fifteen-hundred hours.** | Creates alarm for 3:00 PM. | +| **Create new alarm for 5:30 PM.** | Creates alarm for 5:30 PM. | +| **Create pizza alarm for 7:45 PM.** | Creates alarm for 7:45 PM labeled "pizza". | +| **Change pizza alarm to 8:30 PM.** | Changes alarm labeled "pizza" to 8:30 PM. | +| **Rename pizza.** | Prompts user to provide a new name for the alarm. | +| **Delete pizza alarm.** | Deletes the alarm labeled "pizza". | +| **Set coffee alarm for 5:15 AM.** | Creates alarm for 5:15 AM labeled "coffee". | +| **Turn off the 5:15 alarm.** | Turns off the alarm for 5:15 AM. | +| **Set spinach alarm for 7:05 AM.** | Creates alarm for 7:05 AM labeled "spinach". | +| **Turn off spinach alarm.** | Turns off the spinach alarm. | +| **Turn on spinach alarm.** | Turns on the spinach alarm. | +| **Do I have a pizza alarm?** | Returns alarm labeled "pizza" if available. | +| **Disable all alarms.** | Toggles all alarms off. | +| **Turn off all alarms.** | Toggles all alarms off. | +| **Enable all alarms.** | Toggles all alarms on. | +| **Turn on all alarms.** | Toggles all alarms on. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Podcast Siri Commands + +> **NOTE:** Requires Apple Podcast App. +| **Siri Command** | Response | +| :--- | :--- | +| **Subscribe to the Apple Keynotes podcast.** | Prompts user to confirm subscription to the podcast feed. | +| **Search WNYC podcasts.** | Returns search results of WNYC podcasts in Podcast App. | +| **Search WBUR podcasts.** | Returns search results of WBUR podcasts in Podcast App. | +| **Search Vox Media podcasts.** | Returns search results of Vox Media podcasts in Podcast App. | +| **Search Vergecast podcasts.** | Returns search results of Vergecast podcasts in Podcast App. | +| **Search Leo Laporte podcasts.** | Returns search results of Leo Laporte podcasts in Podcast App. +| **Play Apple Keynote podcast.** | Plays latest Apple Keynotes podcast episode. | +| **Play Pivot podcast.** | Plays the latest Pivot podcast episode in Podcast App. | +| **Play the latest Pivot podcast.** | Plays the last available podcast in feed. | +| **Play the earliest Pivot podcast.** | Plays the earliest available podcast in feed. | +| **Pause playback.** | Pauses podcast playback. | +| **Resume playback.** | Resumes podcast playback. | +| **Rewind 10 seconds.** | Rewinds podcast 10 seconds and resumes playback. | +| **Rewind 73 seconds.** | Rewinds podcast 73 seconds and resumes playback. | +| **Rewind 2 minutes.** | Rewinds podcast 2 minutes and resumes playback. | +| **Set playback to double speed.** | Changes podcast playback to 2.0x speed. | +| **Set playback to half speed.** | Changes podcast playback to 0.5x speed. | +| **Set playback to normal speed.** | Changes podcast playback to 1.0x speed. | +| **Set playback to one-point-five speed speed.** | Changes podcast playback to 1.5x speed. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Calendar Siri Commands + +> **NOTE:** Silent Mode must be switched off for audio responses. +| **Siri Command** | Response | +| :--- | :--- | +| **Show all November events.** | Displays all events for the month of November. | +| **Read all November events.** | Displays all events for the month of November, provides a summary, before reading all events sequentially. | +| **Add a new event.** | Prompts user for date and time details about the new event. | +| **Add pizza event.** | Prompts user for date and time details about the new event labeled "pizza". | +| **Add a conference call to my calendar at 9 AM on Thursday.** | Adds new event labeled "conference call" at 9:00 AM on Thursday. | +| **Add training event at 6 AM tomorrow.** | Adds new event labeled "training" at 6:00 AM tomorrow. | +| **Add pizza party at 10 PM next Monday.** | Adds new event labeled "pizza party" at 10:00 PM next Monday. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Maps and Geolocation Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Location.** | Returns current location, with latitude and longitude. | +| **Current location.** | Returns current location, with latitude and longitude. | +| **Elevation.** | Returns elevation based on current location. | +| **Show Salesforce Tower in Maps.** | Displays information card of requested location. | +| **Show Salesforce Tower in Maps.** | Displays information card of requested location. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Find My Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Hide from my friends.** | Deactivates location-sharing beacon for friends. | +| **Stop hiding from my friends.** | Activates location-sharing beacon for friends. | +| **Ping my watch.** | Locates Apple Watch and activates ping sound. | +| **Find my watch.** | Locates Apple Watch and activates ping sound. | +| **Find my headphones.** | Locates account-linked Apple AirPods and activates ping sound. | +| **Find my iPad.** | Locates account-linked Apple iPad and activates ping sound. | +| **Find my Macbook.** | Locates account-linked Apple Macbook and activates ping sound. | +| **Find my Macbook Pro.** | Locates account-linked Apple Macbook Pro and activates ping sound. | +| **Where is my iPhone?** | Locates account-linked Apple iPhone and activates ping sound. | +| **Find my iPhone.** | Locates account-linked Apple iPhone and activates ping sound. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Reminder Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Show all reminders.** | Displays all reminders. | +| **Show all potato reminders.** | Displays all reminders with the keyword "potato" in the text (if availble). | +| **Show all reminders from February.** | Displays all reminders created in the month of February. | +| **Show all reminders from this month.** | Displays all reminders created during the current month. | +| **Delete all reminders.** | Prompts user to confirm deletion of all reminders. | +| **Remind me hourly.** | Prompts user for details about hourly reminder. | +| **Remind me every 2 hours.** | Prompts user for details about the reminder that repeats every 2 hours. | +| **Remind me daily.** | Prompts user for details about daily reminder. | +| **Remind me weekly.** | Prompts user for details about weekly reminder. | +| **Remind me every other week.** | Prompts user for details about the reminder that repeats every 2 weeks. | +| **Remind me every two weeks.** | Prompts user for details about the reminder that repeats every 2 weeks. | +| **Remind me monthly.** | Prompts user for details about monthly reminder. | +| **Remind me about bedtime every night at 8 o'clock.** | Creates a daily reminder at 10PM labeled "bedtime". | +| **Remind me when I leave Las Vegas.** | Prompts user for details about location-based reminder. | +| **Remind me to buckle up when leaving Las Vegas.** | Creates a new reminder labeled "buckle up" that reminds the user whenever leaving Las Vegas. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Weather Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Sunrise.** | Returns the sunrise time for a location. | +| **Sunrise time.** | Returns the sunrise time for a location. | +| **Sunset.** | Returns the sunset time for a location. | +| **Sunset time.** | Returns the sunset time for a location. | +| **Humidity.** | Returns the relative humidity for a location. | +| **Wind speed.** | Returns the wind speed for a location. | +| **Dew point.** | Returns the dew point temperature for a location. | +| **Pressure.** | Returns the barometric pressure for a location. | +| **High temperature.** | Returns the high temperature for a location. | +| **Low temperature.** | Returns the low temperature for a location. | +| **Wind chill.** | Returns the wind chill temperature for a location. | +| **UV index.** | Returns UV index for a location. | +| **Will it rain today?** | Answers the question based on chance of rain. | +| **Do I need an umbrella?** | Answers the question based on chance of rain. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Live Radio Streaming Siri Commands + +> **NOTE:** Requires Apple Music App. + +> **NOTE:** The response card now prpvides a link to the audio stream provider (e.g., RADIO.COM, TUNEIN.COM, etc.) +| **Siri Command** | Response | +| :--- | :--- | +| **Play KNX 1070.** | Plays live stream of radio station KNX 1070 (Los Angeles). | +| **Play KFI 640.** | Plays live stream of radio station KFI AM 640 (Los Angeles). | +| **Play KCBS radio.** | Plays live stream of radio station KCBS 740 (San Francisco). | +| **Play KMOX 1120.** | Plays live stream of radio station KMOS 1120 (St. Louis). | +| **Play 1010 WINS.** | Plays live stream of radio station 1010 WINS (New York). | +| **Play 710 WOR.** | Plays live stream of radio station 710 WOR (New York). | +| **Play WBUR.** | Plays live stream of radio station WBUR 90.9 FM (Boston). | +| **Play 90.9 WBUR.** | Plays live stream of radio station WBUR 90.9 FM (Boston). | +| **Play RTHK radio one.** | Plays live stream of Cantonese language radio station RTHK Radio 1 (Hong Kong). | +| **Play RTHK radio three.** | Plays live stream of English language radio station RTHK Radio 3 (Hong Kong). | +| **Play Deutschlandfunk radio.** | Plays live stream of German language new radio station (Berlin). | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Music and Music Streaming Siri Commands + +> **NOTE:** Requires Apple Music App. +| **Siri Command** | Response | +| :--- | :--- | +| **Search David Bowie in iTunes Store.** | Returns music search results for David Bowie. | +| **Search David Bowie music.** | Returns music search results for David Bowie. | +| **Play Eine Kleine Nachtmusik.** | Plays Mozart K. 525 (if available). | +| **Play Serenade Number 13 by Mozart.** | Plays Mozart K. 525 (if available). | +| **Set playback to double speed.** | Changes music playback to 2.0x speed. | +| **Set playback to half speed.** | Changes music playback to 0.5x speed. | +| **Set playback to normal speed.** | Changes music playback to 1.0x speed. | +| **Set playback to one-point-five speed speed.** | Changes music playback to 1.5x speed. | +| **Play a random song.** | Creates an in-memory playlist, then plays all songs, shuffled. | +| **Shuffle songs.** | Creates an in-memory playlist, then plays all songs, shuffled. | +| **Reshuffle music.** | Turns shuffle on. | +| **Turn shuffle on.** | Turns shuffle on. | +| **Turn shuffle off.** | Turns shuffle off. | +| **Play jazz.** | Plays all music tagged "jazz" in Music Library. | +| **Play bluegrass.** | Plays all music tagged "bluegrass" in Music Library. | +| **Play next song.** | Plays the next song on the playlist. | +| **Skip this song.** | Plays the next song on the playlist. | +| **Play previous song.** | Plays the previous song on the playlist. | +| **Play the last song.** | Plays the previous song on the playlist. | +| **Jump ahead 5 seconds.** | Seeks ahead 5 seconds and resumes playback. | +| **Seek ahead 5 seconds.** | Seeks ahead 5 seconds and resumes playback. | +| **Jump back 15 seconds.** | Seeks back 15 seconds and resumes playback. | +| **Seek back 15 seconds.** | Seeks back 15 seconds and resumes playback. | +| **Seek to 3 minutes and 21 seconds.** | Seeks to exactly 3 minutes 21 seconds and resumes playback. | +| **Jump to 3 minutes and 21 seconds.** | Seeks to exactly 3 minutes 21 seconds and resumes playback. | +| **Seek to 200 seconds.** | Seeks to exactly 3 minutes 20 seconds and resumes playback. | +| **Jump to 200 seconds.** | Seeks to exactly 3 minutes 20 seconds and resumes playback. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Hourly News Update Siri Commands + +> **NOTE:** Requires Apple Podcast App. +| **Siri Command** | Response | +| :--- | :--- | +| **Play sports news from ESPN.** | Plays pre-recorded hourly news update from ESPN. | +| **Play business news from Bloomberg.** | Plays pre-recorded hourly news update from Bloomberg. | +| **Play business news from CNBC.** | Plays pre-recorded hourly news update from CNBC. | +| **Play NPR News.** | Plays pre-recorded hourly news update from NPR News. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Finance Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **What is the ticker symbol for Twitter?