diff --git a/lessons/pymt/cem.ipynb b/lessons/pymt/cem.ipynb
new file mode 100644
index 0000000..575fa35
--- /dev/null
+++ b/lessons/pymt/cem.ipynb
@@ -0,0 +1,480 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Coastline Evolution Model\n",
+ "\n",
+ "* Link to this notebook: https://github.com/csdms/pymt/blob/master/docs/demos/cem.ipynb\n",
+ "* Install command: `$ conda install notebook pymt_cem`\n",
+ "* Download local copy of notebook:\n",
+ "\n",
+ " `$ curl -O https://raw.githubusercontent.com/csdms/pymt/master/docs/demos/cem.ipynb`\n",
+ "\n",
+ "This example explores how to use a BMI implementation using the CEM model as an example.\n",
+ "\n",
+ "### Links\n",
+ "* [CEM source code](https://github.com/csdms/cem-old/tree/mcflugen/add-function-pointers): Look at the files that have *deltas* in their name.\n",
+ "* [CEM description on CSDMS](http://csdms.colorado.edu/wiki/Model_help:CEM): Detailed information on the CEM model."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Interacting with the Coastline Evolution Model BMI using Python"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Some magic that allows us to view images within the notebook."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "%matplotlib inline\n",
+ "\n",
+ "from tqdm import tqdm"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Import the `Cem` class, and instantiate it. In Python, a model with a BMI will have no arguments for its constructor. Note that although the class has been instantiated, it's not yet ready to be run. We'll get to that later!"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "cem = pymt.models.Cem()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Even though we can't run our waves model yet, we can still get some information about it. *Just don't try to run it.* Some things we can do with our model are get the names of the input variables."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.input_var_names"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We can also get information about specific variables. Here we'll look at some info about wave direction. This is the main input of the Cem model. Notice that BMI components always use [CSDMS standard names](http://csdms.colorado.edu/wiki/CSDMS_Standard_Names). The CSDMS Standard Name for wave angle is,\n",
+ "\n",
+ " \"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\"\n",
+ "\n",
+ "Quite a mouthful, I know. With that name we can get information about that variable and the grid that it is on (it's actually not a one)."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "angle_name = 'sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity'\n",
+ "\n",
+ "print(\"Data type: %s\" % cem.get_var_type(angle_name))\n",
+ "print(\"Units: %s\" % cem.get_var_units(angle_name))\n",
+ "print(\"Grid id: %d\" % cem.get_var_grid(angle_name))\n",
+ "print(\"Number of elements in grid: %d\" % cem.get_grid_number_of_nodes(0))\n",
+ "print(\"Type of grid: %s\" % cem.get_grid_type(0))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "OK. We're finally ready to run the model. Well not quite. First we initialize the model with the BMI **initialize** method. Normally we would pass it a string that represents the name of an input file. For this example we'll pass **None**, which tells Cem to use some defaults."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "args = cem.setup(number_of_rows=100, number_of_cols=200, grid_spacing=200.)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.initialize(*args)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Before running the model, let's set a couple input parameters. These two parameters represent the wave height and wave period of the incoming waves to the coastline."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import numpy as np\n",
+ "\n",
+ "cem.set_value(\"sea_surface_water_wave__height\", 2.)\n",
+ "cem.set_value(\"sea_surface_water_wave__period\", 7.)\n",
+ "cem.set_value(\"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\", 0. * np.pi / 180.)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The main output variable for this model is *water depth*. In this case, the CSDMS Standard Name is much shorter:\n",
+ "\n",
+ " \"sea_water__depth\"\n",
+ "\n",
+ "First we find out which of Cem's grids contains water depth. "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "grid_id = cem.get_var_grid('sea_water__depth')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "With the *grid_id*, we can now get information about the grid. For instance, the number of dimension and the type of grid (structured, unstructured, etc.). This grid happens to be *uniform rectilinear*. If you were to look at the \"grid\" types for wave height and period, you would see that they aren't on grids at all but instead are scalars."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "grid_type = cem.get_grid_type(grid_id)\n",
+ "grid_rank = cem.get_grid_ndim(grid_id)\n",
+ "print('Type of grid: %s (%dD)' % (grid_type, grid_rank))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Because this grid is uniform rectilinear, it is described by a set of BMI methods that are only available for grids of this type. These methods include:\n",
+ "* get_grid_shape\n",
+ "* get_grid_spacing\n",
+ "* get_grid_origin"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "spacing = np.empty((grid_rank, ), dtype=float)\n",
+ "\n",
+ "shape = cem.get_grid_shape(grid_id)\n",
+ "cem.get_grid_spacing(grid_id, out=spacing)\n",
+ "\n",
+ "print('The grid has %d rows and %d columns' % (shape[0], shape[1]))\n",
+ "print('The spacing between rows is %f and between columns is %f' % (spacing[0], spacing[1]))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Allocate memory for the water depth grid and get the current values from `cem`."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "z = np.empty(shape, dtype=float)\n",
+ "cem.get_value('sea_water__depth', out=z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Here I define a convenience function for plotting the water depth and making it look pretty. You don't need to worry too much about it's internals for this tutorial. It just saves us some typing later on."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "def plot_coast(spacing, z):\n",
+ " import matplotlib.pyplot as plt\n",
+ " \n",
+ " xmin, xmax = 0., z.shape[1] * spacing[0] * 1e-3\n",
+ " ymin, ymax = 0., z.shape[0] * spacing[1] * 1e-3\n",
+ "\n",
+ " plt.imshow(z, extent=[xmin, xmax, ymin, ymax], origin='lower', cmap='ocean')\n",
+ " plt.colorbar().ax.set_ylabel('Water Depth (m)')\n",
+ " plt.xlabel('Along shore (km)')\n",
+ " plt.ylabel('Cross shore (km)')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "It generates plots that look like this. We begin with a flat delta (green) and a linear coastline (y = 3 km). The bathymetry drops off linearly to the top of the domain."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "plot_coast(spacing, z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Right now we have waves coming in but no sediment entering the ocean. To add some discharge, we need to figure out where to put it. For now we'll put it on a cell that's next to the ocean."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Allocate memory for the sediment discharge array and set the discharge at the coastal cell to some value."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "qs = np.zeros_like(z)\n",
+ "qs[0, 100] = 1250"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The CSDMS Standard Name for this variable is:\n",
+ "\n",
+ " \"land_surface_water_sediment~bedload__mass_flow_rate\"\n",
+ "\n",
+ "You can get an idea of the units based on the quantity part of the name. \"mass_flow_rate\" indicates mass per time. You can double-check this with the BMI method function **get_var_units**."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.get_var_units('land_surface_water_sediment~bedload__mass_flow_rate')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.time_step, cem.time_units, cem.time"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Set the bedload flux and run the model."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "for time in tqdm(range(3000)):\n",
+ " cem.set_value('land_surface_water_sediment~bedload__mass_flow_rate', qs)\n",
+ " cem.update_until(time)\n",
+ "\n",
+ "cem.get_value('sea_water__depth', out=z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.time"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.get_value('sea_water__depth', out=z)\n",
+ "plot_coast(spacing, z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "val = np.empty((5, ), dtype=float)\n",
+ "cem.get_value(\"basin_outlet~coastal_center__x_coordinate\", val)\n",
+ "val / 100."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Let's add another sediment source with a different flux and update the model."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "qs[0, 150] = 1500\n",
+ "for time in tqdm(range(3750)):\n",
+ " cem.set_value('land_surface_water_sediment~bedload__mass_flow_rate', qs)\n",
+ " cem.update_until(time)\n",
+ " \n",
+ "cem.get_value('sea_water__depth', out=z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "plot_coast(spacing, z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Here we shut off the sediment supply completely."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "qs.fill(0.)\n",
+ "for time in tqdm(range(4000)):\n",
+ " cem.set_value('land_surface_water_sediment~bedload__mass_flow_rate', qs)\n",
+ " cem.update_until(time)\n",
+ " \n",
+ "cem.get_value('sea_water__depth', out=z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "plot_coast(spacing, z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.7.4"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/cem_and_waves.ipynb b/lessons/pymt/cem_and_waves.ipynb
new file mode 100644
index 0000000..a9f2b54
--- /dev/null
+++ b/lessons/pymt/cem_and_waves.ipynb
@@ -0,0 +1,602 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "# Couple two models in *pymt*\n",
+ "\n",
+ "In this exercise we will couple two component using *pymt*. We will couple the Coastline Evolution Model (CEM),\n",
+ "which models the transport of sediment alongshore, with a wave model that provides incoming wave direction and\n",
+ "characteristics.\n",
+ "\n",
+ "* Explore the base-case river simulation\n",
+ "* How does a river system respond to climate change?\n",
+ "* How do humans affect river sediment loads?\n",
+ "\n",
+ "## The Coastline Evolution Model (CEM)\n",
+ "\n",
+ "The Coastline Evolution Model (CEM) addresses predominately sandy, wave-dominated coastlines on time-scales ranging from years to millenia and on spatial scales ranging from kilometers to hundreds of kilometers. Shoreline evolution results from gradients in wave-driven alongshore sediment transport.\n",
+ "\n",
+ "At its most basic level, the model follows the standard *one-line* modeling approach, where the cross-shore dimension is collapsed into a single data point. However, the model allows the planview shoreline to take on arbitrary local orientations, and even fold back upon itself, as complex shapes such as capes and spits form under some wave climates (distributions of wave influences from different approach angles). The model works on a 2D grid.\n",
+ "\n",
+ "CEM has been used to represent varying geology underlying a sandy coastline and shoreface in a simplified manner and enables the simulation of coastline evolution when sediment supply from an eroding shoreface may be constrained. CEM also supports the simulation of human manipulations to coastline evolution through beach nourishment or hard structures.\n",
+ "\n",
+ "CEM authors & developers include:\n",
+ "* Andrew Ashton\n",
+ "* Brad Murray\n",
+ "* Jordan Slot\n",
+ "* Jaap Nienhuis and others.\n",
+ "\n",
+ "This version is adapted from a CSDMS teaching notebook, listed below. \n",
+ "It has been created by Irina Overeem, October 2019 for a Sedimentary Modeling course.\n",
+ "\n",
+ "### Key References\n",
+ "\n",
+ "Ashton, A.D., Murray, B., Arnault, O. 2001. Formation of coastline features by large-scale instabilities induced by high-angle waves, Nature 414.\n",
+ "\n",
+ "Ashton, A. D., and A. B. Murray (2006), High-angle wave instability and emergent shoreline shapes: 1. Modeling of sand waves, flying spits, and capes, J. Geophys. Res., 111, F04011, doi:10.1029/2005JF000422.\n",
+ "\n",
+ "\n",
+ "### Links\n",
+ "* [CEM source code](https://github.com/csdms/cem-old/tree/mcflugen/add-function-pointers): Look at the files that have *deltas* in their name.\n",
+ "* [CEM description on CSDMS](http://csdms.colorado.edu/wiki/Model_help:CEM): Detailed information on the CEM model."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Interacting with the Coastline Evolution Model BMI using Python"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import numpy as np\n",
+ "import matplotlib.pyplot as plt\n",
+ "from tqdm import tqdm"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Import the `Cem` model into your environment."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "cem = pymt.models.Cem()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Even though we can't run our model yet, we can still get some information about it. Some things we can do with our model are to get help, and get the names of the input variables or output variables. In looking at the "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.input_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We can also get information about specific variables. Here we'll look at some info about wave direction. This is the main input of the CEM model. What do you think the more conventional names for these variables are?\n",
+ "\n",
+ "| Conventional Name | Standard Name |\n",
+ "| :--------------------- | :------------------------------------------------------------------ |\n",
+ "| ??? | sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity |\n",
+ "| ??? | sea_surface_water_wave__period |\n",
+ "| ??? | sea_surface_water_wave__height |\n",
+ "\n",
+ "To help us out, we can get some additional information about each of the variables."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "angle_name = 'sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity'\n",
+ "\n",
+ "print(\"Data type: %s\" % cem.var_type(angle_name))\n",
+ "print(\"Units: %s\" % cem.var_units(angle_name))\n",
+ "print(\"Grid id: %d\" % cem.var_grid(angle_name))\n",
+ "print(\"Number of elements in grid: %d\" % cem.grid_node_count(0))\n",
+ "print(\"Type of grid: %s\" % cem.grid_type(0))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We now get the model ready for time stepping. Remember the lifecycle of the model is:\n",
+ "* *setup*\n",
+ "* *initialize*\n",
+ "* *update*\n",
+ "* *finalize*\n",
+ "\n",
+ "For this example we'll set up a simulation with a grid of 100 rows and 200 columns with a grid\n",
+ "resolution of 200.0."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "args = cem.setup(number_of_rows=100, number_of_cols=200, grid_spacing=200.)\n",
+ "cem.initialize(*args)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Changing a model's state\n",
+ "\n",
+ "Because the CEM model has input variables (unlike *HydroTrend* in the previous example), we\n",
+ "are able to change variables within the CEM model. The is done with the ***set_value***\n",
+ "method.\n",
+ "\n",
+ "For our first example we'll set the incoming wave height, period, and angle (in radians)."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.set_value(\"sea_surface_water_wave__height\", 1.5)\n",
+ "cem.set_value(\"sea_surface_water_wave__period\", 7.)\n",
+ "cem.set_value(\"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\", 0.0 * np.pi / 180.)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Grids\n",
+ "\n",
+ "This example is also different from the previous example in that it generates output that\n",
+ "is on a grid (as opposed to scalar data). The main output for CEM is *sea_water__depth*\n",
+ "and operates on a grid whose size we set when we *setup* this simulation.\n",
+ "\n",
+ "*pymt* models can have multiple grids. This allows for models to calculate some of\n",
+ "its state variables on scalars and others of 2D grids, for example. Models could also\n",
+ "maintain several grids of differing resolution. In *pymt* each grid has an ID\n",
+ "associated with it.\n",
+ "\n",
+ "We use the ***var_grid*** function to get the grid ID."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.var_grid(\"sea_water__depth\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Once we have a grid ID, we can then use the *pymt* *grid_* methods to get additional information\n",
+ "about each of the grids. Because this grid is uniform rectilinear (as returned by the\n",
+ "***grid_type*** method below), it is described by a set of methods that are only available\n",
+ "for grids of this type. These methods include:\n",
+ "* get_grid_shape\n",
+ "* get_grid_spacing\n",
+ "* get_grid_origin"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "print(\"Grid type: {0}\".format(cem.grid_type(2)))\n",
+ "print(\"Grid rank: {0}\".format(cem.grid_ndim(2)))\n",
+ "print(\"Grid shape: {0}\".format(cem.grid_shape(2)))\n",
+ "print(\"Grid spacing: {0}\".format(cem.grid_spacing(2)))\n",
+ "print(\"Grid origin: {0}\".format(cem.grid_origin(2)))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Now that we know a little more about the grid, let's plot it with the current values of\n",
+ "water depth.\n",
+ "\n",
+ "Here I define a convenience function for plotting the water depth and making it look\n",
+ "pretty. You don't need to worry too much about it's internals for this tutorial.\n",
+ "It just saves typing later on."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "def plot_coast(spacing, z):\n",
+ " import matplotlib.pyplot as plt\n",
+ "\n",
+ " xmin, xmax = 0., z.shape[1] * spacing[0] * 1e-3\n",
+ " ymin, ymax = 0., z.shape[0] * spacing[1] * 1e-3\n",
+ "\n",
+ " plt.imshow(z, extent=[xmin, xmax, ymin, ymax], origin=\"lower\", cmap=\"ocean\")\n",
+ " plt.colorbar().ax.set_ylabel(\"Water Depth (m)\")\n",
+ " plt.xlabel(\"Along shore (km)\")\n",
+ " plt.ylabel(\"Cross shore (km)\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "z = np.empty(cem.grid_shape(2), dtype=float)\n",
+ "\n",
+ "cem.get_value(\"sea_water__depth\", out=z)\n",
+ "plot_coast(cem.grid_spacing(2), z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We have alredy set the incoming wave characteristics, but we've yet to add any sediment to\n",
+ "the system.\n",
+ "\n",
+ "From the list of input variables, can you tell which one might be the one we're looking\n",
+ "for?"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "for name in cem.input_var_names:\n",
+ " print(\"{0:70s} [{1}]\".format(name, cem.var_units(name)))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The one we want is *land_surface_water_sediment~bedload__mass_flow_rate*. Now have a look\n",
+ "at what sort of grid its defined on and how we could change its value."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.var_grid(\"land_surface_water_sediment~bedload__mass_flow_rate\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Notice that it's on the same grid as water depth. To add sediment, we need to:\n",
+ "1. allocate an array to hold sediment discharge values\n",
+ "2. set values of the sediment discharge array\n",
+ "3. pass this new sediment discharge array into CEM\n",
+ "\n",
+ "I've placed the sediment discharge in the horizontal center of the grid (column 100 of 200) and\n",
+ "along the bottom. The sediment will be routed in a straight line until it hits the coast.\n",
+ "\n",
+ "You don't need to do this, though. Feel free to add sediment in another location (or even multiple\n",
+ "locations!) or change the amount of sediment. Note that the CEM model is sensitive to the balance of\n",
+ "wave energy to sediment input. If you go too far from the defaults, you may get some \"interesting\"\n",
+ "results."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "qs = np.zeros_like(z)\n",
+ "qs[0, 100] = 750\n",
+ "cem.set_value(\"land_surface_water_sediment~bedload__mass_flow_rate\", qs)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Now let's time step through the model. For every iteration of the for-loop we set the sediment\n",
+ "discharge (***set_value***), and then update the model to the next time (***update_until***)."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "for time in tqdm(range(3000)):\n",
+ " cem.set_value('land_surface_water_sediment~bedload__mass_flow_rate', qs)\n",
+ " cem.update_until(time)\n",
+ "\n",
+ "cem.get_value('sea_water__depth', out=z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Let's have a look to see what sort of delta we've created after 3000.0 days."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "cem.get_value('sea_water__depth', out=z)\n",
+ "plot_coast(cem.grid_spacing(2), z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Exercise\n",
+ "\n",
+ "Now play with the CEM model on your own. To make things easier, I've placed all of the steps\n",
+ "to run CEM into a single cell below. Please feel free to modify!\n",
+ "\n",
+ "Some ideas\n",
+ "1. Modify wave energy vs sediment load\n",
+ "1. Change the incoming wave angle\n",
+ "1. Modify the river position so that it moves with time\n",
+ "1. Pick wave height and period from some probability density function\n",
+ "1. Add a second, or third, or fourth river\n",
+ "1. Increase the sediment load or have it vary with time\n",
+ "1. Make a movie of the evolving delta\n",
+ "\n",
+ "Anything else?"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "cem = pymt.models.Cem()\n",
+ "\n",
+ "args = cem.setup(number_of_rows=100, number_of_cols=200, grid_spacing=200.)\n",
+ "cem.initialize(*args)\n",
+ "\n",
+ "qs = np.zeros(cem.grid_shape(2), dtype=float)\n",
+ "qs[0, 100] = 750\n",
+ "\n",
+ "for time in tqdm(range(3000)):\n",
+ " cem.set_value(\"sea_surface_water_wave__height\", 1.5)\n",
+ " cem.set_value(\"sea_surface_water_wave__period\", 7.)\n",
+ " cem.set_value(\n",
+ " \"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\",\n",
+ " 0. * np.pi / 180.,\n",
+ " )\n",
+ "\n",
+ " cem.set_value('land_surface_water_sediment~bedload__mass_flow_rate', qs)\n",
+ " cem.update_until(time)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "z = np.empty(cem.grid_shape(2), dtype=float)\n",
+ "cem.get_value('sea_water__depth', out=z)\n",
+ "\n",
+ "plot_coast(cem.grid_spacing(2), z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "def avulse_river(river_x, stddev=1.0, x_min=None, x_max=None):\n",
+ " river_x += np.random.normal(0.0, stddev)\n",
+ " if x_max is not None and river_x >= 200:\n",
+ " river_x = river_x - 200\n",
+ " if x_min is not None and river_x < 0:\n",
+ " river_x = 200 + river_x\n",
+ " return river_x"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "river_x = 100.0\n",
+ "river_i = []\n",
+ "for time in range(3000):\n",
+ " river_x = avulse_river(river_x, x_min=0.0, x_max=200.0)\n",
+ " river_i.append(int(river_x))\n",
+ "plt.plot(river_i)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Exercise\n",
+ "\n",
+ "### Couple models\n",
+ "\n",
+ "Instead of using constant wave characteristics, let's now couple the CEM component with a wave\n",
+ "gererator component.\n",
+ "\n",
+ "### Waves"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "from pymt.models import Waves\n",
+ "\n",
+ "waves = Waves()\n",
+ "args = waves.setup(angle_asymmetry=0.2, angle_highness_factor=0.5)\n",
+ "waves.initialize(*args)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "angles = np.zeros(1000)\n",
+ "for day in range(1000):\n",
+ " waves.update()\n",
+ " angles[day] = waves.get_value(\"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "_ = plt.hist(angles * 180.0 / np.pi, bins=100)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Now add the waves component to our coupling script."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import numpy as np\n",
+ "import pymt.models\n",
+ "\n",
+ "waves = pymt.models.Waves()\n",
+ "args = waves.setup(angle_asymmetry=0.2, angle_highness_factor=0.5)\n",
+ "waves.initialize(*args)\n",
+ "\n",
+ "cem = pymt.models.Cem()\n",
+ "args = cem.setup(number_of_rows=100, number_of_cols=200, grid_spacing=200.)\n",
+ "cem.initialize(*args)\n",
+ "\n",
+ "qs = np.zeros(cem.grid_shape(2), dtype=float)\n",
+ "qs[0, 100] = 500\n",
+ "\n",
+ "for time in tqdm(range(3000)):\n",
+ " waves.update()\n",
+ " angle = waves.get_value(\"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\")\n",
+ " \n",
+ " cem.set_value(\"sea_surface_water_wave__height\", 1.5)\n",
+ " cem.set_value(\"sea_surface_water_wave__period\", 7.0)\n",
+ " cem.set_value(\n",
+ " \"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\",\n",
+ " angle,\n",
+ " )\n",
+ "\n",
+ " cem.set_value('land_surface_water_sediment~bedload__mass_flow_rate', qs)\n",
+ " cem.update_until(time)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "z = cem.get_value('sea_water__depth').reshape(cem.grid_shape(2))\n",
+ "plot_coast(cem.grid_spacing(2), z)"
+ ]
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.2"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 4
+}
diff --git a/lessons/pymt/cem_and_waves_and_hydrotrend.ipynb b/lessons/pymt/cem_and_waves_and_hydrotrend.ipynb
new file mode 100644
index 0000000..f38d15a
--- /dev/null
+++ b/lessons/pymt/cem_and_waves_and_hydrotrend.ipynb
@@ -0,0 +1,1193 @@
+{
+ "cells": [
+ {
+ "cell_type": "code",
+ "execution_count": 1,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stderr",
+ "output_type": "stream",
+ "text": [
+ "\u001b[33;01m➡ models: Avulsion, Plume, Sedflux3D, Subside, FrostNumber, Ku, Hydrotrend, Cem, Waves, ExponentialWeatherer, Flexure, FlowAccumulator, FlowDirectorD8, FlowDirectorDINF, FlowDirectorSteepest, LinearDiffuser, OverlandFlow, SoilMoisture, StreamPowerEroder, TransportLengthHillslopeDiffuser, Vegetation\u001b[39;49;00m\n"
+ ]
+ },
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "time = 0\n",
+ "time = 100\n",
+ "time = 200\n",
+ "time = 300\n",
+ "time = 400\n",
+ "time = 500\n",
+ "time = 600\n",
+ "time = 700\n",
+ "time = 800\n",
+ "time = 900\n",
+ "time = 1000\n",
+ "time = 1100\n",
+ "time = 1200\n",
+ "time = 1300\n",
+ "time = 1400\n"
+ ]
+ }
+ ],
+ "source": [
+ "import numpy as np\n",
+ "import pymt.models\n",
+ "\n",
+ "waves = pymt.models.Waves()\n",
+ "args = waves.setup()\n",
+ "waves.initialize(*args)\n",
+ "\n",
+ "cem = pymt.models.Cem()\n",
+ "args = cem.setup(number_of_rows=100, number_of_cols=200, grid_spacing=200.)\n",
+ "cem.initialize(*args)\n",
+ "\n",
+ "hydrotrend = pymt.models.Hydrotrend()\n",
+ "args = hydrotrend.setup(run_duration=100)\n",
+ "hydrotrend.initialize(*args)\n",
+ "\n",
+ "qs = np.zeros(cem.grid_shape(2), dtype=float)\n",
+ "qs[0, 100] = 750\n",
+ "qs_save = []\n",
+ "\n",
+ "for time in range(3000):\n",
+ " waves.set_value('sea_shoreline_wave~incoming~deepwater__ashton_et_al_approach_angle_asymmetry_parameter', .3)\n",
+ " waves.set_value('sea_shoreline_wave~incoming~deepwater__ashton_et_al_approach_angle_highness_parameter', .7)\n",
+ "\n",
+ " waves.update()\n",
+ " angle = waves.get_value(\"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\")\n",
+ " \n",
+ " cem.set_value(\"sea_surface_water_wave__height\", 2.0)\n",
+ " cem.set_value(\"sea_surface_water_wave__period\", 7.0)\n",
+ " cem.set_value(\n",
+ " \"sea_surface_water_wave__azimuth_angle_of_opposite_of_phase_velocity\",\n",
+ " angle,\n",
+ " )\n",
+ "\n",
+ " hydrotrend.update()\n",
+ " qs[0, 100] = hydrotrend.get_value(\"channel_exit_water_sediment~suspended__mass_flow_rate\")\n",
+ " \n",
+ " qs_save.append(qs[0, 100])\n",
+ " \n",
+ " cem.set_value('land_surface_water_sediment~bedload__mass_flow_rate', qs)\n",
+ " cem.update_until(time)\n",
+ " \n",
+ " if time % 100 == 0:\n",
+ " print(\"time = {0}\".format(time))"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 2,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "def plot_coast(spacing, z):\n",
+ " import matplotlib.pyplot as plt\n",
+ "\n",
+ " xmin, xmax = 0., z.shape[1] * spacing[0] * 1e-3\n",
+ " ymin, ymax = 0., z.shape[0] * spacing[1] * 1e-3\n",
+ "\n",
+ " plt.imshow(z, extent=[xmin, xmax, ymin, ymax], origin=\"lower\", cmap=\"ocean\")\n",
+ " plt.colorbar().ax.set_ylabel(\"Water Depth (m)\")\n",
+ " plt.xlabel(\"Along shore (km)\")\n",
+ " plt.ylabel(\"Cross shore (km)\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 4,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAXkAAADnCAYAAAD7GCa6AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4xLjIsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy8li6FKAAAfnUlEQVR4nO3de7gcVZ3u8e+bAIKACgYQBY0iiIhy9Rqd4XYcxlHBUZxBhoPKGbzgUccr+ni/oo+ijhckCBIVdXAUAVEUEWS8gYkgAUHxggrkECOiiNySvOePqoZOp/fu6t7d1dXN+3mefvbu2l2rfl3p/Pbaq1b9lmwTERHTad64A4iIiNFJko+ImGJJ8hERUyxJPiJiiiXJR0RMsQ3GHUBERNMceOCBXrVqVc/XLVu27Ju2D6whpIElyUdEdFi1ahVLly7t+TpJC2oIZ06S5CMiulgzJfcQJclHRHRYa7hjzdpxhzEUSfIREesxa9amJx8RMZUMSfIREdPKzph8RMRUS08+ImJKmfTkIyKmlu3MromImFa58BoRMc1y4TUiYnqlJx8RMdWcnnxExLRKWYOIiCmX4ZqIiCnlDNdEREyxzK6JiJhemV0TETHFUtYgImKKpaxBRMSUy3BNRMSUmqbhmnnjDiAiomnsoiff69GLpO0lnS/pSklXSHpFuX1LSedKurr8usWo3kuSfEREF8NI8sBq4NW2Hwk8ATha0i7AMcB5tncEziufj0SGayIiOgzrwqvtFcCK8vubJV0JPAg4CNinfNkS4ALg9XM+YBdJ8hERHfoYk18gaWnb88W2F3d7oaSFwB7ARcA25S8AbK+QtPWcAp5FknxERIc+kvwq23v3epGkzYAvA6+0/RdJc4ywuiT5iIhOHt4USkkbUiT4U21/pdx8g6Rty178tsDKoRysi1x4jYjo0CpQ1uvRi4ou+0nAlbaPa/vRmcAR5fdHAGcM/U2U0pOPiOgwxNo1i4DDgeWSLi23vRE4FjhN0pHA74BDhnGwbpLkIyI6eEiLhtj+HjDTAPz+cz5ABUnyERFdpKxBRMSUyqIhERFTzEOcXTNuSfIREV2kJx8RMaWmqQplknxERIcsGhIRMcWmaY3Xkd3x2oQ6yhERgxrGHa9NMMqyBmOvoxwRMYhhLRrSBCNL8rZX2P5J+f3NQHsd5SXly5YAB48qhoiIQU1LT76WMflB6ihLOgo4CmDTTTfda+edd64j1IiYcMuWLVtle6u5tLE2F16rG7SOcll4fzHAXnvv7R9cdPHogoyIqXGvDeb/dhjtTMpwTC8jTfLjrqMcETGIzJOvoEId5WOpWEfZNrevno4/nSJiAqSsQSVDq6O8dkhlPyMiqkiBsgqGWUfZwB1rk+Qjoh7TdDNU7niNiOgwrEVDmmAikrxt7pySEx4RkyHDNTWyyYXXiKiNmZw7WnuZiCS/1ubW1WvGHUZE3ENk0ZCIiCmX4Zoamem5CBIRzZfZNTWbpjoSEdF8tqdm2vZEJHlDZtdERG3Sk4+ImGbOmHytpmm9xYhovvTkazZNd59FRPOlCmXNjLl9TebJR0Q9bHPHlNyAORFJPiKibunJ12it4dYp+a0aEc2XO15rZmcKZUTUJ2PyNVtLZtdERJ2yaEhExNTKFMqapdRwRNRpmqZtT0iSz3BNRNQnPfmaZY3XiKiVp2dMft64A4iIaJpWT77XowpJJ0taKenytm1vk3SdpEvLx9NG9V569uQlPRH4N+ApwLbArcDlwNnA52z/eVTBtay1ue3O1aM+TETEXYbYkz8F+BjwmY7tH7L9gV47S5oH7AY8kCL/XmH7hqoHnzXJS/oGcD1wBvBuYCWwMbATsC9whqTjbJ9Z9YCDKC6CTMefThHRfMO8Gcr2hZIW9rufpB2A1wMHAFcDf6DMv5L+BpwALLE961h2r5784bZXdWz7K/CT8vFBSQv6Db5fWRkqIupkalk05GWS/jewFHi17T91/PxdwPHAi+x1/6yQtDXwPOBwYMlsB5l1TL4zwUu6j6QtW49ur2l77VjHoSIiBtXHmPwCSUvbHkdVPMTxwA7A7sAK4IPrxWAfavvCzgRf/myl7Q/bnjXBQ8XZNZJeBLyDYjyodUADD5tlt1OYwzhUuyz/FxG1qr5oyCrbe/fdfNuYuqQTga/N9FpJ84F/AhbSlrNtH1flWFWnUL4GeNRMvfZuBh2H6toWGa6JiJqNcJ68pG1tryifPotiMstMzgJuA5YDfSfCqkn+V8Df+m18Br3GoSIixm9Is2skfQHYh2Jo51rgrcA+knan6MNeA7xolia2s/2YQY9fNcm/AfiBpIuA21sbbb+8z+MdD7yT4o29k2Ic6oXdXliObR0FsOlWD0hPPiLqU0yvGVJTPrTL5pP6aOIbkp5q+1uDHL9qkj8B+A4D/rnQ0s84lO3FwGKA++/wSCfJR0StmlPW4EfA6eV8+TsBAbZ9nyo7V03yq22/asAA79LnONRd1mJuXZ3l/yKiJqZJSf6DwBOB5d1m2vRSNcmfXw6fnMW6wzU3zrTDEMahIiLGpzm1a64GLh8kwUP1JP+88usb2rbNOoVyCONQd1mbUsMRUSs3qSe/ArigrEDQ3ske6hTKh3W542rjyiHOkZ0qlBFRs+b05H9TPjYqH32pmuRPom0WjKRNgTOB/fs94CCMs8ZrRNSnuOV13FEAYPvtc9m/aqnh6yQdDyBpC+Bc4HNzOXBERHO56Mn3eoyQpMWSHj3DzzaV9EJJh/Vqp1JP3vabJb1P0ieBvYBjbX+5v5AHN01LcUXEBGjG7JpPAG8uE/3l3F2FckfgPsDJwKm9GulVavif255eDLy5/GpJ/2z7K4PF3h/bufAaEfUa85i87UuB50raDNibu9fzuNL2z6u206sn/4yO55cAG5bbDdSS5NeSnnxE1Gz8PXkAbP8VuGDQ/WdN8rZfMGjDERETa4hlDcat13DNm4CPz1RETNJ+wL1tz1ieYBjs3PEaETVrSE9+rnoN1ywHvibpNoqVoNoH/ncHvg28Z6QRUpzrO9YkyUdEjZozT35Oeg3XnEGxjuuOwCKKgf+/UEyfPMr2raMPsVVPfjpOeERMANOYJC9pJ+C1wENYd9GQ/arsX3UK5dUU9RMiIu4ZmjNc8yXgk8CJQN9DGlXveB0rZ/m/iKhVo2rXrLZ9/KA7T0iSzxTKiKhRA8oaSNqy/PYsSS8FTqdiFeB2k5HkSU8+Imo2/jH5ZRS/blQ+f23bz2atAtyuUpIvB/6PB7axvaukxwDPtP2u6vFGREyQ8d/x+lAoKv7avq39Z/1UAa7akz+R4rfICeXBL5P0eaCWJG/DnZknHxF1caPG5H8A7FlhW1dVk/y9bV8sqX3b6or7zt0U3X0WERNizD15SQ8AHgRsImkP7h62uQ9w76rtVE3yqyTtQDEOhKTnUKxWUp8k+Yio0/h78v8APB/YDmhfBepm4I1VG6ma5I8GFgM7S7qOYpWSnnWMIyImUgNm19heAiyR9Oy5lHbvmeQlzQP2tn1AuSLUPNs3D3rAgRhIqeGIqM3oFwXpwwWS/hN4MkU2/B7wDtt/rLJzzyRve62klwGn2b5lTqEOKmPyEVGnZiwa0vJF4ELg2eXzw4D/Ag6osnPV4ZpzJb2mbPiuRF91Mv5QZCHviKhTc3ryW9p+Z9vzd0k6uOrOVZN8axHvo9u2VZ6MHxExcZrTkz9f0r8Cp5XPnwOcXXXnqgXKHjpAYMOT4ZqIqFOzcs6LgFdRVP81MB+4RdKrANu+z2w7V73jdUPgJcDflZsuAE6wfeeAQffHwJ25GSoiatSQnrztzeeyf9XhmuMp1nb9RPn88HLb/5lpB0knA08HVtretdy2JcW4/kLgGuC5M606ta5G/VaNiGnXrHryorjY+lDb75S0PbCt7Yur7D+v4nEea/sI298pHy8AHttjn1OAAzu2HQOcZ3tH4LzyeURE86x170c9PgE8EXhe+fyvwMer7ly1J79G0g62fwUg6WH0KF5v+0JJCzs2HwTsU36/hGLY5/U9j96AGxMi4h6mIT154PG295R0CYDtP0naqOrOVZP8aymu8P6aon7CQ4AX9B1qUcVyBYDtFZK2numFko4CjgJg0y0ga7xGRG0adTPUnZLmc3dZma2Ayr3eqrNrzivXeX0ERZK/yvbtPXabE9uLKUopoPs/2GSN14ioS7NGD/6TYsGQrSW9m2IK5Zuq7tzPoiF7UVww3QDYTRK2P9PH/gA3SNq27MVvC6zsc/+IiHo0Z3bNqZKWAftTdLIPtn1l1f2rTqH8LLADcCl3j8Ub6DfJnwkcARxbfj2j0l7N+q0aEdOuQWUNJD0a2JmiU3xlPwkeqvfk9wZ2sasPUkn6AsVF1gWSrgXeSpHcT5N0JPA74JBqrRmyaEhE1Gb8Y/KS7kvREd4euIyiF/9oSb8DDrL9lyrtVE3ylwMPoI8a8rYPneFH+1dt4+7GSE8+Iuo1pJ78HO4ZeiewFNjP9tpyv/nAe4F3A/+3yvFnnScv6SxJZwILgJ9J+qakM1uPam8xImLCtG6G6vWo5hQGu2foAOCYVoIHsL2GYsGQShUooXdP/gNVGxqpZtWRiIh7giHlnDncM3SH7fWWWbW9WlLl2Y2zJnnb3wUoFwu5tawtvxPFRYBvVD3IUCTJR0Rdqi/kvUDS0rbni8vp371UuWdo4461XVsE3KtKcFB9TP5C4CmStqD402Ip8C/UtQRgevIRUbdqwzGrbO89oghWsO7aru3+X9VGqiZ52f5bOSvmo7bfL+nSqgeJiJg4o51d0/OeIdv7DuNAlZO8pCdS9NyPLLfNH0YAlWSN14io22jnyQ92z9AAqib5VwBvAE63fUVZoOz8UQXVVYZrIqIuQxwiHu49Q/2rWrvmQopx+dbzXwMvH1VQXQLIzVARUa8hDdfM5Z6hspb8drZ/P+jxq9aTj4i452iVNRhzPfmyysBX59JGPwXKxid3vN4z3HrH3d9vslHxfJON7v7ZJpVLaDfPNL+3OrTOX53nqTmlhn8k6bG2fzzIzpOR5PHd/8gbzYc7OoZuNhriNeCZ2m5tbz/+bD8bRWwzqRLzMNue6f12Ozf9tt/S+vduT47t37eO1es4nbHOdG6qtDWozvfY6721x1WX2c5NXcdvHXOmzwR0P0+j+rdrSIEyYF/gxZKuAW6hmCdv24+psnPVKpTvB94F3AqcA+wGvNL25waJuG/tJ7vbB6B92/x56/b65/cYkVqzdv19Zmq78/lsP2vf1oqh27GqxNv6+WxxVo25Xbf22o/f7VhV33/r+/b3XqX9qlrtt9YZ6Gy/s+3W6/v592w/PzN9jvr9t6lqts90Zzy9PsPdzkm31890blqvnenczvbzXuet02wJfrbX97tfL9VvhqrDP85l56pj8k8tK549HbgW2IlitaiIiOm0dm3vRw1s/5aiEuV+5fd/o4/rqVWHazYsvz4N+ILtG4uLvg3U2UOo0rsa9Xh/e/u94pstlmHH2a29UR9jktqf7d+tVxzDVOXfqd/PTT/xtl470z6z/XySr6U1pCcv6a0U5d4fAXyaIh9/DlhUZf+qSf4sSVdRDNe8tFxj8Lb+w42ImACtKpTN8CxgD+AnALavl7R51Z2rzpM/RtL7gL/YXiPpFooqahHD88z3r7/tzNfVH8eotd7nNL63qdGoMfk7bFtSayHvTfvZudK4jqRDgNVlgn8TxZ8KD+w71IiISTG8evJzdZqkE4D7Sfp34NvAp6ruXHW45s22vyTpycA/UNSZPx54fL/RDkV7j68JvaFuPVCoL7ZRH7+O9zfTMYb5b915jDo/O93e3zjjmU0T/tKY6fPQMurYGrTGq+0PSPpfwF8oxuXfYvvcqvuryrKtki6xvYek9wLLbX++tW3gyPugez/AvO6G2V80l3/02T5Qvdrt9WGs0sZcjPr4VdqfyzGqtj/o8UYd/zBjaBlHcu0nxlHEN+jnANaP55L3L5tr+V9tuJXZosKI9B9OmvOxesYivc/263ttm3H/ikn+a8B1FEtO7UVxAfZi27v1H3L/9ECZo/rYoZ8P4VySwCAfzPZ25joGPdfjj7v9QY9R9XjDTByDGOV76+c4w+iozKX9URxzthiGkeQ3WGDuVyHJ//HkOpL8T2zv2bHtsqHeDAU8l2KNwg/Yvqmsf5x58hExvcY8u0bSS4CXAg+TdFnbjzYHvl+5nSo9+fKAuwFPKZ/+j+2fVj3IXPXdk283Uy9j2D2JOrTey7Bir+PcjKqn3etYdf371vX56jxOkz+/o/7LoZe3M5ye/ObP6P3Cm04ZWU9e0n2BLYD3su5C3zfbvrFyOxWHa14B/DvwlXLTsyjWMvxo5YjnYE5JPiLuWYaV5Dd7eu8X/nnJyIdrWsp1YDduPbf9uyr7VR2uORJ4vO1byoO9D/ghUEuSj4ioVYPWlZb0DIq1Xh9IsUzgQ4ArgUdV2b/y8n9AewWgNay/gnhlZTW1m8t2Vtf1mzAiorKGTKGkKA75BODb5SzHfYGZFiJZT9Uk/2ngIkmnl88PBk7qK8z17Wt71RzbiIgYvmaVNbjT9h8lzZM0z/b55WhKJVXLGhwn6QLgyRQ9+BfYvmSweCMiJkBzevI3SdqMYgnWUyWtBFZX3blnkpc0D7jM9q6UBXKGwMC3yloMJ9hePKR2IyKGozk9+YMoCkL+B3AYcF/gHVV37pnkba+V9FNJD656NbeCRWUlta2BcyVdVS4WfhdJR0E5p+a+QzpqREQVDVg0RNIrKebDX2K7dU10Sb/tVB2T3xa4QtLFFMtPAWD7mf0esNzv+vLrynKc/3EUf4q0v2YxsBjKKZQREXUa/+ya7YCPADuXN0P9gCLp/7CfefJVk/zb+4+vu7JM5jzbN5ffP5U+/vSIiKjFmIdrbL8GQNJGFIuGPAl4IXCipJts71KlnVmTvKSHA9vY/m7H9r+jqGUziG2A08uVpTYAPm/7nAHbiogYjSoDCPX8HtgEuA/FwPV9geuB5VV37tWT/zDwxi7b/1b+rMJ9v+uy/WuKhcAjIhrKMK9CBh/y+uHtJC2muOHpZuAiiuGa42z/qZ92eiX5hbYv69xoe6mkhf0cKCJiYoixJ3ngwcC9gKspRk6uBW7qt5FeSX7jWX62Sb8Hi4iYGPMrXHi9c3SHt32ginHtR1GMx78a2FXSjRQXX99apZ1ey//9uFxuah2SjgSW9RlzRMSEcDEm3+sx6igKlwNfB75BMbtmB+AVVdvo1ZN/JcVF0sO4O6nvDWxEUYkyImL6iFqS+KwhSC+n6MEvovib4fsUhSFPZlgXXm3fADypLIiza7n5bNvfGSToiIiJUWVMfrQWAv8N/IftFYM2UrV2zfnA+YMeJCJi4ow5ydt+1TDaqXozVETEPUcDhmuGJUk+ImI9rja7ZgIkyUdEdKo6T34CJMlHRHST4ZqIiCmWJB8RMa0q1q6ZAEnyERGdhji7RtI1FEXG1gCrbe89lIYrSpKPiOhmg6HOrtnX9qphNlhVknxERKcpmiffq0BZRMQ9UDkm3+sBCyQtbXsc1b0xviVp2Qw/H6n05CMiuqnWk19VYYx9ke3rJW0NnCvpKtsX9thnaNKTj4jo1LoZqndPvifb15dfVwKnA48bXeDrS5KPiOhm/trejx4kbSpp89b3wFOBy0cc+ToyXBMRsZ6hLQqyDcWaHFDk28/bPmcYDVeVJB8R0WlItWts/xrYbc4NzUGSfEREN1MyhTJJPiKim5Q1iIiYUlN0M1SSfETEeqZn0ZCxTKGUdKCkn0v6paRjxhFDRMSs5N6PCVB7kpc0H/g48I/ALsChknapO46IiBkN8WaocRtHT/5xwC9t/9r2HcAXgYPGEEdExMymJMmPY0z+QcDv255fCzy+80VlIZ9WMZ/beXu9d4l1sQAYS6nQDk2IowkxQDPiaEIM0Iw4mhADwCPm3sTkDMf0Mo4kry7b1jubthcDiwEkLa270H6nJsTQlDiaEENT4mhCDE2JowkxtOKYeyNMTE+9l3Ek+WuB7duebwdcP4Y4IiJmltk1A/sxsKOkh0raCPhX4MwxxBER0V1rnvwUzK6pvSdve7WklwHfBOYDJ9u+osdui0cfWU9NiAGaEUcTYoBmxNGEGKAZcTQhBhhKHJNzYbUX2dPxRiIihkWbb2L22qH3C797xbImXIeYTe54jYjolAuvERFTLhdeR68p5Q8kXSNpuaRLhzI9q/pxT5a0UtLlbdu2lHSupKvLr1uMIYa3SbquPB+XSnraiGPYXtL5kq6UdIWkV5Tb6z4XM8VR2/mQtLGkiyX9tIzh7eX2us/FTHHU+tkojzlf0iWSvlY+H8K5qHDRdUIuvDY2yTew/MG+tnevefztFODAjm3HAOfZ3hE4r3xedwwAHyrPx+62vz7iGFYDr7b9SOAJwNHlZ6HuczFTHFDf+bgd2M/2bsDuwIGSnkD952KmOKDezwbAK4Ar257P/VykrEEt7vHlD8oV3W/s2HwQsKT8fglw8BhiqJXtFbZ/Un5/M8V/6AdR/7mYKY7auPDX8umG5cPUfy5miqNWkrYD/gn4VNvm4ZyL9ORHrlv5g1r/Q7Ux8C1Jy8pyC+O0je0VUCQdYOsxxfEySZeVwzkjHRpoJ2khsAdwEWM8Fx1xQI3noxyeuBRYCZxreyznYoY4oN7PxoeB1wHtA+jDORfpyY9cpfIHNVlke0+KoaOjJf3dmOJoiuOBHSj+TF8BfLCOg0raDPgy8Erbf6njmBXjqPV82F5je3eKu8UfJ2nXUR6vzzhqOxeSng6stL1s+I2TnnwNGlP+wPb15deVwOkUQ0njcoOkbQHKryvrDsD2DeV/8LXAidRwPiRtSJFYT7X9lXJz7eeiWxzjOB/lcW8CLqC4ZjK2z0V7HDWfi0XAMyVdQzGcu5+kzzGUc1EuGtLrMQGanOQbUf5A0qaSNm99DzwVxloR80zgiPL7I4Az6g6g9R+o9CxGfD4kCTgJuNL2cW0/qvVczBRHnedD0laS7ld+vwlwAHAV9Z+LrnHUeS5sv8H2drYXUuSH79j+N4ZxLqbowmtj58kPWP5gFLYBTi/+f7MB8Hnb59RxYElfAPYBFki6FngrcCxwmqQjgd8Bh4whhn0k7U4xfHYN8KJRxkDRYzscWF6OAQO8kZrPxSxxHFrj+dgWWFLOPpsHnGb7a5J+SL3nYqY4PlvzZ6Ob4XwuJmQ4ppeUNYiI6KAt7mX2f0DvF375dylrEBExcSbowmovSfIREd1MyJh7L0nyERGdxMTMnuklST4iopsM10RETKvJmSLZS5PnyccYSXqWJEvauW3bQrVVo6wxln1aFQZrONbBkt5Sfn+KpOcM2M5WkmqZahsjkjteY8odCnyP4iaTiVbO5a7qdcAn5npM238AVkhaNNe2Ygym6GaoJPlYT1mbZRFwJDMk+bKe+KdV1Nm/RNK+5fbnS/qKpHPKet7vb9vnSEm/kHSBpBMlfaxLu3/fVov8ktbdxsBmkv5b0lWSTi3vPkXS/uXrlpcFse5Vbr9G0lskfQ84RNIOZUzLJP1P+18obcfeCbjd9qouP3tn2bOfV7b9Hkk/lLRU0p6SvinpV5Je3LbbV4HDqp31aJwpKWuQMfno5mDgHNu/kHSjpD1bJXbbHA1g+9FlwvxWmSShKE61B0XN8Z9L+iiwBngzsCdwM/Ad4Kddjv0a4Gjb3y9/2dxWbt8DeBRF/aLvA4tULOByCrB/GetngJdQVCYEuM32kwEknQe82PbVkh5P0Vvfr+PYi4DO90n5i+q+wAtsu/z98nvbT5T0oTKGRcDGwBXAJ8tdlwLv6vIeo+kmaDiml/Tko5tDKQo+UX49tMtrngx8FsD2VcBvgVaSP8/2n23fBvwMeAhFoarv2r7R9p3Al2Y49veB4yS9HLif7dXl9ottX1sWvroUWAg8AviN7V+Ur1kCtFcI/S+46y+TJwFfKssRnEBxW36nbYE/dGx7cxnHi7zu7eGtOkrLgYts31wO0dzWqulCURjrgTO8z2i6KRmuSU8+1iHp/hQ93F0lmaJukCW9rvOlszRze9v3ayg+Z7O9/i62j5V0NvA04EeSDphDm7eUX+cBN5VlcWdzK0WPvd2Pgb0kbWm7ffGUVjxrO2Jby93/rzYu24xJlJ58TKnnAJ+x/RDbC21vD/yGoufe7kLK8eZymObBwM9nafdi4O8lbSFpA+DZ3V4kaQfby22/j2K4Y72x8zZXAQslPbx8fjjw3c4XlTXffyPpkPIYkrRbl/auBB7ese0cioJXZ7ddH6hqJ8ZbsTQGlQuvMcUOpaiZ3+7LwPM6tn0CmC9pOcWwyPNt384MbF8HvIdiJaVvUwzj/LnLS18p6XJJP6XoBX9jljZvA15AMQyznKIX/ckZXn4YcGTZ7hV0X0ryQmCP1kXdtuN8iaI2+pkqyupWtS9wdh+vjyaZkimUqUIZtZG0me2/lj350ynKR3f+QhkrSR8BzrL97SG0dSFwkO0/zT2yqJO2nm/+ZdPeL/zYzT2rUEo6EPgIxdDnp2wfO5QgK0pPPur0tvLC5+UUQ0BfHXM83bwHuPdcG5G0FXBcEvyEGtJwTXmPxscplg7dhWLtgV1GG/y6cuE1amP7NeOOoRfbNzCEFcjKmTZN/CUWVQ1nOOZxwC9t/xpA0hcphgp/NozGq0iSj4joptqF1QXl/Roti20vbnv+IOD3bc+vBR4/hOgqS5KPiOhU/cLqqh5j8t2m+dZ6ITRJPiKim+FMkbwW2L7t+XYUd23XJkk+IqLT8BYN+TGwo6SHAtdR1ILqnI48UknyERHdDOHCq+3Vkl4GfJNiCuXJtq+Yc8N9SJKPiFjP8O5otf114OtDaWwASfIREd1MyB2tvSTJR0R0at0MNQWS5CMiupmQRUF6Se2aiIgO5fq8Cyq8dJXtA0cdz1wkyUdETLEUKIuImGJJ8hERUyxJPiJiiiXJR0RMsST5iIgp9v8BbszGTH0REiMAAAAASUVORK5CYII=\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "z = cem.get_value('sea_water__depth').reshape(cem.grid_shape(2))\n",
+ "plot_coast(cem.grid_spacing(2), z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 5,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "[0.002789636475236596,\n",
+ " 0.0082716148760771085,\n",
+ " 2061.5453030721555,\n",
+ " 69.692963494932513,\n",
+ " 5.5679804817186733,\n",
+ " 9.8369364792671448,\n",
+ " 24.028156721061226,\n",
+ " 3.1800271236105528,\n",
+ " 1.0028329687279884,\n",
+ " 2.1718279461684493,\n",
+ " 6.5900504293048243,\n",
+ " 0.97279630589925159,\n",
+ " 0.68295731117177105,\n",
+ " 1.5856475832823027,\n",
+ " 0.59192739542400297,\n",
+ " 1.2027394830568687,\n",
+ " 0.14013028154094181,\n",
+ " 0.28735249440422383,\n",
+ " 2.1090236516467895,\n",
+ " 0.15878462552569153,\n",
+ " 0.089549159499981934,\n",
+ " 0.16030114941476892,\n",
+ " 0.21543932335009572,\n",
+ " 0.24586112155091458,\n",
+ " 0.18919824660376355,\n",
+ " 0.16691717959868707,\n",
+ " 0.08182568847750378,\n",
+ " 0.23603450288690656,\n",
+ " 0.37395845427459679,\n",
+ " 0.071190935246522327,\n",
+ " 0.11201040483060556,\n",
+ " 736.34254093119796,\n",
+ " 239.27457907612407,\n",
+ " 6.3969351234890706,\n",
+ " 3.4706340328160286,\n",
+ " 9.0396204806585558,\n",
+ " 18.851034313625942,\n",
+ " 3.3426192641265944,\n",
+ " 4.7108833280407216,\n",
+ " 7.9035238358971442,\n",
+ " 5.5109832665122394,\n",
+ " 0.30710518336535059,\n",
+ " 0.61872686874973626,\n",
+ " 0.45878636691661756,\n",
+ " 0.1432000278271508,\n",
+ " 0.38483134013132347,\n",
+ " 0.43223040768334797,\n",
+ " 0.081722761646173245,\n",
+ " 0.28225381142125505,\n",
+ " 0.24070200465195396,\n",
+ " 0.085501026865737531,\n",
+ " 0.17655740125841884,\n",
+ " 0.29670980093696453,\n",
+ " 0.16426413470036239,\n",
+ " 0.07142779358418111,\n",
+ " 0.033121018313935477,\n",
+ " 0.10222502799442026,\n",
+ " 0.03138218055968154,\n",
+ " 0.13823401439641333,\n",
+ " 0.2952740600580635,\n",
+ " 0.11838232059293122,\n",
+ " 0.016160104962500792,\n",
+ " 0.079948349527419707,\n",
+ " 0.086783017410444807,\n",
+ " 0.023906235775582235,\n",
+ " 0.074427074807476901,\n",
+ " 0.032682118388732674,\n",
+ " 0.014566201735157054,\n",
+ " 0.0088676227757215013,\n",
+ " 0.041941145375860536,\n",
+ " 0.0062458558449178959,\n",
+ " 0.059253852413059291,\n",
+ " 0.038442650935449456,\n",
+ " 0.019530340559793219,\n",
+ " 0.0086414550528861375,\n",
+ " 0.0038244990496172485,\n",
+ " 0.015438310752799162,\n",
+ " 0.014339254727865699,\n",
+ " 0.01412226897839885,\n",
+ " 0.027945501259507514,\n",
+ " 0.042947689884027362,\n",
+ " 82.774833621154343,\n",
+ " 50.073380033615884,\n",
+ " 51.259942965337821,\n",
+ " 17.65737769261623,\n",
+ " 28.325318527552515,\n",
+ " 4.6640426165036883,\n",
+ " 2.5796616595477175,\n",
+ " 180.1188668147191,\n",
+ " 14.762386336469534,\n",
+ " 6.1843196120742361,\n",
+ " 8.557363956478504,\n",
+ " 7.6111466475789369,\n",
+ " 5.2072785105475532,\n",
+ " 3.3546175965364684,\n",
+ " 2.5479975140147082,\n",
+ " 0.26018121340214451,\n",
+ " 3.5589141713933321,\n",
+ " 1.9304158789140624,\n",
+ " 3.2213843963360809,\n",
+ " 11.413173024427959,\n",
+ " 2521.0638358525152,\n",
+ " 54.367331945343565,\n",
+ " 43.404043198573866,\n",
+ " 9.6292811337375017,\n",
+ " 16.759321804399203,\n",
+ " 9.1699809050401822,\n",
+ " 4.0332496860683396,\n",
+ " 59.23911333172795,\n",
+ " 2.6692991829770292,\n",
+ " 8.1880021592098888,\n",
+ " 6.0536094404164507,\n",
+ " 2.2889172277351073,\n",
+ " 1.8042571949710893,\n",
+ " 3.2657230470143048,\n",
+ " 11.553835854535663,\n",
+ " 3.272336775759277,\n",
+ " 65.523655266138917,\n",
+ " 6.5140123181065137,\n",
+ " 76.001072602296119,\n",
+ " 12.067812235734976,\n",
+ " 3.352028838639868,\n",
+ " 6.9265433432735,\n",
+ " 5.1085488031015691,\n",
+ " 6.6124211691348354,\n",
+ " 6.7149472741811973,\n",
+ " 19.546521799250851,\n",
+ " 10.511466161174694,\n",
+ " 6.7905491508700839,\n",
+ " 15.012701654509073,\n",
+ " 6.0866446845575179,\n",
+ " 9.6102833795033984,\n",
+ " 1.7528230101567992,\n",
+ " 1.0856198193936075,\n",
+ " 1.4055517383172127,\n",
+ " 312.56035581076372,\n",
+ " 8.7206618652310013,\n",
+ " 5.8884282820273928,\n",
+ " 12.973426445005297,\n",
+ " 5.6962491848148424,\n",
+ " 2.9055885423056824,\n",
+ " 3.651619496046008,\n",
+ " 5.2630161144903642,\n",
+ " 5.5787239174837682,\n",
+ " 2.1366093185843091,\n",
+ " 0.56205566925437456,\n",
+ " 0.38181249793677163,\n",
+ " 4.9649993525385625,\n",
+ " 0.4515579737727316,\n",
+ " 0.44299658287932514,\n",
+ " 0.26405374820145183,\n",
+ " 0.52967715189276521,\n",
+ " 0.65285615575917921,\n",
+ " 0.099037556992691941,\n",
+ " 0.27968699316654838,\n",
+ " 0.13940832709503004,\n",
+ " 0.15393693887348939,\n",
+ " 0.085557839850704892,\n",
+ " 0.071435783848682669,\n",
+ " 0.48366754538010154,\n",
+ " 0.047494370160390979,\n",
+ " 0.34934021714509877,\n",
+ " 5.5782233952026239,\n",
+ " 24.9977492638535,\n",
+ " 9.3966855685703301,\n",
+ " 6.221949456806823,\n",
+ " 11.999332308419628,\n",
+ " 6.7059016750950571,\n",
+ " 4.9859224974261185,\n",
+ " 2.1033129739041576,\n",
+ " 5.9508488681381566,\n",
+ " 2.7348595694111943,\n",
+ " 0.49733267013126831,\n",
+ " 1.2112128862779814,\n",
+ " 1.4761181519981086,\n",
+ " 0.36710513327222544,\n",
+ " 0.57666513153149412,\n",
+ " 0.64980316750505873,\n",
+ " 2454.7818696630266,\n",
+ " 26.396563048441866,\n",
+ " 28.887422455894846,\n",
+ " 12.339407006029374,\n",
+ " 4.7379275089043036,\n",
+ " 6.6757844432774105,\n",
+ " 5.4771835812520733,\n",
+ " 7.3756771496905289,\n",
+ " 1.0035749417028266,\n",
+ " 1.6495081389009636,\n",
+ " 0.76840449718999826,\n",
+ " 3.8829299174474605,\n",
+ " 0.57963204451685857,\n",
+ " 0.74221644775915763,\n",
+ " 0.75964325070307881,\n",
+ " 0.87889151536711718,\n",
+ " 0.91441117226399371,\n",
+ " 1.139138262528353,\n",
+ " 1.8004058265048064,\n",
+ " 0.48189330383509371,\n",
+ " 1.0764792094144739,\n",
+ " 718.77548047876189,\n",
+ " 658.13666009443943,\n",
+ " 300.83342355891494,\n",
+ " 10.969388545663914,\n",
+ " 53.988655671012737,\n",
+ " 57.623457322441418,\n",
+ " 37.156799863022009,\n",
+ " 12.613325789107236,\n",
+ " 43.150815615675356,\n",
+ " 11.826568908355069,\n",
+ " 33.716569546177922,\n",
+ " 22.159483955550066,\n",
+ " 17.542113491185056,\n",
+ " 64.620876167152701,\n",
+ " 7.3501404080574249,\n",
+ " 14.990966383449178,\n",
+ " 1.3860330935973946,\n",
+ " 0.60299848045795368,\n",
+ " 1.7616044681413798,\n",
+ " 847.86058335096868,\n",
+ " 13.759672724101268,\n",
+ " 48.185319017264248,\n",
+ " 15.597080921596739,\n",
+ " 20.806389286407903,\n",
+ " 18.03644389691976,\n",
+ " 10.169318914032406,\n",
+ " 21.921858924192811,\n",
+ " 6.3954717203181337,\n",
+ " 3.5432442331048417,\n",
+ " 20.60362850280255,\n",
+ " 1.5616132611000033,\n",
+ " 0.93920044405031045,\n",
+ " 8.8561447903017427,\n",
+ " 4.6441748191882803,\n",
+ " 3.4524195772786932,\n",
+ " 2.1851664217075997,\n",
+ " 7.5275642621183234,\n",
+ " 2.9311778190078956,\n",
+ " 1.7094807642287078,\n",
+ " 13.308188217700494,\n",
+ " 18.255891885264294,\n",
+ " 3.726954478403981,\n",
+ " 16.55553312716615,\n",
+ " 7.0884198523702073,\n",
+ " 26.660846931951468,\n",
+ " 2.5480109185255362,\n",
+ " 3.7157908087630553,\n",
+ " 1.9648358636356158,\n",
+ " 1.4764246263862804,\n",
+ " 3.7493739270289907,\n",
+ " 1.0911979693820275,\n",
+ " 4.3681777975654796,\n",
+ " 0.54663941138050398,\n",
+ " 1.9542102348109289,\n",
+ " 2.179490811786637,\n",
+ " 1.0854548787138407,\n",
+ " 0.21835450749723032,\n",
+ " 0.40428495192529768,\n",
+ " 0.48738834268980402,\n",
+ " 0.45211821255689411,\n",
+ " 0.275932244240364,\n",
+ " 0.3016311102444188,\n",
+ " 0.90823387875967887,\n",
+ " 0.13619237401502318,\n",
+ " 3.5266524231562255,\n",
+ " 1506.8280781105989,\n",
+ " 107.6883893023787,\n",
+ " 46.289952167215851,\n",
+ " 28.690524779748326,\n",
+ " 31.479829248660547,\n",
+ " 5.7186680425978604,\n",
+ " 1.7678584750611481,\n",
+ " 8.7152912574151014,\n",
+ " 1.2499849056184673,\n",
+ " 25.852072384168363,\n",
+ " 2.4761783726207827,\n",
+ " 1.4386966079136581,\n",
+ " 15.550412449128924,\n",
+ " 11.520809773687372,\n",
+ " 54.985637662501269,\n",
+ " 18.309231305418326,\n",
+ " 7.0873801322025667,\n",
+ " 15.296487393188418,\n",
+ " 15.451814307882017,\n",
+ " 7.9171407589133738,\n",
+ " 9.0276067236235544,\n",
+ " 1.2999032689219414,\n",
+ " 2.5751665673641515,\n",
+ " 2.1635289511165192,\n",
+ " 8.7789545807025142,\n",
+ " 4.0174490328018786,\n",
+ " 6.8015687016179145,\n",
+ " 3.0627224376556743,\n",
+ " 2.800525943717016,\n",
+ " 2.6623988879488434,\n",
+ " 13.984293136840789,\n",
+ " 4.7522114792937611,\n",
+ " 5.8223441103447602,\n",
+ " 6.7726638171415479,\n",
+ " 9.1477098945529907,\n",
+ " 0.67465510146324636,\n",
+ " 0.51608962162209304,\n",
+ " 1.2909633404086538,\n",
+ " 68.223086140592656,\n",
+ " 2.9540559265511699,\n",
+ " 2.8846952707651377,\n",
+ " 1.1548735953385132,\n",
+ " 4.5589090710063749,\n",
+ " 2.7388528824491538,\n",
+ " 2.7320250812536524,\n",
+ " 5.136980983030468,\n",
+ " 14.55441753099201,\n",
+ " 2.7536195289964365,\n",
+ " 2.6055914396235824,\n",
+ " 3.3686414835597938,\n",
+ " 0.37977707474321054,\n",
+ " 0.77400483457513514,\n",
+ " 0.70222092254625723,\n",
+ " 16.923241522030125,\n",
+ " 0.8703625247046094,\n",
+ " 34.800670905714242,\n",
+ " 10.748366884124296,\n",
+ " 9.2732023224499454,\n",
+ " 6.039913187086829,\n",
+ " 9.9677208209644732,\n",
+ " 10.842343487317288,\n",
+ " 175.66448184398178,\n",
+ " 72.376535410452561,\n",
+ " 27.85937393218342,\n",
+ " 12.222514050878768,\n",
+ " 4.4335050809506349,\n",
+ " 1.443475807103423,\n",
+ " 1.7459369656437822,\n",
+ " 1.4009354517748533,\n",
+ " 5.1862874704797033,\n",
+ " 5.4857511072551297,\n",
+ " 2.1133511917325181,\n",
+ " 1.8861989165878978,\n",
+ " 1.0753645019489395,\n",
+ " 0.45347425217994042,\n",
+ " 1.1035690571173762,\n",
+ " 0.20279407951348877,\n",
+ " 2.2998482994609835,\n",
+ " 0.27183667157212532,\n",
+ " 0.41775626710770414,\n",
+ " 0.049497069918633098,\n",
+ " 0.18873029662340013,\n",
+ " 0.10650466517045734,\n",
+ " 0.3524911822996819,\n",
+ " 0.051358891965582597,\n",
+ " 0.038349975279293902,\n",
+ " 0.042764497183663076,\n",
+ " 0.33966723551582684,\n",
+ " 0.10332686927108405,\n",
+ " 0.055486517739106296,\n",
+ " 2.0286807979537671,\n",
+ " 1508.697952990145,\n",
+ " 109.5338729683692,\n",
+ " 12.0238261771218,\n",
+ " 32.214627765202081,\n",
+ " 21.560295091210403,\n",
+ " 17.037906107768986,\n",
+ " 3.265446195073709,\n",
+ " 7.9369567240660741,\n",
+ " 3.157120000012049,\n",
+ " 9.6763651901440362,\n",
+ " 7.7421284297192736,\n",
+ " 11.114834403235799,\n",
+ " 1.887671579779918,\n",
+ " 1.238590005542562,\n",
+ " 6.5014980054185729,\n",
+ " 6.7019572211135836,\n",
+ " 2.4852086740539341,\n",
+ " 1.6437322859223273,\n",
+ " 0.48641259230258194,\n",
+ " 1.3977538879140639,\n",
+ " 0.95461672157506283,\n",
+ " 0.83935748156293855,\n",
+ " 282.20033570970241,\n",
+ " 41.6476809245653,\n",
+ " 10.394554319542952,\n",
+ " 9.1412068093949284,\n",
+ " 11.11810896891985,\n",
+ " 33.236603081781183,\n",
+ " 3.3752093808358441,\n",
+ " 3.7529819354894349,\n",
+ " 2.5983881144780638,\n",
+ " 1.4896515427357837,\n",
+ " 3.4290211146476879,\n",
+ " 8.6974663613840644,\n",
+ " 1.2382989545178573,\n",
+ " 2.3824049846865187,\n",
+ " 1.4897859868437453,\n",
+ " 1.2378111621835124,\n",
+ " 0.3853345823493165,\n",
+ " 0.31965462447617055,\n",
+ " 1.5950240435976668,\n",
+ " 1.2274519968563391,\n",
+ " 0.93733839111406203,\n",
+ " 0.60373658135570551,\n",
+ " 0.16377647910477119,\n",
+ " 0.48131552629104268,\n",
+ " 1.4641643919708229,\n",
+ " 0.25429169108688626,\n",
+ " 0.23682008331197008,\n",
+ " 0.19669397363501567,\n",
+ " 0.3623376052636299,\n",
+ " 0.24096339759323246,\n",
+ " 0.049491525353001178,\n",
+ " 0.23690199035872883,\n",
+ " 0.18320688503940388,\n",
+ " 0.10824743308595516,\n",
+ " 0.19585993304959692,\n",
+ " 0.58914312307853944,\n",
+ " 0.16811734470880965,\n",
+ " 0.17042833150894318,\n",
+ " 0.17395285693206664,\n",
+ " 0.30788339443010188,\n",
+ " 0.08969909062055996,\n",
+ " 0.27657148467527914,\n",
+ " 0.12335075555175334,\n",
+ " 0.26649647710707608,\n",
+ " 67.947466019239286,\n",
+ " 39.258256150854031,\n",
+ " 50.618006797419945,\n",
+ " 14.94134881264414,\n",
+ " 4.5976886952194027,\n",
+ " 4.4090114133077485,\n",
+ " 10.926445745391076,\n",
+ " 6.4328981155220593,\n",
+ " 74.123501032282519,\n",
+ " 15.956975537737167,\n",
+ " 7.5004545456514666,\n",
+ " 14.434721827005532,\n",
+ " 26.49233318211795,\n",
+ " 16.881760738743719,\n",
+ " 9.4124507232979866,\n",
+ " 6.9120987082469849,\n",
+ " 43.623668183393811,\n",
+ " 115.4623591981281,\n",
+ " 4.5266419711917871,\n",
+ " 17.1656355714039,\n",
+ " 15.152190357675128,\n",
+ " 21.674610619399157,\n",
+ " 13.00900810873245,\n",
+ " 3.3897417321573347,\n",
+ " 40.20886545939603,\n",
+ " 2.3490845807547327,\n",
+ " 2.372339417243293,\n",
+ " 7.6133357607191083,\n",
+ " 11.279497301059722,\n",
+ " 2.2984134965362304,\n",
+ " 2.528799059613803,\n",
+ " 3.313260308565277,\n",
+ " 7.0122182966330389,\n",
+ " 1.1142475231848825,\n",
+ " 4.3368923969094393,\n",
+ " 158.37660287580229,\n",
+ " 41.178551124017098,\n",
+ " 16.317760202327271,\n",
+ " 4.3410106571010472,\n",
+ " 26.030988726762981,\n",
+ " 3.0431579329743887,\n",
+ " 10.65241828332103,\n",
+ " 12.216616956610554,\n",
+ " 8.6160389770223098,\n",
+ " 10.813387903645719,\n",
+ " 7.2573397947714318,\n",
+ " 1.7896402519049013,\n",
+ " 2.4536598665990801,\n",
+ " 1.7321791476564687,\n",
+ " 2.9416762796849882,\n",
+ " 16.307873872947457,\n",
+ " 1.9114802403203048,\n",
+ " 2.2074000447909872,\n",
+ " 1.4769524768348594,\n",
+ " 10.59684259039885,\n",
+ " 3.8005429533801314,\n",
+ " 2.7605750233567194,\n",
+ " 2.6615040569409203,\n",
+ " 2.2614817989192195,\n",
+ " 2.5150403284242553,\n",
+ " 1.126160262380437,\n",
+ " 2.3263397293011199,\n",
+ " 34.834614438139496,\n",
+ " 16.329195644686347,\n",
+ " 3.1862447804276983,\n",
+ " 7.0945993048299654,\n",
+ " 8.6256038209367212,\n",
+ " 9.2121722852514001,\n",
+ " 1504.4826746247679,\n",
+ " 55.52248164196255,\n",
+ " 82.101251520969768,\n",
+ " 21.182235540856958,\n",
+ " 17.80465351765881,\n",
+ " 9.1070714462835056,\n",
+ " 5.4029523557845289,\n",
+ " 2.7656321191442457,\n",
+ " 7.0619619771647573,\n",
+ " 3.7010558116644248,\n",
+ " 4.0083074098873901,\n",
+ " 2.0468196930457574,\n",
+ " 3.1707235923860067,\n",
+ " 1.1776066853333265,\n",
+ " 0.70707208940715149,\n",
+ " 0.58659477411818084,\n",
+ " 1.8370042168770961,\n",
+ " 1.3433680975008337,\n",
+ " 0.46466577945958942,\n",
+ " 2.349757899485208,\n",
+ " 0.55268805855859149,\n",
+ " 0.92270712980348513,\n",
+ " 0.57972508712256099,\n",
+ " 1.2754152431333203,\n",
+ " 0.52010706969847242,\n",
+ " 0.21897605561037381,\n",
+ " 0.073669011645183122,\n",
+ " 0.69341324700790075,\n",
+ " 0.43703836238266008,\n",
+ " 0.59060098977405528,\n",
+ " 0.10004005920415068,\n",
+ " 0.17478345891014993,\n",
+ " 0.32633199871652335,\n",
+ " 0.30926436064735358,\n",
+ " 0.47823262280618634,\n",
+ " 0.1866858161887027,\n",
+ " 0.23580479946746233,\n",
+ " 0.088359759398009274,\n",
+ " 0.19957184870512967,\n",
+ " 0.08816995767269481,\n",
+ " 0.050398487427938093,\n",
+ " 0.2012643271682838,\n",
+ " 0.076752208010090223,\n",
+ " 0.3828223678273559,\n",
+ " 0.076619491992714298,\n",
+ " 0.12170731649706042,\n",
+ " 0.25612033741397611,\n",
+ " 0.082118216893908344,\n",
+ " 0.10093209897502972,\n",
+ " 0.21030505279447415,\n",
+ " 0.06140357100990692,\n",
+ " 0.043764751727327016,\n",
+ " 0.37577564974313321,\n",
+ " 0.16183382839783597,\n",
+ " 28.56289286059873,\n",
+ " 1123.6976571168673,\n",
+ " 25.602059041213025,\n",
+ " 16.413164394617656,\n",
+ " 17.106881019180314,\n",
+ " 39.979321357850857,\n",
+ " 27.324859906447976,\n",
+ " 12.390665789873889,\n",
+ " 21.272100414864866,\n",
+ " 20.037471402010784,\n",
+ " 3.7239087986423209,\n",
+ " 3.0777452691488807,\n",
+ " 2.6019103521376552,\n",
+ " 4.0761300634047837,\n",
+ " 3.1941779169381443,\n",
+ " 0.35264276396085753,\n",
+ " 2.3620856344811698,\n",
+ " 234.70851047072694,\n",
+ " 32.430599041035215,\n",
+ " 10.060253381640516,\n",
+ " 12.14717976765985,\n",
+ " 54.656011336093009,\n",
+ " 41.019372087453668,\n",
+ " 17.896428396772343,\n",
+ " 8.2779453792826008,\n",
+ " 9.4902906338312913,\n",
+ " 3.7742751346264911,\n",
+ " 2.4968101001967775,\n",
+ " 4.6720592781677439,\n",
+ " 1.9800091405250331,\n",
+ " 1.2935738516970008,\n",
+ " 5.5306351701543237,\n",
+ " 468.28534921749957,\n",
+ " 36.258535474409378,\n",
+ " 32.504598783399615,\n",
+ " 7.342808925981573,\n",
+ " 21.516600021223635,\n",
+ " 24.965951743888702,\n",
+ " 6.5434873859102014,\n",
+ " 4.3167795903827386,\n",
+ " 5.3439608493988464,\n",
+ " 21.138561996950081,\n",
+ " 4.1276023725314186,\n",
+ " 12.708148935459031,\n",
+ " 6.1296293234499384,\n",
+ " 5.6248911084191757,\n",
+ " 21.449967391442115,\n",
+ " 81.873703548620554,\n",
+ " 25.712228361123781,\n",
+ " 228.10080526447283,\n",
+ " 37.240013337614776,\n",
+ " 45.05859740349468,\n",
+ " 16.923995275716578,\n",
+ " 31.152981607283838,\n",
+ " 5.6293355410978201,\n",
+ " 11.635885962573251,\n",
+ " 13.544837747001866,\n",
+ " 41.862499404171373,\n",
+ " 3.6213626109841321,\n",
+ " 3.7873885618448457,\n",
+ " 11.00058473321225,\n",
+ " 2.6241602089204843,\n",
+ " 2.838934636412711,\n",
+ " 15.187239197218101,\n",
+ " 2.2401783228647818,\n",
+ " 3.0054162062050369,\n",
+ " 1.9355487372294875,\n",
+ " 2.4581184863344552,\n",
+ " 1.7883014093351584,\n",
+ " 0.44895675213443254,\n",
+ " 1.4604248001394804,\n",
+ " 2.3163766856759835,\n",
+ " 0.34657063052285325,\n",
+ " 1.2974182237946865,\n",
+ " 0.4744000692744193,\n",
+ " 0.20121441786243172,\n",
+ " 0.40888537379542977,\n",
+ " 0.62634317813552798,\n",
+ " 31.714711886777877,\n",
+ " 3.7174356421074735,\n",
+ " 9.0312610775700382,\n",
+ " 3.1810239936594495,\n",
+ " 5.5678841368188179,\n",
+ " 403.43140061066447,\n",
+ " 87.426806138753847,\n",
+ " 24.076121840712574,\n",
+ " 13.268791535878062,\n",
+ " 47.475909220603988,\n",
+ " 7.8586363815152929,\n",
+ " 11.788534557397567,\n",
+ " 4.1269109783899633,\n",
+ " 30.443147403574216,\n",
+ " 12.58668617178491,\n",
+ " 2.2208256417757126,\n",
+ " 2.36456647125316,\n",
+ " 15.150964523800369,\n",
+ " 0.75869627475206192,\n",
+ " 3.7485048455120453,\n",
+ " 4.1927509209130456,\n",
+ " 3.7310190322675427,\n",
+ " 3.581273567279641,\n",
+ " 5.998739594680421,\n",
+ " 8.201202619577348,\n",
+ " 4.676154710135612,\n",
+ " 6.8221872258916907,\n",
+ " 4.1295095363220584,\n",
+ " 4.928102011763893,\n",
+ " 3.8091259509798125,\n",
+ " 1.0437067000496785,\n",
+ " 11.450192867726587,\n",
+ " 0.7835521906385019,\n",
+ " 0.48403068766927648,\n",
+ " 0.86063984264415661,\n",
+ " 38.92063451615779,\n",
+ " 83.273654272235163,\n",
+ " 19.942254150753627,\n",
+ " 11.751082404486029,\n",
+ " 106.04421321994361,\n",
+ " 2.3917064494671667,\n",
+ " 13.432505201536294,\n",
+ " 37.468971792037522,\n",
+ " 14.045322200869737,\n",
+ " 3.5711656236504368,\n",
+ " 15.71890462406124,\n",
+ " 21.244659982892561,\n",
+ " 14.07144365465888,\n",
+ " 22.966112324222514,\n",
+ " 1.1973319116081729,\n",
+ " 26.040327279744432,\n",
+ " 1.348321111894202,\n",
+ " 3.7083595273052827,\n",
+ " 11.37454885284347,\n",
+ " 9.036645402547963,\n",
+ " 2.071380580621883,\n",
+ " 2.61477550331526,\n",
+ " 111.39649733381135,\n",
+ " 41.853737854659791,\n",
+ " 492.14297469880739,\n",
+ " 30.525578125322749,\n",
+ " 177.65718576222307,\n",
+ " 4.9111449454750549,\n",
+ " 15.601039969505289,\n",
+ " 18.942585512142401,\n",
+ " 10.210326678865512,\n",
+ " 3.0463852955147548,\n",
+ " 7.1943690725796818,\n",
+ " 2.1085415072683613,\n",
+ " 1.6926676422937059,\n",
+ " 3.8510950514873841,\n",
+ " 9.5255046984305451,\n",
+ " 4.1686970162699035,\n",
+ " 2.2204724667703162,\n",
+ " 0.3962684837983575,\n",
+ " 0.35268153388581402,\n",
+ " 0.71205845271216384,\n",
+ " 4.9665946207320095,\n",
+ " 33.905600497248876,\n",
+ " 21.962475684600872,\n",
+ " 12.257863045049554,\n",
+ " 13.612235819521553,\n",
+ " 10.540395909106479,\n",
+ " 12.095816326086586,\n",
+ " 2.2443083684230078,\n",
+ " 4.7332641198674539,\n",
+ " 1.5513529126256191,\n",
+ " 3.1655086842605944,\n",
+ " 6.9762581078520585,\n",
+ " 10.628620133670607,\n",
+ " 4.4087480732833315,\n",
+ " 177.9259669284435,\n",
+ " 13.666373535892927,\n",
+ " 7.3745969328794452,\n",
+ " 10.635682580362655,\n",
+ " 8.6052455067954146,\n",
+ " 15.206407324120955,\n",
+ " 6.0718635187907726,\n",
+ " 10.315260062018844,\n",
+ " 2.5296573163912073,\n",
+ " 11.966566850408839,\n",
+ " 5.2896559061849269,\n",
+ " 17.733117362735562,\n",
+ " 5.3422044496545258,\n",
+ " 7.5452456579121039,\n",
+ " 2.9671832375271969,\n",
+ " 9.8039582529827776,\n",
+ " 2.2864289867710874,\n",
+ " 2.530383394011134,\n",
+ " 0.87122873401701373,\n",
+ " 0.59540174594430861,\n",
+ " 0.47644197369144209,\n",
+ " 0.36044102001583528,\n",
+ " 1.1051926054409449,\n",
+ " 1.2503206025514129,\n",
+ " 793.37551408334616,\n",
+ " 27.075145073933431,\n",
+ " 6.3001061779767751,\n",
+ " 15.213103354187901,\n",
+ " 3.5084786229876208,\n",
+ " 1.6689802854867397,\n",
+ " 4.7219732949398328,\n",
+ " 2.3200354517089425,\n",
+ " 0.42633483291849933,\n",
+ " 2.9941927124028389,\n",
+ " 3.342917976337342,\n",
+ " 1.4182471916224302,\n",
+ " 1.7612602241532749,\n",
+ " 1.0514834475630048,\n",
+ " 1.5432638139173041,\n",
+ " 1.9993538793339714,\n",
+ " 0.49779882480254917,\n",
+ " 0.53585224551956601,\n",
+ " 16.090619179017601,\n",
+ " 6.5143983858326528,\n",
+ " 7.0923358046809328,\n",
+ " 2.9783424448977938,\n",
+ " 3.1039307383666839,\n",
+ " 1.6045106821507136,\n",
+ " 2.5784708087578969,\n",
+ " 1.0866233945886601,\n",
+ " 0.61542445069408291,\n",
+ " 3.4647635271367738,\n",
+ " 0.69294259196899077,\n",
+ " 0.75670027555816233,\n",
+ " 8.6549551295140041,\n",
+ " 1.6297647943398343,\n",
+ " 1.8730382215380417,\n",
+ " 1.3476573196642618,\n",
+ " 1.9601684528646084,\n",
+ " 0.40176922758883044,\n",
+ " 1.4074180870668667,\n",
+ " 3.5196146751770985,\n",
+ " 1.587891055178587,\n",
+ " 0.37722688310610591,\n",
+ " 1.0523130483440468,\n",
+ " 0.55132586733065714,\n",
+ " 0.087282622882003558,\n",
+ " 0.33289464166320004,\n",
+ " 0.52567535255080933,\n",
+ " 0.18358349402553475,\n",
+ " 0.12011551793094501,\n",
+ " 0.20673947748818752,\n",
+ " 0.21097556086894334,\n",
+ " 0.21950185788822726,\n",
+ " 101.60897246755394,\n",
+ " 64.591467923952138,\n",
+ " 28.611379001573191,\n",
+ " 1456.1758773917627,\n",
+ " 165.6295029544103,\n",
+ " 51.021290756001854,\n",
+ " 52.561607669431503,\n",
+ " 28.25267166735286,\n",
+ " 8.8690835700194821,\n",
+ " 10.596055735945511,\n",
+ " 8.9777568927165508,\n",
+ " 7.5123256533810912,\n",
+ " 1.4817063388266871,\n",
+ " 8.3294010736438224,\n",
+ " 13.609561313594211,\n",
+ " 2.5713025919394781,\n",
+ " 2.1065254532808977,\n",
+ " 3.761211083083988,\n",
+ " 1.5640244137371655,\n",
+ " 2.9770140879542208,\n",
+ " 0.42905302479654878,\n",
+ " 0.55886624364179682,\n",
+ " 2.1495484085113024,\n",
+ " 0.28685004009797055,\n",
+ " 0.6019766489770717,\n",
+ " 0.22478638756087577,\n",
+ " 0.30643748863585768,\n",
+ " 0.1099585730674183,\n",
+ " 0.37316394305500633,\n",
+ " 0.28385785483978271,\n",
+ " 0.28574817913175166,\n",
+ " 0.32193540194535386,\n",
+ " 0.083244716137986799,\n",
+ " 0.13556876840912682,\n",
+ " 0.31154824034046746,\n",
+ " 1.1783982436097886,\n",
+ " 0.44228194326455489,\n",
+ " 206.80145868783598,\n",
+ " 2.8802924451420515,\n",
+ " 25.987891893113567,\n",
+ " 6.1063612992869416,\n",
+ " 20.396980771832379,\n",
+ " 7.1238803738362586,\n",
+ " 4.4481395028917392,\n",
+ " 3.2360504819038418,\n",
+ " 2.7237142073814211,\n",
+ " 5.3234666341524592,\n",
+ " 24.748524722796517,\n",
+ " 17.638627337313448,\n",
+ " 4.6307993795392379,\n",
+ " 0.90966051277664661,\n",
+ " 5.0063140696348629,\n",
+ " 78.348091044662397,\n",
+ " 6.8055986312027574,\n",
+ " 7.8697037277281492,\n",
+ " 9.5969127908991947,\n",
+ " 2.5974505760194191,\n",
+ " 19.800555404077958,\n",
+ " 15.579922762747737,\n",
+ " 11.807934328597732,\n",
+ " 25.472517908866187,\n",
+ " 3.1474540647274738,\n",
+ " 5.3170600549003328,\n",
+ " 2.944183551715216,\n",
+ " 3.1383417688301569,\n",
+ " 8.911024765123118,\n",
+ " 0.76391120498742271,\n",
+ " 2.0723824757727085,\n",
+ " 2.9420976190961339,\n",
+ " 1.3858284046687173,\n",
+ " 1.6523928797206588,\n",
+ " 1.4789908311253304,\n",
+ " 1.4249119237947929,\n",
+ " 2.3685666210429108,\n",
+ " 0.60804219091687495,\n",
+ " 423.36985940601505,\n",
+ " 30.792501414300979,\n",
+ " 18.108768790001413,\n",
+ " 4.4496105005404933,\n",
+ " 14.757672295025035,\n",
+ " 104.74910598224882,\n",
+ " 4.4333734982805639,\n",
+ " 2.2313807098016873,\n",
+ " 3.9034525360685866,\n",
+ " 7.7117577651210523,\n",
+ " 6.1938556811717156,\n",
+ " 4.2520554317870332,\n",
+ " 3.320979906957982,\n",
+ " 0.54421532627677194,\n",
+ " 1.8976358242377152,\n",
+ " 1.5594876153221644,\n",
+ " 2.1538563521252825,\n",
+ " 0.7604070227438775,\n",
+ " 0.73363873780822941,\n",
+ " 0.21913332484550013,\n",
+ " 891.15756876491446,\n",
+ " 24.106129910195552,\n",
+ " 40.549982251746016,\n",
+ " 16.641952075341461,\n",
+ " 41.22271913895807,\n",
+ " 5.8035740618139275,\n",
+ " 14.174418898652359,\n",
+ " 11.738416251242464,\n",
+ " 2.6172745317267014,\n",
+ " 5.9978935577173216,\n",
+ " 0.99116467374156292,\n",
+ " 1.5916535945179819,\n",
+ " 1.4418379081953687,\n",
+ " 2.0971628761202985,\n",
+ " 4.5806755052692463,\n",
+ " 3.4650652941685549,\n",
+ " 1.1301455963602338,\n",
+ " 8.1914801473741843,\n",
+ " 0.86028055579823315,\n",
+ " 0.5310759373309778,\n",
+ " 0.34612140418335874,\n",
+ " 0.79901496739263989,\n",
+ " 0.25288991597343147,\n",
+ " 0.27589497048441114,\n",
+ " 0.41207016024727572,\n",
+ " 0.99396700069859534,\n",
+ " 0.028782581589787807,\n",
+ " 0.40115808853469181,\n",
+ " 0.62023234760494994,\n",
+ " 0.056774407236603569,\n",
+ " 2.361008327584099,\n",
+ " 12.438128356920537,\n",
+ " 7.2227608186317092,\n",
+ " 11.964806233104646,\n",
+ " 4.2924424242507992,\n",
+ " 5.0596525695310417,\n",
+ " 5.4453602393851366,\n",
+ " 2.9286365581159113,\n",
+ " 423.50902658864288,\n",
+ " 21.921462193880721,\n",
+ " 113.7931607914916,\n",
+ " 29.323322201845251,\n",
+ " 66.648483247019044,\n",
+ " 10.269018255497343,\n",
+ " 18.66065870399666,\n",
+ " 15.910596431059693,\n",
+ " 23.229557861920124,\n",
+ " 3.5914157722229341,\n",
+ " 12.815105541912395,\n",
+ " 127.6200334104672,\n",
+ " 19.621608929637834,\n",
+ " 2.9697097237454662,\n",
+ " 18.337734990967085,\n",
+ " 30.229399069395829,\n",
+ " 6.1986973174024822,\n",
+ " 8.4202421411346453,\n",
+ " 10.893035277255745,\n",
+ " 19.031671851874311,\n",
+ " 9.2278852402523164,\n",
+ " 3.2917412883106643,\n",
+ " 8.0765095953023209,\n",
+ " 43.204224867379672,\n",
+ " 13.283740967101192,\n",
+ " 417.98025083316935,\n",
+ " 71.737027211951073,\n",
+ " 5.8050067213390859,\n",
+ " 24.741978287557671,\n",
+ " 5.6271566194246097,\n",
+ " 27.178181861038315,\n",
+ " 67.945896937352103,\n",
+ " 111.15987019621114,\n",
+ " 5.4454895505888183,\n",
+ " 10.988111810094345,\n",
+ " 10.473053574263009,\n",
+ " 4.9013029610019574,\n",
+ " 4.0171820792294666,\n",
+ " 8.9073567587718578,\n",
+ " 2.6878639994814955,\n",
+ " 1.9237037569575628,\n",
+ " 3.3499856738202616,\n",
+ " 2.7060961664490222,\n",
+ " 2.0666124060761559,\n",
+ " 3.7662084405353893,\n",
+ " 50.305215829430303,\n",
+ " 5.4685807265137685,\n",
+ " 3.7086138534640098,\n",
+ " 1.4483488036938259,\n",
+ " 7.1327752419114994,\n",
+ " 0.87516263232672897,\n",
+ " 2.3836941789899146,\n",
+ " 0.8136700861352324,\n",
+ " 1.3030216443128613,\n",
+ " 0.8322413856951506,\n",
+ " 3.5300857798046148,\n",
+ " 1.8656735347030518,\n",
+ " 2.6913742328152406,\n",
+ " 0.31387230942420385,\n",
+ " 0.30973705222222869,\n",
+ " 0.4302099522047762,\n",
+ " 0.85415076254776612,\n",
+ " 0.17700884709455461,\n",
+ " 0.35559398518210983,\n",
+ " 0.59712496301664952,\n",
+ " 1.496592072083649,\n",
+ " 0.24621983608265074,\n",
+ " 0.43038742818888887,\n",
+ " 0.086826806419915456,\n",
+ " 0.045647400148162751,\n",
+ " 0.21029399656342113,\n",
+ " 1201.9578249593617,\n",
+ " 86.882938492142443,\n",
+ " 19.223454651738933,\n",
+ " 25.565714138828522,\n",
+ " 5.9343702291175315,\n",
+ " 5.4899912391923946,\n",
+ " 8.4396730768861623,\n",
+ " 15.990251539056429,\n",
+ " 7.6240011206820064,\n",
+ " 1.5224493121852121,\n",
+ " ...]"
+ ]
+ },
+ "execution_count": 5,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "qs_save"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 6,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "'kg / s'"
+ ]
+ },
+ "execution_count": 6,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "hydrotrend.var_units(\"channel_exit_water_sediment~suspended__mass_flow_rate\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.7.4"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/child.ipynb b/lessons/pymt/child.ipynb
new file mode 100644
index 0000000..64c8c3f
--- /dev/null
+++ b/lessons/pymt/child.ipynb
@@ -0,0 +1,1007 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## CHILD Landscape Evolution Model\n",
+ "* Link to this notebook: https://github.com/csdms/pymt/blob/master/docs/demos/child.ipynb\n",
+ "* Install command: `$ conda install notebook pymt_child`\n",
+ "\n"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Import the `Sedflux3D` component from `pymt`. All of the components available to `pymt` are located in `pymt.components`. Here I've renamed the component to be `Model` to show that you could run these same commands with other models as well. For instance, you could instead import `Child` with `from pymt.components import Child as Model` and repeat this exercise with Child instead."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 1,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "from __future__ import print_function\n",
+ "\n",
+ "# Some magic to make plots appear within the notebook\n",
+ "%matplotlib inline\n",
+ "\n",
+ "import numpy as np # In case we need to use numpy"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Run CHILD in PyMT"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We'll now do the same thing but this time with the Child model. Notice that the commands will be the same. *If you know how to run one PyMT component, you know how to run them all.*"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 2,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stderr",
+ "output_type": "stream",
+ "text": [
+ "\u001b[32m✓ Avulsion\u001b[39;49;00m\n",
+ "\u001b[32m✓ Plume\u001b[39;49;00m\n",
+ "\u001b[32m✓ Sedflux3D\u001b[39;49;00m\n",
+ "\u001b[32m✓ Subside\u001b[39;49;00m\n",
+ "\u001b[32m✓ Hydrotrend\u001b[39;49;00m\n",
+ "/Users/huttone/anaconda/envs/excom_demo/lib/python3.6/site-packages/landlab/bmi/components.py:13: UserWarning: unable to wrap class Lithology\n",
+ " warnings.warn('unable to wrap class {name}'.format(name=cls.__name__))\n",
+ "/Users/huttone/anaconda/envs/excom_demo/lib/python3.6/site-packages/landlab/bmi/components.py:13: UserWarning: unable to wrap class LithoLayers\n",
+ " warnings.warn('unable to wrap class {name}'.format(name=cls.__name__))\n",
+ "\u001b[32m✓ Flexure\u001b[39;49;00m\n",
+ "\u001b[32m✓ OverlandFlow\u001b[39;49;00m\n",
+ "\u001b[32m✓ Child\u001b[39;49;00m\n"
+ ]
+ }
+ ],
+ "source": [
+ "import pymt.models\n",
+ "\n",
+ "model = pymt.models.Child()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "You can now see the help information for Child. This time, have a look under the *Parameters* section (you may have to scroll down - it's the section after the citations). The *Parameters* section describes optional keywords that you can pass the the `setup` method. In the previous example we just used defaults. Below we'll see how to set input file parameters programmatically through keywords."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 3,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "Help on Child in module pymt.framework.bmi_bridge object:\n",
+ "\n",
+ "class Child(BmiCap)\n",
+ " | Basic Model Interface for child.\n",
+ " | \n",
+ " | CHILD computes the time evolution of a topographic surface z(x,y,t) by\n",
+ " | fluvial and hillslope erosion and sediment transport.\n",
+ " | \n",
+ " | Author:\n",
+ " | - Greg Tucker\n",
+ " | - Stephen Lancaster\n",
+ " | - Nicole Gasparini\n",
+ " | - Rafael Bras\n",
+ " | - Scott Rybarczyk\n",
+ " | Version: 10.6\n",
+ " | License: GPLv2\n",
+ " | DOI: 10.1594/IEDA/100102\n",
+ " | URL: http://csdms.colorado.edu/wiki/Model:CHILD\n",
+ " | \n",
+ " | Cite as:\n",
+ " | \n",
+ " | @article{tucker2010child,\n",
+ " | title={CHILD Users Guide for version R9. 4.1},\n",
+ " | author={Tucker, Gregory E},\n",
+ " | journal={Cooperative Institute for Research in Environmental Sciences (CIRES) and Department of Geological Sciences, University of Colorado, Boulder, USA},\n",
+ " | year={2010}\n",
+ " | }\n",
+ " | \n",
+ " | @article{tucker2001object,\n",
+ " | title={An object-oriented framework for distributed hydrologic and geomorphic modeling using triangulated irregular networks},\n",
+ " | author={Tucker, Gregory E and Lancaster, Stephen T and Gasparini, Nicole M and Bras, Rafael L and Rybarczyk, Scott M},\n",
+ " | journal={Computers \\& Geosciences},\n",
+ " | volume={27},\n",
+ " | number={8},\n",
+ " | pages={959--973},\n",
+ " | year={2001},\n",
+ " | publisher={Elsevier}\n",
+ " | }\n",
+ " | \n",
+ " | \n",
+ " | Parameters\n",
+ " | ----------\n",
+ " | fault_position : float, optional\n",
+ " | Fault position [default=30000.0 m]\n",
+ " | grid_node_spacing : float, optional\n",
+ " | Mean distance between grid nodes [default=1000.0 m]\n",
+ " | grid_x_size : float, optional\n",
+ " | Length of grid in x-dimension [default=20000.0 m]\n",
+ " | grid_y_size : float, optional\n",
+ " | Length of grid in y-dimension [default=40000.0 m]\n",
+ " | run_duration : float, optional\n",
+ " | Simulation run time [default=5000.0 year]\n",
+ " | subsidence_rate : float, optional\n",
+ " | Subsidence rate [default=50.0 m / year]\n",
+ " | uplift_duration : float, optional\n",
+ " | Duration of uplift [default=1.0 y]\n",
+ " | uplift_rate : float, optional\n",
+ " | Uplift rate [default=30.0 m / year]\n",
+ " | uplift_type : str, optional\n",
+ " | Type of uplift (0=none, 1=uniform, 2=block, etc) [default=0 ]\n",
+ " | \n",
+ " | Examples\n",
+ " | --------\n",
+ " | >>> from pymt.components import child\n",
+ " | >>> model = child()\n",
+ " | >>> (fname, initdir) = model.setup()\n",
+ " | >>> model.initialize(fname, dir=initdir)\n",
+ " | >>> for _ in xrange(10):\n",
+ " | ... model.update()\n",
+ " | >>> model.finalize()\n",
+ " | \n",
+ " | Method resolution order:\n",
+ " | Child\n",
+ " | BmiCap\n",
+ " | pymt.framework.bmi_mapper.GridMapperMixIn\n",
+ " | _BmiCap\n",
+ " | BmiTimeInterpolator\n",
+ " | pymt.framework.bmi_setup.SetupMixIn\n",
+ " | builtins.object\n",
+ " | \n",
+ " | Methods inherited from pymt.framework.bmi_mapper.GridMapperMixIn:\n",
+ " | \n",
+ " | esmf_field(self, gid, name=None, at='node')\n",
+ " | \n",
+ " | esmf_mesh(self, gid)\n",
+ " | \n",
+ " | map_to(self, name, **kwds)\n",
+ " | Map values to another grid.\n",
+ " | \n",
+ " | Parameters\n",
+ " | ----------\n",
+ " | name : str\n",
+ " | Name of values to push.\n",
+ " | \n",
+ " | map_value(self, name, **kwds)\n",
+ " | Map values from another grid.\n",
+ " | \n",
+ " | Parameters\n",
+ " | ----------\n",
+ " | name : str\n",
+ " | Name of values to map to.\n",
+ " | mapfrom : tuple or bmi_like, optional\n",
+ " | BMI object from which values are mapped from. This can also be\n",
+ " | a tuple of *(name, bmi)*, where *name* is the variable of the\n",
+ " | source grid and *bmi* is the bmi-like source. If not provided,\n",
+ " | use *self*.\n",
+ " | nomap : narray of bool, optional\n",
+ " | Values in the destination grid to not map.\n",
+ " | \n",
+ " | regrid(self, name, **kwds)\n",
+ " | Regrid values from one grid to another.\n",
+ " | \n",
+ " | Parameters\n",
+ " | ----------\n",
+ " | name : str\n",
+ " | Name of the values to regrid.\n",
+ " | to : bmi_like, optional\n",
+ " | BMI object onto which to map values. If not provided, map\n",
+ " | values onto one of the object's own grids.\n",
+ " | to_name : str, optional\n",
+ " | Name of the value to map onto. If not provided, use *name*.\n",
+ " | \n",
+ " | Returns\n",
+ " | -------\n",
+ " | ndarray\n",
+ " | The regridded values.\n",
+ " | \n",
+ " | set_value(self, name, *args, **kwds)\n",
+ " | Set values for a variable.\n",
+ " | set_value(name, value)\n",
+ " | set_value(name, mapfrom=self, nomap=None)\n",
+ " | \n",
+ " | Parameters\n",
+ " | ----------\n",
+ " | name : str\n",
+ " | Name of the destination values.\n",
+ " | \n",
+ " | ----------------------------------------------------------------------\n",
+ " | Data descriptors inherited from pymt.framework.bmi_mapper.GridMapperMixIn:\n",
+ " | \n",
+ " | __dict__\n",
+ " | dictionary for instance variables (if defined)\n",
+ " | \n",
+ " | __weakref__\n",
+ " | list of weak references to the object (if defined)\n",
+ " | \n",
+ " | ----------------------------------------------------------------------\n",
+ " | Methods inherited from _BmiCap:\n",
+ " | \n",
+ " | __init__(self)\n",
+ " | Initialize self. See help(type(self)) for accurate signature.\n",
+ " | \n",
+ " | __str__(self)\n",
+ " | Return str(self).\n",
+ " | \n",
+ " | as_dict(self)\n",
+ " | \n",
+ " | as_json(self)\n",
+ " | \n",
+ " | as_yaml(self)\n",
+ " | \n",
+ " | finalize(self)\n",
+ " | \n",
+ " | get_component_name(self)\n",
+ " | \n",
+ " | get_current_time(self, units=None)\n",
+ " | \n",
+ " | get_end_time(self, units=None)\n",
+ " | \n",
+ " | get_grid_dim(self, grid, dim)\n",
+ " | \n",
+ " | get_grid_face_node_connectivity(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_face_node_offset(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_face_nodes(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_ndim(self, grid)\n",
+ " | \n",
+ " | get_grid_nodes_per_face(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_number_of_faces(self, grid)\n",
+ " | \n",
+ " | get_grid_number_of_nodes(self, grid)\n",
+ " | \n",
+ " | get_grid_number_of_vertices(self, grid)\n",
+ " | \n",
+ " | get_grid_origin(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_rank(self, grid)\n",
+ " | .. note:: deprecated\n",
+ " | \n",
+ " | Use :func:`get_grid_ndim` instead.\n",
+ " | \n",
+ " | get_grid_shape(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_size(self, grid)\n",
+ " | .. note:: deprecated\n",
+ " | \n",
+ " | Use :func:`get_grid_number_of_nodes` instead.\n",
+ " | \n",
+ " | get_grid_spacing(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_type(self, grid)\n",
+ " | \n",
+ " | get_grid_x(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_y(self, grid, out=None)\n",
+ " | \n",
+ " | get_grid_z(self, grid, out=None)\n",
+ " | \n",
+ " | get_input_var_names(self)\n",
+ " | \n",
+ " | get_output_var_names(self)\n",
+ " | \n",
+ " | get_start_time(self, units=None)\n",
+ " | \n",
+ " | get_time_step(self, units=None)\n",
+ " | \n",
+ " | get_time_units(self)\n",
+ " | \n",
+ " | get_value(self, name, out=None, units=None, angle=None, at=None, method=None)\n",
+ " | \n",
+ " | get_value_ptr(self, name)\n",
+ " | \n",
+ " | get_var_grid(self, name)\n",
+ " | \n",
+ " | get_var_grid_loc(self, name)\n",
+ " | \n",
+ " | get_var_intent(self, name)\n",
+ " | \n",
+ " | get_var_itemsize(self, name)\n",
+ " | \n",
+ " | get_var_location(self, name)\n",
+ " | \n",
+ " | get_var_nbytes(self, name)\n",
+ " | \n",
+ " | get_var_type(self, name)\n",
+ " | \n",
+ " | get_var_units(self, name)\n",
+ " | \n",
+ " | initialize(self, fname=None, dir='.')\n",
+ " | Initialize the model.\n",
+ " | \n",
+ " | Parameters\n",
+ " | ----------\n",
+ " | fname : str\n",
+ " | Name of initialization file.\n",
+ " | dir : str\n",
+ " | Path to folder in which to run initialization.\n",
+ " | \n",
+ " | quick_plot(self, name, **kwds)\n",
+ " | \n",
+ " | time_from(self, time, units)\n",
+ " | \n",
+ " | time_in(self, time, units)\n",
+ " | \n",
+ " | update(self)\n",
+ " | \n",
+ " | ----------------------------------------------------------------------\n",
+ " | Data descriptors inherited from _BmiCap:\n",
+ " | \n",
+ " | bmi\n",
+ " | \n",
+ " | grid\n",
+ " | \n",
+ " | initdir\n",
+ " | \n",
+ " | input_var_names\n",
+ " | \n",
+ " | name\n",
+ " | \n",
+ " | output_var_names\n",
+ " | \n",
+ " | time_units\n",
+ " | \n",
+ " | var\n",
+ " | \n",
+ " | ----------------------------------------------------------------------\n",
+ " | Data and other attributes inherited from _BmiCap:\n",
+ " | \n",
+ " | NUMBER_OF_ELEMENTS = {'edge': 'get_grid_number_of_edges', 'face': 'get...\n",
+ " | \n",
+ " | ----------------------------------------------------------------------\n",
+ " | Methods inherited from BmiTimeInterpolator:\n",
+ " | \n",
+ " | add_data(self)\n",
+ " | \n",
+ " | interpolate(self, name, at)\n",
+ " | \n",
+ " | reset(self, method='linear')\n",
+ " | \n",
+ " | update_until(self, then, method=None, units=None)\n",
+ " | \n",
+ " | ----------------------------------------------------------------------\n",
+ " | Methods inherited from pymt.framework.bmi_setup.SetupMixIn:\n",
+ " | \n",
+ " | setup(self, *args, **kwds)\n",
+ " | Set up a simulation.\n",
+ " | \n",
+ " | Parameters\n",
+ " | ----------\n",
+ " | path : str, optional\n",
+ " | Path to a folder to set up the simulation. If not given,\n",
+ " | use a temporary folder.\n",
+ " | \n",
+ " | Returns\n",
+ " | -------\n",
+ " | str\n",
+ " | Path to the folder that contains the set up simulation.\n",
+ " | \n",
+ " | ----------------------------------------------------------------------\n",
+ " | Data descriptors inherited from pymt.framework.bmi_setup.SetupMixIn:\n",
+ " | \n",
+ " | author\n",
+ " | \n",
+ " | cite_as\n",
+ " | \n",
+ " | contact\n",
+ " | \n",
+ " | datadir\n",
+ " | \n",
+ " | defaults\n",
+ " | \n",
+ " | doi\n",
+ " | \n",
+ " | email\n",
+ " | \n",
+ " | license\n",
+ " | \n",
+ " | parameters\n",
+ " | \n",
+ " | summary\n",
+ " | \n",
+ " | url\n",
+ " | \n",
+ " | version\n",
+ "\n"
+ ]
+ }
+ ],
+ "source": [
+ "help(model)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 4,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "rm -rf _model # Clean up for the next step"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We can change input file paramters through `setup` keywords. The `help` description above gives a brief description of each of these. For this example we'll change the grid spacing, the size of the domain, and the duration of the simulation."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 5,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, initdir = model.setup('_model',\n",
+ " grid_node_spacing=750.,\n",
+ " grid_x_size=20000.,\n",
+ " grid_y_size=40000.,\n",
+ " run_duration=1e6)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The setup folder now only contains the child input file."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 6,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "child.in\n"
+ ]
+ }
+ ],
+ "source": [
+ "ls _model"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Again, initialize and run the model for 10 time steps."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 7,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stderr",
+ "output_type": "stream",
+ "text": [
+ "/Users/huttone/anaconda/envs/excom_demo/lib/python3.6/site-packages/pymt/utils/decorators.py:60: UserWarning: Call to deprecated function get_grid_size.\n",
+ " name=func.__name__\n",
+ "/Users/huttone/anaconda/envs/excom_demo/lib/python3.6/site-packages/pymt/utils/decorators.py:60: UserWarning: Call to deprecated function get_grid_size.\n",
+ " name=func.__name__\n"
+ ]
+ }
+ ],
+ "source": [
+ "model.initialize(config_file, initdir)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 8,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "10.0\n",
+ "20.0\n",
+ "30.0\n",
+ "40.0\n",
+ "50.0\n",
+ "60.0\n",
+ "70.0\n",
+ "80.0\n",
+ "90.0\n",
+ "100.0\n"
+ ]
+ }
+ ],
+ "source": [
+ "for t in range(10):\n",
+ " model.update()\n",
+ " print(model.time)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "This time around it's now quite as clear what the units of time are. We can check in the same way as before."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 9,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "'year'"
+ ]
+ },
+ "execution_count": 9,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "model.time_units"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Update until some time in the future. Notice that, in this case, we update to a partial time step. Child is fine with this however some other models may not be. For models that can not update to times that are not full time steps, PyMT will advance to the next time step and interpolate values to the requested time."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 10,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "201.5\n"
+ ]
+ }
+ ],
+ "source": [
+ "model.update_until(201.5, units='year')\n",
+ "print(model.time)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Child offers different output variables but we get them in the same way as before."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 11,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "('land_surface__elevation',\n",
+ " 'sea_bottom_surface__elevation',\n",
+ " 'land_surface__elevation_increment',\n",
+ " 'sediment__erosion_rate',\n",
+ " 'channel_water__discharge',\n",
+ " 'channel_water_sediment~bedload__mass_flow_rate')"
+ ]
+ },
+ "execution_count": 11,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "model.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 12,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "array([ 0. , 0. , 0. , ..., -0.14213881,\n",
+ " -0.34210564, 0.00178402])"
+ ]
+ },
+ "execution_count": 12,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "model.get_value('land_surface__elevation')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We can query each input and output variable. PyMT attaches a dictionary to each component called `var` that provides information about each variable. For instance we can see that `\"land_surface__elevation\"` has units of meters, is an input and output variable, and is defined on the nodes of grid with id 0."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 13,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "\n",
+ "double land_surface__elevation(node)\n",
+ "Attributes:\n",
+ " units: m\n",
+ " grid: 0\n",
+ " intent: inout\n",
+ " location: node"
+ ]
+ },
+ "execution_count": 13,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "model.var['land_surface__elevation']"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "If we plot this variable, we can visually see the unsructured triangular grid that Child has decomposed its grid into."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 14,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAP0AAAEKCAYAAADZ1VPpAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4wLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvqOYd8AAAIABJREFUeJzsnXlcVOfZ979nNmAA2YZVVJAdxQUEAXHDFdnEXdHEqFnbpk2btknaPu3TNG3zaZumaZtNRdxwQ1BkETcQF0REQUEREZBFBNkEhGGYmfP+MRMe375pTdLYvHnk9/ncHzjXueee+5wz17mv+1oFURQZxjCG8fRA8k1PYBjDGMZ/FsNMP4xhPGUYZvphDOMpwzDTD2MYTxmGmX4Yw3jKMMz0wxjGU4Zhph/GML4kBEEYJQhCniAINwRBqBAE4ftGuq0gCMcFQbhl/GtjpAuCIHwgCEK1IAhXBUEI/CbnP8z0wxjGl4cW+JEoin5AKPAdQRD8gTeAk6IoegEnjccAUYCXsb0AfPSfn/L/YJjphzGMLwlRFJtFUbxs/L8HuAGMBOKB7cZu24HFxv/jgR2iARcAa0EQnP/D0x6C7Jv64m8KKpVKdHNz+6anMYwviZKSkjZRFO3/nTGcRkhEjfbx/Tr7xQpA/QjpU1EUP/28voIguAGTgSLAURTFZjC8GARBcDB2Gwk0PPKxRiOt+ctdwdeDp47p3dzcuHTp0jc9jWF8SQiCcOffHUOjhTk+j//Jp5YOqkVRnPIF5mQBHAR+IIpityAI/7Tr59C+Mf/3YfF+GE8VJMLj2xeBIAhyDAy/WxTFNCO55TOx3fi31UhvBEY98nFX4O7XcT1fBcNMP4ynBoIAcqnw2Pb4cQQB2ArcEEXxvUdOZQDPGv9/Fjj8CP0ZoxY/FHjw2Tbgm8BTJ94P4+nGF13JH4NpwDrgmiAIpUbaW8Dvgf2CIGwE6oHlxnPZwCKgGugDnvtaZvEVMcz0w3hqIPD1ML0oimf5/H06wJzP6S8C3/n3v/nrwTDTD+PpgQCS4Q3tk9/TC4IgFQThiiAImcZjd0EQioxeS/sEQVAY6SbG42rjebdHxnjTSL8pCMKCR+gLjbRqQRDe+MfvHsYwHsVnK/3Xocj7NuM/8d77Pgbnhc/wLvBno9dSJ7DRSN8IdIqi6An82dgPo6fTKmAcsBD40PgikQJ/x+Dt5A+sNvYdxjD+KaSC8Nj2vx1PlOkFQXAFooEtxmMBiARSjV3+0WvpM2+mVGCOsX88sFcUxQFRFGsxKENCjK1aFMUaURQ1wF5j32EM43MhCCCTPr79b8eT3tO/D/wEsDQe2wFdoih+5hf1mWcSPOK1JIqiVhCEB8b+I4ELj4z56Gf+0ctp6udNQhCEFzD4PDN69Oj/57xOp6OoqIju7m5KSi4RHBwMQEnJZfSiyJQpBj+NlN27iIuLx9LSAoCMjCPExsUBcOP6de63tTFjxgwADqamMmvWTFQqFQB79uxl1erVADQ3N3Pr1q2hvvl5eZibKwkJCTF8T8oeFi9ejFJpBsCHH33M2rVrsbQ03MZtSUk8++yzSKUSioouEhAQgFJpxsOHDzl27BgJCQmGG9LYyK1b1cyaPRuAQ+np9Pf3sXr1agRB4OzZc0ybFo4gCHR3d3PmzFmioxcBcOVKKYODg4SEGO7Fli1b8fDwYPbsWUPnJ0+eBMDFi8UMDg4SPm0aAMdycxk1ahT+/n7Ga9/D8uXLkclk9PX1cebMWRYsmA9AQcEZHj7sJSoqynCtH37EoUOHPu8xfi14GsT3x+GJrfSCIMQAraIoljxK/pyu4mPOfVn6/0sUxU9FUZwiiuIUe/v/25PzwIEDfPTRh3h6ejBjxnQsLS0JDw8nIiICa2srxo8bR0REBGq1muc2bESpVBIREQGAlZUVERERREREIFcoGD169NCxs4sLnp6eREREUFtbR0hICD4+PkRERNDV1TU0rl6nY9GiRTi7uBAREYGTkxMJCYuRyWREREQglcqImDaNutraoXlFRS3k5s1KwsPDCQ6egq+vD+Hh4VRX38bHx3doDr29D/Hw8Bg6dnR0ZMmSJVRV3SIsLIz58+ehUtkTERFBY2MjY8e6ExoaSkREBCNGjGDcOH8iIiLo6enlxz/+MSBiampKREQEdnZ2REREYGtry/jx43B1dR36noAJAdjbq4iIiCA0NJSpU0ORSCRERETQ0NCIm9uYob5ubmNQqweGrm3EiBGf9wi/FggYFHmPa//b8SQvcRoQJwhCHQbROxLDym8tCMJnEsajnklDXkvG81ZAB//cm+lr8XKytbVFpVJRWFgIwOzZkVy+fJmmpiY8PDzQiyJqtZq2tjaCg4Npa2sD4HZNDVOCg7l79y5paWnMn29YuR4+fEhzczMhwcG0trZSVVXF6DFjWLV6NaWlpeTk5DBnzhxEDCtxV1cXgUFB9PX1o9FoOH36NHPnzqWt7T4AlZWV2NnZsmLFcvbv3w+AhYUlK1asYMuWrXh6enLvXgtJSdtITFyDk5MTra2tXLx4kalTQ+jr60MURdLT05k3fz7W1tYsX76MTz75BH9/f27frqawsJDQ0FDi4+MpLLzAnTt38PLyRKfT09XVRX9/H+PG+ePt7U1LSwtXrlxBp9MZJaPLzJ8/H7VajVar5VB6OjOmz6CzsxOAtLR0YmNjqK9vQKvVGu+3PS0trRw7doywsDCCgoI4efIkoihSW1v7ZR/hF4cwvKeHJ8j0oii+KYqiqyiKbhgUcadEUUwE8oBlxm7/6LX0mTfTMmN/0UhfZdTuu2MIT7wIFANeRmuAwvgdGV9lrnFxcbi7u7N58xYcHR2or68nJyeHadOmIer17Ni+naVLlwLQ29tLR0cHI11GEhkZyZUrV9Dr9bi4uJCQkEBRURFZWVnMmDkTjUZDQcEZZsyYgUKhoL29nZ7ubsaOHYtWqyU7K2toe7BkyRJ27txJQEAAgiDQ09ODTqfD1tYGnU6HSqXCz9eH/Px8RFGPtbU1y5YtJTMzk8OHDzNnTiTW1tbMmjWTGzcquXGjkoCAAAICxlNTU8OAWo2npyd9ff2MGDGCF154gS1bttLW1s6tW9VMmDABBwcHmpubOX78BGFhYej1Ovbu3cfixYuHrj0qKor6+nrq6mrZtWsXa9YYtiwLFy6gpKSEfnU/Tk6O+Pn5cfPmTTSaAWxsbDAxMeHjjz9h3ry5REcv4vLly7S23sfd3R1LSwtqa2spLy9Hq/0CETFfEcPaewO+CWHmp8APBUGoxrBn32qkbwXsjPQfYoxFFkWxAtgPXAeOAt8RRVFn1At8F8jFYB3Yb+z7leDp6cnGjRvYs2cPN25U4uDggCAIlJSUMC0iArlcDoBEKiVlz15mzpqFRCLhyJEjxMTEAGBubk5HeztWVlZIJBJOnTrFzFmzKCoqIic7m6ysLCQSCTk5ORw8eBAHBweOHj1KVlYWZ86c4XxhIVqtlsHBQSIjI/nDH/5IaGgYOp0erVaLpeUILhYVkZeXT3LydnJzc1EqlajV/Vy9epXi4kuYm5tz8uQJZs2aBUBwcDDbk5OZPmMGSqUSjWbAcB0SCWPHjuXgwYNotVpyco5SWlpKc/NdnJwcEQSB8+fPs3DhAiRGmXf69OlcuVJKf7+ay5cvI4qQk3OUq1ev4uTkxLHcXEKM+pCwsDCuXr2KtbXhpeXo6EhdXR1nzpylvr6eCxcuDOkLtFodS5YsYevWJDQazVd9hI+FwLAiD/5DzjmiKOYD+cb/azBo3v+xj5r/cVv8x3PvAO98Dj0bg4vjV8alS5coLDyPn58/omhYsfPy8lEoFOzfv5879fXU1tZyp64OiUSCUqnkQmEhB1NTuVFZiVwmI2nrVqytrbGwsOD48eN4+/iwb+9eGhoaKTx/ngUL5jNp4gSulJYycdIkHBwcOFNQQPi0aTg4GKIvb926RcklV+zs7NixYyfm5uZUV1eTl5dHXl4ecrkcX19fEhIS2LlzJ+Hh4Xh7e3HtWjmBgUH4+/tx584d/uu/fklraysXLlygqKgIiUSgubmZy5cvY25uTn5+Pi0trYwYMYLAoCBiYmMZM2YMc+ZEcvt2DZdKLtPb20tDQyN36hvIyDjCiBEjkEgk9PT20NjQyOs//jFanQ6ZVEJMTDTXr19n27Zt1NXVUVpaSkVFBVKpjH379jFv3nySk5OJiYkhNHQqCxbM5/Tp01Tfvs2ZM2e5ebOKsrIyuro6kctl9Pf3/zuP81/jKVnJH4enQG3xrzFlyhTs7R2YNGkiixYtwt/fn02bNuLq6opcriAyMpIxY8awKDqahVFRdHV1MXXqVOIXL8bLy4s/v/8+dnZ2rElMJC4+HldXV0KnhhAfH8emTRvp7u5GqVSSkpLCj370I8rKysjPz+ft3/yGzCNHhuZRcPo0//XLX3Ljxg02btwAgLe3NzEx0SxbvhyNRsPESZMouniRN958k5KSEmpqarh0qRgvL08qKys5ceIEa9cmYmtry7z581m+YgUIEr736qs8fPgQXx8f9HqRFStXEhMbi0qlYvTo0TQ2NQJw4uRJ/vjHPxISMhWZXEZSUhIKExOiY2JY98wzyKQy3MeOxdraGktLS0zNlDQ3N+Pv74+Pjw/z5s1j/PgA4uPjCQ2diqOjEwBBQUE4OjoOreIVFddJSkpCJpOxKDqanp4e5s2bR3d3zxN91gLDe3oYZnoA1q1by6FDh+jt7eXEiRPMnj0bExMFbe1trFy5krLSUu7du8flkhICAgKQymR88sknQ2YoM6WSxsZGdu3cycuvvEJ19W1Onz7NuHHjeOWVl9m2bRu9D/vQarV0dnbS2tqKVColYvp0CgoKaG5uxsPTE4lEQltbO2fPnmWshwcvv/IKv/zlr5g+fTpu7u5cv34dAKVSyarVqyksLKSlpZWkpG20t3ewceNGbtyo5Ec/+iGn8/PRarXodTomTJjAhAkT+Oijj3jhxRdJSUkBIO3gQcLCwhjlOor33nuPmJgYHBwcaGxsxN19LAqFghdffJHU1FSSk5OJWrSIRYsWcTA1FRcXF+Lj48nLywOgtraOVatXc/XqVQAOHEhl8uRJvPDii/T1q9m+fQddXV1s2ZrEmsREFAoF/uPG8dvf/paf/+IXZGZm0t/fx+Dg4BN91sPa+2Hfe5qbm/nkk0+QSqV873uvMnnyZPbvP0BNTQ3t7e0cTE1FFEX+/N57DAwMMDsy0mD/rqriyJEjqPv76Ver+eCDD1i0aBEqlQpvHx9OHD/OrFmz2LZtG66urtTU1HIkI4PLJZews1ORkpJCX18fvb29lF65wvrnnqP44kX6+vvZs2cvsyMjh+Zw9OhRHB0cOJ2fj5OzM0dzcrjb3IyFuTklJSXExcXS1dVJc3Mz3d3dODg40N7ezo7t21mxciVFRUU0NTZSW1fH3j17cHR05OTJk2h1Onp7e7l8+TL19fWcOnUKpZkZ+fn5zJs3j/379iGTy5HJZJRcuoSZmRkW5uZkZWXx4UeGNG82Nrbs2LFzyEbf19fHp59uJiYmmr/85QO2bNmCQi5HKpVy4sRJAgICOJqTg06nQ6vV0tjQQHZ2NqZmSlpb76PT6Z7o8x4W74eZfsguXlFxHbVazbx58/Dz8yU5ORlnZ2eWLTNo7f/0p/ewd3AgPj6epK1bmfvyy6jVasKnTUOr1fL8889z7do1GhoaMDM1paKigszMTNauXYuJiQmHDx8mOjoauVzOwMAAa9asQafTcSQjg9zcXLKzspg+YwYW5ubMmjWLpUuXcvjwYd586y0qKioYN348mZmZ3L17l5dfeYWFUVGkHjhAWFgoiYmJALz//vvcvdtMyp49dHd3U1NTA8CChQupq6vj3XffpfjiRQImTOBgaioNDQ1YWlpip1KhUqlYu3Ytvb293KquZsLEiQQEBKDX69n86aeET5vGypUr0ev15Bw9SvK2bYwdO5YBjYa8vDwUCgUXi4q4c+cObW1tWFpaMmrUKGxsbIYsH3q9nod9faxctQqJRMLuXbsICgpizZo1tLS0kHYw9clq74f39MCweI8gCDx8+JArVy7z6quvUl5+jZMnTzJt2jQcHR1oamoiOXm7gbFEEb1ej62tLUFBgTQ2NtLU1ETS1q28//77+Pj4sG7dOnQ6LbGxMVRUXKe42JCaS61Ws2PHThZFRyORSOjs7EQqldLe3s7cefOIjIxk5MiRWFhY0Nvbi16vp7+/H29v76E5+vj6Mnr0aBwdHdFqtYiiSGRkJDdvVrFjx05mzY4kICCA1avXYG5uTmBgIBs2buTu3bu4ubkxcuRI2tvbGTt2LLZ2dgQa99rBwcFYW1vT2trKgf37+cEPfsCFwkL6Hj5k+/btrFy5YogZU3bv5u2330YmkzF/wQI8xo5lTWIiMrmcefPnM2FCABMmTMDS0pLFCQmoVCpKSw0h50pzc5YtW8aRI0fQaDSYKZVIZYZ1JyMjA4XC5Amb7B6fQOOLJNH4tuOpZ3qAHTt2sGbNGgC6u7tpamrCy8uL2bNn85e/fEB4eBhOTo6MGDGC5ORkpk0LB2Dy5Em88cZPcXZxwcrKiu7ubrKzshg3bhxr167F2dkJOztbtm5NoqamFnt7e8zMzFixciVnzpzhwP79RMfEsHTpUvJPn+bChQtMmDABJ2dn/vrBByxcuBCAxYsXczQnBysrK5avWMGJ48fZvXsXixYZFIu//e1vmb9gAZMnT0YzOEhJSQlTpkxhwsSJnD93jtvV1UydavBQDg0L48CBA/j7+zNmzBiKLlxg3LhxxMbFUVxcjIWFBSYmJjy3YQO/+MUvmDRxAjY2Nui0g9y7dw97BwdsbGxYvmIFWVlZXLx4kblz56LVannrzTcxNTXF3FzJ8RMnMDMzY+bMmZSWlnL//n3Mzc1RqVRIJRL+/N57REVFYWpqyrmzZwkKCqK/v49/kWfua8GwnX5YvCcnJwdHR0dSU1PRanXcuFGJTqdjYGAAuVxBQ0MDeXn5XLlSiqmpCefPncXURIGFhQVjxoxh4oQJNDc3k7xtG2fPnWOUqytmZqZcuHABd3d3jhzJxN7enrKyMpRKJelpaZiamXG5pITAwECcnAwabq1WS+mVK4SGhVFfX88NozZeEAQkgkBDQwONjY0o5HJOnz6NKIpYmJuj1Wrx8vLimNFmb2piwvFjx/Dy9ubBgwfk5+URFxdHWpohjZtGo+H4sWPMjozkYW8vFRUVpKSkYGtrS9GFC0yaPJlD6el0dXXR3f2AEydOcv/+ffR6PYfS03nxpZcAg6POxaIi+vv7SU1NpfvBAyZMmEBUVBRlZWU0NDRy9uxZuru7sbCw4PuvvkpgYCAdHR2YmppSXV1N5pEjWJibc+z4cdzc3Ojr63+ie/ph8d6Ap57p58yZw82blaxYsQJBENDpdGg0GjZu3EBtbS0+Pj7U1dWxcuUKrl0rx9vb4D8/ZswY9Ho99fX1PHzYx6rVqxnQaLAaMYLp06fz4MEDbt26xcOHD3n99R9hbW1Nf38/CUuWoNPpyM/L43xhIS2trcjlcmpu36arq4uZM2cyZswYrK2s8PT0ZNKkSajVavrVaiwsLIiNi6O6+hZeXt7ExsawbVsyzzyzjoaGRiKmT+fjjz7iTn09L7z4Ira2tnR1duLm7s6UKVNobm4mKzOT2bNnExMTQ3l5OZ1dXaxatQq1Ws2+vXuZOHECCcuWodFocHJy5OHDPqZOnUp6+iHMzc3JzspEJpdjr1JhY2NNcHAwcfHx7Ni+HblcxsDAAJculRAUFISXlydjxoxBo9FQZ7yXsXFxHEpPJzIykrDwcM6dPcvD3l7WrFlDetpBDE6YTw6SYa4fFu8VCgXx8fGkph5ErVZjb2/PjBnTuXjxIidPniIkxLDfra6upri4mJ/+9Cfk5OSgVqs5efIkgYGB6PU6MjMzmTNnDr5+vpw/fx6VSsW1a9fw8fEhKSmJyMjZmJqa0NrSQvK2bfzsZ2/h6enBkiVLUPf3s2HDc2zatImW1laa795lxcqVlF+7RmtLC9u3b2fZsmX09fWxJyWFNWvWcO9eM3q9Hhsba9zc3Lh16xZgMOf97K03OXniBB0dHYRMncrlkhLKyso4c+YMGzdtYk1iIqdOnaKyspK1a9dy4cIFtFotq1avpr29g4cP+zh48CDh4eF0dXVibm7OtGnhREcvQqFQsHDBAsrLy1m7dh29vb2UX7tGQMB4YmNjKSq6iI2NNXPnzhlKNb5ly1ZeffV7yOVyqm7epPdhL2vWGEyOPT3dJCauobi4mIEBDXq9/sk9bMHA9I9rX2goQUgSBKFVEITyR2i/EgShSRCEUmNb9Mi5z00E803gqWd6AEdHRzw8xvLLX/6KmTNn4O3tzY0bNzA3VyKVSomKWsjf//53nJ2dEUWRTZs2sWfPHhobm3B1dcXf35/srCy6u7txdx/Lg+4eMjIymDhxIuPHj6eo6CL79++nr6+Pt956i/7+fs6ePcsIyxH84Pvfx87OjqtXr9HU1EjeqVOUlpayZctmNBoNP/nJT+jv6+N0fj55eXmIooiD0Yrwl7/8hXHjxgEQEhLCkYwMPD09jGGt/vz617+m+tYtdDodf/vrX7G1saGvrw+JREJ7eztyuRxXV1fq6+tJPXCAyMhINmzcyJ49e9DpdFhZWbFs2TLee++9oei7trY2vvvd76FSqXBwsEejGaCo6AKBgYGoVCrOnTuHr68hpLajo4Pdu3ezeHE8JiYmzJ49iz1792JqYgpAb083JiamBAYG0na/ld7eJ+2cIyCTSR/bviCSMSR1+Uf8WRTFScaWDf88EczXcElfCU+9eH///n2ys3Po7++nubmZ1INpmJmacvduM52dnchkMnp6ehkY0NDQUM/WrVsxMTGlvb2du3ebOXw4A0dHR5ydnbGzteH4sWN0d3dTUFDA9OmGkFxPTw9WrVqFVCqlsvImkyZNZPr06fT29nLlymUWLJiPlZUVAO3tHUgkwpAZ7kH3A0LDwggNDeXq1at0dXWSlJSEiYkply5dwtHRiZKSEvR6kczMTOLi4khPP4RcoeBeczNRb7zBmTNnmB0ZibOLC4cPHUIvipiZmXG9ooK6ujr6Hj5EIpGQm5vLvXv3aG1poaenmwMHDqDX66mouM748eNpbGwkNDSUc+fOI4qQlJTE/fv36ezsIjU1FVGE69ev4+rqyrVr1+js7KS0tAytVodWO4hcrkA7qKGy8gbbtm1DEATKykrZv98EQZAwMKBBFJ/cSm/Y03894r0oigWPpnR7DIYSwQC1xviSEKDwa5nMl8RTv9Lb29uzaFEUra2tJG3bhkwmY9ny5UwJDsbFxYUVK1aQmLiGmJhonJ1d2LRpE+Hh4ahUKsaN8yc+Po6qqpt4eXlibm7O8uXLGDnSBT8/XzZt2oS1tQ2vv/46qampKJVKQkNDUasHKC6+RF5eHt///g/Izc0FDAzj7u6GRmPwSrt2rZzZsyOpuX2b2tpaQ+CPwoSNGzeydOkS4uMNiTZWrVrFmjWrmTVrFkqlksUJCURHRxMTE8PNmzfp7+9nzZo1nD1zhlWrV7N27VpcXFwwMTGho6ODyps3uVlVRUhwMBs3bkSlUjFp0mSWL1+OXC7n1Ve/h1KpZPbs2fT09LJu3VoUCjkbN27Ezk6Fj48Py5YtY+nSJSQkJNDT08OKFcuxsbFl9uzZzJo1k40bN7Jq1UqmTJnCxIkTee655xAECX5+/qxYsYLOzg4kEsl/YE8veWz7N/FdY2XapM+q1vLPy1p9I3jqmR5g585dLFmyBKlUiqmpKc3NzTQ1NrIwKorCwkL27t1r/MF3U1hYSEVFOevXr8fb25vq6mqkUhkrV67kwoUi9Ho9HR0dODo6cuqUQScglUqZPHkyWVlZ2NraMG/eXO7du0dJSQnOzk7odDra29u5cOGCIQGHsxN3797lYnExkyZNwsLCgkPp6UydOpWoqCgKCgrYu3cv8fFxSCQS7ty5Q3V1NYFBQcgVCm5WVnL58mWmTJlCe0fHUMadlatWcfjwYdra2ujq7MTT05OmpibWrVvH3DlzaO/oIPfoUebMiaSv7yEajQadTs+UKVO4ffs2AwMDFBcXExoaSldXFzk5hr6BgZMpLS0lNfUgM2fOYNmypaSlpWNra8uyZUvJyspmcHCQlJQ9zJ8/n76+fvr6+rCxMfjwHzx4kBkzZjAwoOYJ8/wX3dOrBEG49Eh74QsO/xHgAUzCUKfuT0b6cFmr/59QVlaGo5MTDo6OACQkJHDy5EmsbWzw9fXl2rVyZDIZJ06cIDf3GEeOZA6l05o+fTqlpaWMGGGJRCLhwYMudu7cRUxMDN7e3pSXl+Pp6UltbS33799n167dVFVVsW/fPjo7O6muvs3u3SmYmJjwgx+8NpSIY/78+RQWFuLi7ExDQwP379+nre0+RzIyuF1dTWlpGZaWlpiYmBAXF0dubi75+flMnjyZhQsXkn/6NMXFxfj4+nLz5k3c3N3R6/Xo9Xra7t/njZ/+FFNTE/R6g0dgWWkpd+7c4dNPPqG09Apubm74+Pjwxz/+ibi4WACeeeYZ0tMPDUUFdnV10dXVydixY5k0aRJlZWUMDg5ibm5ORcV18vPzqKqqIjMzi6lTQ9i9OwVzc6UxFFjN7t27WbhwIfb2Ks4XFlJbW0dfX/8TVeQJwuMZ3sj0bZ9lWjK2zy1e+Y8QRbHFGPatBzbzP9Gk/1+VtXrq9/RSqYSc7Gz0ej06rRbN4CAXCgsZ6eoKokhzczMDAwO8+ur3aGlpQS5XUFpaytGjRw0r8KHDzJ07l+PHT3DlSimCIAxp++/cucPWpG34+voyPmAC8+fPw8fHh7CwMD755FNWr15NQMB4bG1tycvLJ/90AXqdFnMLC3bvTiE6JhpLSws2btzAzp27iI+Po6enhyNHMqivv4NCoUCj0aDRDFJ58yY5OTnodFqsRozg3LlzpKSkUFFejlwmo7i4GJWdHb29vYSEBBMdHU1y8nZiY2Pw8PBg3LhxJCdv5/KVK2RlZSOVSmlobCAtLW1I5M3KMrzwdu7cRUlJCWZmSszNLdBqtVRWVqJWD6DTaVm0aBGt9w2Zf6I19GjVAAAgAElEQVRjYqgoL+f8+fOMHDmSgwfTuHLlMiCwc9duYmNjGdTqcHBwwNzc/MmG1sLXIb7/UwiC4PxIuaoE4DPNfgaQIgjCe4AL/5MI5hvBU8/048cHEBMTw5HMLNavXz/k8iqTSlmckEBPTzc6nY4RI0ZgYmLKmDGjUalUQ4kc7927x6hRrsydO5fq6mpsbKyJj48jaVsytnZ2JCQkYGVlxScff8wLLzxPRsYRjh49OmQlyM7OpqWllbfeeosqY7LMK1euMHHiRO41N+Ngb8/AwAAWFha0tbWRk3OU1157jaKiiwQFBaFQKEhLS2f+vHmMdHFm8uTJfPLJp4wbN46VK1cil8lQKBQsTkigr6+PnOwsNBoN3d3dQ5LCrl27sbOzA+DPf/4zH/7976xYuZJb1dV4eXkxZcoUNm/ewu9//3vy8/NJTExEFPV4enqi1+uJiIigs7MTpVLJ9OkRHDp0mMS1a0nZvRuNRsO1a9d44803KTh9mtmRkXR0dqLT6Zg9ezZmZmaIokhFeTk6ne4Jr/Qgk309TC8Iwh5gFoatQCPwS2CWIAiTMIjudcCLYEgEIwjCZ4lgtBgTwXwtE/kKeJKJMU0FQbgoCEKZIAgVgiD8t5GeLAhC7SO2zElGuiAIwgdGW+ZVQRACHxnrWWNxjFuCIDz7CD1IEIRrxs98IHxFH05nZ2eeWbeWTz75mA8//JAlS5agGRzk+LFjBAcHs27dOrKysjExUTBjxgzOnDmDXq8nNfUgy5Yto7a2jpycHObPn4dcLuf8+UL8/Px45plnyMjIIPXAAWJjY5BIJDQ2NjA4qMXX19e4JXiArZ0dTs7ONDU10dbWRlVVFf/1y19ib2/PvHnz2LNnDxrNAG+//RucnZ3p7u7G1dWV3//+9/z3f/83Hh5j8fHxprLyJsnJ25kxYzrLly8j4/BhXIz+9qIosnvXTuLi4lixYgWHDx9m5EiDLmnt2kTS09NRKBTo9XqiY2J48403UCqVZBzJ5J133iEhYTEjR45EpVJRU1ODUqkkPDycnp5eNm/egr+/HyqVHb/5zTs0NjVx/fp1ps+Ywe9+9zvGBwTg7u7OumeeIS0tDVNTU1588UVKS0s5ePAgxcXFBuemgYF/6zf3OAgIX5siTxTF1aIoOouiKDemhdsqiuI6URQDRFGcIIpi3KNFKkVRfEcURQ9RFH1EUcx5Yhf5BfAkV/oBIFIUxV7BUNb3rCAIn13sj0VRTP2H/lEYxB4vDKmsPwKmCoJgi+EtOgXDG7REEIQMURQ7jX1ewJAiOxuDDfRL3dBr165x48Z1rK1t8Bg7ljNnzpB28CCmpqakpqayfv2ztLW1o9frh8xJy5cvJy0tDVGE27dv09vbS3Z2NjqdDlNTUzZv3kxkZCS3q6upMZ6XSATjC+E8ILBz5y4UCjlZWdlMmzaNzZs3U1NTw92mJl774Q8BiImNo6CggMDAIEpKSujp6UGhkKPXi7i5jWHUqFGEhYXh6elJR0cHTk6OpKSkIJfLMDc358CBA/zpvfdwcnJiz549TJgwAYCysqtkZ2ezZMkSDhxIRa83rLDFly7Rr1bj6uqKl5cnU4ICGWFpQWHhBbKzcxgxYgT+/n4UFBSgUtlz+HAGDx48oKDgNOPG+TNu3Diqq6vx9PTCxsaGS8XFVN+6Rc1tw33QaDQ87O2l8sYNZDIZCrmcM2fO4DpyJOnp6UYX3Ceo3xKGPfLgCTK9Mallr/FQbmz/6onGAzuMn7sgCIK1YKjxPQs4LopiB4AgCMeBhYIg5AMjRFEsNNJ3YCic8aWY3s/Pj4qKcgIDJ+Pm5kZv70NWrFhOcvJ2Jk+ehLe3N8XFl7h16xZNTU1YWo5AFPUcOXKE0NAwpgQH09jYiEQisGDBAuRyObm5x1ixYjkmJiZs2bIFEIbCSzWaQSQSgSVLltDb22sMtdWwctUqsrOzyTxyhK1btyKXy5FJpRQWnmf+/AVs2PAc7e1tqFQq/P39KSwsJC4ujpKSy8yYMQMXFxeSk7cTGBhEYmIiZ8+dw8XFhdvV1XR2dpJ71JCF90blTWbOnMnChVGo1WrWrVsHwKefbmbmzJnExsby0Ycf8vrrr5Off5rBwUHCwsJYsGA+tra2nD9fSGFhIc7OLrz00os4OTlRWVmJm5sb9++3ERIylds1Nbi7u3P82DE2b9lCWtpBEo0BTbt27cbe3p5Vq1axf98+fv7zn3PyxAmWLl3Kf/3i5/T19X2Zx/elIRGeet31E69wIxUMpXxbMTBukfHUO0YR/s+CIJgYaf/Mlvmv6I2fQ/+8ebzwmfnlvlHB9BlkMhkvvfQSxcWXOHfuPBrNAEeOZBIaGoq9vQOiKBIVtRBnZyc2bdqIk5MjXV0P+N3vfocoijg5OaHXizz//PNkZxvS9c2aNZOzZ8+h1+uxtrYhImIaRUVF9Pb2YmtrS2dnJ4ODg+zenUJsbCwODvZU3bzJgwcPmDFjBjExMaxfvx6At956i7a2NlpbWwkImDAUplpVdQs/Pz/s7GxpaGhArVZjZWWFmZkZFy5coL9fzU9++lNkMikajYbvfu9V7OxUrF+/HoVCgbe3F5MmTebkyVOGzD0eHoh6PZ9++gnPPLMOuVxOX18fKpWKlStXcPLkKQBGjXIlNjbOGEFoR0FBAZs2baS0tJSSy5cJDAqiu7ub27dv4+/vj6mpKaNHj6Gs7CodHR3Y2Fij0+s5evQovn5+uLu7ExkZyen8fKNzzpNb6Q3i/dfjhvttxhNleqP5YhIGE0WIIAjjgTcBXyAYsMWQHRe+oWIXn2Hp0iWGTDkH05BKJfj6+rB4cTxFRRfZvTuFuLg4xo0bx8cff0JjYyPl5eVMnjyJ377zDp6eHiiVSrq6urh48SI+Pj7cu3ePw4cPM336dKPp7xqHD2cQEhLM8uXLSUlJwcvLE7lczpw5czh9+jQPHz5k9Zo1HDt2jAP79xEeHoarqyvPPbee5OTt9PX1YWpqyvHjx4eqy0RFRXH+/Hl27TKE2mq1g+zcuQt3d3ccHByoqrqFiYkJwcHBmCmVlJWVkXv0KCEhIQQEjEet7ueDD/6Kvb2K9PR0/Hx9hwpOnDp1ihkzZhiSYvb0GBSBOTlERy9i3bp1ZGRkUF19m7Fjx1JScplgYyWgadOmsW/vXqYZi4JERERw8WIRKSmGLcaFwvOcP3eO1pYWThw/zu2aGrYlJz/RWHrga/W9/zbjP5UNt8soji8URfGPRvKAIAjbgNeNx/+qqMWsf6DnG+mun9P/S6GtrY2srKxHVjY7ioou0tnZiZWVNdeuXUOn03Ly5Enq6xuYMXMmY93dCAsLo6GhgY6ODvLy8qmpqUGn07F582Y+/PBDrl41uKE6Ohrs2lFRUfz1r39FFEW0Wi1Hc3NZFBXF9u07DAklzEwpKrpIcvI2Sq+UIpVK6e9Xk59/GhDp7n7A9evX8fLyYt++/SxbtpTm5mYEQUJhYSGiaHAy6unpJWTqVFpbW4f89eMXLyYzMxOFXE5WVha9Pd0kJ2/H3MICc6USS0tLdHqRka6udHR0cPRoLmp1PwMDA5w8eQqZTIa9vT2bNj3Pc8+tp6KiAnNzc/r6+qivv0NaWho1NTWcLiig6e5d+h4+5Pr1CnKP5iCVStHrDclHrt+4jq+fH97ePowfP57IOYZS7r29vZSWlnLu7NknqswzaO+fghzXj8ETY3pBEOyBQSPDmwFzgXc/s2UaNe2L+b9tmd8VBGEvBkXeA2O/XOC3j7g0zgfeFEWxQxCEHkEQQoEi4Bngr192niqVirCwUMrLKww58GZHMjCgJjExEa1WS2rqAZ57bgOhYWHs2rmTtWvXcmD/PiQSCZmZWbz33p9IS0tn1apVaDQazp07x7ZtyTg6OpKdk4OTs4th9RDh/v02Fi+O5/79+4wZM4ampiYS164FDMEp+fmnWbN6NYiGuPfExDXIZDLUajXZ2dmYmSmN+eoNIrFCoQDgxo0b+Pn54e7uxv22dh50deHi7ExYWBgP+/oYNWoUU6dORa/XU1tbS21tLTNnzmL+ggUIgkBfXx8lJSX86le/IikpiY0bYigpKSE0dCre3l74+fkNxRNMmjSJ9vZ2mpubqaurQ6VS4eHhyauvfo/SsjImT55MTnY2H/z1bxxKT2fDhucQBIEtW7YSFBhEREQE7W1t1N25AxhSaP32nXcYHxCAtbU13d3dX/7H9oXxdKzkj8OTFO+dgTxBEK5iqEZzXBTFTGC3IAjXgGuACviNsX82UIOhKu1m4BUAowLvbeMYxcCvP1PqAS9jqIhbDdzmSyrxPkN1dTW3blURGxuLXKFg0uRASktLqay8yXe/+z0qb95k65YtQwza3dPDp59uZvXqVZiYGDzbHjx4wN69+3j33XeRyWT4+HizYcMG7t27x/z5C3jw4AGvfv/7XLlSysmTp5g1ayY+Pt4UXzT4aBzJyGDp0iXcu3cPGxsbEhPXcMSYInvXrl1ER0ejVvfT0dHBq6++Sn5+PgD79u1nxYrldHV1UlBwhtDQUBZGRXHm7FkGBwfx8fGhqqoKgIaGBsa4uZGQkICDoyNbtmyhvLwctVqNjY0NCoWC9evXs3//Aaqqqli/fj1nz55DFEVSU1N55ZWXqaqqGjI3RkbOYfToMVy+fJnJkyfz3Pr1hkSiGMqFzZk7l8OHD9Pb28vIkSNZvXoVJ06cwNTMjNDQUEouXeKtN98kPDycNWvWoNVqn2jmHEMtuyfue///PZ5kWauroihONtosx4ui+GsjPdJoyxwviuJaURR7jXRRFMXvGG2ZAaIoXnpkrCRRFD2Nbdsj9EvGcTxEUfyu+BW0QC0tLZSUlAxVepXLZEa30qtcvHgRqUxG/Z073Lp1i7179rB9ezKVNyq5ffs2+fn55OQY9scff/wxfn6+qFQqgoODOXDgAJMnTyY2NpZtSUno9HoCAgI4d/48Hh5jEQSB4OBgKisruX79Os7OTkRFRbFtWzKBgZOxsDBUxi0rK2P06NFIJBKysrKIjY3BwsKCpqa7VFZW4ujogLOzMxcuFOE6atQQ09ja2rJ9+3YmTpiAs7MztbW15B49yoIFC1i0aBGVN27w/PPPc/fuXVIPHqS9rY2UlBQOHTpE2dWr1NbWUV5ezsKFC8jPz8fCwhJ3d3dqampoamri7t27TJsWTktLC15eXuj1ejIyMujv76OstJQD+/dTVlZGbV0dP/3pG3h5eSKTyWior8fM1BSFQsG7776Lqakpbu7utLa2GlJ2f0vi6b/NeOo98np7e9HpdBw8mIa1tRV37twhNzeXqqoqenp6CAoKwtPTExBZv97gF7Rjxw4EQcKSJUsMaaQOHaay8ibOzs5UV1cjl8u5dq2cjIwMlEolLS0t3Gtpobenh8obNxhQq6mtrUUuV2BiYsI7v/kNy5YtIzs7h/r6eioqKrh69SpSqZQ//OGPzJ8/nx07dyKKkJt7DFEUaWlp4Q9/+APz5s0jMzMTmUxKyaVimhobUSqVmJmZkXfqFDqdDolEwtYtWwgNDWVbUhISqZTSK1dovX+fgPHjmRMZia+fH9OnTwcMSTzNlUq0Wi25ucc4ffo0ISEhHD9+nDt36nn77d/w0ksvcvv2bc6ePYNMJqOtrY3Q0Km0tLQwfvw4li41BDDl5+dTf6eehsYm6hsaqayspKmpCStra/z8/FixYgUSqZTDhw