** | Returns the ticker symbol for Twitter. | +| **Home Depot stock price.** | Returns the stock price for Home Depot. | +| **Microsoft market cap.** | Returns the market capitalization for Microsoft. | +| **Verizon market capitaliztion.** | Returns the market capitalization for Verizon. | +| **Verizon 52-week high.** | Returns the highest price over the last 52 weeks. | +| **Verizon 52-week low.** | Returns the lowest price over the last 52 weeks. | +| **Clorox opening price.** | Returns the opening price for Clorox. | +| **Clorox closing price.** | Returns the closing price for Clorox. | +| **P/E ratio for Clorox.** | Returns the price-earnings ratio for Clorox. | +| **What is the yield for ticker symbol CLX?** | Returns the yield for Clorox. | +| **What is the current price of gold?** | *Commodity information is not available at this time.* | +| **What is the closing price of silver?** | *Commodity information is not available at this time.* | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Useful Math Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Heads or tails.** | Returns either heads or tails in a simulated coin flip. | +| **Flip a coin.** | Returns either heads or tails in a simulated coin flip. | +| **Pick a random number.** | Returns a random number between 0 and 100. | +| **Pick a number between 3 and 588.** | Returns a random number between 3 and 588. | +| **Pick a random color.** | Returns a random color swatch. | +| **Roll the dice.** | Returns two random numbers from a pair of simulated dice. | +| **Roll a die.** | Returns only one random number from a single simulated die. | +| **Is 881 prime?** | Answers whether a number is prime or not. | +| **Is 513 divisible by 9?** | Answers whether a number is divisible by another number. | +| **Prime factorization of 24.** | Retuns the prime factorization in exponent form, including prime factors and distinct factors. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## General Math Siri Commands +| Math | **Siri Command** | Response | +| :--- | :--- | :--- | +| *5 = 4x + 3y8 = 3x + 5y* | **Solve five equals four x plus three y AND eight equals three x plus five y.** | Returns a solution for the two equations. May show graph. | +| *x2 = 6x - 9* | **Solve x squared equals six x minus nine.** | Returns a solution for the quadratic equation. | +| 4! | **Four factorial.** | Returns the factorial. | +| *arctan(0.75)* | **Inverse tangent of zero point seven five.** | Returns the value of inverse trigonometric function. | +| *cos(π)* | **Cosine of pi.** | Returns the value of the trigonometric function. | +| *1 + 3 + 5 =* | **One plus three plus five equals.** | Returns a sum. | +| *64 = x2 + y2* | **Nine equals x squared plus y squared.** | Returns the graph of a circle. | +| *SQRT(81)* | **Square root of 81.** | Returns the square root of the number. | +| *CBRT(27)* | **Square root of 27.** | Returns the cube root of the number. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Money Math Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **15% of $200.** | Returns a fraction of the total. | +| **15 percent of 200 dollars.** | Returns a fraction of the total. | +| **$20 plus 8% tax.** | Returns the total amount due with tax. | +| **20 dollars plus 8 percent tax.** | Returns the total amount due with tax. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Local and Navigtion Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Sunset temperature.** | Returns the temperature around sunset for a location. | +| **Sunset temperature in London.** | Returns the temperature around sunset in London. | +| **Wind speed in Palm Springs.** | Returns the wind speed in Palm Springs. | +| **Sunrise temperature in Seattle tomorrow.** | Returns the sunrise temperature in Seattle tomorrow morning. | +| **Sunrise temperature in the North Pole.** | Returns the sunrise temperature at the North Pole. | +| **Wind chill in Minneapolis.** | Returns the wind chill temperature in Minneapolis. | +| **UV index Syndey Australia.** | Returns current UV index forecast for Syndey. | +| **Grocery stores open now.** | Returns a list of grocery stores still open. | +| **Grocery stores open at 4AM.** | Returns a list of grocery stores that open their doors at 4:00 AM. | +| **Grocery stores open 24 hours.** | Returns a list of grocery stores that never close. | +| **Starbucks open now.** | Returns a list of Starbucks retail stores open right now. | +| **Pizza open late.** | Returns a list of pizza shops open late. | +| **Directions to nearest Starbucks.** | Returns navigation route to the nearest Starbucks. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Flashlight Siri Commands + +> **NOTE:** Availability depends on devices. Works on iPhone 11. +| **Siri Command** | Response | +| :--- | :--- | +| **Turn on flashlight.** | Turns on camera LED light. | +| **Turn off flashlight.** | Turns off camera LED light. | +| **Toggle flashlight.** | Turns camera LED light on or off. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Audio Volume Settings Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Volume.** | Returns the current audio output volume as a percentage. | +| **What is the current volume?** | Returns the current audio output volume as a percentage. | +| **Change volume to 25%.** | Changes the audio output volume to 25%. | +| **Change volume to 75%.** | Changes the audio output volume to 75%. | +| **Lower volume by 10%.** | Decreases the audio output volume by 10%. | +| **Full volume please.** | Changes the audio output volume to 100%. | +| **A little louder please.** | Increments the audio output volume. | +| **A little quieter please.** | Decrements the audio output volume. | +| **A little softer please.** | Decrements the audio output volume. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Screen Brightness Settings Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Set screen brightness to 50%.** | Changes the screen brightness to 50%. | +| **Increase screen brightness by 25%.** | Increments screen brightness by 25%. | +| **A little brighter please.** | Increases the screen brightness. | +| **A little dimmer please.** | Decreases the screen brightness. | +| **A little darker please.** | Decreases the screen brightness. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## General Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Battery life.** | Returns the battery charge as a percentage. | +| **Turn off Bluetooth.** | Turns off Bluetooth radio. | +| **Turn on Dark Mode.** | Activates Dark Mode. | +| **Turn on Low Power mode.** | Activates Low Power Mode. | +| **Turn on Night Shift.** | Enables Night Shift (blue light filter) mode. | +| **Invert colors.** | Inverts screen colors for improved readablity. | +| **Read QR Code.** | Opens camera and scan for any visible QR code. | +| **Turn on Do Not Disturb.** | Activates Do Not Disturb Mode. | +| **Hold my calls.** | Activates Do Not Disturb Mode. | +| **Take a picture.** | Opens rear camera. | +| **Take a selfie.** | Opens front camera. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). + +## Sports Siri Commands +| **Siri Command** | Response | +| :--- | :--- | +| **Zack Greinke ERA.** | Returns the ERA for Zack Greinke. | +| **Tom Brady quarterback rating.** | Returns Tom Brady's quarterback rating. | +| **Starting lineup for New England Patriots.** | Returns the starting lineup for the New England Patriots. | +| **Starting lineup for Pittsburg Penguins.** | Returns the starting lineup for the Pittsburg Penguins. | +| **Starting lineup for New York Yankees.** | Returns the starting lineup for the New York Yankees. | +| **Medal count.** | Returns the medal count for the most recent Olympic games. | +| **Super Bowl champions.** | Returns the name of the reigning Super Bowl champions. | +| **Super Bowl score.** | Returns the name of the reigning Super Bowl champions. | +| **Cleveland Browns record.** | Returns the current win-loss record of the Cleveland Browns. | +| **Gold Glove winner.** | Returns the name of the most recent Rawlings Gold Glove Award. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets](https://gsuite.google.com/marketplace/app/markdowntablemaker/46507245362). +#documentation #iP12PM \ No newline at end of file diff --git "a/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms.md" "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms.md" new file mode 100644 index 00000000..7c3d3b9f --- /dev/null +++ "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms.md" @@ -0,0 +1,45 @@ +# The Precarious Legacy of the iPhone 8 — The Psalms + + +## A decade of iPhone has probably ruined my life, but will the 8 Plus finally end it? + +*Is my True Tone bullshit on?* + +“True Tone” is so forgettable, everybody had to mention it first. Quite simply, it uses an ambient light sensor to fiddle with white balance, warming the colors of the display as an immediately-obvious whole, yes, but an interesting contrast to show off is no longer inherently justified in being called a “feature” in Apple products, anymore. Essentially, no matter who you ask (aside from [Jon Rettinger](https://youtu.be/AXU8GqaMsQc?t=7m23s),) you *should not* buy an iPhone 8, though I did last Fall, not only because I had to suddenly decide on a handset in less than 24 hours, but — if anything — to say goodbye to the form, the operating system, and the tech company which I have depended upon and carried with me virtually every day for my entire adult life. I’d originally decided to abandon this review due to a variety of unexpected circumstances, but Apple and its iPhone have maintained their place in the news with their [battery scandal](https://www.apple.com/iphone-battery-and-performance/), and a third of a year with the 8 Plus has included some experiences which warrant a send-off before iOS 12 is released, making it (and myself) totally irrelevant forever. + +As the longstanding benchmark of the smartphone industry’s state at any given time, the iPhone can be easy to reflect upon as a product once occupying a state of universal exemption from criticism, but it has, in fact, [never been so](http://theweek.com/articles/459748/7-reviews-original-iphone-from-2007). As Nilay Patel [noted](https://www.theverge.com/2017/9/19/16323570/apple-new-iphone-8-review-plus-2017), one might regard the 8 as the last compromise of “basically four years” of the same design. Since launch, it’s unsurprisingly stayed a wee bit too far behind on the spreadsheets for most Android-type folks — not that I’ve ever believed them truthfully incapable of comprehending what it means to *package* a product, given where their greasy startups all eventually ended up. ( **You cannot doubt me** — I once took a year-long sabbatical from iOS with a [Sony Xperia Play](https://youtu.be/nSWgS0l0kc8), and my authority is absolute.) The rest are trying to decide whether or not to pay $200 more for “ [the phone of the future](https://www.wsj.com/articles/iphone-8-apples-middle-child-1505322945),” which knows when you’re watching it, and is only good for playing half an hour of stupid video games before it needs a charge. + +So far, I have maintained that my first generation iPhone was the best handset of all time — one hell of an Email Machine that lasted me close to five years — throughout the last two with actual motherboard exposed to the elements in the corner of its cracked screen. That said, who knows how it’d feel to be coerced into using “iPhone OS 2” as it was called, then, for an entire workday in 2018? Two years prior to bringing home an 8 Plus, I [vowed](http://bit.ly/drycast47) that my 6S Plus would be my last ever Apple device, but this one *actually* feels like a last hurrah. Though the ability to *Tweet directly from the swipe-down notification menu* is still nowhere to be found (it’s been gone for 5 releases, now, and would seem to have been forgotten by *literally* everyone but myself,) one gets the sense that Apple’s efforts to add to the iPhone 8 and iOS 11 were to make amends with us by settling a few debts. + +In part, they did. Native apps got a major overhaul — including Mail, which was startling, considering that I’d been looking at what was near as makes no difference the same UI my eldest phone shipped with. As a result, it alone constitutes my benchmark for an email service, and I have been left without a clue as to what *a good one* looks like. (Apparently it was [really bad](https://www.pcmag.com/article/342889/the-best-mobile-email-client-apps)?) Since time began, there has always been at least one alternative email app of the moment that tech journos refer to as the must-have, end-all replacement. [Edison Mail](https://itunes.apple.com/us/app/email-edison-mail/id922793622?mt=8) is currently the smoother, faster, most modular option — at least for another few minutes– but I’ll never know it as I know Mail, and I’ll never want to. Playing around with experimental email apps is too scary. What if I decide once again to kill that massive number in the red badge and need to immediately mark 40,000 emails as read? It took all of my iPhone 4’s 1.0Ghz CPU and proprietary software over 18 hours — how am I supposed to trust a shabby little 6-month-old startup with such an important task? Anybody with a hundred bucks can make an app, you know. + +### Why is the App Store now the best-looking publishing software on iOS? + +One might interpret the App Store’s redesign as an attempt by Apple to control this conversation — of both the trending *new thing* and the old“essentials” that you’ve probably had tucked away in an untouched folder for years. Technically, whoever the hell is writing those gorgeously-presented daily bits has made them a publishing company, though I’m not so sure I’m not the last remaining user who’s continued semi-regularly visiting their “Today” section. If I *did* want to actually read about apps (I don’t — who does?) it wouldn’t make much sense to seek critical reviews from the faceless boffins behind the platform itself, regardless of how much better it may look than all of the tech news sites, paywall or no. + +Native screen recording could conceivably come in handy once or twice, but I see no reason why the red bar must remain at the top of the render, but it has, which could explain the total lack of any such video in the wild. Front-facing 4K, 60fps capture is impressive, but useless — vloggers all have GoPros or DSLRs, these days, and sharing through Snapchat and Instagram will always be ultra-compressed. (Here are two sloppy test clips — [at the zoo](https://youtu.be/VnfkWkotGFw), and [fishing](https://youtu.be/KOs5m6ynKAI).) + +Perhaps some have figured out the new Files “app,” but it’s sat on my homescreen for months, untapped, and it will likely remain there for all time as a sort of soothing trophy — a thanks for my legacy iPhone loyalty. My reward for half a lifetime of syncing, scrolling, and tolling? **I can now view some of the files on my Mobile Computing Device**, and even *scan documents in*, which is mostly novel (though it is fun to digitize excerpts from physical text.) At some point, I must’ve mischecked a permanent option because all file types now open only in an app that does not recognize them. God bless. + + +Somehow, I’ve managed to fill my social circle with precisely zero iOS-using folks. All of my friends and colleagues use Android devices (including [Tim’s supercool Nextbit Robin](https://soundcloud.com/extratonemagazine/nextbit),) which provide a few handy datapoints (like the camera in [my fiance’s Galaxy S8](https://flic.kr/p/BXruaL),) but deprive me of any significant experience with the ostensibly intoxicating cult of iMessage. I’m constantly listening to and reading tech writers claim that it’s [one of the only reasons they’re still using iPhones](https://www.theverge.com/2016/10/10/13225514/apple-iphone-cant-switch-pixel-android-imessage-addiction), but my own food-OS loving biome has forced me to find others, and frankly, I can’t imagine looking at the [gluttonous](http://www.extratone.com/audio/futureland/toomanymessengers/) palate of available mobile, cross-platform messaging services (Telegram, [now Telegram X](https://telegram.org/blog/telegram-x), WhatsApp, Signal, Snapchat, Facebook, Instagram, Twitter, Discord, Slack, *Tinder?*, Google Hangouts, Google Allo, Google Chat, Viber, Skype, Line, Wire, etc.) and thinking… *well, none of this will do*! + +Honestly — even if I’d actually been at all informed in my haste, the photographic capabilities of the 8 Plus, alone would’ve sold it. It’s not the new filters, gif functionality, or even “3D Photos” — it’s those mythical dual 12MP sensors (which it shares with something called the iPhone X.) They’re no less than infallible. After four months of [astonishing captures](http://bit.ly/ip8plus) in all manner of conditions, I don’t even care how exactly they do it anymore — it’s better to be left marveling. This first example was taken at Keystone, Colorado in the middle of a dark, cloudy Fall night — the amount of light they were able to find — “up to 80% more,” [according to Apple](https://www.apple.com/apple-events/september-2017/) — is just *impossible*. + +*The vast majority of the samples in my iPhone 8 Flickr Album were taken within the native Camera app as it ships and left unedited. (Especially before just a few weeks ago, when I [discovered Halide](https://extratone.com/race-day-at-hodges).* + + +Here is an unquestionably sensible progression from which iPhone has never wavered far since its fourth generation [set the standard](http://www.extratone.com/words/inred/iphone4/), but it’s one of an unfortunate few. Siri is still useless and silly apart from its “disable all alarms” feature and its ability to sound itself off in response when you’re hysterically screaming and digging for it through the vast plush of a forty-year-old Lincoln. The customizable Control Center makes toggling low power mode, orientation lock, Wi-Fi, and Bluetooth less frustrating (note the last two [aren’t quite hard switches](https://www.wired.com/story/how-to-turn-off-wifi-and-bluetooth-in-ios11/),) though it should’ve come years ago. Notifications are slightly more sensible -certainly better than they were on Android Gingerbread, but I’ve heard things’ve changed quite a bit since then. + +I *have* been tripped up by the lack of a 3.5mm audio jack a few times, but it just [wouldn’t make sense](http://www.extratone.com/words/inred/mono/) from a hardware perspective, and the new external stereo capability should refute those who can’t or won’t understand. Yes, it would be nice if Apple hadn’t led the industry to quite such a compromising obsession with thinness — we’d all trade *a lot* of substance for exponentially greater battery life, storage capacity, water resistance, etc. — but I don’t see much sense in expending your energy holding up signs in Silicon Valley. + +> I’ll be here long after you’ve died, and you know why? Because I took the time to sync my apps. + +Two years ago, a new generation of social apps and the preposterous notion of a quad-core CPU in my iPhone 6S Plus seemed like the harbinger of a world I no longer understood. Now, most of those services have expanded to the far boundaries of my reach, and I’ve stopped counting chips. Refinement of the hardware design is reverent to the extreme. It’s pretentious, but Apple’s decision to pause on the 8 to consider details like stuffing the legal text in the software and adding a little bit of weight *back in* for ergonomics’ sake leads one to regard it as a monument to all the devices along the development timeline that have led to this… last triumph. Or, it would have perhaps, had they not [sold so many](https://www.apple.com/newsroom/2018/02/apple-reports-first-quarter-results/). + +One could argue that good execution of consumer electronic design means minimizing as much as possible the obstructions in the way of the user completing any given task, and the iPhone 8 Plus has surpassed the vast majority of these for myself — and I am, surely, a “power user.” iOS has changed a lot in the decade I’ve employed it — in far too many ways for the worse — but this pair of handset and software have reached *my* imagination’s limit for what I could possibly want to do. Augmented reality and wireless charging won’t ever have a place in my future, for better or worse. Face ID is much too peculiar. Surely, this iPhone is the ultimate expression of the first and fourth generation’s foundation. + +If the 6S Plus was indeed the key to my immortality, I’m afraid the 8 Plus heralds my imminent demise. Whether or not it’s an early one is for you to decide. This *really is* my last iPhone. + +[The Precarious Legacy of the iPhone 8 — The Psalms](http://bilge.world/iphone-8-plus-review) +#iP12PM #ios \ No newline at end of file diff --git "a/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/C2LLJAl5.jpg" "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/C2LLJAl5.jpg" new file mode 100644 index 00000000..9934f385 Binary files /dev/null and "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/C2LLJAl5.jpg" differ diff --git "a/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/fuMs1a4.jpg" "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/fuMs1a4.jpg" new file mode 100644 index 00000000..9449bc5d Binary files /dev/null and "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/fuMs1a4.jpg" differ diff --git "a/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/tq2eUGT.jpg" "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/tq2eUGT.jpg" new file mode 100644 index 00000000..4cf3d227 Binary files /dev/null and "b/notes/Bear Notes/The Precarious Legacy of the iPhone 8 \342\200\224 The Psalms/tq2eUGT.jpg" differ diff --git a/notes/Bear Notes/The Psalms' 2020 (Draft).md b/notes/Bear Notes/The Psalms' 2020 (Draft).md new file mode 100644 index 00000000..a4324184 --- /dev/null +++ b/notes/Bear Notes/The Psalms' 2020 (Draft).md @@ -0,0 +1,122 @@ +# The Psalms' 2020 (Draft) + +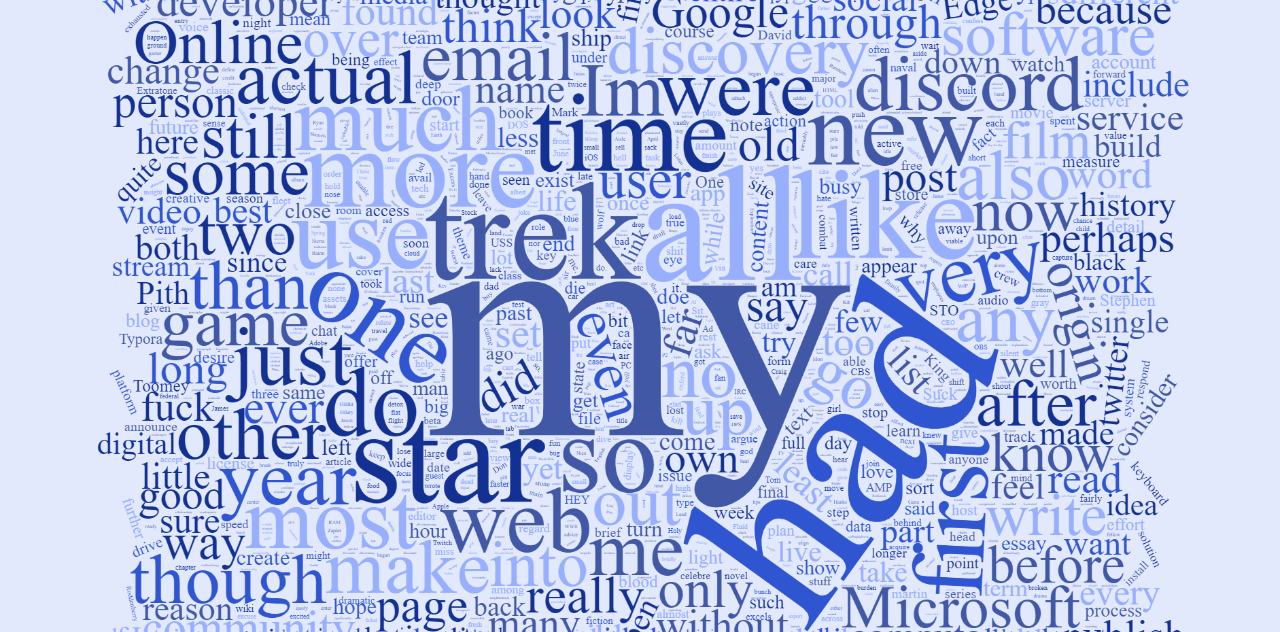 + +## Screens and software subjects big & small discussed within my most moderate 35,000 words ever. + +Believe it or not, the cyclical cold season has once again come to pass! Oh, the [W̘͖̯E̟̹À̫T̯̫͓̪̮͓̼H̝E̪͇̰̘̖͍ͅŔ͍̖̱̟̪](https://youtu.be/qg0ZPmSux_4)!! If the past two days on Twitter were any indication, it is indeed time for a year's-end retrospective on what has been published on this Web Site. Given the extent of the attention I've devoted to bilge.world this past year - both technically and editorially - I thought it would be nice to chuck in a bit more in the pursuit of whacking myself on the back. I understand that 2020 has been one helluva catastrophic marker of linear time for just about everyone on Earth - as I write this on its last day, the [inexplicable news of MF Doom's death](https://www.complex.com/music/2020/12/mf-doom-dead-49) has just surfaced and has already hit my adjacent communities remarkably hard. + +