7R39//hFd64X+FyU4QBFcM8fnTMbj19mPYJmcBOeJj4pOfeqb38PAgLCyUvr4+tm7dipmZGbNnz6alpQWpRMKEiRO5fdugoS4rK8PBwQE3Nzc6O7u4d+8eCoWC7u4HREUtZN68edjY2HDlSilr165FqVQyZ+5ctiUl4eDoyNq1a7G1tUEQJCxdugSA5ORk3nnnN+Tl5fHcc89RXFyMu/tYfHx92bplCzGxsYSGhnLt2jV+/fbb5J06ReLatRQWFqIwMWHSpEn4+vrS1taGVCodsrtfunSJadOmERQURGBgIDqtFplMyvLly7CwsGBwUINKZY9KpSJyzhyKjBF+J06cYPr06RScPo2/vz8TJkwYquoTEhLMnTt3UCqV9PUZFH3W1tbMnj0LNzc3Y9TdHMzMzCgsLMTU1BSdTsef/vRH9u7dR2xcHN3d3QgYtlQ/eO01srOziY2NNW6TnuAqD/A1uuF+UzAqv0cCmcC7GMzhpoA3Bue0nwmC8IYoigX/bIynnunBEMd+6dIlXn75ZTKzskjeto1nnn2WE8ePU1paiqOTExMnTWL3rl2o1f1s2LABiURCSsoeenq6ef755xkcHKSgoGBIZF+1ejVbt2xBq9Vib2/P3Hnz2LJ5M+HhYVy+fAVRFDl06DDh4eG4u7sjk8n461//RmxsDPn5+ZwvLCRq0SJcXFw4YMy64+LigoOjI7du3aKyspLXXnuN9LQ01Go1EokUe3t7GhoaUCgU1NTU8sp3vsOunTu5fv26YR/vYM+HH35IS0srr/3wh6QdPEhnRwcbNm7EXKnk/PnztLa2Mm/ePOzt7SkoOENDQz2bnn+e0/n5lJVdZfz48YSGhrJ1axLR0YtYuDCK/PzTzJkjpbv7AWPHjgVg+/bteHl5k5CwGIlEgrm5OR99+CGv/fCH7N+/H5lUio2NDQKGfP0/+9nPeOutt56wnf7JBtz8h/AnURTLP4deDqQZKziP/lcDPPVMX1JSQnPzXRIT19LR0cGl4kssWboUtVpNcEgIv/jFL3jllVcoKCjgdk0NPd3dZGfnoFb3k5mZSXDwFFJSUli8eDGdnV3sTtnDS8aMsdMiInj717/mlVde4eyZM5w7Z1CKubu7s3XrVkaNGsXFixe5cKEIMzMz8vPzhjLFfhYQ0/fwIZ2dnXh4eAAwz/jysLW1BSBhyRIOpqZSXX2L1atXkZp6kAfd3SxfvpyCggKsrKzIyDiM1QhDKG5gYCCbN28hPS2NB93d1NXWkpycDMDJEyeYNHkyu3btYvSoURQWFjJ79mysrKzo7e2lpOQSGzZsQKvVMmvWTN5557fY2tri5e3N66//mMWLF5OZlY1MJuP69RsoleYkJSWh14vcu3ePvn7DPcs9epQZM6aTlZlJU1MTHcZKQk88xFz49u/Z/wnDP3peg0Gx/U/x1DN9UFAQAQHPUltbx/bt27lx4zp36oJQ9/eh0WgYUPcjIDLW3Y3b1a7IZDJiYqLp6+vDwnIEt6uriY+P58KFIjIyDhMWFk7qgQOAwezWfO8eJ0+dYubMmSQmrqG39yEuI0eyZ08KiYmJJCQkYG5uzrFjxwgODuGZZ9axf/9+/P396VcPEB4ezoEDBwwmO6USvV5vYNa6OkxMTZHJZEhlMpqa7nL0aK7B083Xl1OnTjF37lxSdu8mMXEtKpWKgIDxFBUVsWyZIbtPZeVNlEol8+fPx8XFhea7d5k7dy5eXl6GGP60NHbt2kVHZycnTpzA09OT5OTt6PR67O3tmRIcjK2NjaGAhyAgVyiIjo42XPuA2uBstNpQvz5pWzI21tYsXryYtvv3cXNzZ+7cOXR0dDBu/HjGjxuHg4Mj9fX1T/R5C9L/HXZ6QRBiMFi1xmDgYwGDPnzE4z77rZd1vg5YWFhQU3ObmTNnsX79ejo62gkPD6ey8iZ/+9vfaGlpYdSoUSgUCgYHNeh0OvbtP8CsWbNYk5hIZmYWc+fOITo6Gr2oJ2HJElasXElPTw/BwcEsXbqUrMxMoqKiMDc3x8bGhjVr1tDcfI+GhkZ6e3t58KAbN7cx1NfX425MWd3R3kZ1dTU9PT28/vqPkAgC0dHR2Fhb4+PjQ3R0NAsWLKDl3j0+/OgjRFFP5Jw5WFtbs2TJEvbv28eq1auZNXs2JSUlAJSXVxAdvYibN2+i1el46aWXyMgwBM5MnDSJ8vJylEold+/e5YUXX+Tnv/gF95qbCQkJwcPDg+CQEOLi4uhob2fdunV0d3eTnp7OnLlzkUgk3L17l8uXL+Pl5Y1crqClpZXU1IMsXLgQnU5H6oEDxMXFcffuXfr6+nBwcGDRokUUFBSg0z1ZjzxDEg3pY9u3BO8DzwJ2oiiOEEXR8oswPAwzPWDwZPPx8WHsWHdsbe0IDw/n7bffZtGiRYwYMYKWlhaamppwcxtDfHw8Z86cwdzcHBMTE6ytrRnr4cH58+dRKpU8v2kTydu2sXv3bhKWLBlieE9PT6RSKbGxMZw6eRKFQsG6dWtJT0/jjTfeJCFhMTKZnKNHc5lmLAYZHx/P2TNnkEok+Pn5IVfIuVxSYnhx2NrS3NzM3j17WLx4MQqFgcEEYPmKFbzy8stETJ8+tA3w8fWloqJiyMW2ra2dG9evs337dgRB4IXnn8fV1RVzpZKWe/e4VVWFv78/I0eOxMfXl7y8PAYHB0lPS+PHr7+OlZUV/f39DA4O8rC3FwcHBxISEsjNzeXy5cv4+vqwbNlSsrOzkEqlODs7o9Xp0Oq0ODo6oFLZ8dFHHzNj5kwAXEaOpK9f/cRz5AlS6WPbtwQNQPlXMVM/9eL9tWtXGRwcpK6ujr179+Lt7cPNmze5fv0GeXl52NursLNTsXPnTn7yk58gkUg4duw44eHhFJw+TWdnJ10PHlBWWoqFhTl6UaSurg7NoAYThQK5QkFaejoL5s8nJWUPUqmU6upqmpoaEQSBsLAwcnOPsW1bMv39/Tg6OnLu3Lkh0VuhkHPu3Hmsra0Z0Axw/PgJLMzNef6FF9i1axfBwcE4GqvkLE5I4G9/+xv79+1l+vQITp44TvHFi1haWmLv4MDJk6ewtLRk37591NTW4uvjw+pVK1EoFLS23OPc2bMoFAref/99nJ2d2bljByOsrPD39ydoShABRpu/TC4nLCyMoqIiNBoNVVVVpOzezeDgIA319XR1dRn1Hmpyc4+xYMECMg4fJjsri6iohUOJQKtv3yY9LQ1BEBAkEvqfcIQdggSJ3OTx/b4d+AmQLQjCaQxh7ACIovje4z741DN9QMAEwsJCaWpqIi0tnf/D3pnHRXWeff97hoFhR5BNcUFA3HBBUBERREWQVRBQo6hxyZ40Tfu8iW3SJE3TNn2eNk/SrEYBgxsogoAoiguKgOwiyiKbgLLIvjMMc94/ZqRpP0lj2tC3ad7r8zmfmbnPuc8clnPu677u6/r+fvrTn5KYmMhvfvMbGhrqcXFxITY2lvLycpKTU5DJZHR1d5OXl8eu3buZ5+CgXgIbYeqUKWjr6ODn70/9vTrCwkJpbGykra0NExMT/Pz9ARgcHEBPT4+1a9cSGRmFra0Nu3bt4r/+6//w4MEDli1bipubG4IgsP+LAwQFBTFnzhymW1sz0D+AREODnJwcbhYXoymV0tvTg1IUkcvllJbewmX3bry9vdm/fz/btm1FKpVSXV3N/s8/Z72vLz7rfdE3MESpVNLU1IS1tTXz588HQUJQUBDPPPMMISEhLFmqQrw1NTWxwtWVmpoauru6eOaZZ7h69SoBAQHU37vH3HnzeEKN7L5y5QoVFRX4+vmRkpzMG2+8TmNjI97e3uTl5TF//nwcHByIjY1jlr09gUFB6OvrU1ysQo2N6zq9wA/Jff82exdV6bo2oPVdOv5/9x5obW0lPf0ib7/9FmVl5ci0tbGxtaW2tg5tbW1kMm3efvtt7t+/z+rVnri4uPDsc89x7uxZ9PX1uXfvHnPU7rNCocDV1ZWhYTnDw8OcO3eOXbt2MWnSJNLT08nKymLp0qX09PRw8+ZNFi5cyLJlLhQXF+PmtoLAwADq6uoQ1Pp19vb2+Kgr6dra2pgyZQqiUom/vz/h4eFMmDCBBQsX4uvrS9ODB/zut79FLpdz4MBBzMzMiY8/RVZWFvkFhURFR4+N9OvWrcPf35+LFy9SUnILOzs7enp6KC4u5rnnnqOouJjOzk4AUlNTWb58OT3d3RhNmICRkRFdnZ10dXUhVQtm3Lt3D6VSyf3GRvR0dbl//z6CAPPmzaOtrZ2BgQE8PNzJzs6htbUVfX09Xnjh+bFRv6ioiNHR0XF274X/JPfeRBTFEFEU3xRF8e1H2+N0/NHf9CrQZDLbt0cwadIkEhIS8PRcDYCDgwO3b99GEASmT5/Onj272b9/P6OjCiwsLHhy1y4++fhjPvroI1ofPqSyspLBwUHa29sJDAzk9OnT2M1USU3PnTePosJCjh8/RnNzM7a2tqrRbpY9c+bM5vDhI/j5+eHq6oqGhgZXr14lLe087u7ugErm+VB0NGu9vLCbOZOoyEgcFy8mYvt2zqam8u677/Lkk09ib2/Pw4dt7N27h1WrPIiNjeXEiZP09fXR1NREc1MT06ZORaqWiHZd4caxY8dwcHBAS1OTGzk5LFy4kD179nD0yBGV0o2hIRKJhNraWnJv3CAzM5Mntm4l9cwZDPT18fLy4saNG3z55ZcEBgUxNDTEmZTksUi+KIocOXKEFStWsHy5C7/85S9xd3dXCYn0dHPz5k3mzZs3ruKVAAgCgkTjW7cfiKULgrDuH+n4o3fvi4uL6OzsIikpidHRUTo6OsjJzkaqqYlMJuOzzz5j/nwHoqKikEo1MTU149y5c0ycaIpMJmNUqcTWxoaAgACysrLw8PAg7dw5BoeGOJ+Whp+fH0mnT6NvYMCyZcvQkslY7rqC5ORkWlpaiI+PZ3R0lKamJtLS0hAEQYWuystDLh/huFpiqqKyErlcTlxcHP39/Spm/uTJZFy5wt2qKvp6e4mLi0Vf3wCFQsH//M8fmTHDmqCgIJRKJdu2baW4+CYZGRl4rFpFTEwMolLJ0PAw9+/fJzU1FalUg5KSEo4eOcKwXI6GVMobr7+OkZEhBw4cxMTEBDMzM6ysrMYCdo84AllZWRgZGZEik3H7zh0mGBly6lQCMpkMc3MzTpw4iUymjbGxMcbGxnzwwYfMmzcXQZDwpz/+EZ/165FKpcjl8nH9e39fI7kgCJGAPwhRr3YAACAASURBVNAqiqKDus0EiAWsUYExw0VR7FRXlH4A+AIDwE5RFAv/yUt4Hvg/giAMAyN8hyW7H/1Nv3KlOyBy//4DNm4Mobe3l46ODnbs3Mnw8DCXLl5k3jwHvLzWAnDs2HFe27ePiooK1q5dS3t7OwqFgvNpaTzzzDNkXLlCaFgYx48dI2jDBmxmzGCxkxMAUZGRY/rvfX19fPb550RHRbF37x6MjCYwMDDApk2bAJXc1gRjE0JCQkhJTmbjxo1UlJezafNmWltbyc/LU4Mpe5k4cSKLHB3JvXGDgAB/Dh8+go6ODtra2ri4uNDS0sLt27dZssQZPz8/tHV0xgqM9n/+Oa+99hr9/X10dHTg6urKYicnZs+erdLe27ePDcHBmJiY0PbwIY2Njejp6eHr60tLczOCRML2HTuQy+UMDQ0REBDA8PAw8uHhsVTj3NxcJk404YkntpCQkMC+ffsoKbnFyIic4OBgKioqxvL+xxlR/X3O6aOBj4Avv9L2GnBRFMXfC4Lwmvrzq3wD//Gf+XJRFA3+0b4/evceYPny5bi5reDzz/djbGzMunUqAm3Ml1/yxq9+hXxkhNu3b9PR0YG+vj6zZs1COTrK/77/Pn5+fmzZsoXU1FRsbGxoaWnhzJkzOMyfT2hoKLdu3aKjo4OBgQEmTZqEra0tSadPs2TJEqRSKWvWriUxMRETE2MsLS25desWfX19TJ5spc7+S8VqyhQWLVo0xoRPTEjgo48/Jun0ac6ePYvXunWYm5ujFEU++ugjli5dgq6uLj09vUyZMgUnJyfy8vIRRRFDQ0M6OjoYHh7m6tWrrFjhyty5c6ioqKSx8T4REdvIvHaVnp4eoqOi+P1771FVVUXGlSssc3EhNCyMy5cuoVAo1PJXiygsKMDExITnnn+eQ9HR9PT0sMrTk+xslVTbrVulvP766yQnJzMwMIixsTEeHu5MnDiRyMgoAgMDOHPmzLgjsBEEJFqyb90ex9S57R1/0xwEHFK/P4SKF/Go/Ut1JWkO8Ij/+A/8CIL1t+wX1AU532g/+pH+3r17lJbeQk9PD0tLS/LzC5BKNblXV0t3Ty9nU1ORaWsTF3eCvr4+1qxZQ8KpUzQ0NlB37x7Xrl5ldHSUvr4+4uNVc+f0Cxfw9vbG0NCQiO3bOXjgABpSKVu3bqW/v59XX32VsLAw7t27x6hCwYUL6RgbGzNv3jzu3CkjM/M6S5Y4c/36dSorK9mh5uVp6+iQlJSEh4cHyUlJZGdnY2VlpXbNpVRWVlJTXYWpqSkXLqTz2muvjv2cq1evJjr6EEuWLmX1mjWkpqYyONCPh7tqhDUyMuTBgyZEUcTV1ZWXXnwBj1WeXLp0icKCAoxNTGhra8PMzIxRpZKDBw+yefNm9PT0eOnFF5m/YAHnz59nurU1MTExWFtb09LSQldXN56eq9DXV4li1NfXc/bsOYaGBhkcHCI39wZ1dXVMmjSJ0dHRcY3eg/C4c3ZTQRDyv/J5/2Oq3Fg8wl6rATDm6vZv4jw28d3tvwVBkACngQLgIaoIvh3gCaxBRY9u/KYT/OhveqlUiiBICAwMRF9fn8HBAXx919Pc3MwMG1uCQ1Quan9f31h9uUKh4MuYwzg6OrLWywuZTEZnZwcmxsaMyEfQ0JCwdu0arl+/TvqFC3R3d1NXV8fEiRPR0NDA0NAQT09PNDU1Acai4I+i9Ieio7GxmcE77/ya47FxtLe1EZmZyahCwZUrVxgdHcV1+XJ6e3sR1Fl6Q0NDdHZ0MNPODhcXF7Kzc7h69Rp371YxMiJneFjOuXPnMDQ0pLKigpMnT7LWy4uTJ+MRRZH29nYqKsqJjIykt7eP+fPnExYWhr6+Pg319VhYWpJ57RqdXV3ItLQoKizE3MwMXT09FAoFwRs2MGmSJTdvlrBx40aGh4fRlEpJSDjF2rVryczMpKysDB0dXdavVyk8x8ef4rXXXiM7O4fNW7bwzju/ZmBg/LLyBEFA8nhz+jZRFJ2/z6/+mrZ/aJlCFMUwQSV9vRXYhQpWMwCUoQLRvCuK4tDfO8d44rK0gauATP09J0VRfFMQhBnAcVRQzEIgQhRFuZqK+yXgBLQDm0RRrFOfax+wGxgFXhJFMU3d7oMqQKIBHBBF8fff9TqtrKwIDgkh8uAB3N096O/vJycnh6XLlpGdnY1SqaSnpwepVANPz1XU1NRwIT2dJ5/cxfDwMNczM9FRCz9cuZKBpqaUiIgI0tLS8Pf3x9nZmcOHjxAcHMxEU1OKiop44403OJ2YSGhYGFeuXMFl+XIqKyro7+/nYWsrAQEBDAwMcvJkPMpRBfazZoEgUF9fj7u7O/Pnz8fM3ByjCRPQ1dVVXdOFC+ze9SSamprEx8ezYsUKOjs7Wb/eB0EQ6O3tpa+vT4Wk6u3lzbfeIjc3l42hody/f59Lly7i7u6Bjo42kyZNYtasWWRcvUp//wC+fn7k3rjBxtBQQBUHWL16NY6LF3Pv3j2efvopqqruMnGiCUVFRezYuZOjR45gbGKCn58fWloywsPDOXz4CIsWLSInJweZTIaFhapM+erVq/T3949/aS2Md3S+5Ss4uEmoyl7he9ayE0XxDvDLf7T/eM7pH4ldLESl4umj5tm9B7wviuJMoBPVzYz6tVMURTvgffVxqJ9qm4F5qOqFP1GjtTWAj1EFSeYCW9THfmeTSCTs2fsUNbW1NDbeJ7+gkHnz5rFy5UpKSkqIPX6cDRs2sHz5ct577w94uHsgk8nGUnTLy8qwt7cnNHQjdXV1GBgY0NbWTnNzMzExMezdu4e1a9dQVlaGXC5nwoQJ6BsYUFVVRX19Pfb29vgHBHAxPR2jCRMQUWXXBQYF0dnVxU9ffpnCwkKWL19OcHAwBfn5fPzRR7i4uLB06VLef/99Vrgu/yvPQUNDQkCAPxcuXABUAciI7ds5e+4ctTU1qvRhGxvOnDlDxpUrRGzbRlnZHQwMDFi4cCHa2trU1zcgAFOnTqWvTyVhcCIujnXe3oRv2sSF8+epv3cPZ2dnenp6OBgZyRY1314ikdDZ0a4OJDbz1FNPo6GhQX9/PykpKWRnq+r3ATZv3kzkwYOMjo53Pf24r9MnocqHR/16+ivt29XzbRfU/Md/5ov+Gft/IXaxGnhC3X4IeAtVNDNI/R7gJPCReqkjCDguiuIwUCsIQhV/UQOtEkWxBkAN1AxCpRf22FZaWkpNTQ0ymQxNLU3kcjkN9feIj49ngpERCQkJTJs2lZMnT2JsbIxUKuXq1asUFRdjYmxMT28v2jItkpNTyM3NZXR0lEOHDiGVarJv3y9Yv349KSkpaGhIGVUouHP7NodjYtDR1eWdd97BafFikk6fRj4yogJKBAZSXl7O4cOHkQgC9vazuFd3D2cnJ2pra7l29SqDQ0MUFBaioybZ9vX1kpNzg4KCQnR0dJDJtLl+/TrOzs60tLSSkXEVQRA4euQIOtramFtYkJeXR1NTExlXrqiIOtGHaGtrp/LuXSrvVtHT3cWlS5fx9PQk6fRpDA0NSUpKwtramp6eHpKTk6morEQq1eCLL74gP78AGxsbjh07hra2Ng2NjfT39XHvXj0eHh5kZWXh4+ONXC7H0NCQ+vp6oqKi0NXVQ0dHmysZGWhoaIzrkp0gCGhofqfktb93rq/Tsvs9ECcIwm6gHghTH56KarmuCpUr/uT3chH/oI3rnF49GhegCjJ8jApe2SWK4qOJ21cFKsaCHaIoKgRB6AYmqttzvnLar/b52+DI1y6DCCp98acApk37a76AlZUVCsUIgYGBdHV1oRxVolAoCN0YQltbG7GxsezevRtTU1Oys7P5yU9eoqKikqCgQORyOb/4xS+RSCRs3boVXV0d6urusWPHDs6ePYuPjw8ODvOYO1flgHzxxResdHcnNDSUnJwcfH19MTc3x9PTk5MnTrDS3R0/Pz9qampQKBRjghfy4WGKi4t56umnEQSBpqYmqu7eZc+ePcTFxvLbd39LcXERvr6+gAqkaWNjS2vrQwwM9Dl69Cgv/eQnzJ49mzMpKTQ3N7N582bOnz/Pu7/9LSnJyURERJCcnExwcDDJyclYWlgwdeo0hoaHWbZsGVevXePMmTMsd3XFZdky+vv6ePbZZ8nNzWXz5s0UFhXjHxAw9rNGR0VhZ2vL0qVLOHDgAK+++ip371bh7OyEra0txsYdREREIJVKyc/Px2bGDCrKy3nwYDwVnAUEyffzLy+K4pZv2LXma44VUa2r/1vYv1TsApjzdYepX/+fiF0YG6vwVbW1tRw/HktgYAB9fb0AnDp1ik8++ZjERJWXVl5eMYamGhoa4sDBSH7285/j5OREYVEha9asYdWqVZSUlNDT08umTeFcu3YNhUJBW1sbM2bMYEQuJzc3l/7+fjZt2sTdu3e5dOkSNjY2eHt7k5ubi9uKFSxdupQzKSkolUq0ZDKCQ0I4k5ICwJmUFGzt7FAoFIwqlUyaPJlHyj2VlZVYWVkxZcoUpk2bRk9PD+7u7hgbG3M6MRGX5cvZGBpK+oULdLS3M23aNHY++SQxX36JUqnks88+Y968eQwPq2r59fX1GVEoGBkZwdPTk7Vr11JWVoajoyO2trYoR0fp7+/Hz9eXgvx87t+/z9DQEObmZgwNDVJdXcPMmTOxtbWlubmJ+PhTeHh4EBYWRnr6RfLz82nv6OQXv/wl/f3945uVJ/xHVdkhCIKVIAiugiC4P9oep9+/ZJ1eFMUuVAIVLqjWKB89br8a0BgLdqj3G6FaB/17IhjfS3DE1XU5b775JlOmWCGRSJg+fTrnzqWxaNEiNDU1cXNbwcWLFzE1NWVgYAC5fJg9e/ZiZmZGdlYWSUlJGBkakpFxlYkTTTh27DirV6tSeSMiIkhISCQhIZHVq1dTVFREfHw8IyMjKrdfIiH1zBk6OjowMjLi8qVLrPL0ZO7cuVhYWhIVFaXCZJmbIx8Z4d69e5iZqwi4kZGRrF2rShqaNt2ampparl69iqenJ4GBAcTExGBnN5OtW58gIyODgcFBzMzM0NLS4uixY3R2dpKSkkJ6ejp9fX0kJCSwceNGbGxs6OntxcLSkoCAAK5cvgyiSNCGDbz55pu0t7djoyb5hIWHk5ycjNGECWMpwQfUAp4DAwNcvHhxLJV4YGBgbH1fV1eX06dPk5Z2nmXLlmFsbMzQ0ND4LtmN/5z+X2aCILwHXAdeB/5Lvf3873ZS279c7AK4DISiiuD/bbBjB5Ct3n9JFEVREIQk4KggCH9CRf6cCeSiGulnqlcD7qMK9j2KFTy2tbU9ZGRkBMfFTty7d4/IyCj09fVJTU1l06bwsaSRkydPsmDBQrq7uxBFEf+AAEJDQ+nq6qKnpwejCcYsWLiQ8+fP8/BhK7m5uSgUI4yMjFBYWMjw8DDR0dEIAqzy8MDHR7VsdSo+ntf27cPAwIDXX3+d1tZWFW0XVTAs6/p1mpubKLtzG7lczr7XXmPT5s3o6OjQ2dlJa0sLVVVVyOVy3nzzTdatW0di4mmGh4cpKirCwtKC1tZWCgoKUI6OIpVqYDrRlMWOi5jn4ICnpycAMTExuLu7k3buHEpR5H5jI+np6SiVSqpraujv72eiqSlLnJ3R0dEhJiYGHW1t9PX1uXTxIuu8vYk8eBB9AwMKCwtISEjk1q1S9PX1OHr0GCMjI1y8eJE5c+YQFxeHkZERkyZNYpGjI2lpafT19qqVgcdby+6HcVM/hm0AZqljXd/JxnNOPwk4pJ7XS4A4URRTBEG4AxwXBOE3QBFwUH38QSBGHajrQHUTI4ribUEQ4lAF6BTA86IojgIIgvACkIZqyS5SFMXb3/UiTU3NaGtrUyGoz5xh584dFBYW4ezszOTJk1m0aBHFxcXs2fsUdysr2bZtGydPxtPZ1YVSqeRUfDwR27cT8+WXuLi4MKJQ8PTTT6OhIWXJEmfa2toYHBxidFTBhg0buHTpEhINCbdv32bevHn09/djamrKwYMHsbS05NlnnqGwsJDQsDDu37+Pubk5dXV1hISEIAgCWVlZuLm5sf/zz2loaEAELC0tqaioYP78+bitXIm1tTUnT5zgt7/7HTdu3CBowwba2toYGOhnY0gImZmZ+Pn5cT0ra+z3INPSQkdbm1WenqSkpDDZymrMW6muqkJiaclKNze6u7oYHh5m48aNGBkZoVQqSU1NxXTiRMLCwsjMzGT6dGueeGILSqWS3t4ewsPD0NTUpLe3B2trawICAgAYGFQhtcLDw0lPTx/30tpH7v1/iNWgCo7/+9z0oiiWAI5f017DX6LvX20f4i/Rzr/d9y6q+uG/bU9FFRn9p6y7u5spU6YQGhbGhQvpNDU18cKLL5KclIRcLqe8ooKIiO2YmJjwm9/8hj179iIfGSEnJwcjIyM0NTXZvmMHsbGxaMu0cHFxITr6ELNm2ZOQkMiePbsRBIGPP/6EadOm4uPtTXT0IczNzdHR1eWTjz8mfNMmzp07h42tLV1dXVy9epWKcpUgRW9vL0lJyQwNDfHzn/+csrIypltb8+JLL3H82DEsLC1ZtGgRixYt4rPPPmP79u2AKmhZUlJCXV0dRkZGbNu2lejoQ8hkMjw9PWlubqayspLKigrcVq7E0tKS1NRUNNVKt3K5nKioKHbs2IGWTEZ0VBSmZmZjtQVPbN1KdnY2z7/wAiUlJQBUV1djZ2fHsWPHxlJtExNPExYWyuTJVgiCQHV1NX19/djZ2ZGTk4NSqaSluflfgMAWkHxP0ft/AxsAigVBuMhfQzRe+raOP/qMvIKCAmbNnk1RURG9vb2kp6ejr69PSkoKw8PD/Pf//HEst14QBG7fvsPly5fpHxjgdmkpwSEhnDt7lqHh4TE12FOnEmhtbeWFF15kw4YNxMfHI5eP0NDQQENDA/39/RgbG/PG668zafJkXn31VbS1tcdQVsbGxnz22WfY2tqQlJSEhoaUgoJ8jIwmYG29iS9jDhOsPq8gCCQmJuLt7U1dXR0mJiY88/TThG/aRPqFC2jLZPzyF79g9erVpKam0ni/Ebm6zn/VqlUkJJ5mdHSUyZMnA6oHoJ6+PqE+Pux96ikcHByIO3ECDYlE9TNfuYKpqSkmJibU1tZSXV3N9u3bqa2pobS0lKlTpjBn7lze+/3vCQoKQldXF319fTIzM5k0yRJXV1c+/fRTNDSk7Nm7FyMjI/784Yf4BwSMez298PhpuD8ES1Jv39l+9De9IAgU5OcTHBKCqakppmZmzJo1C39/f1pbW1GKIubm5qxYsYLbpaVEbN+Onp4eK1asYMf27QwNDrLO2xuAUbXUcnBICFlq1zk4eAOCIJCTk4O19XRaWloIDg6mpaWFmppqWlpaSUxI4EFTE5pSKYcOHcLC3Jw1a9ZgYmLMunWqkukHD+7T1tZOZGQkdysraW5uJjw8HKVSiaamJgb6+qz39UWpVHL9+nXWrVs3JnCZnZ3NMhcXHBwc6OzsYsIEI9zd3Tl+/DgZGVextbUlOjoa5egoeXl5mJqaIgBTrKxYv369iqqDat5vZGSEpaUlJS0tvPHGG/h4e3Ph/Hn09PV59913Wb9+PdezsjAzMyM3L5/e3l6GBgc5dOgQmzdvIjk5GUNDI65du0ZsbKz6QXobG1tbdZHQ+ApYqmabP3wTRfGQmnFvr26qEEVx5HH6/uir7BYvXoy/vz/FxcVMmTKFqVOm8PDhQ0RRJOn0aUJDQ2ltaQEgJycHHx8famtruXTpEq+/8QaDg4NIJBLiYmPxXL2azq4uFAoFZWVlBIeEkJl5nZaWFhoaGnF1dWVwcIiGhgbS0tJ4+eWXcXBwYPOWLfR0d7PS3Z0dO3ZQ39BAyMaN9PX1A5CcnMLq1asxNzdnx44dBAYG0tLSgo6ODtVVVSxdtgx9AwPycnM5ERfHz3/2MzIyMtR9k9m1e/eY+21kZEhvbx+6urrMnTuXsPBwlixdys6dO3FdsQJ3d3dmzJiBKMLLL79M2Z071NbWAow9RKZOncoqT08c5s1jsZPTWJXfvHnzVP2tp7No0UJsbW3x9/fHZ/167O3tcXR0xMvLi86uLjYEB7No0SI2bdqEvb09VpMnozHe822B/xiIhiAIq4C7qPJfPgEq/62W7P7dbZ6DAytWrCAqMhIDAwN8fHxITU1l2vTpSCQSurq7GRoawnLSJARBYMqUKdwuLWX27Nm0traqXFJBwMTEhODgYK5mZKCvp4ednR23bpVw8OBBXF2XU11dTXFxMRcupBMREYEgCOjq6tLa2oqjoyOVFRVkZWXh4uKCpqYm/f19VFVVIZPJsLOzY9myZfzxj39k7do1ODo6cuHCBWrr6jAzM2PlypU0NDbS2NjI1GnTaG5uBqCvtxcrKys0pVJKS0uxsrJSRdwvXaKzq5v169fT3t5Obm4u1VVVPPHEFhYsmM/Fixdpb28jLCyUjCuXSUxIwNnZmQ0bNpCdnc2JuDj+z6uvUlhYiFKp5EZODq+//jpf7N8/5ildv54JwNGjR3njjdfJz8/nwMFInnrqKby9vbl8+TJdXV04OTtTWFjIqHKcyTnqkf7bth+I/RFYJ4qihyiK7oA3qvT1b7Uf/U1fWlpKdFQUOTk5mJmZUVBQQH5eHodjYmhsbKSnp4fp06fz5w8/xMHBgQNffMGD+/cpK7vD4ZgY9PT1+e8//GEsj3zixIk03r+PTCYjKiqKO3dU+fYVFRV0dHQgk8morq7m6NFjJCensGzZUv70pz+xZOlSent7KSsrY+7cuSiVSuQjI5w5k4qDwzxKS0tpb2+jurqG5uZmZs60Q1SOkp+Xh1wu54svvkAiCAwPDxMVGcnw0BD79+/HUx2B3xgayicff4wgCAwODhIbG0tHRztHjxzhyuXLHD92jO7ubiIjI8nOzsHAQMVoeJSfkJycTElJCXl5eaQkJ7N48WKUSiXm5ua8+atfoaenx5HDh+nu7uL48eMcPHiQocFBMjMzWbhgPnFxcZw4cRJzc3PS09PJzs4mICCAd379a6ytrTE1M2N4aHjcg3mCRPKt2w/ENEVRrHj0QRTFSlTR/G+1H/2c3sbGhvLycgKDgjAxMVHx7ObORSmKaGhoUFhYSEd7OzW1tdTW1rJr927i4uJYudIdLy8v+vv7SDt3lhs3csi9ISCKIvl5eUybNo0XXnwRTakUQVDVsyuVSpWMU0cnW7c+gVKp5Pz58zQ2NlJUWEhjYyOdnZ18eegQSvV5NDQ0uHPnDtOmTcPOzg5LSwuUSpHjx48jiiK3b98mOSmJPXv3qqgzgsCGDRu4du0aRw4fxszMDKlUilSd1z5p0iRu3bqFl9c6lixxZvr06fT19WJhYcmGDUEAHDwYSWBgAJqamnh5efHpp5/i6uqKl5cXWlpaJJw6Rc6NG/T29fHgwQPaO9oJCwtFKpWiqanJ4OAgfn6+5OTk8PlnnxIevgl/f3+6uroxNDDA28eH1tZWLly4QH1DAyfi4ti5c6eabDPO5ByN/5jofb4gCAeBGPXnrahS3r/VfjCPtfEyXV1dnn/+ORITTnH37l2Ghoe5fOUKISEhDA0OMnfOHPT09fHw8EBPTw+JRMLg4CDbIiLIyMhg6tSp+Pn5ISpFNm4MISQkGBcXF0zVmW9GRkZoaclob28nPv4Ubm5uODqq1v5VijBN7Nu3D0MjIyZbWTF//nye3LWLXbt24eW1lmeffRaFYhR7e3vOnj2HnZ0dM2ZYs2PHDpRKJa6uyzE3NyMvLw9QBROVSiUlN28SvmkTNjNmEBAQwNSpU5k8eTIKxShz5sxVC1GcZWRkBG1tbdraHqJQKMjKymL5cheWL19OScktYmIOE7QhmM1btnDjxg2ysrL42c9/jpWVFVaTJ6NQKNi79ymKiorUv08dtm+PIDX1LH5+fqxes5ampiYkEgnm5mb09vby4MEDHra2gigSGxtLU1MTg0NDKJXKcR7p/6Pc+2eB28BLwE9Q5bE88zgdf/Q3/SPbvXs3t0tvcTY1lcWLFyMIApu3bCE5OZm2hw/ZvHkzTU1NlJeXo6OjA8CIQkFMzGHWrVuHhYU5RUVFfPllDAEB/jguWsj7f/oTS5cuJTR0I9evZzE8PIypqSmOjo7cvFnCuXNpuLi4MG/ePGqqq9HR1maluzt5eXkcOXIELy8v5s93QF9fj8uXLzN58iQCAwPJzy8gOjoaHx8fzMzM8PPzQz48xLmzZxkdHeWLL75gW0QEq1evJjcvj97eXnLz8ljm4kJKSjIrVrgCsGPHdk6cOIGmphahoaFcunSJqqoq5s6dS2npbRITE9HQ0MDS0hI9PT26urqor6/H1taWwMBA9u/fT/29eyxcuJDCwiIaGhowMzNDIpEwc6Zqrd51+XL27N3L0aPHqK9vwNbWhl+98QZJSUlseeIJJBIJS5Yu5XRiIkqlcpzJOf857r0oisOiKP5JjcEOFkXx/cfNzvvRu/e5ubnUNzSgp6uLrq4egiBw584dWlpaGFUoVEir0VGOHjmCfGSEgwcPMsHIiMSEBEYVCrKzs5g2bRqiqOTUqVNoa+uQkZGBQqGgqKgIIyNDjI1VElSzZs2iuLiY4uKblJaWcvPmTQKDgjB68AB7e3tulpRgb2/PoejoMT25kyfj0dTS4tLFiyxZ4oxCMUpcXBxubivGrk1V4NNDSUkJhYVFLHZyIi0tDTtbWzZv3szxY8dUqKp796ivbyAhIRGZTAupVEpPTw/19fUoFCOcO5fGkiXOJCUlMWPGDNzc3Ghubiby4EGMjY25ceMG2jIZR48cwcDQkOnTp2NsYkLS6dOMKpW8885v+MUv9pGcnExzczPJySn09vZRXl7OtGnTKC4uwszMjIULF6KpqUlaWhr9fX0MDw3R3NyMVCplZOSxVp3+MRN++Et2giDEiaIYLgjCLb6mwEwUxQXfdo4f/U0/f/58urq78fP35/ixY7z3BEDJzAAAIABJREFUhz9w5syZsdz4h21tGBoYELJxIwCRBw/S+vAh7h4edHd3U1Z2h7Vr1yAIArW1tVhYTsI/IJCioiJeeOF57t69i6fnKn71q1/R19dHcHAwW7Zs5suYw+hoa+Pk5ER2djaZ167R1t7O4cOHycrKwsDAgNlz5rB9xw6yrl/nlVd+Sl5ePt7e67h+/Tpr1qxBKpWSkpLCvXv1vPDC8/j6+vLJJ58yY4Y1K1eupKWlhaLCQm7evIm9vT36+no4Ojri5rYCc3MVvq28vJxPPvmEkJAQRkeVDA8PsWiRI9OmTaW6uhqJRMKiRYuYNm0aWVnXWecdiJubG599+ilPPbWXlJQzbAgORi6Xs3vXLh40NbN0mQsPHjxAqqlFdVUVISHBmJub09urqvvftGkTOTk5zJ07l+nTVbkL//v+++jo6DAwrtJWAvxAluT+jv1E/er/j57gh+HLjKPp6OgwqlBQV1eHto4OEydOxN7entLSUk6eOMF6Hx96e1WltrHHj+OxahUODg4MDg6Snn6BJ598ksLCQuLiToypuQIUFxWxfPlyFi924ubNEjw9PZk0aRLm5mZoaGhgZmqKoaGhSodeS4tVq1bx/PPPYz19Om5ubkRERHC7tJTBwUGamh6wYMECli1bym9+8y5vvfUmmZmZ2NjYMHfuXDZu3EhJSQnl5eW4ua1AT0+PqqoqZs6cybx5c/Hz88Pa2poJEyawbdtWEhNPj2W+XbuWyTvvvENGxlUMDPR55plnyMnJISMjAwMDAwIDA0lLO09nZyfe3t5UlJdz8sQJ/Pz80NHRob9flUtw4IsveOvtt5FqaKCvr8+tW7fYuXMnCxYsICkpeUwBZ1QtYBkUFDg2HTmdmEhgYCAjIyPjLGslINHQ/Nbt39m+Qtx5ThTFe1/dgOce5xw/+pv+0bLTa6++SktzM9HR0fT395N27hwSDQ0sLC0xMDAg5ssvmb9gwVjCyalTp5g9azazZs0iNzcPAwN9Jk2ahLOzMx9/9BEeHu7k5+dz40YOhw4d4vbtO8yaZc/Jkyc5evQoa728WO/ry+9+9ztqamqYPWcOVlZWlJWVMTIywtDwMAgCzz37LKIIR48do6iomIaGBs6cScXe3p7MzOsYGhqxcOECQODYseMsXLiQVatWqZHWjcTHn8LHx5uysjLs7OwA1CKS5ykoKMDJaTFGRkY0NT1AqRQ5cuQoQ0NDxMQcJi8vj1OnTiGVavDKKz+jrq6OxsZGbtzI4datEsrLK9DU1OTTTz8lLDwcW1tbHjx4QHR09JgufXdPN08+uZPr17O4dOkSrq6u9PT00NXVhY+PN++++y7Lli3F1XU5w8PD469a+58TyPP6mrb1j9PxR+/et7W1MdPeHm/vdRgYGBIUFEhrayupZ87Q1NSkSg3t7SX3xg0mTJhATXUVgiCQnZWFnq4udXV1XL9+HaVSyZEjR1T7srOZOnUqc+bMZteuXWhr66Crq4uJiQkymYzc3Dz09Q3Q09dHUypFJpNRWlpKT3c3NbW1aEgkrPf1paamhp++8gqtra34+PhQUFDA5MmT0dBQRf0TExNZunQp8acS0NLSorKykoTE00jUKrBvvvkWdnZ26jLXW1hYWFBZeZfBwUFu3bqFXC7H23sd8fGnaGhoQE+vk2effYaOjg4mT55Md3eX2u0fJT+/gN27dxMVFYVSKeKz3pfioqKxqP3ly5fR0tTkVEICqzw8xqrmdLR1+O1vf8fMmSqtvLKyMvT0VLGTzs4uqu7epaCggGvXrv0L/to//Nx7QRCeRTWi2wiCUPKVXQao6uu/1X70N725uTnZ2dls27qV8vIKrly5wrp161i6bCmmE00xMzPjwf37BAYGMmOGNbNnz6ajo4PRUSU6urqsWbMGowkT6GhvZ+vWrURGRvLBB//L8eOxrF27lsTE03h6ruLs2XPMmjWLkpJbeHh4YGtnx4IFCxgc6Ofhw4f4+ftjaGjI0NAgMpk2dysrmT1rFo6LF3Pq1CkaGxu5VVLCzief5OCBA+zevYuenh5MJk4kODiYwzExfPjnP5OZmUmQeo49PDSEXC5n48YQBgYGMDY2HlPO/fyzzwDw9fVFoVAglw8zYcIEDAwMOHbsOHv27iXy4EFEUeTkyXheeeWnXLmSwYQJxjgvWULmtWu4e3hQWlqKUqkkPDychIQEgoM3MMPaGkdHR9ra2og7cZIJEybg6+uLjo5KgMPb25uhoSEOHTqEh4cq9fjAgQPjHrn/PnPvBUGoA3pREZoVoig6f5Os1ffyhX+xo8BZ4HeoFHQeWa8oin8rvvG19v/de6USI0NDZDIZCxcuQCbT5uzZs0yeNJk1a9dSUlKCKIqsX+9DRsZVBgYGiI+Px9/fj5bmJk6pcdOLFy/m6NGjzJo1G1NTU/bs2c2f//xnHj58yOTJk3FyWkxJSQmjo6OEhoVxq6SE5uZmtLS02L17N3FxsSQmJrJmzRoaGhqob2hgspUV9+/fx8nJiS8PHWKSuhLOXQ2atLS0oLu7i4GBAbS0tDA3N6e/v5+uri6io6LYvHkTa9euITX1LPoGBnR3dwNw8MABNmzYgKamJqIoEh0dzYYNG1AoRomPj8fXzw9BEHBydub27duMjiqwtbXlwoUL2NvbM336dMorKrh58yYLFi5k/oIFfPzRR8yYMYPg4BBu3iyhsLCQixcv8cwzz/Dc88/z+9+/h42NDUqlkubmFg4cOMiTTz7JzJkz+eyzzwkMDBz/bDzhe1+y8xRFcdFXGPmPZK1mAhf565vyezFRFLtFUawTRXGLeh4/iCqKry8IwrRv6Q6AMF5zKEEQpqLi2FsCSlQqIR8IgvAWsBeVMgfAL9R18d+Zb/9NDP2/d13Ozs5ifv5fxEt+9rOfYWpqirGxMZqaUjQ1tTh16hTe3t6YW1jQ1dXF9cxruLu709XVxdDQENOnTycoKIizZ89yt6qaSZaWDAwMcP78eZYvX46WlhYymRb19Q10dnXhuGgRhoYGJCensHnLFgwNVdrwSadPo6enh7m5GT09vdTW1rBs2TLOnUvDy8uLOXPmINHQQCKREHv8OBKJhLlz56Krp6cq8PFchY2NDadPJ/Hc88+jp6fHyMgIX+zfz7JlSzEzM2dwaJArl6+gq6eHoaEhWpqaBIeEYGlpSemtW1RXV2Fqaoab2woiI6MwMzPDXw25APjvP/yBuXPn0t/fT2pqKm5ubpiYGPPgQRN5eXmsXbsGfX19TiUk4uXlhaZUSnFxMf39/bi6ujIyMoJcLufy5cssdnJSKfKcOcP69T7oGxjwsPUhRUVFODs78dOfvsLIyMjXzusFQSj4ZwUoHOfbiZcT//CtxxnbbfzW71KP9M6iKLZ9pa0CWPUV7v0VURRn/TPX/He+PwB4RJNqBaYDZaIozvu2vuPp3iuAn4miWCgIggFQIAjCBfW+90VR/J+vHvw3fPvJqKR4H5UNfowqcNEI5AmCkKQG/j9i6B8XBOEzVA+MT7/LRS5cuBClcpTw8HAANbJJiZZMGx8fH7744gsWOS4eK2N98cWX6O3to7Ozk+bmZqqqqtm8eTNmZmYYGBrS0tzM3r17EASBI0eOMjIywraICLq7uzl//gJSqRRjY2MUCgWaWlosXLQQP19foqOj0daew7Zt20CQMDo6ytJlKrivXC6n3tWVocFBNm/ZwsDAAPX19cy0n8WsWbM4evQYzU1NTDQ1paO9nZaWFmbY2I6tQqxYsYK2todoamqRlpaGgYEBWlpaaGppcToxkY0bQ0hKSiY9PR0/Pz/iYmORSFRxgeLiYuztZ7JpUzhSqQYaGlI2bAiivLyclpZmNmzYgK6uLgMDg5iamuLt48Pg4AA6OrpjDPza2lqmT5+Gjq4eU6ZM4WFrC46LnZgzZw6RBw/i4OBAREQE+/b9AoVi/BRuePw03MeRtRKB84IgiMDn6v3fJGs1HvYbVMzJdFEUHQVB8AS+idD7VzZu7r0oik2P5HhFUexFJbtj9Xe6jPHtRVGsRcUIX6reqkRRrFGP4seBIDUTfzUqRj78tWDgY5uVlRWLFy8mLu4EAHFxJ1izZs3Y0puJsTG2trbU1dXR09ODr+96Fi1ayPbt2zG3sBxb1x8YGMDIyIiQjRtJTEzk7NlzuK1cyURTU5qamog9fpwPPvyQ6qoqpk2bxsX0dPbt24eGREJ9fT3Tp0/H29ubvLx8tDQ1mWFtTXl5OQBHDh/G29ub4eFhBgcHORwTwwsvvEBNdTUWFhaEhobyoKmJBQsWUHn3Lm//+tcoR0e5fj2TkRE5AQH+LFiwAC0tLXbtehKpVMqmTeGs8nBnpbs7CBICAgNZs2YNEomETZvCCQsLY9rUqbzyyk8ZHh6mpaUFCwuLsXr3rKxsfv3rX3PqVAI3b95k6dIl9PT00NzcPJbk1Nmpms5eTE9n3bp19Pf1ceniRV566SUyr11DFEX09fWJiNhGipr0O97ReySSb9/UslZf2b5Ox26FKIqLUUXMn3/cstbv0UZEUWwHJIIgSERRvIxKVOZb7V8ypxdUSpuOwA110wuCIJQIghApCIKxuu2bRP6+qX0i38zQ/9vvf0oQhHxBEPIfoaK/anZ2dixe7Ehc3AlEUYmJiQn29jP57LPPcFm+nFWrVnHr1i3i4uLw8fGhv7+fg5GRhIWFYWJigre3N6+9+ioGBgY0NjZSW1tL2vnzDA8Ps3r1aj799FMWOzlx4fx5mpqaePaZZ9ixcycymQy5XE5aWhqenp7Y2dlRWVmJpqYm7h4e5KhltQwMDNDR0SEsPJwPPvgAt5Ur0dTUZOu2bXz4wQfY2tpiYWHBT156SVUpmJ/H559/TldnJ7NnzWJkZAQnJydu3LiBnZ0dS5Y4k5CQQEJCIhEREUyfNo2k06eZOm0ai52cOH1aBWQpKyvD2dkZAwNDIiOj1NTaCaSnp7N4sSMSiYT58x2Ijz/FjBkzCA8PIykpCT09XTZtCifjyhWKi4txclKlNTc2NjJzph2CIBAUFEhUVBQ2NjPQ19dHLh8ZdwFL4XvMvRdF8YH6tRVIQDU4tajdev5G1mo8rEsQBH1U0nFHBEH4AJV3/a027tF79YXFAy+LotgjCMKnwDuo3KN3UNUF7+KbOfZf92D6ztx7YD+o5vRf3ffw4UNiY+PQ1NQkOzsLAwNDYmPj0NLSJD8vD5lMphpBK+8yd+5crl+/TnJyCrNmz+b8+fMMDw0xODREW1sbHR0dzJ49G1tbO7Rk2vT09HAjJ4fGhgYVcdfREX0DAwaHhrh06RKtra2U3bnN888/P/bPbmJiTL86K232nDn84b332LxlCxfOn6e7u5vq6moKCwspLipCEARy8/KwnjEDIyMjjI2NWem2AplMxpUrV5gyZSq5ubm0tbWhUCjo6urkyJGjzJxpR2PjfapratA5eRItLS0uXryIhYUFbQ8fUl1Tw8WLF1m6dCmpqakMDw9TWVnJiRMnGR4eJiMjg1WrPLh1qxSpVIP79++TnJyMIEiov3ePSnUZcV9fP52dqorCoqIirl7NwNLSgtOnTzMyMkL6hQtoSATq6u4hispxp+E+gmj806cRBD1AIopir/r9OuDX/IXo/Hv+mvQ8HhaEKoj3U1QVdkbqa/hWG2+FG01UN/wRURRPAYii2PKV/V8AKeqPf49j/3XtbagZ+urR/h/i3puZmbF8uQtXrlxh27YI2tvbWLduHcnJKcxfsID1Pt709/dTUVGBtrY2AQH+LFu2jAnGxoSoFW0LCgpYsGABHR0dTJ06leuZmRgZGTFr1izkcjl6+vro6upiYWnJ2bNnefnllynIz2f37t3s3rWLjIyr1NTWgShSVlZO5d276OsboBwdpba2lvy8PPwDAjh27BhOTk6Eh4ejpaVFyc2bGE2YgJGRER3t7ZiZqbL87t+/z86dT1JTU0N4uIo12tDQgLW1NR0dHWzcuJHa2loOffklW554gvQLF3jq6ae5W1lJWHg4/f39/OqN11m+3AVbW1uampro7u7B1taGmTNnUl5ezqZNm9DR0UGhUJCZmcn69evR0dGht7cXDQ0NNoaG8uGHH1JRUYFUqoGbmxvOzksAgaCgIFpbWzEwMBwDdWRnZ/+QluwsgAT19UqBo6IonhMEIY+vl7UaD3sKOCGKYiOqqe1j27i59+o590FUEcU/faV90lcOCwZK1e+TgM2CIMjUUflHfPs81Hx7NRNsM5Cklgp6xNCHf+LJWlFRwdDQEE5OixkZGaGjowOFQsFPfvITLl++gp2dHd7e69DT00VXVxdT04ksWriQ9PR0AG6VlKhEHLu7iYuNZZ23twoHfe0aFeXlBAcHU3rrFgqFgokTJ2JqakpDYyMKhQqLPTQ8zMqVK1nr5YWZmRkvvvAC+vr6zJ4zB0tLS9b7+vLw4UOmT5tGaGgouTduoFQqyS8owNfXl5aWFjo6OtizZw+ZmZlkZGSweLEjSqVyTPnm3Lk01qxZw8DAAENDQ1y8eAm3FW6cPXsWQZ1f393Tw+joKEcOH+b37/2Ba9dU5JuUlBS2b4/g5s2bJCQk8tZbb5KcrHpWx8bGsnfvU1RUVHDs2HHWr/dBV1eX7u5uzExNcXNzw8vLi46ODnx8vJkyxYrz58+TmJjIWi8vjI1NqKmpobKy8l+gWvv9pOGq40sL1ds8Na0ZURTbRVFcI4riTPXrY62b/4NmCKQJgnBNEITnBUGweNyO4zmnXwFEAKsFQShWb77AHwRBuKXOJvJE5Z6gZtY/4tufQ823V4/ij/j2Zaj4+Y/49q8Cr6hZ+RP5C0P/sU0lTX1jrMBGVcV2gsAgFVDC2NiYO3fuYGQ0gZ07dxIXdwItLS0cHOaBKBIdFTUWZe/u7kYqlWJqaopMJqOtvR0TExPVeUxM+OTjj1WBM1RKrampqejo6LB7926OHj3K8WPH8Fm/nnkODtTW1JCcnMy+fa+RmZnJubNn8Vy9GlNTU1paW4mJiRnzNHp7etDT00NXV5eWllb09PSRSCSEh4dx4cIFUlNTx4qCwsPDuXz5MhMnmmBpaUFCQgK1tbUUFhbi7+/P/v37WabGdc1fsID8/AJMTEyQSCS4urpSXV2NlpYWOjraNDQ0IJVKWbRoITU1NejoaGNmZkZQUCCXL13CwsKcTZvCSUpK4uZNVQWhiYkJmZmZVNfUEnv8OC2trbz11ttYWlr+CxDYgCD59u0HYKIovq1ennse1WpXhiAI6Y/Tdzy595l8/bz7Gzn135Vv/00M/e9iN27cQENDg6ioaAwNDcnLz2fv3r0MDAzQ29vL/2XvzeOiuPL1/3c1zdLQDbILKi6AG5sCoqLgxqK4RMB9XxKzzpi52e7M3JnJZHLvTSaT+SWZJCYqRkUBBTcQEFBAFkUWN1ZZRHbZRKDZ6a7vH91h5js3iSZ3zC/L98nrvCSn6lRXV9epOudzns/zTJw0if98+23mzp1LfX09RkZG3LhxE6lUSl9fP5dSUzE1NaW6upqrV68yecoUIiMi0NHR4dLFi0yfPp0TJ04gqtWUlJZyPi4OlVYs4tLFizg7O9Pc3ExLczONTU1IpToIggS5XE5eXi4pKSnExZ3Hy8trxH0mPS0NDw8Pent7UavV6GgVawYGBhgeHqKnR0lGRgYtLa0UFBQwPKxCKtWlsrIKQYCj4ceYPduLe/dqcHZyYvfu3ZTfuUNeXh63b93C0tKS6upqpFIpEcePM3/+fA4fPsKdO3eQy+UcPnwYhULBH//4R2bNmkVMzCnOnDnDlq1bSEpKQqVScy42lkULFxIefgyVSk1h4Q0OH5Ywzs6OcXbjkRkYsHGTRj2o+u5dlMoedHQkPMnMWs3t+OPo1N8CLcB9oB14rCXCnz0Nd/HixZSWlREUFIRER4fomBjKysqor6/HUCajpLQUURQRRTX29pOYP38+ERERLF26lIMHw9i3bx/paWksX76cvr4+urq62LhpE2lpabz40ksU5Oezbt06RFHk9u3b+Pn7M3r0aJKSLrBt21ZaW9vYvGULn3/++UgOu42NDYmJFxg3bhwrV64kP78AA5mMmTNnIkgkXMvJQRAE7ty5Q+fDh9y4fp3+gQE6u7ooKirGzMyM8ePH4+LiQnNzM6ampvhrpbSPHDnClClT2LPnWWJiYhg/fjx3795lytSpXM3JYcaMGaxZo5kxZVy+jJ2dHU8/vZvjx4+zd+9eqqoqsbKywtHRkYqKCoyM5Pj7+5GRkYGv7wIMDQ0pLipi586dtLS0sHnzZk6fPs3AwAA7du5EqVQyODBAT08PbW1txMfH8+qrr3D+fDxf/Y74F+NH8iZ/FLQc/PWAJZpl62e03JVH4mff6QF27tzJvn37GD16NG+//TZ5ubkEBQURHx+Pg709ji++yGhrK3R0dAgLC+POnXJaW9t4/oUX0NPT435zMxcSE5k1axZtbW1ERUVhZmaGp6cno0ePJjkpicGhIfbu3UtqaioqlYqZM2fg7OxMeno6h8LCWLjAl6lTp7J//36eeeYZ2tvbCQxcyq1bt5gzdy76+vrU1dfj6enJjJkzedjRwaJFizh+/Dgvv/wyubm5NLc08/LLe0lOTsba2pozZ84SHBJCamoqNffukZCYyNq1mtjS5cuXsbWxwdfXl9OnTzM0NIS9vT31dXV0d3dz+/Zt1FrTyo8//pgNGzYwerQ1o0dbEx0djbW1NV5eXoiiSH19Pf7+fiTEa3Lrc/Py2LlzJ/n5+WRkZKBWqfD19aW4uJicnJwRi+qoyEgszM1RKBRIJJLvZU7/E3rTj0ezInbz2zZ8YjTcHyr+mYYbExNDs1avLTU1FT8/P+6Ul6Ojo8PiRYvw8PRET0+PqMgIVCoVhkZyruXkoKMjYcaMmRgYGCCKIklJSSxYuBCZgQFxcXEEBQVhZGTE2HHjKCstpbmlhfnz5vHZZ58xZsyYkTTXwcFBrl27hp/fEgwNjdDX1+PmzZsEBQXh5OTE3r0v8+Yf/8ioUaM4e/YsKpWKadOm0d/fT0JCAqamptja2NCtVHKnrAxXV1fkciOuX7+BwthYw77TlZKUlMzadesYNWoULi4uvPXHP/LeX/6Crq4uYdpkl127d9PZ2cnRo0eRyWRYW1tTW1urYdDNdMfefhLOzs4cPnyYoaEhduzYga6uLm+++UfUahUWFhYoe3pxdHSkq6sLA3194s6fZ/Xq1fj7+3Pp0iWkUimLFy/m3NmzJCcnExgYiEqtQlSr+bd/e4Xh4eEnRsN1nzFdzL547JH7GVp6/K8/6/uAIAjzAUdRFL8QNIaxci2x7Rvxs3/T6+npsWnTJuJiY/nFL35BV3c3mzZv5m9/+xvKnh7i4+PJzspCFEU+/OgjdHR06O/rRV9fn01ammleXh6TJ0/Gz88PGxsburq7MbewwM/Pj/Lycnp7eykqKsLf3x8/Pz+6u7vZsmUzgiCw77PP8PDwICQkBKlUSkZGBjdv3mL69OlUVFTy4EE7yUlJI0YTZ06fRn/DBvr6+rhbVcUvfvES7u7uVFZW4ubqwowZM4iIiKCoqIjf/ObXuLm5kZKSwpo1axgzZgyTJ08m6cIFHnZ2EqFNBS64fh1jhYJj4eH09PZyJTubDRs3snLlSsrKylAND+Hs7ExRUTGHDn1BX18fxcXFyOWn6OlRIpPJmDJlCpZWVkRERLBnzx4sLCwA6Ovrw9bGhtRLlzh39iwLFy7k1q1brN+wgZaWFpxdXHB3d6e2thapVPpkabgIIPw0bnlBEP4AeAJTgC/QyF8fQxNA/0b8ZMY63xVGRkY0Nzcjl8uZ5eVFvdZrburUqQQFBdHR8QBPTw+ee/55EhI0sURDQyOGh4dHVGOKS0p4/Y03OHf2LKIoYmtjQ21NDX19fUyePJlRpqbMmjULhVyOhYUFixcvJiMjg7i48/j7BxC4dCk3btzk5s2btLa2smXLZtzd3Wlvb+O9995jYKCf4ODVODg4MHnyZHx9fejq6uTAgf00NDSQkZHB1atXMTc3Z9++z1iwYAGbN28mJycHgMbGRoKDV1NRUUFvby+dnZ288sorTJs+nW3btzN37ly8Zs9mvo+PJmcgIoLuri6uXLlCd3c3MpkMtVpNYWEhMpkBzc33Wbx4EZs2bUSlUvHSSy8yPDxMZUUF27dvp7KyEoCbN28yZ+5cmu7f56nVq7WZfMP4+PhgYGCAg6Mjd+5opNuTLiQ+eQouoLnlH1V+FAgGVgE9MMIQVDxOwx/NN3ySSExMHPGj27ptG3FxcQwODrLv00/ZumULalHE2dkZmYGBVgTCkHXr1pGZmcn58+dZvHgJACGhoURERCBXKNi2fTtxsRo668DAAE8//TTvvfcec7296R8Y4NTp01y7do3+/n6kUilxcbG0trYSGhrKkiVL+Pzzz/HROsm6uc0gMzOT3NxcnnvuWW7duo2VlTVSqZSVK1diYGDA+fPnycnJ4bnnnsXa2hpDQxnBwcHExZ3HxMQEgNCQYP7z7beZMGECLi4ulBQXa1KLTUwICQnhb3/7G7U1NRw6dIi+vj6SkpL49JNPiIo6QWxsLEuXBiIIEp577jnUapHIyChWrFiBXC5H2dODjlSKl5cXJcXFdHd3U5Cfj7OzM0qlkqNHj7Ji5UrWrltHdLQmz0EqlYIoEhUVSUBAwPczp/+JLNkBg1quiggjLMHHwk9jrPO/QFJSEuPGjSMxMXGk7l51NYWFhfz+979DoVBgZKi5nu4eHrz5hz/g5eWFVCqlra0NiY4OVlZW3Lx5k6amJi6mpDBv/nyMDA3R1dXlyJEjzHBzI/bcOTo7O8nKysLW1pZJk+yRy+UIgkBZWRlVVXfxmj2HpOQU1Gq1VpYql/z8AnR0dIiPj8fLaxaiKHL69GleeulFYmPj6O7uprm5GUdHR+7dqyEuLg6ZTIajoyM3b94kOTkJMzMzuru7EUURa2trMjOslOggAAAgAElEQVQzedChYQ9+8MEHzPL05ItDh5AbGeHj64ufnx8AKpWKf3/jDYyNFSiVPeTm5jFt2tSRB8LUqVNpaGjQav6Z09fXD8COnTs5Fh6OqZkZDQ0NSCQS8vPyMDDQZC46OjiQmZmJrq7uP5hnPnn5aw1+NJ36UTgpCMLnaFipz6Chsh94nIY/+07v5eVFV3c3QUFBI3V1dXWEhIQgiiJnzpwhNzcXudwIE60hhSiKhIeHk5GRwYSJk4iKjMR73jwWLFjA7Vu3mOftja6eHvUNDdy8cQO5kSEhISE0t7RgYmKCs7MzxcXFmBgbY2BgwMDAAG//53+Sc/XqSOpsZUU5bm6uuLq6Mjw8zP37TXh7e5OTcw2lUkl1dTUrVixHIpHw+ef7+dWvXubUqdMEBASwd+/LTJgwga1bt1BXV4eOjg7btm3j5MlonnnmaS5fvsx6rdJNQX4+c+fOZeeuXcRER9PU+Hcmc9jBg7zy6qtUlN/B2NiYDz78iNDQUMaNG8f4CRO0dlRW1Nc3UFNbx80bNzCSy1Gr1RQUFGBoqGEwimo1Lq6uBAQEUH7nDu3t7WRkZCAC9pM0wcHAwACkUimDg98oh/C/w5fknJ8ARFH8iyAI/kAXmnn970VRTHlEM+AxHnuCIHgKgvArQRDeEwThLUEQ1mllgX4SMNWmzl7J1siLZWVlMX+eN0qlEmdnZzo7uzTyVvb26OjosHz5cszNzXnqqacIXbOWMba2rA4OxtHRkWPh4ezctYsHHR3o6+sjNzJi+/btODs7U1ZWhre3N5UVFXR1dWFpacnSZctIvXSJ9rY2JkyYgImJCVVVVWRmZrJmzRpycnJQqVQcOXKUPXv20NzcwoMH7SxfsYKpU6cikUiIjIxi9erV2lTWB0ilUvz8luDs7ExjYyMKhYKZM2dy+fJl9PX1GD16NEplD4ODgwiChN/89rfcvHEDpVKJyahRmJmb09DQwNkzZ1ji58dorUBIbm4en+37lJp795gyZQoODg7IDA1RqVR4zpqFvr4+L730EjKZDJlMxrbt25k1axZLly5FoVCwZ88eLly4gPe8eSwLCmL69Om4urpiaGTEc88/T2Lihe9xye4nMadHFMUUURRfE0Xx1cft8PAN31AQhB2CIFwHfg3IgDto2D/zgRRBEI48rjzPDx2enp7cb26msrKS6rt3cXZ2ZtasWRQXF2NoKGPVqpWkp6dTWnYHFxcXBgYGiIk5xbJly9i5axcRx49z8eJFFixYgIWFBcXFxVy9ks3OnTtYsMCXnJwcrl69ipOT0wijzdvbm4aGBurr67lTXk5tbS3LgoK4dPEitbU1jB49mg0bNhAWFsaECRO0ySxdmJqZa73qsigvL8fGZjTW1hoiVnBwMO+88w5z5sxBJpNx/nw8JSWlVN29S1jYIURRpKSklODg1URGRmIklzN9+nT8/P3ZvXu3pgN7enL8+HHMLSywt7cHIDU1ldDQEAwMDHjuuWcJO3gQtUrFihUrSE9Lo7u7G2NjY1zd3MjOyiInJwcPDw86OzvJy8vD2dkZiUTC1KlTuX79OofCwli/YQPdXV0UFRZy7949xowd+z10ehAFnUeWHzIEQegWBKHrK0q3IAhdj3WMr4uYCoLwInBIFMW+r9k+AzAXRfHSd/4G/z/gn9fpo06c0Eg0qdVER0fz1FNPYWtrg7OzM3/5y/v4+fnR1dVJTs41ent7cXNzo6amhtmzZ6NUKunt7aWjo4Py8nICtKy3hIQEgoOD0dXVRaGQ09nZSUlJKaFr1lBTU0NkZCR+S5YwZuxYCm/fxsLCAhMTYxoaGhkYHKSyogIXF2cQBBLi4wkICGTUqFFERUXh6+uLsbExlZWVdHR0sGLFCoaHhxgeVjE8PERERCTBwcH4+vpoYxUXNK45R4/yq5f3jvDsL11KJTAwkKHhYSZOnEhpaSmjTIwxMzPj2LHjLFy0CEEQaG1poaWlheXLg1CrNczEzs5Orly5iq/W36+kpITBgQGsra1pbWtDbmSEvoEBerq6NDQ28vrrr1NQUEDh7dtcSEpi6pQpzNbaeeVcvcqUqVOpr6tj+/btDA4OPrl1+pmuYlZ63CP3Mxo14UexTv9d8bVzelEUP/mmht+FCfRDhKWFBXPmzuXsmTNs27YNG1tbHB0duZCYSGNjI6amoxgeHmbrtm1cu3aNrVu38vLLe2ltbWXt2jXo6+tz6NAX/OIXv6CxsRE/f39uFxYy3cmJSZMm8fDhQ86dO0djYyMN9XW0tbYybepU1qxdS3V19YjRRmioJnmmrKyM27duERK6hpKSEuztHVAqlSxYsICqu3exd3DAx8eHy5cvU1RYiJ/fkhGn18OHD+Pr60tPT8/I3FilVpOWlsZvf/tb0tPTCAwIoKWlhf/43e/Izsri6WeeoaWlBalUSnt7OzNmzKCtrY1xduNZtGgRh8IOYm1thZ+f38jn7Nu3j9mzZxMaGsrg4CDJyUnYT7LXpOlevEhXVxebtCm7ycnJxMXG4uTszIaNG6msrGTChAkou7uxsbEZ0Rm4euXK/wvkfUv8EznHAlA8Djnnceb0EwVB+KsgCKcFQYj9svwrTvqHgqysLGxsbQlavpy7d+8il8tpf/CAefPmIZMZAuDs7Mz69eu5ePEivr6+mJqa0tDQwIEDB9m5cwcuLs4YGRmSkpJCYGAgSUlJDA4OMmrUKFTDw3h5zcLe3h4DA33MzEzp6+sj9dIl5s2fr02UGebhw4fk5eXhOcsTiURCcVERc+fOpbm5mWPHjvHCCy9gYGDA7Vu3uN/UxNRp02hq0hiepKWl4e7uzvLly/HwcOfmzVvk5uZSUV6Oq5sbenp6dHVqRn9tbe1Mnz6dp556ioiICOLi4pg/fz4hIRqtPFtbW2prajh79iyBgYH4+/uPuOKGhx8jJCR0xHPuzOnT7P3lL7G1teHjjz/Gz8+P/v5+hoaGqKqq4vCRIzTdv4+BgQHHjx/nN7/9LXZ2dgSHhHD0yBGqq6v55OOPWbN27f9bsvsW0JJz3kAz/QbQQ0POeSQeJ3p/Fk3KahwaVdufFBoaG9HX0xtRgO3o6CAmOpqlS5eSe+0aH3z4IR9++CEAcrmc+ro6TE1HsWjRQp599llmz57DuXPnEAQBQZCQlHQBtxkzCQoK4sSJE+hKpaxYsYKkpAvExsayZ88eOjo6CA8PZ9asWQiCwLz587l16xa5uXk8++weWlvb+OSTTwgODgagv69Po7JTWopUKiXl4kX09fXZsXMnUZGR+Pv70aHl4gOcOxdLcPBqPvzwQ65dyx35bp1dXZw/fx7fBQsA6Ontpa62lpbWVk7FxDCsUlFaWsr9+/fR19ejpqaGVStXoKOjQ25uLsPDw0yfPh1raytUKhUXLlzAwcGevLx8ent7KS4qIjw8nDmzZ/PJJ5+wbOlS+vv7mTFjBu+99x7GxsaUFBfT2NjIyZMnMTAwYOw4jWdebm7u90jO+UkgGI0E3Zc6lI1aAdpH4nE6fb8oih/9L07uB4171dW0P3jAw4cPkerq0tjQQFlpKYZGRoyfMAEDfT2iT55AYWxMb08vyp4ebt++ja6uHkuXLsPAQH9ESRc0D42JEydy584diouKGBoaQl9fj7S0NKZNdyLqxAnUKjVZWVno6elx48YNRLWaixcvsmHD+pEodvmdO9y8eVOT8dfQgLmZGZaWlpiYmFBz7x5371bxxaFDVFVVkZ2dzapVKzl/Pp6hoUEuX76MVKqDm5sbIJCXm8uN69epr68nLy+P9vYHKIyNGTd2LDY2NowdO5b169dx48YNJowfT03NPZYsWcK1a7l8cfgwxgpjcnNzsbS0ZN48b5KTk6mtraGqqor58+exePEikpKS2LhxI2PGjqXjwQOKi4owHTUK3YIC/Pz8cHF2Zuy4cdjY2nLgwAH++NZb2Nra0tzcrFkRmDoVHR2dJ66G+1Oh4aIl52jVeP/l5JwPtUOJZGDE//pLpdsfO+bNm0dPTw8ymQzvefMICwtjvI4Onh4e1NTWsnfvXi5fvsxsLy9sbW0pKCigR6lk1ixPsrOvIJcbUVdXx7hx40hLS8N3wQIqKipYvnw5tTU1GBkZERISwvCwira2NtatW09fXx/9/f1IBIFtO3YQGxuLh4cHixcvxtjYmOzsbHx9fbCzs8PT05POzk4G+vsZN24ct2/dYsKECfT397Njx3ZOnoymoqICf39/JBIJTU1N1NXV4eDgQFZWNr/61cvs++xznnnmGXRjY5EZ6OPk7IyHhwf9/f3U19fT2dnJ4OAgt28Xsn37Nurq6sjLy8PVzY3x48eTkpxMc3MzFhbmuLi4YGJiQm1tHXp6egQFBaFWq9HV1SUwMIDw8GNs2ryZtPR0vOfNw9HRkcHBQaytrRkaGuLatWv813//NydPnODZ556jvb0duULBiaio7+cH/5EM3x8D35mc8zhXwAWNOcU7aEQs3wf+8o0t0JhdCIKQJghCqSAIxYIg7NXWmwmCkCIIQoX2X1NtvSAIwkeCIFRqlXLd/+FY27X7VwiCsP0f6j20KjyV2rbfKRLk57eEgYEBsrKykMvlbN+xg5iYGO5VV2NmZsauXbtITk6mvr6eW7du8/vf/46EBA2Db/ny5aSnXwagrr6eyZMnMzw0xIXERBZr7aTPnj2L56xZrN+wgTNnznD69Gk2bNzI6uBg3n3nHZTd3bzyyr9x5swZAMrLK9iyZQt3q6qIi43Fzc0Nx8mTKS0t5fr1AubN88bPbwnp6ekYGRmxe7fm/ADi4xN46aWXiI9PYOpUjc/Cxg3riYuNpbe3lzfeeAPV8BDHwsM5/MUXBAUtQyYz4JNPPmXDhvUArFu3lgsXkjA2Nuazzz5j/Pjx+Pn7s2DhIoqLi+np6WHMmDHY2tpSUVHB8ePHR1YubG1tOHvmDOvXryc9PR1RFImMiMDf34+hIY3VlqWlJdt37CDi+HEePHhAVWUlnp6eP6p1ekEQlgqCcEd77/3LnWweBa1vRAwaDcovyTl/e5y2j/OmDwYmPco55ivwdWYXO9BY/7yjvVj/jiYgsQyNLp4jMBuNacVsLRHoy4wiUXucWFHjEbYPjUBgDhplnaVofL4eG01NjYSFHUIqlXK7sBAjQ0Pi4+OZOHEix44dG4lqGxjo8+qrr+HjM59jx47R2trKnTt3NKYQgsCnn37KipWrAFAqlfT192Nvb4++vj5/eustjE1GYWRoqBG9uHED1fAwxiYm9PX1UVBQgJ6eHgqF5i0/erQ17e3tKBQKjh8/zvLlQQwNDfPJhUR8fHw4eFCjCpaens5///d/MWbMGHJz86iursbc3Izc3Fxu3ryJIAi0tz9ApVKRkpKCnZ0dZ8+eRUdHilqt4tatm0RGRmnSZ1tbOXfuHLq6ekilOtTW1fLaa6+xcuVKyisqKCkuxsrKirtVVaSnX+bVV19BT0+PAwcOMkorzgng4+PD8y+8yKqnnkJfX5+0tDQMDQ2RyWQ0NTbh6uYGMJLgFBkZiY2NDXO9vb/l7fUd8S940wsadc2vM2D53qAl5HwlKUcQhKuiKM79qm2P0+lvAaP4lhreWqePL90+ugVB+NLs4ilgoXa3I0A6mk7/FHBUm0SQIwjCKK2I5kIg5UuRQe2DY6kgCOmAsSiKV7X1R9GYXXyrTm9jY0tISAhtbW3o6koxMTFh+XKN2KTGjVbF00/vpra2FmNjYwwNDfH39+eLL75g2vTpBAcHk5iYSGZmJmbmFuhIJJw/f54lS5Zw6NAhLC0sMDc3G2HQGRoZUVxSwvYdOxAEQRvpHmTZsqXk5eXxwQcfsnDhApTKHoKDV9Pa2oqZmTlLlwZSWFjImjVrsLKyQq1WU1NTw4ULF5BIdDAyMuTf//3XzJ7thZOTEytXrqCtvZ2AwED09fXp6u5GRyJh9WqNH8iBAwdYsGAhS5cGcvr0GRYs8MXIyGjkjRufkMif//xnFAoFaWlpKIyNCQgIIC01lePHj5OamgoI3LhxAysrK4yNFQiCBD09PXp7ekhJTqZ/YIDYc+dwdnYmIiKC7OwsFMYKWpqbESQCEkFCaUkJBvr6REZEjCwJPjn8y0Q0RgxYAARBiEJz/36vnf4RMPi6DY9zBayBMkEQkr7rkp3wf5td/F/WP/xd1+vbml2M0f79z/Vf9fnfaHYxODhIdHQMGzdqUkV7e3s5e/Ysf/rTW0i0DjRJScmsWrWK+/fvaz3fl6JSDTM4OEjNvXts274dV1dXlvj5sXXbNgYHB9mxYwcdHR28+eabXLiQiLW1NaUlJezcuZPMjAwAjBUKtm7dRlKSRq121apVGBnJWbt2DXV1dTg5TaehoZ7+/n58fX1HhvEpKSk8++we1GqRTZs2MnfuXFauXIGLi4uGIiszZPeuXURFRY2YdJqbm3PvXg05OTnMnj2b9es1mYL6+vosWLCA6up73Lt3j4iISF599VUKb99GrVbT0NCAp6cnTU1N3L17l1dffRVzc82DyNfXFzu7ccydO5eAAH/Mzc0JCAxkkr09Pj4+rFy5EktLCzZt2sSqVavQkUhYsyaU0JAQJk2axHPPP89cb2+MjIy+F0beYy7ZWXx5v2jLnn86ytfdkz8kfO1SyON0+j+gGeL/F3+f07//uJ8s/JPZxTft+hV132Rq8a3MLr60KLK0tPwf2/fvP8CuXTsRBIHe3l6++OIwu3fvprKykp6eHv7whzdRq1UkJSVTWlpGXV0dNjY2DA8Ps//zz9m5axfe3t7k5+VxKiaGwMBAtmzdyhdffIGevh4GBgbM8pzFlStX6B8YwNnZmfKKCjIzM5nu5IREImFgYJCOjg5MTU2ZOXMm2dlXuHQplZkzZ7J161bi4+PR1ZUybtw4ysru0NR0n7Fjx7J7t6ZjX7iQxObNm6mtrSMjI4Pp06ehp6fHDDdX/vLee8zy8mLpsmVkZmVSXl6Oq6srEomE1tY2DA01XIQ1a0IJDz+Gjo4O9vb2dHR0cOTIEZ566imWLFlCeHg48+fPw8lpOiUlpZpgn6sLO3bsICbmFKARFNm2bRt3q6qIiY5mdXAwLq6uxMTE4OjoiKOjI/n5+QwPD1Nw/TpLliwhOSmJoOXLv5clO5X46MKjba0e+977IeJrh/eCIAiiBpcftc83bP8fZhdorX/Evzt7fjlt+Dqzi3r+Ph34sj5dWz/2K/b/VkhOTsZu/HjOxWromXFx5wkJCebixYvY2Nji5jaDyspKQkJCsLKyoq6ulrr6BsLCDnHp0iUWLVpETPRJ1GqR0tJSevv6OBEVpckYGxigorwcAU1OfWFhIXZ243n48CH+/v4c2L+ft/9TI/67cdMmfvub3/DSSy8yduxYwsOPIZFINFTY1lZKS0uxsrLi6aef5osvvsDMzExjknH3LjnXrmFrY8upU6ewsrLi6NFwFi1aREZGJjKZATdu3MB6tDW6Ul0yMzKxs7Pj5MlohoeHaW1t4datW4CITCZjxYrlxJw6zbmzZ6mpqWHylClcuHCB3p4equ9WUVFRyf379zE3NyMtLY3XX38dgJkzZ5CQkMCEiRNRq9WcOnWKhQsXciExkaHhYaKjY/Dz80MQoLm5hdi481hbWRF98gQSiYQU7QjmSUIE1P+avvlNxiw/eHzTnD5NEIRTwDlRFGu/rBQ0hhPz0ZhLpAGHv6qxNpL+P8wu+Hrrn1g0HndRaAJ5ndoHQxLwX8LfPe8CgF+LovhAm2QwB820YRvwWNHLf4STszO22kDS3bt3GWViTHd3N0uXLmV4eJjDhw/z61//muzsbGbOnMnYseM0nWPlKqRSKTKZbMRF5tixYzx82MmyoCBMTU05dOgQzi7ObNy4EbVazQcffMDAQD+lJcUolUrq6uo4FBaGjo4EURSpqa0lNzeP24VF9A8MUFpSwqXUNEaZmKCjI6Wzs4uUlBRKSkrR19dnz55nsLbWKNNWVVURumYtQ0NDnD17htDQEAwNDYmMjOKNN16nv7+fuXM1cZ2BgYH/65y7u5Ujue6CIHD/fjM+vr7s3rWLMWPG4O/vT0tLC8PDQ0yaNBFHR0eSk5MpLCxi//4DyGQydHQkxMVpYhl3q6owNjHB2UUj3zU8PMzgQD86OjoEBwdz7do1Dh8+wgvPP8fo0aORGRrR1NjwPczp4V80mBgxYAEa0BiwbPqXHPlfh3Fft+GbrvJSND7xkYIgNAqCUCIIwl2gAo0l7v8niuLhb2j/dWYX7wD+giBUoIl+vqPdPwG4i8at9gDwAoA2gPcnNBc6D3jrH5xDngcOattU8S2DeABjtMtOAGmpqfj7+2NqakpRURGHDh1i06ZNGBgYoFQqiYk5RUCAP5s2bSIhIQFbWxtsbW24fl1DWRAEgRdeeJ7ICI1FtUIuRxAkKJVKPvnkU/bseRZzcws8PDxQKBTsffllLCzM2bFjB729ffz1r39FrlCwbNkyRLWaadOns3jxYhAE/AMCsLCwYGBggM2bN2tkuEaNIjv7CvPmz2fylCmUlJQQGRHB1q1bqamppaysDBub0cycOZO6unoePnyIjY0N7u7u5OcXAKCjI8XJyQknJycOHjxIb28vg0NDREdH88mnn3L//n0UCgWXL19m9+7dXLlyBT09PQwNDfnT228zMDjIuvXrme/jy4YNG5g4cSIKhYLf/va3lJeXU11dTVRU1Ijr7sOHDykpKeXjj/9GcnIKhYWFXMnOZunSpU98eP/lm/5R5ZHH+WYDlh8K6r9uw9d2elEU+0VR/FQUxXlo5HaXAO6iKI4XRfGZRyXciKKYJYqiIIqiqyiKM7Ql4eusf7RTiRdFUbQXRdFFFMX8fzjWIVEUHbTli3+ozxdF0Vnb5qVvmmp8E+zGjyc/Px+78XYIgoCxsTHvv/9XDbW0uISenh7u3bvH5MmOtLa2kZWVRUJ8PKWlZXh6enL7diFtbW0YGxsjkUjYtWsnb7/9NgCjR4/mV7/6N7Zu3YJcbsTGjRtISUmhrOwO7u7uDA4O8Ze/vM/6DRsYPXo0rS0tJCYksHjJEgIDA7mWk0NJcTH6enpkZ2eTl5dPqVaL/xe/+CUmo0ZxV5sOnHH5MmZmpixcuJDy8jtkZWWzcOFCAJTKbqKjo/Hxmc+MGW5cv17A3bt3mWRvT//AALa2tuzcuZPw8HAyMzLw9PTE3Nycbdu3ExkRgemoUQiCwNq1a0lISKCuvoHx48ezZ88ewsLCSExMZOWqVdTW1TGkte9at24dcXFxtLa0YGxszIYNG0hKSkIuN0IqleLm5sq+fZ8hiiItLS3fSyBP/RjlcaC9lydr773/YdDyA8DX9oXH4iSKojiEdvntp4bW1lZ6e3vZf+4cQUHLiI2NZfLkKcyY4cacOXNGrJ9yc/MYHByit7cXb29v/P396O5WjkS/f/Wrf8PJ2YnubiVGRkbcKStjyeJFdHZ2Ymdnx6lTpzGQyTBWyLl9uxCpVMrpU6e4fv06PT1KEhISkMvlFBUVaf3yLOjs6iL86FH8AwJQqVTMmzcPAwN9Nm7cCMDt27fwcJ9Jc3MzudeukZubS1+fC9HRMZw5cxpvbw3DUKVS09vbS1VVJRcvXsTY2JgpU6bwwQcfsOfZ57C0tOTPf36PcePGYmlpxcVLqWRmZJCfn49UR4cbN24gkWiWFwcG+mltbcNk1CjS09Lo7Oqiq6uLjgcPiIqKoqiwkIcPOzTptfr6zJ0zh6ysLBITL9Df38/Vq1eRyQzpVvYwe/ZsZs+ejVxuhKmpxjpLpVI9wV9bRP0zk3z/KvxkiMjfFZaWltjY2lJXX8/MmTNxdnYmLi6OtWvXcvWqZmlLJpPx7rvvcOFCEkFBQfT19WFlZY2zswtqtYolS5bQ0NjIZEdHVq5aRXJSEtt37MDCwoKamhomTZrIylVPoVBo8iFS09KZ5enJ5MmTsbS0oLy8nE0bN9De3k5dbS0IAnbjx9Pd3c24ceNYvny51jrrLiqVipaWFmQyGcuWLaOoqJiVK1dQWlbGn997j9TUVNasXUt7ezvW1lYjSTsnTpykW6lkxoyZ9A8M0NzcjFLZgyiKPHz4EAOZAdu2baO3txeZoSHd3d2sXbuWBw8eYCCT0fHgARs2rEdHR4fIyEgKCq6zefNmjI2NedDejpurK+4eHkh1JHR0PKStrY1Zszxxc3Ojrq6WoKBlnDhxkrVr143Ik0VEaIhH586dY9KkiU/8txZFUP18Ov3XslN/MkTk/w2Sk5N55ZVXyM6+wuDgIN3dSmxtbenq6qS6uhpjY2PGjh2Lt/dcsrKyOXHiJAsXLsDDw53btwv59NNPefbZZ5Ho6HBH680eGBhITk4OU6dOZe3atWRmZgJw/PhxXn31VYyMjCgoyMfX15eQkBA+++xzMjIy+eUvf4G5mRnW1takpaXx1p/+RGpqKsVFRdjb2xMaGkp6ejqnT5/Gx8eHjo4HnDsXi7f3PCwsLOjp6aGiogJ395nY2NiQlZVNU1MTCoWCp3fvorCoiAkTJnDr5k3+9PbbtLW1IarVBAZozvfcuVjmzZuHVCrVZMOdOEFISAgbtXGML51oAgMDaG9vJycnh1leXgwPD3MqJoa1a9diZGTI00/vHgmEVlXd5dNP9zFv/nzm+/iMyHAr5AosLCxYsGABZ8+eQxTFJx7M+1fM6X8oEARhvCAIftq/Zf+UZbf169o9Tj79S/8QOf/JITs7G4kgkJGRwZSpU3nttdeZOHECdXV1yOUKPv74E+zs7GhtbWXy5MmUl99BqVRy8uRJwsOPUV1dTXX1Pc6eOUOPUsmf3noLXV1dqqurqaioZNasWejp6dHe3kZCQgIzZsxAFEViYmIAgejoGLKzs8nNzQVEqqqq0NfXJzY2Fh8fHyQSiVZFNxMXF5cRFp9aLdLY2MTVqzmkpaVx+fJl0tLSWL16NZ9+8gkeHh64urpSVFTIX//6VxYtWoiNjcn6r+gAACAASURBVA319XVkZmbi6emJra0tGZcv4+DoiJOzM7du3WZgYACFQoGjgwOvv/46ZmZm1NfXI5PJ6Orq5uDBMJYtW0ZgYCAZly9zp6wMBwcHYmNj2bp1izbFWECpVOLk5IRKpabg+nXa2tpITk6mt7eX3t5eTkRFsXy5RozUwcGBlJSU7yeQJ4qPLD8GaJNsYoDPtVVj0aTBAyCKYtFXtYPHG96PRsMtvg4cApK+a8DshwiNE4uIg/0khoaGNKYNlZUMDg5RU3MPlVpNV7eSjoed9PQUcf9+M2q1mt1PP01rayujRo2io6ODLVu30tDQQEtLC9OnT6e1pZnq6mqOHg3H0MiQG9dvoFKpmDFjBrZjxuDgYM+sWZ44OTkB0NjYxOjRNjQ1NdHd3U32hQv4+vpy7VoOquFhbt68xbFjx5FKdejvH6C8/A4uLs64u89EpVKxa+cOGhsbuXXzBh0dHYSFHcLQUEZXVxdSqS4nTpwABAz09Tl65AhBQUHcb26mo6ODyIgIyu/cQU9fX0MnNjPD3sEBRwcHJk+ZQnV1NRdTUmhsbKS9vR0jI0MkEgkFBQUYGyv487vvYGBgQEJCAjo6Unp7e3n33T/j7uHBps2bMTc3p+n+fdatW0dWVhZXr17F2sqK2NhYVCoVg4NDdHZ2IpFInnjH/wkJQryIhg58DUAUxQpBEB7LtfaRb3pRFP8DTRJMGJpkmQpBEP5LEAT773y6PyBMmTKFyZMnU1NTw5gxY1ixYgWDg0MsWOCLtbU1f373HQry8/Hy8tJQTsePx87ODj09PRITE1m+YgVSqZSuri4S4uN58aWXKC4pZnh4mHfe+W96e3tZuHAR052ccHFxYeeuXUyYMIHVq1eTk3ONBw80q4/jx9tRWlqCt7c3Bgb6LFu2TMN2274dX18fnJ2dmD59mjaIJzJtuhPTp0/HZJQpa9et5/z5eOzs7Jg5cyYhoaGMGWPLjBkzmTNnDr/73X9gaGjEjh3bkehIWb5iBfYODujq6vLCiy+yZs0anF1c0NfX5/XXX6OpqYmJEyfi6enJrZs3WbBgAaNtbFi8eDFubm5s3LiR0NBQZs+Zzfz585kwYSIffPghHR0PCQ0NQV/fADMzM1atWkXutWu4urmxZcsWEhMSCAgIwN3dnYmTJrE6OITQNWuRGRqycuUKgCc6vBcfY2j/IxreD/xjEpwgCFIekxX4WFdY+2a/ry3DgCkQIwjCn7/9uf7w4O3tTW9vL++88y7e3nPx8ZnPmTNnmTBhInp6eqxZE0pkRASHDx8mNDQUuVzOhx9+yMaNGxEEgeCQEA4eOMDsOXMQBIGHHQ8pL69g4sSJPP/8c5w+dQqJILBw0SLS09PJzsrC2dmZnTt3cOLESdRqNRKJhHXr1nHq1GnkcgVr1oRy92415eXlZGVl8+KLL1JbW0dU1Almec1my5YthIeHM27cOMzMzNDV06OqqoozZ84QGBhIU1MTp07F4O3tjaGhIQqFgvz8fORyuUZNNyODrKwsysrKqKmtJSIiguvXC6ipqWHs2LG89uqrZGVl0dzczLvvvIOdnR3e8+YxZepUKioqOHzkCCEhoeTn5+M1ezYSiQT/gADefPNNXFxd2bR5MynJydy9e5eJEycil8sxMTGh/M4dFHI5Tk5OXC8ooKurCwGRdevWoVY/ecMLlSg+svxIcFkQhN8AMkGjfx+NRt3qkXjk8F4QhF+iYc61oSHCvCaK4pAgCBI0RJ3Xv/Np/wDQ2trCiRMn0dPTpbKykvDwY5iamhIXF8fq1as5deoUEomE6upqjZRWTAw1NTU0NDRw7uxZJBIJOlIpZWVlODg60tTUiJ2dHZcvp3Pw4EEMDQ2xtLQgNTUNuUJBXW0tpmam6OhopJbXrl3DwYNhuLq6UFhYxJkzZ1iyZDEREZEMDg6yf/9+BEEgLu48vb09xCckEtDfT0lxMWlp6QwODlFUVMSMGTNISk5BVyrl6NGjlJaWYmFuTlr6ZQYGBhgcGODDDz8iMDCQw19oFHfGjx/PnDlzUCgUDGmFLgICAujv76exsQETk1EEBAby/vvvc+P69RE+QOy5s9iNn0BKSgoVFZWUl5dTU1ODvr4+FZUa6Stzc3Nu3bzJdO30BcA/IIDPPvsMWxsbPD092f/552RlZ7NieZA2a09rdfWE8OWc/ieCfwd2A4XAs2jIbQcfp+HjXGELIEQUxZp/rBRFUS0IwopveaI/OFhaWjF37hzCwsL45JOPOXPmDJs3b6KvrxcLC/MRi6eOh5r19i1btnDo0CE83N1xcHTEwcGB3NxcjUrt/HmMGjWKw4cPAwK7du0aMaTwmj2bdevWcfLECVJTL2FuZqalhIpcvXqVtrZWLCws+fN775GSksymTZq1+LCwMKysrFi5ciUff/Ip7733HuXl5SxcuBC1KNLf38+uXbuoqqpCVyqlqqqKP739NjExMfT39WmnCwZ8+umneHt7s2XLZpRKJebmFrS2tqJQKGhvb2fChAl0d3dpP/MQzzzzNCe08uALFy5k8eLFqNVq3n33XW5cv86YseNYvXo1HQ8eIBEEAgMDaWlpYevWrehKpSzx86O1pYVrOTmYm5sDoFaruXfv3sgDQiKRcKesFHd3dx486NA8QHWerO78T2hOL0MjUX8ARnL8ZUDvoxo+zpz+9//c4f9hW+m3PNEfJC5fvoyXlxcKhYJJkyZx8+Ytxo4dy717NYiiSHl5OU5OTtjY2nLr1i3s7OxYumwZFy9eRK1WU1JSwmuvvUZGRiY1NTWMHm3Drl07yda65qjUqpGlrgcPHrDn2eewsbElOHg1Tk5OeHvP1dBjPTwYO3YsszxnkZ19hZycHGbOnMnw8DBHjhxh7dq1GqXaWk0qhFwuZ/GiRaSlpuLo6IhaFJnl5UXsuXN4aINoyUlJfP7552zZsgUDmYzBwUEiI6NYtmwpW7ZsJunCBQ0DcPEiBgYGOXz4CGvXrkFPT4+NGzfy2b59jBs3jhNRUcRER7Nt61Z27tpFlzbwZm5ujrKnh5bmZs7HxeHn50dzczM3btzA1c2NpcuWMWnSJPz9/enq7GTXzp1MmjgRP628l4eHJzIDA0bb2CCK4pPt9OJPJ3oPXELTyb+EDLj4OA1/9uv0SqWS5uYWXFxc6O/vZ8yYMbzzzjvY2tqyaNEirl69SmZmFl5eXixevJiPPvqIadOmMTQ0xJo1azgUFoaDvT0SiYTm5vukpKSwbNlSHBwcKCsro7OzEwsLSwYHBzly5AjrN2zA3d2d24WFPHz4kMzMLCZOnEh+wXXaWltJ1urRJSQkjKjcXLqUiqurG1ZWmuCso6Mjt2/fRi6XM8nengcPHnDjxg0c7O2ZOHEisbGx3Lxxg7jYWGJiYrCyssLExAQXFxeuXLmCgzaIJwgCycnJdHZ2kpSUzIULF6irqyM+Pp7Dh49w/Phx8vPziYyMZImfH+vWryctPR1fX198fH1JvXQJXV1dNmzYQEJiIgqFAqlUStP9+yTEx+Pu7k5gYCBpaWnEREfj4urK5ClTCA4JIT0tDZNRo5g6bRqHDx9m/PjxiKL4xIf34mP89yOBgSiKyi//R/u34eM0/Nkz8nJycpDJDAgLC0NPT49JkyYxffp0UlPTsLCwoKCgAAOZjIjjxzExMUFXV5e0tDSGhoZQq9VcSk1leHiY8vJy8vMLsLKyIioqCpnMkDFjxvLqq6+xadMmrl69yrixY4k/fx4AfT09nn/+BXx8fGhpaWHv3r04OjoCkBB/no6OBzhOnoKRXI5UKiUtLY2qqiqUSiVmZmaciIri9ddfJykpiYednbz//vssXLgQ4d49duzYwbBKhZ+fH42NjUilUsLCwrAbN46EhASWLVtKZGQUDx92MHXqVDw9PfD29qakpAR3Dw8WaCWye3t7KSwsws3NjfPnz+Po6KiV4GqnuKiIyxkZyI2MaH/wgJp79+jp7UWuUGBra8v1gnyiT55kaGiQ0pISBEFAoqNDZWUl+vr6JMTHY2ZmhiAI7H76aXKvXUMQBHR1dZ/o7/0TGt73CILg/qVArSAIHsBXulH9M372nd7Pz4+7d+/i5eWFo6MDhw8f4Zd795KYkDDiyDLX23tE+FEQBJru32f37t2o1WqMjY2RGRiwYuVKjIyMGB4eHuHGP3z4kOjok1hYWjJfq7q7ZctmBEHg1q3bGBrKkMvldHcrsbS0pK2tjVMxMfj5LaG1tQ1RFLG1tcXX15e29nYCly5FLpdzv6mJM2fPkpSczNKlS6mvr8fDw4Nt27Zx+tQpFi5axJkzZ6itrcXGxoa+3l6efvppjh49Sn9/PwsWLEAmk7Fv3z5efPEFzp49S05ODsuWLdNKZLdjbm5OeHg4r7/xBuV37rBy5Uo++ugj6uvqmDZtGgsXLaKjowMjuZz169cTFhaGqakpkydPpqqqigULFjBmzBhmzJhBREQkfX29rFyxHD09Pfr6+ricns7CRYsoLy/HzMyMnt5eBEFAX1//if3WIiLDP57h+6PwMhAtCMKXefw2wPrHafizH94DbNq8mevXr5OYmMjESZMwMTFhWKWip6cHFxcXWltbaWlp0chPT5yIj48PmZmZnI+Lw8fHh2atEcXkyY4oFIoR15moqBPs37+fkuJiTExMWLtu3YjCzPXrBaxZo7HFun79OqmpqeRcvcqePc/g4OCAiYkxmzZt5GJKCnp6euzevZuTJ06MCFR89NFHDA8Pk5ycTGBgIObm5hwKC2O1lmsfHBxMQnw8OlIpwyoVMdHRuM+cwfvv/4Xw8GNER8do5Kt0dOjs7KS0tIxp06aybdtWzp09yyWtIaelpSWVlZUcPHCA7du3s+qpp5DJZJiamqJQKOhRKhkcHMRYoSAkJIT09HRqampYvHgxeXn5HD16lKCgZSPqP2q1mrCwQ/zhzTcpLS3lYUcHV65cYdu2bSNS2k8SP5U5vSiKecBUNOnlLwDTRFEseJy2P/s3vVKp5PDhw+jq6pKYeIG53t5kZWYSGhrKgf37WbR4MS4uLhzYvx9BImHnzp3o6OiQnZ2NSqVi9OjRzPL0JPrkSX7/+9/h7u7OqVOnEASBZcuWUlNToxkaT55Me3s7alHkww8/xMnJiaSkJPr6+igtLcHS0pLQ0BAkEgn37t1j7Nhx6Ovra4gykybR2NhIV3c3O3fsYMmSJVxITKS0tBQdiQSZgQH1dXV0dHSQmJCASq1GrVajUqu5lpPDaBsbigoL0dfXp7q6GiMjQ7KyMhEE0NXVpampCaWyh4SERERRpKurk7i4OObNm0dJcTFJSUmsX7+enp4efH19OXDgAD09PTg5O2NnZ0fE8eM4OTsDsHHjRnZs34auVAe53IisrCykUl36+/uoq6vj+vUbeHt7c+nSJfR0dSksLGTqtGlcvXoVURSfeKf/Ec3ZHwdTgOloRDBnCoKAKIpHH9XoZ9/pa2trMTDQZ9OmjZiZjmLGTHckEgkRERHk5eVhb29PY0MDRnI5GRkZCIKATCajsaEBpbKb+PPnUavVNDc3Ex0dg76+PikpFzE1NUUUYcKE8SxevBgdHQmbNm2ipqaG//iP3+Hq6sqiRYvo6+tjeFiFiYkJFRWVpKenk5eXT0CAP9ExMVRVVfJ/2HvvuKqudP//vc/hUA9VekdEEVAELNgrShMUsII1aoqZJGbmm8xMkrl3ZpKpdzKTm8mYxBIFFRQLNgQpdlBUQHqTjohU6fXs3x/7yM3NTTG5Y+69ye95vfbrcNbea58Dh3XWs571PJ93c0sLxkZG2NjY0N/Xx4aNG6Uc/IEBhtQiFufOnaO4uBj/gAB0dKSg7p49e5g5cyYtLc3s2rWL2toaAgMDqampoaysnPDw8FE5LhcXQwICpVz4uro6XCdOJHTFCpKTk/nVv/wLWVlZFBUVkZqaCqLI/n37WLt2LXW1taSmpqKrp0dRYSEqUcTb2xsPj0no6ytpbGxk8eJFWFpakpuby5EjsUydNg1TU1M+/eQTgoOD8VSz9uRy+ahe37MwaZ/+md3+ezVBAtAsQBr0iUgS8teBbxz0z8y9FwRhvyAIjwRBKPhc278KgtDwBSWdJ+d+oQYHlAqCsOxz7V8KFRAksOYtQQJgHFXLeH1rc3Nzw8/Pj48//pgZM2aQn5+PQqFgaHCQGb6+BAUHs8zfH2NjY8aMGcOWLVuYO3cuHh4eTJjgSnBwEL29vcyZM5s5c2YTGhrCpEmTcHd3Jzw8DE9PT0zNzBjrPI6SklKysm7z1lu/RENDgVwu5+jRY4SEhtLU1MSSJYuJjIxEkMmYO28+YWHheE7xYpKHB5M9PVGpVKyPjCQjI4OkCxeYO2cOPj4+5OTk8PDhQ1599VXiYmMBqLx/n4murgwODTFmjCkeHu5qXb0r3Lhxg7CwMO7cuUNnZyc2NjZ0d3cxODjIyZMn8Q8IYPPmzZw/f56OdinYN2vWLDVVZzPWNjYYGhri4+ONXC5nypQpLFy4kE2bNzM0OMiuXbuorLzPjRsZ7Nq1i5SUVARBIDs7BxcXF4yMjKiqqmKCmt57/vx5NDQ0EEURTc3v9DE+tf2A0nAjkIRtHoqiuAXwBJ4qIPIs1/QHkCS3vmh//bySDoAgCG5IOmPu6j7/EARBLvwHVCAA6RttnfpagD+q7+UCtCNlJ30nMzc35/nnn+fUqQSuXbtGeloaO55/HqVSyeDgIBkZGejr67Njxw5uXL9OctIFQkNDsLOzo7a2FlEUWb9+PdeuXePWrVvMnTuHiRMnkpGRwdGjx5g7dy6zZ8/m8uXLKBQaeHh4UFNTw+DgICZjxqBQKNDS0qK1tZXo6Gh++9vfcjMzk7i4OPz9/enp7SX64EFWrFiBvb09FRUVtLS04ODoyGRPTwry85HJJM35Gb6+XEpP59KlS8yeM4ebmZnMnTsHAF9fX4aGhikuLsHTczKlpWUcO3YMPz8/IiIiOHQoBgMDAxwcHGhtbSUnO5v8/HyOHz9OSUkJDQ0NvPvuuxgZGrBz50vEx8cjkwn89Kevk5WVJb3mbCkZqLe3F4VCA7lcjoODPSUlJejq6hIWtpIb16+Tpqb/AoSEhPDb3/wGURTR1v5Kufb/ton8oNJw+0RRVAHDgiAYIAnMjn2ajs/MvRdF8apa7/5pLBSIE0VxAKgSBKECqYIIvgQqIEjgjEX8hxjhQeBfkYg338pKS0upuH8fHR0dLC0tqaqqon9ggLy8PFxcXEhNTUVDLsdzyhQuXLhAeloaa9asGZ2Zf//73xMaGgrAyMgIubn32L59G/39/cTFxVFRcZ/evj709fWRy+WUlJRy5swZ5HIZH330D55/4QUAwsLDOXwoBmtraRZ91NyMvnq7Lj09HXMzs1EKbk52NpaWlly/do1p06fj4OBAlholraenx5mzZzHQ1+fQoUPUNzRw89YttLS0QRQRRZH6+jpOnjxFbq5U+RcbG4empiZXLl9h9erVXLx4ERNjY2zt7FAoFCxZsoTBwUE0FQru5eZSVlZGaWkpmZmZGBgYcvv2bR41PURPTw8PdzeSkpLo7u6hvr6Ou3fv4u3tTVzcUaytrRgzZgxJSUnY2duTeP48ff399Pb00NjYiCAI6Ok9NYfxW5soigypnqUyj2SCIPwrEgruCWThl5+b4H6BNEGNAK+Iopj8HV/mjiAIRkh6kneBbiDraTr+T6zpXxYEYSNwBwl71Y4ECrj5uWs+Dw/4IlRgBjAG6FALFH7x+v9ialjBDgB7e/v/dE5HRwcbGxtmz5lDVVUVFhYWTJ02jdzcXFJTU7lfUUF4eDj3799n0aJFFBcVMW/+fK5du0ZdbS2FhUXY2tpx+/YdioqLedzRQXp6OgqFJo8eNdPc3MzW557D2VkqSjx//rw6//0zKquqRvftAZKTkwkJCeXYsWOkp6fj6uqKqZkZ45ydcXNzY3mIhM3qHxhAqVTiOnEicbGxaCgU3L1zh6NxcTiNHctvfvMbcrLvMjw8zK9//WvKy8pG04kPHjyIj89UwsJWSrp+hoasWSPt9PT29aGppYWfnx8AxSUl9Pf3o6+vT3t7O21tbfzu978nNyeH2bNnUVxcTECAP62trfz97x/h7e1NVVUVU6Z4oVQqMTAwwNTUlPPnz3Pr1i1sbW2pr2/gQWMjmzZvxtXVFYADBw4QFBwsxQae4ZoeYOT7AGpI9ldR4s2N2hc8WmsgVRCE8aIoPvU3kSAIs0VRvAHsUk+SHwuCkIREe8p7mnt831t2uwFnYAqS5t4TaMYzA13A18Mu7O3tKSoupq+vj7S0NLx9fGhra+PWrVsYGxuzecsWWtvaGDt2LBeTk3n7nXdG02Plcjm7P/54dK09xdOT1157FQADA31mzZrJsmXLyM3J4c4dSeezr7cXDQ0NRlQqPDw8WBkWhn9AAE1NTbz44ku4e3hgZGjIz998A3c3N3S0tXF0dGRoeJj6ekngVF+p5PHjxxgaGrJp82aGh4dx9/Cgrb0dCwsL9PX1pZm24QGurq40NUkaAMXFxYwdOxYdXR1aW1uxs7MbTQ3OzMxkzpw51NfX093djUqlwsjIiNDQ0NGMujVr12JiYkLDgwYSEk7zs5/9jBs3MrCxsSEsTJLcdnd3x9LSgvLyCrS1tXBwcKC7u5t33/0t06dPR0NDgw8//JDiIokAlZuby8SJE1m5ciUjIyPPdqbnm137Z+zej3q0oihWIak4T/+GPl+0J9j4zCcNoihWP+2Ah+95phdFsenJz4Ig7AGeTHNfBw/4svYWJESvhnq2/2/BBrZs2cLx+HgMDAw4dvQoSqWSbdu2cfDAgdFCk7fffhsNuVxaj6ank3XrFhGrVlFfX4++gQEv79xJRET4KGFGX1/Jm2++yZHYWNatW8+VK1ckaERfHykpKSyYPx9jExPijx2jt7eHF198Ablczod//wgrS0s0NDRQKvVITk5iaHiEX/7yl3y2fz/PbduGUl+fJX5+XL1yhUePHjFv3jxGRkbo6uwkJzub8vJycnJyWBkWBsDykBCuXr3K/YoKntu2jb6+Pvbv/4xdr7+OIAicOH6crq4ufNW1/ieOH0epr8/UqVPp6+vjyOHDBAUFUVxcjKurK0ZGxtTWVGNpacnly1c4evQY/v7LMDQ0ZO/efWzb9hzGxsa0t7eze/duIiIiMDMzIycnB39/fwwMDKTtS5WKnOxstmzdCkgFOUql8rt+jN9o30Ijz1QQhDufe/6p+F8pN99k39ajfVobEgThM8BWEIR//+JJURRf+aYbfK+D/gnZRv10JfAksn8GOCIIwvtIbo8L0vpE4EugAqIoioIgXEKKYMbxn6EZ38paWlo4ceIE2Tk5VFVWEhYWhoOjIx0dHXR1dbFv7x7GjDHl//3sp6SnX8I/IIDk5GTc3d3p7e1FLpOhraXFmDFjmD59OoaGhtTUVFNcXEx0dAw9PT0AzJ8/n5KSEo4cPoyRkRE+Pj7o6Ghz9uwZli6VuO4jI8NcvXKFZcuW0tnZyezZs6mqqkZTU5OY6GgEmYx33n6bV155hdu3b3MoJoZp06fT39eHnb09eXl5mJiYcPfOHfIL8hk7diz3cnNRqVQkJibit3QpiYmJPGhooLq6msTz55k9Zw4Dg4MoNDURBEH6stHXJycnh4aGBlxdXQmPiGDKlCm0trSwf/9+RFGkurqarKwsuru7RpN1APz8lvDmmz/H1taWnJxsxo4dS1ZWFnK5nIKCfOzsbMnLk2S5fvGLX7ByxQqSkpIYHBh45mt6AJX4VO59iyiKU7/uAkEQUpFUpb5obyF5tL9F8j5/i+TRbuWfg8MKBpYgxbSeKhnni/bMBr0gCLFI+4imgiDUIzHxFgiCMAXpF61GqgNGFMVCQRCOIVE/h4GdT9Y5giA8gQrIkUoJn0AF3gTiBEF4F8hBUvb51mZqakrwzJk87ujA28uLNWtWU1FRwT8++js1NbW88MLzTJs2DZBy0VUqFYsXL6ajowMvLy+A0f18pVIpRdUdHAgMDKSoqIiEhNP87r138fHxQS7XwM7OFmNjEyIj11NbW4eRkRHDwyMEBQWyf/9+PvjgbxQUFODj40N+fgFubhOpr29gw4Yo+vv72bHjeU4lJEiRfAcH/Pz8GBocJD4+nqtXrvDW228zf/58Dh8+zNDQEBs3bQKgrb19lFYTHR3NrNmzmTlrFiUlJXR0dEj4LUEAUeRhUxMlxcXMmjWLuXPnjnoD4RER2NrZcfLkSWbOmo2ZuQUlJaVoaMjVX1ojdHd309rawssv72TMGBM0NbWYMGEC9vb2DA+P0Nvbw+rVq6mtreOXv/wlmpqaLF26lOPx8QDP3L0f/CcF8kRRXPI0130Lj/ZpX7dFEIR4wFoUxYPfpu8Te2ZrelEU14miaCWKokIURVtRFPeJorhBDbKYLIpiyOdmfURRfE8NDpggiuKFz7V/KVRAFMVKURSnqwEYq9RBje9kR+PiCAkNlVzkri7u3s0mLCyMmTN9GR4e5vDhwwwPDzMyMsy5s2fxmToVK2trKioqSExMZPGSJfjOnElhYRGnTp1iyZIl2NjY8ODBA6ZNm8bkyZ6YmZlhY2NNSEgIP/nJy3zyySdcvJjM0qVL6ex8zKlTCXh7+2Btbc0Tsu7t21nMnDmT4eGh0fTVjz/ejaGBAcNDQ8z09SUnJwePSZMYGBjg7x99RG5ODoIgMGbMGObMnUtCQgIqlYqxY8fS9PAhbW1tyGQyBgcG0NPTY/r06ejp6hISEsLUqVMJDArCxtqKHTt2IJfL2bt3L9euXaOtvZ3W1lZOnjzJ1q1bGR4extraGi9vbzyneBG1YcNo0tCePXu4evUqWlrarF69ioyMDPbu3cvs2ZKKT21tLSkpKezZu5dbt25RV1eHoZERoig+W/ee72fLTpAYjU/six7tWkEQtNTe6xOP9tv9HtKEuPy7vr8ffUZew4MHpjVTvQAAIABJREFUGBoZ8eDBAwSZjJ07X+b111/Hw8MDuVzO4OAgK1euZM+evbi7u3H12nVCQkOxsrJi/7596BsY4OjoCMDePXuYONGN/v5+bt68hVwuJycnF19fX86ePcvDh03Mnj2bmpoaLCwsSE1N4/DhIwgCJCScYsGChdTW1qBQaPLxxx8TEREBwPDwCHv27CEycj2tra3IZAJvv/0WHpMmY2FuTkxMDAEBAejp6aGlrU1mRgbm5uaMHTuWjvZ23n//fVatWoWdnR2HYmIwMjZm6dKlpKam8qChgU2bNqKtrc2Hf/87cpmcF154HplMxsHoGLZv3051dTUnjh/nzp07fPDBBxKUQi1vvWnTJo4cOSKVDh84wIYNUcjlckxNTUlJSaGnpwdtbS1u3LiBjo4uhoaGpKSkoK9vQE1NDSpR5E9//CMbNm5EFMVn696L31v0/k/f1qP9DpYhCMLfgaNAz5PGJ1V3X2c/+kFfUV6Ovr4+zs7O+Pv7U11VRXx8PNXVVXR2dlJf34CVlRWamppcvJhCe3s7x44eRaFQjMpCaWhoMDQ4yNVrV9HW1qaqupqQkBAq4irw8vJi1aoIPD0nExNziBUrJOjF7du3GT9+PMuWLZXkqoaGsbOzw93dnWvXrpGfX4CxsVR6mpqaipeXFxkZGZiZmUuqOSoVOrp66Ovrc+TwYRQKBVevXmWgv5/Dhw8TGRlJQ309nZ2dZN26hampKRpyOdnZ2ejq6dHT3U3yxYu4u7lx+vQZBgYGKCstA+DIkVj09HRpaX7Ex7t3Y2hkhKmpKSYmJsTFxaGtrU16ejrTpk2jpLiYGTNm8MYbb+Dh7kZKSgpdXd3cvy/Jf69bt468vHzGjBmDIAh4enrywQcfMDg4xIQJ43nxhee5cOECcpmATCZ7xjP991NQI4riV2rOq73VfwYGa5b68Tefvz3SWv9r7Uc/6OfPnz+anHPhwgVeeeUn1NTUUlhYwKZNm3jllVd57bVXUSqV7Nu3Dzs7W5YtW4qBgQGiKKKjq4utrS0TJ06k6dEj7OztmT9/Po2NjTg7O1NdXY1KpeLq1Wv867/+C/Hx8axdu5aCgkJ27XqN06elRJ2goEAuX77CyMgwnZ2dzJo9m4ULF2Bubo4oSttqc+bMwcDAgN27P2bHju0ciY3FwsKCDRs30tnZydq1a2lpaSE/P4+JrhMwMzMjLu4oL7zwPONcxmNra4sgCPT09BAeEUFnZydKpZLVa9bQ0tKCmZkZgiAwbpwz48aN4+DBaFrbWlm9bA1ZRkZoKBQ4q4U67ldUMDQ8jLaODj09PXR1drJo0aLRfIQjR2JHRS7v3LnD1q1b2LNnD4sWLcLFxYUxY8agpaVNbW0thoZG5OcXIAjCMx308MMh3IiiuPC79v3RD3qADVGRfPLJp2hoaKCvr8+ECeMB+POf/43ly5eTnJyMo6MTkydPxsfHh0OHpJn1SU7+8fh40tLS2Lx5M5cvXaKyspLU1FS2b99OcXEx+fkFmJqOQS6Xo1QqqampwdjYCJlMRmtrK3K5DDMzM1atiuDjjz/B2MSE1atXc/DAAaKiJHTUqlWr+Mc/dqNU6hEWFoZcLkdbS5vbt2/z6quvkpOdTVJSEjXVVfz1r38lNjZWHfjbzvDwMNeu3+DypUuErlghwTROn0ZPqWTy5MlcSk+nvKKCbdu2IZPJ+PSTT8jPL2DGjOn09/fzu9/9jj/96U/IZDKOHj1K1q1b7Hj+eRoaGqiuqsLFxYWdL79MRkYmgiCQm5vLokWLSEw8T05ODt7eUsBz5cqVnDhxAnt7exYuXMiBAwfo6JBkt3bufInXX3/9mRfcjDxd9P5/vQmC8KsvaxdF8Tdf1v55+9EP+gcPHnDgwEF6eyUybUpKKhoaUrVXR0c7ycnJ6OrqkpGRyZo1a7h58yZtba2UlZUzODiAQlMLLU1N8vPySE9Lo39ggD/98Y9M9vRkYGAANzc3fvr6Lt555x1A8ix27tzJzp076ezsZN68uZw9e474+OP09fVRVV1FXX09WlpaWFpa8v777xMZGcXhw4fpePyYsrIy9PUN6O/vp7e3h8LCQmKio9HV1eXUqVMsXLiAxMREkpKSWb16Ne3t7YwZM4YHDx5gppbNAklfr6mpCXMLCz7dswc9XV1OHD+OXL1ll5qaSltbGxoach41NXHq1CkGBwbIysrCwsKCrq4uzM3NefToEf/+4YdsiIrCwNCQf+z+mMGBfiZPnoy5uTk3bmSwceMGTp48RUdHBxcvXiQ8PIxTp05haGjIsWPxREau5+JFiXDzTAe9+M+L3v8vsJ7P/ayNtJX3VJqVP/pBb2VlRXh4GPv27WfatOnMnOmLUqkkOfkiGzduIiMjg0WLFxMbG4u9gwNaWlq0tLTS19c/qoH/6NEjsrKy8A8IQFdXV9JyFwTi4+NRjYzQ0fFYHUUX0dLSxMHBkYKCAmpr6yguLubRo0ds2bIZExMTDhw4iMmYMQQFBXHq5Enu3ctjsmcBK8PCORoXh7WVFUFBgWhra5Obm8vAwCAbN23i+vXr/PKXv6C4uJjly5dTU1OLkZERly5dpr+/j+SkJJYs8ePwoUNoaGigoSGnoLAQT09PJk2ahKhSsTIsDA0NDRITE/Hw8OC557Zy6NAhPvjgb6SmpbNu/Xr6+vspLy/n4cOH9PT00NPdLUlilZTg7+/P0NAQpSUltLa20dPbx/3798m9l8e8+fPR09NDpVJhaWnFnLlzUalUXLiQhEKhICBAqs16tlt2PxwJbFEU//L554Ig/BvS7sA32o9eOUcQBBITL7Bo0ULWrl1Devol2tvb6e3twdVVQlbHx8djYmyMpqYmRkZGPO7s5I033yQu7igAZ8+e5ac/fZ3s7GySk5Px8/NDoVAQERGBt7c3O3e+RE9PD6tWRdDT08svf/kLhodHWLJkMU5OTrz00osUF5cwODiIqakpA/39yGQyhoaGeOmll9DX1yfl4kX8/Zep36OkEZ+bm4uRkREqlYqqyvt4eHjQ09NLW1sbbm4TKSkpITg4CFNTU0JCQnByciQqKpK1a9fg5OSEvZ0tHpMmYWxszIaNG0k4dQqAxsZGdPX06OvrQyaTYWJiglJPVy3Aocdbb71FSXExS5YsobW1lX/785/R1tKiq6uLwYEB3njzTUpKSrC2tsbFxQVHBwfGjBnDkcOHidqwgfuVlQDEHzvGrl27ePjwIfn5+QDPtMoOflBVdl80XZ6yyu5HP+iHh4fp7HyMk5MTGhoaNDc3c/TosdHKOW9vb6oqK9m2fTtpqanczMzEx8cHmUzGvPnzSUtLw9DQCHNzc1qam2ltacHZ2ZmoqCiSk5K4c+cOPj4+7NixgzfeeJOGhgaOHYtHQ0ODV199FQMDA8aPH09RUSFHjsQyd948+vr6uHHjBuNcXJg1ezYFBQWoVCPY2Nigq6vLgweNnDt3nkWLFtHX10fskSMEBQUBsH79Ov76178xYcIENm+WttP6+vpYvXo1/f0D5OTkAJCfn88bb7xBdHQ0rq6u6OrqYmhkRFlpqboENozk5GQsLaWks8DAQP7tz3+m8UEDF5OTUYkiu3btQqVScf36DfT19Xnv3Xdpb2+noaEBj0mTSDx/nm3bt3M+MZH+/n5MTEzQ0tLCydGR8vJyRkaGmTjRFXt7B27duiUFRnV0vvyD+ieYKIqMqBWFvu74v2CCIOQLgpCnPgqBUuCDp+n7o3fvL168yNixThw/fhy5XE5ZWRkymYzo6BhUqhFUKpHW1lY+/Pd/p7mlhY7HjwkODubo0aP0dHdz8+ZNbGys6e3t4cKFJMLDw0lLS0NbW5u7d+8yNDREXNxR+vp68fT0xMLCnLCwMFQqFYWFBbi7u5GamopSqeT69RtY29iQn59PrpohF1dezq2bN3FyckIuP83A4CCPHz8mPz8fTU0F9fX1tLQ0MzAwgFwuAwTKysq4fPkKOjo6ZGRk4ODgQFdXN6KoorGxkdbWVjWBt5zbWVno6GhTef8+02fM4MyZM1hYWKClpcXJk6eYOXMmjx41o6enh46ONjNmzMDNzY3h4WFu3bzJmjWrR72NBw8eqNOQaygrK6O6uprY2FiGh4d54fnn2fnyywwODjJv/nx2796Nh7skjdDT0y0p+T5zYcwfTvQeaQ3/xIaBps9VnX6t/egH/eLFi6mpqR5NhGltbUWpVLJ27VoAjh49xl//9jcyMzMlXbuLF5k8aRL+/v4IgoChoQEtLS1ERkZSXV3D+PEuuLm50d3dTWZGBlZWlqxdu4a4uKMEBgaRlJREZ2cnhYWFvPjii+Tm5hIREUFubi41NTUsWbyIm5kZuE50IyQ0dFQssr29nZDQUARB4NjRo5iZmbF06VJqa+uwsDBn69YtCILA/fuV9PT04Ok5mYcPH/Kzn/0/MjJuEBQUiK6uLtnZ2Xz00T9YuHABPj4+eHlNYfKkSbi4uHD/fiVtra1UVVWhr6+PmZkZ3t5ezJgxA4C+vl7q6+uZNWsWsbGxzJo1a/Tv+NlnB1i9ehVxcXEsDwnBwcEBaysrFJqaREVF8bOf/pT29nauX7/O4OAgN65fR0tTkwcPGrl2/QaGhobfy6D/PurpvyfTAOpFURwQBGEBEC4IQrQoih3f1PFH795raWkxbdo0SfsN0NbWob9/gL6+Ph49eoSeUomVlRWtra1UVVfj7ubGpMmTMTQ0JPbIEVauXMn69etJSEjA09OTzMybiKKIIAi4ubtjaGRMUVER2traWFiYs2FDFMnJFykqKmLcuHF0d/fQ1dVFTk4uTk5OdHR0MGnSZJR6ulRUVJCQkMDcuXPxW7qUa9euASCKKgYHB0ZLUVeuXMmZM2dQqVRcupTOzp0vUVJSwqNHj3B1ncDWrVuJizuKpqYmFRUVbNmymXnz5lFWVs6WLVvIy8tHqVQyZYonDo4OjBs3jrlz5zJv3jyampooUpfB6urq0dXVTU9PD5qamgQFBVJSUkpFRQXjxjmjr6/PunXrSElJIeXiRQICA2lsbCQuLo53fvUr6mprWbRoEfr6+rz62muYW1iwavVqFi9eTFtb+/cgjPk/Xlr7z7QTwIggCOOQ6k6cgCNP0/FHP+hB0skbHBxUSzrpsHbtGi5fvsKpUwkEBgbS0tKClpYWOTk5BAZJs/W5c+fw81uCXC6np6eHW7dukZmZwcqVKzhz5gxHj8Xj5+eHhoYG7733OzQ05BQWFjIyMqJ2xeUIgkBUVCTR0TGMGzeO0NBQ/vjHP7FgwXwCAwNJS02hu7sbcwsLHBwcKCkuJjk5mRkzZhAQEEBqahqWlhZYWVmhp6fk9dd/iqmpGRcuXODEiZP09w8QHXOIAwcOoFKp+MlPXsHLyws/Pz/KysoQRRUmJiZs3ryJhIQEPvvsABERq1i3bh3nz51DqdQjJCSEe/fySE9Px87OlvDwMH7zm98SFBSEhYUF7e1tXLp0eRSQoVAoOJ2QQE9vL1evXKGvr4/a2loMDAxYuHAhaampVFdVMW3aNFqamzkaF8fixYsZ6+z87Ak3orRP/03H/xFTqd35MOBvoijuQtK+/0b70bv3zc3No3vk6emXMDExYXh4mPPnE3FzdyMmOhqlUsnI8BBTPCdz714uV69cQaVS0d3VSUFBIebmZtjbO2BlZcW9e3ncupWFXC7jQmIi3j4+WFpaMn2GL48ePSLm0GEG+vspKysjLk7Ssc/IuIGxsTEPHzbS3t7OpUuXRoUib2dlYWigz8DAICqVivhjx1iwYAGOjg4cO3YMX98ZNDY+REdXl8HBQfz9l6GtrU1DQwOWVlYEBASM/q7bnnuOrNu3KS8vJzc3Fz8/P27dyqK1tZWMjEyMTUy4mJyMXEODEydO4OXlRV1dHXK5Bp988ikhISGkpaXT1tbGqVMJWFiYk5aWzsyZvpw+fYbu7i6GhoaQyeVERUWho6NDfkEBcpmMy5fSGRgY5NChQyxfvpzz587R2tpKQ0MD+gYGdHd3I5PJvhdU9Q/EhgRBWAds5D+Kb57KTfrRz/RPMuFWr5YKUszMTAkICGDSpEn4zvBl06aNhIeHoaOji6vrRMY6OTF1qg9OTo4EBQURGhpCXl4+mzZtRENDjo+PNx4e7oxzGU9IaChKpZLly5eTkZGBu7s7W7ZIa++JEycSFh7O4iVLcHWdyMKFC7Czs2PevHk4OzsTEhKCQqHJggUL8PX1ZdWqCFSqEby8vIiICMfe3h5tbW3MzS1Yt349Pd3dbNm6lfz8Atra2nBxGU9bayutra0ApKam4h8QwIIFCwkLj6CpScotsLe3w8bGhpCQ5UwYP57g5cslVt3cueodgM2EhoYwZoxUDmxgYMDmzZvw8fHG29ublpZmdHV1CQ0NITIyElEU+fmbb1JaWgqAjY0N1jY2arDlQxYuXEhgYADBwUGSpt6kSQQHB9P5+DEAMtmz+5cUEVGpmQBfd/wfsS3ATOA9URSr1FV7h56m449+0AM0NTVx8GA0L730Ilpa2oiiyJQpU6ipqaGtrY2CgkImTBjP/PnzuHs3m6GhIV599VViYg4RE3OIpUv90NfXp6urm2PH4omKimLa1KlkZWVx7tw5pk+fRntbGwAXLlxg8ZIlrFq9mitXrnDmdAJvvPH/KCwspLS0lKioSG7evMnQ0BBGRkZERISTnJzM7t27iYqKwsfHm7q6Oqqrq9m16zXc3d3Yv28fKlFk6tSpFBYVcubMWXx9Z7B+/TrOnz8PSJmHYWFhlJWVkXThAm+8+Sb6+gbIZDIKCwtZvHjxKKfu4MGDPLdtG62trXR2dhIXF4enpycxMYdYuXIFs2fP5u7dbDIyMnjvvffQ1tbmzJkzVFRUMNZ5HA6Ojjx+/JhDMTEEBwczPDTEgQMHWbJkCRER4VIWYcwhSfNPFElJSWHW7NmjsZBnZuoqux/Clp0oikWiKL4iimKs+nmVKIp/eHJeEIQTX9X3+9a9NxEEIUWtVZ8iCIKxul0QBOHf1dr2eYIgeH+uzyb19eWCIGz6XLuPeq+yQt33O/233L9fQUpKKjt2bEehUKCjo8PBg9HMnTuHqKhITp8+PaqJBzA0NIggyNRur5xr165x5epVYmIOceHCBUxMTMjKymLiRFdyc3JQjYygq6vLxImu3L59m76+PsaNG4eRkRGlJSU4qLP8SktLmTBhAgAbNmzg97//Azo62iQknCYtLY3BwSHKysrw9fXl3r171NTU4ujoSFZWFgqFBqUlJcREH6T5UTMdHe2j/PfxLuM4f/48Li4uyGQyOh8/pqOjAysrKxwcHXnrrbcYGRnm4MFouru72fXaa9jZ2VFRUYHf0qX85S/vY21tTVFREVVVVdTXN9Cr1vmrr6+nq6uLmppaqqtr+P3v/8Dw8DADAwOUlpTg5ubGjRs3qK2tJT8/n+zsHCorKzl95gwjIyMkJSUxMDjIhcREHj58+MxnWRUig8PD33j8QOwrE3We5Zr+APB3/jNx4+dAmiiKf1CDK36OpIATgCQo4IKkdrsbmCEIggmS4s5UpB2Xu4IgnFHrje1GUri9iUT48Acu8C3t0aNmZDKBM2fO0t/fT3d3F3l594iOjkahUFBbW0tvbx+JiRcAKTc8PT0dZ2fnUfzzhg0bqayspLunG0/PySiVSqKjYxBFkby8e5w7dw5BkBEbG0tAQAAnThyX8vXz82lqaqKrq4usrNtYWFgSd/QoA/0D3L9/Hzc3NyIiwunt7UFXVxdjY2P27dvPlStXcHCwJzHxAkFBQRgaGhIfH8+qVatISUnh+PETnFOTd4aHhzl+/ARh4eEknDrFmTNnWLhwIQmnTiKTyXF1nUhk1AYEQeDhw4dUVlYyc+ZMHjQ0kJeXR01NDWPHOmFtbY2hoRFa2trcvn2HsvJyHnd0MHPWbNatXy8N4IEBvL29iY2N5d69exgaGbFy5Uqqq6uwHLQgJGQ51dXVPGx8SHhYOD5TpyKXy7mYnIyzGvf9TE2EkZH/GzP5P8G+MnjxLJVzrgJtX2gORdKoR/244nPt0aJkN5FEL62AZUCKKIpt6oGeAvirzxmIopgpiqKI9MWygu9gM2fOREtLm8DAANasWY2bmxuurq5s376dqKgozMzMmTx5MkFBgdjY2NDb28vixUuwsrJEpVIRGBhIdnY2V69e5fnnX6CgoBAbGxuee24rgiDg5eVFcHAw7e3trF69mnHjnIkID2f58uX4+S3BycmRJUuW4OzsjJu7O2vWrKW7u5uPP/mEtra2UTWZlpZWnJ2d8fX1ZfbsWchkcvr7+xgcHASgr6+fjo4OWlpaefHFF7C0tCI4OJgHDx7w8ss7GTt2LPYODqxevYrx411GybrBwUHk5uYCUjrxvPnzGRgYYLKnJy3Nj3j//b+gUqmwtbVj0uRJ9Pb24jxuHO5ubkx0c8PFxQWVSsXgwACeU6bQ3d2Nk5MTHh4erFixAlEUsbO1ZcWKFaSlpXHrVhYffPA38vLyaGluRqVSIZPLSUlJeeZBPJEfTkbef8e+7zW9xROJLPWjubrdhv+qb2/zDe31X9L+pSYIwg5BEO4IgnDniRTV523jpk0kJEi6mmVl5UyYMIGGhgY+/vgTnntuK6KoIibmEI/V2XhTp/rw2WcHeNzZiaOTE3fu3MHZ2RlBEGhvl/abT5w4ybJlSxkaGuLixYt4ek4mJGQ5tbXSejwtLY2pU6eyefNmTpw4ybRpU7mZmcmeTz9l7bp1aGtr4x8QQHR0DNbW1syYMYPY2Fiqqqp44YUXmDx5EhEREVy+fIUjR2JpaGjgyJFY1qxZjaenJzdv3uTDDz9k48aNzJo1i9KSEvLu3WPFihWUlZWxf/9nBAcH4erqyv3796mvr8fFxQU/Pz9yc3KoqqrC2toGExMTvLy8qK2txczUlKQLF9i7Zw8hoaGsXbuWtLQ0oqOjCQkNZfHixRTk51NTU8Orr73GjevXOXnyJAsWSLoAlZVVDA8PYWxszPbt2zh79ixFRUXczMxk+fLliM86sv4DWtM/hX3lN+j/li27Z657D3wKMHXq1P90XWNjI9XV1ejq6hITc4gZM2agUGjwxhtv4ufnR0LCaUpLy2hpaWFw0IeWllbMzExRKtXqM8nJXLt6FYWGBs2PHjHD15eDB6MZP94FDQ0NCgoKuXcvD0/PyWq5akm3DsDGxpbExAsUFORTV1dHc3MzJiYmXL9+fTSSnJiYiEKhgVKpJD09nZCQEFpaWnj8WKrkmzdvLufOnSM/P59x45zZv/8zVCoVpaUljIyoiI8/jkKh4OJFaX//6NFjjIyoKCrKx9bWhhkzZvC4o53E8+fZvmMHgiDQ2dVFeloqixYtIiEhgdraWrq6u+nq7kGpVFJRUcG5s2fp6ekhJTWVadOm0dzcjKmpKdeuXWPb9u1oaGjQ2taGkaEhXV1d3Llzl57eXiorKzl6LJ6RkWF0dHX585/+hKmp6ai38SxN5HuFXfxP25tfdeL7HvRNT2Sw1S76I3X7V6mE1iMp6n6+/bK63fZLrv/WZmVlhZu7O9evXyctLY3+/n7sHRywsrJk3bq1aGlp0dvbi729HVu3Si57VlYW/v7+3Llzl2XLltHW2oqBgQGz58whMzOTK1euMDw8RG9vHy4uLlhYmBMcHExTUxPV1TU0N7fQ0tLC1KlT8fScjFwuo7+/n+bmFgRBYPny5aOu7uPHj7GyskYmkxEUFIyNjQ2XL1+hvLyM9977HfPnz2fz5s1oa+sgyATWr1tHd3c36emWKBQKxo1zxtnZGQ0NOcbGxgQEBPDpp58SGRlJX18/KSmpNDU9ormlhWPHjiGKIqkpKVhZWVFXV8+KFSv49NM9THR1xdvbm+KiIna9/jplZWUsDwmho6Od7q4uSkuKSauv5+HDhxw/fhzXCRPIzclBW1sblWqEwMBAGhoaMB0zhtWrVwNQU1ODTBDQ19dnwfx5z969VxfcPGsTBGEVEmZtIjBdFMU7nzv3pVgrQRD8kQpm5MDez0fiv3DvfL5+gpusfrz4Vdd83+79GSSNevjPWvVngI3qKL4v8Fjt/icDSwVBMFZH+pcCyepzXYIg+Kqj9hv5jrr3IJVz1lRXE7VhAwsXLaK+ro633/kVycnS383Q0IClS5eSmSlBRYqLi/Hy8iI0NISzZ89iZ2dHW1sbCoWChvp6XnzxBSZNmoyPjzfOzmNpb28HwNbWlt7eHhYuXMjMmTPx8vIiMfEC/v7+dHZ2oaWtzaJFi8i4cQOAhFOnCFDTbyorK1m5cgV37twlNDQEZ2dnbG1tmDjRFUEQMDAwQEOuQUtLC7GxsfgHBOAfEEBqWhpHjhzB31/CTyUlJbNo0SJmzJhBe3s7Xt7eWFpZ4Tl5MosWLWLt2rUsWLCAiRMnYmFhwfDwMGZmZvT391NfX4+DoyOOjo50dXZSV1eHlZU1oaEhDAwMMG/ePHbs2I7z2LE4jR2rJvuOZ8mSJRw+fITVa9ZgbWNDbW0tjY2NXLt6lbXr1vG4s5PU1NRnHr0Xv7/ofQFSptzVzzcK3w3U+kULRkrGSVIfkeojETj+NG/uWW7ZxSKhdyYIglAvCMJzwB8AP0EQygE/9XOQ3nAlEuZnD/ASgCiKbUiwgNvq4zfqNoAXgb3qPvf5DpF7kKgqn3zyCVufe47FixeTk5ODUqnE0NCQh01NFBQU4OzsjIODA+XlFcTGxhKo5rhra2tzNC6O8vJynMaO5S//9m9MmzaV6dOnk5+fx5EjsSxcuJCIiAguXbrEtWvXUCgUzJ4tYZ+jo6NZvXrVqDbcyPAwY52dKSwqQqVS0dvXh6WlJT09PRgY6KNSqXB1ncBzzz2Hn58fmzdv5ujRYyScPsO0adMIj4ggIeE09g6OPHz4kJiYGGQyOTdv3iIjI5OiomKys++ip6dHV1cXoaEhXL50CUEQ2LxlCyeOH0cURfQNDIiMiqKRjUa8AAAgAElEQVS4pIR3332XRYsWMmHCeA4dOjRaZLPM35+333qL0tJS8vLyKS+v4MMP/46+vj4eHu5kZmRQW1uDiYkJu3a9jkqloqmpiXnz5pGWmkpSUhKRUVH09PTQ3NxMRcX97/LxfTv7ntb0oigWi6JY+iWnvgprNR01qFUUxUEkgEvoV9y7RhTFGmC2KIpviKKYrz5+jhT4/kZ7ltTadV9xavGXXCsCO7/iPvuB/V/Sfgfw+O+8R5BKa80tLDhx4oSkaHv1KtOnT6e8vJxlS5fy17/+lS1bNnPo0CFKy0rp7pLSRfv6+tDR0WH8eJdRBn1lZSVXrlwhLy8PDQ0FV65cxcjIiJGREa5evYKpqSnz5y8gKSmJjIwMTEyMOXnyFCMjw2hra3O/8j6tra2sWLGC999/H19fXz777DNaW1soKipCX18fJycnjIyMuHv3Lunp6SiVSs6fO0d/Xx9yuZxLly4RGBhIk7ExERER9PX10VBfj6/vDIyMDElOvkh+QSGtra10dXXR+KCB9vYOTpw4gaOTEwcPHsTa2pqkCxdoaWmhtLSMo8fiMTTQp76ujvhjx9DR0cHIyBBbWxsCAwOws7Pj7t27VFRUADA0NIS3tzeZmZn85Cc/oa6uDqVSSV1dHenp6aSmpOA7cyZ79+xB38CA5uZmZILwPUTvn3pN/8/AWn2ZfVtQ69eZniAIc0RRvA4gCMIs4Klkh/63BPL+x2zp0qXcy8tj3bp1CIJA/8AAAtDZ2Ul2djYDAwPcr6xixcow5PIztLe3j1Jec3NzcXV1paiomKnTprE8JATVyAhr167h7t27TJrkQVbWbbZte06tKWc2KgvV2NjIwMAAK1aEYmJiwqVLl6iulurQ29vayLt3D2srS6Ii13PsWDx2dnaMHz8eR0dHvLy8sbCwIDw8nPz8AiorKwkIDMTIyAi5hgZtbW1MVVN5jh+P5+c/f5NTpxIwNDRg4sSJODs74+fnx+DgILFHjmBnp2L58uXU1dVx5MgRPD09eXnnSxw4cJCPPvo7OTm5PO7sZNy4cSxbthRjY2MyM28SGRlJRcV9JkyYQG1tLdu2bUOlUjFp0iT279/Pr371DiUlpRgbm+DgYI+2ji5bt25FqVTS39c3St9BFBkaGnrmn7UoioyMPNUOwX8LayWK4lctNb8qAP1lHvc3vdHngP2CIBiqn3cgobO+0X70abgymYwItVDj1StXmDdvHu4ekgMhiiIf/eMfqEZGqKqStpuWLvXj2rXrAGRnZ+Pp6YkgCLS1taGjo4NMJqO5uZl79+7h7e3Nli2b+eSTTzE0NMTBwYHMTOmLXqnU54UXXiA+Pp62trZRff1p06ZRXV3Np3v2IAiy0W2udevWkZycTH9/P5aWltTU1AISUuudd97m2tWrXL58GW9vbxYuXEh6WhoAAoI6sceIoqJiVq2KGJXb+uyzz1izdi3hERGjeOxPP/0Ue3s7zp07T3BwECYmJjxofEBfXx+vvPoqcXFHEUWR0tIS3N3d6e7uYu/efURGRuLpOZmSkhLa2tqwsrLGycmJ6upqNDQ0mDNnDsVFRTxqakJbSwsPDw/u3r1LQ0MD9vb2mJgYP/stO/557r0oiktEUfT4kuPrYktfF7D+VrgrURTviqLoCUwGPEVRnPI0oAv4/wc9KpWKlpYWCgsKiIuLo7KykuZHj/jLX/6CXC4n4dQpFJqaHI2Lo7a2DicnJ0pLS2hpacHGRvLMVq9eRWpKCjo6OkSsWkVaWjr6+gYIgsDNmzcRBMjKukV+fj7371dQVVWFUil5Yu7u7rz66quoRBEra2veeOMNpnh5UVJSgqOTE6dOnaKuro62tja2bt3Kvn370dXVRRAEYmIOERDgj4aGBi2trdRUV+Pk5MS4ceNoa2ujoaEBpb6kIx8YGEhFRTm9vb3M9PXlD3/4A/r6+qSlppJ4/jwXk5NR6umRlHSBS+mXyMi4QUpKCtHRMWRmZFJcVMT+ffvQ0tLi17/+NQqFgsTERFJT0xgaGiQrK4u+vj46OzuJj49n6VKJcW9iYkxfXy8AGzZEcfzECRQKBd4+PmRnZ3P2zBlmzvQlMDDwmQ96lfg/nob7VVir26hBrYIgaCIF+75W5FJ9j/XAy8CrgiD86qtksb9oP3r3vqWlBSMjQ3WFnRlBQUGj4hmNjY2juOehwUEKCgv57LMDaGlp8dpru9i1a9eooGNqWhoBAQFcu3qV7JwcBEBDQ86UKVMoLS3D13cmq1ZFcPnyZX71q39hxowZnD5zFl1dXWxs7QgLC6OoqIiR4SEMDfRRKBQYGugzYcIECgoKuHTpMq2treTl5dHe3oajoyO3bt2SovYactpaW6itrUNbRwcDAwOsbWzYu2ePtO/+uBNtbS1GRkY4e/YcVtbWFOTnEx4ejouLC/v27WXNmjVMmuSBg4MDzY8eoaOjw4YNEqhlZGQEQRDYtHkzHR0d/Oqdd3B31yQwMFCK6Ds4MH78eOLj49V8+x4SEhLUabUCBQWFHD9+AoVCg/q6WqoqKxkeGcHE2Jjk5GTOnjuHQkPx7NNw4XupohMEYSXwIWAGnBcEIVcUxWVfh7USvhrU+lV2GniMRK79VhzHH/1Mb25ujpGREVbW1jg4OlJfX8/phAQWL16Mq6srd+/eHc1hj4yMxM3dHd+ZsxgzZgwGBvro6OjQ2dmJsZERlpaWTJ02DUcHB+zs7PDz8+PBgwfMmjWTkZFhFAoF5uYWBAcH4ebuTnBwMJWVlezYvp379++Tn5fH1ue2MTAwyIQJEzA3N2dwUNLODw8PQ0NDzjvvvI2XlxcymRx3d3fmz59HUFAQunp6OI0dy7x58/D29sba2hqVSoW9vR2bNm2kpaWV3bt3o6klleuGR0RQUFAg5dY7OREcHMTt27cB0NeX1v537tyhpaUFe3t7Jnt6cvfOHYyMjFi8ePGospBSqU9LSyvm5uZs3LgRW1tbXFxcCA0NZeXKlejoaOPuLtUQODo64h8QwEQ3N5YsWYK+gQFr1q5FW1sHN3f3Z/5Zf1/CmKIonhIlaKuWKIoWoigu+9y5bwVq/RqzFUVxjSiKfxJF8S9Pjqd5fz/6QQ9w9tx5li5dSkBAAKdPn2b8hAnIZDKmT59OTk4O0QcP4rd0KePGjaOwoIDLly6xbds2+vv70dLSorKyivd+9zt6urtHkc4vvvQS+/btp7y8gkmTJjEwMEh5eTmVlZWsWbOGxsZGDhw4wJo1axjr7ExjYyPGJiZ4enpy584dVCop9XfVqlWIokSsDQgIwNbWlr6+PgRB4Pnnd3D+fCIxMYcIWxnGK6+8wqlTpzA1NcXa2hpvb2+cnJz49a9/w6pVEWhqatLb00t6ejpeXl4MDgxwOiGBhQslQlJnZyeDg4MolXpMnTqVgoICDh8+wvwFC/Dy8uJudjY3b97E1dWVjRs3qmdvBSDS1dVFbGwcwcHLWbt2DRcuJAGSSImurh7d3d3k5xcwb958oqKiSEpKoqmpicWLFyOXyfhMzb1/1vYDSsPNEARh0nfp+KN379PS0tDS0uLw4cNoamqSmZGBgYEBp0+fRlSpMDAw4OqVKygUClQqFXezsxljYoxCoUFvbx9GxsZERkUhCAK3b9/G3d2d27dv093VRVV1NQIiR45IFXW3bt3C19eXAwcOkJl5EwO1YoyhoSGXL1/mp6+/DkBoaCgvv/wyLi7jOXDgIJevXGaK5xRSU9MYP96FwsIi7Oxs2b//M+7l5aGro0N/fz+TJk8mMjKSI0eOMDw8jJGhAQ0NDQwNDXH+fCLGxkZ4e3uxd+8+NmzYQHVNDfcrKoiOjsHExJjw8HD+8Y/dLFu2jIKCQvr7BygrK+PE8eP09/czODDA3j2fMnfuXG7evIlCocHVq1eJiAgnISEBU1MzLCykcoq2tlZSUlKZOvX/a++8w6I60/7/OfTeBKRIUywUUYqCYi8giAqKWKLYk2x6sknM7mZj8m7eN21389tkU2xYYgEbKigoIAoCNnpRmiIISJHeGeb8/piR1+xrYtxVE2U+13UuZp5zzszzMHPPec793Pf39iAzM4tdu3/g+eefl+9r4MyZMxgZGnL48GFaW1pob2//yc/oUfGkIvKeEJOA1YIg3EA2vReQrX67POjEAW/0tra2jBg5knHjxpGRns5bv/89OdnZ/br3YWFhODo5sXTZMpSVleWbEoGBgaxbv4GlS5YQc/IkvRIJxcXFuLm7M3v2bOrq6jAwMKC6uprly5fR1taKlpY2z61YAYBUKtLS0sLNmze5fPkytTU1JCYm0t3dTW9vD1KpyJIlIVhYWNDc3MyYMWOYMHEi1dXV3K6pwdjEhN/97nfs2LGDrq4u1q5bx/Xr10lLTZV5yWtr+fDDTVhZWfHDD3tYuVL2vufOnaO5pQUtbW3Mzcxwd3Nl6NCh8pThRC5evIC5uRkjR45EQ0MDZ2cn+a2FCq2trbzzTj5LlixBU1OTnTt3MmnSJExNTdm6dRsBAXMJDw9HKhVRUlLmwIEDTJkyhZMnTzJt2jSio6NRV1dnkJERdra2uMpXGrZu2dK/CvK4eYZSa/0efMj9GfDT+2HDhpGZmUlvby+5ubm4uclkoNJSU2loaMDSwoKgoCDSUlPp6upikLExHZ1dAPj7+aGsooK/3Pm3ePFiRo0ahZKSEseOHsXX1wcHh1H9a9XW1lbk58k0RfT09Fi3fj03rl9HT0+Pl156iaamJpYtW4qJiSkffriJmBjZLZ+DgwPdPT3cvHkTPT09Fi1cyCRvbw4flomjLFq0iLjTp7G2tqaiooLgxYsZ6+rKqVOn6enp6VfO7erqIr/gKlOnTKG1tZWxY8cwe/ZskpKSMTc3JygoEE9PT0xNTXF2dkZXV4dFixaRmpoKwP79+9m0aRMXLlxAIpGgp6eHVNonl8tawKBBg1i6dCnLly/D0NAAR0cH5s0LYO7cufRKJPj7++Pn58dgMzPGjR9PY2Mj+Xl53L59G0/P8QPBe//IuCcyrxPZmv7d7YEMeKMHWLFiBR/8+c94eXkhiiIuY8ZwrbCQvXv3MtvHB3Nzc8rLyzl48CCTJ09m8eLFnD13DmPjQVRXVZKens4YFxf8/P25ePEi58+fx9vbG0EQ8PLy4ujRo4wYMZwpU6aQkpJCbW0tpqam6OjooKSkxInoaJqbmxk3fjxfffU1tbU1mJmZ4eXlxe7dP2A5ZAh+fn6kpaWxZ88eWR04UeTWrVuknD9PTEwMUVFRvPrKKyx/7jnc3NzQ19fnuRUr2Lp1m0xJ91oh27ZtZ/369fj5+xMfF8eYMWMAcHFxITMzk6NHj7J06VLKysrYvn07vr6+mJqaUllZxbVr13Bycsbc3Ixbt26xZ89efHx86Onp4fTpOIKDF1Fff4eamlo6Ojro7Ozi9ddfJz4+AQMDA9atW8fWLVvo6ekhLi6OSZMm0d7WxuYtm1m6dAkjRox4/B/0M5RaKwjCfHk4+w3gHFDGLwxFH/DT+/r6ek6fPk15RQXnkpJISkrqb6+vrycyMhJLuSe8TyLpr6qampJKcHAwdXX17AgLI2DePFJSUmSKM9nZDB06lCtXrqCpqcG1a1dRUVEmOzsHqbSPje++i6enJzU1NYx1dUVZRYUZM2dSXCxbR6+oqGD37h9QVVXh5MkT9Pb2UlxcjIG+PmmpqZw/f54hQ4awfv16PvrwQ1avXo2kt5fe3h4Sz8jUagsLC2lvb8fVzY3w8HCqq6pYtGgRkUeO0NnZSVlZGeHhEejoaDN8+HCSkpLQ0NAkMzMLECguLuHIkSMIghLt7W2cPXuODRvWU1dXR1tbGw0NDXR3d9PR0Ym1tTWCILBs2VK2bdtOX18fa9euobtbVkZLSVmZuvp61DU0eOONN9DT1eWH3bs5deoUDg4OZGVly9/38XJXROMZ4S+AFxAviqKrIAjTgZ8Kff8RA97ojY2NaWlt5aOPPiInJ4eF8nX57du2YWNry6JFi6isrCQqKgqpVMq+fftAFKmqqiI6OoqXX34ZPT09/Ob4kpWVTV5eHlra2vj5zcHCwoL09Axee204ly9fITBwAZqamiQmnkVDQ4Op06ZhYmLCpYsX5bXq65k5cyYFBfmsWPEcIFtX1tLSJjAoiF27dsnTaDUYOXIkhw4exHLIkP5xKCkJuLm5Ymlpyf/8zyds2bKFJUuW4OXlRX1dHdOnT2fwYFM6OjpobGxg9GhnnJycSE9P75fuevfdjfj5zSEsbAehoaF0dHTwl798TGdnJ2FhYQwePJimpmYGDRpEcvJ5Ll++TH19HciVZvPycgGBPXv2oKKiioaGBrZ2doSEhNDc3ExXVxcjRozAx8cHVTVVent6CAlZTF1d3RO5p3+K1G4fRK8oincEQVASBEFJFMVEQRA++yUnKqb3yNRw7e3taWxspKenhytXruAxbhxdnZ1IpVIuXrxI0MKFuLu789zyZRgbG/P737+Fu7u7vPadwJEjR6itrcVrwgS+/PJLEhLOkJubS16ezE+wYcN69uzZQ0pKCn5+fsycOYOE+HiORkYiKClx+dIlWlta8faeyPTp08nIyOjXiG9obKCrqwtNDQ0mentzRR470NPbi5aWFieio/H0HM+CBQtITEwkJSWFOX5+mBgby67CiLz22qscOnSInp6e/nLWKSmpiKKInZ0dU6dOY9GiYMrLbxIeHoG5uRk5OTns2LGTt9/+PS4uLmzYsIGOjk5WrFyJ0aBBTJrkTUhICKamg5k1axa3bt3i7bfflhfj8GflyhUMH26PlqaGLM/+4EFeeuklKsrLKSwsZLj9cEaOHElubi7Hj/+iKsv/EaLIsySB3SQIgg6y9N29giD8A1nAzwMZ8EZfXFzcf2+7bNky4k6fJi83lzFjxjDR25tPP/0UJ0dHPDw86Oho5+bNm0gkvTg4OPQXi/jhhz1YWA5hto8P6mpq/ZVa/vGPr7h+/TrhEQc4fPgI+vr67Nq1m6CgQPLzC1i2bCmzZ8/i0sWLbNmyGUNDQzo6OrC3t+fatUIMDAzQ09PjueXL+eijj5g0eTIAgYGB/O1vf8Pd3R1NDQ1qamqwsLAAZF/q8IgDSKVSPL28+NMf/4Cvryw2ZMOG9ezevZuioiLMzc1ZsGA+8fHxHDhwgKCgQFpbWwgMDCQkZDHJycl89tnnDBliSU1NLWPGuLBjx06G2dvLdAHaO+RSWFNRUhJYu3YdHh4eWFpasmzZUo4ejaS3txd9fQNmzJjBxo0bGWxmRlJSEg4ODuzbuxcPD3e8vLxITU1DX9/gCazTP1O69wuQOfHeRJZXX8r/Fr34WQb89P7mzZv09vZSVFhIT08PsbGxOI8ezaFDh1BTUyMvNwerIUMoKi6m7GY5//jHV0yfPo39+/fT0dFBR0cH06ZNRUDkyJHDXL16ldiYGObMkRlaW3s78wIC0NLWJi4ujhEjRhCfcIbsrCxqamowMjJi48aNbN++nb4+CUeOHEEi6ePUqVMsXLiQ48ejUFZW5mZZGZcuXpAr0Ui5WlCAnZ0dVdXVdHV2cuRIJC0tLZSVleE9cQJmg03Jy8un4lYlJ0/GyBSAOjtpbm7hypUrGBsbU15ezrlzSbi7uxMbG4tUKvLJJ58yYsQIli5dSkREBLN9fCkuKiIrK5vk5GS0tLS4desWWVlZ6OrqkpiYyLJly6ipqaWs7CY5Obno6umip6fPu+9uZOzYsURHn8B74gRMjAfh7u5OamoaN27cIC4uDoDr169TUVHx2MNwRVFE8pR45x+EKIr3Bjbs+skD78OAN/pZs2ZRWFRE8OLF1NTUoKyiQktLC4sWLaKgoICN7/2B88nJLF26hMpbFaiqqDBvnuwH9dq1a9TV1dHU1ISNjQ0ODg7kZOcwb14A4eERzJw1C0NDQ/bt3cuKlSupranB1c0NBwcHcnNzsbC0ZPbs2ZSUlLAoOJiMjAzm+PpgZmZGa2srQ6ys8PLyIjo6mo//+7/JyszAz8+PjIwMrK2tmT8vgK+++gp1dXWCggIRBIGIiAhaW9uwtLQkNjaW7du3ExER0R8fsGfPDxQVFeHg4MCkSZMwNDSkvb0Df39/YmJikUqlBAcvIiwsjD/+8Y/Ex8Uxb/58yisq2LBhA11dXcyYOZM79fX09vbg6emJjo4O48Z50NDQiO+cOVhaWtLc3ExCQgKubm4giigrK5GdncPIkaPo6enmtddeo62tlenTp1NfX49E8mSqyT5FV/L7IghCK/dfmrsbnKP3oNf4Vab3giCUyQtVZN0VK3iUhTAeFicnJ9KvXOFoZCQhISH4+/sTeeQI+fn52NnZseH559myZSvKyiq4urpy7ZpMFOX8+RQmT57M3LlzuXDhIkciI7GxtaW1tRWQlcxSUVHB0cmJjz/+mDl+fsyePZv/9+WXvPXWW1iYm7N3715u376Nrq4uq+SqvFlZ2XhNmEBpqUxNpqW5maFDh9LZ1U1DQwPZ2dmEhoZSUFCAra0tGzasZ9euXcTHxzN+/Hg8PT1JSkpmyBArNDU1cXV1ZcvmzfywezdzfH1ZvHgxAHv37iMgIAAbG2sKCgqor6/D1NSU27dvo66ugba2NrW1NUgkEhobG5no7U1dXR0X0tJwcBjF7373O/bv309DQwPKysosW7aU6KgoOjs7CQ8P5+t//pPzycmkXbiAo6Mjy5cv4+jRo9y5I8v3Ly4u4fjx40yePBkTE+PHPr0XRdla/YO23zKiKOqKoqh3n033lxg8/Lr39NPlOcB3xQruFsIYDiTIn8OPC2E8j6zIBfcUwvBEJje06e4PxcNwN/f9iy++QFtbm9jYWMrKytDW0eHy5ctoaWlRW1uLg6MjiYmJdHV1kZiYyM6du1iwYD5Av+ddVUWVRYsW8cknnzBt2jRSU1M5duwYVwsKqJQn8mzfto3Ozk7i4uLIzcujra2Nb775hmPHjrFjxw40NDT45JNPZHXpVVTYuWMHM2fKxIaWLVvGt99+h5ubTHtPFvE2FXV1dbS1tQkPjyA5+TxX0q+wZetW6urqOHLkCBUVFSQlJaGiokxLSwuTJnmTkHCGkSNHoqamxpQpU4iPj8fY2ISgoEDOnUtCV56SGxwczNGjR7G0sKCrqwsLCwu2bNlCff0dTpw4wdBhw/jgg02kpqaxefNmAF588UWkfX0kJiZiOngw2VlZREVFc+zYMTo6OigoKOBoZCT6BgbExsYSFxdHVVWV4p7+CfFbmt4v4H+Vb3chU73dyD2FMIALgiDcLYQxDXkhDABBEOKQiQ3uf5g31dfXZ9r06airq2NhYcGMGTO4c+cOZ8+e5WZZGTt27MDe3p7Ojg4cHBxwcpLpFSYkJKClpYmKiqrcmFopKioGoKamltjYWPz8/Zk4cSLh+/cz3tOTtevW8f333+Pr64unlxfm5rLKwi3NzXh7e+Ph4UFKSgrOt27Jf0jukJmZia6eHqqqqqioqFBw9Sr6+nrk5xfQ0NDIqVOnUFVVRVVVlWnTpuHu4cGoUaMoKS5horc3Q4cOZf/+/YSGhmJnZ8vNmzdJTU3j1KlT+Pr6Ul1djbq6OiUlpVRX36ahoYGkpHOYmJjQ1NSMIAicPHkCHx9fKirKGTx4MNbW1swNCEBZWRmAwmuF2NhYExgYSG1tLddv3GDJ0qX9Jb7s7OyYP38ePT097Nq1i2HDJjJ//jwiIg4wYsQI1q5dS2trK2+++da/9815CAaCUT+IX+tKLwKnBUFIFwTheXnboyqE8X/4uWIXampqREREsGTJEm5cvy4ThtTVpaamhk8/+wzXsWMxNDREXV2d997bSFlZGQ0Nd/D0HM+sWbNYuDCI+fPno6amxuTJk2lqbubdjRsxMzfH1NT07vujoqzMvr17WRgURGBQUL/ibVxcHEELF3K7upqmpiYqKyt56623ZLcIcsFKExMT5s+fj6mpKRs3bsTc3AIfn9mYmZnh6+tLYFAQUlHkuRUrOHf2LFKpFC8vL+JOn0YqlSIIAj6+vuTm5jFjxgzs7Gzx8ZmNiooy8+YF4ObmSlBQIO7ubixeHIyX1wRGjBiJq+tYVq5cwcSJ3owYMZzQ0FBqa+tY/txz/ToCe/fsYenSJXR3dyMIApGRR/n444+5dPEiAFcLCjA0lHnmt23bTmhoKH19EpqamtDU1GTq1Knk5+cTERHx8N+ih+SuI+9B27POr2X03qIouiGbur8sCMKUnzn2Py54IYriFlEUPURR9DAxMfnRvu7ubkxMTFBVVWXGzJkkJyWxefNm1q9fz9ChQ0nPyOB6aQmzZ89CTU2NpqZm2TLac89x+rTM+7xv334WLgwiKiqKxYsXM2LECESplGvXrhEbE8NEb2+yc3Lo6u7GdPBgABoaG+nt7aW2thZ7e3va29vZv28fwcHBqKurczQyEn/5TKGrq4usrCzy8/JwcXGhuaWFw4cP8/77f+JM4hl2795NYKCsqtei4GBOnjyJiqoqi4KD+fzzz3EdOxaAxsZGpFIpN27cYPXq1SxZsoQtW7byxRd/xdXVlaamZrn01XJCQ1dSUVHBpk2bmDPHl5KSUvbs2cu8+fMZMWIEtyoqSE5OxsHBQa7Y28HJkyeZOWsWqqqqNDU1ER8fj/ekSdja2vGPf/yDRYsWoa6uTk9PLxERB5g71x9XV1cuXbrcr0L0OHnG1un/bX6V6b0oilXyv7WCIEQiuyd/VIUwHoq4+HhsbGzYv38/ykpKREYeISAggJiTJ1BSUubc2bP4+c3h0KFDqKvLSjLPmjWLjo4OlJSUiImJoba2hqioaHp7e7mQlkpPT68sdPXcOaR9fdTW1qKvp8vt6mqOHztGS2sLolTKH//wBxaHhHDq1CliYmLwGDeOPXv2UFtTQ2dnJ8ePH0dXVxc1NTWOHD6MlZUVJ0+cwMjIiJiTJ1FVUyP9yhW0tXXYv29fvxMqIyMDfT09WltbycnOxkToll8AACAASURBVNzMjJLSUiZ6e/PFF18wYcIEtm3bhr6+TPijo6ODuLh4Ll26xKBBg9i7d6/8qtdHaWkpJSWl3Lhxg7q6OpmE96RJVNy6RU9PNxZjxnDkSCT19fVkZWdjbGxCfX09HZ2d5ObkUH7zJhKJhOvXb5CamoKuri7p6VewtrZmx46daGhocOpULH5+/g/70f0bPFNhuP82T9zoBUHQBpREUWyVP/YB/ov/LYTxKf+3EMYrgiCEI3PaNct/GE4B/3OP884H+MPD9sdn9mxyc3MJWrkSDQ0NJH0Suru6+6+cEomEjo52goODkUgkVFdX0dzcRHp6BlpaWuzZs5cPNm3i8qVLfP7FFySdO0tw8CIAjh49SmZmFqtWhXLgwAEGDx6MkZERo0eP5uLFi1y6dAllJQGf2bPIysxk6tSpjB49mq1bt/L95s3s3r2b1atXA9DT3U1nZye+c+ZQWFhIdlYWq1evoa9PNn339fVlsJlMnLWnp4dBRkYsCg6mp7sbIyNDZs+ezeEjkeTm5jF58mTWrVuHIAhs27YdCwsLFi8Opqenm97eXlksv0TCtm3bWbw4hHHjPKiqqsLYxJg5fn6UlpZy+dIlxo0b1x+n0Nvbi5a2NkOsrEhLSyM7O5tBRka89tqrxMfHo6qqgpubG6ampuzfH05Q0EK8vDzp7OxEQ0MdbW2dx79Oj+KeHn6d6f1g4LwgCNnIRAFPiKIYy6MthPGLUVNT44UXX2Sb3Kuuo63DlCmTiYmJpampCWNjY4YPH056ejq7du1i5cqVzJo1i87OTvr6+njjjdcpLCxESUmJQYMGIZHIruxSqZTW1jYWLlzIhQsX+u9fL126RHl5OSUlJXz88V9obm4mPT2defPnU1xURFxcHNOnT0dJSQkXFxeuXLlCQ0MD1tbWrAwN5fDhw1xIS2PlypVcunQJNTU11q1bx7Fjx/oFPseOHUtTczMSiQR9fT1qampRVVXFQF+Pv/zlv8jIyKC9vZ2enh5MTE3plUjIysrCyckJJycncnJy2Lx5C2vWrGbMGBcqKirQ1dVl9apVnD59Gnd3d/z9/dHQ1MTCwgIjIyN6e3vx9/OjtKSEuXPnMmHCBMaPH09p6XVu3brFmjVriImJRRRFQkJCyM7ORhRF9u8PZ/78+dy6devBH9Z/yhOa3guCsFgQhHxBEKSCIHjc024rCEKnfKk6SxCE7+/Z5y5fxi6RL1E/tkSEJ2708ioeY+Sb0109MFEU74iiOFMUxeHyvw3ydlEUxZfl2mGjxXvqgomiGCaKor182/Hv9klFRYUXX3yRbVu3IpVKsbe3R1VVlW+++ZaxY8cwaNAgtm3bTmFhIfv27SctLY3k5GTi4uKora0lMyODa9eukZmZyaLgYE6fPi27/50XgIvLaBISEkhJSWXHjh2oqqrx5z9/gKGhIYIgcP36dbKzc3B0dKShoYG6ujrs7e0BcHNzIyszkwMREUyZOpVKeQZffX09tnZ2FBUVoa6uDsDzL7zAgQMHSD5/nvHjx7No0SJOnz6NtrZ2f05/ff0d7OzseOmll9i5cyc75MuBzs7OnD4dh7OzM5WVVXz66WfY2dmirq6OtbU1586dw9nZCR0dHUyMB5Gfn4+6hgZr1qwhIuIAFRUVWFtbM2zYUK5evcoPu3fjJy+rdfbsWUDmzFyyJIT33vsD3pMmsTgkhMjIoxgaGqKmpsacOb5P4Cr8xJbs7lvWSk6pfKl6rCiKL97T/h2yJem7y9NzHkVH7sdvacnuV6G+vp4TJ06grKxMZVUV5eXlaGpq0NfXx9WrVzl/PgULC3NcXFxQVVVh3TpZPYG4+HgKrxXiPzeAqurbaGtro6WlRVhYGNevX6ezowNlZWU6Ozvo7OrCy8uTwMBAzp47x9ixY3Fzcyc9/QoSSR/FxdfYt28fGRkZGBkZ8cPu3SgpKaGkpER7ezvXb9xg586djBkzBhsbG/T09Ajbvp36+npMTEzYu3cvUqmUshs3+jMB9fT0OBEdzaZNH2Bubs7nn3+Bt/dEDh06TFtbK5qaWly+fBk9fQOkfX1UV1cTFxeHl5cXOTk5SCQS9u8PB0RqampwdHREIpEwcuRINm/egpq6OnW1tSgrK/Pee39gyZIQjh07jpKSEpevXEFdQwNRKkVTU5MLF9KIiDhAZ2cnt29Xs3PHjv4qvC5jXDh67DggPpEClk/COy+K4lXgF49H7sPSE0UxTf58NxDIv1mq7UEMeKM3NjbGa8IEoqKiWLVqFZcuXWLy5Mk0NDSQmZmFq+tYlJWVGTFiBDY21sTHy0o419bIZKJ7enowNDSkrbUVa2tr1q9fL3OS6ekSEhJCcXExDnX1FOTnI4oi5TfLee311zly+DCLFwcTGRmJsrIyXp6eDLG0oLi4mEWLFqGtrUVaWhr6+nro6Oiwes0a0tLS8J40icrKSpYsXUrkkSOkpKayadMmrl69ynB7eyoqKlgsrwp76OBBfvjhB4yNjcnLy2XYsGHMmDkTQ0NDvvvuO9zc3QkODuZARATOo0fj6upGYmIir776CrGxp1i2bCnl5RWcPBnDjp27sLayQk9PD2UVFUyMB7FqVSitra1cvXoVH985qKury+IQfHxwcHTE3t6egoICSktLWLIkhMjISP7617+SnZNLQEAAGhoaNDU1MX/+fOpqawfKPb2dIAiZQAvwviiKyciWmu+9v/nJ5edHwYA3eoDz589jbGyMg4MDw4cP50R0NPX1dbz77jucO3eOmzfLWbt2DUpKSuTm5vL3v3/Jhuefp729nc8//5znn38eHR0dkpKSmDx5MuZmg6mpqZHVsEs+z9q1azEbPJivv/4nc/z8UFJSoqm5md7eXtra2lBTUyUuLo4XXngeb29vwsPDMTExQVtbm7lz51JTU0NKSgo3y8qYNGkSFRUV3LhxA1VVVWbNnElpSQllZWWEhIRQWCgLET4QEcF//8//kJuTjSAo8cEHH5CamoahoSG7du0iODiYrq4u0tPTEYG1a9ey+fvv0dPTxdDQEGVlZerr6zl16hRhO3YQIe/TxYsXefXVV0lMPENdXR3R0dG8995GMjIyaG1tZcb06YwYOZLvv/8ea2trLl64gIvLGOrq6uju7sHa2pr4+ASampowHTyYadOnExsbS2Vl5ePPp5eH4f4CHljL7t8sa1UNWMvz4N2Bo4IgOPEQy8+PggFv9Ddu3KCrq4tx48aRkpJCa0sLcXFxBAYGoqamRkNDI6amMuGJQ4cOIQhKlJaWcvzYMTQ0NLhdXS3zXGtpUVdXJ4t+W7mCO3fu8M9/fsNsHx/y8/O5XlpKZmYmWtraqKurExISQlxcPHp6epSUlFJeXk50dDSiKJKfX0BPTw+TJk0iOjoaZWUVIiIi+K+//AWArs5OEs+cYcXKlaSmpvL3v/+NF3/3ElKpFHV1dS5duoSZuTmWlpacO3sWJSUl7O3tqampYevWLXiO9+RuvMKFNNkPQUlJCdXV1eTn59PZ2cXYsWM4duwYVtbWxMbGIpH08s0332BiakpyUhLa2jp89dXXBAYuwNzcnLPnkmRBQD4+AKxZs4YPP/yQ4EULcXR05KOPPuK992SR1WPGuPD1V1+x8b33UFNTo7GxkY729icWhvsLeGAtO1EUZz30u4tiN/LCFKIopguCUAqMQHZlH3LPoQ8sa/WfMOCNvry8nOEjRqCiqsqQIUOQSCRYWlpy/vx56upqOX48Cj8/P1LTLrBk6TL27duHq6srK1au5OCBAziPdiY3J5uE+Hhyc3MRBIiLi6enp4f0jAy0dXTwHD+epuZmVqxYgaGhIdXV1Vy8eJFDBw8ybpwHenr6jPf0ZNy4cZiZmcmkpdTUCQwKAuDChQuYmJgQGxODvoEBByIiCAwMZN/ePSxYsAAPDw+qKivZsSMMQVDiu+++Izg4mGPHjnLixAn8/f05duwYysoqnE08i56uHlevXaOrs5OTJ08yffp0BhkZYm1thY2NNYsXL+bWrUqSkpKZPHkSAQEBmJmZoaSsTG9PL9NnzEBTU5Nj8iXJ6tu3ORoZiZ+fH0ePHkVNHhZ8584d0tMzqK6upr29g4SEBEQR+vokVN++zYnoaNTU1CiSr37INPQfH7/29F4QBBOgQRTFPkEQhiJz2F0XRbFBEIRWQRC8gItAKLIKOY+FAS+iMXXqVJoaGxk1ahRWVlacio1h48Z3cXJyYvny5UyaNAlJXx8zZsxATU0NfX19BpuZkZmZiZ6+PirKKvj6+rJqVSi2tja4jBmL/9y5tHd08Oabb2JpaYmaujrWVlbMmDmTzMxMPD09mT59Ol5eXkybNh11DQ2WL19OVFQUIHMAKSsr09DQwI0bN6itreWPf/oTI0aOxNHBgalTp6KnJ8vKA3B3d6e1tYWQxYvpk0hwd3PDb44vgQsW4O/vh7q6OgsWLMDBwYFXX32Vzs5OlixZgp+/P5MnT8ZKnsKrra1DaGgoJ06cxMFhFAsWLKCvrw9NTU3a2towMzOX1bE/fJiSkhLWrl2LoaEhCxYEEhISQnt7O1OnTsV/7lxaWlr48MMP0dHRYdKkSWhqauLv709w8CIaGxsZM8YF3zlzGO/piYeHBxaWlo+/rNWTW7ILEgThFjABWVmrU/JdU4Ac+XL1IeDFe5aZfwdsQ7Y0XcpjcuKB4koPwERvb1JTUsjOyWb16jUoKyvT3NxEQ0MDzs5OuLm58f3336Ojo8O8efNobW3lu+++45NPPiE9PZ3i4mLy8vKYN28eSUlJXL9+HSMjI8aOHUv4/v3sCAvjg02bAJg0ebIseCUri9ffeIPdu3aipSUrZjljxkwSEs6gpqpGyJIl7N+/vz9YBmQZgSXFxbzy6qvcuXOHzZu3IJH0smrVKgYNGsS7727k9ddfw8rKisjISIKDgzEwkFWDLS8vJzExkTVr1/aLXxQWFvLyK6+QkpJCSkoKBgb6qKiooKGhTkZGBubmZgQGLuDbb79FXV2DlaGhsluFYcPYvm0bn3/xBfnyjLm7CUTbtm5l5qxZCEpKmJubo6Wtzb59+3jnnbdJSEigra0Nf/+5mJqaEJ9whlsVFTz/wguUlpYikTzectVP0HsfCUTep/0wcPgnzrkCOD/mrgEKo+f27dsUFRURHx/PlCmTuX79Ok5Ojvj4+PDXv/6NTZs+oLq6GlMTY2JiYpH09mJja4uBgT579+xhvKcnp0+dYvRoZ0xNTRk5chQf/PnPzJ8/n507d6KlqYmKigqHDx2ir68PDQ0NTsbEMHXKFOLi4ujs6qa8vILKykps7exIS0ujt7eX8PBwYmNicJbXeg8ICKC1pQUDQ0O6u7u5WlDAYLPBRIRHMHz4cMzNzent7SUpKZlBgwYhinDw4EGcnJxxdHRg+/bt6OrpUl1dzYWLFzl9+jTTpk7lwIEDCEBUVBTjxnlQX19Pb28ve/fuY/Xq1Zw8GYOxsQnJycnyuPluKipuoaKqStj27ejq6XH8+HHq6upQU1dHV0+P9//0JxYEBhIdFYW6ujpXrqTj5OTMsWPHsbGxwcDAkIICiIgIx9l5NDt2yEIsHn8qu4j463vvf3UGvNGbmZkxa/ZstHVk+eOCIPRXpq2vr2ffvv3Y2dnS2NjE+vXr0DcwRJRKWTB/AY6ODuzbt4/k5GSUlARu3brF1KlTGTFiOI6ODowePZq0tDRKS0tYtmwpKioqXLt2jaqqKqbPmIGdnR0J8fFUV1VRXVVFQUEBt2/fpra2ljffegtDQ0MqyssJmDuX4uJiCgoKUFdXp72tlXnz5lFfX0/djBkMHTqUCxcu8v7773Pr1i0mT55EZORR9u7dy/z5XeTkZNPc3EJRUTFDhlixevVq1NXUMLewYObMmURFRfHuu+9QUlLKwoUyP0JRUTHOzk7Y2NgQExPL0KF2hIauJC3tAk5OzuTl57N23Tqam5vJzMjAx9cXGxsbOjo6SEtNJSgoCHV1dfLz8rCwsMDd3Y2ioiKUlZX6pcQKCwtxdnZmxsyZSKVS/vz++4//AxcVRj/g7+lBVrll/vz5IIoMMjZmto8PHR0duLq6snr1Krq6upk6dSre3t6kp6dzJT0de/thsnpsRkY899xydHX1sLW1w8rKivHjx5Oenk5DQwMFBVdZu3YtKSkpSKVSzp9P4a233iQnJweAyspKAgLmoqmlRZ9EwnhPT5ydnTkVG8u0adNYv2EDBw8exN3dHQdHR4YPH86qVaswMjIiOTmZDRvWU1BQgK6uDnZ2ttTWyopNVFVV8de/foGuri5L5bntw4YNY/To0cTHxzN12jTq6+tJSEjA3MwMFxcXGhruIJVKiYqK5pVXXubChQuALIBp6NBhZGRkUFtXh9eECbi5ulJUWMihQ4f4+L//m5iYGLlizn6ee245N2/epKOjg/T0dEaOHMnZs+eYM8eX6dOnc/SobEXLcogl5RUVtLS0EB0V9dgdeXJP3oO3Z5wBb/QtLS1YDRmCqqoqs318+PSTTzibmMiGDetxc3Pl4sWLdHZ2MmrUSABsbWyouX2bgwcPYWhoiKWlJRcvXqK3t5dz587x+uuv097ewerVq4mIiEBJScDMzIyqqmr27NnTfyVtaW6mpKSEUaNG4ubmxpd//zul16/j4uKCoKSEiooKmpqaKCkp8cKLLxK2fTva2to4OTuTnZ1NdnYOY8e6oqSk1C+KGR4eTmLiGbZv384LLzyPra0t3d3d1NbWoqmlybr16zl69Ci1NTWYmJhwJiGBnGxZABLAvHnzOH/+PC0tzVhaWiKVikRGRuLtPREVFWW++fZbPMePB8DN3Z28vDzMzMxQVlZm/XqZZJeBgQEeHh6Ul5eza+dOlj/3HH19fTQ3N2NlZYWdnR1mZoNJSUlBXU2d0NBQjh8/TnNLc78ox+NDBOkv2J5xBvz0/vz589ja2hK2fTsampp0yrXud+3ajY6ONkePHmPWrJns3v0DgiCgpKRERkYGqqqqdHV1oq6ujqmpSX947l//+jdqa2sICwtDVVWV1FSZtnxqahq2trZ0dHRgYGCAvr4+x44excjIiIqKCiwsLPD09OTUqVPk5ebS19eHnp4eyANWOjo7Kblwgfa2Nnp6ulFWVsHGxoawsDC0tDT7i3SUlJTQ2NhEdPQJWtva6JNI2L49DOfRowkPD+dqQQEgK2RpP3w4pqaD2bVrN5qaGmhr63Dy5ElGjhzJli1bUFFR4dw52Tq/g4Mj5maX+uvr6erqcvDgQWbPnk1EeHj/VL5+sClqqmrExMaybNky0tLSOHfuHI6OjuzYsUMegCNQUFDQv0JRVFyMpLcHFZXH/HUUgb5nXyTjQQx4o581axY3y8tZuXIlYWFhfPrZZ1y+fBkfHx8uXbrE2LFj8fHx6deVz8jIoKysjDVrVmNkZMTOnbtwdnbm5s2bGBoaMmTIEGxsrPH09JRXcp2HiYkJvb29aGpqUVhYSGxsLG1t7ZSXl/Pyyy9x6NAh3nn3XaKjoliydCnd3d2oqKjIikkOHoxUKuVOfT1WQ4awcGEQn332ObdrauThtp5oa2uTl5eHu7s7rq6utLd3MG78eCwsLGSVZt9+Gx0dbdasWUNnZwe9vRJ8fHy4XV2NVJT2/2BlZ+dgbm7OlCmTcXd3Jzs7m/LyCubMmcOZM4m8/MorREZGsnbtWtTV1UlPT2fmTJlvAqCnpxttbW08PT2JjIyULR96eHDZ2RlPLy+8vb3lx/WwIywMU1MTFi5cyObNm9HV1SM5+fxj/rTFATF9fxADfnqvpqbGqFGjiI6KwtbWFkNDQxobGwG4WpDP22//nqioqP5osezsbP7wh/dITk6mpaUFS0sLZs2axeXLlwkPjyAgYC5tbW18++13LFkSwoIF86msrKStrU02PXdyYv369Whra7NqVShXrlxBTU0dXV1dVFVV+fbbbwkICGDZsmUcO3YMURTZuWMHwcGLaG9vQ01NDVtbW8a4uKCpqUlUVBTz5s2jvLycAwcOMHXqVEJCFnP8+HHa29vZ88MPfP31V/1S1/r6+ixYsICEhARUVVUxNDSiqqqKK1euUFl5iw8/3MS1a4UIgkB6egYb33uP06fjqKysZPDgwaxbt449e/aQlpbG6jVrSEg4A0BiYiIeHh60t3dw/PhxtmzZTF5+PsrKylhYWFB47Vr//3zb1q2sWbOatrY2kpOTmTDRm76+Pnp7H++SHSBz5D1oe8YZ8EYvlUopKiwkOjqa0pISOjo6aG9vJzMzExcXWeWbwMBATp48yblzSUyYMAElJSXq6uo5ePAQU6dOBaCsrIzGxkaSkpLZuXMX2tpaxMbGcujQIUDmDTcxMSYuLk6eMz+NhoYGNm/eQl1dHUcjI+nu6aGkpITY2FjCwsLok0r58u9/x9V1LLq6ugwZMoTvvvsOH19fQlet4vjx4wiAlpYWHR0diKKIhoYGZ86coauzgzffeAMtLS327dtHT08Pb7/9NmaDzfqr8EqlUgICAggL20FDQwP+/jL1GmPjQRQWFmJmbo6GvIKOmpoagiCgpqaG5/jx7Nu7Fzs7OyZPmUJy8nnKyysYNmwYbW1t6OsbyNKVX3iesLAwVFSU8faeyMWLFwkLC2Pp0iWoq6vLbpUyM3F0dGTpsmU0NjbS1/cY9e9FxT09KKb3ZGdn4+TkxJ/ef58b169z5swZGu7cIer4cV5++SVKSkpobm4mNzeX5uZmQkJCuHTpcv/9uoaGBjo6OqipqePh4U5hYRF+fnNYIS8uAfDdd98xaZI3VlZWHD8exZUrV5g2bRouLi4EBMxF38CQOXPmsG3bNkY7O7Nsmaz4aEVFBX9+/08YGRly9eo1enq6KSwq5spl2fuXl5fTcOcO27Zto6qqmu7ubs6cOYOjoyNlZWVMnjyJ0NDQ/tdKS7tAV3c3Ydu398foa2hqkpeXx+jRzkRGRtLd3U1XVxe7du3mlVdfJS8vD+fRo9m9axd9fX3o6OpiaGDAKIdR7PnhBzQ1NTl79izm5uYcP36crKwsbGxsOHLkCACS3l4uX75Cb6+E2NhYxowZQ3x8PF1d3WRlZ2NmZsa5s2fp6e3t92/Y2to+vg9cMb1/+q/0giDMEQShUK448t6Dz/gxrq6utLQ0c+fOHSqrqggICMBj3DiqqqooKb1OR0cnOrp6SKUiVtbWGBoNwtjEhIbGRgyNjLC2tmLBgvkEBckSdHR0dFBWVqGhQRZduX9/OAsWLEBJSRk7Ozvs7Ozw9PQiKCiIs2fPERgYSFtrKx0dHZiZmWFrK5OpBjgRHcXcuQHMnz+fJUtC6OnpwcvTE/+5c5k5axZ9fX3Y2w9j7dq1DBliyciRsmqwzc3NjBw5it5emdOqra2N06fjmDJlMt7e3qwMDaWxqYnxnp5I+/r44osvUFJSJigoiKVLlzJo0CDshw/HysoKVVVVziYmoqqqirGJCYsWLWLmrFmYmpiydu0aHBxGYWdnx/Lly7CxsWXx4mBGjRrFwoULGTduHCYmxnh6ejJ16hQmenszafJkQpYsZeiwYYSGhjJs6FBmzJxJVVUVbm5uP/k5PTIU0/un2+gFQVAGvkGmqusILBMEwfFhX2fevHncqpBJWNXX11NVVcXG996jrbUVB0dHTsXG8sorL2NkaISamhoJCQm88soreHl5oaenz67duzExMeHw4SNMnz6NJUtCSEw8S1JSEiNGjOh3Au7atZvg4GB6enooLi5m6FA7NDQ0ZEq4+/fj4+PDzFkyvbz9+/YRFBTE/PnzyMrKYuvWrYSGhtLe3kZfXx/ffvst69atQ0NTk7179xEYGIiNjS3FxcVcuHARb++J2NhYU15ezs6du1i1KhRdXT3u1NezZcsWNmzYII91F7GyGsLt29VIJBIOHjyErd1Qhg8fzqBBgzhz5gyhq1Yxbvz4/ko5dXV1iKLImTNnuHPnDq+++gqlpaXk5eUyZcoUWlqaqays5OzZcyxfvhyJREJU9Amef/55cnNy6OjooKK8nLFjx9LS2kpqSgqODqPQ1dV9lF+P/4sogkTy4O0Z56k2emQquiVyCa4eIBxZcYxfTGdnJ+np6VhYWKClqcG7776LnZ0dDQ0NXLp8mXfefhs3N1eKi4vJzcvjn19/zdixY8nOzuZWRQW9Egn29sP59NPP6O3t5ebNm2RmZpKbm0NSUhIgygJ6rlxBEODWrQrs7Yfx5Zf/T64Mm05Dwx1qamrIzc0lPT2dtAsXaG6WGU5+fj6HDx/GykpWekpHR5dXX30VZycnysrKyMvN486dO9y4cQMdHW2+/PJLLCzMSU9PR19fn48++i9Gjx5NdnY2VVVVfPrZZ3h4eJCXl0fK+fPo6OiQnp6Ovb09b7/9Dn19fXR3d6OtpcWbb77JqJEjqaqqoqKigtu3b+Mxbhybv/+ehIQEioqKMDAw4Nq1axw5cqRfS7CoqIivv/4aB4dRZGRkcP78eUxMTMjIyMDY2Jh333kHu6FD+wOYUlLOY2FhQVdX5+P4jvwIURQfuD3rCE/zIAVBCAbmiKK4Xv58JeApiuIr/3Lc88j0x7C2tna/O30Gmdptd3d3//O+vr4fBYnc+/xf94nijyWe7t0vlUp/lDX2c6/7r8c/zLn/euzPnXs3g+zu/p/rw4P6JJFIfrSufu/+u9+pu/+bn3ufvr4+6uvrUVJSQhRFbGxs7pttJwhC+oNy3B+EYKAnMtXzwQcej/+P3+u3zNPuyPtFiiNy1ZMtAB4eHj/ar6Ki8viDQhT8LHp6v6ju4qNB4ch76o3+pwphKFBwH8QB4ah7EE+70V8GhguCYAdUAkuB5b9ulxT8ZrmbcDPAeaqNXhRFiSAIrwCnAGUgTBTF/F+5Wwp+s4jwOIN/nhKeaqMHEEXxJLIqOAoU/DyKKz3wDBi9AgUPheKe/qlfp1eg4CEQn4iIhiAIXwiCcE0QhBxBECIFQTC4Z98f5NGj32LyLAAABV1JREFUhYIg+N7T/h9Flj4MCqNXMHAQeVIJN3GAsyiKLkAR8mrK8mjRpYATslp13wqCoPyoIkt/KYrpvYIBhPhERDREUTx9z9MLQLD88QIgXF704oYgCCXIokpBHlkKIC/LvgAoeBz9G3BGn56eXi8Iws1/aTYG6n+N/jxCnoUxwE+Pw+Y/fuW2zlOczzL+BUdqPKis1UOwFoiQP7ZE9iNwl3tr1lX8S/svCB389xhwRi+Kosm/tgmCcOVpD7t8FsYAj3ccoig+svLPv6SWnSAIfwIkwN67p92vW9z/NltRy06Bgt8SD6plJwjCKiAAmCn+b4LLz0WQPrHIUoUjT4GCR4wgCHOAjcB8URQ77tl1HFgqCIK6PIp0OHCJeyJLBUFQQ+bsO/64+qe40sv4d+/Xfks8C2OAZ2Mc/wTUgTh5puEFURRfFEUxXxCEA8gcdBLgZVEU+wCeZGTpU51aq0CBgodHMb1XoGCAoTB6BQoGGAPa6J9k6OMvRRCEMEEQagVByLunzUgQhDhBEIrlfw3l7YIgCF/J+58jCILbPeeskh9fLPck3213FwQhV37OV8K90j+PbgxWgiAkCoJwVRCEfEEQXn8ax/HM8ks0w57FDZnDpBQYCqgB2YDjb6BfUwA3IO+ets+B9+SP3wM+kz/2B2KQrf96ARfl7UbAdflfQ/ljQ/m+S8AE+TkxgN9jGIM54CZ/rIssFNXxaRvHs7oN5Cv9fyyq+TgQRTEJaPiX5gXALvnjXUDgPe27RRkXAANBEMwBXyBOFMUGURQbkcWCz5Hv0xNFMU2UWc7ue17rUY6hWhTFDPnjVuAqssizp2oczyoD2egt+b+hj5Y/ceyvzWBRFKtBZlCAqbz9p8bwc+237tP+2BAEwRZwBS7yFI/jWWIgG/0vEtX8jfNTY3jY9seCIAg6wGHgDVEUW37u0Pu0/WbG8awxkI3+aRLVrJFPaZH/rZW3/9QYfq59yH3aHzmCIKgiM/i9oigekTc/deN4FhnIRv9EQx//Q44Ddz3Xq4Bj97SHyr3fXkCzfNp8CvARBMFQ7iH3AU7J97UKguAl93aH3vNajwz5a28Hroqi+PendRzPLL+2J/HX3JB5jYuQefH/9Gv3R96n/UA10IvsirYOGAQkAMXyv0byYwVk4gulQC7gcc/rrAVK5Nuae9o9gDz5Of9EHpX5iMcwCdl0OwfIkm/+T9s4ntVNEYarQMEAYyBP7xUoGJAojF6BggGGwugVKBhgKIxegYIBhsLoFSgYYCiM/ilHEARNQRDOybXTf+k5rwiCsOZx9kvBbxfFkt1TjiAILwMqoij+4yHO0QJSRFF0fXw9U/BbRXGl/40iCMI4eW65hiAI2vK8dOf7HPoc8mg0QRCmya/6BwRBKBIE4VNBEJ4TBOGSPPd8GIAoE2ssEwRh/H1eT8EzjkIY8zeKKIqXBUE4DnwMaAJ7RFHMu/cYefjwUFEUy+5pHgM4IEvPvQ5sE0VxvFzI4lXgDflxV4DJyPLSFQwgFEb/2+a/kOUIdAGv3We/MdD0L22XRXn6qiAIpcDdEku5wPR7jqsFRj3S3ip4KlBM73/bGAE6yNRnNO6zv/M+7d33PJbe81zKj3/kNeTnKxhgKIz+t80W4M/IyiJ99q87RZmajLIgCPf7QXgQI5AlrCgYYCiM/jeKIAihgEQUxX3Ap8A4QRBm3OfQ08iy2h4WbyD+P+iigqcUxZLdU44gCK7AW6Iornyc5yh4dlBc6Z9yRFHMBBIfJjgHmQPwz4+pSwp+4yiu9AoUDDAUV3oFCgYYCqNXoGCAoTB6BQoGGAqjV6BggKEwegUKBhj/HzZ7Dg5rfK7QAAAAAElFTkSuQmCC\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "model.quick_plot('land_surface__elevation', edgecolors='k', vmin=-200, vmax=200, cmap='BrBG_r')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "As with the `var` attribute, PyMT adds a dictionary, called `grid`, to components that provides a description of each of the model's grids. Here we can see how the x and y positions of each grid node, and how nodes connect to one another to form faces (the triangles in this case). Grids are described using the ugrid conventions."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 15,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "\n",
+ "Dimensions: (face: 2914, node: 1512, vertex: 8742)\n",
+ "Dimensions without coordinates: face, node, vertex\n",
+ "Data variables:\n",
+ " mesh int64 0\n",
+ " node_x (node) float64 750.0 1.5e+03 ... 1.939e+04 1.96e+04\n",
+ " node_y (node) float64 0.0 0.0 0.0 ... 3.839e+04 3.883e+04\n",
+ " face_node_connectivity (vertex) int32 211 106 107 210 107 ... 79 80 78 79\n",
+ " face_node_offset (face) int64 3 6 9 12 15 ... 8733 8736 8739 8742"
+ ]
+ },
+ "execution_count": 15,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "model.grid[0]"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Child initializes it's elevations with random noise centered around 0. We would like instead to give it elevations that have some land and some sea. First we'll get the x and y coordinates for each node along with their elevations."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 16,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stderr",
+ "output_type": "stream",
+ "text": [
+ "/Users/huttone/anaconda/envs/excom_demo/lib/python3.6/site-packages/pymt/utils/decorators.py:60: UserWarning: Call to deprecated function get_grid_size.\n",
+ " name=func.__name__\n",
+ "/Users/huttone/anaconda/envs/excom_demo/lib/python3.6/site-packages/pymt/utils/decorators.py:60: UserWarning: Call to deprecated function get_grid_size.\n",
+ " name=func.__name__\n"
+ ]
+ }
+ ],
+ "source": [
+ "x, y = model.get_grid_x(0), model.get_grid_y(0)\n",
+ "z = model.get_value('land_surface__elevation')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "All nodes above `y=y_shore` will be land, and all nodes below `y=y_shore` will be sea."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 17,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "y_shore = 15000.\n",
+ "z[y < y_shore] -= 100\n",
+ "z[y >= y_shore] += 100"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 18,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "array([-100. , -100. , -100. , ..., 99.85786119,\n",
+ " 99.65789436, 100.00178402])"
+ ]
+ },
+ "execution_count": 18,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "model.set_value('land_surface__elevation', z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Just to verify we set things up correctly, we'll create a plot."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 19,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAP0AAAEKCAYAAADZ1VPpAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4wLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvqOYd8AAAIABJREFUeJzsvWeUHNl1oPm9iEjvy3vvPbz36G402pFNcihSNKORRI1GmjMz0mokrc6udkbD1WhXI+lIZ8URJcpQpIZskm2ANmgD2wAKQBUKBZT33ldlZaWNdBH7I7NbEKdJdFNd7CFR3zlxquLGy4iXGXkz7rv3vvuErutsscUWDw/SR92BLbbY4sfLltJvscVDxpbSb7HFQ8aW0m+xxUPGltJvscVDxpbSb7HFQ8aW0m+xxQdECFEshLgohBgQQvQJIf5dWp4hhHhTCDGS/utJy4UQ4k+FEKNCiHtCiO0fZf+3lH6LLT44CeDXdV2vB/YCvyKEaAB+Cziv63o1cD69D/A4UJ3evgR85cff5X9kS+m32OIDouv6gq7rXen/A8AAUAg8A/xdutnfAR9L//8M8HU9xQ3ALYTI/zF3+12Uj+rCHxVZWVl6WVnZR92NLT4gt2/fXtV1Pfufc448p6THEg9utx7R+wD1PtFXdV3/6nu1FUKUAduAm0CurusLkPphEELkpJsVAjP3vWw2LVv4YO/gw+GhU/qysjI6Ozs/6m5s8QERQkz9c88RS8CJ2gd/5b/bHVd1Xd/5PvpkB74H/Htd1/1CiB/Y9D1kH1n++5Z5v8VDhSQevL0fhBAGUgr/TV3Xn0+Ll94x29N/l9PyWaD4vpcXAfMfxvv5UdhS+i0eGoQAgyweuD34PEIAXwMGdF3/o/sOnQG+mP7/i8BL98m/kPbi7wU23hkGfBQ8dOb9Fg837/dJ/gAOAJ8HeoQQ3WnZ/w78V+A5IcTPA9PAp9LHXgVOA6NAGPi5D6UXPyJbSr/FQ4Pgw1F6Xdev8t7jdIAT79FeB37ln3/lD4ctpd/i4UGAtDWg3fwxvRBCFkLcEUK8nN4vF0LcTGctfVsIYUzLTen90fTxsvvO8dtp+ZAQ4rH75KfSslEhxG99/7W32OJ+3nnSfxiOvJ9kfhy/e/+OVPLCO/wB8MfprKV14OfT8p8H1nVdrwL+ON2OdKbTzwCNwCngz9M/JDLw/5HKdmoAPpNuu8UWPxBZiAduP+1sqtILIYqAJ4C/Su8L4Djw3XST789aeieb6bvAiXT7Z4Bv6boe1XV9gpQzZHd6G9V1fVzX9RjwrXTbLbZ4T4QARX7w9tPOZo/p/wT4j4AjvZ8J+HRdfycv6p3MJLgva0nX9YQQYiPdvhC4cd8573/N92c57XmvTgghvkQq55mSkpL/6XgymeT65TdYHOugs7ODpgo3AL2jS+i6TktNAQAvXujj5N5KbBYjAOdvjnFybzUAIzNe1jdC7G5KhWPPXR1iV2MBGW4bAGcvD/D00UYAVrwBJubW2d2c6svNnllMRonWmrxU20sDPLKvCrPJAMA3znby8UfasKev+53Xu3n2ZDOSJLg7tEhtWSZmkwFVjXPl9gSPHqgBYHEtwNScj72tpQC80T5MRI3x1JEGhICuwUW21eYhBITCMTp6Zzi6uxKAgfEV4vE4LbWp9/7c6/coyfewtyX1/ganNqgrdQFwb2SZRFJjR32q7dtdk+RlWqkqyQLg5UuDnD5cgyRJRKJxOnpnOLyjAoCO3llCkQhHd6U+x7/97gXOd8y91238UHgYzPcHsWlPeiHEk8Cyruu37xe/R1P9Acc+qPx/Fur6V3Vd36nr+s7s7H+ayfk//u6/8+V//xTSyF9QZbyHmQANOWGa8iI4LTKVuQaa8lWCviU+fiAPgxagKS9CPLCIzajRlK/SlK+ikCA/0/rufpbLSIEzQlNehJnZBRpLzBTa/TTlq/j8QaqKXTTlq0SDyxxsdJLjNtGUF8Eh1jjWlglxP015ERKhRbbX5TIzt0JDboSmfJWDrTmMTi3QkBOmNjdOoT1AQ06I8dkVSvPMNOWlrhsKhijOdbzbpwy7wvEWF+PTi9TnhNlVBnbhpSkvwtziGoWZBuqyQzTlRbCZBRX5qXP5vUt88bEStHgYOZHql8OcpCkvglVfoyrPSK7H9O51qovseOwyTXkR6rJCNFc4SER8qessrFCYZXm3jwVZFtRI5N3P3G42vNct/FAQpBx5D9p+2tnMt3gAeFoIMUnK9D5O6snvFkK8Y2Hcn5n0btZS+rgL8PKDs5k+lCwnh0XgNMXpnUkZHzuqrAzORVjyRijMlNARqLEEflWiscyGP5qy/xYCCo0lFpZ9Uc7fWWdPrZlkLEQkmmBlPUJzuQNvUGNqKUx+lo3H9+QxNBfjWq+P3TU2QLDoDROKydQWGgmHw8QTGt1TCfbU2tmIpK4z45XIcDs40WLmrbtBAGwWEydbbLx0c4OSXBvegMaZW34e3+Ekywbr/hg9EwGaSi2EQkF0XefCHS9762w4bSZONFt4/rqPigI7cz7B3fEALeVWjjQ76J1SmV+NUJQpo+sS/lCMqCZTmWeioiSHFV+Y4fk4yUSSYDjG4HyS3dUmIuEgiaTGhTtrtJUqbASiAFy46+Vwo4PlgE4ioeFxmHGZ4ngDMW70+2guVmiuyqVjVEXXdWaXQx/0Fr5/xNaYHjZR6XVd/21d14t0XS8j5Yi7oOv6zwIXgU+mm31/1tI72UyfTLfX0/KfSXv3y0lNT7wFdADV6WiAMX2NMz9KX480u8hz6rx4c4Msl4mVgEz7cJSWUjM6Eq90+jnWnDLTw5EEG8EY2U6F3fVZDM2qIBvIcRk50eahdyrK1YEw2yptJHWF7qkk2yrMGBUZf1QQTigUZhpJaBrXBlUONVgBON7q4uWbK1TnmxBCEAgGSSY1XDaFpJZSluKMJLfHVXRdwmkzcrzZyrVBlQvdS+yuseOwyOyocTGxEmfGK1GZZ6QyV2Z2RSUhTJTkWFHjOnarkY/vdfHSzQ18wThzPkFVvpEMp5m1kMytsSjNJSaSCN68G+ZIuo/BYIiDDU7m1yLMLnl5rSvIY9vsAOyrtTI4EyGOiUyngdIsmFpS0SQbDpsBWYvw3atL7K4ycrApk6H5BL6okYJMIzarmblVldH5CPFk8ke5he+LLe99io/CmPlN4NeEEKOkxuxfS8u/BmSm5b9Gei6yrut9wHNAP3AO+BVd15Npv8CvAq+Tig48l277I1GSa+HpXQ7O3fYzPLVChjOlfH3jq2yrtGNQUh+VnozwepeP7ZVmJElw8fYMB+ssAFjMBvxRGafdiiQJbvYtsr3CSM9EkOuDYS60DyFJguuDEc5d7iPDrtA+pHJtMMrdGbgztEIikSSe0NhVbeFv35ihqVhGS6aekjaj4O7APO33Jnm5Y4Ob4xoWY2osP7KYpH86jMVsoL1/lW3lqfF/U7mTl64t0FZmwGyUSSRTIyBJgny3zrkr/STiCdoHwwzNhlleXSfLaUQIwZ2BRfbV25HSmtBWbmJoLkw0FqdvZBld12kfVhmeDZHltvB27xr1hSkLpbXSxeiyht2sk9R0slwW5lZC3JlQWVyP0T2yTkNRyuBL6oJjzRZeuDpPMrl581AEW448+DEl5+i6fgm4lP5/nJTn/fvbqPxj2uL3H/sy8OX3kL9KKsXxR6brTi8XZuepKvagI8jJsHLj7iQmg0I8KTG75GN+PZfFdRXQsZpNdA/PcqHXzejkIrIkePH6Ei6XC6tJ4ertEcoK3LypZzG36KN72MS+hgyqrDL9xZlU5ylkOGQ6cly0llvIcKTGsFOLIfKzHDjNSV7t8GK1OZheDNA1kcf1OxNIej7leVaOb8/ibPsyLeU2SrIUhqc3qCkopiLfxPxKmD9/aYJVX5D++XwG5mMgYNkbYGhBY2o5zM2+FdYjBmxmQV2pi5MHGsjPVNhVZWFmKUjP6BLBUISFFStzS16u3LNgt5kRQhBW48wveflXj5eDYkNC42CdhbH5AGfaV5lbWGNkKZvJlTiS0Dh7sZ/DOys4ezPCwXob2xtK2Fuj0DHkZWpuhe4JB9NrGgNjy/iDHgyShPp+5r7+qDwkT/IH8RC4LX4427c1kemyUZVvYH+tmbKsJJ96tJ68bBciGWRvUx75bsH+OjP76ywEVI1tDSUcrjdQXpzJb36mHo/byaltNg43mMjL8tBS4eZAjcSnTlYRTpqwmg283uXnC48WMbqkcXs8xq9+vIK3+/9x/HpnMs6/frqMKa/CM/uy0JMq5YUe9tXInD5YSVKYqS220Tul8gtPlDEwE2ZuLUH/TJTibDMTCyE6JpI8ub8At9PGnkqFEy0WiEf42UcriahxSrMkEHCy1cKhBituu0Kux8CKXwOgYzzBb3ymiZaaHAxGI//lF9swGg0carDz5C4XiixTkp+B3aJgM0kYpDgr/jiVBQ5Kc00caCmgIhsO1RtoKISsDAcIiYYyJ1luM7F4ynSfWpX5vV9oQ1FkDtRZCEXi7K62EIjEkDZxTC3YGtPDltIDcHqXi8s9ASLRJB1jCXZUmJC1CP6owqldOQzNhFj1x+mf9FNVaEPSYjx/bZWTLTYURcIg4iytx3n1lpdPH81lbl2na9hHRY6RTx3w8NINL5FogqSm4/OH8PpVZFmircxA17jKynqE4iwjkiThC8a4MxqgOMfOp4/m85Uzk7SVG8l3aYwvxpCNNiwmhVM7PNybDOMNJTh7a4NATOGZ3Q7G5wJ88ZEibk/ESCQ0dNlIbbGdqlyJb19e5JOH8znXFQDgfNcaLaUmsm1J/v78AoeanGQ4jSz7EhRmWzEoMp84kMlb3Rucub7E/nobB+ptvNW1SqZN41hrBrfH4wAsBRQe35PL+FLKPL/QE6GxIotPHMhEVWO8ejvIRiDISzf9nN7lxmiQKc2Cv3x1ml96qpyr/UHUWJxYQtvUe73lvd9SehaXVvjulQWEHufLf9+Hqsa40BtjajnC2NQyF3pUdCS+/to4Z9sXWA3oLPvjDE0sc3Uowbk7IdaDCb7x1iS5GWbcdgPFHo3eqTB2q8yZm+vkZVqJqjEu3lmmd2SB2UUfr3eH6RoN0Te+xlvdfmoLDfRNBolEorx6Y44lv8714QTrgTA3RhIgKdwe8WMxCq72rPDSjXWMZjO9Q3OYzSb84SQrG1HCSRMZThPrfpWXb3nZX2uidzLMtFdmdmGd17v8JKIhOkZVUGyEozqDcypzS35uj0W52Bflxt1p1gIab91TudQXxSALesdW6J1JMrIEl27PUZ5nAsBpSvDyjSVaylL7oUiY56+tcaDOzPjMKi9cW2HGC5Ks0H5niqW1ANeHorx2O8DEYoKFlQ3ah+OYTQqr3gBJbXNrS2w58rYm3JCbk8X2Q3mML4SJxnLYU2enPNfEy1EXOZkujjUaAANevx2P3cyRBjM+v5l/cayMpICWRhuJhIXf/dt5hqa8LG0kMRstDE8Oc20gg9M7U0+1KwYj+6tkTFYX8SQ81mYlqZm5eGeV6z1LXLFo7KhxYTUr7Gsr41ijiYt31/nSU3VMrGhU5Cpc7FhmccXAp4+XkOM28eYdL9vqCzjVZgF0vvHWDCu+OG+aTIQicabn19DR2V9nY35N5dc/20L/bIKqPIk3OpdZXA1glvPxuOxkuGw8vt1OWI0zMZNBTYGRyjwjmqbxwnWdnc0VnGyxoGkaV26beOnaEiUFGSSFlZv9U5htHvpnwsyvhllbD2AxJCnIy8TlsHK8JRX50LQGImqcR1pTjs5Xbq3RXFPEo21WVn0qr16RSWqb6L1/SJT6QTz0T3ohBJFokqF5jc8ez2N8BW4NbdBSZsRtirK8HuXlWz4e35mFJJvQNA2XzUBDqY3FtRDLvjgv3Vzntz5bT0Whm9M7HMRjYY7tKmZ0Zp2BuZRjKhrXeKXTx4E6E3oiTCAUR5YkgnEj+1sL2duQRW6GFZvdTlhNoGkacc1AWZ4FtBhqXKK6rIDCvEwynQYSCQ2EgV3VdqaWVV7p9LO3pYi6ynwebbNisZhoqi3m4/uzWdlImes5bgO+gEphphGPw0pTTSGZdomGIiM2RcUbiPFml4/PnSjk7nhquHP25jqPtNmJJ1LKeK7Tx799thqDycLeGjP5zihPHihDFhq7KmRqSzKor8jDbjVwrDUDpznJ8HwMAKvZwIkWK2/3h4klklgtZmSRMuffHohgMhhIJjdzTP/gAhrvp4jGTzoPvdIDvNIZ4LHtqXhzIBBiNShTkmVkV62Lb56fo6XCTqZDwSyHefHaAi0lKQOpOl/mj77VQ5Zd4LAaCccV3u71UZlv4ck9OeRkOnGa4pzpCDA9u0qm24rZqPDYjkzuTMZ5o8vLwQYbJ7dl0DUR4+7oBlW5ggxrgn84P8++2pTJfKzVw42hEHa7mZOtdm4Nq7zWuc6BWhMBVeerZ4bZV2+ntsBITA3TN+mnscRKZTbcHQ8w7xM0lqRDeMUKb3SuUFlkJ8+p0zMVoTLfxJHWLAZm49jsdowGmad3e/iz50epLbLisCok4lFW1lUyXGYcVoWTbTauDUbon9PYW+8mpvr5k+8OYTQILCaJ6/eWMBt0dtY4GZwOsh6IYVaSeJxm0OJ8883U+zNKiVT4rsRKJJZAbHLpuC3zfsu85/XzV3Cgc7EvRiKZZGzORzKRRI24USSN+ZUNOoY3GF6wYzTYuTM0hMXmxGpMkuuUqavIZ8UX4cytDTp7psjLsmFUMglHYuQ5k1zu8ZPlcTM4vojVauISCkaRoHdkmcaqbLKcqZBdPBphJCBoMuos+pKMzXnpGM9GEjFAZ3ZhHSF0DHIh7d0zCCGwWs3EwlHKCjNo7/djs9kxSEluDGxQkm0knFBo757ikYMNXOqLgoBYXOHtu/Mc2GElHIwyPLnE691OnGad7qEV6kpsXOqDQChKMBLjRt8q3pCHRDzK5T6JTxzwABBWE3QPzBNNaFywmghEktSV57C/1szgdJyFTCddQ14imgW7zcLvf/0ejVU5+CMCo9HExPwU1wYzMZskrvf7KMiOoaqxTR3Tb5n3KR56pT9+eB9db7/IiaZUQoqm5RGPJ3l6t4O5lTDl+XYWNgQnm02MzAYpL8ygtdRIvkdB0zSW/BBNCB5tsxGPF2G3yGyrsBKMxJha1IioSU7vsOGwNhBLCo42mEgmFW70JujqX2DN50IxKMwsBtkIhNheWUG+O4mtIZeiDEFNgRE1lkCNZmGzGDlUb2Jy3klZrp2DDWbOdsR5co+T1aBMW4WF5y4mmFvy88lDNbjsJvzBGAVuQX2RiRVfhKsDEfY2FXKw1sTITBRf0MMjLRaisSRn3l6nutDM8XojsYRChr0MNSHRWChx/uY6ZlOAa4MWJBK4LDoup42yAjeHGy280uEkHgsTiycZXkhSX2KhJNdGQYaRWEJjeiGf8kI3hxutXOhaZW9DNk3FCncG44TCKqd3lfBGu3HTa8RKW1q/Zd4bDAYON1i52BNBjSXwWAWtpTL9M1E6RsM0lNqwGRLMrMYZnIefe6yIawMBorEkNwd81OZLxNQQl7tX2F1rpzRLpmcmgcdhZnxVoSzXyovXV9hRYUDSInj9cc7c9PGLp0soK/JwvNVFNKbxsQN5PHu4mPWwwBsxcGp3LqNzQbyBBC93bHCixUokpvFap5dTOzysbETRNB2nzUBhloXpldS42ebI4BeerKBjNIYvGKW5wkn/dIjBmQB3J5N8bK+H07sz6RyLMbma5IndWfRMRUhqGs8cqiEQMxCJJjl/x0tLqRF/IITFpLC9sZSjbbkoks6+WivjS3Ge2JNJJKYzMhugMlfhcJODvukoLoeFvfUehuZT4/UzNzf4zJFsZD3G1EqcqG7m8T259M4kCGtGnthbwMBcglgsgaZvYshOpJT+Qdv7OpUQfy2EWBZC9N4n+7+EEHNCiO70dvq+Y+9ZCOaj4KFXeoAsl5F8l85Xzk7TVm6kLM/GxHICm9WBLAkONLr49vlJMmwJdF3nY7tdvNEdZjVsINdjojxb4XL3AsFwjMIsI6FwlEt3vdQUWqgqsHBveJnXO1dQ1Sh/8lw/qhrh7rSG3Wrh97/Ri8dlYWxZZy2kcKNnnt7hWZ5/e5FoPMn/+w+9qBGV26MRrneNoCXiZNgVjjRa+Ob5OcqzU1/ShgK4fM9LkUciP8NMWabOX5yZYHJ+A13T+Yc3xnFYBWosiSRJrPsjGI1m8jKtLPsFb931s6PKwjN7PLxxN4RQbNgtCsdbHHzjrXmaShRaKuysrq7y+9/sw2XR8dhkIqEAfTNxaotMeBxmusf8FHtSj2tfIMyrnT6ONDkwGmR2VNl45foMsp5aRyIQDGEyWqgvteP1hQlFYqBvriNPUeQHbu+TvyVV1OX7+WNd19vS26vwgwvBfAhv6UfioTfvV1a9TM2oqNEYy94AF3tCmC1Wltb8bPhDyHIJwXCEWDLJ/HKAFzcSmC0W1oNhlpZ9uOxleEwSuZkOHCaN9t41wnGZm71z7GrMJxxWKS3K4PFdOQihM7kUp640g7ZyI2E1Qc+wgz2VCnZL6lZs+J0IOYNT21MlCEJRaC2301xmYWg2F38wxkvtq5gsFnrHVsjNzmRoQUXTZS60j/LIgXquDCZQJAMr6yF+/nQp3aMB9jblk+WUuNDtRTLYsBgVhiYXmSuTCIXCyLKR632rrAVhzR8jGAhhNJSg6Toj015qyrJZXo/QWpXB3fEgejLOizfW8AWS+PwrXLRb0IGhiWXyshyMr8XYCMWYW1hHp5B4LIYsEiQSScbm1onEBUI20Dcyi8lYipBMxOIJ9E2071Nj+g/nR0XX9Sv3l3R7AO8WggEm0vNLdgPtH0pnPiAPvdJnZ2VQaDPzvetRvvwLbZy/F+ZEs5l22crYvMKJZhNqTCbbVYqG4GCdmZnlMN0JC267hcP1Js7eDFCcZcJqMfLIThvX+31UlWTw8f3ZnLsT5pEWM6/eDvHUbgettTmo0Sj9sxIraz4+f7KQGyMxTrYojC+EKci2Mb2SehKOzAbYVeNgYlkjwxZme5WT0fkIz+xxokaTGEUlBgMcaTQDEImUY1LgcEPK6+8LVDG1qhHFwhN7HHz3mpdn92UjSYJbQz5MJgP+CIzNriNLEl94tASPw8jz172UFng43mTkfPc6P/toOaoGOypsDM0EeOpAIaGYxMdbrbx000+mx8axxlRMX9KrWN2I8oltVl4MmChuzqeh1EK+x0ksodE+FEWSZA7VGzl700tNeS4nmk08376OJElsbj4eSJufcverQogvAJ2kFrlc54cXgvmxs2XeA692+jje4kCWJRSirGzEWQ0ZONDooGcqmppVV2EkHNW5Ox5kYlXw1C4nRR6NmZUoRrONx3Zl0zeTiq8HYgYy7Ao3B9dpKJKRZYnqfLja58dhSrC3zsmaL0z/dJgst5lEVGUjFKdvJkFrmRGPJc6KL8rgPNQWmjGLCBfv+mkqNbOvzsKd8ShvdPs53GRD16IsrMeZWgpRV2xGIc7Ucoz+yQ0aio0EojI2SypC8Gibnct9Ydb9KkFVpjg7VQ/gY4fL2deUy0ZEcL1/g13VNsJhNZUSK5loLHMwsxQiFk8yOK/RXGYnFJW51r/BzioLNXkwPBfjrTs+tpWnpvxe6g3jdlg40ebman+QeELjXKeP3VUGonGdiJrAaTdjlmO8dXuV7ZX2dG7+5jra3ueYPksI0Xnf9qX3efqvAJVAG6l16v5bWr61rNX/SvT0DpLpNJKRDp0d35ZJ51gUh8VAeb6d0fkIRpOFmwNeLt0a5XL3MvWFqY9te7WbkcXUlFdJkgiqOq92bnCw1kRJloHxxThFmUbmViNsRCReujLK9GqSt3qiBKISU/NeznVHMFtt/Ndv9rG7OhVL39/goWcqRpbbyMJamPUwrG0EuToQY24tydCMH7vVjNEgc7TZzY3BMHfGo9QWWtjf6KJjOEj/bJyyXDMTs17ynRqapqPrOmtrPv7ouVQ8XVLMXOicZWhWZX4tynMXJhiY8pPvlin2wN+/Nc+h+pTVcHqXm0v9ETIcqT76A0FCMSVVbafEwchiHF0xYzYIJpY1bvTOMT67xtVBlaYihXNdAWxWMxaTQiSqcq4rwL4aExkuG91j68x740SicTRt8571QjxY4dNKv/pOpaX09p6LV34/uq4vpad9a8Bf8o+zSf+XWtbqoTfvZUXmSuc8OkVoyCQ0wZ2BGXI9doSUy5I3SDSq8rMnSvCGJIxGA4PTAW5EVGxWC2+0D7OvKY+bQwn6hhdACFxOG5NzceaXNnipXVCWY6Aqz8qhbWWUZsm0lJt4vj3M6b1FVBcLXFaZ9rtWbo8EQE5itSicuTLM8T01WI0Kz+xx8VqXgYN1CqGIzvmODeaW/RgMJSSSEIsnGJteJifTQVIXOBwmOu9O8LrNwtDEErLQ6JuUcVkFkViCttp8DtRZOHNjlaPbCynJNVGRa+MlLcLgdJhrA0EMRhvzy3Nc7s8EIRBCcPHGGC21hbyqJrk7vIjFbMRqLiWpw9jUMtF4gljEw4EGO7sbi0GSONRgZWTGR9fgHHmZDmRFYXBsCU0XCElwpMmGphfhsupYTDJqdLOf9Jv3nBNC5N+3XNXHgXc8+2eAfxBC/BFQwD8WgvlIeOiVvqGumjbXINcGozy5240AwpEiZFniSIORYCiDRFzFZpawmM3kOJO4HS4O5aYsgxVvMfnZFnZXW5hdy8JhNXKozshG0IHbYeZ4qwu7ReF717x8bI+HtwdjXO/3s73STkmWzPUhlVWfn198spSZNdheaWVgOkhdWRbLaxt47BnE4homEcEXNHB9IMIXTpXTOxWkNl9gkDQu+iUOtOSR5YCafIXn2wNUl2ZxssWCItWiyIKjTRYiaoLrgxHUaIRQJIHZYudoi5nXOjdwWmzIJif/8TNFfOeql0faBOOFbooyFeqLDLzQvs5/+HQDXRMxTm2zoWulFHk0kKG1zMhGIAuTkqStws6V/iCnd7p5rdNLPGFifBm+9HQdt0f87KwwsL7uQRcKu2udmAygJSNMrshoukDb5OQcRflwlF4I8T+Ao6SGArPA7wJHhRBtpEz3SeCXIFWp4hQ1AAAgAElEQVQIRgjxTiGYBOlCMB9KR34ENrMwplkIcUsIcVcI0SeE+E9p+d8KISbui2W2peVCCPGn6VjmPSHE9vvO9cX04hgjQogv3iffIYToSb/mT8UPWSv4h5HtNnNqu43vXfPynbdXONZsIRaN0N63Rn2RwumdbtqHYxiMCturnHSPhdA0nbe61zne5mB+Lcq1vg321NqR9Rh3xzYoyzHw5G4PV/rCvHVnnYP1qUkmS94wmjBQlmNIDwkEGS4r2W4rqwFY90eZ8er88jMVZHoc7Koycq5rg6Rk5r+fnSDToRMKR8l1Gfjay6P8+ZkJ8p0JSrJkJuaDvNS+xLZKByfbHFy5t062A3yBVP251277ONRg5tFtHi71BsiypxTs8Z0uLt0LYDQoaDocrLPwx88NYjHKXOya4y9fm+VYi4MctwGXOc7cWhyLxUhrpZNQWOWFq0uUZQkcxjhfPTPE4soG44sRtpWb+Nqr01QV2ijIMPDELg8X7oWw2Bx84mAWQ7Mh3upao3fSz6Pb7MRim6sHgtQw7EHb+0HX9c/oup6v67ohXRbua7quf17X9WZd11t0XX/6/kUqdV3/sq7rlbqu1+q6/tqmvcn3wWY+6aPAcV3Xg+llfa8KId55s7+h6/p3v6/946TMnmpSpay/AuwRQmSQ+hXdSeoX9LYQ4kzaK/oVUqWtb5CqoHMK+EAfaN/ACLeX/bjsZopzLHT0LXCx14bFauP1m8N87HAZPpNGIh4FyQCYONFi5WJPECGMzCwGCISi9I/6OLyrCrPNznMXBzm4vYJZb4LJhXXCYRVJKWF4Mcbt3iloKOScqqMoMhdvDbOrsZjnr4aYml9jadXNzx5PlcI+1GDh7mSCuiITwwsaobCKTAItKchzmyjIzaClwk5RhoQ/opHpEJy9NovJ4sRilHj1+iS/8Zl63FYD524tU13oBGBkXuVy5ySnDtZxoS+Gpulo6NwdmCESdJPtligtzKC+xI7FoHNv3M/1wSh2S4KyLDN3JqK4bRIXu/1ENAsd/YtUFFRSXehgfsNASY4Jpxl6J/xMzHmZXs5kzpskntQJRVSGJ9dR5FIUxULn4DR5mQ4u98dJahpCbKJ/S2xl5MEmKn26qGUwvWtIbz/sjj4DfD39uhtCCHd6je+jwJu6rnsBhBBvAqeEEJcAp67r7Wn510ktnPGBlL62upzO+TtU50sUeIyo8QKON5l4+ZaPhqp8SrIV+iYCTM2HWfSGsFmq0IHzHRNsq82jodjNSgAkKYM9lTIGReLt22ZONKccbS9GVESWk2ONKQdYPFkHus6xJgthNYGkV5LUJR5ps3P5TpwrPau8eF1CMRgxKAZu906xvzWPp3a58fpyyHA7qMgzcXfMz5EWN0PzGtsrLeR44OxNL83Vqam2d8YC5GTamV2NE4obudw9x8FtZsbmN9hVZefw7hoisQRP7EjV93uhPcLetgoO1hn4zttrfOFELl3jKhidtNWY2F9vwmlVuDvqo6tvgWyPhU8dKSDLZWRs2klBhgFvIE5zuY25dSjMMXNjNMZ/+vk2LvaEONWUGg692qmQUVPII60W3ujy8csfr+fWcIhjjUb+UJGIqB/k7n1wJPHQ+643fYUbOb2U7zIpxb2ZPvTltAn/x0IIU1r27mIXad6JZf4w+ex7yN+rH196J/yysrLyT44pisIn9nvonwpybypOLJ7k7V4/zeV2PBYdkDjYkk1ujodnjxSTaYdAIMx/+FQDQjKQ6TSCYuXZfZlcH0p9Y3c35nF3KoamaTgdFlpKZHqno4TVBA6TxkYgkgph3QmkylaZE0yvxAnHJHY15HGoOYOn93hIJuP8wtNVbIR11gMJakrcjCylvNtzPpnyXBMOQ4TF9RhqLIHDZsYoJ7g37ieBmX91qgRFllDVMJ97rAq3w8DH9uehKDIlmRI1eRKdY1FW1iMUZZvQgOeve3lipxODIhONS7itEo9ud9M5lkrzzckwc2JPGTmZLlw2hc4hLx8/mMfIosbwkqCuyIw/GGJ6KURlnhWzUSbPqTO6EGcjGMNlN6HrcL1/g4r8lNm/o8LA7bHUZ7KpyTm8b+/9TzWbqvTp8EUbqRDFbiFEE/DbQB2wC8ggVR0XPqLFLt7heIuTNa+X197uQ5IkSrMVjrZm0Deb4LVOH4carFTlW/n2m8MsrIUYW4hSna/wl69OUeTWsZgVAsEoPWPrlGbJeINw8Z6PtlKF8nwbo/Mql7q9NBQZOdlq59ztdUqyzRgUiV21TjpHg6hxmcd3ZXNrROWNLh+t5RZyXQpP7XbzUvsy4UgERU/5GmoKUkbagaYMemaSnLvtZ3+tiWQywdnrc+S7dDJdZqbXkpjNZprKnJhEgpGFONcHQjSWmKkutBFRo/zDpUVcZo03rg5QkgG2dHZge+88bWUp30MgpBKJJmgfUtlfa+b0DgdvD6jMb0gUZVnom1insTj1+91aauSN22u0lqf2t1W76JkKce5OgMps6OiZoGtojTV/jJuDQea8SV44P0Qiqf2A2/oh8SHm3v8k8+OqhutLm+OndF3/w7Q4KoT4G+B/S+//sEUtjn6f/FJaXvQe7T8QXu86k9OpFNFoHDxOC3dHVgnEZOwmneFpH8lEgk6jhdnFFXY1FVOYaaS51MTiWgSvP8ytwTXmN7JIJhN87+0VfudzdYzMewklDe9Wu91Xa+Jb51OVbFCsXLkzzbE9tbzSGSQWVTGIKPcmAkiyzuCkD1lALJFL57AfLREnEAwzFotRUZLHuZsjnDpQxao/DLrG7b5ZhJBBSIQTRtrqS1jzq3SOhrhxd4rHDjZydUBFFhKXuuYIRRKcvSVw2K2YFCMOqxktmSA3044/otE+FCGmyUTVKLcnNWQRJdNp4nf/uodnj5YzthDGYhCEQwHmVlQu9ZmZnFujw6qwvOEgosYZnlzmWp8LWZHQdUjEY4xMrlKanUdVSQ7VxU52Vafq6YfVOINTOdzqm0aNbbb3/iGocf0ANk3phRDZQDyt8BbgJPAH78Qy0572j/FPY5m/KoT4FilH3ka63evA/y2E8KTbPQr8tq7rXiFEQAixF7gJfAH4sw/az4wMD80WI2NzcRJJF/u3OYnGk5xqs5BIaLx2zcuzR0tpqTDxWtTFE7vdvHk3jCRJXBuK8pufruFyf5xHWszEE0ZuDy5x9uYamR4bl68Ok+2pR6CjawnW/CF+sa2E9WCMXHcNq354fLcLcLERjHJrcI3Hd3iQZBPxeILHttlQZAdqLEH7kAOzSYZkjOJ8DzsrTe/W4p9YyqI8z0KeSxCM2dgIhsnJsNNWZUSN6+Q4oanUjKZpzC4ozC752dNUwN7aVGnraEJicCHIv3mmnBdvrHO42UT/VJDW6gyKMgTlOUaCYcGtPge1RWZ8/hjL3hhzKxHcdhP5zgRfOFXD8LxKbaGJqz1hfucLzVzqDfPULhtCCF7yxWmuzmNHdQahRJx5b2oFHE3T+KtXZ6kpy8RpNREMxX60L9z74uF4kj+IzTTv84GLQoh7pFajeVPX9ZeBbwoheoAeIAv4L+n2rwLjpFal/Uvg3wCkHXi/lz5HB/Cf33HqAb9MakXcUWCMD+jEe4fppQgz6xIH680YDAo1eYLh+RhTS2E+f7qO6TWdF9vXeTw9CSYYDPH89XUe2+7EaJCJRUMEIwlev7PBr326FoPRTIkrwSeOlLG6EWNvrZVAOM7nH61geDFB53icHZVWij1J+mZSX/LLvQEe2ZHHqj+O02bg1HYHVwdSinHutp8DdWaicQhGZT57LI+u8dSxN7t8nNzmJhRTuDet0VRq4mBTBncnY8QTScpzLUyvpkp2LXpVCvMzeWRXMW5rkhfafYwtxojGErgdqeq3T+1289a9MLM+mWf259E9EUbXdc7fC/AvjuYzswrlhU5kg4l9LQUU52cyvAS1RSae2u3ifPcGksGMy25iV5WJK/0qYTVBjsvIo21OOkajmAyC5mKZgRmVP31+nG21WZza7iShafyIUdf3RWotuw8nZPeTzGZ67+8B295DfvwHtNeBX/kBx/4a+Ov3kHcCTf+cfq6sellZSPJIW6pcliwJ6kqcvNzhR9OhtlBnbtFLJBLnnFFCQjA6s44iQ5fLhlEO01Ri4TtXltjTkI3bZqAuP8qbd1b57MkSqhI6Z25tYDZaqC628jevz9Nc4UIIQWO5g1c6fJhlM9luC7urXPzFKzN87EAeVrOCngwyNJMkP9OKEIILN8f4zc/WYjUrLG9EmFgIkem2kO1U6Oof51Br/rtK4zDGOXN9naOtOQzPh5lfi9E+HOXZfW6EsPB6d4hn93u43rvKq5eHObmvjte7BZIQDE2sYDIKxgok9lYb6ZqIYbfZKMo2c28mTK5bZS0kcazZyAs3fFTkpQtm9qyjRjXGZ5axmCswKjKzi2tcu+PncycLkRUTC6t+irLNKIrMn32vh6oiD/luCa9fJZlMpQpvGlshO2ArI49gKEQsAZf6YliNCWYX/LRLLsanlwmpcWrziigtyAQET+1K/TAIWUEIONpoRNMUznctMT6zRk6mi7l1DVk2Mjy1ztXBPMwmA6veIMteP8FgBmMzK0TVMHOrHoxGI2aLhb94oZ/TRxpoH44zv+JncjWPseUoBpOVv3mtn8M7a3jl1hpCwM3hCEI2s+YL8jc9qxzaWc3VQRVZQO/4GssbSaxWK2aDhZu9Y2i6hCTJPH9hjNbaPF5sTyBLMv1jy6z5PFQXOjiwvYqyPAvbKlLhu2gsB7NRIR6PcWsqyc2eGZqrsrg57GRuYZnO3gifPl7KzHKIjp4pFLmCjbBGU5mD9UiEmtIsjtQryJLALOzMrwZZ9sNyUGV4aoX5ZQNHWzOoLsnmsd25CC3G+a5VorHNnXAjED8VITshRBGp+fmHSKX1RkgNk18BXkvn/v9AHnqlLy8tpjBnkIia4KVbEcxGmR2VZrzBHCRJprbExkJAJc8RZ3g+iseikecS+IMRVjcUDDKouo1DbQr76k04bAYGZ4I8c6wWs1Fid42JQNBBhsvME7s8uF1OhNA5lp4Oe/aWj3/7yVq6JlSe2uXk3oibwgyF0hwjL1z3cnx7Ec0VRkZno/zqJ2q5PRbj8TYz3RYbigTVeTJlOQa8G9koRhOnt6WcY32TAXY0FNBYmUldgQFNEygGA4+02rCYJJKawOM04TQl2V3rpGcqQlu5mRsDPtoqbNwe3KCy3k1NEfjDebRU2GgstbKw5sJitqKqMVRVw2W3sqPKQoFH4Vqfn93VNoxSnN4pFUUk0CUTv/6pKt68p3K4wUw4Vookycyth/j8yUKuDcc4WGvBYoOktrkhOz7ENNyPirTzuxB4GfgDUuFwM1BDKjntd4QQv6Xr+pUfdI6HXukBxhdCDMxpfPKAh+sjGmdvbfDkLjc3BjcYnE7isSrUlTh47XYQVVV5eo8bSbLw+p0gITXBx/a4iCdtdE9EqS/UmV4TPLbdyUs3fCQSZjwOhV1VNr53dYW2SjuDs1F03cTle35aK+wUZSnIssq3L69wpC2TzpEA3eOCA40OclwGzt8Lo0aN5LhNuC0RpldiTK8KPv9IERe6fcSSAllRcJuiLK4rKEJn0a/w6aMFvHLLy+i0yrHWDDx2me+8vcqqL8QXHyvj/N0AGyHBM3scmJQk9yZVfKqBfZlG3Ntc3JmIsuhVefZAFrfH4wzNhqgqtNNUYuTFGz4ONjg5vM3C7ZEQUrWFcEKmMFMBFF7pWKQkz87RJiOSJDBJMZ677OdzJ/M536NiMJpx2Awk1FW+cSHGL54u5v/5mr7Jcfofy3z6zea/6bre+x7yXuD59ArOJT/sBA+90nf3DDDes8QTe/Pwh2L0Di9zYmce0XiSxhILf/bCKD9zrJDOwRCTM+uEVI3rHivxZGqM3VpbyGsdqxxr9RCMybzeHeaTBzIAaC5R+Oork3z6aCF3J6J0DcwhKCTPpfP8tUUKshz0jKzRM2XFajbRfncKg1LK5LyPYCSJ2VxBKLSOzx+hvCgLgH0Nbl5s9+FypGLgx9vcvHl7jak5H4+eLuFCn0ooHOdkq42u0SAOm5k326dwuTwYRJS6EivfGV3irW4fITXJ9PwqIl264tqdSRoqsni5PUCuW+HeeITdjbnYLanpsMMBjad2G0kkNXZUmPjq2VE8Tgvlxbn84bf6eXR/LdeH48gkGZpcwiDFePFaAA3Bqk8lGktybSjOxVvD7Gks5mq/YHk9gs8f42JPyjEpNjVO/5Pvvf8BCn//8Rgpx/YP5KFX+rbmeo4VTTK7HOSl6z6GJlaoL7GhqibiiSTRaByBREGmgbwMKwaThYN1JiJqAouhjpnlMEdb7PTOxHjz6ig7moq42BdD1zXiCVhaC3Bj0MfOagdPHalGjUvkZ8m8cqOPpw9aOL49E4tJ4caAn+bqbJ7cncn5XhulGRpJSaa5JZs3Oha4eGsUk6EGHYmNYIjp+RWMShESSWRJZ3ElQPtggMsdU1QWZ3JrIM6eBg/nugI8fbAUjwMq8y3cG/PxyM488rOsTMwHMJdksrcuZVGseKPsa/RQnKWwHohx8c4qL10ewx+p5NqdcUry3bzcoZOMR3FZddrqinDaDByosyBRhUEWHKhLDVtiiVoiMY1H21LDjbOdQVx2C0cajKytF1OQZWJ3tYmNcDY1JQkqCxQyXWbmVwKber+F/NMRpxdCPEkqqlVKSo8FKX+480Gv/Ym3dT4MrGaF+Q2JPY0FfOqxFgIxA60VdqZWdH7ncw14VYX8TCtmu4t4QiOp6bx518+OShOndji5Nhhld7WV4/uq0XQ4XG/gZIuFYChGa3Uuj2zP4upAhP21VkxSDIdV5pkj9ayFYNmfqiEfiisUZllY8IbJd8s0ljnwboSZXUsQTij8y1PlSAIO1JlwWo1UlqXmxO+rs+OLyPwfP9eMkIwc2FaO22HnxI4c3uwOcmqHi111GQzOpxaanFiFI63ZTK/p6AY7nzycx5UeP8FwnLoyF+PLSSwmhRVfnE8er+CXni5ndT1ES3UOpXlOmsrsHGnxEExYOb3TRTgK57u97KpOrcSzvBGjf9JHUQbIWhRvIM75bh/7aq1ous6bXWscabKxGkhVz8mwyxxs8tA9ESWZ3NxiWakiGvIDt58Q/gT4IpCp67pT13XH+1F42FJ6AF7t2KAs10K+W8NhStBULPMXr0xzoNGG3WpgPaix5I2Q7xIcabJyd0LFbkutBOO0GSn0CHqmYphNMs/scXL2lo/XOtY51urg+DYPb/f6KM42I0uCw00uOkYiKHKSx3c4eaNjlj/57ghHGs3IQnBjSKU1vRjk0WYnt0f8KIqR8nwbkqYyNBfDZrdjV6KsbMQ417nOkWY7RkVmzR8FITjZauX3vt5HW4UZpzVlzBVn6IwvxnDYUh56r9fL8MQcZ64toMUj/J9/1U22PYERlTV/jFmfREWeiVyPheJMnevdUyR1hbduL/KH3x7Aqqio0SRRNURMM5LhMHK8zcPNIZWhuSRluSZObPdwdSCCbDCR7TKQSGpowkiGw4DDoPLdtxfZVpGaiJT5/7P33lFyXPed76eqq7qqOndPzjkDMxhgMMiZBANIkZQlynJY27Ls57V8HN6+t8G77+zau372Pq+t57W8XgfZlmzLNEWKJBgBAkTOwCBNzjlP5xyq3h81lCg9USQljmyK+z2nTk/fqltV09237u/+wvdrz5LMbHyJuWCxvOf2EcEM0Gt8HzHOj7153zswTDSZYSGQ4fXL89RU5DOtS4xNrdJT7MNjz+KQErx6PcXPHi1FFAX+7vQ0HbU2bgxECCcMYgmd/ukQdsWCYVQwuxgik05jVTVki8DJq5Ps66rn5N0EFouFibk15pdAIJ/2GidXkzqv3IgSjybJz3NzeyTA9KqOZrdhlSVu9U7hcjaRTitcuTqNZhX51IEyUwCyzkveeqrvwU12njm7wJsJJ9ua8rjau8LArAe7ZsWjWbg2HMYm6Zy652JmOU5thY+HutzIkog/qnN7NIqi2fjqySkKXFZevQVOTaI6305HcyW1xRa8mh2LRWBLnYe+mSRZ3cLw2AIWi4VMJsPsop9wNMkVn0ZaFzl3c4yD2+s535/g9JVB9neUcHVYwGK1M7m4yNm+fETSCKJCMpXdWOY4QUSUlfc+7qOBfw28JgjCOcwydgAMw/jD9+r4sR/0m1oaKaueYimQ5OS1ND/VbONcb5Rfe7qVpTC0lkuc7DEYm1nj0lA+kkUnHInRNwVP7imkQZGwqRI5QaHYZ8VqyXBoWxnzgQyH22QW/Ul2tpfjcVjZ22LOsolkHjZFortR5eUbOpVFTj7R7eQPvr7EcmiVpw+UsbVRQRAEXrwa5PD2MmqLBUp9NuIJLxaLhbtjIQYmlpAsArGUBwOBTNZgYGyJzxz1sbPJwwtXgzy0xYZkEZlejHB3YJb9ncXsapSxKRXkMklWIzqlPgvNNXmAyIE2ld8emOFoVwlt1WZewkogwZY6JwtBgUjMwqf3FXJ7MsP+VgfzK3Eaa4p5uNNUpr0xJDC9nGZPi41z9wJ84akWliMGOxut3B3y0lDuor5U4WRPgNoyLwdaVTTFwuB02Ews2lg/3kfJfH8v/A5m6boKWD9Ix/9l3gP+cIqbY2l++fEaJpfTqJpGeaGdeX8W1SqhyBa+8FQDS/44XbUKnW0VfOZgCVeGU9hUiQV/ktpSGyMzYXRBZnOVlWQiSjqT49pIiid3F+LTslwfSXJnJEhruakcMzQdoaHUSlulwtBMlO1t5RzsLGQxLCIIAgv+JFVFdvZuyuP+VIZAOEWxT8Mw4EBHPo/uacLlUKkvtrC7UWZ5LcpvfKaFdCbLS9eCeO0SZ3rj3B0LM7ws8Tu/uAWXy8ObdyLsaFTZ3+7jxmiSoekwZR6DWDLL4HSEzx5tZGguRSRupu9e7I+yqUolEk/htCs4bVbCkRSReAarZqfAkWPen0LXdVajIjabylIggWiRqS1RCcUhkcyyvSWPvpkU/nAKu03lM/uLuLJejjyyBDndYCMT8kD4UTLvfYZhfNIwjP9oGMZvvb29n44f+0Efjca5MJDk0W1OCrwap2+vsK3OfHDWFMD4YgqLpFGar/GJHW6+cSWIrkOeW+UT3W6ePb/CP5yaIRCDiblVkukcoWiaA5s9nL0XorLQTKGtLdXoG5nntatzrIZTlPngxK0VKvMs1JY6ePX6EnuaFbbUOhGMFHcmM1wfTdNZa5qjVjHF8etrdDdolHl0XrwwR2OpyLHtHi71hfiLV2d4vNtNdbGDUELkyZ0eOmutvHq2j5M3l4jFoqwEk6yFsxT7NCSL+dV3VEmcuLlKXYmKmI3RO5OmsUzhyV1eXrsZJKfruJx2RAGmphe50z9Fz5CfR7rcXBpMYlNEdrX66J/N8cr1AAfaNBKJBBf74t/05OfSUV67vkJ7lZW2MoH//vwIW6okJEkkEo0zOBWitsiKntvQ1ByT4FO0vOf2EcEpQRCOfj8dP/bm/b3efhYXAlwYsqHrEAzH6Zs1sAgJLILIs2dGaShzcfyqHdmq4HPbOHt9GI+jGVnUyWaTVBS72dtk5VavwtYamct9IbKixrmbMxze1cyFgRSqJcOmWjdW1UZHrYOztxdZDcQ51bNMzhBZWo1wdTiBIEoYqNzvnSOdNXhTVcjmcozPhMhkMpy6nyQWzdE7EaKoMI87U3Em5wLEkhlO3Y1it2tk0km+cjJEZWkeD+5pxUDkoS0aI/NJrtweY+eWal67lUPXc6RTaRZWw1weSiNrTgbvTPCGXSOVSiHkkvzxC2M4NSsvRZ3k5XnxuW0U+SSuDqe4N7KATZEIx3zc6pvBaVdRVY2R2SBOTeLcgB1J1PG6FK73L6E53DhVEbdT4WtvLdBYU4xFsvKVN8Y4tLMZi0Ugm93Y7/vDmskFQfgr4DFg2TCMTettPuAfgWpMYsynDcMIrFeU/hHwKBAHftYwjJ4f8Ba+APxrQRBSQIYPELL72A/63Tu7WHVMsxZLc2izjVi8kkA4wePdblOkstdOY1Ue3Y1mvPnErSC/8IkmZtYMuhs1QvF8sukEl3uXefpQOT0TGR7sKuCNW0GOdFdSWSDRVGYFFI5fy2HTQJEtpAUX//FnS3n5RpCndrjxOB0kM/Bghzmz+0NePE6Ng5s0zt8PcrS7nKnVLA+2a/hDAr2jS7RXyUSTGVxaOY3FIn0zGfa2WHn9phVVsSIJabrq7ayGkkwsZ2mttHF4VzNWi8GhzeYa/PlLq3z+WB0p3SAYirKlwUdzmZXqQjuJpIv//vwID24txaEZhJM2FvxxNFVhT4vKWrgQQRR5bLuLdDpHJmuwr0UhHnOQQ+Fgq+lgvD+WwOuycbRD5a3ba3z+kWqGF5LkMDjQ6mBsxkNHpXnshvrx1kN2HxL+BvgS8NV3tP1b4LRhGL8nCMK/XX//b3gX/scf5OKGYTi/374fe/MeoKPOSXuVxDeuhHDZZbrrZU70RHjtVoj/7fFqMrkc40sm3ZPdrlJTbCOXSfF3p+bZ26LyUFceF3oDlBfYWAulOd8bpKHMzoPb8hmaiRKOZ0kks+S7ZUo9BmdvL9NWoSJJIt31Gmf7YjhU8NpyjC1mzFJUj5VwPMmFvhBFeXYaS2US6wRyZ/ri/OZPtXK+L8GVwRQ7GlR8LoVcLs0zZxdpq3Zgs9lIZK0UeWTaqpz0TycwDAOHIhCMJkhncvSMhNhS66CuzMn0qsFKROSxnUX0jISIJbIcvx7i1z/dyEwQbk9m2VSlcHSrj5tjabJZHY/DSkMRDM6m8LptfHqfj+PXAsSTOtvqVO5PmU7lCb/ELzxaycWBJFlBw2mX2VbvxGnN8NLVAAe35HNpIGoKcmzkFy0IiFblPbf3g/Xcdv93ND8BfGX9769g8kW83f5Vw8RV4G3+x+/jXxCq32O/sF6Q86742M/0MxU7JlAAACAASURBVLPzXB9aw263ke9RuTeyiEUsZm4xQCSe5orHgdVi5Y1rcyRSWXZtLuNsX5KFYJa5lTC3p/IxDIFYLMWZ3iSxZJqhiTX2dVZik3M81u3hxathLGR5ZLuXeErki/84xMM2O4uhHLoucunOLB6HSl2pxuRShltGjtZyhZ65ABO6wZP7ygAZq5DizJ0VttW7OXfPz62BZYp8di4PaVjQmVyIML0QJs/r5kLPOD9/rPab/+e2WpnjV1bYXOtma62HSwNxkhmBzjpzhtUsCeKGgGEYtFcp/M5X77Ozo4pb4xl6h+dw2SSCURtep5VcOskLl0I80l2IKqv87t8P0Fjp4eqwl7ICG984NU5JnkYwbhYmbau1Y1MlMukws4tRrgxZTGaetMTd4WnmVhwU5bnIGcYGz0LC+12z5wuCcPMd7//8farcFL1Ne71OAFO43v5uPI8LfHD8viAIIvAScAtYwfTg1wOHgCOY7NGz73aCj/2gl2QJ0SKzr9mKplhIZ4rZ3aSyFvFQoVg5uC4GGY06GZmNsrNRIavD6xGZlpoCuutkrLKFYKgCh6LjUXXEEh9ddVZuj4a4OgiRRI7Z+TW8Hgfk4jjsCl31tm8y34TjFVgsInuarNydTPL8qT7K8xr45U9Ucep+mkAoyd3xFJmczLX+OQTJTkedi6SuACJ7mlWS6SyhuIfKQhvtNRI9gwo9IyHm/AJZRLJZCxfvzOHxeJkJ5nj90jB7t9Zzpt+U3w7GZUan53kJnWgiRWOVlwe2OLGpMgtr+eQ5JXqGA0QzMoqi0N83R0F+HoolRSab43CHh3yPwtBsjIf31JPN6UgWC29en2Lf9gbuTEQYnfajKgq7mszQ5Vt3Q3z+8Ub6ZnIc3WLjj/5WJLmBWXmCICC+vzX9qmEYXR/mpb9L2/dl1BiG8el16eufBD6HSVYTBwYwiWh+xzCM78kpvJF0WSpwHlDWr/OcYRj/URCEGuAZTFLMHuCnDcNIr7PifhXYBqwBnzEMY3L9XP8O+HkgB/yqYRgn1tsfxnSQWIC/NAzj9z7ofZYUF7LN5ealqwG21tuJxWLcG0/TVmHn3ngIXVeJJjJIssr2Jgtz/izXhuM8scNDOpPj7mQKWUjRXq1yayyBRVR4tEvj2kiavS0+4sksr/dEOdJVgs8j0j+Z5Zcer+V8X5wjHQ6uDwbZVGVjaiFKIi0TCKc4umcT6Uyat+4nyCRj1DTkIYgJliIWdmyqoK5Yxuuw4FCzyGKKuVWZq4MRHu92IUsOzvQm2d5WSSiSYFeTGe+PJTJEYzXYFZ1oPM0Xfmwz/TMpDm9SWAokuDEKOzpqkEmyqcZFeZ7A3ck0iUSUPa1OBuZ0jmwzfUTPX1pjZ3sJjSUi86tZnj5cxcxaDpdDZ3RJ57HtPt64HcOhJjnQWYxqNTiyyckbFpGGQuidTmMhTZ7bLMm9NRwkkbKiGwYbLVu7wd75pXfQwZVglr3Ch6xlZxhGP/Dvv9/+G2lNvS120YGp4vnwOp/dfwW+aBhGAxDAHMysvwYMw6gHvrh+HOtPtR8H2jDrhf/HOrW2BfgTTCdJK/DZ9WM/MERR5KndecytplhcizI4l6GuxMqWWoWR+QQn70Q40GZjc42DL786wrY6O1bZgsNmJRCDqVWBykKVw5vtzC76sWsy/nCCtVCK125FeHKnmx3Nbsbmo2R1EafdiirlmFnNsBKzUFVgZX+7j+ujSZwODcPQOdTpY/8mG9Ek/O7f3mNgKsLmcgsH2530T8d45q05NlVKbK528DdvjNJerX7TcghF4ggC7GlRuD5irqtP3olxrNvLxZ45ZpYjOFWdErfOhd4gPWMpHt3qYGx6DYdNo75ERrVKLAYzIMsUe2VicXPyeLMnwK4WFw9tK+D6cIylkEBblYNERuL49TAPdZr+JSMbJ5IQ2FztYGlphd/6mz7IxElk4MzNOe5PJeioNkOjD23z8eKlZVPSaiOL4IQNj9Mfx8yHZ/31pXe0/4v19fZO1vkff5AL/SD4pxC7OAz8xHr7V4D/hOnNfGL9b4DngC+thzqeAJ4xDCMFTAiCMMq31EBHDcMYB1gn1HwCUy/sfWNgcJTeQAxFtmARFTJZnbmlIGfuO7EpFk5enaQ0386ZPg2HomMRLdwcWmVk0YtTEwiFgiiqysWBBHdHV9F1eOXqKlbVxhe/PsShHU1cGs5gwSCXzTIyucLrmoKqqPzp831saijiwoCVTA7OXB3iwd3NjEwt8Uo2iaQ4qC5xMbcSpbXKwcxyjFsjIdI5kb7xNTRNQZNyxJMZ7o35GZx3oCoKqtXKjXvjNBVWsBpKc2s4iaGnOdETQ7aa1Wy9Y2FWozrXeufpbCnl5RtZ/KEEU0sxppYEIrEUV+5MsHtrPWduJ1HFLGfupCktcBFLZLjQn2V0ahmLRSSV9tA7skR5kYMTPTKqKrPkTxOLx6ktLKKrycfd8Qi729ykMzpOu8z8cpjj12RsNg1VFrjWO4tFEMhs4EwvCAIW+QMlr32vc303LbvfA54VBOHngWng0+uHv4YZrhvFNMV/7kO5ie8TG7qmX5+Nb2E6Gf4Ek7wyaBjG29HYdwpUfNPZYRhGVhCEEJC33n71Had9Z5/vdI581zCIYOqL/yJAZeW38wuUlhSxGu1nf6tMOJYmly1CFyQOtlnxh5O8msnyyX1FeOwyd8ZC/PSDVUz7YX+rSjqrc+VeDMGIcGxXKd0thcytRHhsRx6X+kPs6yyhplCkdl3s8oUrCbq31HFkk8q98TAHu6rxuRW66hVO3Q7S1VLEniaF6QUFQ5B4eJ0FJ2OUM7IU55O78hEEgZVAgqnFME/tKeTkTT+//qlmhuYz7Gk218qv34hTVV5AMCHgsKm8cnmcf/FoE9WFMpfsFlaDSfZ0ebjSF+LXP7OJ830Rk9XH5eRAq8K5e0EKvG5KCztIZw3a66z0jKV46+YYW1sENldZiSVy/PgDtfTPpnmwXaNvbJmDnUXUFpsJOccTDqrLfLRVW3nxip/PPVzBzGqWlgqVyrI83OEkj3a5kCwifZMRKordjEwtk1qLv89f1/cDAUH8cH7yhmF89l12Hfkux74r/+M/BX6oYhdAy3c7bP31n0Tswu1yYuhp5v0ZTt2Ns69VIxZLAHC2L8F/+OlmzvWa72f8ItXFGoFIhmQ6y/FrYX7m4Wo2t1QwtJBle73CtlqNkfkEiayVh7YVcHssSjanE4gkKctXyGZ1eicipHQrR7d5mF5OcH0oREWhzdSln4ywtamAtgqFiwMJdF1HJsuhTXYuDpgD4uJgnMoiO9lcDiQrBR6FUNycIqeW4hTm2Sh0ChR7JSKxJF0thTg1kTN31thUKXOo3c714SSRjJUSn5VPbHfz2s0w2VyW5y/6qS+zk0zEaa+SUS1psjmBTCbJzvYydra4mVzRaal0UJ4nkUnGSKSyHNpew8B0guWg+dn4nBaS6Swzy3Eqi2xUFDnxxy2cvhNka43EkQ47N8ZS9E1FiWUVPn+smkQyu7FpuMKPVJUdgiCUCYKwWxCE/W9v76ffDyVObxhGEFOgYidmjPLtx+07HRrfdHas73djxkG/lwjGh+Ic6ahS+dJz/eQ7DEQBipxZLt1fpancpIVur7RwcyyJ1yGRSGVJJiL81lf68bk1emd1Tl0awKnJ9IzF8Dhk3ri2SFetObs/utXJub4EZ3uTdNWp9A7P8ObNRbJZg4uDKSwWkfN3lghGM7jsVm4MBNhWp1JbaserZTl+ZYV8p4DPpZBOp5lfS+NzaeQ7JF68uMj2ejO6UOjIMbeW4s5klm21CgfaPbxy3U9VkZ1jOwq4PZEmLdjwOmSsksjL5wYJhiJcHExxc0Inlsxw4sIAhzsclOXJJHWNPJeVA+0+bo2nsMg2DrV7+OPn+giE45R6zcHx4DYfF3qjOKw5jnV7uTQQ5oWLi2yrtRKPx7k5nqFzXekmmTHAYsNjl1EViVNXJ7jSH6C13ILbrpDe6NLajV/T/9AgCMJ/BS4B/wH4P9e3/+N7dlrHD13sAjgDfArTg/+dzo6fAa6s73/LMAxDEITjwNcEQfhDTObPBuA65kzfsB4NmMN09r3tK3jfWF3zs7Sss7mxjIVAkuPXQjhsdt66NsSx/U1cGkqjGzKvXxikubaQYEhEFGWO7GzkSLuNcCxNZEslTptEQ4nI5b41VgJRBuYNsnMZslmd+yPLpNMZXrEIYEB3exm71k3x0z0hPv9oNZoq8qVvjLESiPLqjZCZSCII3BpcZCXkY3JNJK1L/OEzdzm2tw5NgnA8y1ogxtR8hnTa4EvPT7Cvs4LzgxnSWYO+0UUKfS4CCYH7QzPkDAFJrMCl6rQ1lNJQ4aJr/aHxSlhg55ZaLveHMUQLi8shrg3mMBCZmVshlszg1srpaC5DVURevbaKzeFAlQwu3Ztld5uPF5dT2G027g/Ncc7rYWw6iKJKnLwrk05nuHx3krryPE6LBdisOYryXDRXOrjcu0YiK294co6pZffRGNTvA08CTeu+rg+EjVzTlwBfWV/Xi8CzhmG8IghCP/CMIAj/BbgNfHn9+C8Df7vuqPNjDmIMw+gTBOFZTAddFviCYRg5AEEQfgU4gRmy+yvDMPo+6E3m5/kYG0typNPLxf4Ij3W7TU95fRH5LonGEonBmQifOdrC1FKSx7qdnOlLEQrH0XUbb90Nc6w7j1dvBGircGMIVj5zqBLJatBaYSUQSZFM5pHN6hzcZMdp1xByKcaXJGqLrCR1FY/TyotX1sj3aHzmUDlDCwZHOhwsBRJ4HfXMryY50CojIHGrz8GWWhtff2uGpWAahDwKvDJTwxGaqvPpbPBQ6pN587af33i6lf7ZHAdaVfx+L8kMHGiRuTMWYe8mN/envpXorqgaSibO9iY3Z++tUpzvYnujWVo7s+IiT4SOGoVoWiSViHNoqw+HJqHrOuduivjcdrqbPdweDlJW5OWhDg1DLyUaT3F4k4os2YglyigrsLNvPfchrUukdXioO4+rA0GztPaHYN7/iGAc0zn+z2fQfw+xi3G+5X1/Z3uSb3k7v3Pf72DWD39n+2uYntEfCPGcQpHXasbNR5OshUU+e6SMc/eCZDMyk6sCx7pUnNYsf/HaND+2v4x0xsb9qQQup5lk89h2L2/ejWK1Wmmvs/HKrRiVeQLn+hI8scMUt3j2op/SfBu7mp28cj2I1+5GUySePb/G0a1urgyrlBfaCcfD3J5IMbGQ4MkdLmLlIhf6EySTSX7maDlTKzmqKkv5qYesnLgdwecQaanx0Vhq5blLfo51OREtVkryNEbmg8z707icdh5uknn1RhirIrO9ycpqIM7UcorJxRgdVQ7yXXlcGkygqE5SqSSZrMHx60Ee6/YgWwReuRHG69Y4vN3DyTsxHt7q5O54hJ842sDIoknHNR+2UJlv5Y0bS2yp9+LWrJzvT3Kk3UZxgRNDTzK7JhGLJ6nwKtybjKHrKoGEbMbpNzhkJ35I3vt/BogDdwRBOM23k2j86nt1/Nhn5N2+20eBkqZ/wk88mePK3QA2TeLigEJO0Pjyy/f5xMFmLg8mAQsj035uTRQRjcYYmQnwwNYCLg/oZAyZczfH2dJUwrkBmdW1EP/3363w0N5mzvRnyGRzLK6EmF/0k0iX43Zq/PFzAxR47XzukSoUqwWHakpcuR0Kf3F8mIpSDxeGNCwY9I4s4bTLPLTNy6vX5zm8NZ/Tt4OIgpU3r05waGczi8E0HrvEb//1fR7ZW8u1wQiKbOGL/3Cf3R0VXBt1sbgWIZVKccVeytYaJ+cHs+iGQqHHHAzRJGiqxME2F7/11/dpqPLy5m0BiySTSKYZHJ/HaSnBqQjM+zPMB0U6663MrEQZndUpdFuoznfy5VfHObA5D02VUC0xbo9F8GkW2mvcPHc5gIjBJ/e4sSkGXzs9w8HOInK6saEzvfD+03A/Cji+vn1gfOwHvSCI9E9HOdKZh9cp4HNFqCnPZ2+Lij+cJLu7Fq9doKNWZXQuzJP7a9E0gSOb8/jN+zOk0h52troByGYbEBA42KbitjpAENjfYkUQBO6NRyjZWk4gbnCgxcpqMMVUeR6rgRjnBtIsLi4jyzKvxnO4lSS7t1bjVGFnozkYlwIegqEEL131MzW/hr/Bx4PbvOg6CEYRmmywu9lU3LnRZ1oT8rpC653xQtrrXNSVqAQiTtyOIrZUiZzoCXCjb4nKUjfHUylyeo57w4v4nBroRRTlOdi/pZS6YtMp+cq1FG2N5RT4FEaXdP7o2fvs66zg6iDYFIn/+eIQh3Y20TO4Sp7XycB8hkTGIIXGiyf6eOxgCxcH09g1mZu9U7icDgRBYGQ2SFVZATbFQji3kbW1gqnu+yMAwzC+ss5x37jeNGQYRub99P3YV9lt6Whhf3sew4tQnGejtKSIQNTMRz/Xl+CBTi+BhPlD6Z3R2d3mYcGf5fpQiF96qpUsdkRR5MQtP9vrFUKRONmczuSKzuEOL3en0qwGU6xGLbRXyaTSOgv+JNfG0vzkoUKa60o52qERSxlsbfBwrMvJckTk0CY7qaz5TL7QG2J7g4M8j43Htns53FXNWjSHapWYXoqwudqFasnQN5PmZI+fn3u48psCl2fv+XlqdzGji2ZIz+XQiCVSaKpEbZHGo/vqaK/L5xM7fWypsdG9qZLKsjz0TIKferCMsbko837zt6SoNvRMkiKPRGe1SGNVAa1VLnY2O/HZBRqr8+msVagozaex3EF5npW9LSq7GiRqyn00FAl01YpEYhke6KqkoUTigXaNmvJ8ClwioiAibPSa/keEREMQhIPACGb+y/8Ahv9Zhez+uaO+zEF7lYXj10LYFJGdzSoX+8KU+BREUSAcTZJMZylwywiCQJ49w+hcnJoilbVwCsMwsMgaLpvEoXYnt8eTOOw2qortDM9EeOnqGm3lMLMco29ohhsjSR7das5yshHDH0nTUlvETADujIbYXONElkTiqSzTSwkURaEiX6a13MJX35yhu1GjqUjg2nCC+bU0XofE1gYXy2txlgNpivNsrEXMQZ7KKRR6ZMjFGZ0NkW/XUYQk1wb9xHMyu5vthFMCvRMRZgMGD291UFtocLVvmUA4wZEOBzeGw7zVs0xLmYVDHR7uTyU5fTfGzz1UztB8Bl3X6Z3N8YuPVfPc2TkqfQL7N3u4O2U+LF6/FeYXHi1nYC7N8esRPrnbx+42DzeGw4RjadqqXAzMRM01/YbCnOnfa/uI4A+Ao4ZhHDAMYz/wEGb6+nviYz/oBwbHOH49RP+ciNchcX9kgaEFkZfOj7K4GiYaT1PszPEPb81Tky/wwmU/azGB0elVXrsVQRGT/PWJaTqqTBPY41RYDuWQxRwvXVllbCZAOpVkYiFGMJxEUWSm5v2cvJvk4kCCzdUOvnpihtZykXAkwuSqTk2hhK4bpBIxLg1Gqc43GJ2PEUrAzFKE1XCGikKVXCZJ/1SEdNbghctrCJJMKpPj+PUQiWiQr5+fZ9t6vsCDW308c2YWDJ20YeWNq/OE4gZv9ES51DPGa9cWiCXhpasB7o0EsWlWBFHmxmgCr1Pl9K15RpcNBmbSvNUzR1OZFV038KgZ/uTFCVTZ4PWeKJFYgtevzfPiFT+JWISe4QCNpSpv9gQ4cWmMfI+NG6NJ7o5H2d+q8WcvT1HqFXBb06QyuY2tpwcEUXzP7SMC2TCMobffGIYxjOnNf0987Nf01VVl3J65xcFNdlw2BcEiU52X45FdtYiShaH5DJGcnZnFORZC+Tyx08uJm0tsbymku04mkfFx4Rv99JcX0T+fBMPg7tAcpfk2PnuoDKuigmGws82Gruv4UzbCsRwPbTHlnS/3B1lYCTK8UMrCSphILIORSaAbBr3ja1hECzX5AsU+hTKfSL7PQS6b5cTNKIbFyuDECufzHXxyTxGiKCCKMvtbrdwekzh+bpSCvHwsCyksmGo9+Q4YXzTYs6WctgqREq9CJJJHgc/FgTYN0HjpuoXWGh1JsrCjUeG5SwG2NRfSXWtBkmROXs9wbyxIPKWzHEgTjMQ5srkSSRKRLNUk4jH2tjroncnyj6eHOLavmf2bPcQyEnZFYGeThj+c5NL9VeaX/Zy4IfPkXlNme2Od9wKi5UfGe39TEIQvA3+7/v4nMVPe3xMfmcfaRkFTVT69x8vpOyGmV9JkdOiZyHB4i4d0KkdNoRWFON2tRWiqhCgKpHUrj+0q4fZklkK3hYPbqtCzGQ62yOxvluhsLiXP50GWBOxWEPU4oWiGU7cDdFRL1BcZDM+nEEURf0zkF55owq4YlOS7aa4t5cm9JTy1t4S9nTU8fagCZCfVJU6uDWeoKtQoL7Tx+K5CDGBbSwl5ToWBWdOUzhkGuq4zPJfgkd3VlHoN9jUrFDhyFOU5yRkCtSUaD2zxcKk/Siaro6kagXCSbE7nzliYzVUaW+o9jC8bvHYzyMF2D49sL6B3OsXdsTA/92g9JXkOinwahkXhU4eqGV4wHXCqDMe2e7gylGJPs8rujipWQmlEQSDPbSMSS7AcyuCPZJFUF3/wK92shtOkcpaNZ8750TLv/yXQB/wq8GuYeSy/9H46fuwH/dt4cqeH0dkoZ68M0lQqIQgCD3V5Od8fJ5y28nB3Ecv+OJPLGTTNzKbLpBO8emWRXc0OPLYsw/MZXr0RYl+zQn2hzldPztBabuGBTh/3prPooobHLtNS5WRkIcvlgTDttS7qSx3M+XOomsaWGpn+mRSvXffTXW+locyOIma4OZqgwKuwf7OXgdk0L18PsrvJgdchs6fVQTIV4/JgglxO54UrAY5t99Dd7KZvMko8maV/Lkd7rZML/VE2V5mz3bFtTk7fTyBZZQ6327k1lmI+aKG2SGZ0NsLJS0OI6OS7LGiqTCwtsRyVKc9X2LfJxbNvjjG7sEpTuZ3B2TiLa3Hcag5RFCn1whs3V+motfPULg9v3A4zt+SnzCfxpef7OXN3jYc6HYiiwKZaH2dur/F9iLV8YPyomPeGYaQMw/jDdRrspwzD+OL7zc772Jv3t+70MphJockCmiqBAGNzUQIx0A0Ls4tr5HIGb4gC6bTOc2fGcdlVTudUspkst0fWKC1yYSDxjXNjaIoVr9tOzlAZmBjD5bTjsGZYi4rUFGsMTgYZnk8yMhvBMOBwl4zdmqPck2NkIUN1cQGv3AyjqirLgQSnbidRNTvnb42ypakE3XDyyrlhtjXmM7eUI5tLMzQVIBLNMTLnp3d0hfaGYi7dz1CeL3O008mJ2xEy6TSLSYP5pSDnBz1IIohCjkg4yMJaklTMzuX7S2xqLObCgESJW2J7ew2rwTgvXgngcWr0DMyiyCInRAHNalBW7MblsHK+P4GuG/zZyxN8/lg15+6usBY1OHVtkmSuiSm/uYwYCCfw2AVaakuQRJ2rQwkSWQsZ3cKy37+uKLuRgfqPfshOEIRnDcN4WhCE+3yXD8swjPb3OsfHftC3NTcw0zvBnhYbb9yM869+vJWLA0l2NZtssf5oPk7NysFNKmDnxZzOWjhJV6OHSDzD2Hyc7gY7giAwv1pMvkdlX6vGwHSEnzjayMxaju4mB3/8/AixuIMjnfk8vN2JblFRrQotFQp3RoLcGQ3jjyR43a5xs3cWhyZRVVDG47tc3B2P8LljzQzMJtleZ+Fmn5MdbT4sgsH5+wGWAjo/fqiYfZsLeO6ii7JCGx1VFvzhDIPTCXpH5qgpdmC3O2itK6C9XMC7LoU17nDxj3MRHtiaj2C1k0pnaSgRKfYozIUseO06zRU2ij0yt/oN9rYXsaXOztcvrvLU7jyujOgc3KSSzqrc6p1mLSbQXudhOZhBstQytejn0CYbXqeNRFaidyrG0U4X9ycT1BQrlHglVoMwMSuiWSWS6fQGftsCfERCct8Dv7b++tj3e4KPhi2zgVA1hWw2xfxqAlVVcdtlKrwGYwtp3rztZ3ezRixhrpdP9gTpanLTUKKRyghcH03z5O5CBmdTvNkTZN8mO6GYeezIgk57jY3mEpHRZZ3dW2sozHPhc1kRBQGfU0WT00STBoqqsqO9nJ94oJoSr0T35goe31XKxJJOKp1jLSJQXyLTUiryF6/N8EuPV3F3Kkd5oZ3GujKObCtgdFlgYjFGR62GYskwF4DKIhs1xRKHu6qpKCvC7bbxaJeXs73xb5rSd6d1fuWJWm5PZnBoCp/a7eX+RIyesQQ2KcPBzR6uDiUIxdLs7ShlciXLm7cD7G11oFotxBKmRfnilSC//MlGJIs5eMeXdZ7YXURrXQnn+qIsBLLohg6igs8pc2Czi8v9YXK6zrm+OAfbPWR+GKq1Fvk9t3/OeAfjzi8bhjH1zg345fdzjo/9oNd1A49m8N/+oZcVf5QXL80TTya52LeKxWIlzyljFRO8fHWZhjI75Xky+zvyeOteiOoileoSB33TSRx2hQKXTFMx/MNbs3TWWOmbCHFvKskLZ0YYnlqhwgen78V4oydId4PC3lYPf/nKGLPLcSp8OgUeK+NzpimezoFh5Pitv74HgoU376UYWRZYXI1wZThNhdfk53Mo0FRux8ileePmGo1lKl0NTiYX4ywFM5zpTbKzUWV8JkCZ2xxUO5sUro4k6ZuK0Fqh4rRbWQkkyGZSvHZtmYwu8dKFSe6PBznbn0Y0Mvz+1waZXY6yuBLiztASowsZJpfiiLkYXz+/zIOdbiqLHKzGLLxyI8zRddqsSDTO490e7ozHuNQzxqYKkWg8TTiWZmedhT9/dZpNlSod9V7S2R+Cau2PjiPvwe/S9sj76fixN+/X1vzkuVX2b63CadfY16riD6c4f2+GlbUomrWCRFbi7tAcHpeT2TXTS32rdxrVWsO8P8Otvin0lnJCCQNBkLgztERpgYvqAoUnd7nQNBXNKuJ1CswEUtwbXsbpdKFakkgWC1bJYHQ2QixpMLMQBFFk5PPgkAAAIABJREFUd3MZc36Dzx1rJJAQ2dWs0TcRJn9fNaIksBLUOX1jkI7mUs4hIwkyEzNrnOvLR5QULLLGl74xTE2Zh3ODNgYmFsjzqMwGJRLJLMMTi6QzOvu2VnK2P83CahRVNnj6QCmhWJYCTx3RpMHBViu5nIfe0RWe3J3Py9eDFOW52NNiZ2AqxOBUCAMLPR4XkqRz4sIA3ZsruDGSAnLIos6XX5+mprKISCzNxFIKTbEgCBCOppmaXaXXLXNzKLeh4ToTH/3ce0EQ/iXmjF4rCMK9d+xyYtbXvyc+9oO+oCCPW/fjPNLlYWIpSc9Ehh0NGp2t1biUDD63xKI/x6GtpZT5BKoLZULRNLl9dWiqle31Ki5bM8Fomoe32HjpaoB/8xOtvHknyvZ6N2fvBdlW6+DKYJyqNjdDcwm62wop94nUlzhI5irxB6Psr/dg1ySyoobVKjO9mqGmxEFTqczpOwGWAhLjK/B4t5cXrvh5ottLIivhdlg52Kbw6rUI//5n2rk7meVAq0I6myOZqiSb0znUaiWZbsKlCuxtVQGV59MZdD3H7iaVbFYnnc7DoVmwazJv3kvw5E47L14JYBhWTt0J8tNHK+kZT+J22mku0bkzkWJrnZfJNRldz3GkXeXM3RBHuispy9doKjPLik+vSridNnbXSyhSM4mMwe5WG8l0ltduZdneVsIndhXy4tUwZknMBlfUf0gzuSAIk0AEk6E5axhG17vJWn0oF/wWvga8DvwupoLO24gYhvGd4hvfFf/LvNcNHA4Nq2yhqdyOJKS41B8h36Gzo9nF8EwEQRDZ0+bh1kiURCrLW/fM5JO1iM6pnjU6qiSaSi28fmONmhI7XqeVJ3a4eObcEoGYQYFbprFEZGQugSCpPLi1gOHZKKvhDLKQ44kdbk7eiXDmjp9tdVYWlkMsBbPk2bIs+eO0lMm8fGWRfJf5dW2tUbg7mSTPJROOxEmkMlgVDZ9LIZ5IEolnePlakKMdNrbXWbk8mMSmSsTWAzovXPZzsN2NVZIxDINXbgTZ36qiI/LW3RB7WswU4ZZykfHFJFhsVBTauHxviXKvTmm+ncmlBIPTEepLZGoLBJ55a46yAo0jnXmMLGYZmIpxayLHp/b4eHp/IV8+MUupR0DPpVkLpzl+Lczj211U5Mk8d36RA5sc6MYGk2gIH3rI7pBhGFvewZH/tqxVA3Cabx+UHwoMwwgZhjFpGMZn19fxCUwvvkMQhMr36A5sLHNOBSaPfTEmm/mfG4bxR4Ig/CfgFzCVOQB+c70u/gPz278bh/4Huc+Tp8+jJgO8msxilWUkSeWNCwPs76ommRGxCDq3RxZw2FREIceLl/2UFjqwiAIuOcFcwODaYIAMNs73zNLZXMLoLEiCTjqZYDwY5/XbGnZF5q1rYzyyo5j7oynKPAZfe3MaRdJZXHUQS+r0jwZI5iq5NzzP3o4SFgNWBBFEAULRONd6EwRjpaiKxMtv3ad7UyHlPpk/eHaYHz9cwdxKlLZKmb98dZK2ahe9UwlSOZEb/QtoVgt2zcKzF2Ic7vCS55RprZA4e8dPTYnplAuEE3id67n6QHOlh78+OUdtkcKpu7DsD3N/XGM2IJJOZ/jq65Ps3lqPXZO5PbyEw+lgYinL6PQqdweSdG2u4pUbITLpNHOLQS71SpQWFfD7zwxwcHsdV0dz+MMSY3PL2Gy2DZaphh9Cld0TmAy5YDI9n8XUsvvQIQjC48DbbFLLQBWm4EXbe/XdSPM+C/wrwzB6BEFwArcEQXhzfd8XDcP4b+88+Dv47UsxpXjfLhv8E0zHxSxwQxCE4+uE/29z6D8jCML/xHxg/OkHucn2zS2EJgI80GGG6HRdR8/VY5UldjVrfONSjLaGUo5sVtF1hd/72iCxeJJwzMVqIMnkfIDfeLoZr9OKXWthJRDnqV0macaJOwmyuRyPbnMQiaW5cFtCkiTcToVsTkeWBJqrPOzb7OXlawEUpZCHttiABnQDNtWYzDXpbI5trWWkcyJHO2wkklnmFsupLXdSlW/h9RsrrIZyeJ0WAtEsK2tRyrvyqC6xMTqfYFtLCf5oFjET4lLvDE5NRbZmkSwKp26O8si+Fi4OZrjYM8KhHc28eS+BCAgi9I8uUZlXxgOdGha5CQsG+1utTCxorPjdHGxVUGSReLQcn1NmV7ONeMKBTSvioU7zM51biVPkq8dmtVDohpX6AprLNWqKrLxwKUJTTRGPdrn4f/5KNMk+NwrvPw33/chaGcBJQRAM4M/W97+brNVG4L9gck6eMgyjUxCEQ8C7MfR+GzbMvDcMY+FtOV7DMCKYT6Gy79Hlm/z2hmFMYHKEd69vo4ZhjK/P4s8AT6xz4h/G5MiHbxcMfN8oLSqksVjk1N0YAG/eDpolsuuhN4/TRrkH5tdSRBMZDnRV01JXwLEuF/k+J//7Z5q4OJAgkcxit+ocaXdwri/Jpb4gHdVWXNYMK6E0J+9E+Xc/2cx82EJxnsqN0RQ//2gVstXGwlqS0gIbOxpVBmbTyLJEsSvL5JJpj79+I8DORpVkPLa+Fg7zmYNFzK6m8LkUHt7TgD8u0VCmMeuHL/xYI4ZF5c5YnBxW9rZo1BWCbLXyyf2VCILBkU1WtlTobG8rBQz2tars6axHROeBTVYOb7KSr6X4uWNN5ESN1WASr5ojmjAHZe+Mzi9/opIzfQkGZ0K0VdmIxJKshjPYbQ70TIzI+md4YzTFriY7iayFG2MpPnuohNtjUVNQ02Hj0W0uLg2mNpY1522I4ntv67JW79i+m47dHsMwtmJ6zL/wfstaP0RkDMNYA0RBEETDMM5gisq8J34oa/p1pc1O4Np6068IgnBPEIS/EgTBu972biJ/79aex7tz6H/n9X9REISbgiDcXFlZ+f/tryzSvjnwRckskS13Z3n27CybKiS2t+Qxtmxw6l7MHHxZC8evh3hwixOPQ2Fno5X/9/kRVDHNUjDL9MIal+4vk85k2d7s5tmzczSXyVy+v8ri4hq//Td9PNbtxipbyGDh2kiabbUKVUV2ptdySJLItkYv96dT6LqOw66hWiUe7PTy96fn6ax3IEsij2x187UzC5R5Bby2DL/7tUFc1iT9k1GePTVKKJak0pcjk9Vpq3LSOxGjvEClpdTC2d4EZ+9HeGxnAYXOHOf6YhR7JJrLLJzvNym/p/0CLRUqNinLS1eWaatUsUtJrvav0VSuIIoiNfkCb93xU5qn8GCnm/O9EVQrHN3q5dZEmsHpMM3lKoIgsOhPUJFvkorsa1Y5fnWVUreBTZVIp5Kmws0GQvgQc+8Nw5hff10GXsCcnJbeVqP9DlmrjUBQEAQHpnTc3wuC8EeY1vV7YsO99+s39jzw64ZhhAVB+FPgP2OaR/8Zsy74c7w7j/13ezB9YN574M8Burq6vu2YtbUAg2MpJEmiZ2AShyYjiCXIko37ozNYJQv1ZQqTixlqixVuD/s5fXmO2oo8ro06SWVSJBMJ/KEE4WiKmlKZqmIHqmrOfPfGQswvhcjpBbRUOXFoMqkc3BpN449EGZ5a4bNHKk1SSMBhSZFOG4BClc/gb96Y4eHuAq4ORomlLUzPBxgq8jE8nzEZeQYXqCjyYFdkXJrE1no3Vknk2uAqRS64PxEmGNPRDQvBcJTXbyrUVOSzsBpmZn4Nze7AKlu5dHOcPK9GXWUhk7OrXLoTob2+gMtDMlnRxsT8NGf6CkmmLFy7McWOzVWMLWaQJIml1SiXRrIICMzNBxifyRIIekhkBMIxhYe2WBiciXP97gQFB1q4MJAhqwtc6JlG2F7PYjgNkg19g3Xs3ibR+IFPIwh2QDQMI7L+91Hgt/kWo/Pv8e1MzxuBJzCdeL+BWWHnXr+H98RGK9zImAP+7w3D+AaAYRhL79j/F8Ar62+/F4/9d2tfZZ1Df322/7547/PyvLTbFG6NRHhifw2BSJqdTQrne0M01eSzu81DIpVlbGoWq+Bl/2YvW5pLcTk1DraZrK59E1kaH2slFM9RnKdxbwYcapzaUidZXcS2vRRVkcn3KFwdyfBTR0oZnNd5YqeH/6tvhltDQebzzfXvxGKcyYVFbGodBgpTiyH6JjX2tfs4cSvEpvoCHmjXkCULQzMRnjrcgFODQDiBz61i12SWAkme2lfBfFDggQ5TJWfBn6SsyEk4AYdarcwV2jgejfPQFhtXBsM8/WAdM6s5jrRrxJvL+JMXxmivcVBRoLASSBBqLabUK1DhszE+4+BopxPVaiGb1bl+X2FnnWU9Qy+f/4+8946S47rvfD+3uqu6q3OYnHPEADODMIgkQAAEM0VKsmRZsmT72F7vete7ft5n+x0/++zb87xeP3nX3vdsH69tyUG2ZFEUk0gkggAJYDAAJmAGEzE55+mcu6veHz2kuDYDKBE8JPXFqYPG7brVhaq6de/v3u/v+zVIRh5oMfOtV2eZmluHjIe2Kgs764tA1zjSaGIrmMByogF/JMMDOxT6J4OIez68/9Am8vKB57Zf1EbgH3VdPyOEuMk721rdC/wS8Iyu6wtkQ9u7xj0b3m/H3H8NjOi6/t/eVl74tt2eAga3P78IfFEIYdqelX9T3/4m2/r225pgXwRe3LYKelNDH36MN+vMSpSkLlNfZCSjGwiEk2iSiS8fL6F3Ok1ZnoX799ZisaioZiNul42aPLgxnjV1nNqAxjIz0bSBc70+9teZONHu5dZMirktwfG2PCZXsxr4DosBt8PMylaMdEbn1KF6Usi0VVvoaLCTk+PmSycrsJglyrwZctwqh1s8+MJJinKtnGjzMLQ97B9bynC42YkvaiCUVHjqYD79Mwl6J+M0lFjIpOL4wtm4+vp4kr3VJiKRMIlkhpsTSdqrbVy5vY7RqFBXpBAMhcloGqd7A/z7z9fSvy2RfWUkzmMdOYwvZkOCf/VYOVdGt40x+3x87nABs2txzvb4OFBnRiZCOJ4hx+tlT2MB+5vc+CMZDjY68Fo1bowneH04zr5aC3ZThsXNFAt+6SPIsvtwaLjb80u7trfmbbVmdF3f1HX9uK7rtdt/39W6+Y8IB3BWCHFZCPFvhBD5d1vxXsb0h4CvAA8IIW5tb48AfyiEuL3NJjpGdnjCtmb9m/r2Z9jWt9/uxd/Utx8hq5//pr79bwK/vq2V7+WHGvp3jWg0xtBChgN1WQ82DcGrt2Pc35TtIW1KmqmVGFYlzeP73JzvCyMbBDXFVjKpGM9fXaG5NNvjB0MxZMWEyyZjkg34Ixoua/YhsslJ/unSIm2V206t7Q46R6LIIsWT+12c6QtzpnuTA/UqNcU25lcjvD4Q5BceKuPWdIJrYwn2VJtwO8z4ohIv3/RzrCU7OgjHUlhUE6rZiC8CNpsVSRKcbHNxYyLJlcEA+2pVhBA82OqiZyqBy2Yix23l/I1l5leCDE/7Odxk49k3lmmpyM4ZVOdLjCwkcDrM2RTYUgOzyz5k2YAsUqz4kigmK/XlLha3NMxmM26bkaM73fRMJPDaDJxsd3F5KMLkqk5ZngmnzcjN4WVmlzY53x/FFxH82bPDuJTEvZfLEmSXJN5v+wRA1/X/pOt6M1mPvCLgdSHEq3dT917q3l/hnePud9Wp/6D69u+mof9BcLO3n6Qvyks3NFRDguH5GJ+7v4R4Mk0klqLYI/EXL47TWutlLZDBqsoMTSxjNBQRj0t0DSzhctpZ9uv0jS5QUeTmnCYhSYKrvVPUleeQyAh03czkwiJXRlxkMhEymsa1W7PUlHnYDLtZ90dYW/MjKxaElMJms9A/tkj3lJML16doqXZxZSCJrsO1wU2aKz3E4ll9OlJBDIqVZMpAIhogpUl0j4bxhzPcngyQ0XSMxmLm1xMI4LmL47Q3lbKwHKOhOp+nDuUyuxpndCnD6PQWObk5LPsTGITC8xdvs29HGT/oTjM5t45NVXnx+hYOm5U/f+4OzZVOzvcoXLi5zBP3VdF1B3Qkznbe4WBbBaf7dDRNZ3R6BclgIN+uUVLgxmw2carNiqZpzC17iKV0JHGPU2sRfAr5aGvACrAJ3NUS4U88Dff+I/vpvbTA4WYHEhpnb9xmetnDqtmASZaYXIgg0BBGlWKvTGuFibMGA/tqjLx4Pcrvfq2FnukkhxrMxJPVRGJpHmy1cHMswJcfbmBkLsKJFjO6rjMyobK31kyOQ6ZzKMCTx2rxhzUe3u3g2SspShvyaCkT5Dpkrg75KcpzcrhBYXDSgaqqNJZne/Dbs1FAZ3olQjiWyerkpfwEwx4mlsI4bWaKGr3UlRrZiiu47CoHmrKjgpeub1Fd6uHpQ14uDibIsyZZ3ExQUaAyMLNJU10hD2zPVfTcCVKU6+DJDhen+0J8+WQ5cyshvG4LZTkK00sebDYz+xvt9NwJ0l5rQzXJTCyE+fyJRnyRDA+127nQl6aiOIcnOlxE4yky00ki0Rj+sMLlwQBfPVnE1bF7mVL7NnxCevL3wzYH/wtALtll61/c5q68L37iGz3AE/s9PHNlE48lza/9VBPD82kO77DzxqCf8mIvZXlmvA4FSei8cD3I5NwaWwE3P3UkB9loYMMX5MrtGI0ldnyBNKdvruNx2mgsUXBbMnTdiZOIh/nyyTK6J5Nk0iHqSyxU5ct03wny3NVl2mvcVOTJPNcV4DMdDkJJhf0NEncWYrTW5iCLDOthA40lCs01BQTDKTqaHJy+6eNnH6pmcDrEZjDJl09W0jUaxOswcWkwzAO7XNwc9bO0Lrg6FuNkqxO90ULPeBCvzcTuOjeXBmOkUhHK8q0srQWIxFLcWYqBUeFYq5fvXFzhoX35eO0GvHYXr92O47Ekaal0o2VSrIfg0K4iOkcT3N9sYHgpwxP7bAzOBOmdjIIk01plYHI5xsB0mMf2eTBIKuf6grgcZixmA1om9pEw8j5FPX052RWxWx+04qfmCvyoCPhDPH91GZNs4PyNRfpnU4xNr/L/PTeJRRHU5Es0ljvpmQhx/U4Uq1Ulk4HFlS1e7Y/y2u04NqvCmevz3JpOsho28XrPAsEYXB6OEYhoBEJRljYzrAUydPZNM7PoZ2wxwSs9YRa3NG6NrTK5pnFlNE6+I8Pfn1+irtBAQ5mDFzqXaClX2NvgYGUryoXedUo9OjUF8Bc/mCIQjjG6lCGeMbCyGWRgPo3XofDNcwusb4W5MuhDlxT++Jlh8j0WJlfiSJLg8sA6bVVZ2S9fKM7AXJrWSjNHdrh5oXOVmeU40bjO+LJGOJ5iZCnNxFIMXdcJRaOc7QvQXmVif4OdM9cX6B9dZmVti2+eXcSkR3ju6gbzPgP/cHqYTCZDSa6Z6TUdl91CLJHhpa4NLt6YJhDRePG6n2j0I4jp4dMU0/8WWb79z0HWMHZ7Avx98RPf08uykUc78njjdpAvPVhNPCPz0Geq+PbFFSLRBG/cjtE7tomuZfjtr+zAIEnEY3koJhOndmUn/4am41SV5LC3xkyuUyYcrcShQkedysxyiHg8zdj0KvsbbBzeXUUkmuChtqzazrOdfnbUFHB/o4zRINE9FmF4apXqshzmtxL4AhG6xpPIxgySwcwrlyd44lgz8Xia+aUA9z2UR0OJzOxKnJr8AupLzJy+ucmd2XV+6fEG6kutXBvx8WBHCbkOA6UeA1cHNwmFopzpCSAkiaHxJawWE68YJWLxFD0jKzx6qJQjTSpTi0kyVbnUFJkZnw/xQpePZEpjbHoVu81OOBRCNZmorHTgsuj8oHOez91Xh9ueDRES8Wq8DgNdI0FevTnHgdZyxpcFD+3x4o/o1BTINJQ6WN6IYJBE1trqnkGA+HQ88kKI3wP2APXAN8nKX3+L7AT6e+KT8Vq7h7BYVTaCCSwWMzurnKxsRoknNapL3BzZmUM4YWRHhYMvHK/Y9rMDi0UlGctm3AHMbkn8/EOlvD4YQtd1cpwKy5tR4sk0FYV27BYjLbU5WMxGnKrO7moTfdMJ3hgMsL/RzqFGO3cW44wthAkmVR47WEpdgY4vEOM3vthAIpHi/kaZQnuCymIXu8oE4bjG7/3cDtZ8KfqmkwxMR3Go2ZfI7loHn3lgJ8ML2fPbisgcb8tlZiVMPC2IphW++nA1VYUqj+110t5czq7aHForzQgB/8+/3k08pTAwmyQSS2MyCjRNY3I1iWpRWfeFOdhWzYO7zGiSkS8ezUeTTMyvJ3jySCmL28mko3MBWipUtiIGjrW5OXWwlowGbVUqJsVAeZGbuc1sI782lrjnmvdZSHexfSLwFPAEEIG3GIL2u6n4ifkf3ktcG02wf3vJ7rF9bt4YjpFMpnjm8iaP7HYgZCu1JQ6MIsX0ahKTUedku4tbM0neGAywuyZb94EWK6e7/ajGDI/tdXN5OPuSyEhmnj5cyDdPz9BSrpBMpTjXNcvAxBaJeAqDQXCpfx1/zMgDLRb2NXn53hsrtNfa8DpM1BRI3JpJMrKs8/n7ChlfjOF1mDEaJO5rcWDU41zqXWBoPsPTB5x4bAZMhhT3N6tcGYljtyrb52fjf74wTpFHoa7UwdRqMkvzNUs8sMvFP5ybZmFli+c714glElzunePbF2Y427PJ5ZEEhxod6Jkkn7+/GF1InO31c6TJjmoyEInGkM02dla5mFjMKvCOLulUF5oJhcO8fN3H4SaVEzttvDoQBsAg6WSSEc7cXKOjwfrRxPSfkuE9kNzmqujwFkvwrvDpGOv8GDh/8QpuMlwbi79VNr+0xdj0Cv/qyVosZgMWSzb2bSw186fPTbCz2onRkIc/CgYh47EZGJuPsBkRXOmbY29zEarJgEFP8mLnMvWlDt4YjhEKx+ifjpNjN1Je5MRisQBpppcTzC352Vnl4fodHQ0DyxshhuYSjC6mMRiMXOgcZWddPgg3r/as8sUHirk8HCOahLX1IBVFbhbXQlweMWLUwpQXWBmbD3O5bxOnUyEc86IlY3hdVm6OrBFKSORaUnzr3DzNlXZevJ7GqsrsacxnX12Wo5DJaPzxs5NYzUYisTiDcxLVBSqxeJw3bsxSWeygwJFB0i04lATp7QbzRIeb0z1hnDaFNV8cScDgxAqyIcOhJiclLp1b0wmMIkNpnoXvX5oEg+UjuuOfmEb9fviuEOIvyLJSf5Eslf0v76biT3yj39feyuLIJgcb1LfKljdsVJY0IQxwcTBK7/AiqrEMi5KmMMeOrsPLNzbpGVmjJN/J6XiEXZVW2ipURmc87Kq0YJA0VnwJhidXURUjD7S62PSXYlNlaorNzGxKqFICs1klnUnx7z7fxNB8iiMtNmLxNNPzHmoLFWoKZdJpjdWNAnZVWRmYChCJxVlcj3Oo0YUkSTx3zc6XTzi4OJRgX42R//qdDUryEjzWkctqCIxGhUd32znfm+bpI25uzWY43mLmtVsxBqfWaW3I44kOlQu3FTaCP8zZeL7Lx9dOlTGzlsCiaHzr3AynDlSR75IpKXBRWuAgx2ViPayx4ksxMrOGxVyLjkT/2AIWs4yiu0DXaajM42CTk7mNFMG4zI3bs+hASa6ZurIcOmqNGA2CdOaeelV/knry94Su618XQpwEgmTj+t/Vdf38+1QD7qLRCyH2AEfIsn5iZGmzr95jiuFHBqfTBi7on0mwq8JE33iA1morA9NhqgtdDM9vsrfBS5FHIhDKcH9bHitbGZpLBLkeB2u+OMfbHNhUI89e3eIzB3KZ20iR7zZgs5h58kgZOS41q1RbaWFiLUNFbhKnqrG31sXz1/zIipniHDMzKzEWNpPMLvk5udvL5ZEIlflOXu728/RBL33TSUIJA0fbi6kssm275fq5vyWrdBMIRTAa3Bxpq0AWSTbDEnablRJXiu7xMIrJRI5DJhwOkkorSJKBX366md7xKNX5RmyKhqInWPMpDM1F6Whw4HXKjC0lmV3X+J2vNPLijRAddW4qSnIxGZNoQqa5XObOUoIvnXQRTgm0ZIKnj9ay7E9zaIfKqwMxHthh4nRvmEf3OEinNXyhPAxGA+jwcLuVM73hj4SG+ynq6dlu5HfV0N+Od70CQoivCSF6gd8GVGCMLPvnMHBeCPG3dyvP83FHc4WdTX+U+Y0kS36dqnyZxmIjUytxrBYrR3d66BkPM7clqC4wkdIlXhuMcaBe5cn9Lk73BOkaCbKn1obLrjC+EOL2TJrH9trZU+dkcC7JwEySqkIVkxTnhc5VdpabWd2KsbzmY3JmieWtBId2uLkxFmE1oJPjNPHgLgvPX9ukKMeSTWZJ6rgcFh5oy2VgNsPsapRct4rHln13H91h5RtnsmaYipTm9VvLTMyssRo28+ylSdBhajnKsRY7Z7o3sVhVqvLNdNSa+D//6hbpVJymcisv31zH7VAp8WYpxNdur/JAixWzYuTp/U6ev+Yjo2W4r8VF93iQaDyNw2alvtROz+AS/ZNbNJTIhMIJBqf9VOUJJEmi3ANjiwleuO7jZKuVgH+T0cklljai5Ni1e59lB+jC8L7bxxlCiJAQIvgOW0gIEbybY7xXT28lKxQQe5cfbyWbFDP3wU/944PNTT8TM0mEbOFP/mmA4/tr6JmIUF1g4m/PL7G/0cXrw4K5FR/xRBpN01hY9dNS5eLsjVUSupFYLMWZrgXu31fP1HqK/jurnDrspms8jmrUcMhRZgMaK5sxHBaZN+Z9XBnLJdeuk5fjxO0wM70UoncqCVqamSU/L9/QMZhsdPXPY9pXy+sjSc5dHmN3Uz4/uBZkbiVMz3CKY+3FdI7qaEikNcHo5Bql+Q7aqmx0NHmzctmeDKX5TtorZebXIrw2HeXawCL37THz3BU/RR4DHa2V+CIag1N+BseXsJkNTC0KNvxRkskEI4tJdFKAhMtmoHd4DtVUTYFb4dkr6ySSSV6MuVBM2RyAF64HkPQEN8ZSfO1BB7cnt5hYTnK57w7VpV56p1SO7ipgcD5NOCGIpz+KSFN84of3uq7f1Qz9e+Fdr7QQrip9AAAgAElEQVSu63/6Pj/+gZlAH0d4vS52WRUu9vt58kgleR6F0hyZywMbrG4EsJndZGJJnjxSwcB0kEf3Ovkv31pkK2DmZLsHWTbw0o0gXzpRji+ms7dGYWTcSVWegWKvSjCS5OKSn+X1MEsbMlsRQU15HsdbzCyuR9EKLSz70hxry2qJTC/rjEynOdGew+RSlJ99pJFYWtBWpTC7VEhFiYdd5TLdIxKTK0n21VmQtsUcX7rhY29LCbGkTlrPlulI9Ewl+cXHKuiZiLG/zoU/5udXnmri1nSMpw7nsxmII5t0/KEEDeU2QikzBR6FPTUWnr/mI9dtZV+Num07Bd+7GqW1uZxjO8wk0zKXexepKHLyYKuV7gkj0aTgwVYL1waTXOlf4/XbNqoLzTxU4mB2NUJRjolIPEOO00RqJkVDqY2By5sf0R3/ZDf6t0MIcRio1XX9m0KIHMC+rTr1nnjfK7Cd0vrfhBDfF0K8+Ob2YZz0xwV9E0Hy3BaO7PSy5JewmGXCKYXd9QWoioRkNFNdIHOqzcGN8QT7W6tx2VXWQ4Lnu/w8vtdObbENWSS4Phbl0M48OkdCpNIZHFYFFAdtTRWU5VtRVRW7WSeeTHNzMsWuShOxWJh0RiMYSTK8qLGjyo0kBDMbsLPKylZY55UbPr5wfz6ySDO5quGLm6gotL418XbzTojGUhuHmxw0lpq5sxBleD7JzLKfumITitFANJUdugaTJqoKVe5vtnKmN8Tl4QitVWaOt7m5MhIhxy6x4ktzsd/H/nqVfTUqw/PZAd/pm34e2Gkjncpy5S8NBPnyQ5XkuM3806UV9taYiUYjpNIaSwEDv/+LrWyGMphkmTM9AX7x0TKKch0cbbHwg5t+5pbW+c6lFU60OrjXnrWfpiW7bXLOb5INvwEUsuSc98XdjKmeJ5uy+hJZVdtPFZZX14knjRypya5l+4NRXu2Lc7DRTv9YjG+dn+M3f6YJAItZZnUriMNuob3SzH/65gBtTSVcHssgyCCEwqWbEzRVejm8w835WxEk0hxutHBtLM7lYY2nDjgIRgz84NoqLbU5CCForbQwvhBjaCHJ0wdc+EIy335tnpN7igCIBDfxRTSmV1QMEly5NY9JMfJ4Rxln+8LsrdIJJyX2FsqAzOWRBEdbzHzr1XkGJjY43Jg9/1AoyhuDUdoqsyPEeCLNwqoPXyDGRZuVdCbD+Ow6KxsKZkUhmUxwpLkcgyQzejvBrckQVUVWPHaZVDJO55CPEq+ZoWkfSV3lzuw6L3ebaS6W+fbFBe7fVUAyrVFXbOIbpyexqzLTyybWAynO3gxjUqwU5bmYXdxieN7+EazTw6eop3+KrATdmzqUS9sCtO+Lu2n0cV3X/8ePcXIfa8zOLrA0sUIomoNRllnZCDMRjaKayyjKsyJPBDjXvYHD6SSeyBCOxBiZXMZAOUc7ajDJRo7v+KHCajBSSqHbwMxqlDuzflLJJCa1kq7+GWrL83j1thlN0+geXcWkWhmdDaNpGldvzfDokTq6xpNousTMcpDx1UJmNqKs+RO4nVZcVrCrJpa23Cyshnjxuo/ZJR89g3GO7Snh6miCtC7oujWLJEqpK7UhJAPDc1Em1gwsrwcZGAsTTlRgtWjk2gX5bivFeW6O71AYmQtTeKCcFV+G9grB4GyUl677sNus3BqZx+M001oj6BpLsbQZYX4twp7GQvbVuegcCfHw/mIKc2yEYjqT8z7cTgeSFmd/o4uGijwKPCa8dp3vvjbPr362njyXiQ1/jOr8AsrzZKR7TcMVnx4aLtvknG013g+dnPMn20OJc8Bb/tdvKt1+0rF/bxsL8jSqamZXhcL3wyol+VYaSxQWV2N8+WQZvdNJmookcp0KQzNpookkjSUKt+cNKCLGis9IgVvh5liAtmobC5sah3fYWQtKqGaZo40yWqaOrWCcEztV4skM8VgxQgge7/Dw+m0/O2ry2VttxqoauTUeYE9DHvlOQWOJhUismGQ6RaHXyth8iOIclUQyzaN7HJzvzTC3ZqRjO7Zf98VYyLdS4pHon1H4ygkvz3b6eXCPCaE5MCkS1YUmGkoU4sk0a36NUCxJMq0xtabz6B6F5fVNBqdT1Je7KcqxcG04wGYghtupUlOoYLcorPq9yLKRgw0qmqYhm8wcarZwpi/GqTYL1/Mc7Kq0UJbrIpnO4HUopHUjg7NB/sMXGjnXF+SzB2UCkSSqyci5W6GP5oZ/Qobvd4EfmZxzN1eghaw5xR+QFbH8I+Dr71mDrNmFEOKiEGJECDEkhPi17XKPEOK8EGJ8+2/3drkQQvwPIcTEtlJu+9uO9dXt/ceFEF99W/nubRWeie26P5LKWke9nWQ8Qv9MErvNxuMdXi70B1jaTOK0Gnmyw0XXWJhVX5LJNfjlR8vpHAmhaxnu2+GibyYbV69HZMpzFTK6xNWhAHvrbIhMjNf61mksNnKy1cqlwSgX+rY4tcfL0WaVb5xdIJLQ+MrxQi4NZePmhYCBRztymV8Nc7F/nZpCiWKnxvRyjLGlDDvLFfbVqPSMB7FaVJ48kMP1iWyMfXUszk8fLeLKYJCKvOyS28ldFt4YipLQFH7uVAnJRIxXuoO82LXJwXoFhQTPvLHByW09vZPtbjqHA9jMBr53ZYuiXAuH2ivZW+9iZh1i8TR5TgWvNcXcRorT3X7212ZHO241yYWeNR7ak0/PRARd1znT7WNvjUJGg7Sw4LbJPL7XwZneEP5QkrnVEM3lNvR7rIb7w3X6H597L4R4SAgxtv3sfehONu+Hbd+I75HVoHyTnPP/3k3du+npnwKqPqhzDO9udvE1stY/f7B9sX6L7ITEw2SXAGuBDrKmFR0i6w/2ZkaRvn2cF7c9wv6crEBgF1llnYfI+nzdNVZWN+ge2sIoy4wOzKAqRixKIYUuAy+8sYQk6QTjArPZwte/M8TelnLO9EbY9EeYnFvHYChDT4T4p0sBjrZlhUsi8RQGJEq8RmRh489fuIPDbkGVJfyBAKMzATCYsFtNxGIxhieimExmVClO/7QRj81AIJzCqiq88PoYx/c3kEpJ/MO5IfY2FfJcZ3bAdWNwgV/7XAP5bjPDc34WtyRcNjND80mGJldA6ASTRjRN4nLvNCV5dt4YkZGEQiYdYXRyFVWRWVqPs+mPcHnMhdGgYRA6S+t+vv7dcU7sq2R+U2NidgOPvYC5pU2uD6f56sliZKPK89eD2FUzNjX7KLXV2Pkv/zDGsfZcZCP0TCawqGbMioHVTT91JVnmo8Us01xs5OUbm+R77bTVCHTutXIOH0pPL7Lqmu9mwPKR4b3IOUKIa7quH3in7+6m0fcDLj6ghve208ebbh8hIcSbZhfvZv3zJPB320kEXUII17aI5lHg/JsMwO0Xx0NCiEuAQ9f1a9vlf0fW7OIDNfqC/Bx2Ozz4QgkMUh4Oi8LBeoXNgEZPvh0MJp466GR5M47VVIOqGNhXZ+Glbp26ygLubzJxZSBC99gK3hwvQ4txLnSOcbi9kuc7N3CpGVx2CxX5JoSuYTJamVwI8dheB0IIEqkSkvEoB2qMDM4q/O3LI+zfVU4kmuHYzhx84Wrsqs6BXTbuzLo5uduL2y6jaRpL6xGu3N5CNpmxWCz89+8M0dpQTEWOlRMH6tkKRuioNqLIBsLRUiQB9zVme//numLsaS7gcKOFC/1J2mtdWC2CxpLssS91u/j1z9diVWVujPmxWhT216mIdIQfdK7TPekFIRgaX8TrtGAx5SJ0DdmgE4nGuD4WI43M2Suj1FfkcDYD3bdnsFlq8UViCEkgMDAxu45Jlni5K4Ikwb21qP/QGHlvGbAACCG+Q/b5/Ugb/fvA/G5f3M0VyAdGhRBnf9QlO/G/ml38L9Y//FDX64OaXRRvf/7n5e/0++9pdpFMa1wYiHKqzUYGQSye5vXhOL/6ZBWSJFjxp7k+keK+ZhubYZ0Lt7KKtxktazm1HDTw5OFSavIl9lRIfOaBepJpjScOeAklJH7l8XKujcbJcalMrSb5zJEi+qa203RNgkf3eegaT9LR4OTkoQYsqsLJ9jxWfTEq8w2sbsZIJDPsbS6ia3sY3zXq43P3FSDJKqfanTQX6Tywt4LaEjtleSqKIc0T+5ycu5WdKLSpBhymNEtbKQamgrSUq5xqd9M3m8asWtlT52BxPcqSL82Z3gBffaicieWs6u5m2EBzqZmNQJLloJGvPVyJ02LgQJ3KvpZKCnMd7CxT2N9gw6HC4dZiSjwSO0vgREc1XpeNU60qxw/UI9A41qxwtFGmwJ7kpx/eQVtdLnaH+yOg4XK3S3Y5bz4v29sv/bOjvNsz+XHCu17Mu+npf+/H+WXxL80u3nXXdyh7L1OLD8XsAuD5Lj+f6cj6z8UTGi91R3j6gJO51TCRSJw/fXaW1vpCusZlxqeX8LqsHG91k04neK4zxFMH3CiygVd6QiSSSZ7ocBMrl3nxegCL2YpJMdBQCAOzCTLCQm2JLWsDnY5SmWtFkiQSyQTBiIxdFeTaJAZmk0wuxnliv4eaQjNXRuKoikSuNcPsWgpfVCHfbeKJfTKv9GbX+Z/a7+KF6z66R6NU5FpRjBLVeTp/c3aexw4UkOdyc7onhKZJPFKVjcF9oRQuR7ZTON7q4H++vEBFsZOyPCtzW3Feuu7jZJsTxWjlb88tcLQtj6p8mZd7ImjpBNX5JirynLzcHebxfQ5GlwWPHyigayzGzckITx9wMzYf4dW+LUpzVDJpnZGFFLUFBu4sazzR4eCPn53iyydLP5Ilu7vM59l4mxPtO+Gun72PI9610QshhJ7F6++3z3t8/y/MLti2/tk2+Hu79c+7mV0s8MNw4M3yS9vlJe+w/wfCa5c6cZsVrtzJABqvXhvl1KF6bk7E8Vol6koszK05OL7LiduhsLzhZXUzwgs3AnT2znCgrYILA1Eymsbk3BrxRJrzZhXZKJFKZxi8M48QpcRjacZmpynKsxOMKOyrVXj2jRX+7dNeAB7e4+FPvj/FTx8rIt+t8vJNH0ajjEDHF4wzMbOCx2Hm6SMFvHQzgstuomfMx1LQyK3RJfK9Vi4OW/DaZF66Nk1Hcz59M0lUk4nhyXXyvE6Mcoobt+coyrVzYVAhk9HY8kcYnlhE12swSWnua3Fy4dYWqklhcSNKRb7K5dtbpHQTcyt+Fn25bAYj2AxRuifSfO2kA4CaAokrQ0GKvCY0HU53TtHRnM+1O3EymsyZa2Mcaa/MWlUHAlzq1fG6VF7tjyIJnet3Yvfcyk4HtA+nbb6XMcvHHu/V018UQjwLvKDr+lv8epE1nDhM1lziIvA371R5eyb9X5hd8O7WPy+S9bj7DtmJvMD2i+Es8Pvih553DwK/rev61naSwX6yYcPPAnc1e/l2NDbWYvL72FmhsrAexXK0mmhK50C9hXRa4+WeEL/wcAkDs0nqJSj0qphkOLrTgdFQjcn4QxeZlzNxIgk4VK9gtxh5vjNEY00xJ3ea0TSFQDhBMpliajlGLClYWgvwQucGQpLQdVhaDzCyVMDUepJUWmd8boV8Vwk2VWCUFSLJbOMYn1lFkQWfu78EjyNNeX4NCxtJjreopFIK564Ljrd5UU1Gzvb6+YXHakhqRnZWqOh6OalU5i1uwcsJhUjcgiJl2F9vRggVf8JEW4XM71waJc9ZycEmF5uhJPGYmyKXoNhjoms4wuj0Ks91mjCbTUgGA+c7xznSXsXccgC7RaGu1EVdsUI6rRGP12AwSBxtdnB7ys8LVxf5/NFCchwyZqWYta3wtrXXve0wP6TRxFsGLMAiWQOWL30oR/7wUPpuX7xXTP8QWZ/4bwshloQQw0KIKWCcrCXuf9d1/W/eo/67mV38AXBSCDFOdvbzD7b3fwWYIutW+5fAvwbYnsD7z2Qv9E3g/3pbWu+vAH+1XWeSDziJB1BYkMv8ZtaJtXsiQUe9DbuSZmo1xYs3AzzYms0ui2eMvHY7yr5aMw/vdnF1NEKOXeC1aowtZl1kDLKFzx3ycLrbTyqtYbfZ0FIxovEUz1zx89nDObgcKo2lKqqc5mcfrsVlN/P4PifxlMZvfWkHFkVkc/slmdqyXPbW2UDXOLSzEK/HSTKV5okj5VSWeLBbjAwu6OyqMFHq1phaSXCm18eTh4pY8aWZXo6Q61JpKLOzHhIEI0lybFBXCCML2RUAxWyltiKfipys0m8skSajGzh/K8Tv/txOtsIaFtVI71Scpw8X0j8VRjEaMCtGfu1zTaQygpOtVnaVSzx+pJbiHDNWs5FffqKKmdUoS1spzvZscqDeTDKRIBzPML2e4bd/ponrYxEmV5J0D81zoNF+z2P6N3v699ve9zjvbcDyccHCu33xXgk3ceDPgD/bHqbnADFd1/1384vvYXYBcPwd9tfJunW807G+AXzjHcq7gR13cz7vhTxbiqHZEAVuGSEEFkXnr18apakql+kVhcoCWFwLU19swR9KMruR4kLXJDurPXz2vkLO3wqT77JiNUtIksRje+385Stz1BSq5DgV/vDbo/xvX2hENRk41erkxkQSf8TI4/vsLPRu8fcXAjx5MA+nVebmVIargz721lkxkOb2bISFDagrjNN7ew6P20I8IwOC//vvBjm5r5SFtSg1xRZe6QmR5zSzp0HljeEYvmCGJ/dvu+BEorx6K8PjHV5ko8T3r/mxm9IUuWUmFoPkujw8tsfEi9cDTC9t8dT9lTitMo/ucXC2L4TTZkEIwfGdVjrH4vgiRg7kqDx1SOH5Lj8GSeKpAy5e7g5jNFpwWmUebHfx7ddWSGcyWM0GHmx38upAHJvNjtEgqC0y8Y/nJ7GrRrZCKe75Mj0fHo/83QxYPkb4sSby0HU9xfby26cNGxs+/HEz3311iGMd9VweiVPqUdhRU0hLpQW3DV7r2+DWyArJeA6RiIld1U7u21NNOJqgfyaF2WTmv35rmNpyD/FUHmZFYmpukz1VRURjCYoLPFzoD2CxWLEoMDq1gUGWuDgoMzLtJxKL0znqQDVnGJ2cRzWbcdlkwnGN51+f5L491WhCpq25FJNi5MFdKmBhdGaL+mITG/4IA1Mp+oZXaajMJ6UbOHt5jPbGIp7rTKFl0kTjCRbWIuTawWqCEpfOt86v8fmjRThNKf7m7CxFBbl43Vau9E3RPbrO0IwZg9HI8MQKQkAiWUg8FmUrEMPptNE9HiaSMhKOJPAHQpwfMDMyuUwgHEU11SOLDC0VFvomg1wbi5PSDfQMz2MxK4SjbnaUyOxuKkU1y9hNGSQJtMy9vNv6RyOz/THHp4aI/KMiJ8dNXlxnqaaA+mKZqnyZ1wd8nGhzMriosaPchLqQ4te/sIOuO1GO7HQQS6TxWCUqc0wgGdhbY2Rtq5CyAgf3NVu4NuznM/eV43GZWF+BYq/g6C43VjW7Rt51e5HmchslrgzO1gJmV8Kc2GnGH0qxsu4kk86Q7zJgi0NhrpPDDSp2q8yyTyeViOALGVGMcGRXPjObEocbPczdCPAff7qF7qlsbO8LlON1mDm6I0uGOdfjI5420FjhJJXS2QwmicRWAQOhOJjNKo/uzkp1mQyNRJKCE7ts+MMJzEoxgVCWQmyQLJy5scLw3CYP767CZlEIhG3Ul7tpKJIQegGBUAJfIEZjmUpdocpaROZgg8L5Pj+PHKoiEs9wqNHKKzc2Odho5dJAmJIm1z2/17oOmZ+cRv+u86KfGiLyj4Pr4wl+9sFibk1lU0LjmimrXx+Os7gRw2Yxke8y0lJqoH8mwfk+P21VJprKbdxZCPPMlS0+ezgPoaeYWU0QjBs4uMPD7ekQFbkGTrR7uDWTXV8/fdPHVx+uxGp3MLqYpq3SxLEWO997Y5X+uTQ/dSQHj8dFjkuldzrFr36mgu6pFBMLQYpcGsdb3fROp3mtP0BrpZlgTOP120Faq+24bEYikSizy2Eais14LGn6Z5Ks+2LYrCqf2e9hag2Kci3cWdX4t5+tJxCXMJpdHGzKKvy8PuBjZ4UZSUuxFkjy6q0ox3ZYeWi3k2tjCSRJIBQHh1pyCcYFA5NBmktNaBpc6A9xvMWCxWLmyQ4nqWSCH3SHmV3a5HtX/bRWO2itNJNIGwhFktgsJlxWmbYqhYu3NtF1ENK9fSQ/jJj+4wIhRLkQ4sT2Z/WfZdl95d3q3U0+/a++beb8U4fO631oyTA3R9Ypdaf5o2cmKLAnWd6MIhPm268tkmdNsRWMU5arMrMSJZ6SONezyZm+GIvrEeZXfFwajJLQTPzZ94cwSBqLGwlmV6M0ligoRolAVOfKUID6MhtoGV65cgcEvDaU5PaCTv/4GkIYWNhIImUiXOxbp60qu4YfDEW5NR2nptCMEIJYPIYwmFj3J+gdmuX60Crdo5tcH17naIud71xapKHUTG2xytjsBn97foH2Kplct8rKRoTeMR/NZVbyPSrdYxuUeCVqim3cWYyQllSsqkxJjsQffXsQuynN6lYck2IgHI3z/atrHKhXONDoom8yxvwWlHiNnO8a55HddoQQaKkI0Xia6kKVdDrJ0PgKW8EIncN+YvFswtK5WyEONmT5AeX5Vq4Nbb0r+eLDgg5ouv6+2ycB20k23wP+YruohGwaPAC6rg++Uz24u+F9AVlucS/ZybSz77U2/0lDrtdNKKJQmquS0UDLbDCzGiGZTLGykWWkRdMSYZ/O5GqMdV8ITdP52qlytsJJbGouobjOw+02Vn0x1usLqMwzsunPvgxe6cnBYlEZnFgkk9Zpri4gx5amsshDU6mZqvzskH91sxSPXbDujxHXFK70zNCRkhmcjZDRYHhiBYtqxmg0kNKNTE4vU1VQQnNNIRlN8Ph+B+v+JHeWEgSCMZ7v3MBszBCJpZGNRs7cXMegqJjNJp6/MskDBxrZGo2y5Y/y8tU5ZtcKUGSFGwNTuGx1FLllKotzqSi0srAepms0wLo/iS8Qxm5zgMhw684idrPM3CKYZCNXhsPIJgvJjJFvnpmnpa6QR/Z48DhtrPujnNrtZGAmSe/gPDluC1fGrGQ0nXRaIxiOZHuge97Tf2rwb8jSga8D6Lo+LoS4K9fa973Cuq7/DtkkmL8mmywzLoT4fSFE9Y98uh8j1FZXUJpnYi0sk+s2c2xvOcLoYG9jLvmFBfz7z9UwuphmR4WV9mobpYU5lBTlIhsF18biHG60IJIRwrEUV0fifPFYITMbkNbgP3yhiVgiTXuFgdoSD43VBTyx30WhN2sMeXs6TDCazdAryrUwtRxjV7UThTT3tRVRW2zm0T0OWitkasvcVOYrnNxpBl2ntiKHyjwFp8PKyTYbV0cTFHrN1BXKPHiwgoIcGw2ldlrr8/mlR0qx2e08vtcJWpJj7SWUuAVCS/IzD1bx4L4iaousyCLFzz9SxZovTrFXoaU2l/HlNHsbc8l1q3TsyKexpoiTu8wca1Jobyhmd2M+ZcX5/NbPNBBKwNEmGcUocLtsHGmyMDgdpCZf4pHdDjpH43TUW2iuyaWsyMvRZjPHW1QUKcnR9kJ0cW+H9/pdDO0/QcP7xNuT4IQQRu6S5HBXV3i7Z1/Z3tKAG/ieEOIPP/i5fvzQWmUnGo3xN2fnaSmT2VVu4LV+PwVOgWKUOLbDzJneMC/d8HNshwWTiPGPr63wUFt2OHus3ctzV1Zoqcz60wXDMeY3odhr5nOH3Fy45UcyyLRVyPRMxumfilNdpPL4PifnboXQNA2hZTix08JrA0GsVjMn2rwsrEWZ20jTP5/hC0cLWdmIcubmBs0VVh7Z6+GV7gC5dg2XzYRRpFnYTHHxdpCDDXbWtsKc79uipVRGNRtRDRmGZiLYrCrH2nLoueOjb9zP1JKPpfUwL12dZHBqg9WgIN9l5OvfGeXG7TlWVtf5xuk5Cr2WLB/AozO3HucHN4Mca7EwOB2gucyEJEl01Jr485fmqCu18/AeN9fvxFkKSBTnmLCYZSxymtn1FHabnYpcGF1IEI4mEZLMqT152Zj+R8uOvmtkdP19t08IXhdC/B+AKrL698+QVbd6X9yN7v2/I8uc2yBLhPmPuq6nhBASWaLO//4jn/bHABsbPoanEsgGE1MLPl6+acHpsPJa5zQnDzdyaTiJEBJzKxsEgzFeMyssrSRYWg9waciOJEkYJImJeR8VJXlsBMLkO3S6R/0816lhtVpwO1U6+6axWqpYXNnA5bRhkAQgON6SFZ+sKTIxtZrh/LUpDrZVc7YfUimJZ16bRgi44lSJazIXe6ZICzPTq0Zu3J4nkyljYiFCbZFM12gYWTbx8k0/E/MB3A4TvVNx0hhJSWa+dW6E+/fV8cK1TWYWNyjOc7Gz0onVLJNGIcdpoqPOkhXXCBTisJrpqJX5u3MLjC0mWNpKU5mncrF/i2Kvyo3xJDMLG8xXeln2RZElndllP7ennXicVobGl6ityH3rWh9ocvG9K5vkeVR2VDh49uomveNZObHuiSwN12C4dxLUb8b0nxL8FvALwG3gl8lyBv7qbireTUyfAzyt6/rs2wt1XdeEEI99wBP92CEnx80uq4nnu/z87ldbuDQU46FWC/FEDU5VZ9+2OEQw4qQk38PD7TZevJ6hodxOWb6Z0hyZ25NBygsd7CzRsakmXuj0owvBk/s9SJLgTPcGbU3lnNhp4Uw8QNfQHC573TYlVOLW6ApbQTseh4Xf+NJOrt+JcmpXdqktnUrjdSocaVD47pUIv/HFZua3dHbXWND1ehLJbDbd/HoMiSAz85v86tO1vGZRicWi7KxUMckSz1zZYndTMQ+1qkTjMm57OZvBGFazjD+SpCRHJZJ6U1U3yGf2uzjXGyCdMbKvKY+9dTY0TeOvX5llaHKNvEPlHN3pJBitBjQONFjZCsZ54r4qFFlhX52Z9U0LfSOLOFWBIJsvP7/iY3HFhywVIfQMk3NrNBQVEYgIhBDIxnu7ivwpiulV4Bu6rv8lvKJPId8AACAASURBVJXjrwLR96t4NzH97/7zBv+270Y+4Il+LNE9HqSlworFbKDQqXFnMU6+08DyZhJd15lZyergeyxJxubDFLhlDjW7uD6WndSb3RJ87VQZfbNplrcS5Oe4efJgPgNz2ZBLGLNuNJqmEUoqfOFYJV6rzv2NMhWeFK31ueTYjTRXusl3KzQWGxmYTTIwFaKxzIYmZH5ww8/JNid5HpUVf5bBYjEb2V2l0D0ZpzzfgmRQaKnJ42LfOg1FBh7e66ZrPMGznT4e2etCMWik0hpn+0LsrzPx8G4nXXfidI7E2V1tIpXWeemGnxO77MhGiVPtTp55fYU8p+Bs9yYXbsd48lAhP/XgDmIZM5Ik4bIrRKMJtoIpXh8Ms7/BwVZEZ2Q2TEOFl/tbCynJNdHRYCMUS/H0/eWUFrnZV2fGYDTQUluIosjkeSzoOm/Jed8T6J+e2XvgAtlG/iZU4NW7qfgTv04fiUTxxwxUF8gkkhnynDJ/+eIwXmuK9iqZ23MJBuY0msvMdDS4+Na5KcpzIJXWON5i4cVrG5R4jUiSxFYgzvXxJAfqTZTnW5hejhKKJnFaIJVM8tINPw+1O2mssDO+ECUUSXFrJk2R18jwbBBfOE3XaJitsMbrvfO81ruG0JNc7Z2mvjSrQgtQ4spwZyGC2ZimNM+CPxRjdDZMSa6ZklwzF3sWGV/VuTqa5vTlO3isEjazgeoiE/0TfsryslmAkhC80TNFMBymazzO69fvsLwR4vJwmB/cDHC2L8zA+DJnbmzQ0eDg5C4rN8aCtFVlE3JuTsQxkOHUHg9Xx2LYbVaMBom1tVUu396kvtDAgSYX3eNRXr3lp7bYSnmuzLEdVnom4jhsVspzjbx4dYF8p0BHR5ble3avsxav7//nEwKzruvhN/+x/fmuXEB/4hl5N3r60QIhnu+MYRBpij1G6iryuTkexetxMTA6j9ls5EyfjM0kIRsN3Bz1kdYkdMnIlVtzpLRSZlYi9I8u4nVZeXVAwaQYyHMa+aPvjvPYoTK6h+cpynVwZTh7yRXFyH/++0H27ihnwxfhyw9WUJ6fvWdv3N4iGE5SUeJBVQSybKRreIMFXw6RaAK7kuL0jTV+/uEyrt5eIxwTfPOVMfa3VqCnEzx1tApNgo5aE6ubpRiE/v+T995Rcl3Xme/vVs65OuecgO4GGhkgEnMmJUuWlSjrybIlS288b83Yfh7PjINsv7fG42ePbdlKVrJI2aIokRIpkgCRO3ejc6NzjtWVc7rn/VENmeKQIkgLtCV+a91VuPvcc1HVVfueffY5+/v4fneAfIeWi4OLnNxXxssjCfyBINWlTprLzeyt1DO3VkBzpZ19VVogx4U3teSktljLlckEZa4MKq2RQDjJzEqY/ik/Bp2aYLyQta0A0VgCg6YEl1nN+FKEF/s3ychqZtf8SAJU6gpWvQlUUoZL11exmvTUFJt4z8kKRud8ufD+Njo9/EKF91FJkvbdJKiVJGk/Oa3JN8W73ulPnzzCyNV1WsoNlDpVPNcb4FfOOOmcSnF3m4HFFR3t9S4ON+QKVySpEk8ozaNHrciyjNmgR6uRONFkxGDQk80K7tqdj4ei8EK3wG6UOLi3kng8xT3tucKVqdUomoPlGE0GwqE0DpMafyjB+dEYB2sNBBtLkDNp8hx6Du4txx+IcKRWjV6rYyeY5Fz/Ol0TQY42W/FMRtlTW8QDB2xcHI1xoN7MK0N+Nv1K3A4zKVnm0SN6nuv2kEzLtFdp0WmUfOdanPcct3F5MsXwfIhjTRYmlsNU5yuwGtU8PxDkY/eWsuxJc6rSyLcubLLpiVDuLOZgo4NISonBYODuNiPPxONYSsyUudWQ0nKgXkWB205toZKXhnTEEymO1ipQqxTEk9A3oeFQcx6LG1EsBokUBiRAo9Xetu9aIMj8/ITvb4b/APyzJEk36/gLgfffSsd3fXgPcH+HnRsrMa6OBynNM2LSq0gno8STGRqq8/GFU/jCaW4sBymwQXuFiqGFBJeGfbRVavCF0sytRyh1gE4RwxPMzeVfHo7x3z7SyIJXhVmv5M5WExdGcw/j6Q3BnfscqEkzuRyldzrC2EqGxw5ZKHWpMRu03LPfSu9MEpVC8Mhh+4+X984Ph/m9D7cglFq6p+IcabBgMcD3O32cbM5FC2fa7FydCKNUCNLxKC8PemksM/Of3l/HCwNhXh4KckdzbhUhHEmw4lNQma/lgQN2Lo7G6JkMsr/WgsOiY8WT4pnuII8cyePM/nx0WjUWowaTyUQsFssp+ZhybEL902HW/UkONjoYXwrzg14/Rxu03Ndh5tqN3Gan5/oi/PrDFcxvxAgnMowu5+i8hQDN7R7pf0Hm9EKIPqCBXHn5p4BGIcTArfR914/00Uic7/XkhCeuDq/QsaeCofkYZ9vtfPfqNgcb7NQUOfhupx/kFI8cNaNUKBju9iELJS6rhoaiJC/3e/i1B0ppKNFxYSyBvBjkaKOJdW+CS9c3KXVq8fm1ZLNpvnU+QFWRge7pBFmlnUXPBm6X4FSLEoVCYs0Tw20CrVrDtj9IiUvPtj9LOBzmv3xxiaP7KuiayTC/FkKtM2B1W/HFg+zs+OiatSDLGYQAWRYMji+R77IzNbaO7mg96wHQ69SMLIbRWwqZ8abY9keJRuN0WdQIIRGORJmcC3BgbzULngSXBxd48I5GYsks+2qtfLfLTzQWpypPS0Gdjef7fNQU5yKhe/ZZ+N2/n0WjNWI06BlZiuPwlRD0brK8vMb4HLTX2RlYyKAzmBgYm6D6ZAMjC1EE3Pbw/udozn4rqAeayJFgtkuShBDi62/W6V3v9KtrG2g0Gu5p1WFUOmisUCAJmRf6A4wtBKgoLcA7k0SnStM3vo2kyIW026EMQu9mSj5ItjBLoO95ri4YUYoEV4aWKSwux127n6qmSu7XzBNeG+VMY5YNb5Ivngtw6qFHOXniAPF4kmT5DWrMWTLZJOfGh+jrn+fEvjKuzOtYC+uQzcU0NR5nr2EdydDLAx0qJElCn3eKrELLxz/0ID/40RUGezo5XCOj0+S+1mc6Y3S0VOANRPnw3ZVsR2SONuhY96Tw0cDHP/1JJEnii1/7PlnfDY425AK/DV+MmvI87mhUcW08wKcebWB8LcPcWoK+2STIgu9eWuaBYxVsBbJ0jW9jc7Vxfk6PWm/hxNl8qk0r6JVpUsYW7n/4fgrznQyNTvGNp57n4Sd+FZfTxt/9w/d4/P0tlIoJ1EoJhSRhMNxSLuptIbdOf9tu/45CygnQnCLn9M+To5C/Cryp09+28F6SpK9IkrQtSdLYq2z/XZKktdcw6dxs+91d4YApSZLueZX9dUUFpJywZs+uAMa3d2m83jLq66o4VKvl6c4AeysszG2kUakUGPLqOXXnvXzsM7/NRz7zX6g/8iiFpRU8ctjJ3jIFR07dR3NTCw/ff5JoWtB+z+M88J4P8Kuf+c+0nnmcPQeO8r733kfbnhpcTivtx86wvJNl2qPhv/7uZ1Bp9SiVSr72zCVOPHA/q54wd995nF//9K+jKWzivR//D3z8U5+l6fh9NO9pobWlBllIfPwTTzCylKRrKsapU0fpaKvj+sg0m5tefud3f4vz47lU1cp2lMoCPemswG7WUFNiwqTJMLiQYmQlzXseOUPf9UlCoQjFRXkIfT7pTJbzQ36ONVp48KCNq5MJomktlUUm9papyEh6HjxgwWVVYjHpaShSo1KpOHriNI994Ak+9elPkpV0/MdPfxBPwszEto7/+Jsf5OWL/UiSRNfQHMV1zdisJhaW1misK+eDv/wovcsaFAoJIYFG87a+xlvGL9A23PeSI6PZFEJ8DGgFbikhcjvn9F8lR7n1WvyFEKJt93geQJKkJnI8Y827ff5WkiTlq0QF7iP3RPvA7rUA/8/uvWoBP7ndSW8LDouWx49YuTAeZXDGzyIH+M1PPYHJZCCVStPZPYzZbOYzn/11hhdiTEaqeeyh05SWOFle2SQsDNz9vvdzoWuMrr4xmo/ega2+nWu943z7mXPccayVE8cOMLqlx13VQUtzDUsrm6RSKTTOYtRqNQmtBa8/yBeePMev/f5/o7N3nK8//QoH77mbYDzNV7/1Ao8+cILy0iK2E1aErYmKskJaW+oYnZhHUipyEcsj72dgPs3AfE4Rd3Bihdby3Mi/t8pEOpVkNaChtaWO6dlVvv3Mee4+fYAP/sp7+WGvH7NRT6FDTTCSYnx2g+nlHc5d97G0nWRja4cvPDeHxaDifScLODcSJr/5Lv6vz3yYnoEJLlzu5/ihVnQ6LejdmF2lKJVKykvcTE4vojQ6OPrIY1zpHuXcxQHuONoGwK985CN84QfLIECne0O69n81BL9Q23DjQggZyEiSZCFHMFt1Kx1vW3gvhLi8y3d/K3gEeEoIkQQWJEmaJVdBBK8jKrArnHGGfyEj/Brw38kp3rwlzC9tMp/Mw2zPo/mwmc3+GSJZFSPjc9RVFXPuQjdKtZbW5iqeO9fDuf44H/rlcja3vdx58hCf+/Ov0v7Q+wDwZzVcHVvjgY/dQzKR4MJ3hvHMzuGLy2jMTlLuPYzMbaJ54RoqCf7iS9/jno9/CoBTjzzCs09+CWNxNWarhSFPGNnsQqFQcO5CJ4V5dr76zBUktZahlTQVcowrXcMc3NdIeWkefYM5nQWj2cjleSVGWebSgh1v0sCO5RRhbW4ELdgr8/zUeb70g+sMjdxAzgqyT19FqdUzuCJoPHwHi2oNjgYrTclpNBo173nwDlKpNIPDUzz17EVC2kq84Rij2ytURNL0Dk6yte3FaNTT3FjFC+c6iaFnbWmF/us32N/WwFNPn0NdVIvN4eDL57qpLHbx3EvdRJNZgrEk/rQZkDAab1mH8S1DCEH69lLzALmIlpwU3E2Rhf/7VQPc75IboLLAZ4UQL77N/6ZfkiQbOT7JASAC9N5Kx3+LOf1vSpL0EaCfnOyVn5xQQPerrnm1eMBrRQUOAU4gsEtQ+Nrr/zfsihX8GkBZWdlPtGkcxVTllXLi8F7ml9ZIFzbTtL+dqeFhui5cYmZ+lfsef4zgcpqaUw/QN7VO4fEHeL6zC99qH68MTRGyXeGFV84xNzWD3+ejP5sGpZKdiSnUgRBnn/gkJVWVAFx78SVqzp7h+W98nZ4bi2S/9y+6Ieeev8yZ+80sfvNbXH3pAurCQl7Z3mTHYODY/qOcfOgBAIIJGaPJhKrhCH/79PNY1IJLfWMYn+kmr7yKT/zBnxK+/grprMyv3f1hkjMDPHj6EABfePJlGvd1cPzhhxldWqbM7ebUL+dWeoLxNFq9lntOHwCgb8ZDMJnAbDLgC4SZDUr8xh9+jvDwJY4faub6H3+V2rseIeDz8Z2//hYdBw+hXEpT2HqaAtMcWUsBobwG/uGlHp6/PIAhb5Xn+/tZm17k7AefoLK+DoBnv/GPHH/4MS5c7r6tc3qArPyOrdT/xa7e3I/xmoi2CDgnSVKdEOKWn0SSJB0TQlwDfmt3kPw7SZJ+RE7taeRW7vFOL9l9HqgG2shx7v35rv22CV1ATuxCCNEhhOhwu90/0VZSVkbf1AbxeIIXLg3T0N5K0OfnhfOv0B9NkT2wj8WNLYrKy+k+f4FP/vZ/Yryvn9o9exjaCVD0xK8w4/UQbqrDVF5CxSP3oVIoQKumcE8jztYWvvHC80wM5ER+k/EEKpWKeX+QtNvFdJGLSaeF7hsT2M4cZ0IpMxLyUfjw/RhKClFrtbiLi+hcmmdrbQ0Ao8lEMBjAZLFw34c+zHQwRcTpYmJlHUd+HkazmXAsxY3NGJV1tSxu51RuJqYWsVY0ojXoCXh9eNRKhjbWCQUCDPf00nrkMJPrISLROLIso7U6OfzAI7xyeZCvfL+bs+99L1aHnYXNAE//8Cof/T8/y2h3D/lFRbgOH2Q7naKmuRFXfh6rcwtoNTqKSkuZ29yk4H2PoSkvJSUENb/6IaYncju4bwyPUN3YwKmHHiIry7d3pOfNQ/vbHN7/OKIVQiyQY3E++CZ9XoubsvFdNw1CiMVbdXh4h51eCLElhMjuzkW+yL984J8mdPF69h1yEr2q19jfFu7+8BM8/fw1VBYn33nySf7q20+S2L8Xg8GAY08Tq/UV/Mmf/imz8/Nc7+zm0ssv88d//j9IV5SQ9PlJKJWMffGrZA06sl4/a0MjeIfH0NfXkM1kULY28Z3hQa68+BLxWIyLL71MpKwQ8/5WfN19RPoHcd91EmdTA5GJKRSpFBkJlDotG4PXWZudR9ncwHPf+z6ZTAa92cTZRx/h+tVO/vFrX2fdbcN19DDbLhv/8NxzdL1ygYHhG1S350RaOu5/iEvXhvlR7xytx46QV1TI33/+82ga69Ds38uVl86xeGOaksoK7vzAh/j+i1388OUu6trbicfj/NU//hC9w83CjSlkWQabmxVvHFd+PkF/gGeefAplbSWZg61855vfQpZlLHYb8Xicv/yff0Gwthy1zYJvbgFLZRlqg57JpUVkWWZmZJTmjv0YTDnVWpPJ9Ha/xjfFTY68W3D6N5O1uhX8ppRTX/7Kq5infhZyWGlJkv4BKJFySs0/cdzKDd7R8P6mss3u6WPAzcz+s8C3JEn6n+TCnlpy8xOJ1xEVEEIISZIukMtgPsVPima8JSyurfH9/h7WJiZYWlym8PAB9KXFEI0ikkl8F6+is1pwP3gn8clZOo0qpra3sDTVk0rEkSQFslqFwWrBVFOJxmgk5fGys7SE/0oPyVgcA6Crq+b8+gaRcxcIkcVVW4tOr2Oxq5fqQx34r3STzmaYH7xOyYF2jHYL1roazB4vKpUa/7UufFnB//qDP+JDn/oNhvr6+aevfxNleTG2liZ0TgfpiSn8ajVP/uhHRBcW8FrdqC5fRgGMXbrIyXvu5dKPXmRrfZ2JqWlcBi22xnoGV5fZX1SCJEmoVCpSpjw6h68TntrGbzUgtbbQo5K51N+N9tnvk28y41lcpKZ/gEg4zEI4iLqqKPf3zLfy57/3+xjdLgb6+9HluSnQa0gpJLZm57Dmu4mvrBEIhfnL3/8Dzj78INdeOkcsEQeJ2zrSA8jilsL7N5O1QpKkc+RYpV6L3yMX0f4Ruejzj8hFtL/Kz0YO60HgTnI5rVvajPNa3DanlyTpSXLriC5JklbJaeKdkiSpjdwHXSRXB4wQYlySpH8ip/qZAT59c54jSdJNUQEluVLCm6ICvw08JUnSHwPXyTH7vGVoLCYi9lqyW5tUl5ViOtROeH2D1RfPEd7cpvnh+9BVlQMQTSTRyDIl7W3IsTjm6lyyVFpeR1lYiEqnJxEMoc9zU97ajLy5zer4BMlnwrjqqpElCOhUmKwO7McPEPN4qbn7NFpJgamtGc+Fq+z79V8ls76Jqa6G0OIKppJiRCCE/cQh0skUI3/3Vf7kb/8G+4E2ZJsZ994WEqkkvmvd7IxPUve+x3HtaSTc1cea34/txGEAtKsrjMQjGEwlbG6sUbq3GW1DLamNTcI+HxfmF1gNBchmZfw7XoYHB2i4724MNVW4JIlI/xDWjjaSLicLA8MYSkv456VZAr29xOQM+fEYmWyWRDTG9twszUfaKZYzZBUSqsI8FHY79ZksUjqN7dA+dJ4d+r7+bbYmyrBVVxAYvsFtT+QhSP2MEnlCiDtv5TpJkr4I/GD39F8thyWE2JEk6Z+BIiHE195K35u4beG9EOIDQohCIYRaCFEihPiyEOLDQog9Qoi9QoiHXzXqI4T4nBCiWghRL4R44VX254UQdbttn3uVfV4IcVAIUSOE+KXdpMbbgq+rD3P7XqLJFNlEgszSGuXHD1PS0kQynSJwrRc5myWWTOLpv466vISM2Uhyaxv/9REMLfXoqyuJra4T6R/G2FyHweUgvuOjpKkBS2UZKrMZhc1KxdFDFN57lp1zlwkPT+BoayERibDdM4ClugKd044IRwHILK2gr61EzmSQZRn/pU4aPvlR9GYTClngbqhHXlnHXlGOQVLQ8smPIa+uI0kSSpMJTV0V0cERZFlGn59H3B8gE4liVGtIJFMotTqMVRUYjAa0exrZtlnwVhQT12uoe+Ae0oD/4jViU3MkQmGS4TDRgWHsdxxByFm0dgfmynKK6+qwHD2I7dghNCol7Z/9JImpedRaDY4jHYSmZlk7dwFDXRVplZKkx0d4dJKWT/0fyIvLJHa86MxGhLjN4T3vzJLdrkbjTbw2ov1lSZK0u9HrzYj2rX2O3ID40Nt9f+/6HXnbG5tojEbCO17Sssz1v/kyje95CG1JETpZoJJltPv34n3lKvrCfPzjU+Qf3I/GbiNwqRO1XofG5UQD7Fy8grm0hGwqTXxuEZVKxdqNaapa97De00fQ66OybS/yjh+j08l0Ty9GswmNQsnUtW5qOvYT2NhCiCyhF18h72gu5ZHJZtk5fwXHsUMkw2ES2Qzz33gKW0UZBruNyOVO7G0tqHU6wpJE4MYMKpMBY34eoUiM1R+8SN6RA9idjUQ6+1CZjDg6WklMTpPy+XHccRilRsPmj15BoVDgvvMOJIWCwJUebKePE9v24LnayerkDfZ84qNICgXZTJbotS7sxw/jvdaDNp0hcLUL54nDKJQKUjota0PDFMZi6DQa5sYmsRhNpDVqouM3MBiNxLZ3SMlZNr/7AyrPngQhbm94L96x7P3/+1Yj2reBTkmS/hr4NhC9abxZdffT8K53+qWFeQLRMPbWFoz79pLx7bBxtQelP0Q2EmVnawutw4ZaocA/NEosFCLeP4xCpWB1YhK91YpKrUZksixfH6VOpyewtoG9o43YxhaljfUYDrTiLnKTvtSJcd9eVHodgek5rCXF6FoaUOi1lMTjaJ0ODEV5RKfmWJlbxGa3k0ZivneQ/Loq4jPzYDZi39OIQalCqNVIei0z5y5i1umJ35glnUiw/Molqs+eJDE8hiIWZ2P8BiarjZRaydrUNGqdlsJkkpmuXvKrK0kMTZBMJPEsrSABuq4BVHod/h0vwRfPY7fZ0FktuEpL8Xb2YTIYmOsbwFFfg2J5FXVFOVNf/xbOynJSE9OEYzH8K6sUNjXiOHqA0OIydfeeRa1QYigtYuG5FxAZmdJ8N8X3nMExOkECGUmhuM0j/TtTUCOEeEPO+d1o9XNv1P4WcHT39Q9ffXtyc/2fine90x88eoyVnmtotFp8Q6OU3n83yR0vqbUt7HccZuOLX6fsoXtR63UoLlzFlOdG21KHUq+jIiOTVSlRuByoCvIo2PGgstvJr68m7vNhyM8jvLmNRZZJTs1T8/7HiPQOYTmyH7GxRcXD9xIdHCWazeDoaCUyOYNZziMVjVLQ0oimoQa1xUy9EERDIfR1VagMejwvX8J55gSRrgGSCgXlZ08ip7NYD7ajC4XwLi6iLy5EbTUR6x6k+sG70BcUonXaKUYiFU+g29dKSTiKxqBDt78VQiHKLSZSWRldSQGafDemUJhEKIyqqZ48g56kEBgL89G6XdiWV1DJAq1Oh0ilSMXiOPY2ocvPwwAou/pR7JJcZpfWsJ88guf8FYxNdRSWVyAbdWi0GqIeDxmNGja3QZJuq9PDL47CjRDi9Nvt+653egDXicNsn7uMVqlEbdCjKCwgJAuWn32BwoP7iI9OEnfaMZeXoqkoJdTZRwqBZU8LapORQM8AwaERik7fQWRyCsW2l9jYJI7Tx8iYjESW19BYzSiUStJqFYltLypTTsgiGQqjkiS0Vgvaw/vZOXcZg9mMsaON0LUerEc7kLUaXGdPsPPyRdBqsR9sR6FUEJcEycUViu67k8j8EpGRCZI7OzR//EN4r/WSyGZxnTlONiMTmZohNjmNtaMVg0pFbGgUrVGPVFRA/MYMsY1NHCePYVQo8L1yBaV1FWtdFep4nIXvPkf9h9+PUaEg2nudyOw8RfecJrHjQ+EPkHU6qHnwXiJTcyglBYH5RayNtQSvj5KdW0RXkctdmTta8fdcR+u0Y2yqY+diJ+FQEL1aQ97dp+EvP3/bC26yt5a9/3cPSZL+6+vZhRB/+Hr2V+Nd7/SezU38i7Nkk0k219ZQu+wISUKl0ZIIhYkOjKDWaUmPJig9eYzUzDx+r5/Q6hqqrECj0aDXalhYWCK/aBZ1RubG09/DXlmOLZ3GUlbC9Ff/kZr3PwaApamO4c9/haoH7yEdi6GtryLQP8zmtV70AnzrG8hsUqhQkNTrWXj2BYpOHsNzuYtEKELIt4LJZCaRTiFicXbmFjBe60Gr1zNx8TJl+9pIjN5gsXeQhjN3kI7G0JhNZHwBdHYrql2nyqrVJP1+LJZa5l98BbVOi/H6CEhKVHodSwPXKQhHSCOI+X2EB4ZRyoLF0TGMDju6ynKUFjORQJCd518i746jZFRqZn7wIpl0Gm1pMbLJSGx6Dv3JI2x09qFKJJnv7af5zCniAyMYzSbGzl+g9d67iI1NIoS4vU4vfnbZ+38HiL7q3zpyS3m3xFn5rnd6Z34e9jI3voud5NfXo6+pQq3X4Rseo+LOU0Sn5lHWVeO51o1ks6FSq9AHQpDJYD3UjlKnIxEIYhgdR9Vcj06rpTgWIyPLbHf2olOqiIUjhAeGSWezpBQS1oICYstrJDw7RFfXiQeCNJw9gcZkQmRlVGYj2j1NxHsG2JldwF1Vhe3APmJ919G5HGj3NqDUaBDzS+SnM9iOHyZ4Y4bWX3kv6fUtDG3NlGxuoTDo8Y9NYpBhvqefmkMdRLr7USqV6JVKlucXMJWX4a6uQAFo2vagUCoJDgxRWFNF/tkTbFzqZO+vPUFibBrjoX24olHC6xvEfH7kRAo5lSSTTpPZ2MLW1oICmdjaJtlwFGU6w9bKKvqFVayN9Sh1WsplGWE2oqmrQZZlrJ3dSEolptZm4Pau0/8iUWALIf781eeSJP0PcqsDb4p3PXOOQlLgGxzF2FyH+9gBEpMzJMMRcHZo/AAAIABJREFU1Oks2sJ8lCUFbHf1ojYZUas1aEwmdOkMJQ/fR6ArtzciPDhC5aP3k1hcwT88hrmlAaVKhfvIQZSlRTQ8fB/peALn4Q60GZnK9z6EVpJwtrbgKimm8oG7yaxvk0llkMxGpHQ2x6cvBDUP3ItCryc8OoFxTxPOIx3EJ2YAkFc3MFnMObEMnw9tSRGxaIxkOIyxpIjY6gau/a1kjHrKjh1Cn+/GeuwApsP7SNktmPPcaEqKMFmt2I4dIjaY28mpDEXIKlVkkinMGi1as4mMRkXKs4PJZKL88YdReXzkte9Bm0jS8vEPodPrUCRT6IWC0ofvQ97ykDWbsBQXo3M70VrM+K/14jh+mIzHB8BOdz9Vj9xPwLNDcmU9l0S8jVV28AtVZfdaGLjFKrt3vdNnshlUyRRatwuFSkUqFCbcM4hp3x4ArDWVJLY9FN15isTkNIGpWdTlpSgUClQ1VcTGp9GbLehtVhSxOKp4Ak2eG9vRQyTGbyCvrmGsKsd19gTj//CPhLY9hHoGkZRKhv/+qyTVSoyF+URX1ti+2oWurppUKknoxgzGwgJsDbXEl1fRKBRonDZUOh0Jnx/vwDD6xjpiiTj+zl5Mbbn36zh2iKVnX0RdmIf9jiP4r/WhzGQpOHaQeDJBcmk198E3tqh67AG2L15Dyneh0unIaNWkNrfRGww4DuwnODwBllxizbl/LzPPPMf25ibekRFCqSSTX/4GiUya6PQsGoOeiae+SyDgJ+kPoCwqwDswTPGdJwldHyOTTKG3WFCq1WTtFhKb2xiVSqxlJeQVFxGankMIgV6vf93v6WcBIQRZWX7T4+cBkiSN7m7zHZEkaRyYAv7yVvq+68P7q+dfIWPWE+sbRlJIeJaWQZJQXO0hm82SzWaJBAJ4XrpA2O/HUViA7G5m41o36lSGxfFJTPku0ok4M509VJw4SnB0AoVGw8aNKaRsFq1ORzQWo7CuFr3NivlAG7Issz23gKm0lOj4FDqjkbXBIYwOJ6vTsyiFoKy9lVjvIMvjk9gKC3LqL9ksiWgE/+wcFRo1wS0PqVAIkcmgUObUdrzLKxROzYNWw+rIOLaifEQiSTKVJO0LoAmG0ObnEd/cZnN6GqVOQ3B9E0ttFeGhMQx2Owq1ioWrXRQ1NyCFowitGqVGg7uxFn1JEXI2y+TMLPnHDqIx5YQwyn1+FHoDsW0P2S0PoY0tIj2DpOUMw3/7JcrvvwtdKoO9qYGdcxcxlpcAkE0k0TfWgiShva3EmL842Xtyc/ibyABbr6o6/al41zv90VMn+eFgH4YDrQDY/AGMZhPmQ/sA8Fzro+0TT5CaX0JVmM/W9WFKS4pwtu0FCfQWE8lgGOvRgxRsbWMtKUJdlE8mkUA/NYPZ7cR0aB/xa71Y9zUQuD6GPhYnuLxC+f13kl1dx3KwndD8IvaCPMx7GzBMTWMrLUHf1oIsy9QoFKQiEbStLTma6K4+Smw2zHuasG15ULhduM8cR5IkoptblMViKEoKSQSCNL7vEUI3ZjC0NWNUq4kvLjP+vR9Ssb8dTXkJhXU1OKorULidpLZ3CAeC7KyuU6hWY7RacNRUoquuAKAinUUEI2gajOxc7cFZX8vN7eTeS53YD3fgvdab22XodlNnt6FUa8g70kFgdQMpniA2O4sqK7M4PEaNRgOBMIvDoxRr1UjvgNO/E/X07xBUwKoQIilJ0ingPZIkfV0IEXizju/68F6j0aKuLCM2PgWA0WAgFouRSaZIBoJo9Xo0dhuJYBjJF8RdUYGutASN0UC4ux/T/lasxzqIDAzjqq4iND2Xu7Ek4SgvQ2i1RJbX0Ol0aKwWXHccJjZ2g8zaFobCfBLRGJl4guzKBhq3i0w0Rl5NJZJOQ2rLg7fvOpraKnRNDSSm5wEwKJWkkinkrIzJZMa8v5XI4CiyLJOYmKH43rPIWx6kSBRtYT7u08fxd/aiUKlIbnqovus0lqY6Mls75J85TmRxGZVeh76sFEdRIY7SYoz1NZTvayUeCJBa29z9Y6mIRiOk4wk0Gg2uA+0k17eIrG9iLMxHpddhP3qQ+MQUyYkpLG17yAZDeDt7qH7foyiDISxNDWTUapoffwiV1YJ2fyul7W1IsQRC3F6xC/7tS2t/lngayEqSVEOu7qQS+NatdHzXOz2AubSYRDJJan0TpVaD89hBEjdmCPUNY9jbRCoURlYpWZ2awtTWTGLsBv7BYax7m1AoFWTiCVbGJlkcGcW0fy+R62MEuwbRNdeTkSTGn/ouQpKILK+CLJNMJNCoc+SWrhOH8FzqRFvgxn1oH3NPP4euvgbHvr2ExyfRZrJorBYMeS6ia+t4h0ZRV5djbmsmMDoOZhN6p52MWsnkl7+JMBmIjkwwefEy2WQa/+UePBeukUxnGfm7r2CqLMO9by9iaweDUoHGZMJ+4iiR/hG8lzox7m/DdvgA0ZExMmoV9v1thBeX2BmZQNis2A+2M/9Pz2Bsa8ZgtyHF46Sm5jA01AKgVCuZu9pFNBYlPDlNIBom5vGi1OvQ1NcQHZ8CXwB9ZTnZcARvVx+6xlqEw44QAtVt1LLLldbKb3r8nEDeDecfB/4/IcRvkeO+f1O868N7n89LbGURRSLJ7I/OozWb0cdjbPQM4q6qQNXZS1KtBDlLSX0tYn2T1ZFRhJxFl82SWd8ia9DjKipE67CRXd1kfWwSFAq0o5NYykqwupyoK8uJ+wMELnaizsqsLq+g1uiQhczqyBhmq5VUaIJkJEJmao6MUkk2m2VzYoJakxE5nQaRZeXKNQra9qB2OVm71Im7sR45GEZSKkin09jaWlBpNFTv+NDYrRj2NP34s3r/+gsEp+dJb3nYmp7F1b6H8Mw8UizO6ugYOosF08Q0sgKmX7lMXkMtKa8PtVLF5A9fovnkcbZHJokGQ8QHx8BsZHVwGHtDLbHBUbKJJOFEAqVCifv4EZQaNcGlZVAoiE/NoMrKjLx4jsbjx4kPj+Pz+oh7vRhNRtSpDAqF4h2Rqv4FQVqSpA8AH+Ffim9uKUx614/0DocTw4E2TIfasRcUYLBZce9rpbCuGndjHfY7DlNw5ABmowFlYR4qtxNrdQX2okJ0e5swtreQXFnDefIIGrUKVXkJ+TWV5JWXYmzfg6TVUHR4P+m5RazlpRSePpEb4cvL0La3oKqrxl5WirK+ipTZRN6eZhRuB/q2ZlQaNUWte1BWlaI/0AoCShrqyT9yEGOeC6VWg8lhx3hoH/F4nLKzp0mvbpAIRdAU5pEIhUlHcns4fCNjuNpbMTTWYtjXSsTnwzc1g9JhQ7aaqTx2BHdZGbrWJtQ1VVS0t+EqL8V18ij6fS0YrBbMRzswmI1U3nkKVXkxmvJiov4Aaq0Ww749mI92oEKi4rEHSW1uAWDPy8OZn4dlTyMxf4DCtj3oWxsw7WvBZDSQX12FobWFWDgM3F4BS4FAluU3PX5O8DHgCPA5IcTCbtXeN2+l47ve6YHcCHylm/y7T2LQ6xAILJXlRLe3SUeihJZWUeS7cTQ3kFlaRSdJFN5/J74rPWxd6sTa2oRKryMTTxLuHcB27CCqihIS80v4BocxVFWQieaczz80irmlEduhfcSnZokOjVLx+INk1raQdrwUnTxKaGoOOZNBbzbjOtJBdGQCz8uXcJw4hKaihLTXT3J7h7rHHkBVVEDgUid6pRJrTSXJ1Q38A9cx1FTiPH6Q+HCuqlMdiZF/5ADy1g7+oVHq3/soJrMFSalAXt/C0FxHQs6QDkcJXuvBcfoYMX+ITCxOqKsfR1UFO5e7MB9ow95UT3pxleDULC0f/QAKjZrI4CiR9U0MBfkY890okykCnX0YWpuJJBN4LnZi2dtI/pGDJNc22LncjW1/G7Is8I+MYa6vQQhxe0f63Sq7X4QlOyHEhBDis0KIJ3fPF4QQf3azXZKkp9+o7zvNe++QJOnlXa76l2/SCEk5/NUut/2IJEn7XtXno7vXz0iS9NFX2ffvrlXO7vZ9W7+Wpfl5EhPTOM+cQKFSEZckNi9cRV9fjeP4YSL9I6TmFtHv7h+P7oaviR0fklLB6vAYoYkZAld7mbrWhc5sITK7iKmkiOjiMgalCpVOi5TvIjQ7jyqTRZPvRmMyEd/YQp/nRqVRE1vfQF2QB4D9xCEWn/4BcQmig6PM9Q6SSadJbWxjq68lu7aOMhBG43QQnZtHpVGzvbRMuLMXj8eDHEugVKtz4XKeE9/gCMo8d+48mUCZSKKwWlA6HEx87SlEVsZ3uRs5kWTki18Fm4XY5haGlnoWv/8CWC14Fpbwr28S3vaQSSRIIMAfIhmLEdv2EN7Y4sY/PUM2myGbThNeWUdfXEh8Zp7YthfP3DzppTUynh3Wu/tz3ASDw8iZDBv9Q6QCQcRtdjgZQSqTedPjFwRvuFHnds7pvwr8NT+puPE7wHkhxJ/tClf8DjkGnPvIEQrUkmO7/TxwSJIkBznGnQ5yKy4DkiQ9u8ug+3lyDLfd5BQ+7gVe4C3C5/US9vnRDo0jp9Iokyk8swuotVpUShXhbQ+JeBzjSG5vuN5gZL5vgLp8N1mdhvzqKmxHDxLb3CYvFEJVWojQqNm+1IlKIbE2PYPWYkYrSYy9fJ6GI0dIXh8FlZLNmVmM3h2KkilWxiZpcjiIdA+STqfZWV1FX1qM8WgbDakUqNUIvQ7fxU4WB4awFOShMOhx7G9FbTCg1mowHGgjPTTK2tUe7EPjSAg0mSxTr1yi5cxJEkOjTF7qpPrAfnx9g0gKBa7yMsxHDiBJEgm/H8v6Bqa6auI7PpKbW/g3N1DnObHmudCbzKhUGsKzSwRX10mGI5RUV+I6eoTg8BjuZApNWTE713pYm5mhxKDD1tGG0+sjk3ZgaGsmsrVNaMeL+1AH5qpKFEoFxtEJJJcD6XZq00NupM/+fIzkPwO8YfLidjLnXAZ8rzE/Qo6jnt3XR19l/7rIoZsc6WUhcA/wshDCt+voLwP37rZZhBBdQghB7sHyKG8DbQc60Gp16PY0YDrUjroon7zKCgrvPInzxCHMDhsldTXoW5vIWkzIyQSlHe2obBYMChXm1hZiS8ukZ+YpvvsM2bUtDC4nBWfvAARljfUY2pqJh8NU3HEMbYELQ8deDG3NVB/cj7WwAHVTDe6yEkRBHvoD7aSiMdo+9QnU8dwylqTVkgiGcmWt1RUU720GSQHpDCKTW3dOp1KkIhHUsQRV99+FwmpGu7eJ8I6X2kfuQzjtpK1mas+cwFCcR/6Jw+hVKhwdbSSWc1yNsaExbM0NSJks9qoK1PEEbZ94Aotag7uoEH1FMaqMjNrtwl1Rjqu8DH1hPkLIqIVAW1aMnMjtbnRUlGPvaEcIUDvtmDtaiY5PE5tbou2TT5BZ3SAbyQlyykgkJ6ZuexJP8IuzI+9fg3c6e59/kyJLCLEhSVLerv2NWEJ/mn31deyvi5/Gew9gO3aQyMAolkPtSB4fuqI8El4fwb4hXGdP4LnWS/xyF+Y8F9q2FtKxOIsvnkfrdFF0oB3fhSsYiouQJIl4KIRRCHy9g1hamwmNTeEfHsdUXoq2tAhvdz8Kq5nQ2ibGilKcLgeBy904aqtJzi0SmZrBduQASo0GbXMjnktdGEqL0NitbFzpwmw2U3D3KSx9Q+j278XbPYBWktjZ2MQSjeO68wRIEpsvXoDJGdynjqIy6Al3DiDSKRy7de2htQ1cRw+i0usI9w4RM5jQFeajq6shPjpJNpPG5HKhNhlRlhThuT5CXlkxG529kJEp/6VH0KXTJCdniHm92I8fwahUEBubRBGOUvbA3cTHbhD3+3GfPoZCqcTTP4xOp0NtMpJ35wm2z11BU12O58YUpXeeRtzuzPo7x5zz7wFv+AT997Jkd9t574EvAHR0dPzEdd5tD57ZKdR6LZGL13DW16CWYOTL36T28AESg2OE1zaJBQKk66pRev0orSbUBgMimSQ+Nsni9VGqlGrCPj+6qnLWL17DWVJMFticn0PKCqyV5ah3vJjra/APDCMhIbscxEYm2ZifR2cwEg+H0OiNmGYXySJQyIKF7j4aNcdQG3RsDI5gueMY6VCEaDiCXpIwNtYSGRzFO79AtqSY7CtXSctZfAtLSEKg7NaiVquZ7e6ldE8T0d7rSEKwPTeP0eVEV11BLBxCGh3HcTq3q49EktTkLMbmemKDI0Q3PSSjMWKRGGh0xH2bxIfHEakUsz19uOprSQaDaK0WPOOTFN95CoVKRTYSxWC1kI0niC6skIzH2Vldy1UIZrNodDpmv/ssWouVzOraLf9Y3i4E7yqn/+03aninnX7rJg32boi+vWv/abz3p15jv7hrL3md698ynHluXFZdjqJqsCcXMlttmJxOLEc6UKrVOONx7IV5OHedIjg9h6WjndDMAoaWRuqjMRQ6HebaSmLTC2wMjaDMykhRO/llZehsNoxtzSQCQbI7PsI+P7FgkOLyYnQlBVRI7ZDJEvT50SiUaFubfhzqVkfCKKwWYiJD8eEOVHYrvvFJQqvrRJ5+DndLE65Tx9Dp9UgSmI90kIolsDqcpCQJS3E+qjwXdQoFCqMBY1sz8fNXKDt9AimdxXN9lHQgSMjrR282g5CZ6+vH5HRAngPnvr1Ez1+hsKoSc00V2h0/ioPt4PFibGuhOBginUoSW98gODZJ0OtF3dWHs6yM9ZlZVBoVllQSZ/te9F4/RpsN/f7clufY9g5VCNRGPfqGmtsf3u8W3NxuSJL0S+Rk1hqBg0KI/le1va6slSRJ95IrmFECX3p1Jv419x7lpw9we3dfX3qja95pp3+WHEf9n/GTXPXPkhMHeIpcIi+4+2B4EfiTV4kF3A38rhDCJ0lSWJKkw0APuQ0K/+vtvilJpSGz46Xs9B2YCvIIjN+g8pceITZ6A/O+PejMJkRJEfGZBQx1VYhND7o7DoPVTGBwGLXdSnxrB61SRToQoOb+u9GpVEh5LtJKFfEdL0bA4HIS2PRQ3N5K1h9EX12J75VrOM8eJ3i1B51Wi6q6guTsArraKry9A5j3NpOdmUdKJCg4dZSd85dxnTyKKp5EKBVoiwtycY9OSzQWQxeKEOy9ju3EYYwqFd5XLiM0ahz7W4mPTOAbGsXS0oAm342v9zr26ir8yRRuux11QzVqs5nKTIaMALPTgZzJoLNZiPgDKD1eFA4rhjw3voVl1F4fRrcTCtyw44PmeuxlJSR8PpRuB86KMpRKJba2FgKd/ViOHiQ4PUtqx0dWIZGencdy5ACxngESE9O3PXsvdrP37wDGyO2U+/tXG99I1mq3+W+Au8gNaH27CeuJ17n3zUKbT+++fmP39YNA7Fbe3O1csnuSnPROvSRJq5IkfZycs98lSdIMuQ9482n2PDBPTubni8CnAIQQPnJiAX27xx/u2gB+A/jSbp853kbmHkCWZXwXLmM/dQzn3mayy2uYTSY0RiMxn5/A4hI47RjzXaS3Pexc7cHYliN8UGrUzF68QmBlHdlpY/nZ57HWVmKrz3HW+671Ymiqw3poH7HJGYKT0+g0GnS1laQSCbyXu7Ad3p/LnKtUyNksxoJ8YqtrCFlGKws0NiuRSASN0YAsy2TdDgb/8u/RN9fhOnkUf2cfW90DqCpKcR7qINA3hM7tIu4PsHHxKkKS2JyYIjW7yOrMHIGZBTIqJelYHHtHK+HJKbQqJe6Txwj1Xs/tf9frsR87SHh5nfl//j66xlrUBXl4rnWhr8mtBJn3NDP29SfZXFgkvLjM5tIKSz98iZRGiaq4AO+Nqdzynk7L8N99jXgqRSoQxN5YR3T8BomxSSxHDpBJJNny7OBfXbv9ItHv0Dq9EGJSCDH1Ok1vJGt1kF2hViFEipyAyyNvcO8lIcQScEwI8Z+FEKO7x++QS3y/KW6nau0H3qDp7OtcK/iXJ9dr274CfOV17P1Ay7/mPUKutFZSKogPjJBKpVi+PoSzoR55fQNdcwOrP3iRwjPH8V+8Rnh5NVc9p9YQisdJKRW4y8uwVVUgmYysblxEMzKGcWkFtUbN0vVhTBYL2WyWhevXMVjtFLe1EB2ZYGV0HK05V5KaSmdAo8K3so4hHMbYvpeVH76IpbaKrQtXyYTCLC0tU6rTYHK5MJhNBGYXiE3MoDUYmOkdwCgkZJWSxYHrVBw5iEavw33kINlUiuD2NprqcuxKieDwOIGFFbLRKCSSxL1eEuEYWqMRyWnDe7kLlc1CZnQCEY2ys7SCtWsAnclEaHObxMAwkkaNrNVizc+j4MA+NE47ytk5Ntc3UUkKpKyMubqCrYkpSh+4CykUQqU3ENvZITA2ydrgEEXNjcTPXwKdllQwiFAq34Hs/S3P6V2SJPW/6vwLu3mhfy3eqlDrT4NRkqTjQoirAJIkHQVuiXbo30si798MJ86e5enLFzEe2odJkiCTISsgGYkSWVwmmUyS3vbiOrAPJAXqZBLjoXaMQGh+EVt5CenVTYzVFTSeOJrTcTu8H//sPPWPPkBiYRnn6WMUxeLobZYf00KV+AJk0mnsB/ehMhrwjk6Q3PKS3thGxOJ4ZubR223knzzCVmcP7jwn+sJ81C4nBXW1aF1OTLsRhXVlFc2eRtRGA/WSRCoUxrRbDrvT3Ufl4w8RHxxFUisxlxZjKy5Cm+cik8oQ6eknnc2g2dNMxrPD1CuXcddUU/bAnfgvddP+qY+TXFojFo7gKitB19KAymQgcGOW0tPHyW57URQWoApGKbvnDErAUF6K58JV6n/5cTIb2xgsFmSbBaNGh+ZsI3aLhVQyifV47ndt12qRM7e/5FX8/+y9V5Ak+XXu98vMyqrM8tVV1d5778f09Ng1IBaG8CDBSxcSLy8V1yj0JD0oQhHSk96kBwWvKPKSgGhAkAAIzzWzY3ume6a7p3vae+/L2yyXqYeahUAJwO6COwtyB19ExnSZ7KrpzBP/c/7fOd9nGBQK7ymf+GfZWhmG8bMs1n7WBvRPy7jf7Yv+18B/EQTB9exxhKJ11rvihW/DFUUR1/l+klOzRBZXsLW3YKkqx2Y245Blev/ov0JCQA+GsUsS1u52UivF8dnC3iFqbTVpvUAukQCTTLpQIBuLU9g7xNFUX5R+fuseqt2GUeJC29gGwGKzUvrqNcIPJ8knU0iROFavB2tTPZmzM/r/w7/FJIoUAkEsiJRevkhkZp5CNovF48IIFsemCzv7NH35M2RXNwjNLyLVVGFuaya9tAqA3WwuCntaZJJ7B1SMnie9VMw8I/ce4LgwiOv8IKGHk+RW1hn4D39IaWUF0SdPcQ31YHE6MKJxFAPKPv4K4YePi70DpwGU6kp0LUP49n1cl87hbqhFPwmgxRKoJR7s5aXowXCxru9oJXFwQD4aQ5BlhIpSstt7pANBhBI3GYtcHIN7zvig0nvDMF4xDKP7pxw/z1Px/Rq1/rzPnzIMow/oBfoMw+h/L0YX8KuVHl3XSUdjnGxukQ6Fabl0EbNeYP77P6JpeIjMzDyK2cL+3QcIZpnGkWGCS2OI5X7kEjcA/kvniI5PI9hteC8Mk3r8BKvdjiAIxFbWQRTYnl+grLmRjCwjOO3oz+bGpYoynvzxn1E3PITocrL81b+hbOQ80b19Ch43O/fHEXUDezxBybVRgrfuYamuQsvHSN4Zo6S3G9FkIpdMICGidBen6kJ7B5jCEUzWovyUd6iPg4ePyWcymJrq2P7W91D8PrJLa+hpjb3JSdouXyK3uML65DRmxYKe0jCZZPbnFzDbHThSSTK6QeTr38JWUY40s8D6xBSlrY2kNrZRG2pJxuKIj6bw3rgCQFaRERMpbID/6ginr99GKfXjbGog8PY9dL1A+avXUOG58/S68aFt5P0svC+j1p/3iwRBsABfAOoB0zul0a8ksN8DQsEgJkHAVebHXVKC0ttJJhKl/eOvUojGUQaeaeVl0sR39jm9dR9FVXn6f32N9s99ksROsRTbfDxF+fAgxvIah8uriALUCALWmioSpwEaenuwnesjtbTG7Ff/hurOTijoFAQDu78U17kBott76IUCVocVJBM4RQpVlWh7BwSfLmKkkpytrOGMxPBVV7I1O4/T6SK3s08sHCF2fEpKMBBVC6Ldxt6btzAAfzKJbDaTzec5ffQEa0kJgc1t2i4MoVZVErh9n7rrl7HU12LxeykPhVFUBfez9Btdx0DAdfkC2USS9a//Pa66Wuz9XVQFg6h+H3K5n9OHj8icBUmnkthniiMXNkFic2ML1W5HEEWip6cE9g+oM8DmcrI6PoHL50eXhOffhgsfyhSdIAifo8gm+YEfCIIwYxjGr/2CRq0/C98BohSda9+Xj+MLH/Q+vx8xdobN6yWVzZEJhkg+XcBzbZT4+haZ7T3k2iosiCiXLyKm0+iqim1xCcNiQRcEtHAU1eVEdjux1tfiPzwqmkZ2txHf3sXe2oy2s49oMmE4bLSMXkRyOFHamtHuPKDyletkTs4Qjk+pfeUGkm6g1lWQTWnYBAFPUyPW4V4Ct+7T+VtfIrd3SCIRx99Yh9zaiGyzYjs9Q66qxNPRBqJAIZMjki9QUl2J58pFTt+4zcC//wNS03MonW00axrC8Rkp2YyjvAxrVyuJxzNY/F7sDge6101maxejxI1U4kFXLWhbuygNtTSeGyIdT5CLxbFabRiJFIrbReX1y4RuP8BeKGDp70KUJIJP5ihrrEcd6iW+tUvl8CCJw2NM7c1El1epuTKKLknoPu9zv9YfFk9vGMa3gW//jNd+qq2VYRg/pMhivVdUG4bx8V/k+73wNT1AcmYetasd32Av0amnKBUVCKKIs7WJ+NYOp3fGULrasFWWk947ILu2Qc2r1zEVdEwWM6ZwlJavfBFLXif6+AnOc4N4X77Gyc376KdBlJpKNC1N4uAYAmE8I+cgFid09yHOC4M4KsowYnEsDgfOhloSG9sYuk50bALn+UEwDAK3xnD192B+0ONGAAAgAElEQVT2ehDyeawmM+WvXic5u0Dg7jjO4X7KPv4yiamnmB1OLB4PVZ2tmP1eNv7223guDiHJJlKpJIGn81hqq8lkM8SnZ1E7i6o3yXiCQi4PFhlnSyOxnT2C9ydQ21pwNNST3N4hvLKGUObHd22EyPgUokkimc+SS6UJ3J/APtCNa2SI5NMixWxKplGtNrIpjdz+EUprU1EpeH4JczJNSW8XGV3n5Pb959+Gy0dntJaigWXPL3LiC7/SP7x3j1Q8gXl8EkmW2Z2bo8VmJTO7UHRccdhZn3yCXVEo6DoHy6vYXE6cAhjZHBa7HeezKbXw6jr22mqyW7uI2SyhwyMEAWSzmeDWDsdLq1R0tpO5/YD9+UVkVcXa3Y7JauXk6Tx1n34NALW/h6f/51/gqCxHvzPG7pOn+JsbyczOYSkrI7axiez1kHz7HsH1bUSLmWwmg1xVgePSMPHxSXK5HFgtqFoG8gUSMwuY7VZsDXVsvfE2DpeL9GmA0P4BtvsOMoqM+8IA+6/fxNffS2xnHyNf4Gx3D9eTObIZjbSmcfb621T0daJtbCFKEutTM1RdvkBkcgbV5cTscgIQicbRZuZxNNSS2z0gcm8cz43LAGQTcXamnqA4HciKBSGZJJt+T30l/yx8WCv9h4TLwO8LgrBFMb0XKLLfve924gsf9NXVNYSlSpSGOmIbW3R/4bNoewdYnlFrgTtjlDbWYT0/hCiJNEoSgiRhHexm6n//E9pfuoY2v4Sey3O2t4fSUIerp4NsNEa9VSEXjuK4OIQvkcSsKDhHzgHFGzCXSlEIhUnvHRIPhsivbJDKZUllNNB1Ki+PoHg9FJIaroZaTI31ZMNhooEQfocT38dukNPBbBh4r18mfXpGbnOHwNY2sXCY3t/+MmZfCQbgGi3aXp/NLZJOJMibTLj8PuxNdSjlZShmmezSOkcLy3j9fkzlfjBJlDbWYx3uwS5JOFIpIju7lI1exGQxc3rrPo0DfZg8HuZ+9BYdV0ZJTkyj6zoWk4mNO2M0DQ2w8nCcuv4+snOL6KKEblXwVFXibKhD6Wwj/vZd/M2Nz52nh4/UaO1rv+iJL3x6X91QR2JrFz2fh6NTlPoaxJoqtI0tMvEi9eQa7ie9vkk+mwWblcQzFZzWSxcQJBG1p5N0JErtlRGc1ZWIokh6Zh5bTwdCmQ/t4Biby1mkpvaPAFBtNkquXkYPhNBMEs2f+DjpZALXyDBml5OOf/MlUnNFazJ7TSWalsEIhRGtKk1XLmFvbyExOYtZErEP9ZFeWMFc4kU7C1J9ZYSa9hbiT5co5PKk0hp6QSefzaIfnVLZ00M+lUKpq8Lb101qZQOLx41juI/ang5Epx2lqgLFZqXkwhCptaIKb+TBFC1f+QLa+vaPlX0wdAomE/WjFxHtVmwXBnGMDJNXLXgb6rAOdNM2MgIFHbmrA2tvJ6LTiae5CUnLkNjdJxkM4W5rfu6U3Tu79x8FEY2f6MxLU+T03zneFS980AO4L51j4+vfxNRQh2EYOOtrSOwfER57hLWrHbWkBMJRQuOTWFqb8JwfJrW8hmS3kgqFiG5sYa+vxTfYT2F7l+jyGo72JgRBwN3ewtmjJ4h+L+7OVhKr62jRKLrNimxVSORzHD+extDSmOpqOPrhW0iJJGa3C3NTHUe3H2A4HbgHeoitrRO+P4HS0kgunyNwdMze0wWSc4tsPXjI8p9+FdfIOSx1NZitDuwjwwTfvo/o9ZA6OCT49hgl10ZxD3QTW1hGqS3OLImVZWg7+5xNTOG7dIHk8RnHN+9h6+lAcbsgEie+f4SttgpriQc9HCFwbwJrdxu5bI70wjK+kWFS0SjZaIy8piHndWo+9TG0xVUkq4r72iXCt+5TyOXJLK6gtDaS1zT237hFzfVRzOWlH5k23A8DgiD8+rN29i3gDrDNe2xFf+HT+3AgSPZwj+jxKZaFRaTFZURBQIvFSEeiOJ/MYTgdJLIZLIKI6ZkZw+HCCr6RYfLhCMdv3ab+0gXiK+uETk4orG+QLi9F3dzBYrFwurWFJIlk947QCjl2/vyvqerqQEgk8TY34lFtKF3txA+PSaSSRE5OEGUzsmxiZ+IRzQZowTCq3cHe00VsqzVYPC6qX71G9m+/jffqpSKtli+QW1snnUhytLlNXU87RlUFp2PjxAMBul66TubJPNmMRvjoiOTENFjMyKU+kktrmC0WIhvbmESBwMEh1sezJAt59EwGJR7H+9IVtGiMYCyKnkjiyuZIpJJ4ysuKYp+jFwjdGqNQKOC9fgk9l2d/aRlDELEHQ+iCweGffQ3FZkV88IiNicd462rJ7x6S2z342QPgHxDeEdH4iOB/AS4CbxmGMSAIwg3gZ7W+/xO88EHv8XnRjvfp+u3foLB/iH24H4DInQeUVlZiHeojFQgSm5gsDqOYZTAgcnIGkzNUv/YKJW43an8X4c1tIlu7RXfWwV7MJZ7iPsHnP01sfQv7UA9Os8zh9DMxibbiVFtkYwerKCIkk9QPDRDd28c9eg4DaC8UEM0WlMFezu48oO7la1hUFUtVBYHxx9hLfQBY3U60go65tgaH10345JSjt25TdukCvo5WPIkqrJ0tyC4neU2jJBJFqipHqa4ktLbJ4fomiVCIri9/FudQUXnXfeUCdi3Dxjf+gYiWIXNTR3Q6EFIaqstFaHGZwNI62Wgc8VnIHq0XSwEkiawAktmCt7ISx/lBcqkUsm7gqKrE1t1OuyxTyOWwnu9Hi0bhQ6jp/xWp3b4bcoZhBAVBEAVBEA3DuCUIwv/6Xk584YMeQE6mUctLOVtaRc3lSe7soDTUEV3fxKrrZLZ2qLs8gh4KYTvXT2h2nu4vfYbUWQDjNABAcHwai1WlrLON0utXiDx8hLOxDv3gBMvVi3hrqwi8dRdTZRmV54dQu1qJLyxhNiskC3lYXUcpFFA6W8nZrKS2dknuH1JyboDg+CRKNouqKtg7Wgneuo/5We9A1mwmMDWDu7kee4mH2Ngj0iVuyocGSGxsY/F50Xf3cH3iFU7fuI33xmWiu/v4zw2QXFrFUlWBtayUusFeRENAC4SInYXI2VQS23ukVjdo/PyniE/NUnLtEsGxx5RcG6WwtYvU1ojDakM7C2DpbCF45yEtX/gUsaVVXF3tyG4XifFpNHTy0SiJJ3OUvXqdyL2HGAeHyGU+Crkc2t4hsbVN3mNJ+gvDMD5SQR8RBMEO3AX+ShCEU4oNP++KF76m39nYRK6pBMAzMkx6cRnj6Ayltgq1pYntb30Pe3UV5voasuk0qdMAZkPAXFWOq7eT46UVZn70BoLbgdrVjlW1gqGTBxa//X0C+wckJ56gTT1FsdvYfPM2ZReHyB0c4x+9gNrVSnRtna03bqIrFvJaBkdVOfmTM1SHA5NVxXv5Apvf+AfMzQ0A2Ad62Pve68h1VRgmE6ZkGktJUXIgls2yc2eMXD6PqaGG9b/+BtaeDgBKrl8mcm8C7egUpcSFdbCH1MIK8UdTOIb6SCfieM8PYj8/QGRplflvfAtLiYdsJIJUVc7BzbuoZWXIJR60VJrE5AxKWzPpQoHJ/+0/ozbUInvc+EYvEJ2cLXL+qgV3dydP//wvsXjcJJbX0Ev9nN4fR22oxd3WTGJ1A9Vh/xBa7z9SuvefobiJ998B/0hxvPzTP/eMZ3jhV/qD3T1SsoBxGqCQy7H6cILSxgbMU7OIosjZ5ha+ykpyp2cc7R0grG1QM9hHYnyaZDKJkcnSNDSAWZLQnjzlbGsLk13FO9SLVZbRUmnkng4ki4X07DzemmrSC2ucbWxQiMWRrFbqP/cpDm/epZAvcPLwMYoosTH+iM7r10hOz4MoED0+JrO9g354DIZBcHsbf3UlqWAIrZBHnXqKlkiSOjmhvq8Hk8dBavugaI39ZB7FYiGrZYhGo8QOD5EdNvRQlP2nc1S0tZKaWyZbyLP199/DX19L+dWLGPcmUDrbSRwcoe3sc/h0HpfNgRaNcrS2isVqRVleo/zKCPlojOTxKamdA+wOO7piYflrf0NpcxOpqVlqeruxuByY6qrRllcIHx4Sn1tEEATO9ordih+Gck7+X8nu/LvBMIzkTzz86s9840/BCx/0l25c4zsPH6AO9aGFozQKAnoqjTLYS2xvj44vfY7M+ha+i4OYDg+QzDL2Z/34HBzhSCRJxuKY/V6oKkdY3cAx0MPp/Uc4utuxqirxiWkcI8OIqTRqfR1ydQXC5hay243a1Ubs4Ijyi+fI7u5TOtCL7HaSS6UxPM6ius70LN2/8xUK+wfY+jqJrm/hLC9DHejCcnCIaJJQB3uwCgLyxDSZZAqn10tidpGB//jvSD56gjLUhwJk7j8kenCAo7Yauasdq8uJns1g6+skOZ1DFiQc5/o5efseTV/6ddLzK7gHejgLhWn4tZfBAKWjlYpYjEI+j6W5HllV8LU1k40nsfV1IXvciIkk+1MzWGpryOs6NtlEcncfZ0UpYsGg6dOfQCoUsHW2Up1IYRT0D4Wn/1e0kv9UCIIQ56fXQe805zjf7Xf8UtJ7QRC2nxlVzLwjVvBBGmG8b5T70bZ2SUzP4jo/iNrTSWJqlvTuEUqZH/e1ywRu3kOVLbibG8keFS2bsmubWNua8Q71kd3YITQxjcXvJZtKY5EkzA5HcQUr87H9ze+idnfi7eti+/v/SM2nP07BYSP64BGZcBhBUSi5MkL40TTRzW2sLQ0YZ0WRIDmTRSnzo2kauUQS4/CEihujpPYO8VZWUP7yVcJ3xwnOziM31GJurCOyuILq9WKyWBBrKjl88w7xB4/wDvRQefkiBhAee4Stvxs8brT9I5R0BhxWspEoVtWKrChFerFQQMlm8bS3kopEiayso1RVUPrqNUL3H5ONJ9AFAe/oeWJPnpLPZIlNTNH1b3+f9OoG2voW1ppKfJcvEJucxZzO4G5uJHlwzNmjJ1haGsmqlufehmsYRa7+3Y5/yTAMw2EYhvOnHI73EvDwy63pbzybAX5HrOAdI4wW4Oazx/BPjTD+kKLJBT9hhHGBotzQ//QTWnrvGeFgiMzeAXPf+DayqpCeWyIfDKFJItGNTSSLhVwsiqmijIMns2QzGpG5JU5uj+EYLHY8iqJIJhbHalbwXxxm8++/i9zaSGJ1g/TMHJwGiJ2cEZ6aJnR7jEw6Q35xDeH4lFxaY+uHrxOcnCZ4dwzRbGbpb/8BCgZJvcDxrXtYOosyap6R8xz86G2U+mocFeUc3R/H0t6EKMvkZYnt22OklzfIbO2y9oM30RNJtOmnCJEYh0+fIkoSelrD29lGZG4JW1UlkmzC3dlG6OkCgs1G6YVhMisbiEqRmnRfGCA6OYPodlHIZlE8brZefxMhmSI5s4Ba7mPpL/+Oo/kl9l5/mzwGT//4T8kXCuRW11HcLk43NtBmF9GezJNLaxxtbKLNzKE47Bw8niY6t4gWinwIvfcfqZr+F8a/pPT+M/y/yrdfpah6+9/zE0YYwLggCO8YYVznmREGgCAIb1J0ufmb9/OhdocD94VhGmQZ2ePG2tGKFosTn18kfHTM0a17yKV+xFwOX0Md9poqsvvH7E/P4LDayEsiSBLpZIKT7V2qBEiEwkRm5nH196C4XSTHJqjt7qTk+ijBt+5SOdyP2FiL4nFjA3KpFI6ONuyN9cSW1yhpqCODjpFMcbSyhtvpQpMkDEngZGsLs03FeXBCKhYnObtI2tCRJJnyvm6UhjrMlWVED46wNDVgLvVxdn+cupevIVeUkzg9w7S+xe7jaawXz0M0jiFLhPb2iZ4FqE5rbE5No7jdlCYSiILI+v2HtI6cJ/DwDJPTibOsDLmrA1Eqrhn+/WMUvxfPuX60SJTo/hHeS+eQ7XaiqxuUVFag9neRz+aRQ2Hq+3tR+7sIPHiMv74W/43L5FIphP/jg1Ck+vl4EYL63fDLWukN4A1BEKaeGVHA/8cIA/hFjTD+fxAE4Q8FQZgUBGHy7Ozsn7wmm2Wij6YouThM5uSs6JGuqJiTabp/77dwN9SjOuw4bFbqP/dpCEXJJxKUdbQhd7agDhVvYLPZQk1/D1oiQcvnP4PT5yt2swGKSUaUJMJjE7jO9eM/P0T2mYJOeHYe7/khxHiCbCIBsTh1n/445mweSRBoePU6gsOG0t9FTrXQ/eXP4/B5MXW2YC3xYO3uwHd+CEWSKL9yifjSCoauU97ZTmJ+CUPXscoW/P29FA6O8fZ0ope4qR7qB0nC3NcJ1ZU0XL1EXWc76lAfNT3deGursdbX4rp8nob+XiwVpZRfH8WUSlN6+SLpvaLXSGjsEf7R85j1ovlkYvIpTV/5Itmt3eLf/jSA2W7HMAzCd+7juXoRCgWyiQQWRcHW3kJq75DIw6n3fxe9T7yzkfdux0cdv6ygHzUMY5Bi6v7vBUG4+nPe+882vDAM408Mwxg2DGPY7/f/k9eymSyK04loMmHpbCG1vP5MHfcy9ooy0ts7iOEw1q42TGYTYlpDtTsovTpCen4ZgMD9CdznBtgce4hzeBB7VTmZfJ7c0QnBJ08xN9VzuLqGlslgejaFlo7FKeTzmFJpzGV+yOaIj0/hGO5HlM3sPXyEo68bd1sLmqaR3TvAOA6g1FSRiicITkzR/OXPkFleJzI2gW2wuLloO9dPam6RgihgHe5j9zs/RKqpACAZK5pEisEI1S9fxXlhgJObd9j81veQa6pIJRI/lr7yXR0hfnLG6l//Pc6+TvKnAYL3xrH19eCoqkSIxogureKsrUR2O0kmEwSnZ7F3tSPJJoR0hvDcApaWBvC42f/+G7jPDyLJMnpBJzrxBFtfF+6merTNHVRfyc+6fB8Y3uHpf5Xe/xJgGMbhs39PBUH4NsWa/IMywnhfGLt1m6zVQvLRFKIgsXT7Dm2XRygsrVAQBHZm5mgdOU9q8imCSWLx7hilAz0oWpaMXuB0cgYtHIGpWfL5ApnNLQwDhHSKrZsLCIaOnNKwOu3kolHSs/MU0hqZfJ61//tvKb98kcTcIqtj49R0thN7+JjwWYBsWuPk8RR2ux2z2cLq2D9i9/uxzC0iO+wcTDzGLMscLa0iqyqm8Ul03cAwDI7X1jFbrVRncpytb+Lx+8ifBTA3NrD17R/gbm/m9OY9bE4HJqcTu08jODvP6cIyitMB98fJFQpkszmi+0dUnAQI7h8SD4XJGOBsb0E7C2HxOMl4nIQePCYViRJf3aD+pWsYqoVEMoEe0MgFQsjAycER3o0d0rLM0dIyZp+Xwq37WK0qa+MTtI2O8HOcmD4gfKTacH9hfOhBLwiCDRANw4g/+/ljwP/MB2SE8X6/z5WXX+Jbd++g9Pcgmc20Gzr5bAZ1oKiu3ZEvUNDSWId70fN56gMhspqGtrOLzWpj7s2btP/mF0itb9P+e7+FvrGJ9Vw/ViArm0hs7+G5egHzoxlyNgXZ6cReU4m+sk5wZRVEcPR2UL61ja2jBWtNNYVb96n99deIjz3CdeUiADX5HDktg7mzndzhEU6fD8+VS2RzeUyihK2nA9n1TBhVL6A4HKjDfbQX8khWFbWrjbPxSYJbO/i6O/C8VHTrSb19D5ffT8noBWyChJ7P4756ET2fJ3hrDMvVEeSGGuyhMFa3G9dAL9nTM3YXFyhrb8NT6qOkpwPR0LFabUglLiIr6wQ2NlGdDpo+8TJnT+bwNTdiqq3C7LCRfPsOZReHcLU2U8hkaJVETIqC+Jzlsgx+VdPDLye9LwPuC4IwS1EU8AeGYfwjH6wRxnuGSTbhfekyodtj5DNZZIsFc0sTyaeLxRrbriKUetG2dgneHcdz5QKu3k6kfIFkNkPrZz+FeBrCZrGgOB1omSy5WNGNVdENSkeGSW9sg2zC3dVObG2T1FkAIRCm43e+jJIrEFvfwjPUD6chIrPz2Dvbil7yVeVktnbJxBPIJR5cly6QmJohv7lD6dVLpDd3UBULvhuXiU49JRdPEN/dx1ZXSyoeRy8UkFWVXCyGJJuQ7TZ6fuc3SG/toWcy5LN5LE4XaS1DZGMHU1V5kb7cPSDw9n1Krl/C3lBLLhTBardRcnUEbXEFe2M97aOXUBQFi9eDxWHHKkg4B7oQAmH8Q/3U9nTjbW0ldxqkEI5S+fIVok/mMQxovnaZ3O4RGAbhh5M4B/vQguEPxcDyw0jvBUH4kiAIC4Ig6IIgDP/E8/WCIKSfUdUzgiD85594begZjb3+jKJ+bmnPh77SG4axCfT9lOeDfEBGGO8XosmE96UrBG7exer34qosIxwIcvSjt6j75MdIh6Msf+cHWFQbBUPHMAxiRydkkkmaLp7ndG2TTD5LZakfz/khko+myBbyuIf6MVlVNv/uO2TSGqWRKLLZzMLX/pa2l68hiiKJ41MEQcBxbZTg6gZmVS3W+ICzsZ7ArfsUNjbx37iCdnLKyfoGJlWlvvQiifFJZLlIrfleusLZm7cRRAHHy9cQS70k5paQLDJCaXGmX05pKGV+zB+7xtkbt8kh4L9yEW1nj+jTBWo+/TFikzOs3X1A3UtXkWQZm9/H3vffoHSwF7NVIeWwkj08ApNEydVLBN66jWOwB6HEha28jLP5VRJHJ3jO9WOyWgneuodsKhpZOC8OsvrVr9P821/GVF9LfOopisOBJJtQezqeu63VO5Tdh4Cfamv1DBuGYfT/lOf/mCIlPU5xofs4v6Br07vhXxJl90tBOBhGO96nIEDk9Izw8TGiWcZiQHh7n/K1LUSng8qWFiTJhPelUQAsswtkDk9QejqxBkN4VRXdbCJwZ4z40SlZLYVispBKJSlkclR3deAc7iM0v4SvuRFzfQ3R9U30Qp7Azj5mReFoeQ3V5cQoFBBEEVESKWTSnO0dIkgSpqpKvBXl5M1mwnceEItEsLqcxO4+xGYyETk+plDQUcenEVQz2w/Gaf83X0Qt8bD/nR/ham/haGwCOVfAZLFwML+A0+XCouucBAIk51dwtzWT3j1AMAzi41Ok8zkykShqdSV6Po+5vJS9129hSCJqIICEwfxf/DVdL98g9WQeQRI5XlrBpqpkDB3ZYmHn6RxWm41MWiMeCnF06x42m52d6SeUNjUhCcUZveethvthteEahrEEvOcOw2d7WE7DMB4+e/w14LP8KuifDzxeD0qpk8j0LPUvXyO6tona1kQmnsRTV41UU4kgilgry8HjIrWwgtLRghhPgclEIZdDsTtIJhOU+Hw4qioRb99HtdtwXBhEODjGXlNd9KczDMRwnOpPfIz09FNKLwxwOj5JWWMdUmMNLSUukocnOM8PICkWoivrOFUVSVEpuXKJ2No61tZmMqEwjp5OchOTBBdXaP7Nz6PtH1Jb5kcPR1GHi4lU4dZ9Dm+P4fC4OVrfQPb7cHd3YrbbCd68Q3lbK+pQL2cPHuFrrEepryY0v0T1J15Bm1vGMTKEdBZg++Ekhzfvofp9iIqCIEk4vB7KXrpCLpUmvLOP3NmGJMvEb96lemgAU00V5jI/if0D7PsH2M4PkJ6Yov8PfpfM3iH2vm4URSGdSGDu6yITjX4otlb/Amr6BkEQngAx4H80DOMeRap5/yfe8zPp5w8CL3zQA0SX17C6XJgry/GW+kjPL5KNJaj/7KdIrayROQ3guzGKIIoE9w45++7rlL9yDSmdZvsffkDNr72MYpbJrG5ASwNqiZt0KIKtoJNZ3cB99RIFu5Xd77+Bf7AfURRJxePY83mUgkFSFEjOLeN/5SpKcwPxiWkMmxXFakXp70IMR9DWNhCDEcztreRCYVKnp9gtCuaBHjInpxjhKJbzg4TvjaMCofFJun7nN8gdHpHHoPe3v0xqdasY8Pce4Dw/SE7LkNrawS7L2K6MELp1D6vNimy3ETYKKLEEidklBv7bPyL8cArV5SS2tkntax8jurhMPhYnPj1H4xc/RXprl7yWxtnVjrm8lMDNu3hKPOjbe9jqqslEY5gNUPxekgsrxf0Shw1bSwPp+SWSgeCHsXn/Xtts39XL7he0tToCap/NwQ8B/yAIQhfvg37+IPDCB/3+zh6Z42P0+lqiC8vomsbZ5BPqL1/EZDZBKo3icZPTssW5dslE8OAA15N5TBYzqWAYI5/H5HSgJZJEHj4uWknHYuz98E38/b2k9g4QgmHO1jawqCpWUcR1YYjk/AqGxUw2ECR+coJj1odgGBxvbFHI5WkY6CU9s4AhCiy/eYvO3/4NAAq5HIWldZyXzsP6Jsvf+h7tn3oNQ9fJYBBeW0f1eDB7PeTXNxAFAUtZKelQhMO3buNrb0F2OJAdDpLb+6CqJA+PiZ0FOFqPUZHWkGsqiUzOYPF6CUw/RTYKrHz/R6guN7b1TcyqwuZ336B29BzWkhIiy5uIgLm82FPlvjzC9je+TfWVizgqy1j/+rdo+mLRiFWqrmD/h29R97lPIckmAosr5DXtedP0vI+a/l297AzDeOV9f7phZHhmTGEYxpQgCBtAK8WVvfon3vqutlb/HLzwQX+4vweqiizLyD4rhl7A6fdxOr+EmEyzdOcerZcuoq9t4R05R3jsEVWtrTgunSM0PklFaxPJ3T0yy6scrhU97uwLKwgFndPVdcyqiqWhjnw8Tu2NK9hcLgqxGIXdAxbefpvqzk4ExYy/vQ1TfTWy24UtGMSmqJj7irRhbHUDu8dDZHYep9PJ0ltv03X9KqEHE7iH+6nu7CAbiZC++wCLILL1wzfpuHENbWae5fsPaLk0Qmp6DrMocjgzh9PppHB4SiabYfX+OE3nB7E7bdj8Phxlfjwjw2SDYTaezFLV10PJUC8WjxuTZCKTzWJqa8Ysm9AejBNc28KIxlm5c5+WSxfRZuZAksgjkIxGCa9t4InGyWoZ8svr5A0wFQokQiFy80tkJYno3gGCAJLp+d6Ov+z0XhAEPxAyDKMgCEIjxXmSTcMwQoIgxAVBuAhMAL9L0SHnueCFF9E4P3oJc381GHMAABgNSURBVCaHvaoSq99Ldn6Zus9/iormJhwjQzQM9FHIF7B2tCKYTCgOBwW7lejWNordRkEA30APnisXKakop7y5CaWnk3gySfNnP4nd50Uyy1j9fry93Wg7e9iaGxFaG6jo7MLb04FdteIbvUBseg4AiySR0XVyiQSp01OkZIr6L3wae1UFeb+Pyr4eRFWh7PolABxN9RiahndkGEPXqelow9rbgW2oh7bLlxBlGetgD3qZj/bPfIJ8Jot6fgC1u4OagW6sPi/W5gbsdju+qyOkZhewVlfQef0KEhR19VMaotNBydUREpMzpI7PaPnYyzjdbpT+Htpeuk42ncL07P+fTaVo/8qXcNgdmJrrkM0W5O521OFeUrEY/uYGTJ2tiHXVlLe34iwtfe48/YdI2X1OEIR9YISirdXrz166Cjx9Rlf/PfBHP0Ez/zfAn1Kkpjd4Tpt48KuVHgBTYz3a+hbJnV281y8hSiKpeAw5FketqcJSX03o7bvkZRnvcB9CMs3e6zdp+53fILNuJnV8grZ3iPfcAJGFJZLHp6gOB7b6Ok7ujxOdmaPxy58rflZzA9rGFsntXao+8Qrxh48xPxPbNLc1k1pYxWy2YD0/SGx8EiNfwHN1BIB8Mk32+Izq114lG48TuHmPZEaj8sYVClaFhT//K9o++ylEj5vE1CzO8wOIVivZfI7MWYjkwgrea6MkLRbSS2ukDo8o/9jLxFbWSa1tYqiWohmmKBDd2EZy2PENdHP2xm10ScJ7ZQRRFCmUuDm+eYu23/0Ksd19khOPcbc0Y3P3ELo1hr2rHVmSMHtcxCWR0N2H1H/+E6QXV8imNdyDPYgOO8n5FTLBMCU3LmMcnVB4zjvrH+Lu/U+1tTIM45vAN3/GOZNA93P+asCvVnqCp2fkd3ZY/N4Pir50J2cYuo6tu4Ot7/wIa0sDeiKJ4nZxODtH6NE0yaNjVKedxPgkqt1OaGYe1eVCdNhRqipZ+qtvIGSyhO+NgyQgmiQCE4+J3B9HOjhm+Xs/QpckUgvLRGJxDja3SAWCKKV+EsEQuVyO4IMJNscfk45EOLl1Fy0SJZNIojjs6LkcucMTrB4Px/NLaGtbiIUChVyeyNIK2YVlUvkcJ2OPEHxuSob7SS4sY3M40EJhUutbzH3/BxiGTvrxDOZEkvlv/4D4wSHRscfoyTSLX/8WhWQS7ekSVrebk7UNUo9nOLt1j/D8AibJROjOGFZV5WB8kvD0U2IPHqHabcz95d8g6Qba7CIWi4Xg6jrZgxPW7z0kun9I9uAYbXmdtVt3ScbiBO88QFtZf/4lPQaGrr/r8VHHC7/Se0v92FxWmswWJEEgo+vEbxVv5lQkQuzhFLrbiZ5I0PRrN1DsTtL5LI4Lg9irqzi4M8bezBwmScI4PsXV1UpZfQ3mqjIcNZUEllaRjk6puDqCKEkkDo7wNTfh6GxDKfWTNgxyi1H0aJzk0QnpUIR4KETDr79Gm9NJ+iyEc6CX3OkZJ9s7yLIJRzKOZ2iAfDxG1WAfplIvibVNWn/z8xCJYW9vJj0xxdpbt+i8eoXEwQlaIs7R1jY1Tge+a6NYLGYkjwtrZzuR6Vl6f/Pz5E4COM4V6b7w3gFKdSUWv5fgk6f4qqtwXzlPdGUdV20NkZ09Sq6Nkksmsa+uUzLQg9lbQl7TsM3NYxnoRpJlErv7OP0+1Ppa6ro7MUQRe2/RTrtq7xB7XQ3WjlZ0XUf+L+9L9ekXg/HRD+p3wwu/0gNExx/hGOghmy9gdTqxd3eQTaepamvFfeUCgl7A1dVGSVc72d190pu7qGVlROYWcXs89L/2MUyqiqeyHJvfh6+thdjGFrlkkszBEVWvXCG1uoGu62RWN6n7zMfRD4vqO0oiSelwP7LFgl7Q8bU1U9XcRGx2AUtbCyU3Rok+nkapry3OntfVUnHjCorTTnZ1i6pXrpE7OEG12bCXlyImUuQ1DSMaZ+APfh9JVbBfGESy2fBUVWKpqSI8t4DS1oyYTBNdWMTuK0GprUKLxTB0ncDkDHWffJXc5g4AppSGVFpCeH0LIZ7C3FSPubaK9OExscczNH/li8Rm5shnskTGpyi/ehHtLEBe08jv7OOrqyO2tIK9txO1o5n41FMAnKWlpE7OyKfTBKdmMZnk53uhizt57358xPHCB30ylig2nZhMOHo7WPvmd0kvr+F96TLmumoiqxvIeR1zRRkARomTfCxGZGIKxenEcDnZn1+gkM1xMPOUuT/5KslkEv/1USJjkygmE6rHA9EEoXsTOM8VOzALqRSJw2PkijIcjfUsf/t7xI+PEasqSOZzKGYzJosZURTx3rhC+M4DFKsVynxou/vEtndR62sQRZG1u2NEYlEO7z5k4/ETzm6P4Xv5CmqZDy2TKVpKq1b8N66Qmn6KOZVGcjpYe/SY+FbRihvAPthDanUDczaPxeshlcty/HASpaURSTKx8f3XMTXWAuBubiR3eIxa4kaUREqujRK5P47Vacfb0oQejhIZm8B56RyFQh5zJofZV4K1rBTdYUVb3XzWyjtC6skcaj7/IfjTG6C/h+Mjjhc+vZ8cHydrtZC6PUZOEslrGVLZYj2uqFbm79ylcXiI/L0JBEFAkkQOV9eoM8tkMxlygojNU0L5K0VJgL3v/CPJcJjArftIsomt2Xnyus7B/BLO8jKsmobJqpKRTWSnZjA7HeRCYex+L66WJkIzcyR29onqBSw224/r3IyW4WxuAW9DHTnDQBJFhFIvoVsPMFnMlF8sGmNmTwMkwjGcM4vkNY1cJsPRW3dRqquIjo2T3N1HNwyqHHZc1VUobient8ewWW2Iipn1sQns1ZXE37hVHN2dmcdusWCrKsfuLeF0cgaH2YzZamXl1h0azw8jPn6CZJY53drCGnFTapLYffiYyiuXSKxtsj09g6++nsytsWLXnQDHW9sYokhpLE5wb498voAkP+fb0QAKH32RjHfDCx/0ozeu8/2px/hHzxO4PUbb7/0mua097D0dRFc3KG1uwtXXiexxAxBd36KsuRH/jVHMdjuRexMUatxoZ0FEm4rV70X2lWBvbiD49n1qRoZxlpSQz+dRVZXk/iGRJ/OQzRI6OabrtZcJPnxM3Wc+iTa7QOnIOawI5ACxvhrZ5UTXdRzxGN6KUpTBXja++T3S4TCtw/2YamuokEWy+0dY6mvxNjeQTaeRaqtRStxY0mmW/uKv8ZplKl6+SqhQgIKBtbsDVySKWTLhvFak/uLbu1i9Hvw9HTga64lt7WA7OkXtaScwu0DNa6+SmJrFcXEYSZbxrqzi7u3A4i+67DTkcggWC2J9NbnbD8gXCtjr6yhvbMLd1oza0ghAPpvHyOWxul3YhvvIv5lBsdkILiw956ttvBDp+7vhhU/vTbIJodRLeHoWa5kfi92OqGUAEM4C1H/2E0Snnv547FM/PKbhC58mu7pJNpXC5Hbi7esmv71H9OEUzoFu8mmNwJu38Yyco/T8EMlACEPLoMsy7vpaKl+9hmJVaXj5OrntPVRFxaQqaOgcvfE2al8X3tHzxKdnizJT9x7iOD9AKplCkk34q6oob25GslgITc3gHR7ACEcIPJhAaWvGO3KO+MwcubRG7MEjev/d72NzuTCyWax2B7aBbrTFVWSTTMoskwmFia1tIkTitHzlC3ASQBAE8juH1H3uk6TmlzEiUSxuF+6ro8QePCa8vErVjask51cACM0tIdfXQCZLfPopA//pDxCOTxAkEVepj8zR8Y//5tG7Y5RcGyGX1ogsruJobSaZzT53yg4obuS92/ERxwsf9Lquox2fcjAxSfLomLymkUgkiG5uozyrdW1DvSRnF4ksrKA2NxTVb6MxwuNTqO0tAAQOD0lEoySW11h7/SYmRUWbXyL1eBZFFDnb3cPqdpGaXyE8U5yZzyUSLHz3B8RDYbSZOWQDwvuHZBdWCN4ZI5bJsP+913E11mFSFQy3k90f3UTtbsd9+SKpJ8XU26RYSKZSqCYZSTZxNjNPOp1i8c++hkmxEHnwmHwuy8yffJWMTUUtKSF5doZuGPiH+jl8+y6mTBZ7fxcAeVUhdXCM7HZiMptJhyJYLAqCIBQlwxpqOLg7htnvRWpuILWygRSNYSnzk0wlsTociJKE/5WrRO4+QJQkzC2NpDe2Cd19gGf0HJIsk8znSW7tYKoowzdyjmQsRqFQeH4X2/hVTQ+/Su9ZmV/E6vfS+MXPogeDaCvrZOMJklMzVL/2MrGDI3LJJOH1DfKpFFVXLpFb3yKFTmB+GauiIikWCqKIo7mR7GmAttGLuEfP//gzTt+8Q2N/H4bHyfH4I7LZHA2D/Viry2m5MgqKgtLTSeLWfSqbm7FdGMQGqIEAy3/5d9jdTjJHJ8jZLMd7B5SUl5MXIHh0RD6ZQNM0tFCEsKYhuOzYqyshHKFxsB/3lQsApAJBzAtLFDJZArfuoygqW3NzNJnNHK9vUVJfT2pqDj2fw9AyzL1+k5Zf/wSJ3X2EijK2b92jJl9AtVmRVAvljQ0Ex8axqVZWJ6ex+7zIT+Y5Wd3AVV6KNFVcT/RCnu2FBeoKBZYfTlDR3ERmYZV4RiO8sYXidpFYXoN8AYvTwd7eHvX19c/vgv8qvf/Xv9ILgvBxQRBWnimO/A/vfsY/RWdvD4W0hqxpCNE49r5ubI11xM+CaEdnkMkjKSoSAg5/KbpFQVdVxGQGq8uFyevBOthD+cVhFIsF1WYjaxjkEkXXoeDYIzzDfYiSiK2sFE9lJXU9XdiH+0gsrWEf7MGUy5HXNNQSN3qJC+2saIqpzS5QdWEI53Af9nP9xSGc3m7U3k5sXe1IgLuqCv+Nyzj8firq63D2dJJPpbBXVZLN5QDIpjTS88v4uztwd7ThuXyRVDxGfU83qVyW/j/4XURJxDrUg/3CIIJN5f9p78xi46rOAPz99tieiWPHYxvHxthuEgLBoWkTnBAoYQuCgNrSBx5oESBoVVWCtqgvpUK8VH2AVqpURPuAKLSUsEmlNKJEISlbA1lI2pDNjuPY8TjGseM43uKZ8Sx/H+4xnbjjjXjsmbnnk67m3v+cc31+a/45d878i7+mBk+pn3iOMNh4DE+eh/xFRRSsWcXC+hUs9PupvHUDUllOSXUVVTdeR8xfzOU330BpXQ2+a1ahddV4SxaxdNVXyVm+hKqV9ZRcdSW+hq9DWRnLNt5MZW0NxStXkDs0TNGSutl7Y0yEfbzPbKMXkVzg9zhZdeuB74pI/UzvU9rglKPuDnQwOjhE7vB5Lr/nW3giEXzVlYSONFFz120sKikhN8/DaFMLFbffwmVX1xPOy+Pcv3ZTUFxM5yd78K1YTvn1awk1Hqf/aDMLqy8lz1+CAr0f7sJ/7RpCoTCDpz6nsLKC3Px8QiNB+nftw1u/gvJVK4l1dHHukz2UrFtNxbrVhNpP0fvex5RsWM/I+WHisRhnd3xI5cabWODz0bdzD0UNq4iVFhM63UOkNYDviqXgLyZ4ppdzH+3Cv2E9OT4v4cEh+j7YSdktN5CTm4PP48FXXkqor9+57659+CoqWFBZQX5REeGmFqpv2kBtfT051ZfS9/5OIkNDAPQfOkrOSJjqO28j2H2GWGc3viuXocEwI719hBtbKLl+LfFYnPMHDrN4440EA51EQyGkf4D82mpC50cYOnYcb03VFwU2UoYqRKNTH1lORhs9ThbdFlVtVdVR4DWc4hjTJjIaJtgWwFfmp8Dn5eifNpNbVkrOSIje5hZaX36dwiW1RLrP0N3aRve29/DV1TDa0clI71nyVMkpL6fjrXfQaIzo2T7C7ac4e+Ikg0ePEdc4wbYAp5uOESdOtK8fX9ViAm9vg4ICgm0BBgf6Cfb3E+n8nNDJAN2NTURGgkTPDRDpPM2pnbvIK/MT+fw0+Apoev4lvJddSqz3LKdbWgkPDhM9cxbfgkJOvPUPchYVEWwLUFhcTPOrb+KrrSYc6CTUN0DgrbcpXFpHpLOLjs8OE833EGwLkL/4Eo69+ArxWJx4JILH56X5xZfxVi0mNjDAQE8PMjxM4dI6urZ/wIm9+wl19SALvERP99D58R7yF5cTbAtwpj1A19YdFFRXEjrZQeDAQSgsJNzegRb6OP6X1/CUlxE6GWCw/xyDzS2MFvqIjUZS8iZJRFWnPLIdyWQlReQeYJOq/sBc3w9cq6qPjuv3Q5z8Y9TW1l7T3t7+RVs0GiUcDn9xHYvFyM3NTXo9vk1VL8j2ktgej8cviBqb7L7j+89k7Pi+k40diyAba59sDlPNKRqN4kkIhU1sH3tPjf1vJvs7sViM3t5ecnJyUFXq6uqSRtuJyP6pYtynQkqKlZuunbrjlh0X/bfSmUzfyJtWxhGT9eQ5gIaGhgvaPR7PBW9ey9xTXDytuouzg93Iy3ijn6gQhsWSBHXFRt1UZLrRfwosF5ElQCdwL/C9+Z2SJW0ZC7hxORlt9KoaFZFHgW1ALvCCqh6Z52lZ0haFVDr/ZAgZbfQAqvoOTnEAi2Vy7EoPZIHRWywzwn6nz/jf6S2WGaBzkkRDRH4jIk0iclBE/iYiJQltvzDeo8dE5I4E+UV5ls4Ea/QW96DMVcDNduBqVV0FNGOqKRtv0XuBlTi16v4gIrmz5Vk6XezjvcVF6Jwk0VDVdxMudwP3mPO7gddM0Ys2EWnB8SoF41kKYMqy3w0cTcX8XGf0+/fv7xWR9nHicqB3PuYzi2SDDjCxHhcfjTMc3MbOA+XT6OmdqqzVDHgYeN2cV+N8CIyRWLOuY5x8Gq6DXw7XGb2qXjJeJiL7Mt3tMht0gNTqoaqbZute06llJyJPAFFg89iwZNMi+ddsW8vOYkknpqplJyIPAt8ENur/Alwm8yCdM89Su5FnscwyIrIJ+DnwbVUdSWjaAtwrIgXGi3Q5sJcEz1IRycfZ7NuSqvnZld7hy35fSyeyQQfIDj2eBQqA7SbScLeq/khVj4jIGzgbdFHgEVWNAcylZ2lGh9ZaLJaZYx/vLRaXYY3eYnEZrjb6uXR9nC4i8oKI9IjI4QRZqYhsF5Hj5tVv5CIiz5j5HxSRNQljHjT9j5ud5DH5NSJyyIx5RhJT/8yeDjUi8r6INIrIERH5aSbqkbVMJ2dYNh44GyYngKVAPvAZUJ8G87oRWAMcTpD9GnjcnD8OPG3O7wK24vz+ux7YY+SlQKt59Ztzv2nbC1xnxmwF7kyBDlXAGnNehOOKWp9pemTr4eaV/qKTaqYCVf0I6BsnvhsYq+P8Z+A7CfKX1GE3UCIiVcAdwHZV7VPVczi+4JtMW7Gq7lLHcl5KuNds6tClqv8250NAI47nWUbpka242eir+X/Xx+oJ+s43i1W1CxyDAiqMfCIdJpOfSiJPGSLyFWA1sIcM1iObcLPRTyupZpozkQ4zlacEEVkI/BV4TFUHJ+uaRJY2emQbbjb6TEqq2W0eaTGvPUY+kQ6TyS9LIp91RCQPx+A3q+qbRpxxemQjbjb6OXV9vEi2AGM71w8Cf0+QP2B2v9cDA+axeRtwu4j4zQ757cA20zYkIuvNbvcDCfeaNcy9/wg0qupvM1WPrGW+dxLn88DZNW7G2cV/Yr7nY+b0KtAFRHBWtO8DZcA/gePmtdT0FZzkCyeAQ0BDwn0eBlrM8VCCvAE4bMY8i/HKnGUdbsB53D4IHDDHXZmmR7Ye1g3XYnEZbn68t1hciTV6i8VlWKO3WFyGNXqLxWVYo7dYXIY1+gxHRHwi8qHJnT7dMY+KyEOpnJclfbE/2WU4IvII4FHV381gzALgY1VdnbqZWdIVu9KnKSKy1sSWe0Wk0MSlX52k630YbzQRudms+m+ISLOIPCUi94nIXhN7vgxAnWSNJ0VkXZL7WbIcmxgzTVHVT0VkC/ArwAe8rKqHE/sY9+GlqnoyQfw14Cqc8NxW4HlVXWcSWfwYeMz02wdswIlLt7gIa/TpzS9xYgRCwE+StJcD/eNkn6oJXxWRE8BYiaVDwC0J/XqAFbM6W0tGYB/v05tSYCFO9hlvkvZgEnk44TyecB3nwg95rxlvcRnW6NOb54AnccoiPT2+UZ1sMrkikuwDYSquwAlYsbgMa/Rpiog8AERV9RXgKWCtiNyapOu7OFFtM+UbwI6LmKIlQ7E/2WU4IrIa+Jmq3p/KMZbswa70GY6q/gd4fybOOTgbgE+maEqWNMeu9BaLy7ArvcXiMqzRWywuwxq9xeIyrNFbLC7DGr3F4jL+C1hZ86Nj+NpaAAAAAElFTkSuQmCC\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "model.quick_plot('land_surface__elevation', edgecolors='k', vmin=-200, vmax=200, cmap='BrBG_r')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "To get things going, we'll run the model for 5000 years and see what things look like."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 20,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAP0AAAEKCAYAAADZ1VPpAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4wLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvqOYd8AAAIABJREFUeJzsvWeUHNl1oPm9iEjvy3vvPbz36G402pFNcihSNEcjiVqNNGdmpNVIWp1d7ayGq9GuRtKRzoojSpShSA3ZJNsAbdAGtgEUgCoUCijvva/Kykob6SL2R2Y3IU6T6Ka62EOivnPiVMWNlxEvM/Jm3HfvffcJXdfZYostHh6kj7oDW2yxxU+WLaXfYouHjC2l32KLh4wtpd9ii4eMLaXfYouHjC2l32KLh4wtpd9iiw+IEKJYCHFRCDEghOgTQvy7tDxDCPGmEGIk/deTlgshxJ8LIUaFEPeEENs/yv5vKf0WW3xwEsBv6rpeD+wFfk0I0QD8DnBe1/Vq4Hx6H+BxoDq9fQn4yk++y99nS+m32OIDouv6gq7rXen/A8AAUAg8A/xDutk/AB9L//8M8HU9xQ3ALYTI/wl3+12Uj+rCHxVZWVl6WVnZR92NLT4gt2/fXtV1Pftfco48p6THEg9utx7R+wD1PtFXdV3/6nu1FUKUAduAm0CurusLkPphEELkpJsVAjP3vWw2LVv4YO/gw+GhU/qysjI6Ozs/6m5s8QERQkz9S88RS8CJ2gd/5b/bHVd1Xd/5PvpkB74H/Htd1/1CiB/a9D1kH1n++5Z5v8VDhSQevL0fhBAGUgr/TV3Xn0+Ll94x29N/l9PyWaD4vpcXAfMfxvv5cdhS+i0eGoQAgyweuD34PEIAXwMGdF3/k/sOnQG+mP7/i8BL98m/kPbi7wU23hkGfBQ8dOb9Fg837/dJ/gAOAJ8HeoQQ3WnZ/wb8F+A5IcQvAtPAp9LHXgVOA6NAGPiFD6UXPyZbSr/FQ4Pgw1F6Xdev8t7jdIAT79FeB37tX37lD4ctpd/i4UGAtDWg3fwxvRBCFkLcEUK8nN4vF0LcTGctfVsIYUzLTen90fTxsvvO8btp+ZAQ4rH75KfSslEhxO/84LW32OJ+3nnSfxiOvJ9mfhK/e/+OVPLCO/wR8KfprKV14BfT8l8E1nVdrwL+NN2OdKbTzwGNwCngL9M/JDLw/5HKdmoAPpNuu8UWPxRZiAduP+tsqtILIYqAJ4C/Se8L4Djw3XSTH8xaeieb6bvAiXT7Z4Bv6boe1XV9gpQzZHd6G9V1fVzX9RjwrXTbLbZ4T4QARX7w9rPOZo/p/wz4j4AjvZ8J+HRdfycv6p3MJLgva0nX9YQQYiPdvhC4cd8573/ND2Y57XmvTgghvkQq55mSkpL/4XgymeT65TdYHOugs7ODpgo3AL2jS+i6TktNAQAvXujj5N5KbBYjAOdvjnFybzUAIzNe1jdC7G5KhWPPXR1iV2MBGW4bAGcvD/D00UYAVrwBJubW2d2c6svNnllMRonWmrxU20sDPLKvCrPJAMA3znby8UfasKev+53Xu3n2ZDOSJLg7tEhtWSZmkwFVjXPl9gSPHqgBYHEtwNScj72tpQC80T5MRI3x1JEGhICuwUW21eYhBITCMTp6Zzi6uxKAgfEV4vE4LbWp9/7c6/coyfewtyX1/ganNqgrdQFwb2SZRFJjR32q7dtdk+RlWqkqyQLg5UuDnD5cgyRJRKJxOnpnOLyjAoCO3llCkQhHd6U+x7//7gXOd8y91238UHgYzPcHsWlPeiHEk8Cyruu37xe/R1P9Acc+qPx/FOr6V3Vd36nr+s7s7H+eyfnf/+G/8eV//xTSyF9RZbyHmQANOWGa8iI4LTKVuQaa8lWCviU+fiAPgxagKS9CPLCIzajRlK/SlK+ikCA/0/rufpbLSIEzQlNehJnZBRpLzBTa/TTlq/j8QaqKXTTlq0SDyxxsdJLjNtGUF8Eh1jjWlglxP015ERKhRbbX5TIzt0JDboSmfJWDrTmMTi3QkBOmNjdOoT1AQ06I8dkVSvPMNOWlrhsKhijOdbzbpwy7wvEWF+PTi9TnhNlVBnbhpSkvwtziGoWZBuqyQzTlRbCZBRX5qXP5vUt88bEStHgYOZHql8OcpCkvglVfoyrPSK7H9O51qovseOwyTXkR6rJCNFc4SER8qessrFCYZXm3jwVZFtRI5N3P3G42vNct/FAQpBx5D9p+1tnMt3gAeFoIMUnK9D5O6snvFkK8Y2Hcn5n0btZS+rgL8PLDs5k+lCwnh0XgNMXpnUkZHzuqrAzORVjyRijMlNARqLEEflWiscyGP5qy/xYCCo0lFpZ9Uc7fWWdPrZlkLEQkmmBlPUJzuQNvUGNqKUx+lo3H9+QxNBfjWq+P3TU2QLDoDROKydQWGgmHw8QTGt1TCfbU2tmIpK4z45XIcDs40WLmrbtBAGwWEydbbLx0c4OSXBvegMaZW34e3+Ekywbr/hg9EwGaSi2EQkF0XefCHS9762w4bSZONFt4/rqPigI7cz7B3fEALeVWjjQ76J1SmV+NUJQpo+sS/lCMqCZTmWeioiSHFV+Y4fk4yUSSYDjG4HyS3dUmIuEgiaTGhTtrtJUqbASiAFy46+Vwo4PlgE4ioeFxmHGZ4ngDMW70+2guVmiuyqVjVEXXdWaXQx/0Fr5/xNaYHjZR6XVd/11d14t0XS8j5Yi7oOv6zwMXgU+mm/1g1tI72UyfTLfX0/KfS3v3y0lNT7wFdADV6WiAMX2NMz9OX480u8hz6rx4c4Msl4mVgEz7cJSWUjM6Eq90+jnWnDLTw5EEG8EY2U6F3fVZDM2qIBvIcRk50eahdyrK1YEw2yptJHWF7qkk2yrMGBUZf1QQTigUZhpJaBrXBlUONVgBON7q4uWbK1TnmxBCEAgGSSY1XDaFpJZSluKMJLfHVXRdwmkzcrzZyrVBlQvdS+yuseOwyOyocTGxEmfGK1GZZ6QyV2Z2RSUhTJTkWFHjOnarkY/vdfHSzQ18wThzPkFVvpEMp5m1kMytsSjNJSaSCN68G+ZIuo/BYIiDDU7m1yLMLnl5rSvIY9vsAOyrtTI4EyGOiUyngdIsmFpS0SQbDpsBWYvw3atL7K4ycrApk6H5BL6okYJMIzarmblVldH5CPFk8se5he+LLe99io/CmPlt4DeEEKOkxuxfS8u/BmSm5b9Bei6yrut9wHNAP3AO+DVd15Npv8CvA6+Tig48l277Y1GSa+HpXQ7O3fYzPLVChjOlfH3jq2yrtGNQUh+VnozwepeP7ZVmJElw8fYMB+ssAFjMBvxRGafdiiQJbvYtsr3CSM9EkOuDYS60DyFJguuDEc5d7iPDrtA+pHJtMMrdGbgztEIikSSe0NhVbeHv35ihqVhGS6aekjaj4O7APO33Jnm5Y4Ob4xoWY2osP7KYpH86jMVsoL1/lW3lqfF/U7mTl64t0FZmwGyUSSRTIyBJgny3zrkr/STiCdoHwwzNhlleXSfLaUQIwZ2BRfbV25HSmtBWbmJoLkw0FqdvZBld12kfVhmeDZHltvB27xr1hSkLpbXSxeiyht2sk9R0slwW5lZC3JlQWVyP0T2yTkNRyuBL6oJjzRZeuDpPMrl581AEW448+Akl5+i6fgm4lP5/nJTn/QfbqHw/bfEHj30Z+PJ7yF8lleL4Y9N1p5cLs/NUFXvQEeRkWLlxdxKTQSGelJhd8jG/nsviugroWM0muodnudDrZnRyEVkSvHh9CZfLhdWkcPX2CGUFbt7Us5hb9NE9bGJfQwZVVpn+4kyq8xQyHDIdOS5ayy1kOFJj2KnFEPlZDpzmJK92eLHaHEwvBuiayOP6nQkkPZ/yPCvHt2dxtn2ZlnIbJVkKw9Mb1BQUU5FvYn4lzF++NMGqL0j/fD4D8zEQsOwNMLSgMbUc5mbfCusRAzazoK7UxckDDeRnKuyqsjCzFKRndIlgKMLCipW5JS9X7lmw28wIIQirceaXvPzrx8tBsSGhcbDOwth8gDPtq8wtrDGylM3kShxJaJy92M/hnRWcvRnhYL2N7Q0l7K1R6BjyMjW3QveEg+k1jYGxZfxBDwZJQn0/c19/XB6SJ/mDeAjcFj+a7duayHTZqMo3sL/WTFlWkk89Wk9etguRDLK3KY98t2B/nZn9dRYCqsa2hhIO1xsoL87ktz9Tj8ft5NQ2G4cbTORleWipcHOgRuJTJ6sIJ01YzQZe7/LzhUeLGF3SuD0e49c/XsHb/d8fv96ZjPO/PF3GlFfhmX1Z6EmV8kIP+2pkTh+sJCnM1Bbb6J1S+aUnyhiYCTO3lqB/JkpxtpmJhRAdE0me3F+A22ljT6XCiRYLxCP8/KOVRNQ4pVkSCDjZauFQgxW3XSHXY2DFrwHQMZ7gtz7TREtNDgajkf/8y20YjQYONdh5cpcLRZYpyc/AblGwmSQMUpwVf5zKAgeluSYOtBRQkQ2H6g00FEJWhgOEREOZkyy3mVg8ZbpPrcr8wS+1oSgyB+oshCJxdldbCERiSJs4phZsjelhS+kBOL3LxeWeAJFoko6xBDsqTMhaBH9U4dSuHIZmQqz64/RP+qkqtCFpMZ6/tsrJFhuKImEQcZbW47x6y8unj+Yyt67TNeyjIsfIpw54eOmGl0g0QVLT8flDeP0qsizRVmaga1xlZT1CcZYRSZLwBWPcGQ1QnGPn00fz+cqZSdrKjeS7NMYXY8hGGxaTwqkdHu5NhvGGEpy9tUEgpvDMbgfjcwG++EgRtydiJBIaumyktthOVa7Ety8v8snD+ZzrCgBwvmuNllIT2bYk/3h+gUNNTjKcRpZ9CQqzrRgUmU8cyOSt7g3OXF9if72NA/U23upaJdOmcaw1g9vjcQCWAgqP78llfCllnl/oidBYkcUnDmSiqjFevR1kIxDkpZt+Tu9yYzTIlGbBX786za88Vc7V/iBqLE4soW3qvd7y3m8pPYtLK3z3ygJCj/Plf+xDVWNc6I0xtRxhbGqZCz0qOhJff22cs+0LrAZ0lv1xhiaWuTqU4NydEOvBBN94a5LcDDNuu4Fij0bvVBi7VebMzXXyMq1E1RgX7yzTO7LA7KKP17vDdI2G6Btf461uP7WFBvomg0QiUV69MceSX+f6cIL1QJgbIwmQFG6P+LEYBVd7VnjpxjpGs5neoTnMZhP+cJKVjSjhpIkMp4l1v8rLt7zsrzXROxlm2iszu7DO611+EtEQHaMqKDbCUZ3BOZW5JT+3x6Jc7Ity4+40awGNt+6pXOqLYpAFvWMr9M4kGVmCS7fnKM8zAeA0JXj5xhItZan9UCTM89fWOFBnZnxmlReurTDjBUlWaL8zxdJagOtDUV67HWBiMcHCygbtw3HMJoVVb4Cktrm1JbYceVsTbsjNyWL7oTzGF8JEYznsqbNTnmvi5aiLnEwXxxoNgAGv347HbuZIgxmf38y/OlZGUkBLo41EwsLv//08Q1NeljaSmI0WhieHuTaQwemdqafaFYOR/VUyJquLeBIea7OS1MxcvLPK9Z4lrlg0dtS4sJoV9rWVcazRxMW763zpqTomVjQqchUudiyzuGLg08dLyHGbePOOl231BZxqswA633hrhhVfnDdNJkKRONPza+jo7K+zMb+m8pufbaF/NkFVnsQbncssrgYwy/l4XHYyXDYe324nrMaZmMmgpsBIZZ4RTdN44brOzuYKTrZY0DSNK7dNvHRtiZKCDJLCys3+Kcw2D/0zYeZXw6ytB7AYkhTkZeJyWDnekop8aFoDETXOI60pR+crt9Zorini0TYrqz6VV6/IJLVN9N4/JEr9IB76J70Qgkg0ydC8xmeP5zG+AreGNmgpM+I2RVlej/LyLR+P78xCkk1omobLZqCh1MbiWohlX5yXbq7zO5+tp6LQzekdDuKxMMd2FTM6s87AXMoxFY1rvNLp40CdCT0RJhCKI0sSwbiR/a2F7G3IIjfDis1uJ6wm0DSNuGagLM8CWgw1LlFdVkBhXiaZTgOJhAbCwK5qO1PLKq90+tnbUkRdZT6PtlmxWEw01Rbz8f3ZrGykzPUctwFfQKUw04jHYaWpppBMu0RDkRGbouINxHizy8fnThRydzw13Dl7c51H2uzEEyllPNfp498+W43BZGFvjZl8Z5QnD5QhC41dFTK1JRnUV+Rhtxo41pqB05xkeD4GgNVs4ESLlbf7w8QSSawWM7JImfNvD0QwGQwkk5s5pn9wAY33U0Tjp52HXukBXukM8Nj2VLw5EAixGpQpyTKyq9bFN8/P0VJhJ9OhYJbDvHhtgZaSlIFUnS/zJ9/qIcsucFiNhOMKb/f6qMy38OSeHHIynThNcc50BJieXSXTbcVsVHhsRyZ3JuO80eXlYIONk9sy6JqIcXd0g6pcQYY1wT+dn2dfbcpkPtbq4cZQCLvdzMlWO7eGVV7rXOdArYmAqvPVM8Psq7dTW2Akpobpm/TTWGKlMhvujgeY9wkaS9IhvGKFNzpXqCyyk+fU6ZmKUJlv4khrFgOzcWx2O0aDzNO7PfzF86PUFllxWBUS8Sgr6yoZLjMOq8LJNhvXBiP0z2nsrXcTU/382XeHMBoEFpPE9XtLmA06O2ucDE4HWQ/EMCtJPE4zaHG++Wbq/RmlRCp8V2IlEksgNrl03JZ5v2Xe8/r5KzjQudgXI5FMMjbnI5lIokbcKJLG/MoGHcMbDC/YMRrs3BkawmJzYjUmyXXK1FXks+KLcObWBp09U+Rl2TAqmYQjMfKcSS73+MnyuBkcX8RqNXEJBaNI0DuyTGNVNlnOVMguHo0wEhA0GXUWfUnG5rx0jGcjiRigM7uwjhA6BrmQ9u4ZhBBYrWZi4ShlhRm09/ux2ewYpCQ3BjYoyTYSTii0d0/xyMEGLvVFQUAsrvD23XkO7LASDkYZnlzi9W4nTrNO99AKdSU2LvVBIBQlGIlxo28Vb8hDIh7lcp/EJw54AAirCboH5okmNC5YTQQiSerKc9hfa2ZwOs5CppOuIS8RzYLdZuEPv36Pxqoc/BGB0WhiYn6Ka4OZmE0S1/t9FGTHUNXYpo7pt8z7FA+90h8/vI+ut1/kRFMqIUXT8ojHkzy928HcSpjyfDsLG4KTzSZGZoOUF2bQWmok36OgaRpLfogmBI+22YjHi7BbZLZVWAlGYkwtakTUJKd32HBYG4glBUcbTCSTCjd6E3T1L7Dmc6EYFGYWg2wEQmyvrCDfncTWkEtRhqCmwIgaS6BGs7BZjByqNzE576Qs187BBjNnO+I8ucfJalCmrcLCcxcTzC35+eShGlx2E/5gjAK3oL7IxIovwtWBCHubCjlYa2JkJoov6OGRFgvRWJIzb69TXWjmeL2RWEIhw16GmpBoLJQ4f3MdsynAtUELEglcFh2X00ZZgZvDjRZe6XASj4WJxZMMLySpL7FQkmujIMNILKExvZBPeaGbw41WLnStsrchm6ZihTuDcUJhldO7Snij3bjpNWKlLa3fMu8NBgOHG6xc7ImgxhJ4rILWUpn+mSgdo2EaSm3YDAlmVuMMzsMvPFbEtYEA0ViSmwM+avMlYmqIy90r7K61U5ol0zOTwOMwM76qUJZr5cXrK+yoMCBpEbz+OGdu+vjl0yWUFXk43uoiGtP42IE8nj1czHpY4I0YOLU7l9G5IN5Agpc7NjjRYiUS03it08upHR5WNqJomo7TZqAwy8L0SmrcbHNk8EtPVtAxGsMXjNJc4aR/OsTgTIC7k0k+ttfD6d2ZdI7FmFxN8sTuLHqmIiQ1jWcO1RCIGYhEk5y/46Wl1Ig/EMJiUtjeWMrRtlwUSWdfrZXxpThP7MkkEtMZmQ1QmatwuMlB33QUl8PC3noPQ/Op8fqZmxt85kg2sh5jaiVOVDfz+J5cemcShDUjT+wtYGAuQSyWQNM3MWQnUkr/oO19nUqIvxVCLAsheu+T/Z9CiDkhRHd6O33fsfcsBPNR8NArPUCWy0i+S+crZ6dpKzdSlmdjYjmBzepAlgQHGl18+/wkGbYEuq7zsd0u3ugOsxo2kOsxUZ6tcLl7gWA4RmGWkVA4yqW7XmoKLVQVWLg3vMzrnSuoapQ/e64fVY1wd1rDbrXwh9/oxeOyMLassxZSuNEzT+/wLM+/vUg0nuT//ade1IjK7dEI17tG0BJxMuwKRxotfPP8HOXZqS9pQwFcvuelyCORn2GmLFPnr85MMDm/ga7p/NMb4zisAjWWRJIk1v0RjEYzeZlWlv2Ct+762VFl4Zk9Ht64G0IoNuwWheMtDr7x1jxNJQotFXZWV1f5w2/24bLoeGwykVCAvpk4tUUmPA4z3WN+ij2px7UvEObVTh9HmhwYDTI7qmy8cn0GWU+tIxEIhjAZLdSX2vH6woQiMdA315GnKPIDt/fJ35Mq6vKD/Kmu623p7VX44YVgPoS39GPx0Jv3K6tepmZU1GiMZW+Aiz0hzBYrS2t+NvwhZLmEYDhCLJlkfjnAixsJzBYL68EwS8s+XPYyPCaJ3EwHDpNGe+8a4bjMzd45djXmEw6rlBZl8PiuHITQmVyKU1eaQVu5kbCaoGfYwZ5KBbsldSs2/E6EnMGp7akSBKEotJbbaS6zMDSbiz8Y46X2VUwWC71jK+RmZzK0oKLpMhfaR3nkQD1XBhMokoGV9RC/eLqU7tEAe5vyyXJKXOj2IhlsWIwKQ5OLzJVJhEJhZNnI9b5V1oKw5o8RDIQwGkrQdJ2RaS81Zdksr0dorcrg7ngQPRnnxRtr+AJJfP4VLtot6MDQxDJ5WQ7G12JshGLMLayjU0g8FkMWCRKJJGNz60TiAiEb6BuZxWQsRUgmYvEE+iba96kx/Yfzo6Lr+pX7S7o9gHcLwQAT6fklu4H2D6UzH5CHXumzszIotJn53vUoX/6lNs7fC3Oi2Uy7bGVsXuFEswk1JpPtKkVDcLDOzMxymO6EBbfdwuF6E2dvBijOMmG1GHlkp43r/T6qSjL4+P5szt0J80iLmVdvh3hqt4PW2hzUaJT+WYmVNR+fP1nIjZEYJ1sUxhfCFGTbmF5JPQlHZgPsqnEwsayRYQuzvcrJ6HyEZ/Y4UaNJjKISgwGONJoBiETKMSlwuCHl9fcFqpha1Yhi4Yk9Dr57zcuz+7KRJMGtIR8mkwF/BMZm15EliS88WoLHYeT5615KCzwcbzJyvnudn3+0HFWDHRU2hmYCPHWgkFBM4uOtVl666SfTY+NYYyqmL+lVrG5E+cQ2Ky8GTBQ359NQaiHf4ySW0GgfiiJJMofqjZy96aWmPJcTzSaeb19HkiQ2Nx8PpM1Puft1IcQXgE5Si1yu86MLwfzE2TLvgVc7fRxvcSDLEgpRVjbirIYMHGh00DMVTc2qqzASjurcHQ8ysSp4apeTIo/GzEoUo9nGY7uy6ZtJxdcDMQMZdoWbg+s0FMnIskR1Plzt8+MwJdhb52TNF6Z/OkyW20wiqrIRitM3k6C1zIjHEmfFF2VwHmoLzZhFhIt3/TSVmtlXZ+HOeJQ3uv0cbrKha1EW1uNMLYWoKzajEGdqOUb/5AYNxUYCURmbJRUheLTNzuW+MOt+laAqU5ydqgfwscPl7GvKZSMiuN6/wa5qG+GwmkqJlUw0ljmYWQoRiycZnNdoLrMTispc699gZ5WFmjwYnovx1h0f28pTU34v9YZxOyycaHNztT9IPKFxrtPH7ioD0bhORE3gtJsxyzHeur3K9kp7Ojd/cx1t73NMnyWE6Lxv+9L7PP1XgEqgjdQ6df81Ld9a1up/Jnp6B8l0GslIh86Ob8ukcyyKw2KgPN/O6HwEo8nCzQEvl26Ncrl7mfrC1Me2vdrNyGJqyqskSQRVnVc7NzhYa6Iky8D4YpyiTCNzqxE2IhIvXRllejXJWz1RAlGJqXkv57ojmK02/ss3+9hdnYql72/w0DMVI8ttZGEtzHoY1jaCXB2IMbeWZGjGj91qxmiQOdrs5sZgmDvjUWoLLexvdNExHKR/Nk5ZrpmJWS/5Tg1N09F1nbU1H3/yXCqeLilmLnTOMjSrMr8W5bkLEwxM+cl3yxR74B/fmudQfcpqOL3LzaX+CBmOVB/9gSChmJKqtlPiYGQxjq6YMRsEE8saN3rnGJ9d4+qgSlORwrmuADarGYtJIRJVOdcVYF+NiQyXje6xdea9cSLROJq2ec96IR6s8GmlX32n0lJ6e8/FK38QXdeX0tO+NeCv+f5s0v+plrV66M17WZG50jmPThEaMglNcGdghlyPHSHlsuQNEo2q/PyJErwhCaPRwOB0gBsRFZvVwhvtw+xryuPmUIK+4QUQApfTxuRcnPmlDV5qF5TlGKjKs3JoWxmlWTIt5Saebw9zem8R1cUCl1Wm/a6V2yMBkJNYLQpnrgxzfE8NVqPCM3tcvNZl4GCdQiiic75jg7llPwZDCYkkxOIJxqaXycl0kNQFDoeJzrsTvG6zMDSxhCw0+iZlXFZBJJagrTafA3UWztxY5ej2QkpyTVTk2nhJizA4HebaQBCD0cb88hyX+zNBCIQQXLwxRkttIa+qSe4OL2IxG7GaS0nqMDa1TDSeIBbxcKDBzu7GYpAkDjVYGZnx0TU4R16mA1lRGBxbQtMFQhIcabKh6UW4rDoWk4wa3ewn/eY954QQ+fctV/Vx4B3P/hngn4QQfwIU8P1CMB8JD73SN9RV0+Ya5NpglCd3uxFAOFKELEscaTASDGWQiKvYzBIWs5kcZxK3w8Wh3JRlsOItJj/bwu5qC7NrWTisRg7VGdkIOnA7zBxvdWG3KHzvmpeP7fHw9mCM6/1+tlfaKcmSuT6ksurz88tPljKzBtsrrQxMB6kry2J5bQOPPYNYXMMkIviCBq4PRPjCqXJ6p4LU5gsMksZFv8SBljyyHFCTr/B8e4Dq0ixOtlhQpFoUWXC0yUJETXB9MIIajRCKJDBb7BxtMfNa5wZOiw3Z5OQ/fqaI71z18kibYLzQTVGmQn2RgRfa1/kPn26gayLGqW02dK2UIo8GMrSWGdkIZGFSkrRV2LnSH+T0TjevdXqJJ0yML8OXnq7j9oifnRUG1tc96EJhd60TkwG0ZITJFRlNF2ibnJyjKB+O0gsh/jtwlNRQYBb4feCoEKKNlOk+CfwKpAoPhBqsAAAgAElEQVTBCCHeKQSTIF0I5kPpyI/BZhbGNAshbgkh7goh+oQQ/ykt/3shxMR9scy2tFwIIf48Hcu8J4TYft+5vpheHGNECPHF++Q7hBA96df8ufgRawX/KLLdZk5tt/G9a16+8/YKx5otxKIR2vvWqC9SOL3TTftwDINRYXuVk+6xEJqm81b3OsfbHMyvRbnWt8GeWjuyHuPu2AZlOQae3O3hSl+Yt+6sc7A+NclkyRtGEwbKcgzpIYEgw2Ul221lNQDr/igzXp1ffaaCTI+DXVVGznVtkJTM/LezE2Q6dELhKLkuA197eZS/PDNBvjNBSZbMxHyQl9qX2Fbp4GSbgyv31sl2gC+Qqj/32m0fhxrMPLrNw6XeAFn2lII9vtPFpXsBjAYFTYeDdRb+9LlBLEaZi11z/PVrsxxrcZDjNuAyx5lbi2OxGGmtdBIKq7xwdYmyLIHDGOerZ4ZYXNlgfDHCtnITX3t1mqpCGwUZBp7Y5eHCvRAWm4NPHMxiaDbEW11r9E76eXSbnVhsc/VAkBqGPWh7P+i6/hld1/N1XTeky8J9Tdf1z+u63qzreouu60/fv0ilrutf1nW9Utf1Wl3XX9u0N/k+2MwnfRQ4rut6ML2s71UhxDtv9rd0Xf/uD7R/nJTZU02qlPVXgD1CiAxSv6I7Sf2C3hZCnEl7Rb9CqrT1DVIVdE4BH+gD7RsY4fayH5fdTHGOhY6+BS722rBYbbx+c5iPHS7DZ9JIxKMgGQATJ1qsXOwJIoSRmcUAgVCU/lEfh3dVYbbZee7iIAe3VzDrTTC5sE44rCIpJQwvxrjdOwUNhZxTdRRF5uKtYXY1FvP81RBT82ssrbr5+eOpUtiHGizcnUxQV2RieEEjFFaRSaAlBXluEwW5GbRU2CnKkPBHNDIdgrPXZjFZnFiMEq9en+S3PlOP22rg3K1lqgudAIzMq1zunOTUwTou9MXQNB0NnbsDM0SCbrLdEqWFGdSX2LEYdO6N+7k+GMVuSVCWZebORBS3TeJit5+IZqGjf5GKgkqqCx3MbxgoyTHhNEPvhJ+JOS/Ty5nMeZPEkzqhiMrw5DqKXIqiWOgcnCYv08Hl/jhJTUOITfRvia2MPNhEpU8XtQymdw3p7Ufd0WeAr6dfd0MI4U6v8X0UeFPXdS+AEOJN4JQQ4hLg1HW9PS3/OqmFMz6Q0tdWl9M5f4fqfIkCjxE1XsDxJhMv3/LRUJVPSbZC30SAqfkwi94QNksVOnC+Y4JttXk0FLtZCYAkZbCnUsagSLx928yJ5pSj7cWIishycqwx5QCLJ+tA1znWZCGsJpD0SpK6xCNtdi7fiXOlZ5UXr0soBiMGxcDt3in2t+bx1C43Xl8OGW4HFXkm7o75OdLiZmheY3ulhRwPnL3ppbk6NdX2zliAnEw7s6txQnEjl7vnOLjNzNj8Bruq7BzeXUMkluCJHan6fi+0R9jbVsHBOgPfeXuNL5zIpWtcBaOTthoT++tNOK0Kd0d9dPUtkO2x8KkjBWS5jIxNOynIMOANxGkutzG3DoU5Zm6MxvhPv9jGxZ4Qp5pSw6FXOxUyagp5pNXCG10+fvXj9dwaDnGs0cgfKxIR9YPcvQ+OJB563/Wmr3Ajp5fyXSaluDfTh76cNuH/VAhhSsveXewizTuxzB8ln30P+Xv140vvhF9WVlb+2TFFUfjEfg/9U0HuTcWJxZO83eunudyOx6IDEgdbssnN8fDskWIy7RAIhPkPn2pASAYynUZQrDy7L5PrQ6lv7O7GPO5OxdA0DafDQkuJTO90lLCawGHS2AhEUiGsO4FU2SpzgumVOOGYxK6GPA41Z/D0Hg/JZJxferqKjbDOeiBBTYmbkaWUd3vOJ1Oea8JhiLC4HkONJXDYzBjlBPfG/SQw869PlaDIEqoa5nOPVeF2GPjY/jwURaYkU6ImT6JzLMrKeoSibBMa8Px1L0/sdGJQZKJxCbdV4tHtbjrHUmm+ORlmTuwpIyfThcum0Dnk5eMH8xhZ1BheEtQVmfEHQ0wvhajMs2I2yuQ5dUYX4mwEY7jsJnQdrvdvUJGfMvt3VBi4PZb6TDY1OYf37b3/mWZTlT4dvmgjFaLYLYRoAn4XqAN2ARmkquPCR7TYxTscb3Gy5vXy2tt9SJJEabbC0dYM+mYTvNbp41CDlap8K99+c5iFtRBjC1Gq8xX++tUpitw6FrNCIBilZ2yd0iwZbxAu3vPRVqpQnm9jdF7lUreXhiIjJ1vtnLu9Tkm2GYMisavWSedoEDUu8/iubG6NqLzR5aO13EKuS+Gp3W5eal8mHImg6ClfQ01Bykg70JRBz0ySc7f97K81kUwmOHt9jnyXTqbLzPRaErPZTFOZE5NIMLIQ5/pAiMYSM9WFNiJqlH+6tIjLrPHG1QFKMsCWzg5s752nrSzlewiEVCLRBO1DKvtrzZze4eDtAZX5DYmiLAt9E+s0Fqd+v1tLjbxxe43W8tT+tmoXPVMhzt0JUJkNHT0TdA2tseaPcXMwyJw3yQvnh0gktR9yWz8kPsTc+59mflLVcH1pc/yUrut/nBZHhRB/B/yv6f0ftajF0R+QX0rLi96j/QfC611ncjqVIhqNg8dp4e7IKoGYjN2kMzztI5lI0Gm0MLu4wq6mYgozjTSXmlhci+D1h7k1uMb8RhbJZILvvb3C732ujpF5L6Gk4d1qt/tqTXzrfKqSDYqVK3emObanllc6g8SiKgYR5d5EAEnWGZz0IQuIJXLpHPajJeIEgmHGYjEqSvI4d3OEUweqWPWHQde43TeLEDIIiXDCSFt9CWt+lc7REDfuTvHYwUauDqjIQuJS1xyhSIKztwQOuxWTYsRhNaMlE+Rm2vFHNNqHIsQ0maga5fakhiyiZDpN/P7f9vDs0XLGFsJYDIJwKMDcisqlPjOTc2t0WBWWNxxE1DjDk8tc63MhKxK6Dol4jJHJVUqz86gqyaG62Mmu6lQ9/bAaZ3Aqh1t906ixzfbePwQ1rh/Apim9ECIbiKcV3gKcBP7onVhm2tP+Mf55LPPXhRDfIuXI20i3ex34v4UQnnS7R4Hf1XXdK4QICCH2AjeBLwB/8UH7mZHhodliZGwuTiLpYv82J9F4klNtFhIJjdeueXn2aCktFSZei7p4YrebN++GkSSJa0NRfvvTNVzuj/NIi5l4wsjtwSXO3lwj02Pj8tVhsj31CHR0LcGaP8Qvt5WwHoyR665h1Q+P73YBLjaCUW4NrvH4Dg+SbCIeT/DYNhuK7ECNJWgfcmA2yZCMUZzvYWel6d1a/BNLWZTnWchzCYIxGxvBMDkZdtqqjKhxnRwnNJWa0TSN2QWF2SU/e5oK2FubKm0dTUgMLgT5N8+U8+KNdQ43m+ifCtJanUFRhqA8x0gwLLjV56C2yIzPH2PZG2NuJYLbbiLfmeALp2oYnlepLTRxtSfM732hmUu9YZ7aZUMIwUu+OM3VeeyoziCUiDPvTa2Ao2kaf/PqLDVlmTitJoKh2I/3hXtfPBxP8gexmeZ9PnBRCHGP1Go0b+q6/jLwTSFED9ADZAH/Od3+VWCc1Kq0fw38G4C0A+8P0ufoAP6vd5x6wK+SWhF3FBjjAzrx3mF6KcLMusTBejMGg0JNnmB4PsbUUpjPn65jek3nxfZ1Hk9PggkGQzx/fZ3HtjsxGmRi0RDBSILX72zwG5+uxWA0U+JK8IkjZaxuxNhbayUQjvP5RysYXkzQOR5nR6WVYk+SvpnUl/xyb4BHduSx6o/jtBk4td3B1YGUYpy77edAnZloHIJRmc8ey6NrPHXszS4fJ7e5CcUU7k1rNJWaONiUwd3JGPFEkvJcC9OrqZJdi16VwvxMHtlVjNua5IV2H2OLMaKxBG5HqvrtU7vdvHUvzKxP5pn9eXRPhNF1nfP3Avyro/nMrEJ5oRPZYGJfSwHF+ZkML0FtkYmndrs4372BZDDjspvYVWXiSr9KWE2Q4zLyaJuTjtEoJoOguVhmYEblz58fZ1ttFqe2O0loGj9m1PV9kVrL7sMJ2f00s5ne+3vAtveQH/8h7XXg137Isb8F/vY95J1A07+knyurXlYWkjzSliqXJUuCuhInL3f40XSoLdSZW/QSicQ5Z5SQEIzOrKPI0OWyYZTDNJVY+M6VJfY0ZOO2GajLj/LmnVU+e7KEqoTOmVsbmI0Wqout/N3r8zRXuBBC0Fju4JUOH2bZTLbbwu4qF3/1ygwfO5CH1aygJ4MMzSTJz7QihODCzTF++7O1WM0KyxsRJhZCZLotZDsVuvrHOdSa/67SOIxxzlxf52hrDsPzYebXYrQPR3l2nxshLLzeHeLZ/R6u967y6uVhTu6r4/VugSQEQxMrmIyCsQKJvdVGuiZi2G02irLN3JsJk+tWWQtJHGs28sINHxV56YKZPeuoUY3xmWUs5gqMiszs4hrX7vj53MlCZMXEwqqfomwziiLzF9/roarIQ75bwutXSSZTqcKbxlbIDtjKyCMYChFLwKW+GFZjgtkFP+2Si/HpZUJqnNq8IkoLMgHBU7tSPwxCVhACjjYa0TSF811LjM+skZPpYm5dQ5aNDE+tc3UwD7PJwKo3yLLXTzCYwdjMClE1zNyqB6PRiNli4a9e6Of0kQbah+PMr/iZXM1jbDmKwWTl717r5/DOGl65tYYQcHM4gpDNrPmC/F3PKod2VnN1UEUW0Du+xvJGEqvVitlg4WbvGJouIUkyz18Yo7U2jxfbE8iSTP/YMms+D9WFDg5sr6Isz8K2ilT4LhrLwWxUiMdj3JpKcrNnhuaqLG4OO5lbWKazN8Knj5cysxyio2cKRa5gI6zRVOZgPRKhpjSLI/UKsiQwCzvzq0GW/bAcVBmeWmF+2cDR1gyqS7J5bHcuQotxvmuVaGxzJ9wIxM9EyE4IUURqfv4hUmm9EVLD5FeA19K5/z+Uh17py0uLKcwZJKImeOlWBLNRZkelGW8wB0mSqS2xsRBQyXPEGZ6P4rFo5LkE/mCE1Q0FgwyqbuNQm8K+ehMOm4HBmSDPHKvFbJTYXWMiEHSQ4TLzxC4PbpcTIXSOpafDnr3l499+spauCZWndjm5N+KmMEOhNMfIC9e9HN9eRHOFkdHZKL/+iVpuj8V4vM1Mt8WGIkF1nkxZjgHvRjaK0cTpbSnnWN9kgB0NBTRWZlJXYEDTBIrBwCOtNiwmiaQm8DhNOE1Jdtc66ZmK0FZu5saAj7YKG7cHN6isd1NTBP5wHi0VNhpLrSysubCYrahqDFXVcNmt7KiyUOBRuNbnZ3e1DaMUp3dKRREJdMnEb36qijfvqRxuMBOOlSJJMnPrIT5/spBrwzEO1lqw2CCpbW7Ijg8xDfejIu38LgReBv6IVDjcDNSQSk77PSHE7+i6fuWHneOhV3qA8YUQA3Manzzg4fqIxtlbGzy5y82NwQ0Gp5N4rAp1JQ5eux1EVVWe3uNGkiy8fidISE3wsT0u4kkb3RNR6gt1ptcEj2138tINH4mEGY9DYVeVje9dXaGt0s7gbBRdN3H5np/WCjtFWQqyrPLtyyscacukcyRA97jgQKODHJeB8/fCqFEjOW4TbkuE6ZUY06uCzz9SxIVuH7GkQFYU3KYoi+sKitBZ9Ct8+mgBr9zyMjqtcqw1A49d5jtvr7LqC/HFx8o4fzfARkjwzB4HJiXJvUkVn2pgX6YR9zYXdyaiLHpVnj2Qxe3xOEOzIaoK7TSVGHnxho+DDU4Ob7NweySEVG0hnJApzFQAhVc6FinJs3O0yYgkCUxSjOcu+/ncyXzO96gYjGYcNgMJdZVvXIjxy6eL+X++pm9ynP4nMp9+s/mvuq73voe8F3g+vYJzyY86wUOv9N09A4z3LPHE3jz8oRi9w8uc2JlHNJ6kscTCX7wwys8dK6RzMMTkzDohVeO6x0o8mRpjt9YW8lrHKsdaPQRjMq93h/nkgQwAmksUvvrKJJ8+WsjdiShdA3MICslz6Tx/bZGCLAc9I2v0TFmxmk20353CoJQyOe8jGEliNlcQCq3j80coL8oCYF+DmxfbfbgcqRj48TY3b95eY2rOx6OnS7jQpxIKxznZaqNrNIjDZubN9ilcLg8GEaWuxMp3Rpd4q9tHSE0yPb+KSJeuuHZnkoaKLF5uD5DrVrg3HmF3Yy52S2o67HBA46ndRhJJjR0VJr56dhSP00J5cS5//K1+Ht1fy/XhODJJhiaXMEgxXrwWQEOw6lOJxpJcG4pz8dYwexqLudovWF6P4PPHuNiTckyKTY3T//R773+Iwt9/PEbKsf1DeeiVvq25nmNFk8wuB3npuo+hiRXqS2yoqol4Ikk0GkcgUZBpIC/DisFk4WCdiYiawGKoY2Y5zNEWO70zMd68OsqOpiIu9sXQdY14ApbWAtwY9LGz2sFTR6pR4xL5WTKv3Ojj6YMWjm/PxGJSuDHgp7k6myd3Z3K+10ZphkZSkmluyeaNjgUu3hrDZKhGR2IjGGJ6fgWjUoREElnSWVwJ0D4Y4HLHFJXFmdwaiLOnwcO5rgBPHyzF44DKfAv3xnw8sjOP/CwrE/MBzCWZ7K1LWRQr3ij7Gj0UZymsB2JcvLPKS5fH8EcquXZnnJJ8Ny936CTjUVxWnba6Ipw2AwfqLEhUYZAFB+pSw5ZYopZITOPRttRw42xnEJfdwpEGI2vrxRRkmdhdbWIjnE1NSYLKAoVMl5n5lcCm3m8h/2zE6YUQT5KKapWS0mNByh/ufNBrf+ptnQ8Dq1lhfkNiT2MBn3qshUDMQGuFnakVnd/7XANeVSE/04rZ7iKe0EhqOm/e9bOj0sSpHU6uDUbZXW3l+L5qNB0O1xs42WIhGIrRWp3LI9uzuDoQYX+tFZMUw2GVeeZIPWshWPanasiH4gqFWRYWvGHy3TKNZQ68G2Fm1xKEEwq/8HglkoADdSacViOVZak58fvq7PgiMv/7LzQjJCMHtpXjdtg5sSOHN7uDnNrhYlddBoPzqYUmJ1bhSGs202s6usHOJw/ncaXHTzAcp67MxfhyEotJYcUX55PHK/iVp8tZXQ/RUp1DaZ6TpjI7R1o8BBNWTu90EY7C+W4vu6pTK/Esb8Ton/RRlAGyFsUbiHO+28e+WiuarvNm1xpHmmysBlLVczLsMgebPHRPREkmN7dYVqqIhvzA7aeEPwO+CGTquu7Udd3xfhQetpQegFc7NijLtZDv1nCYEjQVy/zVK9McaLRhtxpYD2oseSPkuwRHmqzcnVCx21IrwThtRgo9gp6pGGaTzDN7nJy95eO1jnWOtTo4vs3D270+irPNyJLgcJOLjpEIipzk8R1O3uiY5c++O8KRRjOyENwYUmlNLwZ5tNnJ7RE/imKkvMCGrMcZmoths9uxK1FWNmKc61znSLMdoyKz5o+CEJxstfIHX++jrcKM05oy5oozdMYXYzhsKQ+91+tleGKOM9cW0OIR/o+/6SbbnsCIypo/xqxPoiLPRK7HQnGmzvXuKZK6wlu3F/njbw9gVVTUaJKoGiKmGclwGDne5uHmkMrQXJKyXBMntnu4OhBBNpjIdhlIJDU0YSTDYcBhUPnu24tsq0hNRMr8/9l77yhLzrvO+1Pp3qqbQ+ec40z3hJ6enKVRtiRjy9jAAsawrM0h7L7vLi+757DA8sK+LHhZzLIEAzZghCxZ0iiPRpqcZ3pS55xz35xD1ftHtWzZa1mSrTbI2u85dW7fp+qpqr73PvX8nl/4fu05UtmNLzEXJOldtw8JZoBe4/uIcX7kzfvegWFiqSwLwSyvXJyntrKAaV1mbGqVnhIfHnsOh5zkpatpfuZYGaIo8PdvTNNZZ+PaQJRI0iCe1OmfDmO3ShhGJbOLYbKZDBZVQ5EETlyeZH9XAyduJ5EkiYm5NeaXQKCAjlonl1M6L16LkYilKPC7uTkSZHpVR7PbsCgyN3qncTk1MlmFi/0zaIrAJw6WmwKQ9V7866m+hzbZefL0Aq8nnWxv9nO5d4WBWQ92zYJHk7gyHMEm65y842JmOUFdpY/7utwoskggpnNzNIZVs/HVE1MUuiy8dAOcmkxNgZ3OlirqSiS8mh1JEthS76FvJkVOlxgeW0CSJLLZLLOLASKxFJd8Ghld5Mz1MQ7taOBsf5I3Lg1yoLOUy8MCksXO5OIip/sKEMkgiFZS6dzGMscJIqJifffjPhz498DLgiCcwSxjB8AwjD96t44f+UG/qbWJ8poploIpTlzJ8JMtNs70xviVJ9pYikBbhcyJHoOxmTUuDBUgSzqRaJy+KXhsbxGNVhmbKpMXrJT4LFikLIe3lzMfzHKkXWExkGJXRwUeh4V9reYsm0z5sVlluptUXrimU1Xs5GPdTv7w60ssh1d54mA525qsCILAc5dDHNlRRl2JQJlPI5HwIEkSt8fCDEwsIUsC8bQHA4FszmBgbIlPHfOxq9nDs5dD3LfFhiyJTC9GuT0wy4GtJexuUrBZK8lnU6xGdcp8Ei21fkDkYLvKbw/McKyrlPYaMy9hJZhkS72ThZBANC7xyf1F3JzMcqDNwfxKgqbaEu7fairTXhsSmF7OsLfVxpk7Qb7weCvLUYNdTRZuD3lprHDRUGblRE+QunIvB9tUNKvE4HTETCzaWD/eh8l8fzf8LmbpugpY3k/H/2PeA4FImutjGT7/SC2TyxlUTaOiyM58IIdqkbEqEl94vJGlQIKuOitb2yv51KFSLg2nsakyC4EUdWU2RmYi6ILC5moLqWSMTDbPlZE0j+0pwqfluDqS4tZIiLYKUzlmaDpKY5mF9iorQzMxdrRXcGhrEYsREUEQWAikqC62s2+Tn7tTWYKRNCU+DcOAg50FPLi3GZdDpaFEYk+TwvJajF/7VCuZbI7nr4Tw2mVO9Sa4PRZheFnmd39hCy6Xh9dvRdnZpHKgw8e10RRD0xHKPQbxVI7B6SifPtbE0FyaaMJM3z3fH2NTtUo0kcZpt+K0WYhE00QTWSyanUJHnvlAGl3XWY2J2GwqS8EkoqRQV6oSTkAylWNHq5++mTSBSBq7TeVTB4q5tF6OPLIEed1gIxPyQPhRMu99hmF83DCM3zQM47fe2t5Lx4/8oI/FEpwbSPHgdieFXo03bq6wvd58cNYWwvhiGknWKCvQ+NhON9+4FELXwe9W+Vi3m6fOrvCPJ2cIxmFibpVUJk84luHgZg+n74SpKjJTaOvKNPpG5nn58hyrkTTlPnjtxgpVfom6MgcvXV1ib4uVLXVOBCPNrcksV0czbK0zzVGLmOb41TW6GzXKPTrPnZujqUzkoR0eLvSF+cuXZnik201NiYNwUuSxXR621ll46XQfJ64vEY/HWAmlWIvkKPFpyJL51XdWy7x2fZX6UhUxF6d3JkNTuZXHdnt5+XqIvK7jctoRBZiaXuRW/xQ9QwEe6HJzYTCFzSqyu81H/2yeF68GOdiukUwmOd+X+KYnP5+J8fLVFTqqLbSXC/yPZ0bYUi0jyyLRWILBqTB1xRb0/Iam5pgEn6L0rtuHBCcFQTj2/XT8yJv3d3r7WVwIcm7Ihq5DKJKgb9ZAEpJIgshTp0ZpLHdx/LIdxWLF57Zx+uowHkcLiqiTy6WoLHGzr9nCjV4r22oVLvaFyYkaZ67PcGR3C+cG0qhSlk11biyqjc46B6dvLrIaTHCyZ5m8IbK0GuXycBJBlDFQuds7RyZn8LpqJZfPMz4TJpvNcvJuingsT+9EmOIiP7emEkzOBYmnspy8HcNu18hmUnzlRJiqMj/37m3DQOS+LRoj8yku3Rxj15YaXr6RR9fzZNIZFlYjXBzKoGhOBm9N8KpdI51OI+RT/MmzYzg1C8/HnPj9XnxuG8U+mcvDae6MLGCzykTiPm70zeC0q6iqxshsCKcmc2bAjizqeF1WrvYvoTncOFURt9PK195coKm2BEm28JVXxzi8qwVJEsjlNvb7/qBmckEQ/hp4GFg2DGPTepsP+CegBpMY8wnDMILrFaV/DDwIJICfMQyj5we8hS8A/14QhDSQ5X2E7D7yg37Pri5WHdOsxTMc3mwjnqgiGEnySLfbFKnstdNU7ae7yYw3v3YjxM9/rJmZNYPuJo1wooBcJsnF3mWeOFxBz0SWe7sKefVGiKPdVVQVyjSXWwArx6/ksWlgVSQygovf/JkyXrgW4vGdbjxOB6ks3NtpzuyBsBePU+PQJo2zd0Mc665gajXHvR0agbBA7+gSHdUKsVQWl1ZBU4lI30yWfa0WXrluQbVakIUMXQ12VsMpJpZztFXZOLK7BYtkcHizuQZ/5sIqn3uonrRuEArH2NLoo6XcQk2RnWTKxf94ZoR7t5Xh0AwiKRsLgQSaamVvq8papAhBFHl4h4tMJk82Z7C/1Uoi7iCPlUNtpoPx7lgSr8vGsU6VN2+u8bkHahheSJHH4GCbg7EZD51V5rEb6sdbD9l9QPhb4EvAV9/W9uvAG4Zh/L4gCL++/v4/8A78jz/IxQ3DcH6/fT/y5j1AZ72TjmqZb1wK47IrdDcovNYT5eUbYf71IzVk83nGl0y6J7tdpbbERj6b5u9PzrOvVeW+Lj/neoNUFNpYC2c42xuisdzOvdsLGJqJEUnkSKZyFLgVyjwGp28u016pIssi3Q0ap/viOFTw2vKMLWbNUlSPhUgixbm+MMV+O01lCsl1ArlTfQl+4yfbONuX5NJgmp2NKj6XlXw+w5OnF2mvcWCz2UjmLBR7FNqrnfRPJzEMA4dVIBRLksnm6RkJs6XOQX25k+lVg5WoyMO7iukZCRNP5jh+NcyvfrKJmRDcnMyxqdrKsW0+ro9lyOV0PA4LjcUwOJvG67bxyf0+jl8JkkjpbK9XuTtlOpUnAjI//2AV5wdS5AQNp11he4MTpyXL85eDHNpSwIWBmCnIsZFftCAgWqzvur0XrOe2B76j+VHgK+t/fwWTL+Kt9q8aJi4Db/E/fh//glDzLvuF9YKcd8RHfqafmZ3n6tAaduyldCEAACAASURBVLuNAo/KnZFFJLGEucUg0USGSx4HFsnCq1fmSKZz7N5czum+FAuhHHMrEW5OFWAYAvF4mlO9KeKpDEMTa+zfWoVNyfNwt4fnLkeQyPHADi+JtMgX/2mI+212FsN5dF3kwq1ZPA6V+jKNyaUsN4w8bRVWeuaCTOgGj+0vBxQsQppTt1bY3uDmzJ0ANwaWKfbZuTikIaEzuRBleiGC3+vmXM84P/dQ3Tf/z+11CscvrbC5zs22Og8XBhKksgJb680ZVpOSJAwBwzDoqLbyu1+9y67Oam6MZ+kdnsNlkwnFbHidFvKZFM9eCPNAdxGqovJ7/zBAU5WHy8NeygttfOPkOKV+jVDCLEzaXmfHpspkMxFmF2NcGpJMZp6MzO3haeZWHBT7XeQNY4NnIeG9rtkLBEG4/rb3f/EeVW6K36K9XieAKVpvfyeexwXeP/5AEAQReB64AaxgevAbgMPAUUz26Nl3OsFHftDLiowoKexvsaBZJTLZEvY0q6xFPVRaLRxaF4OMxZyMzMbY1WQlp8MrUYXW2kK66xUsikQoXInDquNRdcRSH131Fm6Ohrk8CNFkntn5NbweB+QTOOxWuhps32S+iSQqkSSRvc0Wbk+meOZkHxX+Rj7/sWpO3s0QDKe4PZ4mm1e40j+HINvprHeR0q2AyN4WlVQmRzjhoarIRketTM+glZ6RMHMBgRwiuZzE+VtzeDxeZkJ5XrkwzL5tDZzqN+W3QwmF0el5nkcnlkzTVO3lni1ObKrCwloBfqdMz3CQWFbBarXS3zdHYYEfq5Qmm8tzpNNDgcfK0Gyc+/c2kMvryJLE61en2L+jkVsTUUanA6hWK7ubzdDlm7fDfO6RJvpm8hzbYuOP/04ktYFZeYIgIL63Nf2qYRhdH+Slv0vb92XUGIbxyXXp658APotJVpMABjCJaH7XMIzvySm8kXRZKnAWsK5f52nDMH5TEIRa4ElMUswe4KcMw8iss+J+FdgOrAGfMgxjcv1c/w/wc0Ae+GXDMF5bb78f00EiAX9lGMbvv9/7LC0pYrvLzfOXg2xrsBOPx7kznqG90s6d8TC6rhJLZpEVlR3NEnOBHFeGEzy600Mmm+f2ZBpFSNNRo3JjLIkkWnmwS+PKSIZ9rT4SqRyv9MQ42lWKzyPSP5njFx+p42xfgqOdDq4OhthUbWNqIUYyoxCMpDm2dxOZbIY37ybJpuLUNvoRxCRLUYmdmyqpL1HwOiQcag5FTDO3qnB5MMoj3S4U2cGp3hQ72qsIR5Psbjbj/fFklli8FrtVJ5bI8IUf20z/TJojm6wsBZNcG4WdnbUopNhU66LCL3B7MkMyGWNvm5OBOZ2j200f0TMX1tjVUUpTqcj8ao4njlQzs5bH5dAZXdJ5eIePV2/GcagpDm4tQbUYHN3k5FVJpLEIeqczSGTwu82S3BvDIZJpC7phsNGytRvsnV96Gx1cKWbZK3zAWnaGYfQD//H77b+R1tRbYhedmCqe96/z2f1X4IuGYTQCQczBzPpr0DCMBuCL68ex/lT7caAds174f65Ta0vAn2I6SdqAT68f+74hiiKP7/Ezt5pmcS3G4FyW+lILW+qsjMwnOXErysF2G5trHXz5pRG219uxKBIOm4VgHKZWBaqKVI5stjO7GMCuKQQiSdbCaV6+EeWxXW52trgZm4+R00WcdguqnGdmNctKXKK60MKBDh9XR1M4HRqGoXN4q48Dm2zEUvB7f3eHgakomyskDnU46Z+O8+Sbc2yqktlc4+BvXx2lo0b9puUQjiYQBNjbauXqiLmuPnErzkPdXs73zDGzHMWp6pS6dc71hugZS/PgNgdj02s4bBoNpQqqRWYxlAVFocSrEE+Yk8frPUF2t7q4b3shV4fjLIUF2qsdJLMyx69GuG+r6V8ycgmiSYHNNQ6Wllb4rb/tg2yCZBZOXZ/j7lSSzhozNHrfdh/PXVg2Ja02sghO2PA4/XHMfHjWX59/W/u/Wl9v72Kd//EHudAPgn8OsYsjwGfW278C/GdMb+aj638DPA18aT3U8SjwpGEYaWBCEIRRvqUGOmoYxjjAOqHmo5h6Ye8ZA4Oj9AbjWBUJSbSSzenMLYU4ddeJzSpx4vIkZQV2TvVpOKw6kihxfWiVkUUvTk0gHA5hVVXODyS5PbqKrsOLl1exqDa++PUhDu9s5sJwFgmDfC7HyOQKr2hWVKvKnz3Tx6bGYs4NWMjm4dTlIe7d08LI1BIv5lLIVgc1pS7mVmK0VTuYWY5zYyRMJi/SN76GplnR5DyJVJY7YwEG5x2oViuqxcK1O+M0F1WyGs5wYziFoWd4rSeOYjGr2XrHIqzGdK70zrO1tYwXruUIhJNMLcWZWhKIxtNcujXBnm0NnLqZQhVznLqVoazQRTyZ5Vx/jtGpZSRJJJ3x0DuyREWxg9d6FFRVYSmQIZ5IUFdUTFezj9vjUfa0u8lkdZx2hfnlCMevKNhsGqoicKV3FkkQyG7gTC8IApLyvpLXvte5vpuW3e8DTwmC8HPANPDJ9cNfxgzXjWKa4j/7gdzE94kNXdOvz8Y3MJ0Mf4pJXhkyDOOtaOzbBSq+6ewwDCMnCEIY8K+3X37bad/e5zudI981DCKY+uK/AFBV9e38AmWlxazG+jnQphCJZ8jnitEFmUPtFgKRFC9lc3x8fzEeu8KtsTA/dW810wE40KaSyelcuhNHMKI8tLuM7tYi5laiPLzTz4X+MPu3llJbJFK3Lnb57KUk3VvqObpJ5c54hENdNfjcVroarJy8GaKrtZi9zVamF6wYgsz96yw4WaOCkaUEH99dgCAIrASTTC1GeHxvESeuB/jVT7QwNJ9lb4u5Vn7lWoLqikJCSQGHTeXFi+P8qwebqSlSuGCXWA2l2Nvl4VJfmF/91CbO9kVNVh+Xk4NtVs7cCVHodVNW1EkmZ9BRb6FnLM2b18fY1iqwudpCPJnnx++po382w70dGn1jyxzaWkxdiZmQczzpoKbcR3uNhecuBfjs/ZXMrOZorVSpKvfjjqR4sMuFLIn0TUapLHEzMrVMei3xHn9d3w8EBPGD+ckbhvHpd9h19Lsc+478j/8c+KGKXQCt3+2w9dd/FrELt8uJoWeYD2Q5eTvB/jaNeDwJwOm+JP/pp1o402u+nwmI1JRoBKNZUpkcx69E+On7a9jcWsnQQo4dDVa212mMzCdJ5izct72Qm2MxcnmdYDRFeYGVXE6ndyJKWrdwbLuH6eUkV4fCVBbZTF36ySjbmgtpr7RyfiCJruso5Di8yc75AXNAnB9MUFVsJ5fPg2yh0GMlnDCnyKmlBEV+G0VOgRKvTDSeoqu1CKcmcurWGpuqFA532Lk6nCKatVDqs/CxHW5evh4hl8/xzPkADeV2UskEHdUKqpQhlxfIZlPs6ihnV6ubyRWd1ioHFX6ZbCpOMp3j8I5aBqaTLIfMz8bnlEhlcswsJ6gqtlFZ7CSQkHjjVohttTJHO+1cG0vTNxUjnrPyuYdqSKZyG5uGK/xIVdkhCEK5IAh7BEE48Nb2Xvr9UOL0hmGEMAUqdmHGKN963L7dofFNZ8f6fjdmHPR7iWB8IM6RzmqVLz3dT4HDQBSg2Jnjwt1VmitMWuiOKonrYym8DplkOkcqGeW3vtKPz63RO6tz8sIATk2hZyyOx6Hw6pVFuurM2f3BbU7O9CU53Zuiq16ld3iG168vkssZnB9MI0kiZ28tEYplcdktXBsIsr1epa7MjlfLcfzSCgVOAZ/LSiaTYX4tg8+lUeCQee78IjsazOhCkSPP3FqaW5M5ttdZOdjh4cWrAaqL7Ty0s5CbExkygg2vQ8Eii7xwZpBQOMr5wTTXJ3TiqSyvnRvgSKeDcr9CStfwuywc7PBxYzyNpNg43OHhT57uIxhJUOY1B8e9232c643hsOR5qNvLhYEIz55fZHudhUQiwfXxLFvXlW5SWQMkGx67gmqVOXl5gkv9QdoqJNx2K5mNLq3d+DX9Dw2CIPxX4ALwn4D/e337v75np3X80MUugFPAJzA9+N/p7Php4NL6/jcNwzAEQTgOfE0QhD/CZP5sBK5izvSN69GAOUxn31u+gveM1bUAS8s6m5vKWQimOH4ljMNm580rQzx0oJkLQxl0Q+GVc4O01BURCouIosLRXU0c7bARiWeIbqnCaZNpLBW52LfGSjDGwLxBbi5LLqdzd2SZTCbLi5IABnR3lLN73RR/oyfM5x6sQVNFvvSNMVaCMV66FjYTSQSBG4OLrIR9TK6JZHSZP3ryNg/tq0eTIZLIsRaMMzWfJZMx+NIzE+zfWsnZwSyZnEHf6CJFPhfBpMDdoRnyhoAsVuJSddoby2isdNG1/tB4MSKwa0sdF/sjGKLE4nKYK4N5DERm5laIp7K4tQo6W8pRrSIvXVnF5nCgygYX7syyp93Hc8tp7DYbd4fmOOP1MDYdwqrKnLitkMlkuXh7kvoKP2+IhdgseYr9LlqqHFzsXSOZUzY8OcfUsvtwDOr3gMeA5nVf1/vCRq7pS4GvrK/rReApwzBeFAShH3hSEIT/AtwEvrx+/JeBv1t31AUwBzGGYfQJgvAUpoMuB3zBMIw8gCAIvwS8hhmy+2vDMPre700W+H2MjaU4utXL+f4oD3e7TU95QzEFLpmmUpnBmSifOtbK1FKKh7udnOpLE44k0HUbb96O8FC3n5euBWmvdGMIFj51uArZYtBWaSEYTZNK+cnldA5tsuO0awj5NONLMnXFFlK6isdp4blLaxR4ND51uIKhBYOjnQ6Wgkm8jgbmV1McbFMQkLnR52BLnY2vvznDUigDgp9Cr8LUcJTmmgK2Nnoo8ym8fjPArz3RRv9snoNtKoGAl1QWDrYq3BqLsm+Tm7tT30p0t6oa1myCHc1uTt9ZpaTAxY4ms7R2ZsWFX4TOWiuxjEg6meDwNh8OTUbXdc5cF/G57XS3eLg5HKK82Mt9nRqGXkYskebIJhVFthFPllNeaGf/eu5DRpfJ6HBft5/LAyGztPaHYN7/iGAc0zn+L2fQfw+xi3G+5X1/e3uKb3k7v3Pf72LWD39n+8uYntEfCIm8lWKvxYybj6ZYi4h8+mg5Z+6EyGUVJlcFHupScVpy/OXL0/zYgXIyWRt3p5K4nGaSzcM7vLx+O4bFYqGj3saLN+JU+QXO9CV5dKcpbvHU+QBlBTZ2tzh58WoIr92NZpV56uwax7a5uTSsUlFkJ5KIcHMizcRCksd2uohXiJzrT5JKpfjpYxVMreSprirjJ++z8NrNKD6HSGutj6YyC09fCPBQlxNRslDq1xiZDzEfyOBy2rm/WeGlaxEsVoUdzRZWgwmmltNMLsbprHZQ4PJzYTCJVXWSTqfI5gyOXw3xcLcHRRJ48VoEr1vjyA4PJ27FuX+bk9vjUT5zrJGRRZOOaz4iUVVg4dVrS2xp8OLWLJztT3G0w0ZJoRNDTzG7JhNPpKj0WrkzGUfXVYJJxYzTb3DITvyAvPf/ApAAbgmC8AbfTqLxy+/W8SOfkXfzdh+F1gz9EwESqTyXbgexaTLnB6zkBY0vv3CXjx1q4eJgCpAYmQ5wY6KYWCzOyEyQe7YVcnFAJ2sonLk+zpbmUs4MKKyuhfl//36F+/a1cKo/SzaXZ3ElzPxigGSmArdT40+eHqDQa+ezD1RjtUg4VFPiyu2w8pfHh6ks83BuSEPCoHdkCadd4b7tXl66Os+RbQW8cTOEKFh4/fIEh3e1sBjK4LHL/Pbf3OWBfXVcGYxiVSS++I932dNZyZVRF4trUdLpNJfsZWyrdXJ2MIduWCnymIMhlgJNlTnU7uK3/uYujdVeXr8pIMkKyVSGwfF5nFIpTqvAfCDLfEhka4OFmZUYo7M6RW6JmgInX35pnIOb/WiqjCrFuTkWxadJdNS6efpiEBGDj+91Y7MafO2NGQ5tLSavGxs60wvvPQ33w4Dj69v7xkd+0AuCSP90jKNb/XidAj5XlNqKAva1qgQiKXJ76vDaBTrrVEbnIjx2oA5NEzi62c9v3J0hnfGwq80NQC7XiIDAoXYVt8UBgsCBVguCIHBnPErptgqCCYODrRZWQ2mmKvysBuOcGciwuLiMoii8lMjjtqbYs60Gpwq7mszBuBT0EAonef5ygKn5NQKNPu7d7kXXQTCK0RSDPS2m4s61PtOaUNYVWm+NF9FR76K+VCUYdeJ2FLOlWuS1niDX+paoKnNzPJ0mr+e5M7yIz6mBXkyx38GBLWXUl5hOyRevpGlvqqDQZ2V0SeePn7rL/q2VXB4Em1Xmfz03xOFdzfQMruL3OhmYz5LMGqTReO61Ph4+1Mr5wQx2TeF67xQupwNBEBiZDVFdXojNKhHJb2RtrWCq+/4IwDCMr6xz3DetNw0ZhpF9L30/8lV2WzpbOdDhZ3gRSvw2ykqLCcbMfPQzfUnu2eolmDR/KL0zOnvaPSwEclwdCvOLj7eRw44oirx2I8COBivhaIJcXmdyRedIp5fbUxlWQ2lWYxId1QrpjM5CIMWVsQw/cbiIlvoyjnVqxNMG2xo9PNTlZDkqcniTnXTOfCaf6w2zo9GB32Pj4R1ejnTVsBbLo1pkppeibK5xoUpZ+mYynOgJ8LP3V31T4PL0nQCP7ylhdNEM6bkcGvFkGk2VqSvWeHB/PR31BXxsl48ttTa6N1VRVe5Hzyb5yXvLGZuLMR8wf0tW1YaeTVHskdlaI9JUXUhbtYtdLU58doGmmgK21lmpLCugqcJBhd/CvlaV3Y0ytRU+GosFuupEovEs93RV0Vgqc0+HRm1FAYUuEVEQETZ6Tf8jQqIhCMIhYAQz/+V/AsP/okJ2/9LRUO6go1ri+JUwNqvIrhaV830RSn1WRFEgEkuRyuQodCsIgoDfnmV0LkFtscpaJI1hGEiKhssmc7jDyc3xFA67jeoSO8MzUZ6/vEZ7Bcwsx+kbmuHaSIoHt5mznGLECUQztNYVMxOEW6NhNtc6UWSRRDrH9FISq9VKZYFCW4XEV1+fobtJo7lY4Mpwkvm1DF6HzLZGF8trCZaDGUr8Ntai5iBP560UeRTIJxidDVNg17EKKa4MBkjkFfa02ImkBXonoswGDe7f5qCuyOBy3zLBSJKjnQ6uDUd4s2eZ1nKJw50e7k6leON2nJ+9r4Kh+Sy6rtM7m+cXHq7h6dNzVPkEDmz2cHvKfFi8ciPCzz9YwcBchuNXo3x8j4897R6uDUeIxDO0V7sYmImZa/oNhTnTv9v2IcEfAscMwzhoGMYB4D7M9PV3xUd+0A8MjnH8apj+ORGvQ+buyAJDCyLPnx1lcTVCLJGhxJnnH9+cp7ZA4NmLAdbiAqPTq7x8I4pVTPE3r03TWW2awB6nleVwHkXM8/ylVcZmgmTSKSYW4oQiKaxWhan5ACdupzg/kGRzjYOvvjZDW4VIJBplclWntkhG1w3SyTgXBmPUFBiMzscJJ2FmKcpqJEtlkUo+m6J/KkomZ/DsxTUEWSGdzXP8aphkLMTXz86zfT1f4N5tPp48NQuGTsaw8OrlecIJg1d7YlzoGePlKwvEU/D85SB3RkLYNAuCqHBtNInXqfLGjXlGlw0GZjK82TNHc7kFXTfwqFn+9LkJVMXglZ4Y0XiSV67M89ylAMl4lJ7hIE1lKq/3BHntwhgFHhvXRlPcHo9xoE3jz1+Yoswr4LZkSGfzG1tPDwii+K7bhwSKYRhDb70xDGMY05v/rvjIr+lrqsu5OXODQ5vsuGxWBEmhxp/ngd11iLLE0HyWaN7OzOIcC+ECHt3l5bXrS+xoLaK7XiGZ9XHuG/30VxTTP58Cw+D20BxlBTY+fbgci1UFw2BXuw1d1wmkbUTiee7bYso7X+wPsbASYnihjIWVCNF4FiObRDcMesfXkESJ2gKBEp+Vcp9Igc9BPpfjtesxDMnC4MQKZwscfHxvMaIoIIoKB9os3ByTOX5mlEJ/AdJCGglTrafAAeOLBnu3VNBeKVLqtRKN+in0uTjYrgEaz1+VaKvVkWWJnU1Wnr4QZHtLEd11ErKscOJqljtjIRJpneVghlA0wdHNVciyiCzVkEzE2dfmoHcmxz+9McRD+1s4sNlDPCtjtwrsatYIRFJcuLvK/HKA164pPLbPlNneWOe9gCj9yHjvrwuC8GXg79bf/wRmyvu74kPzWNsoaKrKJ/d6eeNWmOmVDFkdeiayHNniIZPOU1tkwUqC7rZiNFVGFAUyuoWHd5dyczJHkVvi0PZq9FyWQ60KB1pktraU4fd5UGQBuwVEPUE4luXkzSCdNTINxQbD82lEUSQQF/n5R5uxWw1KC9y01JXx2L5SHt9Xyr6ttTxxuBIUJzWlTq4MZ6ku0qgosvHI7iIMYHtrKX6nlYFZ05TOGwa6rjM8l+SBPTWUeQ32t1gpdOQp9jvJGwJ1pRr3bPFwoT9GNqejqRrBSIpcXufWWITN1RpbGjyMLxu8fD3EoQ4PD+wopHc6ze2xCD/7YAOlfgfFPg1DsvKJwzUML5gOOFWBh3Z4uDSUZm+Lyp7OalbCGURBwO+2EY0nWQ5nCURzyKqLP/ylblYjGdJ5aeOZc360zPt/A/QBvwz8CmYeyy++l44f+UH/Fh7b5WF0NsbpS4M0l8kIgsB9XV7O9ieIZCzc313MciDB5HIWTTOz6bKZJC9dWmR3iwOPLcfwfJaXroXZ32KloUjnqydmaKuQuGerjzvTOXRRw2NXaK12MrKQ4+JAhI46Fw1lDuYCeVRNY0utQv9MmpevBuhusNBYbscqZrk+mqTQa+XAZi8DsxleuBpiT7MDr0Nhb5uDVDrOxcEk+bzOs5eCPLTDQ3eLm77JGIlUjv65PB11Ts71x9hcbc52D2138sbdJLJF4UiHnRtjaeZDEnXFCqOzUU5cGEJEp8AloakK8YzMckyhosDK/k0unnp9jNmFVZor7AzOJlhcS+BW84iiSJkXXr2+Smedncd3e3j1ZoS5pQDlPpkvPdPPqdtr3LfVgSgKbKrzcermGt+HWMv7xo+KeW8YRtowjD9ap8F+3DCML77X7LyPvHl/41Yvg9k0miKgqTIIMDYXIxgH3ZCYXVwjnzd4VRTIZHSePjWOy67yRl4ll81xc2SNsmIXBjLfODOGZrXgddvJGyoDE2O4nHYclixrMZHaEo3ByRDD8ylGZqMYBhzpUrBb8lR48owsZKkpKeTF6xFUVWU5mOTkzRSqZufsjVG2NJeiG05ePDPM9qYC5pby5PIZhqaCRGN5RuYC9I6u0NFYwoW7WSoKFI5tdfLazSjZTIbFlMH8Uoizgx5kEUQhTzQSYmEtRTpu5+LdJTY1lXBuQKbULbOjo5bVUILnLgXxODV6BmaxKiKviQKaxaC8xI3LYeFsfxJdN/jzFyb43EM1nLm9wlrM4OSVSVL5ZqYC5jJiIJLEYxdorStFFnUuDyVJ5iSyusRyILCuKLuRgfoPf8hOEISnDMN4QhCEu3yXD8swjI53O8dHftC3tzQy0zvB3lYbr15P8O9+vI3zAyl2t5hssYFYAU7NwqFNKmDnubzOWiRFV5OHaCLL2HyC7kY7giAwv1pCgUdlf5vGwHSUzxxrYmYtT3ezgz95ZoR4wsHRrQXcv8OJLqmoFiutlVZujYS4NRohEE3yil3jeu8sDk2murCcR3a7uD0e5bMPtTAwm2JHvcT1Pic7231IgsHZu0GWgjo/friE/ZsLefq8i/IiG53VEoFIlsHpJL0jc9SWOLDbHbTVF9JRIeBdl8Iad7j4p7ko92wrQLDYSWdyNJaKlHiszIUlvHadlkobJR6FG/0G+zqK2VJv5+vnV3l8j59LIzqHNqlkcio3eqdZiwt01HtYDmWRpTqmFgMc3mTD67SRzMn0TsU5ttXF3ckktSVWSr0yqyGYmBXRLDKpTGYDv20BPiQhue+BX1l/ffj7PcGHw5bZQKialVwuzfxqElVVcdsVKr0GYwsZXr8ZYE+LRjxprpdP9IToanbTWKqRzgpcHc3w2J4iBmfTvN4TYv8mO+G4eezIgk5HrY2WUpHRZZ0922op8rvwuSyIgoDPqaIpGWIpA6uqsrOjgs/cU0OpV6Z7cyWP7C5jYkknncmzFhVoKFVoLRP5y5dn+MVHqrk9laeiyE5TfTlHtxcyuiwwsRins07DKmWZC0JVsY3aEpkjXTVUlhfjdtt4sMvL6d7EN03p29M6v/RoHTcnszg0K5/Y4+XuRJyesSQ2OcuhzR4uDyUJxzPs6yxjciXH6zeD7GtzoFok4knTonzuUojPf7wJWTIH7/iyzqN7immrL+VMX4yFYA7d0EG04nMqHNzs4mJ/hLyuc6YvwaEOD9kfhmqtpLzr9i8Zb2Pc+bxhGFNv34DPv5dzfOQHva4beDSD//aPvawEYjx3YZ5EKsX5vlUkyYLfqWARk7xweZnGcjsVfoUDnX7evBOmplilptRB33QKh91KoUuhuQT+8c1ZttZa6JsIc2cqxbOnRhieWqHSB2/cifNqT4juRiv72jz81YtjzC4nqPTpFHosjM+ZpngmD4aR57f+5g4IEq/fSTOyLLC4GuXScIZKr8nP57BCc4UdI5/h1etrNJWrdDU6mVxMsBTKcqo3xa4mlfGZIOVuc1DtarZyeSRF31SUtkoVp93CSjBJLpvm5SvLZHWZ589Ncnc8xOn+DKKR5Q++NsjscozFlTC3hpYYXcgyuZRAzMf5+tll7t3qpqrYwWpc4sVrEY6t02ZFYwke6fZwazzOhZ4xNlWKxBIZIvEMu+ol/uKlaTZVqXQ2eMnkfgiqtT86jrx7v0vbA++l40fevF9bC+B3qxzYVo3TrrG/TSUQSXP2zgwrazE0SyXJnMztoTk8Lieza6aX+kbvNKqllvlAlht9U+itFYSTBoIgc2toibJCFzWFVh7b7ULTVDSLiNcpMBNMc2d4GafThSqlkCUJi2wwOhslnjKYWQiBKLKnpZy5yzAk9AAAIABJREFUgMFnH2oimBTZ3aLRNxGhYH8NoiywEtJ549ognS1lnEFBFhQmZtY401eAKFuRFI0vfWOY2nIPZwZtDEws4PeozIZkkqkcwxOLZLI6+7dVcbo/w8JqDFUxeOJgGeF4jkJPPbGUwaE2C/m8h97RFR7bU8ALV0MU+13sbbUzMBVmcCqMgUSPx4Us67x2boDuzZVcG0kDeRRR58uvTFNbVUw0nmFiKY1mlRAEiMQyTM2u0utWuD6U39BwnYkPf+69IAj/BnNGrxME4c7bdjkx6+vfFR/5QV9Y6OfG3QQPdHmYWErRM5FlZ6PG1rYaXNYsPrfMYiDP4W1llPsEaooUwrEM+f31aKqFHQ0qLlsLoViG+7fYeP5ykP/wmTZevxVjR4Ob03dCbK9zcGkwQXW7m6G5JN3tRVT4RBpKHaTyVQRCMQ40eLBrMjlRw2JRmF7NUlvqoLlM4Y1bQZaCMuMr8Ei3l2cvBXi020syJ+N2WDjUbuWlK1H+4093cHsyx8E2K5lcnlS6ilxe53CbhVSmGZcqsK9NBVSeyWTR9Tx7mlVyOZ1Mxo9Dk7BrCq/fSfLYLjvPXQpiGBZO3grxU8eq6BlP4XbaaSnVuTWRZlu9l8k1BV3Pc7RD5dTtMEe7qygv0GguN8uK31iVcTtt7GmQscotJLMGe9pspDI5Xr6RY0d7KR/bXcRzlyOYJTEbXFH/Ac3kgiBMAlFMhuacYRhd7yRr9YFc8Fv4GvAK8HuYCjpvIWoYxneKb3xX/B/zXjdwODQsikRzhR1ZSHOhP0qBQ2dni4vhmSiCILK33cONkRjJdI4375jJJ2tRnZM9a3RWyzSXSbxybY3aUjtep4VHd7p48swSwbhBoVuhqVRkZC6JIKvcu62Q4dkYq5EsipDn0Z1uTtyKcupWgO31FhaWwyyFcvhtOZYCCVrLFV64tEiBy/y6ttVauT2Zwu9SiEQTJNNZLFYNn8tKIpkimsjywpUQxzpt7Ki3cHEwhU2Via8HdJ69GOBQhxuLrGAYBi9eC3GgTUVH5M3bYfa2minCrRUi44spkGxUFtm4eGeJCq9OWYGdyaUkg9NRGkoV6goFnnxzjvJCjaNb/Yws5hiYinNjIs8n9vp44kARX35tljKPgJ7PsBbJcPxKhEd2uKj0Kzx9dpGDmxzoxgaTaAgfeMjusGEYW97Gkf+WrFUj8AbfPig/EBiGETYMY9IwjE+vr+OTmF58hyAIVe/SHdhY5pxKTB77Ekw2878wDOOPBUH4z8DPYypzAPzGel38++a3fycO/fdznyfeOIuaCvJSKodFUZBllVfPDXCgq4ZUVkQSdG6OLOCwqYhCnucuBigrciCJAi4lyVzQ4MpgkCw2zvbMsrWljNFZA0WETCrFeCjBKzc17FaFN6+M8cDOEu6Opin3GHzt9Wmsss7iqoN4Sqd/NEQqX03vyAJ7O8tYiqiIAoiCSCSe5EpvklC8DNUq88Kbd+neVESFT+EPnxrmx49UMrcSo71K4a9emqS9xkXvVJJ0XuRa/wKaRcKuSTx1Ls6RTi9+p0JbpczpWwFqS02nXDCSxOtcz9UHWqo8/M2JOeqKrZy8DcuBCHfHNWaDIplMlq++MsmebQ3YNYWbw0s4nA4mlnKMTq9yeyBF1+ZqXrwWJpvJMLcY4kKvTFlxIX/w5ACHdtRzeTRPICIzNreMzWbbYJlq+CFU2T2KyZALJtPzaUwtuw8cgiA8ArzFJrUMVGMKXrS/W9+NNO9zwL8zDKNHEAQncEMQhNfX933RMIz/9vaDv4PfvgxTivetssE/xXRczALXBEE4vk74/xaH/pOCIPwvzAfGn72fm+zY3Ep4Isg9nWaITtd19HwDFkVmd4vGNy7EaW8s4+hmFV238vtfGySeSBGJu1gNppicD/JrT7TgdVqwa62sBpN8fK8XQRB4tSdGLp/nwe0OovEM528pyLKM22kll9dRZIGWag/7N3t54WoITSvhwR0uBKkFXdfZXGs6wzK5HF1tFaRyAsc6NZKpHHOLFdRVOKkukHjl2gqr4Txep0QwlmNlLUZFl5+aUhuj80m2t5YSiOUQs2Eu9M7g1FQUSw5ZsnLy+igP7G/l/GCW8z0jHN7Zwut3koiAIEL/6BJV/nLu2aohKc1IGBxoszCxoLEScHOozYpVEUnEKvA5FXa32EgkHdi0Yu7ban6mcysJin0N2CwSRW5YaSikpUKjttjCsxeiNNcW82CXi//vr0WT7HOj8N7TcN+LrJUBnBAEwQD+fH3/O8labQT+Cybn5EnDMLYKgnAYeCeG3m/Dhpn3hmEsvCXHaxhGFPMpVP49unyT394wjAlMjvDu9W3UMIzx9Vn8SeDRdU78I5gc+fDtgoHvGWXFRTSViJy8HQfg9Zshs0R2PfTmcdqo8MD8WppYMsvBrhpa6wt5qMtFgc/Jv/1UM+cHkiRTOewWnSMddk73JrjQG6SzxoLLkmUlnOHEnTi/8VNtzEdkSvwq10bT/NyD1SgWGwtrKSqKnexqsdE3nUJRJErcMLlk2uMvXwmwq0UjnYyvr4UjfOpQMbOraXwuK/fvbSSQkGks15gNwBd+rAlDUrk1liCPhX2tGvVFoFgsfPxAFYJgcHSThS2VOjvaywCD/W0qe7c2IKJzzyYLRzZZKNDS/OxDzeRFjdVQCq+aJ5Y0B2XvjM7nP1bFqb4kgzNh2qttROMpViNZ7DYHejZOdP0zvDaaZneznWRO4tpYmk8fLuXmWMwU1HTYeHC7iwuD6Y1lzXkLovju27qs1du276Zjt9cwjG2YHvMvvNey1g8QWcMw1gBREATRMIxTmKIy74ofypp+XWlzK3BlvemXBEG4IwjCXwuC4F1veyeRv3dq9/POHPrfef1fEAThuiAI11dWVv63/VXF2jcHviibJbIV7hxPnZ5lU6XMjlY/Y8sGJ+/E2dWkkspJHL8a5t4tTjwOK7uaLPz3Z0ZQxQxLoRzT86ucv7NIJptjR4ubp07P0Vph5WLvKsurIX7nb/t5uNuNRZHIInF1LEdXg0Z1iYPp1RyKJLKjxcvdyRS6ruOw21AtMse2+/mHN+fZ2uBAkUUe2Obma6cWKPcKeG1Zfu9rg7gsKfonYzx1cpRwPEWVL082p9Ne7aR3Ik5FoUprmcTp3iSn70Z5eFchRc48Z/rilHhkWsolzvablN/TAYHWShWbnOP5S8u0V6nY5RSX+9dorrAiiiK1BQJv3gpQ5rdy71Y3Z3ujqBY4ts3LjYkMg9MRWipUBEFgMZCkssAkFdnfonL88iplbgObKpNJp0yFmw2E8AHm3huGMb/+ugw8izk5Lb2lRvsdslYbgZAgCA5M6bh/EAThjzGt63fFhnvv12/sGeBXDcOICILwZ8DvYJpHv4NZF/xZ3pnH/rs9mN437z3wFwBdXV3fdszaWpDBsTSyLNMzMIlDUxDEUhTZxt3RGSyyREO5lcnFLHUlVm4OB3jj0hx1FX6ujDpJZ9OkkkmC0RSxjEFdkUpdpR+bw0U8lePNmzEWg0kkm5stNSV4imJkr89za1ZkNRBleHyBn7iv3iSFBJxKmnTWAGxUFwr89StTPLCzmEuDMRJpkem5IENFPobnsyYjz+AClcUe7FYFlyazrcGNRRa5MrhKsQvuTkQIxXV0QyIUifHKdSu1lQUsrEaYmV9DszuwKBYuXB/H79WorypicnaVC7eidDQUcnFIISfamJif5lRfEam0xJVrU+zcXM3YYhZZlllajXFhJIeAwNx8kPGZHMGQh2RWIBK3ct8WicGZBFdvT1B4sJVzA1lyusC5nmmEHQ0sRjIg29A3WMfuLRKNH/g0gmAHRMMwout/HwN+m28xOv8+3870vBF4FNOJ92uYFXbu9Xt4V2y0wo2COeD/wTCMbwAYhrH0tv1/Cby4/vZ78dh/t/ZV1jn012f774v33u/30mGzcmMkyqMHaglGM+xqtnK2N0xzbQF72j0k0znGpmaxCF4ObPaypaUcl0PlULvJ6to3kaO1bguheJZSv4270zouW576Cjd5Q8JT4EVTrRT67Fzoi/GvP9NNz61pPnmknF/vn+H6SJj5kMk8O7GUZmJ2AbuqoGNlej5M34TGgc4CXr0WZFNDIfd0aCiyxNBMlMePNOLUIBhJ4nOr2DWFpWCKx/dXMh8SuKfTVMlZCKQoL3YSScLhNgtzRTaOxxLct8XGpcEIT9xbz8xqnqMdGomWcv702TE6ah1UFlpZCSYJt5VQ5hWo9NkYn3FwbKsT1SL9/+S9d3hd13nm+1un94KD3jsIgCAIkATYexUpqljN6orjxE48ySQ3d1ImZXIn19c345QpdsaJLTseOZZVKUpi7xWd6IXovR6c3suePw4kaxIVyhb1SPKLZz84WGevfTb23uus9a31fu9LNBqnqUvNxiL5CkMvGblMwe4qDS+cG2dkYhFiSdQU6lhTlglSnG3lapbdIXR7V+H0xdi9WkXHsBtx14f3n9hEXhrw+soXtQL4F0mSTgkhmnl/W6u7gd8AXpYkaYpEaHvHuGvD+5WY+wdAnyRJf/ue8oz37PYA0L3y+jjwmBBCvTIr/46+fTMr+vYrmmCPAcdXrILe0dCHX+KbdWzOT1hSUpapICbJcXnDxGVqntyTTdtolNxUHTs2lKDTadFqFFjNRkrSZTQNJkwdxxwKKguM+MNyTjcvsaXSxIGNmbSPBJlyKTmwpYDBKR/RaByrRUeSRce8K0o0JnFo5xpiqFlXamZTZRLJNitPHS5Dq5GTb4uRkqRja1UyDk+YjGQde2uS6JkIEY/HGZiJsbXSjMMvxxNW8cDmNDrGQrQNB1mVrSMWCeLwJuLqxsEwG4rU+HxeQuEYzUNhaosMXOtaRKFQUZqpwu3xEovHOdnm4t8/XELHikT2tb4gR+qTGZxOhARfO5LHtf4VY8xbDh7ams74QpDTrQ42lWpQ4sMbjJFss7G+PJ2NFVacvhiby03Y9HGaBkNc7g1SV6LDqI4xbY8w5ZR9Cll2nwwNd2V+qXplq1xRa0aSJLskSXskSSpZ+X1H6+a/IEzAaSHEVSHEbwsh0u604t2M6bcATwG7hRDtK9s9wF8LIbpW2ES7SAxPWNGsf0ff/hQr+vYrvfg7+vZ9JPTz39G3/0Pg91e08m38XEP/juH3B+iZirGpNOHBFkdwrivAjopED2lQRRmZC6BXRbm3zsrZW15UioTEViwW5tj1eaoKE7202xNApdZiMapQqxQ4/RJWa+I4Rm2cn5wcoL4qGYD79pVytcuJWiHx0O4cTjQtc6Jhnq2rTZTkmJla9HOp08mvHymkYyzIjb4A64vUWE0aHH4Zbzc72VWVmB33BiLotGq0GgUOHxgMemQywb4aC01DYa51u6gr0SKEYP9aC60jISwGNclWPWebZpmcc9M76mRrhYFXr8xSlZ+YMyhKk9E3FcJs0iRSYHPkjM86UCrlKEWEOUcYlVpPWZ6F6eU4Go0Gq0HBzjVWWodC2Axy9tVauNrjY3heIjdVjdmgoLl3lvEZO2c7/Dh8gu++2otFFbr7clmCxJLER22fA0iS9JeSJFWS8MjLBC4LIc7dSd27qXt/jfePuz9Qp/7j6tt/kIb+x0FzWwdhh583m+Jo5SF6JwM8tCObYDiKLxAhK0nG944PsrbExoIrhl6rpGdoDrk8k2AIGntmSUlNZtbhpWNwnsLcNE63B5ELuNk5RXlZHmdaPcQxMzgxzrnGWeKxGPFYnEs3ByjNS2HJFcbuCjAzu4RSpQEhMOoNtPdO0jRk4dyNYaqKzFzrDCFJcLPbTmVBEoFgQp+OiBu5Sk84IifkdxGJy2jp9+L0xugadhGLSygUWUwuhhDA6xcHqa3IYWo2wKqiNB7YksL4fJD+mRj9o8skpyQz6wwhFyqOXeyibnUub7VEGZ5YxKDVcrxxGZNBzz+8fpvKAjNnW1Wcb57l6PZCGm6DhIzTN26zuSafk7ck4nGJ/tE5ZHI5acY42elWNBo1B2r0xONxJmaTCEQkZOIup9Yi+ALy0RaAOcAO3NES4a88DXfHto20XZpia6UJGXFON3UxOpvEvEaOWiljeMqHII5QaMmyKVmbr+aMXEF9iYLjjX7+06/V0DYeY/saM2g34gtEeOzoGq61jPKNr6+h9dYA9+9fjSRJ9NyeZFtNGilJOi42jPPgngqc3jj3bk3np6eDZFZmUp2vIsWi4mrHIhnJRraWqei+bUKr1VKel+jBu8b9gMTonA9vIJbQyYs4cXuTGJrxYjZoyCy3UZqjYDmowmLUsqkiMSp4s3GZopwkHtxi42J3iFR9mGl7iPx0LZ1jdipKM9i9MlfRettNZoqJ++otnLzl4cl9eUzMebBZdeQmqxidScJg0LCx3EjrbTe1JQa0aiVDU14e3luOwxfjYK2R87ei5Gclc7Tegj8YITYaxucP4PSquNrt4pl9mVwfuJspte/B56Qn/yiscPAfBVJILFt/dYW78pH4lW/0AEc3JvHyNTtJuii/+0gFvZNRtq42cqXbSV6WjdxUDckWLTIR540mD8Pj8yy7bTy2Kx2lQs6i3cXV9gi19fXYHR5eO9VBanoW66rySEvScbFxmEjYz9ee2MrlphHCwQlKM1SUFJtp6lni5XNDrCuxUJCu5pUrC3xpWwpOn2DjKgO3p3xUl9hQihiLXjnl2Soqi9NxeyPUV5g42ezg6YNFdI96sLvDPLmvgIZ+NzaTmkvdXnZXW2judzKzKLg+EGDfWjNSuY7WQTc2g5p1pVYudQeIRHzkpumZWXDhC0S4PRMAhYpda228eHGOg3Vp2IxybEYLF7qCJOnCVBVYicciLHpgS3UmN/pD7KiU0zsT42idge4xN23DfpApWVsoZ3g2QOeolyN1SchlWs7ccmMxadBp5MRjgU+FkfcF6unzSKyItX/cir/yjd7l9NDSOotaqeVs0ziBuJaR8QUGxxfYWJlMcZoMhdzMqeYlYkKO0WBAQsas3cPZTjNqpQKDTsXZlkWiRgc6vYGz1we4Z6+NExe6yEo34wnEmJt1kZZi5vK1LlLMSmKBZHpHXIQjUdq6x9FptEwuhcmwCn50YpTN5WYK0038l5eG+frRAow6JRc7ncwsuMjP0BMywPfeGsGs19A/oyUYkzNnd9M5acRmUvHDM1MYNAqudcdQqdT8/cu9HNlZyfBckOJ0JVc7F/m/Hi4BwOEJ4vLJuK9eT1GqjDduzKNVq0hOUjK7FMcbjNA3E8Xtj1CUocHj93P6Voyj9TYUcg3/8OYY8WiMJLOWH045yE2W8/r1CBqdlnPXezmwuYTsFC3Nw1EsRh2BUIyLHU5udEyzbX0RxxudxCKfQkwPX5ieXpKkPxJCbBVCPCdJ0g9XDGMNK8S2D8WvfKNXKhUcrk/lSpebx/cXEYwpOXh/IT+9OIfPH+JKV4C2gYR+2398pgq5TEYokolWb+DI5kQI1Tm0zHLcyoF928hMt+HzR0iyqNi9ZRWDI9MEg1G6+4bZtMbC5po8PB4fB2sTajsvXVmksiiF7WVyFHIZLf0BugdmKMgwM7kcYtnppWEwjFIRQybXcOLqEEd3VRIMRpmccbH9YCqrspWMzwUpTkunLFvDyWY7t8cX+Y17V1GWo+dmn4P99dmkmOTkJMm53m3H4/FzqtWFkMnoGZxBr1NzQiEjEIzQ2jfH4S05bKvQMjIdJlaYQnGmhsFJD280OAhH4gyMzmM0GPF6PGjVagoKTFh0Em/dmOSh7aVYjYkQIRQswmaS09Dn5lzzBJvW5jE4Kzi43obTJ1GcrmRVjonZJR9ymUhYW901CBBfjEdeCPEXwHqgDPghCfnrF0hMoH8ovhhfe78EdHotS+4QOp2GNYVm5ux+guE4RdlWtq1JxhtSsDrfzJf35nN9halm0OuJBHz4g4nlsGmvmd//6iGOvXUJSZJIT09met5LIBimpDALk1FNTWU2Bq0CszrMhmI1bcMBLnU42LjKwJZyIwPTQfonvLjDOo5syaE0XcLhCvAHj60iFIqwo1xJhjFEQZaF6lyBNxjnL55bzYIjwq3RMJ2jfkxawas3nKwrMXH/7jX0TiWW3JZ9SvbUpDA25yUYFfijKp45VERhhpYjG8zUVuZRXZLM2gINQsB/+a11BCMqOsfD+AJR1ApBPB5neD6MVqdl0eFlc00R+6s1xGUKHtuZRlymZnIxxH3bcpheSSbtn3BRla9l2SdnV42VA5tLiMWhplCLWiUnL9PKhD3RyG8OhO665n0CsjvYPhd4ADgK+OBdhqDxTip+bv7Du4mb/SE2rizZHamzcqU3QDgc4eWrdu5ZZ0IodZTkmFHKIozOhdGqZRzcnEnrgJvLt5bYvbUqUXdXGT95+QwmvZbnnryXExcSFAS/z8Pj91bxnZ80UpWnJhSOcOrmCB2DC4SCEeRyweX2eVxBObvX6KmvtPHK1TlqSwzYTGqK02W0j4Xpm5V4eHsGg9MBbCYNCrmM7VUmFFKQS21T9EzGeHCTmSSDHLU8wo5KLdf6ghj1iSST3VUG/vGNQTKTVJTmmBiZDydovhoZu6st/OTMKFNzyxy7sUAgFOJq2wQ/PT/G6VY7V/tCbCk3IcXCPLwjC0nION3mZFuFEa1ajs8fQKkxsKbQwtB0QoG3f0ZKhANeL283OthaoWXvGgPnOr0AyGUSsbCPU80L1K/Sfzox/RdkyQ4Ir3BVJHiXJXhH+GKMdX4JnL14DSsxbg4E3y2bnFlmYHSOr91Xgk4jR69PrLWX52r5zqsD1KzORiG34PRE0Cdlk2Iz0tk3wdyih9PnbrJ9ax06nQaNzsgLL1+iKEPNmRtTuJwe2kdDJJtUFGTZ0Os0yFVyJpaCjM84qSqw0tAfI46c2UUPPRMh+qejyOUKzt/oZ01pGggr51rneWx3Fld7A/jDsLDoJj/TyvSCh6t9ChRxL3npegYmvVy9ZcdsVuEN2IiHA9gsepr7FvCEZKToIrxwZpLKAiPHG6PotUrWl6dRV5r4f2OxOH//6jB6jQJfIEj3hIyidC2BYJArTeMUZJlIN8WQSTpMqhDRlQZztN7KyVYvZoOKBUcQmYDuoTmU8hhbKsxkWyTaR0MoRIycVB2vXRoGue5TuuOfm0b9UXhJCPE9EqzUr5Kgsv/TnVT8lW/0dbVrme6zs3mV9t2y2SUDBdkVCDlc7PZzq28WrSoPvSpGWooZKS5x7PIELT0zlKxS8erbrWzeUMaqkgJ6x/xs2bIFlVLOdFMfDdebiddksm9DOnMzhRh1SkqzdUw4FOiUYTQaLZFYhN9/opqOYT/bqgwEglFGp2yUZKgozlASjcaZX0qnulBP54gLXyDI9GKQLeUWZDIZr9808uReExd7QtQVK/j/X1wiOzXEkfoU5j2gUKg4vM7I2bYoD26z0j4eY0+VhgvtAbpHFlm7KpWj9VrOd6lYcv88Z+NYg4NnD+QythBCp4rzwpkxDmwqJM2iJDvdQk66iWSLmkVvnDlHhL6xBXSaEiRkdAxModMoUUkWkCRWFaSyucLMxFIEd1BJU9c4EpCdoqE0N5n6EgUKuSAau6te1Z+nnvxDIUnSt4UQ+wA3ibj+zyVJOvsR1YA7aPRCiPXANhKsnwAJ2uy5u0wx/NRgNhvAAh1jIarz1dwadLG2SE/nqJeiDAu9k3bWlVnJtilwuiPsWJvGUkBJdaGOjMw07F64755NmI06vv+zm/zaM19maPA2udkZaFUxnnv8XhTeIUamXdSUWOif8FGQGsaqF9RXpPHyxRmUSiVZKXqGZ/xM2cOMzzjZvz6Fq70+CtLMvN3i5MHNNm6NhvGE5OyszaIg07DilutkR1VC6cbl8aGQW9lWk49ShLF7ZRgNerItEVoGvajUapJNSrxeN5GoCplMzm8+WEnboJ+iNAUGVRyVFGLBoaJnwk/9KhM2s5KBmTDji3H+9Klyjjd5qC+1kp+dgloRJi6UVOYpuT0T4vF9FrwRQTwc4sGdJcw6o2xZreVcZ4Ddq9WcbPNyeL2JaDSOw5OKXCEHCQ7V6jnV5v1UaLhfoJ6elUZ+Rw39vfjAKyCEeFYI0Qb8MaAFBkiwf7YCZ4UQ/3yn8jyfdVTmG7E7/UwuhZlxShSmKSnPUjAyF0Sv07NzTRJN/U4m7HFKMtVEonC2zcmOdWk8uCOVn7x8ngvXutm5azfJyUl09Qxw5fI5nv7SZnZsWUPPVJxbgx6Ks41oVXGOXZ9jbbGBuSUf88t+hsfmmF0Ksr06habbfuZdkGxWs3+tkWM37WQm6xLJLGEJi0nH7poUOsdjjM/7SbFqSTIkvrt3rtbz/KmEGaZKFuVy+yxDYwvMezW8emkYJBiZ9bOrysipFjs6vZbCNA31JWr+7PvtRCNBKvL0vN28iNWkJduW4KHf7Jpnd5UejUrBgxvNHLvpIBaPsb3KQsugG38wismgpyzHSGv3DB3Dy6zKVuLxhugedVKYKpDJZOQlwcB0iDcaHexbq8fltNM/PMPMkp9kY/zuZ9kBkpB/5PZZhhDCI4Rwv8/mEUK47+QYH9bT60kIBQQ+4MPXkkiKmfj4p/7Zgd3uZGgsjFDq+K8/62TPxmJah3wUpav557MzbCy3cLlXMDG7TDAUJRqNMbPsZ/2aXI5fvE0wLHC6A7x8e5YDB/R09/TS0NDE/Ye3cfZqLwadkpy8HFqb55ld9GI2qLky66NhTENWeiq5+Wr0ihDjCx5ahoMIITE64+LtRpCrDTR0TqHeUMzlvjBnrg6wriKNt266mZjz0tobYVdtFjf6JeLIiMYF/cML5KSZqCk0UF9hS8hlJ8XISTNTW6BkcsHHhVE/Nzun2b5ew+vXnGQmyalfW4DDF6d7xEn34AwGjZyRacGS0084HKJvOoxEBJBhMchp651Aqy4i3ari1WuLhMJhjgcsqNSJHIA3Gl3IpBBNAxGe3W+ia3iZodkwV29TzhlgAAAgAElEQVTdpijHRtuIlp3V6XRPRvGGBMHopxFpis/98F6SpDuaof8wfOCVliTpOx/x4R+bCfRZhM1moVqv4mKHk/u2FZCapCInWcnVziXml1wYNFZigTD3bcunc9TN4Q0mvvUvcywuOjm4OQeVQsax6ws8WZdCUBVi744aunpGqFq/n8LCfJxOJ28ce5OF5QAL3hjusILK1au4f38l49N2YvFURkam2FObDsDwlIv+kXn216UzNOXluXur8Aaj1BSqGJ/JID87ieo8JS19MobnwtSV6pCtiDm+2eRgQ1U2gbBEVEqUSchoHQnz1SP5tA4F2FhqwRlw8vUHKmgfDfDA1jTsriBKtYTTE2JVngFPREN6kor1xTqO3XSQYtVTV6xdsZ2CV677WVuZx67VGsJRJVfbpsnPNLN/rZ6WIQX+sGD/Wh03u8Nc61jgcpeBogwNB7NNjM/7yExW4wvGSDariYxFWJVjoPOq/VO645/vRv9eCCG2AiUr5JxkwHgn5JyPvAIrKa1/K4R4TQhx/J3tkzjpzwpuDblJterYtsbGjFOGTqPEG1GxriwdrUqGTKGhKF3JgRoTTYMh6qvz0SthwRnjlctzPLSvkLICKxHXAGcvt3Fg/z5OnzlHOBzGYrEQjbip31BNXqYFsy0Lq0lPIBjmavMk9dXZRGJxorE4bm+Q7rEgVSWpyIRgdAnWFBlY9sGJJgeP7khDKaIMz8dxBNXkZ+jfnXhrvu2hPMfA1goT5Tkabk/56Z0MMzbrpDRLjUohxx9JDF3dYTWFGVp2VOo51ebhaq+PtYUa9tRYudbnI9koY84R5WKHg41lWuqKtfROJgZ8J5ud7F5jIBpJcOUvdbp58mAByVYNP7s0x4ZiDX6/j0g0zoxLzje/uha7J4ZaqeRUq4uvHs4lM8XEziodbzU7mZhZ5MVLc+xda+Jue9Z+kZbsVsg5f0gi/AZQkSDnfCTuZEx1jETK6pskVG2/UJidXyQYVrCtOLGW7XT7OXcryOZyIx0DAV44O8EfPlEBgE6jZH7Zjdmop7ZQzV8+f5m62jLONc4nRBeF4NLJ16jbFuGeQ4f42UuvoZBL3LtvHcffPM3lLi+/9ug6nC4fP3urmXVVBQgh2FRbQP/YIF2jPh7ekcayW8e/nBtjX102AH7vMsvuKKNzWuQyuNY+iVql4N76XE7f8rKhUMIblrEhQwkoudoXYmeVhhfOTdI5tMTW8sT5ezx+rnT7qVkR3AyGokzNO3C4Alw06InGYgyOLzK3pEKjUhEOh9hWmYdcpqS/K0T7sIfCTD1JRiWRcJAbPQ6ybRp6Rh2EJS23x5c40aKlIkvJTy9OsaM6nXA0TmmWmudPDmPUKhmdVbPoinC62YtapScz1cL49DK9k8ZPYZ0evkA9/QMkJOje0aGcWRGg/UjcSaMPSpL0336Jk/tMY3x8ipmhOTz+ZBRKJXNLXob8frSaXDJT9SiHXJxpWcJkNhMMxfD6AvSNzCGT5bFzfTE6k5b968zvHs8TKGZVYSq3exrp7uogEg6gUkS50jxIZfkq3ry6SDQaobFtBLVGR+fALFIszpnzndy7s4IbfT7ikmBsxs3grMT4oo9FZwSzQY1FD0atmpllK1PzHo43OhifcdDaHWTX+myu94eISoKG9nFkIofSHANCJqd3ws/QgpzZRTedA168oXz0ujgpRkGaVU9WqpU9q1X0TXjJ2JTHnCNGbb6ge9zPm40OjAY97X2TJJk1rC0WNAxEmLH7mFzwsb48g7pSCzf6vRzalENWqgl3IM7w5DhWswlZPMjGcgur8lNJT1JjM0q8dGGSb3ypjFSLmiVngKK0dPJSlcjuNg1XfHFouKyQc1bUeD9xcs5/XRlKnAHe9b9+R+n2846NG2qYUo6i1WqozlfxmldLdpqe8mwV0/MBntyXS9tomIpMGSlmFT1jUfyhCBVZarqn42gkH7NLATKStTR0LbKltpi5QJAjh3YyOOVAb7Ry+OEnCGDEtbTAY48/RSAQICJpkILTfPnQak5c7GZdzSp2bS/DoFPR3DHNpvpS0pLkVObqcfszCIfCZNj0DEx6yErWEgpHObzexNm2GBMLCupXYvtFR4CpND3ZSTI6xlQ8tdfGqzec7F+vRsRNqFUyijLUrMpWEQxHWXDG8QTChKNxRhYkDq9XMbtop3s0QlmelcxkHTd7XdhdAaxmLcUZKow6FfNOG0qlgs2rtMTjcVRqDVtXGzh5y8ehWgONqWaqC3TkplgIR2PYzGqikoLucTe/92g5Z265+dJmJS5fGK1awZl2z6dzwz8nw/c7wC9MzrmTK1BFwpziWyRELP8G+PaH1iBhdiGEuCiE6BNC9AghfnelPEkIcVYIMbjy27pSLoQQ/00IMbSilFv7nmM9s7L/oBDimfeUr1tR4RlaqfsLqazVlxkJB310jIUxGgzcW2/jfIeLGXsYs17BffUWGga8zDvCDC/Abx7O5XqvG6QY29fYaOpzArAc0lGYayUcCXHi7A027d4HMhnHj73B2vUbOPLIY7x27ASvvf4WX370QR554uv8/Q+v4Xa5+PoTGzl1fQqAsfkgDx2oYM4tcaF1jtIMOVlJMDobYGAmxpo8FXXFWloH3eh1Wu7blEzjUCLGvj4Q5Ms7M7nW7SY/NbHktq9ax5UeP6G4iucOZBMOBTjR4uZ4g53NZSpUhHj5yhL7VvT09tVaudHrwqCR88q1ZTJTdGypLWBDmYWxRQgEo6SaVdj0ESaWIpxscb1LY7ZpwpxvnedgfQZtQwl33FMtDupK1MTiEBU6rAYl924wcarNg9MTZmLeQ2WeAekuq+H+fJ3+l+feCyEOCiEGVp69T9zJ5qOw4hvxCgkNynfIOf/9TureSU//AFD4cZ1j+GCzi2dJWP98a+Vi/RGJCYlDJJYAS4B6EqYV9SLhD/ZORpG0cpzjKx5h/0BCILCBhLLOQRI+X3eMufklWnqWUSiV9HeOoVUp0KkyyLDIeePKDDKZhDso0Gh0fPvFHjZU5XGqzYfd6WVkyo5MpiAWcPOTUz6OHEiI+HhcbpxRPRsKC1GpVPztN7+FwWxKJKu4vfS03yIYA53Jgl9Y6BkeR3NtGpNWQUv3PDazCqc7iNGk56XXO9m1sZhoRPCTkz1sqMjg9RuJAVdT9xS/+9Aq0qwaeiecTC/LsBg09EyG6RmeAyHhDiuIx2VcbRslO9XIlT4lMqEiFvXRPzyPVqVkZjGI3enj6oAFhTyOXEjMLDr59kuD7K0rYNIeZ2h8iSRjOhMzdhp7ozyzLwulQsuxRjcmnQaDNvEorS0x8c0X+thVm4pSAa3DIfS6hIT3/LKL0qwE81GnUVKZpeDtJjtpNiM1xQKJu62cwyfS04uEuuYHGbB8avgwco4Q4qYkSZve7707afQdgIWPqeG94vTxjtuHRwjxjtnFB1n/3Af8eCWJoEEIYVkR0dwJnH2HAbjyxXFQCHEJMEmSdHOl/MckzC4+VqNPT0tmnSkJhyeEXJaKSadic5kKuytOa5oR5Goe2Gxm1h5Ery5Gq5JTV6rjzRZYVaRjZ5WOK+0+WruWSOuap613kTfP97DrwGFee/57pKWYSLbqyCstASFQ6HR09/TxwFNPI4QgFg4jhfK4/+Aqmlp6+B/f+ymb1xXg9wc5uK2IxblVmNQxNlUbGRizsm+dDatRSTweZ2bRx7WuZZRqDTqdjr97sYe1q7LIT9azd1MZy24f9UUKVEo5Xn8OMgHbyxO9/+sNAdZXprO1XMf5jjC1JRb0OkF5duLYl1os/P7DJei1SpoGnOh1KjaWahFRH2/dWKRl2AZC0DM4TbLViF4rQyChkIM/EKZxMExUUnLqcjel+cmcvgWtnWMYtCU4fAGETCCQMzS+iFop4+0GHzIZ3F2L+k+MkfeuAQuAEOJFEs/vp9roPwKaD3rjTq5AGtAvhDj9iy7Zif/T7OL/sP7h57peH9fsImvl9b8uf7/P/1Czi3A0zvlOPwdqDMQQBIJRLvcG+cZ9hchkgjlnlMahCNsrDdi9EufbnWwq0xKLS4SjUebcMo5uzmBVnpZNVSYeuaeSmG+BX3/qKE6Hi7/6k69z5fRJUtPSuN3bx6NPP03jtWsAWIxGnnrm1zh9oZ29OzfwpQcOYzSaOLKrhNl5F4WZahZdYULhGBsqs2lYGcY39Dt4aHs6MqWWA7VmKjMldm/IpyTbSG6qFpU8ytE6M2favYlMOq0ckzrKzHKEzhE3VXlaDtRauTUeRaPVs77UxPSinxlHlFNtLp45mMfQbEJ11+6VU5mjYckVZtat4NlDBZh1cjaVaqmvLiAjxUh1vpZN5SYsWsG2dXnkJCtYm69g35Yykq1GDtTo2LN5FQKJXZUqdpYrSTeG+fKh1dSUpmA0WT8FGi53umSX/M7zsrL9xr86ygc9k58lfODFvJOe/i9+mU8W/9bs4gN3fZ+yDzO1+ETMLgCONTi5v96MEIJgKM6bLT4e3GRmYt6LzxfkO6+Os7Ysg4ZBJYOjM9isBvbW2oiMB3n16jIP7UhFpZRzunOBcAwev7cKXyDM93/0IkajGY1GzbbqAtoaGomEQpStruDF53+EJJNRWVmBTCYjFDfgcHmwmnVUldXR2tvNwNAMR+uTKM3Scb5lAY1SkGqUGF+I4PCrSLOqOVqn5ESbl2gszgMbLbzR6KCl309+ih6VQkZRqsSPTk9yZFM6qRYrJ1s9xOMy7ilMLFE6PBEspkSnsGetiX98e4r8LDO5qXomloO82ehgX40ZlULPP5+ZYmdNKoVpSt5u9RGPhihK05KfauHtVg/3bbTSPytx//Ycrnc7aRrw8tD2VPrGXZxrWyYnWUMsIuibilCSLuf2bJyj9Sb+/tURntyX86ks2d1hPs/Se5xo3w93/Ox9FvGBjV4IIaQELn/UPh/y/r8xu2DF+mfF4O+91j8fZHYxxc/DgXfKL62UZ7/P/h8LFy7dwKpRce12DIhz7mY/B7aU0TwUxKaXJTLiFkzsqTZjNamYXbIxb/fxRsMy12+Ns6W2kLOtLuJSnKHJRSJxJa+r5MjlAofdTb8zSjSwRDgq6Lw9TUZBKW6nk6179vDyD57nyDf/CoDHn3iaP/kP3+C3fu0w2ZnJ3OoaQEgSQsCyO8jw5BJWnYwHt2dxvMmNxaCmdcDBjFtBe/8MaTY9F3t12AxK3rw5Sv3qNNrHI2jUanqHF0m1mVEoIzR1TZCZYuR8t4pYLM6y00fv0DSSVIxaFmV7lZnz7cto1Sqml/zkp2m52rVMRFIzMedk2pGC3e3DIPfTMhTjuQMJV7LiVBlXuxxkphiIS3Dq2hB1qzO40etNDPNvjrF1fRFSNMyS08Gltjg2q47z3UFkMmi6HbjrVnYSEP9k2uaHGbN85vFhPf1FIcSrwBuSJL3Lr18xnNhKwlziIvCj96u8MpP+b8wu+GDrn+MkPO5eJDGR51r5YjgNfFP83PNuP/DHkiQtryQZbCQRNjwN3NHs5XtRXl6C2ulgTb6WqUU/up1F+CMSm8p0RKNx3m718JVD2XSOhymTQYZNi1oJO9eYkCuK0Kjk7K9J6N6/FQ3ijyrYulqPyaDmlfMeyksyuG9XDvF4HIfLiy8Q4HZvP36fl6mpKX7w/I+QyQSSJDE+OU1jSw8daiWRUJTe2xOkGMGoUyCXy/GGJBpv+xkcm0elEDy0I5skU5S8tGKmlsLsqdISiag40yRjb20qWrWC060OvnK0lHBUxpp8LZKURyQSY8/qRE//dkiFL6hDJYuxsUyDEFqcITU1+Ur+9FI/qeYCNldYsHvCBANWMi2CrCQ1Db0++kfnee2GFq1aiUwu53zDEDs2lTPduIjJYqAsP5lVuVqi0TihcCUyJHavSaZzaJnXr4zz2N48ks1KNMpsFpZ9K9Zed7fD/IRGE+8asADTJAxYHv9EjvzJIeeD3viwmP4gCZ/4nwohZoQQvUKIEWCQhCXu30mS9KMPqf9BZhffAvYJIQZJzH5+a2X/E8AICbfafwJ+C2BlAu8/k7jQzcD/85603q8D31+pM8zHnMQDyEhPYdKecGJtGQpRX2bAqIoyMh/heLOL/WsT2WXBmIILXX7qSjQcWmfher+PZIMMmy5G/1RiNl2h0vPIjlTeuDxJJBrDbLEQD/vw+YP885ujfP1rXyXDZmJ1bQ06g4Fnv/FbWG02nn72OXyeef72r76GQa/mwI7VBLwOSguzqC+3IsVjbK5MJTnJTDgc5ejWPAqykzDqFHRPSVTnq8mxxhmZC3G6zcl9W7KYc0QYnfWRbNVSnmti0SvD7QuTbIDSDOhbOWeVRk9Jfhr5yRJvNLoJhKLEJDln2z38+XNrWPbG0WkVtI0EeXBrBh0jXlQKORqVgt97dDWRmMT+WiNr8+Xcf6Ca3CwrRr2Gf//0RqacMaaXQgkTj0oTkXAEbyDKyEKEP/vKOhoGfAzOhmjpmWRLpfmux/Tv9PQftX3kcT7cgOWzgqkPeuPDEm6CwHeB764M05OBgCRJzjv5xA8xuwDY8z77SyTcOt7vWM8Dz79PeQuw+k7O58OQaojQM+4h3apECIFOJfGDt/qpKEhhdE5FQTpML3gpy9Lh9IQZX4pyvmGY6pJkvrQ9k7O3PKRbZOi1cmQyGUfrLXznZ51UlGaRkqTnT//uIn/77f+MQa/jqYcPcez8Bex2Ow899SQnR17n7/76L3nm4Y3YrCaWlv2cPNdM3SodCqGic8TN2EyQ0nSJ1q5xkqw6AtFUQPD//ribfXU5TC34Kc7ScaLVQ5pVw4ZyPVd6/Cy7I9y/KUHU8nl9nGuPcW+9DaVCxms3nRjVUTKtSoam3aRYkjiyXs3xRhejM8s8sKMAs17J4fUmTt/yYDboEEKwZ42eGwNBnH4lW5K1fGmrktdvOFBq1Dx2IIM3rs6jVGmxmDTcu7OQH7zaQTgYQq9VcGhTGqealzEYjCjkgrIcHS+eHUavFix7wtz1ZXo+OR75BxmwfIbwS03kIUlShJXlty8alpYcOIMaXjrXw66NZVzrD5GTpKWqOJPV+VqsBrhwa4mOvnki4VQCAQ1rS63sqCvF5wvSMRZGrdHwzR93sKoog2BMgU6jYHTGy4G9BXgCMTKLrPyv1y9g0qqwGjQMdA0gKTScOfYGvW038drHePNEGJM5ie7+IWQhB9tqM/D4o7z8djfbaguICyU1lTmoVQr2V2sBHf1jy6zK0bHk8tM5EuFW3wLlhelEJCWnLvdTW5HF69cXicei+IMhJue9pBhBr4Zsi8QLZxd4eGcmZnWEH52eICsjBVuSgWvtI7T0L9IzpkGuUNA7NIcQglAkg6Dfx7IrgMVipPm2B39YgccXxGP3cuKmke7+KfyhKJakFFRKOVu3rOd6Ux+t0zqCoRgdg0uoFBKhGKyrymBjvRZFLIhJG0Emg3jsbt5t6dOR2f6M4wtDRP5FkZxsJTUoMVOcTllWIpvuUucye2ot9ExGWZ2nRjsV4Q+eWMvNfi/ba6wEQlFsRjkFKVokoWBDsYIlVy6FOSnsWpfMtc4lnvzSDpKSLMyMxSgtMLHpnsMYjIl8iLcu/SHb64pYU1ZApnKGgSHBPZuSWHb6uN3lIxqPk2qIY5DHyLAZ2bpKi1GvZNYhEQn5cHgUqBSwrSaD0SXYVpHMpMPFHz+7jqbbAfbXmnB6irEa5OxanejpT7faCUblVBYmEY7GsbvC+ILzgBxPEDRaLYfXmwgEo6hl5fjCgr3VBpzeEBpVFi5viH3VeuQyAyebZukdt3PPBisGnQqn10BVRhKVBXpkZBOIq7E7vNSszmF1aTqTMw72bs7njQuDPPrQQewL0+yqy+S1cwPsrsvmxMVBslMNd/1eSxLEfnUa/QfOi35hiMi/DBoHQzy9P4v2YTeRaIxQTE2qWYXHG2J6KYDRqCfNqqQqV0H7SIAzrXZqizSsLjAxNO3llevLPLonFykeZHTGS0hmY8/WCprbRyivrOHRhx+g5foNAF796Ys88Tu/hV6vp/HqW2xZX8D9Bzfw42Pt3Gga44l9udiSrKRYdTT0uvnt+/NpGYkwNOUm0xJnz1orbaNRLnS6qCnQ4PZLXO5ysW6VFYtBic/nY2zGTXmujjSznI6xEIuOACaDnge2pjG8IJGVouf2XIzffbgCV1CGQmNhc7mJ7okglzrsrMnXIItHWHCFOdfuZ9dqPQdrTdzoDyCTCYTSyNbVqbgC0DnsojJXQywucaphnoOb0tCoFDx1fy2RUIB/Od7G2MQcPz7ex7ZNa9i8vohgROD2BDDotFjNGjbXZnGhbQFJAiG7u4/kJxHTf1YghMgTQuxdea39V1l2T31QvTvJp//Ge2bOv3C40XiLeNhLc98iOdYo3/7ZbdKMYWaXfKiEnxcvzpBmjLDsDpKXpmd0zkswLDjTYudUe4AZe4ipRS8X2t2EYmr++4vtKFVKxqeXGZ0NsWHDOlQqFV7HMudPnaJgdSWSJPHSi/8LgcTx8/00dy/Q0j6MBEzO+1EQ4lzTFGvzNchkMtweP+2jQYozNAghCAQDCLmGRWeQtr4xmvqXaOpborFrnt1rk/jpuXEq8vWU5BoZnHTw47OTrCtWk2rVMmf30TrgoDLPQFqSltbby2TbZBRnGRiY9BGTadFrlWQny/ibn3ZjVEeZXw6iVsnx+oK8dm2ezWUaNlVaaRsOMG6HnGQlp6/0cv+OLIQQRAIOfP4g5UXpxCIhOgfmcAaUXLg5hN8fJBiWOH5plN11CdfygtwkrncvfSD54pOCBMQl6SO3zwNWkmxeAb63UpRNIg0eAEmSut+vHtzZ8D6dBLe4jcRk2ukPW5v/vCHFloQnoCE3TU8sLiFJDiYXQ8QlmHOGiQOBiBzPcozgrI/FZR9CJueR/eUsu0OYzTrcvhj3bU9nbtHLsieXitIs7E4fo+NT/OiFl9DrdHS1t+MKR6hYU4UtM4Oi0ko21K6iojRB5BofGyHZHGZh2U8gqqShZZC6Nfl0j/uJxaF3eA69XodcLicmVAyNzFKSmUdVaS4oVDy8O5sFu5/hhRDuQIQ3Gp3o1HKCqFFpNJxsWkCu1KHRaDl2dZh926pwDcdx+aOcuDnJxFIGKpWKxo5hzPpSMq1KCrJTyc/QM7XopaHfxaIrjNMdwGiyIESIztszGDRKnp9ZRCXinLs+ikqtx+8P83ffP8fa1fk8uL+czPwq5hZcPP7Ew1y/2UxLzzRWXZhzTQvE4xCNxvB4A4ke6K739F8Y/DYJOnAjgCRJg0KIT8a1VpKkPxVC/BmJ9fHngP8hhHgJ+IEkScO/+Dl/NlBSnMdSqJ15j4LybDm76wtYcEXYUJHEgl/H04cNHLu2xENbk4nFJJxhNXFJoFTIuNbt5pF9uRy/PIbXF+Jql4tnH1jNhVtzpCbr+f/+49OcujrEoXueZcGxjC8W596nnmBscJC1OXk0Nl0hI82E1WwkN9PG2NgU92+0Mbs8w866ArJsWsoylYzMeAlFbBRmaCjPVvPaDQdlhWkUpGuY92moLzdwuX2ZPeuSMegUHD2wHpkEmSlyMpxmKgt0XLg5zb4aE6dbltm7uYjcVDULDi/PHF3N/JIXlUxibNbNbzxYSVO/lw3FRtaUpDA4G+bIhhTCPS5KijIYn/Vxz0Yb0WgcSSbDqIyz7I3xtftNvHrNzkPr1LzV5MOsV3NgayGN7ZOsrd1HSnISJ05f5OEHDzM+NgnhBY7uKQXg7Qs97N9SzLmb/e9Kf90NSJ+z4ftHICRJUvgdhqsQQsEdkhzu6Aqv9OxzK1sUsAKvCCH++hc63c8Y1hYZ8QeC/PDkBNX5GmryVZxvs5OZpEClULCv1srJFidvNjvZX5eCRhnmxydHObItEyEEBzbn8NLZEWor0xBCsLS0wMiki4K8dL7+5FZe/9mPiCJYt20Lt65dp7ehmcrVq3n6K7/NS2+2E4/HkYk4Dx2u5WzrEjqtkgP1mcw6IkwsRuiaiPL4nhxml3ycbJynqtDE4c2pvNVoJ82qwGLUII+HmZz3c65lkZ11eSwse3n74jA1JSZ0GhV6g5LuUTdGk5F9ddk09y7Q2mdneNLO9JKfEzcm6B2xM+cUZKbq+ZuXbtPUNc784iI/PD1FdpaNmiI9uSkyJub8vHFjkX3rkukaWWb1ShiysUzDd44NUVGUwqH1Fi41jjPtkFOQn43BoMNiUDIwOILRqKdm3UY6+udxewNEQz6O7CxOxPS/WHb0HSMmSR+5fU5wWQjxJ4BWJPTvXyahbvWRuBPd+98hwZxbIkGE+b8lSYoIIWQkiDr/4Rc+7c8A7HYHfcMBlHIVEws+3m5zYzHqOXtjgEO7q7ncF0MIBTP2IC5vgLO3LEzPhZld9HKp048QIJcJhiaXKS4MMm+fIc0co314jh/85Bx6g4k0m4k3rrSh1uuYmpwiM9mGXJ7Qq3vw8d/g+Re/S0mmgt7hJU5dH2BrbQmn2nyE4wpevjwOksTVPgNhSc2ljmnQJTFmj9Lct4DQGBma8lCapeZaxwJqnYHXz48xMuPHqFbRMuAmEhfE5QZeON3Mrs2VvHZ1hok5LwUF2WzbXo1Br+GVk91YdDF2b0gnGI6y7JehlceoL1bw43Mz9E8GmF4KU5im4+KtRbLTjNzs8zAx72bWX8jytEAltzA5P0THoJ0ki5He4XHWb9n77rXev2cT//CD18jMSGHD+mq++z9budHcz45qKze7lhDw7nW5G3gnpv+C4I+ArwBdwG+S4Ax8/04q3klMnww8KEnS+HsLJUmKCyGOfMwT/czBZrNSrddyvNnDN39vN2ebl3hwTz4xuYYkk4zNa5IB8Ibi5CqUPLSvkFcuzFBXn0x+ppGCnCRaOseYWXCxbpURo17FK2eHEXIdv/7sI8hkMv7llTPUbqhj94P3c/q117l1+Spv2ZIBCUmChtbbzE0pSDLr+PNv7OVKywGmlVMAACAASURBVDT37UjE+i9JAqteya5aGy9eWuAvf/8II1MuNlWno9SaCAQCPHKwiLFpF8qFSaYWA/ynP7iP10+24vE4WVdhRq2S8+Pj/ayvLuDo9kx8/jCZOQUsOdwY9BocTh/5ueksL80nPvP0MI/syeXNS2NEYxIb12SxscJKPB7nH48N0Du6TEFhHvfuW0NInoRcHmB7bRpLDh8PHa5BRKNsqrSytOyioaEJm0UNCKS4xOjYBCNTdoQuFfTZDLY0sWZVCt6wHCEESsXdXUX+AsX0WuB5SZL+Cd7N8dcC/o+q+JHDe0mS/vxfN/j3vNf3MU/0M4nWQQ/rqnLQa1Vk2+T0jjjJTDMxbQ8jSRIjUw7KClNItSroGVogPzeDAzurudw4TDwe5/aYg9/5yn6aep1ML3gpXFXLM4/u4lpDBwABNCjlcuLxOL5lJw8+9yyZGek8cP9RVleUUb8mhzSbgYoiMxkpeqrLkmgbcHKrf5HVpSlIQsHxG4vcv6+CjFQz0wuJ+6rXa9hWV8iN9gUKc6wotRbWr13Fm2daqK7M4vEHtnG13c4Lbw1wdEsGanmccCTGySYHezcX88ihtVxoGOXczXG2bSggEoNXzg5zaFMmKqWc+3YV8LPLc6Rb5Zy4OcvZWx4e3FvKM4/twxNKGFgkWY2E4yqWnAHON86wtSaTZS/0jjioWp3HtppkCvMy2LejBpc3yrNf/XcUFhaxe+8+FDKJjZu2oVapsOoFksRdjemRvjiz98B5Eo38HWiBc3dS8Vd+nd7n9eMVJsryDITCUdJsWr77wnXSklRsW5dL+20nbUNBaspT2LY+j+df7aCsMI1IJMqXDtfzwrEWCnOSkMlk2D1RmodlHNxdS3FBJn39A7hcHkzJ6UQiYd7+yU85/MhDVNSspaGjA6fTxeWzr5CfbaOtcwiHK8iV1lmWnGHONwxx+sYYMmJcax1mTWUeNmtCzqowU0/f8BJ6rZyCnGTcvijdtxcoKc6jIDeFt88209E7zdsXujl+roMkgxyDTkFJjpG2vnnKSvJQKuUImeDC1W7c3iAXbw5z/lon0/MOLrXO89qlKd68Pkff4CJv3ZhjS00Ghzam0tTrYHNtLptqsrnaMoFSDg8dqePKrWUMBgMKuYxF+xJXuu2sLjSzrTadcxcbeOXtZirX76W0tJQHHniAK5cukWTSUVlVzYtv3iLTpkBCQqlU3rV7nbB4/eifzwk0kiR53/lj5fUduYD+yjPyWtp7kEU8/G/y3js6rus6+/7d6R0DYNB7J9ErCbAALGCnSHWbkqwSO3Zsp9jJ+lLc4texvy/F/uz4jePEji1LltU7xU6AIAGigygkiN57HwCDaZiZ8/4xkCPrlSxKMZVYeta6C7j73HNxZw32Pfvsc/bzvFA5glIOsRFGsvLyae7zERggp762D51Ox+uXpzAH6NEaQqi9YcPtmsPr9VBdP4BAwdD4Ch1dU0RESjx/sgmtWkFkWABf+ur3OX7iYZrrGoiLiaLx7DkEoFGp+PxnH2NbUQozcza++NgBkuL8Ky7nq3tx+oykJEcRlZyL3jxPXccs4wuwurqCUeuh+uIgX/qDvVTU9rDmlvjpi53sKgtkfF7Opz/9GWwLvezamsD42CgKpY+XLk0SHqzhSuskB/bG8crFXlZW7WTmbacoP51txfn0TzhIi/KxNcv/HHanm+7BKdKTAqlqWyI+VIPaGMyi1UZ33xRXWwYwmkNZcWuZWlayujhBoCWE6Lg4Wm8Mc7pmDLcH+oamUY46kNRhDPT3oVKrOXPqNAGBRlbJ5Itf/CP6+gb84f1tdHr4SIX3a5Ik5b9JUCtJUgF+rcn3xMfe6XeXlXCz6RSFebHERxp5+dIkX/7CvZy+2MyJu3czNDpLQUYYe7alAKAJiGdq1sqnH30In8+HOTACFQsc2ZODwRiAT9Lw4CePA2C1rvDLk03MaxVkFBehc7v59EMnkCSJ9o4OghXLqORubLZlLIEGFpZsvHF5iH27C1j16BA+QUR4MKU7tzA/N8e+nZvQ69RMzy1zvnaM2k47B8vLOX2xjaLiEB597GFefuU0u8tKeOnlOcanrYQGm1h32fnEgRherRplXSjYW7YVrVbDvz9bzxc/9xleffUk9Q3XOLSvhIbGFqwrTswmDS+d6+ULDxbS1z/Lvk3BPHFqgJmlOTanRFNaksmqW4M5JIlP3HeEnz3+LOaszaREeRkcXqe0OIOwIC0ZycGcrJ7E5VVx7NA2VCoVDoeDc5cq2Vq2m5G+mwTnbKbN7q+nV6nVt+27Fgg8vz/h+3vhS8ALkiS9WccfAXziVjp+7MN7gCNbLbS1D1PZMEp6Rh4BRj0et4u1NQfZmZtZtsuZW1zlRvco8XFRlJZkU321kZOnL7JzRwlzS+t0942RkhCKXr3O5JSfkusXL13iuz/+MfP9QwSZA9ly/BgvvOTfNHWt6Qr33bkLU0AwbdeHqW4ZoW3AwWcfOURSfDgBJjMP3n+QyppuVCoFn3n4KC+f68Dn83Gqqo8ffe8reIWM85evc+DQEYKDAvnZ489w1x3+bPk9dx/jYt0YcpmP9XUnZ2snyM/P4Ft/fohfvVzNy6dbuPP4ceRyOcsrNrp6+0lPi+exT93D+fppqptGKM4JI9isZ3R6jRerZjhxLJ/9O5LQaNSYA/SYLbHY1tZwu92YTEbuvecYddetjE1aKStJp71nlhfOdXNwdw6fPJLNG6cv4PP5+NHjL/LHX/0KPd092Bbmqalt4aF7SxECVLd7pP+IzOmFEE3AJvzl5V8ANgshWm6l78d+pLfZ7LzSPoXOFEx1yzQ79Vaq669z77FS/v2Jk+wtKyYro4wf/fuTSAg+tzMBuVxOTf2reH0KwsNDKdq6jRdf+CVf/dL95GUm8FpFG0KmYOeho4yNjlJ/oYLNqamsLC7iEz5+8M8/JDM5gLOVzThdgq7hBUIjormrKAaZTMbw2DTRUSGo1Wqm51dJjNMzOT2P3enj83/zS8r37uRMRRM3u0fwKbTIdM2MjM+wvDjLqXOX8QkvwiuQlEZaOjoICwmkeWwRS5yOmR4vBpOZK82DCF0rSsV1JqdnWVuZ5/SFAHwCXD49F+u72F6cx+iil8sto9x1sBC7w01JbgzPnPZvp83K2kpcdAS/evY1MtP9kdCnHvwkDz7yOTSGQAKDI6hp7EYfNIV9dYnxyWka2rrI2rqN+kuXkSsVtN9oJyNpD3UtPQi47eH979Gc/VaQBqTjJ8HMkyQJIcST79XpY+/0E9NzGAJDuXv/ZoLMGrbvKEAmSfzqpcs0XOsmKSmZielFzIGhXK5p5PFfvoZWb2Bscp6lNRfPn7qET/iYXnTw8rlO1ErB+Yo6lEHRzAkNoTExbNtVSpBSw8MPPcTIyAhf/9pXyM35DLtLy3A4HEx7DSQEWxia93Kl6RpNzc0c3L+L51+vpWdknrlVBeaoQiITC3B5VTzywFEkScLu0bPiXmfH3ffgOXMOeU8nh/cVo9X6Oe/+7ae/pGRrLosrLv78+BFGJ6wc2b+VkbEpOkds7L3zXiRJYmzeSkpSHIcP7gPgx+MzZGblcOfhEi5ebufb3/gyV2sbGZr2cLVjCJ/Xxa9eb+WBT6YwNjbNhYpqtHoD7V0juHxQWLyHnOxEjHoV01YZ5fuPEh4eRlv7dX7yq+fYVJBLYHAwL/38F+w6cjd5eQmoVEpkkoROd0u5qA8E/zr9bbv9hwrJL0CzC7/Tn8ZPIV8DvKfT37bwXpKkn0uSNCtJ0o232L4pSdLE25h03mz7mw3hgB5Jkg68xf6OogKSX1izYUMA47kNGq/3jc2bktmWHcgvXmklPyOKG50DqFRK7D4tBSVl7LnzEXYe/RT60FQsYTE89tin2LG9mMTMXFLSNrH38CFsdjvZO/ays/we7jzxpyRml5C4eTM77zhKUmYG5uBgDHFRdHd309TYyFe++nXkShVyuZxfPvscZUcPMzEzQ3n5AT712BdZVwZSuPtuDtz7KPHZBWzOzCQrOxt8bh544AFqG65z+mI923aWUVy4he72DuZnpnnoj7/EUy+cBWBgaIS0hCA8XkF4eASZmxMJMmu5fLWD6oab3HXPPbS3XGNlZYWIyCgW19Zxu928/NpZDu8r4Q8eOsbpynasNg+b0uIpKy3B5VHwqXt2EBMbjTnAREFeOpJcYlNeAXm7DnD84ccQXsGff/nPGBxd4GpTP1/+0p9woaIKSZKob2kjPjkZY0AA4yMjJKemsf+ee3n1fAMKhQwhgUr1gb7GW8ZHqMruXvxkNNNCiMeAHOCWEiK3c07/C/yUW2/H94UQuRvHaQBJktLx84xlbPT5V0mS5G8RFTiE/412YuNagH/YuFcKsIR/d9IHgiVIzwOHEnnjUjdVNU28UT3AQ3/4eXR6A263m4baqwQbdXz2D/+QmtpGXj1fw96jRwiJimB8dAyPz8fh++6l6soV6urr2VxcRFxqCjcam6h45VVytpeQtXUL5yorUSiVZGZmMjIyitvtRh1oRqlUsq6Qs7CwwH888QRf/MZXaWxo4LnnnqVg3x5W1mz88onHufPYIWJjY+gbnmZ2yUdsXDzZ2dkM3+xGLpOjUqlIKy6j4nIjFRWX2VaYQlNbPzu3ZQFQXLgZ97qDm70TZGZlMdTXx+svvcTOvXs4cPe9PPnMa5gMGuJiwlhYXKb9+gAdNwd44fXLdPePMW918g8/OkVQkIXPPbSH514+g11u5HN/9me0NjVTd/kKu7aXoNFosLvkKDVG5HI5cbExdPf0Itfq2H3sKG119TRXXaZoxzb/cx25i7/99j+DAI3mXena/8sQfKS24TqEED7AI0mSCT/BbOKtdLxt4b0Q4soG3/2t4DjwrBDCBQxJktSPv4II3kFUYEM4Yw//SUb4BPBN/Io37wt9g+MM9rnQm4JITN/BUEMnDpebm9dvkJicRNXFi2iVEunZmZw8fZ6Lly5xx333Mz8zw849e/jRP/4TZUf9Acuy20X1tRYOP/wgbqeT8y++wtjgIC6HA6PRxJrw0n6zE+XrfiLJH/zoXzny2MMA7D5+BydfeBlDeDjGABPTM7P4dGpkMhmVlZeIDLXwxHOvIFcoabzWRXRkDFdraigsKiIlIZGahnoAdDoDPz11hRAdPHOqi/EFF1eu21FrfCAEQhlLz1g1Tzz/HB2trQivj5eeew6VSkXllWbuvf8TvFo9jDkwiKD4bPQqFWUH78K9vo5obaXx5gv0T/roH5viUkMb+8wRtDe3MD8zg8VoIDQrgzNnz7FsdzMxNkpzSyv5+bk8+9xLKENDMQcFUXOhgsjoKC6fPYfL5cJuX2NidhWQ0OtvWYfxfUMIwfrtpeYB/BEtfim4N0UWvvKWAe5v8A9QXuBPhRDnPuCfaZYkyYyfT7IFsAGNt9Lxv2NO/8eSJD0MNOOXvVrCLxRQ/5Zr3ioe8HZRga1AMGDdICh8+/X/FzbECj4LEBsb+xttGmMYcRGJ7CzJZ3BkDBGaQGZBPt3tHVRVVDI40Mfhu+5krXeAtNKdNN/sIr1kK021dcyOT9J2/Tr6sBDq6uvo6+5hfmmJ1SAjcoWCgdFhVmamOfbwp4hKiAeg/vwFEnft4OzTz9De24P61H/SrF04fYryI0c4+cKLVF68QGRSAnaNCm1UOGk5uew54n+5WB0u9EY9xqQ4fvarpzCq1FxrbuGV558nIj6OL3z9qyx13mTd4+ULXytlYWiE3Xv9tIQ/f/xxNuXlUHz0EOMz04QFBXHovnsBWLHbUao17Cr3X9vWeQOry4nBaGRpaYmxuRn+5JvfZLHrJttLimns+QZFe3djXVziwo8qyc3LQ9XdQ8TmzUSoVPj0OmwBZp54/TSXamoIjIykvb+PvrER7nrwBIlpaQC89ORTlBw+yqVLNbd1Tg/g9X1oK/Xf39Cb+zXeFtFGAhclSUoVQtzym0iSpO1CiKvAlzcGyX+TJOksfrWnjlu5x4e9ZPdjIAnIxc+5970N+20TugC/2IUQolAIURgSEvIbbdFxsbR1j+BwODlb2UB6Xi7WxSVqamuxqmTk3nmUmfkFouLiqb1YyR/91f/DjaZm0rKyWPS4uOdbX2HJ5SD5UDkRm1LY89hDyDfkq5Lyc9i0vZhLdTV0tbYC4HY4USgULNjtmJPiMW8vRFeQRc/YCAWfuBtiwrEqJMo/+yihyYmotRoiYmIYX7EyPTEBgMGgZ9lqxWAyccdDJ5h2rmFOimNybhZLWCh6o5FV2xq9Y2MkpKYwMT2Fz+eju6sbQ0wkCo2G5cUldGEWZu1rrFittDU0klO8lYHREexra/h8PpQmA3kHyrlcVcWvnn+OA/fejTkokJHxSV5+7XUe/bM/oa2+gbDICPL3l7OukJOcsRlLWCjjA0NoVBqiYmKYtS6x70tfIDorHWQy7v76X9Hf1QNAV3sHsZtSKT16BK/Pd3tHet47tL/N4f2vI1ohxBB+Fuct79Hn7XhTNr7uTYMQYvhWHR4+5JFeCDHz5u+SJP0UeGPj9LeJB7yTfR6/RK9iY7T/L4kNHHv4UV5++RlUpiBee+FFbHKJjLuP0vLKSTZvL8Hn8/Gv3/sherWatrp6aquucOlqDdkH9mGdngWNhqe+/m3yD5WzOjVDT2MzCq2G0s88Qu/ZCpIP7Ka9uR3r/AIOh52rFZVY8rKIMBroOHsRr8PJ1k/eg0wup+GpFwgIs4BchkqnpeVSFZ51D0e++DkunDzDiT94FI3RQPnuMq7V1DIxPUVg9mYK01NwrzmoqL1KckgY/a2tbL/jCADbDx7g6pVq2nq72P3AJ1i123n5iSfZ/sgJkCSuVlTisK1x5KEHCI+N4czJUxiMBpJysnE47Dzx5BPsO3yQge5uEtPSkBkNjI2NURQWRltNLSdfeJGYLXloDAZee/Z57n/sEQICzSwvWfn5j/+N+PKdGAIDaXjxNdJLt6M16OmZ8b+Irre3cfhB/yxN+HwYDLePK+99cORZJElqfsv5TzZUkt4P3m9Ee6tYlyTpcSBakqQfvr1RCPGn73WDD9Xp31S22Ti9C3gzs/868LQkSf8//rAnBf/8ROIdRAWEEEKSpEv4M5jP8puiGe8LI5OTnG2/xsiNG0yPT1JwoJzw1GTsK6u41xxUP/cCBnMg2z/9EKMNzcjz0pk9dYrkjHxsdhsymYRPpUAXGEBEVjo6o5H58UnG+/vpPHkWp8O/MzK6MIeZwREan6sEnYa4jHQ0Wg1N5y6SX1bKtddO4/F6aK+rI6t0B5rlZaJyMpgbHUOpUlH/yuvgE/zgf/0dD37+j7jefI2Xnn6G6Ox00hJiMEeEM9hbj8yop6qxjpGOTmThoTQ0N4NPUHexkq17dtF0oYK5qSmGB4cwVF4mpSif6dVlglV+imuFQoHQaWi+1oJssA99bBTJ5aWsJ8fSPDvBuctVGOQqRgeHuNHcwuqqjTWZINxkAiCwIJvvfe1vCYoIo+1aK5bYKFTtN0EuY7Crm8DwcCa7+1hds/GPX/9byo4epv5iBU6nC6TbO6cH8IlbCu/fS9YKSZIu4meVeju+ij+i/Tv80eff4Y9o/4DfjRzWUaAcf07rljbjvB23zeklSXoG/zqiRZKkcfyaeLskScrF/0GH8dcBI4To3GDjuYmfpOOLb85zJEl6U1RAjr+U8E1Rgb8CnpUk6dtAK341nfcNdYAJWXwomsVFCjPTyTi0j7mRUSqffJr5iUnKH/okUVn+BQOXw4HP5yN921bWbWvEZWwGYOZGDyEx0Wh0OmyLVsyR4cTv2MLi4Ai9Fyqx/eQJYjM3I2QSsmAzAeYANh0uZ2l6mq16LUokknaW0PrySe782l9iGxwhPiuDyZ4+QpMTsc/Ok3PsEG6nk+e/9m2efO4ZNpfvwhgRSsq2rThcLvreOENvYwuH/vizJG4pwPj6GRbta+Tc6R/tY+Zm8AUaMRXl0P3KGGlF+cTkZTHeN8DC4iL9ox2setx+wcq5OTo62tn9yXuJzs9BkiR6L1Sxef9uzBER3DxfSUR2BgMyL80d7SCXYV934/F6sNvsDE6Nk3n/cbx6LSjkBCXEYAoPY5vbjcfpYtOBPSxOTfPGd3+IZnGK8E2p9F/0q6fd7vDe/TtK5Akhyt/7qvcV0d7q352XJOkFIFII8cT76fsmbtucXghxQggRIYRQCiGihRA/E0J8SgiRJYTIFkIce8uojxDiO0KIJCFEmhDizFvsp4UQqRtt33mLfVAIsUUIkSyEuG8jqfGBMHG5lvhd21h2OXDb7czc7KXw4D5SC/JwuN20nzyL1+PBue6h89JlQtLTUAYHsjA6RtflGmKLC4nKyWS2f4jui1XEbC0gICyM1dl5krKzCE9NRmc2owuxkL23jNxP3k3bi68xUttE4rYt2FZW6KysIiJ9E6YQC2uLfj2R6c4uonIy8Xg8+Hw+Wl56nTu/+TdoDQaEx0NiXg6z3b1Eb0pF8nq555t/zWx3L5IkoTUHEJGXTU/lFXw+H4Ex0SzOzmFfXkEjV+ByuVBptURnZ6DWakku24EuJR5LSQGKwAC2feJe3AJaXnyN0ZY2Vq1WbEtWbp6vJPuuo3i9XsyhFmIyN5GQmU7GHQfJOnYYmUziE9/+BqMtbSjVajIOljN8rYPa518mMi8bSa1ieWqa4bom7v/ON1jt6mdlehaZRoMQ4vaG93w4S3YbGo1v4u0R7SclSVJvRK9vRrTv73P4B8Q7Pujzfex35M1OTWEOMrM0O4tbCJ7+xrfZ/+lHCUlJxCMDr9tDxO5Sml54FUtSAn2NLaTtKsUUYuH6q6dQ6nSYI/1RXuuLrxOcFI/H5WbqeidKhYLejhtsLsjjeuVlrPPzbCosYG1qFpMlmI7qWvRnjMiAa2cukrW9hLmJSXwS1D79IpmH/Fl0r2edpudeIePIAWxWK24heO17PyQqLZVAi4XmV0+RsnMbGp0OlAqGrrWhDgwgOCYK5+oK1Y8/ReaBvSSEh9F96jwak4mUkjJG6ltYmpkh+/hhlGo1DU+/iECQe9+dyGQybp48S/bdd7A4PsnI6XP0trVz19f+EplMhtfjoe3V02QeO8j1N87hca/T9uopsu44iEwuRzIauHG1njWbDaVaRW9TK1qtFrlOz3BdE3qDgaUJ/zu/7udPkbp/Dwhxe8N78aFl7//x/Ua0HwC1kiT9C/AcsPam8c2qu9+Gj73TjwwNMcs6cZFFxJUU4ZqZo+XUOVYnp7CvrjE/OYUh1IJcJudmdS3LVivdZyuQKZV0tbRiDDQjl8vxedbpbGgkV6NmdmSMtD076B4ZIzkrg4TyUoxJ8XS8cYb43dtR63SMX+8kJCGWqOICFFoNNreLgPBQzEnxjLe0M9zVjSnIjAS0XqkhLiOD8bbrqAIDiN+Sj1wIlFoNKr2OhtdOoVYqGW66hsvlpPbVN9h67AgDc1dxrtnobmnDYDb7lxHbO1Dr9LjsdlqrrhCXlspAVQ0uh4vR/n4QAt3Ziyi1Whbm5ql/5iUCzQHoAs2EBwTQcfo8Bq2O9qtXicvKYnpgiPCsdF7/hx8QmZrEaF0zjrU1xoeGScjOYPOhcqZ7+ym6+w5UcjnBqUlcffIZfB4veTGRFNx/J4bLV/FoVEgy2W0e6T+cghohxLtyzm9Eq995t/b3gW0bP7/11tvjn+v/Vnzsnb5wWwmnrzWiUqsZq22i+MFPsDw5zfLgMJnHDvLad/6JrQ9/ArVOR9vLr2MKCyWqpBCVXofX50VSqTCGhxKQEMvc7ByGsFDSCrJZnp0jMDaKhbEJfD4fU60d7Pj8pxmpuELKgT2sDI6y5VOfZPxKHU7hI2lnCZMt7QR4vDhtNhIKcgnLy0IXFIjH68VmXSEyLwu1Xs+1518h++6j9J29BEFmCu48Ag4Xmw/swbZkZfRmDwHxMegCA+g9d4mtn7yb8IQ4TGGhCEngtjtJ3FuKbWUFjV5PYvkubEtW1IEmvAiCY2Mwx0SxurKMfWnF/2Iy6PDKJSyxsZijIhgbGsbr8SBXKfE4nNhWV4gryiMw1q8eLp2pQL5Bcjl3s5f044e4/vJJoovyiElMQBlgRKVWszQ1jU+rYblvGCTptjo9fHQUboQQuz9o34+90wOElW1n4HwVJp0WjV6PIj4WrxBUP/4Um3eXMlrbiC4ijLDUZEI2p9Jz+iJeuUTy9mI0JiPd5y/RU9tA9l1HGW+6xvL4FMP1TWTcfRRVoJm5vkF0GxGBUGtYnpxGHWBEJpNhW7KCTIY+0ExKeRmdL51EH2Aiad8uek6eI/VwOXKdjuzyMtqffw25VkPKnp3I5HI8Shkznd0UPXgfk109DF2tZ2l8ikN/82W6zlzE63aTcfdRfB4P022djDW1krqnFEkhZ6CqBo1ejzk5nvGma8yNjJF11xFkMhltL7yGpneAyKwM7HYHl376C/b/+R8jk8kYvFDFZMdNCu47zvL0LI6pWXQxEWx/6H7G228gk8mZ7O4hqiiXwep6Jm/2ELrJX4GXsHsnPRcvYwgPI6Yoj47XTrNiXWbd6yX6cDn80w9ue8GN99ay9//jIUnSN97JLoT41jvZ34qPvdPPT08z1TCMY83OwvAIhvAwJJkMuUbN8pKVpepaVFoN7pZWcg/vZ7Kjk8XFBeaGRhHrHlRKFQqVkrGePkKbWsG9TsV//ILI1BQ8bjehyYmc+Yd/pvSPHgMgujCbN771T2x98D6ctjVC8zIZvFxL14VK5G4P0yNjyBRyZEolMrOJ2iefJufoIa6/cY7V5WUW+wfQGfR43G5ca3ZGu3vRnzyHWqul4ex5NpdsZbSmgY4rV9ly+ACuVRvaABO2uXmMwUGoDf45s6RWszY3T2RQIJeeexWVVo2u4gqSTIbSoOfm1TrirFa8Moml2Tn6Kq4geX30tbYRYLFgyUxDGxTI3OISHU89T8bRA6DTcPXp51l38tZ81QAAIABJREFUuwlMTkQZaGKi/QbpR/fTefESPpuDG9U1FB86wGBlDQajiSuvvUHeoQNY224ghLi9Ti9+d9n7/wFYe8vvGvxLebfEWfmxd/qg0DAiUmKYq6wmICSI8Ox01Dodg7WN5N95hOn2TiIKc+k8cx5VqAWFSoV5cYl1p5uEvaWotFpsi4sYW9sJLy5EpdWwZrMhfNB5rhIlsLayzFBVDR6vD59CTmBMBLN9AyxNTrMwOIxtcZH0Ow+jNRnxCYEuwET09q30VFxh7GYvUWlpJO0tZeD8JfQhwURsK0KpVjPd1YvH7WbTHQcYudbB3j/6NCvDo8SWljA3OYXSqGewoQWlx0tb1RWyd27j5qnzyOQK5HIZw929BKcmE5WWBAKiy7YhVyjoqa4lNi2VrLuOcv2Ns+z/qy8x39JO/P4ybGs2FkbGWJiexeN04lmz43K7WBoaJWH7VoTHy8LwKI7lFYTDyeTQMJbeAWIK8lBq1Hi8HpTBgUTkZuHz+QitvIRMISeoIAe43Ut2Hx0KbCHE9956LknSd/GvDrwnPvbMOTKZxExzKxGFucTvLWWyqQ378grC6cIcF4MpOYGb5yrQBJhQqVToTEaE3UnRYyfoP1cJwPDlWrY+eoK57j4GaxuJ3pKPpJSzad9uAtOSKXngPlx2B2n7dyG53Gz79MMogISSAsLiYig6cQ+rQ6N43G40ASZ87nVkMhmSx8PWE/eg1OsYqWsksriQ5P27mWpuA2C5fxBDgAmfz4d9YoqgpHjW1tZYs64QnBjH/NAoSaUlSCYD6XvKMEaGk3BwD3H7SpGHWQiICCMoOQGD2UzKkX2MVF0FwD2/BGoV604XWoUSnTkAn1rNyuQ0BoOBrZ99GOfYBEklRfhsa5T/xZ+g0qjw2p3IvT4KHz3B6sg4KkswlvhYAiLC0ZsD6DlbQdqRfayO+7P2PecrKX7kBGtLS6wNjyFxe6vs4CNVZfd26LjFKruPvdN7PF406170EWHIFApWFhbpv1BFbJk/ORqZnsbi+CS5dx9jsvEaY23XCdqUjEwmw5KbyWRTK/qAAIxBQXhWVvAsr2CKjiT10F7G65pY7OojZHMqm+86wpnv/m8Wp2cYvHgZSSbnzP/7fYRGjTk2mvnBYbrOXCQ0Lwu3y8V4awdBcdFE5WYx3zeATIAh1IJKq2F1dp6BK7VEFOZhtzvoOnORmJ3FAKQd3EvDk88QEBvD5qP76T1TgXC52HRgN06Xk8WefgBsQ6NsfewBrr92GkNcFCqtBkmnxToyjk6nJWnvTkbqGlEGmgGI37GVqz/7FdNTUwxebcTuWefcP/4Ql8fDdPsN1Do9FT/+D6xLS6zNzmNMjKH/Si05d9/BUHUd604XepMJhUqFMtyCdXQCpZAISYwnIiaa1b5BhBBotdp3/qJ+BxBC4PX53vP4fYAkSdclSerYODqBHuCfb6Xvxz68r6msYD3IzGRVLUgS4/19KJQqpFPn8Xq9eL0+VhaXaH3mJVYWFgmJjsKwYwudZyuQnC4G2zswh4bitttpq6om58BeRhtaUKhVDF6/gc/jQX3hMja7nbj0zRiDzcTv3onP52O8t5fgpHgmG1vR6HV0N7YQGBbK0M0uuHGTtK2FrIxV0dvagSUmCplcjvB4WVtdZaS7F5VKxdzUNI4lK26nC7lChoSMqeFhIq+1o9Bo6Gm5RkhUJG67A5fXg21+EZt1GVNsFMuj4wxdv45Co2ZuZJzI7HTGrtRjtAShUCppP19JfF4WbusyMo0KmUpBRGY6QYmxeD1extquk1xehsZkxOfzkTQzi8ZkYGFyirXRSeYnJhg8X4Xb4+H1//X3FJy4B4/bTWxBLp3PnyQoOQ4Ar9NFSEEWSBLq20qM+dHJ3uOfw78JDzDzlqrT34qPvdOXlJZReaMNy3Z/sdOK1Uqg2UzcvjIA+s9XcfCvv8RiZzcBibH01TQQkBxP/LYtIIHaaMC5ZCXx4B7mJ6awxMViTIhh3e5A136DwBALcfvK6Dl3icgduYzVNuJaszPbN0j+/Xex0j9E3J6dTHf3ETg2QUhBDkNtHYQnJRK5sxifz4dPJuFcsRG5s9hPk3XuEvGBZsK25DE3PkGQJZi04weRJImlsUmS7GsYkuNZm19gx6MPMtV+g4iSQuRqNQu9/dT96kU2FRcRkJZI9OZNhCQnoouOYHViioWFeSZHRvCqVejMAYSnpRKc4S+B9TrdeOYX0eRk0Hu2ksiczF/vJu89eZ7EfaUMnq8ibUcJqxFhGCzBKFQK4g/uZnZ0DPfKKpNtnUgeD13NTWSqFbgWluhtbiVc8uvY3W6n/zDq6T8kKIBxIYRLkqRdwD2SJD0phLC+V8ePfXivUqtRxMcy33odAINOx5rDzrrThW1xCa1ehz4kGNvSMo6pWSJTkghKTkRjNDB07hKxZduIP7Cb0cu1RG1KZbLjBtJG8W9YciKSXs/8wDAqtRpdkJmUw+VM1TezPDSCOTYah82Oy25ntW8QQ0QYLpuN6LRUJJWa1bFJBi7VYMnJIHRLDrNt/t2cKglcbhc+rxeDwUB0WQljV+rx+XzMtrSRc/+d2EbGWV9axhQXTdqxgwxeqEKuVLAyOkH28UOE52djG5si7egB5voGUOm0BKUkEhIVTWhsLOG5maRsKWBlfoHl4VEAZGoVNpsNt92OWqUiYXsxyyPjLI2MExQbhUqnI3H/LqaarjHT2ErMji04FpboPVdByWcfxj23QFRRLkKrZscjD6ANCiRqzw4Si/KR1uwIcXvFLvjvL639XeIlwCtJUjL+upME4Olb6fixd3qAgLhoVmxr2MYmUGm1JJfvYra1g7GqWiK3FeGwLoNCxsCNm8Ts2MJUfTNDV+qI3lqITC7H7XDSf62dnuZWondtY6y6jsGLlwkvykPIZFT95HGQScwPDCG8PuxOJ0q5AkmSSDm8l943zmOMjiS5bDsNP38aS14mcTu2MNrQguR0oQsKxBwRwcLQCEO1jQSmpxFVXMRY4zVUgWaMIRaEWknld/8FRYCJybomWs5eYN3pZuDMRXpOnsPl8XDu//s+wWnJxBcXYh+bQCVJaExGEg/tZfxKHX1vXCBm9zbi95cxXtMIajWxO4uZ6+lntOkaypAg4sq2U/eTJwgvKcRgCcK7usZc2w1C8/yUXDKFgvYLl1hbszNxrZ2VtTWWp2ZQ6XQE52Yx2XgNx+QswZtScCwt03uuksDcTHSR4QghUNxGLTt/aa3vPY/fE/g2wvm7gR8IIb6Mn/v+PfGxD++XFuax3hgHh5P2V94gIDgYm8tJX3Ud0anJ9J+6iEetwLPuIW5TGsu9Q/Q1NuPzCSSnk+XBYWQBRoKjIjGFhrDSP0x/WwcymRx9XTMhaUmYLRYsmZtYXVyi6/QFpPV1RgeHUGrUCCHoa7mGwWRietGKfWWFhZbrSHI56551Rtt6UBv0+NzreL1eOs5cILEoH31EGF1nLhKdnYFzYRFUSlwuF5FF+Sg0KjZNzqCzBBFRXPDrzzr5rX9k+kYXa2OTjHX1EltcwGxnN95VG/0trejMZuaa2kAmo+XceaI2bcI2M4NCrqDpuZfJ37eHicZWVpeWmapuQBlooq+ukaicDCaqG1h32FlzOpFkMpIO7EahUTPV048AJpvbkLxe6l89TWH5LiZrm1hcXGBxchqlWo1wuPwrFh+CVPVHBOuSJJ0AHuY/i29uKUz62I/0gcEWzMUFBG0vIjgyHH2QmdhtW4hJSyEiczPxB3eTvHsnep0efWwU+sgwQjalYYmKIrykiMgdW7EODJN6dB9KhQJzWjLRKSlEJsQRU1qCQqslddd2Fq53EZaUQObxQ0hIRCYlEllWQlB+FqEJiQTnZyELNhNXmIs6Moyw7UUoVSoSiwowpaUQXlYCXh8JGekk7SnFFB6KTKXEZAkiprwUx9oa2ccPszw0gn15BUNMJGtLVlzLKwCMNrQQV1xEaF4W4aXFWBfmmbjeicoShCzQzOZdpYTHxxFSXEBA1iZSCvIJT4on8VA5oduK0JvNRO0tRWc0kHnsEIbkeIxJCawsLqJQawjfXkRMeRkyAUWPnGB11M/yYwkPIyQ8nIiiPFbnFkkoyseyNZ+w4gK0Gq1/ulSUx8ryMnB7BSwFwp8jeY/j9wSPASXAd4QQQxtVe0/dSsePvdMDOBaXmL9cS+KxQ6jVaoTwYUlLxjo1g3NllfmBIXSxkUTlZ2PtGUANZJ24i/4zFfScvkD0lnxUOh3rdjvDFy6TeHA3ptQk5jt7GLxchyU9DeeG841crSdySx4Je0uZbb3OxJV6tjzyCVYGR3GMT7HpYDmzN7rwejzoTSYS9+xkurGF3pdPkXhwD6aUBByz89imZih84D60sVH0v3EetUxOeHoaK8OjjFbXE5SeSty+MiZrmwDwLS6TvGcn9vEpxupbKHnkBEajEUkuwz4yRkhBNi7hw7m8wtCZShKP7Me2uIR7zc5YxRVCU5MYPFtB1M5iInMyWOkbZPrGTXZ+4TNIKgUTNQ0sjY0TGBOFOTIcn93B0NlKIrYVYXe76Tt9gagteaSUl7I8NEr/2QridhYjITHR1IppUzJCiNs70m9U2X0UluyEEDeFEH8qhHhm43xICPH3b7ZLkvTSu/X9sHnvgyRJurDBVX9BkqTADbskSdIPN7jtOyRJyn9Ln0c2ru+TJOmRt9gLNtYq+zf6fqD/ltGBAeydvcQf3odcocApQc+pC4Rkp5N0YDeT1fUsdfYSlOLf92B3OpBJEiszM0gyGQPNrUy1XmfobCXXKq+gDTAxe7OHoMQ4Zrv7UEsSSo0GbUwk0ze6Ea51TNGRaExGlkbGMEWEoVCrWRoZxRATCUDCwT20/OIZ1hUyJqvruXm1Hvf6OiujE0RmZ7A6MML67AL68BDmbvYgkysY7x9k6EwF87PzuFdtKFQqZDIZqogwRq42oo0K95fE2u0Imx11cCC68FBq/uVn+Dxehs5W4rHbufjd/40yOJDl8QksBbm0/eoFFEGBTPUOMDc+iXVmFrfTyboE63OLONbWWJmaZnF8grqfPYXX68XjdrMwNEpgQhxzHTdZmZ5horsPa98ga5PT9FXV4FpfZ6i2EXxehmobcC5aEbfZ4XwI3B7Pex4fEbzrRp3bOaf/BfAv/Kbixl8DFUKIv98Qrvhr/Aw4h/ATCqTgZ7v9MbBVkqQg/Iw7hfhXXFokSXp9g2/sx/gZbuvxK3wcBM7wPrGwsMjy6iKahmt43W6Ew8lEdy/K02oUCgXWqWmcDgfGej8zkUarpbuuCX1UBGg1RKWlkHxoL9axSeJWVghITkCmUdNz6gJyYKirC22AEUmSUffGy+SU7WC86iqSQsFwVw9Ls3N4HU4G2q9jsgQzXlHDutvN7MgopvgYYvaUku10IVOrQK+l7+Q5epuaCYyIQKHXEbt9K2q9HtVlNRFlJVDfTE/lZYJqm5CEQHi9tJ67SOGBfczUNNJWUcXm4q0MXKpBkskIS4gjel8ZkiRhm19gfnSCoPQ0VmfncIxPMjc+gS4ijMDwMPQmE3KlkvnOXuaHx3CurmJOTyWxfBcT9dcIda+jS4yj92wFo13dKPRaYkq3ETQ1gzE4mNDiAqyT0yzOzpGwewehm1KQyeWoahpRRoQi3U5tevCP9N7fj5H8d4B3TV7cTuacK8Di28zH8XPUs/HzzrfYnxR+1OMnvYwADgAXhBCLG45+ATi40WYSQtQJIQT+F8udfADkbilEpVJjys/CsmMrmqgIIpISSTt2kIT9uzAGBRG3KY2QrflIgSbWHU6SSorQBpnRyGREFRcy39PPXEcnWffcgW1oFFNYCOnHD+ETgoT0dEJLClm1Wknfvxt9VAQRpSWEbysiY1sxwVGRBOdnER4bhz4uhui9O3CurbHvK3+BzOZACIFcq8ZuXcYcHYl5cyrxuTkgSXjd64h1/8i07nLhXLHhs62RddcdqALNBG/NxzozT959d6EMsyCCzaSX78YQHUHSvl2oJRmRxUVY+4cAmKlrJjw3E7G+TlhqMqzaKf3S59HJFIRERmCMj0G+7kEXFUZYUgKhCfGYYiIRXoHc68WcHI/H4UAXEYYlOZHYsm0IIdBYggnfXsRsSwcLN3vY9RdfZKV/GJd1BZ/Ph5DB0rXrtz2JJ/jo7Mj7r+DDzt6HvUmRJYSYkiQpdMMexf/Nbx/1Hvbxd7C/I34b7z1ASFkJ83XNhO0shrkFDDFR2GbnmaiuI+mOAwxWVNN/toKA8DDCthXhWluj7ZVTBISFEh0VQf/r5zDHx/hHS+syQgiGLtUQVZzPVHM7Y/UtWFISCUiKZ6iyGnVQAAujEwSmJRMZHsLQ6QoiMtNY6Oxhuu06seWlKNQqQrbk0n+mgqDEOAJCLfSdv4TBYCD1+CFmqusJ2b6F0cu1qJGYm5zCcfEySccOgCTR+8opZq53knCoHJVex2RlDW6Xm5j9uxg6fYHF0XFidu1ApdMyd7WRVZMRY1QkgVmbWWhqw7vuwRhqQW00okmIYaKhhbikePqqriK8XnIfPYHXvc5C6w1ss3PEH9yDJJcz29SGd3mF7PuPM9/czurCIgkH9iBTyJm+2oBao0NjNJB0x34GTp4nODONiY5O4g/sQdzuzPqHx5zzPwHv+gb9n7Jkd9t574GfABQWFv7GdQuzs0wO96PSaBi6WEVMVgZCJlH1z/9Gxo4SZmsaWRwZxbZkJTI9jeXFJVQBJtQ6HesOJ9ON1+iubyJdLmN5fgHz5hRunjxHSHwsPknGWF8/oruHsJRkluYXCMnOYLymHgQYwyzM1Lcw1tOLdtKA3WpFbTRhvNHNsk+AEHTV1JGrUqLSahmsbyZrbxnO5RXWVlYJlSQs2elM1zUz3ttPSEwU3jfO4/V5me4fQiYEsks1qBRKbl6tJyE7k5nqevD6mOjrxxhqwZSWwqp1GVtDC/GH9yFJEl6Hk6X2GwTnZDFb28TS1BQOmx27zYakUWGbmGauvgWfy83NujqiMtKxL1nRmgMYb7vOpuMHkSkUuFZW0RuNuB0OlnsGcNidTA+PI1cq8Hm9qDQaGp54FoVej2tk4tb/Wz4gBB8rp/+rd2v4sJ1+5k0a7I0QfXbD/m4soeP4GXXfaq/asEe/w/XvG8GhoYSHmFju6mXoaj1Kn0BpCcIUEkzk7u0oVCqcDgfBkZEk37EfSZKY6ewmcdsW5rv6iNiST5Z1BZVRT1BWOvPXb9Lf1ILwelCvrhIRH48+yExYSSFri0usTk2ztLDA2qIVQ0oC+vgYUiUQ6x6s84uoFHJCSwp/HepmLK+gCgzEjZek0hI0liCmWjuYGx1j+YlnicrNIm7/bjRqDUgQsXsHbrsdsyUEj0zCHB2JNiIMIZNQGw2Ebsln8I1zpB7Yg+TxMtHYgmNhCeviAtorRoTw0VXbiNESiDwslIjiAlZPXSA6MZ6wTamIqTnithfjmZ799bRl3e5gfnAU5/w8izMzDF68QlhiAiM3ulCoVQS5nMQUF2Kfm8dkDiB0ozhodWqWTQBqJaaszbc/vN8ouLndkCTpPvwya5uBLUKI5re0vaOslSRJB/EXzMiB/3hrJv5t977Obx/gsjd+nn+3az7sJbvX8XPUw29y1b8OPLyRxS8GljemAeeA/ZIkBW5k+vcD5zbaViVJKt7I2j/MB+S9B5ApFXgXlkg9sJfw/BzWF5Yo+IMHmWtuB0BjMGIpyGah06/K4hibxJySSMSOLYxWN6AJtWBbXEauULA2u0DuPccISU4idFMqxshw1jaW60yhIXhcLpKKCkgryCN0UwozDdcI3ZKP02ZHq1ETlJPJwo1uAIYuXyV0az6e5RU80/Mklm1nobuf2O3FWKKiMYVYMMTGgCQh02txCYFzeYXxSzVYinKJ2lrATEs7wxWXCS7MwWNbY7LxGpbcLEI2p+FYWSU0LQVVsJnw1BRMWZsJLS0htSiPkIR4AixB+DwedIFm1lxObLNzqEKCMUaEsbZqwz47hzHEQlhJIRohCMvJJO/uYwRERaEOCyEkOYGQuFhiivIYr6whomwbmM3YZ+ZYm19gubObqN3bweFkpb3ztmfvxYeXvb+Bf6fclbcaP6BQ69txFP9mnLMbx4Mbx2ngxVt5uNu5ZPcMfumdNEmSxiVJ+jTw98A+SZL6gH0b5+B/4EH8Mj8/Bb4AIIRYxC8W0LRxfGvDBvB54D82+gzwATL34FdVmb14hdgDe4jIz8HWP4TBYEBjMLC2sMhc3yCqiBBMEWHYxicZvFBFeLFfB0GhVtF25jxzI6Oowi1c++VzhKSnEpG5mfn+AYYrLhOal0XMru3Mt95gqu0GaqWKoMxNuJxOBs9UELNnhz+kVinwen2YYyKxDo0ifD5/0iw4EJvNhlqv90tNRYZx4dvfJSg/k/iDexmvqmGkuh5TSiKxu7YzVdOAMTyMtYVF+s5WgEzGWEcntu5+Rnr6mOnqRaiUuO12IkuKmG27gVouJ+HgHqZq6v3733U6YvaWYh0Zo/3J5wjIyUAbFc7QxcsEpqcCEFqYQ82/Pc7E4CCLg8NMDA3T+dLr+NQqtHGRTHZ0sjI9g8+g4/L3/xWXZx3H4hLhuRnMXetgvrmdqL07WXc4mZ9fYG507PaLRH9I6/RCiC4hRM87NL2brNUWNoRahRBu/AIux9/l3iNCiBFguxDiL4UQ1zeOv8af+H5P3E7V2hPv0rT3Ha4VwBff5T4/B37+DvZmIPO/8owA1ZWVSCol83VNrLvXGWxuJTonHc3YBCFFuXQ88zIph/fRe7aCxaER1h1O1HIFDocTr0JGaFwsoWnJqEwmOkfPoGi6hql3AIVCRW/jNQwmI16Pl77GFvTBgSQW5jPTcI3+1nZ0Rn9J6rrHg0ytYnF0lPDlZSJ2bKH96ZcIyUij79QFnMvLTPcPItRqDGEWtEYD8939LLZ3otbp6K6pQ+31IVfI6W1oJq20BHRaYsu24XG5mJucwpCaTIRSzkxTG3P9Q3htNrx2J7b5eVyrNtR6A6pQCyPnL6EOCmKuqQ3Pio2Z4RECL19FqzeyNDnNTHUDcrUSoVETEBJCXPEWtKEW5np6WRmbBAFi3Ys5JZHRjhuk3HWU9blF1Do91qkZplraGWpsJjY7k+7Xz6DQaVlbWgQhfQjZ+1ue0/8uZK3eCe9XqPW3QS9J0g4hRA2AJEnbgFuiHfqfksj7b8OO3Xs4WVtN8I6tSJKE8HiQJFhbXmGxb9C/FDb9f9h77+A40/vO8/O+b+eckXMkAAIgCJIgAIJ5NCNpNJJGsizJa0l7Xq/P562r811dqLuqq7qr2rpQWxdcXq/3LJ3lINmWtPKMNIHDAIIkAklkgMg5NBpodM7xvT+aI8u3kmZGFkf2UN+qp8jut190F57+4fnF7/eQ6ou9iKKEHI9TfKlAsOFdXsNRW01iZx9ncyPtVy+Sy+Uou9zH4eIyp6teJbS8Ts0nrpGMxdBZrTjPdAJQ4z0mk8lQfuEcKqOR/YlpIm4P4e09spEYe0sraO02al+4xPqd+5Q4HBgqStEVOSk/0YzRacfedgLf+hbmrR0s3R2oDXraEUiGw9ia6gHYuTtM25dexf9wkrxahaW6AkdlObpiF9l0msOhUbK5HObudqKHXubfvElxcwNNn/440Zt36f/93yWxsUM8GsVZVYH5VCsqgwHv/CL1L1wme+hFUVGK7AvR9PLHEJEx11ax9dZtOr/y6yT2DtCbjQh2C3qtnrKPX8dkNJFJpSi7NgCARpRIp9LPfK9lWSaXe1/+xD9I1kqW5Z8Wav60BPRP8rjf64P+J8A3BEEwP30cpCCd9Z547ttwRVHEcKaD47FxvHMLuDpaMFRVoFMo0EkSA//Vv0LIQ+rwGI0g4OzuxDdb4B+MrW9jqasmkcuSDEcQVUoycp5kMERsYxdHUz3VH7vC+us30BqNqIrsBBZWANAaDDS88hI7g8OkIxFkfwiT04G9uZHowSHX//v/EoUokvB4UeZlaq5dxPNokmwqjc5uJX3kAyC6uk7nV75AaH6Jg+lZdHVVmNtb8M8U1L80CgVKjYacRk1wa5fKi30/urZ/a4iii+cp6e9hf2iE8NwiF//b/xxXacnTXEM3WpOJTCCEKpen/jOf4GBoFFmWyXqOMFaVk00k2Xn7DsWXerHV1ZDa95AMh9E5bJhLikkfehElCWdbC/6tbVLBEJJSgaaihMjqJhHvMaLNSk6nKYzBPWP8otx7WZavybLc9hPWz8ot/ayE9QeSu5JleUKW5Q6gHeiQZbnz/QhdwK9OevL5PMlQBM/KKplgmLaL/Yi5HKPf+VtOnD/L8eg4GpWKxbdvI2nUlF7pZ2N6Dk1FKVqnDYDqKxc4uDeKZDRQdbkf9+AIeqP+R5l+BIHV6VnKEw1IKhUqqxlZrQJAV1nG7f/9D2ju7UFltzLyh/8PtZcvcLy5jcLlYPnOEHIujzMcoerFK2y+dQtTVQWJfJa1G4MUn+78UXlMjAgYOtsB2NvYRnvsQ/mUfqrk3Gk27o+STabQNdYw/5ffRV/sIjTzhGwiyeroI9ov9hOenGPl4TgKjZpcPI4kKdiZm0djMhGNxcgIMPOn38ZcXsrx+DQLIw8paaonuLKOub6GaDhCdGiUihcLmgtZnZp8uEDcWn51gO0fvoOxpBhnUwNbb9wkk8tS9sJljPDM6/R5Wf5lt9l+IKHWn/WDBEFQA68C1YDi3dDoVxTY7wMBnx8FYC5yoXEV4TjbRdwf5JRSSToQxNl7BoB4Kol/c5v1N2+hUWu493/8W858+XP417cAWBoeo6avh6OZJ2w9WUAQIC8IGGqqiO+5aTjVgav/HP6ZJwz90depbT+JnM2SlUQsriJK+s9xvL5FNp1BpdUhKESUeh2minKCm9u4J2fIRaK4F5cx+4MUVZSzMj+DyWggtiERCgQIHhySEgUkvRaF2cTyD98hm889xfJAAAAgAElEQVSRjMVQqFSkMxn2Rh6hd9jxrK1zqv8clooydm/cof7qAJqaanRFTop9PjQaDcWX+wu/pHweBIHiqxdIRaPMfONbWGuqsHa1U3lYEPhQFbvYHhwmcXhIPJbA+GgKAK0gsrK6ilavQ5AkfAcejnZ2EfI5dGYT8/dHMNntyJL07Ntw4UOZohME4TPAHwBO4A1BEKZlWf7YzynU+tPwGhCioFz7gXQcn3ujtzsdiFEftqIiktkMce8xnoeTVL54Bd/yKqHVDYx11SgQqL52CTkcRTAZ0M/NI6vU5AWBqM+PxmhCaTFhaqjFubFNXpaxnmonsLaBs7WZ0PomkkKBZDbSMtCPxmrB3t7Czlu3aXzlRaJuD8mdfU68/BJiLoe5top0PE5Mhor6epz9Z9l66zbdX/0Ssa09ouEwRbW1GNuaURn0RA4OUZaV4mhvQRAEsuk02UwGW3kZpVcH2PrBDfp+/3cJPprG2t5CSzxBds9DVKXEWFKMpb0F38hjdEXOwvSd00Z0bRPJYUftsiNrNUTWNjHW11B/7gyJSIR0KIzeoCcXiaKzWqh+4RLum3cx5nIYuzsQJQnP+BQldbVYek4TWN+kpucMoX03xvYWvHML1F8ZQBZFhGLHM9/rD6tOL8vy94Hv/5RrP1HWSpblNylUsd4vymVZfvHn+XzPfUwPkJh5gqWzjZIzXewPP8RUUYYgijhONHG8ss7G23ewd53EUl5KaHOH4OwCTS+/iJjNoVSpEI4DnP2XX0GRzuK+N0r5xV7qPvUiG2/eJHlwhLGmkmQqRXB3n/TRMRWX+8kGQmzfGKT0Ui/WslIygRA6kwlHQy3+5TXkfJ692/cpudBTaOt98xbO7k60Tgek02gVCupffgHf4yl2bg7h6jtD7Wc+jnfkMRqzCZ3NSnnLCXRFDma/+VcU9Z9DUiqJxWMcTMxgqK0imU5xNDaO+WRBcjsWjZLLZBBUKmxNDQQ3d3APPcDc2oytoY7Q2ibehWVUpS7Krg3gefAQQVKQyGZIx+Ps3R3GfuYUrv7zBCZnARAjcbQ6Pel4nNSuG1NrEyWX+vBPzCKEYxSf7iCLzM6NwWffhstHZ7SWgoDlyZ/nxuf+pB+9f594PIZ3aBRJoWBtcppWnR45Xhh20en1LD+eQKtWkcvl2X6ygN5iJicKyOk0WoORsqsXCvH74hLWmmpCy+vkk0mO9wqtpUqlkqPVDXbnnlDZ3sbW27fZmp1HrdPhON2OSq9ne3yKk7/+KgCu3m7u/Zs/xFpZzubbd9gYn6SkqYG9iWn0ZcV4VzdQO21E37rN0eo6klpFOpVEW11ByaV+PEMjZDNZ0KlRBhLks1l849OoDQZMddWsvHkTo8VM3HPI0c4uujsPyOnUFPWeZe0HNyg500lwc5tcJsPh5jaW0XHSqTTxRIKNH7xFeUc7oaU1RElk+fEENZf6OBodR2cxo7YUksnxUJj0xAym+moSW7t4BocpfxrnpyJR1h49RmsyoxxVk49FScUSz3yvP6yT/kNCP/BVQRA2Kbj3AoXqd/t73fjcG315RQUprYShvobAyjpnvvwFohs72M4VRvq33r5NcX0troHziJKEpJCQJAlHTze3//W/oePF63gfTZHLZjnY2sFUX4v1dDuJQJB6nZakL4Dr4nmi0QgarY7SK4U4WZYhFYsRP/QSX9sk7PMRmnmCN50mnkogyzlqLvejc9hJx2M46uswNdcT9/lZ9XopMhup+tSLZOU8irxM1YtXiR4cEllZ42h9k4DPx5mvfRmt04Gclym63AfAwfQ88UiUvEqByeHAWFeDoaQYVEoic4vszz/B7nSgKC0ChQJXbQ2mc12IkoQlHse7sUXZxfMo1Gr2bt2lrqsDjcXCxA/e4uTFCxzfH0OWQSlJrN4ZorH7NAsPRqg7fYrg+AyyJIJeh620DHtjLZb2VrbfuoWzsfaZ1+nhIzVa+9LPe+Nz795XVFcTWN0gl82Sc3sw1VWjra0gtLhCMhzG4LBT2neWwOIq2VQahdFANFbIRrdc6EcQRexnOokHAjReGcBaVYEoihw/nMDe1Y6qrJjI7j4GswWF3Up0u9CDoTMYqHrxCqlDL1mVivbPvkwsHKb44nlUFgvdX/tn+CYKbcDWykpSqSSpo2MUOh3NVy9ib2vmaOQxSlHC1duNf2oerdNOzFOYVa860Yxvco5cJkM8mST/lNwive+morOddCyBrroCV1c7kcVltHYr1nNdVLa1IZlN6CvK0Op1FPWdJbK0CsDR/TFOfuULRFc2yGezaA0GBBnyKon6C30IBj3WvrPY+s+S12hw1lRh6m6npb+3wNzb2Yqlqx2FxYSjuR4hniS4tUPw6BhTQ90zL9m9m73/KJBo/FhnXoJCTf/d9Z547o0ewH7hHE+++VcYmgqUTda6GgLbu+zevo+9qx29w0722M/u0DDm1ibKBs7jm11AYdITPT7Gu7yKta6Gip4zxFY2OJpbxN7ajCAIuFqb2RseQ1XiwtXRindukXgggGDSo9LpSORybA6PkYsnMTTWsfr9NyAUQWu3YmiqZ+PGIAqbhZKzpzleWGH39hDmlkayuRzeAzeb0zP4JmZZufeAsf/rjym6dB5DbTUag4Hiy33s3xhE5bQT3t1n78YgJdcv4ezu5GhqFn1NYcxYUVFGdHMb98gjSi+eJ3xwyM6NQcydbeisFnKBEOGdPcxVlehtNrL+APt3hzF3tJFOpwlPL1DSf45EMEQqGCKTTCLlctR9+hNEZhdRGHSUXL+I+51BcpkMkZkFjCcaSScSLL/2FuUXe1EXuz4ybbgfBgRB+NTTdvZNYAjY4n22oj/37n3A5yPh2SXoOcQ9PYc0M48oCMQDQeKhMJbRCSSbmVgmhYoC9RWAe26e8gvnyQaCrPzwHRov9nE8v8iRx0N6eQVjSTGa5XXUajXujU1EQUF0c5tULsvov/0GlSdbyAbDOBpqMao1WNtbCO25iUZj+A48iDeVqJQq1oZHOSHnyXqO0Oh1rE9NY15YRmWz0vjxj5H85reouH6JfDZHLpMhPL9EJhLjYGMT8+mTKKsr2B18QNDrpePaFcIPp0ilUgT2DwiMPAaVClWJk/CTFZQqFb7VDRSCwPGeG93YBIlcllwqiWohSun1SySCIXyhMLlIFGcmTTwew1pSjCAIlFzsxXNziFwuR/G1AfKZDHsLSyCJGI99yILA+B9+HbVBj3h3hOWRh9iqysnuusnu7P/0AfBfEN4l0fiI4H8GeoBbsiyfEgThMvDTWt//Hp57o7fa7SS9brq/9mXSuwe4+gp1+b0bdyiqqMB2/jRR7zG++6PIuTyKQSUyMn63B2HkMY2f+QQ2kwlbdyfe1Q28a5soNBoaX+lCa7fhW16j+/Ofxbe0iqPnNC6Vio1HE2jUGoxtzajNJsLL64iiSDYSpe7cafybO5Rc7kMGWnJZlBo1tvPdbL8zSPPHr6PWaDFVlrM7NILRVeAh0ZlNJOUc+poqNA4bgcND1n94k8pLfRS1NmMLlWFsa0JtMZNJJgkF/ajKS9BXlnO8vMreyipRn59TX/o81rNdyMi4LveRSaZY+MvvkEmmyMiDKMxGSCTQWkwczS5ysLBMMhRGQECQZfZX1kAAeVAkAwhqFa6yMpz9Z0nF4ki5PJaKMiydbUgKiUw6g+lcF4lQCD6EmP6fENvteyEjy7JPEARREARRluVBQRD+1/dz43Nv9ADKWAJdaTGB+SVymQyB9S3MjXUcLxVKZ+HlNRou9ZM+PMbed4bDiWm6vvR5oodHpN2HIArs3x9DrddR1tZCzYtXcN99gLWulvSeG/vVAXQ1ley8cRNtRRk1589ham/haGoWjVpDIp/jaGEFRSqNqaudvEFPaG2T8M4eJb1ncN8bw5JOo9VqcbW1sPPWLYy1VahkmZRaxd7oYywNNdjsNo7uDhNz2ak8101gZR2dy0F8dYPyV15k981blF6/SGBrh9JzZwjNL6GrKMNQUkxNVycSED/0kj/0kjcaCG1sEVpYpfHVTxEan6b42gAH98eouHyB1NoWmpZGDDot8UMvhpPNHNy6x4nPv0JwYRlLewtqi5ng8GOSgkwyGML/aIryl65ydOcBwu4+iiInciZLYmefwPIa7zMk/bkhyx8pow8KgmCgML77l4IgHFFo+HlPPPcx/fb6OrrqQtuza6CHwNQ86V03xpoqzC2NzP3ld7FUV2KsryGVSBD1HKHIy+gry3Cd7mB3boGHr7+JZLVg7WzDoNWCLJMTRCb++vsc7exx/OAhgdEJNAYDi2++Q+WFHhK7+1Rc6sfU0YJ3cZmF198EnZZMMomloozkvgetwYBSp6PsSj9z3/wrjE/HWp093Sx+93X09TWgVCKHo2jthZbgaCbN6q0hMtksmsZapr/+F5g7C8OIJdcGOLo7TMLtQWOzYj3TSWh2geMHD7GePUUsHKHofDemntN45xeZ/NZ30DqspENBpIoStm7cwVBchNphIxmP4x8bR3+ikaSc48H/9n+jq6tCZbdSNHAe/9gkuUwGdBrs7a08+qNvoLZaCDxZRihxsnv7Poa6amwnGggvr6E1Gj6E1vuPFO/9KxSSeP8Fhbn6df5O9OJn4rk/6ff3dgkdKci4D8llsyyNjFFSX4tq+BE5ScSzuoazrJSE+xDP3h77KyvUdHfhvTdKLB4nl0rR0N2FQhQ4Hh3Hvb6BZNBTfOYUalEimYhj6e5AodFwOD6Ns6qS4MwTPCvrZIIhlAYDLV/4LOtv3SKTSbM9NIJaFFkZGePk1cv4H04iiwLHbjfBpVViajXkZY7W13GVlRLz+QmnUmjHJkhGY0TcHqo6TiKYjMS2tgl5jvBNzKJRq0knk4RDIQK7bpQmI1lfgJ3JGUpPNBGZWSCdy7L8139LUXUlFZd6EYZGMHW0Et7dJ7q5w970PEaDgVwgyP7SKiq9Fs3CMuUX+8gEQ8Q9R8S39tAbDcgaNbNf/3OKmxpJPJ6iqv0kKpMRTU0lRwuL+NxugjPzCAgc7e4jSs9e3UaWZbL/RLLz7wVZlmM/9vCbP/WFPwHPvdH3Dlzkh4/HMPV0kfAHaBJEsok4lp7T+Ld26PqNLxBeXMHedwZxbxelQoHjbKGGr9zZxxwKEwlH0LgcSOVliEsrOM6eYnfwAfbONux6HUf3xyi+3E8uHMVUX4OushxhbQO13Yalo5Xg3j5VA73ENrcp7e5EbbWQiSdQ2CyYmxs4eDjB2d/6ConNHaxd7fhX1zEVFWM63cHB7h5KtQbLuS4EQUBxf4xkPI7eaSc4NUvvf/2v8A8/wnK+IG+Vuf0A384+hooy1K3NaIxG8qk0plNtxB9nUIoS1p7T7N68S9MXPkt0bhFbdydpf4CGT1xHyssYT56gNBgim8mgr69FqdXibG4kE4lh7mpHbbeiicTYmZjCUFNJNp/HIEnEtvZQlxYh5vKc+MwnkbJZTK3NVESi5HL5D6VO/0/oJP+JEAQhwk+Og95tzjG918/4pbj3giBsPRWqmH6XrOAXKYTxQSEXO4itbxEan6Fo4BzW0+0cj40T2dpFX1JE2ccus/f2HbRKFbaGOuJ7hanH6OJyoa30XBex5Q32h8fQFTlJxeMoJQm12YSoUKAoLWL+z/8Ga9dJik93sPi912n63MtgNuK5O0zcF0DUaSi7OsDR6GP86xtYmuvJHB4DICXTGEqKSCaTpCNRUtv7VF+/RHhnD2dpKVUvXuXg9n08E7No62vQ1ldzPL+I3uVEqVajqqpg842bHA0O4zzTQfXFAh/A0dAoltPtiHYLiV03ykQS0agnFQhi0OpQajXE/UHyuRzKRBpnazOxQIjjxRV05aWUvXSVo/ujhdFgRFwXevA9niKbSuEbfkj37/0W4cVVosvrGCrLKb54Ht+jSZTxFPameqL7Hg7GxtE01ZHTaZ55G64sF2r177X+MUOWZaMsy6afsIzvx+DhlxvTX346A/wuWcG7QhgNwO2nj+HvC2H8NgWRC35MCOMcBbqh//HdPxQfBH6/j+T2HhPf+i4qrYbA5CypIx8pScK3soZCoyYZCqOtKGVnfIp0KsXh9Bzb7wz+qGtPFEUSoTA6tZry/vPM/+V3MLQ0ElhcIfhwkqz7iMDhIZ7Rx+zfGCSVSBKdXSS3e0A6nmDx+2/gGXnM/s27SCo1U3/xN8h5maScZ/PGHczthd74skt9bPzgbQx11ZjLStgevI+xtQlRpSCnUrBye5DwwgqJ9W0WX3+LbDhK6OEkciDI9tQMoiSSiyVwnWzBN/sEU0UZklKJve0E3uk5RIOekt6zxBdXkTQFnXhbbzfHY+MobBay6TRau5XlH7wN0RjhyVl0JS5mv/lX7M/Ns/nmTTKyzNj/+Udk8zniCytorRY8q+tEJ+eIjM+QjqfYX98g/HgardHA9tg4geknJHz+D6H3/iMV0//c+Mfk3r/C3zHffpMC6+1/w48JYQBjgiC8K4RxiadCGACCINykQDb47Q/ypnqjEVvvWZQaDUqbFfPJEyRDYYIz8wT33Wy8fQdtqQtSGZw1VZirKkjs7LM5PolepyMhSSCKxKIRPFs+ymWI+Pwcjk/jOnMK3YlGdgbvU32yjfKPXWbnjZtUnj2NtrEWjc2KnUI7rrPtBJbGOnwLSzhra8ggk41G2VtcxmI2k5AkZFHkYGMTpU5Pcs9NPBIhMDlLEhlJIVHR1YmpoQZteSmBPTeGpjq0xS727j6g/oUrqEuLCR14kFbW2Hr4GG3veXLBMIJSgXdnl6D3GOJJVscn0JotuCJRJFFg+f4ITb09hDweJLMJS0kRmo4WREkCwLHrRutwYD93imQgSNDtxtl3DpXRQGB5FWtZCbpTbWTTGZQ+P9UdJ9F3neTw/kNc1ZW4rvSTiccR/uCP/6HfoffE82DU74Vf1kkvA+8IgjDxVIgC/n9CGMDPK4TxH0EQhN8WBGFcEIRxr9f7966plSqCo+MU9Z8j7vYUNNK1GsRIjK5/+RUcDbVojUZ0ajWNv/Zpssd+0pEIJS3N6E+ewHKuC8uZTlQqFZWnOkhEIrT9+mcxO53orBYANJISQRLZvzuM43w3pX1niS6tAXA4MUNJ71nyoQjJaJSsL0jjqy8jpdKICDS+eB3JaMByppO8XkvXl34No9OOtrUZnc2K6VQbpX1nUYsSlVcu4J9fRM7nKWtrwT89j5zPo5GUlHR3ktndx9nZhmyzUNXdjSCK6LvakCpLabjUT1VLM8aznVSdbMNeVYGhphLrhR6qO9pRlbgouXwBRSxJyUAvsZ2C1sjhvVGK+s6ilAsxeXh8hhO/8Wsk1rYAyB94URn0yLKM9859HBd7IZ8nFY2i0WkwtTQR393HNzLOs8a7ibz3Wh91/LKMvk+W5S4Krvt/JgjCwM947T9Y8EKW5X8vy3K3LMvdTqfz711LpVPobRZEhQL9yROEnizhvjFI6QuXMJWUEF7bJHt4jKmjFYVKBfEkGoOBssv9hKYL2pz7dx/g7DnN6r1hnL1nMJWXkcykie+6OXg8ga6plt3lVZLxxI+m0GLBMLlstlBuKylCTqU4vDuK/Xw3klLJ2tAI1u4OHK1NJOIJYps7pPcPMFRXEI9EOBx5SMuXPkdsfonDwWGsT0MNW88ZghNz5EURW083q999DXVVQSIgGooUaKaPA1S9cAlb7xn2bwyy9J3XUVdVEI9EObx1D2v/WYou9RI+PGLhL76D+VQbmUMv3nujmE93YK4oQ/aHCDxZwlRRhspqIRaN4R2fxtzegqRUQiLJ8fQcuqZ6JLuVrdffxnG+G0mlRM7mCIyMY+xsw1pfQ2JjG63d+tO27xeGd+v0v3LvfwmQZdn99N8jQRC+TyEm/0UJYXwgDA8OkTRq8T94iCBKPBkcomWgj9j0ExAF1icmae49T3BsAlGpYO7OPUq7OzAmkyTzOfYfThD1Bcg/miKTyRBZXichg5yIszR9E0EGwnH0BkPB9X08TTaeIJnNMPUnf0blQB/+yTme3Bumuq2Fo6FRAl4vqWSC/dHH6A0G1EoVs/eHMbqcqCfn0JhMrA6PoVSqcC8uodBqke6NIct58nkZ98oqap2WimQS9/IqJruD9KEXQ1M9y995DXtLE/vvDKI3mVBazBgSCTyTMxw9WURtMiLcHyOdzZBOZwjs7VN26MW7t0/MHyANWFsaiR/7UJlNpC1m/MNjxIJBIqtr1JuNoNUQjcfIe49Je/1IyPj33ThWN4mplOwvLKJx2Dm4fR+tVsvKyEMa+3r4GUpMvyB8pNpwf2586EYvCIIeEGVZjjz9/wvA/8TfCWH8L/zHQhi/JwjCX1FI2oWe/mG4AfzrH0vevQD8dx/081y4cpnXhu9jG+hBoVLRki9Mo73LWtuWyZFJJrD0nCafzVLr9ZFKJIlt7KDT6Zh9+xYdX/ki4ZU1un7nq8QXVrA85cVPKxWEN7dxXe5DOTJOzqBDZTZhqSqHxWU8i8vkkbF3tlK8to6l7QTG6gqyN+5Q99nfwT80StHVCwBkMhkyqRTGjhbCe24sLiel1wZI57IoBBFTZ+uPvAg5m0VjNmHqOU1LJouk02Fsb+Zg5DHHG1sUtbfiuH4JQRBI3LyLxeXE0d+DHpF8Nov9Ui/5bJaj2/epvtiHurYKk8+PwWLF0d1J0nPEzvwTXM2N2IocONpbEfKg0+lQ2qz4F1c4XFtHazTS9MnrHE7OYq+rRVFZispoIPpUO8DcVE8ulaJJFJE0BWntZwmZX8X08Mtx74uAB4IgzFAgBXxDluW3+cUKYbxvKJVKnNcucHhriGwqhUKjQdtcT3ByjmQ0imDUoSx2Elvf4mDwAUWX+7CfOomYzRLPZGj73CvkD47QK9VoTSbSmSypUEGNVZ3NUdZ3jsjKOoJSiaO9Bf/yGtFDL7nDYzq++kWU6QyB1XVc506T8RxxNDGDpe0EoigilZcQW9skGY6gcthwDJzHNzpBfGWD0sv9RFY20KrVlF6/iP/hJOlwhNDWLsbaKhKRSKHUptOSDoWQlEpUBh2nv/ZlYhvb5FIpsuk0aouZRCpFcG0TVVkxYkkR8Z09Dm/dw3m5H1NNJWmfH53BgPNSL7HZBcz1NZzo70Or1aJ12FEbjWgFEUvXSfJHPorPdlHV1oqjuYH00TFZf5DyawMEJ+eQZWi8MkB6dx9kmePhR5i7O0j5Ah+KgOWH4d4LgvB5QRCeCIKQFwSh+8eerxYEIfG0VD0tCMK/+7Frp5+WsdeelqifmdvzoZ/0sixvAB0/4XkfvyAhjA8KUaHAde0ihzeHMDid2EpLOPb6cL/+NvWvfJx4MMDcX7+OWq8jl8+Tl2VC+x5S8RiNvT0cLK+SyWVRlLoo7jvL8f2HpLNZnD1dKHU6lr71PZKJOK5QEKVSyeQ3/oKWa5cRBYGg+xBJFLFcG+BgaRWlRoumpAgAa0MtB+8MkV1eo+yFS8Q9h7hXV1FotbiK+wg8eIRSWWDVLfnYZfbfvIUgipS+eAVFiYvg9DyCSolU4iK2d4AinkRb7KL0pau437pFVhApvtRLenMH/8w81a+8ROjxFAt3h6m7OoCkUqJ3Odl8/S1KTp9CpdMiG3Qk99ygkCi6coGDt25j6m5HslkwlBTjmVsm7PZgO3cKpU7H0a0hlGKh2858vpulP/0WzV/9IqqaSkLjM2hNRiSlAn1HyzOXtXq3ZPch4F1Zq59UjliXZbnzJzz/RxRK0mMUDroX+TlVm94L/5hKdr8UBPx+Ykdu8oKA//CIgMeDoFaikmW8W9sULa0imo2UNjcgSRLF1y8CoJmaJek+xHiqjYj3GI1OR1ahYO+du4Q9nkLSTqUkHouRSacob2vBdrarwK3fWIe2thL/6jr5bJaDnV2UGjV7C0tozWbyuSyiKCGJAplEAu/eHsItEU1VOfaSEvJqFZ7b94kEAugtZiJ37qNTKPEfeMjlcmgfPELUali/P0rbP/s1tHYbm9/9IdbWRnbvj6LM5FCqNezNPcFkMqKUIeQ9Jjy3iLW5gdjWHoIsExx5TCKTIRUMo6soJZ/NoikpZvPtW6CQ0PqOEYHpr/8FJ69fJTIxiyCJuBeX0Gk1JPKg1GjYnJ5Do9eTSiaI+APs37qPwaBjY3yKovpaFJKELPPM2XA/rDZcWZYXgffdYfg0h2WSZXn06eM/Az7Nr4z+2cBqs6EvteIfn6bhhSsEVtcxnGggHY5gr6pEXVmGLIkYykoQ7RbCc4sYWpsQonFQKshlMmhNJqLRKCUuB+aKMnjnLkUGA9bebhT7bsxVFUR29pFlGdEfou6VjxN6OEnp+W72Rx5RqqhGVVtFs9VCZP8Ae083kkZNYGkVs1aHpNVQcvUCgeVVLCcaSfr8WDvbyA0/4ujJAq2/8QViO/tUFznJ+wOYzz/NKdy+y9btexitFtxrq6iLndjbW1EbDXhu3KH4RCPGc1147o3hqqtFV1PJ8ewCVS+/QHxmAUvfGZTeYzbHHrN7awid046o1YIkYbBYKb58gUw8gX97B01bE5JSSeSdu1Sc7UJdXo6q2Elkdx/jzh7Gni5SDyc49S9+k8zeAaZTJ1FrNMTDEdQdrSSDoQ9F1uofQUxfIwjCFBAG/gdZlu9TKDXv/dhrfmr5+ReB597oAYKLy+itVjTlJRQVO4lOPyEVCtPw6stEFlZJHHkpuTaAIIocbe/j/f4bVL50DW0iwfLfvEbdJ17AqFERXVzF0FyPzm4l7g9gzuVILK3junoB2Whg/fW3KDtzGlEUiUcimLNZtNk8MVEgPLtAyccuo2+qwz/yGAx6tDod+tMnUfoDRJbWkY/9aFuaSfn8xDxH6FUqFKc6SRwckj32Yeo9w/HdA8yA5/5DOr/2ZZI7e2Rlmc7f/CKxlXXURgOHgw+wnz9DOpkkur6FTqnEcrkPzztD6PRaVAY9fjlPOhwhPP2EM7//u/iGH6G1mGNmXqAAABtZSURBVAsyXZ98keDcAtlwhNDkHLWvvkxsY5tsIoHl5AnUJUUcvnMXh72fzPo2+qoKkqEwijxonQ7i88uko1EwGjA11hGbXSDm9X0Yyfv322b7nlp2P6es1QFQ+XQO/jTwt4IgtPIBys+/CDz3Rr+7s0vq4Ahqq4jML5BLJPE8nKRuoBeFSoUcS6C1WsgkUxyNPEItKTje3cf0eBqVWkXU50fOZVFoTOTDUY7uj1F0qQ9VOMLGD29Q1tVJdHuXnNfH0fIaWq0Wi0LA2XeO0OwCskZFyndM8OAQw6QD8jIH6+vkMjlqT3UQnpxFFkTm37lN1z//DQBy6TSJuUXsAz3IyxvM/c33af30J5DzeZKCzPHSKjqbBY3dSnJxBVEQ0BS7SPiDbL99h6KWRpQmI0qTkdDGLoJeS9TtIez14l4LUppIoqwsxf9oCo3DyuHkNMp8nsXX3kRnsaBfWUel07L++ttU9p5Db7cRXNlABNRP8xG2i32sfft7VFzowVRazMq3/wMNny8IsUrlJWz98B3qXv0UklJBeH6RbCr1rMv0fICY/j217GRZvvaB312WUzwVppBleUIQhHWgkcLJXv5jL31PWat/CJ57o3fv7SBrNUiSAoXdRj6Xx+R0sD87jxCNM393iOb+XqIr6zj7z+G9P0bZiUZsF87hHX5EaUMd/vVNlHOLuFfWkQG93YaYy3O4tIJao0VbX006HKHm+iUMJiNpf4jM+g6zN29T0dqKoFFR0tKMsrocldWCwefDoNWiP11gMw4ur6K3WTkcn8JsNjNz4xYnr1zEMzSC/WwXZS1NJH1+Yjv7qESR5dffovXqJaLjMyzcH6G5/zzR8RkUksje1Axmk4n0vodUKs3SgxHqursw6HTonA70RU6s57tJ+wKsTE1R1n4S++lONDYLCoWSdDqF+kQDWqWC1INR/OsbEImyePc+Tb09xCZmQSGRQyAWCuJbWccajJBOJEkvrJJGRsrliRz7iU/PgyQR2N1DEAQkxbP9Ov6y3XtBEJyAX5blnCAItRTmSTZkWfYLghARBKEHeAj8JgWFnGeC555E42xvH8pkGmNFKTqng8TsIrWfe5ny+jpMvd3UdnaQy2YLgy0KBTqDAdloILC+icZoIC8KuLo6sA2cx1JSREljHYbONiLRKM2vfgqD045CWciCuzraiG3uYmqsQ9lcT3lbG46TJzBodRQPnCc0URCIUCkkUuRJR6LEPEcIkTiNX/g05vIy8kUOKjrbETUaSq8Uavjm+lryiSTOvrPk8znKTzShbz+BobuDExd6EZRKDN0dUOyg7bMvk0mlMJ3vxtDZQmVnGzqHA31DDQaDHtfAeWLT8+jKS2i9OIACkDQq0vEkktmA41IfoUeTxDyHNL54HbPFgr7rJC1XLpFJJFA11aPvaCUVj9H2G7+OyWhE1VCNUq1C3X4C/ZlOYqEQzrpqVK1NSNUVFDc3YnY6n3md/kMs2X1GEIQ94DwFWasbTy8NALNPy9XfBX7nx8rM/ynwJxRK0+s8oyQe/OqkB0Cqqya+sk50a5eiK/2IkkQ0EkEdjqCrrEBbU4HnnbvkVUpcZ7uQ4nE233iHtq99mbRaRezgsCBXfa6LwNwSUfchWpMJU201+0PDBCZnaP7y5wDQNNYSW1knsrlD1ac+hv/eKCpNQWRS29pIZH4JtVKFue8M/uFH5LM5XE8569OxOKmDQ2o+9SLpcITDm0NEU0kqr14kr9My/fU/p/Uzn0SyWQk9nsbacxpJpyOdy5Dy+ojML1NydYCwRk3kyTLRPTdlL10ntLRCfGUdWatBVChIiyLB9U0kkwFn10kObwySV0gUX+pDFEXydgvbNwZp/edfxrOzS3T0EbbGeozWDry372Fqa0ElKVBZzQQlEf/QMFWf+TjxhWVSsQTmrpOIRgOJhRVSx37sVy8gHBRITJ4lPsTs/U+UtZJl+XvA937KPeNA2zP+aMCvTnp8R0dkN7aZe/1N8qJA4vAIOZ/H1N7C2n/4AYbGWnLRGBqLmd2pWQ5HHxPa3UelN+B/8BCd0YBvahat2YRkNKAtL2bmz74NySSHd+6TFQSQJNwPHnJ05wH5HTdzr72BLEmE5xYJRmPsb2wQ8/rQFbmI+/xks1k890dZHXlILBBg9+ZdEsEgiWgMrclILpMhue9Bb7NyMLdAdHkNslly6Qy++UUScwskslncD0YRnDYcZ7oIzy5gNBqJ+wJEV9aZ+f4PyefzxB5OogjHmP3+D4nsugkMP0KOx3ny7e+Ri8WJzyygs5g5XFknPDbJwa0hfDMLSAoFx4PD6LRa9kYe45uYwX9vDI3ewNSffgshlyM2NY9Go8G7skZ6/5CVoWGCe/uk9z0kl9ZYvj1ENBLBe3eY2NLasw/pkZHz+fdcH3U89ye93eVCb9PToNEgiQLpfJ7g7XvotTpigTD+4UfkbCby4Qh1H7uC3mQikclg6HFgqChj7+6Dwqy6ICIfeDC3NeOqqkAqLcJRUYZ3YZmk20PJU4WcyJ4bZ30dxtZGtEUuErk8qUCAdCBIau+AuD9A2O+n7tOfoNloJOE9xnK6g9TBEZ71DRQqFcZwGFv3KbLhMOWnOlC4HERX1mn+4mfJB8MYmhuIjU2wdmuI1osXCO4ekIhFOdjaptJsxHWpH5VKhdJqwdDWjH98mo4vvkrm8BjT0/bjwM4+mrJSNC47x5MzOMpLsV44R3BpFVNVBaHNHRyX+8jEYhiWVrB1nkTlsJFNJtHNzKHpOomkVBLd2cPkcKCtrqSqrQVZFDG0twBQvuvGVF2JrrWRfD6P8k/+9NlvuPzRN+r3wnN/0gOERh9j6W4nncuiNRkxt7eQSsQpba7HOtCDlM1jaW3G3tpMYmuH2MYW2uIiAnMLWKw2Ol98AaVWi7W0BIPTgbOpkfDaJplojOT+AaXXLxJbXiefz5Na2aD6lZfI7nkAUIYjFHV3olKryeezOJsbKauvIzQ1j+5EI86rA/jHJtDVVOKsqcZVWUHplQtoTAaSKxuUv3CJ9J4HjV6HobgIMRonm0xCMETXv/gKgkaNsacLSafHVl6KtrIc38w8uhMNEIsTmH2CzmZFW1lOIhRCzufxPp6i+pPXST+V4VbEUyhdDgJrGwjROJq6alRV5cTdBwTGJmn48ucJTs2RTaUJjI5TPNBD0ntMNpkks72Hs6qK8OIyhvYWtCfqiTzNXZiLXcSOvGTjCY7Hp1EolM92owuZvPdeH3E890YfjUTQuxyICgXmjlaWvvMa0YUVHFcuoK6uILi8hpTNoS4tlKLyNguZUJjA2ARakxHZbGBnfpFMNsP+1Cwzf/ynRGNRXJf78Y88QqNUorNaIRTh+N4o5jOnAMjGE0TcHpQlLoy1NTz57uuE3YdI5SXEs1nUKhUKdWEIpejaAN7BB2j1Oih1kdjeI7y5jb6mElEUWRt6QCgcZu/eKOuPpji8M4zz+kW0RU7S6RSZcBi9XkfR1QHC4zMo4wkUJiPLjx4R3NhG9XT01tTVQXRpDVU6g9puI5FNcTD6GG1jDYIosfqDt1E+VcWxNtSS3jtAbTUjSiKOy33474+iMRiwN9SR8wUJ3BvD3HuGXC6HKpVB5bChK3KRN+pIrmyAJGG/eJ7o1ByaTPZD0KeXIf8+1kccz717Pz42RkqvIXb7HilRJJtMksxkCvGpTsvs4BD1Z07juzeGKAooBAH30gpVCiXpVJKMKGCwWSi9VmjP3f7bN4kHAnjvPEBSKNmcniWXy7M3/wRzSRGGVBKFXktKJRF5NInKZCTjD2J02rE01nE8OUNoa4dgPo9ar/tRnJtKJDnansdZW0U6n0cUJXA6iA0OI2lUlJw/C0Dac0Q8HCIx/YRMIkk6lcJ98x76yjLC90aJbO+Sl2XKjHrMJaWozSYO7tzHoNcjaTSsDo9iLC8lfGMQpUJif3oenVKFrqwIg93G0cQUeoUKjV7Hwu271J7tRnw4iaRUcri+ic5qwaWQ2Bp9TPnFPqKrG2xOTuGoqSY1OIwgCggIeDa3kEUBVziCb3ePbCaLpHzGX0cZyH30STLeC8+90fddusSbU49x9vfiHXxA81e+SHZrD1NHC4HlNYoa6jB3tKGyFVhwgmsbOOvrcF3pR2U04BsaJVduIXl0jGjQoXfaUTnsGBpqObp1j/KeM5jtdjKZDFqdlsieG//UHCTT+A48nHzpGsejjwtdbVNzFPedQyMLZAQZRW0lKrOJfD6PPhjCWlyE/nQHa999jUQgREtPF5q6SoolgeSeG211Fbb6GtKJJMqaSvQ2C7pYgrlv/Dl5pYKSqwMImQxSXkbfdgKzP4RSIWF6SpQZ2dpBZ7PiaDuBsbaa8OY2Rs8R2pPNHM8+oeoTLxAen8Hcd6bArbe0gvnkCdROBwA1mQyCWo1YXU7m3gjZXBZDTR3FdXXYmhvQNdYCkE1nyWcy6Cxm9N0d5G4NodHp8T1ZfMa7LT8X7vt74bl375VKJaLLQWBiBr3LhdpoQEgmAZAPjqh65eMEJ2Z+NPaZ2/NQ8+onSS6vk47HUVjM2DvaSG/uEhh5jLmrnWwiyeGNQaznz+A620XEe4ycSpJTKLBWVVB+/RJqnZbqqxdJb+2g02hQaDUkkdl76xa6zlYcfecIPp4u0EwNPsB49hTxaAxJqcBZVkZxQx2SWo330TT27lPgD+IdGUPTVI/9fDfB8WkyiST++2Oc/O2vojebkdNp9CYT+q6TxBdWUCqVpNRKUv4A4dUNhGCEhi++CofHCIJAbsdN9aufJD6/hBwIobaYsV3sI/jgEf7FFcqvXiT2ZBkA/9wiyuoKSKWJTM5y6vd+C/ngCFESsbgcJN2eH/3O/XcfYLt4nkwiSXBhBWNTA/F0+pmX7Aqbmn/v9RHHc2/0+XyexMERuw/HiRx4yCaTRCIRgutbqCoLMw+6rpNEpp/gf7KEpr4aURRJhsL4R8bRNtcD4HXvEw2FiSyusvT2LRQaDcn5ReKPZ9CIIkfbu+gsZmLzS/im5zG0NJKJRpn72zcI+wPExmdQ5vL4d/dJzS3hHXxAOJlk+7W3MdZUodBqkC1mtt+8ha79BNYLPUQmZtEplSg0aiKxGBpRgaRU4J2eJxaPM/3v/l8kjYrgyGOymTTT//6bZHRatHYb8UMvspzH2X0K9517KFJpDJ2tAGS1GuL7HpQWMwqViqQ/iEajLfDqqxQoairZGxpG7bKjaqglvryOFAqjLnISi8fQGY2IkoTz6gV8d4cRRQllfQ3xtS2OB4ex9hY8hVg2S3xrG1VpEc7+s8TCYXK53LPbbPlXMT38yr1n6ckTdC47dS2fJnfsI7G4SjoSJfpwgrKXrhLePyATixFYWyMXi1N6oZfM2iZx8hzPL6LRaJA0anKiiLG+muTRMU19PVj6zv7oPY5uDlHb2YFsNXEw9oh0OkNNVye68mIaLvQiajVoOlqJ3L5PSUM9hvOnMQA67zELf/Y3aI0GUgeHKNNpDvf2sG4WkRXA5z4gE4mQSCZI+oMEkklKzQYM5aUQCFL7/7V3rsFRlWcAft7dTfaWhOwSSEIgCSgKIQGBcNFCvY6i7Wh/2BlbRx2t0+mMtnX6p3Yc/3T6Q9uZztSx/eFURxxvdaa2MlaLeKlXLoIiBAIJIZAQISSBhITsbvbsvv1xTuyShlwkgb18z8yZPef9vu/ke2Hf/c5++15WXEXx+jUAdnqrfY3E4zG6PvgEr89H654GFuTl03molXB1NYO79pK04mg0xt533uPKO77HwNF23HPLOPLuR8xLWPgCQVx+L6ULqun6aCvBQICmnV9QMHMmeV820NnUwoyy2bh32etJ0rI4sm8flYkEjdu2U3b5ZcT2NTEQi9Hb0oovPIP+/U2QTOArKqS9vZ3q6urp+w83j/eZv9KLyAYROehkHHl0/BHnUlNXhzUYwROJIL39FC6vI7igmv7uHuKd3bgsi/xgAI8KhbNLkYAfCgK4z0YJFM/AMzNEYEUdZWvr8Xm9+INBhlSJD9hVh3o+3UGofhkut4tg6WxCc+ZQVbeEgvpldlTeijrcQ3GsaBRfuBhCxUS77CIXg182ULFmJUX1yyhYdRWJuMX8ulqCy5ZQWLsYD1BcUcGs69dROGsW5dVVFNXVYA0OUlAxh6F4HIChwSiRhgPMqq0hvHgR4XVriZzpZ/7SWqLxIZY9eC8ut4vAyjoK1qzAVeAnPG8eeTNDJFwu+hoOkpfnIb+okED9UgprF1FYHKL0hvVQWkLxnHLKv3s1iVARl1+3jnDVPPwrl6JVFfiKZzB/aR2uhfMpr60hXHMlBWuWI7PCXH7zdZRVVjKjbjGu3gEK5ldN3RvjfJjH+8w2ehFxA3/GzqpbA/xIRGome5/QymUMdvXQ2d5O7Ew/7v4BFv7wdlzxOIGKcgb3NjL3tpsoCs3A7fEwtP8QZbfeQFXdEixvHqc/3oa3qIiOz7bjX7SQkmtWEW1spnd/EwUVc8gLFaNA94dbCa1ZQTQa48yxrwmWzcadn090MMKpz3YSqF1MyVVLsNq+pvuTbRSvXs7s1cuJHj1G9/ufUrx+LWcHBkgmEnRt+ZDym64lGLCTehbWLyURLiJ64iTxw234r1gAoSIiXd2c/mgrofVrcfm9xM700/3+x5TcuA5xCT63B39JmKhTyaZn606CpbMJlJeSX1hIrLGZuTesZ15tDe55FfS89zHxM/0o0Lt3P67BGBW33kSks4tERyf+Ky9DIzEGu08RazxE8TWrSCaSDO5uoPyma4m0dWBFo8jpPryVc4kNnKX/QDPeeeW4nAIb04YqWNb4R5aT0UaPnUX3kKoeVtUh4FXs4hgTJj4UI9Lahi8cIt/rY99zL+IuCeMajNJ9sJnmja8SqK4k3tlFZ0srx//9Hv7qucTaOjjb1YNHwTO7hGNvvIVaCayeU8SOHqOn5Qhn9h8kqUkirW2cOHCQJEmsU734y0tpe3MzeL1EWtvo6+slcrqXoWNf2333HyB+NoJ1uo94xwmOfbKVvJkh4l+fAL+X/c9sxD93DlZXDycOHSZ2ZgCrqwd/IEjLP/+Fa0YhkdY2gkVFNL3yOv7KCmJtHURP9XHk9TcpWFDN0LHjtH3VQDzfQ6S1jfzSWTQ9/zKaTJKIW+T5fTQ++yLeOWVYvX30dZ5E+gcIXlZNx+b/0LJjF9HjJ5GAD+vESTo+3U5+aQmR1ja6jrZx/O138VaUET3STtvuPUhhkOiRdhJBPweffxXPrJJvdD99oJmhoJ/EUHxa3iSpqOq4R7YjmaykiNwJbFDVB53re4A1qvrwiH4/xc4/RmVl5cqjR49+02ZZFrFY7JvrRCKB26ncMvJ6ZJuqnpPtJbU9mUyeEzU21n1H9p/M2JF9xxo7HEE23D7WHMabk2VZeFJCYVPbh99Tw/82Y/2dRCJBd3c3LpcLVaWqqmrUaDsR2TVejPt4SHGRcu2a8TtueveC/1Y6k+kbeRPKOOJkPXkGoL6+/px2j8dzzpvXcPEpKppQ3cWpwWzkZbzRn68QhsEwCpoTG3XjkelG/zmwUETmAx3AXcCPL+2UDGnLcMBNjpPRRq+qlog8DGwG3MBzqrrvEk/LkLYoTKfzT4aQ0UYPoKpvYRcHMBjGxqz0QBYYvcEwKcx3+oz/nd5gmAR6UZJoiMgfROSAiOwRkX+ISHFK228c79GDInJLivyCPEsngzF6Q+6gXKyAmy1AraouBZpwqik73qJ3AUuwa9X9RUTcU+VZOlHM470hh9CLkkRDVd9JudwG3Omc3wG86hS9aBWRQ9hepeB4lgI4ZdnvAPZPx/xyzuh37drVLSJHR4hLgO5LMZ8pJBt0gPPrceHROAORzXyyu2QCPX3jlbWaBA8Af3POK7A/BIZJrVnXPkI+AdfBb0fOGb2qzhopE5Gdme52mQ06wPTqoaobpupeE6llJyKPARbw0vCw0abF6F+zTS07gyGdGK+WnYjcB3wfuFH/F+AylgfpRfMsNRt5BsMUIyIbgF8Dt6vqYErTJuAuEfE6XqQLgR2keJaKSD72Zt+m6ZqfWeltvu33tXQiG3SA7NDjacALbHEiDbep6s9UdZ+IvIa9QWcBD6lqAuBiepZmdGitwWCYPObx3mDIMYzRGww5Rk4b/cV0fZwoIvKciJwUkYYUWVhEtohIs/MacuQiIk85898jIitSxtzn9G92dpKH5StFZK8z5ilJTf0zdTrME5EPRKRRRPaJyC8zUY+sZSI5w7LxwN4waQEWAPnAV0BNGszru8AKoCFF9nvgUef8UeBJ5/w24G3s33/XAtsdeRg47LyGnPOQ07YDuNoZ8zZw6zToUA6scM4LsV1RazJNj2w9cnmlv+CkmtOBqn4EnBohvgPY6JxvBH6QIn9BbbYBxSJSDtwCbFHVU6p6GtsXfIPTVqSqW9W2nBdS7jWVOhxX1S+c836gEdvzLKP0yFZy2egr+H/Xx4rz9L3UlKrqcbANCpjtyM+nw1jyY6PIpw0RqQaWA9vJYD2yiVw2+gkl1UxzzqfDZOXTgogUAH8HHlHVM2N1HUWWNnpkG7ls9JmUVLPTeaTFeT3pyM+nw1jyuaPIpxwRycM2+JdU9XVHnHF6ZCO5bPQX1fXxAtkEDO9c3we8kSK/19n9Xgv0OY/Nm4GbRSTk7JDfDGx22vpFZK2z231vyr2mDOfezwKNqvrHTNUja7nUO4mX8sDeNW7C3sV/7FLPx5nTK8BxII69ov0EmAm8BzQ7r2Gnr2AnX2gB9gL1Kfd5ADjkHPenyOuBBmfM0zhemVOswzrsx+09wG7nuC3T9MjWw7jhGgw5Ri4/3hsMOYkxeoMhxzBGbzDkGMboDYYcwxi9wZBjGKPPcETELyIfOrnTJzrmYRG5fzrnZUhfzE92GY6IPAR4VPVPkxgTAD5V1eXTNzNDumJW+jRFRFY5seU+EQk6cem1o3S9G8cbTUSuc1b910SkSUSeEJG7RWSHE3t+GYDayRqPiMjqUe5nyHJMYsw0RVU/F5FNwO8AP/Ciqjak9nHchxeo6pEU8TJgMXZ47mHgr6q62klk8XPgEaffTmA9dly6IYcwRp/e/BY7RiAK/GKU9hKgd4Tsc3XCV0WkBRgusbQXuD6l30lg0ZTO1pARmMf79CYMFGBnn/GN0h4ZRR5LOU+mXCc590Pe54w35BjG6NObZ4DHscsiPTmyUe1sMm4RGe0DYTyuwA5YMeQYxujTFBG5F7BU9WXgCWCViNwwStd3sKPaJst3gHcvYIqGDMX8ZJfhiMhy4Feqes90jjFkD2alz3BU9Uvgg8k452BvAD4+TVMypDlmpTcYcgyz0hsMOYYxeoMhxzBGbzDkGMboDYYcwxi9wZBj/BcfFZ0HlRaBTgAAAABJRU5ErkJggg==\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "model.update_until(5000.)\n",
+ "model.quick_plot('land_surface__elevation', edgecolors='k', vmin=-200, vmax=200, cmap='BrBG_r')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We'll have some fun now by adding a simple uplift component. We'll run the component for another 5000 years but this time uplifting a corner of the grid by `dz_dt`."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 21,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "dz_dt = .02\n",
+ "now = model.time\n",
+ "times, dt = np.linspace(now, now + 5000., 50, retstep=True)\n",
+ "for time in times:\n",
+ " model.update_until(time)\n",
+ " z = model.get_value('land_surface__elevation')\n",
+ " z[(y > 15000.) & (x > 10000.)] += dz_dt * dt\n",
+ " model.set_value('land_surface__elevation', z)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "A portion of the grid was uplifted and channels have begun eroding into it."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 22,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAP0AAAEKCAYAAADZ1VPpAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4wLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvqOYd8AAAIABJREFUeJzsvWe0Jdd15/c7latuvu/dl1MndG40gG4kggAIMICZQyuMRsmzJMuekZbHlu0Zyfpgjz3ysmZ5RrK8bHmokaywZCUORYIgQAAEARBE6G40Ogd0fjnffCtXHX+4FxREgwRA4ZFD9vuvdda7tevUqVP3vl1nn33+Z28hpWQTm9jEzQPlB92BTWxiE99fbCr9JjZxk2FT6TexiZsMm0q/iU3cZNhU+k1s4ibDptJvYhM3GTaVfhObeJcQQowLIZ4VQlwQQpwTQvyznrwshHhaCHG597fUkwshxO8KIa4IIU4LIW7/QfZ/U+k3sYl3jxj4b6SUu4G7gV8WQuwBfg14Rkq5A3imdwzwUWBHr/wS8Hvf/y7/LTaVfhObeJeQUi5KKV/rfW4BF4BR4NPAH/eq/THwmd7nTwN/Irt4BSgKIYa/z93+FrQf1I1/UOjv75dTU1M/6G5s4l3i+PHja1LKyt+njaG8IsP47evVPHkO8N8k+pyU8nNvVVcIMQXcBhwBBqWUi9B9MQghBnrVRoHZN10215MtvrsneG9w0yn91NQUr7766g+6G5t4lxBCTP992whjeHjn2//Lf/5k5EspD72DPmWB/wD8V1LKphDiO1Z9C9kPjP++ad5v4qaCIt6+vBMIIXS6Cv9nUsov9MTLb5jtvb8rPfkcMP6my8eAhffieb4XbCr9Jm4aCAG6Kt62vH07QgB/AFyQUv7bN516FPj53uefB770JvnP9bz4dwONN6YBPwjcdOb9Jm5uvNOR/G3wPuBngTNCiJM92X8P/K/AXwkhfgGYAX68d+5x4GPAFcAF/vF70ovvEZtKv4mbBoL3RumllN/krefpAA+/RX0J/PLf/87vDTaVfhM3DwQomxPajZ/TCyFUIcQJIcRjveMtQogjPdbSXwohjJ7c7B1f6Z2felMbv96Tvy6E+Mib5I/0ZFeEEL/27ffexCbejDdG+vfCkffDjO/He++f0SUvvIHfAn67x1qqAb/Qk/8CUJNSbgd+u1ePHtPpHwJ7gUeA/6v3IlGB/5Mu22kP8FO9upvYxHeEKsTblh91bKjSCyHGgI8D/753LICHgM/3qnw7a+kNNtPngYd79T8N/IWUMpBSXqfrDLmzV65IKa9JKUPgL3p1N7GJt4QQoKlvX37UsdFz+t8B/jmQ6x33AXUp5Ru8qDeYSfAm1pKUMhZCNHr1R4FX3tTmm6/5dpbTXW/VCSHEL9HlPDMxMfH/O58kCS89/xRLV4/x6qvH2Le1CMDZK8ukacKBW0YA+NLXL/DBe7aRsQ0AvnbkKh+8axsAV2aq1Joeh/d1u/bki5c4vHeUcjEDwJefO88nH+waIqu1Ntfnqty5v9uXI2fmsAyVW3cMAfDo8xf48N3bsUwdgD997Bj/4KF9ZGwTgL9+6jSffXgvioCTry/Sb0aYho4fxjx9fIFto2UAWm7IasNnYqD79V+abxBFEXun+gFYrPkMlywAgihhbrXFtpHus680POIoZqS/e+2payuUsva32qr7UOxeykrdI04lI2UHgBvLLbKWRn/BBuDCbI2dY0UUIYjilNnVJluHu/eZW2vjeT47xrt9eualU8w30rf6Gd8T3Azm+9thw5ReCPEJYEVKeVwI8eAb4reoKt/m3HeSv5WV8pYspx6F8nMAhw4d+jt1/vyP/28uv/ooD+8z2G5pnKXFngEDRRHMzKv0ZRX2DXkcudjgM/dVCKIW+4YcTlxukNFj9g15AFyfSxjus751fDynM5L32D6k8PixGnvHDEYzDUo5g7+53mLHWIF9Qx7HLzd53+4ss2spewc7TK+4PHigiAwb7J2wOXm1we07+plZWOOzd+ZQFMHCnhJXppf41B0ObiUkWp2mrAmevO7QZ4aEy+cA6Kij5CwFb6l7rMkBHFllcVWhEC9AqFD1dGwlpKaOoKc+ncU5FCEQ5jgmHt7SDI0kz6QTs9wJaVbb6GGVxJ7Aq8/gRip6ph9Ntb51n5w5hkKIt3SNNE3JaxVqaws4SZ0qFWxV+1Zd2xpn3XNxF88ihEDfwJFWsOnIg401798HfEoIcYOu6f0Q3ZG/KIR442XzZmbSt1hLvfMFoMp3ZjO9JyynnC3ImxFnZ7vGxx3bHS7OeyxXPUb7FCQKfhjT9FX2TmRoBt3/ysWmxt4Jh9V6wDMna9y9wyQJO3hBzGrNY+9Uhmo7ZXrZZahs85HDA1xaiHjxbIPD27tD5FLVpx2o7BzW6Lhtojjl1HTCXdttGr37zNVUysUcD+3ReeZcAEDGMnjwFsmXj7tMDNi0EpPnbzhsMZfIiBZBDPXQICubeB0XKSXVOI+TrGOaGk4wT00ZJaenCLuPVmSSEW1ycg1fKeJGEi1uo6oaYZyiWRlMXAb6irh+QqSXSOKEME5JnAGscJl2q0EqJdU4i+4v4UcJANU4Ty5dBz1PmqZYhkbUqRImCrXIQfNXGSrY+HoFKSWdYAPZqWJzTg8bqPRSyl+XUo5JKafoOuK+LqX8aeBZ4Md61b6dtfQGm+nHevVlT/4Pe979LXS3Jx4FjgE7eqsBRu8ej34vfX1gf4GhvOSLRxr0F0xWWyovXwo4MGkhheDxV1s8uK9rurpeRKMd0p9XuHNXmYsLIUIxqRR0Hrq1xNnZkG9ecLlti00iVU5ej7htSsfQFJqBwI1VRss6cSp56YLH+3d1TfYP7M/y2LF1tg1oCCFoNdskSUreUUlSSTFrMF6MOHEjRgpB3jG4f4fkpcsJR69H9Ck1TDVlKh8RqjlEZhBLtjDjKn5qYGULZNQIUDE0hWIyR10bIUoVRKaCETexdAXVKhBaI5hxFRSNjjGGE3bfpZ1Oh7yo40ZQa7q0jVGyvXOZtIovcljZMrqIwV3BlyZOvoyugtuqshyXMf1F+kyP2OzDyPZh4GPZJn6i4UmLdGN1ftN7zw+GhvsvgF8VQlyhO2f/g578D4C+nvxX6e1FllKeA/4KOA98FfhlKWXS8wv8CvAk3dWBv+rV/Z4wMWjzqcM5vnq8yaXpVcp5EyEE56+tc3BbFl3rflUydvnqa3Vu32qiKIJnj83yvp7i2pZGy1fJZx0URfDK2SVum1Q5M+3y0qWAr798CUURvHwp5IlvnKOUVXjlcsSLlyLOzCmceH2ZOEmJ45RDW3X++Ok59o5AknZltiE5eWmRV87O8ZWTPsemVQw1xfNjWqKPZd/B0AVrnoYTdWnfJSthOXDQ/CUUBaT4W/tZdtY4d3WJKIroqP24qUWt2cZQUoQQLNV8stR4YyOJEa3iSocoilmutUmTBN8cppOY2LqgGlhoQfe+RSMhNfsg8ZFS4hgKbT/B1/sJpUHVBT1c73ZEKNjRAvMdm41MwyDYdOTB94mcI6V8Dniu9/kaXc/7t9fx+Vva4ref+03gN99C/jhdiuP3jNdOnOXrcwtsHy8hEQyUHV45dQNT14gShbmlOgv1AZbqIUKk2JbJidfnebZY4MqNRVQFvvjiCoVikYyt8MKrV5gaKfA12cfCUo0TlzXu2V1g+4DChbEi2/tTShk41p/nwLhGKdt9mUwvewyVHLK6z1eOd8hmc0wvNjgxU+HlkzcgLDNVMbh/l83jr4XsG4LRYsjl+Q7FW21GCyErTZOvnjdptJsM9Q+T9Nwh7aUlZHkAN2iz0kxQK5OINCDPKnu2DaGnPnayTjtMWWqB66tkrBLVegtLz2GZXSdcKFJqzQ6TmTZ7t44jZYodzNGMJatKiWqrw0B5iAhJmsScu77KtpEyHhUywQqTw1vQWtdZ9xVWW01yo32kWoGV9Tal/ASK3yLaOB9el5xzE4zkb4ebnpF3+237ONW6yPZhnUpeY37N5cc/vJtqWyKSNnfvG2S4INg21PWkP3rE5/a9E7x/l0oSlfnFj4zy3AWfD+7vevTPXylwYFuRncMKeXM7N5ZDHEvnqVNtfubBAV68ItHWUv7pJ0Z5/KTPJ+/o/gQnZxL+s0eGefFywicP63ztdJupkRKHJyIsdZx6J2XHsMbjr/n8pw/2881LMYqi8PpSwq19krma5Pxajn2VVVYaJrY/g6FIlsM8u/piglSihVWgiOXdQBGClBRNhiSaA8k6oTPCHmOFSCsQSINbB6rUtTKZeBWNmLo2Sjlno8kmKQme2yJSNPJmjIx9BnMK0l3BkC5BIsnaeQSQ0yN0oeAmEg1QssMcsOfxVAXDmyGMS9jhKlFifUdu63sBATfFnP3tsOnLBD52uMDzZ1p4QcKxqzF3bDVRU4+mr/KRQxUuzbqsNSPOT3fYPuIgkoAvvLjOQ/tsNE1BExHL9YjHjzf4ifv7ma9KXrvcYOuAzo/dW+TLx1p4fkySSurNDuvNAFVVODAmODkds1oPGO3TUBSFWivi5DWX0X6bH7u3xO8/ucSBUcFQLub6WopmZLEMlQ/uMzm/mLLWTPj6NZP5mmSXPcdSU7Kn1MLTh0jTFM3MkjNjzGCF5ajMqN2gY3SXFdfjPEa4StRaYSEskZM1DCUlwsDREhRFUE4XaYp+VqICTryGHS2zHmeJvRpFtUlodwPAaJkKg6aLtLpLb64xSn9OpySX8DyfljFGu9Ohro5QSJZRFYW0s8KsX2Iq06Sl9hHF6YbO6aHrvX+78qOOm+ARvzuWllf5/DcWETLiN//0HL4f8vWzIdMrHldnVnn2XEiqKPzJk9M89vICqy3JSjPi0o1VXryc8uQpn3o74c++PstQyaKY0RkvxJybccnaKo8ebTBYtgjCkOfP1jl/ZYX5lQZPnY04ed3n3PUqz5ztcMsgnJ/18TyfJ44tsdpSeOWaQrXpcmxGAUXnxI0AS5e89HqLx09HGJbD6avLaKpKkKi0AoVAKWLrgjCWLCUDOME8bZlDy4/iBwGuNoTbqhEYAzj5ElIxiLQcrU5IbA4QZiaZXmqQKDa+M0mYmURIyVozQjrDkBlmvp5ipm0Aok6VJT+DHq4CXWffuuzHDpdYb3mspf1g96MogumlBm0vJHTG6JhjpEaJphsSOROoMqXZ7mx4ZIlNR96mec/gQD+3v3+Ia4suQTjAXbuybBk0eSwoMNBX4AN7DcCg1uxQcnQe2GPSaDkcfmiKOJXs320Rxwb/8k+XeH1mnaVGEdu0uTR9hRcvFPnoQQdDV3lBG+CerQLTyhGlgg/v00nSIs+drvHiuVUKZpGDWzM4lsrd+ye4f6fg+bNtfuEjk0zXUrb0wfMn1ljWVX7s3n4qBYOvn3M5tHuYB8ZbICWPnc2yUO0gKhPIwGe17mH19zNmrlPD5FP7VK61DUwHrrdiqi2XiZJFMWuTcyxy0SyRl9Jf6ENPGhhBkzRNQR9j63gF073RW3YrshIVKNsZHM1g+sYKxfIgHhKv1qHlepRti3Ixh20oZMJ5iGHXllHiGGxvGiEEVVlmrFLA9qZRIomq6iQbOKcXN4lSvx1u+pFeCIEXJLy+kPKPHhri2iocfb3BgSmDohmwUgt47Gidjx7qR+g2aZpSyOrsmcyyXPdZacQ8+mqLf/6TO9gyUuJjtzlEocuDt49zZa7OxcXuf3EYwxMn2tyzQ0NGHVpujKoI2rHF+/YOcPiWIoMlm2wmixfEpGlKJA0mB0xIQoJEZdvkICODJco5nThOQTE4tM1mqaXw4qzD/oksO8eL7LTn6Mvp7J3qY0+hRiAtBrMKWTUkkRJb8SlkDLaOFNGET0bWSTpr3bVzKoxZVTqpTSKhoQ6Ri5ZIku66e0MZYFuujZPJYQaL+PU5JssKSRyitKYpZw2G+nIYmkKJOonXINK67DtD17DDWTxjuPvyME3SNALAs0YxdH2D5/RvH0DjnQTR+GHHTa/0AF95tcVHbs8C0Gp1WGurTPQbHN5Z4M+emefA1ix9OQ1b8fjSS8scmOgaSDuGNX7nry/Ql5HkHB0v1njhfIttQxk+cVeFgf4ieTvly695TM+tUc5bWIbKhw4WODmb8rWTTe7ZofGBA3lOzsKZG222VlJKls9fvLDGXVu7/bt/j8ORqxFZx+QDu1SO35A8dcbn7kmJFyr89dE2O0ttRjMuSeSx1NaYKsSMmTVWfIfU7GNAqwIwoq0w62ao2Al2UqMtCzi0GXY8IqOfbDaDqgj65CLX2nkyaoCmpARBgB9JbF1BU1KcYA7fHAZniKLm0qpXuVxzUESKIhOWmikyDcmrLu3EIEwVYr+DpSmEgctC1I/pLxD5LrVAxaFDFKeb5v33ATe9ef/kM98gh+TZcyFxknB1vk4SJ/heEU1JWVhtcOxSg0uLWQwjy4nXL2JnCzhGwmBeZde2EdbqPo+92ubY2RmG+7NYusANFIbyEd847dJXLnDxxgqOY/ANVDQRcfbqGnumyvTnugvDYehypaWwZyRgqSm4Ntfg+EA/QnTN0vnlJpCgqwMcOTOPABx7CL8dMFbJcn5NJZPJoIsVlsM8Sc0l1Yucml/n8C6DOTkBEiIrZa66RDbv4KYpy+ttilMTELmsNlMcsQaFMcIwJYwarLkWxUyFoOWCM0opnAchiFNYWG2RomIOTZC4awyWs1jhMmEgyGdK1IMUO9+Hramcva5TyYeI8gSWkMxMr9A3MYkaLFJPckSeJIzjDVX6TfO+i5te6R+6/x5ee+GLPLzPQAhBmg4RRQmfujPH/KrLluEsiw3BB/ebXJ5rs2Wsj1snDYZLGmmastwEP5R8aL9JGI+RtTUObrFoexEzocQNEn7mVpOstZMwgft3qiQJHDsvOXlpmfVGHl3XmV12abRcDm4ZY6jgkdndz2gxZfuAwA9jfL9Ixja4dxtML+aYqFjcuw2+dMzg/VsiqqHO1sI6X1tOWW1G3DOakDFrNNwcA7bPiN2m7qacWi+yY8ikwjwrEkr5HOVohiSRnGtksHJguXPoacqWgUGEZqK2Z6j5efSwTWZwjDj0Qe2QzToUbA3Tm6FQGsdrt0iQpPYwjlclY0mMaJ40SBnpHyBvqzj+DGtRhoGcguovEkYxQRIyYXUwNGvDY8Qqm1q/ad7rus79exyePePhhzElR3DrpMr52YBjV1z2TGbI6DGzaxEXF+Aff2SMFy+0CMKEIxfq7BxWiEKP589UObzDZrIsOTuXUMqZXKvqTA05fOlIlTumFNTUo9qKeew1l194ZJjJkT4e3OsQxJJP313hs/cNU/dUap7Jh28vcWUpoNpOeOJ0wIO7NLww5alTHT68z2atGZOmEkNJGMhrrHpdVqDmlDg85HHdq9D2U0azIdN1lbm64GK9yDZrkZ2FJjUG6ZBn2KjRlAVSYMeIjWbnSSSsxwWMcBXXC9BUweRgicFMCklMJlkn1PL0q3WkqtOKFPSoTk5UCdQilqlSMgNSuxv2va6NUlFWiQKXSM3i5PoYsj0SawgjU2YsE5FYFeIkYSO5Od3IOeJtyztqSog/FEKsCCHOvkn2Pwoh5oUQJ3vlY28695aBYH4QuOmVHqC/YDBckPzel2c4uMVgaijD9ZWYjJNDVQTv21vgL5+5QTkTI6XkM3cWeOqky5qrM1gy2VLR+MapZdpuxEifQbvt8/zpGjtGLLYP2Zy+vMZTJ6r4QcT//jdX8HyP07OQy9j867++Silnc21NYd01OHJuhXNXF/nikRpRlPA7X7yK7wWcmE54+dR1kjig6Aju2y74ixfrDGg1ACYzdU4uqBhRg4IlUd1FvnpFZ6kREkSSx8+FqARECSiKgh8m6KZDxhCkZpGlpIIdr1KWC7T0UZxcCU2BXLLMvF9ED9bIiiara6ucW3cgclFlSKfVILGHMNMWlqbQiExwu/Ra14+oyT5y6TqqIsjSZK6l4Hca3fOei+PYZI0IL0wIo2TDHXmapr5teYf4I7pBXb4dvy2lPNgrj8N3DgTzHjzS94Sb3rxfXasyPevjByEr602+frqFbWdYXm/SaLZR1UnarkeYJCystPhiI8aybWptl+WVOoXsFCVTYbAvR8GSvHKhRifSOHZunsN7RnBdj6nRPh65vYwi4MZKyK6JEgcnNVw/5uzlLIe3SLKWAFRqrTyqWuLDB7o78Toh7J8w2TuqcXmxQqvj89hrbUzH4cSlJeSWPi7VIJWCF07PsWtygLUoR5qELFTXOdgfs+jClopB4re5GGbJ5osYesDl+RpqNqXpN0AzWWnrCLNAEAW4rs/48BQA1etLVIolvECnz2nT7uhEQZt1u0KCoF5t4gxPAYKV68vkx/oINJWw6VFt+owN9NMIfKLQJ41T6nGKKA5haBqzy1W2jExhkhLHaxvLvRegvEeMPCnlN94c0u1t8K1AMMD13v6SO4GX35POvEvc9Epf6S8zmrH4/Es+/+oXDvDMGY+H9hm8rFhcW1J5eL+JH6pUCpOkCO7bZTG74nIytilmbe7fbfLlIy0mKha2bfChOzK8dL7J9vE+PnNPH0+e8vngPoPHX+vwidttDt4yQBD4XFxQWKk2+OkPDHP0quShvXB9yWO032J2LQTg8nyHQ9tsbqxB0fI5uMXmyqLGJw6oBFGCWyvRaXuMm8sgYGagnygMKKRLAOwaH6YtfVQnxy5tnvPtQcadBUTSYtF3QNVxU4ulVoiixIzbMYZYo26OUs5nMDs3qKUFtpRiSGMyNGmlCmP5CEUr4iRrNIwxHMfE6HTX8HeMjhDECU4wi6kPM1IysJMqedUnNVP8wgiqqmF6c1T9Pob7i5juDWrKEIqqgtxQAx9l4yl3vyKE+DngVbpJLmt890Aw33dsmvfA48dqPLQ/g6p2KbWrzYi1js69u7OcmQ548rU6t281cAPJqWttrq8JPnk4z1gpZXY1wLAyfORwhfPzCWma0op0yjmNI6/X2T2ioqoKtwwJXrzgktUj7roly2rD4/ysT3/BJIp8Gp2Y8wtwYLw7H15thLy+orBjWMcQLt+44LNnROOubSqn5+CrJ10OVlyylqAVmay2JQ4t0qiDLzLUfIWsrBHr+W8FpthqLLLGMG6Y4socGT0lSDWm+lQGnBTFzNFM82RlHc/3SdMUw3TI6zFupJCkksQeIitcFDNLI8nhJGvQWSRQctSSInqwghMu4JojWKZKUdRoUSKVkhoVDH8RhEacplimTug2WA0dsqJNkkg2mhr/Duf0/UKIV99UfukdNv97wDbgIN08df+mJ99Ma/UfE86cvUhfXqec626oeejWIsevhuQzOluGM1xe8DBMmyMXqjx39ArPn1xh92j3a7t9R5HLSykZQ6AoCm1f8MTxJvfdojPer3J9OWKsT2N+zafuKTz64nVmq5Jnzse0A5XpxTpPnQlxnCz/+q8uc2hrt927d+U5N5fSn9NYqno0PI31hstLVwWLDcGlBReFBF0THBpPmI/7ueH1kVM6DOht6nGOQO8nq/osVD3MaB0pJRJBu9nkpbkMghTLyjFXSwmVHKGwubae0oo01LgN7hrzYR9W0N0vX0wXcY0hDKVL0ul0XDQrhy598npCpBexc0UUEhKjzNxKm/WGi2cMoUfrtLRhLMtEUwR+ENBUhjGDRRxLp+apRFhEycZy74V4e4XvKf2alPLQm8pbJq/8dkgpl3vbvlPg9/nb3aT/UaW1uunNe1VT+caxRVJGkWjEqeC183MM9eUQygAr1TZB4PPTD09Q7SgYhs7FmRaveD4Zx+aply9xz74hjrwec+7SQjfART7LjfmUhZU6X3oFJisa2wYc7rttgok+hf2TGl886vGxO4fYMSLJO/DyKYcTV13QBY6p8egLV3jozm04hsonD1l89ZTKvVsTOp7k2eNNfN9FUyokEjStw9XFFtvHRhGKSkaVXJlbJTM+wczKCklkcwMFjZBWKBns666nryUFRssaZtomqzTwNYNOoNA0itiFLPPzNfrHJxFCIITg6o0lRvsLJMYoS0t1dF1jamgMhMbqSp0oTig5RXLJGmMD4yiKhu3P0Iwlc40WuYyBVp5keaHanWAXBnGCJcZK/SR+HU1Iog0f6TdunBNCDL8pXdU/AN7w7D8K/L9CiH8LjPC3gWB+ILjplX7Prh0cLFzkmxd8PnG4u7XT9UZRNY0H9pi0O2XiyCdjKdiWxUA+oZgr8P7BrmWwWh1nuGJz5w6bufUKWVvj/Ts1Gm2HYlbnA/uzZG2NL7zS5DN3FvnmxZCXX29zcIvNRF+Gly/HrDc8fvEjI8zWBbdNmVyc89g1WWa12qKUKRHGKZbwaHQMXr4S81MPVDgz7TGo1hAyYH7Z4sCYTpB2sKM68/EQfQWHYjTDvi3jCJnSLxcI4xRhj+B2XGIgky1gezPUkwpZDPKFfkaiOWrqKMJdoODkUOMWRlSjJobYOyAJDZ1MMMfE8CjSXYdYxwjX6CuOkUY+WVq0xAh5f56a7MMUktTqZ6fj0pIaanuWgpNDNyzySgspwA8DVKcCYh4pkw37rYUATXtvlF4I8efAg3SnAnPA/wA8KIQ4SNd0vwH859ANBCOEeCMQTEwvEMx70pHvARv22hNCWEKIo0KIU0KIc0KIf9mT/5EQ4vqb1jIP9uRCCPG7vbXM00KI29/U1s/3kmNcFkL8/JvkdwghzvSu+V3xXXIFfzdUihYfvSPLF16q8flvrvLgPpvAd3n53Dq7xzQ+dqjIy5dCdEPj9u15Tl7tkKaSr52s8dDBHAvrAS+ea3DXziwaEaevNZisaHz8UJ4XLgZ87VST+3Z3o+ksVT0SDKYq3a20rQCKOZP+osVaW6HeCpmrCf6Lj49TLma5Y4vgqVMuierw+08uUTID2l7EcEnni6cC/uxIhOEtUdbaCKEy6+bJiQ5FucZKmEeLm3hhgpSSuXiYbLjAoF6jpfQjgyYARblKU+lD01UQAjtY5FLVRiFhvpYw65fJyTV0GRB1qkRYGLpOXvPx/JDlMI/iV4ncKhfXVOotFx8LI1xjxi+SUWO01KOQLOOaw+TyBcos005NqmmBZmyQDRdINnhfraA7DXu78k4gpfwpKeWwlFLvhYX7AylhlhZGAAAgAElEQVTlz0op90spD0gpP/XmJJVSyt+UUm6TUu6UUj6xYQ/5DrCRI30APCSlbPfS+n5TCPHGw/53UsrPf1v9j9I1e3bQDWX9e8BdQogy3bfoIbpv0ONCiEd7XtHfoxva+hW6EXQeAd7VF3ruwmWOrzQpZC3GBzMcO7vAc+dy2BmHJ49c4TP3T1E3U+IoAEUHTB4+4PDsmTZCGMwutWh1fM5faXD/4e1YmRx/9dx57r1tKwu1lBuLNVw3QDUmubQU89r5GW7bPcKTp0HTVJ47doU79ozyN0c6zCxUWV4r8o/u74awvm+nwZk52DWscmk5pe36KNIiTSQDOZ3JsQqDeoM+Q8FNNDKhypnZDs7YAMIocPH6CveOBeRpMt3K4pjdwJodmeHGUptdU/2EahEJyLbLzGKDolNAxA3KxQwFpUnTT2gFEr8whCYklreKbwyghi5rgYWdL7A4u8r2fpWckaJmC5j4SK9BM1apuQH9uTzSmQChELQ8lpp1JkYmcQTMra2QdVSi7BbS9Apyg6NobDLyNlDpe0Et271DvVe+26v808Cf9K57RQhR7OX4fhB4WkpZBRBCPA08IoR4DshLKV/uyf+EbuKMd6X0O3ds4dWFE+wYVhgpGfjRKA/tM3nsaJ0924eZqGicu95iesFlqdohY29HAs8cvc5tOwfZPVpgpSlRlDJ3bVPRNYUXjjt8cJ+Joat8ycsgKkU+sLv7VUfxLiDlA3stXD9GZTtRKvjQAYvnT0d88/Q6Xzoq0HUDTdd59dwM79tT4WO3OqxXK5SLFlP9CmemPe7bYXBqpsSObEgZyTdvKNwyWmBAzLLm2+Qdk1qkozlFLs+tsWO8QD2MKaoNdk6OE8Uptn8DAFcbZstoP3r7BjVrlP5kHk/to1DOYrotLG8BTUmpRSqL1Rq2pTLqxBjBLIVsAV22iaRKRrhgFjHTGpE9xHBmHk8pY7gzAGiij5GBIpY7TYM+dg1AR8mgt6+jKnSN3w2EIm563/WGZ7hRe6l8V+gq7pHeqd/smfC/LYQwe7JvJbvo4Y21zO8mn3sL+Vv145feWH5ZXV39O+c0TeM/ubfE+ek2p6cjwijhhbNN9m/JUrK74fXvO1BhcKDEZx8Ypy8LrZbLf/0TexCKTl9eR+gOn72nj5de9wG4c+8Qp6ajbsz3nMOBcYWzsyGuH5MzE5ptjyhOefJEm/t2W5SchJn1BDfUOLR7kPfvKfCJO7KkccgvPjJFwxfUOgk7xrJcW+++PBbbJhNl6HNCap5KGKeoQqBLjzVXR7Vy7Co0UVUFz3XZM5BiqjHjmVZ3N1nURPNXCIwKXiSxRIQiFGrqCIV0CVVRUDQDkQaURJXQ6ibisNSELcNZClkHjYhqoDFkdkjMMjIzjJk0aLsunQhsJUBVFKRXI9IKhHGKaWoIodJI8zha1+zXvHk8tUySyo3dcMM79t7/SGNDlb63fHGQ7hLFnUKIfcCvA7uAw0CZbnRcePfJLt7x2qeU8nNvLL9UKpW37OtDB/KsV6s88cI5FEVhsqLx4K1lzs3FPPFqnffvcdg+7PCXT19icb3D1cWAHSM6//6JWcaKvUi47YAzV2tM9qtUXcFzpxvcOqEwNeRwdTHguTM19ozpPLw/w1dfazA+aKNrCod3ZHntaocgUnjkUB9Hr0V87XSb/ZMWAwWFj9+R4bHjTVzXRZMuRy632FbuDon33uIw65d4ac5h0lyGJOJSVUX41W4EHSWLZRmUrYjEb+MrRepiACttkNVjgiBkMSySBE3O31hGeGtoovs1LtQCDH8ZRVHwgoA4SQmMEaxgiXyygG+Ootj92GpM3ReYcR0AI1ylmpQwoy4dN6d5tBKbpjqMcFe4vrBC1VMIpUpbFEm0PJeXfFL5nfM/vyd4D7n3P8z4fkXDrffM8UeklP9bTxwIIf4f4L/tHX+3pBYPfpv8uZ587C3qvytUqzVuzISoIiaIoJS3OX1lnVaokjUll2bqJHHMq4bN3NIqh/aMMFax2T9psrTuUW15HL24zkKjnySJ+Q8vrPIbP7OLy/NV2on2rfX/u3fo/MVz6yBBqjYvnJjlA3ffwuMnXALfQxMhp280URW4MN1AESl+PMBrV0KSJKTddrnuC7aMDfC141f40B2jrM+kIBMuzlS74a4qA7hKzJYRDa+zSE32c22xxr5tAzTkCCL1mKnHBHFKmh3CsS3M2MeMY6LAI2eXSRWBq5fQdJtgfY10YIIgTTDDgNPLNlv767jCRCQhrWYDP9Wx+sZYm6miFE1yZplYS1hd6VAcKqMqAqkoRJ7PWjNkMKNQKebImxI7XgMgTlIGSv3MrjQ3fGvtu+DW/8hiI9NaVYCop/A28EHgt95Yy+x52j/D313L/BUhxF/QdeQ1evWeBP4XIUSpV+/DwK9LKatCiJYQ4m7gCPBzwP/xbvtZLpfYbxtcnY+IkwL33pYnjFM+enuWOE554sXrfPbBCQ5sNXncy/PxO/N87YyPoii8+HrAv/jJW3j+fMSHDlhEscHxi8t8+cg6faUMz7/wOpXiboRIIU2pNjo8+NExau2QofItrDYlHzuUA3I02gHHLlZ55I4SitaNqffh/QaaauGHMa9czmJpAhKP8cECB8ckuqYCKpfmCxjFGjlWSYIMHa+FYxtUxDrBwCCRW8ORTZQ0hTRP200YLhnY3o1uTPviJO22YEquUldHsIIFWm2DvqyJ8GsYaRsRp+QzJSxZI4whjAWuFJhGStxcZOeggysNjKSKmzjs7ffxtT6y0QIiFUT2CEOaRlF2iLIZwjDCBtI0ZS6u0JfVMTRBEG+sgX8zjORvh40074eBZ4UQp+lmo3laSvkY8GdCiDPAGaAf+Fe9+o8D1+hmpf194J8C9Bx4/3OvjWPA//SGUw/4J3Qz4l4BrvIunXhvYGbZY7amcN9uC13X2DEIr88H3Fju8LMf28nMuuSLL9f46O3dZJStdocvvFTjI7fnMXSVMOjQ9mKePNHgV39yJ7phMVGI+eyDk6w3A+6+xablhvzMh6e4tJRy/HrKHdscJkqS87Ndnv03znX40KEh1psROUflkVsdXrrUXcp98qTHPdtUggSaoc5P3FPg9EJ3xHr6jMdtAx2kUeCKO0iOGsOOh6tVSNIUSwmItQIAfqxQzmcYLwpSt0ZNGSNQ80Rxgm128/flk3k61ihqfoghs01HKSClpKVUGLbqYJbJWwqmaTFatugvFbpz+bRFSS7TUvuxMjlMTUH3F/HMYeI4xSCiIFcIzCEUYhR3EV/Jc90r0e9ALl7acPO+m8vuvVmy+2HGRnrvTwO3vYX8oe9QXwK//B3O/SHwh28hfxXY9/fp5+paldXFhA8d7IbLUhXBrok8X3m1RZJKdo5K5pequF7Ak7qCIuDqbA1NFbxWyGCoLvsmbP76G8vctadCMaOzazjg6RNr/NRDY2wfSXn0aBPbctgxZvFHTy2xf1sRIQR7t+R47FgNU4uolBzu3G7w7x6b5tN39eNYOjL1uDQfM1wyURR49tUb/OqnRnAsjbVOxI3VAF2GFMyEyzNrZPUEYfbUxq9xvWOTUWu4vkdgmnhWH8VwHlsXuNY45XCW1bbJ6yuL7JwYQFgTCEVhZaWGKiRqxsb05wjsCo7qYPvrdIw8XruGkiljhIvUk2Es0eXpVyOHVE9YWW+wZXQKjYTVapOml2MsXkYxwIsFRtRCUxXOzQcUMxoi8vBTSSo31pG3uWTXxU3PyGt3OoQxPHcuxDFi5habvKwUuDq9TMeP2Dk0xuRIHwCfPNxN0yxUHSHgwb0GaarxzGvLXJtdZ6CvwHwtRVUNLk3X+ObFQSxLY73WZqXaotUqcm1ujSD0WVgvoesGtp3hc188x8ce2MPLlyIWVppcX69wbS1Btxz++KkLvP+2rTx+vAUSXr2eIHSTaqPJ547OcmBbheWqhusF1NsKbnGAjGNjZhJm5pYZKGRRFIVTiwFDRZfYqKAKhZXlOqX8IFk7Zvuohq0EWH6XSzJYHkPTVML6LKk9wsxCnUo+JrXLrKyu44ZZpkSVTiK40aizdaQPaVvkxSqerlMpZjA61xFCkNVs2kIlURw6Qmel1kITkj49olLKM2C6hIHPemiQJvGG76f/UViyE0KM0d2f/366tF6P7jT5K8ATPe7/d8RNr/RbJscZHbiI58d86aiHZajcsc2i2h5AUVR2TmRYbHoM5QIuLQSU7JShgqDZ9lhraOgq+DLD+w9q3LPbJJfRuTjb5lMP3IJlCu7cYdBq5ykXbD52qECp1N1X/4F93dztjx6p8l/+xB6OXw345OEcpy+VGCkqTParfPFoiwdvHWTvGFxdEvyTj49xYhY+tD3hlKbQWLcpyjVKZofVShkvMciHs9DpZq0dLNn0ZwRqsI5WGUI3dDLRAkqSIMpjGJogbq6SNW18iphS0oizOGKNppehrCUQzTNYHiantHHSDoXcAHacEsZ10jjBMXTsZB098mikOZy0Shy7eGaJJPSwMjl2KEu45hiWN8dkZQRV02h3DIapEdljWMk0xewAcmZ6w0f694qG+4NCz/k9CjwG/Bbd5XALuIUuOe03hBC/JqX8xndq46ZXeoBrix0uzKf82PtKvHQ55ctHG3zicJFXLja4OJNQsgU7x3N89YSH7/t86q4iimLz5Ik2HT/mM3cViJIMJ68H7B6VTK/BR27L8qWjLeI4pZzTOLTN5gsvrnHbjhIX5jyktHjudIODW3OMVXRUVfCXz6/ywIEyx695nL6Rcu9Oi/6cw7PnIrzQplJQKa66zNUMrq3AJ/fB0dkcSayhKDppcw1fM0gjn9gsMywWqUcVvCBDv1VFDUNW5SAdL2CCJVrpAIozjBXPk3gdAquEbmYxwjny0sU3+vETlT65QuKM0W4GZJ0Ygyo1Y4h8uo6tmXRSG0dGqHYWPVxE1wWLkUHWNDD8ZYSiEPstVtIKQ+EigTaFY2fRgzrrtTUC+phQ50nTjd1v+sac/occ/0ZKefYt5GeBL/QyOE98twZ+6L+Bvy9OnrnAV15ZZveoSrMTcvbSAnsnHYIoYe+EzZ8/M40uAl69uMaN2QWuzq7x0qWQZ8+FfP3IVXw/5oljaySJpB2qPHmyw4du7Tr8DowJPveVaXaPKJy+EXL84iKnrjUp2SFf+OYipqFy+so6X3m1yYUFhZdPTXN+ps30/DonXl/i5Ax86WiD2cV1nB6F6c7tDidnUgyla8HdOR7hpzoLa236zA6h0U9DG0V3F+iQwzQ1VloSmRkhMIfJKB5tP6KpVIgTyfxKjboYJDQGuLAYUGs0WfJsGoGg6ilkdIkmJAmC1BnCiKqkUmKFK1xZ11muBxi6ydllDUWoBPYortbP8nqLWr3FYpBlMchR8wUdzyfOTHJpdhU/ivHNEYK4G/gzcCaBjV6n/+En53wHhX/z+VBKeeW71bnpR/qD+3fzgbEbzK20+dJLdV6/vsruiQy+bxLFCUEQoSgaoxWTubUI3bS5b5eJ58fY+i5mV1wePJDl7GzI09+8zO37xnj2fNcjH0aClVqbV15vcmh7hk8/sBM/FowMaDz+0lk+9X6bh2/vxzY1Xj5fY//2Cp+4s8wzZ7NMlmIiqbB/b4mnX4t5/vgMpjZFikKz47Iwv4KYLCLSCF1z6QQh7WyZS3NVChmLSFr0qQ2aUZapUgp+FTttUw8VhrMqjujQCqE/b5Ghji4D/PIgZaODGruEiaDa8Wm5CluHx7i2sE7JsUidIXy3gwxaDFdymCqY3g12jI6ATDG9LlVi5/gIKQqZoEuabGXHMA0VozPNxOAwJiFWsE5f/zhxp4rqLWPp0Ao29vcW6o/GOr0Q4hN0V7Um6eqxoOsPz7/dtTf9SA/gWBoLDYW79o7w4x85QCvUuXVrlulVyW/8zB6qvsZwn4OVLRDFKUkqefpUkzu2mTxyR54XLwbcucPhoXtuQUrB/bt0Ht5n0nFDDuwY5EO3lXnx9ZB7dzuYSkje1vj0Q3tZ78BKU+L6EZ1QY7TfZnHdY7iksHcqR6MdMl9LcROdn/vwOELAPVsleVtj+9QQ+8pN9vZ7VAObnzmYYpgWu8dLFHM2Q45PUxuhyAp9Vkiod2kO0h6kYkVglckUygxoVVqUiFIo2JLEKKEpgkgabCnDVLaBF6ZUsgZZU5AVLiWtiVMaoZAsgWZRi/OY0SqB3yFCpx4I8NYJ3RaR1KilRaxoGRDU4gLZeBWpZ4jTFCX1KWodQnNgw5NXdoNoqG9bfkjwO8DPA31SyryUMvdOFB42lR6Ax481mBq0GS6m5MyYfeMq/+4rM7xvb4aso1NrpyxXPYYLggf2OZy67pPNZDF0lXzGYLQkODMdYpkqn74rz2PHmjzxap0H92d5+NYiL5xtMDFgoyoK9+8vcfRSB01N+fhdRZ4+Osdv/9UlHthroSqCVy553DrRTXv9wP48J657aKrOliEHTcRcXpZkMhn6MpK6L3h5xmBHptpzUCkIoTCqLHCumsOO19FEb9t2Z5lQyeJY3bar1RoLS+sseRk8t8WpZYO4s4rfqhFJDTU3iJG2sTVB2lliZq2DZtr8f+y9d7Qdx33n+anOfXN4OT/kQBAMIACCBJhEUqQCKcmyLdnyeO11GHvGXh/t2J5wzs6Mw1pn19J6jsOMZmWPgmVZWRSDxAxmgAhEzg8v55tT59o/7qNEaUiBlAjaFPd7Tp97u6qru9/rW11Vv/D9zjd0zhTjOPUlQimp18qY8TSGEpJVKjhGN5HZhSUcsmqFpt6FaVro+ERSYsST6CLArS0x52Yw3UUA/PryZdWxexlCVS+5vU0wBRxfcXW/Ibzjp/fHT52l7vjMlXweem6W0cEOJiONCxPLHOrJkYkHJLQWD+x3+eU7+lAUwRcem2TrqhgvnqpRbUkarYiTkxXipoqUg0wvVPE8D9OOoamCR16c4qbr1vHw0RaqqjI+U2R2Hog62LIqSb0VcP+LdZp1h45cisPnS0wWJLF4HN3QOXBsnFRiDV5g8fzpeWw15N7tGb72jEWn7ZHQ23H4W3NFHjxn4SX7GMrWKLZMQqsbXRNoVKhGNmGpSCo9SL1SJp+Ok4kWUATIfCd16RGPJZksKRjNIiI3iCoiYnKZoa4sirNMTIaIdJKM0sRVc6iKwexymYHuIXzfp1Cs4PkR8d4+hGpy4fw8a4byuPYApycW6U9riFgHcUtnbqZMPttey1sxQRBVL+/DFgqKbl76uLcHfh94UAixl3YaOwBSyk9equE7vtNfsXEd/SMTLJQcHt7n8YsbYuw9Xud3f3YTC1XYNKDx8CHJhakCz57pQFMjqrUGJybg3hu6WGtqxCyNUJj05AwM1eeWa/uZKwfctsVmvtikvHWIbNpi9xVtP7/jhVi6YMd6i/v2hwx1J3n/9iR//pUFFsvLfHhPL9esNRFC8I0Xytx2dQ/DXQG9GY1mI42iSI5Ntjg3W8bJmfiZLiQKgZTMFabpTSt0puqc13tJB9MooaDqhEwVXXqSoNYnGOjowXNaRJqNKh06UhZgYrammfaT9GQi4m47ubEVSJJGC8XKoxkRna1pAruXuL9A00/Tk88Qc9qps8KO4cVjmM4c5SjFpl5BJF3M1jK5ZAcps4kZLFPys+QSJmZzElWBqq9eXiMeKxTYb5/p+6XwJ7RT1y3AeCMN3/GdHqBYdTlwweO33jfK+KKHZdsMdMU5PF7hmlUWpq7y2x9Yy3NnmtyzI02pPsjOtSZPnmjyvuss5ooOq/piHDlXZONoji3DBmOzZTzf5IWzPh/c3cupiTr7TtcxFI8rRmxeOlvi9KTP2l4dJYo4M1Xnus0DZGyfhZpKX14wV2wx3Gly9YjOw8dcbM2jK2OwVPXZvTmF0+qmUHXp0Mok1BZPzOTYORxQ9QImwh4Smk9N9CJbJaJ4jq1qW3SyHmTIePPEVEFVHaDVnEGXBUKrA8/XWNMhaMg0ZtgWr2zqXeSiRepBEkNTMTSFuhdgIIinMriVBTzDQIscFDuHLQSthsS0bSy/SkvvJPAiOuISV8lBq4EZM0jLOVpWP3FvDhHvRXLxMj9p8Xaavl8KOSnlHT9Ow3f8mr5eb/L0KYe7r03SmbV57PAS165uvzhHO2Fs3kXVbPo6bN6/I83Xny8TRZBPW7x/e5ovP7XEPzw6RakBF2eWcbyQSt3jpi1pnjxSZqQnhhCCVX02x87M8MAzkyyXXAY7dB5+cZ6hvMqqvgQP7F/ghg0mV61OIaTHSxM++8/5XDXcfi+bONx/oMa2EYXepM+3Xlim365x06jHeDPF/efi9ChzZCxAT9KrzhMLFjk2vsRUzaDZaOIE4KNha9H3RB+U1izLXhqLJq1qkcDsxIxqZMJZKmo3UkoSdjuQaH6pwNRCiZKjkI4Wccw+FOmTNRxCq5uK0oPtTNNoNHDNfiyvzb9fr5ZZ8lIY/jKiMc3Zcgy9OddW2nE9Kq5AD2uXVegCACEQinrJ7W2CR4UQP1anf8eP9EePn2R+rsTTZ2JEEZSrTU5MS1TRQhUKX37iPGv7U9z3QhzdMMmlYzy5/yyZxAZ0JSIIHAZ70ty43uDgcZNrRnWeO1EhUGz2HpzgXbs2s/d4A0sL2bIqjRVPcM3GHI/vm2K54vDo4WWiSLBYqPPCWQdF1ZHEOHJyBs/1eMTuIwxDLk418H2fveey1OuCoxfK2GvTnK8ZzBQWKNU9ZsxuYraJ51U51UqRTsXZOCRAKMTcSRyR5OJ0gZG+LIHRjwwj3Min0qjh5vtJWIILMyWsgSFc16HVqHIhTGE6VZKJfjLpkJilo7YcXLOXuUIdXRXkkr1MzRaxdA27a5jqYhndaxLvHCUMPYyoxnwlJNU/hAiaWI7HrJelJ5XGDCLOL+qsz2YQLF/25/1mjfRCiL8F3gssSimvWCnLAf8IjNAmxvxZKWVpJaP0L4C7gSbwy1LKQz/hLfw28PtCCBfweQMuu3d8p9+1cxvLiUkKDY9btsRoNIcoVVu8b3u6LVJ5PM664Tzb18UA+O7BMr/2/vVMFSTb19lUmh0EXovnji/ys7cMcOiiz+3bOvnOwTK3bRtiqFNn42Bbouq+fWXitoqpqzjS5j//+ja++ewCH9iRIZtJ4QZw+xXtY4vVPClb46aNOk+fqHHH9j4mlkJu26RSrMY5fFphVapOww1xOnWu6yxxshIn6UxSE3ksU8Nt1IgrLi0/wrfSxMIq60eGkKFPzJsBoKT1sibrgV+n0WjSGTfR/SIxWSdpRpyvp+g1PXDKWFaWZsMhqYLlL9Kd7UdRFBLeFL25bqRQ0BsTxLU4ViKN3hhHBxxPIWYmsJ1JCm6ckXgTR8SQgUvCWyCTzKM151d+tpfvWb/ssnuT8D+AvwQ+94qyPwQek1L+mRDiD1f2/4DX4H/8SS4upUz+uG3f8dN7gK2rk1w5rPH15yuk4jrb1+h891CNBw9W+I33jeCHIWMLPpW6RzxuMdoTI/RdvvDoLDdutLhzW56nj5cY6IxRqHg8dbzM2v44t1/bwZmJCtWGT9MJ6MxZDOQ1Hntxhs3DNpqmsGNjiieP10nYgnxCcn7eo+n4dGUM6i2fZ07W6M7FWNuj0XLadFxPnvL49z+/lmOlLAfnYgyZS6QshaTmcqGeJqE4bct/LI0uPFJGgCuSSCkRkUfLDQgjSSWwSYgGSUMSGRkUO0u33aAWJQikoKL3szZZQ8TyBHY/ZlAkp1XwrB6iKEITIVFjFlfNELN1suEMZbWXCBXDmcPV2rEBaqqfYbuMY/Rhp3LoKiSVJkGjSJFOOm2XhtrxlkzvFcO85PZ6sBLbXvyh4nuAz658/yxtvoiXyz8n23gBeJn/8cf4E8TIJerFSkLOa+IdP9JPTc+y/0yBeDxGR8bi6Ll5VKWHmfkStabH85kEhmrwnX0ztNyA67f08+QJh7lywMxSlcMTHUgpaDRcnjju0HA8zlwssvvqQWJ6yHuuS3Pf/gqqEvH+PQM0Wx6f+Oxh7t69lvlSSCgVnjs2SypmsKYvzti8y+EoZPOwzaH5Mr7nce8uA1DRhcuTRz2uGrJ4+lSdM3MNEopHtWM1igzxRY1SvUoqlefsxCKr0873nrDuzLAYpUnKEmlatPQeVEVD99sRdK3qMoqZQkYSKyxyYtlkqDciiPUzu1hCUwS2LTBUcJp1qn6aTuax1IjTyz4Zq0wm2U1MkVwsOVgdNqq0aYUecX8JTVOoOk1qLqipTlQzhiphfmqZmK2Tikdvgc6TeL1r9g4hxIFX7H/6darcdL9Me71CANO1Uv5aPI9zvHH8X0IIBfgWcBBYom3BXwPcAtxGmz16+rVO8I7v9Jquoag6uzcY2KaK5/ewa71FoZZh0DS4eVP7zV+vJzk3XWfnOpMggodqOhtHO9m+WsfQVcqVQRJmRMaKUPtyXLfW5tC5Ei+cgWozZHaxRDaXRkQOmUyS3duHV5hvoO5KRBRw4waTl8aafPWR4wx2beBff2gt3zlYodx0OXLQxw8M9p+YZvc1I1y5KolLjFa9ztbOZbwgYq5kcM2wTuDMYRtxSoGCtGMomoliK8yMLbBptJNIUTl7YZE1gzm8+Ej7/6D6zC2XCNOduL5HNilJ+vNokaAj24ciXcoNEz2WwYobzE4tkRsexnUbRFGLnFbDCurUfY3VPQmiMETIkMliyLrhHmq+T7FawdB17GAJAiiTZW1nRGCmsFuTKHBZ9emFEG2RzEtjWUq57c289KuU/VjvOCnlh1ekr38B+BXaZDVN4BRtIpo/kVI6P+ocl5MuywKeAsyV63xVSvl/CCFGgS/RJsU8BHxMSumtsOJ+DrgWKAA/J6UcXznXvwV+FQiB35FSfnel/N20DSQq8P9KKf/sjd5nb08X16bSfOuFEtesidNoNDg65rF5MM7RsQpRZFFv+Wi6xXXrVWaKAfvONrlnRwbPDzky7qILlytHLA5eaKEqJrAlzEQAACAASURBVHdda7PvrMONmzpoOj4PHaxy6zV9dOY0Tl70+Z2PbePRFxe56/penj8yx5WrYlycrtL0Ikp1n3ffcg2+CHjsSI3AazG6JoVQWixUNHZcOcTqLoVsXJAwQ9K6YKmhcmBKZ0NsBjUueGGxh9F+jWrDw3SnEZ7ADyJGurvAd2j5AVf0CjwBRmOcViARVh+rBjpwamXSCQXhFfGMLmqeT4ICMt7OqkMuUHR76M3oKK1FAg9GspJQpIiiCpHdRTaYpRUbolUv0psSyMAh6c+jdA4i67N4eg6vWSMWV9G9FuVaBV1cZgKNFVxm6/zCK+jgemmnvcKbrGUnpTwJ/Psft/3lXNO/LHaxlbaK57tX+Ow+AXxKSrkWKNHuzKx8lqSUa4BPrRzHylvt54HNtPOF/3qFWlsF/oq2kWQT8JGVY98wFEXhA7vyzCy7zBfqnJ7xWd1rcNUqk3OzLR5+qcZNm2NsGU3wmQfOce3qOIaukogZlBowsSwY6rK4dUuc6fkicVunUGlSqDg88GKVe6/PsmNDirPjRSJhkk7axC3BxFyDuYLPaE+cW7f18uLZFpl0EiLJ7dv7uG1bN01f5c8+f4yTExWuGFS4+coUp+dCvvLMMpt64YqhON843KRTLaCuZIhJ6YMMSQUzuEY3AHWjjwxLTJc9aq4k8hqEjQKVMElg9ZH0ZylUXSzbRA/KaIqCL0xM00bHxXF9AMrkSIoKHXqNpppHTXSQUBxUK05Z6yexYiCs10qoZoqEaLC4tMTxZZtGrQiqyXTRp6WmMby2tT6vlFgOMpe/0wtxucNw76MdD8/K57deUf5LK+vtnazwP/4kF/pJ8E8hdnEr8NGV8s8C/5G2NfOele8AXwX+csXVcQ/wJSmlC1wUQpzn+2qg56WUYwArhJr30NYLe904dfo8x0sNTF1FVUz8IGJmocwTx5LETJWHXxinryPOEydsEmaEqqgcOLPMufksSVtQqZQxLYtnTrU4cn6ZKIL7X1jGsGJ88sunuHXnBp497aMKSej5nJ5c4uuPW8Rsg//nc8+zZV0PTx4x8SN44sUx7ti9mXMX5/hm6KKbMVYN5piaK7FpKM7UYoND56p4ocLxi0UsU8fSQlpOwHgRFuJdWJZO2vbZd3qJ1SmJH0Q4voEXtWimhtBaVWxDUHN0Is1gbtmjL+8TaF00mi6WatBUunG9iLGpBdYMdND0bKJWAU+xSSUkvgthrI/FxQqKouAlulicb2AboGX6MXUDv1yjWqrQk0iQtxvUQpuMUiUKwNCyVBoeeroHyzJRiJg+v/AWROQJVP0NBa/9qHO9mpbdnwFfFkL8KjAJfHjl8Adpu+vO056K/y9vyk38mLisa/qV0fggbSPDX9EmryxLKV/WMXmlQMX3jB1SykAIUQHyK+UvvOK0r2zzw8aRV3WDiLa++K8DDA39IL9AX283y/WT7NmkU214hEE3kdC4ebNBserwgB/wwd3dZOI6L12o8LHbh5kswp5NFl4Q8fzRBkLWeM/1fWzf2MXMUo337sjz7MkKu6/qZbRLZVV3+4f2jecddly1hjuvy/HSuSJ33LyFfDbBrqu6eeCpCW7csZ479qxmZqmOVDTes7MDAKfVz4WFOvfuzCOEYKnUYnwmyT07snz3UJFfviHG4WlYZbdnkweqabo60kgUYobByUWF9d0BWmuC7kw/TiDJ2SXKYcCmbklN0UmHs8S7RzCaE1SCDCnbYOvqTiQKuvTwjA7G5iqgqxh+i8iJMZqN8I0sVnOCRdL0JAJMfxZ8SMT76cgkMJoTFI0ehmJzBGoSS6mRS8ZxvIBUOIfSFNQCk3TCZLHUoOm//t/XG4dAKG/OT15K+ZHXqLrtVY59Tf7Hfwq8pWIXwMZXO2zl859E7CKdSiIjj9miz6NHmuzeZNNotAB48kSL//CxDew93t6fKiqM9NiUaj6OF3Dfvir/4t0jbNk4yJm5gOvWmFy7yubcbItWYHDntZ0cPlcjCCNKVYeBTgvfDzh6vkRoZHjfLau5OF3iucMzjA51ccv1qzl8cp7tVw2zbesge4+WiKIIVQTcsjXJs6fb9pmnTzUZ7LIJwginGZCNK4RKO2pusQ5hJDGjOgYuTcenO6UhQpdimERzFoh7szhGF0Yij4FLOpihpvcTRgFFpZe45tFqNtCcRbxWFaGotFot+rIWabWONHMkVA8tbNCoVQgjGOmO0yKBL0yCKEIjIJCSZiCI6QFJS0G1M5SjLHprnoQ/j2N0UQ9NjHiG4ViF4HLruIqfqiw7hBD9QohdQog9L2+vp91b4qeXUpZpC1TspO2jfPl1+0qDxveMHSv1adp+0B8lgvGmGEe2Dlv85VdP0pGQKAK6kwHPHltm/UAMXVO5ckjlwAWHbEKj5QY4rRr/6bMnyaVtjk9HPPrsKZK2zqELDTIJne/sm2fbqrbIxV3XJNh7vMGTJ5pctzbG8dOTPHJgDj+MeGxfOxT1sefGKFWbpJM2zx6a5PqtPawb7aSnJ8PXnpyiM6mQT1v4QcBswSOfNOhMKHzzuQU2ZtqqMj2xBiVX50ytk1S0TK9VZ97LYqohXWYd3+ohlsyjKxEKcHqmTr3exLX7iZIjBEHIybFFEsE8WtTETObb6bJqDc/sJpHKkqHIyUWFphugBO2MuJxSoq52Ero1MnKROlkW/SymO0+j3sAz+zFX1u5SaNjJNLoSoSkwvtig6FkozXkMTSF8K8Jwf0o6vRDiE8CzwH8A/s3K9r//yEYreMvFLoAngJ+hbcH/YWPHvwCeX6l/XEophRD3AV8UQnySNvPnWmA/7ZF+7Yo3YIa2se9lW8HrxnKhyMJixJZ1/cyVHO7bVyERi/P4vjO8Z896nj3jEUmdh54+zYZVXZQrCoqic9vOddx2ZYxqw6N21RDJmMbaXoXnThRYKtU5NSsJZnyCIOLYuQV8P+Q+RSWKJDu3DrN7SwaA7zxb4ff+19uI2wZ/+jePML9Q5CvfOY0QCkIIDp6YYWEhzvhym9jiU18+zt07BrF0WFquUdRcppcj/FDh2yck/dkm1cQQEYLZ8TnswS6IJZieL4BUGOoZJvJq9HfqJHUfs9U2vMkwy6qBDuqBi6LoVAololgKVdVYLlXxgoj+XDf9nSqKDChEORKJFDJwmJ6vkzNbuIkuYrbFzFKFzMAw5cUqmlPHyA/heR7jEwt0pGw68sOETp1kTBIXLQquhWYl4TLbttpadm+PTv06cC+wfsXW9YZwOdf0vcBnV9b1CvBlKeX9QoiTwJeEEH8MHAY+s3L8Z4DPrxjqirQ7MVLKE0KIL9M20AXAb0spQwAhxL8CvkvbZfe3UsoTb/QmO/I5LlxwuO3qLM+crPHe7WlOTdTYsqabjpTGul6N01M1fu6OjUwsOLx3e5InTrhUqk2iKMbjR6q8Z3ueB14ssXkwjRQGP3fLEJoh2TRoUKq5OE6eIIy4eUucVDJG0CxxdjLGuqEkbmSRy8T54reP0dPdwa995FZePHyKu3b1MbdYJamsYnqhzk2bdAQ6Lx5LcNXaFF95cprFio8yaJI3I47NwWiHRYfVIkaZyWaa64cDCjLAas2SjWcRqonRHKcW2GR0gad2QNAOKovH49QrRdJ6i0LDJpVIEA/nIYBkYgBkiOkvoMRHaDXrZJUyWmtl+aGkiFkmGRYplw0yyTgxZ5K+rkFcL8BqTRITgv58JwlbxWpMAKCmB0AkyLUmqQTqZQ/DfXl6/1OCMdrG8X8+nf5HiF2M8X3r+yvLHb5v7fzhuj+hnT/8w+UP0raM/kRohibdWYPbtibYf96hUFX4yG397D1aJvB1xpcF79lmkTQC/vuDk3xoTz+eH+PYRItUMoauKbz3uiyPHKljGAZXro5x/8EGQ3nB3hMt7tmRRgjBl58pMdCdYtfGNN/eP01HZpR4PM7fff0IP/OebTzyzFlGhroo11q8eHKBM2dnuXdnisaQzlMnG7gth19+9zATSyHDg3384u3DfPPJKWzqdMY9tuR8HpvIoYfTqEab+67iNPCERTIex2hNUtUG0CwVw52iWVvANSwagUayNU9e93DMXlK2ieM0kQgqWh/pcBYhI6rGADEi0tEiLWsQzZuhFtqszgsCoxOcSbRkN3p5kQUnRlYuYErne+mzqaSNUy+jaTFaLQedAk2SmFKiJzqQsvKTPsofDSFQ3iTr/T8DNIGXhBCP8YMkGr9zqYbv+Ii8w0dO0Gl6nLxYpOmEPH+kRMzWeOaUSShsPvPtY7z/5g08d9oBVM5NFjl4sZt6vcG5qRLvuqaT505F+FJn74Exrlrfy95TOsuFCn/6hSXuvHEDT5z08YOQ+aUyc0tlHH+IVEznE59+mqGREX7v1+/GMnWS8Xb0Xyad4DNffJyetMLeEzqqIjlxfoGkrXHnthz3vzDJLVfleOylMvFcnsefGGfbpj4qQYKupMNjRyw29DZZFmkMVXJ4BgY6HdTEKLVCDdfz6Mt1kowW8awhLEB3pkAooJpoQpKOFjheSJBNNhCxLjRNx/cCLiyV6Y2ZICv4qoUa78DwpqnXJZFUUdQGKaXJeCNO3nDQFIV6q0aNGGqzRFqpUaKPyAhJynkImkz7SbrF4mX304vXH4b7dsB9K9sbxju+0wuhcHKyzm1X58kmBblUjdGBDm7caFGsOgS7VpGNC7ausjg/U+XePauwbcFtW/L8u2NTuF6GnZvaWnFBsBaB4ObNFmkjAUKwZ6OBEIKjYzX6tg1Rbglu2RJnudxiermbcsvl/sdOML+whKZpfOl+j66OBDfv2oLmzHP9pnYy1WK5k1KpyX37K4zPFiiuzXH7NVmiSFKdzaBFHmsSBSIzYp9t0KFVvhes05nNk1SbmM1lErEeunJJ1PpFijLPwkyBVNwkaXcRhiHzM0vETIOeXD+JWJPelED3F8EHN8rS35XF9JeRdobjE8sM5upILY2uRpxZ0Fg/HGc5DEnEFFzFILSS2KrOifNzbBrN4ikZND9iYrFKfGgEBahMLtKVy6GKGpdVvxJBe7X59oeU8rMrHPfrVorOSClfl8PzHZ9ld9XWjey5Ms/ZeejJx+jr7aZUD5BSsvdEi3ddnaXUav9Qjk9F7NqcYa4YsP9Mhd/8wCYC4iiKwncPFrlujUml1iQII8aXIm7dmuXIhMdy2WW5oXHlsIHjhcwVmuy/GPHL713DhlV93Hvraqp1jz27ruKXPryHqekl7r55NU7UnorufanA9Zs76MgnuXd3H3fcsIFSPcIyVCYXaqzrbGfozbopDs7bbM7XqIi2j3+mGafbqBCZbWmumGXg+QGaomDTYlVPnI6ESlYuYkdlhrsy5LMJmvUqg7EGTV8jWHEHxhMJ3Fa9zZBTm6QzbZPSfZJUEEGDjpSJ6S3RmU2RUD10WpjODGpljFwmhmguoNQn8IOIgUxbecdsTpBPxxBBk8tOOS/4qSHREELcDJyjHf/y18DZf1Yuu3/uWNOf4Mphlfv2VYiZCjs3WDxzokpvzkRRBNW6g+MFdKZ1hBDk4z7nZ5qMdlsUqi5SSlTdJhXTuOXKJIfHHBLxGMM9cc5O1fjWC0WuGFSYWmhw/PQ4B8YC7tnd144QC0oUSnWuuXoz58eXef7Aaa7e2ImuqXihYHK+jhWLM9RtccWozd/df46dmzJsGo2z/7zL7LJLypJs7gVP6sxWBQkDQrXdUUMjg45Hs1am4kLYKuHUChRdHT2WJuYvIfQ49dBEiXWSDOegPsdiHVquTzJaoiZTLLkWamuJNCVcLUfDGKDfKOKbOaIoIrR7GI5VmWnEEG6RrN4gtNohwDWjj0GzgGfkqKr9ZKJZMlqTWpTACyKSuk8jtN6aLDuhXnJ7m+DPgTuklDdJKfcAd9IOX78k3vGd/tTpC9y3v8LJGYVsQuPYuTnOzCl866nzzC9XqTc9epIh//D4LKMdgm88V6TQEFyYLPDQoQam4vB3351k63DbL59JmixWQnQl5FvPL3NhuoTnuVycq1OuOViWxfjMMg88v8wThwpcuSbNX37uGa67eg3VeoMTpy6ybiRLFEX4oeTp41VW96icnaxQbURMzldZKrsM9yRBgeMXq/hBxEOnVBwPPD9iOuynUlrmbCWB1mq7wXJqmZlWiigM0a0kMzUNqVo07SHG5grMOzGkalASXZQ8DdPQ0A2TlpbHMhTmKhIZ68I3OpitRhhBCSklfq3ARDNDFLg07SEcx2W2rlOgi1qtRsnTsEWLUpjh/HQV29RwjR7qMo7lzjLZyiK8Cm596fL76QGhKJfc3ibQpZRnXt6RUp6lbc2/JN7xa/qR4X4OTx3k5ivipGImQtUZyYfcdf0qFE3lzKxPLYwzNT/DXKWDe3Zm+e6BBbZt7GL7Gp2Wl+fpr53k5EA3J2cdkJIjZ2bo64jxkVv6MUwLEOzcHCeKIkphkroD793VSRRFPP3SHPNLFY6eWmB+sczi3DRflj5SSo6cnkEhYrY5TH9vhh5TY9/pEoqh89CLSwihc3p8CRo61/UuoAhBsbODpDtBKZHi0KTDuuF+HBkRBT6eV0b4TYhlGMxbbVUZ6dARz5CMmcRak8QANddPVF9AVVXMqETR66EjpaHWJtCFxA/SlD2dyEjjSmi6AcOxMkqrwOjACPVGnWS0hG93cmbaYcOgRUZdQOvvR0Qulr+IE0QUPINSrYEuEvRYjbck9l5Rf2qs9weEEJ8BPr+y/wu0Q94viXd8p7ctiw/fkOWbL5S5dm0SP4JDF33evz3DwwfLjA7bnJyosX1TN7aloSgCLzK45/ocjx5psGezxc3XDhEFPrdeGSOKIhy3jyACXRPETYVWs0GlrrP/bJXrt3Qzu9zg1ESNjcNJSi2Lj//Ge2kSp7+3l57ODB+5ez1SSlIJm9H+BIsll9XDnfz9t0+wdlUfg70p1o928pVHLrJ9yxAZWWPeU+kzqwhFtF8uQYx1XR44JYywSs1XSNgJhOpiCQdLlCiJPtLRDLZt4wQhlpTUAwtLrWKYAY7dQ9n1SEVFUrqDp+dxnSarMz4taWNrPpGeYkhZxDdymEEZIp+sXKRhDhJ3pxjq7MCLVEzAtnSatSaqYhAQksp00hef5UIjiWpEvE471E+Anx5DHvAvacfz/w7tCIenaK/tL4m3zVzmcuPenRnOT9d58vnTrO/TEEJw57YsT51sUvUM3r29m8Vik/FFH3uFHdZzW9z//DzXb0iQiQWcnfV54MUKuzeYrOmK+NzDU2we1Lj92jxHJnwiNUEmabBpNMvp8SrPHF3mhl3XsHFdP+fPT2DaJrfuuY7DJ2f5x/sPsWdbH5vWdmEZ8OyhaXq60rz7po0cv1Dla4+Os2tTinzaZueq9rJirJkHCZNeLx1ing6zhYNNGIG0e0ioLeqiE9MvAJAMpmka/WiaTsKfx9W7UOJdGGGNqgtnJgv4bgtVumiqgmqnMJJdGDgkZYGxgqRQqRFXPBqRRdONCJx25p1sFVj2k8SokfKnqag9FMt1DFqcKqgUgyRxbwYhBFlbUvATb1E+/U/H9F5K6UopPyml/KCU8gNSyk+93ui8d/xIf/Cl45z2XWxdYFsaCLgwU6fUgEiqTM8XCEPJdxSB50V89YkxUnGLx0KLIAg5fGaJvs4EoPH1vRewTYNsOk4oLU6Nj5FOJUnoPssNhTWDaU6OFTkz1eDcVAUj2UG+v8pc0mCk1+LMRIN1a4d56pkXMQ2NucUq9+8dJ5bI8sQzh7l68wBhpPCV+w5z7YYOZuYDwsjnwnyLekthoRKw73SBzoyNp5hYNOm05ljQB3AcF6GnKNWaZPpHkFFAGHhUKhWcUCGuJZkv1unNg57oR5dFRnpzNFsOZaWPmKUxtVBESOjrGibym2SSElVIHKufyI8YqycZpcpiaIBuMbZYZ8NIN5qpYnlNWkhE0KQv10sYejhGN6oRR3VdSqX6ZQ/IQ7z9R3ohxJellD8rhDjGq/y7pJRXXuoc7/hOv3nDWqaOX+SGjTG+c6DJx39+E8+ccrh+Q1tuuljvIGkb3HyFBcT5ZgSlmsOOzV3Umj5jcw471qcQQjBb7KMja3HTFQlOTlT5xXdvYmLZZfuGBP/l62dpuR63X9fDe3aluX+fRaZrkCs39LD/8AX2HZ6g0gj5wleeZN+RCyRsk6uvuY5f/9Vhntt/gmuvu459L+znjj2beX7fUW7cNoCmCJ44OMex+YAbB5vcMCCotvIYGsTcKq40cITNwmIVSwtJplJ0pUHUZzFEeyodKJKqm2Q4VifeP4AfRihOAVM4qIaKdAPiNNBbDpCiJymxWxMUlV7yYpYgMYLZnMCKIqaDGJEeJ6U08UWd1T1xStUG8ViDmPBRM8M0qj5pCjTVBEZUR2sugS9RlCyaCt5lzbQT8DZxyf0I/O7K53t/3BO84zu9ZZsEgcvssoJlWaTjOoPZFhfmPMbm6+zakOSF0w5g8fDhCjuu6GBsuoLjw76zLh+8eZDT0y2mlhxuujLNcycbAJybj7hnR4q4KTk/L9l11SpajkcubaIIQT6TQPGXqdVdLCvGu26/nZ6uLMulOjfK69l6xRpOnDrFyFA3c8tNbr5pDaqQfOKvvsTv/+atfPXBo3xwdzejPRl29jS5uBSjVl0mrdZwPAiMLMmojONVGe7qBlUHoWA2FyhrfaT99tQ6SvSzWpvGs3oxDZ1kY5wyOUzNJmpVyagVKuoAtjNJf8bAw6Qc6CRlCVURNH0fEyhr/azNzCJEAk2V+GYXnf4sidQQdcckKapICVYshe5WSVOmRB8Z2aRl9ZOVC2+Naq36ugzc/2zxCsad35JS/sEr61Yy7/7gf271g3h7LGAuI6JIkrElf/4Px1kuNvjms7M0HYdnTiyjqgb5pI6htLh/3xIbRrIMdprcdHUXj71UYrQvxqr+FCcnXZLxGJ1pg40DKv/w2BTXrLI4Nlbk6HiTbz51jvPTBQZykkcOlHhw/zLXb06x+8oO/vy/PchMETasG6K/r4vTZ8YIggDH8RCqxW/9m79AovLFrz3BoeOTzC6WefyFadat6uTQ2QpGWGd1l0Y2ofLClElKbdBtN2kGKm5ksij6sLx5yjWfqNlOcTXdWRyjh5qnYcs6hqbg+OC0miy4CTQjxthCQMlRcOPD+G6DU8UYNSei1nRYrHj4WopmqNOslVmKOkiFS8RNgRrLUdUGiK/QZjWbTTLRPHUZ58J0AdGYxQsivCBCb04x5WaxZY2sEb41qrU/PX7621+l7K7X0/AdP9IXCkXyaYvd146STFjs2WRTrHo8dWyCpUId2xikFWgcOztPLpdnutAm/Tl0YoZ4Is5Ctc6hM3NIpZ/vvKQihM7hswv09+YZ7U7yoXUxEskMlino7jGYrdU4enqKVCqFpYcokYsaVjn84jNUay7nz11EtzLcfccexibm+fjv/iYLhSrvvuNmDh48Sn9PjqA5x+LSMt+8/yjrOxQOGF3oyYDFyjxdyU50M0ZSF7x0vk4i7mJnR5kfW8DoSqLEugg8n/lClUjaDCdjeFaOeqFG5LfojzXwm3VW93aCamE2JtAIWTLSdKoFSno3CTuF5U5T8RXKjo7wXFK9A0RITo8vMdgRxzW6icKQoNViIkjR0xHD9eq42Ghqe/XuyYhCoYmOTiRTtPlQLyfe/rH3Qoh/CfwWsEoIcfQVVUna+fWXxDu+03d25jl4rMXd12W5ON/k0JjHjnU2V28cJmX65NIaC8WQW7cNMNCpMdpjUq47qHdfg20Z7NraSa4zx/JSiXt29/DVx6f4T//6Vu57cpIdG5I8fniJ7Ru7efpokZHeLKcna+y8ZpjBTo11AykCJUa5VOSuW68mmbBxQ5VYsouzFybZsH4112zdyNfve4Tp6TmOnjjJr/zC+/ivn/4cH/vADmqNAJbPcsOqkAcPN/j43XkePx3Sr0wTEOEP9VJ1IeFMsHF4kNB3MRvjmICf6iaMIix3nqgVkU8OoAod3WvS1PpJudOUlV5MKSlHWYZiNRytA1s3kbVJPLuDrFJET+eRkcRsjFMROQYyCnHVwfRruKFEt/sQfoDWnGLDQCdS0Yh7swRRhG8O0JOp0W1Wqaj9CAqX2YL/5hnyhBDjQI02Q3Mgpdz2WrJWb8oFv48vAg8B/ydtBZ2XUZNS/rD4xqvi/5/eR5JkIoahq6wfTKLh8+yJKh2JqM1gO1VDKBo3XJHlxVNFWm7A40eb3Lq9n6Wyx0PPTLD9ii6u2tTLt5+aZPVwlmzK4ufvHOWLj81Qbip05Ww2DtmcmayBFuPuG0c4O91gqeyha/Chm7v5yref5/5HDnPLDRuZnJ5kcnqOvp4OZmbmufbqTXz2i1+hv6cdP3/bLbvZd+giXfkkYayTlhsgJGRiCnFbww0E55s9DBnzjJiL1PVuNEWC3pbMKik9pCiiaQpSSspKL5Y7g6LplGWWWLDUDhF2FnBEDDuZJm5I5muSqLlATBc4kUnNV9H9MrIxx6yTIqZG5PU6vp6h7hv4Vh/ZcJYOscBUK410y7hOEy9Sqaj9pIMZ9LDOnJsmIS93h29LVb/JLrtbpJRXvYIj/2VZq7XAY/xgp3xTIKWsSCnHpZQfkVJOAC3aVvyEEGLoEs2By8ucM0ibx76HtobBp6WUfyGE+I/Ar9FW5gD4dyt58W+Y3/61OPTfyH0+/NhT2F6JBw746LqOrpk89NRJbt6+Bu9CgG6ovHRsmkTCBhnwtb2zjK4aRFUVsvGIyaWAZw9N45Nk7+FZrnYF56crGJpAKioT83Ue2F8hZig8/PRx3n/rWo6cnmOoW+fzD50jFjOZW6rRaIUcPzNFwxMcOnSSO+7oYWJqHlVVUIRCtVTm8b3PsrBYIB6z+eI/PMju7WsZHerhv/zjAe5YGzFfjtjcJfnHfQm67BpLIoFUDZYrLTyvgaZI6lYnmaiAho/qLFHAJhkPUH1B05eYhoHutwenlCmZC5JYA83GHwAAIABJREFU9RIiMUhtqYCtWmjZXvx6k/GSzuqBFGYmy4WLCyQSeXwrxWKxhucZDCV1qlofrutQrrVQMenMpzk54bC6XxDEhtG0gMWlMjE7zffJky8XLrvL7h7aDLnQZnp+ktdhWPtxIIR4H/Aym9QiMExb8GLzpdpezul9AHxcSnlICJEEDgohHlmp+5SU8v9+5cE/xG/fR1uK9+W0wb+ibbiYBl4UQty3Qvj/Mof+l4QQ/5X2C+Nv3shNbt2ykcp4hXddlQAgiiKk3ICpq9ywOclX9zbYsn6Q27fGiaIYf/r547hSpVJzWCjWmVl0+KWPfoCOXJp0JsP01DgfvWsUIQT37Z3BDyJ+7q4NVGotnj++TLJziL6uNEEQkjgbsGVtnttvWMNXHh4j223z0Q/eiKIlkEJlx3VbAfA8j13T22k2a3z0Q7fSbDqcO32Ytav6WT2cw/Gg5mkIVaHS8qk0HFbFXTpMWHZtOtMp3EilWphhesHHHuom0jWsuGTs4iKbMjE8I875sSXWD3fi6iMgJALBwsV5BjIC25li/fAQURRgNiewQ4V0IontzqI4IYP5DLoIsJ1ZUmaOWC5HzJkEoClhTXcGIQNkdYKuVAorrKI36lSjDno6UqSjORTab/vLhtcfhvt6ZK0k8LAQQgL/baX+tWStLgf+mDbn5KNSyquFELcAr8XQ+wO4bNN7KeXcy3K8Usoa7bdQ/49o8j1+eynlRdoc4dtXtvNSyrGVUfxLwD0rnPi30ubIhx8UDHzd6O3pYl2v4NGX2qPMI4dK7FgXo9xoG+wyqRgDecHMskO95XPLzrVsXtfPB28bYXBwhD/+w4/ywKOHaTYd0gmDn3n/Hh5+fpYn9k+yfUsPuaTCwnKdB56a5pN/9KtMzjkM9GR56uAMH/+Nu9B0i+n5MmvXbeTOW67lwJELGKbJyFA/p85cAOALX7qPO9+1G9cLaLUc/u7zX+XXfn4nY1NLdOVT3LlzmJpMMZKTnJwX7Bny0O0Es60klTBOMphHay1gGBbDmRCiCLMxDpWL9GYMwsDHaEwy2pshCgPM5jhmYwKnPMuGHh0rkcXxIoJmmUi2R8oo1sewVaJl9FF2BTHRxPV9fHQSySStehk/akfT+3Y/CVlCM+P4dh+9ZoWGSCGlJB6PkfJncYzeV+c3frOhKJfeVmStXrG9mo7dDVLKa2hbzH/79aa1vonwpZQFQBFCKFLKJ2iLylwSb8mafkVp82pg30rRvxJCHBVC/K0QIrtS9loif69Vnue1OfR/+Pq/LoQ4IIQ4sLS09D/VD3XHv9fxFSNOKq4zkIn40iMX2TJssH1zFxcWQh55qc6uTQmarYCvPTHHh96znVwmwa3Xr+Hf/snfEbc1ZhbKzC577N03juf53HDtCP/j64e55so1PPr0MRaLTT7+x9/gF+7djmnoBCE8e6zBLTdeyZpVA5y/uIhumNy0ewcv7D9CFEUkk0ls2+LnPvRePvXXf8/2LV3ousaH79rK33zhSXoSPh1pwZ8/VMarzDHfMNk3LpmrRojmYtstaQZUwxiW4qM48zSNXioiT6dWJmou09S70XERzXYdgBLvxopqhK0ai0EGW1ZwqssUXB0zrKIoCjSXKAVpLMUnQ4mG1omIArJKmcDqoeqr2LKOEAInUDCkgxAC25mhGObBKaFpCq7rXnbVWvEmptZKKWdXPheBb9AenBZeVqP9IVmry4GyECJBO+b+74UQf0F7dn1JXHbr/cqNfQ3436SUVSHE3wB/RHt69Ee084J/hdfmsX+1F9Mb5r0HPg2wbdu2HzimUChzZsxBVTUOnhonmbBQNRVdsTg2NolpmqzpM5iYb7GqP8HB00s8/NQMm6/YwmPPncVxfBzXoVR1qYc5Nq5dxcaqhR1L4pLku8/PsVgKUGLD7NjUT75nEl8+y75THnOzFzh+/Dy/+1sfoz1xgVw2Qctv3+LG9av5xCc/zUc+/H4efuxpKpU6586cpiujcOz0HAjYf+AYqStyxHSdMAjJJAJUESBFgsitsCh0pGKiaDq1Zgst0UFnOkml7lCoeiT6RtAVwYXpAjFLozs3yGKpSqMZoyNZRk/0YmsGExcX6ewYQVVdxudKjFg2gZlCj6nUi0sEPaMIoLRYZimMyMTy4ARYsV5Ud4oGFhcXy2wazuErwwgEU2MLrB7I4xl5bAmXt4/wPRKNn/g0QsQBRUpZW/l+B/Cf+T6j85/xg0zPlwP30Dbi/R7tDLv0yj1cEpdb4Uan3eH/Xkr5dQAp5cIr6v87cP/K7o/isX+18mVWOPRXRvsfi/c+n8+wNW5x4GybCqviSnZtSrH3SJErNgxz+02raTgeY0+9gKFJbrm6i6uv+P/Ie+9oSar73vezK3VX53RymnwmD5OYwAzMMMMMSTAIBAgRhKItB3n5XVv2dVr32tdXT5b1HK5l60pCEpIAgZCGzGRgGCbneM6kk1Pn3F1dXfX+6APGNjCDBCxA37X2Or12166us6t27fT9fb8KTW0N3LquNpo6dLyHBVdeSzIRo62liVd3HSAYitA5uQ3TFIQbpuBy6TQ21PHCtv189Q/+kIN7d/DlL9zPg7/9F7xyYICekZqB45kLac6eO4TH56Vatbhwvpf9B47wiRtX8cjPnmbxonncumYimqpwomuI++5cQ6nnIMlUgeaIE83OkitDZ8QkJ1rwVIYAk4Jh426sx5Z1tHwPropNwRlCL/aSsb1MqZOpql6cxV6aVYuLSgCvUsJRzlLM2TQF/EjlDC4jjtcVxFsdQS7XIvocDj9y9iKyJKgLtiLJEo58L/0FnXimSFD34iZDS7i2d+8oDlI2baY112ELGy3fQ6Zyeb7wvx7es4W8BuCX4y9qBXjEtu0XhRD7eWtbq/cDXwKesG17gNrU9rLxvg3vx+fc3wdO27b9rTflN73psNuAE+OfnwbuFkI4xlflX9e338+4vv24JtjdwNPjVkGva+jDr/Fm7RnJY1gqnS0qVUsilSsh6QE+f/sc9pyIM7ElwJoV8/D4/Oi6SiQUYHKDwfZdpwE43VNk0cJ5pLMFfvbk86xfs5i7PrmO3YcucG6gwG23XMexk12YpkkoXEckEqZ/KIFpmmzY8AnK5QorVyzhujVXU1cf4Xe/8jk8bhczpk+isamOG9dfTTSWoKMtwp2fXMehk0NYlsXRrhjrVkwna3lJFhTWTYO0iBCzw/iVIrZZxLBqD3nR0YqjPEY+n6Nq2RjOJrwiT6ys4XC6UM0UuXwB27bJqi1M9qapOGrKN0WtgYiapohORkTocCUpOWszqaQdotGRpWTrJO0gzvIIhUycKgrhUIQGv0bIUca0FXxShmo+QUlroKg1o5tRKsU0FeFEctd/IMo5kqxeMl0K4+tL88bTrHG1Zmzbjtu2vca27anjfy9r3/xXhA/YJITYKYT4HSFEw+UWfD/n9FcB9wHXCiGOjKcbgW8IIY6Ps4lWUxueMK5Z/7q+/YuM69uP9+Kv69ufpqaf/7q+/deAPxzXyg/z7xr6l41CvsiJfpPl08flpSzBtmMlrlvaDIDPBef6knh1uPP6qWw6mELTVDonhimmB/nREztZurRmoZdKZ1FkQSQUwOFwkMgY1NXX7kU46OZfvvsYV6+srffc/el7eG7TLnTdyecfvIdHHtvIoz/7JTesW8XsmZ1cuNjPM89u5b//0W/z6u5DvLB5B9eunEckHCCervL4c8e45dqaS5ghnKiKhFOTkJ1eXC43QghatCQVZyMJ04urGkcIQYAYJbUOhyJw6yrDGZlswSRVFrjNMYYMPx6RRxIC8sOUJD+6Vgs1lgpDJHNlZElQKeYwcOBy+wjoUFXcODQHijAJyVnKWh2yZRAUCfJyPbjqcFBClW2GUyVi6TxFvQPJ4ePUKJSzIx+Aay01xd9LpY8AbNv+H7Ztz6IWU98MvCyE2Ho5Zd9P3ftXeet599vq1L9bffu309B/N9h/6ChGosDTe21cSpnTQyb33TafQqlCvlCmrcHFPz68m0Vz2hhJVgiEgpzoHsLh1CmWLV7ZfYKGlk4uXuxl974jdE7u4Ke/2IksCXbsOsHMWVD85SvY2Jw6c4FnnnsOq1rFqtps3baZ2bNmMjoSZXQsyvBAD4pURhICry7Yt/c0m7ft4pnntrBk4TRe3LoH27LYue8kc6Y1kS+UqFpVnB43mb4MFbeNUs2RTFcoVGyqkoPRfJqyCS0BByUcIGzODeVoawhQyEFj2EtYjFJSHNiOOpKjWepCDRh2EM02OXkxQUeTTkVtIZrLoesKcVvH43HR1RfD7xzFITsYyhaYXK9R0OqQZYVzPUkmtASxtFYso8JIIovU0IKZixH0unCoKnqpD8uyCPoj2KL0/ofWIvgY8tHGgBFqHObL2iL8jafhXrNyKYdeGmDFTA8SLrYc7OJ8X4phl4KuKXT3JFAcLhQ9zIQ2H1fObmDjDo1Vi1v42eYe/vF/fpbdJ3LcfP3VFA2FTDbPPffez44dL/O7vzeXAwcOcuddd9fkr453cd2a1TQ2NrJp0yYeuOcWxhIl7r3nU3znO99hYts8ViyeRnNjmOc3v0ZT2Mn6ZQ3s2+3AoUrMm9mGJAleedWNhM3Zi6Nk8iWOnOolOVwGycNYPoeEzYSIhEM2cXkjWEIQMGsBMMNmHXU+CNnDuCMdGJlhKqoTXS6TNKEp7EPL9wCQqbrwuVUC1WGyNNLhLZArgUuS0YoxwsE6nFIVrx0nbfpxWUmUikSuINPZEsCSBB5jgKTlJ+x346sMYcoWhlQln8uiSBJZEaFFjWG424H32ewCPjI9+aUwzsG/C6ijtm39xXHuyiXxG9/oAW5ZGuKJV+OE3VW+etcsuqNVVl/Zyo79I8yYPZdZs6fT2NiIIgSPbz5CV/cFUlmT37p/PZqmMDRwghe2vMriJcuJxWI89tjjhEIhFi1aSGNjA5s2b6VSqfDVr/4e27ftoGqWWTC7g9kzZ/PSzv18/wcPc83y2cyY1s53frCRL91/A8PDA1yzuJVjp3pZfMUkNE2mfyjBoiumMHd6C6lskauXdvKzp/fz4C0zOHwywMWui6ybLnN40IPHztBbDtPhStKbdZI1ICc3EZGjBD0GY4YHzcwRdFQoOFvJZ4dx6VVS2SIV1aaEG6fuJliOMmwEadRTKHaFgANKeiNGtpegw6JimqAFaHFolKUWpNIglt6MxxwiU9IoOoNoqhM5P0ZJ1snLboLlUZySTU5pwqmAbEAhm/oA7vTHqqfvoLYjduTdFvyNb/TpVJYDB4dxqDpb9vdTFj7O9w1wri/OtVcvZPa0elRF5skXj2HZCqG6CUj9eYajeX65+SQOTcbrVnjil5u4JlVFdzp5/oUXufHGG3jm2edoa20lmUoyNhqjqbmZbVtfoLneh1UeZf/+fRgVg737juDWDM6d72NiexPf/OdHWXFFiOmTIvzFP2zhz37vFnxeF89tP0lf/xATm12Uygrf+PZzuJQKRyt+Mpki/aMpnFqYOm+RQ+d9KKJMxXTj0HX29eWZO0mQN1zookK0qDBFjoMQlAwTSW/CXRkgqFiMVfxoioIiFHA1YeYLmI46KsU4Tgrk8wWqRIhUYjgFXEx6qVZz6A6FpFSHXIxi6BF0r4vTPVGmNGvoikXFGUG3q5j5WhDPUDTN5LYISdFAuZL9QOSyPi49vW3bfyKEWCGEeNC27R+MG8Z6xolt74jf+Eavqgo3LannlRNZ7rtxBmXbwc3LZ/HEqxmy6QTPbxllz+GLKM4A//x3X0OWZUoVcDg1PnPHWgD2HzxFX0xh7dq1NDU1kc1miITDrF27lu7usxQKJU4cP8K61Qu4dsU8Mqk4d6zrRAjBd3+ynbmd9ay/MoSiyLx2oJt9e/fSFl7IuZ5RxoaHePb5V1FVGWx4eusBNqydS7FY5MThI2xY6GOy1cegVUZtF7T5o+zqVeiP5Vk5SaJOz9GTdjAxLKFaRRQrxUjJQb5QJu1rRJIkhqM5NFVCBFswJYvRWIJWfxm9nKRSsqhzOHCW0mQqgqLWgAWMJTN4OyaQy+RwOGy8DoFVzjCQMZkaMHBIMSjBxJYmZCtPpuqlvzdGe6MPpxoiXE1CXRNqJYXXzuGQ7Q9mTi8+Ho+8EOKvgEVAJ/ADavLXP6G2gP6O+Hi89n4NuNw6sUwZj8/D3CkBBseylMpVpk5uZu2KGWTyVRbOmchXPv8pntu8GwC3x4tp2uTzRQBOno/zta99jac2bsS2bZqamunt66dYLDJt2lSCfj9XLpqDx+0h7FdYvbyTXQcu8vy2I6xc3MyqJW0c7xrm2JkBRmMZPnn9LGZN8hKNJvjjz8yhWMizeo6LZn+JCY1e5k/UiEeT/OE6L4mCxKmYk1Mj4JTKvHjWhaOSZlFnE3G7DoCiEqLZVSJryCA7kJ0hJgfL6FIJf3WYjqYgYY+Es1rbYZrfUEJ1+TDUMJUqCNvEsiwMUWMG5gsGk1vD6IVeFFWlUUngcHkx0Gn3VRB6LRowY0jo1RSyM0BQTjGlxY8QMrpVU90JeJ3YzhAAhqv1A7rj0mWkjwRuA24B8vAGQ9B7OQU/Mv/h+4m9Z02Wz6gF3NyyLMKOI0mMSoUfPHmYu26+AqF6mT1rKrpT5dSZHlwuN3fecQs7dx/lmU2vce2a9QDcfvsneeSRR/B6PTzwwAM8/UyNd2SUMnzhs3fzjb//Z5bMn0jZqPD0C3vYe/AUpVIZRZJ4fscxRkfj3LCinZULJ/DwxiMsnOwmEnQyrUXhQFeGExcK3LWmjTO9GVx2HlkSLOmwkK0cL51KcGjIQZvcj1MUqRo5ItYgg2YD0rhfVEhEOTGmIFlFfJqNIXmxLAthGQRI0pNWSGbyRM0g5ZJBX7xIX85BrOqj7GzBL2cpl4q0eIoIWSVFeFw2C0qlMm6fH59mkqsoVC2w9AacFMnl8yRFA7oxjKsySF6rbYdim+QzKaIVL27r/dzSfh3iY7NlBxjjXBUb3mAJXhY+HmOdXwNbdrxKWLbZNa5tB9A7EGP7vgt87Su34HY58fprPdfCK6bzl3/zb1y5dAmKohBNZJHVAPX19Rw5coSh4WE2b97MypUrcLncqKrGj370MFfMmsBTz24llYqxa18XjXVeJk5qQldBlmTO9ac4f2GAOZM87NhdxLYFA4NjHLsQ4GRPEVmW2PLqYeZOawRcPPdyNzd2muwfdFM0ZUajcSJejdG0gRxspJwZxetIEyupXBjNosgyYV8z+XwGzaEymjUR4VYqmTH6TS+eSpyKpxVVzlHvk3GZNQUbS7W4IIVQBZTKZSTcuCSDUr5IT2wUv1OhShFdhXI1hvDUwigC1gh5ZzsOyaZkyNi2xWimiOn24JezUIhjuCOYRhm3anM+XsHdUg9kPoA7/pFp1JfC40KI71BjpX6RGpX9u5dT8De+0V+54AqGu19hxSzPG3nDMS9Tp7RRSA3z5DM9HLtQwuNxEvDqtDSFsW348SO/4JWdu2mf1MmPHnmERcuWMf+qq9h/5AhXLb8KVdMYGBzk6MFduLXl3H7z1YyO9ODz6czqbKXrQhyPDk6nTsXM8Jd/eBt7DnRx85X1FEoG5/vamN7uZVqbC9O0GIu3c8UknSPnEmRSaQbjDq5oyyFJEhvjGjdPLbNrJIJa6uNo0ovLoVCnZvC5vGgOJy5jgLIcpFHNUA004yz2UZK9RBMV6lrcOI0BHJEOTMuG8UaflptpcwxTUXzYlSwX0xoT6zUk1UXA7cTrEGimjC07KVcEY2M5prU0IskyQyMZZCCgSAhN0BB046tGqcg+VJefvrEctm3jlEzCHgdqcfADkMDmo9STvyNs2/6mEOI6am/KTuAvbdvecoliwGU0eiHEImAlNdZPkRptduv7TDH8wOD3e8BX4ciFAldMcnGwK8mCziDHLuSY1tHEiQu9LJoVZHJHA8lUhpuvv4re0QrLliykvr6egZEYN95yK16/jx9+97vc88ADjF7sob29DY8uc/99n6YhKHOmu4fli2dw+OgZOicVCAd1rlk6jYce3YGmynS0hOi+EKBvOMfFgTg3XzOFLbsHmNzsZOOrQ9x13QT2HBslU5RYt6KTOnMISbLZfhYm6bFawI5dQRKCiY0BysUsQgngVtwY6QFyqheHw4FaSZLJ59CwkSSZGY0VCpYLuZqgWs5RKhZQVYWC8OIRGVRVUFUcGKqPmUo/aQIERJxwsB4zn0F1uFCtPGV3HWE9iUSVci5HZ51GWbhxFDMUdQ+OfA95vQ1vZRArn6E+2Ioky9jYuIt9FB3t2Fxy4fnXxMdqy47xRn5ZDf3NeNsaEEJ8VghxCPhTQAe6qLF/VgBbhBA/ulx5ng87ZnV4GYum6RsrMZyCKc06s1pVzval8fiDrFs+ka3bd9F1foQ5MydSLpf4+S+f54b1q/jiA3fwi0d/ykvbt7P86qsJRcKcPHGC3Tu38uD9d3DN1cvZc7CL1/adYNb0CXh9Xn7y810smT+BoZE4I/EiZy8M0D+c4rqVM3jtRJKheJm6kItbVnXwxI5+Whu8OB0KeQMCPp3rlrZyLhegP2kiVQ08ai2i8opQitMpH0pxhGo5z0jWZiyeQQ+0cW60pnNfsDS8Vox41Y/D4cBpF9HKQxyNOikVC7hFgbgZwKXJqFYBgJF0FXdlBEWSCFYHScnN2JaFT0qRs71ULfB43PhUk6FEgWRJwmEmKZsmaUNGKkZrYbjFGBU1SFppxlUeIBGLMjKWomBAtfDB9CG2kC+ZPswQQmSFEJm3SFkhxGXNj96pp3dTEwoovs2PX0EtKKbv3V/6hwfxeIpzPQZC0fnWTw6wfuUs9p9JMbVF54cvnueaq6byws5eznafpWyepVRIMRg1WLJkMT97fCOFYh4jF+eZnx9j9drr6D55kj17dvHJDTexacsreD0u6huaOH3yKH0Do4QCPl64MMrWvXHa2tpo6+jE7zLpHS6y+8gwquaku3uQjTt60Vwh9p+K4vL62XY4wwsvn+LKWY0882qWoVSJ1y5mmdOiccGKIBQVW5YZS13E0+DH50jRqCRJiAbMdA9+txe1NELeFBTUevrHckzRPWTMAFI1y8TmCFgG2UKFoUwWCR1BgEK5StkoU/EFMcc15BTToncoxeS2DtRihqgVxoylCAZa8ehlVMUmrbZgpJOUCOC1oiTLEobsoLu/QMRv4wo00SzHqThDSOUqturh/WfkiY/88N627ctaoX8nvG2jt237Xy7x4++aCfRhRC20VmPH0RS3rphAQ1jQXifYeWSQ4WgCt1qlUqxw+/qZHOmKcdvqZv7iW1uItgf51K2rcGgKDz32Cn/wpWsYiFusWXsdp06cpHP2QiZOmkQqleLppzYyOBxjcDhNLFFi1uwF3HnHrVzs7cesWlw4e5pb184BoOv8EKfOjXD3nTdxunuQGTNnER06y7IrWhiMFpjSorNgmpe9x4aQTIPrZpm1XhR48bTF/EkB8oZAxgF2AUmWMfRWJsh9FNV6AnKMpFlgRoNFybKpU1KUbRtblCjbFl63jNMXRqGEbiZIu1rwGGX0cbFMgKLUzITmCI5CL6plMVTwEXJrBCr92L4mLMlJqNrHkCoxEK3iqQvhULN4RYqCN4RDrlBFxykqGMi4pSJRM/AB3fGPdqN/M4QQK4Cp4+ScCOC9HHLOJWtgPKT1W0KIXwghnn49vRcX/WHB4XMZ6oMuVs4NM5S0cTk1smWVBdPqcDlVkFU6O3zctKKVnQcGWb5oGrqcZ2Aoznd/sp3P3Xc7c2ZPw6uV2LplC2vXXcfmTZsxDINAIACmwZWLFzF5UisOd4RgKECxWGLbjt2sWLYQw7QxzSqpTI4jXTGuXDgXSRJ09aRYtngGiazFz188wefuXIzHH6B7oEg8K5g1exLJYm04eqTfos5RZE59kYiWx5ScFOUQ6XwVh51FliRkR22xUvOE0YWBqzxETmumoDXiNOMERIqcFEaySpiym5QVwFWJ4apEKYpaB5OiDk81ilGpTReych1TQ1Ucis1QKUjAHiWXz1C1bKp6AyvbigjFiaYoZJQm2pwJArqGyxgkKTUyFk8zbITxWbEPJLT247JlN07O+Rq16TeARo2cc0lczur9Rmohq89QU7X9WGF4NErJUFg5pSaYmMwU2HLIYMXsAIe7Rvje4wf5H19dDYDHpTE4PEQwFGDp7Aj/7a//mRVXXc1Tz21HIEAINm/dycz5S1h3w/U88bOfoaoKN9+0jk0vPM9TLx7kS1/8LMlkmod/+iRXLp6PEIKVK5Zx/MwxDp8a5cufvYlYPMW//egZ7rittv9fqcJINMXZnhiKqvLygRFkq8xtt83gsY1xZhBlLCcz058Hp8K5tJc2uZ9jUZ3eaJoZdRXQBIVCkULVg7s4CgJMG5LpPAXDwt0ygWrVJjqaQlMlNLVMuVzF7y6haoKSHiKbreLWTdRKhXIxT1rx4HZWSRQEutdDKhljzN+Azx7hbMZLizdN1RL45TznkiqyVMTh8lAVGoWKD59fQ1dgJFHAEwgChQ/gjn80GvVl4DZqEnSv61AOjQvQXhKX0+hLtm3/069xcR9q9PYOMHRuhGwhgqKqjERznC8WcWoKTSEnsp1m4/NH8QWClCs2mWSaU2f7kYXNulUL8YWC3Llh1RvnS2UMWid1cr77LMdPnMAyDRyqzLaX9zB95ix+8vPnsapVdu4+gObUOXTkFLZtsWXLZu66fT0v7jiCZcH5vgRHTw/TdSHB4GgWn8dFJODG69XpG0hw7nwvT+4YoD9ZZffhHFe0O+nOhrCEQvfQKG3herxqgqaQi4KwkfUgmViaXNGmo6keSwZBEreiEA66cOR7yVVUJkR8mIobKd1DTtFJUIdbd9E/kkR3OBBGhYocIl+pUKgIdJeDiCNDzsgzq8Gm3pPH8IQ51V3A7w1hlKHBmaW5rhmqBnY+yXBGsLi5jEvnIc22AAAgAElEQVQeJF+18ITduBj9QFxrPy40XMbJOeNqvO85Oecfx4cSm4E3/K9fV7r9qGPp4vkMqBfRdSfzJmj8IqejKm5mtGoMjha5b/0EDp4vM7NVpj6gceKiQaFiMW96mGMXqridVfoHRmhrbWTHKwe4etUqTp+9yPobb+R8z0U8bg/X33YHxapNPBbnE5+6i2KxSLFkIqQqn733Np5+djMLFy9jzfV34PN62LVrL1dfo9DeWs/iBTNIRAcp5NO0NIU40TVEU1AmV+dlw1WNPGsVKSacdAZzSFKBRN7EqwkcVo6cs4F2eYAYzTgKvfg0L7Kkolk5NCOFaVng8FIxLSzLwtLr0CpDZAoGpiHjc5RxiTLpvIdCxUJ3qGjVDJqQCPmbcagyXnMAC4ugz0WbmmbEamCi2k9TwEdITqP7CpgWqFULW3OSK/pZ0FhmtNpAhzxEqQKSUmHEbgF63/8b/hEZvl8GfmVyzuXUwBxq5hRfpyZi+ffAN9+xBDWzCyHEDiHEaSHESSHEV8fzQ0KILUKIs+N/g+P5QgjxT0KIc+NKuQvedK4Hxo8/K4R44E35C8dVeM6Nl/2VRJSXdHoxSnmO9hh4PR4+sSTMtqNphuIGfrfKhmUhXjueYCRR5mJM5vfvnsvWV88iJIkb1yxkx86aRHr/SJFpU6dSqZhs2rSZK6+5GksSPPvUU8xftJjb7vwUz218imd/sZFP3n0X1224k//9d98mm83x/3z1C/xy4zMAdF/o4d577+Z8f4KnntvO7Gn1TJlQR/fFKIeOXWRBp58V8+rYeyqGlUtw8zyF8/kah/1IzM/0QJaxshe5WpP19pkDFLUmNE+QVmcKo1Qkq7aQpB5HeZhSLknUbnjDdDIkp0mbHhRhkZCacKkwsTGAXymAXodZtXBIJuVcnLJwkSJMgzQCQLUQpzvhYFakRFYEsG2bwXIEpzGCEAq+QAiHVKXOHmTYaqZkQqpo41MLH8yc/j3i3gshrhdCdI0/e++5k82lMO4b8XNqGpSvk3P++XLKXk5Pfxsw6d06x/D2ZhefpWb98/XxyvoTagsSN1DbApwKLKFmWrFE1PzBXo8ossfP8/S4R9i/UhMI3ENNWed6aj5fl42R0RgHTiZQVJUzx3rQNQWX1kRTQOapV4ZQNIl0QeB0uPjGjw6y7MqZPPXKILFYgr6xk8iyCtUi//Ldn/OJDTWvgXwui1HIsWD11aiaxre//g18fj+6SyedTnH8yDFsu+YXnymZHDhyCs3hxOtxsuu1vTTWR4jHk3i9fn788EPccO1iKmaFF17YzuK5rTy2+TwCeOnlwzx4lU69T6U7ComSgl21SNsB+sayBH06IX8bAonewQRel4Ya7EC1qhTyRUYTBdS6RkqUKKQLBNomYgGWVSE5NErVDjCh3sZWAkSjORr9HmKZMlURokWMoiuCnGgh7ANNrm23Neh5Xu330BnO02GPMGLUoTgcKGVBrmQQ1kogQFMkHOVRBip+PLoD2Yh+AFF2vCc9vaipa76dAcsHhnci5wghdtu2veytvrucRn8UCPAu9YnHnT5ed/vICiFeN7t4O+ufW4GHx4MI9gghAuMimquALa8zAMdfHNcLIV4CfLZt7x7Pf5ia2cW7avSNDREW+kIks2VkqR6fS2N5p0Y8bXGowYuNxm3LAwzHi3j0afjCPlbMq+MXryg0tExgw/ULeHHHIXbuO0m4/jUkWebZ555l5eprefrhn9LY0EAkHGRy5zQQAqfbzemTp7nrvprsdalUolIxufaGDRw8sJ9v/dO/ce2qa8gVDG7bcCuxsUHC7jJrV87g2PHT3HTNJMJ+J5Zl0dM7wv6eGKpDw+Vy8ciuYeoDHkKkmTGxjVSujJztQ5YErQ2tCEDL14bQRa2FRn8Fd3WMiuonqNpIRga1ksCyLALeIJM9KdRKhkzRhVN14DbHKFoSI0UnTS312EJiZCyD7VNwR8LYlomwq1i2Sd7RhnA5OXN0AK/LRvK1M3ghSmhyHXnVjxCguiA+OoSuClI4gXfbr7xbvGeMvDcMWACEEI9Re34/0EZ/CTjf7ovLqYEG4IwQYtOvumUn/qPZxX+w/uHfdb3erdlFy/jn/5z/Vr//jmYXhmmx7ViB9fM9VBEUSyYvnyrxO7dOQggYSZrsO1dh1bwA0XiRF3cPsvrKVkyzimEY9A9luO+OFcydNZm1qxbz4D0bkCplPvfgg6QTCf76r/6cHZs2Ud/QQNfJ09x9/33s3vkqAB6Pjzs/cw9bNm1i1bVrWH/LLehuL5+643b6+weYNWMyQzGDUtlgxbK57DpWU5jZeXCAz9w4HT3cwsoJBpM9CRZO8hF0Wni1KtVykQYxREFrwbIsJKoY+QQVHGQqGi47S0hOUXE24vb48CkF8hWoSDopEaFNz7wRhSe5gjjtHIat4gg0Mz1koGLgs8aY3h7C63JQryRp0zM47CKLJnsIa3naHKMsmRIg7HNQV+1n9qQI2FXqrT7qqn1I+UGum+VnSp2MO1D3wYloXHrLLvL68zKevvSfzvJ2z+SHCW9bnZfT0//Vr/PL4r+aXbztoW+R906mFu+J2QXAxj0pNizx13ressUzB/J8cpmfvtEc+UKR//NkH/NntrD7dJ4zZ/tpam2hPuyicjbF9x99hS/cuw6HQ+OnTx2kXKny+ftuJ58v8OMfPITH7cbpdHLV4gUc2LMHo1xi+uxZPPrQD0GS6Jw5A0mSKBpl0skkPn+AptYWdr22mzOnTvDgPTcwd9Y0fvHk42gytLc1cGEgSzRVZflMF/fcNINHNpZIJDJcEc6wy/AzkpBRlASKLOEsRxksB2lgDJdSJe9oQ9JAM/pBkjAtgXP8KfDbcQbyQbwuGbdmU3L6SBV1AuVBPA6LgXKQDlcOp9MmIfso5fPUO+N41DwXSq1Mdw9juNu4ui3L8aiH0aTNbO8wuqVyNu7FoyapVCpkvEE8VhzczXS4RnnuvJfOwOh/vi3vC6qX92aJvcmJ9q1w2c/ehxFv2+iFEMKu4eVLHfMO3/8XswvGrX/GDf7ebP3zdmYXA/z7dOD1/JfG81vf4vh3he0vvUbQqfFqdxWw2Lr7DNevnM7+8wZhj0pnq5uBaJnrFoQJ+RwMxcOMxTM88swJXjvUy6qVi3h84w4sC86c7adsSjz6mIWiSBi5GEe6Ugi7RLlc4cjJLpraO0inUqxYs4affv97/MXf/DUAt999N3/z53/Ovb/1WzS1tvDUI4+iUkUIQTSaoPtiFL9e5oE7lvHw46/i0VX2nRhhMAkn+1LIRp5urYmIO8/RCw4afBJldx3OgEa8a5hAayOmItM3ksDncuAITqBqW+TTBQbGkkxtm4BpFPAbMWJZN1qwkULRQlctEjkdhzdILpWiWhcmU5IopUaxtAA+OQlIKOUoPXhpb7KxEezqSjE5LNGnNYBT42J0mM72EKVKgVisiiIFaQxVGJYn43LEKWpNwNl3e/veFWzAem/a5jsZs3zo8U49/Q4hxJPAU7Ztv8GvFzXDiRXUzCV2AD98q8LjK+n/xeyCt7f+eZqax91j1Bby0uMvhk3A34p/97xbB/ypbduJ8SCDpdSmDfcDl7V6+WbMmDEVRyrJ3Ak6A9ECnjXTyJdh+XQ3pmnx7MEMX7h5Asd6ynRKNs31XnRnkfVLGlBVDbcTNqydDMDjZpZ8SWL9qhkE/R5++Nh25syeyj13rMOyLBL/miZbKtF1+gz5fI6BgUF+/IMfIgkJbJvB/gEuHD7K0JkuRMXk+ImjbN/hx+/3IAuTXK7ES7vP0nVhCNkqcff1nURCBu6ch3MjGlOdA1TUKpKQ6HDlUaQCw4UQsxuqmFYWVznDpOZ2TMtGL/UAUJQDuHSdaqWE0xhFdwhUj45aGuJMVGdSvZOAWsAolGj2ewgpWXQ9TZ+p0psuMOJvQFNVJI/Eid44wXonh6NQH3bS2Vql2VXAtHIYM0OUTZjpSTOUlbiQcXPVRAm/M4kiAgwnih/IQt575Jf3hgELMEjNgOWe9+TM7x3a3u6Ld5rTX0/NOfhRIcSQEOKUEOICtdfxp6lZRP/wHcq/ndnF14HrhBBnqa1+fn38+OeBC9Tcar8LfAVgfAHvr6lV9H7gf74prPe3ge+NlznPu1zEA2hqrKM/XjNIPnDeYOkMP17d4vxIhaf3pbhhoR+nplCsyGw7kmPZDC83La1nx6Ex6sM6YbfJsa7adpWs6HzuziU8+uQOKhUTry+AsAxyuTz/53tP8qUvf4WmughzFszH7fbwhd/9HULhEHc/cB9GMc//982/xev1cOMNN2CbOWZNn8K11ywCu8rVizuIRIKUikU2rJ7M5I4G/G6NXXt7mB4uMjlYIlrWORwNMC1UpmQ7yJQlnIqE31FF0oMYpoVSzaMVhynJNa672+ujMezBKoyQVlswLRtZdZJVGplTX8FSXCgyVJ31TPblGCu7kGWBQ5G4fZ6CpigsCEWZoo9y21VtdNQ58HkcfOnGVspahERZ48Cgkwl6DNs0MKoyRTnEfVcqnIo5GMo7OXY+Tr2cfP8bPLWe/lLpkud5ZwOWDwsG3u6Ldwq4KQHfBr49PkyPAEXbti9Lq/gdzC4A1rzF8TY1t463OtdDwENvkX8AmH051/NOqPdUONmbpTnsRAiBS7P5wbMnmTGpkQvDRSY26gyOZZk+MUgya9AzWmLrzjMsmjeJe26Zy3Mv99Da4MXr1pEkiU/fPIf/9a1HmTtnBk0NIf7gT77B3/3dN/G43dx79908vXUbiViMT91/H89evMj//Yd/4Av330M4HGTbjld54flnWbtyDpoms2f/CU4dP8zsqQF27z1KwKMwbWITNvBn/7CNJe2C4RRMiMg8N+qnYhVo9tkMmyEKFUGkWht1FgsFTBGh2YwjaYIx24VppFBEimxJIijbOI0BUkoLidE0HQEb1bbwGoNk9Rb8LhVhCULVIcbsJhSPQsiVYpEW51Aigtfr4raJNtvOCRx6CL9L5voFPn68vUSikKLTazM3nOJYupG6sI4sxWn2lNl8qkqlXKFQ/WBCWt8rHvnbGbB8iPBrLeRh23aF8e23jxtisSSpkpPHt59m7VVz2HXWoqM+wrw5CrPbndT5JHYcS3C0e4gqNkbVz6JZjUSLTnKFMvuOj6KqCn/69SeZPXMqJVPC7XLTfXGMtetuJJ3N09QxkUeffBKP7sbv9XDm+DGQFV745VOcOnKYcjbB888/g8fr58Tx47gcFhHvMjKZHA/98KdcvXgS+ZTB/BmtaArcuKQm33X8VC+dDXniOZPuKHT1pfDqKrK/g5PnR2iJuBkhjFmtUC4XSJdtZF1G2CbYg/QZPlqraaoli168NNaHCGoSPcNF4g4dp7MeRVcZSeZIZ8Gqb6VQyVLIF2ir83Ah46Iqe8iXixQqWV7paaTrYpSSpBFqvAJNE6xa1cTOXaew6idQqgpGBnvJGAWcvkksWhDBjJRJDg2i5y98AHfbxnq//bA/AvjYEJF/VUQiQepLNqMz2pg5ycfUFhfbD4xw44o2Dp2KM3eyB1d/mb/4/fW8vG+Aa5e0USxXqAt76Jzgp2oLrrrCTyxnM33aVD6xbhGbXz7GZx+4l0gkxPnBOO0TJrD+5pvweGvxEM9v287iKxczbdpUOiJuzp3t4p7blhGLpeg5exCznCPsiKK5SjSEHKxeGMHncdA7mME0CiQyZRyKYM2yKQz2XmRRW5H+U/DAMhe7+zWaRT+VCQ0gbCLUph5DJT+uisRkd4mqLde09UZNfG4HFQGq6qKeQcyCxdz2EBV03MYQ5ZJFR6SFqmXTQD+SFy6knAzGcswJltG1LGm9nmltPib5UpSa3SihZhKZEnOnBpk+wUtvbwPL2ou8fE7w4P2fID48xIrZXp59bYTlnQ6eizqJeH4lMuW7gm1D9Ten0b9thX5siMi/Dg702Hz+lk4OnYljVKqUbQcNIZ1swaB/LE+wLkJTnYsFs8IcOJPi+V0DLJ1bz9zpjZy5kOCnL/Ty5XvXISyD7vODpAoa69etZs++w0zqnMmGO25nz65dADz+00e576u/g9vl4tShPVyzcim3bbiVf33oSba/tJPf+sw1RMIR6iMBXt59hj/+4nJeOxan62KUtojM+qUt7OvKsnnfMAun+SlJHnZdsOnwlvA4bGRMYnmbiJbFL1JkRJh82SbodTLDl6So1uF3CkpaPWsmlhGaj0CkiSZHmrwUZLTsw2MlsSsFDFRKzlYC1WF85jBxGhFC4AlEaPTYlHHSm1ZodGSoWvDKRYWFDRkULO64ppFyIc8vdgzSN5rm+XMeVl+3hCXzGjFslWzewKXJBNwqV80LcTJas6p+XRvg/cJ7Maf/sEAI0SGEWDv+Wf9PUXb3vV25y4mn/903rZx/7LB73xGwSuw9PkRHRPD1Hx2kJSwxNJbBpZk8tn2A5ohCPJVnUmuQi8MZihWJZ146z9OvjDIcNxgcy/PM1mPkSxZ/8/ePomoOLvb0cuZ8P/MXLUTTNFLxBJuff4EJc2Zi2zbP/OLnSELmiY2b2bX3GHv2Hgbb5mJ/FIcGz207wuJZISRJIpMvsf94jGntvnEWXxlkB6OJAsf7Ehw8n+XMqM2JwQqLWw1OJDxEHHnq9DLpXImzGS8RMYbXKZE3YCiv0KgX8esS0Rw4qylCepVC1YHD40eVBZqV5uSYDOUcBVNCkQXpgsGFnJ86RmnS81zMB0jbYfxyju1HBlnSnEUIQW60l3yxwtR2H+V8mq7+LCUlws5DYxRKBqWKzQv7YiyfXiONTWj00JV4//3pbcCy7UumjwLGg2x+DnxnPKuVWhg8ALZtn3ircnB5w/tGatziQ9QW0za90978Rw0N9WFK8ShTpjZQrVpIR3MMpjUUj4doXgPZQcl0MBCtcrY/RTxdQpJdfPGBdcQSWcKRCOmswWfuWMXgcJREusTMWXMYi6W50NPHjx/+MR63i8OHDlEyTWbMm0OkqZmWiZNZvHgJs2bNBGCo/zyN9RojYynyRZtXdr7GsoXTOHRqjGrV4kT3AG63C1mVqUoOzveMMmd2B/PndJKNDrG2LUUspzGcU6naQ/Qa9ThEBZdLULItLmRdaLoPt67S1Z+nsbWOEkFULU1vEqp1TXh1mRMXo3gmNhGQk7Tgw69myRdtUlU/hg0VwyQUaEEA5/pT6Cqcr2qUzDKbTkh4fH6Mism/PnaYGe1eVk2o0jZlNfGcxAP33cmrew5z7OxuXCR5tctJtQoV0ySdq6myvf89/ccGv0ONDrwXwLbts0KIy3KtvWQN27b959SCYL5PLVjmrBDib4UQk3/ly/0QYcrkibSGBENxk4awh5uuuxJLOLhqcScNjU389R/dwbGzSRbMbGLpvGYmTZpCe1szmqqw9dWz3LB6LhIGmWyeF146ye9+6U5OnTyJWTH55te/TrVUZOHKlUyaPoPpc2Zzy3330tzRxtpP3MyO3XtJJJIAdLS30N2TYunCaWgqrF+9mM4OP59c1cqi6QFmTGth6sQQt67uQFIczJo+kWkdQbxuB7ddP4sDI24a/ApTQhXWXhGhKeRmQkgwoc7JjVNL+Hw+ZnhGkaw8S6fo1DnyCDPPDbNdrJrhptVr4KDA+ilVyqbAJZVo9CmUtTCNXvA6YW6LwuRmP1Mc/XSovUxvcdMS0miqD7OqrYBha9SbFzFKJeRqmWUTbE4NVZjd2cQnr5vEc5t3sn7NchYuns/E9iauniazeoYM5Qzzmmr9yPvZ6O3LGNp/hIb35TcHwQkhFC6T5nBZNTzes4+MJxMIAj8XQnzj3V/rhw/zpwXJJOL82xPHWDK/g+ULJ/Ls9pO0N/vRNIUN6+axcft5nthykQ3rF+DRFf71R9u565YlCCG4df1iHvrpFpZfOQ8hBMn4KN1nLzBx4kS++pXf4aWnnkaSBVeuvIpDO3dxdPdeps6ayc333sNPf/ZEjd8uSdx91x1s3HwYl65w6/ULGE4LeobzHOrO8NlPzmMomuHpHedYNHcin7ppPj/fdIb6AAR8TnyNjQxnZXZeEMxvMsmWKuztk2h2JNAUCZdUZqTopD7k5Ypmi+6YxLm4xGC8TKokcXJU0J8wKalBmtwmB8fc9IykicdiXMz7mRiWqZfjuKpRUobG+WIDU12jjOVswlISSZKIMMLuYR8dAYt2qYd9PTYpqYWO1jAelxOnSNB1rhePy8mi5UvpGjLJFQyGB0eZ11B7fn/F6OjLRtW2L5k+InhZCPHfAV3U9O+foKZudUlcju7971NjzsWoEWH+yLbtihBCokbU+eNf+bI/BIjGEnT1plBlmd6hHI+/cIqA38Nzmw9z642reHHXEEIIhmIVUqkMz2w7Q+9AgoGhBM/uOIeQQJYEZ873M23aCEPDMTqaA7y05xz/97vfw+Vy0VhXz3PbtuDyuBnoHyAUDCHLtX3plRtu4bvf+wHzZrRw7GQ3z7ywl2tWzGPj1rOUTY3HXjiJVa2wbV+Qsqmxfe9FJL2Nc4NDvHbgLPmpdXRfiDN9ko9DA14skeW1YRdRo4TTUWGkEsKWHeh+mZePDrLUr7J32Es8azBlSivrrgzjdqq8cCgN6RFmh7MYpkVFjlAsW/grA5zNOEmYXvKSG68jysW8l7DTYNBsJJXLorZGMGwTr7NMcjhHX0bH72lh6HAPq2+Y+UZdr1oymR9t3EFzQ5DF86fz7QMn2LH/OA3mCH2VmlyWorx/G0qvz+k/JvgT4PPAceDL1DgD37ucgpdTwxHgk7Zt/wdZE9u2LSHEze/yQj90qIuEuLI+wMa9Wf7xf32J51+5yAP33Ixhe6iL6Ky5ei4A2VyZCRNV7r9rLd9/dCcLl65h2oQGpkzuYN+BIwwMJbhqyRQCPjc/fHQLNjb3P/ggkiTx6GOPccWixay5bQMv/mIjB196mcZIGOzakHPXnt0kom2Ew0G++bf/jRe37uTTt18NwEOWIOiTuf7qTn74y5N86//9M7rP9bFqxQIk2UGs/zSfWOyif6yA5tQYGrP4vdsnsO1IlnQiyUS1D1UusKPXw/Q2PwvCMUqGxdRpV5DMGbidKqm8QVuDl4FCFsjyUo+LBaEYB4ZdWMCkiKBdH8KyLF4bcjOWyzNzQR3XznQSamqmmIwy1ZMmU7RYMdWDZVdpVwZICh+7du1Dt+IgJGwE57oSDA5FcHn9eMLt9Ay8RHO7B8vUEPDGy/D9wsdoTq8DD9m2/V14I8Zf5zKEBi9nTv+X/7nBv+m70+/yQj+UOHAmzVXLr8TjdtJer3DkeDetLc30DqWwbZvu8wPMmjmJ5oYgR0+cp33CZG5Yfx1bduzBsixOnTnPH/3hb/HKa6fo7R+jsW0Gn//sp9k9vk1XqJSRZAnLsigmknz685+joamJG269hc6ZM1mxdHEtrn/uJFpbGliyaC67D3Sz92AX82Z1YFkSjz9/irs/9Qmam+rp7a/FKLldGjfefB37zxbpaHQjUJg7tYEdh6N0NsAnlkU4lw/zcp+XWb4YolLErNqcKbawrNPJ9Qu87OkusbvLYPFUF7as8EqPk1n+JIosWNScpzsTwEWOroyXIdpZ3m5x17VTMRU/kiThd6s43W5yhsTplI+pwQJlycNwXmVGu4+p3gxtDW6Wzw5RqKj89pfvY+LEVtatWohVLXPVikWomkx1XF33fW309sdn9R7YRq2Rvw4d2Ho5BX/j9+lzuTwlRyuzO5splQ0a6/3877//Ps1NDay+djV7DnSza383SxbOYM01C/nH7/ySGdM7qVQq3HHH7Xz/4SeYPKkd6f8n773D47quc+/fmd4ADHrvvZMgOkCCvVeR6t2SHZfEvv7s2I4TO45j59ppTlzibsmSbUqUSIkFJMECgmhEIxrRe++Djukz5/tjIF9ZV7IoxdSNpZfPeYCz9tlnBjyzZq+99l7vK5EwPbfC1ap+9u3dRUxMFL3dHSwtLaHz9sJqtVL825McfPB+kjdu4E5rK8tLSzRXlRMVEUZjYxNzhkVKSmuZnl3k8vUGXr9YhUwiUnarjU2ZOfj5ulZOY6ODabnTi04tIzoiCIc6iI6hJYK95YT4qihtGKN73E5Fh4XK9jmUogml1ImP0kjfnEhCbABymQSJIFDeOMyqyUJNt4lbd8aYXjTSs+JO87wXnct+TC2a6FvRk+RjI8lthlGTG2nBDlIDrbRMKpDiYF+GO4MWf3RaLVKJgGHWdV2QeoV4TxM360e51rxM/tadxEUHc2x/LqUVzehUkL4hhus9TpSOZUS45+G9eBf//kygEkVx9Y2T9d81d9PxI78j73ZLJyqZjRfO1KJUyIiKCCAxZRNXypvw9dbTVHcTjUbDb05X4qH3RKrUcelGNTa7FRxOSkursTugp2+chqYufP0DOfny62g0KkKCAvjiF7/AngdOcLumlsiwMCoulQCgVCj58l9+hi35OczMjPG5z3yM2JhwAC5cvsn8KsTHJqMPzkSpbaG0soX+EQMrK8t4e6p5qfQyX/iL/Vwta8Rok3P22igFm2JYFNQ89dheZnva2BhsYzDaB4VtgdZFP9wUVvqXlYTYRa512FlaWSMjt5BNaZHkZsQwsihBv9ZLnH4VcGC22hia9iBEZ6Lf5IenbQ1v/wCW1iwMzthoGlrG0y8Qhy4Su07BzHAvwb6RxIUbaB+30TwpxyFRMmroY8aswc1/iN6BCVRKORev3ELvoUOVmsInH86mf3gO4VoVcrn8nj7vD1F4vyYIQsYbBLWCIGzCpTX5rvjIO/22ojx671SwuWAjMVGB/PqVKv7qc/+LixcvcuKhB+kfGKIwO4XdOwtdHWQejE1M8sjTT+F0OlG5u+OjVXLowD40Oh1Wh8j9jzwGwOLiIs+dOoNE705GXi5Si5WHHn0EQRBob23FR6vBTSOwsrKCr68nc4YFXjl7g507tjO7YEcURYICA9iyeTOGuVn27N6KTruqavUAACAASURBVKthcmqG0+fLuV4zyt5dW5koKSM3N5sn7tvA2ZLbFGZGcs6wzMxKP3qtCrPZixy3KZpmtDhEKTlpvqiUcs7eFvn0M4d4/WINNY3dbMsJp7bByqqlHZ0SyofVHEhYZHhRSbjbDLem3DGuLhIb4ENWvIBdHYR/TCrH96Xx61eqSI71x2ulA9EqY1OEFHeNghCdkbbACFDCtgwPFHIZJpOFm24e7NixmbaW2yS5w+KCEQRQKu/dJh0REfufT/j+bvhfwCuCILxRxx8IPHg3HT/y4T3AoaIgqqvKuXStgYj4TDw8PLA77KytrZGWmsbsvJGZWQMtrR2ER0SydcsWaiqrKCkuJrsgn/HpWTo7u4mLicRDq2Zy0lWb9MLJ3/EPP/xPJrt70ev1FB49xLkzLi6RjqYm7j9xDKXak8bmO5TerKe6vpdPPPs0MdGRuHt48vCjj3O1tAKFQs4zzzzJy69cwOl08urZa3zvBz/G5pRy5Xole3dk4e3jzQunq9m7ORKAw3vSaZrWgdOGxbjMnTkt6VEePJnp5FzlFNdaVrnv6E6kUinLK0a6uoeIjfDksRPZ3Fnyo3UcYj2tuGukGEwK2leD2JeiIDMUlEo57ho5vmGxrK2tYrXacdNpOLY/iz5rMFPLsDFcwdCClOpxLQXxSrbFmCkp78HpdPLy1XH+4WtfpLNnhOmJEaoaRsgPWgKRez/Sf0jm9KIo1gMJuMrLPw0kiqJ4+276fuRH+tVVE6829KNx9+PyjWbyN/tSWVnJieMn+OnPfsquHTtJTU3l5z/+TyRSgSc+VoRUKqWiqhKzzYZfQABJGzdx6pXTfP1vv0DGxjROvX4FB5C1czsTI6OUl1wlNjaWxXkX6eRPf/ADNiYlcLnkKkaTifbOfnx8AzlxLAeJRMLQ0AihoaEolUompwxERYQyMTHF0qqRJz/+Rbbv2MWVy5dp7x5CKtiQqzWMTy4xPTpGaa3OxWEvglzvw5077Xh76BhdEYj3SWBSBI1goXXIhr55GNmdESanDSzMjnHDTYooCkg8/GlqnycrLZpxuYyu6QZ2bNBjsVlJDoDyQRMms4SUglBCAj04VdxAXLgr3/DwiS187K+a0XkF4hfqRnP/AneWglg2zDAx3UhH/yKbi7Zz7cYtFAolTQ1NFKX40GNwfRTvtdP/Gc3Z7wbxQBIuEsyNgiAgiuIL79bpI+/0E5MzuHmF8vB9Rfj4NJKxeSsSiYSXTp6kob6e2JhYJsbH0Xr4Un7zBg7Jc6hVakbHxplfWeZycTFOp5Px6RlePnMBlULB9avXkHt4kGy3EhAWRsHWrWhkUo499BBjIyN89+//gYTUVPKKCjGZTMxYrAR4etHcP8q18lvcrm9g95799L/yCm19A4zMzoHeB31QGAkWJ48/8QSCILBsMmKxWtn34JNcvViMRFyjaJMPKqXLcZ5/eZzM5FDWbFIOpAUwa5RStMGb8ell5hxaTtz/IIIgYFh+ichgT3avRwnj00ukpyeyN9uDqjYDX/3cfmrrBlhATvekBJvFyOUOFQ8lrTI+tcSNila02kIGro8jSFRs2bWP1MBV1HInZoU/e/bkE+Crp6VjgFdKesjMzsPHx5uf/uxXPPjUJwh1NCKXOhB+3YNGc1e5qPcF1zr9Pbv9B4p1AZqtuJz+Ii4K+UrgXZ3+noX3giD8ShCEGUEQ2t5k+4YgCONvYdJ5o+1v1oUDugVB2PMm+9uKCgguYc3adQGMl9dpvN4zEuJjyE9x4yfPXyA7I5Y7d1qRy+UsmY2kZGWSsWs7Kdu2IOq0uHv58PCTT5JdWEB4UgIxCXFs3ruHNaOJ1Lw8UnILKDh4hPDkJKITE9h6+BAxKcl4+njhExFGf3cPzfUNfPYrf41cKkMqlXLm1CtsPbif6ZlptmzfweGHH8UsSInOyyb7wF4iU5KIT04mITUFOXD/ww9xq7qay5cusSk/j5SNG7jT3MzM5BRf+OIXOXfTJVgxNGYg1MOOU5QQEOhDXJgHOpmZ+s4lmnpXOH5kBw23W1leXiE4OBCzqMVqs3OhtJ2tWUE8eCCF8vZV1pxqokM9yc2JxiF353C2O6HBnnjoVCRHu4NoJzO7gL0HTvDss89iczj4/KfuY3JZTuuwlc994jA3qroRBIHmrjliY+LR6z0YHBomIS6CRx59mLpRNRKJAAgoFO/rMd41PkTbcE/gIqOZEkXxaSAduKuEyL2c0z+Pi3LrrfieKIob1o+LAIIgJOHiGUte7/NfgiBI3yQqsA/XN9rD69cCfHf9XrHAAq7dSe8LPl5uPLo/ltOvl3Kz7AZnSi5x9OknUWm1WK1WGmtq8fXQ8+Szz1BTVc2FS5coOrAP38BgJkZHQXSy/4ET1FZVcbuujtTcHMLi4mirrefqmdfZUJBPem4uleU3kcnlxCcnMzoygtVqRefliVwuR5TLWZif53cv/oZPf+1vaamt48KpV9m0cztra2u89tvfcfDIEULCwujr62Nidpbg8DAS01LpbGtHJgGFQsHW3ceobp7mZnUfaWFy2keW2RTrUqtNj/HEbFxhaFYkPSWent4BTp0uZteOzTzw4IO8UtyKRikhxF/HwrKRriED3QMzXKoaZnB8mSWblF9dm8HTW8/xfE/OXe9E55vCFz73cWobWrhxs4qC7FhUKiVOuRsadz+kUimhwV5090+i8wjkvvuOUVlVw7VrpWwpzALg4ac/zfdfcZFiqlTvSNf+34bIh2obrkkURSdgFwTBHRfBbNTddLxn4b0oiuXrfPd3gyPAS6IoWoBBQRD6cFUQwduICqwLZ2zn/5AR/hr4Bi7Fm/eEnoER+vvW0Oi8CI3NoKu6GZPZQk9bO2ExUVTfKEMnVxCVlsaVy5cpu3GD/cfvwzA9Q+72In75L//OtkOugMVks9Pc3MyhJx/HajZTcvo1xvoHsJqM6NzcsYoiXV2dyC/IEaUSfvHjn3D0Yy6Vrh1HDnHl1KvoA/xx83DHMDOLVOOi36q4UUagvz8vvfwSUqmc+tv1BAQGUl9VTVrmJgLCQulqqAVAq9VxvWEeuXmNm8PhrGBgQp6LwakERHwSRMZbSzh5oY6G5nYQ4eQrl1AoVVS1TPHw/YdpnlDi6ZVC7AZP5FInxw8VYbXaaGrt4vTZG8xLgpidt1DfMUNAhIm6261MT8+hVTlIiknh8rU6zA4lI0PjNLb2k5Eaxalz1YREZ+Pt7c3lK9cJC/al+HIZZouNtTUTq04NgiCg1d61DuN7hiiK2JyOe3b/NyAIwjdwScG9IbLw1TcNcH+Da4ByAJ8VRbHkfb5MgyAIelx8kreBVaDubjr+v5jT/6UgCE8ADbhkrxZwCQXUvOmaN4sHvFVUIAfwBhbXCQrfev3/hXWxgk8AhIWF/UGbUutDeFA0hflZDAyPYvMOJWnTRrpaWrl1o4zh/gH2HT1Kc283yZvzae1oJ60gj6bqW8yMjdPW1o53UACNdQ30dnexuLCIOjgQmUzGyMQ4M7MzHH7iMUKiIgCoLrlK2o6tFP/2JN39fdy4dNn1RkSRK5cvs/PgAUpOn6HsRilhsTHI9e74RISSlJzC1gOuL5cVkxE3Nx0hcbGcefkUKrmChttNvHT6BpHRMfzjP/0bjRUvY3eIbD3yaXo6atm13aVw9IvfXSQ9M4t9x44zMTePn96TRx9el+MyWlCpNOzaUQBAS9cYJssabjoNC4vLGFbk/O/v/jtN1WfIy4yn/9/Os/fAUQyGOf7jJy+SvSmLgRl3NuQfR+vXh5t3P36xW7lcXUFFywhBBoHWkREGxid56vGHSUiIA+CXz5/k0AOPU36r5Z7O6QEczg9spf5763pzv8dbItog4JogCHGiKN71N5EgCAWiKFYBn18fJH8iCMJlXGpPrXdzjw96ye7HQDSwARfn3r+t2++Z0AW4xC5EUcwURTHT19f3D9pCw8No7hzAZDJz+XoVCRvTWZpfoKm2DrQatjxwAoPBQFB4ODXXS/n4l75Ie30D8WlpWBD59L/8E0a7nZxjh4hMSuToJz6GBFBqNSRnZ5G1rYjGxtt0NTYBYDWZkclkmKxWguJiiN1RRFh+DiMTE+x8/FE8oyJwqFUc/8wnCYmNQaVSERIWzqLJxNS4a76uc9OxtLiIm7s79z32KEarhaCEBCbm5/H398fNzY01o40Jg5OEhHimDauu7cLdgwREJ6FSq1kwGPAMCmDBamF+fp5bt26RX1jI8OQ8q2tGnE4nWk9Pdh4+zo3y25w6X89DDz2Ml5cX4zMmzl6u44tf/Guqqm8RHBzClsOHcaqUJKek4B8QQE9vD0qlkvDwcGaWV3n2a18nLiMDpFI+/y/fpa2zB4Dm5jvEJKVw4NgJ12vey5Gedw/t73F4//uIVhTFQVwsztnv0ueteEM2/tYbBlEUh+7W4eEDHulFUfy9jIkgCD8HLqyf/jHxgLezz+GS6JWtj/b/LbGB408+zWtnXkKm8+Dy6TOgVFD48AlunjrDhs0FOJ1OnvvOv6KUy2mpqaW2rJzamlryD+1nbnISmUbFD770txQe2odhYorWW7WotGqO/eWnqD1bTM6RA3TV1LFw9Tomk5FbpTeIzslE5eFOzfmL2ExmdjzxCFKplCu/egHPAD+QSVHptNRcL8Vht/Pw5z9L2akznHj6STQ6HdnbimiorGJmZprEvBxisjKxrq1RUltNZG8vTW393He/a+pw+PB9lFdV0TY0xwNPP4PRZOK5557jxGf+AkEQuFJ8hbXVVR5/6inCwsM598oLuOnUpGUUYDSa+dWpqxw9dIjOzk4SEhLw9AljaLCXgIAAbpYt8NtTp0gr2oLWzY0XfvtbPvnxj+Pp6cnCwiL/9qMfkn1oP3pvL7paW8nbtQOtm47ROQNOp5O65g4eeeopwJVZ1+l07/cxviveA0eejyAIDW86/9m6StJ7wXuNaO8WNkEQngNCBEH4/lsbRVH87Lvd4AN1+jeUbdZPjwFvZPbPAb8TBOHfcYU9sbjmJwJvIyogiqIoCMINXBnMl/hD0Yz3hKHJCa7caWLoThszYxMUHNhHeFQ4a8vLmNdWufqbk7h5eXHwU8/SXVVDeEEOV0tKSMlIY2Vt1UX6oJCj89QTtSEdrbsbU6OjDPf0Uvnq65iMrqKnhNxsxnv7qXj5FRRuWuLSUlGp1VReKiFn21YqXn0Nu8NBQ2U1mdu34La0SERGOpNDwyhVKkpPvYroFPn+P3yLxz79SVobbnPu5CkSN20gxGzGLySYphs3Ubu7c7XuFj1tA+gjG7h5+zai00np5UsU7dpN6eUSJibG6R8coKLkKhvzcpgzruImlyMIAjKZDI27L3WNt2kbW8AnOoKsg/vRpCTRPG/g3M9+ilqQMDAyRV1dHaurq5jkUtw8PACI2pzLl778JXyDgmi43UBQZCRuDbcRJBI62trxDQ6it62dNZOZL3/1m+w7eoyrJSWYzBYEuKcjPYBTvKvw/t1krRAE4RouVqm34m9xRbT/iCv6/EdcEe3H+NPIYR0EduLKad3VZpy34p45vSAIJ3GtI/oIgjCGSxNvqyAIG3D9oUO46oARRbFdEIRTuFQ/7cBn3pjnCILwhqiAFFcp4RuiAl8GXhIE4VtAEy5mn/cMtd4DeUwQ2oUFCjakk31oP5ODw5z/1fNMj4xz6KnHidrgKq81m0w4nU42bi7AvLxKTKqLcn+4tY3AyDBUWg3L8/P4hgSTsm0LM30DtBdf5tX/+CHR6akgkeDm54OHlyfZRw8yOzHBNo0GKSKp24uoePlVnvn2N5ju6SU2PY2Rjm5C4mNZmZoh+/hRLGYz//XXf8PZM6+Rt38P3sGBpG8pxGgxc+X0GVqqa3js//scxz71CcpefY0ls4ntD54AYGx2DoWfD5FF+fSePEVabg5xmRn0dHYxtTBPa/8Qq047olNkZmaaxvYODj+eQWJOFoIgUFN8mYJD+/ELDqbifDERmzYyoZJRc+cOgkyK0W7HYXewtrZK/9QkW596FMFdh0yhIDg2Gt/gYCw2GzazmS1HDzE9McGPvv5NfFcMhKcm0lhcAvc6kYeI9U+UyBNFcefdXPceItq7fd05QRBeAYJEUfz1e+n7Bu7ZnF4UxYdFUQwURVEuimKIKIq/FEXxcVEUU0VRTBNF8fCbRn1EUfy2KIrRoijGi6J46U32i6Ioxq23fftN9gFRFLNFUYwRRfH+9aTG+8JYWSVJO7awYDJhXjMy0tZB/v59JGdtwmS1Un3mHA67HYvNRv2Va4SmJqH282Z6aJim6zdIKsgjOmMjYz29NFy6SmJhHj6BARimp4nP2EBoUgJuXp7o/f3J3bebnU89xs3fvkz7zUpSigpYWVqmoeQqkWkpePr7smJwUWgN32knOmMDdrsdp9NJ2clX+dQ//xMaNy0Ou53krEwG77QTlZSIw2rjM9/5FkNtHQiCgJuXJ3HZWdRcuoLT6cQ/IozZqSlWFpeQy2RYzBbUGg3xmzaiUqvZsHs7/smJxG3bjMrXm/1PPI5NInL5xd/RUVPH0vw8S/MLVJwvZutD92O32/EK8CM6LYX4tFS23X8f2x88DsDnv/cvtFXXolQp2XzkIHdq6jj/6xdJzs1CplIxMzpGY+lN/uYH32P6TgfzE5PYVXJEUby34T0fzJLdukbjG3hrRPuQIAjK9ej1jYj2vf0drgHx0Pt9fx/5HXnTk5P4evkwPzWDVXTyk698jWOffJbg+FicEgG71UbK7m2U/u5lgmJj6KipY+PuHej9/Kg+dQa1VotPqGtaVvbbU4TExWCzWBhobEEqkdHV1kJaVhZ1JdcwzMySnpuNYWwcTx8f6sor0J4tRoJAdfFlNhUWMjU6hkMq4eoLvyP30D4AHHY713/zEoXHj7Ayv4ADgV9+6ztEJSXg5evLtVOvsmnHNtRaLRKlgva6Bty8vQiMCGN1aYnTP/4ZhYcO4hMcSOXp13HTe1CwdQvNFZXMjk+y7YHjKFRKLvzyeURBYNcTjyKRSLj5yhm2Pfog0yNj9Jw7R2tjE5/69jeRSCQ47HZKX3qFogeOU3HmLDarlSsnT1F0/31IpVLUnh7cLqtgZXUNhUJB061aVCoVKnd3Gssq0LrpmB4dQyGVcu6/fk7C/p2Ionhvw3vxA8ve//N7jWjfB6oFQfgh8DKw9obxjaq7P4aPvNMPDwwyJ3ESE5xDdEEOlqk5Ks8VYxgdx7iywvTEJHo/P6RSGU1l5SwtLtFw/hJSuYw7DQ14eHohSKU4bHaaa2pQqJWMDw2Tvms7E4PDxKemkrZ3Jz5x0VSdPkvqrm2otFr6m1sJjoogfnM+So0ak82KPjAA/7go+upuM9DZhaenJ0gE6spuEpuWRlddA1pvTxLys8DpQKXRoNLpuP7qa8hkctqqa7BYLFx55TS7ThxnceoaaysrNNc34OapRyaT09nUjFqjZm1llerSMqIT46kruYLFYmGgpwcnAtoz51BqNRhmZ7j0/G/w8NCj8/QiOErPzdfPolNrqCuvJH5jOiO9fURvTOfHX/8mEQnxtJZVYFxbZWhwkISNGyg8dpjB9i52PeyOQiIlPCWJ1376C+xWG76REex94jGUly4hqLVIJJJ7PNJ/MAU1oii+I+f8erT67Xdqfw/IX//5zTffHtdc/4/iI+/0Wfl5lDTfRqFUMVhVy66nH8MwMcFszwA5x4/wwte/xZ5nnkCl1VL58mm8AgOJ3ZyHSqvF4XAiVSvxDgzELzqS2ZkZ9AEBxORkMj89g194KNOjozidTvrrGjn6+b+k9dJVNh3ax3RvP7ufeYq26zewA2nbi+itrcPP7sC4ukJ8ZgaROZm4eXvhcDhYWVgiJjsDtU5H6YsnKXrkARrOXUTr7c22+49iN5rJO7yf5fl5etva8YmOwMPLi5pzF9j7xCOERkXjHRSAiIjZaCJj/24Wl5fRarVkHtzH0vw8Gk9PnAIERoTjHxHO8qkllhYWSDpSgNJdhyiTEBwRiV9YCIP9/dhsNgS5DKPJzPLKCin5ufhHujgBJK+dR7JOcjl05w7bHrqfa785SUphPuHRUWj0epRKJTNjE0h1Goa7ulxCGvfQ6eHDo3AjiuK299v3I+/0AMHbN9Nx8RpeWh1qnZaAyAhEUeTSz35Fxq7tdJZX4xkcSHBCHKEpSTScLQaJlORthWjd3Wm8VEJLRRVFDx6nu7qOudExOiurKXzofrQ+3ox39+DupUcqlSJVqzCMj6P1cNFNrcwvIkgkuHt5smnfHip++zJunp5s2L+bujPnyDxyAIVWS+G+3VT85mWUGjWb9u1GKpUiymUMtLay95knGbzTTuuNcqZHx3jym39H7dlibBYLRY88gN1uZ6C+ic5bNWTu3Y1MLuP2lWtotVqCEuPoqLrFxMAQRY88gEQi4fqLJxnp6iZqYxomk4lX//NHPPZ3X0YikdBw4RJ9Tc3sevxh5iYnmR+bwCcyjP1PP07nepa+v62dhIJcWq6X0d/aRmRKMgBZ+/dQfeES3sFBJOfncePlV1lcWGDRZibqyD7Eb//rPS+4cdxd9v5/PARB+Prb2UVR/Obb2d+Mj7zTz05NMVo/gslopH94FO+KagSpFIVayeLiIvNl5SjVaiwNDeQdOsBgUwvzhnkmBgdx2m0oFApkCgWDHV0EVtVit1h47Uc/IyIxHpvVSkhcDL/5h//Nkf/1aQBic7J47u++wZ4nH8O4skpUVgYt18uoK76MaLUyPjKCdHwSqVyOysuTSz9/joJjh7n12nkWFxdZ6OtDo9Nhs1oxGo0MdHZR9errqNRqyi5cYkNhHq3Xb1J7vYxtRw+xtryMm17PwvQ0eh9vNG6ukVSmVrMwM4e7tzeXf/0iSq2O+uLLCBIJCo2G+hvlxBrmESUCczMz1F+8gmi309HYiJevH+Hpqbh7e7M8O0/xL35N4X2Hkes0nHvu19gsVoIT49F4e9HdcJutJ+6jqvgS5qVlasvK2H7oIPUXS9C5uXHulVfZdHAvMw3NiKJ4b51e/NNl7/8HYO1Nv6twLeXdFWflR97pffz8CU2KYvpaOT5+fkRsTEel1dBeUUXhfUcYaWwlKj+L2teL0Qb4IVcq8J6fx2Yxk7p3JyqNhqU5Ax63m4jZnIdSrWZtZQUEqL1wEZkosLK0RGtJKTanHYlcjl9ICKPdvcyOTTDR38/inIGcE0fRerhjdzhx03sQu7WQ5pLrDLR3EpGUSNqeHbQUX8HT34+4rYUolEqG2zuxWixk33eY7vrbHPvsJ5nrHyR5RxFTY2Mo3LS0VlQjsdm5da2U7K1FVJ4+i1QmRSKV0tPRQWBCHOFJiYhOSN61DalMRlNpGZGJCRQ+eJzqM+d49O+/ymBNPRmH9rFqXGNyYJjpyUlsJhOWtTXMVgvjvf2kbN2M1WpnemCQlYV5bEYjIwMD9Ld3kJCbhUqtxuZ0oPP1JT57k2tF4soVpDIZATmbgHu7Tv9hosAWRfHf3nwuCMK/4lodeFd85JlzBInAZN1tInIyid27nb6aelaXlrCbzPhFReCTEEP9hcuo9R4olAq07u4414zs+ouP0XTBtW++/XoZez7+NGPtXbSXVxJfkIdUJidr/14CkxLY/dRjmIxGMvbvxWG2sPfTzyI4nSRuziMwLIwdTzzMXP8gdqsVraceu9Xq2vRjd7DjiYdQajV0VlQTvzmPjQf20HvLtcoz2dWNzsMDp9OJYWSMgNgYVteMrCwsEhQTzXjfAOk7tqLQe5C5ZydeIUFsOrKfDQf24BYUgE9QIEEJsbjrPck+dpDWK6UArM7MIVEpsJrNyGVSdHo9gkLB3Ng4Wq2OQ3/5SeYHh0ndXIB5cYVH/vZLKFRKrGtGBLuDvZ98hpn+Idz8fQmKisQ3JBh3T08qXztHwX1HmB0eAeDW+WKOffLjmOcXWR5wES7fyyo7+FBV2b0VGu6yyu4j7/R2ux213Yl7cAAymYyFuVmai0tI2lEEQERKErMjoxQ8eB991XX0N7YQlJSIRCIhPHMjvbdqcdN74OHjhWlhEfPiMj7hoWQeOUBXRTVjbR2EpSSR/9BxTn77u8xOTtJ66SoSqYQXv/4tJBo1vhHhTPT2UXf+EmGZG7GYLfQ3NOEXEUbMpgwmunuRiqAP8Eel0bA4PUPL9TKic7MxGU3Uvl5M0jYXT37moX2U/PJ5fKMiyLvvMPVnL2A3mck+sBezxcJYuysCnOrpZ98nn6Xy1Gv4RIWj0miQuWmZGRxGpdGwcfcuOsqr0Hp7A5CyvYhzP/oZU5MTtFZUYrLbeeHv/wmrw05/QxNqrZbT3/shiwvzLE7P4BMbReO1G2x/5AEar5diMZtx89QjVyrwCAlianAYwSkSEhtNcHgYS119iKKIWq1++wf1J4Aoijicznc9/hwgCMIdQRBa1492oBv4z7vp+5EP7yuv30D092bg2k2QSBjo6UOtkFP/2gUcDgcO0cHi/DwVL5xkyTBPYGgI3ts2U3fhEg6jmZ7GZrwD/DGtGakvvUHegX10VdcgVyjpbmnFYbWhLC7BaDQSnZyM3teLlN07cDqdDHX3EhAXTW91LWqtltbaOjz9/ehv74C2dlLzcmi5UELH7Ub8wsIQpBIcNjsry8v0dXQik8uZnhhnZX4es8WMRCpFIggM9w3QX9uAQqXkTm0DviEhmIxr2B0OFqdnWF5YxC8yjNnBYbqaW5CpVUwMjRC9IY326zfx8PVGppRTdfEy8ZsyWDXMI1OrkCgVRKSnERAbhdPuoPd2ExsO7HZFP04nc1NTuHl4MD06xtzgMJOjo9ScLcZut/Ojv/4qe59+HKvVSlJuFqUv/I7g+FgAnGYzETkZCIJwj4kxPzzZe1xz+DdgB6bfVHX6R/GRd/rcoi1UdbURst3Fdru8uIiPXk/Kvl0ANF+8wgNf+zKTre34RkfSUVmNX3wsiVsKQBDQuLuxF3XH8AAAIABJREFUOr/AxsP7mBkbxy8iHN+YSCxGI+rGRrz9/Ejdv4vbFy4Tv7WQrptVmFbXGO/pZcujDzDT1UvKnh2MdnTiMzpKZG4mfbebCIqJJnpbIU6nE1Eqwbi8TNz2LQiCwO3iEnRenkQV5DA9No6nry9ZJ44gCAIzw6Osrq3iGx/D8twc+/7iGQYam4jZUoBcpWS8o4uS539LWkEeAcnxRCQnEhQfi2dYCLOjY8zNzTE6NAQqJTpPT0KTEwhJc203tpktrM3Mot20gYZzF4netOH3/4+1p8+ycf8emoovU7C9CN+QIPR+viiUCjKPHGBsYIjVxUW6ahtw2mw01dQikStYmp6lrbaBAJwfiNN/EPX0HxBkwJgoihZBELYCxwVBeEEUxcV36/iRD++VSgVCWAgTta6NTG4aDSajCavZzLJhHrVGg7ufLysLiyxPTBEaF0NgfAwaNzdaLlwmaedW0g7tpf1aGeHJCQw0tbjKuQSBkNhY5G46Jnv7UapUuHl7senoAfoqbzHbO4BveBjG1TXMa0amO3vxCg7GtLJKWGICMo2K+ZFR2q7eIDRzA5H52Qw3NAOup221WHA6HGjdtCTuKKKrtByn08lgbQMFjz6AYWAI49wCPlHhZJ84SnNxCTK5HMPwKPknjhKVtQnD0ChZJ44w1tmFUqshKCEO/7BgAiPDiczMICU3m4XZWWb6B12vq1KwsryM2WhEoVSSvG0LMwNDTA8N4x/uqj3YeGAv3VU1dFfWkLJtC8vTs9SeK+bI5z/D8uQ0Cfk5yLQaDj77Mdy8vUjZu5OEvCyENSOiKN5jYsz/56W1f0qcBhyCIMTgqjuJBH53Nx0/8k4PoI8IZW55mcWhUVRqNan7dzFc30TXtTJituSzurCIIJPRe6eN2C2F9FXcov1GOXEFuUikUixrRjoaGmmvbyBp1zY6S8tpuXiF6PxskEg4+8OfgERgsqcPp8OB0WxGKpUhCAIZR/bT8Np5vMJDSdlZxNUf/5Lw7AwSiwrpqarFabbg5u2Fd3AQk/39dJZXEZiWRPTmPHqqa1F7eeHh7wsqBae+9c+oPN3pq7hFxcVLWCxmbr9+gdrTZ7HZ7fz2779FYGIciZvzmB8aRgZoPdzJOHaQzmtl1J8+R9LuHaQe2E13WQUStYqk7UWMdXbRdasWTYAfyXu2c+H7PyauqAC9rw/WpRWG6hqJynZl36VyGdWXrrC2tkZPbQMrJiNz45OotFqic7LoqqphYWyC8LRkVhYWqD13keDsDLzCQxFF8d4q3Iiudfp3O/5M4FwP5+8D/kMUxc/j4r5/V3zkw/t5wzyG9jGcJjM1L5/Bz88Xk9XKnbIKwuJiaDl7EVGtxG53EJWUyEx3D201da6kj9HETG8/Cr0H/qHBePj5MtXVQ9ftJgSpBLfyKvyT4vH09SUoPZmluXlqz15AsNoZ7etHoVYiOkXaaxvQebhjnDOwurzESM1tJDIpDoeTzsZmVDotdqsVh91J9YWLJOZm4xEUSP2Fi0Rv3MDq7BwShQKb1UJMQS4KlQrD5BRaH2+iN+f9/m8d/Oo3GG5pZ2F4jMH2DhIK8hhpacOytExHXQM6Tz2D1bUIUimVxZeISE5iYXISqVTGjRdfIm/fLnqralmcX6DrWhlqby9aK28RnZFO5/WbmNeMmKwWJFKBzCMHkCmVDHV14RSgs7oW0W6j9PRrFO7eTfuNchYMBsbHxhBVChwmMxKJ5AORqv6QwCYIwsPAE/yf4pu7CpM+8iO9l7cX3vlZ+G7OwTsoEK3ek5jNeYTHxxGxIZ3UI/tJ270DrVaNPioMfUgQwckJ+IeGELMln7htm5nu6WPj0YPIZTL8k+IJTYgjODqS+O1bUGrUpO4oYrzpDsGx0eQeP4IIBMdFE7eziNDcTQTFRBGStRGlrzex2Vl4hAYRs60QmUJOfF4WfimJxO7ahkN0EJOaQsqeHeiDApEpFHh4e5G4fxdra2tkHj/CbN8gq4tLeIWHsbqwhHFxCYCeqlriC/MJz84gZmcRczNz9DW3ovb3ReHjSfrObQRFRRKxJZ+AjWkkZmcSFBNF+tGDRG8rROepJ/nAHrTubuSeOIJPYhy+CTEsGAzI1Sritm8h7dBeJCLs/uSzzA4MAeAT4I9fYCBxBTkszs6RmJtD1JY8YosKUKhVhCXEE7Y5l/lFV2WhRHLvPpIioksT4F2OPxM8DeQB3xZFcXC9au83d9PxI+/0AMb5eQw3q0l94AgKlcIlJ5UYj2FsHNPSMpM9/ejDQ4nO2sR0Rzcyp0jBEw/ReLaY268XE1OQg1KrwWI00nb5GmmH9uKfGM94aztt128SkprE2rrzdd6sIiY/i9S9O11TiOs32fGJJ5nrH2RpeIyMw/sYam7FYbOh9fAgdfcOBqpqqTv5KikH9+ATH8vy1AyLY+MUPfUY7lHhNJ05j1wQCEtJYra3n87ScoLTk0k9uJu+my7lXPPcPKm7t7MwNEJ3eTW7P/EUbm46JBIJhr4hwnI2YXGKGBeXaHm9mA33HWLZMI9lbY324iuEJMbTeLaYuB1FRGZsYKq9i+GmFo588a+QKOR0lt5kZmgY3/BQfIKDsK6u0Xj2InFbN2OyWKg9c46Y/Bw27tvFTO8A9a9fIHlHEVIB+qprcYuPQRTFezvSr1fZfRiW7ERR7BBF8bOiKJ5cPx8URfE7b7QLgnD6nfp+0Lz3XoIgXF3nqr8qCILnul0QBOH769z2rYIgZLypz5Pr1/cKgvDkm+yb1tcq+9b7vq9Py/DAIJaOPhKOHUAqk2GSCDS9XkxwRhopB/fQff0mky1tBCTGA2C0WJAIEhYnp0CQ0l5bz2BdI63nLlF7rRSdhwdjre34R0cy1tGFHAkKtRp9RCjDrW04LRa8wkLRuLszMzCEZ3AgcqWS2YFhPCNc/Apph/ZT+tPncMik9JZW0FJRic1qY35ohPANqcz19LE2PYt7UADjrR1IJBKGe/u4c+4SM7MzmJZXkCsUSCQS3MNC6L5ZhT40GIlEgm3NiG1lFY2PN+6BAZz/1+/jsDtoPXcR69oar3zzu2j8fDCMjBGRl0XZL19E7evDaHcP0yOjGKamsBrN2CUSVqfnMK6uYRifYGp4lOL/+jl2hwOb1crEwAD+MZEMN7ZgmJhisKOTqY5uFkYnuH21FLPdTntZFU6bk7YbFZgMi/d8lHUiYrXb3/X4kOAdN+rcyzn988AP+UPFja8A10VR/M66cMVXcDHg7MNFKBCLi+32x0COIAheuBh3MnGtuNwWBOHcOt/Yj3Ex3NbgUvjYC1ziPcIwZ2DRuMxEZS0OqxWH0cRIZxfycxeRS2UYJiexGE3oK24hAhq1mtaqW+hDg5FqNYQlxJN6eB+G0VFiVpbxjo9GqlLRcLYYCdDX0YHW0wMEgRtnz5O5bStdV28gkcnpa+/EMDODbc1IZ2MzHr4+dF6+hs1iYWxwCK+oCOL37MBqNCFRKpDqdDSfuUBnbR1eQYHIK7XEFuWj0umQXy0jZtdWeqtqaL1yHd8blSCKOB12qosvU3BgL/2lFdy6fJW0wjzarpUiSKUERkeReHA3giCwNDvHzOgoQRtSWZyeYWZ0jKmREXRBgej9A3DXeyCXKxi908b04BBrK8sEpqeQfmAvvRXVBNls+CbEUH+umN47Hch1WpJ2bmV+bBy9zZvorQXMjY0zNz1N2p7thKUkIZFKUd64iSYk6J6G9oBrpHf8eYzkfwK8Y/LiXjLnlAPzbzEfwcVRz/rPo2+yvyC6UIOL9DIQ2ANcFUVxft3RrwJ719vcRVG8JYqiiOuL5SjvAxuzM5HJ5fjmZBC8rRBdWDAhMdFkHD9C4sE9uHt5EZGYQHhhLgpvL8wmM4kFeeh8vVEIAjFbcpnq6Gbsdis5Dx7H0DuIZ4A/mceP4BBFYlKSidySz/LCEpv27cEzNJjYnVuJ3lpA+pYCfEKDCc3NJDAyHI+IMOJ2b8e4auSBf/w77Curroy2Rs3a4iLeYSH4pya61sclAg6LBYfNNTLZLBaMy8vYllbIefA+1N6errny1DQFjz6AOtAfmZ83mQd34xUWSur+PcgRiCnKZ7rbJTQxeLOKiMwNOKxWQhLisC0uc/irX0AlSAgICcI3JhLRYkUfFkxIXAzB0TH4RIThdDrA7sA/IRar0YRnSDDBCbEk79yGKIq4B/gTv6OIwZp6xlrbeeDrX2G6q4+1BdfoLgoCw1V19zyJJ/Lh2ZH338EHnb33f4MiSxTFSUEQ/Nbtwfzf/PbB72Ifexv72+KP8d4D+GzNZ7z8FmHbN+OcmsMzIpTl6Rl6SytIP36I9pLrNJ+/hHdAAFFb8jGvrVH98hk8/P2ICw6m5dVz+ERFIAgCq4uLiKJI59UbRBXkMlLbQG9VDUGJsfjGRtNWch2NtxfTwyP4JcXhHhhA27lLhKckM3mnnZHGFlL270KuVBJRkEPLuWJ8oqNRBfrSWuyqTMu4/yj918uJ3FZI97UypE6YnpjEeOkq6eubdG6//BrDTS2kHt2PUqul53IpdquFpMP7aDl9gZnhYRL37kCp0TBwvZxFvQde4aEEZaQxUl2H02pH7++L2t0dz7hI+itrSUqKp63kOk6Hgx2ffhabxcJITQMLUzNsOHoAiUzKUHUtxvlFCp94mOGqWpbmDGQcdU2dukbHUKvUaNzdyHrgKPWnXidsYyr9jS1E7tmOeK8z6x8cc87/BLzjN+j/lCW7e857D/wMIDMz8w+um5ueYXKkH7lGTffFa8RlpINUwrl/+Q82bNnM4I1KpgeHWZ2fx5qSjGF+HrXeA6VGjdVoYqCqltaaGtKkEhbm5vBLTaTh9fMERESARGCwuxexo4uQxDjmZ+YIzUinp7QcAHd/PwYraxjq7ELj5sbK4iIadzc8mu4gOp2IokhzWSVZMgVKjZqu6ho27d6BcXGJteVlBEEgOCOd/vJbjHR1ExARRvNrF3A4nYz19AEispJS5HIFLeWVxG1Mp+9KGaLTyXBPD/oAf/xTElleXGKlooa04wcRBAH7qomR+gZCszIYKKtkbnwS09oqxtUVJBoVSyNj9N2swmE201xeRVR6Kivz8+i8POmtbyT7gWPIZDKMi0u4ubtjMZqYbO/EYjQxPjiMVCHH6XCgVKm49NPnEDVKTIOjb31sf3KIfKSc/svv1PBBO/30GzTY6yH6zLr9nVhCx3Ax6r7ZXrZuD3mb698zfPz9CAz0ZKGjm4GqWhROEZW/D3ofX+L2bEOmUGAymXAGBZJ+/DCCIDDa2kb85nwm2joJz8/GvLSEQqcjcEMqE8136LpVj9PmQLu2QnBUBG7eXkRuzmfFYGB+bIL5OQOr8wt4xceij4kkWRBw2mwszBmQS6WEb8n7fai7srSI2scLGyKJ27ag8fVhoK6RqcER5n/6PJFZGSQe2oNKpUIQBGL3bsdsNKL39cUpFfAJC8U9JAgkElTubkQU5tD66nnSDu4Fm42eyhqWDQYWDfNorpbhdIq0VFTh6eeLJiSIiKIClk6fJzQmhtDkRIwj4yRu28za2CRRRQUsLy5hXTMy2T/AatUMc9PTtFy8SnBMFP132pEplazaLMRuzmdxcpoIvZ6YXS7Sl/nxSdIFcKoU+G1Kv/fh/XrBzb2GIAj345JZSwSyRVFseFPb28paCYKwF1fBjBT4xZsz8W+59x3++ACXtv7zyjtd80Ev2Z3DxVEPf8hVfw54Yj2LnwssrU8DSoDdgiB4rmf6dwMl620rgiDkrmftn+B98t4DSOQynIYFEvftIjwnA/OsgS2f+hgjt1zPSuOmIzQ3k4kW10LE0tAIvvExRG0toK+sEq2/H6sLi0jlMlZmZsl76DiB8bEEJyXiFRLM2tIy4KqSs5ktJORlk5iZQXByAkNVtYQX5GBeXUOpVBKQmf771+m8dpPIwlzMC4usTUyTuKOIyfYuYrcW4Bsegj7AH31kGAgCMq0aK64lt+5L1wnJyyKyIJfh2tt0XLpKSEEW1uVl+qtqCcneSHBqEsalZYKSE9B6exGSEIffpnQid28lOTebgKhI9D4+2O123Lw9MZrNLExNowvwxys4iLXlVZanZtD7+hKzbTNyh0hEZgYFD57AOzQE9+BAAuNiCIgKJzYvh86LV4nfsx2FjxeLk1Mszcwy0dRK0r6dONdMTNU33fPsvfjBZe/bcO2UK3+zUXh/Qq1vxUFcm3Eurx+Prh8XgVfv5s3dyyW7k7ikd+IFQRgTBOEZ4DvALkEQeoFd6+fgesMDuGR+fg58GkAUxXlcYgH168c3120AnwJ+sd6nn/eRuQdwOp1MXb1JzKHdhGZtZK6zD51Oh9pNx/LsHJPdvWiDA/EMDmRxZIy2i1eIKMwFQK5UUnfhElNDw2iCAqj41YsEpyYRlp7KVHcvHRevEZK1kfhdWxlraGL4dhMKuZzA9BTMZjN3zl0kfvd2BEFAVCpwOhz4hIYw2zeA0+lEsFrR+XiztrqKSqvB6XSiCQvi9Ne+RVD2JpIO76W3pJTu0gq8k+KJ27WV/tIK9EGBrMwZaD1/CSfQ39TKbGsnA929jLd3IigVWNbWiCoqYLS+CblESsqR/fRfv4koiii1GuIP7GJmYIjKnz5PYOZGPMJDaLtQQmC6i/oqoiCL4u/9gNH+fia7exntH6DqNy8jqFXooyPob2xhfmIKiZuOM9/+V6x2O6uGeaIyMxiuaWCoqo7kA7uxmkwsGAxMrO/vv6f4gNbpRVHsFEWx+22a3knWKpt1oVZRFK24BFyOvMO9h0VRHAYKRFH8kiiKd9aPr+BKfL8r7qVq7cPv0LTjba4Vgc+8w31+BfzqbewNQMp/5z0CVF4vRaZVMVleg81mpb++kcgN6XgMjxKSl0XNCy+x4eh+ms5fZqZvAIvRhEIqxWQ04VDICIgKJzghDqXeg7qRUXpq6pnq6kEmk9FeW4ebhzt2h53OmnrcvLxIyM1iqKqWnoYmNB7uOJ0idrsdiVLB7NAwkQtLRG4toPrXv/v/2Xvz4Djz+8zv8/Z9d6Mv3Pd9ECBxkCAIEOA9M5pDMyPLkiV75JVXa2+ySSUVZ5PNVly1W4l3k002u+uUbK9sy/dYluYkh+TwBEgCJEDiIG6gcaMbR19A3/ebPxqy5exInJHFkTzUU/UrsvvtF/0Cb3/79z2fh8LmRsbeuUjEv8/G4hKCRo0xPxeNXo9regbXwzFUWg1Tt+8gS6WQyqRMD92nobcHdGoqz/SSjMfxbG9jbqyhTCVnY2iErUUH8UCQVCTK/raHaHAflUGPOi+PmfevoLVZWB8cJra3j3NpmYWrN9HqdXhcWziu3UamVCLRqjHn5lJ7sgtDXi4b07MH5BgiYjJJbn0Ni6NjdPzS60R23ai0GtybLhYHh5m9d5/qtsPc/85bKLRaAl4v8WTqU8jef+yY/icha/VR+KRCrT8KWkEQukVRvAsgCEIX8LFoh35WEnk/NZw4fZrLDwYpOtODIAhIMiIShCz99ewCiXiMfecWdadPIhEEUuEI5ef6ANianSevsoLAyhr5jXV0nDtDJp2h5sIpNqdm6a4oxTuzwKFXP0c0FMZgyaGkK6vJvr/rJpVIUH26G7XBwPKDh+w5XXjX1kkGgqxMzaK1mGl44TzTV29SnGcjp6QIfUEepY31mOw28lqa2F1cwrS6Ru7xdlR6HRlBILIfwN6QbSZyXLvN0V/9Cq6BIVJqJebyUuylJRgK80klEjiu3iKVziX/RAf7Wzs8vHiJ4to6jnzpVfYufsjn/uVvEnAsEwqEyC0rpbCzHZVBz8bEJC0vPUd4cwtLWQnxXQ9tX3gFISNir6lk4q1LnPqNr7O/uoHOaERlt6JVa6h4/SUMej2JeIyGl54DQCqKRKKxp36vRVEknf5YFYK/l6yVKIo/LNT8YQnoj/K4n3ShXwf+UBAE48HjPbLSWU/EM9+GK5FI0Le34BwYYmtskuLWFkwVZaikMlQSCS//r/8cSQZCWzsoECg+1s7WWFYg1De/hK2mkngmQzQQQKpUkCBD2L+Hb3GJ/IZaal86z/h330Vr0KPKy2X78QwAGp2W5l94hfmrt4gGAsQ9Pgw2G3mNdfidW3z+X/+LLFuucxtpJkPD8+dYHRwmGY+js1qIbmVzoP7ZRY5/4w3c45OsjYxjrKnEfqSZ7YMxXKVchkKtQtSo8S2tUXvuFK6RrILu/PtXKTvXS/mZHhY/vM32owle/K3/idziAtbv3aespxONyUDE7UOSTNLy5deYv3oTURQJr7uwVJaTiEZ5/NZFap4/S35tNcH1TSJ7+xjsFixFhQS3tpFKpRS3trC9tEzY60OqkGMoK8U9s4B/Zxe53YrEoHv6JTt+cu69KIpnRVFs+oj1o3JLPyph/YnkrkRRfCSKYgvQDLSIonj44whdwM93ejKZDNH9AK6ZOcRgGO3pXjLpNNf//K9o7u5ivX8QlVKRnUdXKql67jRTj8bRlxWjz83KXtddOM3yjX4UBgN1506xfO02Gp0eQRDYnJxGlEiYG52gJBpFqlCituSAKksWYSgv4e1/9X/SdLILjc3C1X/3O9SdP8Xu8irKPDuPP7wB6QyR/QD1rzzP9NuXMFWUEstkmL54lZLOdqQyGbG9AIIQxNiRJbZwLC4T3vWgVGfZZct7Opm7NUAiFsXYWMv9P/hTdHl57I5MkIyEmb5zjyOnetm9P8r03SHkajWJUASZTIpj9DFak5FwOExaArf+329hLStm9e4Dxu/co7S+jp2pOez1NQQCAQLXbtH06gGxi05L7GDuoOHFC0x85x1MBfnkN9Xx+Hvvk0ylqHr5AhZ46kafEcWfdpvtJxJq/VE/SBAEJfA6UAbIvh8a/ZwC+2PA7/UilQiY8nLRFkjJP9FByOejUyEn5vNT1JsVEgnFYrgXl5l89wNUShWXfvvfc/JrX2F3YQmAqYFBanpPsDk6gePxFAgSRImAqaaC4JqT2rbDlJ0+ievRBJf+n29SdaSZTCpNWi7FlJ9Lxeketh3LJFNJlDoNEpkMhU6LqawE3/IKyw8ekgwEWZ+exezzk1dSxNzEFHqDAf/CEnt+Pz7nFkmZFJlWg9xi5PH33ieFSDQUQqaQk0omWeofxGizsjG3SM+pHiylxcy9+wGNF86QU12JPj8Xv8eNWq2h8oWsRqOYFhEkAjUvnicaDHL7P/1nbPIKCrraqdzewZifi7o4j8krNwht7RAOR9D3DyIIAgpBwDG/iPqGDolMyq7ThWttHTIZtCYjj24NoLWaQSp9+m248KlM0QmC8CrwnwAbcEkQhHFRFC/8KFmrHyHU+sPwLrBPVrn2E+k4PvNGb7HZkEX92ArziSeThHbcrA8OU/fK82zPzOOZW8BcU4VUFKl94SyZYAiZwYB24jGCWkVGEAh7vKiMelTmHKx1Ndgdq2QyafI729idc1DQ0oh7YQmpXIYix0jL6V40lhzyW5uZeecSLV94mb0NF8GlNdpeewkhlcZSVZFlqMmIFFdXUXKqm9n3LnPy13+VwNIqwUCA/OpKrIebUOp17G1tIS8poqC1OdtgE4+zlk5iLy6m4vkzTH/3Pc79i/+enXsj5Le10BIKE17ZQKqQYSgsILe1GVf/IPr8XHR6PYqCPLyzC6jy7Gjz7Eh0WjyzC1jra2g40UkkECTi20Oj05LYD6Azm2l6+QJz717GkMmQ130UqUzGyuAwRdWVlJzuZntukbqTXXjXN8ntOMLG6AStnztPOpVCUfhR4fFPFp9WnV4UxbeBt3/IsY+UtRJF8QOyVayPiyJRFJ/7ca7vmY/pAcLj09jaWig+3sHyrbuYS4uRSCQUNNWzNbPA1HuXyT/Wirm0CM/iMluj47S89hJCKoVCoSC2s8vJ/+afIEkkcVzvp/xMD42/8DJTb18k5HRhrqogEYvjXdsgurVN9YVTxDx+Zt67TMW5PizFhcS8PtQmA/a6anam58lkMjgu3aD8TA9kRGbe/YCizg60uTYy8QRKqYym11/CeW+YhUvXKOvtpukXX2Xz9iAakxGd1UJpYwOafDtDv/dtSk/1IJXLCYbDrA0/wlRdQSwRY/32EPYjhwAIBYKkk0mkKhV5DbXsLi7juHKT3NZD5NZXszO7wObEFNqSQmpePM/yjX6kMimxTIp4OMLClRuU9HRSca4X11BWOj29F0Ct1RKLRNhfXiX3yCFqnj/D5tAImb0A5Z0dyKQy5t67/A8qpv8ZwKAgCId+nBOf+Z3+/p07ROMRnNcHkMrlzI+OodTrSA7cBzGDRqdh+sFDlIpsHd0xOY3eZCAjCIiJJGqDjqrnzhzE7zNYqsrwzs6TjsTYXt/MurhyBZuLDpYnJik/3Mz0e5dZGpvI8tJ1tqHUaVl68IiON34RgJLeLi7/b/8X1rISZt67wsKDhxQ21LLy4CGG4kKcC4uo7TZC715ma34BqUJJPBbHWFlG2YU+Vj68ndWZ06iRuaOkUylcg8Oo9XosNZVMvXOJQy9eIODcZmdtHc2Vm4haDcV9XUz89buUdXXgXlohfTAim3PzLolEgkg0yuJfv0t5+2F2J2dBImVqaJjG506xcuseOrMJjTkHgODeHstDI1jqa9hfXGbh4lXqXv0cAJH9ANND99EZjchv3SUSDBKLfErZ+384Rv0kdANfEwRhhax7L5Ctfjc/6cRn3ugLi4sQ9SpMtVW45x30fO2r7DmWKejJlknn3rtCYXUlped6sxTTB6vgZCcXf+u36XjxOTYHR0glkzhXVsipqyKvs52wz0+9VkPU46P0fC/BUBCVWk3V89k2BVEUiQXDhLZ22J914PN42Hk0QSKeIBqPk8mI1F44jd5mJR6OkFdbhaWpnpDHi3/XjcpkpO4LL5N6N4Mklab2pfPsO7fwTs7hcizhd3vp/idfQ5drI3M1Q+mFbOvr5qMJgoEQGYUco82Gua4KU0E+glK7J4joAAAgAElEQVSBZ3SS1fFJLHYbmuJCJHI5+VWVFPQeRyKTEQ+H2XYsU33uFHKVkvn3r1Lb0YbGlMPIm+/Qeu4Uyx/ehoyITCpl8so1GjqP8fjWALXHOnDeHQaFDJleh7WggLyGWvLbDzP9vffJr6l+6nV6+EyN1j7/4574zLv3xWXluOcdpFMp0k4XltpKDFXluCdniewH0NuslJ7qZndyhmQsjtyoJxSJANDS14MglVLQ1UHY66Px3CmsZaVIJBI2Bx5Q0NmGuqQQ/0GtWm634F9aBbIlu9rPP0d4a4e0WsnRL71GeD9I2fk+1GYTPb/+NbYGs/0h1rISYtE4ke1dFFoNzedPk9/cyPrte8gkEopPdrEzMo4u10Zge4fas32UN9azMzJGOpnMfomk06RiCSLrTiraDxMPhTFWlVHU0Yrn8Qxaq4WCnk4qWg6hMJkwlZeg1moPfvesQMbyh/10fuMNvNPzZFIpNHo9YkYEpZzGs33I9HqKz/RQfO4kglZNfmUFhSeO0tzXTTqVJLerncLjHSjNJvKb6smEwuw6lnFv7aCvLvvUsvefBRKNH+jMi5Kt6X9/PRHPvNEDWE928uhbf4qlsQ5RFLHVVuFZWcNx+Qb5x1ox2KzEdjw4rvdja2mk8kwPO6OTKI1GAjtutqfnsNZUUt7dyd7MPJtjj8k73JCdgmtpZPHmHdRF+RS1tuAanyTk8yMzGVFqNMQyGRb775IKR8hprGHyzbdJ+ffRWi2YGmuYvXgVhc1MyYkOtiamWfjgOraWRlJihl3nFosPx3E9eMj0rQFu/h//kZKzvZiqK1DptJSe7WPp/Q9R51rZW9tg6dKHVL50nsLj7bgejmOqKgdAW17C3uIyqwP3KDvbi9/pYva9K9iPHUFnziHu8eFdXcdcUYreaiHq9rB45Qa5x46QTMbZGR6n8sxJwnt7RHx7JGIxhESKli+/zs7IOAq9npoXLzD39iVSySTbw2PYWxpJRKI8fPMtSvq6UOU//UTeZ4kuSxCElw/a2VeAfmCVj9mK/sy7936vh/COC9/2FusPx9kcncjOxfv8hP375NweRGE1E00lkYoi8gOttY3xSSrPniTj8TH+9kUaT59ka2Kane1t4nPzGAvzcc8soFQq2XQsI5FK2FtcJpZO8eG/+x3KDzeT8PnJr6tGp1CQ19aCd32TYCjIrtOF5AMZcrmC6Vt3aEqniW1uo9ZpWRgdwzw2hcpupvHzzxP8vW9T8fxZMqk0qWQS78QUiUAIp2MZ27E2NJWlOK7dZm/XTftzZ9keuE88HsftdOK8eQ+pWom6MA/P+DRylZKd+UUkgGdtA/XtQRJihmQ0Rnj0MdUvXcg2Hu3tkwyFKI4niISjWArzEQSBqvOnWHzvCul0muqXLpBOJFmamkaQSPB5PGQkAtf+7X9ApdUiuXyD6YF75JQWk1xzkVhzPvV7/X0Sjc8I/jXQCVwXRfGIIAingB/W+v538MwbfY7FSsK7TefX3yDt2qa07wQAC+9eJq+4iLyTnQR23OzcyopJyD6UAyIelwuh/x7NX/w8JqOB/M52tucW2V5YRK5W09L1ebQ2Czsz83T/8hfZmZ6j6ORxpEoFC0PDqFRKLEeaUJtMeKbns/x1+wFqOo/iWVql7PwpRCCVSiNXq8g/2cnCpWu0vPw8apWanNISlm4MYMzNBUBrMpEQRQyV5WjtVjxb20y/fZHKs70UHGrAshcgp6URVY6JRCyG3+tHU1qIobyEndl51uYXCHq8dH7ty1g72+EylF44RTIWY/gP/oxULE7ivcsoTCZS4TBqvZ61h2OsT04R9vuzpPKiyNr8AoIIfHCdtASkcjm5JcWUnu4mFgpDKo2lpIi8o63IZDKi0Rj2k51E/PufSkz/D4jt9klIiqLoFQRBIgiCRBTFW4Ig/NuPc+Izb/QAslAUfWE+61OzpJNJdhccmOtr2J6ZR8xk8M0u0HCmj+j2LgV9XWzcf0TXG79EYHubyOZWlizj1l2UGg0lzYeoe+kCK9f6sddWEV7dIPeFsxirypl/6xK6smJqT3Zhb2vGOTyGUqkklkmzNTmDGI1hOdqKYNThnXfgX1mnpLeLtZt3SMUTqFUqClqaWHz3MqbqcmTpDFKVktV7D7DVVaG1Wdi4dptgrp2KrqN4Zhezcf7sImWvvcjye1eoePEc3uVVSk4cxTMxjb6sGENhPpVtrUiB8JabhGsX0ajHs7iEd3KWI1/5AjuDDyl/4Syr1weoff4s+7MLGFsa0Gm0BLd3sLY147j4Ie1f+SI7E9MUth9Gbclh4/odUlKBiMePc3CYhtdeZOniNbxrG2gK85GnkoRW19mdnvt4AenfA6L4mTL6PUEQdGTHd/9cEIRdsg0/T8QzH9OvLy+hrywDIK+3i63hUcIrG+RUlWM7VM/It/8CS3kpOXVVxKNRAls7yDIZDGXFFB5tY2V8kntvv4/CYiK34zAalRpEkbQA9/70TbbX1tm4cQdX/xAqvZbxdz+gqq+b4MoGVedPYe84zNbULA+/+y4SrYZkLIa5pJjwhguNQY9Sq6Hi3Cke/Oc/xtKcHbEu6Olk7M+/i6GmAkEuI+MPoLVl1WVDySQzV66TSKfRNVTz4Jt/hKW9BYCS506zfvU2wQ0XGosZa2cb7keP2bx5j7wTHYQCQUp6j5PXe5zt8SkGv/0XaKxWor49VGXFzL5/FVNRARq7lVgkinPgPuaWBuKZNJd+699gqq1EbbNQfq6X9YEh0skkMp2GgvbD3PgP30RrNbM9PomqtICFyzcw11WRd6gB/+wiCu2n0Xv/meK9f4VsEu+/IztXv8Tfil78SDzzO/3mxgY+t4LY5hbpVJK5e/cpqq3GefseGakU5/witoICQptbuDY2WJ+dp/pYOxvX7xAOh0jFYtQdbUMQwdk/iHPRgUyfrb8rJFLikSi2423IVSo2HjzCXlbC7sPHbM7NE/H6Uei1HPnlX2T2vcskk0kWb/SjQMLM3UFaz5/BdW8YBAHPpovd6TkUSiWIIq4FB/aiQoIeL/vxBJo7D4gEg+xtOik/3IzUoGN/eQ3f1jY7D8ZQKRUkYnH8e3vsbTqR6/UkPF5WRscpbqjD+2iCZDrJ2B+/SW5ZGeVnehFv9GNuPYR/bYOAY5nV0ccYdTriHh9rM1n9O+3oJBVn+4j69tjb3MLvWEWn1yNoVAz8x9+nqL6WwMAQ5UcOoTDoMdRU4JqYxr2xgfPBIwQEdjc2SYoZJJ8Cc07qH0h2/kkQRTH8Aw//+Ie+8CPwzBv98d5erow8wHKig4jPR4NUSioaI7enE8/yGl2/+hW8k3MUnjrBunMTmUxK7vHs1KVvdR1zIEQgsI8mz46ktBhhdp784x0sXx+goL0FhU7L5o27lF7oI7W3j7m2CmN5KZvzi2hsZmxtLXg3Nqk63cO+Y4WSY+2ozTnEQhEUVjOWxjrW7j6g+ze+Tmh5ldxjrezOLWIqyMd87Aiu9XXkSiXWrnYEQUB28x7RcBi9zYZnZIKz/8v/wPbAEPaeLPFH8sPbeNc3MJYWo2xuQK03kInHyWlrIfQgiUwiIa/nGI4PrnP4V34R/+gk9uPtWbGMV15AIopYjxyiyOcnmUySU1+NQqMmv7GORCCI7WgrapuFWDDI4tAwhqoyUpkMJomU/eVVtMWFkErT+qXXkCVS2I4cIurbI5lK8vDnMf0TIQhCkI8uzX2/OcfwpJ/xU3HvBUFYPRCqGP8+WcFPUgjjE6PATmBxGd/wOEWnu7EePYxz4D57K6voC/OpfOk8jveuopHJya2tIbiRJeLdm57H0txAadcx9mcXWesfRJdvJx6OoJBIUJmMSGQyVMX5jPzBn2E/2krx0VYe/cV3af6l15GYjKxd6yfs8SLVaKh47gwb/UPszi9hbaolcjA+K0Sj6AvziEVjxAJBwstrVL9wmv3VdeyFhVS/dIG1q7fYGB5FX1uBobaSnYkp9Lk25ColmvIS5t+9wsa12+S2H6ayLyvL7bx5F9uxI0itOYTWNpGFokgNeqJeP1q1CoVaTdjnJ5NKI4vGyD3UQMjrY3tqBl1JEZUvP8fa9QHigSCiIFBy5iSuwZEsb//Ne/T95j/D+3gW//Q8pvISys724rxzH8IR7PW1+Nc3WRkYRNdQjajTknnK7r0oZmv1T1o/yxBFUS+KouEjlv7jGDz8dGP6UwczwN8nK/i+EEY1cOPgMfxdIYxvkBW54AeEMI6RpRv6re9/UXwS+D1ewivrPPjzv0KpUeEeHiO64yYpk7Azs4BcqcqyupYV4XgwQjweZ+vhYxyXrlNwItu1J5FIiOzto1EqKevtZvTbf0lOSyPuqVl27g4T2XDh3dpmbWAIx/sfEo1G8D2cILq6QTwSYew777DWP8jipWvIlEqG/ujPEDMZYmKG2YtXMR/JdlaWnj3J7Pfex1BdQU5hIYvX+8lprkcilyPK5cx+eBPv41mCC8uMv/M+8b0Au3eHSXh9LD0aRSKRkIpGyGtuYnf0cVawQy4nt6WJrdEJpAYdxd2d7E3NIlNnS5P5J4/jvPcAhdlMKp5AY7Ew/t33yQRDuIdH0RbkMfR7f8T6+CQzb18iiciN3/73pDJp/OMzaMwmNucW8Nx/hHvwIbFIhM2FRXbvjaDR61m4M8Tu6GOiXl/WKp8qPlMx/Y+NnyX3/hX+lvn2j8my3v5zfkAIA7gvCML3hTD6OBDCABAE4RpZssG//CRvqtPrsZ/oRKlUoTSbsRxuIrIfwD86gdvpZObiVbQFuYiJJPbyckxlJQTEdZaHH6LRqJHKpCCVEgkGca2sIooZ9rxeNoaGKehsR9tQy/K121Q0H6Lic+dYfOcy5Z1H0dVXo7GaAYgGQuQdbsRaW8325Ax5VeUkRUgGg6xNzWHUGQhIJSCVsOlYRqbVEF7dILwXYHd4nLgAMpmUktYWjLWV6IoL8W5sYqyvQZNvZ/XGAHXPn0VdkMeecxvJ7CKOoWEauo+T8O8hyOXsrK7j33GTCUdYGHmINieHaCCIRCJl6vYdGnu68Lu2kOcYMRXkYWxrRiKVAuBf20Sfa6Og+xgRnx/v+iYlp7pR6vXsTs9jLSrEfLSVVCLBvsdLxZHDWI61sn7rDnnlpRSd6yMRiSD837/z9/sEfQw8C0b9JPy0dnoR+FAQhEcHQhTw/xPCAH5cIYz/AoIgfEMQhIeCIDx0u91/55hcqcA7OExe73GCTheiKKJQqxACIbp/49ew11SiMehRK5Q0f+ULJHY9JAIhChsb0Lc0kNPZTk7HEWRyBRVth4nsB2n7yhcx2W1oD4ZPVDI5UpmU1Wu3KejppLTnOHvTWd5E5/AoJSePk/btEwuGSLp9NP7iawjxGBJRQtOLF5AadFg728jotHT+ypcwWq0YDjehs1qwtB+mtOc4MkFCxblTuCemETMZipsb2X00gZjJoJLKKOpoJbbmJK+1GaxmSo+1gUTA2N6CvLSI+tM9lDbVk9PZRllzM9bSEoxV5eSf7qairQV1QR5lF04hCUUoP3OS/ZV1ADZuDFDc14U0k0EQBHbujdD69a+yP+cAILbpQqnLZubXrtyk+FwvpNPEgiGUKjXWliaCa5ts9Q/9OJ+jT4TvJ/KetD7r+GkZ/QlRFFvJuu7/lSAIJ3/Ea//egheiKP6+KIrtoii222y2v3MsEY+jNZuQymRoD9XjfTzNyqVrlL1wFmNhPv55BzGXG8uRQ8gUCjLhKGqdlvJzffhGJwFYvzFAQfcxZm7eJb+3C1NJEbFkkuC6k/X7DzE11rA+t0A0GkNlylKahfcDpJMp0vtBtAV5ZOIJNq4PkN/TiUwuZ+HmXXKPHSH3UAOxWIzg0hrxDRfGijLCoRCb/YMceeNL+CemWL92C9tBcjG3+xiekXEyEgn27qNMv/k26vLiv3lPMZMhs+uh6rkz5PZ0svLBdab/6nuoy0uIBEJsfnibvL4uis6cxL+1zaNv/Rn29sPEtnbYuDGA9VgrOSXFpL1+dh/PYCotQWXOIRQMsTk0grX1EFK5nHQ4ivPhOMbGGuQ2M7PffY/Ck8ezf8NUiq3+QWxHj2CrqSLkWEZlzvnU6vQ/d+9/ChBF0XXw764gCG+Tjcl/UkIYnwh3b94matDgHriPIJEwdfM2h/p68I2Mg0TC/MgjGru72L37AIlczviNWxR3tGKJxUhkMqwPDhPy+kkPPSSRSuKfmSeUgXQkwtSjKwhkYD+ISqcl4vWxMzhCKhIlnkxw/5t/QMWpk+w+HOfx7X5Kmw6xeX0Ar9tNNBpl+c4Qeq0OpVLJ6K27GHNtqIfHUBv0zN0ZQqaQ45yeQ65WI719j0w6QwYR1+wCCp2GkkgM59wcBquZ2NYuhvpqpt98G1tjLWuXr6PR65EZDeiiVlwjYzinptAYjUhv3s0OnySTeDY3KXJus7u+QdC/R0oA26EGQrseVDlGUjlGVvoHCfh9OBcWqTeeRaJREwqHSO3uEt7eQSqKeDac2GYWCKgUrEzPorVZWb58A7VaxezdIWq6On+4DtNPDJ+pNtwfG5+60QuCoAUkoigGD/5/HvhX/K0Qxr/hvxTC+K8FQXiTbNJu/+CL4Srwv/9A8u488D9/0uvpOX2a94cGMBw9gUyh4JAokkzEye9sA6AlmSQZi2Hp6iCTSlG56yYejuJfWEKtVjN6+UM6/tFX8M85OP5Pv05oeh5bdzbBl+qX43OsUHi2F2n/EKJBi8poQFdWgmR6Huf0LClRxHa4ibx5B5ZD9RgrSkl+cJ3G33wV140Big6YdxPJJIloDOORJvY3nBjtVkrOnyKZSiETJFjbWlDlmLK/VCqFymDAcuIojckkMq0GQ0sDznvD7DiWyW1uoPD8KQRBIHL1FiabjfzeE6gECSRT5J/rJZNKsX71JhWnutFWl6N3e9GZc8g/2krEtcPS40kKG+qw5dqwtzRCKoVGo0VmNrE1NYNr3oHGoOfQK8/jfDiGtboCdUUJMoOB0OVrlHZ3Ym2oJRmP0wCgUCA8ZboskZ/H9PDTce9zgbuCIEyQJQW8JIriFX6yQhgfG3KFHPvZXlxXb5GKx1GoVZga6/A8HCcWDCEx6FDm5xJcXGH9Wj9FZ09mJZiSacLJBC1ffI2kcweNXIHaaCCWTBDz72f79JMpSno62ZtbRFDIsbc04ZldJLjjJrnj5ug/fgNZPIF33kFRVweJrV22Ho5jPdyERCJBXVJEYGGJWCCA2mah6GwPO/eGCc05KD93mv15ByqFkuLnTrN1b5j4fhDfyhrGinIiwRCZdBqFRk18bx+pXI5Co6Hj618l6FglHYuTSiRQm4zEEnG8i8uoiwqQFOYSXFln7fJ1Cs70YqooJ+b2otFpyTvdzd7oFDk1lTT1nECpUqK2WVAZ9CgFAWvHEVI7Hoo7O6g4fIjchjpi27skPH4qnzvDzvAoIFJ/7jThlXUQRZy37mI+eoSEz//0s/efknsvCMIvCIIwLQhCRhCE9h94vkwQhOhBqXpcEITf/YFjbQdlbMdBifqpOT6f+k4viuIy0PIRz3v5CQlhfFJIZDLs53pxXr2FMT8PS1EB27tu5t6+RP3rLxHx+Rn9y7dQ67Skb2SFJfdc28SCIep6unDNLZBMp1EU5lHUc5yd/kHiqTT5x9uRazVM/ul3iEbChP17yBVyHvz+t2m+kGXbCTi3kEok2J4/g3d6HqVGjbYgO2Zqqa1i9YPrpOcXKX/+HMGtbVzzDuQaFUX5drbnF1EoFACUvHCW1YsfIggCpZ87h6IwF8+jx6CQIc/PJbThQhKJos3PRf3iOTYuXiMtESg+c5LUyiqescdUv/Yiew8eMXnrPWrO9SFVyNHn2lh85xKF7a0oNBpEvYbw+ibIZRSe62Pj4jWsx1qRW8wYCvJYn5hiz+mi4MQxFFoNax9cQy6VIggCtp5Ohn/3j2j7x2+gqypjZ3AEhU6LVC7HeKTpU9iFxU9rp/++rNXvfcSxJVEUD3/E898kW5K+T3aje44fU7XpSfhZKtn9VOD3eQntbpERwLu9i397B4lCjlQU2V1dpWB6HqnJQFFdNVKZlOLnst9L26MTRDa2yGlrJrDrRq1Rk5HLWL58g4Brm1gkglImIxwJk4jHKTnUhP14OzvjU+TWVKGpKGN3bpFMOsXW0gYKlZL1mVm0RgOpZAqpRJKdvItE2dnchKs30JQVY87PRVSpWLtyk6Dfj8ZkJHDtNmqpFM+mk0wmg7p/CKlaxcLAPVre+BIai5nF77yLtamOtf57yBJJ5ColG5NTGIwGhIyIf9eNb3yKnNoqbMuriOkMuwNDxFIpYr59dCWFZFIp1AV5LFy6hiiT4PV4kAoi93/v2xy5cAbf8BiCRIJzahatSk1GzKBQqlkaH0ej0xKLRAl6vDiuXEen1eEYfkRudQVSiRRRFJ++e/8pteGKojgLfOypwYMclkEUxaGDx38CfJ6fG/3TQY7Zgq7QgmdkjJrnzuBfcGBsqCUWCGIpKc5mviUSdEX5SMw5+MenMDY3QCCEoMzSSqsNekKhECW5NkwlRaxdvk6+ToetpxPFppOcshICaxuIokjG56fu9ZfwDI1QfOIYG3fuk1cpRVldQaM5h/1NF/YTHchUKrzTcxg1KqRqFcXn+/DMzGNqqCXh82NtbWbtzhDbj6dpfuNLhDacVOXnkfT6MB+o6CSu3WTl2m305hxciw5UeXZshw+h1OvY/OAGBfW1GDvbcPYPkltVgaailN3xSSpfeZ7g+DSW7mOEd90sDz1g5cpNtLk2pGoVSCUYc8yUnD9FIhLBvbKGvqURqULO/qVrlBxrQ11agrogl/21DXRr6+R0deC6N8yxf/prxNY2sXQcRqlSEg4E0bYeIrq3//R77/mZiOnLBUEYAwLAvxRF8Q7ZUvPmD7zmh5affxJ45o0ewD8zj86cg7ooH2Wejb3xKWL7Aeq/+HmCMwuEd9wUnutFkEjYXt9k+7vvUfXieeKRKNN/8T2qX3oOvUrB3tQ8hsYa1GYzYZ8PSzpNaGaRwvN9CAYDc29dpKSzI9vBFwiRTqWQp1KEEfCPTVLywlkM9dXs3LmPRKdFpdVg7DiCyudjb2aB1K4XbWMdCa+f4PY2GrmCoo4jhLd2SLm9mLs7cd3IklG4+gdp//pXia27SCHS9rVfIjjvQKnX4bpxB3v3URKxGAHHChq5HMvpHlxXbqLRalHodMQyGRKBAHtjU5z4H/9bdu8MoTYZ8c8tUvPK83gnZkjsB3A/GKXui68SWFohEYlgbmlEXZCH8/INCm19RBZXMFSWENvbRy6KaO1WAhPTxEIhJAY9loYa9senCO664Wn33h+04X4MPFHL7seUtdoCSg7m4NuAdwRBaOQTlJ9/EnjmjX5zfZ3Y1g5CZRnByRnS0Sg7D8ao6stm8zOhKOocE8lYDNedB6hkUtwbm5juj6JQKgh4vFm3V2VgPxBk6+Y98s90o9oPsPDOBxR1tLK/sk7S7WF7fgG1WkNGKqGg5zj+sSlQKol7POxu7aAfGc9O0C0ukUmlqGo7gn9kHFEiMHHlGu1f/xUA0okE/okZ8vpOsD+7yMSbb3H4tZcQMxkSArhnF9BYzKisFsKziyCAOj+XsM/PygfXyW2oQ2HQozDo8S2uItGoCThd7LndhBYdRKNRVGVFeO6PorFbcI2MIk1nmHzrItocI7pZB3K1mtm33qe8+zhaqxnvvANEEfVBPsJ+upupP/kryntPYC0uYPJP3qTpy18AQFFaiOPtS9R/6TWkcjn+iWmS0finNlr7MfBELTtRFM9+4ncXxTgHwhSiKD4SBGEJqCG7sxf9wEufKGv198Ezb/SujQ0ErQa5TI7CZiWTSRNZWWdzfBIxGOLxzQEae7oIzDvI7z3O9u17FNfVYu/rwjVwn8KaKryOZWSPZ3DOLyIKoJ0wI6TTuOYWUKpVaGsqie4HqDp7Cr3ZRMLnJ7a0yviH1yluaEBQKchvqENZWYoqx4Te40WvVmM4kKjyzs6jMeewPTKGwWhk7Oo1Wk73sXHjDrbONooa6gi7vQTWNpBLpMy8c4mms6fYHxlnauAedSeOszc8hlQisDY6jt6gJ7rpIhmPM3PnHtXHOtBrNWhtVnR2G7YTHcQ8PmYfjVF8+BC2jiOozDlIJVKSyQTaplokcjmOgUHci0tk9oNM3bhNXXcX+8PjIJOSkUDQ72N3bpEc/x7xSJTw5CwhEcR0moDHx/6jx4hSKZ7VdTKIyGRP9+P403bvBUGwAT5RFNOCIFSQnSdZFkXRJwhCUBCETuAB8CtkFXKeCp55Eo2jXV3IojEMJYVo7VZCEzPUfPHzFFVXYu4+RmVrC6l0CmNTPRKZDLVej2jQ4nEso9JpyAgCee2HsfV1kVOQT0FNFYbWQwRDYRpffwW93YZcIUdrt5Lb2kzQsYqprhp1fQ1FjQ3YWhrRaTQU9J3AO5wVllRIpcQyGeLBEMHtHTKBEPVffh1DcSHkWyk+0oKgUlJ0NtvIaKquIB2NYe/uJJVOUdxQh+FwI8aOwzR0dyGRydC3t0CujUOvv0wiFsfU1Y7uSCNlrS1orRb0NZXotDryT3UTGJ1EV1xI06mTSEWQKpUkIlFkJgP20z14Bh8Scm1T98JZjCYTxo7DNJ49RTwSRdNQhbH1EPFQhJZf+TJ6vQ5VbSVypRLN4UaMna2E9vexVZahaW5AUVlKXl0NJrv9qSfyPsWS3auCIGwCx8nKWl09OHQSeHxQrv4u8Os/UGb+DeBbZEvTSzylJB78fKcHQFZVSmjOQWBlnYIzJ5FIpYQDQVSBILqyYrTlpTiv3ERUysk71o4yHGbx4lUOf/2XSaiVhLe2Ca9ukHesDc/kDEHXNhqjHlNVGRu37+J7OEbjL38RAHVdFYE5B3tLK1R8/gXct+6hOJho0zbWsvd4BqVCQU73Ubx3HpBOpcg70wNALBQmsrVN1bllN58AABZwSURBVCvPE98P4rx6i0g8Sum504gaNaO//20aX30JqSUH/4MxLF3tSDVq4ukUMbeX4PQcBWd7CaiUBKfmCW66KPncefwzCwTnHaBRIZHJSEol+BdXkBj15LW34PrgBqJcSsGpHiQSCRlrDouXr9Pya7+Ma22TyN37WZ6AHBM71/rJaWlEJpGgNOfgl0rw3bxD9RdeJvB4jng0gqWtBalRT3BqjvCuG/u5XiRbO6TTTzez/ilm7z9S1koUxe8B3/sh5zwEmp7ypQE/3+nx7LpJLK8z8c5FRKlAZHsHMZPBeKSRhe++h6GmilQohNpoZG10AtfdB+xtOFFqtLj7h9DodOw8mkBtMiI16tAU5fPoD/+cTCyO6/pAlrRMKmFjYJCtGwOk1jYZe+t9RKmUvYlp9sMhnEsrhNwetHl2Qm4PyWQS5+1B5u4NEfL5WL16i4h/j2gohFqvJ51MEnO60JlzcE5ME5xdhFSKVCKJZ3qO8OMZwskEGwODSOxm7Edb2Z+YRqc3EPH6CMw7stcgptkbeoQ0EGTie++zt+HE0z9EOhRm4i++QzoUJjQ2hSbHxNb8Iv6hETau3sQ9NolUImXr+gAatYrVuw/YGRnD3T+IUqtl+Ft/CukMgUePUSmV7M4tEN10MT9wB/+Gk6hzi9DMAlPXbxIMBNi+eYfgzMLTn6xFRMxknrg+63jmd3qr3YbeqqNaqUImlZDIZNi4dhuNWkPI78d95z6ixUQqGKTmuXNoDHpiqSQFJzrQFxexfvMOK6MTSAUJPtcOOYfqyC0tQVGQh7GkkN2ZOSLObYr6upFIpQQ3nNiqKzA21aHJsxPNZIj6pkn49ohtuAh5fQR8Pmpffxm1QU/U7cHS0ULMtc3W0goyhQJjMIi14wiJQJDC1sPI86wE5h00fvUXyPj2siXHoWHmP7xFY28PqXUXsXCIrZVVSk168k51o1QokOUYMRxqwDMyRsuXXye548Z49AgA/vVNlIX5qOxW3I8msBQVktPTiTC3iKmslL3VNeynu0mEw+hm5rAdaUZps5CKxdCOT6Bta86W8NY2MNhs6CrKKG5qQJBIMR3ObmjFa5voyorRNdaSyWRQ/P4fPf0bLn72jfpJeOZ3egD/vWFyOlqIp1JojAZyDjcRi0QoqqvF2teFJJUi51A91qY6IitrhByraPJy8U5MYzLn0PbCBeQaNTmF+ejsNuwNNfgXl0kGw0Q3tii5cIrg3CKZTIbI/BJVr36O5EY2OSvZD2LvOIJcqSSdymCvr6Wougrfo8foG2vIO9eLZ3AEbWUZtvJS7MXFFJ05icqgJzLnoOTCKeLrW6g1WvR5uQihCKlYjLQ/QPs33kCqUmI43o5Eq8VcXIimpBDvxBTahhqEUBT/42m0ZjOa0iIigewU3s7wKOUvXSB+oMYji8SQ2234HMsI4Qia6nJUpcWEnVv4749S/8aX8I0+JhWP4747TEFfN1G3h1QsRnJlA3tZCf7pWYwtTWjqq/GPZHMXxjw7kV03qUgU98NRpPKnvAdlM3lPXp9xPPNGHwqF0NqtSGQyTC2NTL/5Pfan5sg714uqvBjf/CLSVPpvSlGCxURibx/P4Ahqgx5MBtYnZ0gmEmyMTvDom39AKBym4EwP7rv3UcpkaMw5iHtBdvsHMR/Lsn0lIxECri0U+XaMFWU8/uu3CWy5UJQUEEmlsjuxUolEIiH//Cl2rg+g1mgQCuyEV9fxL6+iLStBIpEw13+HvcA+67fv4Rh+hPN6P7nnelHn2onFE1kpaY2G/LO97I9MIAtFkRn0zA+P4F9aRVmWrRaZWpsJzTlQJFIoLWYiyURW+LKmAqlUwuJ7l1FVlAFgrqkkvrGFOseERCol90wP7tuDaPQ6rDWVpLx+3LcHMfccI51OI40mUFrNaPPsZHRawnMOkMnIPdVN8NFjFPH000/kZQv1T16fcTzz7v3I0BBxvRrX9X6SUgnJaIxIIsHu7XuoNRombvRTdayNnVv3kEoEBIkU59w8JXIZ8WiMhFSCNsdIwcE03MpbFwl7/Wxd70cql7My8ZhUJs3m5DTG/Dz0sRgyrYa4QkZg6CEKvY6Ex4/OasFUU8n2yDj+lTV8mQwKrfrgKgWi0Sjba+vYK8qJZzJIpBKUNiu7N+8gUyjIP34UgOjWDuH9AOGxqexOm4jhvHYbbWkR+wODBFfXSYtQpNdhLCxAYTTiujGATqtFolSxMDiIoaiI4JUbyGUynGOTaORy1IV5aC1mXMOP0CoUqLRaZm/cpuJYOww+RKKQs7W0gjbHCDIZy0MPKO3rITDvwPFwDHtFGbEbA9muO0HC5soKCBLs+/t41jdJJpJPvWSHCDzlZOE/BDzzRn+ir4/L4w/J7TvB7o07NPzqV0iubmBsacQ3v0huTSWmliYU5uzY6p5jGWtVBXmne1DodXhv3yNVVEB0141Uq0Vjs6CyWdHXVLJzvZ+Co+0YrRaSyRQqlYq9jU3cjyYgHse7tU3zC1/FPThCxWsvERmfIr/7GAoR0ojIK0pRGA1kMhm0e3tY8nLRtrcw/9fvEfP7qT96BEV5MXlSKbFNF+qyEixV5SSiMRQVpejNJrThKBPf+mMyCjmFZ3qRJFIIYgZ9cz0J/x4ymQxTXxcAwZV1tDlmbE116CvLCaysodveRdPcwO7jKco/d4H9kTFMXR1I5XLMM3OYmutR2qwAlCcSSJQKpGXFJPvvkEglMVVVkl9ZgbmuGm1tJQCpRIpMIoE6x4S+vYXUtX6UajXe6dmnfLfFZ8J9fxKeefdeLpcj2Cx4R8bR5NlR6nUIB1rpadcuZZ9/Af/D8b/pFkutu6j8wstE5x0kwhGkJiPWw00kVjbw3nuAsbWZVCzG1pWbmI93kNfZRtDtJh2NkpHLMJeWUHz+FEq1hvKzfcSX11EplcjUKqJihvUPrqE/0oStp5O94TFEUWT31l2MR1sJhcJI5XLsRQXkVVUhVSpxj4xj6zhCxrvH7r0HqOuqsXZ14H84RjIaxTMwSMuv/yO0BgNiIoFGr8fQ2kx4ah65XEZcKSPu87O/uAz7AWq+8gUy2x4EQSC55qTiCy8RnppD9AdQ5hixnOrBf+cBvpkFSs72EZycA8A7OY2irAQxnmT/0QSt/+wbZFw7SKQSjHYbMdf23/zNvbfvYOnrIhWNsTczj7GuimgySfrToKoSM09en3E880afyWSIbu2y/mCEoGubVCxGMBTC71hGVZqNdbWtzQTHpvBOzaGuKUcikRDbD+AZGkFTXwOA2+kkuB8gOLvIzAfXkamURCZnCY+Mo5LK2F3bQJNjJDg5i2dsEn1DLfFgiIl3LxLw+Qk/nECezuBd3yTyeIadG3fYi0VZfucD9OWlWXbaHAMrl66ha67H0nuc4KPHaOUKZColoXAIpVSGVC5jZ3yScDjCo2/+IVKlEt/dB6QTSUZ/99skdWrUFjNhtxsxI5Lb0Yrz+m1k8Tj6g6x6SqsivLmNwmREplAQ8/pRKZVZXn2FDHlFCeu376K0W1DVVBCedyD1B1Hl2YiEQ2gMeiRSKfazJ/HcuotEKkFRXUZ4cRn3zbuYDzyFUCpBeGUdRUEeuT3HCAcCpNPpp3ezxZ/H9PD/tXemsXFd1wH+zsyQs3FGMzTFRaRIipK8UKQ20hLtSrXjqInsFrF/pIDbIDaSBkWBuG3QP3UR5E/RH0kLFGiQ9ofRGLHhJK6Buo2QxlFkxZUtW7tji6RIcRElLuI23KlZ38ztj/fojFiKS0RKs9wPeJj3zr33zTnknLl37jv3XD28p7O9HU/pA2yvf45kaIL59i5is7PMnfuYrc8cYWZomPitW0x192CEw2w9/Bjx7muEVYqx1g6cThd2ZyEJEXwPbic6FuLhQy0UW9lzAEaOv0fd3t0kN/kY/ug8sXiCbfv34K4qZ+ehx7G5Xbj27GLu5AdU7NxBUUsTRYB7PMSV19/C4/MRHRrGkUgwMjBI8FoZKRFCN4cx5uaJRKNEJiaZiEZRPg/eLRWoiSnq9u0lcNjUIxyaoKD9CvFYjNGT7+N0uuhrbaOuoIDhnj4CtbWkLnyKMgxULMrlX57k4ef+kLkbA9iqyrn+7imSRhKX14NyOSmtq2Hk1IcUub30XvwY7+ZiCj5uZbSrF395GfYL5hoSI5Ggr7WdasOg88w5ynfsINrWxUwsylRPH67gJqbbOiGVxOX3MTAwQG1t7cb9w/XwPvt7ehE5KiJXrYwjL6/c4nbqGxsxwhEckQgyPYdvXwOeulpmx0NEh8cgHqfA7cKuwLe5lJTbTcrrRuYjeDb5cRQH8OxvpLylGZfTidvrJZZKkZgzdx0KnT5HoHkPNrsNb1kpgS0V1DTWU9S8h/mObor2N2KPJzCiUVzFAQgGiI6HAAj/po3Kg034mnbjfXQvRjxBbeMuPLvr8ex6CDvgr9xCyZOP49u8mYraGvyN9RjhMEWVW4gnEgDEw1EibZ1sbqin+JGHCB5qITw7R21jA5FEnD3feAGb3YanqRHvwX2I101waxX24gAJYLq1E4fDQYG/CHfTbnwND+MLBCn73GFU2QP4t5RTfugxEgEfdU8eIlhThatpN6maSlyBTWzb3YBtxzbKG+oJ1j+I58BepKSYHX/wJOXV1WxqfATb9DxF22rW7XNxR/TwPrudXkTswL9iZtWtB/5EROrXep9g0x7C4xOMDgwQm53DPjfPzj/+ErZEAk9lBeHWDqqeOYI/uAm7w0H8Sg/lTz9FTeMuDGcBUx+cxen3M/TRb39Thzu6mGrvxLOlgoJgAAWETp0heHA/0WiM2cGbeMtLsRcWEg1HmPzoIp6GRyjZuwuj/yah02cJHNhH6YF9RG8MEvr1hwQOt3Br3kyDNX7iFBVHnsDrcTN5+hy+5t0ki/1ER8ZIXOvH/WAdBP1ExkNMvX+G4OEWbG4nsdk5Qr/+gJLPH0JsgsvuwF1STHRymlQyycSZi3jLSvFUlFHo8xHr6KbqqcNsbajHvrWSiZMfkJidQwHTrVewhWNUPn2EyOg4yaFR3A9tR0VihEOTxDp6CDz+KKlkypykPPIEkf4hjGgUmZrBWV1FbP4Wc53dOLdWYHM51/cDshilwDBWPnKcrHZ6zCy6PUqpa0qpOPAm5uYYqyYRjxHp68dVHKTQ6aL91TewlxRjC0cJXe2m+7U38dRWkxgdZ7S3j+FfnsRdW0Wsf4hb4xM4FDhKSxj82S9QRhJjYpLYjUFCvX1Mt18lpVJE+voZ6bxKihTG5DTuijL6f34cnE4iff3MzEwTmZomPnjTrHulk8StCMbUDImhEQZPn6HggSCJmyPgdnLllddwV23BGJ9gpOcasdl5jPEJ3B4vvf/9P9g2+Yj09eP1++n66du4qyuJ9Q8RnZzh+ts/p6iulvjgMP2ftpEodBDp66ewbDNdP/oJKpUimTAocLvo+OEbOLeUY0zPMDM6hszN491ey9Dx/6X3/CWiw2OIx4UxMmbuglNWQqSvn/Eb/Qy/8y7OynKi1wfo/+Qy4vMSvT5A0uvm6o/exLG55DPbpzq7iXvdJOOJDfmQpKOUWvHIdSSbjRSRLwNHlVLfsK6/ChxUSr20qN6fY+Yfo7q6uunGjRuflRmGQSwW++w6mUxit3ZuWXy9uEwpdVtKpPTyVCqFLS3YZLn7Lq6/lraL6y7XdmEF2UL5cjqspJNhGLc9V08vX/hMLfxtlnufZDJJKBTCZrOhlKKmpua291lARC6ttMZ9JSTgVzxxcOWKx9696/fKZLJ9Im9VGUesrCevADQ3N99W7nA4Nj4oRLMsfv+q9l1cH/REXtY7/Z02wtBolkDlxUTdSmS7018AdorINmAIeB740/urkiZjWVhwk+dktdMrpQwReQk4DtiBV5VS7fdZLU3GomAjg3+yhKx2egCl1C8wNwfQaJZH9/RADji9RrMm9G/6rH9Or9GsAXVPkmiIyD+JSKeIXBaR/xKRQFrZ31nRo1dF5Itp8ruKLF0L2uk1+YPiXi24OQE0KKV2A11Yuylb0aLPA7sw96r7NxGxr1dk6WrRw3tNHqHuSRINpdSv0i7PAl+2zp8F3rQ2vegTkR7MqFKwIksBrG3ZnwWubIR+eef0ly5dConIjUXiEiB0P/RZR3LBBrizHXe/Gmc+cpzTn5SsoqZrpW2t1sDXgf+wzisxvwQWSN+zbmCRfBWhg78beef0SqnNi2UicjHbwy5zwQbYWDuUUkfX616r2ctORL4NGMCPF5otpRZL/8zWe9lpNJnESnvZiciLwB8Bn1e/XeCyXATpPYss1RN5Gs06IyJHgb8FvqSUCqcVHQOeFxGnFUW6EzhPWmSpiBRiTvYd2yj9dE9v8rv+XsskcsEGyA07fgA4gRPWSsOzSqm/UEq1i8hbmBN0BvBNpVQS4F5Glmb10lqNRrN29PBeo8kztNNrNHlGXjv9vQx9XC0i8qqIjIlIW5qsWEROiEi39Rq05CIi37f0vywi+9PavGjV77ZmkhfkTSLSarX5vqSn/lk/G7aKyHsi0iEi7SLy19loR86ympxhuXhgTpj0AnVAIfApUJ8Bev0+sB9oS5P9I/Cydf4y8D3r/BngHcznvy3AOUteDFyzXoPWedAqOw88ZrV5B3h6A2yoAPZb5z7MUNT6bLMjV4987unvOqnmRqCUeh+YXCR+FnjNOn8NeC5N/royOQsERKQC+CJwQik1qZSawowFP2qV+ZVSZ5TpOa+n3Ws9bRhWSn1snc8BHZiRZ1llR66Sz05fyf8Pfay8Q937TZlSahhMhwJKLfmdbFhOPriEfMMQkVpgH3COLLYjl8hnp19VUs0M5042rFW+IYhIEfCfwLeUUrPLVV1CljF25Br57PTZlFRz1BrSYr2OWfI72bCcvGoJ+bojIgWYDv9jpdTbljjr7MhF8tnp72no411yDFiYuX4R+Fma/AVr9rsFmLGGzceBL4hI0Joh/wJw3CqbE5EWa7b7hbR7rRvWvX8IdCil/jlb7chZ7vdM4v08MGeNuzBn8b99v/WxdPopMAwkMHu0PwMeAE4C3dZrsVVXMJMv9AKtQHPafb4O9FjH19LkzUCb1eYHWFGZ62zDIczh9mXgE+t4JtvsyNVDh+FqNHlGPg/vNZq8RDu9RpNnaKfXaPIM7fQaTZ6hnV6jyTO002c5IuIWkVNW7vTVtnlJRL62kXppMhf9yC7LEZFvAg6l1L+soY0H+FAptW/jNNNkKrqnz1BE5FFrbblLRLzWuvSGJap+BSsaTUSetHr9t0SkS0S+KyJfEZHz1trz7QDKTNZ4XUQOLHE/TY6jE2NmKEqpCyJyDPgHwA28oZRqS69jhQ/XKaWup4n3AI9gLs+9Bvy7UuqAlcjiL4FvWfUuAocx16Vr8gjt9JnN32OuEYgCf7VEeQkwvUh2QVnLV0WkF1jYYqkV+FxavTHg4XXVVpMV6OF9ZlMMFGFmn3EtUR5ZQh5LO0+lXae4/UveZbXX5Bna6TObV4DvYG6L9L3FhcrMJmMXkaW+EFbiQcwFK5o8Qzt9hiIiLwCGUuonwHeBR0XkqSWq/gpzVdta+T3g3btQUZOl6Ed2WY6I7AP+Rin11Y1so8kddE+f5SilfgO8t5bgHMwJwO9skEqaDEf39BpNnqF7eo0mz9BOr9HkGdrpNZo8Qzu9RpNnaKfXaPKM/wNo1mqCPiRKugAAAABJRU5ErkJggg==\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "model.quick_plot('land_surface__elevation', edgecolors='k', vmin=-200, vmax=200, cmap='BrBG_r')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We now stop the uplift and run it for an additional 5000 years."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 23,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAP0AAAEKCAYAAADZ1VPpAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4wLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvqOYd8AAAIABJREFUeJzsvXewZdd15vfbJ9+c3n05dc6N7gYaGSDYAAgCzBQljUbJMsdyWVTVyLI9ljxV9ow9si2XNJLl8tBFDeUhZUkkRZEACAIEkQE2Go3OOXe/nO67OZ28/ce9ICEOwAZIPGLIfl/VrvfOOvucu29YZ6+91rfXElJKVrGKVdw4UN7vAaxiFav46WJV6VexihsMq0q/ilXcYFhV+lWs4gbDqtKvYhU3GFaVfhWruMGwqvSrWMW7hBBiRAjxghDinBDijBDin3flWSHEM0KIS92/ma5cCCH+QghxWQhxUgix5/0c/6rSr2IV7x4+8N9IKbcAtwOfE0JsBf4AeE5KuQF4rnsM8DCwodt+G/j8T3/IP8Cq0q9iFe8SUsp5KeXR7v914BwwBHwC+FK325eAT3b//wTwZdnBa0BaCDHwUx7296G9Xy/8fqGnp0eOj4+/38NYxbvEkSNHlqWU+Z/kHv1JRbr+9fuV2/IMYL9J9AUp5Rfeqq8QYhzYDRwE+qSU89B5MAghervdhoDpN10205XNv7t38N7ghlP68fFxDh8+/H4PYxXvEkKIyZ/0Hq4P92+6/k/+68c9W0p5yzsYUxz4B+D3pJQ1IcTbdn0L2fvGf18171dxQ0ER12/vBEIInY7C/42U8htd8eIbZnv371JXPgOMvOnyYWDuvXg/Pw5WlX4VNwyEAF0V123Xv48QwBeBc1LKf/umU48Dv9n9/zeBx94k/42uF/92oPrGMuD9wA1n3q/ixsY7ncmvg7uAXwdOCSGOd2X/A/C/A18TQnwWmAJ+sXvuSeAR4DLQAn7rPRnFj4lVpV/FDQPBe6P0Usrv8dbrdID736K/BD73k7/ye4NVpV/FjQMByuqCduXX9EIIVQhxTAjxRPd4jRDiYJe19FUhhNGVm93jy93z42+6xx925ReEEA+9Sf7hruyyEOIPfvi1V7GKN+ONmf69cOT9LOOn8dz753TIC2/gj4E/67KWysBnu/LPAmUp5Xrgz7r96DKd/gmwDfgw8O+6DxIV+L/psJ22Ar/S7buKVbwtVCGu237esaJKL4QYBj4C/PvusQD2AV/vdvlh1tIbbKavA/d3+38C+IqU0pFSXqPjDLm12y5LKa9KKV3gK92+q1jFW0II0NTrt593rPSa/s+BfwEkusc5oCKlfIMX9QYzCd7EWpJS+kKIarf/EPDam+755mt+mOV021sNQgjx23Q4z4yOjv5H54Mg4NWXvsvClUMcPnyI7WvTAJy+vEgYBuzcOAjAY8+f44E71hGLGAA8e/AKD9y2DoDLUyXKtTZ7t3eG9vT+i+zdNkQ2HQPgWy+e5WP3dQyRQrnBtZkSt+7ojOXgqRksQ+WmDf0APP7SOT50+3osUwfgr791iE/dv51YxATg7797kk/fvw1FwPEL8/SYHqahY7s+LxybZ+NoBoBq02WxZDPS1/n4L81UcVyPreM9AMyXbQYyFgCOFzBTqLNusPPel6ptfM9nsKdz7YmrS2TiEUZ7O8cVG9KdS1mqtPFDyWA2CsDEYp24pdGTigBwbrrMpuE0ihB4fsh0ocbagc7rzCw3aLdtNox0xvTcqyeYrYZv9TW+J7gRzPfrYcWUXgjxUWBJSnlECHHfG+K36Cqvc+7t5G9lpbwly6lLofwCwC233PKP+vzdl/4fLh1+nPu3G6y3NE5TZ2uvgaIIpmZVcnGF7f1tDp6v8sm78zhene39UY5dqhLTfbb3twG4NhMwkLO+f3wkoTOYbLO+X+HJQ2W2DRsMxapkEgbfvFZnw3CK7f1tjlyqcdeWONPLIdv6mkwutbhvZxrpVtk2GuH4lQq7N+SYml3m07clUBTB3OY0lycX+PjNUVp5F68wSVYTPDcdIx91YfksALYcJBVVcRbPAKDTS0wps1BQSPpz4CqU2joRxaWiDqKHNs35GRQhUMwRTNq0F6aoBknGoj6LTZdaqYHulggio7QrU7Q8FT3Wg6ZatBc6r5Mwh1FwaS9cJQxDklqe8vIc0aBCiTwRVft+34g1QrHdojV/GiEE+grOtIJVRx6srHl/F/BxIcQEHdN7H52ZPy2EeONh82Zm0vdZS93zKaDE27OZ3hOWUyIiSJoep6c7xsfN66Ocn22zWGozlFOQKNiuT81W2TYao+Z0fpXzNY1to1EKFYfnjpe5fYNJ4DZpOz6Fcptt4zFKjZDJxRb92QgP7e3l4pzH/tNV9q7vTJELJZuGo7JpQKPZauD5IScmA25bH6HafZ3pkkomneCDmxWeO+MAEI0YfGBdwLeOtBjtjVAPTF6ZjrMlXiCpNLA9KNo6Ob2O224hpaTkJ0lTJGJopIM5KuoQCT1ERHLUPZO4aJBiGVtJ0/IketBAVTVcP0SzYpi06M2ladkBnp4h8ANcPySI9mK5izTqVUIpKflxdHsB2wsAKPlJEmER9CRhGGIZGl6zhBsolL0oml2gPxXB1vNIKWk6K8hOFatrelhBpZdS/qGUclhKOU7HEfe8lPJXgReAz3S7/TBr6Q0202e6/WVX/k+63v01dLYnvg4cAjZ0owFG9zUe/3HG+oEdKfqTkkcPVulJmRTqKgcuOuwcs5BC8OThOvdt75iurbZHteHSk1S4dXOW83MuQjHJp3T23ZTh9LTL98612L0mQiBVjl/z2D2uY2gKNUfQ8lWGsjp+KHn1XJt7NndM9g/uiPPEoSLrejWEENRrDYIgJBkRhGFIOm4wnHQ4NuEjBSSjOves9Xn1UsDhaz7DVgVLC1mfDWiJOCLWR0I0SFLCDg0isRRRzScUKoam0MssVW0QL1RQ4nmMoIalK6hWCs8axPRLCFWjaQwTdTvP0mazSVJUaHlQrrVoGEPEu+diYQlbJLDiWXThQ2sJW5pEk1l0FVr1Eot+FtOeJ2e28c0cRjyHgY0VMbEDjba0CFdW51e997w/NNz/Hvh9IcRlOmv2L3blXwRyXfnv092LLKU8A3wNOAt8B/iclDLo+gV+F3iaTnTga92+PxZG+yJ8fG+C7xypcXGyQDZpIoTg7NUiu9bF0bXORyX9Ft85WmHPWhNFEbxwaJq7uoobsTTqtkoyHkVRBK+dXmD3mMqpyRavXnR4/sBFFEVw4KLLUy+fIRNXeO2Sx/6LHqdmFI5dWMQPQnw/5Ja1Ol96Zoatg4IwlPh+SESXHD8/x8FTszx5wuHQtIGhhDh+QDnMMdeKYGiCxaZOhmUAemMhc+0YEXcRTQEpfmA/q3aRs9cW8DyPhtpDM7So1BoYSogQgvmSTZwyb2wkMbwCLRnF830Wyw3CIMA2B2gGJhFdUHIsNKdDN08bAaGZg8BGSknUUGjYAbbegysNSi3Q3WJnIEIh4s0x24ywkmUYBKuOPPgpkXOklC8CL3b/v0rH8/7DfWx+QFv84XN/BPzRW8ifpENx/LFx9Nhpnp+ZY/1IBomgNxvltRMTmLqGFyjMLFSYq/SyUHERIiRimRy7MMsL6RSXJ+ZRFXh0/xKpdJpYROGVw5cZH0zxrMwxt1Dm2CWNO7akWN+rcG44zfqekEwMDvUk2TmikYl3HiaTi236M1Hius23jzSJxxNMzlU5Pt3DgeMTSCfDeI/OPZtNnjrmsX1QYSjtcnm2yfDeNCNZwUIlwWPHTRrNKnZfP7YAENjtBfzePHa7yVI9IDIwii5cxqLLJLID4NskwiI1GVJqQOBruJEstVqDmBHHMtMoQiFQApaqTcbjDWLrRgjDkIgzQ82XFJQMpXqT3mw/HpIw8DlzrcC6wSxt8sScJcYG1qDVr1G0FQr1GomhHKGWYqnYIJMcRbHreCvnw+uQc26Amfx6uOEZeXt2b+dE/TzrB3TySY3Z5Ra/+KEtlBoSETS4fXsfAynBuv6OJ/3xgzZ7to1yz2aVwMvyzx4a4sVzNg/s6Hj0z15OsXNdmk0DCklzPROLLlFL57snGvzafb3svyzRlkN+56NDPHnc5mM3d76C41MB/8WHB9h/KeBje3WePdFgbCDF3hGHiDZKpRGyYUDw1DGX/+yDPXzvoo+iKJxfDLlnDKaKHmcKMe5ZG9JoWoxoM1ia5FItyV1jARUF0lqFop6knykUIfCVkCguDS0KbhEvMsi9qWWKQRQnNHloQ4Wrjk4yWEbHp6AP0pOKoIU1fAKcdh1PaCRNH+nb9CUUZGsJQ7ZwAkk8kkQACd1DFwqtQKIBSnyAnZFZ2qqC0Z7C9TNE3AJeYL0tt/W9gIAbYs1+Paz6MoFH9qZ46VSdthNw6IrPzWtN1LBNzVZ56JY8F6dbLNc8zk42WT8YRQQO39hfZN/2CJqmoAmPxYrHk0eq/NK9PcyWJEcvVVnbq/OZO9N861Cdtu0ThJJKrUmx5qCqCjuHBccnfQoVh6GchqIolOsex682GcxZfOaONH/5zBI7hwT9SZ+JokSzEliGygPbTc7Oh5QaIU9fVCk0NG7rKzNdcrh3rMlS2IsfhggjSm88IEuBS40Mu/INFmUnBDnTTJCkiOkUmLazjFhVLC3EkQYpM0RVBBsiS1RFD3N2krQskpGLFP04YbtMVq3hRjoJYLRYnj6zhbQ6obeWMURPQicjF2i3berGMI1mk4o6SCpYRFUUwuYS03aG8ViNuprD88MVXdNDx3t/vfbzjhvgLf5oLCwW+PrL8wjp8Ud/fQbbdnn+tMvkUpsrUwVeOOMSKgpffnqSJw7MUahLlmoeFycK7L8U8vQJm0oj4G+en6Y/Y5GO6YykfM5MtYhHVB5/vUpf1sJxXV46XeHs5SVml6p897TH8Ws2Z66VeO50k419cHbapt22eer1BQoNhdcmNcq1NoemFFB0jk24WLrk1Qt1njrlY8WSnL5WwNB0Wr5CpS2xRYKEqWD7cKGep1/MU3Dj+NFhbD9gkQFCu0JF9BJJZAmFQVNJ4HouDT1PWR/l2lwNTzFZ1kYp66NEdFisejhWP0F0gJlKiBk2AAiaJRbsGLpbADrOvqLsIeIuUKy3WQ57INKDoggmF6o02i5udJimOUxoZKi1XLzoKKoMqTWaK55ZYtWRt2re09fbw557+rk638Jxe7ltc5w1fSZPOCl6cyk+uM0ADMq1Jpmozge2mlTrUfbuG8cPJTu2WPi+wb/+6wUuTBVZqKaJmBEuTl5m/7k0D++KYugqr2i93LFWYFoJvFDwoe06QZjmxZNl9p8pkDLT7FobI2qp3L5zjA9sVnjpdJ3PPjTGZDlkTQ5eOl6kUDb5pQ8MkE8ZPHeqzi3bhnlokwMSvn5YZ7ZYRx0cIcBmttQmMtjPTf0tplohn/tQntPzCnnd48CEZL5UJj0UYySfZCgbY2NkFtsPKfelSKt1ktQIg5BiMMi60V7i9iRhGGIaKZa8FNlojGjM4NrVJdLZPtpI2uUm9VabbMQim04QMRRi7iz4sHnNEL4PkfYkQghKMstwPkWkPYniSVRVJ1jBNb24QZT6erjhZ3ohBG0n4MJcyD/d18/VArx+ocrOcYO06bBUdnji9QoP39KD0COEYUgqrrN1LM5ixWap6vP44Tr/4pc3sGYwwyO7o3hui/v2jHB5psL5+c6v2PXhqWMN7tigIb0m9ZaPqggavsVd23rZuzFNXyZCPBan7fiEYYgnDcZ6TQhcnEBl/fggQ/05sgkd3w8RWpTbt2SYLcPzl3Vu3Zhhy5o8tw9U6cnEuHnLEB9c61LzDEZ7o+SiIbYvyFke/dkYu9bnSeouI7EmRlil6QkuVDPc3lel4lp4IVxu9ZGRC4RBJ+5eCHvZkGhgRRNE3Hnc8gxjWYXAd1Hqk2TjBv25BIamkKFC0K7iaR32naFrRNxp2sZAJ2ZvmoShB0DbGsLQ9RVe018/gcY7SaLxs44bXukBvn24zkN74gDU602WGyqjPQZ7N6X4m+dm2bk2Ti6hEVHaPPbqIjtHOwbShgGNP//7c+RikkRUp+1rvHK2zrr+GB+9LU9vT5pkJORbR9tMziyTTVpYhsqDu1Icnw559niNOzZofHBnkuPTcGqiwdp8SMay+cory9y2tjO+e7dGOXjFI5mIcP9NUQ5d9Xj6RJO7NpvUHfjyK0VuHvZZm/UIPJuJEmwb0NiQdbhS0mlrOdZnOkzBzbkWx2Z11vXpDMQdFtwEfVGbnf0BS0GOWDSGpgp2ppc4OB/HEja6EuK6Ni03RNcEmhISc2doGQP40X7SWot6pcSlchRFhCgyYKEWIkOXpNqiERi4oYJvN7E0BddpMef1YNpzeHaLsqMSpYnnh6vm/U8BN7x5//RzL5NA8sIZFz8IuDJbIfAD7HYaTQmZK1Q5dLHKxfk4hhHn2IXzROIpokZAX1Jl87pBlis2TxxucOj0FAM9cSxd0HIU+pMeL59skcumOD+xRDRq8DIqmvA4fWWZreNZehKdwLDrtrhcV9g66LBQE1ydrXKkvwelO/fNLtdZKDXQtUFeOzGLUDViiThu02Z8IMWRGUEsFsNQWlytxnCVOg4xDl6qct+eCEeXO+Qizw84PX+NSFqn3lCYLlQ5lR7ApMXEskOvVeKaGKThBDTsMrP1CPlED67TpmIMkXZnQQj8EOaW6oSomH2jBK1l+rJxLHcR1xEkYxkqTkgkmSOiqZy+ppNPuojsKJaQTE0ukRsdQ3XmqQQJvLbE9f0VVfpV876DG17p9917B0dfeZT7txsIIQjDfjwv4OO3JpgttFgzEGe+Knhgh8mlmQZrhnPcNGYwkNEIw5DFGtiu5MEdJq4/TDyisWuNRaPtMeVKWk7Ar91kErc24QZw7yaVIIBDZyXHLy5SrCbRdZ3pxRbVeotda4bpT7WJb80znIb1fQLb9XGDPIl4lHu3RZhcyjGej3H3VotHX1HYtxGqgcm2fptHD0kWSk0+flOSZDSk2sox1h9l84BgqeLwwskWu9Yk2ZIqMun7lNIWe/vKuJ7kpbNtkmrIKLNk9ZBmXw+BYqI0p1hsJFBFA6tvGN+1QWkSi0VJRTTM9hSpzAjtRp0ASRgZINouEbMkhjdL6IQM9vSSjKhE7SmWvRi9CQXVnsf1fJzAZdRqYmjWiueIVVa1ftW813Wde7dGeeFUG9v1yUQFN42pnJ12OHS5xdaxGDHdZ3rZ4/wc/NZDw+w/V8dxAw6eq7BpQMFz27x0qsTeDRHGspLTMwGZhMnVks54f5THDpa4eVxBDduU6j5PHG3x2Q8PMDaY475tUVwfPnlnP5++Z5BKW6ViWzy0J8fleZtSI+A7Jz3u3xmj7QQ8+XqRh2/OsVx1CUNJRPUZSOvMVDo/Zj2S5iNbFY4vRqm1fDb3Cc5O1rkw2+LguTr3DNe4e03AxVqShabJTT0NrlUtQuDWtTEwU7ghXCgniPgFWraNpghG+jL0xUIIfGJBEVdL0qNWkKpO3VPQvQoJUcJR01imSsZ0CCOdtO8VbYi8UsBzWnhqnGgiR3+kTWD1Y8SyDMc8AiuPHwSsJDenkzlHXLe9o1sJ8VdCiCUhxOk3yf6VEGJWCHG82x5507m3TATzfuCGV3qAnpTBQEry+W9NsWuNwXh/jGtLPrFoAlUR3LUtxVefmyAb85FS8slbU3z3eIvllk5fxmRNXuPlE4s0Wh6DOYNGw+alk2U2DFqs749w8tIy3z1WwnY8/s9vXqZttzk5DYlYhD/5+6tkklGuLgtKbYPXzy5x5soij75eJgjhz79xFcf1OHrVZv/RS4SBRzau8YEdMf7m2VmGozUA1iXrvHrFJ6M1yMUFca/Al/Y3mVpq4ToOX3ryMpbi4fgSRVGotwM00yITVah4UQ7OWgwbRW5KLzHpDICVRlehRywx2U6j28vERY3CcoEzxSh4LVTp0qxXCSL9mGEdS1Ooeia0OvTalu1RljkSYRFVEcSpMVNXsJvVzvl2i2g0QtzwaLsBrhesuCNP09TrtneI/0AnqcsP48+klLu67Ul4+0Qw78Fb+rFww5v3heUSk9M2tuOyVKzx/Mk6kUiMxWKNaq2Bqo7RaLVxg4C5pTqPVn2sSIRyo8XiUoVUfJyMqdCXS5CyJK+dK9P0NA6dmWXv1kFarTbjQzk+vCeLImBiyWXzaIZdYxot2+f0lQS3rdeIRzpfRa3dg2ZEeOS2LAC2iHDT2iQ718W5tOBSrbV4dP8iViTK8XOzGLKXs0sgMXjp6CQ3b+pjsR6FwGO2UOIXduc4u9DipvEYXmOZFxc0YukeTM3n3LVl+oZ9Kq0KoVC5WNdoiTgV22Gp0mZsYBxpSApzC2RHMrQdnVy0QaOp4zkNipE8AYJKqUZ0YBwQLF1bJDmcw9FU3FqbUs1muLeHqmPjuTahH1LxQ0S6H0PTmF4ssWZwHJMQ319eWe69AOU9YuRJKV9+c0q36+D7iWCAa939JbcCB96TwbxL3PBKn+/JMhSz+PqrNv/mszt57lSbfdsNDigWVxdU7t9hYrsq+dQYIYK7N1tML7U47kdIxyPcu8XkWwfrjOYtIhGDB2+O8erZGutHcnzyjhxPn7B5YLvBk0ebfHRPhF0be3Ecm/NzCkulKr/2wUFeu+zxwA6NqwstRodyTC50iC8Xp6rcvrOPS1MNMokGt2zKcmFS4+N7Y9hOgFfJ4TgO27MVABZGUkivzai1BCr4G4eYb+p4qsG966s8eT7Lzp55FNHiUsnEDmCyLDhfqKOoCrvyLj16kVYwyEhfBKs1wbKfZE3Gh9AnRo16qDCc9FC0NNFgmaoxTDRqYjQnCMOQDUODOH5A1JnG1AcYzBhEghJJ1SY0Q+zUIKqqYbZnKNk5BnrSmK0Jyko/iqqCXFEDH2XlKXe/K4T4DeAwnSKXZX50IpifOlbNe+DJQ2X27Yihqh1KbaHmsdzUuXNLnFOTDk8frbBnrUHLkZy42uDasuBje5MMZ0KmCw6GFeOhvXnOzgaEYUjd08kmNA5eqLBlUEVVFTb2C/afaxHXPW7bGKdQbXN22qEnbeG7DtWmx7klnd3rY+TiIUtlm3Ozki1jCaJGwPNHiuxYG+eeHSmOXXN46vVl9g45WFpAydaYr/j0GnXwWpT9GLNVheFog2YQwdI7s9u94y0ut3pp2CGLtkXCCGl4KtsGdcbSEGhxplsJkrKC4zj4YYhiREnqPi1PIQglQaSfuGihmHGqQYJosAzNeRwlQTlIoztLRN05WuYglqmSFmXqZAilpEwew54HoeGHIZap47aqFNwocdEgCCQrTY1/h2v6HiHE4Te1336Ht/88sA7YRadO3Z925atlrf5TwqnT58kldbKJzoaafTelOXLFJRnTWTMQ49JcG8OMcPBciRdfv8xLx5fYMtT52PZsSHNpISRmCBRFoWELnjpS4+6NOiM9KtcWPYZzGrPLNpW2wuP7rzFdgufOBTQcjamFKk+fdIlEo/wfX73InVs7qaju2tnHyast+nJR5gsNyo2QYrnOSycbTBd9Ls40MfAwNIU71ypcrCS4UIrRZ7VYn2oy1YhSpIe+mM/V+SpZrUYoJVIKbMfmqydVBCG6GefsvE/Ri1H1TY5Oh8zWFAzZwHCLTLWzWHZnv3w6nKdl9GMoHZJOs9lCsxLo0iapB3h6mkgijUJAYGSZWWpQrLZoG/3oXpG6NoBlmWiKwHYcasoApjNP1NIpt1U8LLxgZbn3Qlxf4btKvyylvOVN7S2LV/4wpJSL3W3fIfCX/GA36X9SZa1uePNe1VRePjRPyBASDT8UHD07Q38ugVB6WSo1cBybX71/lFJTwTB0zk/Vea1tE4tG+O6Bi9yxvZ+DF3zOXJxDCkEyGWdiNmRuqcJjr8FYXmNdb5S7d48yllfZMW7yzYM2j9w+xMZRjVRM5dDFDMcmHcKrPhFT5dEXzvHgvTuIRVQ+s2+ER1+EezZrNNshT79SxLcbaGoffgCK0uDSTJnI2gEQCrEInJsscizex6WZAkLGubAQEtV8mnbISG+cdLjERCvFUEZHD+okRI26olNtC+aNFJFknPK1EtmRMYQQCCG4MrHAUE+KwBhiYaGCrmuM9w+D0CgsVfD8gEw0TSJYZrh3BEXRiNhT1HzJTLVOImagZcdYnCt1FtipPqLOAsOZHgK7giYk3orP9Cs3zwkhBt5UrupTwBue/ceBvxVC/FtgkB8kgnlfcMMr/dbNG9iVOs/3ztl8dG9na2erPYSqaXxgq0mjmcX3bGKWQsSy6E0GpBMp7unrWAaF0ggD+Qi3bogwU8wTj2jcs0mj2oiSjut8cEeceETjG6/V+OStab530ePAuTp71icY7VE5cNGl1HD43C/vYLqscNuOXk5dLLBjywiLiyVyCRPXC4hakkrd5ZVTFX71vl5OT8QZj9VQpMeLBZVdo1EM2SKnVDjeyNObsthgzWJtG0NXJTf1VLFdwQEvAkETLwArliThTFHw8iiqQSyVYzScZSEcQrfnSMfiaH4d3StTFv1s65W4hk7MmWF0YAjZKoKvY7jL5NLDhJ5NnDp1MUjSnqUsc5hCElo9bIq2qEsNtTFNKppANyySSh0pwHYd1GgexCxSBiv2XQsBmvbeKL0Q4u+A++gsBWaA/wm4Twixi47pPgH8l9BJBCOEeCMRjE83Ecx7MpAfAyv22BNCWEKI14UQJ4QQZ4QQ/7or/w9CiGtvimXu6sqFEOIvurHMk0KIPW+61292i2NcEkL85pvkNwshTnWv+QvxI2oF/yjk0xYP3xznG6+W+fr3Cty3PYJjtzhwpsiWYY1Hbklz4KKLbmjsWZ/k+JUmYSh59niZfbsSzBUd9p+pctumOBoeJ69WGctrfOSWJK+cd3j2RI27t3Sy6SwUW4SqyXiv3gmd2dCTz5LPxllYtilV2kzOt/n9//xOenIpblmn8viLk/jS5N998wJpvU2j7dGX0fm7AzW++FKDaFAgH7Wp+zrHl+P0mS1GrWXOlWOkjTb1doCUklcmI4wZ82zLVimEOaTTCZ3lRYGin+uEq4QgEcxzYsFCJWC2HDBtZ0nIZXTp4DVLeFgYuk7c9Bu1AAAgAElEQVRSs2nbLotuEsUu4bVKnF9WqdRb2FgY7jJTdpqY6qOFbVLBIi1zgEQyRZZFGqFJKUxR8w3i7hzBCu+rFXSWYddr7wRSyl+RUg5IKfVuWrgvSil/XUq5Q0q5U0r58TcXqZRS/pGUcp2UcpOU8qkVe5PvACs50zvAPillo1vW93tCiDfe7H8npfz6D/V/mI7Zs4FOKuvPA7cJIbJ0nqK30HmCHhFCPN71in6eTmrr1+hk0Pkw8K4+0DPnLnFkqUYqbjHSF+PQ6TlePJMgEovy9MHLfPLecSpmiO85oOiAyf07o7xwqoEQBtMLdepNm7OXq9y7dz1WLMHXXjzLnbvXMlcOmZgv0267aOYoF5cCjp2dQWwf4jvHQdNUXjp0hTtu28rXnp1gYnqJxUKZf/aZnQB86O5xjp2ZZcuwyZmJKrVaAxGaBJ5PX8JgaDDPaLRB3rJp+ipDlsbxqxWsWC+qluL41QI7ByGeFLw6oZJWmwAsO1EuztTZNNZDU80QIgmbba7OVMjEkihelUwqRlLUqDsBVUdip/rRhMRqF7CNXlS3xbJjEUmmmJ8usL5HJWGEqPEUJjayXaXmq5RbDj2JJDI6CkLBqbdZqFUYHRwjKmBmeYl4VMWLryEMLyNXOIvGKiNvBZW+m9Sy0T3Uu+1HPco/AXy5e91rQoh0t8b3fcAzUsoSgBDiGeDDQogXgaSU8kBX/mU6hTPeldJv2rCGw3PH2DCgMJgxsL0h9m03eeL1ClvXDzCa1zhzrc7kXIuFUpNYZD0SeO71a+ze1MeWoRRLNYmiZLltnYquKbxyJMoD200MXeUxO45QdT64rZNHLwi3ggi5f2eClu0STd5MqFh88oENPPvKeb7z0mm+8m0NTTPQDZ3XXr/C7RtTfGRXguVinmw6xpq8yomJJvdsNDk1rbI54ZMDXrwi2DSaZWt0lum6RcLSOT/nIs0MBy8W2LU+T7kBG7I2t27pp9x0SbsTALSUftYO9WC0JiiaQwzKWZoiRyIaR2/VsdpzaEpI2VOZL5WJWCpDUR/DmSYVT6HLBp5UiYkWmGnMsIwX6WcgNktbyWK0pgDQRI7B3jRWa5IqOTb3QlOJoTeuoSp0jN8VhCJueN/1ile4UbulfJfoKO7B7qk/6prwfyaEMLuy7xe76OKNWOaPks+8hfytxvHbb4RfCoXCPzqnaRq/cGeGs5MNTk56uF7AK6dr7FgTJxPppNe/e2eevt4Mn/7ACLk41Ost/utf2opQdHJJHaFH+fQdOV69YANw67Z+Tkx6nZzvySg7R1VOTzm0bJ9kJKRWt/H8kO+esHng9kHS0YAr0xXqLZ+7b9vOvluH+cwDY/hOm9/+1BZqvk65GbBxJMWV5c5XNlfTGc0KshGP5ZaC64dYuoYhHKZrOtJIcPtAA1VRsNstHtlpkYoq3DnmoygQDasMGUUaap6WKzGFh1AVisogGRZQFQWhGYjQISNKuFanEIelBqwZiJOKR9HwKDka/WaTwMwiYwOYQZVGq0XTg4jioCoKsl3G01K4fohpagihUg2TRLWO2a+1Z2mrWYJQruyGG96x9/7nGiuq9N3wxS46IYpbhRDbgT8ENgN7gSyd7Ljw7otdvOPYp5TyC2+EX/L5/FuOdd/OJMVSiadeOYOiKIzlNe67KcuZGZ+nDle4Z2uU9QNRvvrMReaLTa7MO2wY1Pn3T00znO5mwm04nLpSZqxHpdQSvHiyyq4xjTUDMS7P2bx4osy2UYsHd6d46tAy69b0oWsqd+3q59Ujk7SckM88sof9J8s88dIEO0ZN+jMGn7p3gCcOV2m12+jYHLxQY122MyXesTHKRCPFKxMWm9JlZOBzZFYQCUokTEE5iJNOxRnPgiabLNoxzhaT9Bg1eqMBluJxtZFGOnXOXllEsZfRROdjnC07GPYiiqLQdhz8IMQxBrGcBZLBHLY5hBLpIaL6VGyB6XdIQoZboBRkML0OHTehtakHEWrqAKK1xLW5JUptBVeqNESaQEtyacEmlG9f//k9wXvIvf9Zxk8rG26la45/WEr5J12xI4T4f4H/tnv8o4pa3PdD8he78uG36P+uUCqVmZhyUYWP40EmGeHk5SJ1VyVuSi5OVQh8n8NGhJmFAnu3jzCUM9gxZrJQbFOq27x+vshctYcg8PmHVwr8y1/bzKXZEs3wB/H/OzaZfPWFIkJVQI+z/+Qisd5xHn1pHsduYQiXY+dL/O3jOifPTxM6TVobBnj9nIsMPOpNmystn7Uj/Tx96AIP3jxMqSmRMuDMRBHH8ZCkaIUO60cSlFvzTLezXJgrct+uEc5VMiBcjk3bVBo+XqqXRCxCOuGSazkkRIV8Kg1Coa1lUQwLZ2mZMD+KEwaYrsPJxQhreyq0hIkIXOq1KnaoY+WGWZ4qoaRNEmYWXwsoLDVJ92dRFYFUFLy2zXLNpS+mkE8nSJqSiN9J0+0HIb2ZHqaXaiu+tfZdcOt/brGSZa3ygNdV+AjwAPDHb8Qyu572T/KPY5m/K4T4Ch1HXrXb72ngfxVCZLr9PgT8oZSyJISoCyFuBw4CvwH8X+92nNlshh0RgyuzHn6Q4s7dSVw/5OE9cXw/5Kn91/j0faPsXGvylJPiI7emeeZEC0VR2H/R5Q9+ZTMvnrZ5cKeF5xscOb/Itw4WyWVivPS9i+QzWxBIZOhTajh86M5xSnWHtevvpVAJ+MWP7QagXG1y4NSjfPKBjQSuQ6tR5+G9GTS1U2HnpSM6hiohsBnpTbJrKOjm4le5Mp8kZ9iktTpzoaTedEjEdEa0EjUvj1svkNZrhGFI4MRotCVbhyzGrBmEEDR6BqlUBbf2LXOh1U/Em6PWMsgnDIRTxggaCD8kGctgyTKuD64vaEmBaYT4tXk29UVpSQMjKNEKomzrsbG1HHFvDhEKvMgg/ZpGWjbx4jFc1yMChGHIjJ8nF9cxNIHjr6yBfyPM5NfDSpr3A8ALQoiTdKrRPCOlfAL4GyHEKeAU0AP8m27/J4GrdKrS/iXwOwBdB97/0r3HIeB/fsOpB/xXdCriXgau8C6deG9garHNdFnh7i0Wuq6xoQ8uzDpMLDb59Uc2MVWUPHqgzMN7Ooy5RqPJNw6U+fDNaQxdxXObNNo+Tx+r8vu/vAndsBhN+Xz6A6MsV11u3xSl3vb4jYc3cn66zZGrAXfuHma83+TomU5U5zsvX+JTH76VpWKTeETh4/cM8fKpjh/0W/sXuHO9ihcIam34pTtSnJzrzFjPnbG5fcTFVeKcK8Xp0ytsyNgsh3n8MCShelT8zrhrrkIuHWdjr4IZVjlV62PZi+P7AbGIjqoINlrzNI0hRLyPwUiDtkghpaSu5BmwKmBmSVoKpmkxlLXoyaQ6a/mwTkYuUld7sGIJTE1Bt+dpmwP4foiBR0ou4Zj9KPgorXlsJcm1doaeKCT8hRU37zu17N6bkN3PMlbSe38S2P0W8n1v018Cn3ubc38F/NVbyA8D23+ScRaWSxTmAx7c1UmXpSqCzaNJvn24ThBKNg1JZhfKtG2X7x7TQAiuzFZQBRxNJzAUm+1jMf7+5UVu25onHdPZPODwzLFlfmXfMOuDkMcPVonEEmwcTfJXT85w261bEEKwa+sAX3/6PDFLMNDXw323r+N/+4tv8Mm7+4lFDRRFcn6ySl9SRRGC516/wu9/bICopbHc9JgsOMT0kEwk5PTVBUbiHsLqVqPxy5wqWaT1MvVam7pmMOdn2RCZR8QFi2KEXZl5LixrHDxXZff6XpasEVRTUC1WsN0ANRbB8mdwrDxRNUrELtI0krQbZZRYFsOdpxIMYAmbMAwpeVFCPWCpWGXN0DgaAYVSjVo7wbC/iGJA2xcYXh1NVTgz65COaQivjR3KDlX4J/kyr4fVkB2wysij0Wzi+vDiGZeo4TMzX+OAkuLK5CJN22NT/zBjQzkUofDx2zsJHlWts06/b5tJGOo8d3SRq7Nl+nozzFYkmmpxcbLM/gv9WJbOcrnO8uQybdtmYr6KODXHbMFB1zWsSJw/+cJ3+NRH7uWZ711icmqeK3MZLs44aEaUv/zmQe7ZvYYnDpdASg5fCxC6Sala44tH57h5Uz/LVRXXdbm0GFJ2syRiUYxYyIXL8wxlYiiK4KnzLiM9LU47ORRFYbZUZCmbIWV4bFubIB/x6Dc6JamaPQN4gYJbmcEzB7k2XyGf8AkjWZYKRVpunHFRohkIJqoV1g7mkBGLpCjQ1nXy6RhG8xpCCOJahIZQCZQoTaGzVK6jCUlO98hnkvSaLVzHpugahIG/4vvpfx5CdkKIYTr78++hQ+tt01kmfxt4qsv9f1vc8Eq/ZmyEod7ztG2fx15vYxkqN6+zKDV6URSVTaMx5hsug0mPi7M26ahkIKNRq7dYrmpoqsQRCT5ws8md25IkYzpnJ2p86sHtGIrk1o0mjXaafA98at84fUPj6JEEn3iwQ8D5/75xgP/x936Jlw6e5Zcf3syBV1P0JwPG+yy+/uIc993Uz7ZhwZVZhd/56ChHJ0MeXB9wUlOwG1F6jSq9VptiPoUdKAwr0xDAYsNgKBchF1Uw/SJBrg90g2gwh+IHZJLDxEyBsAuMxKMU3BR9umSiHiVBkfl2hIQeIMI52tl+4qJBNGySSvQS8UNcv0LoB0QNnUhQRPfaVMME0bCE77domxkCt40VS7BBWaBlDmO1ZxjLD6JqGo2mwQBlvMgwVjBJOt6LnJpc8Zn+vaLhvl/oOr+HgCeAP6YTDreAjXTIaf9SCPEHUsqX3+4eN7zSA1ydb3JuNuQzd2V49VLIt16v8tG9aV47X+X8VEAuZrB5LMmTh+vYjsOn7upFUeI8eXCZliv5hXv78IKAoxcbbB2WTBUlD9+a5RuvLOL7IdmUxW2bonztmWvcecd2Tl8qI6XkiWdPctvuNYyN5Lhf28pfff0I+24Z4tCFCsculLljvUU+FePZky1sr1MZNx1pMlMWXFsWfHq3xqvXVHypIFQVo1WgoRvg29RkhnXxBWa9HgrNGFmzjOa5zAe9NNsOaxMLVNu9xMx+xvQF8FsseRmaSoReZhm2Wiy4OZqeQlYsEUSHadQc4lEfgxJlo59kWCSimTTDCFHpoUbi6O48ui6Y9wzipoFhLyIUBd+usxTm6XfncbRxopE4ulOhWF7GIceoOksYrux+0zfW9D/j+FMp5em3kJ8GvtGt4Dz6o27wM/8J/KQ4fuoc335tkS1DKrWmy+mLc2wbi+J4AdtGI3zl+SkM4XDo7BITU7NcnV5m//kWz51q8+LRKVyp8u3XFgmCsLO19miVh27pBBp2rbX4yycn2TZicPxKi8Nn5zl8epGeJHz5718hYqocOnaZv33sEK+fnOKFV45y8sISV6cWOHJ6imOTPt88UGR6vkTE6HxVt22IcXw6JGJ0DOE71wTUHMHsUo2RRIsKPUz6g8S9eUp+nKipMV8LCWODtI0BEmqbcsujEPbQtCVX58qcqeVZcHt46WKL5XKNK1WL5aag3FKIaBJNSAIEYbQfwysRSonlLnG5qLNYcTB0k9OLGopQcSJDtLQeFot1ypU6806ceSdB2RY02zZ+bIyL0wVsz8c2B3H8TuJPJzoGrHSc/mefnPM2Cv/m866U8vKP6nPDz/S7dmzhg8MTzCw1eOzVCheuFdgyGsO2TTw/wHE8UDSGe01mSh5WLMu+W/pp2R6Z/J1MTJf5yL5RTlws88yBk+zZNsSzJzocd8+TLFVsDl1ucuv2PL+cuhcn1Bkf7+UfPv8P/OIje3j4njGiEZMXDlzipg19fPyOPN89ajCa8fGkwo7tWb57ZJGXj08RjW4gBBq2y9LcEobIIUIPQ2vTcHwKQQ/np8qk4gaGZjESrzPhJtjVL3GdEgnZoOQpDCcULJrUXYhFTBJUsBSH/p5eRmMtoti0PcFE3abcFKwdGObabJF01CKM9mO3mkinzkA+gamC2Z5gw9AgyBCz3aFKbBoZJEQh5nRIk/X4MKahYjQnGe0bwMTFcorkekbwmyXU9iKWDnVnZb9vof58xOmFEB+lE9Uao6PHgo4/PHm9a2/4mR4gamnMVRVu2zbILz60k7qrc9PaOJPL8C9/fRuVtsJAT4xIogfP8wmCkKdfX+L2nb186oF1vHBoibt29fPQ/TeDorPvpgQP3ZyiaXvs3jrMw3eN8b3TLR64cx2m4pJORfmnn3mI5TrMLbVotGxKhTJDvXHml5v0pwTbxhNUGg6z5QA7MPmtR9YhhOSujQapmMX6tUPs6W+xe9Cj5kf5vYfS6KbFng058ukY6zMOU94Ao2aBoaSPr3esD9/spTfqgZUlnsqSV0vM2SncAPpi0FSy6KqgFRhszMPWbA3bC8knDOKWIC5aZLQa0cwgqWABNIuyn8T0Cjh2Ew+diiOgXcRt1fGkRjlMY3mLgKDsp4j7BaQe62TmCW3SWhPX7F3x4pWdJBrqddvPCP4c+E0gJ6VMSikT70ThYVXpAXjyUJXxvggD6ZCE6bN9ROUL357i7m0J4lGDYj1gsdhmuMdk3y09HLlYI5nJYhoayYTF6GCMo+fLRCydX/3Edh47sMy3DxR4cG8/H7q1n+cPz7N+7RCqqvDQPRt54dXz6LrCr37qHp56+RL/6k8fY9+eDJom+N6ZGjeNd7Yj3LcjxdErLVStk8VHEx4X5n1iiTjZpEqpCS9fUbhl0MbQFDypI4TCLfkyz0/EyIjS9zPdJIIlbBHHMjsltcvlMrOL/z977x0lx3Ve+/5Oxa7O3dOTBzMDDHIkiMgAEgSTmERFyqJM2ZKWo5zek9O1vZ6TvK687rVlP9vX98kKVjBF0ZQoUswkwACCyDkDMwNMjp1TVVd1nfdHDyVKJkVSImhTvHutWt11qk51zXSfOt/5wt5pJqohCsUiz1/UENVZvEqaqqdSNVoIUiJsCAxnipHZCrphMVXROZsJYZdmqEtJqZjDDMUwlDoJJY9ttOKbLQSETULNU9FbMM0AOi6+lBihCLrwcIozTDhxTKcRMXBLs5dUx+5lCFV93e0dghHgxFyo+03hXW/enzh9jpLtMpF1efylcebPSzHsa/QPpzk4kCIeqhFWqzyyv8ivfGAFiiJ48dFBNlzWxe5DF8mXPYqlGifOTREK6kgEUzkXu1LGisTRVMlTu4e5eVs7391xAU1TGbg4zujYOH6tzOUr2piZGuPBFyaplCo0RQwOn88ynJaEwmFMy+LQyWGSqQQ1P8LuAyNYuuRDWzp4YHuaRS0acauhB3fV/Br377Px/BbWzS8xloaq1oymKah6nkLVws5lkLF5VLI5ktEQMX8KRYCvNjNSdYlEIhydKaKJNDI5D0PxiYdm6WmLozqzhGQdYhHiSgVHTaIqBuOzObpau3Fdl3QmT831CbV3IFSTgf5JFnY34VhdnBmapjOmIYIpQgGdibEcTYnGWj4QFHh+4dJ+2UJB0c3XP++dgd8HHhNCPE+jjB0AKeXfvl7Hd/2gX7lsMZ29Q0xlbZ7aW+PnlwZ5/kSJ3/7wMqYKghXdBk8dLDMwkub5Yzl0FfKlEsfOZbj79tWEggZBy8QX5+lqj2Pqgltv3MjQaJr3bVvA+FQOR4mSSjXxnusaeURVp05Al1y7aT5f//YeFnQ18f4t7XzuK2NMihIfub6HjasshBB856UMN17ZR19XgM5UgKpdg7rL0QtFzo8WUGQYux4FBG5dMjR9lqVdQZYlfXbIJAv0UVRFMOvWOTBl0xyBkD1MV6oVx67iqxaqtGmOBdDVAFF3lGE7wpJmSYJR8KHs+cR0G6k3oeg+zdVRPKudkDtFxY3R1hQnaDdKZ4UVpBYKYtoT5Pwoy9sFvnQwq7MkIymiZgXTmyXrJkiGTczKMKoCBVe9tE485iiw3znm++vhr2iUrgcA4810/D/mPZApOBwYqPHrd8zn4nSNgGXR1RJiIlsnYGiYhspvfnApE9MFNi6Nsn7lAj52Sx/P7hshaJmMTuZZuqiDMwOTSNViw+p5OI6NU/N4/uA0H//wtbSkQuzYdYbdB86xfnUvxbLN8TNjLJ5ncdnSBKcv5Fi/qpttm+YxmW9w0k2kyyzoinHt5e0cHaiQKTi0pYKgaFy7JsVN69uJhw26o1XWNOcZni5y50qVYrHMzuEwqbBg2G1ntGgyIzq4aaFDPBYnLdqwvCmSepGqlqJQE8hKGrcuyNVUlrcJSkRx/cbPIy1bCPo53DqYmoqhNeoBPCkIRePUK2lqGPi+j2IlsQIWVU9imhYBqgg9iOf7pEISR0vieBLTNGjVM9iBdgBEqP1toIcVP0vmfVJK+QEp5Z9KKf/85e2NdHzXD/pSqcLO0za3rovQnLDYfniGdX2NB2dvymdgwkEzQnSkgty5Ocq3tg9Tl9CcCPKBa9v5yrcP8ZUHDjKbrdB/cYqq7ZLNl7n1upU88cI5Fi/qRgjB8kWdHD12nvu/9yJTM1kW9rby7Uf3ML8jyMKeFA89P8g1KyNcvjiJIuocGqiy57zL+iUxAEzN48EXxrhieYzuVo0HXxhhfrTCDUt8Do8rfPUlh9WxGVojCo6IcFl8mhYm2XN6miNTKuVyhXIN6miYmv990QejOs6sG8MSFYr5DDWthbAo0a5MMuG14EuJGWhwB07NpBmeypK1FWL+NLbZgSJdEoZNPdBKXmnDskcpl8s4ZieB2mTjf1zIMVOLYriziPIo53JB9MoEiqJgOzXyjkCvFy+p0AUAQiAU9XW3dwieEULc9JN0fNeb98dOnGJyIsvOs0F8H3KFCidHJaqoogqF+587x+J5cb67y0YzTBIRk+f2niYe1NBViVPMMK89wXXrkuzep3L1+vk88+JpHFdh+84z3Go18+j244SCGhvWLsA4E+CKdUt49Ol9TKdLPL5zkLovmS3YHBwzUYRC3Wzj4LHzuI7Dkwdj1H3JwHAZu1rlqUNFSiWXo+emiS5LcjKtc3FygkyuyhEzTjQcoOYU2HEhQCwSZOUCkCi0iVEK9TDnR9PMa41T1zrxfZ+a7lJIF6kmOwmZgqGJLPq8Lmqeg13Jc9QPo4oC0XAH0Wgdy9RRqzaO2c5EuoSuCpKRdkbGMwR0Daulh8J0Dr1WIdQ8n3q9huEXmczXiXZ2I7wKAbvGeC1BWzSG6fn0T+ssScQRzF7y7/utmsmFEF8GbgempZQr59qSwLeAXhrEmHdJKbNzFaV/D9wKVIBflFIe+ilv4dPA7wshHMDlTYTs3vWD/srN65kND5Mu17huVZBypZtsocodG2MNkcqTQZbMb2Hz0kal2hP7ZvnlOxYzVvC5YnWCbNGl7rk8t2eAe+5cxYsHLvK+my/ngccOcut1q1g0v4XLVjRoAr7+wG7CwSCmqVMoO/y/f/Exvv7t3Xzkpm6aWtqwa3Xee918ALIll1jY5Jar5/H0rgHuXH45Z85P8J71MWazIQ4eGWBJskrJKSG6w3Qs9jg65tPFRXIygWHouNUiSa1G2fEpm1ESepHlvV24rkvEa8TTp0QbfYkauCXK5QqJoIlayxCTJUKGT7oQozfmIuwJDDNOuWwTViHgTtOa6ERRFMK1EdqTrUihoJeHCGkhAuEYevkiOmDXFIJmGMseJu2E6A1VsEUQ6TmEa1PEI01olcm5n+2l+65fDtm9RfhX4B+Br72i7Q+B7VLKzwkh/nBu/w94Df7Hn+bDpZSRn7Tvu968B1jTF2F1j8Z3dueJhnQ2LtR58lCRxw7m+ZXbe3Fsm4EJh1zJJhg0md8RomYX+ddHzrN1TZRbN7ew/aUBejoTTE7N8uTzJ1i6IMUd16/gyPFzZPNlKhWbttYEC3qaeOSpfaxd2oymaWy7ajlP75kkHjZpSZicGcxQqth0tCUpVWo889IgHW1JVixswqk1vPRP75viz35pPQemouwbMVmWKBIPagQDgn1TUVIBh2AwhG/ECKo1moMeOT+ClBJZr2E7LnVfknMtQqJMxJD4RhzFStAaKJP3wnhSkFM7WR4vgtWEY3Vgehma9Dy1QBu+76OJOn55HEeNE7R0EvUxcmo7PiqGPYGjNXID1GgnPVYO2+jAiibRVYgoFbxyhgzNNFsOZTX1tpj3imG+7vZGMJfbnvmR5juBr869/yoNvoiX278mG9gDvMz/+BP8CaL3dY6LuYKc18S7fqYfGR1n39k0oVCQVDzAsfOTqEobY5NZipUau+NhDFXnsV0XqTgeV62Zx7PHK0ykHYbHZtl3Oo4vBYV8ge89c5bcTI5TZy9yw5Y1RCyVu96zjG8+uh9N17j7/VsoV6v8wZ//C+9/zwZGporU64IXD4+SiFksXpDi3MUse48J1q2Zz95DGc47JT56R0PM0gqoPLNnmHV9Fs8dmeXkeIW45jDsdaBQp+AWmCwUiUYTHB2cYW2LA3O/4UR9nMFyBI0sTaJKUWujrqlYtcaMXy3MophRpC8x6xmOpU16233sQAcjU1lUBQKWwFDBqZYo1GI0M0lA9Tkz6xIP5IhHWgkqkgtZm0DKQpUW1XqNkDuDpikU7ApFB9RoM6oZRJUwOTJL0NKJhvy3x5H3xmb6lBDiwCv2v/AGVW5aX6a9niOAaZlrfy2exwnePP6HEEIBHgIOAjM0PPgLgeuA62mwR4++1gXe9YNe0zUUVWfLUgPLVKm5bVy5JEC6GGeeabB1eWPUlEohzo+V2bTIwPMlj+U0ls1vYtOiButtLt9J2KgTD9ZRFIt1C+DAyVM8s1NSqNQZnyqQiAVRcUkmkty47Up0fU6ptuyiKj43XbmAvUdHuPfBl1iydDF/8Yf38M0HXyBXEXzr8bPUXMHB/RfYunkZ61d1YkuLWjHHxnklnJrPbD7A1csihLxpzgdMRsuCkhpE0UwIKJwdmmR5bzO2onK6f4q+riS1UG/j/6C6TMxmqceacdwa8bAkWJtEqwua4h1o0iFbNtGDcQJBg7H0DMmeHhynjO9XSWpFAlOA9GQAACAASURBVF6JkqvR1xbGr9cRss5wps7injaKrkumkMfQdSxvBjzIkWBRs49nRrGqwyhwSfXphRANkczXx6yUcv1b+dGv0vYTPeOklB+ek77+GPBJGmQ1FeA0DSKav5JS2j/uGv8ZYhfzhRB754QrvjVXFYQQwpzb75873vuKa/23ufazQoibX9H+nrm2/rk11JtGe1sL790U48nDRYZnXcrlMscGC6yYZ1EulfB9SaFcQ9MDbFgcZSzj8fCeHLeui3D9mhhHBiscOZ9hzXyLoYkCNWlw28YkLx5Nc8WaTm7Z3EIooHDjld3Ma4sym63wx5+5h4efOgzAcy8dZ/2qDqIhnVKlRjpv897br8epwXceP4AAFi9oY/H8FqKhAFdfsZrFXUGSUYOwCa2pABMFeOyUz7rWIpt7Fab9VpbNSxIKR0n4U8TdEYLVCyxoC+HVqpSLOVa0CRQBRvki9fwFhPBZ0JXCoEZLWKEjkKdmNpP1Y0T8NKYVpjXokGQKp+bSHtdRqtN4dpneRJ26FsX3fXyrhYSSIxhJUK+VaY8KpGcTccdob06Q1ArU9CQlVydoqOh+lVIxj1e/xAQac7jE3vupl832udfpufa3VMtOSnlKSvnHUsqtc+IZa6WUd0spv/F6Ax4u7Zr+ZbGLNTRUPN8zx2f318DnpZSLgCzwqbnzPwVkpZQLgc/PncfcU+3ngBU06oX/1xy1tgr8Ew0nyXLgo3PnvmkoisL7r2xibNZhMl3izJhLX7vBZQtMzo9XeepIkWtXBFk1P8wXv3eGy/tCGLo6l6IruTjj09NqsW1VkJGxWUKWzsxMltlMmYd3TfHR25Zw9bpOTpw6hyd14rEw0YjFwMUphkdnWdjTzM3XruSFA0Mkm5qR0ud9t13F+269knzJ5Q8+ey+Hjp3n8mVN3LZ1CWcmPL7x2HlW9Zqs7ovxzV1Z+qJVNLUxoTRo5nx6zQlycxbmhN9BizbLRL5GwZb4tTL1cpp8PYIX6CDijpMuOAQsE93LoSkKrjAxTQsdB9tp+BOyJAmTJ6UXqahNqOEUYcVGDYTIaZ2Ea2MAlIpZVDNKWJSZnpnhxKxFuZgBtVG4VFVjGLWGt75JyTLrxS/9oBeXPE7/MI18eOZeH3pF+8fn1tubmeN//Gk+6KfBf4bYxTbg7rn2rwJ/RsObeefce4AHgH+cC3XcCdwnpXSAC0KIfn6gBtovpRwEmCPUvJOGXtgbxukz/ZzIljF1FVUxcT2fsakczx6PEDRVntpzkY5UiGdPWoRNH1VROXB2lvOTCSKWoJDLEwgF2XmyxJGzU0jf58HnhjGDIT735d1cf81lPLN7FFUBp1Lk1MUJvn5/gFAoyF99/pusWdHN4y+cx6tLnn7uCHfcupWzZwf5xv0+CIW+3mYGBuKsWpLi4vA0uw9VcFzJsfNTmIZK0JBUanXOTUmGChFM0yASdHnp6BDr2yW+7zNl6zhumXykG00UsAwo2jq+ZjAxW6OjycXTWihXHAKqQUVpxan5DI5Msagrhe1aePU0NdUiEpK4DtSDHUxP51EUhVq4henJMpYBWrwTUzdwc0UK2Txt4TBNVpli3SKuFPA9MLQE+XINPdZGIGCi4DPaP/U2ZOQJVP1NJa/9uGu9mpbd54D7hRCfAoaBD8+d/hiNcF0/DVP8E2/JTfyEuKRr+rnZ+CANJ8M/0SCvzEkpX9YxeaVAxfedHVJKTwiRB5rm2ve84rKv7POjzpFXDYOIhr74LwN0d/8wv0BHeyuzpVNcs1ynUK5R91rxhcbWFQaZgs2jrscHtrQSD+kcGchzz409DGfgmuUBap7P7mMlBAVu29zBxhVtjE0XeO+Vzbx4IsvVq1pZ2BlkcXcjuvKtp6tctXEl779lDfuPDHLrTVfRkkqw9aqVfPt7L7Hl6nXcesM6BofHcWsOP//BqwAo52c4PZjhY7ctRgjB1Eyec+eG+dB183j0pTF+47Z5HL9Q4rLWCmCz/ZygqyWGp6rEQgZ7RgWrO3wC9WFksp1yDZIiS67usbxVUlR0YvVxQq29GJUh8l6cqGWwpq8ZiYIua9SCKQYn8nRoKoZbxbeDzE/4uEaCQGWIaWK0hT1MdxxcCIc6ScXDGJUhMkYb3cEJPDVCQCmSjISwax7R+gRKRVD0TGJhk+lsmYr7Zn5hbxYCobw1P3kp5Udf49D1r3Lua/I//mfgbRW7AJa92mlzr/8pYhexaATp1xjPuDxztMKW5RblchWA505W+ZN7lvL8icb+SEaht80iW3Sxax4P7y3wC+/pZdXSeZyb8NmwMMC6viDnRitUPZObN7Sy98goXt0nk6vQ05WkVnM4eOwCdj3AXXdu4fyFcXbsOkbf/E5u3raefYfPcdWGFWxat4wnnj3RCI2pktuuXcCOvQ3T+eldQ/R2RvC8Op5TozmqUak3HI4j2Tq6XyOuVwmrNsWKR0+TgSIdxu0whj1NrD6ObbRghJswcIh5YxT1Tuq+R0ZpJ6TVqFbKaPY0tWoBoahUq1U6EgFiaglpJgmrNbR6mXIxT92H3tYQVcK4wsTzfTQ8PCmpeIKg7hEJKKhWnJyfQK9OEnYnsY0WSnUTIxSnJ5jHu9Q6ruJnqsoOIUSnEOJKIcQ1L29vpN/bEqeXUuZoCFRsphGjfPlx+0qHxvedHXPHYzTioD9OBOMtcY6s6Qnwjw+cIhWWKAJaIx67js+ypCuIrqms7lY5MGCTCGtUHQ+7WuTPv3qKprjFiVGfZ3adIRzUONRfIh42eGzPBBsXWQDcsjbMU7vHeXr/DFetbefIifM89PRRPM/j0WcOoQqFJ57ZRzpbJBYL8/yLR7j2yuUsXTyP1tYkX3tgJ61NFqlkhJpXZ3SySCKs0xw1eWDHIJd3NIym9lidqaLgyKhOt5VlecrmVD6GpfksjJWp6K0YoSYM1UcB+idKlEoVHKsTP9KL59U5NThN2JtE8yuYkaZGuaxapGa2Eo4miJPh1LRCxfFQvEZFXFLJUlKbqTtF4nKaEgmm3QSmM0m5VKZmdmLOrd2l0LAiMXTFR1Pg4nSZTC2AUpnE0BTqb0ca7s/IoBdC/DWwC/gT4Pfmtt/9sZ3m8LaLXQDPAh8C7uM/Ojt+Adg9d3yHlFIKIR4G7hVC/C0N5s9FwD4aM/0iIcR8YIyGs+9lX8Ebxmw6w9S0z6rFnUxkbR7emyccDLFj71luu2YJu87W8KXB4ztPs3RBK7miiqKY3HDFUm64LEyhXKNU7CZiqSxsV9h1Ms1spsDJ0TrecBWvXuf4+Ul8ReP+xzVkvc41m1dy45aG0fPdx/fxR7/7SSKhAH/8l19gYmKSr933zBy1k8KuPUcYuRjl3IV2arU6f/kPT3DHNQuxDEFmtkA6oTM0Vcf1JP/6Yo4lbSYDTicSwcjMCOGeFBllHqNjaWo+9HX2oHpFFmg6uuISfNnx5idY0JWi5Dkoik4+ncUPRlFVjdlsgZrn05lspbNZRZEeaT9JOBxFejajkyWSZhUn3ELQCjA2kyfe1UNuuoBmlzCauqnValwcmiIVtUg19VC3S0SCkpCoknYCaIEIXGLfVkPL7p0xqN8A3gcsmfN1vSlcyjV9O/DVuXW9AtwvpXxECHEKuE8I8VngMPClufO/BHx9zlGXoTGIkVKeFELcT8NB5wGfllLWAYQQvwE8CajAl6WUJ9/sTaaakgwM2Fy/NsGLp4rcvjHG6aEiqxa10hwzWNyhc2a4yEduWs7QtM0dm2I8e8ImX6zg+yG2H8lz+6ZmHtmbZsW8KFIYfGRbL4YlWdETJFtwcNxmpFC46YoO4okk9VqOU+fGWL64k0pNkErG+OLXH6OttYlf/cVb2Lv/BO+7aRXjExmiZpXh0TQ3bkghBOw7GueqK5fzte8cZLoMZqyFeIvgwNkCK5cIVsYLNAWKHBjV+dQ1MY6MS3r0UezmONW6TtIbYtaxaA3YpP0k1Bt6c8FQiHIuQ0Svki5bRMNhQvVJ8CAS7gJZx3SnUEK9VCslEkoOrZrF931UJUowYBJnmlzOIB4JEbSH6WiZh1PzCFSHCQpBZ1MzYUslUB4CQI11gQiTrA6T99RLnob7snn/M4JBGs7x/zqD/seIXQzyA+/7K9ttfuDt/NFjf0WjfvhH2x+j4Rn9qVCpm7QmDK5fE2Zfv026oPDRbZ08fyyH55pcmPG5bb1JxHD5wiNDfPi6Lmq1EMcvVoiGg+iawh2bmnjyUB7DDLC6L8SjB8v0NGs8d6rCh65tRwjBfU/3s6Cvh22b+7jvsRO0pKKEQiH+8Yvf5ec+cD2PPf0S83s6yGRLvHTwAqfOXuCjt/RRWprkmX0jOLUan77nKvqH8yyY38uvffx67r3/OeIBl6U9Me7Y1MQ3nhohrE6hGBapkEsi6FOsmkTDQZarYxzJd6AEVYJylKIzg0OAkqsRqk/SZNSoGO1ELRPbriAR5LUOYvVxhPQpGF0E8Yn501QD89BqYxTrFn1NAs9oBnsYLdKKnptmyg6SkFOY0qYa6CRUmyAasbBLOTQtSLVqo5OmQgRTSvRwCinzP+1X+eMhBMpb5L3/L4AKcEQIsZ0fJtH4rdfr+K7PyDt89CTNZo1TFzJU7Dq7j2YJWhovnglQVyy+9MgJ7rx+FXsHfcBiYDTHoaFOSoUKZy/OcuP6Fnad8nF9jecPDLJ2SQfPndKYns3x2a8Ocsu1K3nmUIGaV2dsbIqpTIVy1SMWNvh//vtX6eru5g9/5+MEAibRUACAZCLK//7KQ8xrtXh69ziqCsfPjBKLhenuSHD/4yd477Y1PLL9NKFUF08/vYNrNyxmIufR3tbEvzwzzJZlNqdmDCwDvndUsrTTYTLag+3kyRZraM1NNAVmmWIehi4xnBEQCqgmmpDE/ClOpMMkImVEsAVN03FrHgMzOdqDJsg8rhpADaUwaqOUShJfqihqmahS4WI5RJNhoykKpWqRIkHUSpaYUiRLB75RJyIb1NujboRWMX3J4/TijafhvhPw8Nz2pvGuH/RCKJwaLnH92iYSEUEyWqSvu4VrVgRJ521gOcmIxtpFUc4P5/jQrasJaAo3r+/m9/5uGLvmccWKhvKN6y5CINi63CSmB1FUla2rwwghONqfZd6WJRTdELduXcj0bIGh8S4yeYfvPPoiExOTqAp87f5naWmKsG3LWoJala0bGyHG6YxDrljj/icHuTiSZqbg8v5b1+H7PtXJcwQ0j819Kr4veG5PgBXNzveTdQ63xOgIVWhSM6StFLFIhFD1ImNukqF0mpBl4ARaqNfrTI7PYBkGbclOwsEK7VGB7k6DC46foLMlgenOIq04J4ZmmZcsIbUYuupzdkpjSU+I2XqdcFDBUQzqgQiWqnOyf4Ll8xPUlDia6zM0XSDU3YsC5IenaUkmUUWRS6pfiaCx2nznQ0r51bls1sVzTWellG8o4Pmur7K7bM0yrlndxLlJaGsK0tHeSrrgIqXkhVNVbt7USrbcGDwnR+pcc3k7Y2mXPSdn+Y27L8cTQRRF4Yn9s2xYaJIvVvDqPhdnfLaujHC4v8Rstsps1WDt4hiVapWxyRw7j2T4zU/czIplC7j7g9dRKFa55oo1/MJHbmB4dIo7b1pDZc5oe3rXINddtZKWVIyP37WVO2/dynSmihUwuDA8zeoFEcJBjdMTPk8ezHDzYo/zuRAAB0YES2N5crJR8abpBrbroWsKQcVmfkuQVEglyTRBP0d3c5ymRJhKqcC8YJmKq+EpjUhEKBzGqZbQfBulOExzzCKqu0TII7wyqaiJWZuhORElrNbQqWLaY6j5QZLxIKIyhVIawvV8uuIC1UljVoZoigURXoVLTjkvLnka7tsGIcRW4DyN/Jf/BZz7LxWy+6+OhZ1hVveoPLw3T9BU2LzU5MUTeTpbIyiKoFCqYtc8mlMhhBC0JVTOjRRZ0B4mU/SQUqJoAaJBjetWRzg8aBMOBelpC3F6MM2Du6a5rC/E8HieIyfOs+tomrvftxkhBKZiMz2b5bI1Kzh3YYqX9p1g/eoedF3DrtW5MJImGEmyoDvF5cs7+fsvPsa2LSvZcNkintszyMWRWRIRnXWLY8yWfIbG8qTCCgWvsXbNOSZhrYZTzjFRBGnnqJUyTJR0XC1CqD6DYoQoeiYEm4nUJ6A0wXQJqo5LxJ+hKKPMOAHU6gwxsjhakrLRRaeRwTWTDQlsq42eYIGxchDhZEjoZeqBVgCKRgfzzDQ1I0lB7STujxPXKhT9MDXPJ6K7lOuBt6fKTqivu71D8DfATVLKa6WU1wA300hff1286wf96TMDPLwvz6kxhURY4/j5Cc5OKDz0fD+TswVKlRrtCcG/PTXEws4ADzw7TtYNMTCS4Xt7s1iGx5cfH2JNT0PUMh4xmc7X0ZU6D+1J0z+coVZzGBjOkMmWCJgmgxfHuP+RAzy24zjrVs/nb/7hXjatW06xVOHk6QGWLWzUq3uex/Z94yzrS3Hy3BiZQoXBoUkmprL09XagaCaHjl/Aqfn8+44xvKpNxa6xayxGITPDU2ckSdlIXeiLFNg7FsT36mhmmLOzCnUlQNHopn8izYQdRKoGWdFCtqZhGhq6YVLVmggYChN5iQy24Bopxgs+hteQ5nKLaYYqcXzPoWJ1Y9sO4yWdNC0Ui0WyNQ1LVMnW4/SPFrBMDcdooyRDBJxxhqsJRC2PU5q59HF6QCjK627vEOhSyrMv70gpz9Hw5r8u3vVr+t6eLg6PHGLrSpVo0ESoOr1NdW65ehF6MMSZUZdSPcTY7BSzdpR7PrCUh585ztWblrFxcYiqHeH5/S9xel4rpydqIOH4uXE6UmHuvrGbQDCMoulceVljIOf9JCVb4a7bL8f3fbbvPMno6BQHj55nbHya9OwU/yYkSDh4/AKqbnBxqk531zw65lns3H8RJPz7w7vwUTl1ZohwzeL2NQEURZDPJugLjDGoWzx1NMey+R3g1Kl7NYrVPLZRwQ5E6WoKoFQa3vVUME4kaBKsDhME1GQnfmkKVVUx/SyZWhupqIZaHEIXEteLkavp+EYMR0LF8egJ5lCqaeZ39VIql4j4M7hWM2dHbZbOCxBXp9A6OxG+Q8CdxvZ80jWDbLGMLsK0BcpvS+69ov7MeO8PCCG+BHx9bv9jNFLeXxfvmMfapYJlmdy1pYkdR/MMz7i4PhweqnPD+hR2uUJfVwhLc7l609I5zXiFak3hrtvXcfB8mdakybYrF+P7HttWWWxdaXL5ih6aUgl0TRC2VJR6mVzR4am942xa3criboNjp0dRFIWpjMMffeYXiEVDdHa0sXrlcj7x0Zv4xY/eyA1br+BX7rkFz1dYvLCHx7cfZMniPnq727jnruup1102b1hOR08X52YbZqlUBL7vM5JT2dijorlZ4t4oVGeJBA1QVALCbmTSiSS+lFiWhe35+FJScE0CfoG46SGtZnJKC1GZoUUvUtOT5D2LvrhL2FSxdEnAitAdq+EaDaIPfJeEnMYxO7Bqk3Q3h6j5DXprK6BTtV1cDDx0ovFm1rc39lXDuvTMOT9b5v2vASeB3wJ+m0Yey6++kY7v+kH/Mt53RRP9YyWe23OWZd0BhBC8Z2OKHQdmKNbDvP+mFUxM5zg/lMWyGo4tX6h897kLbFnVRCqmc3bM4ZF9Oa5dHWFxh8pXn7jIyt4AN21o5dC5Ir4WIxELsnpJO8dOD/P0ztNcfeV6Viybz+DgCKZpcu2WdRw4Osi933mB669ZycplvQR0nx07j9DR0cZ7b93KweMX+Nf7nuG6Tb2kklG2bezCSLayf0RDSsFLEzGWR2ZYmKhRkRZuHUpaK0m9IXBpuo2EnFh9lIrRiabphN1JHL0FJdSCUS9ScODscBrXqaJKB01VUK0oRqQFA5uITDOYlqTzRUJKjbIfoOL4eHaj8k5W08y6EYIUibqj5NU2MrkSBlVOp1UyXoRQbQwhBAlLknbDb1M9/c+GeS+ldKSUfztHg/1+KeXn32h23rvevD94+ARn3AqWIbDMRlZY/2iRzFzceXh0EtUq852ndWo1h288uIdoNMRjz7n4UnDo1DgdzWEkKo8/349lmhxMRPGlyemLWeJHE4RNj0xJYemyXo6fGeNU/yxnBqc4bTURS7QRjwZZ3NfCsVNDLF7Yw67dRwgEAkxMzvLgo/0YgTA7nt/DhvWX4Xk+937rYa64fAEjYxbS9zkzOEuxWmMw7fHSvkG6mwzOVjXiepXV8RkOptupVByEHiWXq5Do7EX6HnW3RqGQp+ophLQIk5kS7U2ghzvRZYbe9iSVqk1O6SAY0BiZyiAkdLT04LsV4hGJKiR2oBPf9RksRZhPgem6AXqAwekSS3tb0UyVQK1CFYnwKnQk26nXa9hGK6oRQnUcstnSJU/IQ7zzQ3ZCiPullHcJIY7zKv8uKeXq17vGu37Qr1i6iJETF7hqaZAnDlT4zEeWseucx1UrGnzzuXIbiZYWbt/aB8B9j+lkix43bNtCvlDiTP8E126ejxCCyaJCczzIjZvbOHF+hk99dAsDF2e5cnUrf/tvh7B9hVu3LubOG5fiPa0TS7axZlkrew6e5KX9Z8lky3zj/qd4af9xIuEQK5Yv5xP3fIhde4/xmd/8JfYfPs7N29byws6dbNuyFk1TeOK545w5Ps6Hr0yw7qoo9XInIVGj05ih6IbJeybTmSKqqBMJR5iXEljOBIZwQYBmSgbsMKlgiVBnF27dR7HTmMJGNVSk4xGijF61gShtEYlVHSKjtNMkxvHCvZiVIQK+z6gXxNdDRJUKrijR1xYiWygTCpYJChc13kO54BIjTUUNY/gltMoMuBJFSaCpULuklXYC3iEhuR+D3557vf0nvcC7ftAHLBPPcxifVQgEAsRCOl2xKv3jVQbHSmxZ3cbuc0UAvvfsINuu2cDZ/jEqtsv2naf4hQ9fy8mBIYYmitx81Xx27G3IO50ZqvDhmxcQDgrOjpa4esMSKo5HcyKEqkBzKo4qqhTKNqZpct21V9DWHGc2V+HqKzexZkUfJ85cYH53O+NTea7dshlFEfzlX3+JP/39e/jmt5/j4x/czMKeVuZfnmBwdJpkucLCuE3J9snXY7SFigTtCks7I5Q9jbpUSHjTjLjtNPkN09oOdLJcHaWktWEaOpHyRXIkMTULv1ogrubJq11Y9jCdcYMaJjlPJyKzqIqg4rqYQE7rZFF8HCHCaKrENVtodscJR7sp2SYRUUBKCASj6E6BGDmydBCXFaqBThJy6u1RrVXfkIP7vyxewbjz61LKP3jlsbnKuz/4j71+GO+MBcwlhO9L4pbkb+87wWy2zEO7JqhUbXYemUI3g6TiJoYs88ATp1i7ZhULelq45fq1PPzkQfp6m1jU18Gx/jzRWIyWpiCrFyf56kMn2LiqmSOnJzl8JsODO87QP5yht1nle8/2891nBti6oZvrr1jAX//dt7g4lmPpoi46O5o5c3YQz3OxHQeB4Fd/56+QEu594EkOHz/HyNgEj28/wtJF89h9cICgVmNZXxOBaIxH9kzTHfNY0SYpeCb5ms6RTJI2dZpsyUV1Gmv5hJygrLWSczTClDA0hVod7GqFKSeMZgQZnPLI2gpOqAfXKXM6E6Ro+xQrNtP5Gq4WpVLXqRRzzPgpovUZQqZADSYpaF2E5qr3KpUKcX+SkgwxMJpGlMepeT41z0evjDDiJLBkkYRRf3tUa392HHk3vkrbLW+k47t+pk+nMzTFAmzZ0Ec0ZHHtqjDpvMOLJy4wm6timRoVB06eHyXV2kX/0AQg2L3/BFZwI2Mz5zh4ahKhBXj4hUkEkoMnx5jX1cbC7jh3r+olFIljmRrtPVFGCxMcPnmBSDRGMCBQlDqGWufo0eMUSlUGBwdQdYtbb7yKwQsTfOa3P8nUTJ5bbryKA4dO0NH2YYSsMjmd5jvf28HqxSmePRZEN5NM5Fz6nXaEahBN1XlsX46msEs5tpD0hXGSbRFcq4W67TI2kcfxLHpDFlUtSXm2SM2p0Bks41ZK9LU3gxrALA+hUWfGiNGspsnqrYStKAFnlLyrkLN1RM0h2t6Fj+TMxRnmpUI4Rit+vY5XrTLkRWlLBXFqJRysufRgSU36pNMVdHR8GQXSl/jbfufn3gshfg34dWCBEOLYKw5FaNTXvy7e9YO+ubmJgydsbr+ihQuTFQ7222xeGmLtqvnErDrNCZOpbIUbNvfS193E4gWtZHIlNOv9WKbBDddeTlOqmenJCT72/g187YEX+bvP/jrffPB5tqzr4MkXB7jq8gXs2D1IX3cvp87PcPXG5fR2hlnel6LmB8jki9x640ai4SCOqxCwIpwbGGHp0j4uX7OE7zy8ndGxSY6fHuATH7uTf/nyfXzy7m0UyjUCSp5brurmgSdO8Td/cQ9PPvIcV8+vU/MkaCuYnZxhVWIaVnVhl/LMM0YaGqd+ikK1TjPT+DUfJ9qJ9DX0WoWK1knUGSWntGNKSc5P0B0sYmspLN1EFoepWSkSSgY91tTgyi9fJC+SdMUVQqqN6RZx6hLd6kC4HlplhKVdzUhFI1Qbx/N9XLOLtniRVrNAXu1EkL7EHvy3zpEnhLgIFIE64Ekp17+WrNVb8oE/wL3A48B/p6Gg8zKKUsofFd94Vfwf896XRKNhDF1lybwImnDZeTxHc1RwxYomzgxmELrJ1o2dPLfrMJWqw/e2n+H2m65icjrLAw8/y9WbV7Jxw2q+9fAeFi/oIJmI8KmP3cRXHjxFOl+nvTnC6iXNnOqfAdXifTdfxpnBDNOzZQxD5effdzn3f3cnDz+xlxuuuYyR0TGGR6foaGtibHyKdWuX8q/3PkRHa4Pqa+s1m3hp/2naWlM4nkm1WiMQCJJKhFHCLZRsn6fOwE1rQtywqZ3zxSSWLkBv5OOfyDfTG8xiGSpSSsa8VoK1MRRNJycTBL2ZBomkPYUtgliRGCFDMlmU+JUpgrrA9k2KqP3SQQAAIABJREFUroru5pDlCcbtKEHVp0kv4epxSq6BG+ggUR8nJaYYqcaQTg7HrlDzVfJqJzFvDL1eYsKJEZaXesA3pKrf4pDddVLKy17Bkf+yrNUiYDs/PCjfEkgp81LKi1LKj0oph4AqDS9+WAjR/TrdgUvLnDOPhs5XGw0Ngy9IKf9eCPFnwC/RUOYA+KO5uniEEP+NBhV2HfgtKeWTc+3voSEAqAJflFJ+bq59Pg0GniRwCLhHSll7M/f51PYXCNazPLKvjm7oaFqAJ188zfXXrGL/sIIZCbHvyBCPRqP4ns1XvvUci5asRlVVUk1R+gdG2fHCYSo2PPn8cTatXcSZ/glMQ8UXBhdHc3z7mQFCAZXHtu/nrtvWc/D4IAu6Ynzx/t2EQiEmJjMN6epTg5Rsn4OHT3LTDU0MjUyiKiqK2lDQ2fHCS0zNpAkFLe779+9y3ZZ19M3v4s//8WE+9eGNDI+n2XR5L1/48ilWLUhwYtimVlcYKgi88iyGCke8JH2RLEHVo02f4UI2gBWooyFwXIlpGOhuY3KKmpIJL0KglEWE51GcSWOpAbREO26pwsWsTl9XFDOeYODCFOFwE24gynSmSK1m0B3RKWgdOI5NrlhFxaS5KcapIZu+ToEX7EHTPKZncgStGD8gT75UuOQhuztpMORCg+n5Od6AY+0ngRDiDuBlNqlpoIeG4MWK1+t7Kc17D/iMlPKQECICHBRCPD137PNSyv/5ypN/hN++g4YU78tlg/9Ew3ExCuwXQjwspTzFDzj07xNC/G8aD4x/fjM3uWb1CoqjFW7e0ATQIKIMrMM0dbZu6ODeR8+xZuUCbr+2G9/3+ZPPb8d2dTLpDJMzGQYuTvFzd/88zakk0UiY8bELfOrurQgh+OaDu3A9j3s+dDX5Ypmd+4cJJ+fT2Z7C8+qEYjNctryTW7at5Rvf2UMk3sY9d92IoprU67BpfSPkWqvVuHLzOipVm7s/dBOVSpULg+dYsqiXJX2dfM2pM5NzSQqTTKbMbLFGd5tFb0eEsyNlehMKpWCCenGKXUM2gb4WdKmiBqG/f5LlvUGqRpD+/hmW9DTj6L0gJALB1IVJuuICyx5hSU83vu9hVoaw6gqxcATLGUex68xriqMLD8seJ2omCSaTBO1GJKMiYWFrHCE9ZGGIlmiUQL2AXi5R8FO0paLE/AkUGk/7S4Y3nob7RmStJPCUEEIC/9/c8deStboU+CwNzslnpJRrhRDXAa/F0PtDuGTmvZRy4mU5XillkcZTqPPHdPk+v72U8gINjvCNc1u/lHJwbha/D7hzjhN/Gw2OfPhhwcA3jI7WFpa0C57c33AiPXkgzZZ1beQKDaGQRDxCT1uYkYkCxbLDTdetZc2K+fz8h66mra2D//HZ3+XRx56iUqkQiwX58Afu4KGnDvHUjkNcuWEhTYkAE9NZvv3YUf7hb/6YweFZurua2b7zGH/0f9+DqhmMjM8yv6+Pm7dtYv/hs+i6QW9PF6fPXQDgG996lJuvvxLHcahWbb5270N8+pO3cX5gmNaWJO+96XIm0zYrFrVy8uR5Pn17L1IJsP90jtH+YZbHszRrGYxAgMs6Glz4HWKEiDNEZ8LA91ys6ggL2uP4dQ+zchGzPISdG2dpm04gnMCu+XiVHL5szJR+sIOeQJaq0UHOEQRFBcd1cdEJRyJUSzlcv5FN71qdhGUWzQzhWh20m3nKIoqUklAoSNQdxzbaX53f+K2Gorz+Nidr9Yrt1XTsrpJSXk7DY/7pN1rW+hbClVKmAUUIoUgpn6UhKvO6eFvW9HMSVWuBvXNNvyGEOCaE+LIQIjHX9loif6/V3sRrc+j/6Of/shDigBDiwMzMzH843t0a/v7A10NxYpEAvW0Bvv7dY6xf1sxV63s5M1Tg0RdG2Lapl1Kpyle+tZO7PngLyWSc92zbwB/80V8QCQUYHZ9maCzPk88fpOa4bL1yJV/4+jOsW7uap3a8xPhUll/7vb/nE3ffjGkauJ5k++4LbNuyjoV93ZwbGEfXDa69egN79h3H930i4TCWFeAjH7iZz//zN7hywyJ0XePu91/N3/3zv9PTFiIVFXzmT79GqJ7h5FCRrz9ykv4z50mqGby6ZH6ToD+tEDNckkwx4rbSX0zQZuQR1VmKagsaDqIyScVoCKoqoVYCfpF6tci0F8eSeezCLGlHx6wXUBQFKjNkvRgBxSVOlrLWjPA9EkoOL9BGwVWxZKmRE+ApGNJGCIFlj5GpN4GdRdMUHMe55Ln34i3MvZeyUb4opZwGHqQxOb2WrNWlQE4IEQZeAP5NCPH3NKzr18Ul997P3di3gd+RUhaEEP8M/CUN8+gvadQFf5LX5rF/tQfTm+a9B74AsH79+h86J53JcvZCGU1VOHhyiEQqwaM7DXRV4eiZSSwrxJL5cS6OpFnS18Hewxd49MkjLFtxGU8+8xK2Y2PbVWbTGdJ5h6VL5rFw0RICpkmlHuDhZ04xNlnAxeSyyzcRjrXg1CTbXxpkenqSkyeP85u/eg8NwwWSsTBlp2HkLlsyn7/+/Jf46Idu5akdu8kXSgycH+BIS4yjJy8ihGDv/sO0xlYTDaloTo4FloKuCbbbFfxSjiNFHds3EZrBdLpIxUrSnoqQK9mMztp0t/egqgoDw7METI3W5DymswXKlSCpSA493I6lGQxdmKY51YuqOlycyNIbsPDMKHpQpZSZwWubjwCy0zlm6j7xYBPYHoFgO6ozQpkAF6ZzLO9J4io9CAQjg1P0dTVRM5qwJFzaMcL3STR+6ssIEQIUKWVx7v1NwF/wA0bnz/HDTM+XAnfScOL9XzQq7GJz9/C6uNQKNzqNAf9vUsrvAEgpp15x/F+AR+Z2fxyP/au1zzLHoT832/9EvPdNyQRrYyH2n8vzwZtWUJYW/z957x1dx3Gf/X9mb+8VuOgAAYIFIAn23sROqpISJapZUmRbLvrZfpM4zptiJ84bJ7GdOPEb23GRrC5SjRIpir0TJNhJkAQJohC9XuD2fu/u748LyXoTSaRsSUeSn3Pm4J7Znd3F7s7OzHeeeZ5FM4rZc6ydmppqbl4zh2g0QdPWS+g0alYsrGRaTQW5BR7uvHU+AKfONjBp8iyGvQMUFxVQe+wkdoeDcZXlpFIKZmsuRqORPE8O23cf5pvffJzTp47zxUfu55Evf5sDxy7T2hVEQaGhqZfmllbMFguyLNPa2sHJMxe5dfUiXnj5LaZPq+GuW+eg1Wqob2jF8eAdqJI9DPn85BflY9QNMhhMs7xaT5uvkPGGPiCGLxpFX+oklNJjS7YjZAWvzo450YE/bWFsnpqEZEEXbadAI3NNbceijqNLhIiFFfLtNqREEGNyCIvRgSXThyqRXdGn09lQha6hkgQ5jiIklYQu0k5n1MBQMIbDYMFEkEJXdu5eF+smkVYYU5CDIhS0kTaCqRvzhf/D8JEF8jzA5pEPtRp4QVGUHUKIk7y3rdXHgS8DLyuK0kV2aHvD+DhdawVZWevLiqL827vy89+121rg4sjvLcAGkXWvHcXv9O1PMqJvP6IJtgHYMmIV9LaGPvwBX9a23jCyZKKq1EQ6A/5gFJXOzeOPrORQ3VUqyvJYuXQOFpsTk16Hy+li/Cgje/afAODClV6mT5tCIBhh0ytbWbl0HuvXreZwXT2Xm3tYe8cqLlxsJJ1O43K6cLtddHb1k06nueOOm0kkUiyYN53lS+aTk5vD4199BLPJyPixo8jLy2HNigUMen2UFhewfu1KTp5rRpZlzl7sZM3yWXgDMsO+JPfePJ4rQwYu9GkodWRJP5FU9hG3xPPIlQZJxsOkZQW/lIdbG2UgpkFrMKJL+4lGYyiKQkhTSIUlQEqXVb6JaT24NQFiGAgKN6VGH3F9diTlU5zk6ULEFQM+xYE+0Uc0OEQGNS6nG49Ni1OXIK2osUpBMpFh4loPMW0BhvQgqViAlNAjmXI/EeUcSaW5broeRuJLNSOpekStGUVRhhRFWaooSuXI3xuaN/89YQV2CiEOCyG+LoTw3GjBj3NMPw94EFgihDg3ktYAPxRCXBhhE91EtnvCiGb92/r2OxjRtx9pxd/Wt79MVj//bX377wB/OqKV7+J3Gvo3jEgsxqVumD8pG1rIZBTequ3nluU1ANisWhqbe7CZNTy4fgFbDrSi02upHlNMMjbIk89uYebMrIVeIBhCo1bhdjnQ6XR4h4I4XdlZAafDzM9++QwLF2T96TZsWM+2HQcx6HU8+vA9vLBpKy++tIU1KxYyoWoMrdc62bJtL3/17cc4UneO7buPsGThNNwuB/3eCM+/eoh1t2aPFUsKDDo1Rr2WiLChNxiRhGBOSZrudB6NPiMe9TBCCEabhwngRqsWWI1aesMqIvE0/hhY5QF6kjbMIoIkBER6iUs2DFp1lrce7cEXTqCSBKlYmCQ6jCYrdgNk1CZ0Wh1qkcapCpHQ5qCSkzjEMBFVLhhz0BFHo1Lo9cfxBiLEDKVIOisN/ZAI9X0CrrVkFX+vlz4DUBTl7xVFqSbrkVcAHBRC7LmRsh+n7v0R3nvc/b469R9W3/79NPQ/DE6dricdCvPawR5M2hSXezI89vBtRKNJQpEoo4pc/MtPX2LmtDH0DISwOnOov9SGTmckFkuw79AZHDnFXGu7xrHjpxk7uoznX96JShLsOXScqvFVbHplJzIyl680s3XbDuRMBlmW2bNvPxPGj6F/YJD+AS+93V1oVAIhBBaTnpMnm9m9r44t23Yza9oEduw5giIrHDxymmmTxxCNxJAzMjq9nnQiTjJlRmhV+Lr9XIoniGS0dA2G8YUzjM3VEhBaUBTq28OUFdiJpxQq8qwUaQcIpbUkVHb87UFynB5SONDJaS5eG6Y030BKU8hgOIzBoGZIMWA2G2ns8GLT96NT6egJRanI1RLV5qBSqWlu81FW6EDWFiEnU/QNh5A8haTDXhwWIzqNBkO8A1mWcdjcKCL+8S+tRfA55KMNAH1kOcw3NEX4R0/DXTR/Fmdre1k02YUkYNepCzS29NFt8mPQa7nc1AUaI5LWTvmoAubPHMvGzbWsWFjNU6+c4L9+8jfsO9bIzauXEYulCYbC3Hfffezff4jHv/Y4p06f4e6716EoCvX1DSxfuoi8PA87d+3hC/fdyeCQnwfuXcsvf/U0o0qmMW/WRAryc9i+6xBFBS5uXT6JEyeOo9ermTKxHEkIjtadQghBY3MH/mCEM/UtRELDpCQLHT4JjcHG5EmFmAwqVJdllFiQSfZskOx4j5mq0ihVlgE6M8Vok/1EUzqsmiSdCYUSjw1jvA2AQNqI1aTBnuklRB6llijhOBglFdqYF5cjB72UwaIMEUjbMMo+1CmJcFTF+EI7aUlgTnbhk224bCasqR7SKpmUlCEWDqGWJELCTaHGS9JUAnzMZhfwmWnJr4cRDv49QA7ZaesvjXBXros/+koPcPtsJy8f6SHHpuU7jy3mcmeMVTdNZvuBC4ytmkrVhCl4cnNRqQVPbTrElatXGfLF+Ppj96HVaujv7WL7zn3MmDEdr3eQjZtewel0Mn361GwF372fVCrJN7/xNfbtO0gmk2ZKzRgmVI3hwKE6nnjqRRYtmMb4MaP45ZMv8eWH1zI46GXFokmcu9DMrOnj0GvVdHYPMmPKOCZPqMAfinHTgmk89/JevvWVOzlxqoHu7k4eu38Rew6cw2n1cbgxzZIpLo7WpxkMyTSFnMwqiqPkCw41mDAZohTZ0pwP5pGO9mLRKSQDYVJCIaqY0OhMOGKD9CYd5Bn8qJUUdh3EDXkkQ+04dDKpdBq0dgp1WhJSIVK8G8VYgDHdQzChJaZzoNXoUUUGiKsMRNQmnMl+DCqFoCofvRpUSYiG/J/Ak/5ctfSlZGfEzn3Ygn/0ld4fDHH6TDdatZ5dde2kdTk0XeuhsaWXZUsXUzOhEq1WzYsv7SAtK1jsuWToorPXy6bN+9DrtdgsBl55dSuLh0LoDQbe2r6DNWtWs/XN7RQXFeLz+RnoHyQ/P5/9+w5QkO8EOcrZM+dIppLUHT+FxaihuaWdUSUF/PinT7FiYRXVY0v40+/+ir/9sw3YrSa27jxNR2cPo8tcJJJp/uFHT+OwmThxppFgMEBXdz8nLpjIL8znuYOtmI1ajlz0odVbeOVoiqVTNHQF0xSZ07T61Sy3DQOCdEYmps4nX+lGZ5S57LeiUakxCzUY80lHoqR1OaRiQ+iJEolEyeDGnfKiF3DNZ0GWw+i1avxSDurYIGmTG5PVSEPbIOV5WgxqmZTejVHJkIkqBBQHXQNBKopd+ISHRCr0ichlfV5aekVR/lIIMV8I8YiiKL8dMYw1jxDbPhB/9JVeq1Zxy5w8Dl4M8vDayaQ1Nu5aNYGn32wiHPTy5rYdHKm7gCK0/N9/+3tUKhWxWFb44v57bgbg5Ol6xoz1s2zZMvLz8wkFQ7idLpYtW8LVq01Eo3EuXrzI8uULWLpkNqGgnwfvylJ1f/brl5g6sZy1K2tQq1UcOnqe06dOUVli4WpTB4P9vby14wharRoUhc1vHuHOW+cQi0W5fPEsX7pvIRPHaLjWqaKyqIoJYzy8tPUUV1r7+cq6CYwtNlF7cYgZo3TY9SlytQnOdMtEYjEaIx4kSdA1GAIEkquAhCLT5xuiyJbAkPCRisvk6HTo4wGCKUFM60EGBnxBLKVlhINhTAYFu16gpEK0+9NMcKfQa4ZAHmJMcT6kwoRkCx3tXioKbeiNLooUH5I2D03aj5UwEbXyyYzpxefjlRdCfA+YDowFfktW/vo5sgH0D8Tn47P3B8BoNOINxLG73Ewen0t7Rw+xeIpxY0axZvksfMEoM6ZW8dXHvsC2nQcBMJlMpDMpIpEoAJeudvOdv/g2r7+xBUVRyC/Io72zk1gsxpgxlTgcdmZMn4bZZCbHaWbpoukcqq1ny1uHWDpvPMsXVnPuQgvnLjbR19fHhtvnMWlcAQODA3zvG8uIxyKsmldMiUdDWZGNmROc+IYD/P2jk2lv7aTufB+nL/Zit2r4ryd2US7aWTG1kPpr2evrGUgwtVCh3ZsiqagJJfXMKc6Qa0gwwTpARaGdArsKG8NkZJhREEdntJLUuEhlQChpZFkmKSwYDHoi0SQVRS4M0Xa0Og0leh9ao4WEome8O42syyrj+uISZsWP2mDHofIzvsSOkCQs+FBLApfNgDBk900aij6hJy7dQPpMYC1wGxCBdxiClhsp+Jn5Dz9O1LXILJiSXba6dnEh2w9dJZlI8fMn3+TBe5YjCw0Tqsei1+touNKM0WTg7jtv5fDR02x96yBLliwF4M51d/DCCy9iMZt56AsPsmXrNgAS8ThffPQBfvTjf2fuzAkkEkle3bKXY8fPEIsnUKtUbNlxhJ7ubm5bPonFc6v4zfN7mDXBRY7TzPgKG3XnujlzqYcHb6vmUtMgdr2CWiWxaJKDTKCbbXvOsm/bIVaWB7HrZdRKjEmWPg43RJHkrEjqJHeIjcdDqFIh8swKQykTsixDOkWx3s+lQRUDvjD9SQeJZJKu4RgdER3ejJWEvhCbKkQiHqPQHEOoNASFG7fKj1qCWDyB1WbHbcwQSWtIy5DSeTBJMWLRKH7JgzXTiz3Vg08qyN74TJpkxM9AyoJR/jintN+G+NxM2QHJEa6KAu+wBG8In4++zh+AvQdqcZtVHDzjfSevtbWHXUca+N53vojFZMRosgIwbcoEvvf9f2XmzKmo1WoGh/yo1CZyc3M5d66e3t5edu3ezYL58zCaTGi0Wp5+5jlqJo7jja07CAb8HD52jgKPi4ryEgx6gVqtoulaH82tbUypymfv4UvICnR2dXOmwcH5xiEklcSuvWepqcoDctiy6yz3LSniwHk/kYSSjReYobltALWUgyodwGNI0TSQ4UjLRQySQp/NRCjoJ56WuNSXJlFQgCo2QK3PgE7x0qotABEm3yZhHPGsl9UyzcKJRkA8kUDChFFKEo/EaPf24zKpUcVjmHWQTnpRVE5QQ5Gmnx6lCJ2kEItLSGToH04grGZc6hAiPkTE6CaTjmPRKbQPpTAW5gLBT+CJf2Yq9fXwkhDil2RZqV8iS2X/9Y0U/KOv9NOnTGKw8ySLp+W8k9c3HKdq4kQS4WE2vfoWJ851YDGbRgwpPCiKxLMvbObQ4WOUlVfy/MaXmTN3LnMXLeZs/QXmzpuHVqOhu6uHs2dPYdJLrLtjFQMDPdhtZiZWj6bhajsWkxad3kA8KfNP332cw0dPsmrxOKLROC1t46mu9FA12k06nWZwKML08XbOXfUSCgbp6Akwv9qFJEls3OXlK8tdvHk6jDPRyotnJQxqQaUliFXtQKXR4cp0EsBKsTFEQleANtpJf9JCY2+C6kJT1hDDXUIqrcBIpfdJBRTrekmprSipEG1BLeW5WrR6I261njyLCqvQkBY6grKgczBMTkU+ilAx1BZARiHfqEKnkSjMNZMjvMSwotXbae0PokLBrk2T79CjiXV/AhLYfJZa8g+Eoig/FkIsJ/ulHAt8V1GU3dcpBtzAZ08IMV0I8b+EED8SQnxfCHH3iCzQ5wI2m5lcbZjTV7LCEacuDTJnWgWhYICqMYWEQjEWzZlA+agiJCFxy5qluFxObr91NXfddQcFBR5uv+MORldW8vxzz/Hgww/jG/ah0+kwmfQ89OD9TKgey5WrzcydPY2m1l6CwTA5LitrVsxj/9EGfIEEZaV5uFxurnUOcfR0M2tvns2ZywNkMjIvbb/Mw+vn4g2k8IcyrF46jfJCK5Ikse1YL3NL0wghSCkClSSozLfgMWVIauzojQa0iT76k2ZQ6dCJNOFQmIysICuCKo8glDGQyshk4mESoUGSihq/4sAsgug0AkmjI2PMY7wzQgIdehEjx2EFJYNGa8CljWOxmJhXmkFNBuJ+ZpdrGV1op9SWQW80U6wZIKguwKGOYE12MirXQnm+HYsjh9FmPylD/idCw/0cjelRFGW3oijfVhTlz2+0wsMH/IdCiIeFEGeA/w0YgEay7J/5wG4hxNM3Ks/zaUf1KAs9nT209YTp9asYV+5mUqWDy009mG0Obls9h4MHarnS1MbE6jEkEgle2fwmq1cu4dGH7mbjC8+zd88eFixciMvt5tKlixytPcQjD93LokXzqDt5jmN1p6keX4nJbOW3z29l7swJdPf009MboLG5nY7OAdasmMPBEy109frJy7Fzz+3zeP6Nc5QWezDotURiMk6HjVuWTqS+Q6atJ4RVlcRuyhIfl4zX8tYVNXa5D5GO0jYk0zMYRFiKON2RRFEgnNLilLx0x2yo1DoMIoYh1UNdj454LIpJRBlM29FrVWjkbCCwL5DBlOpDLUnY090MiwJQZHI1AXrjZlIZsFtMeEwZWgei9IQk3Go/6XSGgYgKbWIQSZLQJL1EJRuDooAidTfDQ4N09fsIJ0Gf/qil5N4bilBdN32aIYQICSGC75FCQogbGh99UPfeRFYoIPY+J59MdlFMx4e/9E8Phob9NLXHQWj5l18d4NbV86k93cH4Che/2FjLymXzeO3NozQ3NxJLpEmnorR3DTFr5nQ2vbSZWDRGLDzMqy+/wrIVy2m4dIm648dZe8et7Ny9H4vZRG6uh4aGS3R09uBw2Nmxs40tuy9SVFxEfnE5OXYDV9u8HDjWgFproKmxk41v1CFprRw914XJlstbB5t4c+9p5k4bwyvbL9A2EKLuZDvzqxyc7NSiCA0ZTFzrb8amt1HiCjFJH6Z+2Ik20UGuxYwh1U84CXF1Dq19YSqKjHhjNqR0iNJ8N7KcJBBN0RMMIbkMCOxEExmSqQRpm4OMyGrIqdMybd1e9GUl6DMhmiNO1FEfiquQXJeCTg3NSSckvfQlLVQYhxgIS0SFjvNtUcpzBSl7PjNLfPSm7VgVhQhm4OMm6IjPfPdeUZQbitB/EN630iuK8rPrnPxDM4E+jXA57Uy26Nl33s8di8opLdBQWqBjf+0FevsGMOsUEpEE966dx6n6dh68cy7/669/gXeggPVrV6PTaXjiuW184+ur6e7zsXTZMi7WX6BqwhTKy8vx+/288cYb9PT009Xdz6A3wLiqGtavX8e1tnbSaZn2a83ceftiAK5cbaX+Ujv33HMPDVdaqBw7iXCgj8XzJtPRn2D86FzmzRjNwWMXOa2VWLGiPCtmAby6t43Fs8cSC/hBlQDiCJUav6qAOYXdNAbdOFVeBhMxJuUrBGSFHLWfhKKgiDgJRcZiUqG3ulATx5AeJmAsJJ1MvCOWCZBQFVBelEOO0kFaLdPtN1PuMTHVPcSwuphwQmKy00t9t4ZL56I4DU7spjD5qiChpAOrJkVSNmHWplHSEh5DgpNDtk/oiX+2K/27IYSYD1SOkHPcgOVGyDk3MqYfJYT4NyHEa0KILW+nj+KiPy040xwgL9fGoike2nujmI1aglGJGRNLMBp1yApUVRawbtUU9hw6z4K5k7EbFbp6+vnV06/zyMP3M3HCeMx6iT27d7N8xQp27thBMpnEbreTSSeZOXMGFeVl6I02nA4HsViMvfsOM3/eLBLJFOl0Gr8/yIkzjcyYXoMkSVxo7GLO7GkMDIZ49qVdPP7l+zBac7hwpZt+b5hJNZMZ9GV1QOsu9DO2yMBN0/KoyleR0LjoT9nxhlI4NVHUkoRan53VkQwujKoktnQPEW0BUW0e+vQQduEnLLmQ5DhplQm/bMeY8mJIDRIT2QbGRw4OBkkms+cdyrhZPEpgEBnqeixMyImQiEdIZxS8KTtfma9CrTOh06jpSnmotg3jMGspUHVTH8ihbzDAiX47eerBT2ZM/zmZshsh53yH7PAbssLmz91I2RuJ3r9OdsnqVrKqtp8r9PZ7SWYMzCgzAjDk9bHtYIYlc0Zx6nwLP/3VZn783YcBMJv0dHb14XTYWTC7kq9958fMnruAN7ZuH5FiEux2n2xcAAAgAElEQVTctY+Jk6ewavVqNm3ahEat5pZb1rBz+1ts2XaAL3/5i/h8Pp59fiMzZkxHCMG8+XM5f6GRE2eu8Nij9zLoHeY/f/kia9etBSCaSDHY7+VyYysajZY9+xvQqdI8tP4OnnzqZWZLMv5Agqk1VkBH/SUL80b5eO2MoKVziKLyDKgFyUScSMKMQdUPAmRFEAhFCcczmArLyGQUBvv9aDUSWk2CRCKDzRRHoxUk9E5CkQx2UxptOs1QNMKAZKLYodAVUFAZTfQOxznWm0u5c4DdV2GsO0w6Ixhlj1PboiGTiWG2mREZLS0hGw6XmRyniYZWLya9ixGeyceMz0alvgGsJStB97YOZc+IAO11cSOVPq4oyk//gIv7VKOto4ve1i6C4SQajYae3iFa2vrRksFjVpCUBBtf243VZiOekAn4A1xoaEGr1bJ65WL0Jhd33/U7PU5fIExxWTlXr16l/sIFMukUGq2avfuPML66ihc2vUpGljl85DhajZ6zZ+uRMzJ79uxhw923sX33EWRZobGlh3P1TVy52kl3jxeXw0yO24bNaqG1tZXmpiae2rSflu4Qx05cY8mMEg43RMhkBCeuDJIsNVJoizLKY8SbVIjqHISjAXr9MqV5uaRUgOzDJKmw2yzoIu2EUxrK3FbSahNSoI2w2sAwOZgMRrr6fJgMejSJFEmVk0AyhYiCy6alwhaiK5lkxUQz5ePyCcXy6Dh1HLPJgZJWqMpJUZ5vJ52Iok8O0jys4ZFFVlymFMPhNB6DCY8hybMHPn7X2s8LDZcRcs6IGu9HTs75j5GuxC7gHf/rt5VuP+uYPaOGbs01TGYdNaP0hEMGNFot1aNMdPX6ePSuGk5c8jF5rIM8t5nzl3uIJ2B6TSVHz3ViNuvp7OyiuLiI/fsPs2DhYhqbmli9Zg1t7e2YTEZuWbuOREZmyOvl9rvvJhaLEYvHESqJh++/ny1btzFtxkyWLL8Nq9VCbW0tCxcuoqSkmOnTpxLw95OIRykuyuf8hSsU5eiIBB08sG4OL72eQokPMbfajiRJ9A9FqTcpuA1xzg+bmJk3xIVgHgVSJzGjiXhKi54w+oSftCKT1OaTSsvIsoxsyEGb6iEYTZJOqrDqEhhFgkDETCwtYxEaTITQigiKswCXSU2ZvgdZlsnPdzOjLEVtR4zVM2yMLstntD1AjkEhnZbQkELRGAgkrdw1WUVdh45VY5OEYhl0QuFop+GTeeCfke77DeD3JufcyB2YSNac4p/Jilj+K/DjDyxB1uxCCLFfCHFZCHFJCPHNkXynEGK3EKJp5K9jJF8IIX4qhGgeUcqd+q5jPTSyf5MQ4qF35U8bUeFpHin7e4kozxpnJRYJc7Y1htli5ra5bnYc66HbG8Nh1XP3inL2H2uhZyDE1a40f/n4rWzbVQtI3Lx6CQcOZi3EOnoGqBwzhnQqxc6dO1mwZDFCrWLr628wdfp07rz7brZufp0tr73G+g0bWH37Ov7pX/6NUDDEn33r62ze/DoAV5taeeCBB2i51sGWLduomVDBmIpiLl9t5cTx48yeOoolc8dyqO4yIjnM3csrOHYlO72293gvK8cpnO9Ro5ezMzij9T10pz0kVXZGW/ykkzH86gIGMjno4r3Ewz4GFc87ppNOVYBA2oxayPhV+Tj0UFngwK2LkdHlkErLWLUZ4uEhfCk9LbFcZhRlx/jGZC97Tw+wemYOHXEniqJwtFOHR+oHSYXL7cGsU6ixD3K43UAwnqbHl8Sujnym5umFEKuEEI0j795H7mRzPYz4RrxCVoPybXLO/72RsjfS0q8Fyj+scwzvb3bxMFnrn38euVl/STYgsZrsFGAlMIusacWsESLQ2yuKlJHjbBnxCPsFWYHAOrLKOqvI+nzdMPr7vZxqGEKt1tB4/hpGgwajTpBnF2w51ILOZMIXTKHT6fm7n2xj4fxZbNx6kkHvEE3tPiRJgyDNz3/xBGtuWwdAJBwmHIuysHwpGq2Wf/3HH2Cx2TCajASCAerPnstKW1uthOMJTp+tR6vVYTEbqK09Rp7Hw9DQEBaLheeffZpbVt9EKpXirZ89wZzpY3nqpUMA7DtwlD9/YAp5bhMXWgJ0e+Oo02GuBQxcaOnHadETtBeCkGjoHEKv0YCzBJVaJhaN0T8cQZObTyoZIxSIYi8ehQzIcgpfTz+KsFNhVsioHQwOhSi0mRgIJpGFk8kMoDIIOmKFjC62YdKlAKjOU/jZwV4W3lvJkskOTl+IgpRBoxIEw0lK7FmVZr1Wwhkf4Fy3HjVqyo2Dn8AqOz6Sll5k1TXfz4DlE8MIIec9STlCiGOKosx5r203UunPA3Y+pD7xiNPH224fISHE22YX72f9czvwzMgigjohhH1ERHMxsPttkcGRD8cqIcQBwKooyrGR/GfIml18qErv8biZZnPhCyZQSx6sZi3zxurw+hVOuy1kUgp3Ly2jZzCMzTYVhzuPpfOreP6Ns0xwF7H29tVs37mfQ7W7cbo9nFCp2LZtG4uX3MSLv30KT64Hp8tJxbgxCCHQGY00XLzEhgezstfxeIJMKsWy1bdw+tRJfvIf/8FNi24iHImydu0dDA4O4nTqWLVsLvUXLnDnzbPIcVmRZZlrbZ3sO34NjdaIyWLmX548TplbyzhrgOljPQz4olhTnaglQXFuIemMgj464jqjLqDIkcSuDJDR2HA6FKRkEE1qGFmWcVodTHAE0MghhiNGrDo9FaYhNClBq19HRp+DLCQ6uwJo9BrOmWygZFCrZOIZmYtBN8mMwrFrLVhVaYSthI7+AcoLSmiOObOBcr2gc6ibUR4jQ+oi4OqHeXS/Bz4yEY13DFgAhBAbyb6/n2ilvw7077fhRu6AB7gihNj5+07Zif/X7OL/sf7hd7peH9bsonDk93/Pf6/zf6DZRTKdYW99hJVTzWQUQSye5mBDlG/ePY5MMk6vN07txRAr5pbR2z/Elp1nWL1sFplMhmQySVt7Nw/fv46aSdUsX7qYhx64m0wyycOPPILf5+P7f/d99u3YRa7HQ2NDA/c/9AWOHj4CgNVi5p4H7mfXzp0sXrKUm2+9HaPZxPr1d9HZ2Ul11Xi6enzE4wkWLZjP3qNNAOw9fIEvPbgCna2A1TMdVBdkWFSTT6FVkGtREKko01wD9CsFyLKMpKSRY8MkFB3+lBarFMKjDZDQejBaLDg0MRJpQUoyEBBuSkxBYiIrw22wOHBowsQyatTWApaPkTGo0pTqvcwYn4/DqKYqT2Z6mYRVJ7PspsmU5hmZXuVg2YxReFxWJlr7mD3egxAwsyDAjLwAbq2PR+9byOwJHpC0n5yIxvWn7Nxvvy8j6cv/7Sjv905+mvC+t/NGWvrv/SFnFv/T7OJ9d32PvA8ytfhIzC4ANh/1sXaOI9vyJmW2nAiwfrGH9t4gkUiUf32qlhlTx3HwVDcNDc0UjxpPQZ6LdKqZXz7xIl/+kw3odDqeeXEbiUSCP3n4XiKRCM/89reYTGb0ej1zZ87kVF0dyUSCcRMm8OwTTyIkwdiqKiRJIp5M4vf5sDnsFBYVUVt7lMuXG3jk4QeZNGkiW19/EaNeS9moSq629tPvDbN0vpMvPriSJ367mYhvmFsmanntuJEr/UNYhQ+1JFGsH+Jcn5U8/QA2fYYBUYykVTBkukCSSMoSes2I0YY0RGvEgdWowqxWSOqsDCf1jJZ6UZtlLvntzCiN4dYKLgQspMMRRhVHyTMlOdBkYM0E6JRLefDmSew53Mi1o82sqRLkGGwcP+sn1xIiHRvmmt9DsTWK3ziO5RPdfG/POaY4/ufH+ONA5sa+LN53OdG+F2743fs04n0rvRBCKFkcvN4+H7D9f5hdMGL9M2Lw927rn/czu+jid8OBt/MPjOQXvcf+Hwr7Dh7DbTFytEUAMnvqrnLzompONsVwW7VUjXLQPZxm9fxiXA4TXb1+Bvt7+dVTm9l3pJ6li+fz8qtbUGSZy1daiMWTbNqkQq1Wk4r7OH+1EYFMIpHk/IWLFJaUEPD7WbxsKc888QR/93/+DwDr793A3/313/Clr32VwqIiXn7+eSRJQgjB4OAgVxo7yHUZ+eLDd/Gb3z6HzWLkyIkrtHcHOHulD7sqyuEWN/kuI6/VK5Q7tfSmrOh1Wnr9XViLPSRUKrq7fLhtOhRXGemMQtofpXXAx9iyMpRUlCJ5iI6wAa0jj3g8Q75JoTOsR2tyEE0GieAkHJaQ4v0MCBfzLGlAwqkMUNfqpqKmGFlW2LLrDJNL9Jxst5DByoU+hWlmJ/FEmNYrfnRWB6UVKvbUJ9CbTQwb7GQFXT8+KID80dTNDzJm+dTjg1r6/UKIV4E3FEV5h18vsoYT88maS+wHnnqvwiOR9P9hdsH7W/9sIetxt5FsIC8w8mHYCfxA/M7zbgXwvxVFGR5ZZDCb7LDhC8ANRS/fjaqqsZii9UwebaGjP4zl5slEIinmT3SQTstsOT7M1zbUcP5agGqVoKjAhckY5fblE1CrVJjMOu5ZtwSAZzelCIRirFk5H4fNyhNPv8LECVXct+FOZFnmJ/8xTCQe4/LlBqLhCN2dXTz95JNIkpT1ie/s5NLpMzQ3XCadTHH5Uj379uZis9tQqSAQirB7/3EuX+1CIyV49L6leNwOnNIArW2DzC9PkkopvAZMcMfQquNcHLaxqAyiSgSnKoS5ophMRqZM0wkakA1WEmk9IhPHJg8gDALFYMSQ7OV0v4HcUgPl1jjRVD+OYjfjirTkmyycuqaltk3hVKAArUaD0MOxi15W5id4ZXcr7rxCJk/OYVypiXRaJhFPkI6FmFmd4UpPkiMDeu5YOZVctxkp6ae1uesTCeR9RH557xiwAN1kDVju+0iO/NGh+P02fNCYfhVZ5+AXhRA9QogGIUQr0ETWEvcniqI89QHl38/s4p+B5UKIJrLRz38e2f8toJWsW+2vga8BjATw/oHsjT4JfP9dziFfBX4zUqaFDxnEAyjIy6VjIEs/OHk1xvyaXGwWNU3dUTYfGeC2BUXotWoiMdh+pIdF04tYu3wMO/ZfJD/Xgcep5sz5RgCEUPG1R9fxwotvkEqlMFusCCVFOBzmP3/+Gx597DE8OTnUTJ2KyWzmS//f4zhdLu596AskY3F+8m//isViYc3q1SiZOFXjK1ly00KErLD8pum43U4SsSj3rZ3PmNGlOGwm9h+oY0qFkYoiM+1DCjvroywYJQimtAyEBVqNhMecQdbaiadkTFIUFwMMp7PCIAazlZJcK+Z0dvyfzihIGh0BKY+Fo9LEMKJVQ0ibx6IKhYs9CmqVwGjQ8FdfX4rKaGPtkhLmT83lgXtvpqy0AKvVwt98+yEGUk66B+NsPdzGzDLICIlIQtCXdvL3X53D3trLXG7xcqTuMoWi6+Ov8GRb+uul6x7ngw1YPi3oer8N71vpFUWJK4ryc0VR5pGV210KTFUUpVRRlC9db8GNoihHFEURiqJMUhRl8kh66/2sf0aGEl9XFKVCUZSJiqKcetexnlQUZfRI+u278k8pijJhpMzjHzTU+CB4bDIXW3wUekwIITDpVfzm9YvEUxmaOkNE4ym6eoaoKHUz7I9w4nwP23fXcqX5GtMmlnPu/AW8w36sVjOSJPEnD6ziH/7pPwGZvDw33/qzv+TeBx/EZDKxYcMGDu/dS0tTEzVTpxBPJvivn/w799+7gby8PAYGBtj+1pssXTyblcsWUHf8FJcunUOnkThcW8vRo4e4dKUVWZb5xnd+hp4gnf0hxpRYqe8RyMk4Y/NUBHHSkcjBo8oqAsXjEfpSLvI1w3jMGYaSRvwxBZMSIBENYNIqjNL1MCjyaesNkmuMo1PJeEQ3bck8PE4zQghqnMOc6TUQ0RVSmGvh3lUVbK4d4lSHnjVLa+ju8yMLPU67hQ13LedIY5KBfj9GvcSKGjPnB024C4pRqyTGFpt4ctNhIn4focQn4VOd5ZFfL90IRt7lMSPv3v8waPkU4A8K5KEoSoqR6bfPG7zeYXxRDRt3XWbV0tkcvSoYXTqW6dO1TB5jx+PSs/dMO2caOskIFYmUwswpFSz1q4jGEhw+3oBWLfGtv/ghE6rHE4qkMJlNXGluZ8nyVQQCIQpKSnnl1VcxGYxYLWYu1V9AUqnYtvl1Lp47TyIc5K1tWzGbrVy8eAGjXoXbvphgMMQzzzzH0oU1xIJdzKqpQKuRWLdiLADn6xuYOCaXweEoF9uHOd/YS65RQRYejjV2UO4x0ZhykM5kiMVjBBIK19QqVEoSEz2c9pmZnBNAnZS5EDRTnOek2KLiWs8QfSEjen0uKpWarv4QwVgaWXESi4YIx4cpHZfL8YuDhGKCUDTOQHiIV7adof5SI+GYgs3mRKvVsHjJUvbtEzSr8ohF4zR6T2JJBlCOx5i/YD7zI7lEO8wUqrs/gaetIH/cftifAXxuiMi/L9xuJwVoGAyNYfKEUsZX5LD9YANrV02j7tQVplbnYzT5+MFfP8zuQxdZsWgisViCvFwnOU4TGRlumjuOXm+CMePGctvNS9m5p5aHHn4Ut8tJS3sXpWVlrLrlFsyW7HqIXXv3MXXmdMorR1Ps8dDUeJl7169haGiIjmsNCDlOaZ6asElLYZ6F1YvHY7OaaGnrIZNM4B0Oo9OpWLl4Ki19nSyenEfLnmt8+/5qdh/tZJJtkND4fDRKktHGbJz0it+GCwNLxiqk0gq+mELz2TD5HgfJIZlck44pjn6SaZnFVXaCSR35ql6iSRlTYQEgMcU1jJQDx9qgubWHNdNsmI1aBnokpk+sYtL4PFKRAVKaHAaHfMyYOp7JE0bT0nSVFQsreX13Aw9/8YuEQgHWLJvO8y/vZvVNk9i4sZ1C28cfvVcUyPzxVPr37Tp9bojIfwhOtip87YG51J1pIZlKE0uqyM+1EYokae/x4XTlUpjnYPbUcurOdfD67kssnD2WqZMquHilg1+9WMtXv3w/aqHQeLWVYX+ClStXUnf8JOVjx3H7XXdSV5ul6r70wgs8+q1vYDaauHLuPIsWLmTdnev5r18/zeFDB/nmV+7C5XaTl+ti94E6vvund7H/2FUarnZQVmDl1uUTqT3dzpt7LzJ7ahnhpJo9dd1MLFJjN6kRGh09/jQlliQ5xiQ9SQeBmEJhgYulY2S6omY8dg09CSffvK2MhDBjdniYVqTQl7RxedhEoS6AlijRjIYBpZBSbR8Fqm4u+axIQqA3O6lyJQlEFc43+RibmyGVzrD5rZOsnpuHTqXwxQdWkE5G+e3z27nW2c/Tr19gyZIlLJw7mWgsSSAQwmTQ4nJYWLlyIYca4wDvaAN8XPgoxvSfFgghSoUQy0Z+G/7bKrsH36/cjaynf/xdkfPPHepOnEVSwdHTLZQX6PnbH71BSYGVrh4vZpOGp1+tpzjfinc4yOgyDy3tQ8QS8Mq2k7z45nk6esO0dw2zees+QtE43/+nn6LR6rh27RpXmlqZMn0aWq0W39AQO7dvp3LSBFAUtrz6KgCbXn6ZA4ePcOLEKRSg5VoXWq2aN7YdYO7UUUiSRCAYpvbEVaoq8xBCEI3FUNDQN+jn7KVujtZ3cro5wInLXpZPd7G/RU2pNUGJTWYwmOHUoI0qVwSXWcVAIMXFnjRVpXpyHTqu+QRuXYxiu2AoaURtsKHXCByqMEeuSagzIUIJCY1K0OsNcbhNw0R3iOqcJMcv+WjtjVNoh607DnPHwuz1JUN9hCNRqseVkYoHOXuxlaGQip37zxCNxolGE2x8bT+rb8o6A48uL+JoS+pjf9YKICvKddNnASOLbF4BfjmSVUR2GTwAiqJcfK9ycGPd+zyy3OIzwJPAzt83YPZphDsnBzmWYsyY0aQyMuJoP51eFSqThT6/GtR6Ehk9nf1JrrQFGfInUCQ9X/nKfQx6h3G6PPh8YR64bx3d3b0MDIYYPX4c3f19tLa18dyzz2Iymjhz5iypdJqqmknk5OdTVF7OlGnTGF9dDcBgby/5ebn09gcIRZK8dXA/82dP5OTZZtKpFOcuNWE06lGr1KRkNa1tfUybNpnpMyYTDQxy15I8Brxh2vuiJFFzxmfFqAG9NYVFleZEpwRqA0aTmkMdMqsqK2iU1QirzOm+YSIaNw5nhrpzrTjHF+JwhCjxqMk1hQlGFbqjVsKZDEPhDDZ7HkLAxWvNmAxqrlxOkIjJvLRxH0ari2ha8IMfPUlNVRG3LyklJ6+Y3qE46+//Eodqj3Ds1BVy7Cq27jlLJp1lNfr8IeCTaOk/N/g6WTrwcQBFUZqEEDfkWnvdO6woyt+QXQTzBNnFMk1CiB8IISp+78v9FKFydBmFjgydvSEKch3ccssqUhnBovkzycvz8KN//DanL7Qzc+oYFs6uZlR5JSWlxWi1Gt7aXcstqxajUskEgyG27TzM41//MlcuXSKVSvMPP/gByWiMGQsXMHr8OMZNrGbtFx6goLSEZbfewuG6OvzD2dnHkrJSGq52MXf2FPQ6DTevXsL40bncc0sNsycXMWHsKMaNLmT9LdNQqfVMqBpH1ZgS7FYL921YzYHTgxR4rFRX2Ll9+SSK8hyMLdZTXWLhoXkmzBYrayZI6HVqVi+pobzUgUoNj94zl/Vr51M9Ogeb3cDX140lktbgMqQpdWvwK07KnQKHSWJhtZMxJS5mFwSY5vExudLFGI+WglwXD89SE04Iamw9yBEfeiKsnF/OmUtdTJ44li/ctZjtb73JihUrmTJ1OqVFHlYvKOOWmyoQSR/zK3XAx1vplRvo2n+GuveJdy+CE0KouUGaww3d4ZGWvW8kpQEH8IoQ4ocf/lo/fZhWlYNvoJ1/f2Iv82ZPYeHcqWzeso/S4jy0Wi3r161k4+u1PPNyLXfevhyLUce///xZ7rv7VoQQ3Hn7Sn791EZmz5qFEIKgf5jmpquUjRrFY1/9KntefwNJkpi1cAEnDx/h7LFjVFZXcdsD97Fx00vIsoxKkrh7w4O88voezCYt6+9YRo9XpqVjiOP13Tz24HK6e4d4+c0TzJk5hQfuWcmzL+2hMN+G027F6iqmvTfC9sNtzJvkwhcX7DsfYrw7iV4roZOSXOmTyS2vZOW8MurOtHPyfBfN7QP0DsXYdd5PQ+sQgzEdleVuNl/W0tgVYNDr5dSgnapyJ5WOKDnaAL0hwdFOM/PKUrQOpBlljSJJEmOsfjaezDCuxMK8PC/7j7XS7RWMKivEbDZiN6a52tiIxWxg0rS5nLvcQzAcZbDpHIvGaADe0eH7uJBRlOumzwgOCiH+CjCIrP79y2TVra6L63bvhRDfIMuc85IlwnxbUZSUEEIiS9T5i9/7sj8F8Hp9NNf3olYLWjuGeGbjDhwOK1vf3MEdt63hlTePI0mCtp4QvmEfL79xgI7Objq7+9n85mFUkkBSSVxpbKVydDu9fb2UFhew71AdT/3mCYwmI253Dnv37cVkNtHV2Ynd4USlykotL157G0898QRTa2q4cOEir2/dw5JFs3j+1cMkU4JnXjmOoqTYfuAysaSKHQcbkbX5XLrax4Hac8RjUS5dbmNSdRnHjrchpxXePBmiM6jGYLHQGLGQUjRIFoXXjreyzJJk4/YrtHX5GFM9heU3r8JiNvLcSzsxZLq5aVoO8WSaoYgg5vNSqu2ltkdLV0jHUFxLninEmS41JTkS5/uNDEQixM1ldKtB687QU9/MpY4oDpuZlgtHmb30tnfu9YqbZvDz326jID+XGdOn8ItfnGL3W3sZqx3m0mB2UZha/fFNKL09pv+c4C+BR4ELwGNkyW2/uZGCN3KH3cA6RVHa352pKIoshLjlQ17opw5ut4N55fm8dmCQX/zkz3ltRwP33/8Q4Ti43W6WLVkAgN8foqS4hAfuX8+vn36FCdPnMb6yktGjR3PixAlGd/Qxf+5U7DYrTz2zCQW475GHkCSJVzZuZPKMGSxft5a3Xt1M3YEDuF1ZvxBFgaPHjuEbGsLtdvPDH/4zu3dt5/4NWX2836Qh123ktlVz+c8n3uLHP/xHrja3s3jRfGShJRro5qG7ZtPa1o/eYKOp28vffutm3thVT8jvZWJxGJ1G4uXDQ0we6+HmmQ4i0SSllTUM+aNYzEaGhv2MKs2n91p22uyF1y+wplrF9lNaMhmYUmpgQl4EWZZ59Ry0+9NMnDWF25ZPQrO3A8nfyuzREsPBBLfNL0OORajxDOMdlqg9chibIYkyMoN0rekqHR0d6HQ61Do7Ta1dVExxEYvLCHjnY/hx4XM0pjcATyqK8mt4Z42/AYher+CNjOm/+98r/Lu2Xf6QF/qpxPGLAyxYvAiLyUhFoZ5z5+rJLyqmpb0XRVG42tRCddUYCvJzOF9/icLSMpatXMGu3buRZZnLDZf49rf/nEOHj9Pe2UVu4Sge/ZM/4cTRYwAkMhkklYQsy4R9wzzwxUfJzc9jxW23Ulk1jnlz5pKbl8f0qTUUFRUxfeYcauvOUHfiDFMnjyOTUXhq0x7W3303BQX5dHRkV3WaTQaWrljDgWONjC7PRxEwc+p4tu07T824HDbcMZsT12RerR1m6ThQZ6IkUxl21SdYtWQq994xlx17T7BtVx1LFkwmpajYtO0yC0craNQSa6Y7qO0y4dAlOdquoj5UyLoFhTzywBqiKS2SJOF0WJBshQyHMxxpjDOjQk8oY6RpUGZyTQWzK3WU/f/knXd4XNd17X93CgaDQe+9996JXgiCvZMiqUZJtuxnW7Edx7ETx35yiZPYjkucWJZjFUu2JPZOkUQhAIKoRAcIgOi99z4zmJn7/hgwT9aTLEox9WJp8bvf4O5zz8WAd/acffY5ey0PB3JSQ1hcXufZZ5/Bx9uT3JwMJBIdaZlZyGRSNAvGYpuH6vTiJyd7D9zE6OT3oQQKH6Tjp36dfmV5FY3Mk8hQH9RqDW4utvzLj3+Mk4szaZu3UFldz+2yOyQmRLM5O79DjOEAACAASURBVI1/e+FVAoNDWF9fZ++hg7z6yiv4+fkZ+emmZsm/Wc327Tvw9/enp6OTxYUFrGxtWdesc/mNE+w9coTwmGjaWu6yuLBAc0U13r6+NNXVMTU9Q35+IRMTU7x94xYXLl1HKhEoLL5DZFQijg52AAT4edPc3IK5uRl+vt4srJnQeLcPHw97vL2cuVZYT9O9ca6XdpJX0Y2liRaVQsDL1kDDvUnCIiKQy2UIgsCNwlIWF5fIK67j5q1GhkamqOoVyWszUNQlZWhaS/WYGRlRTuSEymgcEUiJ9SA5xpOyuhFkUoEj+1OpHTTBXKVCJpUwMz9H05Q5Qa5ykoJUFN2q52JBCzEJGQQF+HBoXy7FtyqwtlASHRvHudIRVCwi8vDDe/EB/v2FwFQUxeX7Jxs/mz1Ix0/9jrzaxjbMFAIvv5GPwkSOr487QSFhFBbdxNHOgbv1NajMTHnz9HWsrGyRyBXkF91Er11HFEVKi26i0a3T1tFBdW0Djo6OnDh5ApXSDA93d779jb9j59FHqKuuwsPDg5LrNxBFUJgo+PaXv0pmegazU1N89atfJSDAH4Crb19jem6JwKAgFLb+SBSWFJfeoadvjOXlBWxtLDl55jzf/PoXySsoYWFlnX97qZDMtARGZuBzn32C+YkO0mNdGOj2QS5b5NpdEUcLMxrurrLLXc+py1UszM0REhpHfHwMKcmJtLb142MzT0KIsRhnVa2la3iWYDcplX0C7tZ6LBy9mJ1f4V73OBV1PVjYOLGkNWPW4MDcZDdO3iH4hllR29RHfv0MOlFKz9ACqnEtJmbOdPf0o1CY8Pb1IuxsbRBk4Xzx2e10944hcAW5XP5Qn/cnKLxfEQQh9j5BrSAIccB7qlG9G596p8/OTKKntYq09E0E+Hnw8pvX+MyX/4rC63nsOXqYnp4eMtPS2Lp1KwAaUWR0bIxHnj6+wSpjjo3KnO27dqFSmaNdX2fvkSMAzM/P88bJk5jb2hCfkoxubY2Djx5DEATam1uwUplja2nJ8tISDg72TE9Pc/rsOTJzthC3aQq9wYCLqytpmRnMTs+wbccOzM3NGR8b49zFa+QXVrF9ey7DI3nEJyTzzFNHOHfhCtkZ8Zy9MMvI5DIONmaoVwzsixe40byKRqsjMzkUpdKU/3yrgue+8AUuXrxMVVU1O7YmUVl1h/nlVazNTbhYPMSzewLo7B4n08uUM3dWmVWPERrqTUZKKEtaU2wc/Tn6yB5eefVNoiL88bFboBctqXHeOJjLCHQ3pajTCx0K9uRGYWIiZ21tjZIyS7Kys2lrrsHTS8bE4CgIoFAoHtqzFhHR/eWE7x+EvwbOCIJwv47fBTj6IB0/9eE9wL7N3lTezuda/m2cfUOxsLREp1tndWWFiIhwRiYmmJycpLmpCQ8vT1LS06gpL6fg2nViU5IZmxin4949/AMDsLSyZHzMWJt05tQpfvDCL+nv6MTSyorNB/Zx7bxx01R7UxMHDh9CIpdTX1/PzaJiSiurePpzn8PP3w+VhQX7jh7hZmEhcrmc4595hlOnTmEwGDh19iz//MtfoNHryS+4ybZtRiXdl187yYFdaQAcPrCVkrppBIOOdc0qxe0a4uJC+bsnQ3nj9E3OXatl3/7DSKVSFhYXudfeSmiQN5996gjFDctUNI4TH2iJrZUpw7M6bnQqeOLwJrameGGqVGBtqcLGwZPllRW0Wi2WlioeObSb2s51RsYWSY1xo3VwhavVs2zPCObwlgCu5JVjMBh4+UQp3/vud2nv6GZqvI+C69UkO8+ByMMf6T8hc3pRFGuAYIzl5V8CQkRRrHuQvp/6kX5leZVT9e2YWTpzJb+ChJQsqssr2H3wAL9/5RWyszYTGhHOW6/+DqkgcPj4k0ilUqorKtCsr+Pg7ExgTDQXz57jH77zbaJjYjh37hwGBFK25jA6OEjx9Rv4+vkzNz2DHpGXf/UCkWFhFObls7a2RnNrK9b2dhw4eBCJRMJgfz/Obm4oFAqGR0fw9fFlbHSUucUFjj/1FBmbN1N0I5+W9nvIJRJkCgUDI2MsTA3xdkE1BoOIKBqQmljT2FSLo60ld8f1uIU7MzsuYqpcpbymG4lpLXKZlLGxSZYXxrhWYI5BFNHJbChpuEtKUgyTYybc6W5g/7YAVta0JEa5ciqvk9VVNRERyXi5u/DmyYuEBxs5G44/cZRHn/wiJkpL7Jy9qWroxqZuhpXFeYbHJmi4O0BKahY3bxYjlyuorawiM8yWzhnjR/FhO/1f0Jz9QRAEhGIkwYwRBAFRFH//QZ0+9U4/PDqJua03TxzdgYNTBX6x6QgSCRdPnqaxto4AX3/Gx0YxUSopL7uNVhQxVSoZHh5mYXmJkus30Ov1jI2NcfbsWUwVCkoKC1FYWbGmX8fZy5OUrEzkEil7jj7C6OAgP/3+DwgKDyMhK521tTVmNavYWdvQ1HGP4pIS6mtqyMzdwpXzF2jr7GZ4YhLRQoWlsyMBIcEcfeJx4x58jRq1Rk32oUMYrt1g7J7Izpw4lEpjiPziy6dJSgxnflnPnoPRjE6tsSM7moHhCfrG2zl0+BEEQWByZo4AXyd2bjUuT744PEFkdDyH9qZTcKuBH373bymrqGZgSkLl3REMOg1vXW7isWNBDA2NUXjzFuZm22jtzEdEyqaUzcTEuGCulDGzLCV3SwYuTvY0tnTw5rlyEhISsbe35z9/+xuOPfMl/M27kctEhNc7MTN7oFzUR4Jxnf6h3f5jhWAUoMnC6PTXMFLIlwEf6PQPLbwXBOFVQRAmBUG4+w7b9wRBGHkXk879tm9tCAd0CIKw7R329xQVEIzCmtUbAhinNmi8PjSCg/xJjTDnhZdOsik+nPbWu8jlclbXNUQkxJOwbQsxm7OQW1pgZWPLoaeeIDY1Gd+wUPyDgkjfvpW1tTWiUpIITUwgecc2vMNCCQgJJnvfHgLCw7C2s8XJx5O+zk6aauv5wje+gVQmQyqVcvXMWTJ37WR6cpLUzdnsOPoIGkEgMCWJTbt3EBgZRlBYGIHh4UhFgb1Hj1BTWUlhXh6xKUmEx8TQ1tjEzMQ4z33t73nrvJHSsKd3GH8PK3R6cHZ1JSzQHWuVhNKqe1TUdnPw4H5qa2tZXFzE1dWNpVUDWu065y/fZGduMs88sYe3bzYwv7ROcJA3WRkpaPUynjychoenB1YWKuIifZBK9ETHJpK1ZTdPP/M5tFotX/vKZ+gfWaSyYZC/+fLTFBTXIggCdU29BAQEYG1tTV9fP8GBfhx77AnK2/VIJRJAwMTkIz3GB8YnaBvuYYzENuOiKD4DRAEPlBB5mHP61zBSbr0bv3gnkw6AIAihGHnGwjb6/FoQBOk7RAV2YPxGe3TjWoAfb9wrAJjDuDvpI8HBzpIndwdx5sxlKkpvkXezkAPPPIVSpUKr1dJQVY21lQWPPfsZaisqKbxxg4yd27F3dWVsaAhEkZ1HDlNbUUlDTQ3RyUl4BgXRXF1D/rkLxKSmEJOURFVpGVK5jMCwUEYHB41zYVtbY0grlzM/O8fZN97kS9/5B+5W15B35hwJuTmsrq5w5a0TbN27GzdPD3p7epmansbN05OQyAg6W9uQSaSYmJiQkLaTotuNFBRXsinak4b2EdISAgFIiPZDo1mms3eaqMgIOjo6OXX6NFtyczn4yGP84eTbWFqo8PJwZmZ2geaWHlraejhzuYR73UNMzWv5ya+vY2Njw+cfT+f0hQIEEwe+/jdf486dGoqLS0hNisTUVMGaVorC1AKpVIqXpwvtnf2YmRunMGXl5RTezCcjLQmAR578Ev/6ajUApqbvS9f+34bIJ2ob7pooigZAJwiCJUaCWd8H6fjQwntRFEs3+O4fBPuAk6IoaoA+QRC6MVYQwXuICmwIZ2zm/5IRvg58D6PizYdCV+8gvT2rKM1tcfOJoG2sDrVaQ9fdVjz9fakoKkZpYkJwRDjFefmUFZew4/BBZiYmScrO5NWf/oLNe4wBi1ano7WpmX1PH0ejVpN/7jyDPb2o19awsLRAJ0DXvQ7kb19DL5Hw+9/8lgOfMap05ezbQ/6ps9g6O2NhZcns1BQKlRkSiYTykls4Ojpy6fRZpHIZ9XW1OLu4UFNeQWR8HC6eHrTXNwCgVKm4cKMOCxM15woHmFiQUN+vxHRcjghIrcLpHbvLm2cvUtdYj6gzcOLECUwUCorKGjl69BjXywaxtbXDxTcKhVxgy/a9aNe1yBqaaWw7T9/oOj3DY1TU3mOXvQ81NTVMTEygUgqEBW3ien4JK2oDw4P91Da0EhcVxslz13D2CMXOzo4bN/LxdHfk7euFqDVaVlZXWVhXGKnKVA+sw/ihIYoi6wb9Q7v/fQiC8D2MUnD3mUH+4R0D3LcwDlB64CuiKOZ9xF9TKwiCNUY+yTpgGbjzIB3/f8zp/0oQhONALUbZqzmMQgFV77jmneIB7xYV2ATYAfMbBIXvvv7/wYZYwecBPD09/6hNYWaLp6s/aamJ9PYPsG7nSmhcDPeamqkqvkV/dw/bD+yjo7uHyPRU2tvbiEpJpqGiiqmRYdpaW3Fwc6Wxtp6ee/dYmF/A0dsLmVzGxMQkU9PTHDh+HHdfbwDKbuQTn5PNlTfeoqe3h9s38rlfHFWQl0/unl0Unr/I7ZISfAICMLO1wdnXm9CQEDJ27gBgdW0NSwtzPAMDuHTqNAq5CXU1tZw4eRIvb2++9f0f0FlXxrpBz3e+v5f+7k62bMkB4JXXf09EbCxb9+9lYnoKeytrjhwzrvQsra5iojBlS24uAE1td1lRq7GwMGdubp6p2QV+8C8/obXuFqmbYmjt+RVbt+9gZmaGf//Vr4mOjaV9cInguEzkll2YWTli6RLGxZuVlNa04za+TMfgIAOjwzx9/BjBwUbar5d+9we2HXqM0rL6hzqnB9AbPraV+l9s6M39F94V0boChYIgBIqi+MDfRIIgpIqiWA58bWOQ/I0gCDcwqj01P8g9Pu4luxcBPyAaI+fezzbsD03oAoxiF6IoxouiGO/g4PBHbR5enjTd62ZtbY0bN8sIjoliYXaO5ppa5BYWbHvsKHOzc7h4eVJdVMyz3/xbWmvrCIqKwCBI+PovfsK6Xk/W4QMEhodz9Ev/CwFQqsyJ2pRIWk4OrU2NtG2MxFq1GplMhk6vxyckmJgduYRmpTMyNs7uZ57EOdAPmYUZj3/1r/AODkRhaoqHpycrGg0TI0YeOXNzc+bn5zG3tGT/E4+j1mnxCglicmYGR2dnLCwsWF5dY2h0lsDgIMbGJzAYDLS1d+Dg5Y2pUsnczAyO7m6s6NeZn52luqqKTakp9A8NsbK8jMFgwMzKkszduyguKeX0ucscOXYMW1tbhkdnOH/lJn/99a9TUV6Om5sbW/btQaY0JTQsHCcnJ3q7uzFRKPDw8mJuaYmvfvd5IuLikMik/O+f/4y7bUYG4cbGJrxCQti6bz8Gg/hwR3o+OLR/yOH9f0W0oij2YWRxTvyAPu/Gfdn4yvsGURT7H9Th4WMe6UVRnLj/syAILwFXN07/lHjAe9mnMUr0yjZG+/+W2MDh409z4fxpZOaW5J2/gMzUlC1PHKPwxBniMtMxGAy88sMfIZfJaK66Q1VJKfV37pC5bw9To2PIVEp++rd/R86+PUyNjNBQXolSpeKJr32F4nMXyT60n8ayCioLCllbXaWqqJjwlCRUNlaUXryCenWVHU8/YUzsvfIadk5OSKRSTFUqKguL0OvWefLrf03RidPsf+o4SgtzErIzqSsrZ2pyiqiUFEISdawtL3O7pgaXri4aG1rYc9C4SWj7nj2UlpbR0tXNoaePs7q2yluvv86Tf/UcgiBQeOVtlpeWOPbUU3h4enLlwkUsLCwIi4lBvabmtTdPsXv3btrb2wkODsbSxpGB/l6cnJ0pL73NydOnid2cgbmFBSf/8BbPfPazWNvYMj83y69ffJGc/Xuxsbfn3O/fIGNrLuYWFozNGDXzyuubOfzUccC4hm5ubv5RH+MH4kNw5NkLglD7jvPfbqgkfRh82Ij2QbEuCMLvAHdBEP793Y2iKH7lg27wsTr9fWWbjdMDwP3M/mXgLUEQfo4x7AnAOD8ReA9RAVEURUEQijFmME/yx6IZHwr942MU/KGJvuYWJkdGydqzGx9/P5YXFllbWebt19/Ays6WQ899gbtl5YRmplGSn09gYAxLy8tIJFIkchOsbKwJjInG3MqS0YFB+jq7KDh5htUVY9FTdFoK/Z1dXD51GjMLS4JjIjE1VVJy7TrJm7MpOnMevU7HndIyEnOysZybxz8uhpG+fkxNTck/eQZRFHnhBz/kiS9+gZa6eq6cPE1EXCxqtRonNzfutLahsraioq6O9pZ2HHzqqW5oQDQYuHnjBulbcii5kc/Y2Cj9/f3cyssjNimZhbU1TOVyBEFAJpOhMFdRU19Hx1A/7gH+ZOzbg1tkBP0zsxS8/BImQO/gELV37rC0tISokGNpZQVAVFY63/n7b+Hs6kpjQz3uPj401dYiCBLa7t7F2d2Ne3dbWFFr+NtvPc+WvXspyS9Ao1EjwEMd6QEM4gOF9x8ka4UgCIUYWaXejW9jjGj/EWP0+Y8YI9rP8OeRw9oNbMGY03qgzTjvxkNzekEQTmBcR7QXBGEYoyZeliAI0Rj/0H6MdcCIotgqCMJpjKqfOuC5+/McQRDuiwpIMZYS3hcV+DvgpCAIPwQaMDL7fGgora1QBnlgOT9HcGwMqft2M9LXz7mXXmV8aIiDzzxFQIyRy029uobBYCA+I4PVpSUCIyMA6GtqxtXbG6W5ivmZWZw9PYjP3cxIZzdNb1/ntX/9OcHRUQhSKbbOzljZ2JB5cD8TI6Nst1CBXiRuSzYFb53iiz/6IaP3OgmOiaK/7R5eQYHMT0ySc+QQGrWan/31N7hx+TLpu3bi4u5OwuYsNBoteecvUldezrPf/DoxaanknT7DskbDzseM8/WJmWlsXJyJy87iypsniE1KIjwxga7Oe8zMzzHU04tGBAMGpiYmaG5r4/BTx4lKTkYQBEouX2Xzvj24uLlRdPkKIYkJaKwtaGi9i1QmRaPTo9frWV5ZZmRyggOfewalrRVyExO8/ANw9nBDp9ejWVtl28EDjA2P8C/feR4/7Qq+8ZGUX7kGDzuRh4j2z5TIE0Vxy4Nc9yEi2gf9vdOCIJwBXEVRfP3D9L2PhzanF0XxUVEUXURRlIui6C6K4iuiKD65IWQRKYri3neM+oii+E8bwgFBoihef4f9PUUFRFHsFUUxcUMA45GNpMZHwsDN24TlZDK3tsraygr9zXfJ3L2DiIR4NOvr3Dp3Eb1Oh1anozKvAJ+oCCwcHBjr6+dOYRFRGWkExcfSf6+TiqvXiMpIw8HFhamxccJiovEPD8HK3hZbFyfSd25n77NPc+P1N2kovkVsVibLi4tUXMvHLzICOydHFjcotHqbmgmMj0Wv02EwGMh74yR/8/OfoLKwQK/TEZ4YT3dTCwFhoei0Gr7+0x/R3XwXQRCwsrUlLDmR0qvXMBgMuPl4Mzk+zuL8PHKpFI1Gg9LMjPC4OEzNlKRt34pPdDjxuTlYOzlx+DNPI0oELr7+BxorKpmfnWV+dpaiy1fY/eRj6HV67J2dCYmOIiQqmu2PHmHHY0cRBIHvvvDvNJRXoFCYkntgP/VV1Zz53etEpyRhojRjbGiIyqIifvybFxhpamVqZBSNQob4sMN7Pp4luw2Nxvt4d0R7TBAExUb0ej+i/XB/h3FA3PNR39+nfkfe5Ogojna2zExMojUY+Le//RZHn/sCHsFBGCQSDOvrxG/P5cYfTuARFEBLVTVJ27di6+TIzROnUJmb4+hh1NHMe+ME3kGBaNUaOurqkUkktDU3E52YSNn1G0yPTxGdvInJoWFsHO2pLClFde4SggC3rl4lISOdsaFhRImEa6/9gfR9Ro4S3fo6119/k81HDrIwN4sOkRe+/0MCwkKxd3Dg2slTJObmoFSpEExMaK6+g7W9PW7e3izNL/DGr35N7v59OLm7kX/qLJbW1mRtzqK2pJTx0VF2PHoUhakpZ3/7MqJEwv4N8o8bJ0+x+6knGB0cJO/iJZrr6vnmv/4LEokEnU7HtTdPsvOxo+SdOcu6VsuVN06w49GjSKVSzG1tqSoqYWVlBYXChNqqKpRmKlRW5lQWlaCysGBkcAilVMbp/3iRsN1bEcWHm8hD/Niy9z/5sBHtR0CFIAi/Ak4BK/eN96vu/hQ+9U4/0NvPrBQC3d0IykhhfXKKW5cuMz04xPLyMlMjo9g4OSKRy6gpvsXi/Dzll64ik8tpqW3A2tYGqUyOTqulvrwSpamS4f4BkrblMtzbR3BkJEm7d+AeEkjBqXMk7NiKUqWio6ERD39fwrPTUZqZsaLRYOfijEtQAB3VNXS3tWNta5TPriy5RUhkBG1Vd7CwtyMyNQXBIKJUmaE0N+fG6bPIZDKayivQaDTcOHWGncceYfraGCvLyzTeqcHG1haZVEZzXT0qcxUry8uU5hcSGBZC2bUbaDRquju7EBEpPHsepZkZ05PTnH/lVaysbLCytcPaxpq8s+cxUyqpKCkhIi6Ovo5OQuPi+Pm3n8c/NISam8WsLC/T19tHRHwcWw8fpPNuK/ttbJBKJARERPDGr19Ep13Hzd+PQ599BpPLdkjNVEgkkoc80n88BTWiKL4v5/xGtPrnkMFK2Xj9wTtvj3Gu/yfxqXf6hNQUCprrUJgq6L5dwe5nn2F6eJSRji4yHjnAb7/zPXZ9/hmUKhVFb53Gwc2ViKwMlOYqDKIBuakpjq4uuAb4MzU5hZ2bC2HJm5gen8DZ24uxgUEMBgOtlXd4/Jt/Q+WVt0nbv5fhe13s+/yz1OUVohdE4nKzaa+oxlmvY2VpidDEeIKSE7G0s8OgN7A4P09IUiJm5uZce+0PbHvyMcovXMHC3o6tRw+zvrJG2oG9zM/M0tl8F5cAP6xtbbl14RJ7nzmOf0Agjm6uiAKsra6SsXc3C4uLmJubk3VgH/MzM1ja2yMKIh4+vrj5+rDw1inmZ2fIPpCJysoSQSbF09cXFy9P+rp7WF9fR2qqYG11lcWlRWLT03D39QGg8Ow57nNcdjY0sfvJx7j42u+Jz8zAx88PS1sbFKYKxoeHkapU9LTeQxCEh+r08MlRuBFFMfuj9v3UOz2Ax5ZMmq8W4KCywMzcHBc/HwyIXHrxJRK3bqGl5Da2Hm54hwbjHRlOxfnLIJMSuzkbcytLKq9co+5WGVsfO0JLWSXjA4M03Coj94ljWDra0992Dys7IxmmiZkZk0PDqKwtkUgkLM7NgQCWtrZs2r2Dm78/gaWtNQm7d1B29gKpB/aiMDdny56dFLz+BqZmZqTs3mGklTKR09XQxL7Pf5bu5rvU3ixmbGCQL/zz9ym7cIV1rZrtxx9Hp9PRdaeOhrJyUnftQCqXU3btBiqVCs/QIOpLyxjq6WHXU08gkUi49LvX6WlrJyg2CrVazes/+zee+8F3kUgklF64RGttHXs/8zSTI6NMDg7h4uvD0c9/jpY7d5BIJHQ0txCTnkFlfgH3mpoJijImPLP27KLo0hWc3N2Jy0jj6htvMTs3x5RWTeChXYj/+KOHXnCjf7Ds/f94CILw/HvZRVH8wXvZ34lPvdNPjI/RVzfI2uoKXX2DONy6jSCVIDc1ZX5+numiEkyVSjR3asncv4eu+gZmZmcZ6enFoF3HxMQEmYkJ3W3tuNwuZ12j5cQvf4VvWCjrGi1egYH89vnv89jXvwZAeEoS//HNf2D3Z59mdWmJoE0J3MkroOLKNQwaLSOD/YyNypHKTTCztePyb14m+5GD3Dp3gbm5eea7ujGzMGddo2V1dZWu9nsUnTqLwsyMoitXiUtPo6awiIqbN8k9sI/lhUUsbayZHh/Hxt4e1YaentxMydTEJNYODlx69XVMzFQUX7yMZOOLqezmTYKnp0EiMDUxScmlK4g6Pc11tdg7OhEYu4iNgx3z09Oc/s1LbD/2CKYWFpx66WXWNVr8w0KxcrCnuaqanY8e5eblqywvLFBeeJNt+/dy68rbWFhZcfrUKeL37mDsTj2iKD5cpxf/fNn7/wFYecfPphiX8h6Is/JT7/QOjk74RAYwmleCwtEJ39golCoVzbfKyH7kAN21DQSlJBlDaRcn5AoFs7NzrKvVJO7ejqmZGfPT09ytryMiOxNTpZLlxUVEQeDW5StIDSIL8wtUX89Dp9cjkctw8vBgoP0ek0NDDHf1MDM1RcaxQ5hbWaE/q8fC2pqIzZnUXM+jq7UN37Aw4nds5c7la9g5OxG1ORsTUwW9La1oNRrSjxyivbqGx772Fca6eojNzWFiaBilpSX1pbcRtevczi8kJSeb/FNnkMlkSKRSOtpa8QkPwScsFNFgIGHHVqQyGdWFRQSEhrLt8WPcPHOOL/7T92grqyD1wB6W1lYY7eljfGwc9coKa6urqLVqejs6SczJRruuZbi7h/m5WdSrK/T19tLRcpeo1CQUZmas6/XYOjkRmZyEwWCg4MYNZDI5bskJwMNdp/8kUWCLovizd54LgvBTjKsDH4hPPXOORCJhpLIWv6R4grfncK+imuX5BXRralz8fHELCabq6jXMbawwUSgwt7JEt7jMvuf+FxUXjcuvdfk32f+Fz9Hf0kp9cSkRmWlIZTJSd+/CMzyMvZ95irWVFVL27kKv1nD4q19CMBiIykjH1cuTXc88xXhXL1qtFnMba3QaLRKJBINOz46nn8DUwoyWW7cJz0ojae9OWssrABhobcfCygqDwcDkwCDuQQEsr66wNDeHe2AAA51dbMrdgsramtSd23B0dyPj0H5S9u3G1s0NB1dXvEKDsbS2JvORg1RdM9Z+zI9PIFeaollTI5fKsLSxQWaqZGJwCHOVimNf+zJj3d0kZGey5MH8+gAAIABJREFUOjvHl37wPRQKBeqVZcT1dY5+5TmGurqxc3bGw98PZ08PrGxtKTh9jq1HDzPS3w/AzQsXeeLLz7E+v8B8j9H2MKvs4BNVZfdumPGAVXafeqfX6XSY6fRYu7kik8mYmZqi5up1orcak6C+kWFMDAyR8/hR2ssq6ahrwCsyDIlEQsCmeFpvV2JhZY21vT3Ls7Oszs3h5OVJ2qF9NJeU0tfSgm9kOJsfP8or3/sh46OjVF6+hkQi4cVvP49MZYaLrzfDnV1UXriCf2ICWrWajju1uHp7E5wQx2B7BxjA1tkZUzMzZsYnqCm4SWhqEmtrq9w+f5HoXGNeJ3X/Hi7+5yu4+PmSceQQJecvolGvkb53N6saDb0txr1NQx2dHP7yF8k/cQpnf19MzcwwtbRgpKcXpZkZSTu3U19ciqWDPQDxuZs5+R+/Zmx0nNriW6h1en71D8+jMxhorb6DmUrF737yc+bm5pgeHcM9OIiyvAL2Hn+C8rwCNGo1VjY2mCgU2Hu4M9Tbi6g34B0YgKenFwttnYiiiFKpfO8H9WeAKIroDYYPPP4SIAhCiyAIzRtHK9AB/PJB+n7qw/vbN4uQuNjTWVCMIJXS09GFysSEsrMX0ev16A16ZmdnyH/1D8zNTOO24IlzbjZll66gXV2jtbYeJxdn1lZXqCosJmPPTppvV2BiakJbYxP6dS2Vl99mZWUVv7BQrB3siN2xFYPBQHd7B+6BAdy9XY7KXEV9RTU2zk50trZBy12iUpKYuDRAS00drt6eCFIJet06iwsLdLW1IZfLGB8ZZXFmFrVGg1QiQZAIDHR3c6+yChNTU+qrqnH18GB1ZQWdXs/c+AQLc7M4+3oz1ttHS20DMhNTRvv6CYqNoTa/EFsHB+QKBSVXrxKeEM/C9DQmSjOkJnICY6LxCApAr9fRVltH6t7dmFtZGqONsTEsrawZHRpmrKeHkf4Bbp67wLpexz9/9esc+txn0Gq1RKemcOWV1/AKMVbZ6dfUBCcbJcEeLjHmJyd7j3EOfx86YOIdVad/Ep96p0/OTKeyow2fnAwAlmbncLSxJXa3kf+j5uoNnvn+dxhsaME5wJemW2W4hgQSmZVhrP+2tGBpdpbkA3sZHxrGyccL5wA/1CurmNXUYe/gSNzu7VRdepuonEyai0tZW15m8F4n2556nOH2e8Tv3Ebf3TYcB4YITNlEe009noF+hOZkYTAYEKVSVhcWiMjNRhAEqi9fw9LOhoC0ZEYGh7F1sCfj6CEEQWB8YIDV5VVcQ4KYn5zm8HP/i86aeqJzspCbmtLf0sqFl39HXHoanhGh+EaE4hUShKOvF5P9g0zPTDPQ14+gNMXC1gafiDD8N7Yha9VqFsbHCUmMo/T8JYLjY//r/7Ho5GlS9+3h9oXLbNq+BWcPN2wcHVEoFGQdPsBgTy8Lc3M0VVah165TXVGJ1ETOzPgEDdXVuAj6j8XpP456+o8JMmBYFEWNIAhZwCFBEH4viuL8B3X81If3JgoFgrcHg9XG2gULlYrVtTXjB3x6BqXKDGtHRxbn5pgdHsUzKACPkCBUlhZUX7xKzLYtbNq3h/obhfiFhdJV1wiiiCAIeAcGorCyYLijC4WpKVb2dqQe2kfrrXLGOrtx9vFidWWFtZUVhtvuYe/hzurSEr5hwciUpkz1D9KQV4hfQixB6cl03zG+Rwkiao0WvV6Pubk5Udu20FhQhMFgoKPiDrlPP85Edy/L0zO4+PmSeeww5ReMG4pG+wbIOXaY4KQEJnoHyDp2mN62dpRmZniGheDs4YG7jzdBm+KJTklmenKSkc5uAGSmpiwuL7G2soqJiZz4LZsZ7u5mtK8PF29vlCoVafv30HDrNg3FpcRv2czs+ATF5y7y5Df+htnRMaLTU1GozDj2xc9j5WBPyt5dhKcmwfIqoig+ZGLM/++ltX9OnAP0giD4Y6w78QHeepCOn3qnB7D19mR6YZHZvgGUZmbE795OV3Utzfk3CctKZ2luHolcRkdzC2FZ6bSWlFFfWExYRipSqRT16gottTU03akhZtsWmgqKqbl6nZD0ZASJhDO/fAFBKmGooxODXo96o6ZeEARSDuyl7MxFHL09id+Ww+UXfovvpngisjNpK6tEp1ZjaW+HvZsbw909NN8qwzM6krDMNFpvV2Bhb4utkyMSU1Ne++4/obK1oaX4NkVX3katVlN65gIlJ8+iNeh48e//N55hwURlpjPZ248EEXMrK1Ie2U/tjUJKTp4lbudWNu3bRdPNEmRmpsTmbqantY3m25VYuziRsGMbJ376C6JzsrF1dGBtfpG28mrCUozUVzK5nOKrxulMS0UVy2trTAyPoDRXEZqSRGPpbSaHhvCPjmRheobCsxfwTkrAxccLURQfrsKNaFyn/6DjLwSGjXD+IPBvoih+DSP3/QfiUx/ez83MMtnagk6tpuzkeVwcHVGvr9NYVIJ3UCDV5y8jUSpZ1+nxCwtltL2TxopKDAYD2uUVhjs6UVpb4+zujo2zE0Pt92irrUeQSmgquoVbWAg2jg54REUwPzXNrbMXMKzrGOzuwcTUFNFgoKm6GnMrK5amplleWKCnsgaJVIZWr6enth6luTk6jRa9Tsfti1cIT9mEnbsbZVfeJjg2hoXJaSQKEzRaLcFpyZiYmjI1OoqFox1hmen/9bf+5ze+TVdDI5P9A3S3tBGZmUJ3QxOr8ws0VlVjaWtDe2kFglRK0eWrBESEMj08hlQm5crvf0/mju20lJUzNztH3Y0CLBzsqC29TUh8HDV5Bawtr6DWakEiIf3wfkwUCrra2jEI0Hi7HP36OtdPnSV753ZqC4qYmZllYGgAnUKObm0NiUTysUhVf0KwLgjCo8Bx/m/xzQOFSZ/6kd7GzhbH9E04ZyTj4OaChZ0t4ZnpeAcFExATReLBvcTvyMVMqcTBxxtbdze8IkJx9vAgJDuD8JwsRjo7ST68H7lMhsfGHNndz5eILdmYqMyIzd1MX30jHkEBpB85BCJ4BAQQvnUzvsmJuPv74ZsUj5mTAyGbErH2cCMkJwO5iZzQ5E24RoYTtn0LeoMB/8hwYnZsxdrVBbmJAit7O2L2bGd1ZYWso4cY6+xmeX4eBx8vFmfnWZk3TvHu3q4gMjONgE2JRGzNYXpygnu1jZg7OWLmYE/Cti24+/sSnJ2OV1w0kUmJuPn5k3R4H2E5WVhYWxO3bxcqCws2HzuEW3gwrqHBTE9PIVcqiM7dTPKBPYgGA4f/6kuMdvcC4OjijJOLCxEZacxOThKVlkx4diaROVmYKE3xCg7CLzOFqTljZaFE8vA+kiIiBoPhA4+/EDwDJAP/JIpi30bV3hsP0vFT7/QAKzOzTJWUE3fsEHKFCaIo4hEWzPjQCCsLCwx3dGHn40VgUgIjre0IOgObn3mC6vOXqTh/mdC0VExVKtTLq9RevU7i/t24R4TS39RCU0ExXpHhLG84X0txKcGpScTu2kr3nVqaCorY9YVnGe/sZqp3gKT9u+lraEa3vo65lSXRO3LpuF3B7TdPEbd/Fy4hgcyPTTAzNMz2Z5/G3teXyjMXkAkCPpHhjHX20FBQjHdUJAl7d9JSZKTEXpmeJmHHVqb7B2gpuc3eL34eCwsLJBIJ453dBKZsYt1gYGV+nsrzl0g5cpCF2VnWlleovXoN79AQKs5dInr7FgIT4hhoaaOztp4nvvUNJCYm1OUXMtrXh6uPN04ebqgXlyk9d5GYLdmo19cpPn2WiPQ0UvbsZLizi1tnzxO/NQcTQcK925VYhQQibuRCHho2quw+CUt2oii2iaL4FVEUT2yc94mi+KP77YIgnHu/vh83772tIAgFG1z1BYIg2GzYBUEQ/n2D275ZEITYd/R5auP6LkEQnnqHPW5jrbJ7o+9H+rT09/ahbesk6vBepHIZaolAxbmLeMdHE7dvJ835RfQ3NuMeGgzAmlaNVCJhdmwMJBJaqu7QVV3LnQtXqcgvxNzKmv7GFlz9fBm8245UBIVSib2PN71NLeg0Ghy8PTG3tGSspx97NzfkCgXjPf3Y+3gBEL9/F9dffAmDXE5b4S3qSm+j1WqZ6h3ANzaa8XudLI1PYuPmwkBzM4JUSl9nF9XnLjM1PcXa4hJyxYaUtKcnTUWl2Ht5IJFIUC+voF5cwtLRHht3F078+GfodDoqz11GvbrCq8//I5ZOjkz0DxKclsz1l17F0tGRgXudjA0OMjU6hnp1FYNUwsLYBCvLy0wMDTPSP8ipX76ATq9jXaNhqKcb9wA/OmrqmBweoqu1lf67rUwNDFF5PQ/Nuo764hLQ6akrKmF1gz7rYcKAiHaDF+FPHZ8QvO9GnYc5p38N+BV/rLjx98BNURR/tCFc8fcYGXB2YCQUCMDIdvsisEkQBFuMjDvxGFdc6gRBuLzBN/YiRobbKowKH9uB63xIzE1NM6dZYaC0Ep1Gi3Z5lcHWdkwuXEEqlTE9OopmdQ2bW2WIgFJpRsPtCmw9PZCbq/AJCSLhwG4mB4aYX1rEMSgAuVJJ2fnLSASRzrY2VLZWSAQJeecvsSknm+a8QiQyOV2trUxPTKBdXaG1vh4rRzsa385jXaNhuG8ARz9fwnbkollbQ6ZQILdQcefMRVqqqrF3c0NRcpvQzRkozc0xuVFI+PYttN2uoOZ6Pi03SxBFEYNOT+nVt8nas4u7BUWUXb9BbFoaNdcLEGQS3Px8idm3E0EQmJucYrRvCM+YSGbHJxgZHGR0YBAbN1fsnB2xsLZGKpPT09jMcE8vq4tL+MRFk7x/N80lt9Gua3ENDabk/CXuNd9FYa4idnsu44ND2KyvE7klm4mhYSbHx9m0czu+UeFIpVJkeflYeLg+1NAeMI70+r+MkfzPgPdNXjxM5pxSYPZd5n0YOerZeN3/DvvvRSOqMJJeugDbgAJRFGc3HL0A2L7RZimKYqUoiiLGL5b9fAREJyYglctxTU7AJzcTax9PPAL9STpykJh9O7GytcUvNISAzFSUDrao19aIzEjF0sEeuQBhWRkMtbbTW1NH1uNHmezqwdbFidRHDqAXISAijOCsDObnFkjcvR07L0/CtuYQsjmD2Iw0HD3d8U3ehJu3F3a+PkTszGV1ZYXj//xdtItLiKKIiZmS5fk5HLw8cY0OJyAuFgTQabXotesAaDUaVhYXUc8vkvn4EVT29gRmpTE9Mc7m40+gcnVG7mjPpj07sfP2IH7vDmQIhG7OYKTdyEx7r7gU/8Q4dFotXqHBaOcXeOz5byFHwNndHZcgfwwaDfaenngHBeEZ4I+zjzeiwQDrOjxCgtGsruLg6YFXSBBx23MREbFxcSZyaw5tZRX0NDTxzA+fZ7C9naXZOeM+BEGgs6zqoSfxRD45O/L+O/i4s/dO9ymyRFEcEwTBccPuxv/Lb+/2Afbh97C/J/4U7z2AY3YqfSVl+OdmoRubwMHHi7nxCdoKikk8epDGa/ncuXgVO1cXgrPTUS+vcOutM9i6OBPl4UblybM4+/siCAJL8wuIokhjXiHB6cl0VdbQdrsct2B/nIMCaLxWgLm9LWP9A7iEh2Dj6kLdhSv4REUw2NhMb209Ubu3I1coCMhIpubCFVwC/LFwdaH28jVUFhYkP3qI9vwiArdkcTf/JjIDjI+MsHr5OpsePYwgCJS/eZruunriD+1Daa6i5e08tBotcQf3UH36PON9A0Tt2oapyoz2/CLMbKyx9/LEOyGWntuV6Nd12Dg7YWZliVNIAG23ynEJD6Xueh7iup79X3uOdY2GjooqZkbHSXlkP1KZjHul5SzOzLHts8e5d6uM2alpUg8b25oHhlAolagsLUl/9BFunzhDQHwMXXX1+O7KRXzYmfWPjznnfwLe9xv0f8qS3UPnvQd+CxAfH/9H101PTjI81IuJmRmtV28QHhcPMgln/uVnxGWm01FYwlhvP0uzs3hHhDM7M4OZjQ0KlRL1ygrtpeU0VlQTK5UxOzWNZ3Q4lecu4ezrDVIpffc6MLS24xEayMz0DN4JMbQWliAANk5OdJZW0NvWgZnlCEuzcygtLbGsa0Q0GBANIg1FJZjI5SjMzGgpqyRpey4rc/MsLy4hCAJecTF0lJTR39aBs48XlWcvoNfrGezoRBBBdi0PExM5DSVlBMVGc/d6IQa9gb6ODmxcnHCPCmdhfp7FknISjx5AEAQ0y8v0VN7BPzmBtoISJkdGWFleYXl5CZmZkqn+IZoKS9Ctqam9VUpQbBSLM7NY2trSWl1D9mNHkclkLM8vYGFliXp1lYGmVlZWVxnq70d2VY5Br8fE1JSzL/wnOlMFyz0DD/pZ+cgQ+VQ5/d+9X8PH7fQT92mwN0L0yQ37+7GEDmNk1H2nvWTD7v4e139o2Ds64uZuz2xrO11l1SgMYO7shI2jA2G7cpGbmLC6toaTmyuJR4xO0d90l/DMdIaaWwlMT2Zlfh6luTle8TEM1DfSUlGFbn0dy5Vl3Hx9sLK3IzArjcWZWWZHxpidnmZxdg6H0CDsAn0JB0StlrmZWaQSAf+s1P8KdZcWFzCzt0MnQNSWLMwd7emqqmGsr5/8F18hMCGO2AO7MVWaAgLhu7aiXlnFxtERg1SKk5cHNh5uiBIpZpYWBGSkUHPqAvF7dyKs62m9Vc7C9Axz0zOYXS9AFEUaSm9j4+iIhacb/jnpzJ46j2eAPz4RYSz0DRK9JZv5gWHCcjJZnJ9nbXmFwc5u5sfHmRifoOLKNTwD/OhobkamULCqXSciK53ZsTGsbK2J3rkVgOmREQwC6EzkuCXEPvzwfqPg5mFDEIRHMMqshQCJoijWvqPtPWWtBEHYjrFgRgq8/M5M/Lvu3cKfHuAiN17z3++aj3vJ7jJGjnr4Y676y8DxjSx+ErCwMQ3IA7YKgmCzkenfCuRttC0JgpC0kbU/zkfkvQeQymUYZuaI2LOVgOQElicm2fbc5+gtN4oqmlta4pOayGBjCwCzff24hgQRsiWLu0W3sHR2YnFuHqlMxtz4JFmPH8EtJBCv8FDsPN1Yml8AwNbZiXX1GiEpmwhPiMcrPJSOW+X4pyextmwkkPRKjGOowShW0pxfTEB6MmtzcyyPjBGZm83w3TZCczJx8vTA1skRez9vEARkKhXrwMrcPC1v5+GbmkRQejI9lXdouHoDv7Qk1AuLdJRW4JMUj2dUBMvz83hGhGBub49XSCAem2IJ2bGF8OQkXP39sLa3R6fTYWFnx5p6jZmxcazcnLF3d2N5aYm5sXFsnB2IzMlGotMTuCmeLY8fxdHLHVt3NzwCA3Hz9SUsPZnaq9eI2bUNlb09s6OjzE1M0nOnnrjdO9CvrDFYVfvQs/fix5e9v4txp1zpO43CRxNqfTd2Y9yMc2PjeHzjuAacfZA39zCX7E5glN4JEgRhWBCEzwI/AnIFQegCcjfOwfiGezHK/LwEfAlAFMVZjGIBNRvHDzZsAF8EXt7o08NHyNwDiAYDo3klhB3YhfemeEbbOlCZq1BaWjA/OcXIvS4s3F2wc3NlZmCQ+qvXCcxIBUCuMKH80tuM9PVj4e5C/m9/h2dkGD4xUYzc66ThynW8E+MI3b6Zvjt19NypQyqV4RETiVq9Ru2FK0TsyDWOcEoT9Ho9Dl4ejHf1YjAYELQaLBzsWV5eNnLyGQxYernzxre+i3dKItEHdtN8rYCWwhKcw4IJ355D+80SrN1cWJiepvbiVQwIdNU3Mtp0l97OTgbutiGYKlCvrBC0OYOeqlqkEoGYg3tpzStCFEVMzVVE7dnOWE8fBS+8hHdSHLbentRcuorXRvFNcHoKp378cwa6ehjs6GCgp4eC195AZqbE0d+P9tp6pkdHMbG04PXn/4l1nY6F6RkCN8XTXlZN260y4vftQrO6xsLsLEPdPR/l8X3Ih/3xrNOLotguimLHezS9n6xVIhtCraIoajEKuOx7n3sPiKI4AKSKovhNURRbNo6/x5j4/kA8TNXaR9+nKec9rhWB597nPq8Cr76HvRYI/++8R4DSm0XILM0YKCpjXbtOd20tATHRTPYP4pu6iVu/e4PEQ/uoufg2Y109aNbWMJHIWFtbRTQxwdXX6/+w957RceXnmefvVs4JqELOOQeCBEmQBMDMzq1WK1qywlhO6znrsx+83vXueO0Zn/Xs+pxZh/XKljyWLFt2tzoxZ5AAARI555xjoXIOdz8U25ZnWupuSeyWm3rOuQeouvdW3VN13/q/8XnIKitBbTaxubKC8mE3a5NTyGRSRrsG0RoNxKIxxh/1oLdYKD9yiLmOh0z09qM1GYnFYkRiUWQKBdtLSxQ7nJSebqbt239HTm0VPW9cxGd3sjozh0StxpSRhkZvYHlknKVHfai0GobvtEE4glQqZejBQ2pbm5HotJSeaSUSDCVovitLiagUzHc+Ym1qmpDbS9jnx7W9g8/tQqPXoUtPZeCty+htycw9eEjAkRDBGL16C51ez87aBiPXbiJXqpDqNFjSUihvPoY5PZWl0XE2F5eIiyJiJEJWRSnT/QMc//LncW1to9Jo2FpdZexBFyMPOimrP0Db919DodXi2LPjDYc+guz9B47pfxayVu+FDyvU+uOgFQThmCiKDwAEQTgKfCDaoZ+XRN7HhuOnWrnR84icsy0IgoAkFkcqCHjcbjYmJgmGQuyvrlN5phUkEqJeL2UXEr9bqxNTpBXmsT+/RFZVOY1nTxGLxam4cJrl0XFa8/PZGp+k4dMv4Pf6MCRZKHisye7Y3iUaCVN+ugW10cDswx7s6xvsLi4TcrlZHJ9Ab02m5rnzDF+7hT4theTcbIwZaeRWlCf6+euq2Zyexby4RFZTI2q9jrhEwO90kV5RBsDEjTuc+JVfZuleJ6JGhTU/j9TcHMyZGUTDYcav3iIaTyPn+BEcm1s8fPsyueVlHP3iq9jfucpnfv9/YW96Fp/bQ1p+DnlNh1Eb9CwMjnDoxedwr22Qkp+Lf2uXY5/9NIIokl5SxKPX3+LZ3/o19haW0JuMiXZfjYaSz3wKvU5HOBSm4eXESLgYj+Px+5/4dy2KIrHYB6oQ/FSyVqIo/qhQ80cloN/L436/C/068DeCIBgfP3aSkM56Xzz1bbgSiQTjoTpW2jpZGxgi90At5oJclBIpSomMz/wf/yuCKOJe30QuQv6Rg6z0DwGwMzlDakkRYTGOz+VGplQSRcTncLA7PU9GZSnVLz1Dz2tvoTXo0aTZWB9ONChqdDoaPvspRq/fJuBy49+1Y7JaSa8sw762wWf/0/+ORBBwr28gicepfvYsMw8eEgmFMFiT8G5u//M1tPz619kYGGahpx9rSRGZDXWsPh7DVcnkKNVqBI2GnblFqs6dZqU7oYcw/PZVSs6fovRMC+PXbrPWO8jn/uP/RmpmBjPtXRQ3N6E1GfHt7iFEIjR96fOMXLmBKIq4llZIKcon5PPR84O3qX3+AlnlJdgXl/E6nRhtVqxZmTjXN5FKZeQfrGdjdg6v3Y5MqSSpIJf1sUnsW9soU5JRmIxPvmTHz869F0XxtCiKle+x/bjc0o9LWH8ouStRFPtFUawBqoEaURRrP4jQBfxipScej+N3ulgbn0D0+NCdbCEei3Hte/9I7bEm5u52oJQr6L54BYVaTeWzZxjoHcScl4PelpC9rr5whskbd1GZjFSfOMrE9dto9ToEQWBleIw4AhMDg+QFgsiUCjRJFiRqBQDm/Bz+8ff/iOrmY2itVi7+5/9C1dlTbM0voklPof/6LcRYHJ/LRfVLzzLwxmWSC3IIiXGGLl4l9+ghZDIZAYcTwSXBdOgAADtTc3i2d1FoEvRTxc1HGbtzj3AwQFJVGW3f/K8Y01NZ6+4n7PMz0v6Ag6daWensYfhBJ0q1mrDHi1QqY2ZgCJ3ZiM+XaL+9+v/8JbbcHGbbuxjoeEBeeRmro2NkVJThcbkZvnqLQ595GQCpXkdgPzF3UP/Sczz8h9dIyswgu6qC7tfeJBQJU/byc6TCEzf6uCh+3G22H0qo9ce9kCAISuAVIBeQvRsa/YIC+wNg376PVCpgTk1BnyEj78QRPPZ9jikVBPbs5LceA8AXCrI9O0/fG5dQKRW89od/zOmvf5mN6VkAhto7qWw9zmLfIDMjYwgCiIJAUkkBjqUVyg/UU3S6hZW+Qd7+kz+jqL6GeKSDmFyGKTWV4lPNbM7OEwlHUGi1SOQyFDod5twc9uYWmX3UR8jlYmlsHJfDTlpWFhODI+j0BuzSWRx2B/ubm0RlMhRaDcpkMz2vv0VcBL/Hi0yhIBQJM9HWjtFmZWV6htNnmrHl5jD05iVqnjlLcnEBxvQ07Ht7aNRqKp9PsAfF4zEkEgk1Lz2L3+3h6n/5c9KVhRQcP4J9YwtTRhrarAx6L9/AubVFwOvDeKcdBJAJAotT06hv3EEilbK1tsHa4jKxaAytwcjI3TY0yUkglT75Nlz4SKboBEF4GfgzwApcEQRhSBTFcz9O1urHCLX+KLwDuEgo134oHcen3uiTrcnIwh5smRmEoxFcW9vMP3hE1cvPsT42yfbENNbSIqRxkarnzhNze1CY9OhGRpFq1CCAZ9eO1qhHaTZiKy0hZWaeeDxO1pGDbE7NkFVbxfbULFK5DJXFRM3JFvRWC1kHahl64xINn30Jx+o6jrklGl99CSEaw1ZcQNDvRx6Pk1NcSMHpZkbeusKZ3/gVHPMLeFweMooLSKmvQqXXsbexiTU7k8wD1QgSCZFgiEgsii07i5LnzjL0T2/z0u//z6zc7yKroY6wz497YQW5XIE5M4PMg3Us3mnHmJ6GTq9Dk5HG1vgUuvRUDGmpyPU6NsenSKsopbqpCZ/Hg2/fgUanJeR0YUiycODlZxl64xLxeIy8lqNIZTKmO7rIKi6i+EwLG5PTVDYfY3d5lezDB1jsG+TICxcIRaKoMt8rPP7Z4qOq04ui+Bbw1o/Y956yVqJUqGZtAAAgAElEQVQoXiVRxfqgyBRF8fxPcn1PfUwP4B0cJe1QHblNjUzd7SApJxuJREJWdQXrE1MMvXOFnCMHE+W06TlWegY59MqLiOEICoUS38Y2Z3/7NxFCESZut1FypoW6z77M4BsXca+uYy0qIBwKsbu8inttk7ILp/Dv2hl6+wql509izcrCv2dHYzKQVlrMxsQk8Xicics3KDrdjIjI0JuXyG06hD7VSiwYRiGVUv/qSyw9eMTYpRsUnjzOgS98moW7D9CajBisSeRWVaBPT+H+X36b4jPNyORyfD4/8496sRYXEgyFmG3rIKOhNvE5uD1EIxFkahUZlWVszswyduUG2Q21ZJSXsD4+xeLQCMbcLKpfuMDo9dtI5XKCsRhBn4+RyzcpbGmi7MJpFh4kehyCDhcarYagz8/u7AJZDbVUP3+O+QePCO87KTl6GKVMxshbV/5NxfQ/B+gSBKHqJznxqV/pH7Z3EIiGWLzRhlwuZ6qvD7VOS/jeAxBFNDotY929KBWJOvrM6Bh6o5GYIEGMhNEY9FQ8ezYRv4+MYi0qYHt8iqg/wObyChKJgFyhYGVmjtmhEQpqaxh5+wqzA0OodFpyjh5EqdMx86iPpq8kwriClibe/sM/xpqTzcg715jq7iW7rJTZRz1YsjNZnp5Bl2Jl+K0rrE1PI1UoCQWDWIryKXnmFDPX7xCORJBqtUh29olGoiw+6EZr0GErLaL/jYsYXjThWN9gc3kFzeWbSHVaCk+foPcf36ToWCPbj0ON1fkFTLfuEQ6H8AUCTP3jDyhqOMD68BiiRMJw10PqLpxl6vZ99BYzuiQLAC6Hg6kHj0gtL2V3epahd65Q/2piJsrrdDPS+Qi90YDiVhs+j5dwMPjEv+uPaqX/iHAM+IogCIsk3HuBRPW7+v1OfOqNPiMrG7lFg6WsmO2pGU5+/Zexz8yR25wQBR156woZRQUUnm1FIpUilcqQSCTktBzl9d/7jxx5/pnEgEo0wtriEsllJWQdPYh334FMp8W7t0fhuVY8Hg8qjZqyZ848fmeRgMeHe2ML+8QM9t1d1vuGCIdCBEIhYvEY5edPYbBZCXq9pJUWk1JVjmdvD/vOLhqzidrPvkzsLREhEqPi5Wdxrm2wNTLB6uw8zr1dTv7Gr6BPtSFejVP4zGkAlvsG8HjcRJUyTDYryWXFJGdlIlUq2OgbYn5wmCSbDUNOJhK5nIyiQnJPJsQ7gj4fG7NzVFw4jUKlYvjty5QdakBjNtHx/ddpPHOKiau3iIsiUomEnsvXqDrayOCd+5QfOcT8/S4EuQyFQY81M53M8jJyD9XT89pbpBYVPvE6PXyiRmsv/KQnPvXufVZeDpsTM8SiUSLL61hLizCXFLI1Mo7f6UJvSya/9Tgbw+OEg0GURgPeQKKmXNtyAkEqIedYI569fWrPnyElPweJRMLS/S6yjzSgz87EvriMwWxCbUtmb24RALVOR9WnnsWzsYWoVnL086/idbkoPn8SjcXE6d/8BmtdvQAk5+cSDAbxbm2j0GqpO3eKtNoq5u+2IxUk5Jw8xkZ3P4ZUG66tbSrPnSSvooL1R/1EIxECoSDxWIxwMIR7cZWCg/WEPF7MRfnkHm5gc2AEnTWZ3JZjFNTVoLIYMRfkotZpKT7dzOZwIqc0df0OJ3/z37E1OkE0GkWt00FcRKJUUnOmFbnRQOG5kxSfP4VEpyG9sIDc40epO9lMLBol51gj+ccOo0mykFVZQdTrZXNmju3NDbSFuR9Z9v6TQKLxQ515ARI1/Xe398VTb/QASScO8+gv/4aU6nJEUSSlpIjthWXGr9wi83ADRpsV//YuUzfbSKurovRsK+v9w6jMBpzbu6yPT5JaWkTxiaPsjk2xPDBCek0lgiCQXVvF9N12dJnp5DbUsTY4gse+j8JkRKXREIqLTLR1EPH5SC4voffvXyfscKFLTiK5spSRS9dQJSeRd+wwa0NjTF65SUptFdF4jK31Tab6+lnt6mX4zj2u/tGfUHjuJEklhWj0OvLPn2Tq7atoUlOwL60w9c5VSl58huyjjaz2DJBUXACAviCHvek5Zu62U3ruJPa1DUbeukzWkYPokiz49+zsLi6RUliA0ZqMb3uX8cs3yDpykHA4zMqjPsrOtOLZ38dn3yfsDyKGwzR+6TOsdveh0uupfOlZBl9/h2gkwvLDXjLqqwn5/Nz/h9fIPNGEOu3JJ/I+SXRZgiC88LidfRG4DyzxAVvRn3r33rG3j29nHfvGNvM9Ayz2DSIg4Nt34HU4WL7XiTLZgj8SQQ4o1AmttaWhEYpONRPfs9P7xkVqT7eyOjjC9tY2galpzBnpbE5MoVQqWZ2dR5BK2ZuZJxSPceX/+lMK66oJ7DtILStCo5CTfrCOveUVPD4fO+sbSC/JkSvkjN7toDoWx7++gVqvZbp/AMvQCBpbMjWfehb3/7tN6fNniUUiRKMRNvuHCbk9rM0tkHa0AX1RPpPXb+Pc2uHgM+dYv99JKBhiZ22NpVv3kapUaLPT2B4YQ65Usjk5iyAI7CyuMHengyhxIv4A/t5hql95Du++A4fbRdDtIRoO4/P5SM5IRxAEyi+cZuytq8RiMSpfvEAsHGZ2bBypRIJ9b4+oVMJb/+n/Rq3TIrl0neH77ZhzcoivbeJd23zi3/W7JBqfEPwhcBi4LYpinSAIrcCPan3/V3jqjd6cbCG8v0XTr/0y0dVN8k8mlG4m3r5CanYW2a3HcG3vsH33PmJcRHrtNgA7GxuI9x5w4HOvYDIYST/cwMbkTELYQq2k/jMvo7NZ2Ryf4viXP8/m+CQ5LUeRKpRMdXWjUKlIqa9GbTaxPTaJRCIh7PJQeuQQO/NL/xyDh6MR5GoNWS1Hmbx0nZqXnkWtVpOUm830rXuYUlMA0FpMhEURc3EBWlsye9/6HkM/uETJuZNk1VSSnO3CWleF2mIiHAzicDjQ5Wdjzs9lY3yKxckp3PZ9mr/+JfKOHUIURUqeO0M4EKT9m/+VaDBI6M1LqM0mQh4PaqOR+b4BFkZG8ew7Eh+mGGdpciqRUroiJf44iZmSlUXR2RYCHi9Eo1hzssk60oBUJsMfDJJ16jg+h+Mjien/DbHdvh8ioijaBUGQCIIgEUWxTRCEP/4gJz71Rg8g9wXQZ6SzNDxONBJhZ3qW5LISNsenEhptY1NUnGnFv7lD7qkTLHX10PzVL+Hc3MK7ugESCbN329FotOTXVlP10jPMXL9DSkkh7sUVSp47i6W4gLEfXMSQl01ZcxNpDbUsdw+gUiqIiHHWRseJBwJYDzcgMRnYnZrFvrBEbksTc7fuEwmFUKrUZNZWMfXWFSzFBUhjcWRKJXPtD7GWF6OzJrNw7Q7KNBuFxw+zMz6NIdWGY2ya4s++zMQPLlHy4nl25xcpOHGErf5RTHk5mDLTKW5sQCKCa3ObwNoWUrOR7elZtobGOPKVz7Ny/yFlL15g+sZdKp47z/7YFMl1NWjVGlwbW6QdrGP8nasc+eoX2RgcIedQPdokC3M37hKRSPDt2lnqeETtZ15i8p1r7C4uo8vOQBmJ4JxfYnN04oMFpD8FRPETZfROQRB0JMZ3/14QhB0SDT/vi6c+pl+en8dYmAtA+snjrD3qx724QlJxPik15XR+67skFeSQVFZM0B/Aub6JJB7HnJdDzuGDLA6P0PHmRTRJSWQcPoBGpSIuisQFgfvf+T5by8ss3LrHStsD1Dod/W9fofjkCVwLK5SeP0lqYz2rIxN0/9ObSHVawoEgSTlZeFZWUet1KLUais6fpPMv/5akmgoAMpuP0vfdf8JSWoiglBFxONE9Vpf1RyOMXb1FJBrFVFFC+5/+FamHE+TCxS+cY/7aHdwrG2iTk8g4doit3kGWbt0n68QRvB4PBa3HyDx1jNWBYdq+9R10KVZ89n10hTkMv30Fc1Y62hQrAX+ApbsdpNZVE47Hef33/oDksmJ0tmRKzp9i/m4H0UgEqU5L9qF6rvzJn6GzJrHWP4w2J5OxKzdJKSsmq6YS+/gUco3mI6jTf6J4718kkcT7bRJz9fP8i+jFj8VTv9Kvr67htO/gX9skFoky1fmQjJIilu90IMqkrE7NYMtIx72yzsbaKiuTU5QcPsT8jTa8Xi/hQJDSww3EBZGFux2szs4h1+vIbjqETCIQ9AdIazqEQq1i6WEfKbnZrPcMsj41i9+xj0Kvp/FrX2DkzctEIlGmbt1DDox1dNJw/gyr7Y8QpAK7a+vsjk7gVCkR4rA2O4ctKxP33j7xYAjN/S78bg+O1XUKD9ShNJvYnV/EvrnFamc3aqWKUDCIy+1if2kVpUlPcHuPhf4BssvL2eweIBKN0P3tvyM1P4/is63M3LxH6qF67EvL2KfnmOsbRK/VEtixszg+gUqnRds/SNGFk/gc++yvb2CfnUen1yPRabj5J39BVnkp3vYuCuprkRt1mEuKWB0aYXtllYWuHgQBtlZWCcfiSD4C5pzov5Hs/PtBFEXfDz38zo888D3w1Bv9keZmbgz0YDvWiM++T4VcRtQfIKO1iZ35RU78ypfZGR4n6/QJVtfWkEplpB07BMD+4jIWlwevy4Uu1YY0N4uViSmyjjcyfeMuGQfrUOh1LN28R+GFU0ScLpLLijHnZbM+M4MmOZnUQ3XYl1cpOdOCY3qevCMH0SRbCPn9qKzJ2KrKWGzv4uRvfQPn3DzphxvYnpzBlJZCytEGNlZWkCmUpB1LSD3Lbt8n5PNjSLGy8aiPZ/7D77B6t4O0lkTfQfjaHXYXl7Hk5qCqqUJt0BMLhklprMff1Y1UEMhuaWLy4jUav/ZFtnoHyDp+BN/2HvWvPI8sKpJ6sBaPw0EkEiW5ohSlRkNmRTlBt4f0o4fQ2ZIJeDxMdD7EUlpAPBbHLJXgmF3EkJOFEItz+Jc+ixAKkXagBq/dQTgc+UVM/wEgCIKH9y7NvducY3i/1/hY3HtBEJYeC1UMvUtW8LMUwvjQSLXinJlnv3uAvNMnSD18gJW2TvbnlzBkpFP60jNMvXUFlVxBWlkxruUE38HeyCQptZXkHT/C/vgMC22d6DJSCfp8yAQJarMJqUyGJieDrr/6LmlHGsg93EDv917nwJc+hyzJxPz1O3j27Mi0GoqfO8vSvU62puewVZbhX98CQPQHMWSmEQqGCLo9eOaXKH3mLPsLy9gy0il98RzzV2+x3N2PqbQYU2kR6wOjGFJsyFVK9EV5jL95iYVrd8hoPEDRyWYAFm+0kXqkAZnVgmtxBYnHj9xoxLe3j0alSVBv7+0Ti0bB5yejpgr3/j4bw2OY8rKp+PTzzF6/S9DlRpQKFJxrZam9i0gwxPyNNs7/7v/E1uAY26MTWPJzKTx/kqV7ncTdXtIrS9lfXmW2rQNTZQkSo474E3bvRTFRq3+/7ecZoijqRVE0vMem/yAGDx9vTN/6eAb4XbKCd4UwioA7jx/DvxbC+AYJkQt+SAijkQTd0H9494fiw8Bht+NdWuXhd7+PQqNm81E//s0dIgoZW+OTyFVKfA4nxtxsZh/2EgqFWOsdYvLSDbJOJAgxJBIJfqcTjUJB8akTPPzW32Gtr2JrZJz19of4ltbY39pkse0Bk29fJeD3s909gGd+maAvQO/3f8D83Q6mLl5DqlDQ/ld/ixiPE0Rk9OJVbAcSFFUFZ1sZff0dTMUFWLIymLp5l6SaCiRyBXG5nJGrt9gdHsMxPUvv628SdLrYuP+Q0Lad2d5BJFIpUZ+PjJoq1vsGMeVkJjj/66pY6xlAbtST39LE3vAYMk2iNJnTeoyle12oki1EQyF01iR6X3ubiMvDemcPpsx07v3Ft1joH2boB+8QQeTyH/wx0Vic3YERtElmVian2ezsYaP9EUG/n5WZWdbudaE1Gpi4/4CNniF8e3sJq3yi+ETF9D8xfp7c+xf5F+bb75Bgvf0dfkgIA3gkCMK7QhgtPBbCABAE4RYJssHvf5g31en1pDY1olKqUFuTSKmvxu90sd8/xM7aBqNvX0GXkYYYCpNamIslLweXuMTsoz60GjUSqQyJVILP62VjYYk44NzdY6HjEZlHD6IrL2H2Rhv51VWUvHCeyTcuUdTUiKG8GG1yok894HGTXlOJtayYzeFx0osKCItxwm43y+OTGHUGnFIpolTC2sw8cq0G79IqXqebze4BQgLIZDLyDtZhKS1Cn5PJzvIq5soSdGmpzN9so/K5s+jT03BsbCKOTzPV8ZDKE02E9h0IMjkbS8vYd3aJenxMd/ehtZjwu9xIJBKG2+5T3XyM/Y0tlGYj5vRUkg/VIZFKAdhdXMaYlkJO81F89n32llcpONuCyqBnc3QCa2YGqUcPEg2Hce7uUXigjpSmQ8zdukdqXh55F04R8vkR/vOf/lQ30AfB02DU74ePa6UXgZuCIPQ/FqKA/0YIA/hJhTD+OwiC8A1BEPoEQejb3d39V/vkCgV7XT1ktjbhXFn/Z0UZ0emh5d//KinFRWiMBtRKNQe+/DlCW7uE3B6yqsox11WRcuwQ1iMNyGVy8g/U4He5OPzVL2CyJaOzJBwPlUyGRCpl7vodspubyG85hmNsCoCV7n7yW44R3XcRdHuJ2Pep+6VXIRBCAKqeP4/cqCel6SCCXkvT176I0ZqMqb4SXbIF28E68k80oRAkFJ8/xdbgKGI8Tk5NFZvdg4jxOEqpjOzGBnxLq6QfqEVqSyL/8EEEiQRLYz3qgmwqTraQX1lOyvFG8uqqseZkYy7KJ+tMM4UH6tBlZFD8zGnibg+FZ1txzC8BMH/9LgWnmpFEowiCwFr7I47++tfYH0/wQvpX1lEadIiiyMzFG+RdOAmxGEG3B6VKSWp9Fe7FFVbudnzom+jD4t1E3vttn3R8XEbfJIpiPQnX/TcFQTjxY479qQUvRFH8K1EUG0RRbLBarf9qXzgUQmcxI5XJ0FeXsTM0xuw71yl64RyWzHTsU3OENnZIOViDTKEg5vOj0ekoOn+Knd4Ebdb8zTaym48y1tZOdusxzNlZhGNR3MurLD3sxVJZyvLkDP5AEJXFBIDX5SYWiSK6POgz04mHQizdvkdWSxMyuZzJO/dJa2wgtboCf8CPc24R3/IapoJc/B4vy22dHPzaF7EPjrJ44y5pxxM8ihktR9jq7keUSEg9cZih772ONj8hjOlzuRHjcSLbu5Q+d5aMlqPMXbzO4PdeR1eYg8/tYeHKLbJPnyD/wimcW1s8/ObfknawHu/6JvM37pJ29BCWnCzCu/tsDo6SlJ+DOtmMx+tj6UE3qQ01SOVyol4fK939JFWVoUyxMvT9N8hpaUp8hpEoS7fbST3cQEppMa6ZBZQW00dWp/+Fe/8xQBTFjcd/dwRBeItETP6zEsL4UOhsayNg0rF1rwuJRMLI3XvUtJ5g51E/SCRM9/ZRdaKJjftdSORy+m/dIfdwA7ZgkIgYZ7GrG499n+iDbsLhCFsjE8jjIuFAgOHeIQRRRHS6Ues0+PbsrHc8IuIPEI6GefDnf03JmRY2ugcYamsnv6aSxet3se/tEQwFmWvvRKfTo1TI6bnTjjHFhupRPyqjnon7nchkclbHJ1Fo1Ehv3k8w0Yoia5PTKLQacv1BVienMSYn4d/cwlRRzND3XielqpzZizfQGgzITUb0/gCr3f2sjo6iNRoRbt4jEokQjkbYW1nFu77J9soK7n0HUSClpgL39g4ai4lYkomZu+149h2sTU5T/fx5pE4XHr+fyM4O7s1tpPE4OyurWMenQKViYWwcrdXK9OWbaFQqRjs6KTna+KN1mH5m+ES14f7E+MiNXhAELSARRdHz+P+zwB/wL0IY/yf/vRDG/yAIwj+SSNq5Hv8w3AD+6IeSd2eB3/2w19PUepIrjx6QdvI4MoWCalEkGolga0qU5WrDYSLBECnHDxOPRinY3iHs8bMzOYtSraL/8g2avvEVdiemOf7vfxXHyAS244kEX1QpxzG3SPa5VqRtnYhGHRqTCUN+Dpujk6yOTBITRWwHqkmdnMZaU4m5IJfIxetU/c7/yOrNe+ScPwlAJBwmHAphOVCNc2UNk9VK9rlWQtEIcomU5IYaVOaEFxGPRFAbjViPN1IdjiDTa7DUVrHc8ZDN2XnSaivJe+Y0giCwePU2JpuN7NbjqESBeCxKzvmTxKNRFi7fovhkM4aSAry7e+jMJjION+Dd2GJ2cJjMynLkqTZSaiuJRyKo1GqkZhOrgyOsTkyjNRk4+MoLLPf2Y6MIbWEuCqMR79Ub5J04SkplGZFQiApRBIUc4QnTZYn8IqaHj8e9TwEeCIIwTIIU8Iooitf52QphfGDIFXKSTx1n+dptoqEQCo0aY1Up290DBN1eJEY9yowUnDPzzF+7Q87ZFlIbahEiEQLhCPWfe4Xg2gYamRy10UAkGiHocBGPx5GHI+SeOMr+xDQShZy02iq2x6dwb20T2tzmyK99BUkwzM7kDNnHGgmubbHW04+1rgqJRIImNwvn9DwBtxuV1UrmqRNstj/CNTFD/rlWHNNzqBRK8p45zUZnDyGXm/2FJcxF+fg9buLRGHKdhpDDhVQuR6HTcvhXvoxzZoFoMEQ0HEZlMhIIB9mdmUObnYEiMw3X/BLzl26Sda4VS1E+gZ09NFotWWdasPcNk1xSSHXLcZQqJVprMmqDASUCqYfrCW/ukNPUSEFdNWmVpfg2twju2Cl59gwbD/tAFKk4exLP/DKIIos372M7fIDwvuPJZ+8/IvdeEIRXBUEYFwQhLghCww89nysIQuBxqXpIEIT/74f2HXhcxp57XKJ+Yo7PR77Si6K4ANS8x/N2fkZCGB8WEpkM66kTLF+9jTkjDVtmOptbO4y+cZGKTz+Pb99J79+/hkqrJXozjiiKODY2CHp8lJ9oYm1iikg0hiIzjezmJtbuPCASi5J5rBG5VsPQd75PwBvA63Aik8lo/4tvU3fhNIJEwLm2jkQiJe/Z08yPTaMMK9E11AFgLS1i/tJNopPT5F84g2dji9XpGeRqNVlpqWxMdqBQJFh18587y/zb10AqUPD8eVTpqez0DyFRKFBkpOFeXUNw+9A1pKJ5/hxLl24QlwjknG4hvrDEdt8Q5Z95if2uXgZut1F+/jQyhQJ9io2pNy6SefAACo0G9Fo8S6sgl5F7/hQLF6+RcrQBZXISxox05gdGca6tk9V8BIVWy/ylG8gECYIgkNbaROef/zWNv/E1DCUFbHQ8QqHTIJXLsdTXfASrsPhRrfTvylp98z32zYuiWPsez/8liZL0IxIL3Xl+QtWm98PPU8nuY4Fj3453Z5O4AHtbOzi2d5AoFAjxOFtzC6SPTiE1G8ksKUYqk5P7ePpto28Q39oGSQ21uLZ3UWvUiDIZc5dv4VzfIhjwo5TL8fl8hAIhcmoqSD16iM3BUVJLCtEW5LE9MUMsGmN9aR65Ssny2Bhak4loJIZUKklM3vn9bK2sgpBY+ZPS0hBVSpau3cbtcKIzm5i/eReVRMbe+jqxeBxN2wNkGjXT7Q9o+OovoU1OYuL7b5BcUcri/QdIghFkSiULI6PoDUbEWBzHzi47/cMklxfjmFtAjMXYaHtAKBbDZ3dgyM0iHo2izUhj6uJ14nIZ9h07gijy4M++zYFnzrDT1YdEKmFxdAK1So0oxpErlMwNDaPWaQkGgjj39pi5fBONTsfMw15SigqQCgOIiE/evf+I2nBFUZwEPnCH4eMclkEUxYePH38XeIlfGP2TgdmShC4jCXvfIOXPnGZ/eh5zRSlBt5vk3Gw0edkIEin6zAxkyRb2Bkex1FQQd3kQZHKikQgqgx6f10tyqg1zThaLV26h0mlJbT6Ka3UdU2427qXVxE23t0/ZKy+w19VLzokjLLV3kVZQgKY4jwqzEffaJmnHG5GpVOyMT2FQq5CqVWSfbWVvYpqkilL8e3ZsB2pYvt/F5tgE9V/9Au6lVQrSbET3HFgfZ/JD1+8wff0OBrOZlalZVKk2bLXVqPQ6li7fIL2sBMvRBlbuPSClMB99YR6bAyMUf+pZXANjpLQcxbuzy+yDh8xevYUuxYZMo0aUSjCYTOSdayUc8LO7sIy+phKZQo7j8g3yDx9El5+DNj2V/aUV9Msr2I4fZqXjIU2/+Q2CK2ukNB5IkIq6XRgO1hBwuJ587z0/FzF9niAIg4Ab+D1RFDtIlJrXfuiYH1l+/lngqTd6AMfENMakZDRZGajSUrAPjhJ0uqj83Kdwjk3j394h61wrgkTCxtIqE//0NoXPnyXkDzD8969T+sIFzColjtFJTJWlqJPM+Oz7xGMxnGNTZJ5rRTAYGHvjIjmNDYkOPreHWDSKPBzFL4js9Y2Q8+wZjGXFbLU/QmLQoVKrMR6sQ2PfxzkxQ2RnD11VOcE9O+7NLVRKJZkH6vBubBPesZNy4jDrK/cBWL3/gMZf/WW8S6tERTj89S/hnpxFpdexeusetmONRAIhnLMLqKVyUk41s3rtNmqNFoVOR1CMEXS52e0d4sTv/jab97vQmI3sTcxQ9qnn2R0cJeRys9PdT9UXP41rboGwP4CtthptZhqLl2+Sd+EUnqk5jPm5+J0uZNEYuhQrzuExgh4vUqMeW0UxjoFR3Du78KR77x+34X4AvK+W3U8oa7UJZD+egz8AvC0IQgUfovz8s8BTb/RrKytEtncJFebhGRkjHgiy/rCP4tZENj/u9aEym4gEg6y1d6GUytlZXcXwsB+FUol7d494JIrKZCTi9rB6p52s081oXG4m3rxM1sF6XIsrRLb32JycQaVSYZVKSTtxlL2BEVApCdr3cKxvoutJBlFkfXaOWCRCYUM99p5BRInA0PXbHPnGVwCIhsL4hsZJO3kM1+QM/f/wGrWfegExHicsEdiZmEJjtqBOTsI1No0ggCY9Be++g9nLN0kpL0ZpMKA0wP7cElKtGtfaBo7tXbzOWfyBAJrcTJxyn+wAABpmSURBVLa7etDaklntHkASjTPwg7fRmczox6dRaNSMv/EOhSea0CUn4X3cjKPNTAMg80wzg3/zPfJaj6PNymDob79PzRdfBUCVk8nEGxep/PwrSOVy9oZGCfv9H9lo7QfA+2rZiaJ4+kO/uyiGeCxMIYpivyAI80AxiZU984cOfV9Zq58GT73Rb6yuEteqQJCgtFiIx2PorcmsDI0geryM3LlPxYkmnJOzZLQcY+1uB5mlJaS2NrHe3kVGUSG7c/PIhsZYm0mo3eiGkhGiMdYnplCoVeiL8vG5XRSeacVgNhHadxCYXWDg5h1yKssRlErSKspRF+Sgspix7+2h02gwHUok9PYmp9Gazaw/6sVoNtN/7Sa1J1tYvt1OypEDZJaX4dvdw728hkwqMPLmZarPnGS/e4Dx+x2UHT+K/VE/UomEpb5BDAY9wbUtwqEQEx2dFB9qwKDVorMlo0uxknK8keCendG+AXJqa7A11qOxmJHJJITDEbRVpUjlcqbaOticmiG872Tkzj3Kjx1l72EfyKTEBAHnvpPtyWksdgchvx/38HjCxY7GcO3sst8zBDIpu0urxMQ4MvmTvR0/bvdeEAQrsC+KYkwQhHwS8yQLoijuC4LgEQThMNANfJmEQs4TwVNPonHo6FFkgSCG7Ay0tmT8wxOUfu5lsooKsRxrJK++hmg0hrm6HIlMhkavB72Wvdl5lFodMYlAxsF6Uk8dx5yeRlpxEeYD1Xi8XqpefQm91YpMIUdns5JWX417fhFzaRHqihKyK8tJqalCq1aT2drE7mNhSYVUSigeJ+Tx4tnaIu70UvHFVzBmZSKmJJNdV42gVpJ9JjEtZy4qIBYIknq8kVgkRnZ5CYbaCowHayk7fhSJTI7pUB2SNBvVn36BcDBI0rFDmOqryKmrQWtNxlhaiFarI731GI6+YfRZmVS3tiBFRK5UEvb7kRmNpJ8+wc6DHtwbW1Q8dw6jyYzpUB2Vp04S9AfQVRRjPlBDyOen4atfQK/ToykrQqpUoK+vwnKkAa/Lia2wAH1dBaqiXNJLizHbbAjCE74dP7qS3cuCIKwBR0jIWt14vOsEMPK4XP0D4Nd+qMz868C3SJSm53lCSTz4xUoPgKwwB+/UHJ6lFTLOtCCRSvG43ajdHvQ5Wejyc1m+egtRoSD1cAMKn4/ZS9ep+3dfJjqtwLO+hXd5hdTGA+yOTuDe2ERl0GEuzGOlrQN7Xz9VX/4cANrSQlxTszjnFil4+Vl22jpRqBITbbqqMhzD4ygUSpKON7Lb/pBYNEb66USXst/rI7CxRfFLzxJyeVi7fgd/KETu2VbQqun95t9S8+kXkSeZsXf3Yz16CKlWQzgaI7Czh3N0gvTTzbhVSpyjk7hX1sh97iz749O4pmYR1SokMhkRiYT9mXkkJj0Zh2pZvXILUSYl61RzQm/OamH68g0O/OpXcCytsNLxEGtZCRaLiY0bbSTVVSKVSFBazNhlEhZv3afsMy/jHJ4g5PdjPViPzKjHNTKJd2eXtHOtSDc2icWebGb9I8zev6eslSiKbwBv/Ihz+oDKJ3xpwC+Mnr2dXcJLK0z39JNbX4tvaxtDdiaW2kqmXnub6i9/jpDDgdJgZKqzi1g0iizJgkKrY/deF9rifLb6B0nOz0Nm1KPNTKfnr79LdWsz67fuE5NLECVSVu53opRIUSlVjHd0kXegFsfwOC6vl+D6OoriPHSpNjan5hDicdbvdbLQ1U1acSGL1++S0lhPwOtDZdATi0Twr2+gtViYvn4LS1YmEpOBWCjMzsg4erOZQCTK6v0udDmZpGRnsn27Ha1Oh9++j3t6jrlH/RQ21OHs6kWCwOC9djIryol6PMTCEfpv3Kb2+Qu4BkbRmI3M9g2iVqkJBIM4t7aRSqSs3WxDrdUy8aCLmMeLUqlCqdXS9c3vUHOqGWffMEqlivmpGSz5OUzfb8eUmopCp4VVGLt5h+T8XGK37oMoPvnJWkTEjz97/7HjqTf6ZJsVfbKOQqUKhVRKJB5n+UYbGo0Gn9PJdvtDSDIRcbspunAGndFAKBpFf+QghpxMlu92sNA3hAQB++YmlqpybDk5KDJSMeVksj0+hXxti6zW40ikUtwr61iL8jFVlaFJtRGIxfDvOwjZHQRW1vHa7bjt+5S9+gIVBj2+nV2SD9YSWN9ic34BmVyOy+0m+WAdYa2HrAO1KFKsuKZmKf+lVxGdbszlJQS6epi8cYfKluNEVjbwezysLSySZzKSevI4coUSudmIobqc3Z5Bar/wKqGtHcyNCY6S/ZU1lBmpqGxWdvqGSMrIILn5CPuTsxhzs3AurpB2upmQz4duYhJbfS0qWxKRYBDN4DCGhlqkCjmupRUM1mS0edlkV1QgSASMtYkFLXNpFV1uFvrKUuLxOIpv/tR9Vu8P8RdG/9TH9ACOzh7MB2sIRiNojAYsdVUE/T4ySoqxtTYhRKOYqspIrijFv7CMe24BbXoKe8NjGM0mDjx7DplGTVJ6OnqbldTyYpyz80Q8Pvyr62SdbcE1OUs8Hsc7PUvBy88SXl0HQOrykHGoDrlKSSwWI6WshMziQvb6htFXlJB+tpWdzl50hbnYcnOwZmeReeoEKoOewNQcOedOEljdQKnRoE9NQfD4iASDRPddHPq1ryJVqTAdbUCq1ZCUmYE2J4PdoVH05cXg9bM3PI42yYImJ5PA4ym8re4B8l84R2BuKXGN/iCKlCTss/OIbg/aonxUuZl41zfY6+yl4pc/z27fENFQiK32h2S2NuHf2SUSDOKfX8aam83+2BSmmgq05SXsP85dGFJt+Hf2iPoDbPf0I33CibzHmbz33z7heOqN3uv1orUlI5HJMNZUMPIPP8AxOkHK6WaUeVnYp2aRRKKo0xMlWdFiJOR0s9PZg9qgRzAZWB4dJxKJsDQwTO9ffAuvz0f66Wa2Ox6ilMnRWMyIThebbQ+wHEqspGGvH/f6Bsr0VIyF+Qz905u4NrZQ5GQQjEZRKRTIlEokEgmZ51vZvHUftVaDND0F39IKjoUldPkJdd3ptnacbhcr9zqZ7e5j7eZ90s61oEmxEQyFCbvcaLU6Ms624OgZQuoNIDPqmeruwTm/iCo3US0yN9TgnpxFEY6gSkoIfKx39aAtLkAikTHx1hVUhXkAJBUXElzZQG0xIZFKST19nK27D1Dr9FiLC4nY99m6+4DkE4eJxeJIAyGU1iS0qTbiBi3eqTkEqZTUk8dw9w+jDEc/An16EeIfYPuE46l373sfPiSkV7Nx+z4RqSSxOoXD7NzrRK3RMHznPoWNB9hu60QqERAkUtYnppBJpQQDQSISAa3ZROaZFgDm3ryEZ9/B+q17SOUyFoaGicSirI2OY0xLRR8MItNqCCpkeB72oTQYCO3a0SVbMRUXsNU7yP7SMvFoDJlG88/X6ff72VpaxlaQRyAWRyqVItiS2brdjkShIO1IYiowuLmN1+XE3T9CNBgkEgqxcuMe+pxM1u514lpaQUREYtBhykxHYTSwdrsdvUaDVK1i+kEXhqxM3NduI5fJWR0cQS2To85IRZ9sYaO7D41cgVqrZezWXQobDyJ29iKVy1mfnUdnMSPIJMw97CGn9TiuqTnme/ux5ecSvHUfQSJBEGBtYRFRIiXF5ca+ukooHEEq+whW+iecLPy3gKfe6JtaWrg21EdKSxM7dx9Q9bVfIriwjLGmgv3pWVKKCzDVVKJ4TH7hnFsguTCflJPHUeh12O91EctMJ7Czi1SrRZecjNKWjLG4gM2b98g63IDBkkQkGkWtUuFcXWO3fxhCIXY3t6h79gzbnT0Uffo5fANjpB87jDIOEUTk+dkojAbi8Tg6p4uk1BS0DTVMv36RoMNBxeF6FPnZpEklBNc2UOdmk1SUTyQQRJGfg95iQusLMPzt74JCRuaZZoRIBCEuYqguJ7jvRC6VYmk9BoBrYRmNxYKtqgxDQR7OhWV0W1voqsvZGRkj77lzOHsGsTQdQqqQY5mYxFRVjjIlwbmfGw4hU6mQ5WUTuddBOBpBV1hAamE+SWXF6EoKAYiGI8TCYTRmM4aDtURutKHUarCPTT7hb1t8Ktz398NT797L5XIEaxL23iF0qTaUeh1CIKGVHtvYIfelZ3D0Df1zt1h0ZYOCT79AYHqOsM+P3GLCVldFaGGF3QfdGA9UEwsE2bh2h+SmQ6QePoh3b494IEhcLseSk03W2VaUag35p5oJLqygUiqRqdUEiLN85Sa6+ipSThzB2TOIKIrstHViPlyP3+dDKpeTmpVBWnEhMoWS3e5Bkg/WEbc72ensRltWhLXpEM6+ISKBAPaOh9T/+tfQGA2I4QhqnR59fRXesSnkMhlBpZzgvgPnzDyi003xF14hvrWLIAhEllbJf+V5vKOTxB0uVGYT1pPHsXc8wj4+TdbpFlxjEwDsjYyjyM0mHgrh6Buk7re+QWxtC4lUgslmJfBDWnW7dzuwthwj4g9gn5jCUFqIPxROsO4+aYjx998+4XjqjT4ejxPY3GGluxfP+ibRYBCv14tjbgFVTiLW1dZX4xkcwz42hbo4D4lEQtDlZu9hL9qyIgB21zfwulx4JmYYv3oTqVKJd3gcd/cASomUneUVtGYT3tEp9gZHMVSWEvb6GXrrEm67A0/fMLK4yN7yGv7hCbbudOAKhlh4+yrG/BxkajWYjSxdvYWuuhxrcxP/f3vnGhvXcR3g7+wu98kllxTFhyiRFCVZEkWKpkRZlCPFjiMkslDE+ZECboPYSBoUBeK2Qf/URZA/RX8kLVCgQdoGQeLGRuy4QZs2QvNQZFm2JcWSLCmySJqUKIriS3y/l/u8u9Mf99JdsRQfESlxd+cDLnbumZm7c8h7dubOPXNm+sqHeJ05ONwugrNB3HYH9pwchq5dZ3Z2lqvf+zccLhejZy+QiMW5/K+vEM/14NlQSHBoBKWSlBxooO/UO9giUfyPmzvoxDxuZvsGyAnk43A6CY1P4Ha6zLj6zhyc1ZXcOXMWV/EGXDuqCbZ1IBNTuEs3MhsM4vH7sdntFB/9JMNvn8Nmt+N6bCvBjtsMvn2WImukMJswmO3sxrmplJIjB5mdniaRSKzdP1vpZ3rQw3vaW1vxFm9gW83nUWMTBFtvEJ2eYfriVbYcP8pU/wCx2VkmOm5hhEJsOXKIWMdtQirJSHM7brcbm9NJFEVg53ZCQyPs/EQThYcPfvwdgyfPUF2/l2S+n4HfXiQWjbG1sQHP5lK2Hz6Ew+fFW7+Hmbfepeyx7fgP7ccPeEdGaX71TXz5eUT6BrAbBhM9fWwoK8MQGL07QGwmSCgcITw2zlgkQtLvxV1WijEyzraGxymwwnSHRkZxtLQSi0YZOv0eLpebruYWqh053O3oJL+yguQHH6IMAxWN8LuTp9n13HFmunuxlZdw+633MBIGbq+XhNtFcXUlg++eJ9fjo+PKVXxFheRcbWboZif5ZSXYL5v9iRGL03m9hYr4Lm6+f5GyHdsJNbcTi8WYuNWJM5DPZEs7JBO48vz09vZSVVW1dv9wPbxP/55eRI6JyA0r4sjLS9e4l5q6OoxQGEc4DJPT+Bvq8G2rYnpklMjAMMRi5Hjc2BX4NxaT9HhI+jxIMIwn34+9IIB3Xx2lTY24XC68Xi/RZJL4jLnr0Oi5iwQa67HZ7fhKiwmUlVK5dw/+xnpmWm+Qu68OezSOEYngKgigAvlEhkcBmLnaTPkT+8nfX0/uwQbi0Shb99bira8ht3YXohR5m8ooevpJ/Bs3UlZVSV5dDUYoRG75JqLxGACxUIRgcxsba2so3L2TgsNNhKZnqKqrJRyPUf/VF7DZbXj31+E72ID4PBRsLsdeGCAOTDa343DYcfhzce/fi792F/5AASWfOoIq2UBeWSmlhw8RD/ipfvowhRUV+BrrkcpyXIE8tu6txba9itLa3RTW7CS3aR+2ogKqjz5NaUUF+XW7sU0Gyd1auXo3xv3Qw/v0NnoRsQP/jBlVtwb4IxGpWel1CvbXExoZY6inh+j0DDI1w44//By2eBxveRmh5jY2Hz9KXkE+doeD2Ee3KH32GSrr9phx8M5ewJWXR9+5C7h37aDoyQOE2m4y0dqOd1MZOQUBFIrhd35L4OA+QpEI03138ZYWY3c6CYdmGTt/CW/tbooe30Osp5+RsxcIHHic4icaiHT3Mnz6LIEjTQSDQZKJBEMnz1B29Cl8Xg/j5y7ib9xLojCPyOAw8ds9eB6rRgXyCI2MMvbOeQqONGHzuIhOzzD69lmKPn0YsQluuwNPUSGR8UmSiQRj71/GV1KMt6wEp99PtK2Dzc8cYUttDfYt5YydPkt8egYFTDZ/hC0UpfzZo4SHRkj0D+HZuY1kJEJodIxQWweBJw+QTCQJXWuh7OhTzHb3YkQiJMcncVVsJhqcZaa9A9eWMmxu1yrfIfNQCgxj6SPDSWujx4yie0spdVspFQPexNwcY9nEY1HCXT24CwvIcbm5/oPXsBcVYgtFGL3RQcerb+KtqiA+NMJQZxcDvz6Np2oz0Z5+ZkfGcChwFBfR9/NfkjQMjLFxot19jHZ2Mdl6g6RKEu7qYaD9BgmVwBifxFNaQteJX4PLRbirh8nJKWbHJ4n13SXc1cNgaxvR4CzGxBTx/kG633sfR2EB8buDKLeT5u/9CPfmTRgjYwzeuk10OogxMobH66Pzv3+BLd9PuKsHX14e7a//B+6KcqI9/UTGp7jzs/8ht7qKWN8APR+2EHc6CHf14CzZyM0fvYFKJknEDXI8btp++GNcm0oxJqeYGhpGZoL4tlXRf/IdOi9dITIwjHjdGIPD9J+/iLOkiHBXD8N3uun7xSlcm0qJ3Oml59p1xO8jcqeXhM/DR6+8gWOjWXZqapKJ9g5iPg+JWHxNbpJUlBUxeLEj05F0VlJEvgAcU0p91Tr/EnBQKfXSvHJ/ihl/jIqKiv3d3d0f5xmGQTQa/fg8kUhgt3ZumX8+P08pdU9IpNT8ZDJ5j7PJYtedX34ldeeXXazu3AqyufzF2rBUmwzDwJHyXj01f+6emvvbLPY9iUSC0dFRbDYbSikqKysXdNIRkStLrXFfCgnkKZ46uHTBE2898HetZ9J9Im9ZEUesqCffB2hsbLwn3+Fw3HPzah4+eXnL2ndxddATeWlv9PfbCEOjWQCVFRN1S5HuRv8BsENEtgL9wPPAHz/aJmnWLXMLbrKctDZ6pZQhIi8BJwE78IpSqvURN0uzblGwls4/aUJaGz2AUuqXmJsDaDSLo3t6IAOMXqNZEfqZPu3f02s0K0A9lCAaIvIPItIuItdF5L9EJJCS9zeW9+gNEflsivyBPEtXgjZ6TfageFgLbk4BtUqpvcBNrN2ULW/R54E9mHvV/YuI2FfLs3S56OG9JotQDyWIhlLqNymnF4AvWOnngDetTS+6ROQWplcpWJ6lANa27M8BH61F+7LO6K9cuTIqIt3zxEXA6KNozyqSCTrA/fV48NU4wfBJzl0rWkZJ91LbWq2ArwD/bqXLMX8E5kjds653nnwZroO/H1ln9EqpjfNlInI53d0uM0EHWFs9lFLHVutay9nLTkS+ARjA63PVFmoWCz9m673sNJr1xFJ72YnIi8AfAJ9W/7fAZTEP0ofmWaon8jSaVUZEjgF/DXxOKRVKyToBPC8iLsuLdAdwiRTPUhFxYk72nVir9ume3uT3fV5bT2SCDpAZenwXcAGnrJWGF5RSf6aUahWRn2JO0BnA15RSCYCH6Vma1ktrNRrNytHDe40my9BGr9FkGVlt9A/T9XG5iMgrIjIsIi0pskIROSUiHdZngSUXEfmO1f7rIrIvpc6LVvkOayZ5Tr5fRJqtOt+R1NA/q6fDFhE5IyJtItIqIn+ZjnpkLMuJGZaJB+aESSdQDTiBD4GaddCuTwL7gJYU2d8DL1vpl4FvW+njwK8w3/82ARcteSFw2/ossNIFVt4l4JBV51fAs2ugQxmwz0r7MV1Ra9JNj0w9srmnf+CgmmuBUuo9YHye+DngVSv9KvD5FPlryuQCEBCRMuCzwCml1LhSagLTF/yYlZenlHpfmZbzWsq1VlOHAaXUVSs9A7Rhep6llR6ZSjYbfTn/3/Wx/D5lHzUlSqkBMA0KKLbk99NhMXnfAvI1Q0SqgAbgImmsRyaRzUa/rKCa65z76bBS+ZogIrnAfwJfV0pNL1Z0Adm60SPTyGajT6egmkPWkBbrc9iS30+HxeSbF5CvOiKSg2nwryulfmaJ006PTCSbjf6huj4+ICeAuZnrF4Gfp8hfsGa/m4Apa9h8EviMiBRYM+SfAU5aeTMi0mTNdr+Qcq1Vw7r2D4E2pdQ/pqseGcujnkl8lAfmrPFNzFn8bzzq9lht+gkwAMQxe7Q/ATYAp4EO67PQKiuYwRc6gWagMeU6XwFuWceXU+SNQItV57tYXpmrrMNhzOH2deCadRxPNz0y9dBuuBpNlpHNw3uNJivRRq/RZBna6DWaLEMbvUaTZWij12iyDG30aY6IeETkXSt2+nLrvCQiX17LdmnWL/qVXZojIl8DHEqpf1pBHS9wXinVsHYt06xXdE+/ThGRA9bacreI+Kx16bULFP0iljeaiDxt9fo/FZGbIvItEfmiiFyy1p5vA1BmsMY7IvLEAtfTZDg6MOY6RSn1gYicAP4O8AA/Vkq1pJax3IerlVJ3UsT1wG7M5bm3gR8opZ6wAln8OfB1q9xl4AjmunRNFqGNfn3zt5hrBCLAXyyQXwRMzpN9oKzlqyLSCcxtsdQMfCql3DCwa1Vbq0kL9PB+fVMI5GJGn3EvkB9eQB5NSSdTzpPc+yPvtuprsgxt9Oub7wPfxNwW6dvzM5UZTcYuIgv9ICzFY5gLVjRZhjb6dYqIvAAYSqk3gG8BB0TkmQWK/gZzVdtK+QTw1gM0UZOm6Fd2aY6INAB/pZT60lrW0WQOuqdPc5RSvwPOrMQ5B3MC8Jtr1CTNOkf39BpNlqF7eo0my9BGr9FkGdroNZosQxu9RpNlaKPXaLKM/wWO9B1vAqELhQAAAABJRU5ErkJggg==\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "model.update_until(model.time + 5000.)\n",
+ "model.quick_plot('land_surface__elevation', edgecolors='k', vmin=-200, vmax=200, cmap='BrBG_r')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 24,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "array([ 3.03531373e+09, 1.33561968e+10, 5.64027942e+09, ...,\n",
+ " 0.00000000e+00, 0.00000000e+00, 0.00000000e+00])"
+ ]
+ },
+ "execution_count": 24,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "model.get_value('channel_water_sediment~bedload__mass_flow_rate')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3 (pymt)",
+ "language": "python",
+ "name": "pymt-dev"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.7.3"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/ecsimplesnow.ipynb b/lessons/pymt/ecsimplesnow.ipynb
new file mode 100644
index 0000000..99a889b
--- /dev/null
+++ b/lessons/pymt/ecsimplesnow.ipynb
@@ -0,0 +1,169 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "# ECSimpleSnow component\n",
+ "\n",
+ "ECSimpleSnow is an empirical algorithm to melt snow according to the surface temperature and increase snow depth according to the precipitation that has fallen since the last time step.\n",
+ "\n",
+ "## Details: \n",
+ "\n",
+ "**Brown, R. D., Brasnett, B., & Robinson, D. (2003). Gridded North American monthly snow depth and snow water equivalent for GCM evaluation. Atmosphere-Ocean, 41(1), 1-14.**\n",
+ "\n",
+ "**URL:** https://www.tandfonline.com/doi/abs/10.3137/ao.410101\n",
+ "\n",
+ "## Source code in Fortran:\n",
+ "\n",
+ "**URL:** https://github.com/permamodel/Snow_BMI_Fortran\n"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### load module"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import numpy as np\n",
+ "import matplotlib.pyplot as plt\n",
+ "from scipy.optimize import curve_fit\n",
+ "from tqdm import tqdm\n",
+ "\n",
+ "import pymt.models\n",
+ "ec = pymt.models.ECSimpleSnow()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### load example configuration and inputs"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, config_dir = ec.setup('.')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### initialize by using default example data"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ec.initialize(config_file, config_dir)\n",
+ "ec.set_value('snow_class', 2)\n",
+ "ec.set_value('open_area_or_not', 1)\n",
+ "\n",
+ "# List input and output variable names.\n",
+ "print(ec.get_output_var_names())\n",
+ "print(ec.get_input_var_names())"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Implement the simple snow model for the first year as an example"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "plt.figure(figsize=[4,9])\n",
+ "h0 = plt.subplot(3,1,1)\n",
+ "h1 = plt.subplot(3,1,2)\n",
+ "h2 = plt.subplot(3,1,3)\n",
+ "\n",
+ "h0.title.set_text('Snow Depth')\n",
+ "h1.title.set_text('Snow Density')\n",
+ "h2.title.set_text('Air Temperature')\n",
+ "\n",
+ "print('Air Temperature Unit:', ec.var_units('land_surface_air__temperature'))\n",
+ "print('Snow Depth Unit:' , ec.var_units('snowpack__depth'))\n",
+ "print('Snow Density Unit:' , ec.var_units('snowpack__mass-per-volume_density'))\n",
+ "\n",
+ "for i in tqdm(np.arange(365)):\n",
+ "\n",
+ " ec.update()\n",
+ " \n",
+ " tair = ec.get_value('land_surface_air__temperature') \n",
+ " snd = ec.get_value('snowpack__depth', units='m')\n",
+ " rsn = ec.get_value('snowpack__mass-per-volume_density')\n",
+ " \n",
+ " units = ec.var_units('snowpack__depth')\n",
+ " \n",
+ " h0.scatter(ec.time, snd, c='k') \n",
+ " h1.scatter(ec.time, rsn, c='k')\n",
+ " h2.scatter(ec.time,tair, c='k')\n",
+ " \n",
+ " \n",
+ "# ec.finalize()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Comparison with Observations at Barrow"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "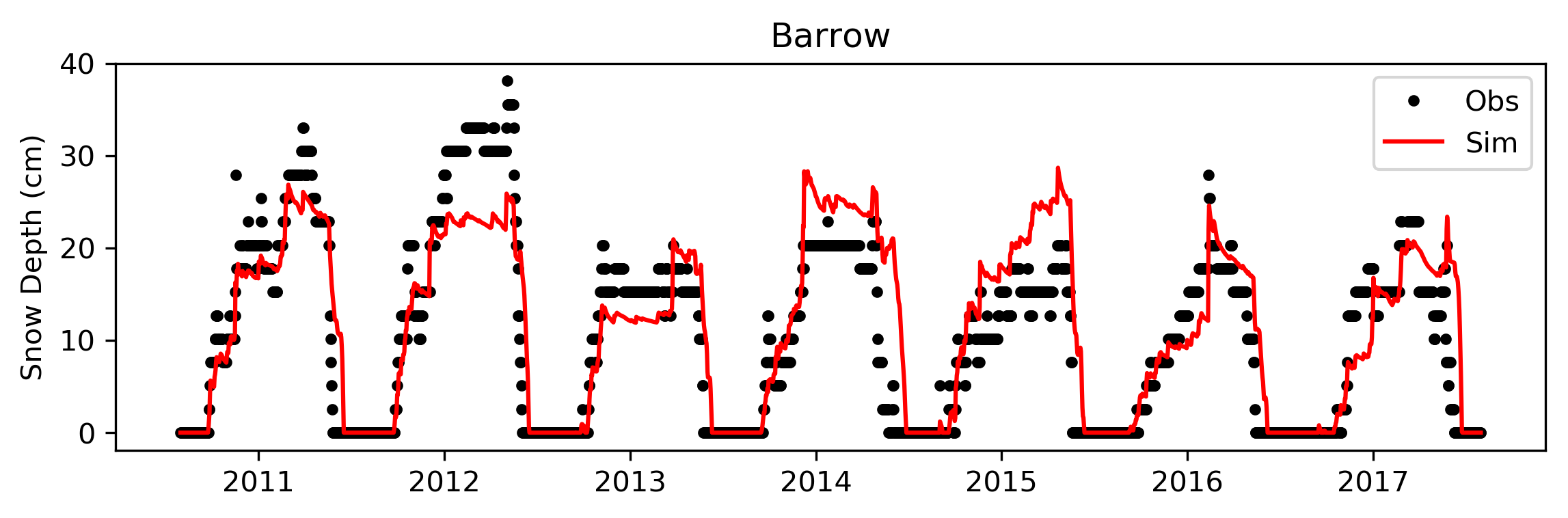"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.7.4"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/frost_number.ipynb b/lessons/pymt/frost_number.ipynb
new file mode 100644
index 0000000..33e957a
--- /dev/null
+++ b/lessons/pymt/frost_number.ipynb
@@ -0,0 +1,331 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Frost Number Model\n",
+ "\n",
+ "### Introduction to Permafrost Processes - Lesson 1\n",
+ "\n",
+ "This lab has been designed and developed by Irina Overeem and Mark Piper, CSDMS, University of Colorado, CO \n",
+ "with assistance of Kang Wang, Scott Stewart at CSDMS, University of Colorado, CO, and Elchin Jafarov, at Los Alamos National Labs, NM. \n",
+ "These labs are developed with support from NSF Grant 1503559, ‘Towards a Tiered Permafrost Modeling Cyberinfrastructure’ \n",
+ "\n",
+ "### Classroom organization\n",
+ "\n",
+ "This lab is the first in a series of introduction to permafrost process modeling, designed for inexperienced users. In this first lesson, we explore the Air Frost Number model and learn to use the CSDMS Python Modeling Toolkit ([PyMT](https://github.com/csdms/pymt)). We implemented a basic configuration of the Air Frost Number (as formulated by Nelson and Outcalt in 1987). This series of labs is designed for inexperienced modelers to gain some experience with running a numerical model, changing model inputs, and analyzing model output. Specifically, this first lab looks at what controls permafrost occurrence and compares the occurrence of permafrost in Russia. \n",
+ "Basic theory on the Air Frost Number is presented in [Frost Number Model Lecture 1](https://csdms.colorado.edu/wiki/File:FrostNumberModel_Lecture1.pptx).\n",
+ "\n",
+ "\n",
+ "This lab will likely take ~ 1,5 hours to complete in the classroom. This time assumes you are unfamiiar with the PyMT and need to learn setting parameters, saving runs, downloading data and looking at output (otherwise it will be much faster).\n",
+ "\n",
+ "We will use netcdf files for output, this is a standard output from all CSDMS models. If you have no experience with visualizing these files, Panoply software will be helpful. Find instructions on how to use this software.\n",
+ "\n",
+ "### Learning objectives\n",
+ "\n",
+ "#### Skills\n",
+ "\n",
+ "* familiarize with a basic configuration of the Air Frost Number Model\n",
+ "* hands-on experience with visualizing NetCDF output with Panoply.\n",
+ "\n",
+ "\n",
+ "#### Topical learning objectives:\n",
+ "\n",
+ "* what is the primary control on the occurrence of permafrost\n",
+ "* freezing and thawing day indices and how to approximate these\n",
+ "* where in Russia permafrost occurs\n",
+ "\n",
+ "### References and More information \n",
+ "\n",
+ "Nelson, F.E., Outcalt, S.I., 1987. *A computational method for prediction and prediction and regionalization of permafrost.* Arct. Alp. Res. 19, 279–288. \n",
+ "Janke, J., Williams, M., Evans, A., 2012. *A comparison of permafrost prediction models along a section of Trail Ridge Road, RMNP, CO.* Geomorphology 138, 111-120.\n"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### The Air Frost number\n",
+ "\n",
+ "The Air Frost number uses the mean annual air temperature of a location (MAAT), as well as the yearly temperature amplitude. In the Air Frost parametrization the Mean monthly temperature of the warmest month (Tw) and coldest month (Tc) set that amplitude. The 'degree thawing days' are above 0 C, the 'degree freezing days' are below 0 C. To arrive at the cumulative freezing degree days and thawing degree days the annual temperature curve is approximated by a cosine as defined by the warmest and coldest months, and one can integrate under the cosine curve (see figure, and more detailed notes in the associated presentation).\n",
+ "\n",
+ ""
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import numpy as np\n",
+ "import pandas\n",
+ "import matplotlib.pyplot as plt"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "frost_number = pymt.models.FrostNumber()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Part 1\n",
+ "\n",
+ "Adapt the base case configuration to a mean temperature of the coldest month of *-13C*, and of the warmest month *+19.5C* (the actual values for Vladivostok in Far East Russia)."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, config_folder = frost_number.setup(T_air_min=-13., T_air_max=19.5)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "frost_number.initialize(config_file, config_folder)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "frost_number.update()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "frost_number.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "frost_number.get_value('frostnumber__air')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Part 2\n",
+ "\n",
+ "Now run the same simulation for Yakutsk on the Lena River in Siberia. There the warmest month is again *19.5C*, but the coldest month is *-40.9C*. "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "args = frost_number.setup(T_air_min=-40.9, T_air_max=19.5)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "frost_number.initialize(*args)\n",
+ "frost_number.update()\n",
+ "frost_number.get_value('frostnumber__air')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Questions\n",
+ "\n",
+ "Please answer the following questions in each box (**double click the box to edit**)."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q1: What is the Frost Number the model returned for each of the Vladivostok and Yakutsk temperature regimes? \n",
+ "\n",
+ "*A1:* the answer in here."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q2: What do these specific Frost numbers imply for the likelihood of permafrost occurrence?\n",
+ "\n",
+ "*A2:*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q3: How do you think the annual temperature distribution would look in regions of Russia bordering the Barents Sea? \n",
+ "\n",
+ "*A3:*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q4: Devise a scenario and run it; was the calculated Frost number what you expected?\n",
+ "\n",
+ "*A4:*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q5: On the map below, find the how the permafrost is mapped in far west coastal Russia at high-latitude (e.g. Murmansk). \n",
+ "\n",
+ "\n",
+ "\n",
+ "*A5:*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q6: Discuss the factors that would make this first-order approach problematic? \n",
+ "*A6:*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q7: When would the temperature in the first cm in the soil be significantly different from the air temperature?\n",
+ "\n",
+ "*A7:*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Extra Credit\n",
+ "\n",
+ "Now run a time series."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "data = pandas.read_csv(\"https://raw.githubusercontent.com/mcflugen/pymt_frost_number/master/data/t_air_min_max.csv\")\n",
+ "data"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "frost_number = pymt.models.FrostNumber()\n",
+ "config_file, run_folder = frost_number.setup()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "frost_number.initialize(config_file, run_folder)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "t_air_min = data[\"atmosphere_bottom_air__time_min_of_temperature\"]\n",
+ "t_air_max = data[\"atmosphere_bottom_air__time_max_of_temperature\"]\n",
+ "fn = np.empty(6)\n",
+ "\n",
+ "for i in range(6):\n",
+ " frost_number.set_value(\"atmosphere_bottom_air__time_min_of_temperature\", t_air_min.values[i])\n",
+ " frost_number.set_value(\"atmosphere_bottom_air__time_max_of_temperature\", t_air_max.values[i])\n",
+ " frost_number.update()\n",
+ " fn[i] = frost_number.get_value('frostnumber__air')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "years = range(2000, 2006)\n",
+ "plt.subplot(211)\n",
+ "plt.plot(years, t_air_min, years, t_air_max)\n",
+ "\n",
+ "plt.subplot(212)\n",
+ "plt.plot(years, fn)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.2"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/gipl_and_ecsimplesnow.ipynb b/lessons/pymt/gipl_and_ecsimplesnow.ipynb
new file mode 100644
index 0000000..c561473
--- /dev/null
+++ b/lessons/pymt/gipl_and_ecsimplesnow.ipynb
@@ -0,0 +1,340 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Coupling GIPL and ECSimpleSnow models\n",
+ "\n",
+ "Before you begin, install:\n",
+ "\n",
+ "```conda install -c conda-forge pymt pymt_gipl pymt_ecsimplesnow seaborn```"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 1,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stderr",
+ "output_type": "stream",
+ "text": [
+ "\u001b[33;01m➡ models: FrostNumber, Ku, Hydrotrend, GIPL, ECSimpleSnow, Cem, Waves\u001b[39;49;00m\n"
+ ]
+ }
+ ],
+ "source": [
+ "import pymt.models\n",
+ "import matplotlib.pyplot as plt\n",
+ "import seaborn as sns\n",
+ "import numpy as np\n",
+ "import matplotlib.colors as mcolors\n",
+ "from matplotlib.colors import LinearSegmentedColormap\n",
+ "sns.set(style='whitegrid', font_scale= 1.2)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Load ECSimpleSnow module from PyMT"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 2,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "The 1D Snow Model\n",
+ "('snowpack__depth', 'snowpack__mass-per-volume_density')\n",
+ "('precipitation_mass_flux', 'land_surface_air__temperature', 'precipitation_mass_flux_adjust_factor', 'snow_class', 'open_area_or_not', 'snowpack__initial_depth', 'snowpack__initial_mass-per-volume_density')\n"
+ ]
+ }
+ ],
+ "source": [
+ "ec = pymt.models.ECSimpleSnow()\n",
+ "print(ec.name)\n",
+ "\n",
+ "# List input and output variable names.\n",
+ "print(ec.output_var_names)\n",
+ "print(ec.input_var_names)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Load GIPL module from PyMT"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 3,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "The 1D GIPL Model\n",
+ "('soil__temperature', 'model_soil_layer__count')\n",
+ "('land_surface_air__temperature', 'snowpack__depth', 'snow__thermal_conductivity', 'soil_water__volume_fraction', 'soil_unfrozen_water__a', 'soil_unfrozen_water__b')\n"
+ ]
+ }
+ ],
+ "source": [
+ "gipl = pymt.models.GIPL()\n",
+ "print(gipl.name)\n",
+ "\n",
+ "# List input and output variable names.\n",
+ "print(gipl.output_var_names)\n",
+ "print(gipl.input_var_names)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Call the setup method on both ECSimpleSnow and GIPL to get default configuration files and data."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 4,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "('snow_model.cfg', '/Users/mpiper/projects/GIPL-BMI-Fortran/Notebooks')\n",
+ "('gipl_config.cfg', '/Users/mpiper/projects/GIPL-BMI-Fortran/Notebooks')\n"
+ ]
+ }
+ ],
+ "source": [
+ "ec_defaults = ec.setup('.')\n",
+ "print(ec_defaults)\n",
+ "\n",
+ "gipl_defaults = gipl.setup('.')\n",
+ "print(gipl_defaults)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 5,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ec.initialize('snow_model.cfg')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 6,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "gipl.initialize('gipl_config.cfg')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 7,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "# Get soil depth: [unit: m]\n",
+ "depth = gipl.get_grid_z(2)\n",
+ "n_depth = int(len(depth))"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 8,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stdout",
+ "output_type": "stream",
+ "text": [
+ "Final soil temperatures will be (176, 365)\n"
+ ]
+ }
+ ],
+ "source": [
+ "# Get the length of forcing data:\n",
+ "ntime = int(gipl.end_time)\n",
+ "\n",
+ "# Define a variable to store soil temperature through the time period\n",
+ "\n",
+ "tsoil = np.zeros((n_depth, ntime)) * np.nan\n",
+ "\n",
+ "print('Final soil temperatures will be ', tsoil.shape)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 9,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "[]"
+ ]
+ },
+ "execution_count": 9,
+ "metadata": {},
+ "output_type": "execute_result"
+ },
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAt0AAAGSCAYAAAA/0X3WAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4xLjAsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+17YcXAAAgAElEQVR4nOydeZgdVZn/P1V3631LAkk3JEEJhyWsgagIiEZHVHS0AVEx4PhzQ0zEwDAdlagJmpbRDEPcl2EkgwPitNuIykyUGWHUYBAwLId964QsnV5v375r/f44darr3r739u3l9no+z9NP0rVXdVWdb73ne97XchwHg8FgMBgMBoPBUD7s6T4Ag8FgMBgMBoNhrmNEt8FgMBgMBoPBUGaM6DYYDAaDwWAwGMqMEd0Gg8FgMBgMBkOZMaLbYDAYDAaDwWAoM0Z0GwwGg8FgMBgMZSY43QcwUxBCvA64B/i2lPJjvukfAL4ipVxY4nZGy8F4jJTyuXEe5oxBCFELXCKl/Jcp3m8Q+D/gvVLKp4UQ9wL3Sinbpmj/pwH1Usr/EUIEgD8B75FSPjUV+zdMDkKI54BlvklpoBO4E/iclDJahn1+HrhQSnlmCcsuB54FTpZS7nF/P1VK+TN3/nOo99LXJvs4fcfwz8CDUspbxnLsk7TvRcCbpJQ/dH//GnC/lPIHU7H/2YIQIgJ8EngfcCzgAA8CN0sp75zOY8tFCPEJ4Fop5fI88+4BXldk9b+TUv5reY5s6hBC2MBHgO9LKZPTfTwaIcTfAxVSyi2+ae8BPgacDFQBTwH/BmzTxy6EeCPwX0CllHJICHED8Bnfph2gH/gtsEVK+YDbhieBt0gpf13+swMhxOuBLinlw5O0vXHrABPpHub9wJPAe4UQlb7pdwAnjmE7S3w/3wT+kDPtxUk52unnOtTLY6r5FKrxfXoa9g3wC+AEACllGrgB9Xc2zD4+zfBzuQz4IOo98M9l2t9XgDeXuOyL7nE97v5+C3Cub/5ZQNk+eIUQZwJrgOkSuTcCrb7fNwObhRALpul4ZhxCiApUoOj/AV9GiaPVwC+BHUKIjxVee8bRyvCzuNad5m8375im45psXo9qLwLTfSAaIcQy4OPANt+0rwHfAn4GnIPSQDcC61HvomI8wPDfrQV4NWABv3GDdVOKK4p/CzRP4mbHrQNMpBsvWnAxcDXqRrsY2AEgpYwBsVK3JaV82bfdQSDhnzaHsKZ6h+7H0D+gXgLTRe55/wy4SQhxjpTy3uk4IMO46c95Njvd6O4/AB+a7J1JKQeAgRKXTQP+Y7Ny5h+cxEPLx+eAb0kpM2XeTyFyz/eAEGInsA74/LQc0czjM6jo9glSykO+6Y8JIWLAF4QQ33XvpRmNlPKw/r8QosedZtrNqaENuEP37gkh3owS4a+TUv7et9yzQohHgT8LIb4opXyswPaSOX+7fUKIdcALqN6MKYlu+yjHNR+3DjCiW3EhUAfcBdyNinjtgGx7iRDifODHqC+9jwC3Syk/OtadCSEsVKT4KqAJ+AtwjZRylzv/34CD7ryLgcOoDwIL9bW5EPWl9QEpZdLt0jkR1T3+d0A3cKOUcrtvnxcCXwSOA55259/qzrsBOA2IoCJonwB+iorM/a27v5eBb0gptwohPoTbheTaaY4Gbsdn88jtQnJtIA8Cb3TP6xx3m9tQUY4MsBP4ZJGX7WXAASnl4/lmCiGORfVWXARsRX3Z7gI+JqV80jf/ve78hcCvgI9KKbt980/Q+3DP9QYp5WL3HFqAbwohLpZSvlFK6Qghforq4jWie/aTAuLg2UFeheoRfDWwXkr5AyHEtSjxtwB1T18rpfyju04AFUH/MOo+vx/4hJTyEb9Fw32X/BT1XG8FKoEfoe7/mN9eAlyLaqxe5953y/32Evd9sg713C5FRcc/LaW8yz2mfwUG3X1cDHQB35NS3pDvAgghjgHegupazou7/38C3oWKrj4FfEZK+Qvf/JuBS1DvlgeBq6SUD/jme/aYnPO9GLjCne5IKXUD1wF8323wZ0zX/HTg2hQ+hLqGh/Is8h3gTi243ff0Dah262XgDOAVqHf8eaj3bwewQUrZ796fvwNq3Y9Fcu7f5ai/1yXAFlQbcD/qXSvd5c8CtgOnuPP+bxLOu1g79iH3mtyJEpJh4GvAvwPfR91bDwCXSSmfd60Rt6M+sm9APR+3A1dLKYfcbR6P6vk6Fzjgzv+clDLurv9vwA9RvQ3/JqW8SghxDerZWYayVvwnSsQuQVkxAGJCiLXA8cAbpZSv9p2jZ5ks0DbfRnH9cLJ73meinvufuOc0mOd61gGXoyLwmo8Cv8wR3ABIKXcLIVaMw06Zcv+Nj3E9fZwCda+eg7IC/gfqnAdyLS7u8jcwfF2fczfzKyHE94F2plEHGHuJ4v3AfW706Ceoxu0VBZZdgHrgz0DdBOPh4+7PR4HTUUL/d0KIpTnLPIp6Ye0E/hVlrbjIPd6LUDeN5m2oh3o18FngH4UQlwEIIU5BNehfB1aiXlo3CyEuzll/J3A26kv0n1EP+TsA4a77JdfLdBtwE8PdSHtLPO8Po8TBhVLKJ1AvwlcCf4N66IPAr13hko+3UdpX8udRL8E3okTIjTnzt6Ku/euBFahrUwrvAPahXtKX+Kb/GvibIsdtmOEIIWwhxGrU/flT36wLgP9Fie67hBAfRb1YP456du8CfusKVYBNqC7Yq935LwK/LHBv1AB/j3qW34G6X7+RZ7lPomxq30Q9k7l8GviCu+9T3OP/uRDiVN8yHwJeQjXE3wO2CCFWFbgcbwUelVJ2Fpiv2YzqGTwT1UjdIoQI++ZvQQUvzgAkcLcQommUbYJ6r/4IJVaW+Kb/N+r9W+i45xOvABYD/5NvppQyJqXMfS9fBrwB9UFTjxIHSZSgbEUJmrFalj7P8Lt0CW6b6P6dfw08hPr734pqv8ZNie3Y6ag28LWo5+LTqI+Jz6PO7whUL46mHvWsvgt4J+p53+7urxL4DerePR113S4Evupb/0hguXuON7lC+rOoZ3YFqh1qRT1/zwLvdtdbjgrglUJu2zyafrgd2ONeo3egLG1/X2DbrweGUB9FmrMpcF8BjFVwCyGORH2g7wf+OJZ13fUXou7VQdS9eglwPvDdEjeh35nvAzb4pk+LDpj3kW4hRCOqkfkHd9LPUV9SfwdcX2C1rRP0FH8aFVH4lfv7Ftfo/3HUFzrA41LKL7vH+B3UA/8ZKeVfgL8IIf4MnOTb5gBwhdtF9KgbZfg4SiD/A/ADKeV33GWfFkKsQEXQfuxb/8tSSsfd5++B7VLKB9357UKITaivvweFEFF83UjqQ3RU/ktK+V/u8itQEa3FUsr97rTLUFG4N5FfXJ+JaohHY7Pu4hFCfBP1UvXzaSnl3e78DwP3u8dTdBCslPKwECID9Ekpu32zHkX1lKxg2INrmPl8VQjR7v4/gvr7/5zhdwEoa9lWbbMQQnwaaJNS/tKd/yU3KniVUIORrkRFRDrc5a9CNfiNefYfQEUG/89ddgPwIyHEJ/0LSSl7hRAJYDDXVuJGua8GviilvN2d/HkhxKtQ0bDL3GlPSyn1++wGIcSnUOJ1d57jOhN4JM/0XP5d71MI8QWUwFoOPOHOv11K+Q13/keB54FLGcX76EavYkDA3+vlDtR6xj2+MTfec4xF7r9deoIQ4gjgmZzl3uKLWH5HSvmIu+wnUEG3ta6FUvfq/lEIcdwYjuMGKeX/uut/g+H261JUVPMTbq/E426bdMEYtp1LKe1YCPi4lLJLCPEkqif1h7qtFUL8CNW+aILAR3w9VdcCt7nPx3uBqJRyvbusFEJ8HPWR7X9HfEnrASHEUage6Lvcec+7kdETpZRpIYRuN/a793Mp553bNo+mH5ajesqfl1I+K4R4O4UjzGeiPrD9bd9CfPeVu8+HUAEyzWYpZW4wS3OWEELb6AJABep9conbizJW3fl+lCa7whfJ/iBwrxDiM0XXVOh3ZreUss99TmCadMC8F92oL88w6mtYX9B7gA8IIT5XYJ1xC24hRD3K9nCL29WhiQC9vt/9X5O6W8j/Qh1y19H8RWZnXNjF8ICUk4AT3K9wTZBsr/ozOQ/ercDb3RfxcagurkomNgDEf930B8PTOS+eSlRkPZ/oPgLI15WayxO+//ehXsR+/N1mf0F1fa0E/lrCtvOhX1BHYET3bGIrqnsYIIFqCHMbp2d9grsG1XPyXSHEt33LRFCN2kKUGPKiRlLKfuAad/3c/adREWzNLtS9KhhuKEbjCHe/f8iZfi/ZUZgnc+b3M/K50BxJae+43OeMnG16z5nbHf8w6jmbCF2oc57v6HdOY86009z/V6E+gvzv69z374NacLvcj3oOTgR6SjyOQu/alcBfc2xAu5iY6C6lHeuWUnYBuF3+MYq3m0lU5gn/MYZRwukk4DifgARl8QygvPQa77pKKX8nhDhLCPFF1HN8kvvvRAY9P+MT3KXoh39A9VR/Qgjxa5TN6GcFtn0kI9vUw4wMErwddV1ABSbCFOZhht89GeCwlLLU+ykfJ6H0zZBv2h9R7faJqL/peJgWHWBEt/qKAnjG1yjaqIfrbwqsU/LAyjzol9L7UTenH7/nKp9nsdigplTO7zaqUQf1d76Jkd0xfpGde063onykO1AZDD6K+pIrRO7XYb57K5Yzf4jhRsJPV55poM6/lEERiZzfc9fJvVYW6lrl+8It5RnRDduMH7BkyOJQCV2l/ntW/52vQL2kc5fT991oaUM1GbKfaW33G8t9VOhdZJFtH8x9JvQyhY5rPM9Z7jaLvZNKeV/kI4B5zkAJyYMoG8Uu8AbfPgXeB2IusQL/96Pvm1LfhcXetbn3UL77ZSyU0o6Ntd1My+zBwv5nMIj6eM03qPolhnsbvGvpen+3o0T2XSgL1peK7L+U6+z/W42qH9xxHj9DWWYuAO4UQnxfSnllnn3le9b/hLqvvGwmUsoX9P/dXrdixMfh+S5GvnvVYuz3ai7TogPmtadbqFQ5r0V1/57m+1mFigR9cLL36Q56OQQ0Symf0j8oD9ibiq9dlJOFEP4o02pUpAPgMeCVOftbQ4GBUkKIBpT/6TIp5aellHegHs4ahh/Q3BszgfLHaQp54jWPobqdKnzHtB/4R7KjCH72M/yimwhn+P6/CvWwPMxwo1DsPPI9kDqH+1wcbW9wkVL2ov7GLTnP0ieAN7vzD+C7v4QQlUKI/a7dI5cQ2ZHf1agPUZln2bxCXkrZhxpT8ZqcWWcz/l6Xl5nk50yo9HanMPxOGu19UejDRQ/qntdIKVMo8XmNyJ9G8ahRNvEYcKrITo97JuqefJzS3oXFeNjdvj+qfEahhUtkTO1YiVQIIfwpgVejRN6T7v5WAC/69rcINRCvUC/RVSg72lVS1bD4q7uNktpNoQbILi90sKPpByFEgxDi6yjr581Syrei7GeXFdhkvmf9G8A78r2zhBDV5LfKlZPHgNPdd4hmNardLuVeLfQumRYdMN8j3e9HNXL/nNv9IYT4AWqk95/yrThBbkSlc9qP8lSuRb04zi26VnGaga8JIbahGtwPonx1oITsH4QQbSjv2+mowSCF7DMx1FfzRUKIF1AjdfXgEf0SHQCWCDXg9AVU1+SVQmVeGUANnCj4RSxVNoe7UPlk16Gi219CPUz5RAeoa3VqgXlj4Z+EEL2oqMh3gJ9KKZ9zX3h7getdX+1pqKim/wEbQHVxHiGlPOBOOxX1Inx2Eo7NMLO5EdgkhNiHuuffhxLdurDHP6Hun+dQXe+fQXX7/gWVESSX7wohrkR5Ab+KyioSFao4jJ8B4FghREueAY7tqBzWL6GekUtRg6fOH+c57kY14hPlo+7YkwdQ41iSDA9Wut+dfxeqq/oGRj5nK4UQy6SUz4PXtb6M7EFf85kvoAb47nI99f+Hej+/EzVw7nmGMzfkchtqzNIOobKSNKLE1n9LKR8VKqtFDOUX3oIabPY2RtqUCnE7qn35rhDiSyiB8yGUdWG8jLUdK5XvuWMvGt19fFuqDEK3ogZF3uLaRepRg/+fcr3J+bbVBawRQtyJEnHXoOyZ+p7VVpVVQogH3embXU/xb1ECuZ7iFNQPUsoeIcQa4AihxmDZqIF/hZ6Z3cCnhBC2jvhLKX8lhPgq8N9CZQH5JepeOA/1PlsA/HmUYyyFVXmu4UNSyn0503ag/g4/EEJsdvf/TeDXUsonhBAHUDruBvdeW4OK8D/qnk9aCDGECkzu8m13WnTAvI50o77+7ijgN/oa6mu2HKmpvopqnL+KujHeBVykB3OMk7+gotEPoB6Mj2ofl1SphC5BCYRHUCPMvyil/Gq+Dbm+1stQN+5jKHuJTqeoMwfcibrRdYaVf0S9NP4b5fm6BTXCtxjvR0W+fo7qIq1GVaHrK7D8LyletaxUbkEVW7ib4ZcW7kvnA6iv2kdQmSw+nbPuTahBtr/0TTsP+JWcvpzGhqnjn1HPz42oe//dwMVSyvvc+f+Iapi/h3omlwBvk1IW+gC9HZUh4ceoNFgbCiz3TVSv3ENuo+Dna+7x3IiKrP0tKkPQiJRfJXIXysu6eJzra76POp8HUB/ua1yPO6h31BMoL/qtqN5G//Pzr6jsHI/5jkOnGc219sxL3Hvqb1DtyFWod9kuVHvyRWClLFD9WKr0cRegPvbuR41puhe3IJH7Dv4g6sPtUZSQ/8IYjq0XlY1nGervdS2qzRs3Y23HxsCPUCnn7nB//t7dXz/q+upxGj9D3a/vz78ZQLUZAdTf4m6UxrqR4XbzQVR6ut+iMpvcjXpnfBklZOOotrUYo+mHd6KE+x/d4x1geHxXLjtRH72n+ydKlfr3UlR7+zvU9W5DtdUnyMmpJHkD6lr4f9bkLiRVyso3Myz2f+we0yXu/B7UB90bUdfjQlTmJD//iLp/v+ObNi06wHKcUu2HhpmKyM5JOWdxu7aeB94gx1HOVeTJvzkJxxRApYW7SEqZO5jNYMiLyJMHeSYhhPglsFNKuW3UhfOv/xyTXKZeCPHvqEwLuQ2qwTBmRJ78zvMRd1B4VEpZ6IN/TjHdOmC+R7oNswg3O4uO6swUWoHHjOA2zDE2Ax8RMyT3vBBiCSrq9vXpPhaDYY7RDrxbTEOJ9jlEyTrAiG7DbOMrwJnu1+q04nbzb0TlZjYY5gxSyj+hrGJ/N93H4vJZ4LPSVy7cAEKIU4UQfxBCRIUQfxUqF7bBUDJSymdRFrVrpvtYZiNj1QHGXmIwzCGEqqr4n1LKI9zfdSnki1GpjLZJKbcWWLfkZQ0Gw/TiPq9PoPylX0dVNv0msKzIuBiDwTCNmEi3wTAHEEJYbo7Yu8kuXPAFVHGGV6LK4V4hhLi8wGbGsqzBYJhezgdCUsqbpJRJqaqDPsJw1iqDwTDDmLEpA3fv3h1BNfz7MMUQDAZQo+KXAPevWrUqt3LiF1ApvW5AdcVrrkCVJe4GuoUQX0EVOro1z/bHsmwW5nk1GEZQ7HmdDE5EZZfy8zhwcikrm2fWYBhBuZ/ZmSu6US+D8aa8MhjmMuei0nv5+ZaUcpObFQPwihwtIbuSaN5GeSzLFsA8rwZDfvI9r5NBDdlVjHF/rypxffPMGgz5KdczO6NF9z6A4447jnA4PNqyBsOcJ5FI8MQTT0Ce/OdSyr15VtGloP0Nc6FGeSzL5mO0nOwGw3ylXM9GFKjMmVbFcAGW0Zi0NnZwKMU9u19g5/0v0htVKemrK4K85qSFnHPqYipCFolEgsHBQQ4fPkwsFiOdTmNZFtXV1QSDQWKxGPG4Ci5WVFRQVVVFVVUVFRUVOI5DJpMhlUqRSqVIp9OEQiFCoRD79++nubmZVCpFJpMhEAhQU1NDIBAgFosRiUQIh8NkMhni8bi3D4BMJkMmk6GiooJgMEgikSCVShEOh4lEIti2zVNPPcX69eupqanh1ltvJRQKkUwmGRgYvswDAwOk02ls28ZxHJLJJMFgkMrKSsLhMOFwmEQiQTAYJB6P093dTSKRwLZtbNsmmUxy8sknk0wmyWQyJJNJkskkiUSCdDrtTbcsC8dxSKfTZDLD6aATiUTW75ZlEQgECAQC3jH5p9u2TTAY9Pbvn2dZFpZlkclk1HUfipPp78cajEJ0EAajOP1RiEbJDAyQ6esnMxAt6T6xwiFCdXUE6+oI1tcTrq8jVF9PqL6eYH0dkfp6IksWE5gmzVesjZ0sZrLoTgPezW8wGDxK7QrWb0J/w1yoUR7LsgWPaeXKleZ5NRiAeDzOnj17oHzWjUeBT+VMO54S7GAuk9bGRiIR3vWGE7jwPMG9D3XS8bsneW5fPz/+305+dt9eXrNyIX9z1hIaXDF84MAB+vv7cRyHwcFBKioqCIVCxGIxHMchGo1i27YnhisqKkilUgwNDREOh0mlUiQSCWKxGKlUCsuyCAaDOI7jLVdZWUkwqCROKBTCsiwikQjxeJyhoSFSqRSO4xAIBEgmk1iWRU1NDYlEwpsfDoe56667OHDgAOeeey4VFRWk02lSqRSghGo8HiccDtPQ0IBlWUSjUaLRKPF4nL6+PoLBIHV1dYTDYWzbJhKJUFNTw+HDh0kmk0SjUYaGhujq6iKRUB8s+mNAn6dlWZ441mLYfxzpdJpAQGX31MJZnzvgietAIIDjONi2rYR7Mkmmt5dQbAj6+kj29uH09UFvH05fP05fH068BJeFZUFNDdVHHkF4wQIiC5rUvwsXEFmwgFBjI+GmRgKVlViWNaF7bYoom91qJotug8EwAaSU3UKIl1GDI3Xp8OPJtpCMeVmDwTAj+B1gCSE+hco6dBGqOvBPpuuAQkGb1686mvPPOIqHnzpEx++e4AF5iP996CD3/vUgr125kLesXsyiRYtIpVIMDg56gjcSiRAIBDwhOTAwQGVlpRfZjkQiXgRWR7S16B4cHCQcDnuR4GQy6YnQVCrlCXfLsjwhH41GPcEeDoc9sR+JRKiqqvLE81133QXAhRdeCOBF5CsqKohGoySTSerq6qirq/MiyMFgkMHBQQYHB0kkEhw6dIiqqipqa2upqqryjvHw4cNkMhls26a3txdQoln3AqTTw9pPR6u14NbRbz/BYNATtfrcAOx0Gqe7m0xXN4HDh8l0HYZDh3D6VUylWNltOxwm1NREeEETkQVNhJqaiCxcSGSB+je8oIlwQwMPPPggp61aVWRLBpjlorujo4P29nb27t1Lc3MzbW1ttLa2TvdhGQwziR3A54QQD6MsJNeiSplPdFmDwTCNSCkTQoi3AN9CFTN6DninlPLgtB4YKgJ86opFnLpiES/u7+NH/y35n7/s5fcPH+JPjx7m/FMX8GqxgHQ6TTwe96K5kUjEE92O49Df3091dbVnJbFtm1AoRCAQ8CK5WqRqu0YgEPAixlVVVV7kW4trbbuorKzEtm1isRiJRIJQKARANBolFApRU1PDvffey6FDh2hpaeGEE07wPhIqKyuJx+OkUilqamqoq6sjkUgQDoepqakhFAoRDAYJBAKeeO/t7fWsLPr4dQQ7FAp5Alqfv76OehltN8kV2ppMJkMiHifY14d9qItAdw/B7h7sw91YrqAfgW0rEb1oofpZ6P67aBEVixYSXrCAYE3NbIlOzwpmreju6OjguuuuIxaLAdDZ2cl1110HYIS3wTDMJlQVz0dQKUK/g2qkEUIsRUWy3yKl/H2xZQ0Gw8xDSrkHOGe6j6MYRx9ZxzWXncXFb+jjB7/cw/2PHeTu3Qe5d89hzjuphmMaU2QySnxrYaxFcywWY2BgIMsCoy0hVVVVnmANhUJ5fc5aoGtLhraUaIGvhfHAwADxeJxIJOIJ4Hg8zq9//WsA3vzmN9Pf3086naaqqsqzlQSDQRoaGrwPAC28w+EwdXV13n4ty2JgYMCz1FRUVJBMJrP81Nqzrb3h6XQ6y0KSF8fB7usnuHcfoX37CO19GcvVRFkEAlQsWUzV0qVUL19G9bKlVC1bSsURR2AFZkTR2XnDrBXd7e3tnuDWxGIx2tvbjeg2zFuklPcADb7fh4Cr3J/cZV9geABl0WUNhrmM6TUtP8uW1LHpQ2fz2LNd3PKLPTz2fA+/fqCXRXUBXv0Kh+ZGNdgykCMCu7u7CQaDnv1E20ts2yYcDhMMBqmvr2dwcNDzYuvIcDQa9USytrHodfWAwkrXZ9zT00MsFqOyspJQKERPTw/33HMPlmXxtre9zbNrZDIZL3LuF9x6wKI/4l5XV+etk06nGRgYYGBggFgs5glrjR48CcNWknxYAwOE9r5MZP9+Ap17sfqzh90EGxqoWXGsK66XUb3saCqWLMF2I/mG6WXWiu69e/Mlayg83WAwGAyGXEyv6dRywjEL+PK68/jDX/fyvZ/t4WDPEL94EI5bbHH2sQ4VYTXQz2+3GBgY8DzRGh0ZtiyLqqoqT6zrzCihUIh4PI7jOFRWVno+72Aw6G1bDyrUkene3l4GBweprq7mwQcfJJFIcOqpp1JXVweoAaP9/f0kk0lqamo8n7k/2wcoAa0j1tXV1TiO40XytTDPFdY6up+XVIrqlzoJ7XkUa9/LWbMCNTXUrTyJhlNPpuGUU6hsaTZ2kBnMrBXdzc3NdHZ25p1uMBgMBkMpmF7TqceyLM4+pYVVJyzmP3Y+wY9/9yRPvOzQNeDwrlUQsLNFY39/v5d+LxKJZKXnA7yItY4wDw4Okk6nCQaDno1DC2ydKs+fhlDbVOrr6+nv7ycWi3HwoLLGL126lFQq5fnD9Xa1DcW2bU94A56dREfk9fHp7CW54rqyMjfrI946tek0oUceJ/PQQziD6h61IxFqV55Iwymn0HjaKVQtXYplm+Lis4VZK7rXrFnDjh07sr4WKysraWtrm8ajMhgMBsNswvSaTh+RUID3XXAC557ewqZv38eh3gQPPO9w1jEjI7Xd3d2EQiHP0mHnCE3Hcbz0fLZt09/fTyqVIhAIeBFvnUpPR6i1vUNnCwmHw9TW1tLf3080qrKohkIhb4Cn9nFXVVV5PnIdvfb/6G3rqLaOsudaZ/JRVVlJ9aHDOPf/mfRTT5F2JU7VMctZ/JYLOOJ15xKoqJjYhTdMG7Py86ijo4M777wzS3BblsUll2n8nPsAACAASURBVFxiIhOGGUdHRwerV6/mqKOOYvXq1XR0dEz3IRkMBpdCvaOm13TqOPrIOja87ywAHnxeRbxz0QVpBgYGPI804Ilejc633dDQkGX/GBoaYnBwkGg06g261DYWbQ1xHMfLQKIj1zqyrQc1BgIBb4Cml+/aZy3xR+CTyaRX0EcXx8klFosRjUYJWhYNL3ZScfudpH54O+knn8IKBFnwuvM4+catnPZPX2HJm99kBPcsZ1ZGuvN1BzqOw86dO6fpiAyG/Bi/qMEws2lra8t6RsH0mk4HJx+7kDe/6mh+86cX+R/p8M7Twc6xmfT29nrZQerr6z2LiMbvZdZ5uaPRKIODqtCuHuQYDoe9+X6RrG0rtm1TX18P4KUJ1Pm+teD2i/1cQa2Ftraj6EGS8TyFZqxUiso9j1D77PMQHcQBgvX1LH7rBTS/9QJCrp/cMDeYlZHu0boDTWTRMFMo5hc1GAzTT2trKzfeeCMtLS1YlkVLSws33nij+SieBj74jpNZUBfmYJ/Dwy/lX6a3t5eenh7i8bgnuP2RahgeIBmJRLyCNDpardMQ6sGOuXmvde7wmhqV2CkajXrvcO0p92dQ0XYSnY5Qb1OXpdeCW+fZ9mPFhlj4q7tZsOcxiA5SsfRoXrnuKs76/rdZ9p53G8E9B5mVke5igyg3btyY5fU2kUXDdGL8ogbDzKe1tdW0DzOAqooQ6y89g89994/8+dkMxywKUl85MsvH4OAgXV1dLFq0yLOP+P3U/qIywWCQmpoagsEgfX19DA4O0t/f73mzdZEaf7Q7k8l4mVIGBwc9Ya6j3H5x7S/PrqtE6nn6/+l02ou2Z53vH/5IqLePvmCA1Z/eSNMZp5nMI3OcWRnpbmtryzvi9+DBg9x6660jUvGYyKJhumhoaMg73XEc0wtjMBgMOZxx/JG8/oxm0hm470lnRHsOKvo8MDBAT0+PN2BRo1MJagGuhXdFRQV1dXVeNcm+vj4vOq1//JHvpqYmQEXW/cLcL+yBEfv2e7y1xSQWi404D7uvn9DTz+LYNrdE+1iw6nQjuOcBs1J0t7a2cskll4yYXizPZb7IuMFQTjo6OhgYGCg4X/fCGOFtMBgMw3zonadSUxnkxa40zx3O3yGfSCTo6enxSqPn2kv8/+r83BUVFTQ0NFBRUcHg4CDd3d1ZUWntEQ8EAjQ2NgJw+PBhzwOuo9b+ZbXo19vwR7cTiQSJRMKrKukX1eFnnsUCehYfScwUrpk3zErRDYx50GQpqXoMhsmkvb3dqzBWCNMLYzAYDNnUVYf5u7efBMC9jycZSo6Mdg8NDZFIJBgaGqK/vz8rg0i+6LgeIBkOh2lsbCQcDtPf309vb6+XV9tfJr62thbLsujr6wPwKmL6RTRk20r8EXO9nJ6XS7BTWQwXveZVfP7zn5/wNTPMDmat6B5r5NpfbjUfevBlS0sLS5cupaWlxXT/GyZEqb5t4+82GAyGbN60ehnHL6tnMOGw+/n8UkW36wcOHCAWi2UJb43fCqKzjoTDYZqamrAsi+7ubvr6+rzotCYQCHgZUgYGBrx83XrwpH8//qi3/tHZSvQ2Q/5odiZD8IAqvnPG375j4hfLMGuYlaK7o6NjzN6nlpaWotu77rrrPCGvHxLT/W+YCKXm+TX5gA0GgyEby7JY9+4zCNgWe15M8XLvyOi1jiBHo1EOHDiQZTXxl3rX2wO8qpWRSIRFixaRyWQ4dOgQiUTCW0Z7sf0WE/+6Ome33keu5USnCvTbVXRhHgC7pwcrlSJdW8PX/uVfuP3228t1GQ0zjFkputvb2/N2HxVitJyr+dK6aWKxGNdff/2Yj9FgKDTg108oFDL5gA0GgyEPSxfX8Y5zlwNw7xMO6Ux2u69tHrZt09fXR1dXlyd+/YMpgayMJnpaZWUldXV1pFIpuru7ve3qwJseTNnT0+NFt7U/3J8WMNfjrUW3zvutRbcW6cEDhwDIHHEE9913Hw8//HCZrqBhpjErRXex7vjt27ezffv2MeVcHa17v6enx0S75zHjzfuu8/8WE97JZJL7779/sg7VYDBMMqbuw/TyvgtOZGF9hK4Bh0cLNNW69Htvb68nvP0+6twS7Vo4A9TW1hIOh4nFYp7NRK+jRbeOdOsUgXqMmBbauuy79nf7rSp6X7r6JUDwoBLdjSeeMMlXyzDTmZWiu1B3vLaQtLe3s3fvXpqbm70oYrGXZind+2aw2/zEbz1yHGeE5aiUBnloaKjoPnbs2GEacoNhBjLa828oPxXhIB951ykA/OX5DMnUyF7uVCpFfX09mUyGrq4uBgYGvAGNfhGt0YMqA4EAgUCAhoYGbNv2iuaAiowvWLAAUKJbi3W/cNei21/2Pdfv7V/Wy6ZySInuo846c7Ivl2GGMytFd75u+8rKStasWTPiBblhwwY2bNhQ9KXZ1taWPcghD2aw2/ykUEXJ66+/vqQGuRQrlOM4xsJkMMxATEXZmcGrVy7hlS21xBLwSOfI92lPTw9VVVVUV1d7Hm1/yfVC72BtQQkGg9TW1pLJZOjr6/MyjuR6unN94oDn3dZWE7+XW+cIz1onkyHQ3QNA/bGvnOCVMcw2ZqXo9pftBdV9E4vFuO2220a8IHX5VT/+l2ZHR0dJqd3MYLf5SaEsOT09PWzatClvg3z11VfT0dFBR0dHyVl2jIXJYJh5mIqyMwPLslj7lhMBePBFh0ROtDudTnP48GFqa2upqKggHo9n2Uz8gyn91Sf9mU2qqqoIh8MkEglisVhWpFtvCxjhGdeC2x9R18Lbb0XR69v9A1jpNJnqKoLV1TQ2NnrVLw1zn1kpukEJbx3x1jf7aGkB/XR2do7IWlII27bNYLd5yGgi2D/wxk86neaTn/wk69atG9P+TPTMYJhZFAq2mCDM1HPG8Udy3NF1xJOw56WRkeve3l5s26a+vp5gMEh/fz89PSqi7LeBaA+2P5uJTgNYWVmJZVkkk0lSqVSWp9sv3rWY19vIHUyp5+kBlHpwJUDAPaaMG0X/7ne/y8aNG8t45QwziVkruqF41pHRsCwrb6QyH/kS2xvmPhMRweO5Z0z0zGCYWRSyMpogzNRjWRZr36oK5jz8okM8J9rtOA4HDx7Etm2qqqq8HNzRaLRgGkG9XR2RDgaDBINBL8e2tpcccj3Yue91fyl4HeHWvwcCAUKhUFZEHMB2rSWRFvPhNh+Z1aJ7IiLFcZyCkcp8GM/t/GO0+2usueJHw0TPDIaZhd/KWGo2LEP5OO24Izh+aT3xFOx5cVg4RyIRAPr7+4nH41RUVFBVVUUqlaKrq4tEIuEJXy2c/YJZY9s2FRUV2LZNKpXybB9dXV3AcERbr5f7fx3R9gt5ndVEoyPdC45bAcDWrVv5wQ9+MPkXyzAjmdWieypFivHczj9Gu7/Gkit+NCzLMtGzWYRJIzd/aG1tZdeuXbz00kvs2rXLCO5pZu1blbf74Zcc4slhu4f2TutgWiQSoaKigmQySW9vr+ezBrKi0toCoqcHAgHC4TCZTIZIJEIoFGJgYIBYLOal/fPbSfz+br+g92c3yap02dMLwBHHHw/A7t27kVKW9ZoZZg6zWnSXUnxkMjGe2/nFVN5fa9euNY35LMGkkTMYpo9TVhzBicvrSaSUzQRUwoRwOAyowexDQ0MEAgEqKyuxbZtoNMrg4GCWUIbsaLX2doMqWmbbNul02vN1Hzx4MCsziX9gpX9b+gMgFAqNSB+I43iZS6qWLi3zlTLMRGa16G5tbeWSSy6Z9G7+QhjP7fxCdy1PBVu3bp2S/RgmjkkjZzBML5e/bSWgBlT6o91aeHd3d3sp+yorK3Ech4GBAYaGhrzIc26lShgW3qFQyKs6qX3dBw8eBBghpPVASf8gTR091xF1PYjSikaxUikylRWE6kzGkvnIrBbdADt37pzUbv5iGM/t/KO1tdVLTVkuyr19w+Ri0sgZDNPLSa9YyInLG0ikh/N2x+Nxr95GPB737CChUIhIJEIikWBwcNATwNpr7Y9U+8vEh8NhbNumoaEBUIMp/ZHy3MI4/lSCOmLut7QABLqVtcRxo+eG+cesF92l5kGeKGbE+vyllOJJ4yUUCpX1vhJCXCaEGMj5SQsh7s6z7Bvcef5lzQjiHEwaOYNh+rn0TQKAv77kkEwrYZtKpbxot7+ku84ioq0nupBNbr7t3PzdoVAoK9Kda0/xC3a9rWAwSCgUGpEjHIYHUYYXH+lNW7JkiZcP3DD3CU73AUwUPTp4sqmsrCSRSJBOpwkEAlxyySXGczsPKbV4Uik0NDR40RaAxsZGNm/eXNb7Skp5G3Cb/l0IcTpwN/D3eRY/A7hTSvmesh3QHKCtrY3rrrsuy2JiPsoNhqnldHEkxyyp5tl9UeQ+h5VHWcTjcWpra72Uf/F4nKqqKgDC4TDJZJJYLJaVzg/wCtlo24kW4oFAwPN06wI5uYMmdTTbK/HuK4aTO4jS7usDoHb5Mm/a9u3b2b17d5mvlmGmMOsj3eUQ3IAnuPU+7rzzTjNQag7Q0dHBSSedREtLCy0tLaxcubLg33Xjxo2sX79+UnpTGhsbeeSRR3jyySfp7Oyks7OTPXv2TOmHnBAihBLgn5dSPpRnkVXAg1N2QLMUk0bOYJh+LMvi0jepDCAPveCQzgwPqqyoqABUwRydwk/n4U6lUsTjcVKplOe59keltb1E/+uvSqmX1QLbn5dbr6vLvvt93ppAXz8ATcccMwVXyDATmfWR7paWlrJYTHLFvB4oZRrW2UtHRwcbNmzIilp3d3ezYcMGgKy/bUdHBzt27Ji08QKbN2+elO1MkKuAGPCNAvPPABYJIa4ELOAO4LNSyvgUHd+sobW11bwLDIZp5jUnt9BY/QDdUYen9juIJRaJRILq6mps2/a83TU1NVl5sxOJBIFAwBPWuV5sHe1Op9MjRDdk20ly0wTqablFcWA40l2/fLk3bdOmTRw4cIBVq1ZNxSUzTDOzXnS3tbUVLbe9fft2WltbvTRf461gCVPnHzeUh0I2kWQyybp162hvb6etrY3W1lba29sndYDudAs0IUQYZSn5mJRyxIkJIYLAS8BPgFuAZuBOwAGuK3U/e/bsmZTjNRgMhtGwbYtzTqzlF/f38eALDsctVpHlVCpFJBIhFosxMDBARUWFF4HW0e6hoaGsyLRfPGvbid9e0t3d7dlPcgdT+nN+A55nPKuCZSaD3T+AA1QceYQ3+dFHH6W/v7+s18kwc5j1oru1tbWo6NZiR//b3t4+bvFsWRYdHR3TLqAM42O07BKdnZ2sX7++6P00HmZIdpILgAzwy3wzpZQpYI1v0lNCiC8CX2YMonvlypVedTiDYT4Tj8fNR+gUcNora/n9o4P0RFO80AXLFg5bTGzbJpFIEIvFvOqSOuKdyWRIJpMjPNz+FMR+e0l3d3eW2M7NXqIj3RptX9HY/QNYjkOmpgbbHexpmH+U3dMthDhVCPEHIURUCPFXIcRZk70PndJntOm6slhnZyeXX355wfzehTJVOI5jcvHOYkrJLjHZ6SfLnZ1kDPwt8CMpZSbfTCFEixDiK25EXBMGhqbk6AwGg2EcBGyLt5+jPNIPv6hebzotoA4ADA4Okkgk1PK+gY6JRMITx4X0QGNjI7Zt09fX53nBC2Utyd2GP9KtrSU05tcrhvlBWUW324D/DOUNbQC+CNwthKibzP1s2bJlhFAOhUJs2bKl4Dpbt27l5ptv9qKQ+kFsaWlh27ZtBdfr7Ow0AypnKWvWrBl9oUmksbGRbdu2zZSekVcD9xWZ3wVcBnxWCBEUQqwAPouymhgMBsOM5W3nriAchL09cKg/O293MBgkmUxmVaQMBAJemfZEIkEymcwS0vpfbT/RATx/tNuftSTXpuL/0ehBlKFFC6fsuhhmHuWOdJ8PhKSUN0kpk1LK24FHgEsncyetra1s27YtK5tAKWLHH/l+4YUX6OzsZNeuXbS2thaMngNs2LDBCO9ZyM6dO8u+j+rqarZv3z4t2UlGYTmQ5a/RObwBpJRDwFuA81AC/H9Rnu7CX6AGg2FaEUK8SQixWwjRJ4R4Sgjx0ek+pumgujLEeaeq3NcPv+hkVYkMhUKk02ni8bg3TQ+gBJU0wf8Dw4Jbo3N19/T0eBUm/RlP/CIehgvl5BtEWZVjN3zFK15hcvzPI8rt6T4ReCxn2uPAyZO9o8nOJlCstHwymeTqq69m/fr1NDc3e4PvDDObyagYqL1/hXjiiScmvI9yIKWszjMtK4e3lPJB1IeywWCY4Qghjgb+A7gC1aO8CviNEOI5KeVvpvXgpoH3XHAKO3f/F08fcHjVKzPUVgaIx+NUVFQQCoVIpVLEYjGqqqq8cu/al51KpbBt2ysdrzOQ6Eh1U1MTTz/9dFakW4tsXUTHn34wn36w3Uh3gy9zCcCNN95o8nTPI8otumuAwZxpg0BVqRuYroEoPW7lqELoL+LOzk6uvfZann32Wc4///wpODLDeIlEIgwNjd+iHIlEeMMb3sBvfvOb7FHpLosWLTIvT4PBMFUsB34opfyJ+/v9Qoh7gNcC8050H9lUxUnLqtjz/CB7XnJ4zYrhKHYwGMyykfij2DqQkk6ns6YDnhD3R7pzo9m61HwikfAymWjx7SfgRrqbXmFydM9nyi26o0BlzrQqYKDUDUxXNoTm5uaSs5zE43HuuOMOrrnmmjIflWG8bNy4cUKCG+Css87ie9/7Xt70k5WVlWzatKmsuVZNNgSDwaCRUv4e+L3+XQjRBJwL7Ji2g5pm3nPBSj777V08vs/hVceCjRLT4XDYs4Ukk0kvmu3Ps61FtLagwHCPd24GE3/aQMuyCIfDxOPxrKI5WT2ijoM9EAWg4ojhdIEA1113HQcPHuSWW8zwmflAuT3djwIiZ9rx7vQZzVgzTpgc3jOb2267bfSFRuEPf/gDYCoSGgyGmYUQoh74OfAnlNVkXnLKisUsrLUZSsJzB9NeaXfHcQgGgziOw9DQUHZpdje6rUV5vsI3Olf34cOHAbLSBerBmn6vd242FCsex0qlcEIhgjXZTr9nnnlmUqyPhtlBuSPdvwMsIcSngK8BFwGnoApwzGhaW1vZtGkT3d3dJS3vz88JqqJhe3s7e/fuHZPvu6Ojg+uvv96ztzQ2NrJ582Yj6CZAR0fHiAqj48G/DVOR0GAwzASEEMehhPajwGWF0oIWYi70nvltfcceCYf64cmXMyxfqFIH+oui6UwmOsWfv3qkP9e2Fs3+QZX79+/32ma9/NDQEL29vV46wXzYA6pzP1NTPcKCqAvjzAVr4lw4h3JTVtEtpUwIId4CfAvYDDwHvFNKebCc+50sNm/eXHKhFL8gy7UfdHZ2ct11qr5IMaE2ljLlhtLQ13QyyP2wMhgMhulECHEeSnB/C/h0vmqzozHbC1rt3r07y9a37JUn8sctd/N8F8STDtWVIWzbpqKigmQy6Xm8q6urvei3Hkip820HAgEvBaDjOF6EOxqN0tTU5A2+DAaDNDU1UVtbS2dnJ0NDQ17RHZ0XHPCsJYGmphEWxNraWvr7+2d9Gfjcv8NsZCosnGUvjiOl3COlPEdKWSulPFlK+dty73OyGIvI9Vcd3LRp04hy87FYbNTCOsXKlJuiPOOj0DUdD5dddtmkbMdgMBgmihDilcB/ApuklBvHI7jnIgsbKll+RJh0Bp456GQNlAyFQl46wVQq5UWm9YBJwBsE6U/75/d0w3CU21/dMl+VSo2OdIcXmhzd852yi+7ZTqklvLUHvKOjo6AlpZhvq6Ojo6gv3Hi+xsdo162Uv28gEODyyy9n69atk3VYBoPBMFGuAmqBrUKIAd/Pl6f7wKabN65eDsCTLw+XbNd+bZ0qUP+ue6m18PYXx9GZSHTdjp6enqzy7roojhbb2j+em7nE7leR7sojswdRApx44okcc4zJaDJfKLene9bT1tY2wvKRi3/AxKZNmwouVygBvrajFMMkzx8fDQ0NBT+CAoEAbW1teTORmIGRBoNhJiOl3ABMjndujvGm1xzLLXc9wb5e6B5I0lQb9qLXWlxre4jOsa3FOAxnHwkEAl4ebm0DGRgYoKamxhucCcPl4PX/R4juqIp01x111Ihj3bx5s/FCzyNMpHsUdLXLYhUqHcdh/fr1XHrppUUHXhbKiNLe3j7CjuLHsqwxZ1MxKIoVskmn0yYTicFgMMwxqipCnLhUlQN58uXhNsBf/EZHsf0Rby28/bm4QbUj/lzd2lqiRbc/N3duJUoYjnTXHz1SdBvmFybSXQJagOVGRP04jsO9995bdDval50r6EazQBQTjobi9Pb2FpynrSUmE4nBYDDMLd78mmP563MP8/QBh9XHDpeGDwQCXirBdDqdlbHEL7z1PO3fbmpq4oUXXqC7u5ujjz7aK/0O2WXf87XXOtJdkcdesm7dOrq6uvjhD39YxqthmCmYSHeJjBaNLoXOzk42bNhAR0dH1vRSrCNmIOX4KHRtTe+BwWAwzF3OPm0p4SAcjsKhvmTWgEotmHWUW6cNzFe+Xa/j93UDXpaT3AqVI0R3KoUdG8KxbcJutNzPvn376OrqmuSzN8xUjOgukckayJhMJrn++uuzprW1tVFZmVu4szz7n2/ku7aWZbF27VoT3TYY5hgdHR2sXr2ao446itWrV48IcBjmD6FggJXLawF45oCT5deG4bFYOuLtn55b6CbXXqKtJX5PN5A/c0lUWUuc6ios20iu+Y65A0qkmKd7rOgvZY32FRfbx2QNpJxPjZIuUBSLxbxuwJaWFm6++WaTicRgmGPoAemdnZ04juPVR5jL7zhDcV5/lsoK8sxBJ6twjbaOaIGdOwjStu0R/m5/pNufz1sP0CyYuWRQ9ZBbtbVlP1/DzMeI7hIpt6+6tbWVRx55hMsvv3xEF1dlZeWkWCHmU6PkP1dQL1l9HU2E22CYe+SzAJZSH8Ewd3ntaUsJB6BrAHqimayItL+d1YMgtRDXIloL6kwmQ11dHZAtujVabOeLdFvRQQAC9fXlPl3DLMCI7hIpNiBvrDTm8XVptm7dys0331yWbBrzqVEqdK651h6DwTA3KGTBM9a8+UsoGOAkbTHxFcrx59n259jW/+pIN+CJ6XpXNPf09HipBjXFgnL2oBLdoab87f6qVasQQkz8ZA2zAiO6S2Qy82SfdNJJRee3traya9cuXnrpJXbt2jVpkdlyNkpTZVspdT+Fzqmnp2dORvYNhvlOoXe0qXEwv3n9WcsB5evWvm0tpLUI1xFqPS2fXcTv6fZnLslNL5iLFt2Vixblnb9x40auuOKKyTlZw4zHiO4SKTQgbzzcd9990yL8ytUoTZVtZSz7KXZOxQoYGQyG2Um+d/RkWfMMs5ezT1tGKACHBqAnmvam+ytRQvYgSF0sB/CEud9eEg6HPdGdGz3PRYvumsVHluHsDLMNI7pLJF8Rlde+9rXj2pbjOHktHeWOFperUZoq28pY9lPsnIoVMDIYDLMTU+jKkI9IKMCJy2oAFe32F7zx5+X2R7d1tBuGo+I1NTXYtk00GiWVSpUcdNOe7tolS/LO//CHP2wG9s8jTHGcMZBbRGXlypXj3lZnZyerV69m7969NDc3s2bNGu68805PVOoort7vZKC3097e7u13MgYWTpWXciz7aW1tZd26dZO6f4PBMLMxha4M+Xj9Wcfw0DN/5fkuh9OXDw+i1BHq3EGVuuJkbgS7vr6e7u5uBgYGaGpq8tbTwjwfOtJdsXBh3vnd3d309/dPynkaZj4m0j1OOjo6Jhwx9dskduzYMSXR4nL4xafKSznW/RRKwTiZ6R8NBoPBMLN5zSlHE7Bgfy8MxrML4Whh7U/9pwW3Xi43baBu+/1iPa+9xHG8lIHhpqZynqJhlmBE9zgZTQxrv1epFPKDzYaR91PlpRzrfrZs2ZI1whwgFAqxZcuWST0ug8FgMMxcqipCLF9cgQO80JU9cFK3vX5LSb7Bkel0OiuDST5RPoJkEiuVwgkFCVYVL4BnmB8Y0T1OionhUCjEZZddNmqVyVJwHGfGF7GZSi9lJBLx/t/Y2Fh0P62trWzbti3ruLZt22a6nw0Gg2GecfYpLQA83+VkZS7xD57UP/7qlFqYW5bliW4d6favmw/b9XM71dXlOzHDrMKI7nFSyNJgWRbbtm1j69at3HjjjWOKeBcamDGXi9iUis5c4q/mOTQ0VHT51atXs379egBuvvnmSU2/aDAYDIbZw/lnvQKAlw5DKp0ZIZhz/dnaauIX59pe0tXVNcILng/t5y5WjfK1r30tp5xyysROzjBrMKJ7nBSyOtx8882esGttbeWmm24qOeK9YsWKgiJ9JhexmYqUgWPJXLJx40bWr18/LypvGgwGg2F0jmis4siGIMk07O0mb4Rb/w7DAyr9ubt1ru6urq6sZQuhRXegvq7gMp/61Kd4z3veM7GTM8wajOgeJ6VaKnKXK8aTTz7pdWvlY6YWsZmKlIGlZi7p6Ohgx44dI16G87kapRDig0KIpBBiwPczohqDEGKpEOK/hBD9QoinhRBvnY7jNRgMhnKwSqgCNc91OV56QH8Gk3yDK2G4bLw/0q2xbbtg2265gyhDRapQG+YXJmXgBCg1PZVe5vrrr8+yR+Qy2lfzZBWxmey0hFORMrC5uZnOzs680zUdHR1cffXVBa+jrkY5Dy0mZwBflVKONrL1duAPwNuAc4CfCiFOk1I+U+4DNBgMhnLzhlcdy11/2scLhxycFcPtRK74zmQyXgYTYESk+/Dhw956xbDdtraiQAl4gPe///309vbyi1/8YkLnZpgdmEj3FJDPjzxWRssGUkoEu1wR6alIGTha5hJ9jYv1FMDoWWfmKKuAB4stIIQ4DjgT2CSlTEgpfwv8HPh/U3B8BoPBUHZWHN1IdYXFQBwOR9U0fyl4GBnl9pMrukfrvbZiatxRfb7p6AAAIABJREFUdYES8KDGJiUSiTGfi2F2YkT3FJBP7I6F0bKBlOqpLldEeipSBo5m5yn1Gs+GFIyTiRAiAJwCrBVC7BVCPCWEaBNC5LYWJwIvSCmjvmmPAydP1bEaDAZDObFti5OWqQwkL/osJkDWwEj/ND2o0rKsLNHtF+UFs5cMjS66DfMLI7qngPEKPcuy2L59+6hZN0qNYJcrIj1VKQOLFfbJZz3Jx2QX7JkFLAL+DPwAOAa4GLjS/fFTAwzmTBsEqsp5cOUYY2AwGAyFePUpRwHw4uGRAylzUwn6fweorq4mFAoRi8WIRqNZojwfliu6KxcsmIIzM8wGjKd7CijkRx4Nx3FKEq6lRrDb2tqyPN0weRHp6Si/3NHRMapP3o9lWZNesGemI6V8GXidb9KDQojtwEXAN3zTo0Bump0qYGAs+9uzZ0/Jy95zzz18/etfJx6PA+rD6dprr+XZZ5/l/PPPH8tuDQaDoSRedfLRWHfu4eVeSKYdIjmhx0LZTEDV4GhqamL//v0cPnyYqqriMQnt6Q4VyV5imF8Y0T0FtLW1sWHDBpLJ5JjWa2hoYPXq1ezdu5fm5mba2tryCttSBhkCWVaM0bYJStSWuux4lp8IHR0dY76ma9eunXeDKIUQJwHvllJ+zjc5DOQmOX8UWCqEqJRS6q+y493pJbNy5cqsAkbFuPLKKz3BrYnH49xxxx1cc801Y9mtwTDjiMfjY/oINUwNddVhmheE6exKsLcbli1UgyaBvIMpdVl4UIEbLboPHTpEc3NzVtaTLBwHa0i930JuUZ18vPGNb+Sll16a/BM1zEiM6J7BRKNRL4pbLNNIKRHsXEHszyeej7FmOilXZpR8+2lvbx9zz0FDQwNbt26dtOOYRfQA1wghXgK+D5wOrAc+4V9ISimFEA8BXxRCbATOBv4WeE25Dmwqst4YDAZDLqetWEhn115ePOywbGF2msDctIG2bZPJZAgEAti27aUN9FelzCu6k0msdBonGCRQJBDxsY99jN27d0/yGRpmKsbTPQW0t7ePKSJrWRbV1dUj1imUaWQ0T7WOCvsHWm7YsKGof3bTpk1jynQynswoY/Xz+geMjgXLstiyZcuY1pkrSCk7gXcAHwX6gP8AtkgpfyyEuEwI4bePXAScABwAvgf8Pyll2UJ1U5H1xmAwGHI55/RlALx0ONtC4s9gotFl4G3bxrZtrxS839aYz9Ntu5lLnBKL4xnmBybSPQUUi9zpQRqBQIB0Ok1LSwttbW1e+fJSt1XMU71p06YRAj6ZTLJp06aCUWv9FV/q/sc6fePGjVlFbEqJjI83C0yp3vi5ipv+78w8028DbvP9/iLwlqk6rnKOMTAYDIZCnHDMQiIhi96YQ1/Moc7Vxbo99g+mzI1i5xPd+bKX6EGUVBUX3RdffDH9/f385je/mcAZGWYLJtI9BRSL3OmHevHixVmZSnQX1li2VYhCArrQ9GLR6bFGJ/NNL1Y1sti+x2s7aGlpGdd6hvIyVVlvDAaDwU8gYLOiRQ2C1NFuyD+IUkextXdbi+7e3t4Ry/vRgyjtmpqynothdmFE9xQwWuQuN7d2R0cHvb29I5YLhUJTEgUsZt8otP+x5Opub28vmNe02L7H88FhIqczm2JpIA0Gg6FcnHWiak9e6i5cVbJYpFuLbshfJEdHugO1RnQbhjGiewpobW0dNbUQqEjv9ddfz6ZNm/J6xJLJJO3t7WPKZVxs2ULR9EAgkHe6ZVkFRdFYopaj2W0KsWbNmoLz8mEipwaDwWDIx2tOVb7ufT2MiG7n5u6GYetJPtFdzNNdLHOJYf5hRPcUUaoXuaenp6DtAyhYbRLyD0wsZtcoNLiwUCn1QtFpTWtrK21tbTQ3N7N3796CHwij2W0Kndudd95ZdP+5DA7m1noxGAwGgwEWL6iittJmKDlcEh4YYRfx/25ZFrW1tQB5e6P96Eh3uMGIbsMwRnRPEZOZkSGf97lQKfhido1CEeBCHujRvNGllqNva2srGtHO96EwnkGU3d3dBT9QDAaDwTB/sSyL445S1o+9PdkBpdyot2VZ2LaN4zheKfienp6sEvEjtu/WIKhqaip6HBdeeCHnnHPOhM/HMDswonuKyOd5ngi5Fo1CKfsKUchCAvmP1bIsOjs7s1L75UbWC6UZXLduXdZ6ra2trF27tuRzKzStFEYbnGmYm5jy8gaDYTTOOGEJAHu7s0W2xp/FRM+vq1PVJf0DKfPZS6x4AoBKV6QX4gMf+ABvfetbJ34yhlmBEd1TRD7Pc3V1dd5lGxoaCIVCRbeXGzkfa+7qdDpdUJD4jxWGXzx6P9dddx0bN24cEdUeiy1m69atXsRgtHMrNK1UTLGV+UWpPS4Gg2F+86qTlwLDvm4/Oj+3jmLrfyORCKFQiHg8ztBQbmHfYWwd6V6woOgxxGKxEZV5DXMXI7qnkNxMDRdddFHe5VauXMl73/veghaMfNUmCy1bLKJdTJBof3YgEMib2u/WW28ds90jN+r89re/Pe9yxxxzzIhpE+kpMMVW5hfjKdRkMBjmH0c2VVFfZRNPQddA/mX8gyW1lcSfq1uL81x0pDs0SsrAtWvX8oUvfGECZ2GYTRjRPY3s3Lkz7/T77ruPX/ziF3kf5EAg4GXk0F3o69aty//QWxaXXXbZqGK1mEe80KDK8eKPOhc7/9Gi76ViUgbOP0x5+dEx9pu5hRCiQQjxghDiA9N9LLON445SAyP3dmcPnszFtpVcsiwry2JSCCuhotdBk6fb4MOI7mmkkAhwHKegVUOL4JNOOol169YVtZU4jsPWrVuzbC2lHst4qz82NDQUFcY66tzR0VHw2B3HyfsR0N7ezt69ewumOtToIgYmZeD8xJSXL46x38xJvgWYKmDj4PTjFwPQmWcwZW66QMdxSKVSWZHuQlm9dKQ7aPJ0G3wY0T2NjFcEbNiwIasEbSG0+PXbWgoJYv+xFBPExQiFQmzZsoVdu3YVHI29Zs0ar9Evhv8jIFckjHbuN998sym2Mo8ZS6Gm+Yix38wthBBXAHXAX6f7WGYj2te9vzd/hDu36qRt257o7uvryz+QMpXCSqdxbBs7HC7vCRhmFcGJbkAI8Ungk8ACQALXSCl/7847FfUFfgrwDPBBKeX9E93nXKGtrY3169fnfdAbGhqyku/7SSaTo247n8jo6OggGo0WXbYUQTwaHR0d3HfffXnn7dy5k507d44aRfd/BIwl6t7Q0GCE9jxH//11z0hzczNtbW3mvnAx9pu5gxDiGOBzwNnAr6f5cGYlixorqamwGBhy6BmExjz5DXTvaTqdzrKX6ABQbjut0wU6kUjRHmbD/GNColsI0QpcB7wJeBy4AvhPIcSxQC/wM+Am4DzgIuBuIcQyKWXfhI56jtDa2sr999/Pjh07sh7ayspKtmzZwrp168a13ZaWlhEiQ4vpXPHa2NjI5s2bs4TKeGwlMFwxEwoX0im1YfdXnyx1HcuyChb8McwvWltbjcguQHNzc96eLGO/mV0IIQLAvwHXSilfFkKMazt79uyZ1OOaDnbv3j2h9Y+sU6J7f59DY/VIkRyPx4nH4wQCAe8HVFauffv2jcg+oq0lTiQ86rGtXr16Us5hJjAXzqHcTDTSvQT4kpTyUff3W4QQXwVOdrcdklLe5M67XQjxCeBS4LsT3O+cYevWrZx11ll5o3Lt7e1jsnlUVlYW9DAXEtNVVVVZy0802jXa+rphH+28brvtNnbs2EFzczMNDQ1F0xFq1q5da4SWwTAKbW1tIz7Ajf1mVnI9IKWUEzLjr1y5kkgkMkmHNPXs3r2bVatWTWgbj78c5OnfPsfLvXD8kpHzI5EINTU1BAIBQqGQ146l02mOPPJIotEoXV1d3vI6XSCVlaMe26pVqyblHKabuXAO8Xi87B+ho4puIUQYyFdSyZFSfj1n2fOAGuAR4L3AYznrPI4S5AYfhaJy+RrHQti2zSWXXFJQdBYSw52dnRx11FGe2C8UBSuVYqLasiyvYR/tvPSA0c7OTm/U+Ghs3bp1rIdrMMw7jP1mzvAeoNntcQaoBb4hhFgtpfz4NB7XrOP0E1u4/bfPsb83fw8tDPfeptNpz17S16c67XM93dpeYpeQ5vbw4cPedgxzn1LUzNnAvjw/WapKCLESuAP4rJRyP0p8D+ZsaxComuAxzxt0mrzRsnWAeuhvvfVWVq5cmTcLQbFt+DMYrFmzZtz5sHW0rFBObX9WkrGk/8tX7SuXsaYSNBjmM7k1A8YjuE3awelFSnm8lLJOStkgpWxADaT8uBHcY2fF0U0EbOgZhKFkgWwkbgYTy7K8wnYDAwP50/UmlL3Erhpd7nzkIx8xg5jnEaNGuqWU9wBFRwIIIS4EdgDtUsob3clRIFd5VQEFUtDnZy74zSbCsmXLRq1O6ae7u5trr72WZ599lvPPP9+bnkqlRl03Fovxk5/8hCuvvJIdO3Zw8ODBUdexbRvHcf4/e+8eJ0dV5v+/u3t6JjOTy0wICNNgBCVHSQQ0EndXwMTsqqir0IARQiJfvO7qJDBgnIFFEZSMUQIkaBBvmBghv0CLK7soSwzorJdkRxHC5UQEBCYgAXKZTOY+/fvjVPV091T1bap7qqef9+s1r6SrTlU91V2n6qnnfM7zMGvWLJYtW8bs2bMBXPfR1dXFpZdeysqVK9mwYQMbNmzgvvvuy/n8nKipqWHJkiWiJxOEEpE+R8R+aQckYi6UHeGqIJEjqnlu7wB/PwCzZzm3CwQCjIyMJJzu7u5ux+wlgT4T6Q5Nda46LVQuXmUv+Spwsdb67qRVjwOXpTV/M7Axn/2Xu97MC3JxfpPp7+9ny5YtXH755Yn81t3d3Tlt293dzfHHH8/DDz/MggULskpN4vE4L7zwQsqyWCzGli1beOWVV1JKyNsMDw/zgx/8gMsvv5xHHnkkr3NLJhAIVNTQeCn0ZoKQC5nSDlZCX/QjWutTJ9qGcmbOcTN4bu9e/n4wzuxZY+OMIyMjCanjtGmmoI7bc9WOdGerRilUHuPNXvJR4HrgPVrrP6St3g4ElFKXAbdgspecDPx0PMesREKhUN6VIffs2eOasSQb9oMzl0mV6RkP2traxmRjccJOtTSeiZvr1q2TB7wgTACSdlCYbJz65mN44I97M+q6beos2UhPT49LCXgT6a6eMd1bI4WyZ7zFcVqBGmCbUupQ0t+HtNYDwFkYZ/s14CrgbK11fmFboaBS7E1NTQWn/7MfnNlSiKVnPIjFYjk53Ol2Foro4ARhYpjMVT9Fq16ZnHyiSVuyt9sh77aVp3tkZIR4PM6UKVMIBoP09vYyNDQ0tv2AqaUxZfqM0hgvlA3jinRrrd+eZf0uwLk0oZAzjY2NOaXMs7GzhKxYsaKg49kPTqfsKbZcxCkXeHt7e84Od2NjY+IYheYjl6iaIEwMkzXtoGjVK5fG6VMSRXIO9EJD2hzIeDxOKBRK/FtfX093dzc9PT1jCuAErAJ2NdOnZT3usmXLeOaZZzw7D8HfSBl4nxOLxTh0KK+5p8TjcaLRaEFRp+S0fnb2lEgkQiAQIBKJsGzZMiKRCHv27KG9vT0lCpSrExwKhbj22msTxyiUyRBVE4RyxOne4FYjoJzIpFUXJj/HzpoCwN6DqcEjuwz8yMhIIuI91dJrHzhwYMx+bKe7PofMYx/5yEc444wzxmu6UCaI0+1z2tvbcyr7noxdLcspdV9tbW1i5rUT6QVmklOLtba2snXrVrq6uhJpBlesWEFbWxuQuxNcXV1Ne3t7YvjWjnrnw2SIqgnFpRQygVgsxty5c4lEIkQiEdeUnfnusxR2j/cYXqQdLDb5nqdo1SubE48zTvJeh/mRdqYu2/G2nW6ntIG2vCSXiZRdXV15J0sQyhdxun1OITd7WwPuFo06fDg9ffoomQrMOEWB4vF4Ij94V1fXmGE2J3p7e1Mc90OHDuVcAAeMNGUyRNWE4mHLBJKvs1WrVnnqwMZiMVpaWhKTgsGk7GxpaSn4OKWyu9jH8AOFnOdk1qoL2XnrHFvXPVYmaTvb9v+Tc3WPSRk4aLKXhOqy17xYuXIlN95447jsFsoHcbp9jtvN3o6sOZFcCMcpGjVjhvPkjmxFeDK9ANia83wmUdoMDg7mlRYyvXS9IKRTCpmA2yjU4OBgwccpld2VIKEo5DzdRgdlVK0yOOmEowB45RCMJD3LbHnJ8PBwokCOHel2Shtoy0tCBRaaEyYv4nT7nMWLF4+JHidXfnQqnHPw4EFOPPFE1yFvt2h0tih1MaM9+WRZkaHe/FBK/YtSqlMpdVAp9ZRS6jMu7S5RSg2mZSL6eKnt9YJSyAQy7avQ40yk3ZOtXxVynpNVqy7kxoypNUyrDTA0DAeSBoTtKLf9jBweHs5YldKWl4RqpQC3kIo43T4mFouxdevWlA4dCAQ4//zziUajRKPRxNt2MiMjIykSkvQh7+Th8GTcltu6yGyFckqFDPXmjlLqOOBuTAGrBuACYLVS6n0Ozd8O3KC1npr096MSmusZpZAJZNpXoceZSLvj8fikSpFX6HdZDlp1oXgce4QZdU2XmNjPYSdNd0rAamSEgFUBOjSlsgv7CWMRp9vHuGmot23blvjs5iinkzzk7fbQCQaDxGKxlMlHc+fOpaWlJW+HOxAIJCZ0eokM9ebNG4CfaK1/qrUe0VrvBB4E3uXQdj7wcAltKxqlkAm4jTSFw+GCj1Mqu9OPYTOZ9N0iFREK4U3HmYn9yZMpbXlJPB5PTKi0C+SMiXRbDnc8HCaQx1wloTKQK8LHuA2DdnV1ceyxxzJnzpy8NNT2/tweusPDw7S0tCSc7Hg8zv79+/POngLmJrV06VLXh3s6DQ0Njg5MOjLUmx9a699orT9rf1ZKzQTOAP6U3E4pFcJUjF2mlNpjyVBalVLZZ8b6kFLIBKLRKGvXrk2ZC9HY2MjatWsLPk6p7LaP4cRk0XeLVEQohLlvOhqA1w45T6a0M5jYpeDTq1LaJeDj1dmfZwCf/vSnOfvss8drtlAmjKs4jlBcmpqaXCPM8Xicnp6evPcHo7mxL7300jHVLgtxsN3YunUr559/Ptu2bcsYKQ8EAlx33XUAXH311a7R+8bGRnlgjgOl1AzgP4E/AD9LW30k8H/Aj4Ao8BarzUHg27keY9euXZ7Y6gWzZ89mw4YNKcs6Ozs9