+ +## Spring + +The earliest archive.org capture of this particular Writeas blog dates back only to [May 1st of this year](https://web.archive.org/web/20200501203946/https://bilge.world/), but its stylistic contrast with the site's current version is immense. I believe I was using [Max Henderson's Anxiety theme](https://write.as/themes/anxiety) virtually stock, albeit with some color rearrangements. By the [29th](http://web.archive.org/web/20200529000327/https://bilge.world/), I had already arrived upon the colors you see today. "[You Know I'm Blogging](https://bilge.world/you-know-im-blogging)" was just a meta update to say *howdy* after such a long drought, but "[Dirty Dave's Poweruser Tips](https://bilge.world/poweruser-tips-software-shortcuts)" actually proved popular (for me) among my newly-connected Fedi crowd, especially, thanks to [an annotated share by Writeas on Twitter](https://twitter.com/writeas__/status/1252704432497836034). Honestly, it was entirely worth it just for the accomplishment of [exposing my platform peer Dino Bansigan to **Alt+D**](https://journal.dinobansigan.com/dirty-daves-poweruser-tips-david-blue). Writeas Community Manager CJ Eller and I began corresponding, which led to [an interview](https://write.as/community/chat-with-david-blue) regarding writing tools, managing fiddly compulsions, and the wonder of Markdown. + +> I think I'm just now coming around to understanding personal blogging and the freedom that entails. It's been a long while since I've had the urge to write about things on which I don't consider myself at least somewhat of an authority. I think – like many people – I originally just used my personal blog as a guinea pig for messing around with themes, and I'm just now actually catching up on some of the lesser items on my “to-write” list. + +I dug deep in my long-overdue todo list for "[Z̴͏a͞l͟g͝o͏ ̕G͟͝e͞n͞҉è̛ŗ͡a͝͞t̴o҉r͞ for iOS](https://bilge.world/zalgo-generator-ios-app-review)," which - along with [my 2018 review of Unichar](https://bilge.world/unichar-for-ios-app-review) - I felt was a necessary celebration of digital text as a weapon, which is very dear to me. "[Preferred Writing Instruments](https://bilge.world/preferred-writing-instruments)" was probably the singular analog departure *The Psalms* will ever see and my condensed commentary on [*Star Trek: Picard*](https://bilge.world/star-trek-picard-review), [*Star Trek: Discovery*](https://bilge.world/star-trek-discovery-review), and [the current state of *Star Trek: Online*](https://bilge.world/star-trek-online) should fulfill all need mention of science fiction, here, for a very long time. Given [yesterday's news regarding Slack's acquisition](https://www.theverge.com/2020/12/1/21719666/salesforce-slack-acquisition-purchase-announced), perhaps I should feel revalidated in my insistence that "[Discord is Better Than Slack](https://bilge.world/discord-slack-comparison)," but a developer friend of mine actually addressed my curiosity regarding why startups never mention Discord as a workplace option, despite its identical functionality: it's all about fuckin' *enterprise security*. + +I had wanted to write about [*The Langoliers*](https://letterboxd.com/film/the-langoliers/) - Stephen King's flop of a made-for-TV movie/extended *Twilight Zone* episode - for a long while. It was probably the first program I watched as a child that genuinely disturbed me and always returns to my thoughts by barren-ish experiences. I had planned to watch the whole three hours all the way through and write whatever came to mind, but I only made it halfway or so in "[Craig Toomey's Coronavirus](https://bilge.world/the-langoliers-stephen-king)" before I ran completely out of steam. I dropped what I had without revision, to this day. Perhaps one day I shall return... + +> In the right hour, the woodland springtime metamorphic processes of the neighboring Lake Geneva suburb’s in-betweens were in a paused state – the toads again hushed; the crickets tired, and the human populace, too. In the right hour, the fickle wind and the social owls were the only sound, and nothing moved but the sparse, light-footed doe in careful segments with her fawn. + +These two sentences begin the sixth chapter of my indefinitely-postponed novel, [*Blimp's Burden*](https://github.com/extratone/pith), and are almost certainly my best writing, ever. My vanity simply wouldn't allow them to go forgotten, so I [published the chapter as an excerpt on *The Psalms*](https://bilge.world/blimps-burden-chapter-6). It may seem an intimidating wall of text, but give it your attention and you'll be amused, I think. + +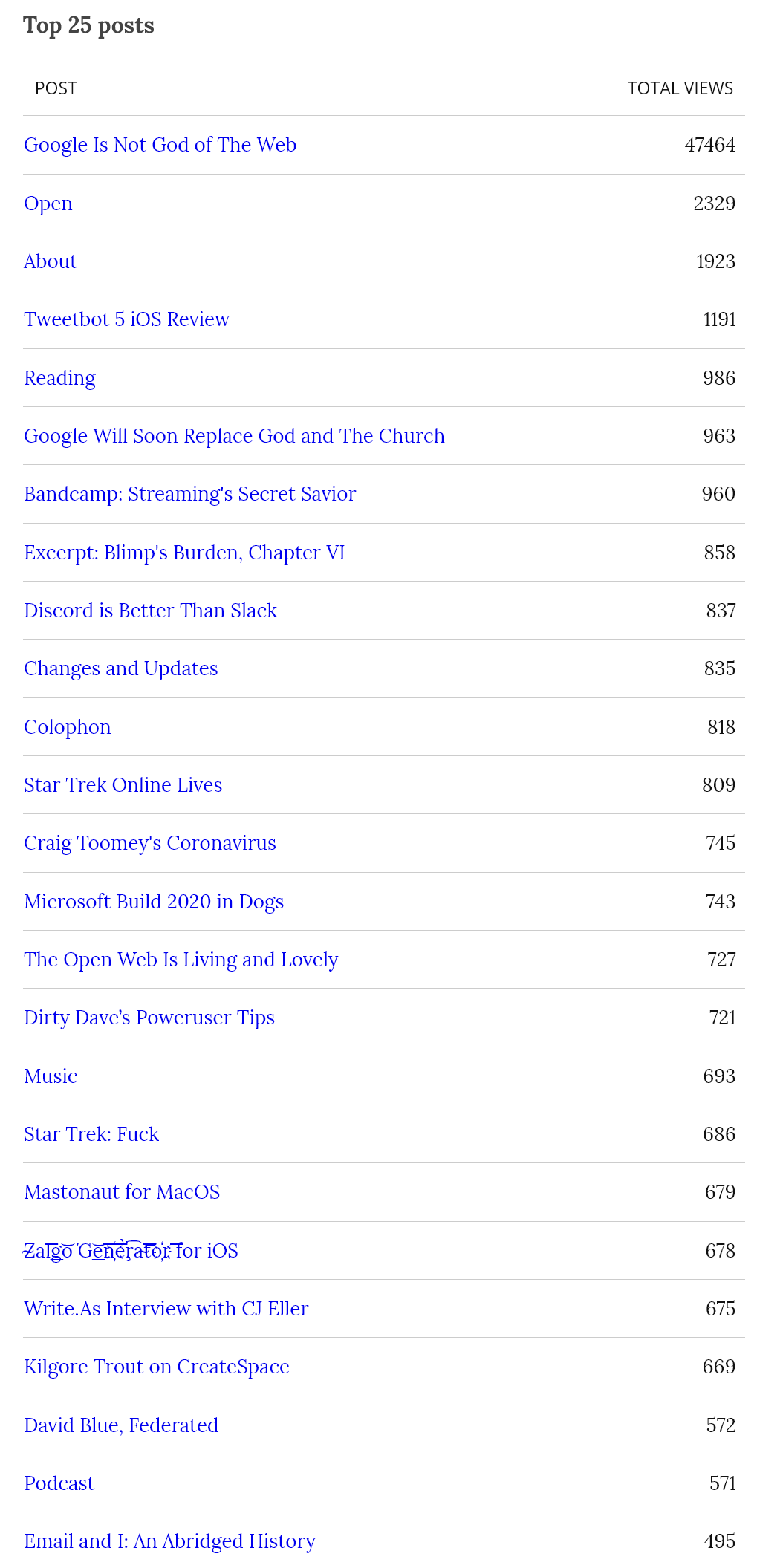 + +## Summer + +The general, distinctly 21st-century wisdom regarding one's content popularity seems to be something like *you never know what's going to blow up*. I experienced this phenomena quite severely for the first time in my writing life after posting "[Google Is Not God of The Web](https://bilge.world/google-page-experience)" on [HackerNews](https://news.ycombinator.com/item?id=23383548) right before going out-of-touch for some 13 straight hours amidst a local BLM protest. I did not intend to write a baity title, nor did I expect anything to come of sharing it, there - I only did so simply because the thought crossed my mind. Honestly, it is probably the least-considered content I'd ever published on *The Psalms* - I only put it up when I did because I knew *all* of my energy was about to be absorbed for an entire weekend or more. I only started the thing because I felt it important to raise a small voice in reminder that tech media had started using language when discussing code/online development which had begun to assume the existence of a single, objective standard of measure for *good web design*. (Fuck your bootstrap baloney, [*The Drywall Website*](https://iowa.neocities.org/test) was a *real* *paradigm shit*.) + +And yet... That single goddamned post would end up accruing more pageviews than all of my writing on the web, combined (I suspect.) Tens of thousands of words were written in hundreds of comments, which I did my best to parse in [a *for the hell of it* substack email](https://davidblue.substack.com/p/witness-google-god-and-local-history): + +> Out of everything I’ve shared on *Bilge* recently, it’s hilarious that this particular post - on which I spent a cumulative total of perhaps 25 minutes writing and made less effort than ever to edit - became probably my most widely-shared work of all time. + +Once again, I *am* thankful for the readership and correspondence, I just *really fucking wish* it had been on literally *anything* else I've written about technology. + +In retrospect, it really is bonkers that I was excited about yet another vague promise to *re-imagine email* in the days surrounding [the launch of Basecamp's HEY](https://www.theverge.com/2020/6/15/21286466/hey-email-basecamp-price-availability-platforms-launch), but I feel it's important to nurture what paltry genuine enthusiasm remains about technology and the future, so I decided to respond to the service's homepage prompt, asking interested visitors to write in with their own thoughts on email. I spent a morning clacking out "[Email and I: An Abridged History](https://bilge.world/hey-email-history)," which I hope will one day serve as a part-foundation for a thorough, composed extension to my [*Why I Write About Technology*](https://gist.github.com/extratone/91ebe16b0c620f309e70b40f5d4dcd9e) series. This would be *The Psalms* last relevant work for a few months, while I played around with [an experimental WordPress website](https://www.davidblue.wtf/wordpress-experimentation/) and dove into [*fuckin' school*](https://www.davidblue.wtf/college-return/) for the first time in seven years. + +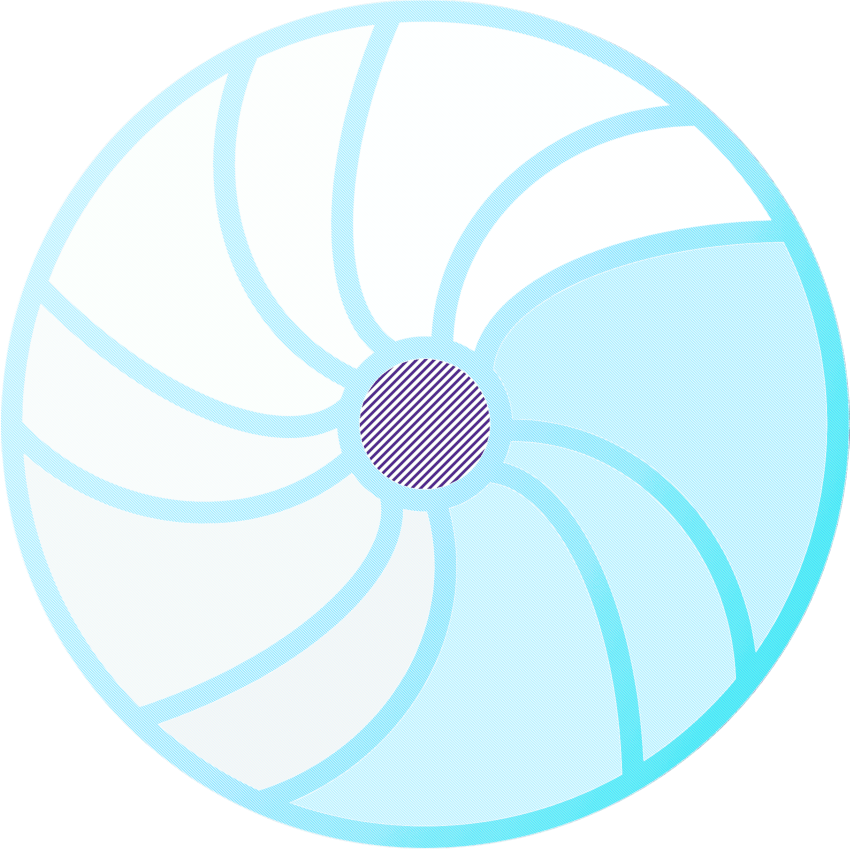 + +## Fall + +I returned in late September with a partial recount of what I'd discovered: "[Indulging Once More in Digital Excess](https://bilge.world/digital-excess)." After exploring the concept of [*Digital Gardens*](https://tomcritchlow.com/blogchains/digital-gardens/), buying [my first pro-ready desktop PC](https://www.davidblue.wtf/hp-envy-desktop/) in 10 years, playing with Big Boy Adobe software for the first time, and reading [a delightful book](https://www.hup.harvard.edu/catalog.php?isbn=9780674417076) documenting the history of word processing, I arrived upon the term *Software Historian* with which to partially describe myself. It doesn't mean all that much at the moment, but will no doubt be explored imminently on this Web Site. + +Addressing "[The Social Dilemma's Dilemma](https://bilge.world/the-social-dilemma)" and "[Software Thanksgiving](https://bilge.world/big-thank)" in detail would be awfully redundant, but I would at least note how they represent diametrically opposed perspectives through which to discuss this stuff. Going forward into 2021, I think it's important that I hold short on the progress I've made in moderating my voice since 2018. *Digestibility* is not a virtue I am at all interested in, nor is it an appropriate one in which to invest any more effort. Any non-*radical* argument will inevitably prove enormously unoriginal, which makes for a huge waste of all our time. For the moment, that's all the editorializing I've done. + +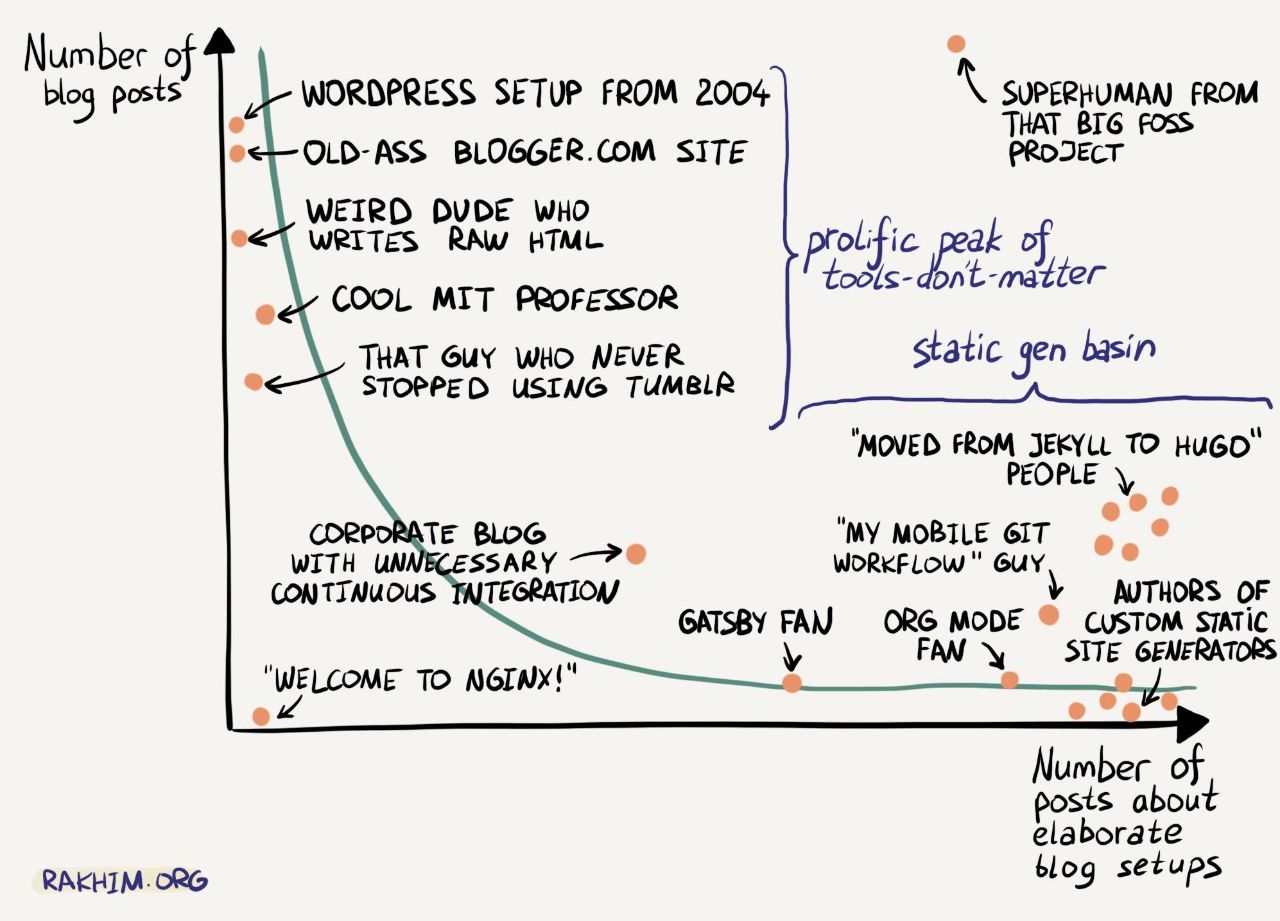 + +## Three Point Oh + +Somewhere along the research process for one of my school essays, I came across the concept of academic [revision tracking and peer review via Git](https://www.davidblue.wtf/git-peer-review/). Having dipped my toe back in academia, any such circumvention of its penchant for the most archaic practices possible was naturally intriguing. *Just wait until I show these goons how to use hyperlinks.* Perhaps inevitably, I was launched into the Git-Gone Bender I'm still riding simply because I finally had ample reason to actually understand it. In just two months, I've created and forked a total of nearly 80 repositories on [my new GitHub account](https://github.com/extratone). Though I'd created one for each of my web properties ages ago, I had absolutely no idea how to actually make use of them, so they were all quickly abandoned. In the past two weeks or so, I think I've actually begun to get the hang of the system's core intricacies, best exemplified by [*The Psalms*' Repository](https://github.com/extratone/bilge) as it stands. + +The "automated" [Project Board](https://github.com/extratone/bilge/projects/1) I set up has so far been a very impressive replacement for the simple todo list. It tracks and categorizes Post Ideas in their various stages in my process: Mulling, Documentation (research,) Drafting, and actual Publication. Technical "[Issues](https://github.com/extratone/bilge/issues)" regarding the CMS and/or the Repository, itself, have also fit in these categories well, so far. Just today, I discovered that one can simply tag Issues by their number to describe [Commits](https://github.com/extratone/bilge/commits/main), which form an impressive revision-tracking changelog when aggregated together. I also figured out the general idea behind Milestones and set [*The Psalms*' first one](https://github.com/extratone/bilge/milestone/1) for my 27th birthday, next month. The goal is to have figured this all out well enough by then that I'm no longer randomly stumbling upon entire featuresets multiple times in a workday. [*Thinking in Public*](https://tomcritchlow.com/2020/07/23/thinking-in-public/) - as my friend Tom Critchlow and his crowd say - is what I'm trying to do here, I think. Obviously, this really means that you are more than welcome to hop the fuck in at any time, in any manner you'd prefer. If Git is still no more than a conundrum for you - as it was for me just weeks ago - I would suggest the directory for [In-Progress Drafts](https://github.com/extratone/bilge/tree/main/Drafts) as a starting point. + +https://t.co/qb7NsZ8os6 - May 1st of this year vs. today.
— ※ David Blue ※ (@NeoYokel) December 3, 2020
I had forgotten *a lot* more about CSS and HTML than I originally thought. glad I ended up comfortable enough to find myself with a completely original stylesheet before the end of the year, I suppose. pic.twitter.com/4QwlbEJECU
+ +[GitHub Actions](https://github.com/extratone/bilge/actions) are still more or less a mystery to me, but I am unbothered - *The Psalms*' seems to be producing green text instead of red, now, in the midst of whatever I set it up to do. I poked around the livestreams for "GitHub Universe" a few weeks ago, when dark mode and GitHub Discussions were opened up to more users. At the moment, I cannot imagine a single application for forums in the context of my blog's repository, but [I turned them on, anyway](https://github.com/extratone/bilge/discussions). It's very possible that all of the documentation-generating functions of Git are entirely unnecessary in this case, but - as I entrust GitHub with the eternal task of archiving such work - it is my hope that it will help me add further structure to my process. + +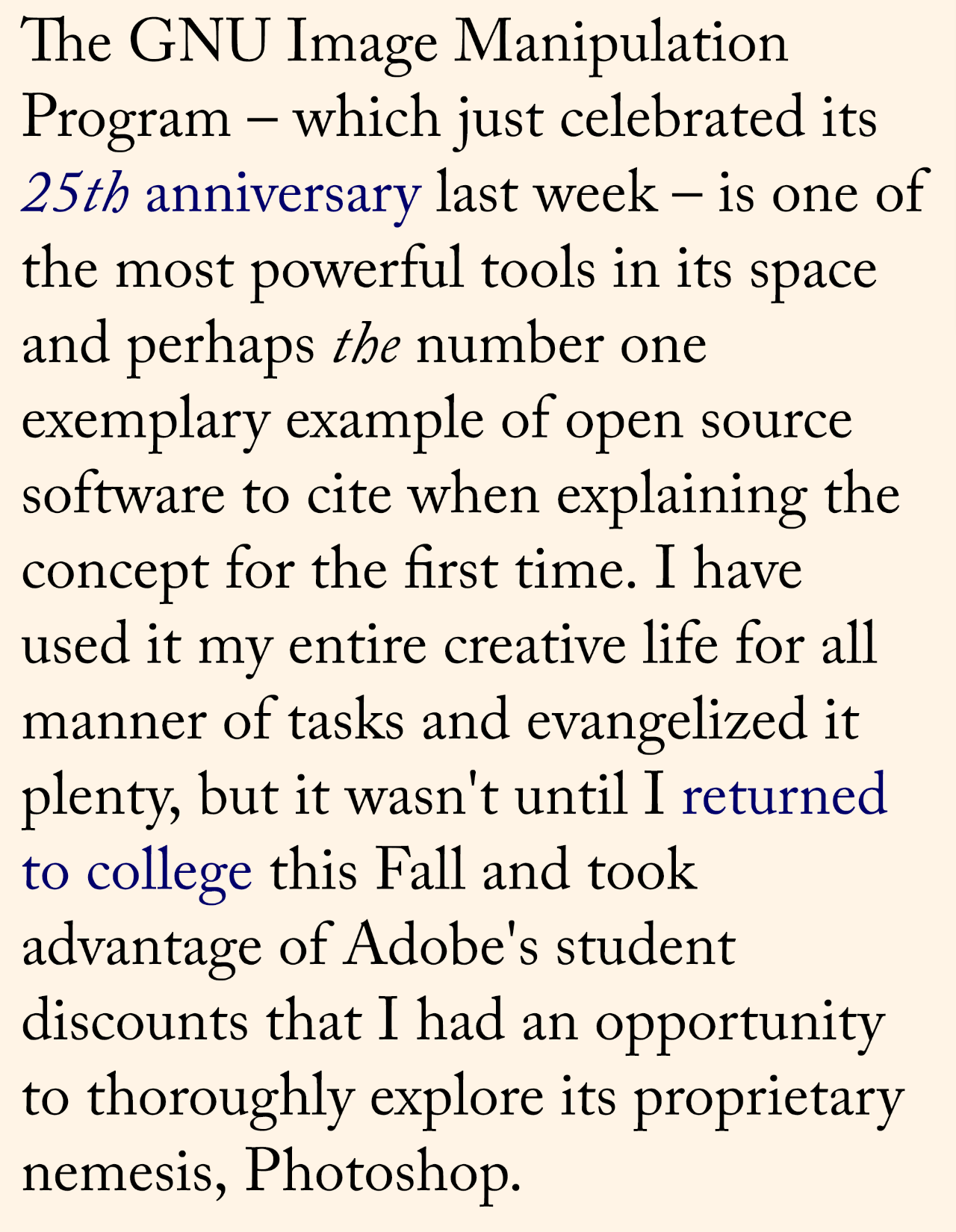 + +If you've found yourself in possession of a .edu email address and are interested in GitHub related experimentation, see [this list of discounted/free dev programs/services for students](https://education.github.com/pack/offers), which includes a free subscription to "GitHub Pro." (Apparently, equivalent to a $4/month service.) Adobe has [similar discounts](https://www.adobe.com/creativecloud/buy/students.html), as you’re probably already aware, which have allowed me to pursue my goal of learning InDesign. So far, I’ve created a sort of [commemorative 404 page for *The Drynet*](https://www.behance.net/gallery/104230341/Four-Oh-Four) and a [font showcase of Georgia](https://www.behance.net/gallery/104229721/Moms-Georgia-Type-Specimen) for my mother. The corresponding posts for this *will not* be imported from WTF here. As much as I’d love to continue discussing the current state of Adobe apps, I’ve realized 1) I am quite distinct from a *professional user*, which is why I genuinely find GIMP more useful than Photoshop, for instance, and 2) given the length of my to-write list considering my current pace, I don’t know if I’d manage to get around to it in this lifetime. + +## Coming Soon + +For better or worse, all of these new exploratory avenues have so far led directly away from the business of *actually writing* + +- - - - + +## GitHub + +- [x] [The Project Board](https://github.com/extratone/bilge/projects/1) +- [x] [In-Progress Drafts](https://github.com/extratone/bilge/tree/main/Drafts) +- [ ] [October 18th, 2018 Capture](http://web.archive.org/web/20181101000000*/bilge.world) +- [x] The [list of discounted/free dev programs for students on GitHub](https://education.github.com/pack/offers). +- [x] [May 1st Capture](https://web.archive.org/web/20200501203946/https://bilge.world/) +- [ ] Migrating WTF here, moving the domain to a NeoCities landing page. (Gonna really miss those redirects tho...) +- [ ] [GitWord](https://github.com/vigente/gerardus/wiki/Integrate-git-diffs-with-word-docx-files) ([My backup Gist](https://gist.github.com/extratone/72ad6ae736ae871fb3026bf73f229750)) +- [ ] [The Bilge Wiki](https://github.com/extratone/bilge/wiki), especially "[David Blue on iPhone & iOS](https://bit.ly/dbipwiki)" + [David Blue on Television & Film](https://bit.ly/dbtvwiki) +- [x] Let me loose with a .edu email account and I *will* find new and interesting ways of utilizing student discounts. (I guess I would normally be paying $4/month for the same features?) + + +- [x] "[You Know I'm Blogging](https://bilge.world/you-know-im-blogging)" - April 17th +- [x] "[Dirty Dave's Poweruser Tips](https://bilge.world/poweruser-tips-software-shortcuts)" - April 21st +- [x] "[Preferred Writing Instruments](https://bilge.world/preferred-writing-instruments)" - April 29th +- [x] "[Star Trek: Jowls](https://bilge.world/star-trek-picard-review)" - May 3rd +- [x] "[Writeas Interview With CJ Eller](https://bilge.world/david-blue-interview)" - May 11th +- [x] "[Star Trek: Fuck](https://bilge.world/star-trek-discovery-review)" - May 12th +- [x] "[Z̴͏a͞l͟g͝o͏ ̕G͟͝e͞n͞҉è̛ŗ͡a͝͞t̴o҉r͞ for iOS](https://bilge.world/zalgo-generator-ios-app-review)" - May 15th +- [ ] "[Microsoft Build 2020 in Dogs](https://bilge.world/microsoft-build-2020-edge-browser)" - May 19th +- [x] "[Craig Toomey's Coronavirus](https://bilge.world/the-langoliers-stephen-king)" - May 20th +- [x] "[Discord is Better Than Slack](https://bilge.world/discord-slack-comparison)" - May 24th +- [x] "[Star Trek Online Lives](https://bilge.world/star-trek-online)" - May 25th +- [x] "[Excerpt: Blimp's Burden, Chapter VI](https://bilge.world/blimps-burden-chapter-6)" - May 26th +- [ ] "[Changes and Updates](https://bilge.world/changes-updates)" - May 27th +- [x] "[Google Is Not God of The Web](https://bilge.world/google-page-experience)" - June 1st +- [x] "[Email and I: An Abridged History](https://bilge.world/hey-email-history)" - June 22nd +- [ ] "['Greyhound''s Top-Side Pot Shot](https://bilge.world/greyhound-tom-hanks)" - July 29th +- [ ] "[Indulging Once More in Digital Excess](https://bilge.world/digital-excess)" - September 24th +- [ ] "[The Social Dilemma's Dilemma](https://bilge.world/the-social-dilemma)" - October 19th +- [ ] "[Software Thanksgiving](https://bilge.world/big-thank)" - November 25th +- [ ] ~35,000 words, ~2 hours of reading. +- [ ] Most Overdue Drafts! +- [ ] [Dinkus Discord channel](https://bit.ly/extdinkus) + (I may or may not have made the *Extratone* Discord totally uninhabitable with automated posts.) + +d + +### Archive? + +- [ ] [David Blue Draws Gallery](https://www.notion.so/rotund/7f4664a737d1429eb94d33cb3486e9e0?v=aad96df288034d2dbe4dd667d6b7f543) +- [ ] Imported "[Through an iPhone 4's Lens](https://bilge.world/iphone4-photography)" +- [ ] [Tevin *Futureland* ep0q0isode](https://anchor.fm/extratone/episodes/Esporting-Psycholiterates--Favored-of-Email-en398f/a-a3vklt8) + +## Notes on Upcoming Work + +- [ ] [Siri Shortcuts Repository](https://github.com/extratone/shortcuts) +- [ ] [iPhone 12 Pro Max Review](https://github.com/extratone/bilge/issues/45) +- [ ] [Underdocumented iOS Manuevers](https://github.com/extratone/bilge/issues/56) +- [ ] [The Notion Cult](https://github.com/extratone/bilge/issues/12) + +#meta #iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/Walt Mossberg First Generation iPhone Review.md b/notes/Bear Notes/Walt Mossberg First Generation iPhone Review.md new file mode 100644 index 00000000..5ea358c1 --- /dev/null +++ b/notes/Bear Notes/Walt Mossberg First Generation iPhone Review.md @@ -0,0 +1,40 @@ +# Walt Mossberg First Generation iPhone Review +Walt Mossberg’s First Generation iPhone Review.mp4 +## Transcription +Hi this is what Maasberg personal technology columnist from the Wall Street Journal and this is the week for months people have been going crazy about this forthcoming device called the iPhone from Apple. +Here it is it goes on sale Friday at 6:00 PM. +And in the 16 years I have been reviewing tech products, I cannot remember any product having this kind of hype and high expectations surrounding it. +So it was quite interesting for me to be testing it the last two weeks I conducted my tests in a number of cities on both. +At East and West coasts I used it in airports and Starbucks and my house and office and Fenway Park in Boston on streets and. +::It is certainly the most beautiful and the most radical smartphone or handheld computer I have ever tested.:: +Starting with the physical you can see it's very thin. +It's actually thinner than, for instance, the Samsung Blackjack, which is quite a skinny phone. +And yet it has this enormous 3 1/2 inch screen which takes up most of the front of it. +It does not have a keyboard, it uses a virtual keyboard on the screen and when this was first announced by Apple in January, when the phone was disclosed, I was among many, many people who thought this was a real dealbreaker feature. +I have to say that. +Three days into testing the thing I wanted to throw it out the window because the keyboard was so difficult to use and I was so slow and making so many errors on it, but. +Five days in I suddenly found that I could type as well as fast as I have been typing on my trio's physical keyboard for years, so my conclusion is that the keyboard issue on the iPhone is a non issue. +The phone has a tremendous web browser. +The best I have ever seen on a handheld device. +It actually shows you the real layout and the real totality of the web pages you're looking at. +It doesn't rearrange them or stack them or do all the funny things that you're used to on a phone. +And if you want to zoom in and read a section of a web page, you just double tap with your finger. +The email program on it is also very PC or computer like it shows you a preview of the email and if, let's say there's a photo that's been sent in the email that photo you don't have to click separately to see it. +It shows up right there in the email. +The phone part of it is fine. +I wouldn't say it was the best voice quality I've ever heard on a phone, but it was OK. +The big drawback to the iPhone, in my opinion, is the fact that it only runs on one carrier in the United States, and that's AT&T. Apple, an AT&T, did an exclusive deal. It is not a short term exclusive deal either. I do not expect anytime in the foreseeable future. +To see this phone in a version from Verizon or Sprint or T-Mobile. And in fact it's locked so that you you can't even put in a T-Mobile card an use it on the T-Mobile Net. +Work now AT&T is the biggest carrier in the US, So why do I consider that a downside? Well, we all know that a coverage batteries, and if you're in an area without a great AT&T coverage, no matter how much you lust after this thing, it's not going to be very useful. If you can't make calls on it. The other problem is. +That's the data part of this, and remember I said it has a wonderful email program and a wonderful web browser, but those depend, of course on a fast connection to the Internet. +And this phone runs on a cell network that AT&T has that's called Edge, which is quite slow compared to the faster networks that even AT&T has on some other phones, and that Verizon and Sprint have on some treos and Blackberries and other competitors. So if you are relying on the cell phone network. +For Internet access on this, it is fairly slow. +The saving grace is. +the iPhone also has Wi-Fi. +There are a few other smartphones with that, but not many. +And Apple has designed the Wi-Fi part of this beautifully, so that when you walk into a room or a Starbucks or someplace where there's a Wi-Fi network, the phone knows about, it instantly switches you. +To Wi-Fi for your Internet access and of course. +That really flies, but when you're on the move, if you're in a cab, going through a city and you're trying to do web browsing or email, you're pretty much stuck with the slower cell phone network called Edge. Well, there's a lot more to say about this, and I've covered a lot more of the details in my column in the Wall Street Journal an on wsj.com, and on. +All things did come, but this is a fascinating product that I think raises the bar for all other smartphones. +This is Walt Mossberg, an I'll see you next week. +#reference #iP12PM \ No newline at end of file diff --git "a/notes/Bear Notes/Walt Mossberg First Generation iPhone Review/Walt Mossberg\342\200\231s First Generation iPhone Review.mp4" "b/notes/Bear Notes/Walt Mossberg First Generation iPhone Review/Walt Mossberg\342\200\231s First Generation iPhone Review.mp4" new file mode 100644 index 00000000..8e7ac445 Binary files /dev/null and "b/notes/Bear Notes/Walt Mossberg First Generation iPhone Review/Walt Mossberg\342\200\231s First Generation iPhone Review.mp4" differ diff --git a/notes/Bear Notes/iOS 14 Review Notes.md b/notes/Bear Notes/iOS 14 Review Notes.md new file mode 100644 index 00000000..c3a4bc07 --- /dev/null +++ b/notes/Bear Notes/iOS 14 Review Notes.md @@ -0,0 +1,41 @@ +# iOS 14 Review Notes +Dec 25, 2020 at 18:28 +- [ ] Apple needs to make app library folders renameable asap! + +- - - - + +- [ ] [iOS 14 Full Features List](https://www.apple.com/ios/ios-14-preview/features/) | Apple +- [x] [My WWDC 2020 Twitter Moment](https://twitter.com/i/events/1275137514609823751?s=20) +- [ ] "[How iPhone Hackers Got Their Hands on the New iOS Months Before Its Release](https://www.vice.com/en_us/article/5dzpxz/how-iphone-hackers-got-hands-on-new-ios-14-months-before-realease)" | *Motherboard* +- [ ] [r/iPhone](r/iPhone) +- [ ] [The r/iPhone Discord](https://discord.gg/iphone) +- [x] "[With iOS 14, Apple is finally letting the iPhone home screen get complicated](https://www.theverge.com/2020/6/24/21299924/ios-14-iphone-wwdc-2020-apple-home-screen-widgets-app-library-clips-pages-complexity)" | *The Verge* +- [ ] "[iOS 14 beta: It's complicated…finally](https://youtu.be/d6ai8ZGoYjY)" | *The Verge* on YouTube +- [x] **Additive instead of transformative**. +- [ ] "[iOS 14: Complete Guide to All the New Features](https://www.macrumors.com/roundup/ios-14/)" | *MacRumors* +- [ ] "[iOS 14 is filled with accessibility improvements](https://www.theverge.com/21302891/ios-14-accessibility-improvements-disabilities-hearing-blindness-motor-control)" | *The Verge* +- [ ] "[**What WWDC’s announcements mean for the future of the