P8bGjRvHLB/PcYpl94MPPsimTZt45ZVXmDVrFu94xztc++WePXs8/65KRfp5Llu2jIULFybWl+t5CaVBveFIAF7rITFpckx2kkAgoelOn0gZGByNdOfCe9/7XrkmKwhxun2MU9W3Qkkf8o5GowVXrMyV3t5etm3bxo4dOzJqwu1iPrZddiq25BeAcDicKKgj5I9Sag7GiX4cWKq1TnmKaK1fAt6dtOhhpdR64FzycLrnzZuXVyYaoTTEYjE2bNiQuJfs3buX++67z7V9U1MT8+fPL5V5nuF0nhs2bOD4448v+Qt7f3+/r15Chdw4YsYUaqqgbxAOD0C9dTtLdsADgUBiFDf9+ZyIdOfodD/11FO88MILZdnfhPwReYmPyTYMnCuBQMBxyLsUExJtSUumjAHp52cP2ycPC49nyL7SUUqdiYlu3wOcp7Xuc2gzVyn1lbTF1cCYtkL54TQ/xI1y1j1XSjpEoXgEAgGOmmHika9axaBtPbe9HmDKFFO9sqenJyUSbqcLpLo6p+O1trby7W/nHNcQyhyJdPscO0vJ61//+jFSkFyJx+OJh06y4+oUSbd11clR5mAwOGZ4LVfsyZkNDQ2JXN7pOD3g7fMWxodS6o3AvcBVWuv1GZruBy5XSr0AfB94G7AC+HzxrRSKTT7pAMtZ91wp6RCF4nLsUfU8/+oBXjsU5/VHjE5rsfXcwWAwIS8ZE+m2n50y4ic4IJHuMmHp0qUZ19fW1mYsp97V1TWmUp7TRKO1a9eOiTLffPPNrF+/PhGRtrOS5FJ90p6ceeDAAcf1xchwIqTwOWAaJk1gcv7tryulrlRKPQagte4CPgx8BqPjvhu4Tmt914RZLnhGrqNakUikbB1ukIqSgjfMmX0EAK8mTZuKpxXLyRbpDorTLTggke4ywS7PvnnzZoaHhwkEAtTV1XH48GGampoS0eJMGvDBwUFWrVqV8lB1iyjnsmzevHmu0ev047oxPDxMe3t7WT/o/YzWugVoydDk+qS2vwLeUXSjhJKTy/yQcpaV2Did52Q4L6G0zD2xCX7xNPt7Uh1tm4yabtvplhzdggMS6S4jVq9ezXPPPUdXVxcvvPACu3fvTingYEeuM5Vz7+3tHZODNzkvdz7FMXLNEZ4NGfoVhOLiNKq1fPnySZdOT9IECl7w+tdNB2D/4bERbvvfqqoqQqEQQ0NDqYGlISMDDVmRcEFIRiLdkxA3KYdNcmQ5FoulRIbs4hjgHO22icViBIPBgnXmycjQr+AlsVgsJfVkY2Mj1157bcU7XpUyT6IY5xmLxWhvb2fPnj2JkcVK+C4rlfraMHXVJntJdx9Mr011uMHMV6qrq6O7u5vDhw8nNN6j1Shzc7pXrFjBX/7ylyKcheBHJNI9iYjFYqxcuTJrwZzkyLLbbP/m5mbXqLftqOfqcIfDYdfCNzL0K3iJnW4yeRRm3759Y+YzCEKu2Pc7u2DYZKraKbgza7qZb7TP0nWnO93JVSmTn6G2012VY2G4M888k1NPPdUTmwX/I073JKK9vT2nLCPJkeVM0g63h4tb+rFAIJCYzGlPkLQnZ15wwQVjJk3K0K/gNe3t7Y5zCAYHByVtnFAQkoawMmk6wjjU+w8bJ3t4eDjF4Y7H4866bqs4TrXlkGdj165dPP30016ZLfgccbonEbloo9Mjy9mkHU4Pl0zH2bVrF11dXQnt+Y4dOwBTnTI5Mm7bIQ634CWZrk2ZOyAUgqQhrEyOP9YEkPYdTl2enLPbjnQfPjzayI50V0+tz+k411xzDd/73vfGa65QJojTPYnI5kA7RZZbW1sTb+tupD9c8k3LJZEioVRk6gMzZszIe8JwoZOMvWAij12uFOM7c5uYLnNRJjdz3nAUQCKDie1s26ly3SLdCXnJlNzkJUJlIU73JKK1tZVg0PknXb58eSLLSTK5VL1Mf7g4OeqZtNkSKRJKRWtrq+P8gWAwSE9PT1663InU8oqOOH+K8Z3FYjEOHTo0Znk4HJa5KJOcNzTNBOCg5U+nSzcDgQA1Vi7uAav0O4w63TU5RrqFykKc7klENBrl5ptvTnGIg8Egy5cvT+T5dttux44drF+/PidnOt+0XFKwQigV0WiUtWvXpkQnGxsbmTFjxhitd7bRlokcoZHRofwpxnfmNkegvr5epHGTnJnTpxAKQu8gDAzFE9Uo7Yh3coGcvr6+0Q0TTvfUiTBb8DnidE8yotEoTz31FF1dXXR1dfH8889ndLhhdEh2xYoVAIloeSgU4vzzz3ctlLNjx46UPOFu5BsZFyY3yRKAuXPnMm/evILkAG5Sgmg0ymOPPZboA7t27XLNKd/V1eW6f7eRmK6urqJHnL0eHaoEqUqh31mm69Ht+siWllUof4LBANOtx9bBXhLSkmSJiR3p7u/vT2xnR7rDOU6kFCoLcbornPQh2d7e3sQw2vDwMFu3bh33A1oKVgg26dfb/v372bdvX95ygHylBG6jKoFAIO9tgKJLPbwcHaoUqUoh31m269F2rvLZpzB5OGKakaodsAZQkqPcbpHuwGB+ebq/+MUvsmzZMg+tFvyMON0Vjlv6PxuvhrTziYwLkxevrrd8pQStra2ODlQ8Hs+4jdsk42JLPbwcHaoUqUoh31m26zE5qpnrPoXJw9FW2sCDvaOTKe1odzwed4x02/KSUE11Tsc47bTTeMtb3uKh1YKfEae7wslluDrTELwg5EMu19t42rgtj0ajrkWjMm2zZs2avG3wAi9HhyplInMh31ku30E8HpdRugrl9UfPAEYj3ZCaxSTTRMpgjpHunTt38sQTT3hkseB3xOmucHIZJk0vaiMIhZLL9TaeNk1NTa76ZbcMPU77Sp7n4Hb9u6Ug9Eo/7dXokNcTmf2sD8/2naXb7pYOMJlIJCKjdBXKG19v0gYePJyaNtD+vy0vcdJ0B6trcjrG17/+dTZt2uSZzYK/Eae7wnFLsZZMruXeBSEb2fLC5zp07yYlWLx4sat+OVf5QbrO1+n6D4fDjikI29rafKef9lKqUs76cCfbDx06lPH+J1KSymZ2kymQk6zpthkZGUlEuhOa7ng84XTnKi8RKgtxuiucaDTK1CypjTLl8BaEfEiXADQ0NNDY2Jj30L2blGDbtm2u+uVc5QduOt9QKJTYburUqY4pCDdv3uw7/bSXUpVy1oc72T44OEh9ff24r0dhcjKroZZgAA4PwNDwqMNty0uqq41jnYh0Wy/o8WCQgIwQCw5UTbQBwsTjlk4NJNIjeE80GvXEkXHaj532Mp1k7W5PT08i0vnFL36RL33pS+zfv5+mpiZaW1tddb7Dw8OJ+Q1uL6Juo0ITrZ9O/q5isRjt7e2sWLEicc65/h5e6sNtO/bs2ZO3HYXgZuOBAwd47LHHinZcoXwJBQPU10B3Hxzqh9opqZMp0+UlAbv/i8MtuCBOt0BTU5PjZMlQKCSRHqGscLuWba13S0tLSoT68OHDHD58GCAhlWhoaGDfvn1j9pGcXtB+6KYTCoUcHW+/pJizJRZ2xNc+ZyCnfp7p+y2lHYXgle1+Qil1DLABWAT0Abdpra+eWKsmF9OmBOjui3OoD2ZOHSYQCCQy2ozRdNuR7ipxugVnRF4iuGo+b7rpJnG4hbIik37ZrbpgMr29vY5p4mA0vWB7e7ujwx0IBFi6dKmvC0GNVx7ilT58ImQqk7RI18+AF4HXAf8AfFwpdeHEmjS5aLRydXf3xcf0e3s+wGik29S4iOcR6b7mmmva997wAAAgAElEQVT45Cc/6YWpQhkgTrcgxWuESUOmazlXCcSBAwcyphd02088Hmf16tW+7kvjlYd4da+YiDSGk+0+p5R6J3ACsEJr3ae1fgZYCGyfUMMmGUc1mlzdhxJzJUfLwbtFugnm7nTPmzePE044wTN7BX8jTrcgCL4gWyo6p/VOy9zSxuWSHg6M3CBTesFM1S3nzZuX0JWvW7eOHTt2ADB37lwikUjib968eXln/Mg3VV8sFhtzXLeXiXwkFl6kMvQ6jSGMfj+RSITXv/71RCKRMd9Tobb7NE3ifOBR4BqlVJdS6q/AOVrrFyfYrknFsUeb+0Z3X2rKQBhbBj5QgLzk17/+NQ8//LBX5go+RzTdwoToKwUhmWzXoNP6lpYWgIRkJNN1G4vFOHToUE62LF68mNNOOy3leJAqRUhfB+aBbGvBbVt27tzJHXfcMUbWsm/fvoT9ufSxfPuok37djYmQWLS2tmb8fvMl/fuxdfVe3Mt8fH+cCZwBPISJeL8Z+IVS6kWt9U8m0rDJxOymI4BnOdQ36mzbczrs7CUDAwOMjIwQKmAi5bp16+ju7uYTn/iEl2YLPiXgFvmYaDo7O98APDNv3rzE26RQHBYsWOA4wcguCiH4g/7+fnbt2gVw/Pz585+dYHNSGG9/zXYNuq13wum6LWT7TNk1YrEYl156adYc9m4TKzPZ6kS+fTTX841EIkXPGuKGl9lLsp3veO5lhd4fi91flVJfANq01jOTll0HvEVrfV627e0+67Vdk429Bwb51n/9nem1cME/jHWmP/e5zzE0NMT69eupe/U1pt17H4NHv45pn87Nib7yyisBuP766z21WxgXRXvGSqRbqJgy0YI/icVirg5TV1dXXg5z8ja2E5dp/27bw2iaPds5bG5uTjjaDQ0NORWNytbGtnXx4sVs27Yt4YCmf3azP72P2rbmcr6BQCCrI+qFY5xsk/0SEolEWLx4caJNT08PLS0tNDc3p9hnl2DPdtxs96rk9W7n1NbWxqZNmxLygerqaurr6x0z2eRyzN27d/Pzn/+ce+6555be3t6pwDDwErATuFdr/VTGHWTnSaBOKVWttbbrkOf9TC/3wFZnZyfz588v2v4P9w3yrf/6bw73m9GsUChEMBgkGAwmot1DQ0NmVCkp0p2rTdOmTaO7u7uo51AKiv07lIKkF+WiIU63MClTaQnlgS2DcCMQCOTlMNskyzu2bt2a17Z2akAnWYvtRGfKbZ9MMBhkZGQkq60bN27M+NktRWFyH023NRvZ+rcXsopMso/kc3T6Pu3zzeW4mV5M7PWZzmnLli10dHSkbDMwMMDAwMCYfaXvM52dO3eybt06/vSnP3HCCScQCoUOAI8AIWAWcBGwRin1EHCd1vrXrgfJzP8Ae4EblFKXAwr4BPBvBe5PcKBuSphwCAaHYWAIakMkHO54PJ7IYDI4ODiq6ZY83YILMpFSmKyptAQLpdQpSqnfKaV6lFKPKqVOG087L8mWxm888je3CpHZsFMD2vblu30yNTU1GcuM52NTehrD9D6aj63hcDhr//Yird94v79cj+t0D7NJ/p7czind4c6G2/2xra2NNWvWcO6557J9+3a+/OUvc9ttt12ltb5Sa/1FrfUntNbvwDjfdwE3KaVuz+vgFlrrPuDdGD33i8AvgDVa67sL2Z/gTp1V0b1nIDV7STAYTHG6GZLiOEJmJNItJKJHpawOJ5QGpVQ1JpfvTcCZwLnA/Uqp2Vrrg/m285piS5hykYA4Yds1Xvv6+vpYt24dV199dc7RcTdsqYVbH83H1rVr12bt317Izrz8fTPtK/keli5jSf6evLAnk9xl4cKFrF69GkhKI+eA1ae+A3xHKZVVf51hP08DHyx0eyE3pk4JcKA3zuF+mFlvAgH26FNKpHsk/0h3e3u7VEStIDxzuq2coR3AiVrrZ61lpwC3AicDTwOXaK13enVMwTui0Sg7d+5k8+bNdHV1cemll7Jz587EA0QoWxYCYa31TdbnO5VSnweWAN8toF1B5Ct9mGhsB9eL/STrlMeLLaHo6uqiubm54H2PZ1uvvptiHjdZxjKec02ntrY2ow7+fe97X9771FrfNR6bhOIzvT5M174BevrjQKrUKyXSbV13gTxSBr7pTW/iwIEDntor+BdPnG6l1FRgY/L+JipyJhRGW1tbisZyeHg48Vkc77LmJOCJtGVPAm8tsF3exGIxVq5cmVXbLAh+p7e3l7a2tpzuiUNDQzz00EPceeedbQcOHBgT9tZaryiKkYLnNE6tBgbosX7FZLlXSqTbkpcE8pCU3X///fz1r38t+0mIQm54peleD6RXC1iIFTnTWg9qre8EHsNEzgSfsXnz5ryWC2XDVOBw2rLDQF2B7fKmvb1dHG5h0pDrPfGqq67i9ttvp7e392TgxLS/NxXPQsFrjjpiKgCH0+bVpstL7Eh3MA+n+7bbbuOee+7xxlDB92SNdFsR65kOq+Ja679berQ3ApcDyTNLihY5E7zHTftaqCZW8A09QPoMszogvVJMru0y4pRuSVJPCpOJ4eFhOjs7s7Z78MEHWblyJaeeeuoFfsurL+RH5KgG4CVLXoKrvCQwbIILQatojiCkk4u85J+A7Q7Lh5VSs4FvAIuA9FBW0SJngve4FfEIySzscudx4LK0ZW/GyMEKaZcRp5y/2dK5CUI5EcoxB/OsWbNobGwsgUVCsTmu6QgADieJhEZGRggEAqmRbssLEqdbcCOr0621fhAIpC9XSgWAB4Ava62fVUo1pDUpWuRM8J73vve93HfffY7Lc4nqCL5lOxBQSl0G3IKZW3Ey8NMC2+VNa2uraLqFScPSpUtzatfS0sKGDRuorq7+B+s5ltIBtNbPFcE8oQjMajCxwh5LXmJruuPxOFVVxo0aHBwkYCl2Q+J0Cy6MZyLlccC7gPlKqXWMOuaPKKU+SxEjZ4L3fO9736OtrY3NmzczPDxMKBRi6dKlMonSRxRSLUtrPaCUOguTReha4FngbK31XqXUlcBSrfXcTO3Ga7edWm0ispdkGsHxUjrV0NBAIBBwrV4olD/BYJCLLroo53vi8PAwzz//PL29veki8AAQxxTLEcqAGfXGie4bHJszv9pysIeGhiBoXKpQ9fhz8wuTk4KdbustfYr92Yp07wNOtiLf1RQpciYUh9WrV4uTPQnRWu8CTndYfj1wfbZ2XmCXVBeESuEb3/gG73znO+np6Tln586df51oe4TCmVJTRVUQhkZM/ZtwFYniOHake2BgAKrNe1SwKnen++abb+bRRx8tit2C/yhacZxiRs4EQRAEwc8cPHiQc845hyOPPPIRmUhZ/kyphkN9JtodrnIpjlNlRuWr8xidj0QivPTSS0WxWfAfnjndWuv9pGm/ixk5EwRBEAS/8v73v5/f/e53fPjDH55oUwQPqK0OcqhvhL5BmFY7KjOxne6hoSGw5q3kkzLwZz/7Gc8884zk6a4QpAy8IAiCIHhMbW0tW7du5a677vrF0NDQE8Bg8nqt9UcnyDShAOqnhNh7cIRe61e00wamZi8xTndVHhMpN23aRHd3N5deeqm3Bgu+xM9OdwgsnZQgCMl9wY8TsKS/CkIS3d3d/OM//iN//OMfdx06dKh7ou0Rxse02ipgkL5BUwo+vSLlwMAAAcvplomUght+drqPAdi9e/dE2yEIfuMYwG8Ts6S/CkISF1xwgf3fm+bPn98xkbYI42f61Bqgl76kSHcgEEhMpEyWl4TykJcIlYWfne6dwBnAi4CURRQEE00+BtM3/Ib0V6Hi+dSnPvWN97///Tece+65L5FDf1VKvQG4Vmu9vEQmCgXSMM0ka+tNG8yzUwYmy0vCU6YgCE741umeP39+PyDRAUFIxW8RbkD6qyAA9PT0bL777rs333333Z1ADPil1ro/uY1S6ijg3cBFwNsAEfOWAUc01AMkIt3A2OwlttMttUUEF3zrdAuCIAhCOaG1vkcp9QDwGUwO/M1KqZeAVzDZvY4EjgKewaTTvVBr3TNR9gq587ojZgBYmu5RkitSUoDTfdttt/HnP//ZIysFvyNOtyAIgiB4hNb6EHADcINSai4wH3gdpgz8S0Cn1vrJCTRRKIDG6bVAaqQb0rOXGIc8GM7dtZo5cybTp0/3xkjB94jTLQiCIAhFQGv9GPDYRNshjJ/pSaXgkwmFTDKp4eFhAnET6Q6EcnettmzZwt/+9jfJ010hBCfaAEEQBEEQBD8zfWp2p9uWlwRCuWd13bp1K9u2bfPGSMH3iNMtCIIgCIKQgWl1xunuH4SR+Kiu28npDlaJiEBwRpxuQRAEQRCEDFSFgtSEIY5xvBPLk/J0BwqIdAuVhTjdgiAIgiAIWairMS5TssTEjnSPjIyMykuqxOkWnJExEEEQBEHwGKVUFfD/gFOAWkzKwARa60smwi6hcOqnVLHv0ICj052q6RbXSnBGrgxBEARB8J5bME73r4H9E2yL4AHTaqsAd6c7UECke9OmTfzpT3/y0kzBx4jTLQiCIAje8zHgXK31vRNtiOANJm3gYatAjhm4GG+ku7a2lhqpYFkxVJTTbQ33XYGJPrwBOABsA67WWj81gaYBoJRKLnUVBw4DjwKrtdb/6eFxpgJLtNbftz7fDkzVWp+X534uAxq01l+29vFxl6b/q7U+PWm7E4D/AN4HNGJKm38H+LbWeiTD8ULA54BPAHOAg5jS49drrTvzsDvl/MeLUioMfEpr/W3r83nAB7XW/8+L/VcC0jdzsuF2rH6qlAoAnwQ2aa37CtzfccB/AguAdwHbMzQ/0f4drN/qUkx/fyPwKvBL4Mta664sx/xH4CrreGHgSeA24Lta63imbdP2cw7wf1rr53PdJsv+3g0c0Fo/rJSqB34PLNJavzKO3Q4BT3hhn+APptcb59gp0j00NDRaHCePSPftt9/O888/L3m6K4RKm0h5PfApoAVQwIeAacCvlVINE2lYEsuAY4BjgXcCvwBiSqnzPTzG5cC/jWcH1gN7JfCNpMX3YmxP//tw0nbzgU7MC18UmIep3vZVYF2G4wWBGMYxuwE4CXg/sAf4X+shnCvjPv80LgSutT9ore8C5imlFnp4jMmO9M3srMQ42gBnYpzV8QRObgHWaK2TMw+/Eec+/AwkHO77MS++12D674WY3+w3Sqmj3A6mlFoCPIhxtBcCpwLfBr4C3Gm9SGRFKTUbcy+YkdNZ5saDmN8Vqyz7d0i9txXC94FV1r1LmATMbKgDoHdgdJmjvCSP7CX33nsvHR0d3hkp+JqKinRjHhSXaq3/y/r8rFLqo8DLwNnA7RNlWBL7tdYvWf/fAzxmRV5uVkr9p9a634Nj5PRwy8Iq4C6r5LFNf5LtY7AeqhuBe9KiwE8rpXoxD94NVhW3dD4LnA6corV+IWl5s1JqL/B9pdRvcoxMeXH+2fa3HuOULPT4WJMV6ZtZ0FofSPo4rmtYKfV24B8wL77JvJzWp9NpwTjaJyX1taeVUh8AnsK8FK9yON5RGCf0MntEyOIppVQH8GfMKMcPcjDf6/7rxPeB65VSX9Va/zXXjZRSOzEjIWCer6cC5yql/gYMJ7fVWi/wylihNMycbpzurBMpJU+34EKlXRkjwHuUUndorYcAtNaHlVKnAnsBlFLXACcDTwOXAP3AVszDYthqcyHQCpwIPIeRN/xIKXU2sAlo1FoPKaWOBP4OXKW1Xm1teytQp7Venofd3wK+gBmS/ZUlZ7gOuBgzK/73wAqttbaO8SBGdvEO4N3AX4DLtdb/o5S6GPiy1S4OHG8do04p9X3gfOucv6O1/g8nYyx5xsUYeUg+/AMmQn2uw7qtmN/A7QH3WeD2NIfb5uuY6PXHgFus8/8/rfUVSTbHgX8FZjH2/K/BnPNU4ByMQ3W11voOq93tpMlvlFLPAt8EdgE/TNrfIq31g8DPge8ppU7RWv/Z9RsRbCqpbz6EyWjxXuB54Bta6+9Z68/AjOS8FdgH/Bho01oP29chxrG1pSDdSqnPAquBLyRLppRSvwJ+o7X+soPdK4Cf2d9bHnwC+EH6y63WuttyvF902e4iwI4gp6C13q2U2gj8O/AD6x71Ta31rKRzuZ3RPviMtfhRpdRXMFHqezCSl9WY7/3/A1ZqrXutEaftwDT7hcK6lj6ktX6H1ZcBfq6U+pHW+mJru/uBzwOX5fjdgBntS+ZneWwr+JxZjfUAlqbbMN5It1BZVNqw1w2Yh+HzSqnvK6WWKqVmaa2fSosifQhoAP4R+BJGR3w2gFJqKcbJ2oBxANYD31VKfRB4AKjBaCQBFln/npG07/cC/0UeaK3/hnlgzbUWXQt8EFiCGebWwENKqeTh1i8AvwPeBvw3cK9S6kRgi/U9/BkzbGxrIs/CPDBPBdqAq5RSbk71uzFRm9/lcx7Wvru11k86nOOI1vpXTvpUpVQdJrr2e6edWhHG32F+r2y4nf/FGI342zHD7j/OUR7yW8zD/jVrf7+1bNoH7MB8r0J2KqlvfhH4H0zffADYoJQ62pqzcA/GcXsLsBwjubk47bDPM/ri+kbM6NFdwAV2A6VUE6af/sTF9LMw8picUUrVYuZS7HRar7Xu1Frvcdl8AeZF2M3J3w68XSlVnYMp9m+4EPPiC+Zl5AuY7+XDwD9jpCu5cJr17zKMhMfmF+TZf7XWX7H/MOd0ffIya3k78Eg++xX8wfSp7pru4WFzaccDAQLBSnOthFypqCtDa90OnAc8hrnB/hh4USl1k/XAs+kF/l0bvotx0OxZDpdhJv1s0Fr/RWt9C2Yo8koritIBvMdquwjzAH2XUipoOb2vx2gi82U/MN168F0GfFZr/ZDW+kmt9QrMxLNlSe07rJv8k1rrVsyEnk9qrXuBQ8CQ1vqlpIfgo1rr/9BaP21F3f6KicY58Q7gCYeJT2crpQ45/C211jdiHNt8acAMKb+Woc2rmCh2RjKc/9+Az1nf102YSHVW3bfWegDz3cet/SWp/Xgc810JWaiwvvmg1vpbVvS7DTPieDJGozwTeFFr/azW+gHMi8ADad/VMKN94WXrmv4xsFAp9Tpr+ceAP9oR9mQsTfRRmO86nZcc+u991rpG698DDttlYybZ+28AOCKHfe21t0mSwoQw3/tvtda/xshgliqlpmfbmdba3t/+tBe8xwFljewVwnbMvSudE3B/GRJ8zIwMEymHh4eJx+MgDreQgUqTl6C1vhu427qRLsREk1YCL2EiEAB/S3OeDmJm2oOJaN2YttsORqNM92GiI1/FPNg/j3nQn4zRJP/WioLmy3TMw+6NmIjd/So1o8IUzGQmm9+kbb8DEy12I13WsR8zTOvE6wAn7fT/AM0Oy/9u/fsKzg+hbNgP60wP0AYXm3Lltzo1c8oOYKlb4xx5FXjzOPdRMVRQ39xt/0drfVApBRDWWr+mlFoN3GbJH/4buENr7RhZTuM3mAj4+ZiRmgsxjrgTtmPu1F/+CZOZJZle699XrX8byZ/XyN5/40nHyJf0kbcdmOtCOTfPCduWozAv6llRSv0bZmIomJeIx9OuBTBReUnMXIaYlIHQm+R0B4NBgsEgIyMjDMfjhIL5TTm466676OzMOfmWUOZUjNOtlDoZ+LTW+vMAVoTkXozs4k5MJgz7wT7gsAu7J/W6rLNfb+8DrlVKHY9JfdaBkRyciRny/O8CbH8jJpPDnxn9zf4FM8ksmeQo8lDauiBpE3nScFrndvcYcVnXozOnd/sDUK+UOklr/XjyCmuG/38BN2utU4a9tdZ9SqmHMY7RT9N3ag1Jv5PRh108bX0u13mm78splVku+wyR+TsXqMi+6XoOWusrLf3yR4APAP+jlLpaa319Jju01nGl1E+AJUqpX2KkXB9yaW6/XDr14ae1y0RKrXW/1Q9Pw2jpU1BKrQJep7W+3GHz3wFtSqkqbWn20zgD+JPWesDBSYXs/W2E0fOC0d98mPH1X3sfufJdjNwoiJkUeh2pIwNxjAO/LY99Cj6hbkoVwQAMDcPQcJyq0GiubtvpDkqkW8hAJV0dIeBzyuRjTecgo0OW2XiCsdrhf8KkwUJrvcva1yrgD5ZG+SHMQ30ReWpGLT4FvIBxEJ7COIhHWXrXpzBR6msY1TqC0SYncxrGMQDnh1A+vAQcme9G2kwo/BMO2Q0w0gI7BaAT64FPKpPjO50WzO97h/V5gNR0YunbOJ1/+ve1gNHvK2V/ViQ2OTWa2/c5C/NdCZmptL7piFLq9UqpDZho/je01oswk4QvcmjudM1twpz/xcCvtHsmIXt53n0Yk0XmEqVUipRLmbSOK3F/Ud8MVJOqmba3fRMmc8kGa9EAMDVNVpTch53OPUzqSN4CoA+jqbdfcjLdE5ywzzHnPqy1HtJab9Ra3465pr6ttf5R0t9GrXUsTcYilAmBQIC6GuM2OUpMCpCX3Hrrrfz0p2NiScIkpWIi3VrrPymlYsBWpdSVWLPZMQ/cpda/udAO3KWUegyjtVyMmdF/SVKbX1ifv259fhAT8XhBa/1olv03KKWOxjy8Zlq2tQAXWFrOQ0qpb2PSlPVjMpNchplMlpyl4CNKqc9jNKqfxAx9f9dadwg42nJgn8vxvJPpBFqVUqG0iVE1lu1jSHIA/g3YZkW212GiQO/F5Gm+SWvtNsHoh1a7X1u/368xw9Ufx0ymu1CPZlTYCay0MiIcANZiMl3YOJ3/25RSX8VMSvsQRobwrqT9fcLKgPEEJqI+nLa/qUqpkzCRQnsy6CkYZ0PIQAX2TTf2Yl4+UUrdgLm+/wXniYt2NHq+UqpTa31Ia/2kFYluwWT7caML40iewtjiLUe5aJgPWNrxb2Mmif7G+q0eBt4EfA0z0vA1pwNqrV9RSl0C3GFN8vwR5oXqTEzGkV9g9PdY51sDfEWZjEofxUw6tV/I7XM/VSmVXBznu5a8YzpmYu73tNY91vXQC1ynlLoO4wx/EPP7kLTPeUqp32qtbTnbKZi5LgWlgtRaP6SUOtOyaR7mnvFnYJ3Oo5hXJpRS/4C5jyrM9dNuzckRikR9bYhDfSP0DcLUKWZZokBOAU73Aw88QHd3t9dmCj6lkiLdYHSO6zDZJh7F6CDfD7xfa51TJg6t9c8xqa1aMOnimjHVCJOdq/swUZ2HrM87MTf9+8jOJkwWkS7gV5iJeB+w9K42qzDZCn6ImQX/duAsnZpP9g7Mw/7PmCwG/6K1th3Mu4BuzESht+VgUzrbMY5H+iTBD1m2O/0BoLX+A0YmUo+REPwZ+DQmo4PTsLS9XRyjzb0Ok+5sF8axmg28S2sdS2p+A+Zl45fWMX6MiUbaOJ3//Zg0c3/GOGXnaK13WOs2YSJ8GzHXzMPA/ybtbxsmgv8nzMMcK1vFyYxNISY4U0l9083+XkwfeivmGnvAOo8VDs0fxVxb92P6j409QS82ZovR48Qx5+s0svBXnPvvEmvbQczL792YF5fHMM7yw8AZWmtXTbbW+h6MjOR4zPwP+9yuBT5qT8y2Rgguw/TDRzFpRm9K2s+r1jG/x6ikDOBOTJ+/y7KvxWp/0NrXQkyfPzttOzBZUL7EqOMP5oWg4P6rlPoY5jqZgvldtmK0679VSo07q5EVuPgZxomfgbk/3qKUcpsAL3jAtFozhaTXqSplPE48UGlulZAPgXh8vEoDwW8ohzzVRTjGt4BhKztDWaMc8nB7sM/PYByJxV7tUxCyoZT6BnCs1vqCLO1Ow2jYI2kTU8sO5ZCH24N9zsC8qJ+stX4mW3uXfewGvqW1vjlteQtwsdb65HHaeARmMuxyTGBhPmbk5jStddby852dnW8Anpk3bx41NTXjMWVC6ezsLGkJ9S/f+hB//Mt+3nNSgBNfZxzs1tZW9u3bx7fe+jZmHnkkZ276Yc77O++88+ju7uaXv/xlsUwuCaX+HYpBf38/u3btAjh+/vz5zxbjGPJKJhTKGkylNS9LMU8KlKm8+VlMlgxBKDpKqQVKqU8AnyGH/NRWRpQdWBFsYQyXALFCHW6LCM4jKD/HSHLGhRXxvwUj1RnEjNpcmYvDLRROwzSjKelzKgUvKQOFLFSMplvwFq3135RSN2IKUjhWrqxgzsNoQbdnbSkI3vAe4GpgvdY6PV2oG58DfqaUutOSjQiAUqoeM0F24Th39VOMfObzacuX4UGlSkte0oeRZt2NmTQcU0o9qbXOOd+8Fdkra0qZcq+vZ7/512UiZTwYyMue/v5+qqurJ0XawMlwDsVG5CWCIAiC4DFKqVsxmWSexWS3GcJo/N+G0eInZs9prT9awP7PA1q01v+UtGwN8Eat9bnuWxpEXlIYP92u+cG9T/KWpgBnKhPVvuaaa3jxxRe5Ye7JHHPccbz7+7fltc/JIM2YDOdQCnmJRLoFQRAEwXtqGE1jGsCkNXzU+vOC46xjJDOEkZoIRWLmdFMzrm9wNGAZCJhMmSPxOATyK44jVBa+dbo7OztrMLmlX0QKjAgCmHzWxwA758+fX1Aas2Ih/VUQUvnJT37yVYrbX+8HViulPo1JB/t2jCzmk0U4lmDRYDvdSZpuuyDOCOTtdN94443s2bOn7KPEQm741unGPMBz1SYKQiVxBqaaop+Q/ioIaezdu5c1a9b8pKurqx5To+B9wJNa69+Pd99a68eUUlFMGtVvYHKvt2qtx60XF9yxS8Ena7rtSHe8gJSB//u//yt5uisIPzvdLwLMmTOH6upq10a7du1i3rx5ruuF4iO/QWkYGBhg9+7dkJT33EdIfy0T5DcoDX/84x9ZtWoVgUDgOOCdQC1wKnCbUupcK6/8uNBa/zcm9aNQImZMNYqeZKc7EemOA0GRlwju+NnpHgaorq7OOsmjnCeBTBbkNygpfpRvSH8tI+Q3KD7r1q3jnHPO4cMf/vDyCy+88FEArfWlSqlXMNHpcTvdQumZVmdFuodMZDsQCCTJS4A3wlQAACAASURBVETTLWRGEkoKgiAIgsc8+eSTvPOd73RatRmYU2JzBI8IVwWpCQeIx6F/yCwbjXSL0y1kRpxuQRAEQfCYhoYGXnrpJadVpwF/L7E5gofUTzF5uW2JSSJ7CeRdHKexsZFp06Z5aJ3gZ/wsLxEEQRCEsuRjH/sY3//+96mvr/9XTMrAU5VSHwK+BHxzYq0TxsPU2ipe6x4yGUzqUiPd8Twj3d/97nelqEwFIU63IAiCIHjMxRdfzIEDB/jhD3/4RaAOuAuTYeSrwM0TapwwLqbVhYE+eh0j3SIvEdwReYngObFYjAULFnDssceyYMECYrHYRJskCMIkIRaL8aY3vYlIJOL6N2fOHF/cdxYvXsyPf/zj04FpQIPWuklrfZPWWkpBlzHT681E5P4h8zOmarrzc6tWr17Nj370I28NFHyLRLoFT4nFYrS0tDA4aEIAXV1dNDc3s3PnTlavXj3B1gmCUM7EYjGam5uztuvp6eHSSy8FIBqNFtusMbz22ms88MAD/P73v+ehhx76GtAFPKyU+i+t9YGSGyR4iol0Q78V6R7PRMrOzk7J011BSKRb8JSrr7464XAns3HjRl9EngRBKF9aW1tzbjs8PExzczMnnHBCSe89P/zhD3nPe97D9ddfzyOPPMLAwMCJmKI4PwBeUEqtKJkxQlGYloh0m8/JFSnjIi8RMiBOt+Ap+/fvd123atWqjNuKLEUQBDdisRg9PT15b9ff38/KlStLcj+5++67ufnmm1m1ahUPPfQQX//617n99ts/qrU+BWgAvgB8TSl1btGNEYrG9PopgKQMFPJHnG7BM7I91Hp7e13b2LKUrq4u4vF4QpbS1tZWDFMFQSgz8olypzMyMkJ7e7uH1jizadMmrrjiCi688EKmTJmSsk5r3ae1vhX4CrCy6MYIRWO6VZVyIG0iZRzy1nQLlYVcHYIn2E5zNtzaZJKlLFmyZNz2CYJQvhQa5U5mz549HlnjzrPPPsvpp5+erdl/Am8pujFC0cg4kTJPeckxxxzDEUcc4a2Bgm8Rp1vwBDenOZ3BwUFHJzqTLKWjo4NFixaNyz5BEMoXL6LUTU1NHliSmb6+PqZOnZqt2QFgZtGNEYrGtLpUTfdoysD85SXr16/n8ssv99Q+wb+I0y14QianOZ2Ojo68ZSO7d+9m9uzZovMWhAokU5S6traW9evXJ6KNTgSDwXHJU/IhIJreSU99rUn8NjZ7CcRFXiJkQFIGChPCxo0b2bJlC9/85jeJRqM0Njayb9++jNsMDQ3R3NzMli1b2LJlS4ksFQRhomloaHC9P6xZsyaRFnDVqlX09vamrK+pqUncZ0pBLBajrq6OoaEhXnzxRe67776LXnrppdeSmkjN7zJnal014DKRMk95yZe+9CVefvll5s+f76mNgj8Rp1vwhFyc5nT6+/tpbm7miiuuYMmSJWzcuDGn7To6Opg9ezY33njjhOTgFQShtMTjzrVkamtrE/eAaDQ64feDpqYm7rjjDsDYPDg4yGuvvfYZYCit6XMlN07wjKm1Jk/3wJD5nUdTBuYvL3n88cclT3cFIU634AnXXnttTkUrnOjv72fTpk2cfvrpdHR05LTN0NAQK1aYdLcT/aD1O0qpU4BbgZOBp4FLtNY7M7RvAB4BvqS1vr0kRgpCBtzka319fSW2JDO/+tWvEv/v7+9n165dAGfMnz//2YmySfCe6nCIqlCAoeE4QyNJ2UviSMpAISMiPhJ8QTwep6Ojg4aGhry2ySVjSiWjlKoGfgZsweQJ/hpwv1JqeobNbgUiJTBPELISi8VcddKlmBwpCE7UVhv3qX+QlEh3PMPcAkGQq0PwBK9y4NoRrfQct24MDg7KBMvMLATCWuubtNaDWus7gccAxzyMSqmPA9OBR0tnoiC4097e7igvCQQCJZscKQjp1NWEAKPrluI4Qq6I0y14QqbsAsuXL897f319fRx99NE5tbUnWJa63HOZcBLwRNqyJ4G3pjdUSh0PfBm4pAR2CUJOuN1b4vG4SMuECaNuipXBZCg5ZSB5O90nnHCCjNhUEKLpFjyhqamJrq6uMcsjkQirV6/mtNNOo6WlJadc3jZ79+5l/fr1rFy5kpGRkazt7YmZtrY8GAxy0UUXsXr16txPZPIxFTictuwwUJe8QCkVAn4MXKG1fkkpVdDBLA1rRjo7Owvat+Ad5fQbzJo1i717945ZfuSRR5bVeQiTCztt4MBgevaS/GKZa9askeu4ghCnW/CE1tbWMem6amtrE8O/dmYBu3JlLs738PBwYrslS5bkPMnSZmRkhI0bNyayogQCAZYtW1ZpTngPUJu2rA44lLbsakBrrcc1VDBv3jxqampc13d2dkpqrAmm3H6Ds846yzGz0VlnneXr80iaSClMQqbW2mkD4yl5uvNNGShUFiIvETwj2dlqbGxMyZ9rE41Gcy2VTCgUSvx/y5YtOW2TiXg8Xoll5R8H0sPWb7aWJ/Mx4Dyl1H6l1H6M/OTbSqlvl8BGQXBl27ZteS0XhFIwrX40V3dyRcpAMJRpszGsWrWKW265xXP7BH8iTrcwbh588EFWrVqVktYrWyqvLVu2ZIyIAixdunTMNnPmzCncUIuOjo5Kcry3AwGl1GVKqbBS6mOY1IE/TW6ktX6z1nq61rpBa92AmUj571rrf58AmwUhgZumO9M8EkEoNtPsAjlp8pJAKD+n++mnn5ZruYIQp1sYN5s2bRpTBa63tzdrRpNvfvObruvC4bCjDGT79u3jjniDcbwXLVo07v34Ha31AHAWcC7wGnAVcLbWeq9S6kql1GMTaqAgZMFtkplMPhMmkulTTYatlOwlQKgqP6dbqCzE6RbGzSuvvOK4PNvbezQadcxsEg6HWbt2ret2W7ZsYf369YkbXaHs3r27IiLeWutdWuvTtdbTtNZv1Vr/ylp+vdZ6rss2p0phHMEPLF68eEye7uT5IoIwEUyrc5CXFBDpFioLcbqFcTNr1izH5blEolavXs369euJRCIEAgEikQhr167NmgosGo3y/PPPjzvq3dHRIWkGBcGnxGIxtm7dmpKnOxAIcP7550u6QGFCmT7VyCMHBuPjkpcIlYU43cK4WbZsGbW1qQky8olERaNRduzYwQsvvMCOHTvyepjaUe/04+eDV4V9BEHwlvb29jHStXg8LpMohQnHMdINBPN0uk866SSOP/54r80TfIqkDBTGzcKFCzn++ONpb29nz549NDU10draWrJIlJ1W0IlYLJbI2+2GTGIRBH8ikygFv1I3JQzA4DCEk6SOwVB+btW1114reborCHG6BU/I5PhOJLZNmQrsyIQsQfAnbkW3pM8KE01tjXGfBodHl8XjcYIhERAI7sjVIUx6Mum/ZUKWIPiX1tbWcUnXBKFYODndkL+8pLm5mRtuuMErswSfI063UDHY+u/kSZtOBXwEQfAH0WiUNWvWSJ8VfIftdA8kabrjkHdxnBdffJFXX33Va/MEnyLyEqGi8KsMRhAEZ6TPCn4kXBUkEDCl35OS64w7la0wuZGrQxg3Dz74IHPnziUSiRCJRJg3b55v0/DFYjEWLFjAsccey4IFC3xrpyAIBumzgh8JBALUVNn5uUcj3cEqiWUK7sjVIYyLJUuW0NHRkbJs3759tLS0APgqQhWLxVi1alUiBVlXVxerVq0C/GWnIAgG6bOjKKUWAPdqrY+yPlcDtwDnAcPAWq312DK+QtGoCQfpGxxmxIp0x5GJlEJm5OoQCqatrW2Mw20zODjou/zXTjl/cylXLwjCxCB9FpRSAaXUJ4H7geqkVV8BFPBG4DTg40qpsSV+haJRU20XxbEqpsYhkKe8ZP78+SilvDZN8CkFRbqVUqcAtwInA08Dl2itdzq0+xegHTgReBn4htb6O4WbK/iJzZs3Z1zvt1y6kvNXEMoL6bOAca4/CHwV+I+k5R8HLtZa7wP2KaW+CXwG2Fh6EyuTKdUhYJDhRKQ7f6e7ra1N8nRXEHlHuq0hrZ8BW4AG4GvA/Uqp6WntjgPuxtwoGoALgNVKqfeN12jBHwwPD2dc77dcum72+M1OQRAM0mcBuFVrPR/4P3uBUqoBOAZ4PKndk8BbS2xbRTOl2sQtE5FuZCKlkJlCro6FQFhrfZPWelBrfSfwGLAkrd0bgJ9orX+qtR6xIuEPAu8ah72CjwhlyUfqt1y6kvNXEMoL6bOgtXYK60+1/j2ctOwwUFd8iwQbO23gsFV3rZCJlJ/61KdYvVqk+JVCIfKSk4An0paNecPWWv8G+I39WSk1EzgD2FTAMQUfsnTpUjZudB7JbGho8N1EJ9ueiSpXLwhCfkifdaXH+jf5jaQOOJTvjnbt2uWJQRPJRMkzBvvNz5Bc7Pi555+nJw97nnvuOWDizsFLJsM5FJtCnO6ppL5dQ5Y3bKXUDOA/gT9gpCk5k8sNQX7oiWHv3r2Oy2tqarjkkkt8+bvMnj2bDRs2pCzzo52CIBgkT/dYtNb7lFIvYSZSdlmL30yq3CQn5s2bR01NjZfmlZTOzk7mz58/Icd+6Ik/8PgLLzGcSBkY5y0nncRxedgzbdo0uru7J+wcvGIifwev6O/vL/pLaCFOdw+pb9eQ4Q1bKTUH42g/DizVWo84tXMj2w1hMvzQpaKtrY3NmzczPDxMKBRi6dKlBQ9rLVq0iN27d49ZXl9fT3t7u68fkrFYrCwjZ6W4IQiCUDZsAr6slHoEEwy7Arh5Yk2qLOqmhAFGUwbGR6tTCoIThWi6H8e8XSfj+IatlDoTE92+BzhPa91XwPEED2hra2Pjxo2JyY/Dw8Ns3LiRJUvSpfi57cvJ4Qbo6+vztQNr5/3t6uoiHo8n8v5KwQ1B8B9SGCcjXwJ2YeZU7cQkLrh1Qi2qMOpqbad71NEOhcMTZY5QBhQS6d4OBJRSl2ES85+LSR340+RGSqk3AvcCV2mt14/XUGF8uKX36+joIBaL5eUoZ0oVmC2jyUSTKe+vn18WBKHSkMI4qWitH8RkArM/9wGfs/6ECaDeinQnT6Qkz0j3u971rkpLgVnR5B3p1loPAGdhnO3XgKuAs7XWe5VSVyqlHrOafg6YhkkTeCjp7+teGS/kRltbW0ZnuLm5Oa9IUqZ9ZctoMtFI3l9BKA+kMI7gd2qnpEa640Awz2fgZZddxsc+9jGvTRN8SkHFcbTWu4DTHZZfD1xv/b8FaBmXdRWKl5pjW1aSDVtq0dzcTHNzc0ZtdjAYZGTEWZq/dOnSguwsFU1NTXR1dTkuFwTBP8gLsuB36mtNgVC7OA7kXxxHqCzk6vAZXmuOs1WNdKOnp4fm5mYikQhz5sxJHH/JkiWuDvecOXN8n2908eLFeS0XBGFikMI4gt+pm5KapxvieY/2XnTRRVxzzTWe2iX4F3G6fYbbkOrVV19d0P680FgnO+AdHR2ObcLhMNu3bx/3sYrNtm3b8louCMLEIIVxBL9TN8WKdNua7jiQZ6S7r6+PgYEBjy0T/Io43T7DSfoAsH//fiKRSIruOhaLMXfuXCKRCJFIhHnz5o2JiJdKYz04OFiS44wXGbIWhPIgGo2yZs0aIpEIgUCASCTCmjVrKnISpeBP7IqUyZpuv89rEiYWcbp9RCwWy5rj09Zdv+Utb6G5uZn9+/cn1u3bt4/m5mZOOOGEhPPtd411qZEha0EoH6LRKDt27OCFF15gx44d4nALvmK0DPzoc1s03UIm5OrwEe3t7cTj8ewNgYMHD7qu6+/vp7m5mba2NlavXs2cOXO8MtGVhoaG7I18gNOQdSAQEE23IAiCkBfVYeNC2Y/tOHFxuoWMyNXhI7yWOGzcuJFIJMLu3bsJh8MErZtBKBTi6KOP9vRY1113naf7KxbRaJTzzz8/ZUQhHo+zdetWKbwhCIIg5ExN2EhJhhmVl+Sbp/uf//mfOe200zy2TPAr4nT7iGJKHAYHBxkZGeH000/nueeeo7Ozk/Xr16foJZcvX044z2paVVVVtLS0lNWw77Zt28aMKEj+X0EQBCEfqi2nO5HQK55/nu7PfvaznHPOOR5bJvgVcbp9RClm5Xd0dNDW1gaM1UuuXr2aZ599lq6uLtavXz9GhpHOnDlz+Nvf/sbChQuLbreXyGRKQRAEYbyEqyx5Ccma7vwi3UJlIU63z8g2kdILcsndHY1Geeqpp+jq6mL58uUp6wKBAMuXLy+LFIFOyGRKQSgPYrEYCxYsyKtiriCUikAgQFVSYNvIS/Jzq8477zyuvPJKT+0S/EtBFSkF77GL4uQ6kXI85Ju7e/Xq1b4vepMPra2ttLS0pKQ5DIfDkv9XEHxEW1sbmzZtStwT7UJhQFnJ2YTJTTgUIJCk6S5F4EwoXyTS7ROciuLkSiAQ4PTTT8+5veQRFQTBz8RisRSH20bmXgh+oyoUZFRdEgeRlwgZEKfbJ2TSEye/OadPdKyvr2fdunVs2bKF9evXJzKUZKLSc3e3t7ePKeYzODgoD3NB8AmZ0qfK3AvBT4SrApAS6Ra3SnBH5CU+YcaMGSmFbtJxq1SZTDQaJRqNjhmWtQkGg1x00UWTSipSCG4P7Vy+Y0EQik+mvihzLwQ/EQ4lp59FIt1CRsTp9gmZdGD5PmQmmwbba5qamhwf6oFAgFgsJnpRQZhAsk2WlLkXgp8IVwVTcnPnWxznQx/6EM8//7zXZgk+RcZBfEKmKLc8ZLyltbXV8SUnHo+LxEQQJpgrrrgi43p5KRb8RHU4mDSRMp53cZyLL76YD3zgA8UwTfAh4nT7BLdodkNDgzxkPCYajbrqRUViIggTRywWo7+/33V9JBIpoTWCkJ3qqtTEBPlqunt7ezNe88LkQpxun9Da2jqmGE1tbW3ZlFcvN9wyuEhmF0GYOLKN6smon+A3qsOjblQc8tZ0L1u2jK985SveGiX4FnG6fUI0GmXNmjUpZdnXrFkjUe4i4ZarPN8c5oIgeMOSJUvo6enJ2Ebuh4LfqA6HUjXdkr1EyIBMpPQRdvYRofg0NDQ46ugbGhomwBpBqGwWLVrE7t27M7ZJr4wrCH6gJjw6OirZS4RsyCuZUJG4ZYsZGBgosSWCUNnk4nCHw2HJyCT4kupwCKQipZAj4nQLFYlbtpjDhw9nTVkmCML4aWtrIxKJZHW4AdauXVsCiwQhf2rCodSKlCIvETIgV4dQkWTKfS5pAwWhuCxatIiNGzfm1La2tlZkd4JvqakOJaUMhECe8pLzzz+fxYsXF8EywY+I0y1UJJmyIEiZaUEoHrnISWwCgQBr1qwpskWCUDg11VUky0vyzdO9ZMkScborCHG6hYokGo1SV1fnuG7GjBkltkYQKoMlS5bk7HBXVVWxbt06iXILvqamuipJXkLe8pLXXnuNgwcPemuU4Fske4lQsdTU1HD48OExyyfbRBil1CnArcDJwNPAJVrrnQ7tPgRcDxwPvAys0Vp/p5S2CpOXJUuW0NHRkVPbOXPmsH379iJbJAjjZ0p1khsVz19e8ulPf5ru7m4WLVrksWWCH5FIt1CxuE2mdFtejiilqoGfAVuABuBrwP1Kqelp7Y4B7gK+qLWeBpwP3KSUenuJTRYmIYsWLcrZ4Q6Hw+JwC2VDavaS/MvAC5WFON1CxeI2mXKSyUsWAmGt9U1a60Gt9Z3AY8CS5EZa6xeBI7XW9ymlgsARwBDQXWqDhclFPhrucDgsmUqEsqI6PBrpDiAOt5AZcbqFiqW1tZVwODxm+f79+1myZInDFmXJScATacueBN6a3lBr3a2UqgP6gfuBb2mt/1J8E4XJSj4a7kgkwtq1a0XDLZQVVaFUNyoQFLdKcEeuDqFiiUajjk43QEdHx2RxvKcC6cL1w4DzLFLoA+qB04BLlFKfKKJtwiQmFovlJCkJh8N0dXWxY8cOcbiFsiOcVJFSELIhEymFisZpIqVNR0cHsVis3B2BHqA2bVkdcMipsdZ6BBgA/k8pdRvwEeD7uR5s165dWdt0dnbmujuhSJTiN/jCF76QU7vm5ma5JoSyJZwW6c5X071s2TKeeeYZDy0S/Iw43YKQgfb29nJ3uh8HLktb9mYgpTKJUurdwFqt9fykxTVAXrNK582bR01Njev6zs5O5s+f77peKD6l+A1isRh9fX0Z21RVVXHjjTeWe/9ypb+/P6eXUKG8SY9055v96iMf+Yi8dFYQ4nQLFU1jYyP79u1zXd/V1VVCa4rCdiCglLoMuAU4F5M68Kdp7R4GIkqpFuBm4J3AJ4BzSmirMEnIVHwKJCWgMHlI13TnS1dXF3v37vXIGsHviKZbqGiuvfbajOvLPWe31noAOAvjbL8GXAWcrbXeq5S6Uin1mNXuAPABIGq1uw34pNb6oYmxXChX2tra6OnpcV0vKQELQym1QCn1ctLno5RSdyilXlZK7VVKbVRKNU6kjZVIVSjtGZHnM2PlypXceOONHlok+BmJdAsVjT203dzc7Lg+Ho8TiUSIRCK0traW5VC41noXcLrD8usxxXDsz390aicIudLW1sbGjRsztpGUgPmhlApgRp2+mbbqe8ABTDGrMLAJ+BZwYUkNrHDGRLrLPFAjFBdxun1CLBajvb2dPXv20NTUVLYOXjkSjUZpb2/PKCXp6upi1apVifaCUKm0tbWxadMm4vF4YlljYyNz587NKVuJ9J+8+QrwQeCrwH8AWLn0R4CvaK17rGXfxUjIhBJSVZWWMrAETvfBgwd5+eWXGRwcLPqxcqWqqoonnkjPTusvwuEwRx11FNOnT8/euEgU5HTnWlY6qf0C4F6t9VEFWTkJicVirFq1it7e3jHrxMErPa2tra7Rbpve3t7JMLFSqABisRhXX311SnXVxsZGrr32WmbPnl3wft1Kue/bty8nh7uhoaHgY1cwt2qtv6SUWmgvsLIMnZ3W7mzgT6U0TBi/pjtfDh48yN///ncikQi1tbW+kUD29PRQX18/0Wa4Eo/H6e3tTQTXJsrxztvpTiorfRNwJkYrer9SarbW+mBaW7dhsYomFouxcuVKRkZGXNuIg1daotFoVqcbJsXESmGS4ybx2LdvH83NzZx88sncd999ee8317zbmbjuuuvGtX0lorXek62NUuoKjNP9T/nufzJkWJnI7B/7Dg0BZtQnXoAt3d2m6G+u21VVVXHssccSj8czprydCDLN5fALM2fO5JlnnmFoaGhCjl9IpHshVllp6/OdSqnPY8pKfzet7ZhhMcGkocvkcNuIg1dasmUyAQiFpBCC4F/cItHJPPLIIyxZsoQtW7bkte9sGUmysXz5cgkieIxSKgysB/4VeI/W+sl895Etzaffmeg0pK8e6AV+Dhg5d762XH755fz1r3/NebsnnniCmTNn+ibCbeP3SLdNXV0dL7/8MqeccsqYdf9/e/ceJ1V553n8U5ZFAy03ucUujM7o5Bcu0USEDbutNoJcokDS6hDDggYN2egQjWOwmzhEIaZbzLgIGZMQM3HtMNFlKERxRxxvEScTEUbx/iAxiDQOXoe0QIA0vX+cqra6u6rrVFXXrfv7fr140XXOqXOeupxTv/rV73mefAzzmcnvIr6nlcb7WWwssDWD43RLkUjEdzCtAC+/Uo1kAtDc3JyHloikz0/AHROb+MmvSCSSVRZr3rx51NXVZXx/6cjM+gH/ijd77Hjn3AsFblKPlG15yZQpUxg/fnxa9ym2gLuUFPq5y+Td4ntaaT8/i/UksbISvxTg5Vd1dTWVlZ0P3hEOh/PUGhH/Min9iPUb8SObLLcC7py5D+8z/BznnH4WLZC2QXf6Ad3OnTvZs2dP1zVIilom5SVpTSudre40rfTSpUt9lZXEDB06tGQeW6m0M5VFixbRr1+/pDWvZ5xxRrd5rNI9+BmmL5FDhw5RW1ubMiBONe52ZxRw54aZnYE3rv5h4F0zi636L+fciII1rAdqP3pJumpqamhqamLWrFld1KLiYmaEQiGeeeaZDh2pp06dyq5du3DOFah1+ZdJ0O1rWumu0p2mlX7//fd9bxsMBlmyZElJPLZSeg38uPvuu4HEQ6M99dRTfOlLXypIbaqmlZaYTAPt9tasWdNpUJzNcRRwdy3n3FPAwOjfL5JJWlW6XJtMt16RhMrLy9m0aROzZ89uXbZ9+/YeORNnJl/RWqeVNrOQmX2VxNNKSzt+h8sqLy9nxYoV6nRUYI8//nibgBs+GVVGJN8ikQijR48mHA53ScANXglbstpuBdwiqQWPC7TG2i2dbtlzTZs2jY0bN7ZZtmHDBqZOndpm2aZNm7j44osZP34848aNo7a2lqNHj/LBBx8wYcIEGhoaANi3bx/jx4/nkUceydtj6CppZ7qdc0fMbDreON1LgV3ETSsNzHHOje7aZpaWRBPdAOzfv7/T+4XDYbZs2ZKPJooPe/cm7pKQbLlIrkQiEb797W93+BLox3HHHddpWdvChQt57rnn2gTJCrhF/Psk6C5MqvvVpbfy0bb/yMuxBo09i1FLvpfWfaZPn86CBQvYt28fw4cP5+jRozz66KPU19e3fumPzU/yi1/8grPPPpu33nqL2bNn89hjjzF9+nSWLVvGokWLmDRpEjfddBMXXHAB06ZNy8VDzKmMJsfxO6103PKniP4s1t1FIhGuv/761pmiGhsbWbhwIb169er0g69Pnz5ZD8klXauioiLhSDMVFRUFaI30ZDfccENGAfeqVat47rnnUgbQ9957L+PGjaO6uppIJOIr4A6FQpSXl7dOwBObfEe/0ElPE2itGVB9SSIDBgygsrKShx9+mPnz57N582ZGjx7N4MGDW7cZOnQoGzdu5OSTT6apqYkPP/yQQYMG8e677wIwefJkpkyZwte+9jXKysr48Y9Lc/JVTQPfBeIz28k+GI8cOZL0/sFgkOXLl+vDqsjU1NR0mDVUX44kl9rPJDlo0CBmzJjB4cOH095XZWUl1dXVrdeVVIF0bDKuBCRdqwAAIABJREFUG264wdf+77jjDl2zRPikTrclg+Hovv3tb/PGG29kdfx0M8+FMHPmTFavXs38+fN58MEHO3QcDYVCrFu3jn/+53+md+/ejBo1isOHD7eJqS677DLWr1/PggUL6Nu3w4B5JSG/85d2Q7HMdmNjY0aZKIBjx47pw6sIVVdXc+mll7aOlx4MBrn00kv1WkmXq62tJRwOs3DhwjZTt3/00UcZlXnMmzevzeQ3fso99u7dy+zZs30F+LGAXkQgEPhkRsp0nXvuuXz+85/v2gYVoYkTJ7J7925efPFFtm7dyqRJk9qsf/jhh3nooYdYt24djz32GCtXruSEE05oXf/nP/+ZpUuXMmPGDH71q1+V7IgnCrqzdOONN7aWkmRK5QrFKRKJsHbt2tbx0pubm1m7dm1ak4qIdCYSiXDyySd3ScfIQCDAvHnzaGxsTBhkp+rIPWDAAF9jfVdWVqY9m6VId3ZcLMGdQab75Zdf5s033+zaBhWhsrIypkyZQm1tLVVVVR1GpWtqaiIYDNKrVy+OHj1KQ0MDzrnW+Oquu+7iyJEj1NXVMW/ePBYtWtRpBUGxUtCdhUgkwsGD7ecJSk8oFFK5QpGqr69vU1oCGr1Euk5tbS0LFy5Ma+z+RCorK2lsbGTPnj2dZrSXLVvGccclvuSHQqEO7/VE2mfQRSS7jpQ333xz6zC13d2MGTPYuXNnwjHJv/KVrzBq1CgmT57Mueeey+9+9zsuuugi3njjDV566SV+/vOfc+uttxIKhbjmmms4cuQIq1atKsCjyI5qurOQbfAVCARUF1nENHqJ5IrfzoqprFq1yvf1I7bd4sWLaWpqarMuEAikLCvRqCQiicUS3IUavaSYxZeBTJgwoc3tkSNHtt7u3bs3K1asSLqfl156qfXvXr16JZ3Artgp052FbIOvAQMGKOAuYsnKflQOJNlasmRJ1vtIJ+COqa6u5hvf+AZ9+rSdVDjVz7SVlZUKuEWSaM10K+aWFBR0ZyHb4CvVuN1SWDU1NR2CE41eItmKRCJ89NFHWe2jT58+GX9hb2ho8FVKEhMKhVRSItKJT0q5FXVL5xR0Z6F979t0KWNa3Kqrq1m+fDnhcJhAIEA4HNbQjpKVSCTCwoULs97P8uXLM77v+++/n9b2d9xxR8bHEukJCj05jpQO1XRn4aGHHkq6LhAIMHfuXMaNG9dmspwYZUxLQ/w4xyLZ8jsGdirZvCeHDBnCe++952vbbDLqIj1GbMjADGLuG2+8sWSHv5P0KdOdoc5+Ig4EAq0jCVRXV7Nr1y5WrVqljKlIDxQbgzscDmc0yU17qYb+S2Xu3Lm+t80moy7SU3wSSKUfdY8bN46RI0d2ZXOkiCnozlBnI5ckKhuprq5my5Yt7Nmzhy1btijgFilikUiE8ePHM2LECMaPH5/x2OwTJ070PUpJ7At5KsuWLcuoLTFVVVW+tlOWW8SfT0YvSd9zzz3Ha6+91qXtkeKloDtDjY2NSdepbKR76aoATEpD+1lmGxsbuf7669N+3WfPns2OHTt8bz937tyEnXdjYpPfdEUgPGjQoJTbKMst4k82HSlvu+02GhoaurQ9UrwUdKehtraWESNGdJqNCgQCyg51I5FIhEWLFrUJwBYtWqTAuxtbsmRJhz4YR48e9T3MXyQSYfTo0b5md4yJDckX33kXIBgMAhAOh1m5cmWXDdu3dOlSQqFQwnVdGdyL9ATRku6MMt3Ss6gjpU+1tbW+fiZuadFp1510NiulgpLuKVlfDT/D/MWy5O2D9s60H5IvH513Y/uvr6+nsbGRYDBIc3Mz4XCYmpoavbdF0hGLujOYBl56FgXdPq1Zs8bXdn5qMqV0aFbKniXbXzBqamrSCrihcEPyaWQeka6hIQOTMzNCoRDPPPNMh07gU6dOZdeuXTjn2Lt3LxdeeCFPP/00/fr1K1Brc0/lJT41Nzen3CYUCqmeu5vRrJQ9S2fnb7Ja65hIJMKBAwd8H6u8vDyjWSVFpLgo1O5ceXk5mzZtarNs+/btbYYuraio4Pnnn+/WATco6PYtVlvZmeOPP14foN2MZqXsOVIFzYcOHWL27NlEIhFOP/301mEATz75ZGpra33VfIdCIRobG2lsbGTHjh26Xoj0cDfffDNXXXVVoZuRU9OmTWPjxo1tlm3YsIGpU6e23t6zZw9mxh//+Ef27NnDF77wBX75y19SWVnJhAkT+P73v8+xY8fy3fQup/ISn+bMmZOypjudqZWlNMTXvu7du5eKigrVvHZTnQ0DGvPMM8906CB57Ngx38MCanZHke6ntbwkg5ruMWPGZD1+/y13/46tr+3Lah9+nT1yON+/6otp3Wf69OksWLCAffv2MXz4cI4ePcqjjz5KfX190pK+gwcP4pzjscce480332TOnDmcf/75nHfeeV3xMApGmW6fxo0bl7S3v3RvGmO9e0g19GNnw4B2BY17LdI9BQKZD6Dw9NNP88ILL3Rha4rPgAEDqKys5OGHHwZg8+bNjB49msGDB3d6vwULFtC7d29GjRqFmbF79+58NDenlOn2ITZsXKoOUn7GvhWR/KutraWhoaF1dKHY0I/gfanK9RCQoVBI416LdFPZdKRcuXIlTU1NXHnllRkfP93McyHMnDmT1atXM3/+fB588EFmzZqV8j5Dhgxp/TsUCvnqW1fslOn2IdGwce2FQiGWLl2apxaJiF+RSKRNwB0TG/oR8D0GdyaCwSB33HGHstwi3ZV6UqY0ceJEdu/ezYsvvsjWrVuZNGlSoZtUEAq6fehseLjY1M36UBUpTvX19UnHz4+d237G4M7UihUrdG0Q6cY+yXRLMmVlZUyZMoXa2lqqqqooKysrdJMKQkG3D8mGhwuHw6rzFSlynX1prqioyGlpiWZ2FOn+PpkFXinvzsyYMYOdO3f6Ki3prlTT7UNNTQ2LFi1qU2KiYeNESkNFRUXCTpKBQICamhpfo5Zkom/fvl02bbuIFLHoL2nKdHfknGv9e8KECW1ujxw5svX2iBEjWv/u379/m+0AGhoa8tDa3FOm24fq6mqWL19OOBxuLSdZvny5MlgiJSDRWOuBQIC5c+dSXV2d9eyiffv2Tbi8V69eWe1XREpDLMEdyKC4u76+nquvvrqLWyTFSkG3Txo2rudKNdScFLfq6mouvfTS1gmugsEgc+fObc1CJwua+/btm3JSrEAgkLST9f79+7NotYiUmkwy3aeffjojRozo8rZIcVLQLdKJ2HCRjY2NtLS0tA41p8C7dEQiEdauXds63FRzczNr166ltraWU089NekslL169WLOnDmd7nvu3LkMHDgw4bpkfUFEpHvJppL70UcfZcuWLV3WFiluCrp9UrazZ0o0XGT8UHNS/JK9hvfee2+nY+/v37+furo65s2b1yHjHQwGmTdvHuPGjUuY0Q6FQurzIdJDxMpLMpmRcvXq1TzwwANd3CIpVupI6UMs2xn74G4/sYZ0X8nqfbOtA5b8yfS1imWq6+rqknaIHDNmDMeOHeuwPBQK6dog0mOoC6X4o0y3D8p29lzJSgRUOlA6kpV/pOInU51sfO+DBw9mdEwRKT0ap1v8UtDtg7KdPVeikS80XGTpiEQiGXdoVKZaiomZjTezd5OsW2VmT+W5SRIVSPCXSCIKun1QR6meS8NFlrYlS5YkLP9IJRwOp9ymtrY26bpMs+si7ZlZwMyuAh4FOoxDaWbTgW/lvWHSgTLdkopqulOIRCJ8/PHHHZaro1TPUV1drSC7RGUyvbufXzIikQj33ntv0vXLli1L+7giSdwCXAj8ALgpfoWZDQVWAT8BPpf/pglAoDXcTj/Tfeedd/LSSy91bYOKiJkRCoV45plnOiQjpk6dyq5duzpMhNOdKdOdQn19fcIRDsrLyxWIiRSxTEYYCgQCvn7JSNWfQ9cG6UI/dc6NBbYmWPePQD3w+/w2SeJlU1QSDocZOnRol7WlGJWXl7Np06Y2y7Zv3857771XoBYVjoLuFJLVbWviCykVZnammf27mR0ws5fMbFyS7S4ws21m9kcz22lm38x3W7MViUQYPXo04XCYhQsXpn3/lStX+gqY1Z9D8sU5l/DNZmZXA8ecc3fnuUnSQXQa+Ayi7w0bNrB58+Yubk9xmTZtGhs3bmyzbMOGDUydOrXNsp07d3LFFVcwbtw4pk2bxkMPPdS67p133uGaa66hqqqKM844g0svvZTXX38d8K77X//616mtrWXs2LFMnjyZ++67L/cPLAMqL0mhoqKCxsbGhMtFip2Z9QI2ACuAc4GLgUfN7BTn3B/jtjsZWAdcHt1+LLDJzHY55zZ13HPxiUQiXHvttRnVcIOX5faboU52XQAYNGhQRscX8cvMRgLfBcZnu6+XX345+wYV2LZt2wrdBMCbBj7dttx1110AnHPOOb62P/7445NO6FVoydo1ceJE1q9fzx/+8AeGDRvG0aNH2bRpE7fccguRSIQDBw5w8OBBrrjiCubMmcOKFSvYsWMH1157LQMHDuSss86ipqaGU089lQceeIDm5maWLl3K7bffzsqVKzl8+DC//e1vWbx4MTU1NTz00EPceuutVFVV0a9fvw7tOXLkSMHeMxkF3WZ2JvBT4AzgTWC+c+65TLcrZpMmTUpYuzlp0qQCtEYkbVVAyDm3Inr7PjP7G2A28PO47U4F/sk5tz56+7noaAj/A8g66G4/1n0xamnx3w0q2XXhuOOOY+nSpV3ZLMlALt9vgUCAuXPnJh27PU+qgWHAG2YGUAaEzOy/nHNp9eIdM2YMZWVlOWhifmzbto2xY8cWuBW/Brx8d7pt6devH01NTb7v99prr1FeXt5m2dy5c3niiSfSOm6mzj//fBoaGjosP3DgQId2xQwfPpzKykqefPJJ5s+fzxNPPMGYMWNaO6yXl5fzm9/8hn79+vGtb3l9gsePH88ll1zCAw88wDnnnEN9fT0DBw7k+OOP5+2332bw4MG89dZblJeXU1ZWxtChQ7n88ssBmD17NsuWLWP//v186lOf6tCeXr16ceaZZ3ZYfvjw4Zx/CU076E4jc+Zru0zV1tZ22pEp1x5//PGCHVvyL5fvt/Lycurr63NVBzwKeK3dstdp1+nKObcZaP2N08xOBM4BOl5d05RtBjpf/IxYEpPs/O/fv7/quQss1++3lpaW1mtBoQJv59ytwK2x22Z2HfBl51xVQRokksLMmTNZvXo18+fP58EHH2TWrFlt1jc2NrJ7927OPvvs1mXNzc2MHj0agF27dnH77bfzzjvvcNppp1FWVtYmUTJ48ODWv0OhEEBRfuZkkumuwl/mzO92aSt0wA0k/WlZup9cv98OHDjAddddB+SkA94JQPuZWg4CfZPdwcwGAA8Cz+J9cc5KfX19UV782ktnNCL19She+Xq/rVmzptDZbhGAhJnnYjNx4kRuuukmXnzxRbZu3cptt93Gm2++2bp+2LBhjBkzhvvvv7912b59+wgEAhw9epSrr76aH/zgB1x44YUA3HPPPaxfv77DcYpdJkG3r8xZGtulbc2aNdnuImvBYLDQTZA8ycf7rbm5OVfZ7gNAn3bL+gIdx8EEzOwzeIH2q8Ac51xa0Uuin+ZKpdPhKaec4rvOb8iQIQl73g8ZMqRo6ks7UwptzFS+3m/Nzc15fR6dc08BCUtHosmtFYnWSe5pSpzUysrKmDJlCrW1tVRVVXUoaTrvvPOor68nEokwc+ZM3nnnHa666iouuugi5s+fz+HDh+nduzcAr7zyCvfee2/r7VKSSdDtN3OWdoYtkUQf4s3NzensIifyfcEtdt35ucjX+23v3r25eB5fBb7TbtlngQ6pezM7Fy/g/imw2DmX9lwPiepDO+t0mG+f+cxn2LFjR4fl8+bNS6sWc8mSJR1qhvv06cOSJUuKoL60c8VRA5s7+Xq/BYPBTp/HfNSHSulbvXo127dvL3Qz8mLGjBmsW7eOm2++ucO6gQMHcvfdd1NfX09dXR1lZWXMmjWLq6++mmAwyNKlS7nllltYtGgR4XCY2bNn85Of/KRoO5Umk0nQ7TdzllaGLZlEH+LBYLDggXc4HO7WH1zp6O4f4vl6v1VUVOTiQ/xJIGBm3wF+jNe34gygze9yZnYasBH4nnNuVboH6UxNTU1R1HRXVlZy//33U1tby5o1a2hubiYYDDJnzpy0ywRiv0jU19ezd+9eKioqqKmpUT13EcjX+23OnDk53b/0DCeeeCL9+/cvdDNyJn7imwkTJrS5PXLkyDa3R48enbRU5pJLLuGSSy5ps+yb3/RGtU00gV2xTriTyTjdrwLWbtlno8sz2S5thb7YBYNBzUbZg+Tj/Zar95Rz7ggwHS/Y/hD4Hl6Hq/fMbLGZvRLd9BqgH1BnZh/H/bst2zZUV1dz55130qdP++/guVVeXk4gECAcDrNq1arWWsG6ujp2797d2nEn07rc6upqtmzZwp49e9iyZYsC7iKR6/dbIBBg3rx5queWLnH//fdrYIYeJJNMt6/MWRrbpS12sStEZ8ocjzQhRSjX77dcv6eccy8DlQmW/xD4YfTv64Hrc9IAPslEdPdfRaQ4JMp8ieRKNjXda9eupampiUWLFnVZe6R4pR10O+eOmNl0vLrPpcAu4jJneJ2vRne2XVc0vK6ujrq6On2IS17E3m8iIiKJqUuldC6jyXH8ZM46205ERESkO2gh7T7n0kNlUtMtIiIiIvGU6JYUFHSLiIiIZCrPie74mRglPYV+7jIqLxERERGReOmnuhsaGnj++ed9bx8KhTh06BB9+6Y15YlEHTp0qHWa+EJQpltEREQkQ9lUlfTp06fDXCSdGTZsGI2NjRw8eLDgWdtS0tLSwsGDB2lsbGTYsGEFa4cy3SIiIiIFcM899/D222/7HoUtNpHO3r17OXr0aC6blpYjR47Qq1evQjejU6FQiOHDhxd0MiIF3SIiIiKZCmSecd64cSNNTU1p3ad///5FN4vltm3bOPPMMwvdjKKn8hIRERERkRxT0C0iIiKSKZVWi08KukVERESypNhbUinmmu4geMX5qRw+fDjnjZHO6TXIvbhzIVjIdiSh87WE6DXIvSI/XyGNc7bYFfr93L9fOcOGDWPQ4EFpt2XAgAEEg8GCP4auUOqPIR/nbKBYh5zZtm1bJbC50O0QKULnjB079plCNyKezleRpIrufAWdsyKdyNk5W8yZ7ueAc4B3gOYCt0WkGASBk/DOjWKj81WkrWI+X0HnrEh7OT9nizbTLSIiIiLSXagjpYiIiIhIjinoFhERERHJMQXdIiIiIiI5pqBbRERERCTHFHSLiIiIiOSYgm4RERERkRxT0C0iIiIikmPFPDlOp8zsTOCnwBnAm8B851yxTkJQkszsAqAe+CvgXeB259zPzGwgcDdwAfAxcJNz7pfR+wSAZcACoBfwS+C7zrk/F+AhSJHQ+Zp7Ol/FDzO7FrgWGAw44G+dc5uj60rqPDWz7wDnOee+HLfs08AvgC/inQcLnXP/r0BN7FSpPd/xzGw8sNE5Nyx6uxfwY+ASvMmW7nDO1RWwiZ3K5HrZFUoy0x19cTcA9wMDgVuBR82sf0Eb1o2Y2cnAOuAHeM/xZUCdmU0FfoJ3Up0EXAjUm9l50bsuAKqBs/DezOOAxfltvRQTna+5p/NV/DCzamARcBEwCO+9sdHMhpbSeWpmJ5jZ7cDfJ1h9H/Ai3peKbwD3mdlf5rN9fpTS8x3PzAJmdhXwKN4X9ZhbAANOw7uOXG5m8wrQxJSyuF5mrSSDbqAKCDnnVjjnjjrn7gNeAWYXtlndyqnAPznn1jvnjkW/fT8FTML7Jvt3zrmDzrkXgJ/jfXgDXA6scM7tcc69B9wMfDPfjZeiUoXO11w7FZ2vktpJwA+dc69G3ye/xAswPkdpnacPA38B/Cx+oZl9BjgbWOKcO+KcewJ4ELgy/01MqYrSeb7j3QJ8Cy9gjXc5cKtz7iPn3C7gRxTvteRUMrteZq1Uy0tGAa+1W/Y63oVDukD058bNsdtmdiJwTnRZC/BG3OavAzOjf48CXm23rsLMTnTOfZjTRkux0vmaYzpfJSaaQT0xwaoW59w/tNv2XOAEvGDvMorkPE3xGPYBlznn9prZzXhfJGJGAbudcwfilr0OjM9ZYzNXqtfFnzrnlphZVWxBtCTjJDpeS4rysWRxvcxaqQbdJwAH2y07CPQtQFu6PTMbgJcteBbYBvzJOdcSt0n8c9/+tYn93RfQh3jPpPM1j3S+9nj/HXgywfJm4j7zzWwMXmnDTc65fWZWTOdpp4/BObc3yf2K6TGkUkptbZXkuT8h+n/7a0lRPxZI+3qZtVINug8Afdot64tX9C5dKPpz3Qa8b7BzgJFAbzMLxL0x45/79q9N7M2q16bn0vmaJzpfxTn3FBDobBszuwhoAOqdc8uji4vmPPXzGJIomsfgQym1NZXYLwvtryVF/VgyuF5mrVRrul/FK9iP91na/rQhWYr+9Pgs8ABwiXPuT3g/uwTw6uli4p/79q/NZ4F3nHP/lfsWS5HS+ZoHOl/Fj+joJb8GrnLO3Ra3qjucp68Cnzaz+OCvWB9Dd3i+AXDOfQT8Jx2vJUX7WDK8XmatVDPdTwKB6HBBPwYuxhtyZ31BW9WNmNlpwEbge865VbHlzrmPzWw9Xk/fK/F6Kn8DuCK6SQNwg5k9jvft9+boMum5dL7mmM5X8cPM/hr4IXC+c+7ZdqtL/jx1zjkz2w7cama1eGUqs4AJhW1ZQiX/fLfTAHzfzF7EKze5AbizsE1KLIvrZdZKMuh2zh0xs+l441suBXYBX472vpeucQ3QD+/NFz/W5j/g9Ui+C3gL+BNej+V/ia7/KTAc+C3ezzJrgSX5arQUH52veaHzVfyoAcqAx83aJFm/6pzb2E3O04uB1XhjL78PXOmce7mwTeqoG14Xl+AN4fgKXhXFarzHVowyvV5mLdDS0pJ6KxERERERyVip1nSLiIiIiJQMBd0iIiIiIjmmoFtEREREJMcUdIuIiIiI5JiCbhERERGRHFPQLSIiIiKSYwq6RURERERyrCQnxxEREZHSY2bH481W+HXgVGA/8Djwd865nT730QLMAF4G/gB8rv0EOGZ2D3B5J7u5xTl3c5rNL0pmdgYw2Dn3ZJ6P2w/4N6DKOfehmf0V8HNgKPAecJlz7h2f+zoO+B0w1znnctXmQlOmW0RERPLlh3hTa18PGHAR3uyAT5vZQJ/7OAn41xTbXBvd7iSgKrpsfNyyH6XV6uK2ARhdgOP+APh1NODuBTyAN7X6aLzp7GviNzaz081stZm9aWZ/MrO9ZvaImc1wzh3Dm5mzWGex7BLKdIuIiEi+XAlc55x7OHp7l5n9Nd607V8G7km1A+fcfwK0m8q+/Tb78bLomNmQ6OL3YvftZgL5PqCZfQrvtTw5umgG8Dvn3L9Fb78KfDFu+68AvwYeARYAO4HBwMXA7cBDzrmNZnanmVU5557KywPJMwXdIiIiki/HgPPN7NfOuT8DOOcOmtnn8UoSMLOTgOXAVKA3XqB2baxUoV15SVaiweNKYDrwMfAw8LfRoD12rMuAWrzM/FbgfwLfBeYCfwRqnXMNcdt/A7gO+Au88otvOuf+kOp4ZnYqXrnM3wHfAZ51zn3JzMYB9cB/w4vbXsT74vJbM3sKOAVYZWaXAFfQruTGzK4AfuScG9LJMTp9HhL4X8BvnXMfRW+PAl6KW/85vMCb6Gv7a+BO59yNcdvsAraZ2d/HLVuP9yvFU0mOW9JUXiIiIiL58vd4geHbZvYLM5tjZkOcczujgWcIr8b708CXgPOBMPCAmeUioxvBi4Um4AXypwH3tdumHi+I/mK0Xf+BF2yPi97/Z2Z2Qrvtl+EFyceAR6KPy+/xLoqu/250v/8CvACcGW1DE/Cz6LbVwB5gcfRvv1qPkUa74l2I92UophEv8MbMTsH74vGP0XUr8AL9xYl25Jz7IO7mI8DkaO1/t6OgW0RERPLCOVcPXAK8gpcp/hXwjpmtMLMgXnb7dLxOeFudc1uB2cBZwOSubIuZTQTOAOY4516OHmsOMM3MxsRt+g/OuSedcy8AG/EywYujHf7uAPrgZbVj7nTO3R/NNF+O12F0chrHW+Gc2+GcewXoi5f1v9E59/toG+4iGuA65z4EmoGm6N9+tR4jjXbFnrcg8AW81zBmDTDEzF4G1uFl9xvN7C+A86LPYbOPdr0KnAB8No3HUjK65TcJERERKU7OuXXAumgWtwqYh1dS8J9AC/CWc25v3PZ7zGwXXmfBVB0o0zEaL6j9IEF9uPFJ+Ur8qCoHgV3OuZbo7T9F/y+L22Zz7A/n3D4zewsYAxxKcbxt0b9/H3f/d83sbuAaMzsT+AxewJtt0vT3cX/7fR5iBgNB4P24dh4mcab9rOj/z/psVyzrPczn9iVFQbeIiIjkXHRouwXOub8BcM59jJc53mhm9wHT8MocEgnQ9b/OHw+8BVyQYN2+uL+Ptlt3LMV+/9zu9nF42ehUxxsc/ftQbGG0vn0b8Bpemck/4QWka5IcuyXBskSx3qF26/08DzGxx++n3KdP9P8mH9vCJ6+xn6x4yVF5iYiIiORDEC9je16CdX/E60j5GnBKNNgEwMwq8DoLvt7F7XkNqMArzdgZHSf8CF7deTaZ1lh2N9Zx8tPA9gyPVx3dZrJz7kfOuceAEdF9x4Le+ED7SPT/AXHL/jJFe9Nt1wd4X0SGptgvfNK5sirRSjPr225RbJ/dcZQZZbpFREQk95xzz5tZBFhrZouBJ/HG6J6MV0M8GW+ClO3AfWZ2ffSudwA7gMe6uEn/ileXfJ+Z3YCXoV4FnIg3skambjKzncDbeIHrK3ijcbSkON7JCfb1ATAcuDBaL10F3BRdV4ZX3vIxMNLMhuFlpt+OtuFv8Gq1v56ivWk9D865FjPtZLxwAAABiElEQVR7Hq9j5790tmPn3HYzWwfcFq0FfyLa5lHAV/Fe6/gx088EPqJtSU+3oUy3iIiI5MvX8Iamuw4vC7oZr6xkmnPu36O10l/Gy3o/hTeSyV5gknPuSMI9Zig6IctM4EO8LwC/wQtyv+Sz018yq4H/Dfw7cCC2vwyP93/xZnm8B2+owG/ijQzSAoyNbnMnXqfUTdFjfB0vu/4q3rCACUcNicmwXQ/jdZD04zLge3jjej+LF2gvxwuu17bb9lzgkSyf/6IVaGlJVP4jIiIiIumIjSHunNtY6LbkkpmFAQf8pXPu3S7a53F4teVfc85tTrV9KVKmW0RERER8c841Av8HL/PeVWYBb3bXgBsUdIuIiIhI+hYDXzWzwSm3TCGa5f4e3kyX3ZbKS0REREREckyZbhERERGRHFPQLSIiIiKSYwq6RURERERyTEG3iIiIiEiOKegWEREREckxBd0iIiIiIjmmoFtEREREJMcUdIuIiIiI5JiCbhERERGRHFPQLSIiIiKSY/8f3cGLy2QqBJQAAAAASUVORK5CYII=\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "fig = plt.figure(figsize=[12,6])\n",
+ "\n",
+ "ax2 = fig.add_subplot(2,3,1)\n",
+ "ax2.set_title('Air Temperature (Input)')\n",
+ "\n",
+ "ax3 = fig.add_subplot(2,3,2)\n",
+ "ax3.set_title('Precipition (Input)')\n",
+ "\n",
+ "ax4 = fig.add_subplot(2,3,4)\n",
+ "ax4.set_title('Snow Depth (EC Output)')\n",
+ "\n",
+ "ax5 = fig.add_subplot(2,3,5)\n",
+ "ax5.set_title('Snow Density (EC Output)')\n",
+ "\n",
+ "ax1 = fig.add_subplot(2,3,(3,6))\n",
+ "ax1.set_ylim([15,0])\n",
+ "ax1.set_xlim([-20,20])\n",
+ "ax1.set_xlabel('Soil Temperature ($^oC$)')\n",
+ "ax1.set_ylabel('Depth (m)')\n",
+ "ax1.plot([0,0],[15,0],'k--')\n",
+ "\n",
+ "for i in np.arange(365):\n",
+ " \n",
+ " ec.update() # Update Snow Model Once\n",
+ " \n",
+ " # Get output from snow model\n",
+ " tair = ec.get_value('land_surface_air__temperature')\n",
+ " prec = ec.get_value('precipitation_mass_flux')\n",
+ " snd = ec.get_value('snowpack__depth', units='m')\n",
+ " rsn = ec.get_value('snowpack__mass-per-volume_density', units = 'g cm-3')\n",
+ " \n",
+ " # Pass value to GIPL model\n",
+ " gipl.set_value('land_surface_air__temperature', tair)\n",
+ " gipl.set_value('snowpack__depth', snd)\n",
+ " gipl.set_value('snow__thermal_conductivity', rsn * rsn * 2.846)\n",
+ " \n",
+ " gipl.update() # Update GIPL model Once\n",
+ " \n",
+ " tsoil[:,i] = gipl.get_value('soil__temperature') # Save results to a matrix\n",
+ " \n",
+ " ax1.plot(tsoil[depth>=0,i], depth[depth>=0],color = [0.7,0.7,0.7], alpha = 0.1)\n",
+ " \n",
+ " ax2.scatter(i, tair, c = 'k')\n",
+ " ax3.scatter(i, prec, c = 'k')\n",
+ " ax4.scatter(i, snd , c = 'k')\n",
+ " ax5.scatter(i, rsn , c = 'k')\n",
+ " \n",
+ "ax1.plot(tsoil[depth>=0,:].max(axis=1), depth[depth>=0], 'r', linewidth = 2, label = 'Max')\n",
+ "ax1.plot(tsoil[depth>=0,:].min(axis=1), depth[depth>=0], 'b', linewidth = 2, label = 'Min')\n",
+ "ax1.plot(tsoil[depth>=0,:].mean(axis=1), depth[depth>=0], 'k', linewidth = 2, label = 'Mean')\n",
+ "ax1.legend()\n",
+ "ax1.set_title('Ground Temperatures (GIPL output)')\n",
+ "\n",
+ "ax2.set_xticks([])\n",
+ "ax3.set_xticks([])"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 10,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ ""
+ ]
+ },
+ "execution_count": 10,
+ "metadata": {},
+ "output_type": "execute_result"
+ },
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAh0AAAETCAYAAACSgrQKAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4xLjAsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+17YcXAAAgAElEQVR4nO3dd5hcZfn/8fdmk01hUyAkJEAgheSGAAGyUqSEon4RRKoCCkgHEUERVJpIAEFEEKT8AhaaUkRAlN4SuhBWQwu5qQlCCAmQJdkUUnZ/f5yZMDuZnZ165pyZz+u69srOOWee85zszJx77qfVtbe3IyIiIlJu3SpdAREREakNCjpEREQkFAo6REREJBQKOkRERCQUCjpEREQkFAo6REREJBTdwzyZmW0BTALGAe8AR7n71DDrICIiIpURWqbDzBqAe4DbgQHAr4CHzaxfWHUQERGRygmzeWUXoIe7X+7uy939NuA14KAQ6yAiIiIVEmbzyljg9bRtM4DNu3pic3NzT2Br4ENgZemrJiIiVa4eGApMbWpq+jyskzY3N38L+LCpqemZsM4ZZWEGHY3A4rRti4E+OTx3a+CpktdIRERqzU7A02GcqLm5eUzbypV3LJ73Kc3NzT2bmpqWhXHeKAsz6FgE9E7b1gdozeG5HwL88+izWDT3k1UbrbGBrSdswPBvfBmAhqavsaz5EQBm3vccU598b7WCtp6wAcCq5ySPTZW6Lx/JcpLPT9YheU5gVZ3S69Fj/Fc55rSLefr5lznj7J9wwLe+WVAdKqFladuq3wf0yt5il3psLsfnUka6eYsKe1+/v+Bz1u/XM+P2dDM/XZS1rOFrrZHzebsq6+25X7xF5szreOyQQR3PM2pwY07lFCvTed6e29phe/J8nR1bqnOX8rq6Olf6ObP9fxeiq9dNptfnoDUaSlqHpNT35iMPT+GMn01kl8024PdH70Zdt7pV+xqavgaw6rM3Veq+9M/HpJn3PdflZ276Z/TUJ9/DW5etugekGv6NL6/2+b/1hA1o79uPtkOPg8T9JAxv3jfFBwxfn37DhgAcCVwb1rmjKsygYzpwStq2jYGbcnjuSoD1Fy+k+cN5AGzWryfb7DiS0btvBSuWAtDQvduq3+sWtLDNlv146qF3OhRUtyDot9qQOA5gzO5b8eYdkwEY/e1dV5WRr7oFLR3KTtaBxHYgeAyrtiWPbehRzx8uPo29jzidH598Jjdedz2bbLEF45u24MCD9qWhoTwfLKUwqAd8smQlA3vX53xsUo8eXT8nXbcV2VvY1unfgzmt+Qce663Zg0zLHy6vW7HatiVt2YOl5XW5X9d6A/vx1rzOb5wLln/xe58Ba/D+nC+O7bO847HZ6rVgeae78pbpPOuu3Y8lbR0fB8eu/vzkvhkfLsjrvBsP7bdaeeuu3S/vcnLV2f9ntmsrRlevm/b6Hqttm7u0nSGNpf98SH1v7rHn15g69b9cfeUfOWGX0Ww5YjAADTvs88UTMnxuNnQP/v8att2dMcn9K5bSsMM+LHvmHiD4/O3qM3fM7lut+v3NOybz+UcfMxz4fNEXn+mrzrli6Wqf/2nHhNJE39zcPGbU13figZMuYJ1xxsb7fmVSc3Pz9bWe7QizI+lkoM7MTjGzHmZ2MMHQ2bvzKWSzDJF+quSbYPS3dy2wmoUb/e1dizpvr14N/OP6CznxiP1Ys39f7rn7Xn7y47M47JDvE/XVgHMJOOJ4rs5sNKjzb7jZ9pXC+kMaO/wbZxsP1eC1OKirq+PoYw6jvr4bP7v7ZVaM371jwEHw2Zu6LX1/ukzPL4eddh9ZlnK78uZ9U3zuK28w+4WXee22++jRpzcE2Y6aFlrQ4e7LgD2AA4BPgbOAfd19Xr5ldRV4pEp9wSV/zxQYVCJIyaRP715ccs6J3Hvzb5jz0j18aQtj8uNPMWvW/ypdtZJJBg3lDB7K8c0vXSmDi2xlZboxFxJwpJaz8dB+uuFHULaMVzaFZPbytf7663LNpEv5d/NrXPeXf3Z6XHrwUWqjv73rqs/yzgKK5Od5pQKOZJaj+bq/AbCsdTGv3HIvrXPmTWpubo5u2joEoc5I6u6vuvuO7t7X3Td398fzeX56212qbC/ynXYfmdOLr5DAI583V77l19fXc/iBewLQo8fqadU4KzbgiEK2A4JgoVTBRynLyqYag41qvKZMZrYs6XRfqQOP1GbQpP3234v/23kbTv/VJI497WKaX3Y+/zy/82b7zEwGLLkELqmf6blkmQvtq1eI1CxHkrIdgdhNg95VhBslXb0JcglCXnxpBgP6NTJ06DqlqlbNKFW2Y/iA9P7P1SMKN+so1EFy9/9umMRhhx/EHf+azA57n0B/+zr9xuzONnscy1V/vpOlSzMHIZkCia4CjHwzJlHIWKdnOZJKke0ws23MbG7K4wYzu87MPjWzeWZ2RpHVL7vYBR3QdcCRrV9HKV+U5cxyJPnb7zFu7EZ06xbLP1XFDWlsCLWppRSZikxl5HJjLtUxUdVV3eN8baUSRjNL3359ufSyC3jptWf4/VUXc8aZp3D894+kV88GTjvvasZ95XBu+NsDvP/hPJYs/Zx33pvNjLdmsWxZYT2ZM33Opn6edvbZmssx5ZApy5FUaLbDzOrM7BjgYSD1A20iYMAogqklDjez7xVa9zCEuvZKsYZ/48vMuvWBVY+TL6Ryth8WK/3FPvrbu64aKZOLtpVt1PfvW+pqVYWBveszpoAzGdLYUPYP5DCaRsph46HlG/0RpzpIftZccwAHf2f/lC0/5aknn+P8s8/j+z+7ZLXj+/Vdg+MP24ezTv4evXrl90Ug9TM+OeoFSpNNLqXUESuZJLMdBYxkmQh8A7gAODtl++HAEe4+H5hvZr8Fjie3UaEVEaugA/J/ESWPf/OOyas9N/lCTn0Rp+vsxZ7eSztbGZ3VKRfLli+nf/do9F+Iu2ICj+EDemdtTy+1jQY1rtapUDfmrtXC/9HMliWhNfnlOhQ+aacJX+bBKffx0rRX+fe/X2T5suUMGjSQ+u7deeL++7nkmlu4/A9/Y8SwoWw0Yj222HQ0O2+3JTt/eUvq6uq6PgFffN5GoSklg5/MffXNjFmOpNduu4/ND/km8+fPP9LMHspwSIu7t6Rtm+Tu55jZLskNZjaAYIbV6SnH5TTLdyXFLujIVXogkO0F2lnQUMyQrnwDkc4sWbqMXr16FV2OSDZRuFmXqg5RuJZKmtO6LJQmxc5069aNrcaPY6vx4zpsP/CgfTlwyjNMmfI0s2b+j7dnzODByS9w0e9vZszIYdioDRi89pqM3HBdhq07mC033YjRI4flHIwk5fJlsowGt789s4sRlitZPvsjXp7zvwMIVl1PNxE4N3WDu8/OcFwytZo603eus3xXTKyCjmWb7EhjvzU6zTiky3bjzxRQJI8tpLmmmOdnO7510WIaG3Of3bLW5NPEAvHPdpRTFG7WCjyq28677MDOu+yw6vGiRYv51z8f5J7b/8Y7783mueZX+fjTz1btHzJoLTbfZBS77TieIw/6BgP6B/fZ1MAiys3r2TQ1NT0JHJdhV3qWozPJ6YlT0165zvJdMbEKOvY96kyuOu9kxhX5IitVT+munl9stmPFipV89PF8Bg1au6h6Vbt8A484i8LNtBIdNgs5Z/I5lf7/irt8m1jyscYafTj4O/t36BuyYMFC3n9/Ns//+0VenDqN119+mTMuvJZzLvkT4zYZxQ5bb87R39kL22iDvL50NjR9DealL/9VOY2NjUvdfWahz3f3+WY2h6Aj6QeJzRvTsbklcmI1JOLzzz9nmz2P5bCTzmf+Zwtzek7qcKxyT1pTak+/8DIrVqxkk7FjKl2VqlJM6rmah8/C6jf35CRiYQYapT5X3Ea1lCK7FcYolnLp168vY8caRx51CFf/v0t4/JmHeGzyPzjhB0fTu1dPrr35Hrb46hHscchpTJ2WvnB5R7nO+RFjNwO/NLO1zWw4cFpiW2TFKtNx861/5rprb+TaSdfz7IuvcurxB7H1lpuwZv++rFzZRsuCVhYsXERdtzrWWXtNbNQGNDQEk2pV6kWXjLZz6ZCa6ldX3MQVf7yD9dcdzO6771b2esZdmM0sYcq1Q2kpb6ydZVPCzLKU+lyp/z/VkPkIszNpFGw+blM2H7cpAB9//Ak33XAbN/zxJibs90P233MC/7fzNmy12Rg232Rk3n1AYu4c4FLgNYIkwnVk7icSGXVRX9MDoLm5eTjw7vojRtGjRwP//c/LnHXambz4kmd9Xt/GPhx3yN6c+aPDWKNPfN6gy5evoL99nWHrDuam2/7EJpso05GLQptYCgk+wuzb0dk339SbZ6m/zc/4cEHFMwTJ6ytHPXIJPCp5/bkMv84l6Chlh9KozAKctHDBQi677Bpu/+sdq/qBDB08kB23GcfgtddkzKhhfO/bX6d3r6BT57IVbbweNK+MaGpqmlmOOjU3N9/14V0P7jfzquwjVjf/fxfQaCN/2tTU9Nty1CPKYpXpSNpq/Djue+xfvPnG27zzziwWLmylW7c6+vfvR99+fWlva2P27Dk89q9/cdl1t3P/489x4xVnM27sqEpXPSdvz/qAtrY2Tj39FAUceailvh1Q3sxDpQOOZB3KeX3VkPGoZX379eWX5/6cs39xGrNm/o8Xnm/miQcfoPkVZ94nLSxsXcyVf76TS37xA76+67aVrq4kxDLogGDVwzG2EWNso06P2f+Ab3LQE8/yw+NPYcd9f8CJR+zHsYfuzYhhQyOdgnv6hVcA2Hrrrbo4UtIVEngU0tQS5kiWbKNYohAclFM5r6/amlwyKeXw2XJ2KC1GfX09I0cNZ+So4Rz83QNWbZ8y+WnO/Okv2O+oM1lv6CD22n0njjr+iMpVVIAYBx25mrDz9kx+5gF+dcY5XP6HO/jddX9jjT69GDJ4IP0a+7DWmv0YPWJ9vrTFJuy243jWXafyI0UefXIq6w1Zm5Gjhle6KjUjLn08pDziGLzl2q+j0vN2VMouu+7IlGcf4o6/3cMTU56hvS36XQlqQdUHHQADB67FZdddxQmnvc0zzzzPW2++w8cff8KCBQv5bO5H/PWuR5h0U9C5c6MR67PNVpswbpNRjBg2lDUH9GPw2gMYvv7QvKfuLcTiJUt54PF/c+j3Do50NibKwmpmCXveDpFClSrwiGq2ozMNDQ0ccui3OeTQb7N8+TLef/ftSlep5tVE0JE0eswoRo9ZvV9HW1sb06c7Tz7xLC88MYXJT/+HW+56pMMx3bp1Y8zIYWxqwxk9chhDBq1Fr149WblyJd3r69l2/Fg23mjDous46/05fL5sOU1f2qLosiQ/YTWzpH47zfW5YU8UJtWnVjMeEi01FXR0plu3bmy22SZsttkm/ODEowH49NP5fPD+bFpaFjB37jzefPMd3pj2X/776pvc/cBTtLW1rVbOmJHD2K5pU8aOGc7gtddkyOCB7LzdFtTX5/7N4PU3ZwEwatSI0lxcjQqzU6kyHhIXpQg84pbtkGhR0NGJtdZak7XWWjPjvhUrVjB//mcsXbqU+m7dWLJkKY8+OoVnHn2M+x97jpvueHDVscPWG8x+X5/AsYd8k9Ejh3V53vsf+zdr9OnFppttXLJrkdyF3bcjn4BF2Q5JV2vzdUj8KegoQPfu3Rk0aGCHbaM2GsHx3z+S9vZ2PvtsAZ9+Mp9XXpnO32/+K5Nuvoerrr8LGzWMzWwke+++Iwd8Y2e6des4Iexzza9y6z8e4cijDqGhQWnQYhWa7Uj9JphrAJJr8KAbhFSash1SSQo6Sqyuro4BA/ozYEB/Ro4azj777sm8eZ9w4w238OrUF3j2xVe4497JnDrxKnbcZnN22/FL9Ondk/+87Fz3138xZPBAfnb6jyp9GZKQT+ajs8Cjq0BDzTMSRwo8Mlt3g/4M231k1mNWZF2Ftrop6AjBoEEDOe2nJwGwcuVK7v3XQzz80GSenvIUd93/JADdu9dz4N67cfZF5zNgQP9KVlfSFLsybSmpiUVKQZ1KpVIUdISsvr6effbdk3323ZOVK1fywQcfsuzzZQxddwhrrNGn0tWrOmHPUpqatcgn4FC2Q+JI2Q7JV6xWma029fX1bLDB+mw0eqQCjjIK+0Nx+IDeZe27kcu6HFI7Cg1WS9VhupaWHpDiKegQyUEYqWh1MpWwKfCQsCnokJoQlxRwroGHsh1SKgo8JEzq0yGSo7Dm8Chl/4704ESdUKWc1MdDuqJMh9SMavsw7CrbkWm/MiTVqdggtZTBtDIeko2CDpE8hDXMsNj+HdmCCwUekokCDwmDgg6pKXHKduQSeBSazdhoUOOqH5EkBR5SburTIZKnsNdn6UrqhGGFBBGacExSlXLiMPXxKA0zOwq4Fvg8ZfOJ7n5jhapUMAUdUnPCnjCsGLl2Ki02Y6HAI/6iuvibAo+SGA9c6u6nV7oixVLzikgBqnEKaTW1SFKpM3lxCfIjrAmYVulKlIIyHVKTqjHbUQrKeFReVIK/Uq/Pkny/KesRaG1t7WVmwzPsanH3luQDM6sHxgGHmdllwGLgj8DF7t4eSmVLSEGHSIGi1rejVBR4SDlVe3PLgNHrM2StXbMe8+5afXni380TgPMz7J4InJvyeBDwInAjsD+wCXAPsAC4pgRVDpWCDpEYCHtBOAUeAuVbjbbaA49cNDU1PQkcl2FXS+oDd58D7JyyaZqZXQkcgIKO3JjZNsC97j64EucXgdI0sVRrtgPyCzwKbRJQYFNaUe1MmkmtBx6NjY1L3X1mV8eZ2abAge7+y5TNDcDSctWtnEINOsysDjga+G2Y5xWpBmFnOyC3wKOYPgjJ5yr4qE3q55GTFuBUM3sf+BOwFXAy8MNyntTMugEbA4OBlcAc4K1i+5GEPXplInACcEHI5xXJqBQfdtU4kiVVGBOJRaXzZKVF8f8hjExeXDp1V4K7fwDsDRxP0I/jTuB8d/97Oc5nZhPM7E5gPvAq8DjwBDADmGdmfzGz7QstP+zmlUnufo6Z7RLyeUWkBNIzE6W8SaofSW2r9eaWbNz9ceBL5TyHmY0mmIBsA+Bugk6r04FPCBIUg4AtgAnAbWb2NnC8u7+Rz3lCDTrcfXaY5xPJRZz6dlSiiSWTcn0jV+ARTeXqUJpOgUdF/QU4z93v62T//xI/95rZz4F9E8/ZJp+TaHIwEYmUKDYxhKFU1x2FoLQYamqpmO2yBBwduHu7u98NbJvvSRR0iJRIXFagjYNaDTwk8MmSlQo+Qubu7Wa2l5lNN7M1c31OvudR0CGCes9HUS0FHnG41koMDVfgEboTgbvcfX76DjPrbWY/MbNhxZxAQYdICVX7SJawxeFmLOWlwCNU4whGx6zG3ZcAWwKnFnOCigQd7j7F3Qfk+7yWpW3lqI4IEK9sRy00sSRVe+BR7ddXCgo8QrMWwWiVztwOfKWYE2gadJESq+ZZSisl06iWbDfruIyAKVfAUa6ZScMaxZKJJhILxWxgI+C9Tva/BhTVvKKgQySmojJ8Niz53KDjMNOpMhyF0bDasnqUYKbTxzvZ36vYE6hPh0iKUn2YqW9HNET1xh7VesWFmlvK5hJgDzO7MLFsSbrdgLeKOUHsMh2KckUkH1HLesQ94KhkE0uqqN4L6oeOpGHjTbIeU/fxYlgevT6K7v6WmX0XuAXYx8yuAl4AFgK7AL8CzirmHMp0iKSJU7ajljqUFqvc68fkWgcpHc3nUXqJSb++DMwDriYIOl4HJhE0v1xbTPmxy3SIxIk6lUZPJaZaV7BRXlHNesSVu08DdjGz4cB4oDfwiru/XGzZCjpEYq7WOpSWQlhNLpUMNso1ggWi08SSSoFHccxsHeAMgoXdpgN/cveZwMxSnieWzStKp0m5lfLDS80s0VXOheuU3QifmluKcivwDnA9sBh4zMx2KPVJlOkQCUEy8FBTS/QUk/VQYBFNynoUZLC7/z7x+6NmdhdwB3muItuVWGY6RMJQjg+tcmY9lO0oTj7ZCWUyoh9AK+ORt3lmtl3ygbvPIujLUVKxzXQokpW4UufSaMvW0bTWA4240X0iL8cBd5vZc8ArwFjgjVKfRJkOkQooV8ZD2Y7SyJTJUMART+rnkRt3fxPYCngA6Ac8D3yn1OeJbaZDJAwDe9eX7QOrXP08NJqldBRoZBfFUSyd0YKh2ZnZAHdvAe5K/OTynDXdfX4+54l10KHUmVSDcjS3JDMeCj5qWzmHzUp4zGwLgsm5xhGMMDnK3aeW+DRPmNktwCR3/6yL+qwNfB84CNg8n5PEOugQCUM5sx1J5ernoeBDJN7MrAG4B7gcmAAcADxsZhu6+4ISnmoH4ALgfTN7BniQYFXZj4E6gvk7tgB2BnYCbkw8Jy8KOkQiopwdTFO/7SoAkVKKUxNLlLS2tvZKzPiZriXRzJG0C9DD3S9PPL7NzH5IkGX4Q6nq4+6twI/N7NfA8cB3Cfp4JJsTlgP/Be4DjnX32YWcJ/ZBh5pYJAxhZDsgnJEt6vMhUnnNzc0TgPMz7JoInJvyeCzB2iepZpBns0au3H1Oog4TzawbMBBoc/dPSlF+7IMOkWoTVuABynqIlNqyhkYW9B2S9ZiVn75LU1PTkwTDVNO1pD1uJJghNNVioE/BlcyRu7cRLPxWMlURdCjbIVIYZT2kFNTEkr/GxsalibVNurKI1Sfp6gOEu2phiWieDpEchRnYhvkBrtENIpE2HbC0bRsntsdOVWQ6RKpRmDOXKuMhElmTgTozOwW4imD0yjjg7orWqkBVk+nQjHMShmpuxhs+oLeyHlUorGBSU/uXh7svA/YgCDY+Bc4C9nX3kva1yMTMxprZfma2hpmNMLO6YstUpkMkwiqxTouyHiLR4u6vAjuGdT4z6wfcBnwdaAPGAJcBI81sT3d/v9CyqybTIVKtKtFBTxkPKYSyHVXjUqAnsD6Q/AZyMsHImss7e1IuqiroUBOLhKGam1hSKfAQqVnfAH6aOgGYu78HnATsVkzBVRV0iFQrDUcUkRA18kWGI1U9RcYNVRd0KNshUjrKdki+1MRSFR4EzjWzHonH7WY2CPgt8EgxBVdd0CEShko0sVQq26HAQ6TmnAQMAz4hmIjsUeA9oB/wo2IKrsrRK5qhVKpVJUazgEa0iNQSd/8I2N7MdiVY+6U7wfovj7h7ezFl5xR0mNnmBOOEvwQMBlYCc4CpwL3u/lYxlRCR6FPgIbnStOjxZmb3EnQknUwwOVnJZG1eMbMJZjYZeBH4JjAfeDbxeClwKDDdzB4xswmlrJhI1FUqm1bJD3NNICZSE7YjWMq+5DrNdJjZn4FNCaZd3c/d01e+Sx7XD/gOcLmZvezuR5SjovlSE4tI+SjrIV2p1WzH0hXtXQ5oWNFWVAtFGH4H3GRmvwPeIW0ki7sXvO5LtuaV+9z9qK4KcPcFwLXAtWb2rUIrIhJHA3vXV2TEVKX6dqRS4CFStc5P/Ht7yrZ2oC7xb8Hf6DsNOtz9znwLc/e/Z9tvZl8Dfg2MBuYCl7j7tfmeJ1fKdoiUlwKPeJjZskTNYpKPEeUqONeOpN0J+m9sSjA1agfufnIOZQwD7gQOB+4BmoCHzGymuz+UT6VFJBrZDvhiSK2CD0lXq00scefus8pVdq5DZm8C9gFeIOhAmirXxqnhwC3unlyOd6qZTQF2AMoWdCjbIeVWqSaWqFHwIVIdzGweWe7t7j640LJzDTr2Ar7l7g8UeiJ3fwp4KvnYzNYCdgJuLrRMEYkeBR8isXda2uPuwCjgCOCMYgrONeiYC8zu8qgcmVl/4J/A8wRNLWWlbIdUq6g0sWSi4ENATSxx5O43ZtpuZlMJZiTNuD8XuQYdPwWuMbOzgXeBtrQKvpfrCc1sDEGgMR04xN3buniKSOSpiaVzqR0YFYCIxNpLwLbFFJBr0NEd2Jxg/vVUeQ2fSUwgdg8wCTiz2OlU86Fsh1SrKGc70in7UZuU7YgXMxubYXN/4GygqBnIcw06LgP+RjAfx+JCTmRmo4B7gbPc/cpCyhCR6qDgQyTSXuWLeTlS/Q84spiCcw061gQucPeZRZzrRKAvcJGZXZSy/Wp3/3kR5eZM2Q4pp0o2scQp25FKwYdIcczsJuBAYEXK5nHu/k4RxabP09EOLAM+CmXBN4JZyQ4mmNirIO7+E+AnhT5fRKqXgo/qpyaWshkP7OvuD5awzOuB/dOXPzGzQWb2oLs3FVpwrkFHK3CumR1C0J7TYSEYdz+w0AqETdkOqVZxzXakUvAhtaK1tbWXmQ3PsKuls7XO0plZb2BjYFqx9TGzXQiWsQfYGTjOzFrTDtuEYOhswXINOvoBtxZzIpFaoFEspaHgQ6pdc3PzBL5Y4yTVRODc5AMzawDWynBcOzCSoFnlD2a2HUGfi3Pc/d4CqvQJwfwcdYmfHwKpH2btBAmIUwsoe5Wcgg53L6rjSNQo2yHVqhqyHam0tkt1qYUmlkXLV9LSxXuwZ1s7TU1NTwLHZdidnuXYHpic4biVwJ4Ek25OJBjOujfwNzP7sru/lE+93f0VgiAGM5tM0LwyP58ycpFtafvrgV+4+/u5FJRIE53n7t8rUd1ERBR4SFVqbGxcmsvgDHefwuqjSFI9nPL7nWZ2JEHwkVfQkXbOXTNtT2Rdmtz9uULLzpbpuAd4wsyagbuAh9KjHjMbTND2cyiwFfDjQisSNmU7pFwq3cRSbdkOUOBRTWoh2xEWM/smsFbaDKINrL5GWr7lbkcwRcZYoFva7nZy75qxmmxL2//DzB4FjgcuBP5qZnOAjwmirkHAYIIZSicB33X3RYVWREQkG/XzEFlNPXCFmb0ONAMHETTHHFNkuVcA8whGrd4IHAsMA84qtuys0Yq7twKXApea2aYEy9GvQzAN+hyg2d1nFFOBSlK2Q6pVNWY7kpT1yF/qVPRSPRLJgbMIBnoMAWYAe+WzNEknxgHbuvvLZvZjgvk5bjWzuQQtGncUWnDOKRJ3fw14rdATRZUCDymHSjexgAIPiS41sZSOu18NXF3iYlcACxK/vwFsCTxO0KH18mIKTm+rERGJheEDeusbvEh5PA/8wMy6EXRI3SOxfTOCmUkLVnBnkGqibJP5G7IAAB3VSURBVIdUq2rOdiSpr4dIyZ0B3A/MBf4E/NzM3iHoXvGHYgpWpkOkTBTIhktZj3ip9mA4ztx9KjAcuDExarUJ+C1wFHBKMWUr05GgbIdUq1rIdiQp6yFSPDO7F/ipu78O4O5zgGtKUXZOQYeZdSdYznYLoDdpE5W4+1GlqIyIlEctBR6g4CMu1KE0srYjbY21Usk103EVQdDxJKtP0Vo1lO2QUovCKJZapuBDpCC/A24ys98B7wAd3kDuPr3QgnMNOg4GDihwEZlYUeAh1arWsh2pFHyI5CW5EN3tGfa1E0xKVpBcg44VwOuFnkREoqGWAw+ozeAj6h1s1cQSSSPKVXCuo1f+BPwsMWa36ikdLqUUtcyZPuCjfyOW+Frw+QpmtizJ+rNsZVulq5mVu89y91nAGsB4guVPugHvJbYXLNsqs1MJ0ijJ47YEDjCzWQRL6qZWcJtiKiEiErZazHqI5MLM+gG3AV8nWPZkDHAZMNLM9sx19flMsjWvpPffuKfQk8SR+nZINav1ZpZUmlK98tTEEjmXAj2B9QFPbDsZ+AvBNOjfKrTgbKvMTkz+bmYTgOfcvcMQGjPrCexZ6MmjToGHlEoUR7Eo8PhCtWY91IwkBfoGwcJxs80MAHd/z8xOIlh/pWC59tGYDAzIsH0kcEsxFRCRytG3y450kxYBoJG0YbIJ9RQ5k3m2Ph0nAMlsRx0w3cza0w5rBP5bTAWiTtkOqXbKeHRUrVmPqFMTS6Q8CJxrZocmHreb2SCCqdAfKabgbH06/gAsIohq/kwwbvezlP3tQCvwWDEViAMFHiK1R309pIadBNwNfAL0AR4F1gWmA4dmeV6XsvXpWAHcBGBm7wLPJLaJSAGi2K8jSdmOzOKc9VBTkRTK3T8CtjezXYBNCWKF14FH3D29xSMvOU0O5u5PmNmERJPLZgRDZl8Cfu/uzcVUIC6U7ZBqp8Cjc8p6hENNLNFhZvUEI1h6EdzzS7IWS04dQszsYODxxMlvAe4g6Fj6rJntUYqKxEFUv6WKlIo+8Ds3fEDv2GQP4lJPiSYzGw68RjBVxnHAiQT9PKaa2TrFlJ3rNOjnAae6+xVpFfsJcDHwQDGVEKkVUW5iSVLGI7s4N7lI9TKzU4Cd3X3flG0bEMwovh0wFzjJ3e/PobhrgXeBCe4+N1HWEIIuF1dTxDwduQ59WY/MgcW/gI0KPXkcRf2GISLhiGrmI4p1yocC3vyYWaOZXUIwoVe624CXgYHAscBtZjYyh2J3IEg0zE1ucPc5wKnA/xVT31yDjrsJZiNLdxg1NlMpKPCQ6qdmltxFNfiQmnEfwQJt16ZuNLMxwJeAc9x9mbs/DvwTODqHMt8FxmbYvj4wu5jK5tq80gocY2ZfBZ4lWHV2PLAV8LCZ/S15oLsfWEyFRKpdHJpYQM0s+UoNPCrV9KLgJz5aW1t7JfpOpGtx95bkAzNrANbKcFx7YpTJdxIzh54LDE3ZP5ZggbZFKdtmALmslXY5cE0icHmaL+75ZwPXmdmqmchzbK5ZJdegoydwa+L3OqAH8EripyZpNIvUAgUehalEAFJNAUecR7F8tmQ5by1qzXrMuv3beKm5eQLB/FfpJgLnpjzensxTj68Eurt7Z5mHRmBx2rbFBPNudOUPiX8vyLDv7JTf2wlmKc1ZrkNmj8yn0FqhwENqgQKP4oQRgFRTwFErmpqaniQYGZKuJfWBu08h+LKfr0VA+gujD0HLRVbuXtRU59nkmunAzDYkGDYzBjgB2B2Y4e7/LlPdRKpWXJpYpLRKHYAo2IivxsbGpe4+s4ynmA5sYGa93T35Yts4sb1LZtaHoK9Iz7Rd7e5e8PInuc7TsS3BmN0tgD0IoqctgSfN7JuFnrwa6MYhtSCuqe4oS3ZALaQjai10XlV2rTju7gSTeP7KzHqa2a7APuSwSGtizZW5BCNfXszwU7BcMx2XABe4+6/NbCGAu//YzD4maJP6Vy6FmNlewIUE0dNc4Dfufm32Z0WfmlmkFqiZpbyqPYiQijgAuI7gfvsxcLS7v5rD8y4imN/jMmBpKSuUa9CxFZCpX8dfgTNzKcDMhgJ/B/Zz9wfMbDzwjJlNdff/5FgPkaoRxyYWBR4i0eTu52bY9j+C1ol89QOucvdZxdYrXa6dRT4BRmfYvjXwUS4FuPuHwKBEwNGNYLKSFcDCHOsQaXG7eYiIRJ0C3Iq5GTiiHAXnmum4CrjWzE4n6EW7ZaKp5Bzgt7mezN0XJjqnfJY498Xu/maedY4sNbNILVC2Q6TqXQL8x8wOAWYCbak73X23QgvOdcjsbxN9OS4iGHLzd2AOwRjeK7I9N4OlwBrAOOB+M3vT3f+UZxmRpcBD8hHHJhZQ4CFS5W4mGFp7H6vP9VGUnIfMJjp8XmtmawD17r6gkBO6exuwDHjRzK4j6E1bNUGHSK1Q4CFStbYGtnX3l0tdcJdBh5mtDewFbErQueQzYJqZ3efun+V6IjPbGbjM3ZtSNvckbSKUaqBsh9QKBR5SbnGenTTGHBhQjoKzBh2JpevPJ5jq9F2CAKEfweJvy83sLHf/fY7nmgaslyjzCmBbgoVn9iuw7pGmwENyFdcmFhGpWhcBN5jZVcDbwPLUnfmut5Kq06DDzI4kCDhOA25ImdEMM+tF0LP1EjP7wN3v7OpE7v5ZYpGY3wO/BP4HHOPuTxRa+ahT4CG1QNkOkaqTXGst00CRvNdbSZUt03Ey8HN3/3/pO9x9KTDJzBqBHwFdBh2J5/0H2LGQiopIdCnwkHJSE0u4KrX2yhjgoS6e/0/g56WrTvVRtkNyUQ1NLAo8RGD+omXM+PDzrMds06cb9ChkDbdwmdlYwICHgcHATHdvL6bMbNFMb6CrESqfAWsVU4FaEPebiUiu9G1UJP7MrJ+Z3Q+8SjBFxjrA74CXzGz9YsruKoVSVEQjX1DgIbVCgYdI7F1KMLp0fb6Yp+NkgsEklxdTcFdDZo8ws9Ys+/sWc/Jao6YWyaYamlhEykX9OkL1DWAvd59tZgC4+3tmdhIwuZiCswUd7wEn5FDGe8VUQESqj/p3iMRaI7Akw/Z6cl+zLaNOgw53H15MwZKZsh1SKxR4iMTWg8C5ZnZo4nG7mQ0iGEL7SDEFl21YjHROKXTpTLUFpEqHSykpiA3NScAwghXm+wCPErRq9COYJqNgOa+9IqWljIfUCmU8ROLF3T8CtjezXYGxBLHC68AjxQ6ZVdBRQQo8pFYo8BCJNjM7B/itu69aVdbdJ1Nkx9F0al4RiZhqDUTV1CISab8k6EBaVsp0VJiyHVJLlPGQYmnobGZmdgqws7vvm7JtN4KOn6kjUS529/MzFBHKFKkKOiJAgYfUEgUeIqWTWAPtl8CpBEuTpBoP3OHuB+dY3PqJBV2zcveCp8pQ0BERCjwkVbVPFKbAQ6Rk7gPmAdcCQ9P2NQHT8ihrahf76yjjKrMSMgUeUksUeEgta21t7WVmwzPsanH3luQDM2sg8xpn7YlRJt9JzBx6LqsHHeOBQWZ2AkHAcDtwtrt3tiLdrgTDZMtGQUfEKPCQWqLAQwoR5X4drYuX8/6cpVmPWbbhGjT/t3kCkKlvxUTg3JTH25N5BMlKoLu7z850DjPrDrwP3A1cD6wL3EGQqfhZhqe0AzPcfW7WyhdJQYdIRFV7E0uSAg+pRU1NTU8Cx2XY1ZL6wN2nUEAnT3dfAXwlZdNbZvYr4GIyBx3qSFqrlO2QWqPAQ2pNY2PjUnefWa7yzWw94BTgTHdPvrkagM7SMDeSeb2VklLQEVEKPKTWKPAQKalPgEOAxWZ2HjACOBv4c6aD3f3IMCqlycEirBZS65JdrQWeUW2nF4kbd18K7AFMIAhAniTo03FZJeulTEfEKeMhtUYZD8lFlDuTVoK7n5th2zRgl9Ark4UyHTGgjIfUGt1MRKqTMh0xoYxH7aqVUSzplPEQCY+Zjc31WHefXuh5FHSISGQp8BAJzasEc3V0NXRWM5LWCmU7pBYp8BAJxYgwTqI+HTFTi2l2qb1RLOnUx0MyUTBaUpsCs919VuL3zn5ybobJRJmOGFLGQ2qRMh4iZXUvMASYm/i9M2peqUUKPKQWKfAQKQ9375bp91JT0BFjCjykFinwEAmHmX2FoEmlG/A68FhiTZeCKeiIOQUetaNWh85mosBDpHzMbAjwD2A8MJNgRMuGwAwz+2oxK9Eq6KgCCjykFinwEIjezKSfL15Oy9xFWY9Zubx3SLUp2BXACmCEu38AYGbrArcQTKN+aKEFa/RKldA3YKlFUbrZiFSRrwMnJwMOAHefDZwK7FlMwQo6qogCj+qnjNbqFHiIlNxSglEq6YoauQIKOqqOAg+pRQo8RErqYeAyM1snuSHx+6XAQ8UUHHqfDjMbALwMnOPuN4R9/lqgPh5Si9THQ6Rkfgo8Dswys1mJbcOBl4DvFlNwJTqSTgLWq8B5a4oCj+qlUSydU+AhUjx3n2Nm4wj6dowFlgCvu/ujxZYdavOKmR0O9ANeCfO8tUo3JqlFamoRKYyZ9TOz75lZ38R8HPcndu0HHGtm2xR7jtCCDjMbAfwSOCqsc4oCD6lNCjxqi7JbxTOzUQQTgF0FDEpsvhS4CPgIaAEeM7PtizlPKEGHmdUDfwFOc/c5YZxTRGqbAg+RvJwP/AdYx93fMbOBwInAHe7+HXc/HpgInFvMScLKdPwCcHe/K6TzSQplO6qP+uvkRoGHSM6+Akx09yWJx7sTDI+9KeWYR4BtizlJWB1JDwbWNbP9E4/7AteY2Tbu/oOQ6lDT1LFUalUy8FAKXqqRmf0I+BEwEHDgVHd/KrFvC4LBG+OAd4Cj3H1qJ0X1J1hhNmk3YDkwJWXbQoqcpyOUoMPdN059bGbTgMs1ZDZcCjyklmlki1SbxBf5nwFfA2YAhwP3mtlGwGfAPcDlwATgAOBhM9vQ3RdkKG4mwUiV9xJdIvYEnnT3xSnH7EoQvBRMa6/UGAUeUssUeEhUtLa29jKz4Rl2tbh7S47FDAUudPfpicfXm9mlwOYE9/ce7n55Yt9tZvZD4CDgDxnKugG40szOJchyDCHo0wGAme0E/Ar4fY51y6giQYe7b1mJ80pAgUd10HwdhVHgIVHQ3Nw8gaDzZroOnTXNrAFYK8Nx7e5+deoGM5sANAKvAd8hGI2SagZBQJLJJYnzXAG0AWe4+92Jcq8kCEDuTBxXMGU6apQCD6llCjykXD5fvJiWDz/MesyK5f1pamp6Ejguw+70LMf2wOQMx60k5R5uZpsBtwNnu/tHZtYILE57zmKgT6Y6uftKgqaan2XYfR3wJ3eflum5+VDQUcMUeEgtU+AhldTY2LjU3Wd2dZy7TwHqsh1jZnsBNwO/dvffJDYvAnqnHdoHaM23ru5esgk9teBbjVN6Pt4UNBZHQ2ol7hKjV24FjnH3i1N2TQcs7fCNE9srRpkOUcZDapoyHhJXZnYgcCGwm7s/n7Z7MlBnZqcQzDJ6AMHQ2bvDrWVHCjoE+CLjoeBDapECj/ib07qsFjNXpwM9CaYnT91+sLvfa2Z7EMzTcR7BkNh93X1e6LVMoaBDOlDWQ2qVAg+JG3cf38X+V4EdQ6pOTtSnQ1ajfh7xoiCxdGrwm7JIqBR0SEYKPKRWDWlsUPAhUiYKOqRTCjyklinwECk9BR2SlQIPqWUKPERKS0GHdEmBR/SpX0f5KPAQKR0FHZITBR5SyxR4iJSGgg7JmQIPqWUKPESKp6BD8qLAQ2qZAg+R4mhyMMmbJhCLJi11Hw5NIibZLGudz4IP3sh6TNvy4cAaodQnapTpkILo5ia1TBkPkcIo6JCCKfCQWqbAQyR/CjqkKAo8pJYp8BDJj4IOKZoCD6llmjZdJHcKOqQkPlmyUsFHBKiDb+Uo8BDpmoIOKSkFHlLLFHhUjv7v40FBh5ScAg+pZbr5iXROQYeUhQIPqWUKPEQyU9AhZaPAozLUryMaFHiIrE4zkkpZafZSqWWavVTKzcx+BPwIGAg4cKq7P5XYdxRwLfB5ylNOdPcbQ69ogoIOKTsFHlLLFHhIuZjZ/sDPgK8BM4DDgXvNbCN3nweMBy5199MrWM0OFHRIKJJNLQo+pBYlm1oUfEhSa2trLzMbnmFXi7u35FjMUOBCd5+eeHy9mV0KbA48DjQBVxRd2RJS0CGhUtZDapmyHlWvdd2BjVkP6FHfjUH9+/DMM09uB5yf4ZCJwLnJB2bWAKyV4bh2d786dYOZTQAagdfMrB4YBxxmZpcBi4E/Ahe7e3vul1RaCjokdAo8pJYp8Ci9CHXavfrLm6532IYDuvHG/z7OeMA3dtmM7vV1LFy48AdkHsyRnuXYHpic4biVpNzDzWwz4HbgbHf/yMyGAC8CNwL7A5sA9wALgGvyu6zSqWtvr1jAk7Pm5ubhwLuNQ0fQrXuPSldHSkSBR3lp9FC0KfAonVyCjrYVy2n98F2AEU1NTTPLVZcrb7irffnylZx+zQOr7evRvRt/v/Aw7n7iVa674MS6Up3TzPYCbgZ+7e4XZznuNGAPd/9Kqc6dLw2ZlYrRTVFqWYS+nUsJbb/5htvtPH4kY4atvdq+b+44ll49u3P8ftsNKNX5EqNXbgWOSQ04zGxTM5uYdngDsLRU5y6Egg6pKAUeUsu0WFz1aWpqev7ZV2Zx1De37rC9R/duHL5nE7c+PI2mpqbPSnEuMzsQuBD4qrvfmba7BTjVzI41s25m1gScDFxfinMXSn06pOLUx0Nqnfp5FC6KQdv2m2+4HfDvMcPWXtW3oxxZDuB0oCfwmJmlbj/Y3e81s72B3wC/Az4Gznf3v5fw/HlTnw6JFAUfpaMsUvwo8MhfrkFHWH06klL7dpSrL0cchZrpiOLsaBItynpILVPGo3qkZjs2GzWkHFmOWAq7eSVys6NJ9CjwKI2BveuV7YghTSRWHZqamp6/8oa7OG6/bRm9/trc+vA0rrvgxJL05YizsIOOQmdHq4cgPSa1Yd7C5QzopX7OxWpb0VbpKkiBBveqY94iBR7ZDFqjIef7QspxoX2jSWY7FixaqixHQmh9OhKzoy0ApgBbkcfsaM3NzTsCT5W7jiIiUvV2ampqejqskzU3N58BvNvU1HRbWOeMsjAzHYMofHa0qcBOwIcEs7CJiIjko55grZKpYZ60qanpojDPF3UVHb0ShdnRREREJByhNZpHdXY0ERERCUeYzSvJ2dHeB/5E0K/jZOCHIdZBREREKiTU5hUz241gdrSNCWZHuyR9aV4RERGpTrGYkVRERETiTxMhiIiISCgUdIiIiEgoFHSIiIhIKBR0iIiISCjCXnslb2a2BTAJGAe8Axzl7qHOKFdKna20C9wKXAV8i2DW1cvcPRYz2ZnZNsC97j448biBLNdiZicBPwf6E8xKe7y7Lwq94jnIcG09gYVA6qIYz7r7/yX2HwhcSDDz4RPAEe4+N9xaZ2dmXwN+DYwG5hKMIrvWzAYQLE3wNaAVONvdr088pw44HziOYH6d64GfuvuKClxCp7Jc20jgLYLlF5Juc/djEs+LxWvSzPYieH2NILi+3ySuL/bvuSzXFvv3nHwh0kFH4o10D3A5MAE4AHjYzDZ09wUVrVzhMq60a2YXAQaMIvhgeNDMPnD3mypQx5wkbkRHA79N2zWRTq7FzHYHzgK+AswCbgCuBI4Kq965yHJtmwOfuvuQDM8ZSzAHzR4EU/5fDNwG7Fbe2ubOzIYBdwKHE7y3moCHzGwmcATBDWsoMCax/R13f4Ig2Nif4PX7OXA3cCZwXrhX0Lkurq0v8IK7b5fheXF5TQ4F/g7s5+4PmNl44Bkzmwp8mxi/57q4tm7E+D0nHUW9eWUXoIe7X+7uy939NuA14KDKVqsoTcC0DNsPB37l7vPdfSbBze74MCtWgInACcAFaduzXcvhwJ/d/TV3bwVOB75rZo0h1TlXnV1bZ38/gEOBf7n70+6+FDgD2MHMRpevmnkbDtzi7ne7e1siaziF4Ib0LeAX7r7Y3acBfyAINiD4u13u7u+7+zzgXKL3+hxO5mvbgex/t1i8Jt39Q2BQ4qbcDRgIrCDIAsT6PdfFtcX9PScpoh50jAVeT9s2g+DbZuwkVtodBxxmZrPN7C0zO93M1iT4djk95fA4XOckd28i+IYBQCJFn+1axqbte5vgdTimvFXN22rXljAeGGxmL5vZR2Z2h5mtl9jX4drcfTHwPyL0d3T3p9z9+8nHZrYWXyym2A68mXJ4tr/bDGDdxPMjIcu1/Zfg77aZmb2ReO/9MfFahfi8JnH3hWbWhyDb9DBwNTCPKnjPZbo2d3+TmL/npKOoBx2NdGyDJfG4TwXqUgqpK+2OIPhmeQJwUmJ/6rVG/jrdfXaGzclvT51dS4e/qbu3E6y/E6lr7eTaABYBzxBkBgxYQtDUADF7vZpZf+CfwPNAM7A08fdI6vTvlvJ7HK7tHmA+8AiwNcFNbAPgusThsXhNplgKrEFwLUcBP0psj/V7LqHDtZnZ0VTRe04i3qeD4MXWO21bH4JObrHj7nOAnVM2TTOzKwnaI6Hjtcb1OpOd0zq7lg5/00TfiV7E5Frd/Sepj83sJ8C8RH+C2LxezWwMwc14OnAIsAnQy8zqUgKPTv9ufPGhHvlrc/c24OCUQz4zszOBp82sOzF7TSauZxnwopldB3wpsSv277kM17aPu++dekxc33MSiHqmYzpBZJtqYzqmCmOji5V259DxWmN5ne4+n+zXkv43HQXU0TGtH1lmdp6ZbZKyqSHx71LSri2RKt6AiP0dzWwCQQbgH8C3Em3hbxL8HUakHJrt77Yx8KG7t5S/xrnLdG1m1sfMfmNm66Qc2kDQZ2AlMXlNmtnOZtactrknQRYn1u+5LNfWUg3vOflC1DMdk4E6MzuFYDjYAQR9Iu7O+qzoyrbS7mvAL83sZYKU4WnAFZWqaJFupvNruRn4k5n9nWAI9K+Bu6I2fC+LccCXzOy7icdXAPe5+zwzu4Xg2/MuwHPARcB/3f2NylR1dWY2CrgXOMvdr0xud/dWM7sbuCiR0h4FHEswogWCv9tpZvYYwbfLcxPbIiPLtS1ODKUdaGY/BAYQvO5ucPd2M4vLa3IasF7im/4VwLYEI6z2Iwg64vyey3ZtpxDj95x0FOlMh7svI2h6OAD4lGDY176J3vOx4+4fAHsT9CpfQDC873x3/ztwDvAqQfAxNbFvUoWqWqxOr8Xd7yeY7+Ee4AOCb1xRGwWRzdEE3yzfAmYSpIIPA3D3Vwja2CcRrKK8KcFQxig5kWD46EVm1pryczHB36GNYFjl/QSjIR5IPG8ScAfwLME35OkEf+coyXZt+wGDgdnAK8DLBDfm2Lwm3f0zYE+CocufEvRJOSYxpDnW77kuri3u7zlJoVVmRUREJBSRznSIiIhI9VDQISIiIqFQ0CEiIiKhUNAhIiIioVDQISIiIqFQ0CEiIiKhiPrkYCI1K7Ek+4Ypm5YAbwC/d/c/V6JOIiLFUKZDJNrOJFhBdF2CGWz/DFxlZqdVtFYiIgVQpkMk2hYmFgqEYPl5N7MVwG/N7CZ3n1vBuomI5EVBh0j83ABcAuxlZrcnft8XGESwBse17n6BmW1DsPjZaHd/C8DMegNzgb3dfXIlKi8itUvNKyIx4+6LgXcJ1pm4DPgyQdBhwO+B882syd1fIOgDkrqs+z7AZ8AToVZaRAQFHSJx1QL0A54Bjnb3F9z9HXe/BGgFxiaO+ysdg47vAre6e1uotRURQc0rInHVjyBj8ReCZpbDgDHAlgRLm9cnjvsLMNHMNiNYYXV3orc6rIjUCGU6RGIm0S/DgJcIRrNcQzCc9iaCppaW5LHu/g7BcvQHAQcAb7n7tLDrLCICynSIxNHhwArgSYJOpV9z98cBzGwI0B+oSzn+L8DxwHsEzS0iIhWhoEMk2vomAgkIgolvAhOBswmaSxYB+5vZuwRzeVxCEHD0TCnjduByguaXk0Oqt4jIatS8IhJtFxLMz/EhQTPJvsCR7v47d19O0DH0q8B0guaVh4H7gaZkAe7+KfAQ8B93nxlq7UVEUtS1t7dXug4iUmZmNhX4o7tfW+m6iEjtUvOKSBUzsz2A7YGNgFsrXB0RqXEKOkSq20nANsAx7r6g0pURkdqm5hUREREJhTqSioiISCgUdIiIiEgoFHSIiIhIKBR0iIiISCgUdIiIiEgo/j8EeZ6Au+EVxgAAAABJRU5ErkJggg==\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "fig = plt.figure(figsize=[9,4])\n",
+ "divnorm = mcolors.DivergingNorm(vmin=-25., vcenter=0., vmax=10)\n",
+ "plt.contourf(np.arange(ntime), depth, tsoil, np.linspace(-25,10,15), \n",
+ " norm = divnorm,\n",
+ " cmap=\"RdBu_r\", extend = 'both')\n",
+ "\n",
+ "plt.ylim([5,0])\n",
+ "cb = plt.colorbar()\n",
+ "plt.xlabel('Day')\n",
+ "plt.ylabel('Depth (m)')\n",
+ "cb.ax.set_ylabel('Soil Temperature ($^oC$)')\n",
+ "\n",
+ "plt.contour(np.arange(ntime), depth, tsoil, [0]) # ZERO "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.7.3"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/hydrotrend.ipynb b/lessons/pymt/hydrotrend.ipynb
new file mode 100644
index 0000000..2600f4f
--- /dev/null
+++ b/lessons/pymt/hydrotrend.ipynb
@@ -0,0 +1,407 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "# Run a single model in *pymt*\n",
+ "\n",
+ "In this example we will run a single model in *pymt*. We will use the water balance\n",
+ "model, *Hydrotrend* (written in C). We'll setup a model simulation, run it, and then\n",
+ "analyze the output. You will also be given a chance to do your own simulations\n",
+ "and explore the HydroTrend model. \n",
+ "\n",
+ "* [Explore the base-case river simulation](#Exercise-1)\n",
+ "* [How does a river system respond to climate change?](#Exercise-2)\n",
+ "\n",
+ "## HydroTrend\n",
+ "\n",
+ "HydroTrend is a 2D hydrological water balance and transport model that simulates water\n",
+ "discharge and sediment load at a river outlet. You can read more about the model, find\n",
+ "references or download the source code at: https://csdms.colorado.edu/wiki/Model:HydroTrend.\n",
+ "\n",
+ "### River Sediment Supply Modeling\n",
+ "\n",
+ "This notebook is meant to give you a better understanding of what the model is capable of. In this\n",
+ "example we are using a theoretical river basin of ~1990 km2, with 1200m of relief and\n",
+ "a river length of ~100 km. All parameters that are shown by default once the HydroTrend Model\n",
+ "is loaded are based on a present-day, temperate climate. Whereas these runs are not meant to\n",
+ "be specific, we are using parameters that are realistic for the [Waiapaoa River][map_of_waiapaoa]\n",
+ "in New Zealand. The Waiapaoa River is located on North Island and receives high rain and has\n",
+ "erodible soils, so the river sediment loads are exceptionally high. It has been called the\n",
+ "*\"dirtiest small river in the world\"*.\n",
+ "\n",
+ "To learn more about HydroTrend and its approach to sediment supply modeling, you can download\n",
+ "this [presentation][hydrotrend_presentation].\n",
+ "\n",
+ "A more detailed description of applying HydroTrend to the Waipaoa basin, New Zealand has been published in WRR: [hydrotrend_waipaoa_paper]. \n",
+ "\n",
+ "[map_of_waiapaoa]: https://www.google.com/maps/place/Waipaoa+River/@-38.5099042,177.7668002,71814m/data=!3m1!1e3!4m5!3m4!1s0x6d65def908624859:0x2a00ef6165e1dfa0!8m2!3d-38.5392405!4d177.8843782\n",
+ "[hydrotrend_presentation]: https://csdms.colorado.edu/wiki/File:SedimentSupplyModeling02_2013.ppt\n",
+ "[hydrotrend_waipaoa_paper]: http://dx.doi.org/10.1029/2006WR005570"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Exercise\n",
+ "To start, import numpy and matplotlib.\n"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import matplotlib.pyplot as plt\n",
+ "import numpy as np\n",
+ "from tqdm import tqdm"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "And load the HydroTrend model."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "hydrotrend = pymt.models.Hydrotrend()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "HydroTrend will now be activated in PyMT.\n"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Exercise 1\n",
+ "\n",
+ "### Explore the base-case river simulation\n",
+ "\n",
+ "For this case study, we will run a simulation for 100 years at daily time-step.\n",
+ "This means you run Hydrotrend for 36,500 days total. Later on we will change\n",
+ "other input parameters but, for now, we'll just stick with the defaults."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "hydrotrend = pymt.models.Hydrotrend()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, config_folder = hydrotrend.setup(run_duration=100)\n",
+ "hydrotrend.initialize(config_file, config_folder)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We can now see that, indeed, we have set up model simulation that will run for\n",
+ "100 years. Notice that HydroTrend has a time step of 1 day so we will be\n",
+ "generating daily hydrographs."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "print(\"Start time: {0} {1}\".format(hydrotrend.start_time, hydrotrend.time_units))\n",
+ "print(\"Current time: {0} {1}\".format(hydrotrend.time, hydrotrend.time_units))\n",
+ "print(\"End time: {0} {1}\".format(hydrotrend.end_time, hydrotrend.time_units))\n",
+ "print(\"Time step: {0} {1}\".format(hydrotrend.time_step, hydrotrend.time_units))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "If we look at HydroTrend's input and output variables, we notice that HydroTrend is\n",
+ "slightly unusual in that there are now input variables. There are lots of output\n",
+ "variables, though."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "hydrotrend.input_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "hydrotrend.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Of this list of output variables, we're going to track water and sediment discharge, sediment\n",
+ "concentration and bedload flux. The mapping of these variables to the Standard Names\n",
+ "reported by *output_var_names* is given in the following table.\n",
+ "\n",
+ "| Conventional Name | Standard Name |\n",
+ "| :--------------------- | :-------------------------------------------------------- |\n",
+ "| Water discharge | channel_exit_water__volume_flow_rate |\n",
+ "| Sediment discharge | channel_exit_water_sediment~suspended__mass_flow_rate |\n",
+ "| Sediment concentration | channel_exit_water_sediment~suspended__mass_concentration |\n",
+ "| Bedload flux | channel_exit_water_sediment~bedload__mass_flow_rate |\n",
+ "\n",
+ "What do you think? Are you able to figure out what the other output variables are?"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We use the ***update*** method to advance *hydrotrend* one time step at a time and the ***get_value***\n",
+ "method to retreive values from the model. Feel free to add other variables if you like."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "n_steps = int(hydrotrend.end_time / hydrotrend.time_step)\n",
+ "\n",
+ "q = np.empty(n_steps)\n",
+ "qs = np.empty(n_steps)\n",
+ "cs = np.empty(n_steps)\n",
+ "qb = np.empty(n_steps)\n",
+ "\n",
+ "for i in tqdm(range(n_steps)):\n",
+ " hydrotrend.update()\n",
+ " \n",
+ " q[i] = hydrotrend.get_value(\"channel_exit_water__volume_flow_rate\")\n",
+ " qs[i] = hydrotrend.get_value(\"channel_exit_water_sediment~suspended__mass_flow_rate\")\n",
+ " cs[i] = hydrotrend.get_value(\"channel_exit_water_sediment~suspended__mass_concentration\")\n",
+ " qb[i] = hydrotrend.get_value(\"channel_exit_water_sediment~bedload__mass_flow_rate\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Now plot the complete hydrograph."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "time = np.arange(len(qs))\n",
+ "plt.plot(time, qs)\n",
+ "plt.xlabel(\"Time ({0})\".format(hydrotrend.time_units))\n",
+ "plt.ylabel(\"Sediment discharge\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Calculate mean water discharge Q, mean suspended load Qs, mean sediment concentration Cs, and mean bedload Qb.\n",
+ "\n",
+ "*Note all values are reported as daily averages. What are the units?*"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "(\n",
+ " (q.mean(), hydrotrend.var_units(\"channel_exit_water__volume_flow_rate\")),\n",
+ " (cs.mean(), hydrotrend.var_units(\"channel_exit_water_sediment~suspended__mass_flow_rate\")),\n",
+ " (qs.mean(), hydrotrend.var_units(\"channel_exit_water_sediment~suspended__mass_concentration\")),\n",
+ " (qb.mean(), hydrotrend.var_units(\"channel_exit_water_sediment~bedload__mass_flow_rate\"))\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "hydrotrend.var_units(\"channel_exit_water__volume_flow_rate\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Identify the highest flood event for this simulation. Is this the 50-year flood? Plot the year of Q-data which includes the flood.\n"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "flood_day = q.argmax()\n",
+ "flood_year = flood_day // 365\n",
+ "plt.plot(q[flood_year * 365: (flood_year + 1) * 365])"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "q.max()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Calculate the mean annual sediment load for this river system."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "qs_by_year = qs.reshape((-1, 365))\n",
+ "qs_annual = qs_by_year.sum(axis=1)\n",
+ "plt.plot(qs_annual)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "qs_annual.mean()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### How does the sediment yield of this river system compare to the present-day Mississippi River?\n",
+ "\n",
+ "*To compare the mean annual load to other river systems you will need to calculate its sediment yield. \n",
+ "Sediment Yield is defined as sediment load normalized for the river drainage area; \n",
+ "so it can be reported in T/km2/yr.*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Exercise 2\n",
+ "\n",
+ "### How does a river system respond to climate change? A few simple scenarios for the coming century.\n",
+ "\n",
+ "#### What happens to river discharge, suspended load and bedload if the mean annual temperature in this specific river basin increases by 4 °C over the next 50 years?"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "from pymt.models import Hydrotrend\n",
+ "hydrotrend = Hydrotrend()\n",
+ "\n",
+ "config_file, config_folder = hydrotrend.setup(run_duration=100)\n",
+ "hydrotrend.initialize(config_file, config_folder)\n",
+ "\n",
+ "n_steps = int(hydrotrend.end_time / hydrotrend.time_step)\n",
+ "\n",
+ "q = np.empty(n_steps)\n",
+ "qs = np.empty(n_steps)\n",
+ "cs = np.empty(n_steps)\n",
+ "qb = np.empty(n_steps)\n",
+ "\n",
+ "for i in tqdm(range(n_steps)):\n",
+ " hydrotrend.update()\n",
+ " \n",
+ " q[i] = hydrotrend.get_value(\"channel_exit_water__volume_flow_rate\")\n",
+ " qs[i] = hydrotrend.get_value(\"channel_exit_water_sediment~suspended__mass_flow_rate\")\n",
+ " cs[i] = hydrotrend.get_value(\"channel_exit_water_sediment~suspended__mass_concentration\")\n",
+ " qb[i] = hydrotrend.get_value(\"channel_exit_water_sediment~bedload__mass_flow_rate\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### How much increase of discharge do you see after 50 years? How is the average suspended load affected? How does the bedload change? What happens to the peak event; look at the maximum discharge event of the last 10 years of the simulation?"
+ ]
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.2"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/index.ipynb b/lessons/pymt/index.ipynb
new file mode 100644
index 0000000..c818745
--- /dev/null
+++ b/lessons/pymt/index.ipynb
@@ -0,0 +1,63 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "# The Python Modeling Toolkit (*pymt*)\n",
+ "\n",
+ "The Python Modeling Toolkit (*pymt*) is an open source Python package that provides the tools needed to run and couple models that expose the Basic Model Interface (BMI).\n",
+ "We'll explore how *pymt* works through a set of Jupyter Notebooks.\n",
+ "\n",
+ "* [Introduction to *pymt*](intro.ipynb)\n",
+ " * Why use *pymt*?\n",
+ " * The *pymt* library of models\n",
+ " * Setting up a model simulation\n",
+ " * Initialize, Run, Finalize\n",
+ " * Getting data out of a model\n",
+ "\n",
+ "* [Run a standalone model in *pymt*](hydrotrend.ipynb)\n",
+ " * Introduction to Hydrotrend\n",
+ " * Setup + Run + analyze\n",
+ " * Adjust for a changing climate\n",
+ " * Adjust for human impact\n",
+ "\n",
+ "* [Couple two models](cem_and_waves.ipynb)\n",
+ " * Introduction to CEM + Waves\n",
+ " * Setup a simulation\n",
+ " * Getter and setters\n",
+ " * Run\n",
+ " * Grids\n",
+ " * Plot + analyze output\n",
+ "\n",
+ "Additional notebooks:\n",
+ " * [Flexural Subsidence Model](subside.ipynb) \n",
+ " * [ECSimpleSnow component](ecsimplesnow.ipynb)\n",
+ " * [Frost Number Model](frost_number.ipynb)\n",
+ " * [Kudryavtsev Model](ku.ipynb)\n",
+ " * [Sedflux3D Model](sedflux3d.ipynb)\n"
+ ]
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.3"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/intro.ipynb b/lessons/pymt/intro.ipynb
new file mode 100644
index 0000000..494fff7
--- /dev/null
+++ b/lessons/pymt/intro.ipynb
@@ -0,0 +1,641 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Why *pymt*?\n",
+ "\n",
+ "*pymt* provides a standard, easy-to-use interface to a wide range of models. *pymt* solves\n",
+ "several problems often encountered when a user wants to find, run, and/or couple\n",
+ "models to one another. Below I'll go through some of the problems a model user often\n",
+ "encounters when trying to find and try out a new model. This is certainly not an exhaustive\n",
+ "list.\n",
+ "\n",
+ "Can you think of other issues a user may encounter in trying to use and/or couple\n",
+ "a model?\n",
+ "\n",
+ "Problems:\n",
+ "* [Source code](#Getting-source-code)\n",
+ "* [Compiling the source code](#Compiling)\n",
+ "* [Documentation](#Documentation) (or lack thereof)\n",
+ "* [Running a model](#Running-a-model)\n",
+ "* [Debugging](#Debugging)\n",
+ "* [Model coupling](#Model-coupling)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Getting source code\n",
+ "\n",
+ "Even if a user doesn't *need* source code to run a model (i.e. they been given\n",
+ "a binary program they can simply run), it's still important for them to be\n",
+ "able to have access to it: they may want to modify it, or have a closer look\n",
+ "under-the-hood to see what's going on.\n",
+ "\n",
+ "#### Problem\n",
+ "\n",
+ "This is certainly less of a problem nowadays. However, it can still be an issue.\n",
+ "A would-be model user has heard of some mysterious model and would like to try\n",
+ "it out but can't find the source code. Instead, they are left trying to\n",
+ "find the email (phone? fax?) for the *master-of-the-code* and then trying to convince that person\n",
+ "to send a floppy disk of the source code to you. It's hard to believe, but this used to\n",
+ "be a thing.\n",
+ "\n",
+ "#### Solution\n",
+ "\n",
+ "All of the models within *pymt* are open source. For the most part, the source code for\n",
+ "*pymt* models are available in repositories on\n",
+ "[GitHub](https://github.com) but some may be housed on other publicly\n",
+ "accessable websites like [bitbucket](https://bitbucket.com), and\n",
+ "[SourceForge](https://sourceforge.com). We don't enforce the use of *GitHub* but\n",
+ "we do use it extensively for our code and it seems to be the most widely used\n",
+ "version control system in our community. In any case, the source code for all of the\n",
+ "models are freely available and not located behind a gate keeper. \n",
+ "\n",
+ "CSDMS maintains a database of model metadata on its website:\n",
+ "* https://csdms.colorado.edu\n",
+ "This is not the source code but descriptions of models that have been contributed\n",
+ "by the community to CSDMS. Here you can query model by, for example, type, process,\n",
+ "or name.\n",
+ "\n",
+ "The source code for many of the contributed models is on *GitHub* under the\n",
+ "*csdms-contrib* organization.\n",
+ "* https://github.com/csdms-contrib"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Compiling\n",
+ "\n",
+ "You have the FORTRAN source code, it looks great (maybe you've even modified it), but now you need to\n",
+ "be to run it.\n",
+ "\n",
+ "#### Problem\n",
+ "\n",
+ "Depending on the model, this step may not be much of a problem. However, oftentimes this\n",
+ "is the *biggest* problem a user encounters when trying to run a new model. At it worst,\n",
+ "this step is extremely painful and is often enough of a hurdle that this is where the\n",
+ "user stops.\n",
+ "\n",
+ "After getting the source code, there are still several issues to solve:\n",
+ "* Do I have the necessary compilers installed on my target platform?\n",
+ "* Was the code ever intended to be built on my target platform?\n",
+ "* Do I have all of the necessary dependencies installed? If not, this step is\n",
+ " increased in complexity by the number of dependencies needed to install.\n",
+ "\n",
+ "\n",
+ "#### Solution\n",
+ "\n",
+ "All of the models distributed in *pymt* come pre-compiled on a range of platforms. Although we\n",
+ "distribute *pymt* through *Anaconda*, which is primarily a Python package manager, models\n",
+ "written in C, C++, or FORTRAN are also available. Although we try to build all of the models\n",
+ "for Linux, Mac, and Windows, all of the models aren't yet available on all of those platforms.\n",
+ "We're trying though! Most all of them are available on Linux, and Mac.\n",
+ "\n",
+ "We use [conda-forge](https://github.com/conda-forge) to build and distribute models that\n",
+ "can then be installed using *Anaconda*. One nice side effect of this is that we also provide\n",
+ "a recipe describes how each piece of software is built so that you can do it yourself, if\n",
+ "you need to.\n",
+ "\n",
+ "And, as always, we accept pull requests! If you've built a model on a platform that we\n",
+ "haven't, we would love to hear from you."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Documentation\n",
+ "\n",
+ "Perhaps the number one thing that keeps a user from experimenting with a new model. Is a\n",
+ "lack of documentation. A user has found some source code but there is no documentation.\n",
+ "Unfortunately, we are seldom paid to write documentation. Instead, we are funded to write\n",
+ "some code to solve a specific problem and that's it. Encountered with a new mode, if a\n",
+ "user isn't told how to build, run, or modify a model, they'll most likely just move on.\n",
+ "\n",
+ "#### Problem\n",
+ "\n",
+ "A collection of source files without any documentation.\n",
+ "\n",
+ "#### Solution\n",
+ "\n",
+ "Both [*landlab*](https://landlab.readthedocs.io) and [*pymt*](https://pymt.readthedocs.io)\n",
+ "are well documented. And, as such, a user using a model from either of these\n",
+ "frameworks is able to tap into a either of these documentation bases for information\n",
+ "about how to get and run a model."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Running a model\n",
+ "\n",
+ "Getting, compiling, and installing a model isn't all that useful it the model can't be run.\n",
+ "\n",
+ "#### Problem\n",
+ "\n",
+ "Every model has it's own idosyncratic way of running. For example, model generally have\n",
+ "model-specific input/output files, or command line arguments (or a GUI?). Or, even worse,\n",
+ "there isn't an input file: instead, input parameters are changed in the source code and\n",
+ "the code recompiled.\n",
+ "\n",
+ "\n",
+ "#### Solution\n",
+ "\n",
+ "All of the models in *pymt* have a uniform interface (based on the [Basic Model Interface (BMI)](https://bmi.readthedocs.io)). This means that if you know how to use one *pymt* model, you know how\n",
+ "to use all *pymt* models."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Debugging\n",
+ "\n",
+ "It looks like there may be a problem with a model but you're not sure. Or, you are sure,\n",
+ "but you don't know where exactly the problem lies.\n",
+ "\n",
+ "#### Problem\n",
+ "\n",
+ "Trying to track down bugs can be a difficult process: particularly in codes written in compiled\n",
+ "languages like C or FORTRAN. Debugging oftentimes means inserting a bunch of print statements\n",
+ "into the code, recompiling, examining the output, and repeating.\n",
+ "\n",
+ "#### Solution\n",
+ "\n",
+ "Because *pymt* models are written in Python, a user can interactively run a model. A model can\n",
+ "be updated one time step at a time, it's state examined (perhaps using Python tools like numpy\n",
+ "or plotted using *matplotlib*), or even changed, and then updated for another time step. This\n",
+ "ability to debug a model by playing with it in Python has proven to be a valuable way to,\n",
+ "not only get a feel for how a model works, but also to see if it's working properly or as\n",
+ "expected."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Model coupling\n",
+ "\n",
+ "A user would like to couple two models (or a model to a dataset). \n",
+ "\n",
+ "#### Problem\n",
+ "\n",
+ "There are potentially many.\n",
+ "\n",
+ "Some examples:\n",
+ "* Models are written in different languages\n",
+ "* Models don't provide a way for another model to access it\n",
+ "\n",
+ "Can you think of others? Perhaps some that you've encountered? \n",
+ "\n",
+ "#### Solution\n",
+ "\n",
+ "The *pymt* brings models from different languages (currently C, C++, FORTRAN, and Python)\n",
+ "into a Python environment. Because of the BMI (*pymt* models all expose a BMI), we can\n",
+ "mostly automate this process. Through *pymt* users are able to write Python scripts that\n",
+ "run single models, or multiple models together.\n",
+ "\n",
+ "We'll show you how to do this today."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## The *pymt* model library\n",
+ "\n",
+ "All of the models that are available through *pymt* are held in a Python module that you can import, `pymt.models`.\n",
+ "\n",
+ "To have a look at what models are currently available, we'll import the library\n",
+ "and print the names of all of the models.\n",
+ "\n",
+ "For more information you can look at the [pymt documentation](https://pymt.readthedocs.io)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We'll now have a closer look at a model and see how a *pymt* model works. Rememeber, because\n",
+ "*pymt* models all have the same interface, and so if you know how to use one, you'll know\n",
+ "how to use all of them.\n",
+ "\n",
+ "Let's begin by picking a model from the above list. Pick one that sounds interesting to you."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "Model = pymt.models.Plume # <- type the name of the model you would like to use"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "`Model` is now a class of the model that you've chosen. You could create multiple instances\n",
+ "of a `Model` but until it's an instance, you can't do too much with it, so let's\n",
+ "create an instance."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model = Model()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We can now examine the model a little more. For instance, we can use the `help` function\n",
+ "to get some information about the model. This will give us a brief summary of the model,\n",
+ "the author, a version number, license, references, etc."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "help(model)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Scroll down a little in the help message and have a look at the *Parameters* section. These\n",
+ "are input parameters to the model. That is, things that are set at the *beginning* of\n",
+ "the model and cannot be changed thereafter. You can also get a view of them programmatically\n",
+ "using the *parameters* attribute."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "for name, value in model.parameters:\n",
+ " print(f\"{name} [default = {value}]\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## The lifecycle of a model\n",
+ "\n",
+ "Running a model in *pymt* involves four steps:\n",
+ "* [setup](#setup): prepare input files\n",
+ " * *setup*\n",
+ "* [initialize](#initialize): read input files\n",
+ " * *initialize*\n",
+ " * *input_var_names*\n",
+ " * *output_var_names*\n",
+ " * *var*\n",
+ " * *get_value*\n",
+ "* [update](#update): advance one time step\n",
+ " * *update*\n",
+ " * *start_time*\n",
+ " * *time*\n",
+ " * *end_time*\n",
+ "* [finalize](#finalize): shutdown\n",
+ " * *finalize*\n",
+ "\n",
+ "Below we'll briefly go through each of these steps."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Setup\n",
+ "\n",
+ "Before a model can be run, it's input files must be prepared. If you haven't done this manually\n",
+ "(which you are definitely free to do), you can use the model's *setup* method to help with\n",
+ "this.\n",
+ "\n",
+ "By default, *setup* will create a temporary folder with files containing default values. However,\n",
+ "for this example we'll specify a folder so that we can see what's going on. The following will create\n",
+ "a new folder, *_my_model* (you can call it whatever you like), and, in that folder, will\n",
+ "be model-specific input files. *setup* return a tuple that gives the name of the main configuration\n",
+ "file and the full path name of the folder. "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, config_folder = model.setup(\"_my_model\")\n",
+ "print(f\"Input files are located here: {config_folder}\")\n",
+ "print(f\"The main configuration file is: {config_file}\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "To double-check that something was actually done, you can use shell commands (hint: `ls`, and `cat`)\n",
+ "to see what files were created and what their contents are. If you don't like the shell, you can\n",
+ "always use the Jupyter tree-view.\n",
+ "\n",
+ "The set of files that you just created depend completely on the model you chose. All of these files\n",
+ "a model-specific. However, note that we all used the exact same command to create them - regardless\n",
+ "of our chosen model.\n",
+ "\n",
+ "Now, let's now change an input parameter. This is done through passing keywords to *setup*. The\n",
+ "keywords that you can use are specific to a model and can be found through *help*. "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.setup(\"_fast_river\", river_mouth_velocity=2.0)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "You'll now see a new set up input files. If you look closely, you should be able to see your\n",
+ "change.\n",
+ "\n",
+ "A couple of notes about the *setup* method.\n",
+ "\n",
+ "***Note***: It's not strictly required to run *setup* before *initialize* - it's just a\n",
+ "conveient way to get a set of input files. One pattern that is sometimes used is to use\n",
+ "*setup* to get a base set of input files and then edit some of the files by hand.\n",
+ "\n",
+ "***Note***: It's not strictly required that you run *initialize* at all - sometimes\n",
+ "*setup* is the goal. For example, *setup* provides an easy way to programmatically\n",
+ "create a large number of input files for, say, a Monte Carlo simulation.\n",
+ "\n",
+ "```python\n",
+ ">>> from itertools import product\n",
+ ">>> velocity_samples = [0.5, 1.0, 1.5, 2.0, 2.5]\n",
+ ">>> width_samples = [100.0, 200.0, 300.0, 400.0, 500.0]\n",
+ ">>> for n, (velocity, width) in enumerate(product(velocity_samples, width_samples)):\n",
+ "... model.setup(f\"_sim-{n}\", river_mouth_width=width, river_mouth_velocity=velocity)\n",
+ "```"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "from itertools import product\n",
+ "\n",
+ "velocity_samples = [0.5, 1.0, 1.5, 2.0, 2.5]\n",
+ "width_samples = [100.0, 200.0, 300.0, 400.0, 500.0]\n",
+ "\n",
+ "for n, (velocity, width) in enumerate(product(velocity_samples, width_samples)):\n",
+ " model.setup(f\"_sim-{n}\", river_mouth_width=width, river_mouth_velocity=velocity)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Initialize\n",
+ "\n",
+ "Now that we have a set of input files, we're ready to get the model ready for time stepping. This is\n",
+ "done through the *initialize* method. The model is not in a state that we can query it, until\n",
+ "*initialize* has been run. This is important to understand.\n",
+ "\n",
+ "To better understand this, consider the common pattern for a model to read from one of its input\n",
+ "files the size or resolution of its solution grid. Thus, in such a situation, we cannot ask\n",
+ "about the model's grid until its read input files, which is done in *initialize*.\n",
+ "\n",
+ "To run *initialize*, we must pass the name of a configuration file and a folder - both of\n",
+ "which we got from *setup*."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.initialize(config_file, config_folder)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Now we can ask some questions about the model:\n",
+ "* what variables do you provide as output?\n",
+ "* what variables to you use as input?\n",
+ "* what is the grid like on which these variables sit (if there even is a grid)?"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Input and output variables\n",
+ "\n",
+ "Input and output variables are different from the parameters we talked about above (the ones described\n",
+ "in the *help* message, or the *model.parameters* attribute). Input and output variables are\n",
+ "able to ***dynamically change with time***.\n",
+ "\n",
+ "To get a list of the available input and output variables, you can use the *input_var_names* and\n",
+ "*output_var_names* attributes. Depending on the model you chose, you may have not have any\n",
+ "input variables. This means your model is configured once at the start but then can't be changed.\n",
+ "It could be part of a 1-way coupling but not a 1-way coupling with feedback. A *dataset* would\n",
+ "also be an example of a model without input variables."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "print(\"Input variables:\")\n",
+ "for name in model.input_var_names:\n",
+ " print(f\"- {name}\")\n",
+ "\n",
+ "print(\"Output variables:\")\n",
+ "for name in model.output_var_names:\n",
+ " print(f\"- {name}\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "These are just the names of the variables. We can get additional information as well. This can\n",
+ "be obtained several ways but the preferred method is using the `var` attribute. `var` is\n",
+ "a dictionary of variables names mapped to variable descriptions.\n",
+ "\n",
+ "Pick a variable from the above list to find out more about it. We see attributes of the variable\n",
+ "such as its data type and units. This also gives us information about the grid that the\n",
+ "variable is defined on (i.e. the *grid* and *location* attributes). We'll get to grids later on."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "variable = model.var[\"sea_bottom_sediment__deposition_rate\"] # <- replace this string with a variable for your model"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "variable"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "You can get it values either with the `data` attribute or with the `get_values` method."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "variable.data"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.get_value(\"sea_bottom_sediment__deposition_rate\")"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Run\n",
+ "\n",
+ "Our model is now initialized and ready to be advanced through time. The *update* method advances\n",
+ "the model's state by a single time step."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.update()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "That's it. There not too much to it. You can see that it's done something either by seeing if\n",
+ "an output variable has changed or using the *time* attribute to see the current model time -\n",
+ "if there is one."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "print(\"Start time: {0} {1}\".format(model.start_time, model.time_units))\n",
+ "print(\"Current time: {0} {1}\".format(model.time, model.time_units))\n",
+ "print(\"End time: {0} {1}\".format(model.end_time, model.time_units))"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Finalize\n",
+ "\n",
+ "There's not much to this method, and often we don't even use it. This is where a model will free memory\n",
+ "or close files. If you're model uses lots of memory and you notice you're running out, it may\n",
+ "help to call this method. Calling *finalize* will put your model in a state where it is no\n",
+ "longer usable."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.finalize()"
+ ]
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.2"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/ku.ipynb b/lessons/pymt/ku.ipynb
new file mode 100644
index 0000000..21d3c4a
--- /dev/null
+++ b/lessons/pymt/ku.ipynb
@@ -0,0 +1,363 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Kudryavtsev Model\n",
+ "\n",
+ "\n",
+ "### Introduction to Permafrost Processes - Lesson 2 Kudryavtsev Model\n",
+ "\n",
+ "This lab has been designed and developed by Irina Overeem and Mark Piper, CSDMS, University of Colorado, CO \n",
+ "with assistance of Kang Wang, Scott Stewart at CSDMS, University of Colorado, CO, and Elchin Jafarov, at Los Alamos National Labs, NM. \n",
+ "These labs are developed with support from NSF Grant 1503559, ‘Towards a Tiered Permafrost Modeling Cyberinfrastructure’ \n",
+ "\n",
+ "### Classroom organization\n",
+ "\n",
+ "This lab is the second in a series of introduction to permafrost process modeling, designed for inexperienced users. In this first lesson, we explore the Air Frost Number model and learn to use the CSDMS Python Modeling Toolkit ([PyMT](https://github.com/csdms/pymt)). We implemented a basic configuration of the Air Frost Number (as formulated by Nelson and Outcalt in 1987). This series of labs is designed for inexperienced modelers to gain some experience with running a numerical model, changing model inputs, and analyzing model output. Specifically, this first lab looks at what controls permafrost occurrence and compares the occurrence of permafrost in Russia. \n",
+ "Basic theory on the Air Frost Number is presented in [Frost Number Model Lecture 1](https://csdms.colorado.edu/wiki/File:FrostNumberModel_Lecture1.pptx).\n",
+ "\n",
+ "This lab is the second in a series of introduction to permafrost process modeling, designed for inexperienced users. In this second lesson, we explore the Kudryavstev model and learn to use the CSDMS Python Modeling Toolkit ([PyMT](https://github.com/csdms/pymt)). We implemented the Kudryavstev model (as formulated in Anisimov et al.1997). It is dubbed the Ku-model. This series of labs is designed for inexperienced modelers to gain some experience with running a numerical model, changing model inputs, and analyzing model output. Specifically, this lab looks at what controls soil temperature and active layer thickness and compares model output with observed longterm data collected at permafrost active layer thickness monitoring sites in Fairbanks and Barrow, Alaska. \n",
+ "Basic theory on the Kudryavstev model is presented in [Kudryavtsev Model Lecture 2](https://csdms.colorado.edu/wiki/File:KudryavtsevModel_Lecture2.pptx)\n",
+ "\n",
+ "This lab will likely take ~ 1,5 hours to complete in the classroom. This time assumes you are unfamiiar with the PyMT and need to learn setting parameters, saving runs, downloading data and looking at output (otherwise it will be much faster).\n",
+ "\n",
+ "We will use netcdf files for output, this is a standard output from all CSDMS models. If you have no experience with visualizing these files, Panoply software will be helpful. Find instructions on how to use this software.\n",
+ "\n",
+ "### Learning objectives\n",
+ "\n",
+ "#### Skills\n",
+ "\n",
+ "* familiarize with a basic configuration of the Kudryavstev Model for 1D (a single location).\n",
+ "* hands-on experience with visualizing NetCDF time series with Panoply.\n",
+ "* data to model comparisons and how to think about uncertainty in data and model output.\n",
+ "\n",
+ "#### Topical learning objectives:\n",
+ "\n",
+ "* what are controls on permafrost soil temperature\n",
+ "* what is a steady-state model\n",
+ "* what are important parameters for calculating active layer thickness\n",
+ "* active layer thickness evolution with climate warming in two locations in Alaska\n",
+ "\n",
+ "### References and More information \n",
+ "\n",
+ "Anisimov, O. A., Shiklomanov, N. I., & Nelson, F. E. (1997). *Global warming and active-layer thickness: results from transient general circulation models.* Global and Planetary Change, 15(3-4), 61-77. DOI:10.1016/S0921-8181(97)00009-X \n",
+ "Sazonova, T.S., Romanovsky, V.E., 2003. *A model for regional-scale estimation of temporal and spatial variability of active layer thickness and mean nnaual ground emperatures.* Permafrost and periglacial processes 14, 125-139. DOI: 10.1002/ppp.449 \n",
+ "Zhang, T., 2005. *Influence of the seasonal snow cover on the ground thermal regime: an overview.* Review of Geophysics, 43, RG4002.\n"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### The Kudryavtsev Model\n",
+ "\n",
+ "The Kudryavtsev et al. (1974), or *Ku* model, presents an\n",
+ "approximate solution of the Stefan problem. The model provides a\n",
+ "steady-state solution under the assumption of sinusoidal air\n",
+ "temperature forcing. It considers snow, vegetation, and soil layers\n",
+ "as thermal damping to variation of air temperature. The layer of\n",
+ "soil is considered to be a homogeneous column with different thermal\n",
+ "properties in the frozen and thawed states. The main outputs are\n",
+ "annual maximum frozen/thaw depth and mean annual temperature at the\n",
+ "top of permafrost (or at the base of the active layer). It can be\n",
+ "applied over a wide variety of climatic conditions."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import numpy as np\n",
+ "import matplotlib.pyplot as plt"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "ku = pymt.models.Ku()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Part 1\n",
+ "\n",
+ "We will run the Kudryatsev model for conditions in Barrow, Alaska in a very cold year, 1964. The mean annaul temperature for 1964 was -15.21C, the amplitude over that year was 18.51C. It was close to normal snow year, meaning the average snow thickness over this winter was 0.22m.\n",
+ "\n",
+ "Adapt the settings in the Ku model for Barrow 1964. Make sure you request an output file. Save the simulation settings and submit your simulation. Download the model results and open them in Panoply."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, run_folder = ku.setup(T_air=-15.21, A_air=18.51)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ku.initialize(config_file, run_folder)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ku.update()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ku.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ku.get_value('soil__active_layer_thickness')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q1.1: What was the active layer thickness the model predicted? \n",
+ "\n",
+ "*Sketch a soil profile for winter conditions versus August conditions, indicate where the frozen-unfrozen boundary is in each two cases.*"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q1.2: How do you think snow affects the active layer thickness predictions? "
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Part 2\n",
+ "\n",
+ "Run the Kudryatsev model with a range of snow conditions (0 m as the one extreme, and in extremely snowy years, the mean snow thickness over the winter is 0.4m in Barrow). Set these two simulations, run them and dowload the files."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "args = ku.setup(h_snow=0.)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ku.initialize(*args)\n",
+ "ku.update()\n",
+ "ku.get_value('soil__active_layer_thickness')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "args = ku.setup(h_snow=0.4)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ku.initialize(*args)\n",
+ "ku.update()\n",
+ "ku.get_value('soil__active_layer_thickness')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q2.1: What happens if there is no snow at all (0 m)? "
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q2.2: What is the active layer thickness prediction for a very snowy year?"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Part 3\n",
+ "\n",
+ "Run the Kudryatsev model with a range of soil water contents. What happens if there is 20% more, and 20% less soil water content? "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "args = ku.setup(vwc_H2O=0.2)\n",
+ "ku.initialize(*args)\n",
+ "ku.update()\n",
+ "ku.get_value('soil__active_layer_thickness')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "args = ku.setup(vwc_H2O=0.6)\n",
+ "ku.initialize(*args)\n",
+ "ku.update()\n",
+ "ku.get_value('soil__active_layer_thickness')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q3.1: Is this selected range of 20% realistic for soils in permafrost regions?"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "#### Q3.2: From the theory presented in the associated lecture notes, how do you think soil water content in summer affects the soil temperature?"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Part 4\n",
+ "\n",
+ "Posted here are time-series for climate conditions for both Barrow and Fairbanks, Alaska. Time-series are annual values and run from 1961-2015, the data include mean annual temperature (MAAT), temperature amplitude (TAMP) and winter-average snow depth (SD). \n",
+ "\n",
+ "These are text files, so you can plot them in your own favorite software or programming language.\n",
+ "\n",
+ "Choose which case you want to run, you will now run a 55 year simulation. "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pandas\n",
+ "data = pandas.read_csv(\"https://raw.githubusercontent.com/mcflugen/pymt_ku/master/data/Barrow_1961-2015.csv\")\n",
+ "data"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "maat = data[\"atmosphere_bottom_air__temperature\"]\n",
+ "tamp = data[\"atmosphere_bottom_air__temperature_amplitude\"]\n",
+ "snow_depth = data[\"snowpack__depth\"]"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ku = pymt.models.Ku()\n",
+ "args = ku.setup(end_year=2050)\n",
+ "ku.initialize(*args)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "n_steps = int((ku.end_time - ku.time) / ku.time_step)\n",
+ "thickness = np.empty(n_steps)\n",
+ "for i in range(n_steps):\n",
+ " ku.set_value(\"atmosphere_bottom_air__temperature\", maat.values[i])\n",
+ " ku.set_value(\"atmosphere_bottom_air__temperature_amplitude\", tamp.values[i])\n",
+ " ku.set_value(\"snowpack__depth\", snow_depth.values[i])\n",
+ " ku.update()\n",
+ " thickness[i] = ku.get_value('soil__active_layer_thickness')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "plt.plot(thickness) # This should be the same as the above but it's NOT! But now it is. BOOM!"