Mac**](https://www.theverge.com/2020/6/26/21304226/vergecast-podcast-apple-ios14-macos-wwdc-2020)" | *The Verge* +- [ ] Safari is super efficient at making PDFs for some reason. +- [ ] "[‘It Shouldn’t Be Hard to Get a Smoothie’, With Special Guest Dan Frommer](https://daringfireball.net/thetalkshow/2020/06/30/ep-288)" | *Daring Fireball* +- [x] "[iOS and iPad OS 14 public beta preview: something for everybody](https://www.theverge.com/21317904/ios-ipados-14-public-beta-preview-scribble-home-screen-widgets-apple-maps)" | *The Verge* +> For the iPhone, the overarching theme is that they're finally letting it get complicated. The home screen starts simple, but it can be complex if you want it to and Apple's also finally putting different elements on top of other elements so you can see Siri on top of your current screen instead of taking over the whole screen. +- [x] There is less to iOS 14 that's relevant to my life, personally, than any other release I remember, but that's okay. +- [ ] "[iOS 14 Hands-On Tour: Hidden Feature & Widgets!](https://youtu.be/z2wNLoS0-HU)" | Soldier Knows Best on YouTube +- [x] I'm pretty sure I do not think widgets belong on mobile... Then again, do I really *believe in* widgets in *any* context? +- [ ] "[What we know so far about iOS 14 from previewing the beta](https://www.theverge.com/2020/7/10/21319878/ios-14-public-beta-google-pixel-3a-samsung-note-20-event-rumors-vergecast-412)" | *The Verge* +- [x] "[17 Things You Can Do in iOS 14 That You Couldn’t Do Before](https://gizmodo.com/17-things-you-can-do-in-ios-14-that-you-couldn-t-do-bef-1844975020)" | *Gizmodo* +> Apple says Siri knows more facts than ever before, though it hasn’t offered up any specific examples of something it knows in iOS 14 that it didn’t know in iOS 13—you’ll have to try and test it out yourself. According to Apple, Siri now knows 20 times the number of facts that it did three years ago, and we haven’t been able to catch it out with anything so far. +### App Library +I'm not sure an alphabetized listing of all apps is really that useful. As iJustine mentioned in [episode 2 of her new podcast](), *Same Brain*, I think most users would use drag-down and search before looking through the list. +### iMessage +- [x] I'm afraid the text conversation pinning feature doesn't really apply to someone like myself, who never messages anyone but my girlfriend and my mom. +- [x] I thought you already could set a group message’s photo? +### Maps +- [x] [My guide to Columbia Missouri](https://guides.apple.com/?ug=ChNDb2x1bWJpYSBFc3NlbnRpYWxzEg0Irk0Q9afRyfG15J1VEg4Irk0QjZS24KKtt%2FaIARINCK5NEM3DzPio6eW5bxINCK5NEIiv4bu1z92nCA%3D%3D). +- [x] I thought you could already share your eta? Maybe I was thinking of Waze. +### CarPlay +- [x] Wallpapers for CarPlay is one of those features that didn’t occur to me to want, but I did/do. +- [ ] Though my personal experience with CarPlay has been limited, I can definitely imagine more layout options (“Horizontal status bar”) will be useful in *fringe* use cases. +### Reminders +- [ ] "[Everything Changing in Apple Notes and Reminders in iOS and iPadOS 14](https://www.macstories.net/stories/everything-changing-in-apple-notes-and-reminders-in-ios-and-ipados-14/)" | *MacStories* +#software #iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes).md b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes).md new file mode 100644 index 00000000..0ddb7bd9 --- /dev/null +++ b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes).md @@ -0,0 +1,53 @@ +# iPhone 12 Pro Max (Bear Notes) +[This user-generated Siri shortcut will help you record the police](https://www.inputmag.com/tech/hey-seri-im-getting-pulled-over-is-a-user-generated-shortcut-to-record-police-interactions) +README-2.md +[[Siri Voice Commands Documentation]] +[[iOS 14 Review Notes]] +- - - - + +- [ ] [Twitter thread](https://twitter.com/NeoYokel/status/1335313244949508101) +- [ ] [Twitter Moment](https://twitter.com/i/events/1335684188654231552?s=20) +- [ ] Full hardware specs +- [ ] [Photography Raindrop collection](https://raindrop.io/collection/15007128) +- [ ] It was completely irrational for me to get a new phone at this point in my life, considering I never leave the house. +- [ ] "[Wireless Charging Is a Disaster Waiting to Happen](https://debugger.medium.com/wireless-charging-is-a-disaster-waiting-to-happen-48afdde70ed9)" | *Debugger* +- [ ] Face ID... oh boy. +- [x] "Pacific Blue" +- [ ] The [battery percentage issue](https://www.imore.com/how-get-battery-percentage-your-iphone-x). +- [ ] I wish part of starting over included re-downloading all my shortcuts, tbh. ([Shortcuts Repo](https://github.com/extratone/shortcuts)) + +### Physical Controls +- [ ] [morganath on Mastodon regarding the Home Button](https://social.tchncs.de/@morganth/105330295770710815) +- [ ] “Gliding cursor” and 3D touch? +- - - - +[October 27th Vergecast](https://podcasts.apple.com/us/podcast/the-vergecast/id430333725?i=1000496205050) Notes +* LIDAR! +* “Higher typical screen brightness compared to the 12.” +* 2GB more RAM than 12 +* “The shiny one” +* Dieter compares 5G to a concept car. +* You have to use the 1x lens if you want to take a portrait in night mode. +/Photo%20Dec%209,%202020%20at%20173019.jpg) +/Photo%20Dec%209,%202020%20at%20173033.jpg) +* Idk what magsafe is in this contact. +* Get mom to get video of me on “wireless charging.” +“[Oh my god, what have I done](https://podcasts.apple.com/us/podcast/iphone-12-pro-max-review-leo-mikah-share-their-thoughts/id381972795?i=1000499104699).” - Leo Laporte +(Also, what the *fuck* is an “ESIM???”) +The test if the handset is too big: OPERA TOUCH MAYNE. +- [ ] My rule about the phone not leaving the house without a case on. +## Software (iOS) +- [ ] They finally removed that awful texture background from Apple Notes!! Holy shit!!! +- [ ] Does this make the case for Apple One? +- [ ] Is it really a walled garden anymore? +- [ ] It’s tempting to say that stereo playback on iPhone’s speakers may even be better than my Surface Laptop 2, but I’m too unsure of my own ear to commit to that. +- [ ] [lol](https://twitter.com/brendohare/status/1340096543181910018?s=21) +- [ ] Most of the shortcuts I use most are quick fixes for abilities that iOS *should* have natively (like shutting off wifi and bluetooth.) +- [ ] Not having to worry about dropping my phone in water is actually amazingly freeing... +- - - - +I’m afraid we must look back *yet again* to the [iPhone 4](https://youtu.be/cER36crkkVw) in this review, and not just because the 12 Pro has returned to those beautiful edges. (I don’t know if I’ve said it yet, but hey! This is the first truly beautiful phone I’ve owned in a long time. +“It’s scientific fact that a phone [screen] over 3.5 inches is impossible to use.” +- - - - + + #software +[12 great apps for your new iPhone in 2020 - The Verge](https://www.theverge.com/22187376/best-iphone-apps-2020-apple-ios) +#iP12PM \ No newline at end of file diff --git a/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/Photo Dec 9, 2020 at 173019.jpg b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/Photo Dec 9, 2020 at 173019.jpg new file mode 100644 index 00000000..cbad3ae5 Binary files /dev/null and b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/Photo Dec 9, 2020 at 173019.jpg differ diff --git a/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/Photo Dec 9, 2020 at 173033.jpg b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/Photo Dec 9, 2020 at 173033.jpg new file mode 100644 index 00000000..f78df205 Binary files /dev/null and b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/Photo Dec 9, 2020 at 173033.jpg differ diff --git a/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/README-2.md b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/README-2.md new file mode 100644 index 00000000..774dc3e0 --- /dev/null +++ b/notes/Bear Notes/iPhone 12 Pro Max (Bear Notes)/README-2.md @@ -0,0 +1,731 @@ +# `siri voice commands list` +# Table of Contents + + + [Wallet Siri Commands][page_wallet_siri_commands] + + [Web and YouTube Search Siri Commands][page_web_and_youtube_search_siri_commands] + + [More Phone and Notifications Siri Commands][page_more_phone_and_notifications_siri_commands] + + [Contacts Siri Commands][page_contacts_siri_commands] + + [Affective (Fun) Siri Commands][page_affective__fun__siri_commands] + + [Notes Siri Commands][page_notes_siri_commands] + + [Translation Siri Commands][page_translation_siri_commands] + + [Dictionary, Reference and Trivia Siri Commands][page_dictionary__reference_and_trivia_siri_commands] + + [Science Reference Siri Commands][page_science_reference_siri_commands] + + [Geography Siri Commands][page_geography_siri_commands] + + [Clock App Siri Commands][page_clock_app_siri_commands] + + [Clock Time Siri Commands][page_clock_time_siri_commands] + + [Timer Siri Commands][page_timer_siri_commands] + + [Stopwatch Siri Commands][page_stopwatch_siri_commands] + + [Alarm Siri Commands][page_alarm_siri_commands] + + [Podcast Siri Commands][page_podcast_siri_commands] + + [Calendar Siri Commands][page_calendar_siri_commands] + + [Maps and Geolocation Siri Commands][page_maps_and_geolocation_siri_commands] + + [Find My Siri Commands][page_find_my_siri_commands] + + [Reminder Siri Commands][page_reminder_siri_commands] + + [Weather Siri Commands][page_weather_siri_commands] + + [Live Radio Streaming Siri Commands][page_live_radio_streaming_siri_commands] + + [Music and Music Streaming Siri Commands][page_music_and_music_streaming_siri_commands] + + [Hourly News Update Siri Commands][page_hourly_news_update_siri_commands] + + [Finance Siri Commands][page_finance_siri_commands] + + [Useful Math Siri Commands][page_useful_math_siri_commands] + + [General Math Siri Commands][page_general_math_siri_commands] + + [Money Math Siri Commands][page_money_math_siri_commands] + + [Local and Navigtion Siri Commands][page_local_and_navigtion_siri_commands] + + [Flashlight Siri Commands][page_flashlight_siri_commands] + + [Audio Volume Settings Siri Commands][page_audio_volume_settings_siri_commands] + + [Screen Brightness Settings Siri Commands][page_screen_brightness_settings_siri_commands] + + [General Siri Commands][page_general_siri_commands] + + [Sports Siri Commands][page_sports_siri_commands] + + +## Wallet Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Show my wallet.** | Opens Wallet App and displays payment cards and/or transit passes. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + +## Web and YouTube Search Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Web Search dogs.** | Returns a response card showing Google web searches. | +| **YouTube Search cats.** | Returns a response card showing YouTube video searches. | +| **Bing search bicycles.** | Opens web page with Bing search results. | +| **Bing search dog food.** | Opens web page with Bing search results. | +| **Search Yahoo for silly straws.** | Opens web page with Yahoo search results. | +| **Search Duck Duck Go for running shoes.** | Opens web page with Duck Duck Go search results. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## More Phone and Notifications Siri Commands + +> **NOTE:** Silent Mode must be switched off for audio responses. + +| **Siri Command** | Response | +| :--- | :--- | +| **Read all notifications.** | Prompts user regarding notifications. | +| **Do I have missed calls?** | Checks for missed calls and returns the total number. | +| **Check voicemail.** | Checks and reads voicemails and saved messages. | +| **Read voicemails.** | Checks and reads voicemails and saved messages. | +| **Play voicemails.** | Checks and reads voicemails and saved messages. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Contacts Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Abby is my sister.** | Prompts the user for confirmation before adding the label "sister" to Abby's contact information. | +| **Bobby is my brother.** | Prompts the user for confirmation before adding the label "brother" to Bobby's contact information. | +| **Cammy is my lawyer.** | Prompts the user for confirmation before adding the label "lawyer" to Cammy's contact information. | +| **Danny is my plumber.** | Prompts the user for confirmation before adding the label "plumber" to Danny's contact information. | +| **Eddy is my broker.** | Prompts the user for confirmation before adding the label "broker" to Eddy's contact information. | +| **Freddy is my chef.** | Prompts the user for confirmation before adding the label "chef" to Freddy's contact information. | +| **My nickname is Goose.** | Prompts the user for confirmation before changing the personal name that Siri uses in social graphs and query discourse. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Affective (Fun) Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Hi.** | Siri replies. | +| **Hello.** | Siri replies. | +| **Hello world.** | Siri replies. | +| **Good morning.** | Siri replies. | +| **Good afternoon.** | Siri replies. | +| **Good evening.** | Siri replies. | +| **Good night.** | Siri replies. | +| **Good luck.** | Siri replies. | +| **Tell me a joke.** | Tells a joke. | +| **I am tired.** | Siri replies. | +| **I am drunk.** | Siri replies with recommendation for designated driver. | +| **Rock, paper, scissors.** | Replies to the challenge. | +| **I am feeling peckish.** | Replies with nearby restaurants. | +| **I am hungry.** | Replies with nearby restaurants. | + + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Notes Siri Commands + +> **NOTE:** Silent Mode must be switched off for audio responses. + +| **Siri Command** | Response | +| :--- | :--- | +| **Make a note.** | Prompts user for details about the new note. | +| **Note that the ocean is deep.** | Creates a new note "the ocean is deep". | +| **Note that the sky is blue.** | Creates a new note "the sky is blue". | +| **Make a note that the grass is green.** | Creates a new note "grass is green". | +| **Show notes.** | Display most recent notes. | +| **Show my notes.** | Display most recent notes. | +| **Read my notes.** | Display most recent notes, read the most recent note aloud, and prompts user for further action. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Translation Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **How do you say hello in German?** | Returns translation. | +| **How do you say wonderful in French?** | Returns translation. | +| **How do you say Germany in German?** | Returns translation. | +| **How do you say twenty-five in Greek?** | Returns translation. | +| **How do you say Happy Birthday in Chinese?** | Returns translation. | +| **How do you say Happy Birthday in Cantonese?** | Siri cannot translate into Cantonese yet. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Dictionary, Reference and Trivia Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Define geometry.** | Returns definition of the word geometry. | +| **Spell Mississippi.** | Returns the audio spelling of Mississippi. Requires Silent Mode off. | +| **Words that start with the letter G.** | Returns response card with partial list of words starting with G. | +| **Words that start with Q and end with R.** | Returns response card with partial list of words start with Q and end with R. | +| **Words that begin with A and end with S.** | Returns response card with partial list of words start with A and end with S. | +| **Words that begin with S and end with T.** | Returns response card with partial list of words start with S and end with T. | +| **Words that begin with the letter Q.** | Returns response card with partial list of words starting with Q. | +| **Words that begin with the letter Q.** | Returns response card with partial list of words starting with Q. | +| **Fruits that start with the letter A.** | Returns response card and (optionally) provides audio response listing the items. Requires Silent Mode off for audio response. | +| **Fruits that start with the letter T.** | Returns response card and (optionally) provides audio response listing the items. Requires Silent Mode off for audio response. | +| **Vegetables that start with the letter T.** | Returns response card and (optionally) provides audio response listing the items. Requires Silent Mode off for audio response. | +| **Words that rhyme with grace.** | Returns list of words that rhyme. | +| **Words that rhyme with hat.** | Returns list of words that rhyme. | +| **How many letters in Mississippi?** | Returns the total number of characters in Mississippi. | +| **How many characters in Mississippi?** | Returns the total number of characters in Mississippi. | +| **How many syllables in Mississippi?** | Returns the total number of syllables in Mississippi. | +| **What song is this?** | Prompts user to provide music for audio scan and search. | +| **World's oldest person.** | Returns information about the oldest person in the world. | +| **World's tallest person.** | Returns information about the tallest person in the world. | +| **World's shortest person.** | Returns information about the shortest person in the world. | +| **Airport code for Heathrow.** | Returns IATA Code for an airport or aerodrome. | +| **Show planes above me.** | Returns sky map with civial aviation data. | +| **What is the ceiling for a SR-71.** | Returns the ceiling for the aircraft. | +| **What is the ceiling for a Piper Cherokee.** | Returns the ceiling for the aircraft. | +| **Where can I buy some headphones?** | Suggests a visit to Apple.com. | +| **Tell me about the iPod touch.** | Suggests a visit to Apple.com. | +| **Tell me about Apple TV.** | Suggests a visit to Apple.com. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Science Reference Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Ask Wolfram Alpha about copper sulfate.** | Displays response card about molecular compound from Wolfram Alpha. | +| **Ask Wikipedia about copper sulfate.** | Displays response card about molecular compound from Wikipedia. | +| **Show structure of benzene.** | Displays stick stucture of molecular compound. | +| **Show structure of acetylcholine.** | Displays stick stucture of molecular compound. | +| **Chemical structure of nicotine.** | Returns the stick structure of molecular compound. | +| **Chemical formula for glucose.** | Returns the chemical formula of molecular compound. | +| **Chemical formula for hydrogen peroxide.** | Returns the chemical formula of molecular compound. | +| **What is the density of aluminum?** | Returns the density of aluminum. | +| **What is the atomic number of calcium?** | Returns the atomic number of calcium. | +| **What is the melting point of potassium?** | Returns the melting point of potassium. | +| **What is the boiling point of water?** | Returns the boiling point of water. | +| **Tungsten melting point.** | Returns the melting point of tungsten. | +| **Symbol for samarium.** | Returns the periodic table symbol for samarium. | +| **Atomic weight of platinum.** | Returns the atomic weight of platinum. | +| **Distance between Earth and Mars.** | Returns the distance between the two planets. May show telemetry graph. +| **Distance between Earth and the Sun.** | Returns the distance between two celestial objects. May show telemetry graph.| + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Geography Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **What is the capital of Georgia?** | Returns the capital of Georgia. | +| **What is the capital of Italy?** | Returns the capital of Italy. | +| **What is the population of Brazil?** | Returns the population of Brazil. | +| **Show me the flag of Bulgaria.** | Returns the flag of Bulgaria from a web search. | +| **What are the official languages of Canada?** | Returns the official languages of Canada. | +| **List the official languages of Canada.** | Returns the official languages of Canada. | +| **How tall is Mount Everest?** | Returns height of Mount Everest. | +| **Where is the highest feature on Earth?** | Returns latitude and longitude. May show details. | +| **Where is the lowest feature on Earth?** | Returns latitude and longitude. May show details. | +| **Where is the lowest feature on Europe?** | Returns the place name in Europe. May show details. | +| **Where is the highest feature on South America?** | Returns the place name in South America. May show details. | +| **Distance from Salesforce Tower to the Space Needle.** | Returns the distance by car and by bird. | +| **Distance from Tulsa to Chicago.** | Returns the distance by car and by bird. | +| **Distance from Dulles to Heathrow.** | Returns the distance by bird. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + + +## Clock App Siri Commands + + +### Clock Time Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Time.** | Returns the current time. | +| **Current time.** | Returns the current time. | +| **Time in London.** | Returns the current time in London. | +| **Daylight saving time.** | Provides the calendar start and end dates for DST. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +### Timer Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Show timer.** | Displays timer. | +| **Set timer for 20 minutes.** | Begins countdown from 20 minutes to zero. | +| **Stop timer.** | Pauses countdown. | +| **Pause timer.** | Pauses countdown. | +| **Resume timer.** | Resumes countdown. | +| **Reset timer.** | Pauses countdown and resets to start time. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +### Stopwatch Siri Commands + + + Works on iOS or watchOS. + +| **Siri Command** | Response | +| :--- | :--- | +| **Show stopwatch.** | Displays stopwatch. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +### Alarm Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Delete all alarms.** | Prompts user to confirm deletion of all alarms. | +| **Create new alarm.** | Creates a new alarm, then prompts for more details. | +| **Set alarm for fifteen-hundred hours.** | Creates alarm for 3:00 PM. | +| **Create new alarm for 5:30 PM.** | Creates alarm for 5:30 PM. | +| **Create pizza alarm for 7:45 PM.** | Creates alarm for 7:45 PM labeled "pizza". | +| **Change pizza alarm to 8:30 PM.** | Changes alarm labeled "pizza" to 8:30 PM. | +| **Rename pizza.** | Prompts user to provide a new name for the alarm. | +| **Delete pizza alarm.** | Deletes the alarm labeled "pizza". | +| **Set coffee alarm for 5:15 AM.** | Creates alarm for 5:15 AM labeled "coffee". | +| **Turn off the 5:15 alarm.** | Turns off the alarm for 5:15 AM. | +| **Set spinach alarm for 7:05 AM.** | Creates alarm for 7:05 AM labeled "spinach". | +| **Turn off spinach alarm.** | Turns off the spinach alarm. | +| **Turn on spinach alarm.** | Turns on the spinach alarm. | +| **Do I have a pizza alarm?** | Returns alarm labeled "pizza" if available. | +| **Disable all alarms.** | Toggles all alarms off. | +| **Turn off all alarms.** | Toggles all alarms off. | +| **Enable all alarms.** | Toggles all alarms on. | +| **Turn on all alarms.** | Toggles all alarms on. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Podcast Siri Commands + +> **NOTE:** Requires Apple Podcast App. + +| **Siri Command** | Response | +| :--- | :--- | +| **Subscribe to the Apple Keynotes podcast.** | Prompts user to confirm subscription to the podcast feed. | +| **Search WNYC podcasts.** | Returns search results of WNYC podcasts in Podcast App. | +| **Search WBUR podcasts.** | Returns search results of WBUR podcasts in Podcast App. | +| **Search Vox Media podcasts.** | Returns search results of Vox Media podcasts in Podcast App. | +| **Search Vergecast podcasts.** | Returns search results of Vergecast podcasts in Podcast App. | +| **Search Leo Laporte podcasts.** | Returns search results of Leo Laporte podcasts in Podcast App. +| **Play Apple Keynote podcast.** | Plays latest Apple Keynotes podcast episode. | +| **Play Pivot podcast.** | Plays the latest Pivot podcast episode in Podcast App. | +| **Play the latest Pivot podcast.** | Plays the last available podcast in feed. | +| **Play the earliest Pivot podcast.** | Plays the earliest available podcast in feed. | +| **Pause playback.** | Pauses podcast playback. | +| **Resume playback.** | Resumes podcast playback. | +| **Rewind 10 seconds.** | Rewinds podcast 10 seconds and resumes playback. | +| **Rewind 73 seconds.** | Rewinds podcast 73 seconds and resumes playback. | +| **Rewind 2 minutes.** | Rewinds podcast 2 minutes and resumes playback. | +| **Set playback to double speed.** | Changes podcast playback to 2.0x speed. | +| **Set playback to half speed.** | Changes podcast playback to 0.5x speed. | +| **Set playback to normal speed.** | Changes podcast playback to 1.0x speed. | +| **Set playback to one-point-five speed speed.** | Changes podcast playback to 1.5x speed. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Calendar Siri Commands + +> **NOTE:** Silent Mode must be switched off for audio responses. + +| **Siri Command** | Response | +| :--- | :--- | +| **Show all November events.** | Displays all events for the month of November. | +| **Read all November events.** | Displays all events for the month of November, provides a summary, before reading all events sequentially. | +| **Add a new event.** | Prompts user for date and time details about the new event. | +| **Add pizza event.** | Prompts user for date and time details about the new event labeled "pizza". | +| **Add a conference call to my calendar at 9 AM on Thursday.** | Adds new event labeled "conference call" at 9:00 AM on Thursday. | +| **Add training event at 6 AM tomorrow.** | Adds new event labeled "training" at 6:00 AM tomorrow. | +| **Add pizza party at 10 PM next Monday.** | Adds new event labeled "pizza party" at 10:00 PM next Monday. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Maps and Geolocation Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Location.** | Returns current location, with latitude and longitude. | +| **Current location.** | Returns current location, with latitude and longitude. | +| **Elevation.** | Returns elevation based on current location. | +| **Show Salesforce Tower in Maps.** | Displays information card of requested location. | +| **Show Salesforce Tower in Maps.** | Displays information card of requested location. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Find My Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Hide from my friends.** | Deactivates location-sharing beacon for friends. | +| **Stop hiding from my friends.** | Activates location-sharing beacon for friends. | +| **Ping my watch.** | Locates Apple Watch and activates ping sound. | +| **Find my watch.** | Locates Apple Watch and activates ping sound. | +| **Find my headphones.** | Locates account-linked Apple AirPods and activates ping sound. | +| **Find my iPad.** | Locates account-linked Apple iPad and activates ping sound. | +| **Find my Macbook.** | Locates account-linked Apple Macbook and activates ping sound. | +| **Find my Macbook Pro.** | Locates account-linked Apple Macbook Pro and activates ping sound. | +| **Where is my iPhone?** | Locates account-linked Apple iPhone and activates ping sound. | +| **Find my iPhone.** | Locates account-linked Apple iPhone and activates ping sound. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Reminder Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Show all reminders.** | Displays all reminders. | +| **Show all potato reminders.** | Displays all reminders with the keyword "potato" in the text (if availble). | +| **Show all reminders from February.** | Displays all reminders created in the month of February. | +| **Show all reminders from this month.** | Displays all reminders created during the current month. | +| **Delete all reminders.** | Prompts user to confirm deletion of all reminders. | +| **Remind me hourly.** | Prompts user for details about hourly reminder. | +| **Remind me every 2 hours.** | Prompts user for details about the reminder that repeats every 2 hours. | +| **Remind me daily.** | Prompts user for details about daily reminder. | +| **Remind me weekly.** | Prompts user for details about weekly reminder. | +| **Remind me every other week.** | Prompts user for details about the reminder that repeats every 2 weeks. | +| **Remind me every two weeks.** | Prompts user for details about the reminder that repeats every 2 weeks. | +| **Remind me monthly.** | Prompts user for details about monthly reminder. | +| **Remind me about bedtime every night at 8 o'clock.** | Creates a daily reminder at 10PM labeled "bedtime". | +| **Remind me when I leave Las Vegas.** | Prompts user for details about location-based reminder. | +| **Remind me to buckle up when leaving Las Vegas.** | Creates a new reminder labeled "buckle up" that reminds the user whenever leaving Las Vegas. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Weather Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Sunrise.** | Returns the sunrise time for a location. | +| **Sunrise time.** | Returns the sunrise time for a location. | +| **Sunset.** | Returns the sunset time for a location. | +| **Sunset time.** | Returns the sunset time for a location. | +| **Humidity.** | Returns the relative humidity for a location. | +| **Wind speed.** | Returns the wind speed for a location. | +| **Dew point.** | Returns the dew point temperature for a location. | +| **Pressure.** | Returns the barometric pressure for a location. | +| **High temperature.** | Returns the high temperature for a location. | +| **Low temperature.** | Returns the low temperature for a location. | +| **Wind chill.** | Returns the wind chill temperature for a location. | +| **UV index.** | Returns UV index for a location. | +| **Will it rain today?** | Answers the question based on chance of rain. | +| **Do I need an umbrella?** | Answers the question based on chance of rain. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Live Radio Streaming Siri Commands + + +> **NOTE:** Requires Apple Music App. + +> **NOTE:** The response card now prpvides a link to the audio stream provider (e.g., RADIO.COM, TUNEIN.COM, etc.) + + +| **Siri Command** | Response | +| :--- | :--- | +| **Play KNX 1070.** | Plays live stream of radio station KNX 1070 (Los Angeles). | +| **Play KFI 640.** | Plays live stream of radio station KFI AM 640 (Los Angeles). | +| **Play KCBS radio.** | Plays live stream of radio station KCBS 740 (San Francisco). | +| **Play KMOX 1120.** | Plays live stream of radio station KMOS 1120 (St. Louis). | +| **Play 1010 WINS.** | Plays live stream of radio station 1010 WINS (New York). | +| **Play 710 WOR.** | Plays live stream of radio station 710 WOR (New York). | +| **Play WBUR.** | Plays live stream of radio station WBUR 90.9 FM (Boston). | +| **Play 90.9 WBUR.** | Plays live stream of radio station WBUR 90.9 FM (Boston). | +| **Play RTHK radio one.** | Plays live stream of Cantonese language radio station RTHK Radio 1 (Hong Kong). | +| **Play RTHK radio three.** | Plays live stream of English language radio station RTHK Radio 3 (Hong Kong). | +| **Play Deutschlandfunk radio.** | Plays live stream of German language new radio station (Berlin). | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Music and Music Streaming Siri Commands + +> **NOTE:** Requires Apple Music App. + +| **Siri Command** | Response | +| :--- | :--- | +| **Search David Bowie in iTunes Store.** | Returns music search results for David Bowie. | +| **Search David Bowie music.** | Returns music search results for David Bowie. | +| **Play Eine Kleine Nachtmusik.** | Plays Mozart K. 525 (if available). | +| **Play Serenade Number 13 by Mozart.** | Plays Mozart K. 525 (if available). | +| **Set playback to double speed.** | Changes music playback to 2.0x speed. | +| **Set playback to half speed.** | Changes music playback to 0.5x speed. | +| **Set playback to normal speed.** | Changes music playback to 1.0x speed. | +| **Set playback to one-point-five speed speed.** | Changes music playback to 1.5x speed. | +| **Play a random song.** | Creates an in-memory playlist, then plays all songs, shuffled. | +| **Shuffle songs.** | Creates an in-memory playlist, then plays all songs, shuffled. | +| **Reshuffle music.** | Turns shuffle on. | +| **Turn shuffle on.** | Turns shuffle on. | +| **Turn shuffle off.** | Turns shuffle off. | +| **Play jazz.** | Plays all music tagged "jazz" in Music Library. | +| **Play bluegrass.** | Plays all music tagged "bluegrass" in Music Library. | +| **Play next song.** | Plays the next song on the playlist. | +| **Skip this song.** | Plays the next song on the playlist. | +| **Play previous song.** | Plays the previous song on the playlist. | +| **Play the last song.** | Plays the previous song on the playlist. | +| **Jump ahead 5 seconds.** | Seeks ahead 5 seconds and resumes playback. | +| **Seek ahead 5 seconds.** | Seeks ahead 5 seconds and resumes playback. | +| **Jump back 15 seconds.** | Seeks back 15 seconds and resumes playback. | +| **Seek back 15 seconds.** | Seeks back 15 seconds and resumes playback. | +| **Seek to 3 minutes and 21 seconds.** | Seeks to exactly 3 minutes 21 seconds and resumes playback. | +| **Jump to 3 minutes and 21 seconds.** | Seeks to exactly 3 minutes 21 seconds and resumes playback. | +| **Seek to 200 seconds.** | Seeks to exactly 3 minutes 20 seconds and resumes playback. | +| **Jump to 200 seconds.** | Seeks to exactly 3 minutes 20 seconds and resumes playback. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Hourly News Update Siri Commands + +> **NOTE:** Requires Apple Podcast App. + +| **Siri Command** | Response | +| :--- | :--- | +| **Play sports news from ESPN.** | Plays pre-recorded hourly news update from ESPN. | +| **Play business news from Bloomberg.** | Plays pre-recorded hourly news update from Bloomberg. | +| **Play business news from CNBC.** | Plays pre-recorded hourly news update from CNBC. | +| **Play NPR News.** | Plays pre-recorded hourly news update from NPR News. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Finance Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **What is the ticker symbol for Twitter?** | Returns the ticker symbol for Twitter. | +| **Home Depot stock price.** | Returns the stock price for Home Depot. | +| **Microsoft market cap.** | Returns the market capitalization for Microsoft. | +| **Verizon market capitaliztion.** | Returns the market capitalization for Verizon. | +| **Verizon 52-week high.** | Returns the highest price over the last 52 weeks. | +| **Verizon 52-week low.** | Returns the lowest price over the last 52 weeks. | +| **Clorox opening price.** | Returns the opening price for Clorox. | +| **Clorox closing price.** | Returns the closing price for Clorox. | +| **P/E ratio for Clorox.** | Returns the price-earnings ratio for Clorox. | +| **What is the yield for ticker symbol CLX?** | Returns the yield for Clorox. | +| **What is the current price of gold?** | *Commodity information is not available at this time.* | +| **What is the closing price of silver?** | *Commodity information is not available at this time.* | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## Useful Math Siri Commands + +| **Siri Command** | Response | +| :--- | :--- | +| **Heads or tails.** | Returns either heads or tails in a simulated coin flip. | +| **Flip a coin.** | Returns either heads or tails in a simulated coin flip. | +| **Pick a random number.** | Returns a random number between 0 and 100. | +| **Pick a number between 3 and 588.** | Returns a random number between 3 and 588. | +| **Pick a random color.** | Returns a random color swatch. | +| **Roll the dice.** | Returns two random numbers from a pair of simulated dice. | +| **Roll a die.** | Returns only one random number from a single simulated die. | +| **Is 881 prime?** | Answers whether a number is prime or not. | +| **Is 513 divisible by 9?** | Answers whether a number is divisible by another number. | +| **Prime factorization of 24.** | Retuns the prime factorization in exponent form, including prime factors and distinct factors. | + +> **NOTE:** This Markdown table was generated by [MarkdownTableMaker for Google Sheets][mtm]. + + + +## General Math Siri Commands + +| Math | **Siri Command** | Response | +| :--- | :--- | :--- | +| *5 = 4x + 3yrequesting support on how to "leverage discussions" to talk about pee pee and poo poo. #GitHubUniverse
— ※ David Blue ※ (@NeoYokel) December 8, 2020