+ ]
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.2"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/sedflux3d.ipynb b/lessons/pymt/sedflux3d.ipynb
new file mode 100644
index 0000000..cec19d4
--- /dev/null
+++ b/lessons/pymt/sedflux3d.ipynb
@@ -0,0 +1,328 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "
"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Sedflux3D\n",
+ "* Link to this notebook: https://github.com/csdms/pymt/blob/master/notebooks/sedflux3d.ipynb\n",
+ "* Install command: `$ conda install notebook pymt_sedflux`\n",
+ "\n"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Import the `Sedflux3D` component from `pymt`. All of the components available to `pymt` are located in `pymt.components`. Here I've renamed the component to be `Model` to show that you could run these same commands with other models as well. For instance, you could instead import `Child` with `from pymt.components import Child as Model` and repeat this exercise with Child instead."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "# Some magic to make plots appear within the notebook\n",
+ "%matplotlib inline\n",
+ "\n",
+ "import numpy as np # In case we need to use numpy\n",
+ "from tqdm import tqdm"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Instantiate the model. If you wanted, you could have multiple instances of the same model - each with their own state. A PyMT component is instantiate without any arguments."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model = pymt.models.Sedflux3D()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "PyMT adds a docstring to the model. It is important to us that credit it given where credit is due. We want to make it clear that when we include a component in our framework the component is not ours but belong to the model's author. To that end, the model documentation includes a list of model authors, DOIs, as well as full citations to use if someone uses the model. In addition, to keep models from being black boxes, and because they are all open source, we provide links to source code. "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "# help(model)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "rm -rf _model # Clean up for the next step"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The following code is generic for all `pymt` components - not just `Sedflux3D`. First we instantiate the component and then call its `setup` method to create a model simulation with the necessary input files (note that this is ***not*** a BMI method but something added by PyMT). `setup` takes an optional argument that gives a path to a folder that will contain the necessary input files (if not provided, a temporary folder will be used). `setup` returns the name of the config file and the path to the folder containing it (we'll use this information later when we run `initialize`)."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, initdir = model.setup('_model', run_duration=3650000.)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "If we look inside the `_model` folder, we can see all of the necessary sedflux input files ready to run."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "ls _model"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The `initialize` method reads all of the input files and gets the model ready to update through time."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.initialize(config_file, dir=initdir)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.set_value(\"channel_exit_water_flow__speed\", 1.2)\n",
+ "model.set_value(\"channel_exit_x-section__mean_of_width\", 400.)\n",
+ "model.set_value(\"channel_exit_x-section__mean_of_depth\", 4.)\n",
+ "model.set_value(\"channel_exit_water_sediment~suspended__mass_concentration\", .01)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Here we update the model for 10 time steps, printing the model time after each step."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "for t in tqdm(range(10)):\n",
+ " model.update()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "From the output it appears that sedflux is operating on a 1 year time step and is using units of *days*. We can double check that assumption."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {
+ "scrolled": true
+ },
+ "outputs": [],
+ "source": [
+ "model.time_units"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Yep, *days*. And the time step..."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.time_step"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The `update_until` method gives us more control by allowing us to update to a particular time. Using the `units` keyword allows us to specify the \"until\" time. Here we update the model until 20 years."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.update_until(200., units='year')\n",
+ "model.time"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.time_units = 'year'\n",
+ "print(model.time)"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We've covered how to setup and run a model, now we'll have a look at how to get data out of the model. This is done with the `get_value` method but first we need to look at what variables the model provides. This is held as a tuple of variable names and accessed through the `output_var_names` attribute."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.input_var_names"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "So to get the values of, say, water depth,"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.get_value('sea_water__depth')"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "As with previous methods, you can use the `units` keyword to specify units. Here we get water depths in units of parsecs."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.get_value('sea_water__depth', units='parsec')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.quick_plot('sea_water__depth', vmin=-200, vmax=200, cmap='BrBG_r')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "model.set_value(\"channel_exit_water_sediment~suspended__mass_concentration\", .5)\n",
+ "for t in tqdm(range(20)):\n",
+ " model.update()\n",
+ "model.quick_plot('sea_water__depth', vmin=-200, vmax=200, cmap='BrBG_r')"
+ ]
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.2"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/sedflux3d_and_child.ipynb b/lessons/pymt/sedflux3d_and_child.ipynb
new file mode 100644
index 0000000..b57cf32
--- /dev/null
+++ b/lessons/pymt/sedflux3d_and_child.ipynb
@@ -0,0 +1,278 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Sedflux3D + CHILD\n",
+ "* Link to this notebook: https://github.com/csdms/pymt/blob/master/docs/demos/sedflux3d_and_child.ipynb\n",
+ "* Install command: `$ conda install notebook pymt_sedflux pymt_child`\n",
+ "\n"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 1,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "# Some magic to make plots appear within the notebook\n",
+ "%matplotlib inline\n",
+ "import numpy as np # In case we need to use numpy\n",
+ "import pymt.models"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 2,
+ "metadata": {},
+ "outputs": [
+ {
+ "name": "stderr",
+ "output_type": "stream",
+ "text": [
+ "/Users/huttone/anaconda/envs/child_sedflux/lib/python3.6/site-packages/pymt/utils/decorators.py:60: UserWarning: Call to deprecated function get_grid_size.\n",
+ " name=func.__name__\n"
+ ]
+ }
+ ],
+ "source": [
+ "child = pymt.models.Child()\n",
+ "sedflux = pymt.models.Sedflux3D()\n",
+ "\n",
+ "child_in, child_dir = child.setup(\n",
+ " \"_child\",\n",
+ " grid_node_spacing=500.0,\n",
+ " grid_x_size=40000.0,\n",
+ " grid_y_size=20000.0,\n",
+ " run_duration=1e6,\n",
+ ")\n",
+ "sedflux_in, sedflux_dir = sedflux.setup(\n",
+ " \"_sedflux\",\n",
+ " river_bed_load_flux=0.0,\n",
+ " river_suspended_load_concentration_0=0.1,\n",
+ " river_suspended_load_concentration_1=0.1,\n",
+ " run_duration=1e6 * 365.0,\n",
+ ")\n",
+ "\n",
+ "child.initialize(child_in, dir=child_dir)\n",
+ "sedflux.initialize(sedflux_in, dir=sedflux_dir)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 3,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "gid = child.var[\"land_surface__elevation\"].grid\n",
+ "x, y = child.get_grid_x(gid), child.get_grid_y(gid)\n",
+ "z = child.get_value(\"land_surface__elevation\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 4,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "array([-100. , -100. , -100. , ..., 99.99964955,\n",
+ " 99.56988064, 100.34489894])"
+ ]
+ },
+ "execution_count": 4,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "x_shore = 20000.0\n",
+ "z[np.where(x > x_shore)] += 100.0\n",
+ "z[np.where(x <= x_shore)] -= 100.0\n",
+ "\n",
+ "child.set_value(\"land_surface__elevation\", z)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 5,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "sedflux.set_value(\n",
+ " \"bedrock_surface__elevation\", mapfrom=(\"land_surface__elevation\", child)\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 6,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAZsAAADxCAYAAAAdgBpwAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4xLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvDW2N/gAAIABJREFUeJzs3XeUXcd94PlvvZzz65xzRKMRGpkACEYxKlAjy0kej+31yjues5M8490zYdd77HXYHa2DRrZFmbZEUYEiKQaJBJEz0EDnnHN4oV/Or/aPbtLwmCJBEk2a0v2cU+fdrlc39R/1exXuLSGlRKFQKBSK7aT6uC9AoVAoFD/9lGCjUCgUim2nBBuFQqFQbDsl2CgUCoVi2ynBRqFQKBTbTgk2CoVCodh2SrBRKBSKTwAhRLkQ4rQQYlgIMSiE+O2tfJcQ4g0hxPjWp3MrXwghviKEmBBC9Akhdn2c168EG4VCofhkyAL/WkrZDOwHviyEaAF+B3hTSlkPvLn1N8DDQP1W+nXgLz76S/57SrBRKBSKTwAp5bKU8ubWdgQYBkqBJ4C/2Sr2N8CTW9tPAM/ITVcAhxCi+CO+7LdpPq4TKxQKxU+7IptKprN3VjaYkINA8rasr0kpv/ZOZYUQVUAncBUolFIuw2ZAEkIUbBUrBeZv221hK2/5zu/g7lGCjUKhUGyTdBZONN5ZNfu9nkxSSrnnvcoJISzA94F/JaUMCyF+YtF3yPvY3k+mdKMpFArFNlKJO0t3QgihZTPQfFNK+fxW9upb3WNbn2tb+QtA+W27lwFLd+OePggl2CgUCsU2EQK0anFH6b2PJQTw18CwlPJPbvvqJeCXt7Z/GXjxtvxf2pqVth8IvdXd9nFQutEUCoViG91pq+UOHAJ+EegXQvRs5f1H4PeB7wghfhWYA57a+u5V4FPABBAHfuWuXckHoAQbhUKh2CaCuxdspJQXeOdxGIAT71BeAl++O2f/8JRgo1AoFNtFgEoZrACUYKNQKBTb5m62bD7plGCjUCgU20j9k6cm/0xRgo1CoVBsEyFAo/64r+KfBiXYKBQKxTZSutE2KcFGoVAotolAmSDwFiXYKBQKxXYRypjNW5Rgo1AoFNtEmY3295Rgo1AoFNtEoEwQeIsSbBQKhWK7vI+XbP60U4KNQqFQbBOBMmbzFiXYKBQKxTZSZqNtUoKNQqFQbCOlG22TEmwUCoVimwhlzOZtSrBRKBSKbSK4s4XRfhYowUahUCi2kdKy2aQEG4VCodgmSjfa31OCjUKhUGwjlRJtAFAm5SkUCsV2EZvB5k7Sex5KiK8LIdaEEAO35f1nIcSiEKJnK33qtu/+gxBiQggxKoR4cJvu8I4pLRuFQqHYJgKB5u69r+YbwJ8Cz/wP+f+PlPKP/sF5hWgBvgC0AiXASSFEg5Qyd7cu5v1SWjYKhUKxTTbHbMQdpfcipTwHBO7w1E8A35ZSpqSU08AE0PXB7+TDU4KNQqFQbCOVSnVH6UP4LSFE31Y3m3MrrxSYv63Mwlbex0YJNgqFQrGN3seYjUcIceO29Ot3cPi/AGqBncAy8Mdb+e/UVJJ35YY+IGXMRqFQKLaJEHc2+L/FJ6Xc836OL6Vcve1cfwm8vPXnAlB+W9EyYOn9HPtuU1o2CoVCsY22sxtNCFF825+fBt6aqfYS8AUhhF4IUQ3UA9c+1I18SErLRqFQKLaJEKDR3J3f9EKIZ4FjbHa3LQD/CTgmhNjJZhfZDPAbAFLKQSHEd4AhIAt8+eOciQZKsFEoFIptIxAfdvD/bVLKn3uH7L9+l/K/B/zeXTn5XaAEG4VCodguQnmDwFuUYKNQKBTbSCWUoXFQgo1CoVBsm81uNKVlA0qwUSgUiu2jdKO9TQk2CoVCsU02Z6PdtXejfaIpwUahUCi2jdKN9hYl2CgUCsU2EXDXpj5/0inBRqFQKLaLMmbzNiXYKBQKxTYRiJ+Kqc9CiDI218c5wub6OAk2X43zCvCalDL/Xsf4mQs2Ho9HVlVVfdyXoVAoPgG6u7t9UkrvBz7AXXxdzcdFCPE0m8sTvAz8AbAGGIAG4CHgd4UQv7O13s5P9DMXbKqqqrhx48bHfRkKheITQAgx+6H256dizOaPpZQD75A/ADwvhNABFe91kJ+5YKNQKBQfmfe3xMA/ST8h0Nz+fZrNlUDf1baFXCFEuRDitBBiWAgxKIT47a18lxDiDSHE+NancytfCCG+IoSY2Fp1btdtx/rlrfLjQohfvi1/txCif2ufrwhxB2urKhQKxUdIqNV3lP6pE0I8KoS4JYQICCHCQoiIECJ8p/tvZ/suC/xrKWUzsB/4shCiBfgd4E0pZT3w5tbfAA+zueZCPfDrbK5AhxDCxeartPexuYb2f7pt6dO/2Cr71n4PbeP9KBQKxfuyuXia+o7SJ8D/C/wy4JZS2qSUViml7U533rZuNCnlMpvLlCKljAghhtkcZHqCzTUZAP4GOAP8+638Z6SUErgihHBsLQx0DHhDShkAEEK8ATwkhDgD2KSUl7fynwGeBF67W/fQ1dXF9evX79bhFIqfGrd3Ivyk7dvHKtS3/XLXaP6+2tFqtW9v63S6t7cNBsPbxzKZTG8fy2q1vr3tcrnePm5RUdHb29XV1W9vt7e3v719+PDhf3Duj8onodVyh+aBga06+n37SP7zQogqoBO4ChRuBSKklMtCiIKtYqVs3sxbFrby3i1/4R3y3+n8v85mC4iKivccx3rb6vr6HZdVKH6W/IP6RkoQmx8qweZ2HqTMoRKCfF6Sz2dRqwS5nCSXSaPRCLJ5STYt0GpUZDJ50hoVeq2aVCZHIqrCbNSSTGeJhlTYTHpiqQy+FRUFLiNSwuJ0mooiO4lUhtG+LK01XhDQc+kVulpLyEvJD7/95zxyuA6TUcvaTC+f/9Jvf7T/KKFCpdV/tOfcPv8OeFUIcRZIvZUppfyTO9l524ONEMICfB/4V1LK8LsMq7zTF/ID5P/jTCm/BnwNYM+ePXcclf/bX/w5fzVwE00qQ3BlBYPdhrWqkszUDDm7BaPBgG96FmtNJZp4knQwRCaTxnPsEOGL18lkMriPH2Lj3BW09TWwto5pRwurF65iMhgxdbYTGRgms+7HvnsnMy+/Ru1nHmXj6i2MLfWogmESK2sYOlrJzS6QMRnQaDWIwAa2rk7CtwZIrvpwnThCcnCE2Ooa1r0dRAbHsBQWkMnn0JmtRAZHcBzeR25ojEQyjrW1mdTYFPFwGOexQyT7h8lks1jLiglPTqMyGrGUlxIencB1z358b5wlrxKYbTas+3YRvd5LMhzG1NFCfnmNhM+Pc99ugle70VdXogpukE8kydusGC1mEgvLZLVaLJVlBAaGMBQVYTCZCczN4tq5g/Dl6xjbm8n6/IhQGMueDpLzS6SWV7G3NTP9+mmqHrqP4NAI5oY6MqOTZJ12UsEgnqpKIv4AMi+xWC0kshnMDjvRwREcB/eQGZkk6vNj7eokt7pOYmUda2sTDZEkO3bsYHlmFkt6g/WxizzcLjg3nCYcSXCo1UbfbBq9KoXJaGTJnyYn4dG9Nk4PxPFvJPj0fjunB9NUubJMrkkisRh76630L+RIxGM0lFrICgOzyyHyUsWTBxz8uCdGJp3hwd0OfnwzhMxLHtlr583+FI0lsBJSE4xkONyk5c2+OMfaDLx5009bnZeRuQhNZTpSOT2r/g121dk51R/n0/vtfOP1JR7qKqB7IsJDnXZevBriyf0Oro6nCUbTPLHPzoWRFNF4ksYyM5NLCXQ6LXvrdPTPJphbS/PZgw7ODWdYD8R48sDmvUWiCR7otHJ5PEsonOCJ/XYujefQq9OYjQaWAlkisQRP7ndweiCFIM89LXoujybJZDO0VJi51L+GWmPkod02Lo2mqSuUjC3nicUiZKSWTx/08EZvgmQyzRMHHLzZl8BrzmIw6JlaTZNJp3hwl53TA0kai8EX12FVxxmaj9PV7KZ7MsGxVjPXJ9McazfxzI9nOdrhZmEtwoO7Gzg7GOfxBj3r2g/0g/xDEYJPShfZnfg9IMrmtGfde5T9R7Z1Tp4QQstmoPmmlPL5rezVt9bN3vpc28pfAMpv270MWHqP/LJ3yL+rrPW1ZA06QjNzaEqKmXvjDJFUAmtDHTF/kEw4gs7jYaF/gNW5OcztzUT7hlBZLRia6ohPzKC1mrGUFJI16okODGMu8CLcDkI3elGbTNgPdDH50iug0RC51Y/B7YBMltFTZ4jFYmTXfcTjcaJDo9jrqskZ9Kxd60btcmA7uIfA2YtkNGpkYSG9X/0G9uZ6MiursOpHX1KI68QRgheuINUC9z0HCPX2k06nsB3sYuTr30RdWYpjbyeRuUWi/gCurk4ik9MkwiHWXz+D++hBdHo9iUSc9d5B1KVFWLs6GXzmObQVpbiPHiRyowe93oC1sozg6hpLE1PoM1mmTp9jfXkFa3sz4b4hzAYT9uYGonNziHgSvdWC7cBexr7zAwKjE4QSCaa+90N8/cM4dneA2UTBznaSoxMYVWpMHjfqihJ8125QfHAficlZ1KEwnp1tRGfnYXkNY0kh3vuPErhwjWQySb7AS99f/g05jRpzexOh/kGmR0bxLy9z7LFH0Os0HHvoCc70hXCbsnxqj42vvzrFzPwqKiHoH1tlZHqZcmeWs31+Cq157m03cn44gVGbo7bUTH2h5Hr/It1TCcKRGNcHlhhbjNE9OM/SWoT7d5q5PJrEbNDyyB4754eTmE0mHui08rcnl6jwwEYMrg8uMj2/xsCCxG6UfO3lWZrKzYzMRdHp9TSVW1nyxdmIqfDY9TzeZeXFqyHaat30zWawmU0Y9Bo+e9DJi1dDaDWSPbU6LowkUIscrWVanjs5SffwIoGNCC9cXKG9Qs+JHSZODyRQqySf2mPljZ4YKpWKJ/fbebU7jEDwyB4rP+qJolfn2FNnYXbJz/DEPPd3WHijJ4ZBK0gl47x4aZ2uOh33ddi5Mhwhmc7xWJedP/7uBBZtgmRGolOluNi7xKEGA+cGE1iNgns7zHz1h7M0FgusJg0/vDDL3OIareV6nv7xIq0VWmqKzeQzCc4ObPD4wWKuj8dxW/U4bXqqvYIfXFjjRKeHnpkU/riGIpeBpw45eOlaiFA4crerhzsgfmomCAAuKeVnpJT/SUr5X95Kd7rztrVstmaG/TUw/D80s15ic5Dp97c+X7wt/7eEEN9mczJAaKub7cfA/3XbpIAHgP8gpQxszYbYz2b33C8B/9/dvIdkMkVsYIhMMsnGuo+ydBph1BFZWCF35gJalYb1qRncS8tYS0qQsTjRwVGmr9ygYmc7+bV1VvoHKD98gHz/ENo8TF26jtntpLCqguVbPZi9HtzpNHavF41GzeTVbrx11ThdDiraWtFZLWhLiggvrbA+O4/z0g30Wi2jb56l5dg9sOpjZWQcSyyOd28nVZ07mX31JDq9gVg0QrXZBPk8Wp2Wmd5+ypJJZC7P/OgwVXY7iUiY1avdGPQ61ELFyugkrivdmMxmhk+fpfXYUeIjE4TX1tFXVbBw8gzNhw8QisdxFHhZOnsZp9NBNLhBaG2dklQKUmnKGusxdrbh9QcQeh2Bq92IfJ654RFqDHpMJhOjfQMYTCZSGhUV7W0YnQ60DbWMPvMsKrWa9PA4/lUf8ViUbCyGzOepUqkQeh1hf5Dg2UskQiHCwQ10Djsag56l0VFM1yxkMhmiGyHis3NUP/4QjsJC0tE4yXCEZDLJ8NgknooK1p/7LsGhHlKxWn58Y5V9rUWMLQZwWI3UVjjZU6+npcLAn70wzdRqlos9czx6tIX1qIrXL4/TUluAL5TCqE5jsZq5f6cNg1ZNKiNpq7bjtdn5w2dHODfs4ebwPJWFFvRqFxPTa8SSafL5Sibmg+g1kscOFDJb7MRs1HOwUU8oKrgxIhhdiJFM5xiaiuE0V2OzGLkxPMmr3QaklMwuBVhYBslml1Qi5SaXzTK/HGRpVZJrq+By7ywOqx57u5em2mIEglKPjhfPjFJa5EElVIzMrJJMpAiEvCyvhwiGE0SjBeh1am4MTKPV1jE4toLVrGPFZySdzmI06jh1y8fMahK1KkdbQxk9Y5N0FzhRiRRFLh3PXlvGZnOgRhJLSso8EqtB0FpXyPnhKMPTAeoqPEQTFmYX/PRPGWipMFFX6cVm1pJMpVhcj/DShTzVZW7UGi3Ti0He7C9gZHqVvnQOjaaOPFp6RldJZQpIp7MEQlFcVgOZvAqZl1y60s0v/ebdrCHugBCIn56WzUkhxANSytc/yM7b2Y12CPhFoF8I0bOV9x/ZDDLfEUL8KjAHPLX13avAp9icrx0HfgVgK6j8H8BbI/X/9a3JAsBvAt8AjGxODLhrkwMADAY9xuYGEqcvUPvIA2jMJgrKykgLgaW6EpXVTDoWQ22xYNkIk3Y5MRd4qM1kMRYXYmptJBOOkNsIY9rdSehWP7Un7iEfjaFva6YsnSGfziKtFpz1tYSXlqnbtwchwF5ZTmzNTzwUxmI1o5eS+s89jiqeJJlI0PbEo5DOkLOaqXvgXnLhKMmVNeyd7egWV0CrhtkFhMmAqaWB2OkLVLa1YOnqxHfyLEX1dQiHjebHPwXJFLraasKXr7HrX/4aqbFJMjYzJY0NOI/sY6N/hGQ0hi6TpqCxDmHQo1ILKh65n9CNXiz79yBWVkmcvYi2thJPJkt0doHUzT6s7S0kxiYoPn6ItdOXcJeVomltJnD6HEU72jDvbEdOTmGuryUxPEGqd4DWL36OxMgEhj2dGE6dw1FaTCIaJZfJoHLYicVilB3ch7G6Ejk5g0qjwdDSRPzCFVylZeh3NGEyGJDncrg7WlCnMhTtaEPEk9gO7sF38hzmo4dYcFrILCzT0lDPFz5znMjcDVKpFPd32Lk4ksAfTiGEgVO9YWrLXNy7w4rVXE86neX+nWYi8SqMOjX3tht57UaQLz1YzsRyhtm1KF84WsDZ4TRDc0mO7irmYLOeTKYYnVbN/kYzy34HyUyOe5q1qNSNhMMxhEqN3WogFI7iDwnODiX5X5+q49p4Cn80xwGvlbpiNQurMaqKbOxvMHCyN8KvfKqac/1h8pk4OaHjvh0mtBrBGwYd2ZzEZcxwbHclkUSedCpNR7WZRCKJUMGxPdWsBeI8sd/OasCGEFvder0CtVrFQ7vsdI9H2PDa6KpVkUiWo1YLOsphbBWeajLyZl+Cak0Ss0FFsT1PeaGNUpegukDH8GyWI7sqqfaq8dgqWQmmUKlUrEe1fOmBMl7vTdLVbiOeTHNfh4lYopaSAgNabZ6aIg1Ty3GOtTp54IAZqzZNMq+lygNjU1YONWhQqyrorFRzcSTJQ7ttpNL1qFUQT6TQ67UcajYipeTFq1oOHTp2N6uHO3a3Wi1CiK8DjwJrUsq2rTwX8BxQBcwAn5dSBrd+7P83NuvUOPAlKeXND3kJXwb+nRAiBWTYHMqQdzojbdu60aSUF6SUQkq5Q0q5cyu9KqX0SylPSCnrtz4DW+WllPLLUspaKWW7lPLGbcf6upSybis9fVv+DSll29Y+v/VBZ0m8yz3gP3Ue57FDuJoaWTx7AW1NJa49O0kMjxG4fIOSB4+zfO4y2ppK3O3NZOcWMHlcJFbXCfQOUnBoP6aWJm595aukkwl0NVXkE0kC5y5h3t2BLPYy+cLL5OMJLGYzyzMz5Bx2knOLoNVg2dXOzA9ewVhTBdkc46+fwj8yhtRpWZucJD2/jL62CplKo43F0Xs92DtamT93kbxasO7zEbx8A6PJDPk8vrOXse/bjbG+Gt+1bkx1NcSXVole78Zz4h50ZhOpYIj84iqFTQ1s9A5iMOiwFRVCMIzO6cA3PcPilW4y/iDWrk7ifUNkJ6YpPn6YiRdfJRWPEwptsDE1g77QQ9pkILq4hMFmRVtRyuBfPo3zngMUHNpHcmwCTSqNVKlYnpwkuLCAMOhJZLOE5+YxlZaSDkUwaHVYWxoJTE2zdK0bd1M9qelZDCYj7uOH8Z29gMnjpuDeI8RuDeLv7sXY3IC9sZ7BH/wQ3C4SApYvXcPSVIfBZsX30ms8+MjDpPRW/vTPn2Z/nYoiu+Trr82xp1ZPV72e3ukkFrMRtSrH6z0xju+wUuSA3ukkLouaeDxBIJzCZNRT5jUx70tT7DYQjWe4fHOStUCEhjIbT/9ojvt3Wqj2Cr59doWOWgtPHXLxancUjRqe2GfjD58dZt23gdGg50++M0pDsZZ8XjIwFaC9QsfxDifdk2kWQhru31vE//2tQaoKtJj1guM7LEwux3hiv4vTA0le6w5xoF7H2qqPFy/M47HkCEViLIXUlLrU2C0ann5lmEA4iV6n5ne/1oPFqMaqhz96boy6EhO/cKKYK+MpwhkDv3CilFeubVDmVpNKRHnlepDWMjW+jST+gJ/ltRAWo5a/emEYnVbFpcEAV0c2CKc1fP6eQl6+uoRRncZmVPPnL0xiNWkYnouRTUVQq+GxfXZ+fCuKy2FiKZCleyrNjko9x9pMnO3bwG7I0llnYSMY5GsvjfP5o8V899wiHRVqPHYdBeY0/+XrfSDznL0+hduq4UibjcG5FC9f2+C+DuvdrBru2F2e+vwN/vHjHe/rUZIPY2uqs0pKafwnNfX5p8HU5CSZdJrkzX4SiQSRlVX8g8MAxFfXSW2EsNss+JeX8I5Pk19cYaq7F0dlOXqrhYWzF6ndvYu4zOMqLkIKFf4zF1kcHMHmcaK9fhP/3ALeqkqM7c2E5xewr7vJ+/wMnzmPq6oSTzrD6sQUKosVW3M9JY0N6J12sNuIboRIRWLo9Xomr3djKy5Ac/0WsWgMk92BzenAWF7CzGunMFjNRFbWsRR40Gm1RP1+ov4AwfNXWBgZwVZcSObUBVRqFatj45idDmKDQ5isVopqa1gdn8ReXEjZPQfQGPSE/+pvETYzoRu9LA8OY3W7kKtrWAu8GIsKKCkpYuzbz+M/fQmj2UT/3zxH05FDxFZX0Rj0rJ67hN1mY/TCZSo72knNzOGtqkBnsbB+7RZmnZah7/yA1hPHWZ4YR63T47GaMHjcuDJZIhNTTJ67QPPhQ+THplgaGKQkm0OVSrM8MUkqGqU2L9noGyKfzhJfXEKdSLIyOIwqkSSTSmNvqGN6bIJoNM7wjWtoEuVksyqml4JcHC1Ap9PwypkBHjxQy5IvTjwZ5mSvCaHS8ublYQ7trMRu1vP73xzi8aN1XB5Lcn1gnuP76hmcS3Cwswq1WkM0nmJxNcStqQR6rYa+0VWsJhMTK1rCkQQz86toKKeu0kOhx0ZHhZqRKQs2Y54f3fAxs+hnotzDnD/N9IIPfyiBRVdModtCkVPNG91+NDozgVCck31x5pY2CIZjpLMSndHIzmIv6WyWyTkfOSCXcVLh1VJa6ORIu5NCh5aNSAmVBXoais2c71nk5miQcZuV8zcmaaj2cmlMS/fQPGZTPUPTAbL5PH2TavQaQSKZxmIxsr/RhD/aiEEjKbDBN380zqFdVbxwOcbGRoyc9KBT56iv9HKgycyyL8bEQph0dgONppKNSIL+kQUsFgNS5rkypkclcrx2aZp9OyoJxFVUFVmYXU9zcTTN5EKIm9MFmPV5wtE8lWUujraaCYSKuDywRkNNEZdvjVHksXJ10oi12PfRVyJCoNLdndloUspzWzN7b/e+HiV5aybw+yGEqJJSzrzL9wIolVIu/KQyoASbd1VbV4d9eQFzVyfpm724q6pw11ShKyrAf7Ub3E7yJYW0PPow2XUfps423AsL6O021E47BXW16CtKSS0sUfLgvaRHJrEcP4wqn8dQVozK66bEZoeiAhKjk6SWljFaLLiOHiAZCmMp8JIr9NDyuSdJjE9hKSoiueojmclgQEV5fR1avR7T7h2URCOotVqMezvRptMYhscQyRSmkmLKW5vBYsJss6E1GDHs6iB96RoV9xzCaDHRbLUQC27gOnaI8MgY9VUVJFZXcZSXo5Z5NC311JpN5LRacgvLBFfWKDl6ANJZXAf2ohYCbYEX6Q/gPHqA9VPnochLwa6dGBwOsiYTRc2NCJ0WjdlM3T/7LJHL1zF0tFKv1ZCLxnF27iB5s49MMknBkQMELl6lvL2NtFZN3YMnyCytoa2qIDM6jqitBLWG1kcfJrG8ir6lkYbDh0gEN9B1tOLY2EBrMGDY1UHsynUKd+/E1lBL9NYAJU31mJvqIRRFX19DdCWAxWxk5649HK4O4gslMGoqsJohEk/SWOnmQJOZWFpg0UsKXWpay3XIXB25PFR6BR2NRQiVhr01Oi72GNhVoeLkgIrPHrTx4mU/zcUGfvHhJsaW03z2oJ3plWJqi3X4QikaKx2sWg20lgl0Gjv+jSTn+xN88UQJPTN57u1wYjQYsBkF+xqM+IIOykvclDgllYdKGFrYHOsZX4ii21eNxagh7THRWOWmrVQw65ebk0EsVuoqveRR8ch+J9PLUR7eV8zwUp6R2QCPdHm4MpYiFIryLx6rZWpNYDfkePxYA5ks6FQJqkoc3NdhQqcpx2XKYTbraSjWspGx4jCkuTayQU2hkZmVOCv+NL/56Xr65yVP7rfjcljwxXMg4aE9JnpnEuRzkvv2VTGxEOZQo5YrGNCqnJS6dAzPxznQZCSezNBT6aWi0ExXvYEXr/gpL3Fj0+eoqyjgwZ1GNqJpbs1aKJAJXrkR5NF9Tq6Paakq0hGJFKPVqjneqmdR4/kYapH3NWbjEULc/uLGr23NpH037/dRkvcdbIA/FEKo2Bxf7wbW2ZyRVgccB06w+eD9uwabT/wb4rZbPJ0ktrKOFkHFYw8Rn10gPDqB1eNGmE1EegYwN9QgCjzM/OAVvAe70OTysOan/JH7iM7NE19eQWe3k8hlCc/MYqosRR1PEO8dwtjWhMnrJrmygtliJpHPs3bpOkWH95HNpAn3D2OqLMd+aD/rJ8+QN5tw7dtNtH8QndFILBpl/dQF3PccwFBfTXxwhODFaxibG4hm0qycPIdpZwsbU7M4d7Sgriih50//O2qjAXUmw/hrb7C+vo62qZ71U+fJ+AKkU2kmLlwlthFmdXGJsWefJ280QDbD0uAw6xNTOBsbwBdg48IzByGZAAAgAElEQVRVHIcPkJhfQl9SBID72CHmXz9DPBzBPz5BqPsWOq+b8Oo6C1eukRkYRqjV9H7160TCUTJOG4Fzl8ibTSR1WhZffQPhtLM+N4d/aAxpsxFLJtg4fwVLZzvWqnJmXz9FaHUdodUy/r3nWZ+bQ1jNjH7j77A11ZNKZ0jH4ujUGlxtTfi7+7BWV6IqLWX6lTfI262kI1FuLsyjT4X4X37rV7k4IbkxkeJwq5Pnz0ywsBbhiYOF/PVrcxxsMrKjUkf/+Ar/5zP9aGSCaGSDZ9+cp63aji8Q5T9/o59ij5k/eHaYvbWbv2bv77Tx7OlF6koMPNhp5S9fnSeZTPLMj8aZW41T6sjTWa3l704us6tGz4mdNq4M+dBp1Zi0Wb57fo1jO+ws+uKc6glyoMVKmRPO9AaoKDThNueZWUsxvJjjxE4nM0thzHot6SxcGEmyq8bAwRYHvTNpzGYT9+0wcXUsyeBCnoZSA6FwnHjOgNumo8SeY2ghiUmn5sKtac71rqFT5RmZXSecVHFsp4tnXp9nf6OBXQ12BmdidI8GaSzR4LWpeeXSAhtxcFgNnO9ZIRCBYmuWr748w+rqGreG55heWGN6Lcup6wvMryUocUiO7bDxzBuLqISkq9HO69eW8JolNyaTvHojwq8+VEo2k2ZqNYPFYiGTzpDOaXhoj5ObU2ne7E9wvM1CcCPM9IKf1675CCfhK9/px27RUFeo4vsXA6TSmY+8/hBCoFKr7ygBPinlntvSewWadz31O+R9oGEGKeVTwP8ONAJ/BpxnM/D8C2AUuFdK+cZ7HUdp2byLVCqNWadn+FvP0XhwP8nuXkxmE4Ovn6bxQBeaTJa1xSUMF68RCWywPjmDw+NhbWqaXD6PSqPGNzmFQBA8ewmTxcTw91+i5cETjJ65QNnOdlL9wyAhn80yNzyC3eVmaWkRi16P2WBiYW4Q65UbZLJZ1mdmiPgDFAaCJMMR1mbn0BsMJKNRrH0O1BoNi0OjJKJRtDotemB5bh7LZAFrU5M4PG6Ew46jsBBDXTU6lxP36hpoNMQXl1jo6ce7owWTy4HV46bgYBeoBcNPfxOMekxlZeSlJNc3SOR6L2PnLlDS2UF8aITFwSGsDhvxcJScRoWjpAiT0QhuF4GhEaruvQdknmQ4jKGlHqvVwtrUNN69nWQ3Qoydu4ynKoylqJDFySlKy0pwl5VicDoIDo8SXVgkn8thHhwhuLSKzmym8PA+1DoduWwWY2EB1rYm5i5dIzQ8ht5sYujpv6PmoftIr/pY6e3DYTGRTaUQahWJ4AbZ+UVis7PcWshj1WuZWhcEltaxWQw0VHlpqLDSNx3BF4wyuZTEoocjbS5mV2Lsa7Lz2o0glSUOago0tJa76Jvwc7jVzkY4xcxyhJtTCcwmE2uBGNcm8yTTGVbWQzy4r5y1UI5H9nmZX4sxsphiIxLnh1f9qGSWfC7P+GKSVF7N1IKfM4NeMlnJxd4F8qo6sjk1s8shrk+kkej5s+8Ncqizhpevh5leDLK0HiaWzNJR78EXSgKQS4ZYDedYtpsZmoyQyuT5wcU0gXCacNSH2VhNLqdjYSXI4KyO1ppiitw66orUnLweJxxJk2soYWktxPkhNwA6nZa//fEwjx5rpbpAy4Gd1RxqMnKmL0RjbTEtlWbG5oOMTfv47aea0Jns6LRquhrMXB/WEAgn6JmQJFJ55leCNJToSaWhpb6YQDzBtVOjtNUVcnk8h1AZeea1McqLXcwtBylwWVBri7jQPU1jdQHPvL5I77iPz9/XxKFWO7mcJJmqpNBpwKhLk8ukePX1szz1K//2I69Htnk22upb3WN3+CjJByKlHAJ+94NfptKyeVd6vY6UXkfJzh0Yqssxd+0kIfN46qox7ekgl81S2daK8/A+TGYj9U8+jK6yFFdZKcX1dVBWRvnuXThLS3Hcc4CswYC7sgJdVSXVnTsx2u0YdrRg6GhBoKKqYwd6k5HipkZUdTVQV43RYkXf3kw+L2n8wucorKrAemAvWrOF4ppqPOVllO7bi6a4AFVNBbZCL97qSiy7dpDN56nqaCdvNGIvLMK8fw/JtXWqPvc42ek5suksFrcbq9mC0WSi/eefwq7XExkdp/qzj5HoHyKXzlB2+ACqNT8anQZ1KIp7dyeakkIKa6txFBdjaW+hsKYKTYEb0+52UtEY5Q/ci1mnIxcO4yjwojWZiN7opepzT5BeWMJ36Tp1n3+C7Mw8mVic9s88gsPjIaPT4amuxlVTjcPjQZuTFO3bg7eiAk95Gdb2FqxGIzWfe5zk6CQAFpsVjczju3Kdhqcex1Regq6+Fo1GjTQYyKysUrlrJ4a6aqwtjThqa7EaDBTt3cWephbqdnTy1JPHaKwtoaTAzr3tJiqKXaxs5Dm+00NNqZMCh5bWKjPdU0lqy10Mzydpr3FiMuqxmnW82h2mrtzCzakMv/l4JfGciSf3O6lxZ9nbWkSFW+K15PjFTzUSTmvZ1VxKz2yW9loXOp2OltpiPn3Qi9AY+I3Ha9FpVfjDGf7lZxsxavNoVIJ7d5dSWyCw6bI8sK8Ckx6c+jSPHK6hyK3ngU4z+3ZUsG9HKSWFdqaXo0wtbaaVQAKJJJbMk89JvE4jTx5w4XaYqCz1cKRZQygSp7W+hK4GB0VuPcEYWEw6drVWUl3uosiSorHKQ0OJloc6TSSSaY7sqSUSS6FWq8nnc1weClHsNmIza9HrVIwsC/79z7eyupGj0KGjyJpnbj1Dc20x9eUOqktdeBxGasvdHN9VxPiK5OE9DooKXBzd24BWr+dIs54jLQY6WypornJyvKuO3U0ubHrobCqhrlBDQ5WTBw42EcloEULw2o0Aj+x1Mu9LU19mx2QycN+xAx99JSK2/Tmbtx4lgX/8KMkvbb3geD9bj5J8uJv5cJRg8y6klKjjSYrvvYfQ0DiZeAJtJkfxscP4u3swlRaRTCbw3+jBuqMFU4GXwe++SDKVYmlikvnXT6JvqEVTUUZwcARNOkPlZx4j0t2Lxu0ko1KRXlkncLUb6652Zq53Y927E8/RQ4SuXCfYfYvqzz3O2Le+h6W+Bq3dRtZqYWNoDFNxAWsLi6zPL6CTeSZefYPBbzyLvqIc8852Ni5ew2gyo5KQX1im+MQxEqOTmMwm1DodsVCYwIXL6FsaiUUjiI0Q+uJCcoVeIrMLaIxGclYTS6fOYmtqIBEI4u8ZQFdTiaOhltziCq6aalKZFKnZRVw1VcR8fny3ejF73GgdNqLxGHqhxr53F4uvvYGloQ61VoM6lcWk12NwOsiFwqg3QugrypAOG5HRcUofPkFicBip05E2G1m9dA1DTRWG1mYmnn0eU0crBpuNhD9AamODvMmIyOUxqtRYK8pQRWIk+wepe+pJIn0DOJvqKTxxhMjYJMHBYezN9USn50iGQrQ3NnHgsSf5/T/6GqXaBR7tcnF+KIFOnaelVPDatTWOdrgZXsiwEUnhdRjZiCSIZlQ0lmopc0pujYcochvx2rQ4LGpUKhUuc5bplRRDizk+d7iQidUc62EVNYU60sk4uVyOYDhFNJ7BbTNQ7lFxdchPeYGJbA7+7kcTeGx6xlZUXO5dZMUXArWRZ340QfeoH4dFx9WBZXqm49zTZiccSfLcmRUONOiYWYqwr8XDfbu8GM12uprdlJcUkkznGJhL8qufKsdp0XJ1OEhLpZn7dlo4eStMkdOA15LjexdW2FWtw6RK8MPLK7RXaLi/w8qPb/h4ZJ+Xc72r/PFzE+ys0iCQ7KzScOrGPK9fHKZ7zIeWBPlsgm+f8/H4PidFbhNne1fZW7/Z/dY/m0KrUXGkzU7fVIR5fw6nRcO1kSCN5RbsZi3JaIBkPMJ97WZO98fpnQjRXqHn+vAaBhFD5FL0jCxz+uoYQqPjQIMBvUGPSZVkbWPzrQ9GvZrGIhUjCwksJgNWi/kjr0OEEKi1ujtKd3CsZ4HLQKMQYmHr8ZHfB+4XQowD92/9DZuPkkyx+SjJXwL/83bc3/uhdKO9i+npaWIBP7ruHrR6Hd1f+e+U72xHdaOH+Z4+Gh84TmDNR3JohKpkio1YHIvbheeeQxhX11g8eYbYwDD6bI7hC5doOXKY1K1+Zm/1UVBbhc3jZfSFH6I3GCCZJB6NER+bIq/RoDMYWeztR5PJk02nWbvVh21qBoPByNC501Tu7MBot+IsKMDU0YpnzY/OakHYLERm51keGcPq3Hxo01ZTi7p/iLXePorb2xD9Q2gMeuZ6+rBYzATnF8jlcqhsVrR6Pal4lPUzFzGbDARn5/He7EWt0TB74SIVO9oJj4wx39NLQVMD7opypk6fRqPRUtHSzMCb52k6uJ/8rQF0FiuL3T2UCYF/chpvaQnRYJD5W704qspR3exnqrubooZ6dDd60Ws0bCwuErhwheWBISwFXjxlpUxfu45Np0el1RCYX6RweIJkNksmm2Xk775D4y/8M6a/9yLuPR3Er99k8dJVXKWlGGcWWBsdx1tWSsIXxGQ0MnbhElaDCbXNwvK3vk/y577A1ZOn6L3Vjbm9BJ1Ww62RBWxGLap6L6dvLFDmqkInsvzpC346mstZ8cfxh1JAJbGklnPXx3ngUAtXRzZQiRAarZ5szsh3fzDIvfsaOD2Y4dSVUQ7urOH8cAq7VU//+Aondnn46kvTPHWsBF9Ey/Pn57h3fyMWl5Yir5VjbUaEEPjDhTitWvbXa1j2F1HsNlJbrOXmSJapRT8/uCiRqJhZDHBxzMXg5AoGnRqDJkcgkuH1K3FqqwpQq9R01Jp56YqfdE5wpnue+w61sbwBp65NcWR3DTazhcn5FV7rNiAl9Iz4MJhd6HUqfIEIowsJOqpNnA0nWQsmGRhfx2oo5MnDpRhNFpwWLVJoGF8IE0/kuVVkAVTE4inOj2TIZHKs+IJMzaXI5ipYXIsQTyQxGPTcGvNxz546hueCLPjTaNRZzg/pWfFHOb8WZP/OGpZWA9Bop6bYRKHTSDiRpX8yQCBiJxhKcLjZxF+/Ms1De71c6IuxEc1yunuJI3trWV7zfwy1iECo7k41K6X8uZ/w1Yl3KCvZfC7mnwwl2LyLmpoaLLOTmLs6SV2/Re29h7E4XIhCD7FAEJ3Tic3jprCsBPuhLjJvnqf0i08RHxgm5Q/Q8MXPkRgYQd3WhHV4FE1jDajV1AmByOZQ1VRQns+iMRhR11RQX+iFjTCGPTuRPj/2lRWERk3b//TPiV27ie1gF8GeftyV5bju2U+qZ4BYNIo+nsDodpIMbuBwudBotVi1OlQyz2L/IHqtBveBveikxORxY2pvIX7qPOX79qJxOvBUVKAu8KDV6wiHI1gLCig6cZhQ3xAND92HOi/JplIUNDZib29G53GhkRKdxYyquICypiZyuRzauiqahCSXymDe0Up8aBS9xYRpVzuF8RgqtwNrZRmq7l40eYmuvYWGdIZ8NotpTwexlVVKW1uwV5ahU2vI53Nk7Tbq7ruXpD+I/VAXTek0uVwO28G9qNfWWRkcIjgwSDabRcQT2Nuaya36sHq9yLIiak4cRWpUmNpb2OgfoubIITRlRbARprCslGOPPsJrzzzNr/3al5ALp6jw6gjHvJgMWrrq9FwZcOKPSVYCaWwWHfe2mzAb6/CaU2QltJdpmJx1cLRFSzJdSrkThBrUpPnC/Q2sh/Mcb9WSzTWgVgmONOuZWEjRh+B8v59MNsPIfBSBmr0tRZS51dQXa6gqcTA8n8JthXKvntWNLC9fD/PwLhuv90SwNpkoLi7maEeecEbP7EqU1hoPXXVqJuac7G5w4LUJTnb78Bs07Km3MbpkpKFMw+RqmGKnwFdgp6NCoFODVtXIajCFTZeitszF/mY7Rq1kaT3GnlotN8bD/MKDtag1asbm87TXOCl2G3j4UC0LviROq56SQgcyE8dk0NFYWcjeegPdk2mOtBiZqnBQ4xVUFph4IWlFX+iixJbF0VZILLUZyEq8FppLNaSSOqoLS1jw53h4l4mTPXlKvBZ2Vanw2loIxiQFLhPPXwry25+p4c3+JORTrAci+CN6stkMc74MB1vsrAYyHO6soK7EhK3A/dFXIuKn6q3PCCFKgUpuix3vtRz0W5RutPdS6GH+tTcxe9x4u/YQXVzGf+kalU8+SmhsknwqTUpCaGYOY0khGp2G9EYIk9GI1mRCWi0svPI6NU89TqJ3iI1L1zDtaGFpeorlN07jPNxFOpth7eJVzDVV5Ax60mvrxPuH0ZhM6L1u1BoNuJzEJqfRZLIUP3AvseFxpF6Hs6uTyeeex9TWiHVPB8mxCaI3+zG2NpIKhSnq7EBlt9L31a+jKikkEQrh6+7F2lCHra2ZlfFxlscniCwuMnf9JuGRcVy7OggMDKPOSxxtTQTm5kmsrVNy/zFCPQPE1/2YyopZW1pi7FvfR2sxY3DY6P/Gt0hkMginjfTCMprgBjVf+Czj3/o+jh0tJGcXiC4sU3H0ILZ9e1h+7SThWJSc00FycZnk4BgFRw8y8vqbhIMBkuk08+cuopISXA6mvvsCwXUfeauZ8PAoqdFJSg4dIB+J0vbPfx69xYJqI4yzqAjpsLJy+gKunW3E/EGCgyPo1Rq8B/bgu9mHXq8n39rASG8fNR4j9584zFTAxOneDQ40GpHZOK9c8/HFE+XcGAmQzOQ50urgO+dWyWXi1JUYCUXiPHd2hS89VM7V8RQWo44dNRaW1yP86NoKDcVaqgtUXB1LYjVIkok4kXiGoUVJPJ4ilREc3VPJ8FyU68OrtFUZWfLFGV9KU+Y1MheQXBpN0lGlp3tghtmlACqV5FCjnpuTCSx6qC01c3VgnumFde7rdPC1l6f54vFSro9FGZiOUVHi4jcfq+bywAoXr49xbTzJ4102/JEsv/apCm5NZ3mjN0JXvZFdNTp+eGmRR/d7uDAY4fXuDX7jsSrODkRRa/TsqHWwtCEwmS2c2OXmGz+epbVcy/E2E+cGYxjVWY53OLk4HEGn11PsMrCvTs1/fbqPfY2b3Wd9U2Gayiys+oKMLKbYXWNgPRijotDI412bM9G+f26elnId+XSMiYUIdqsRlVrNhZE4O6sNRKJxfnTDx6EmI4Fwmmv9s8wsR6gvsdAzGeHffKGZApeJydU880HBpw8XMLEYZSP0MbwbbfvHbD4yQog/AC4C/xvwb7fSv7nT/ZWWzbtIp9OoNsIs9g+g02nRLSyjUatYnJ5Dd+EKwaUVMrEYVR3tDH3nBZofOkF4ZJyM0YBvcgZhNqPP5QjOL1IyOMb63DzZdBrz0AgqvR6tRkOsbxiTVsPM5BSGC0byBj3RS9dIRiIkQiESgQBlUqLL5Rh4+TRV/z977xkkWXbdd/5emvdeep+VWVnemy7TVd1dXW2ne6ZnMBiHAeEJGpAiRBCidqldyqxiJUVIGwtF7JJLSUuCSwuQAmEHGIzvae/Le+9tVmWl9/7thx5FQBQCHEowJAK/iBMZcd69ryrzw7lx7j33/I/3khqbJLi4jK2xDls6TSaRIjMxi0qSWLv/EEuVD/3sIivDY7SeG6SQTuOor0fJZJHNZpZu3Kamt4vC6hqiy4UxlcH31CXi27vsvHUNZWuHteExOi+cJTU5S1mtIr6zh/7uIwRBYPZLX6H93BkEoO5EH4aeDjKhCGZPBUW1BsEfYHXyOp7Gekr3hkgn4sRm5innC4Ref4fGgZPk/QESoRBi1oDZYmX6rXep6+ogNDKJxWbD1tdD3h/AVulFrq8FATJ7forZDFpBYOGtd2k/M4gql2N37wDX4ioyApu37oNajaPKR3B3F8foJAaLhaXrd2g+2U/+0RiHiyvYXU4y2SxfvXuff/75TzI6scBaoEBoL4Ao1aIR9YwtbOKwWdBJGqrdJiocMvJempmVQ4r5LOWywtZ+lAfLHmaX9zDqNOTyFcxvRbFbjTycj5Iuarg3vsW5/jrcFonf/cYSFU4zoiTxxPEKOqpl4gkzwbiBsqJGo1HxrZsb2Cw6ookskqTh/qKOep8dnU5mYiNPIqvi2oM5etp83EZA1mqp81qZ3S4SiqYZXi9jtci88WCF4+0+tv0FVJSprLAQTRa4t6hi/yjJ2FKZlc0U2bzCLb2O3f0QDpuRibU4KgRW9iIMrdqZXdnDYTGQyhTY2guik7W8VbKjUQt8+/4htT4X14bWaap2EE4pqAUYndlAEpswaKChxsnMZpJUTmB0NsRATz1H4Qw7BwlsZj1js5tYB9t4uJzDbdFQKuv57lAYSdTxpasb/NrzzWztxVFUGr778BBBJTE0s0uuUIndUKajyYtaKBNLZ1ncOGKs2okKLd94d4bT3bW8PlImkytx9+EYv/hjPrkQEH6auj5/CGhVFCX3N478PvxssfkBiKJIJpni+Od+lezsEpbBfgI37uFqqMN6bgDt8DglmxWN2URVSzNatQZNTRXRjW10RgPG7g6iswvUXjxH2WbBUuFGQUFua8YYDKNVqTD2dJA5CuGLd2H2elBXVbL5rVdxtzajRaAoaVG7XIgeFx2lEtlYnIpzAxwtLGFvbiQTCOJsbUTqaqOYy2PfrMRS5cN4vJPGSAS12YQhn8fy0rOEbtzFcOkcHQIUUmn0tdXkVzcQWxrJHYUob2zjbGlAOtaOKxhCU+FCqq1CHwpjPnEcva8SQW/AGQohVVXiLpVJZzPkU2kSE7NUPvsUBX+AokZL3dlBdE4r2WCYmgtnMFX7yAdDZFMZ9L3HiI5P0fCBp8murqFpbcS1vYO5s5XUwRHWS+dJbO2iBXzPP0P8/jDGk71YKlxkUin0jXU4fJVomutJz8xT0dKEUFeDIGpxRGKIskQmnaH3858lP7dEyWnH6LAjHe8iNDJO+y99kvzCEq6LZ+HVOFs7B/Qca8Iq5qhs9nK2TeKbDyL0t3tor9JSLPsQSlkUlYjVasdmhWf7Tbw+FOTjl2uxWtQEgmZMBg2tXnCaqyjks/icOjK5ApK2jgavTCpTQq1W8enLXqY2s2wHFTqqQdDoOd+hEM2oOdOqJRz34LSIHEZzqFRqLnTquVoQEFUZmjxaXBYt2/se+pqttFZqKZWdqNVqap1FnjhRg1mnEAyn6W5x82SvBYOs5u3xFHanlqe6ZL79IIzVbKDBZ2JyPUNbnZmnuiS+m7FAKcuFbievPorS016LU5/nw080sx0q8ES7xJ8fRpAlDT21Kmqddawf5Oj0qUj11ZNI53i6W+bOXI7zPRUYZYVivsBLZ72sHZbQpdO4euq40CFh0VdT6xBY9pfweRw0uaHGpePNcQWXo8QLJ028MRKhpdZFLJlheTuKLGv5zZcb2TxIEAiZuNRjYWo9zqlmPcNLCdQaDb/xchMzO3n667U8d6YRp1Wk1qnhzkKJwUsXf/xB5KdrG20d0PI9WjZ/G362jfYDUBQFndGAZDKimA1EJmfQV7iwDvSz9Z03kZobsLY1k1vfwl7to6BWkZ5bwup2Ixr1jxtHxhM4+3soB0LIFjP2i2eJPhxFFkWktmbSq5ukZxZxXzxLJhwlMjpBwwefRgFK5TL2/h4SC4tkwhEw6DEN9BO6/YCmS+fJrG8hKVB56SLphRVS49NUv/Qsiqhh8ztvoq31sfTOVVRVlQDoujrILiyDRo3t/ACRsQlC+360kszWm1cxdnciN9Sze/UGtS9+gNThEXn/IQaDAUdfN/mVdWLDo9R9+HkiSysc7u2hNuqZ/OKfIrU0IhoMBKZm0ZvN2E72kt8/RNRqsbW3kNraRVsq4+ztJDIzj1arRet2kJMlAtdvU/X8M+Q2dtAWCohOB/ujE6hqq8iEwmRkLWtfewVjbyeus6eIDI3j7u+htLOPwWik4tJZYsPjBN69hbahjp3FZZLRKCqNllypSGR8mpqXniO7sIxeUGNwuzD097Lw+3+Mt66edx7N8a/+1f/BYEMZo1Ti2/cOOd1q5Jl+G68Ph3Aay7RWifzl22tIQg5VOU00kUOWJDrqTPzRq/OohSK7h1H+4uomHnOZEy1mprfzLPnLfOCEnVuTQbSSjs9/qJE/fH2D3joRnTrDzckgrV41NRV6VvczvDEa41KXgVhGocKmo6dWw8R6FlkUeKLbzthGgf1ghlNtdtYOCtycjnK8TiSTKzK8lufKcTv+UIqyoOXlsxUMr+Z5bSjCE8d0lEtl3h6LcLrdTJVTy1GswMlOD4WShq3DNPUVImfaTfzFu7sMtJkBheWDMi2Vaja3/Py7L8/yoUEnFoPEndk4bVUybT41X/iLKYr5ND21Gt6dSlIsa+msMbK4ccT1kX2UYp54qoBG0pPPl3hnIs7JJhmfS4ekykK5wPRWgXV/ioYKLZIqy+xGFK9Dz8uDdsbWMnz4chOf+UAtb4zGmd3K8Zlnq7kzlyaV1yBrythNaubXjxhff3yX6T98a4nTbUaCCXh3KsVz/eb/SkX0x4YgoNKK78v+HpAGJgVB+ENBEP7Df7H3O/lnmc0PYHVtjUw0hjg2jSwITL17g7ZzgwjxJJHtbZzLHhJzi0SCIdjO4aqpZm1knOquTjQ2C2tfewXP+TMkZxZYuv+Amu4ucvceEQ2GSYdDuM1G4lOzIIqoH4ygURR25xbQCgKZZIKj9S1a1WoMNitT/9+f0XbpAuVYnNXhUar7ekgdBsinszRJEovv3qTt3CDJR2OU8wUOV9fQ1/iwVniJb25R2vUj6XXszC8CUJXOUDF4kqL/iKxaTSoSJTO/hNagJ5/JcrSwhCIobFy7TalQwBSLk/Qfkk+n0et1lNUq7D4vmkoPNq+XXCxObHae6L4fx/4+2YMDVh+OUNPTiTqRYGNohK5nriDnCszeuEP7uUEyo5MU/Iek43GKc4tsTE5icrpIplOUCgWSe/sIag35dIZioUjg1kMMBj1rQ8NUdXcR2d7F3lSPemSS4PbO46wxl8Xm81JMZQjce4hRkjja2EJ8OEI+HkXqRH0AACAASURBVEcRRVTj02QTCSSTmSdfeIG9jQ1mX99j8zDN9mGO9b0ooqRjaj3D0voBaqVIT4MZj1PP3GYUr9PCf/zWCk21TkZWBSwmHR+76OHBQpzdYIGDSJbh5SRDs/u0N1TwxojC5n6Yaq8df0RDMpVjeF1BLRq58WCJTElmyV8iGs8SiScwm4zcHF7mg+fa8MfU3B3fRNQIGFQOTFp4azTNLz7lJRaPM72RoqlSz8LKLjqdxO15PYlUkb2DQ0SxnoeTWzisMtObMndG16iusJAvKgiouDa8yUsXGjCJGV5/lOJkh5uZPTU7BzGW/W5uDC1z8lgNbwwHsZgkanxOjhIgSRKTS/vcXzTjMqowmXSc6zQzv5Xg3YfbdDQ4kAUdsqSjo9lEuqhhbmULg0FCKZdJpLKPS5NFFfFEkWA0jUYTYX07z0cuVuJx6PjP76xy8VQriZyG5a0QRp1I0G1DL4vcH9/GZrUyNruN06pDVhnJFlQ011bw/EkLe0EtmVyJG1NRbo5uc+VsG7cXChi9P/5qNOFv167m7zrffc/+u/jZYvMDaGps5G5gD7m/m8jCMp0f/RBKJIrictD0wacpBUI4Lp5FmF0gNr+E4rTT+fEPk9vYQu2rJHrjNpaDAK7+HhqDQbTeCgxtLXDvEeV8Dp3LyWE8SeOHn0fv9RC+eZeKthYc5wcI3n5IwzOX0OgNqJw29A+GEVubSY1OUnvhLOaGGqwBD2WDHkFQ0XZ2kFwmi/PyeUL3HlHZ243GYMB79hRKIIhxoA8AazBIyH8AGg0aq4Xy7gFsbdP2yZchk0flsJF/NIJaI6Jr8FEKxxA1GpyXznJ04z6xwCFIEp6BPpJDE2SX1vFePgexFEW9nrqnnkDW6ZAaaukQRTLxBPYLg5g3t1FVVIBRh3VlDVVdNdn9Q2xtzdhTKQo2C63PXCG5vYt5oJ9SqYzFYkGuryV65wFySzPWk8fJ7u/T/sxTqFQqIptbmJvq0dfVUFMqkVPA4HBQjMQpNdYhIiBUuHDFE5iqfazf2cReXYW+r5vyzh7emmrmJqeQkkF+5dd+jenrX0YXT9PRKPPBEyauTsT5zY928mgxRXuNjqOkilwRLrRrmV7Z49l+K6CgESoYXc+RLUk0V6rwOg0cb9Zgs5rI5BX66lRIah82q8jJJpnJBZGBJg1jqylqfXae6tYhatW8mi1S5bUy2KwmFK5GLwmcahI5DDnIFsDj0DO0GOUwGOeNITX7oSyCWk02l0eSRZw2ExfaJV6Ni/S013GlR0csUUGlU8epFpn1PTfNtVZONsnsHaXYOTDTUaPjqzeOOAqnqHOp2NhP0VFvp6tKINnXgFFWc7lLz9sTGbLZNE0eCwu7CfrbPByr0XB3LsPPX/axFyqQzGv4J584xspBEUlTpKfCwNx6hEavTG9HFal0AbNc4iBqYLBFxKzX8OqQjn/6iRZG1kq8fX+Z7cMkCApVHhuXu3RIohq1qpXDUJrTTRqOogUOjuy0eASKfQ0oZbjQLXNtKk2NM48/nGN0tYjHaeB8pxGzsZlgLMfTp3Xsij+BajQEBOGnY7FRFOVLgiCIQMt7riVFUd53D6CfbaP9AARBIJtIUi4WUYUiGBtqyeVyxKfmMLU0oW2oJT2z8PhSos9LZHYBjdMOFS4O7z7k+Oc/iyqeILV/gFxTRTkaJ3jtFtaeDho/8gLhoXFaXnoOZfeA+PIahsYGLH09JFfW0VnM2NpaSK1uELr5AEdPJ7N//GXE9hZUskxsbBZ9dyempgZShwEKpRKmgX7Wv/4dVKKI98Ighw+H0ddVk8rlKKTSHF2/g7m3E7VGQyoaZeUr32Ly6nXEpjpMvkoIRkiMTXPss79EcXuP9PYujuYGyho1wYkZTC2NVHZ2IDbWErxxj6D/AJ3ZhNFTQd5/gF6jxd7ZRnJvn0IiCaIWfW8nR3ceUn1+kPT6OvtvX8fW283i116hXCoiNzdQzOcpbu6ia2nA8cQZtr/9JvbuTjJbO0Qmp9G3teA4dZzIyCSqeBJ9ayOrd+7T8NwzKHsHxLf30FS4cA72E7h9D+wWTPV1JDa2CT0YwfvkBdL7fiobG5AsZgrhCIX1LeS6apbW16lyGTkMhPjGzW3SqTjHqrV8846f+kojHquWnloNX3x9i/56DaV8iu8+CvMvf76dt8fj3JoO09tg4K17q7R6BQbabczvFnlnLEx/vYhVLvD2SICznXbiyQz+cJ5TnR7uzyUoKRo+damSsbU89+djnGo1kMul+O7DIB88aeUokiaaLGA167nUbWDZX0SS9XQ0enDb9Dw7WM1zA26iGQ3HW1w83WvgW/eD2IwiqXSKtf0E7TUGgvECb41GeOG0jUgsTSBWYGStQFe9kdmNOJ1NHv7NZzq5N5dh46jERy54GVvP4zCJGMUiG4EielnF2XYD//pPZzCJOU63m7k1k8RlkamvNDG6FMVr11Np17Ltj5PMa2j2ajnbYeRLV7foq9PSXQ0jCwGeP2XjrdEoNyeCHK8XKRTK3BhZ4+mTXvxxkYOYhs8+X8vVySSru3G8ZoXnTli4Np1kaCXPL16p4o2hQxrcKihn+NbdQ063ygx22nlrJER7rYxaKfDOeJTBVgNPdht4bSTOD1mB5H0Gkcftat6P/V1HEIQngBUe90f7fWBZEIQL73f+zzKbH0A+l6eYz7P8lVdw93WRnJxFyBcJ+w8xPnrcnHXl0TCeznYEnUw+myPwcBghXyLq9+OensdgMTP7tW/RdfE8a4+GcdbXkZxZQEDgaG0du9vJwfoGzupqtOcGUEolNm7fx1RThXJ/mMO1NRRRRC4V8TY3ImSzJJZXUUplEg9HUBQoJpMcbm3jyuXJZ9IkIxHk+RUK2Sy5iVmMJhMTf/AnVLe1sX9/CNlgwP3EWTwaDYt/+p/JB46I7foJbO9ir/KRXt1ALWrZvn2XltMD6CWZteFxGq9cQigUiCyvIggQ2tymmMvi02jIpFIEt3dxFPNo7HYO3r2JympGGwzhn53DGY3jqKkmsruH62QfJrudVDAIdx6yPjJK06kTpIbGKRaLRA4OMIxNI8kS20OjtAycorxTZmdsjPq+XpJj02hFifDULPlsFvXBIc7OdpJD44T39shmM7gOAsg6Pfsz09jtdoRyif21TXxtLWy8+S5aSUQ3Ncf+zBTr+iLPP32OvmO1CIU4sayG2bUQ3gonR7EMAmoOgknuLeXJl0W2/X4erdlw2vXcfLQMaj2NNS5eueunq8XL+vYRtT4HKhV47SLfvhXCrBfRSyr+6LV9WmssjC8c8tzFNtb8WXaOUqhUanL5MNlcmY3dMBMVZuwmLf/uSzM8ebqJ+X09b96d58KJRnaDCZY2swwebwA0vH5rhitn2pneUdjYCbF3GKXWY+LWVJSfO+dmaSvF4k4Mj0VDk0fDW8NH5HI5ylYDY/ObXB5s452pDMlMHv9RhLcnDEws7GE2iNRV2nj70RJWs56dfYnmWidNVRb2QkVGZrfpaa0kkYPFrRAOm5nV3Ribe2EOQ3HS2QokSebgKMWSv0wmlcdmkplejaIGbo0dEElXotcUqXKbCUSLSDqRqUU/lIsI5TzfuZ/h/DEH0xtJlrZC6CQtb41rWdo4oLnWgSgbWZ5fxWq1UirnWN44pM5nx6JXk4mWmFyJsBspkysIvP72TT706d/6MUeRn57MBvi/gacVRVkCEAShBfgroP/9TP7ZYvMDECUR+9lT7P/JX2BOZTD19ZC5dZ+6rk4Mp/qIjEzQ9fMfp7CzTzIew+714jx/htCt+zR96DnUhSLZSJS2Fz+IoBJof+Ic6UgM67kBwkPjNH3gSUSzCV08zvbMDK5yEYBcNkvjyePoXE6qBBXIMkomg9x7jNT4NMbqaqRSCfOZUxTzeUr3h6nv6iKvFGn+yEvkFldJ53K4ujoQfB5ymzu46+txP30R+dE4poHjJMdmMA8cp/p4D+VyCdO5fiJvxPEvreDzuVFrtdRcPI/KU0FiY4uK+joSoTAHQ2M0f/QlQKDVbkPIF9AfP0YqFMZdV4O9r5tiJkPgwSNcPg/WzjZ0JtPjuzL11egmLSiiFndfD+VgCKmjhVazCRUKhv5ucokkHYbHQm9ZtQpbrAKxq4PE+gYNl86j1+nQ1FRiNJsRVALpeIyD6Xncl8+Ty+fp+OjL5Lf20J/sJXj9Dk293VBbiXY1h6+1GfOZU0T9BxhqqxCqKviFnl8lt7PExOQMg40wu6mirkJDW50Tiw66akUezEepr7Rw+ZjMW+MxGmvdXGjTsHGQZ9Zu5pnjRqY2RYo+I7UVItGImsmFHYSSnWiywOkuLx31dnwONTuhPC6HmScGTEQSBVq9IgvrB1S5LVzp9XJ/IUlLg5fBFpmhpQSnu300+gy0VorEEw3UOjWUymYkr5kWj0IuV+DyiVoqTKBVPe6TdhAro1cnGJ7dZ6xC5FynlZ3DJC0+id2jJIfBGO11do43yKTSdupdavRahWnBRJXHxoU2DYrio1hSuNwlk0hX0FCpYy+Y4ZOXHLzyKMpLAzYuDbSiKGWe7NKTzVRi1ms4f8KE1WKkkMvR26hnYTuJz21ioEnDW2MSHqfA+W4Hrw9HaWtw89KAnTdGY3zsopPxzTI91bDjN3Ch204uX2J4cZEaTw1loUBTdSPJTBF/KMP/+olO/DHIZJLU+hxc6dFxdybCyxfrsJhgfj3DxFKYpso6PjRo5tsPozx55ifQGw0QVD81G0ja/7LQACiKsiwIgvb9Tv6p+RV+VCSGJuj5/D9EnUjif+s65tP95LIZYvOL6J12tG4n6XgMg06PsaeDvXdvYWqsR3Y4WL56k/juPiqjEf/ENGWzCUN/N5GhMUSNBkd3B4nFZZw11bS8/DwOrxcZgd7P/wNyi2vE1zdReT2o8gUEnYxGlhHcLqKra5TNRnJHISK3H2A9fxr/xgaCVovGZCSVTCIWClScPsnaa++ASo33g8+Qml5Ao9ehkSSKWg3pnT1KopZ8PEW5XMZms2KyWsmubWMZPIm9u5PSxjZyqQx2K8HJKSovnyc6NkVubhFj7zFS6TRHQ2NYjrVRceUi6clZCuEoTR+4glmvJzk8gbGvm3QqRXR4nNoXPsDe1RvoG+tQ3E723rmBue8Y+UKBfCRGfGgcfVc75UwGdSxBxZMXycwvIRwc4ezvIR2JcXD9HmJdFavX72BwuvANnEDI5RFSKSSvm1QqSfDWfeynjpOJxsmvbmAZPEHJbGDv9XeoevZpTDo9Pn+Y/nNn0HhqePPV7xCMJBhoMfDdhyH6W8zsBFJEkiXiOS0vnXGzsJvFbNQx0Cwzs5VjblfhmZNO/uLdHXrqJc51WhhaTGAwWWitr0CjlehrdfPy2QqW9nIUCiW6Gp0sbYRIxWOcbpEY3ygy0N1IjcdMJi8giSImqcjV0SAmvcxHzlcwu5Hg9mSQM+0GgmkVdpPEsyes3J9Lcms6xjP9NraCBdaOBDprROLxJIcxLb/9qU4Kip5QLMvlXgcTm0W2Qyp+6Zl68mU1wytpPnHJx72pQ94cCXOlx0hZUXhtNM7lbj2VthK/98oqH73gIpnTYDIaUakEXjxl4e3xBKKoxqgt8O7YASdbzJh1Zb5++4AGt8CVPgt35pKkCiIfu+jhW/eOaK2WaPZqeDAXosajZ7DdyGsPD2mqNGDSqUmkctyaSfLrL9YxvJpneS/LyTYnI0sJ7BaZGofC/cldznaY8Tr1RFIqojmJRo+W/WCWdEHNQLuNvQiIehtf+PU+UnmBP3pzG59TxKDT/djjx2OJAfF92d8DRgVB+BNBEJ54z/6Ix/o274ufZTY/gK3NLdLJBIb5RQwuB1vXbmFx2DlYXEFQb1B7qo/M9DwIKraXlqhTqwmtrKKTJQq7ezgqPRirfeQyGWIHQTQrG9hSGdbvD1Hd04Vqao6duQW8ZQVz0srG5AyyzYpufpl4NkP24QjOs4NsDg1jaawnHY4iyxLJoxAV8QSzX/oK9f29RB4MkUskiIdC5K/dIRsOE0wmsWSzCCqBRCCAOKdi5fZ9Krs7EKbnUKlVzHz1FVpefh51TSVLX/4arrpaEtEoehVkp2Yp5/JsTEyhlUUQBKxuN0a7lY1HI0RLZdSSiFZQsTs5g15QkdvYJhEMEZlfouH0SRav3cLd3ETpzkOUcpngxhbGCjeZeILM2CS5VIbYQYDIvWFUisLG62/jam+jlMsRS6XRCAJKOMLR7BxqrQZxcg6dRs3Ozg6aqTlUWi35VBq5UGb6S1+h/eJ50sOTZEIhUpEYWq2GzelZak/0cXDtDuGtHQqZDIbRCZJ7+3ScPMW1b32H5blpKlxWTHotK/48m7tHmHRqLGYT/883FjjV7sCsr+DG+Ca//EwV2VyJr11boanKgkn7OJhPbOTJFkrsB6Lkcjl0sshyOodabCAQyyEUU/zO1zc51V1Hb5OFcCLH+mGRQChJMByns7WK3/36LOeON4CgZWhqk6fOWNmbfnyl4erwDuG0j3vj25zrq+fWnJpwNEEgkuLbD1SMLx5SX+Xk+oxMKJ4hlc6w7fbiNOR4YzjMi4Nu7j3cwmSQMek1eC0K10YDaEUd0USOgiLwzkSKqaU9nFYdN2dlDgMxiiWFVx6ECUUSHG92MLkSZiNQ4iiWY30nQEOVi5vDO2RLWgx6AyPzfiqcZnbDObb3g5QVAC+zawEqPQ40Kh1vPFjk7PE6DrRaJleCuBxW5jbzyCTJavSks0VSiQhjmzF6Omu4P7qCy64nm1M4CicYWkphMcksrO3QWuuktcrAH7y6wT/4YA2JVB7/QZBEtsTNWYl4CtI5hfmNKIL96CcQRX6qttE+x+N+a/+Yx3o5d3h8dvO++Nli8wOoratFNz+F1NZEqVTEvdeK1ufBkUqhVhR0nW0Us3mERyPUdR2jYDVjcruwnx8kOj2LrXWA5PQ8tp4udGcHQKOmZNTT/tLz5Pf3MfR00pbJUchmMQ2ewFcsohEljD2dJEcmOJpfwpXLYbBasdpsWE+foFwu06JSoRZF3PV1GJsbEex2JJsNdaGI8WQPkTuPcMsypUoP1poaigcBpK426tMp1KIWY3cn5XKZiqU1oksrCMUS+VwOy+l+JIuJXDiCvreT+Nom3mPtiFYLqnSWks2MplCkqqUZHHb0eplUPo/pKIj+RA+CSkXm2h1qnE7E1iZcG1u42lqQmuoJ33uEr7UZVYUTo82KfLyb/MMR+n7r1wnfuI/jyXPsLy8T3t0jlUoR39lDq5PRNzeCWo212oeht5Oj63ep7eygoNXS+omXya1vozvRgy8QQJBltJ1tWAsF9G435r5ufNEYhsZaJF8l+tEJilotproqosur9J89Q31rC3Ihhk3rQZ8bpdKh4bCvHpdJoKteYttvQ9SKjG/kCITi3JqOUu1Q09FQQVOVGbOURa1Sc7pFh0olkMu70UlqkpkCxWKBM81acoUSrx9qEFQCkijQ02BgeE2Hy1QgXWGiymultxpUNKAS1CRTGT79gUbSBehv1LO+XyYUsXHhmJVMXsBr1zHQIpLOOjjTaUOjlZAkA2UEnuyS+HZCT2OVg1qXindHgxwEI8xvSlzsr2M/lOFks443hlIY9TJXunXcEStJpdOcaRWZmC/R3+qit0HHq4+s/MJTVbwxmqKQzzG9GuLZUy66G2VeHY5jt3i40K5lfc9FX5MFl1lApTSgl3hcLVaqIpYq8kyvjnDMjcdUolgq0FLv5FSLAa26yMGhmSqHGlFV5tpoknwpgay2kswItDd6aHMVcV+o4ShRpLfbyAsXmlAELaeaNcytaplbD+G1eYgn00yuJVCp4DCco7nOxqVjMnvBEvUeH/uREl6P6ycSR35attHe6xzwO+/Z3xrhJ1Kh8RPkxIkTyujo6N88EPjO22/x9XyU4LU7lO0WTLW1ZKbnkXQ61B0tZGbmKabS2C9fID0yTjFfQGptoJzOUj44wjR4gnwyyeGNu7hO9FLa3qdYKGA5f5rExhaSSqAcilJw2ikFAshWC8n9AxxnThJ7MIb5zEli94eRTEbKNguqZIp0IoWlq4Pwo2H0Ph85/wElFJwXzhC5P4TGakZf5SM1vwzlMtaLZ4gtrxGdmaf2pWeIzS5iqq8hub2HodJDYWOHTCpFSVFArcI5eIJ8OsPmq29RfbIffXsLO6+8huv0SeRKD6Gbd9GbTOhO9BIdGiPuP6Di0hkUf5CiWoVWkoltb1NMZai4dI7YxAyKVoPRaiW+tY0KAcvZ06QnptDIEqa+LjLhKPm1jcdtPcoKuWwWY1szsYUVLD2dFBeWyWcyqH0VyLJMcHkNgyRjPT/A4fU7qDwV6EyPlTf37j+k6WMfRmPQk3w4gspsQpXJkc7ncPT3oDHqidx6gM5swllS+PinP01y6g5PXezni7/zBZKxME/2WpjcfHx58vmTZm4vFCgXszRUqEkVRHYCKa70Wrg3n+AgkuPF0w7mdkpY5AI6SeT2dJiBDhsmscSX3t5ioLuap3rNjC5H8dh1TKylGJrZ5tLJeq4ct/LGSJxcociHTtu4NxNici3Gb7xYz8OlODVuA5PraV4asPDn7/o52WIlEM2Rz2VoqbFS5xZ55UEUi0lHpTmPSiPjj5ZIpAsIShmfS2Ju9ZBArEhvo4lwLM/kWoQzXV7Oddn49r0jehqNhCNJro8f8k8+1srCXgG33cB+uMATHRJLuyluTobxOo1c7jYxu5Wiym1kbidPMZ/jUo+F23MZ8vkcz52wcH0uRzqd5+VBG4vbCabXIlzqdTG+WaZQLPHigIVv3A1CKcsLp928Nhqn1qHCaNCzGynj1Bcw6iWy2QwTKxFePOOhWBL44mvr9NSbWfKnUKPmH75Qx9Juhq2DNI2VEpmSjMdSJpJWsxdIcaHLwvXpJC8NWLg+HkTvG+A3fvv//FvFC0EQxhRFOfHfE2sAejprlatf+Rfva6yn93P/Q3/rR4UgCF9XFOVjgiDM8H3UPhVF6X4/7/mRZTaCIPwp8DwQUBTl2Hu+fwP8Go81rAH+N0VR3nzv2b8AfhUoAf9YUZR33vN/APg9QA38saIoX3jPXw98FbAD48AvKIqS/2F+h1w+T/z+CNl8nv1rt/Gd6CcfDpNJJKnXatgYHqWiuZHM3AKxSBSb142x0sPyl7+Oqa2F9I3bGHR6DldXcHgqWBsdw1zhJn3jDrqGehJrm0gWM+mtHbYfDdN29jT5bJbpL/4Z9oYGVEPjFIsFoqtreJuaCG5ucrS7h1EnoZZlDqamcVb62J2bRa/Xk89k2Jmdw9veRmR3D6VUQjLo0QgCUf8+FVPziHqZyNg0WkkiUthl/9Ew1mofxgo3exNTGHQ6SoJAJhIjdnBA9uiIwOYWrrpacoEjVCoVW3ML1AkqZK3I+vYO5ulFYoEAol6Psb4Onaxja2kV28wCBlFk7vZd2s+fpZBOEz0IIOlk1h8N4+tsRzsxCwIs3rxLfV8Pu3ML2OrqEHM5rCePE759D32lh1AgQPKdeZxtTaRicZKFIqVVF9qGWrbfuErDwADlZJJ8Kk1wbBJZpWJ/YQHZaCKXzmCucJE5OKScyZDMpNldWeWgws3Gb/3PfOrFi7z17n02giXkYh61WmB86YgneuxMr0UYmz0CVBi1djaOUkRiGa6rtciSxMbOHmNuG+vbR6g1ahqrrCysHyCqi9RViMiSFv9RlDdHQafTMXRvn85mD2a9zPZ+mDfLah5MrdNS4+bOQo5gNEcqk2NoOUUJmT9/Ywmv08zbExoWV/0YJQGv28bVR+sgmtk4yiOoBKYXt5C76/jOjTkunGhkbG4Hg6ylWDSzd5Sgvc5FX7MFvaRhZT9NrUvLvZkQi+uHWA0qzrRbGVqKMbYcI1uWuDOxitMqo1Z5SaaL+I+iVHtsfOPOAZUVVjpkhcXVPVRqFcVijm1/glyxzIjNwKPJDTxOE29PaCmXFabXw9RU2lnd9JPKlhAooZe1PJjYwWqzI2o1XB/d5Vx/A3PLO7gdJi53qTBo1MyshTEZTVhMBkSVgNthQFCVCcQUrk8EUASJ+9O7WK1tbPnD+E0GPjRooLtW5LWROHaThtHFMNF0mbmJuR9meHifCPD3oKz5b+B/eu/z+f+Rl/wot9H+HPhPwJf/mv93FUX5v77XIQhCB/AJoBOoBK69V1YHj2u6r/BY5nREEITvvidR+u/fe9dXBUH4Io8Xqj/4YX4BSRSxnh9AWFkjG09gbW4gvQwmmw25r5vWUglBo0bubGHt/kNy0SgqjYZEMIhdbMfx5AVS/gPqyqdQ3E7q+46TS6exnT9D7jBAMJUmGgxibWmi9dkraHUy9uZ6diemsdgsmE4exyaJJKfnUVVXYojHEPU6MrE49oE+DGYTqVCEmnNnkawW5J4uMrE4jo42BLMZdSKB/uRxArcfUH3hHBqvC7Xdxvqdh5gqvVRcGKQuk0Wl0WA6fYLQyhqGk70c3X1Ex2c+RWl7l3y5TMtLz1HK5jB0tpG4eRdfSzNyfzfR8WmczU3oezoJvel/nOX5vCRGJ6lsqEfX30N0aYWq06cQnHbEWJzq9jaMp0/QBI9v/B8/RmR6nsbLF5AcNmrVatSiRD6VIrG0ws7EFPUmI7KoxdnTheXsKfbeuUk5n0PS6yjn8qCAtrYKrU7CC5h8HvSN9WjUGkr5PFmL4XE5t8OGwVJLPhTBUVON++wAxqEJBJWWvp427t64yv5Riqn1NFv+IKlWM1UuPS11LgSVlrY6HcMLW9RWWXn+hImro0Faa+2caVETj6lY30+iqtTxmx/pYMVfJJjM8I9erueN0QQf6DehEmB6sUxvrZZ8sZpyWeHZ43py+VqsJpEnOmW+O2zicy85md8tca5JZH3HxtljVma3svzLX+5mbK1AR6WCcLGZcLzA0ydMfOtBmroqB8dr1TywGznXJqGTm1CKWXobjchaFbGMZqfwKwAAIABJREFUgMUg8mghyqefrmErCCdadXgcZvbCRRwWkXM9HkqKyNPHdCgKGA0yZ1vUvPKwzPHWSp47YeQPvhtkdtmPiBmDXofZJPP8aSdvjYgks0V66rTEM/Wk0jkudOhIZPKsbZs4025iN5hDKSs8fdyCoIJYsoIKM6wlC/R21nKuTWJ2RaJUyLF9qCKbL+Iw6zjVqmdxJ8NnnmtkYrNIQSlit6h5fsDC3GYSz+VGREnFfCrNtj+K2yZTUmBkZpNar43nT1ewH0tx8eyPP2l4XCDwvgu2/k7yPQqfv6Eoyj/73mfvdYL+Z//trP+WH9lm4nsaB+H3Ofwl4KuKouQURdngsbrcqfdsVVGU9feylq8CLwmPmxxdBr753vwv8bgj6Q+d6OwCYkmh5VM/R3R8CmOtD6mlifTKOmVRJBOPUy6WaRocwF5fi1hTRfulCxCOUUymyC1v4D5/GiEURaWTMQ30k56cQa70YLFZqGxqRJvOYOvppByMkJ1fpvdzv4LGZCLyYJjU7CKp/QC5+RUcl8+hNRiwPXGO6OgUy/cfYWyqx97dQXxtg8D4JL4PPElya4fs9i7aCjd5/yFGnQ5HzzEyyxuEb96n7Rc/gd3ppJjJIXo9FGWJ7EEAZ2cHyfEZDHo9OpuFxOERSjyJqbGebCBIPpVGZ7eRzmTIxhJogKorT3B4d4iqgX7clV5KmTQGbwUqn5fsvh/hKIT79AnCU3MYq3wUJJF8OIpKlika9ERmFtCWFex93ew/GMHUe+zxpUxJQhJF+j77y+h0MhabnRxl0ocBbNWVmOtq0KrVZPf8dH7mUyQmpjkaHsd+shclGCa6uIzUUIt0rJ3M+jZGnxdNNkdqz4/B68Fz5SKrX/kmjW3tzOwl+N//9b/nYquKTz1ZRa6k4flzLQTiKjx2GYfNSHetiD8q0NVeTZtPx/h6nrLGwIunXdyaiWO1WKnz2YhlVPjsWhKpDJIkIYtqnu0zcnMmzd2ZMJ9+ysfw6mMZZb06xzujAU40G0imMsysxWjyiliNEsFohm/eC/KpyxW8PXzAYLsZk05DMpPn1kySU80Gmrxq/t/vbNDsUXG2Tcd//M46v/R0NVNbOUxSmaf7bdyejVMslTndIjOxniOSFal2ykQSBe4vZDjeINHiFbg6GqLSpiWXzRJLFTGbdCRTab79MMIzx01Y9WV2DpP0tFRwrMkFqHn6pIfzHSbeGo3gtMl8/LyDN0eiGGWBD5+x8fpwlAeLWfqazfzltT2u9Jr48KCV10divDMa4qUBB3ObcawmiWwmy9JukovHK6hwu+hrcRBIyfzTT7Yyu1VArRFxWTSUC0kQ1Ay2SkxvZtkMCZxqNbNxkOdYcyW/8HQd2UKR/aMk//znj9HgM7O8m6LBa8JuNf8oQsTfiCCo35f9PeDK9/E9+34n/yQKBP6RIAi/CIwC/4uiKBHABzz6njG77/kAdv6afwBwAFFFUYrfZ/wPje3tLQ5HJvF1tBG9O8ze0hI2n5dyLsDiwyGaPvwSglHH9qtv0vCxlzCp1QSu3qKs1aLoJab/5C+x11ajXVplY2QMe3cHumCIdDqF/6++ibunk/l3btBy4SzpyTm2ZudQqdW4bGYIxbAMniBw8y67C0t0XbxA+M4j9lZWyWazWKxWyoU8yV0/hCOIksja7XvYTEYktZrAxgZWt5PNsQlUkkRFscjGxBRmtxvd4hrxXAZlZALfC8+gEwR2XnkNsaaK7fvDOGurQaMlvL1LKZdD1uko5LMsfvmvaProhxDNRla/+k1qBk+Rm10ksLyMyWzkcHcf3WGA6g89h04QiNy6T7Zconz7PofrG+SzWQwuB7tXr+M43o2YzbF07Tqdly6QnV4gHY6QnFlApVIzO3wDZ101uj0/S9dvU3duEMlkZvFr36b9kz+HxeUgdm8YWRIpFYsUdFoOhidQqVWUMlnkVIqcyURqdZ2jlVVsjfUERidJhyI46mooJ5IU0mm6TvSzvbRIcm2Yld04qYKWmZVDqt0mDDoV/+k7AT583kehJPDGg3VkjZpMtYONvSOgjEZdzd2xHc701TO/eoQkqclk02zuxymXFVSqGvSSisOjMJm8woUuGxYxxkGsjFVX5uFMAIPJjtVs5CvvzvPsxWNcm8lyEEwRjCZ4e1RFqVRmbqfI2kGRSCTKYSTL2+MiRp2Gw0iSRNJIJJ4lEEowvFZgdG6H3/5EGwAvnLLwha8s8eRxB4+WouglhbFVOAiEyWSLPFzWIQgqrg5vYzc30t8k89VbB1Q5tKQyAjsHYbwOPW3VBr56y89gu41CWc2DST959BSLBa4/WmWwu5K9A4GlrTA7fi1HIRuHRwmyhSLUuNjYDTHisKB+rwPy1PIhGq2ete0goaiMLKoZz8l87Lye5e0Iv/9ampNtDh4u57k1tsHFk828OZYkX1Qxu7JLuehidOGA/g4fN2a1jM1tcrzNg6QxcX1ojZoKM9cnHh/O3xnd4Olz7aT1wR92iHgf/P3vjSYIwud4LCvdIAjC9Pc8MvFY3+Z98eNebP4A+Lc8PmT6tzy+kforPC6j++sofP/MS/kB478vgiB8FvgsQE1Nzfv+Z2tqarF4PYjH2tDJMo25LFjN2Foace3tE1tYolwsET04JDaziAIc7e4h6vX4nnkSVSyFrtJDHgGj24mQy6MVtRTjj88WtE4H3qZGZJcDubGO6mSKQqmI1mgktntA4u3rmFubqLGYUVe6cVRVko3H0VkslKsqaXvxg5RCUeTeLjIPh3HU1SB3thGdnqPzUx9HOTpCI8tYvB5MZ05SXSyitprQWq2QShDY2MA2PYdKpSJ+FMJmNuHpaMVoNiN1tlJVKKBYTai1Wrwnujn8vT8kubVN6uAABQVdcwOCIFCbSiGaTVR3tDD/pb9C/fZ17L5KVoZH6Xz5BUoqgfa6WjKb21jODeD/wz9DvbyGsdJLw7NPo6BQMhkwOexYz/z/7L1nkBz5eeb5y3JZvqqrq9p7b9ANoA0adoDB+OEYUqIokeJKtxt3t6vT3Zq4D6f7cKfdW+1KWqNbKk4nURIlUkdPjh8OBgNg4BqmgUZ77315X5VVWZVZeR8avFBsxA1BiaQUCj4RGZWV+f9nVlVE5Zv5vM/7vMPoDAaaSyomlxNdfQ0VrS3YamsoKAqO8nLS+37ic4vE1tYxmi3UedyU9XSRWFqn8sQAOx9cIZpOU9/ejKu1kar9NvQuB6m5BfQ6He7TQ2QDYWrPn+Fgdxdj0s8Xvvh5QlNv0ugzIRqN2Cx6Bpr0/Nv/J8jkapyuBhutdT7sVpEXjll4Uzn0KjPrcrQ3uLnQa0ZvaKJYPEyAX3rkoqgoPH/0sLbDoJr5eDLE/RWJmKSxtBngs+fr6Gz0UOGERErm/EA95TZo9OrQVDe1PjP15dDbWENrtcjlR3GSUgmHxcinBm0s7aRpqnLS1+rm2nSGf/KpVkSTjqklPd+7cUB3cxmlkkYmI3F9JsnGfoSacivPHitjJ+zEYjZzutPE6EKKZ040U1RKLO5k2NqPMtDWhCJoXByswWHVcX0qTDKdo76qjn6rnmzWR3OFgfZqM7FkHcOdTvQ6aK33kJXhdIeRy1NWCkWVziqNTNrFUIsRh0XHG/dUTvS3ohTz/Pevt7HmL5JMpRlfijKxYcMfyVIsljjabGXtQOJETyW9dUYaKuzcmElQW+nhF85WsB2UuNDnJCUV+ZXnOtiJqAy1WcgrbYCOC0cs3JqJ85uf7WE3plFd6X3i//5PDj9Z6bMgCFtAmsPctqJp2pAgCB7gO0ATsAV87vFN/E8K3wQuAb8L/NZf257WNO1J2aufrhpNEIQm4P0fCgT+//Y9FgegadrvPt53GfjXj4f+a03TXni8/Yeyjt/jUGRQpWmaIgjCqb8+7pPw46rRvpWLE716E8/TZ8ktrqIvFsnrBZyNdSSn50ED85EehGAYzVuGkEiSDUUx1FRi1ukJPpzAWlmB5/Qw8TsP0fQCrrYWdN5y5Jl59EYjOSlLPpulcmQQo9tF+OotVFXFardRLCqUXzhN/PodSmYTjs42EnNLKOks1S8/w+6HH6NXVcpPDpOXclAoQDCC4+QgkY9HsThslLxlGCQZJZXCcWqY2INxIktrNH32NTIPp3CeOkF+Zg4lKyEYDBSKBdRSCe+pExgsZmJ3x5DlAq7OVoRkmmI0juvMMJFroxSNeipPD5OcWyKxs0/DixdJzi4S39rG19uFzmhC9YdwnjtJ9iBAemmNsuZ6YmsbKFKO2ldeJH73AUo2i/fps8iLK8hKEWdHK9LcMoosU/7MOeI37qEBzrMjSA8ncJwcJH3/EZrVisXjJrmyjrG5Hmk/gKeqkmw0jqOrleTDKcqfPnsYaAoq9sE+Ih+PkstksXW14xgf58//z9/CZDLy5f/yH4nHorw44ODmQgGtmOd0r4Mb83kGmw1EMjpUtcDYQpRfe7aWsY0SckHh4hGRR+t5VE1PXZnG3FaG3mY3WSmP3WrCbioxs1tCkXOc6nZyZSaL2aCSllQ+e87Hd24ESWYKvDhSyd35KNGkzH/zfB12i4Hf+foyx7sqsdusDLeJXJtK0ldvJFM0shWQeGHAztujYfrby+mpN/En7+/yqZOVLO/l6Ki1cG8py/PHHXz4MMRAh4+VA5mXjlu5syxTUvL4ozKnj5TTVGHkxoKMWSdT47Ox7lcQdHChR+T9B3HSksJTR+ysBkCSMjx9rJwPJ9K4zQp9zXZW/QqhpMrrI06uzspIUo5njx3WE71/18+vvdDI1ZksUq7Ii4NOvnp5l5dG6uisNTK6VCCTU6h0KLicNpYPitS5VYxGExvBAp8acnJtJkezt0RM0lPpVLg9E+NMn5fNUImkpPDasIOp9RTjK0m+cLGOqzMZXh128tFUlhcHHLx1N0LP4PP88n/7ROmF/w9/WzXa8b5W7eO3fu+JxnraP/cjz/U42Axpmhb5a9v+AxDTNO33BEH4LaDsv86t/CQhCEIFYP7he03Tdp5k3s/0yUYQhOq/lmz6DDD3eP1d4JuCIPwBhwKBduABh08w7Y+VZ/scigi+oGmaJgjCdeCzHOZxfh145yf9eWVZJnH7HvmSysJXvk7t+dOokTB6QUcklUZJpEkn4lSbTATWNlDkAu7WJvRobL93iY4Tw8QO/Oh85QTvPiAbDpGPJbCKIvpAmMDqGqVSiZq2VrYWVnBVVpJjj2w2SyYaxWgwYjSbKV69hZrJEl0J0mowYhPNbCwu45peIBuPIwUjOMrLMVR62bkxSs2FM2Sn5ylKEqGtTRp7e/FvbJHPZWkymoiubqAz6CmubqCpKtN/9GXaXnkR1Wohu7SK0WknsrGJu6wMRQOr2cLe1Axl5R52p2cxOu1kb9xBKKmEFtbweDxYdXo2NjapnF/BZBFJHfjxVFQQ3vdT1tdD4PptbGYz+5NT6DSVvCxTTKcJ3RpFX9IIbm1jeeTAv7qKwShiFkX2FpcQrVYs47NkEnGS4QiyoqDqBRLvf0TluZOk9/3sfHgVo9FIvdNJeGYej8OB1Swy/Wd/RVVvN8ndffbvP8Ld3ox2f4JMNEGhmMdhNCDodHx4bYy8XOTO1B42g8SkvYg/mMdpt7IXTCNn0/zx20meHunkIJghnsxya7HA3al1PnOunlLJxJ0ZPyOdbqY2dUwsBPCW2emqtfBgrUhGkvmFUy4iCR2/981Fnh9pwGiyMLm0xehSGcl0Fp1BRFOL9DZY+P7NKKPzUeSiQDSeRinVYjOBUQ9Oh5XWWhPv3o8QiEiMb4gs7USpqChnPy5zEEywsO9FEEx86XvzPDXcxswerO7EGWh30+JT+YvLu1R5LOgNZqZX/VT6yphaiyDJJTxuG40ojE6sU1/l5opSztpuHFUtMbNrIxRNsbUXIy+rZPIKKxsZBtsdjC2E+cLFWpKZAotr+3Q3HXrD1fmshJMFrs8k2fWn0Qsl7s0rhGNZptaTyEU7M6sRXj1Vgc9p4k8/2EFRVNTGSu5OLTHQU8O9JR2rWyGWNgWGunzsxAys7CRwOp3cm96ivtLFh1MGRJOVveAu4+sFKmwKX7m0y69cqOEgmqPMoefy9dEfO9j87fEzKep8HbjweP1rwA2eMGn/40AQhFc5rLGpAUJAI7DIobDrR+KnKX3+Foc/gFcQhD3gt4ELgiAc45Dy2gL+KYCmafOCIHwXWAAU4Dc1TVMfH+d/BC5zKH3+C03Tfqhf/F+AbwuC8DvAJPCVn/R3EEURz/kzmANBSvkCqeU1cskEVk85tc8/TfLOQ9xVFTjPDKNqGtlIlOoLZ0nNL+FtasQy0E+3WURRVOwjA0Su30aoqMA6fBxBp8OdSmJ2ucglk7S9+jw6TYepqR5XKk1lYwOJZILExhaNn3mZ8PQcbr2AqacDvcWMbnYOsasNXyKB89OvkLgzhrGygkwshpzN4T3aSz4Wp7K5GeuJ4zQc72f7jXcwH+ulUilS0OsxNtVTLKmU19ait1sI3biLnE5T09uJWy5gbKrF5C0nen+CytYWDM31iOsb6PUGai6cIReNIadSWI71En80Te8vfRo1HEXnLcdW7sF9boTNL30Zo9NB7QsXSa9t0PvLn0b1h9DpdWC3433qJNEbd6nsaMV+YgCXQU9h34+lr5eaZAZFVTB0tCAmE1TYbJSdO0Vud5/lt9/DUVeLuaoSb3MzDl85uWiMxr5eLMePkFxZp/WZC5CVMBoMFAoFKk6fpJjOUi6KyKk0tnCU3/yd32X39iU+9+mn2V0ao5iN01prZmw5BYDNWo5Kge7WSp7pM/F2RuR4TyMvHDOzue9k/UAiGJfJZPOkCwKfOe0lni7gtgpMrsa5OX5Aa72Pt+8W0VGgraGcc30erk0n6W6poLdORzZXSV+9gWBGTzIt8z9/roMb8zIvDdnYDWa52CciGvT80dvrGAx6EgkHWwcJ6ipdpDN5upqruHjExOWJOBeOV9NRq0evK1H2XCeJnEZvlcKCx4aUK7AZLLDtT/BLT9WwF0zz8slaPE6BC73l/MdvLxNNpvFa3HQ0VdJcbaXKWcJlrSUr67nYJ/KDBwqKovLamSpS2QJ/+p7E6FyMQDjJ3XkLLTV2FEVheSeOXMiTL2iEIgkM+mp+8/Umbs7nqXIW6WzsZD2o4nUI7BxE2A6XE0wUsRoNVFR7eGXYQUmrJ1/QONYsMrFQwGKxMthqRSmVGH1k4vljNiS5Dr1O4LmjVlLZAgcBN00VOlZ3S/jDKa5Mmqkv13EQ0XjuwtM/6UvEj8Zju5onhFcQhL9Ou/yppml/+l+N0YCPBEHQgC8/3l/5w5t4TdP8j588fhr4HeAkcFXTtOOCIDwNfP5JJ/+8qPMT8PaHl/jzpWmEeAr7QD/R66OYRBNaqYSuvhZDqUQ6EkFnMOD0lFFyOshvbKMrge34EaSZBQw6gXRWwt3fi7q6jtBcjxqOoxoNmAXIbO5hrvBg7+8hcu02qiBQfnKQ1NgEYnkZYlcbsRt3KaJR/ex5krfHKNVUYBJFiMTRKQq2E8fJpzLsvPch3sFjEI7iOn0C6dEMQmMdWjRGNhCimM9jMplwnx7GIIqsf+O7+Pp6kHJ5ctt7VAwPgNXMzg8+ousf/yqxj29jH+qjtHNAYHUNk9FE3Wsvkg9HULb2KWSzFFQV99FeSgdB7Md6iT2cJL3vR6yuRInEqHr6LLlACHlzl1wmTeUzT5Fa3aAYCOF7+gxbb/6A2mfOo4ki6t4BSixOyW7DUlWBuh/AfOwIsY9HsbicGLvbKK5vkctksXd3kp9fwn3uJNmHU2TjMVytzWQScVztbWRnFnCdPUn2IIi0vILgsGMxmZCjccounCG5sU1XrsCv/bN/yo2330IILtPj3CaVkbk9E+Wp41XMbGQxGjQG213sB9NUlYss+zWSyRQGvZ4L/S4+nknz7FEHjzaLiHoFq9VKLFMikZKQCyqvDLu4MXd4939vMY3RqOdoo5FgSsdQm5nv3Y7icVm5eMTExzMp9sISv/ZMFRv+DF+7tMU//8U27i2kUPVmLvQe0l+ZrMyrJ1y8cz9GY5UdfUnC6zKzG9dxvtfCtbk86Uye10dc3JxNsh1I84WLtbw/nkZPieMtIpsRgWQiyXNDPj6aSHLxqJMHawrZfBFVUXhhwMnNBRlZVnj9pJNLkxKqUmSow4lDLDG3qxCI5Xhx0MXvf2OJf/HZNu4uSvQ2Wln2lzDrZFqqraz7s4j6EgUVIhkdJbWEyWTktREX1yajlEoqFS6RbEFPtbtEWjZgMSqkZAPBuMJz/RbeHkthMgo4zQI9jXbGV+IMtlpY3C1gt4n01hn5waMMglbk9dMVXJ/LkcvlUBSVl4Y9vPcgwavDLsK287z+q//yx7pe/K1ptP527eb7X3qisa7GTz0JjVajadrB44ByBfifgHc1TXP/tTFxTdPK/qaf+RPOPf44RzQNHNc0rSQIwgNN0048yfyf29V8Ana2tgjdG6eiuYnsg0mUXJ7w3h4Wl4v0/CLe9lYsJpGtBxN0njxBKRhhffQeVe2tSGMyuryMrdxD+alhQh99jLuzDau3nMTSOkU5j6Gqio3JCTpPn0J6NEMxnyceiuB0u9iYmqF18DiFB5PojUZ2p2cRjAaM5R6idx/S8cXPEVvbwux2Ebo3jkXTSOztI9rsgEb6g48oP9aPxeshubCCaDKRiEVJHvix2ayk8jkSwSC21iYKmSzJcBhrwA9qiXw6jTy7gMliZu4vvknHiSGkaIKS3UZmfgm9qpEM+EnHk1grfGy+e4n64QGkyTnI5kgGQ7hUFSmdpnxlA51OIJ2TkBNJItNzKLkc0c0tLHXVRPf28K5uYKipQo3F0dttOI8eYft7b6Po9ZRns8iZDJGDAxoNBvxLS7gqKjA67ChNdWy9+R4Wr5e92XkMJhNFvY6lb71B9eAxctPz6IpFtsenqOxqY3t5g5rudpJ3xshnJZZCUe59dBWT2cb127dIt3kpFgrMrYexiAa2AmlURaWk6alwaLx5O8jLJzxc30wRiMsUikVsJoH/9O0l+lpdoDPij0SRCwqKpmHS67izYsNus/D1K5t4XRacVj235w0cb7Vxd0lCLhaZXtpBUWsplQzs7Ee5u+TGoNNQtRKj82kezPt5+alu5vdUbo+vM9RTzcx2nt1AAqvFhNNq4S8urfH8YAVXx1PcX4giigbeRcNgMLJ1kOTmYgWJVA5/KA5aOTazHn+8gNmk55UTbv6vd3awmCCVL2FAY9Rm5e7kJq0N5VyZMXFnYoO6ShfFYpGBNiuhZImConFtWqJUUrk5m8DldPHBWIBMNkdLjZPx5QhtdWW8OlLG6FKBV4dN/O9fncVhNqITNPR6kTsTawx01xBJZNl32vnsWRsg8vF0kmxORS2ZqfXAx+P7/OOX27mzlMEqWnHZjfzg7gon+2sJJi1YLEYmZvep8LqZW92jUNBornHy238xzVBvLVen0/hafvbeaMJPmEbTNO3g8WtIEIS3OCwPCf4wRSEIQjWHFNdPAwlBEOwceqJ9QxCEEIdM1BPh58HmE1BbX09lVwf2gUM3Bvn6beo7OlArvRSSKTxdHZhqqrDt7KBvbsTscdNj0CMlkriOH2H38seEN7doA/yLK5jMZvTpLBsPJ7H5PDSPDNBz/imKch7rYD+pq7ep7+lCrSzHWeHDMXQUg9VCan0T2+4eFaeGyW3vkQ6FSd8fP3QJCIZoevk5stu7HPmVX6SQSmNpbWbiD/+Esupq8pEYu8tLGEURq8+DtbUJU1sTBpORjnIPekVFsloQrVbKW5pI7fvxNDdgPdpDfHaRitZmLEf7aDWJlKp96JIpbMd6ycXimOwOZKsFQyKBva8bnU6HdPUWzf1HkHN5LC4Xpt5O9EYD7ngK1WbDe2aE4M17+JoasbY20/n8s+jyeUo6geX7D6jp6EBOJskmk1R0dOA+dwrl+igOnxfL8SPYwmHi+/sod+6T2tsnE43SfXqYLruFfDSBd3iI0Mw8RocdS2c7pVKJ9mKRol5HV1MjaiqN88wIwZujGE4PYXO7qGlsZP5yJa+ecHB3Ps6/+NwRtiMlbHY7ol7hWKsZRVF543qUaKYcn8eG21POZ065kWSF+Z0MdVVuBtudfDyVIBCVMBtLCHoz57sNmIx6grFyYqks4Z04JUHgqR6Rrjo7oYSIu7ma549aeOtenI5mH6e7zLxzT+LiQCU+lwG9rhKHGcosKp9/vpN1f47hNgv5Yj01LoUlfwkBgaYqO2UOE5GMRpnbzgvH7HzwMElrvZdn+0TekSz43CInO21shops7+9yedpHOJJCLhQ43VfHbiiL1Wrn6R4jRl0LsVSes51GCnIDkqzw8pCL7ZDM7Mo+PpeIsdzN//qr3Xw0k+N8j4msZKekuXhp0ME3rvpZ3Y3ynqBjZtmPVqrnVJeHmKTnhQEne2EJOV9JV6OLnQOVG5N71Hgt6HR6YhmF1a0wH+kVVFWjpd6LP5phdz+JRgm3rZrG6jKOt3torTLxgwcxWht9nOsWOQiXYdAJvDjgQMoX6Wooo7/RyI7h78Ab7XHztJ/IoQTBBug0TUs/Xn8e+D84zHn/OofCqZ9K/voxXgdywL8CfhVwPT7/E+HnNNon4O0PL/FHH12ibGSA5NwC9uYmEjPzGBAOqZjpOVQph1hdRXFnF+tAP2ogiNhQy8Gla3hbW1AtIiYE1FQGJZ+jJIrYG2pJzC0hdrSgLyqopRKiXk8xFEPOSaCB69xJpIkpHMPHSdweg7oaRJMBaWUT++BRIjdG8TQ3UEymcZ49QereOO4zJ8iMz5AJR7D0dSNkJYrJNLbqCuTtvcM2Ak+dJDo6RjIQovH1l4jcukdBKVL77Hmi1+9gMptRfB5Euw1lcxfn6SHSY5OHppjHekkuryIHI9irKjG3NDD75a9R2d2J9+Qgick5XD0dJGfmMVttmI92k33zIOCzAAAgAElEQVQ0i7G9CTUUo6DXYbFZUXf9KNU+wg/GaX7lBdIr60jBCBVHuknML6PZrDhbmpBTKYxKiVImS04ngNGAwyyS3NkDnUBZZzv6Si/7H17DN3gMc6WP8NVbWDweCpqGzVdOOhDE0d3J5tvv0/HFXya5so7VYae4e4Dt5CA8mKLVZuZcVxXy5iXmdxVeGrTz1r0oZXaR870W3rqf5GSHiZ2wSkE1kMopdNXoyBUNBGMS/c027q8WeKbPwsO1PKc6zfzeN5f5rS90MrqQJyUVeLrfxUFY4tpkmJpKN6+PuLj8KMmZHjurexn8cZneZg/BcAqv20Qka2C4VeQPvr/GP/+FVm7Mpomlinz+gpdossBffbSLr9zOYIeHzhoDD1YlQsnSYzVYHpuhQCCW42i7j2A4gd2iQ9WZ6W849FIrCQKCKvNUfxnj60U+NeTk/bE4iqoh6lXSuSKvjPgwmwTeeZDCahZ5qsvIx3M5umv1xHN6grE85U6RkQ4zi9tp4pKGoDfij0hUlRmo9VqZ2pCwiyU6as08WpfR6eC5fju3lw5dsl874eLtezFKCLw04ODyZIbuWiPBFGTyKq0VAtmigZ1AhtdGynhvPAuAzagw1OnixkyKRq8e0WylWMgysZbmM2dr2ApkGZuP8Osv1DO3JWE0iZS1XfyZ02gDRzu125f+5InG2msvfuK5BEFoAd56/NYAfFPTtH8nCEI58F2gAdgBfunHkSQ/KQRB+FfA9zRN2/ubzP+HYUf6U0IuL6OaTSQeTGAU9OSkHOlEAv/6OtLYBOaSxvbYOMm5BVLxBJtvvgeCgDyzSGBljbjfjxoMs3T5GtGdXQIrGwTnF5HWttAEgYVvfJf42gbZrR2WPviI4O4u0WCIwOYW2WCYvFwg8NENbAP9OBrqWH//MvlcjuL8MsHNLWJbO6QzGTa/9w66mirS0/MsXL+J0elAS6bZvzuG2e3EWFONbDajFAuEJ2fQayDFouQmZ7HUV5Pe3Se5ukFoc4vt2Tk0SWLvyk1sR3vIBkPsLiywv7iE9GgGXTzF8rUb7I2Nk11cQWfQYxQEdi9dQ2fQkQ+F2Z2eJRmLIq1ukS3IBK/fwdLRiruthciDCXS1VTjr61AzEtGxCYRkhuDyCsVoDKvXw8HkFMWtXQyJFJujowTW1zErRXY+vokSSxBcXSeyvokaiSFPLRBcXkPZ3ic/OY+qquwtLGI16Ak+msY/PUvi/jiZWJzcxCzFgwDR6VkEUSQXCpM2G1l49AjRLHJ9OkmDR+CduyHW92JMLB5wZUZGyuX547dXafTqiCYlLKKRlmob9+dD+KMSilKkXJT4g+8uE45n+Hg2TzKdY3RZ4dbkNpG4xKNNlUBWZD+UpljI88btICYD3JiJE8wYmV2LcRAv4bCLvH8/yEi7GYNBh16v59ZsgnBKYXHDz9hylvVgke1AHIfdQjit8cFYgCqXjr4GPXcWMxg0mXhWY3Y9wlZYwWi28Paon839DNfmCsyu+onG0mRl+L/fXEWv13NtNk++qLG6HUQvWtnYizOxpXJvtYheUHk4s8mVqRTBaIpvXt0iGC9yc3yLeFblyoyMPy3y3u1NckU92bzCzYkD9mMlpJzM+GKI7ZgenU5gfs3Po60Se8EkG9shrs/nMZn0rG0FubmQJ57K8/0bW+gE2PdHWQuUGGgWsRgUvj8a4dmjdipsMvfnAyzsKbhdNt66vc1OpMDKfpHlzTC351McxMEfzXJnRSWrmhmbD3Hj9v0f/af/iePQruZJlh+Fx04qRx8vvZqm/bvH26Oapj2jaVr749efeKB5DCdwWRCE24Ig/KYgCJU/zuSfB5tPgMUs4u5sY2dqmnQ0il7TcDQ3UVZTg+3kIKZjR2g7PYKzpYmyIz3kEkk0q4Wi3ULf53+Rsvo6TM0NVPd2UzbQR0VbM1WtLThODeM+fRJfYyPe/iNUXjxPWWU1voGjlPm82F1O1GQSTdMIrKwjr28RG5+kVNKoeuEiBbOJvi/+Ena3C9/pYaK7+8iZDNaejsOq+4Z6xLpq8uk08Y0tcvOLCMkEaw8eUcpIuM6eoPLEEIaWBuSigpTJYnE4qOvuorazA0dtDclwmPTiKqWsjKe6Gl99PdbBflS3i5qjfZT19SAoKt6+buxDR0n4/UQ3tjFWV1HT04WjogJTQz15uUBkd5fIjTuk7j4ktLmNsnvA3ruXyGWzlI0MIysqdSeHMVRXkctk8LW2YD7eh66jhbpjx6hqa0M8egRnZSViZys1PZ3UnhxCX+HF0NFMTW83xkov5sE+RKuVpr5erIP92MpcNA8cR2800vqpF9FVevFeOHPY62VqGikSxVzS6BkeIScXWNgMsh7I8OKgm44GHy11Xp7tM/GZU+VUljuIZTTC8Sx3JjcYW5HIFwqoaom9UJaNgyxOm4lfOO3DIBT5/HMtVDsVzg60UFddxjN9IkPNAk8PN1DpsTO1EqKoFHl5uJySItPbUce5HivRZI5wXOLuUoZ7KzkisTTDnU7sFiP/w2faQWegvlzPr73cjddp4nyPyG5Y5upEGH/KyLs31wnEcuSLGn1tVVzoFTHrCugEeGXEw3P9Zp4+0UptpZPOaj0dzRW8NGBnsEmH1WxgqL+FbE6mrbGCUx1GznSKWEUDrY0+XjvpodJjpae1hpcH7Zzoa8CoF3iuX0SvSbQ3lHGu04DNYmK4r4nTnSIOs55jPQ2c67agAV2N5fQ3GuioFWmsKefpHhPFQoH+rnpeGnDgcVk4dbyNM91W4pkiEwv7jC7JHMRL7IdSTG8VWAvINNWWc6bLgs+ap6O+jJE2Eae5xG98uhOLaOCZfgtWi4kznUYu9JrxuO30H+n62V9EBEDQPdny9xyapv0bTdN6OexpUwPcFATh6pPO/zmN9gl4+8NL/PGNqzg620lOTOM+d4rEvYeYGusRBYH0wQGuviPs/eBDagaOYqivprC5i5pM4zp7gsSte2iCgPXYETbf+oDm1z9FZm8fi8NOenmNsnMnkcanUO02LB4P8ZU1XDXViO3NRK/dQtDpsLQ2oldKZPYOMDfVodcElGAI95lh0jt7FEJR8tkMnrpa0tE4rvYWstMLiEd7KAVDFEJR7F1tpOeWKBn0iE11FNe2MbmcJHb3cFZWUMjl0FmtuLraKORliqEIJU2j6A9RcthxN9aTDYdx1FSRX9nA2NOOGksgWC2kZxdIhWM0ffplcgd+dOksgqKiFArYTw2TvHUP24njFBZXUW1WMvsHWKsrMGTz6BrqEZJJhEwG+/Ax0vcnQBAQ+zrJr22TD4TwPH2WxK37yAYdnuP95CamMYoijlODhK/dRikW8T1znsStu2hA2elhCrNL5NQijvZWsnNL6D1lhyaidx7gOHOCzL1xBJ2Arqme58sqGLlwnm//+/+NU7Ux1g8y2CwmCkWN9koIZwQ2/RI6vZ7OGj3pgkiVs8TYcobGKht7IYnnjzu5tSDTWlFiditLV6OHnnoTf/7hHs8PVRGM56j0WJlYS/PqiTJ+8CCOKJowCAoNPjO7UQUpm+G5IR9XprI822/m6nSOp3pExtckYmmZ7kY3vfUiVyaTBGJ5vnixgnfGkrx2wsmthQKZvMLLA1auzOYJR9IMdZfjMinE8wKrBzLP9Vu5vZjHYylS47NSXWbk3/7VEq+fqaWkF9kOyXzmVBl/+dEBIz1ealwaqwEFOa9QU2Fjei3O88edTGypuExFBB0oJR1uKyzsFXHaRBo8KlPrKXpavMxvpXnlRBk3FopkshIem56mGjv15XquzUjkZAVRpzDSaWd8UyOVyWA2GTjd7eDWfIZz3WaWD1TqPBoz2wpyoQiCjgt9diY2ilTYS9hsInPb+UNl23QWSS7y6gk3K3tp3rt7wD96tpbtqMBOKM8zR12knOd47WdNox3r1u589JdPNNZaeervZYuB/xqCIFQBv8Rh3aPjSVsM/P0Pp3+H2N7aJrq1jaoT8Fw4Q/reQ2w2K87mBqStbdI7e8hz80T395HDEZSldVauXicRiSCNz7B05x4CkJteIJ/JkBifQApFCN5/iN5hIx9LEEskCExMo2glAgsLqAWZwswiosXMweoqulCUnbv3Ca2ukd3cYvGtd1FKKtmZBXSJFCtXruFsqCOzvYshl0csc2FoqmXvg48Q62sxlbmZ+stvYLLZsNRWIwQjqKKRQjLNwfIqOp2ObCqFCTC6XVgrvGzfvENqZx+zw8HBw0dogRBGKcfM179LwWrGIIpk9/xkFlex2BxIqRTS5BxaKEZ8c5udpRWS6TT773+EY/gYAhAPh9m7OYrH62Xryg2MRiO6YIjlDz4kun9A/NYYy6P3SAaC5CbnWLlyDdxO1KKCUFdFMRbDZLNSqqlkc2aW1J2H5NIpwjt7JEYfEN09ILK7j7y8xvqjSZIHAYIT0yzff0A6J1GU8hSddvYuXcXU1UY6J2F6OINaKPDx2++wNDtFMC5jMxu4M7nD9LKf1ZDA5EoMp01kzx/lzdt+pILA/D6MTe2wsp3EbBb5N1+bRS4q7CZFJpZC7EQPqaXNvTjTmxJZqcDEahaHxcT6QY4yp8jU0i56k5Wvf7hKIJKit8nGVy/vcKJNxGwy0Fun40tvrKPTGXg45yeU1BhdKmAxi+wcRLk5n6exXOMrl3Y53myg2afxlQ/3MOtU5jcCbAfzaDoDUxsZWqtErGYDXrvClUdBNkIaV2ZkFFVlaV/iOx8tYdDr+cHDJPOrfnaCeR5ulHh/dINQMkeDV0+TT893boU43WXhaLubd+4coBc0NiMG7s/ssrQVZS1s5OFSBEXO8VSvja9f2aOtEkqqwu3pAx6txBlbjCLLOYwGHc8NevhwIkGFo8TGTpi1nTAlrYSoy/PBeIKhVhORRJ5QPM3yVhiP28bvf2Mej02jo97Gwr6M235IP0m5HAa9gem1BKsB2AsmGFtT+d6VZbLZHNdn4uz5f1oirR+BfyBPNoIg/IYgCDeAa4AX+O+eNNDAz9Von4j6hnosZjPS1g4lnZ5cOkNoZ5e6kkZwbQNnXQ2usyM0yjJiZQXWzlY8m9v4jvejmc00DA9hbKwns75JdVszvvOnUAtF5r/6TRRBwGG1ojMaEa0WCsUCer0BS1c7eqOR9Og9qo/2YepooVyWMYkm3KeGEAoKZk855q42EpMztF28QHJtE0EuEN7dO2zsJuhI+UNExyZwHjtCRUcbxvoaFE1j5fZ9ev/JF0mNT9H5i69jQCM5Nk4hmSYnSRQVhUK+gPfEAPlgGFd9HcbeDvSyjDcQRC0W2fnoOrlwlI5/9CuUVAXb7i7OM4dSe+X6KN6WZmKZNMGJGQz3zGC2oORlfM2N2AeOUL6+jrHKh6m2isqtbcr7ejBUV9FhESmkM9hPDmNbXMVUWUHk/kOMSolkKIz99n3CW9tUNjcdFtLevEdZRRXWkQF0dwQEBAydnRinZihrbsR1tBdNktFlJPLbOxjlIuH1dXx1NSR39jjS08vZF1/g2ne+xdlzJzlavYcggCTXYjCaeKrbxNX7cYoFmays8vyJOobaRdb209S/1ks8q9Lk1djc83Cux8rcVoaLg1W01RnxOXWk0lU0V1mpLYMvv7uGyWik7alqSmqRhupynu4VKRZbyOdlFrez7AaSjK97UZQMwXASo0HA54Bff7mLQLLE2S4zN6ajtDT4eKpH5PJ4Gn8kzdiaQiJVZH0vzsvDXl48200wkmZjP8/U/C5OSyur+xkC4SQ2i5GzXSKBuIzP0U5aBr3JSoPPxLEWCzZLF6WSxtluI7l8PVsHCd4aDYDOyK4/wb3VKhSlSDyRxiZCOifzzz7dxfhajkZPkRqvE02nZ+EA1g+SeMvsqJqe7tYqXh0pI54qEFhIsLLtx2BoYHxhj+ryDuqrPYiiyNRqkr2Iyn4wyv1VO/UeMz31OmwWMx1VJRar3ViMGh89CvNwIUyVz4lKFQXVwNLmHt0Xmwmnsrxwoo7+Zj2ReAW9LT4G20R2dD+tWsdPgsA/oHv6RuBfapo29TeZ/HMa7RPwQ2+02PU72Pt7yM4tYvOUURBNmM1mJCmLvqSit1iRd/fRTAbcx/qQF1bIZyXKnz6DND2PJhcxH+1GmllEzuZwDPSTejBB2fkzZMceYTrSSXFtCw0NrVDEeXKI7NgEtpEB4jfvYHI6kJMpXCPHyW3ukQ9H0AwGXI11mOpqkR5MkE9nELxl2Hw+Mrv7qEYDDp+X9PomvnMjyHNL5AFFVTG53eiSGWzHj5C8dQ+j1YxqsWCxWxEqypFWthB0AqVUButgP4WNbfKBIN5nnyJx6z4IAo6TQ8Ru3oW6aqS9A3xHepA2t3G0t1Jc26Qgy4i9nSgb21j6j5CbmUPwlWPSSiiSRD4YQbNZcbS2kFtYoqQqeC6cIR+JUgrF0AoFNA0MrY1IEzOYzGYKqoqzp4Po7CLmhlpEILW5jc5qxdHRRtYfJLm4TOPrL5GeX0IwGNGZTJTQMBQUpEAQU0cLxqyEmkpTaTLzy5/7HPGJGwwdbef2G3/Iul/m5SEn12YPm5g9d9zF3FYOTacnnlZ5bcTJuw9SvHbCyZXpHBkpx+snnLw9lsIiGnnxuJUr03ly+RwvDZXx/sMkr424uTqTx4BMb5OD6a08xpJENq9wqqcMr8vEd25HsZgEnA4rRxsMjK3KFIsKOr2RV4Yd3JyJ015jYWqriEiW/hYH12YlRKPAUz1W7q0WeOGojTfvJajxipzutPD9OwnsVhNOq57eOh2TmyqtlSWysoHVA/nwu4yl8LotJLJFKh0KNV4rj9YySHmFXzjjw6AXeONunJyUY6jTSSSjxx/JcaLNQFyCWFbPM/1WtgJZ3rsf4jdeaeTaXI5yq8Lyboanj/vYCqkcbzLyYEPhwhEbb9+L43FZ0Ws5yuwGgkkooaPeXSRd1HMQVWjx6ThIwDP9Vi5NSuh0OvL5Aq+POLk2J+O1q5hFMwdhieZqCxPrGfRovDjo5s5SjvM9Zv74vS2++Fwjm0EZo0nE3Xz+74BG69HuXP36E421+gb/3tNogiCcBdo1TftLQRB8gP1xW5gfiX8wIfengVwuR+zWPSx2G9Nf+Sus9bUUMhmEdAZ8HvKxOMuXrpDf3UcrqfjnFslOL7AxPkUiFkNTVdL7fgRvGYVogp3pGUJr60Rn57Ec6yM1PonOYcdgNhPe2uZgYYmiVST2cALZqCf88W12ZuZJHPjR11Sw8eYHGLweipJEYHae/M4+2bEJVsfGkTIZhLTE6g8+Irm3T/mxPpT9ADa7Hb3JRD6VQZ+Tcfd2svbuByiCRm56nsjePvuLy2iJJJujY2x8/z1svZ0UwlEM5WXkojGWLl9BEyD3cJrtmTkSgRDZh5NIeQn/x7eoHBkiO7eI0WRCjsVZHL2D3mpB3Q8QD4aY//OvIjsdmCt9pDa2ySaSKFqJg8kZSls7hHd2iewdIE/NI/hD7I6NszMzR2B5he0338Ps9bD2cIJcIkHeH8BcW8XB9duk0xlS0TgHU7MoKxsYM1lSkQjFpTUsOj2rl68g7x8gSnl2xh4QDwQwBCKsXLtBaHObhc1Nvv+l/8ynnjtJVaWXtUCR+gozczsFRifWURWVTF7lzmyAoVaR3jodb9z2c6RBpKiUmF/eJRBOcGe5SDCcYmUrwJ0lmUAojMFoxGTU8+KAg+szadwWjY4aI//l29NksxKixcqjpRDjmyrX5wv4gwm29uOsbMX40hvLqKrK2OwO8USC+e0MRxot3F3MYDWp1Pos/P43FygVcyxtHPCfvr2M3WLi4/kCB6EEc6tBHq7JZLISSxsBHswf8MadCKe7xMdtpjO4bTC1kSUSS3JnaguzIDG1kWUnJJHMKoctAdYL3F+RMQoaG/sxVg8KfHhnlXRWYjsh8q0ra2TzRa7OyofOCmmJ6wsFNnajjM5GONVTxp+9t8bxZiMWs4F8Ps+lB0EG2yzshZIUVB2BaJpbExusboXYSZj49uUlTEYDuwkd0VSOf//1RaLRCAeBGBUuHdNrccZnt7l0b59oRkNvsvJHb8xhNYu47Eb+w7cWaPQo7IRyRBI57i9E2Q2muTK2xa27D/8OriICCIYnW/6eQxCE3+bQJuyHhshG4MkiKT+n0T4RFosF74UzxG7fo+X5iyRDYfbHHtL7zAWUAz+eoz0YCkXM9bXkd/epOXoEW1cH3nwOJSORXVhhb3mVCkXB0d2Or6UZvcGIuaEWLRhk7fZdGvp7ycRjmC1myutqMVisLF39kIaTJyg7M4LF7ULL5ShIebLxGNmdPSw2O1WdHdiGjlHMZmm3iJTyMvYTA6gFGTkvI80usjU9i7OiHEHTOFhewWSzUeVxHRZ4dnWgNxnxZTIIgoD73AjOU4NM/uGfER0dY/vRJO3WU+RjUWr6+3A01mNpb6FVUVFLJewnh7AqCqmvfZP9H1wmfhDA196K58QA1S0tGD1lmJsbKKoK+Vgco06HtLlDeGsHi9tJzXMXSPlDmAeP4pNyh60VjnRhEE2URWLYyss4mFnA09aC/WgvddEYljI3tt4u9u8+BJ0eW3UVaiSOp8KHdWSA8I07VPcfQd9Yi8ntoiMvIwgC+q42aotFCpkM5qGjNORzlBQV91On2f3Lr/GnX30Lu8PB2OwuwpEa6st1fP6FbvxxBX8kRyojcX0uj8Gg5+F8AAQjM2sKRpOBo+0+umo0CkUP6VyJsz1WwhGBR3O7lNlNqJqejx9uMtBVjdNipb7aw0ink2A8R0N1Gee7TYhGgUKxGrdVIJYq4LIbudjvoFCoQdEEPJYScxtxxmYO6G1x4Q+bOH28mU+fdPPdW0ZE86EqDUBRqyiz6xloE4lLZXQ0ixRyKSZXYlyZiCArMLMcQGgv59iJCrbDTqoqy6mp0HN9YoWXh8sJxEsMdFXSXWNAoEQsbWSkp5Jz/S4sFjMWo0BjuUJ3k49jLTYavAbeuJtjoLeZ5/rNlIpO4pkiRUWhqJZ483YAm82C0WhibGYH9FamFnapO9tER72dbNGMwybyVI+InG/FZREY6TAztqygx0tzhZF37x7gtOo41e2mpa4cl9PK2S6RUqnExIKTk+1GBAzcnBDIySrxdJ4yp5mBdiceh4nLEyKepvq/oyvJP5h7+s8Ax4EJOHQzEATB8aSTf06jfQLe/vASfzJ2B3tFOcaaaiLXR9FZLVitFmzHeglfvXloX/9oBoPJiP3YEcJXb2GymNFcDiyeMpQ9P1IqjfvUIPLsEvaTg4ctBIwGnE1NqJEwYncn8vIquqKClMngHjpG7N4jFE3D+9QpMlu76PV6Du6OUXXuFKZSCWN9LfL8CoVEgvJnzhK6PYaay+M7PUTswQSes6fIPJigkMtRdmaE/PQcitFILpvG3daG4g8dtqge7Ce9vYvVU0ZqbRPNYsZWU422d4CSy4MGZRdOEXswiaWuimIoRr5QwNHcRGZlDUdHK8mpOax2G1IyRdmFsyjzS6TjcUxNDRizOcSeDqRH08hZCefRXtKLK0jJBObaGoqZDJ7GRoyVPrLjU7jPDJN5OEUhk8FU4aOQkbC3NCCvbSFLEuUXz5K4eY8CGq6hAeTZBYoWM7ZK3//L3psFx7FfZ56/rCWz9gWFfd93giBBguvdV21XV3aPw/ZMh9sOd0f3TM/048zDxHjCMQ8eR4ej3e6ZkGXJlmRJ1nL3y7vokpckSIIEQQDEjsK+FJZC7XtlZVZmzgOvYhQOWaLsa0l2+Iv4B1An/5l1ohB1DvLLc75Dee8A97lTZCemkQXw9nSSXlqlFE9S8/JzyIkUQjwOBRmjqQFle5cvjZylfLCG06xgid1nLwGqBq+MevlgMo7LZtBY5WJqI8+XLvq5HVSIxHO8dNrDwq5CJCWj6/BrF3zsHBdQdJGNI4XGCvC4bJgMlUReYC9aosJp0NNgYzNikMrrvDxs48OZAmZB5Xyfl5mtIhazmct9dj6azaPrBpd6rIyvlmjyQ74EkhWiWRMlucBgi514wYJLKqNoIm1VsHxgoBhmIvEcr5zzcWe1jFwqY5SLPDHo5fZSjvM9LiY3SrwwJHF7pYTTUmI5VODZIR8rhzpWi5mnB+28PZlB0wy+dMGPUtb47vUDnhmuYvlAp6SovDLq5q2JFDarxnBnBVNrWc51SWxFIRwvcLbLTlo2sX5Y4oujHj6aSeC2QYXXRShhUCyWKKkaXzzn5f3pLE6rxmivl+vzGexWaK1109Ng4U5QRtMFYqkCzw05CB6Crim01jqY30pzptPFblRnL1biQreN44zATqTEF844eW8mj1s0aG9wIdQ8+Uug0QaN8evff6y9jsDArzSN9iMdNEEQZgzDOP2JisG9f6lG+xQQ2t8nurCIEk9RWFhmb3YONZ/jaHuL3Ssf4ezqQC0W2X0wg+awkZlfQpBEduYXKSSSpOaXMSwWqp5/ksTt+6SjMSIf38bmdnE4v0Q5GiMXTZAcn8TSWM/KnXuUiyXU4KMu98jGJuWFFczxBCuvv429KsDGm1dIbu+SvjfF2s0xyqqKPLOA0+kgsrFBcX4Fpayx8Z3XsPX34L04SvjqTY73QpAvsj85g7YbYndqBjmbwSRJVAz0ou6EcIgiNWeGST54SNHQ2Z6ZJRmNIM8sIAkC83/9PZR8Acnv4+jmLeRMFmX/ELvbxdbDOcoWE8lbd8Ek4GysZ/PKhyDLyNPzrN+5SymbQdvaxWGzEd/awZrNczT5kNTBIWVZRnM5CX10nRw6O7MLGEUZqa6a5MMFEMA+2MfOWx/g6OsicPEcu2++g9jVjv9EPwcfj2FUeCkrCgVFIbuzR3pmnmIkSuIojDw9h+ngiO079witb5BdWGb7zj1WZudZDWe4+sEVogWRla1jVjaOuLlUAovEhxMhFraStFVqvHUvCZrCQJPEH/7lLMmcxuZujN2DOGMrCjlZYCVUpMJt4VSnh5n1DDdmk8VGf+IAACAASURBVNjMZfSyzMTCEQ7RxN5xgeZKE6mcwt5hhIXNGPlimZngMRd77ZhMJipdOqlsgeXdLPtHKd4eD9HfZOPqVJhLvTYuD3r51tU9euvN9DS62DkucGUywekOicX1Y0Y63VgtZg4iGRorBF4+U8G3Pz6kvc5JhceKS1R57VaYzhrIqVa2DxLsJC1MLx+wuHnMvdUSU4u7xJJZrs0XmVjX2NhLsLArc39+m/3jJNcWFKxWK1NLxxzEVUqlEuPBEue6JM522nj99hF9jXZOtlh5uF1CMNu40F/B0l4BkwCboWMi8TT3NxQ8ksLGYY4b82lMZgvj8/vsRFXemYhwf/GQ5gqdapfK//3tIB6pxIU+Dw82ipgsTqr8drbDBTrqH2mmff/aKppaYnztER14fynM7GaWvYPwLymSmB5z/crj+4Ig/DngEwTh3wLXgL943JP/hUb7KaiurKKyuQn3yT4EQcC/uEzF8BAVFhMLX/0mBmB2u9A1Dc0QcPf1Ii8s4a2toeLkIKGrN4kfHBJIpUklEmAYdH/+RWJzi1Q0NmAf7Cb8+hXkTBaT30PHyDBWrwfH8CCxmXkq2loQB7pxupxU7exRdeokxeMIlcMnECsDmASwWC3YR4aIB9dxV1fhPHsSUZYJf+WblA4PEUwWMtEINYP9VFw4S4/FhMnlpLK9FbVQ4GjsLg6rld2Zh1R3dmKanudofZ3Bvm66Lp4DwHb6BGWlTEsqjeTzojudJPYPabh8HvtgH4nbE1S3t1J1+RyxiSm2pmZwVwVoPH0SS1c7arFIz8svgCzjPHOSVHCNQEcbzoEeXBubeNqaUI/CiLpG8MFDOi5foOd/+A1IplC1Mpl4nKP1TXpsEvHtHZwuF6LdRjYSQ17fRHU5yWdyJFZWMRSV9OERFouFyk96dOxeD67zpxEEAdfWDu6eTvwDvdglEWfAj8cm4c138kRPmWy+GrcNuutMqIrAWsDNiyMBwgmZm3OH7JkEnjkZoKulirPtFtyOJopFmdOtZhRF59sfbHLpdAv3gjo7B1FcDisVHj9mi0RXWy13V1JML+7jc3Wiayb8Pg8NdolwPEsmU2BqvQBAJKmwvRflmRPtHKZM1FR5+WgmQUlVuTaXpZDLYpMsvHbrCI/bidliYesgydU5D1uhKJstAXbjMLeyR7W/l4xc4jCa5dYcRLO1aIKDpe1dXE6Jsmbw3Ok6mgMCJ7rrMQsa3fVmEifbUNUyl3pEMkWFo85K2uvslEoVSDYXLw47eH08watPtlAqq8yvH+P3OLmxZEfTzWRyMtfmC4CJG/eCDHTXc3XBjKbDnel1BrsbcdhELvfamFzJc3Cc5ndeqGdhK83p7mqGWiUq3XYeLEdYPygw1ObF7U5jMpm5v1ZkdTuMaDIhis3MBvdxODrYP8zR21ZNU62H0S478ZQPwzB48ZSXY1vtLz6I/Kip858BDMP4z4IgvABkgB7g/zAM4+rjnv8zaTRBEM4AT/CoY7TIo4Fn1/4RJRH+UfHz0mjfDG+jbu7hPnuSwsIKKCpFwNvRQmZmEd0k4D1/ltTte/ieuEDp4TyOs8MUF5Yx5BKKXHpE/dyawKgM4PS6yG/sYDvZT2ZhBafDgZxKE3jmMsWFZfKpNIHzI+QezOG+dJb8zDyK2YyrsZ7S1h5mUSQXi+M7P4K6so7q82I3CyS2dglcOocSXEdOJBF7OrHKJXS3A1MqS/EwjNTfhZAvUtgJYXM6KZnA1dZGYW8fye9Di8cpJpIIFT6K4Sh1T54nObtA4NwIifEH+C6OUpxZoGQScHd1UFwK4hwdQVlaRmisQ8gVKOzsUcrlqBwawNbeQvruFOWijPepiyTHxql89jLpO5P4njhHZmIa7Db0fAHfxbNEr90CQGysw4glUOUSJrcTh9+P5rRjSqYRyhq5WBzd58VZWUk+uIbn7DDacRQ5GsN9coDiyjq6XcLicGDWNHSPC5OsoCoKosOJFk9QlEt4ejoph485Wczz+7/5Au99+78iKwJPD9p4fTyBIMCpVjPJHKyHVT4z4mFqSyeTl/nsGTd/9voG/8uvd2I2CXwwk8Nn06j224hmyvgdGppg5zilU+FQsUsi66EUZU3g4qCHscU8LQETVRVOtg/T5AsKfq9EbcBDR62VNydS2Exl7JJAe72bxe0UWVnAKQk8O+Tl6lyWV0Z9fDSTYajDyWQwi1UUsVnKnGxzMrEm89yQg6mNEolcmVfP+7i9LGNo8qNJnAdZwrE8v/1sLTeXZJ4fcvD+dB6HzcrTAyKv3U3idTl4fsjGu5MZdMPgi6Nu3rmfQlE1+holFnfzXDpRTXOVlTfuJjELOpUeE06Hg63DHIpS4tcuVTG9kcPnsrMVURhpF5lcl3E6RKyCgUkv0tnoZCKY46XTft6dTCHoJX7tyTrevp+h1gstNQ4erBf4/BkP94J5DMFMQS7R0+gimSliskjcWzjk8xfqWN7JcrbHzcPNAoOtbpYPVC53W/lovsiJ0Zd+8TTaqRPG+PU3f/ZGwFHR9StNo/1D8XemXEEQ/o0gCDM8qjywA6s8kq6+DFwVBOEbgiA0/2Lc/OWgJBfJHUUo2axsf/9tLI31FDJZxNKjslzNYiKytY0aXEcMVLDy9W+RTKcorqyxcWuCaPgYqa+b2MQ0luoqvL1dZFY2sIhW9NABBzOzYDbhONHL/ntXEaoCVD55gfTkLGafG5PJRDGdxZIvIFUFKORyaIZBxdOXSI1PgtmMp6OV7H4Yk25gaAbr4xOUBBOOmhrU4wjKygb2ng6k3k4OPrqJomkU02kONjZwihKr33ud+Moq+nGE4PWbGAY4DYFCLEZp7xDfyEmityZw1NUhWCyEDw/J7h+gYWBUVrD3xjvYT/Thqq0mtbxKMnzM4eoGajRBYXqenYfzZDMZ8sF1zG0tbP3gHRwD3RTjCULLK8T39snkC+y+9QG+00MIVgve3i5USWRjcorkxjZkc5ijCQ7nF9ldXMLp97N9/SZGOILosLPwl99CUBScPi8Pv/xX6CUFS6FE8K33SR8dY3V70KNxjGgSqbEeo6RgAZLLq6ixFIsLi3w8NsOt+TSL6wd8MJNHNwy2QxHWI1Zeu7lNhdfOzI7OtfFlzCa4t6ZyHM8zvqoytqJyGM1wb/GYer+JvGJi41hgsFkinkxzez5KNKuRKhqshuLM7mgYusHVqUP24xoOu8jiToYLfQGWQzJvT6QYbpWQbBLj80esHekcxVW2QzGsZoPxoIzf/Wgqb0+jyF+8s0oynUXTVHRBoson0VNn4r+9ucnZLhsj7SLvTyVxSyoVTnhzbIuV7ShPDrj58yu7nOuwchArsnsQYXnzgDtBBZfdytzKDneCZeLpAmvbYa4vqdhsNhbXj4gWJGaDYdaPFD6cyZEvFFnaOEJH5L3xTY6iKVpqHHz/VpiiAtuHCXZCMb56ZZNTbRILa8d01Zq4NOhnMaThdjmx2ywMt1l5sHzEew9SFIsyY9MHyKUywy0WvnF1D5ek8t7tIOG4zHZUJymLvHNrg0JR5eGWDGYbfpeV8z1O/uKdNbLpJBtHMuVi5pdUjQaGYH6s9asKQRCygiBkfsLKCoKQedzr/DQazQlcMgyj+Hc4MMyj8c2PNX/6nyIsVhFndQA5Gud4e4fagyOSoX0URaHKZkMoa9R3dWE/OYCQTOFcW8fT3oajq4O2TI5SqYQRibF15x4DTz1B8cEs2Xic8E6G2tERavp7sdRWkVnf5nBlhUBtDfLBMVv3p2g5fRLLw0Vyx1ESZRWzxUouEiW1v0+LSaCsldmZmaXDbEK0Wthe3sZWV4O/ugpBEomO3eE4uIa3pgrpwSyaqpJLpmmy21F9XgKtLdiHB5BmZmn5/AuoapnBCi/Z7T2sQwM0W6xY2lsIvX+N8OoqXSYTZUWBsoamKuR2dihGYiQOD3HcuosJ2Ftc4vT/9PtUrW4hZ3L4n75ITSqD3elAamnm+O4Eif197NNzmAN+fLW1OP1+FI+TnQ+u4asKcLQcxCQIeE700V96NMjLPjKEXi7jPgrjrKmiUFLo/dIXoVRCbGinNpPF7PVga2umM5HC4nTgOTtM7VEYZ2MjpdABa7fv0nRiEHl6juDtO3RcvoRvsA8tuMHL//p36fOVCe/3YJUPeW7IyZt3ZU71t2K3FOlsquDZAQkwODyup7VapL1awPREG+lCic+f9ZJOi7TW1nN9LsXYTIiLpzq48iDNylaU4a5KLvfaSKRL1PS18MJJG6+Ny5zorOJMuxmz2ckHd1WmN3Js70coFFUafDUUZJ3B7iZeHHbwjqLSWOvnQvej8t6e1moSqRzddVZG+psoFEvMrhxSHfBwW7JgEQQy+RIfTkUQBJGppQO6WwI8dbKCnmYfqm5h+UAhkshxbS5Na6WZ6ko/9ZUOLvbauXK/SFtjFU/0momlbFT5bIx2WlEUgf0jH921BsLFdkDnpWEHsmLlqx8UuNxnI1dqpqzptFSbeed2DIdk4oXTAXTS/N5nm7k+G2V7/5jbK24CPoHrkxuc6Krj9rKArqpcOtVKjd9OW5WJouxB01TmQwpLm1FOdgR4+VI3sVSJp/tFyrqOprWAAXPBED63nXGbgN2s4XKKVHolvA6BsmHC7/f9jG/8PwaEf/I0mmEYj11x9tPwL9VoPwVvffgB347vk52eo2jouBsakAyD/OERtqEBCEfJxmK4hwYpzi7gf+oSxZl5jMY6zPkCpWwOOV9AtNmwOWxYmpspzi+hlUqYzAIVT10kMz6J68IZkrcnsAUqEKor0SMxjKKM2NVGcXULk6ZRzObwnDpBZnGFwIUzZCemKYsWPAO9pCdn0E1mBJOJwPkRisvrKKqCq7EONZHCcaKP6EdjWJpqcVb4Ke+HMQsm8rqKu7MddTuEnH5E5WmqRvLWPawuJ2ouh7ujFT2RJp/NIjXUP7rLaG5A295ByeSw93cjZHLkQ4c4Bns4Gr9P/ZOX0Mtl9KMIRlGmqCr4zwyTHn+A49QgHIZxDQ+QnZxFzmYpW8zYG2rJ74TwNzYgh4+pePoS+ekFylUBLPk8ud0QgWceUXBiwI/jRD/Jm+Pohk7FU5dIjI1jeN342luQ4wlMioqOCSOVxjrQg7q5i6DpOE6fIDMxhdVux3nqBF17x3zpt3+Tm3/9NczxRYqZCGe63czvqsgq6LrOcBMkCgL70SKX+tzcXCnhk2T6W7xkixpHaRM5WUfTDDySSqVXZCuqMdxq4SCmsxst8eo5L7eDJVKZHAImnhhw47KbubtawmGRyRZ0agMShykT2bzKK+d93FiS8Yolqv129uMauXyeU21Olo8MFNXgxWEHb91L8fLII2l+q9WKaC7TVOWkzm/w+q1DXh6t5zBewuOysbiT50sXfHw4W6DaqRDwOhhfiHKqO8DGYYGnh7zM7mgEHCUMQWTrIElPox1FtzHQLPL+VA6lXObV837+yxub/PfP1LOwW+Rku5N7yxnKZZVKr5X2Bi9FWWEllGew1U00pZBTLESSRbwuG+VSlpZ6P2v7RV694OfKgww1fpGLvQ7enEjx6jkvN+ZS7MeKPHfSRzxvIl+QsYsmbs4e828/14ailnmwqZItKHxh1Mf7DxLoOhiY+MKoh51wnjIi8ZxAlUujpIv4238JTZ2nhow7N997rL1OX/OvPI32t5o6K3mkjfbpNHUKgtAmCMKfCILwhiAI7/xo/UOd/qeAeDzGztsfIDU3kovE2Prhx8QPj8gUZVa/8wPK6Fgddha/+k2k/m4EQaCQzVJaWcNaX40cjRF+OIfNZOJwbpHD9z4kj07iOEJoZZX0zh6q007o/Wt4Tg0hR+MUF4M4B3oopDNsvf4eJkEgeHscBAF9/wipuorddz6k7LQhul3Mf+1b2LxeCokkid091LUt1m6PowsgNtZjKmvEr9+h4slzBAb6OB5/gKm2GlVVEDUDqcJPMZVGsNk4ujtJcnyCslJi9c44rv4exJZGymYTgtvB0g/eRCsWMIUOOFgMkopE0Xf3Cf7wGiVVoRyLk947ILm5TXpzm6PgKkfbO9ga61j5+t9g7+/GHqhAlmX2P76Fpa0Zqa+bzM4e5mSG0IOHFA/CZBWF+MQ0imilsLnDwttXEO0OlPkVNqemQS4hT88hZ7OP9NAWVhDdbg7uTqKFjpDyMsvv/RAtnSGRSBK9fhvXyQHkXI7ovUkcg30UsllS84uMjJ4hFj4muHvE8uoWbpedP/nuMtVOle29MLUeqK9ycJwxYbbacTutdNUYzG/lCHglBMFgbHqH9Z0jphf3uL8cJZlVSadSfO3dLRr8Bs+dcPDld/fQSlmSmTLrezGCBypySSOZKXKUsXC+3893rm5T4zF4ot/OX30YorvWzGjvI3tHNQy2OPh/39lgtFNCNKtcm4lyos1JSdHQlDzzwT1SGZnrD/b4s9c3+K1nm5jezJMtmemuF7nUZ+P+WhGXJDDS7ef9iWP6m2x856M1isUSDslEQdbYieoMNFm5PODh/fsRRLPGxGqBg0iS7VCMawsyR8cp5vcNqnwS91aL2J1OnjjhZWI5zmFcIZqzMDF/xF7CTFqR2NpPMru8x+k2K5rJzolmkc+NuHhnMkl9hUgyp/HBdJIL3Tbevx8lp5rZDydYDQuMzexza/aYcMZMMlPgdvCRdltwN8ZBOMnUZonWaiupbIHPnHZxc6lI8FCgr1Eili6xHdWo9ZRJZXK/hCgi/HPSRvvbTZ0in3JT51vA14B3Af3ndfCfMiwmC2azBd3pwl5VRW1rC54zJ0ltbKGl03j6uolNz1PX10N+cxfTYQStpBA9CiNVVWIyDGp7urAN9SMmEuR296jt78GiqFQ11iNgwlRSSe7uUb1eS3R7BwMBY8xEZH0Dd309zpGTDFjM5CIxxMFehEKR2Lsf4uzupJTK0DI0iOfcadSCjKWpEVNXO625LNm9Q0xyia0HD2kc7ENZXCNbLHK0vokzUMHOzCxNg/2Y7s9wGFzFVVND3fNPI7ldRG/cZvCZpyhEY+xfu4nk9RI4e5reZ5/CapewnxygPpfH6nRgG+rDNr9I5cVRMFuQXA9wBCqwVVdhUsuoqRQqArquUdg/gHgCu1VkZ34Sv8+PIsuUFAVxsJ9uA0y6TlVbMwt//R1aRkYQ62vpeuE5LJKEtaeTDlVFKRapePoimRtZGmpqsJ0cQNncpqqlCcfwAPnwMf7GRmz9PYjLQcITU1TOLiKKVrYfziHqBmYeKSIs+quxu50MtdWCq4nznXDnoYP9WIFUtsCdhTDRXB23pjboaKrgPdWDySQQTeQYWypR6zXR11GHaLXQEEhR1ERGupxUe2D7MMtBQkU14CCa4cKAn6ysUFfdzJluGzdm42SLOrv7YSxGFS9d6qHKq/M3H4dYD8Wp9DuYWi2TzBSYXktR4TYjWiyshGQsFhs3pkIUVAsuUUPVRXra63j1nAdZqeAPv7HMh1MJxh+GePFyHzeXSphMZt6/tUR/Ry1lw8xxIs12xMpgRxWSZOHKRIy7cwec7m/g7prKdiiBphnYLDojnXYiaQ89rXbOtJtYWfdypk2gWDJ46+Y+dVUuTJobTYeT7Q4kq5mN/TpqvdBZa+P9opvetmrG5uMcRvNctUto5TKzywdEqj0YmEhmcvidLTwz7OfDmQxnBtt5ekBibhWePdOIZFbpaangTLtIpVckX/Tid9u50C1y42GKg0iG6Z0qFtaOsVoFfjhrZnppl7Z6H82VAYTAL4vF+dVPJI+Jf9ymTkEQ7huGce4f5OKvEH5uGi0bJXnzLopeRpQkqp66QObeFObmBiyCifJ+GOf5EWI37uA7e4r0g4dYrRY0m4SrpQlZKWNSFfTDMEJLE0IiiaGoyJnMI/rn4zF0ScTd14VxGKGYSOG7fA754QJKsYj30jmKa+s4utpJ33+I4bCT3N6h6vQwkq6TOzxCcNhwtbWTWlhGL8nUvvAUhdABJlmhFE+ilUq4Rs+QHr+P/+mLyAsrqJk0UiCArb+L4sIKpXwB18kTmB12CjPzWAQB1WbFZrNTOAij6Dr+C2cpzc5j7etCP45SjiVRVBXPmSHknX3kWALf6OlHn09dDTZJJH0cQY0nqX3haXKTD/FePkfi3gMEjxu7zUYutE9Z0zEkkcDQAPnNbVyN9RTXthDMJjRFwffkRRI376BX+HDX16ED+l4IQdUoFIsEnrpE9u4kcqlE7fNPkrhxF5rrsEh29K1dpMFe1K1dhIZaEg9mqBsZRqjwErs6xn/83d9j5c5N/sffeo6//suvUkiEON/rYXxFxjA0zBYLLw/buTKVxdDhC6NulnZz3JiN8e+/0MbYQpqT7S42DnMsbqb4jadqubemUCiqfOGclx8+zNMSAIfDzsxGFqfDRlkp8tJIBWaTwFv3k7RXmcmXBA5jGVwuL2c6RD56mGa0200oKjPc4eThtoosF6hym6ircrOyl6NQVHjudCUHMRkEC5JZpaBaSGVkirJMQ6WTtGIimdN5YdjFuxMpRrrdPFjNMtgiogsiwVABSTSjKCqXeuyEkgLRpEx3k4tgSKaklHj1QoDX7yb44jkf1+aLlGSZi702wimdcLJMd6OD9SONYjHLcLuLSNZMR41AKKaxGy2jlst85rSLqW2dREbG0OGL59zsRYqkSyKbhwVaA2UiqTK62UlPvUC6aCWW1cnlZT5zxsu12RRFxeA3nqjk5mKBvnqB/YRONGPwzKDEDx/mEFG42P+IrnTZLVS7VQxBJJIs0VrnwlT7S2jqPD1s3Bl7vOpgp6f6V5pG+0U0df6pIAh/IAjCBUEQTv9o/cPc/qeBcrlM9P40JknkcGEJVVXZeeM97J1teFqbSc0tIQQqKCZT2FqbWfj6t8kmEhxvh4gsr6KZzbgbaskF1xEqK8hvbrH83keohQKqprH0F1/HWleD3edj5duvkU4m0QNetr/3JmJHC7aBLnbf+wixvhZMJuLhMEdzC6hljfzqOkJNJfF4gvDsEtnFJWwWM9HdPeS5ZcyJNBsf3yAbi2OyWJj/8tewBfwUtnZJHR4hVlVSSCaJ3hjHcaLvkVLB1Czxu5OI3R2s358msbKGXiyiKDJHK0FyeyHyZY3E3Qc4ujtIpBIUc3kktxtTQcbmdKLm8hRyWXZv3MJIpnFbRKI7u8Tv3KcoWkhMPcTu9eAf6CW3s4fN46HiwhkKoQNEjxv/qSGyCyuYbSK7cwuEt3Y4un0Xo66G9PIqJqeDcjxJZHuXg80tTNVV7L3zPq6hPqx11ey98yGe0WGc1dWEb9/FPtSP5POiWUzE7tyj5ZWXyW7uEP74DhVPXuC//dEfY5YzhI/jyJqIVbThc1lpqjBIZYvYTTI/uBXmYo+NwSYT99dltqPwm0/XcT+YQpJEqrxWLvT5iaSKTCyn2D1KEtw55sOHeQ7CCV4fC3GYBJfLzr2HG1T5TMztlHjzXoLnTzhQdDNv3FzlKF6is9ZEpcdMe52TwxTowqOH3Hq5hGaInB+oZP1Iw2yx8eqlGma2VDaPdfoaRdrrnKwfFMmVrTx3uor3JsOcbLGRzmT5g6/N43Hb2IuD3W7ltRu7pPMaHqnM1t4xfXVm/vT1VfbCWTBb+cH1LUJHcVySwZ9f2eHJATeabpBLJzAMDZMg8OH9A/qa7bRWW1HkPILZRkuti8NYno+mE7TViISOohweJ5BVg9WdGCda7Iy0W5jfVZjb1TjZKvFEn427yxnO91fgEMv8+VtBYjmDscl1sgWZ2yslHHY7y+sHvDsRJZ4qMr5S5FSbRKFQ4J2JNC8NO3nuVAVf/SDE+W6JnKyxHn5EpT11wsPKbobg+mM9Wvj08c+ERuMX0NR5AvjXwLP8/zSa8cnrvxOCIPwl8HkgYhjG4Ce2CuB7QCuwA/yGYRhJQRAE4E+BzwIF4N8YhjHzyTm/A/zvn1z2/zIM4xuf2EeAr/OoLPt94D8Zn3K1g2EYVI6cJL8YZPC3fx3tOM7GyhqejW3K0TjHO7ukYnF8g33Y62oJ1NRQcfY06tYuBgaZnT3yC8scrK3T01CHo62Vbrcbs82Grqo4IhEcrU1k55ep7eqk7onzyKk0Ox9eJ7C0it3lJLa2Rk19LYok4XK6qO/s5CAYJB2JIQQ3UNJZqpoaqXrmMuHx+1R1tmPtasPqcdOmqGAy4TgzTE08gVTphwofu2O3kbNZ6nt7WB2fwO33YbFY0MpljlaCyLEENr+H2uefQfJ5EeJJajraEO12zJLE3koQaewuaipL2TDIz8yz/PFN2kZP40x7sDXWExAEbCd6SAQ3qB0axNPciKZprLzxLh2jZ8iPjXO0to5ZFKnOZMmn08izS4BOPpkiEYkQaG3CarHiPnkC9ThK6ugYx+QM7t4efJWV2L0ecvkssa1tapqasGFwcBSmKriBpukU0xnKa1toFjP5/UNyiSSFhRVEh4Od6YeIAR+tgwMM9LcR2g9ze+IhLQETV2SVYqnM7mGchpoOFjb2qaqqQEDk5v11WhsrWDmq5N2xLZ69+IiiEihTKMhc6PchL+So8Nh4/qSbzSqBiRUrl/tEZtby9LT4Ec0if3N1meHeRm4vZXjupI/w2S6aAmYEo8x/ef2QCq+d5a0ol0+38fGCzFFcJnScpMLn4OaDTRprPBhUcX1ijeG+eq7OW9E0jY1QFMlqRqCWUknh+mwCq8XKSF89l7ot2CUzE8sqvW01XOx18MFkAZNgQi6pBDwOzvT4aa6yUtZqKSsq/c12rk+FuLskIVkEdo/zuB0q06sqmazMw40MuzEPedXE5u4BkihilSSmlvZx2kVaG6qwSRYerGXZ2Y+xXutD13VuT63T2VLFO/cfhZT13Th3VirRywoXTjRwstnEUbSWkx1ehttsjM3FaG+s4Il+N2/cPSaeKnLTY2fzMAmawdU5C2VV4SiW5t5qFTNLB1QGnNwK2rAIOvmCTKZ0/GmGH1xKoQAAIABJREFUh8fEpztiQBCEl3kUK83AVw3D+KNP7eI/A7+Ips4gMGQYhvLzOCYIwpNADvjmjyWbPwYShmH8kSAI/xvgNwzjfxUE4bPA/8yjZHMO+FPDMM59kpymgDM8SnDTwMgnCWoS+E/ABI+SzX81DOODn+XXz0ujfXXpITaTCVtXG5Ebd7A7nZQyOVxnT1LeO0Qpq9i8fnJb23gvjrL31rs0PvMUilLCIisUs1lSW7s0f+ElMncf4HvqIvLMPKosI1QHkGNxfHW16F43RjyJCqj5IlZJRD0MY64OYHc4yW7tUvH0ReS5ZTRBQFFVXN2d5BdXsPe2YxxFMIol3BfPkLp9Hz3gw+H3kV5Zxzk0SPk4ghqJ4j97CnlhmWKhiOZ24mlpJD+/gufUEKmph1gkG4au433yAsX5ZYT6WoREAlNDPeWdPfKpDJIAtr4uSCQpqSqOygCl7RAlpYSztho5HMX35Hlyk7OocgnfE+eJ376HKgg4G+ow5fI4T/RTmHpIMV9A93kphkLUPfUkZrtEdmIKqygCBo4zw6TuTGJua0LLZLGUVOR4gqoXnkSeXiBXknH3d5OZmsPc0kh6YYXGz7xAemIKXdfwX76AfBzBnE6TiyepfuoC8Zt3kTpbsShlzvuqEHNRmv1WhOO77MXKjHZY+WAmA0YZl2hQ4bKgm+2c7ZS4Ni8jyzKX+px8NJPG6bTx8mkXVybTKIrMC6d8BI90zn2yt6QZnGoxkyyY2Q4X+PwZNzeXFPJFBbWs84VRDwvbj2T+d2MGx4kCF/vcTATzaIZBtc/GUwN23p16RMfZ7RK7ERXRVMZmEzmKybidVp494SAYyqLoNqIZHVXJk8gofOliJQ82VS52S1xfLPLisJMPZ4sopRIWs4nBNhcLO3kMTeW5kx5uLOQ50+UmeKBwuVfkynSefC7LSF81XbVWri8U0TFRyOf43GiAK5NJ+hutrB8buCSD7kYn6/t5Ng8y1AXsnO12czdY4FKvxK2FNE11PkbaJd6fyZPLK7xy3s/YQhqrWafGZ2M/afDisJPxYBEEM5lMgcuDbu6syDzVLzG7XSSe0bGadc52u5jaVHBYVZqqnWzspxlqdbK4r1NUNMyCzok2N02VVt6ZzDB8/pfQ1Hn6lHHn9s3H2ut0+X7qewmCYAbWgBeAfeAB8FuGYSz/ff37NCEIwj3DMC78XccfJ+XOAT93gbphGLeAv60y8EXgG5/8/g3g1R+zf9N4hAke3abVAS8BVw3DSBiGkQSuAi9/csxjGMa9T+5mvvlj1/rUUMjnOb4/RWp3j/T4JBYDdubmMQydua98g3w0iilfYPPDj0iFw6jzS2SOY2i7IbTdfTY+vkkpEqXu+SfZe/8qzk8q1tLHUXK5HNZCkcPpOcrpLGbBRDkaRw9HsdntrL7/EXavB6lUZuGtKwiihcLGNltTM9gGeqg4N0J+dgGbTcJeGeBgZY294BrRG+Mch0Ik5peQaqsJXDrL/ns/xFpXg+/yeZJ3J9FtNkytjRzcmyS/vIbN5WLmK38FJhPbM7Oko1EyG1vohSKl5VVsPV1IbhfFaByHaKVUVsnNLWHv6cR/op/c8hoWUcQ7eoroyhq7Sytk705xuLpK4uiIwvwyTqeT8PwCZrVMMRYn9nABa2sz/vMjHI7fo/mzL6Csb5G8dQ/P+RHyJRnDbMZstWBqqmPzrfexKmXWb91GMwnoJQVVVXCIIvZABb6LZ1l7810kv4fj62PY66rxXhjl8MpHGMcRXMODeIYH2LvyEZ6BXqRABWsfXmN9aZG54C6vf+87iKYymbzCO5MpXjnn43y3k3BSYaTHh6qUePvuMd11Ap894+W1W2FiyTSFfJ4//ps1LKYyXoeJjx5mOddpY2k7w/35XY4jcQIekZ2IgsvxqBFzYnab7VAEtyjz7RvHzG8XOYhrvHtzBZsksnygkyuq7B3EWNoI862PDwg4FLbDeb765iKZvExRs7BzlGdqaZdwJMlxssR6GIZarGSyOSwWG587W8GX393lXKeIxWLCYSnzwwcRPJLK/GqYg2gGs6BTVmRE0Y5dstBWCf/5b+Y5iue4FdQIboTRdFjYyvHD6QSjXRJyIYfNZufhWgqLxcLXP9jEJomYrXa+9dEG08EojTVeJhYOKCllGnwGb9yJ8LlzVRTlEu9NRGmthJZqkf/zr+ZIZFXSuTJv3trh6cFH2nCHcRmfrcznzlUwtljALprYCKtcub2J0yHicdn5szeCbOwekc0Vee/uHsHdDOv7WTb3jtkORWmu0HgQjPPa2BEnWkQUVf20Q8Rj4Ed3Np+KNtoosGEYxtYn//x/l0ex81cFtp928HFotBogKAjCA6D0I6NhGK/8PZypMQzj6JPzjwRB+NHovAYg9GP79j+x/TT7/k+w/0QIgvDvgH8H0Nz8+KIHyXQKf3srVZdGEQSB6LXbNHR14XvyPLG9ENXPXMbQNBoREAywnz1FfTKFyeehorcTpVgkG4vj2wmR2NmlqraWwtomx5tb1J7oRRrsxz6/hNTdTmptg/D8EljM+AyNzheeAbMFW38PXcUiVp8Hc2M9aqlEdOwu/upKNF1je2GRFqsVu8+L1+PBe3kUy3KQ8OQcxx/fxuVykjoIU7u8CpJIeGsbezJFzbnTOLxeqp65TC58zIDrM5h0nTbpFBgGNpeLzZvjOLxupKk59LJGPLSPyWJGkmzk0inc0350XUMrl9meW6DFJuJobUYHnGdOUmExIx8d4z41QOzeNIH2NlTRilEus39nHMe5UWLZDHaXi8Sd++zNzBNobcIxswCFIqHNbVokkczBIZ7qAK7Tg/RgUM4XSTyYYXdyhvrebtTb98kpCjWtrXg62ti88kMEiwUPkEsmKBXzqMUi5aJMMrRPZWsLelrC11DPyIULJPZ3UaQE4USC8ZldOpoCvHvfIBROcRjNMbboQrSKTCzuoGgmpgWddK6AIAi8PFpFtMvBxmGBhc08ZrPBvXUPTQE7w31NOO0mxuaS7BzlUUslRHMjDTUeWhv8DDWZidyPEM8pnGqrIhSpp7HSynCrxFsTVpprWzjfZeYPv77EiVYvo+f8aIKNxoCV0S4781sqPkcDiazKn3x3hafPdzO2ohDcieO0WXDZ6gjHstxbKz2iSc127szv8dkLzVw41YIBHEZzrIey2KUcDlsd9V6R0/1N9DXaCLhVpJFmZFVn5yDF6lYKk9WBXBZY2w3x2y+0MdRhYWXTwVP9IiVFY3XbxUi3l51Imb72Oo7TOomSjf3jfT5ekBFFG7fmttH0Gp4/XclmdyPDHW56GiWmg8e8P5nA4XTyYG4H9BZiBSvroShoZX7nxSaqKtycbjXjtps5ijdQ4Ra52Gvj7lKMvUiZvhY36YJOQTHhdjnASDG7FqXSJ+KwPHaz+6eLx38eUykIwo/TLl8xDOMrP/b6J8XDX6XirZ9Kkz1OsvmDT8mRnwbhJ9iMv4f9J+KTP9hX4BGN9rhONdQ3IAQXKecLaLqBvSZAsayxf22MwOkhivtH5Ne28Fw4S25zm9LRMa7GWvLhY4SqClw11YiVAQrodH/uReTdA3wXRumSJBTg4OoN2r74GeT1LZyNDbSeA0GHsizjGx4kMTOPvrmLpbKCYiSKFo4w/B9/n9iNcax93dhTacTjCLaBHnxllUKxiFOWIZaiurUZ3xPnSS8Gabt8DktdLXIkSs+vv0J2MYjJ6cAkWlHyBZSVDfxPXyR9ZxKr1YpRWwNFmZbBPhRdR+xsJbu6QftLz1OORiml0ng6WzHX12KvqyZ39RaBxgac/d1o4w9o+txL5OaWsAkmhLpqlHQG0TBoeP5pCosrCCYTtd1d2IcHKE5M46uvw9nfTZfXgxyNI53so3R/htr+XnSPC3+pkpLdTuzuFP7hAXJbu3iqArSpOhanE/foKco3blP/r15h94136f+NL5FdDOLp76ajVKJQKCA1N1PeC9H1xc8+GhMdjdHw4tPMr65ypsbFM7/3u7z33S/z8uUeSiq8dMrJlQcS/+HzrbwxkeJSr4X/7rkuYlmdZwftjK96EC0G1+cyjPa4cTgE+joc2K0GDRUWlnayPDXo5t6aQmeDA8nuRFZK9NRbUfRqUrkC2xGN4b56tg4L3FtJ8cKwn/FgnkYfVHpFcnKZpd0i//5LfUxtFtCMDKNdDqbWc2gdNkIJE587E+CNeymGBxrxOUyMdFgwtCZKpRLd9RY+c7kLWdV5eVDkzlKazqYA8bzOs0M2rs0kuXzaw1HahKLqXOiWCIZynO1ys5/Q2YwofOm8i2vzMn0tTqxmg9EOEzfndPraqrCJZq7PJvjt5xqY286zHVb53Zcb+P5YhMF2P121Zu6tyjw7ILKz76UhYKWt0sBq6kTXBbIFhaF2JztRDb+zyGcuNGKzOcgWSvyHfzXE1Fqacx1mCsVqTCYT6XyZz52rYSFUJp7O8fmzHqbWc8SyOrLuotInYxPNWGwuPDYTkaRGS0Mlr16q44cP87RXBR73q/+pQnv8J8mxn0HZ/Vxx71cNP00bTQAwDGPsJ60f3/Nz4PgTCoxPfkY+se8DPz7ZqBE4/Bn2xp9g/9QRuHyOzNQs2cmHOPp78fV0El/bwNPeSnx6DmdLExZJxNffQ3ZhGcMqYmltYv6r30TTykgWC9vXx9AjccySlc0fvMF+cBVR18nsH6Juh8iFIxSXVnGdHqKQSSPYRI5nFyglkiy99gZaIoVkFTne3CI2dg/HYC/p8Unyy6u0/NorbH/vTaTONiovnmXz+29jG+xFtpqIzS4gWa1UnBth7+YtzA4Ja2UFUn8nofeu0v6lzxG+cRtnRwuCIFD2uSnk8rhaGtm5eYejnT1MwOI3vkMxl8NSU0kxlkCsrsY3PERqeZXI3Um8/b24zwyTmJzBHPCjRmOsjY1RyudR5BJr332TgkmgtLzG2sc3UcsqvpEhCvPLOFxOdLeDg2u3ECp8iPU1rH7rB8RDh0iCwPrb72PyuCjpGpJJwOpy4h/qp7i2+YkCtZ2ja2MUijLZiSnS0TjqQRhLWzORm+OUXU5sPh/B195CEiXM0QQ79yaJbW5RXttifmGB1eUg4w+W+eD+AbuHKZRChrfHw5xuE4lnZCyGzP/zxjqGoWMyinzr4yNGO61ohoVXz/u4sxjnjetBikWZ/P/H3nsGOZKed56/THhvCigAZVDem66uqvZuenr8DIcjiZIoamVWonZ1kvYibjf27nbvbvfDnqSN2DidtFqtKImiETkSyZkh2cOZHts93V1tq6uqy3tvgAIK3iWABJD3oVp3iovgsLWiyCWlf0RGApn5Zr4fkM+L53n+z/8pKrxxfZ3FzQizOxXGZjb55s1dEukseanI599aY2UjQCqT54MHAXx2qHNW2ImUcFi1HG/V8buvzuKzlpFyWYJJFQ0ePV21ai6PbDOzFqPGJvMHb6xh1hxK+qczBXYDMcYX9vnPb6zRUwtPH7Xy/sMUVr1CjVVhfqdAVlZTbamwFUiwvB2nzqXw+2+s8USPgeeOGrkxJ7FxIFApF3jr5iImdRFFUchJErGsmn9yqYYbsxImi5kXT7i4OZdD1JrQaHR848MVnDYDIwsyU0tBVneTSIUShUKB90bDvHLaQzYr8eUPApzqMJGTilydStFVb8BhlLly/4DjHTZeu7rE+l6ag0SRxmotf/KdDbRCEXVF4u5imrZaI9fHtgiGE1x5ECWcKPDH31qkIKXoqdfx+6+vYtHK3Jlc497CARu7MT4YD5HLJJhZWP37MBEfCwWooDzW9hj4bvbwRwIf59l8JAjCG8BlRVH+X/0zQRC0HIpx/hLwEYeMsMfFm4/G/cdH+8t/4/hvCYLwNQ7dwuSjMNt7wO8IguB4dN0zwL9RFCX2SATuJHAf+EXgD/8W83gs5KU8ubmFwxxLLIFKoyZVyCOq1Ei7+4Q2NtAaDQiZDIqiENraxCJJOAf6cNT40Pvr0bmdmEfHsAz3ozEaUeQSBqcDbVcrTWYjitPOyptv421ponz9NvtLqwgqFc2vvIiut4dyVkKs8ZLdDeBuasB98QzS5g6iRs3B4jJGg5FEOMT+7VF0JiOJYBjXzAIWjYb1BxO0HRsmu7CCXJSJbe+RDUXR63Tksxnk2WUia1u4a2rIReKYgOWNDUyTTgwOG05/PZbhAeryBRSdlviNO6yNj9OhPY2USmOwWFi8dZcORFRqNas3b9MyPIR0cIB/eBhzQx1qr5u9sYcYajyUsjkaL13EaNSjpLOs3LlLdXMz8WAIvd1GSVRhaGmmOniAodqFvrMF0+IKKo+b5I3bJAQBre6wI2VocwtRraWqrobg7BxdP/sKgkaLaXcPbVsTuYUVNu4+oO5oH6audiyuKvSDvYhqNb50BkQRw1A/9UWZvrZafurFM+zN38KikzFq4esfLOJ0WHDoFZ4ddrNzcOiVfOdujGAkw60lFxPzW6A00FVvZDto4yfPuBBFgcuKgMmg40ynjmiqjnyhxCunnIiiwF8UFPqbzGyES7SbtDxYiFFWmdgO7nFj3kmVSaCjycv0Vp5ktkx459CWqCs5LGYDp3ucJLMlDuJ7tPi81FXriKSMuBxmXhgy8n98YY7taJmNgzw7e1EiMS39nTW8/cE8T55sZTsiYzPr8ThNFMsVspLMe2MxfB4H00sBRJVIR209J480UihVuL2YZ20vTkUuAjXshhJI+QKC0EAuX2B2eQeT6MHvtdNZIyLLBZ456Uev17NxUEHUGLj2YANBZ0Wn1bK5F+Pbdy1YLWbWdgK899CCUhHYCCQZWXBzrKeOKpuBk50mSqUKC9sJVneTNPs9rGztcX3eicth4sJRL71+PcVSGbmiwunQkcgeEi/a64zEpCb0GoFLfQYyUonZjRTTu3vfbxPxWPg+cmQfAG2CIDQBe8Cngc983+7+d8fHtkL9rmw0QRD0wK8APw80AQkOE0Aq4H3gjxRFmfyuNxaEvwKeAFxAiMNw3LeBbwB+DgU8f/rRwiEA/wV4jkPq8z9VFGXs0X1+Bfi3j27724qifPHR8WH+P+rzO8C/eBzq899eG22H7MwSQpUTjSyTCR9gGRwgMz2Hyecmvx/G2tNJqVyiFIwgFPJk80WqhvuRZhYQG+spxxOIpTKyXMJc6yW/ukm+UEBlMWEwGMhFYwgaLZbOFgpL64hNdRTXtilbzRirnJT3wyj5AtruNsqBEKbudsLvfYTOaiWfzWE7MYj0cAbrmROkJ2cpHEQoO+2UAvvUvPQs8YlpzE0N5BeWsZ45TmJmntTaJvbuVjK7+1T3dqHzVRO5PYqUSFJ94SzFuSXypRKWzlZKa5tUZBltXxeJBw/R6fVYTw4TuTeOaDZiNBjIBYLklTLV/b3kZhYOw3Ij9ymUS9gH+8kvr1GWClhPHyd2fYSqi2eJ37yH2uFASWco5vM4nzhD7OYdbMODlBaXSEkSZVHA3d9DfmYRweNCiSWwHBsgdecBauNhd1DbsQGSt0epCOB+8hy5qVk0LY3IGzsoskwhk8E00Ev5IEpJymP0VZNPZ9Gp1XRUVLgFmWqDjCs3ysPNMuVSnl6/jqlNmReHLbz9IIaUr/CJkw7ee5ilUilj1IrUu1SE02q05HBY1ORkLXZDmQI6VnbSaNUCJ7psqAWFe8sFrIYKNS4jo4txOv1Weuq1vD2WRiWUUFGitc6G01hhPVxhN1ZGrUgIooa2eivzmwme7LcxtVXCZ6sQS8uU0dJVK7Kwp6AVipSVMqJKQ6ag4nyPkavTOVKZPH31amJ5NXIJ4hkZjVjmZKeV0cUEJzos3JzLMthqZGK9AEqFM90WFnaLlGSZtjoT9xaSGAw6TrRqub8sIcsyT/RZ+WgmgUknksoUONHl4N5yHkEQ+IlTDt6biHO8zYLNpOL3vrHCr73UyI3ZFBd7LYxvlikUioRiWX7x6VpGZpIEoll6GswoooZ0tojfY6LepeHqdI6KAt2+MiNzaToaq0hkD2ugXjlp51t34rx4zMaVsQQqocyJDgsLwTIqFMplmY5aI16Hhm/eTTJ09gVe+Sf/02O9+3+NvysbbWBwULl288ZjXVtlsX7PZz1i7v4+h3b4C4qi/PZ/69y+3xAE4aGiKEe/2/nvGkZTFCWvKMp/VRTlDNAAXAIGFUVpUBTl1z5uoXk0/ucURfEpiqJRFKVOUZQ/VxQlqijKJUVR2h7tY4+uVRRF+U1FUVoURen764Xm0bkvKIrS+mj74t84PqYoSu+jMb/1/a6xASiXy4Su3UICpO1dYmubRDa3qCyvsTX6ADkcRUBg/a33WL/8DmKth0w6g0GjRmMyki/LJManDvumxBOoSyW0HjeJXJbI+gaW+lqMvZ1o1BqqTg+z8LU3CG9vU1hep5jJsHX1BsV8gUpWQjQZ0NttyJEoB1dHcJw+Riq4j6Wx/tBjclexf/seKrcTy8lhNq9ex9DeSn5tE21RRuu0o7irKOwGUGWyaKwWlFCMmifPk5lfPixMNZuoffYiiftjKFYTzpNDSLMLoFaTrVSIjtyl+okz6FobSN59gK5SQW+1MP+dK8j5PFU9nWy/8yGqxjoCt++zv7FBZH2T0uomSx+NUFEgPbMAPi+rr76OaaAPQcoj6nUYervYe/sDLC2NqE0GMqk0JrUG74khAldvoGtpwNzkJyVJjP/+HyO4nBTicXRWC4nlVcoChFbXiYyOU0ikyIxNYT7STV7KodbqMDodCMkMYk5C56nG1tpEZnUDt9NJxexgfX4MnVphZXOH7UAKg1bkWJuOD6Zy6PUm1GKZy/eSHGlQk81kmFkNky6oiMbj3JwO0+QzsX1QYD4AKqVIsVRhejnE3K7C1A4Eo2k+Gt9jN1phailIUhK4vSQTOEgytRymVKowvZ7irftR+hp09NUJbIcLXBqwc28myPx6hLKicJCQmNrMM9RmIRiTuDqdYbhNz2CHnfcfhDjSbCaVkXjrXpihFi2NbhV/dW2bDp+Gg0Qeg17HM4MORleLlEUDZoMKrarE731tFq1GhcOi5//+2hw7gQOqTGWuTkQQRYFINMYfvL5Eb53Ai8fs3JyX0OkMuO0G7k4HWNwXURSFudU93r4X4kKPlZvzWd55EOPXP9HAnSUJh8VAKlfko9FVwtEUp7vMvHUvhM1q5JkhF195bw2fQ8WZHjujSynGFuO0eVWkMxnurRT5qXNexmYDjM1sUpHTfOWDXWqcItcnD5DlErNrBzzcqvDOjWU2A3GOt5p4sFpgfitNj9/A3z7q//1B5TG3x4GiKFcURWl/ZPf+u1loHuHvTBBAURQZCH5fpvMjhMDeLmUEXD2daC1mouOTONRqjMcG8EejaNwuDG3NlHQ6yrEYme1dIuubaPQ6VIKAUaUluLOLc3yG5bujtJ4YJnRthNTGJggi0toWpb19AktLqNRqrO5q3J1tmPu6SG3uYIvHEfJ5lu/dp/n4MOLYNLtzS2gtJsxrm4Q2NrF6PCiJFDpRZPHWXboQKZqMVDc3kVtdZ3NlDW9vJ8wsoEZh9q33aDjaRzYUJpbJIKhUZNJp1r/wKl2XniA/u8TW+ASdxrPkH848ClepcdXVshcIYht9iAJE1jcoSBINfh/OaheO4SNkdgLE90N48gWqjvQgFGWMNjuqrg48OwG0dV70tTXs3bxDLpOlOL/Mwsgt/IMDmEtlwqsr2JwOlEiMbDpLdHcPv9lIYjdI9e4+6VAEs78O0+oGcrnM2tgEjceHsPV3I3a2kwqFcfZ2s375bcqlMuKt+xysbVIuFNHq9SzcuEnD4ADZ0Ukq5TLh7R3mVFp8vhpWFzeotfjQaXSYjFpC8RLpAkwt7mI16Ymm8ogCdPiqafLqMRhNnGg3IipFlrdSPFjJEU9miSaz9PobafeVkUtVnO/SAhCN63C01nKmU8etCR2n21QoikIkbsbntvFEr57pzTzvLQW5v1aNIGgJRZK8N6bDYVGTl02s7ZfQajXMLe9wx2miKFfYDsa4ZbdSKOSJp7K8eTeKqNYwuRigpKhJpTP0d9YytpIgnpJZ34kgyzXMruyhVokYND6GWi3sRT20eUSavDoezJvpabRhMWs5iEfJZgR0BgOdzV6ysorR1SJTSwGMehXmDjuDXV46vRXupWCo00tfg5HpLYlSUWZx5wCzxcz10XXODjaQNGvpavHhNIvMbhd5uLhPGQ3RaInmOifv3tvHZrOi02i4fGudE/2NJDMy+wcRLpdL2O0WqqudnO828B9fnaO32crpXicrOznKiovnjpooFJox6xWmt2VS2Rxfvxri3LE2TNrwx77vfz9QqPwDU9b/bviR0Ej4YaHe34Cl2o1YKiEIArpiCX1bE/lAEIu3GjkcAcBQqaDS69BpNbQ//QT+/l7Mp4bIpdP4u7soaFV0vvISOo8btVZLx6dewd/Vie3MMbKJJPa2FjT+GjxNDcixBACVrV2aP/UyqnyB1mNDqDRqjMP91PV24WyoR93cQOfZM0ipFJqeDlRtLbSfPYMiQCkWx//JF7E7ndT3dmF1VqFtaSB/EME32I+5twuPv46Gi2dRGfS4zp3C4najaWpAbGmm5ewZ0OkoGg3UHR2gprODUqmEt7kR08lBFF81dUMD+NpaMdfV4O7tprS7D4kUff/05xGyOXRmM2a9ATUC8Tv38Dx9AaIJsju7VLldNA8cQdPXibulCXNzE3m9lt7P/AzFvIRhsA+z3YrDU41Y58NW50Os8WHu66a8sU3Lz3wSnUpFz5NPIMgltDYr0dEJjLW1SPEkvq4O6jvbsZ89QX1XB7XHBxHqa2g7dxZBFFHVeCmqVTQ9fZHqBj86scSFp56lXJI52evFpNfS7NMhy2V+6mIL3mo7F4/5OT/gw2IxE0qC165ici1FpqjhX3+6nTJaXFVWLhytRVbUbEUr1FapiSSLLGynaa8347NXuD0b4WS3g4XdPFfGU1zqN9GkwcujAAAgAElEQVTqVtiJltiOyAx2VDPUJJLN5Gj1u/jEKQ8qrYVzvVYsBjVOU5lmfzXVZhmnVU9vex3nuzSkciX+l8/0Ul9tQJbLnOqrYahFT2OtE1EQeHLAhc1ioLutjqePmGiqc9NUX82FHiN3lzL87Hkv2zGBUDzPiW43+dJh6+XPvtiMRqvl/BEPTR4tZqOWoVY9x3vr6GuvJSZp+ZkLPu4sJGmtMfL8sJOJDZnjbUZUKoGj3fW0e8p86lIbUrFCi1dHjcuIVm/GYRZpb3Dx7KCNdFHFLz1dg9Fs4rlBM3JJptXv4vlhK2ajnlMDzVQENa+cctBXryKYqPDcmQ4iGRUWo5ZgWkSnhmSmgNehJlcUONFuwGPX0ttaTXetilpf9ce87X8/UBQoK8pjbT8G+FjX8R8Xm+8Bx8lhkhPT5JNJRLsVe1sL5d0gJZWKst1K4OpNVPU1lIoygdEJ1HU+kEtER+7jGDpCTpIohqOojHpWPrhOOhpDCkc4iEaZ/qMvIFuMaH0eAiN3KZtNCDUewqMT6Op8iGo1+UQSndkENguF3QBakxHXuZMk7o8jiAJVT54jev024Q8/QuW0E98LEgsEKM4vEdrdJRYMEd3YZO7PvoyuqQHf+dOkpmbReqsxNTUSTyaY/fO/oOb8adLjU6Tuj2Hq72bj/ijxxSV0HS0U0xmMVU50bc3Ep+dQ9sMYezow9nex/u0raP11yPE4BqcdQ5UDWalQOIiAXosiFzGYLah1OrYnp5GDYYx93VRKJVJ3HlD/8otUQmE06cxhIebxo0Sv30FvsaBra2b1G9+m4eUXSC8sEp9bxNLRis5qITI5i8pfg/OJ0yTuPMCk0WBubmDl8lsYezuRlDLBqzcx9HZiamth5ot/SbFYxGC1s3blfUKz81QEkfn9ADadSEtbG5dvB9gM5SmVivzBN9eYXIkwv51jdHqLg0SBdE5idjNFrqAgFSu8/tEmKrWGsbU8y5thRMr43SqmliOUShXO9zmZ2ymxsl+hp17H8U4H1ycPONtbxa2ZKEZNhZVggYSk5ktXlulv1PPMMTcPNwqotTraarS8dTdIr19DR72FtXCJWFbN1l6UK/f2OdqoplzM8trIAUcatKyEynzzoxWcNj0ms4XPfWsJh6GCRsnx6rUQLwzbEMpZ/upGiE8ct3OkQcPkZgGd3ohRryKdk7m9mOdos4FvfLiMgMKdpQKhaIZ0rshQm4WpzQJvjyaoNsu8c2sJFIU7KyUmF0MsbycYmQrjt8u8PhKi029Cyhe5t1xguNXA84Nm/sNX5uirV5HNFciV9Hz6iRruLuWxmvRo1SJdNWr+/Rdm0Iglev16fverC9iN8M7IPFI+z7WJAxxmNQvbOayGClZdicWdLC6LmlNdZr783hZHm3TUOSqMLiawmPT89HkPo8sZQo/+HP6g8X1ko/3QIQhCgyAITz36bPj/qT7/wseN/Z5hNEEQfgt49VEF/z8o5PN5pKlZtEYj05/7Im3PPEl5fpH10TEs1dVU1XjZfjCG1WXH++RZzGNTh8WJkzM462rQaTTsLy5hqa5GU27E5a+nqrMddb0P1fYOVq8bq78eSmViwRCKWk21z8fqvVFajw1RCIZJH0SJBoPU9HWzeuVDRKMeazQGpRJrH92gR5axuF3szs5TXe/DcRBBrdFgHuzFVsgh5mUqLidqrRZBpyVy4zbbE1N0WMyUC0Xc/d1IO3ukg0Gk/RC5VIqSKFBV40PtdhMeuUtgYhL/kX7McpnlkRG6z59FmphBEAUSW9vUbO6wOjpBx5kTJG8/oFIsMvmFv8R/cpjtew9oO3Gc3OQsCJAIhRFv3WPt/gNaThyjML/IzsLiYeMvo4FSocjqvQc0nDmByWamVJQ5uHEHs9XC8vUROk4MU9oLkgiH8Qb2kQP7ZCMx0skUxlQKV10tB/cm0IsCO3NL2KqqKOv1mOw2rEd60ZhN+NIptBYLUjyBtB9iJrzH6YGfpKneRVe9gQa3mf9rN8VLp2tp8ujYPajiaLMZh9nC7KbE6MweL57sYWUvR6tXhc+hY2IpzPpunBavl1qXlusP9xlxWLhya4mjnbVcm82jERVyuRzfvnPA6laEzrpaOmr1LOxI9Da72DvIshaqcOPBCkfbq0lqRaZXI7jtRnYjMjOLe5TKFcwGDU31VSxspVjZTZHLyTS6q2mpNtDgs9PfoEWnVhhzWdCpKyxspZBLMLVhJSuVWFwLM1rjpFhSc/3+EvVeK29KFhbWwiDAq4kUbX4XlwbsOC0aVrcMNLhUvPMgxuJ2DJNOTX9TLU+f7qJcljnboeX+jIVLA07K5TITa2nG5oK4nHY29qKgwJVxkVA4hkGj5qOZNMtbMVQqqJR93BxbZ7C7ljfv56h1ClS7rDw37Oby3SAWk47z/TbKqg5S6Twnu81shmXmV4Mk0zYaaqr41lsLPHe2k+V9kUgyx7W5AgJabowu8+TJTt6blFCAW/cmDyu7f4BQ4McmjPZIfPOfAU6ghUPq9ec4zOejKMrsx41/nJyNF3ggCMIE8AXgvb+PZPx/j9Dr9RgGesnfuk/d8FFMPh/FkkxNVwdGpxNZUKjv7qIQSWCIJSjmCzjOHqcsyxgdDszHBmiQJKiUKW3u4n3mAon7Ezj8tThNJip1tahLZWRFoeXCGUSDnsJBlNrhIxjaGtF5qpE/uIFaq8XS34N1fRtzYx2OgT4Ort2i5egA+byE2V2Fy19HbmMLU3c7mcA+xUgMIyI5RSG/F8DW34O6UMB2tJeuaje5QBDX0BFitx/Q+NOvUJxfQfBWY/dWYzl1AnlpmUpOIq/R0f8rv0BqYhrdQA+OtTVU9T50nmpioxM0nDuN4LTTfvokaLWYh45QSGeoSaWxeDz4e7vR2KwY+7tpKJdRyhX0g33UF4oYPC6MnW1Uhw9QaXXoBvrIjtyj/9d+kcpekFzogO5f+nlyD6egzoezqQGNvxatz4P64TS6/m4q5TKGWByL20W5XKbicmJtbSK1skHDk2dRKmDuaMWfzlDY2CKVSmMd6KU4v4Kx2c+Q3kSVUY8sywy1mJhaz5BMCXzmUgOjyzm8djV9rdUs7JV4+oiB7UiJX/+JNnYiZfrafExvynhsarpavFi0JXQ6HeFAga7mao63qiiX2oim8gzUC6wGcjT4HPQ1GujwdxBKCWi1KmJZkc9cquXmfJ5uZ4V8vpZ2v5NMOkO+qZpj7SZ2DvIc7apBKlQQhAoOg0CN20amZCBXkBlut/Pm/ST/7KVGPpzOkc3l+c1Xmrk5J9HeXIteXaLJoyUcF/nMM00UKmBW5/nkhVYCsSKfOHao6qwgoBbhE8dtvHY7wQtDVnRqBbks8NKJKlBpEQWRFp+eQFzCYRRZ2JU52VfL/dUiffUigsrIv/mFHhb3SjR4rGj1Rs51arglOLk06KRQ1iAIakQRBlqMaLTtZHMFzvVaGJlL88lTHkZmYtRUOzHqMhRlBasOzndaeXcizSeO2wgf8SOKIk90q5mcN9NaXebecoGfONtARaWit15N6MBNjUOkq07Hm/clnr10+odiR36MmoD9JoeSOfcBFEVZ+RsqMN8T3zOMpijK/w60cdhA7ZeBFUEQfkcQhJb/pun+CEFRFCLXRjB3t+M6eYztqx8RGrmLyl9HbGcH0llEiwX7+VOkdoNkEwl2Lr+D++I5cNqRFlfR6nSUq5wE5haJ3Dnsg5O6P45oNmHvbie7tIq8uoF9oJfI9Bxmfy2+c6fJLR0WcNpPDCLWeln7+rfwXjqHrlQhdn8ce28nerMR95PnKBn0HGxssjs6gVyuYO/pIHD1BprGOpynhskGQlga6slt7ZJ5OIuxu4Oqi+cIXPkQlU6LRq+nlM1i1ulRXE52r7yHWFPN8p37ZMNhpOA+6o5WQh9exzU4QH5lg/j0PCa3C/exo+Q2t1GbjBSKRYrJFOn74/heeJp8YB+L14OsFilu7hyy2iSJ0M07OE8fJ7sbJHzjLtbho6jqvOy98wHmJj86hwOhKKNTqdFYTKham9j78AZ1zz9NYnaRyPQsvtPHyW7uEP3oNs4LpylqNaBR4z52lOLqBrpSGVd/DxWTnujteygOO/lAGIPNhtpkIhYOYR2f5eixY1T5G/h3/+EPmdtMkM1m+ObNLQIHaS726Xl7LIVGkKmUcnzlWoiXT9jxV5vZChcRBAWvrcQX39vmVLuegRYzNyeDCEqF050m/uztbc50Gbh0xMzXbwSY28zw9KCTq5Mxuuv15HI5Xh+JcLHPiCiKxJI5prdknh10Mr8WZm4zjUUvsh7IsrKv8FS/iVYPhKIZlIrMl66sUOMQeKLHyMR6HofNiCgKFKQMqYzEyp7E/alNsukkl47YuL2QQVDp6fJbePfuGh893KfKXKHVIzC5kafKqqNSyDC/ts+370ax6wp8/so2zx73shio8J3ROE/1m2ioUrg6fkCNXaG30cLK3mErhdnVXf7kzTUGmzXYLXqCsSIGoxm7Xubz72zz3FEzDV4LK8EC9dUGXjnl5PpEiOmFHQSlzJfe3WBjJ8JuUsvVsT2kXJZLRx189cMdmj0qjPpDBYL/7U8f0uAsk0xneXs0zrl+B3/0zWV8Dg0Wo5p0OstfvL/LJ0+5WQ1kSedKmEwmLCbjD96GPGYI7UckjFb4m4LMgiCo+VsoGDwuG00RBGEf2AdKgAN4XRCEDxRF+Z//lhP+kcH2zjaxvQCFShnRaKCUL1LV331YPCmXCK2tU1VTy+Y338LTUIfV7WJjYgrP9DwUi+ysbyKl0nh6uzHYrVSdOUHhIML6/CJ2rwcRiIfCFHI51HodiWAIXySOFIlzsL6BLJdQCQLRYIBsIoU0vcDq6DhGpx1JypPZ2cWZy2OwmFGbjLi9XvLpNIn5JfY3trD769HpDpBSqcM2yqk08YMIGr3+sOI+kSSTSKAVRKLBIEVJosrvJ7m7R3UoSm1fDya3C11rM9HZBYILi9iiMdQ6LanpOdpODJOLJUhHooQ2d6htb2Pmz79Cw8ARMvfHySXihNY3qO1sZ/3+BKIo4Gr0E1hawWY2o1Kp2Z6dRw0UZJm92QXsHg/l/TALN29R39eLanwalVpNOhonfecBWoOB1Q9HaHvqAvOvX6b99Amyo5PEVlYpZDLotVrWbo9iclehKAoarZaF2/ep7etmf2kZRzaNdn+f6NYO557qQAHqW1o4c+40fnGVgSYdv/uqRK3LwG60wup2iHBET53bwMZ2lOuzFvQ6DTkpx9J6gCNdDaztxHjrnh6NukI6mydfKDIil0iks0ys5TBpFVwWNTsHBWZ2IRzNcGOhhM1iZGJ+jSs6ERSFxfUIogpMKjseW4Wt/SxN9Q7+5DtLPH++lxsLMiJa1rYjfOJkDxIWTHqZkcUCI2MrDHTWcVVR2AymycsVHCYH7Y1VhGJ5bs7nkUtlphb30OtaafQ5qau2kJUKLAdL3Jlc4exRP7VuA4m8wE+cqSaZKXJjaoEb0ymWtiL4nEauFPMIgorbkzu8/GQv6wcSm4EEVTYtnX4nOVnFXlxhdq+AlJeZW92jv82LKAi8OZrAbDJyfXyDp052MjKfw6iDxjonr5xy8uZ9hSqbEZc5j99npa3WyL3lIms7MR5ueTDvSwhlhTqPHVlRoygl5jZCSHU2Opo8nOo0sh5Isx/NsLIV4e6qG4tJz29/dZaeVh+m/R8GG40fl+Q/wA1BEP4tYHjUauA3OOzg/Fh4nJzN/8hhtX8E+DzwrxVFkQVBEIEV4Md2sWnwN+BsrMc+0I/aYsauN5BPpdDZHXibm1ApCul8HlOpiGmwn4OJaerPHEesdqHSqHBmJfIWC+VCEf/LL5KfXURsrKfl7CmK0TiG/m5cyTQVg45sUcbd1YHocaPxuKjO5ZCLMpZjAzCq4K6to+S003TuDKVUCuvxYfL7YQzVLnTtLXhQkKQcVQ11xAP7DP3GZ5EWlslmczQ/cxFRp0OfzuDzedA3NVKYmqH1Uy9TmJ7HcnqIXEFCUKkRdTpqhgdRW8wYzWbK2SxqrRYtCj0/+ymym9uk4gmq+30oRiP69hYsyTTmKigbddSfOobZ40Hf3EDx+i10NhuGvh48hSLlcgnbiSGSoTDqOh/ZcISq5gYsR7opzS7Q+4s/BwcRVM0NNObzaPR6DEP9REfH8bQ0YT1znNTiCo4aD6LdiqejDVN7C1qPG1+lQtlsRO2txtvZhtnrwTzQQ+zeBEd/+TMkllfpfO5Zcrt7OC+exWIwYrBaae3q5N0vfYH/4Zc/yef/9PP4UwkuDnrZiYkU5SKfvtTCw/UcZUXm7EAN/S1aqm1ark7qEUQXp9vVWLQtBONlnhmyML5uo1RRkU5nOHfEh9+twaxTiElOLJYczdUC5aITvbrC8VYtE/N6zvdauLeU41debOHOQpoLAzauPIhxpFXP0UYNpaKfREo6ZI4t5vjsSy0E4hU0apHWWisboTjN9W60Wi3HmtWUSj6Otah5uCljsVjprDMcCqiq1CRSdp7qN3JjDlJSiXPdNtZCCf7Vp3u5s5gjlIHnhquY2y6wtJvjX326m5W9AhqNCkWBY512PHaRpa0EJ1o17McK2E21JLIKxVKJgVooCSLVxgJps47muirKpTw1bgsvHXdwaz7Ni6eb0OlETncauDJWplA81Pc1m8xUULEULPHZFxq4MSdxsc9ENt9BKi3xRLeDd8bz1HmsNHs0zG5K9LTVgQDn+zVMbxdxm7VYrHY++5IdQS2iFhWGuupoqbXh9v4Q2Gj8+ORsgP8V+FVgBvjnHLZ2+fzjDn4cNpoL+ElFUZ5VFOW1RzU3KIpS4bA52o81XOdOkxwdR4rHKZtN6Hu7Wf36Gxj6u5HzBUxqNb4XnyY2chddqYy5pYnZ1y+z9faHaBr9mAZ6yUdjaI0GcrkM6QcPMfV1oetsJbuwgspiQlPjYe/eA1SCwMH4FAcf3cJ87Cjmo73M/vmrHITCLN97wP6dUdBrqRgNLH/la1i7OsgkU2xffgdjXxdVp06w89b7YNATfjDB2ugYqWAQlVxi7epNIoEAipRn+kt/STadppiTyKIQnZnH5q9DKBbRCALuk8OkVtYQRJGCVktkahaD0UxJLZLPS4Rn59BXFIJTs0z+0ecplEvks1m2bt3D0FBHZmsbKRLD6HLivniG6K076FwO3E+dJ/LRLVz9PWTWt5G2d6h77hLpyVn0Gi1Gj5vcQZTk7QfYTx2nrFVTDIbQKYDZRC4SQ0ynqToxTPTBQxpfeYHk7CKZYAiV045zoJfUzAKmaje5aIzE0goGpx2MerZGJ6jYLdhOnyAzNnno8ezusDQ9xfHOGswmAy998mW+8sEO/Y1GPry/wdhCmKXtGHZNlnBM4qnBKsbXiiQyBTQaHc0eNa/fDNDXoGOgUcXvfGWOfr+abK6AyWTk4oCb6W2ZD6clznUbeGawitduBHjheDU2fYl3J1JcOFLNH76xQq1Tjceu5rmjFu4s5dEbzDw96ODdsTgtPiMvH7PwlQ922AomcVp1bIULQIXXbkXpazLT1VLDp844+MNvLrO1F2EhoLAdTLG4tkeqqObNkXVmNhK8dLyKr76/TbtXwK4v8HuvrVGQ0tyeiyHlC6xth9mKqXn13UU0YolsvsSNySDHWw30Nhh5d3SP//PLs/zqC35mtyWmNmWOteqRJAmTXoPToubLb81zdTKCw6zF77Fwfy7MyU4zX/soiMtm5Il+B3pR4r98a5UjjRp81grfvhWkr15NQS5jM2kQRZFURmJqNU57jYbnBy38+z+fpCznCUUS/MUH+xxv1VIqpJhf2WN0IcmbNzf45s0gx1s0tNVbmFrPsR5W+JkLHkLxHIH9gx+KDfl+FnX+kGHgULXgpxVF+RSHOXzD4w7+np6Noij/7mPOLTzug34Ukc8XkManKMklFr70l7ScOUV0epZysUhy5D47szNYqz24ymXUWh07C4u0eNw0DvSjs1vBpCM2NkUqcoA0O09qN0ghlcY2OYdQKvPw3ffx9/bgstvwXziLqb6W8IMJ4hvbyBUQzCaMNhu+gT50egOOlkZ0jfWoMhmKd+9TKOTJx+PEtrdwzi+h0mqIbO5gaW/FPdCPqlzBVOPF1N2OenySugunMdZ4D3vE6PQQT2IU1axdH6H92DF25xaweqqpFApotFq2Zudo7O1m4aObdF+8QCWRxtzeiml1A8upYdjYpnj7Lu4LZ4nduEVddydquYRotjD1p1+i98nz5CfnSMbjSMUi2lCY+M426lAI/8ARNscn8DycJbCyjkanxZUvkD44IBWOYHbaUcsys9/4FlZvNWqPm6Wvfp2moSPowtFDCvnDWQwWM3N/8TW6z58lNz5DYGEZS60Xi9XK2jsf0jBwhHI8jtFqgXSGcjBEYneXbDKN1mHjjT/6z3z6p57jO+/doVyucJCUGV8v0lRfRVONlSMNamLJAjemN3lnIsPWXoTFNZkXTvlI59SsbMe5s+JmJxinud7FnfkEd6f26Wyq5tqciofze1iMWq7PHr6T+UKR28tlBEHDncl1+lurMBm0LO9miUtaspLMwnqAphorOwcmHs7voSh1BBMCv/aCn4nNEmNLSQ5iMrfGVnjpQgdboTxTiyGUihe/14HfZ+N0u4ZMRovfW8PpTjPBqJdIPM1aWGBpO05tjYfljSyCoPDccS9mg5rJ1SRWkxujKs+zJxswGHQk03kisTR7ERtdDVaeGXLz7pjInUWJO5MbvHCmlXuLGZY3wxgNOqx6Lyf6G/E4tJzp1FGpVLg2pmdtN8PcWhizScfSloxJJ7IdSHB3wYrDamRp54ChDgf3Z7Z4briaxa0i/iqFr1/b5MxgCxGrmrYGD0dbrVweSWM1lUikC6hEgfbGal444SBbEIEKoaTCWlhidGabM4NNvD2WJJGS2Ztd/MEbEeXHyrO5CjzFYVNMOFxo3gcei3nxWDmbf6jQ63UYho9QnJnDlkygra/FWS7hHOhFYzRSKygosoxpoJfU5hY2n490JEJVXxeJmXlsrU1YjEZMx4aQNWpq+nspKgqqKgeCzUyXdIbo1h5y6ADHkW6y8yvYLBaqepyYTg4RHblLwyvPk7g3jtlqQYknUXe2kRibpP3nPkUlEkOnz1D/m79OfnIa89E+OkoVcoF9hI42LA47SAVSqxs0PvUElUiUyPo2zpODxO5PYOtsJRkO0zJ4FKGlgVaLhXwkgqGng8TMPK66OnI5ifaf+iSF7T1s/d0krt/GeaSX1M4uQjRGzaULJJdWMLhclM1GBERKGjWelgaKuRyG7naaWpuQg/uUMlk8fd0k17eQbVb8x4bAZKShr5tSoYjjwinEG3epPn2CSiSGeegIjbkcWqed5G4QR40X+6ljJDe3MTsc6Ad6yEViNOePI9otGLvaaSoWEIwGpPABLU9fxKDXIykK/kvnEaQ8puEjmBNJNAYjrotncdy8xcnhbpx2K+++f4Nnhj2olBLdDVYCUQlFsTK7U6Cn1cfzg2auiUVWdhJoKZHOlqn32umpUTBoXKSkMpcGzKg0BjQqkQ5PCc3ROiLJPMeaRdI5mSmLniP1Fa7OSPzLn+3ka9d2+KVn/dycz3G+W4co6pGLDtYDCbpqNFjNRsrlMm0+NSaDGrlS5qlBF2/cSfCJJ3u50G3g9VsRmmqdPNlr4IYgkMrKVCoVBLUelVrDO2NxLvZZ2AqpEMUyHY0u6qtE8kUX/fUCc7tlTrZr2E2o0Oo0hNMVnhu08f54nJY2Cy9d6CCWkXHZDdxeSlLvtdNcLRI4cHK6y4JOq2IvWo0oCgy2GEjlIZHOksppuLeQ4lee9xNMwLG+epq8Fgaa9bw7FuM3f7KdSEYkECtitZiJpySEikIsU6HKZmAvWqClzkltlQ63uYSpt4pgEga6a8nlSwy0m9nPShQKEnKpQoPPgt1QpoQWiy7PZ1/uYjlY5PlBC5fvlzl75twP3IYoHJIEfkygVxTlrxcaFEXJCILw2KyLfyzq/B6I3xlFpVLjG+hn/q9eQ9vWhL2jlUpgH5PFjOfZi8TujpJeWsPR1EBwfAppaRWNSs3k576A3mrFotWy+s6HJAP7yAIk55dI3h3HceYkDp8XfX8v8bvjLF+9gaG3i0whTy4aQ2+zoTYaMQ8dYW9pGUmAbCCIwWJB73Sy8v5VhIY61Fo1JYOBnfeuoaqrwXHhDIlb96hoNeSzWdg/QO+vJZdMozcYUOv1uM6dJDM+hcF4+FuRphcwdLTgPHuSxOgEKrlMKi8RWl6hsLZBNpHg4R/+CQUUSpksW+98iFhfg0qvJ3DrLkKtF5XFwu7IHTRaLTqPB+uZk6TmF1H2w6TWNtEbDGC1oHPaWb38NgaTifl33ke2mBDrfKTml1E57JjqaiibjMx9/suUNWrW79xHAXxPnuPg2i2kzW1cp4fJrW+Rn17Ade4kuUCI5PYuqmoXwclZbIP92Hs6yUbjJKbmsLQ0UkmmCL9/HevwUUy9XexfvcmnfuNf8KXXrpHJZFmbGqGnwci3bqwSOEjxRK+Z20sFNDozDpPCym4KvV5PU72XVF7AYTPS32jg/YkYZzr1yEWZt0cTnO/SE0tJ3F3Kc6ZTz8vHbbw/meHOUp7uRiv/6WuLqCoFtoJp9DoNLpuWS31Gbi1IBCI5aqotPDnoZS9e4clhL8PtVma2ckxtFpHyRW7MJjnVaUIuwbvjcc712PBXqfj27SBtPpEae5mvXg1wsl3PVjCJ3awhkioildT85fsr+H0OvvLeIjpy1LoMyMUCG6ECGrHMtbuLSPkil28HaXQLXJ9OoVWVGWjQ8Gdvb3G02UBZllkPK/zq837uLef54GGc871WjrfquL8soRXLPD9k4/ZiAUGtp9ZlJBgv0+w1sB7MIhVktFoNdW4D37m1QbVV4CfOuHiwkuWXX2gjXVRT49IjlQ386gsNSFKGz11eQa+uMC1iGKsAACAASURBVLkUosMn0uRWuL+UxOsQeWbQzufe3OBIo5aeRguReIYPHx4QTaaJRqP8zlfnudT7g2ei/TV+jMJoWUEQBv/6iyAIQ4D0uIP/0bP5GOxsbxFeWcPR3UZVfx/etmbC98ZxuqrYW15FpdNRnc+TjsSRiwWqvG4cTY3oa33oGutxbO+ga6pD5XTgWFjG2dVOSa1if3GZXDiC0WYDAdZf+zY17W34j/SRejiNTqtl8atfp+PcWdJ3x8gU8lRkGb1KZP7V1+g+f4aD67fQW62UQmEkScJQrrA6s0CVo4qCUia4vIIp6qSQzKAzG9GMPmR/efVQt013qNe1OTlNVY2P6O4eVp8Xw9QciiyTPDigLJcx1Pkw19XiOn8SlUZD6nNfxHvhNMFrNzFbLeRiCRRZPsz9bO0gaDXIkkR0eQVEkd3rIxjUGgrxNXbm5tAZjBQiMTQWE+5GP6YjPdgWFsntH2C3Wpm7+hHdF8+TvTdGNpU69HaOD6FSQKPXkQ0EEDVq9uYW0Kg1hFbWcA30EJ6dRzbqWP+rN+g+d4b4fhD3boB8YP9Qx217F8NHt9mbmcVZW4t5ZQOtRkV2ZZ1b731IWW3hFz/7LznR6WJ6vcTx/ibMZi1LwQqTC3t4XYeU3S9eWeXFk3XMrCQYK5QZ6vGztpnGbtbw7kOJsfldXDYjN416NveiFOUyl4UyKlHF3n6cfLFIo9dEX3sNzx1zEk4UmFlZ54MaF+UyzK8FuDtd4dxAHemSmusTAS6dauP9sV0avDZWt6MsbURpa3Rh0IiMjG3jdVspVURUKhUTSxEcThflioHdUJA7S3mmF7ex6Wvw2czUOlT0tXpo8arYqq1i+yDP3UWJisrAH78xzwvnW+ls/X/Ye9MgubLrvvP38r1cXu6ZlVlZ+77vGwpAFYAG0PvC7iabrSYtkhKpESfGUmg8npiwNeMIL5LHnrFoBxW2RUm2uIqkKHY30Xuj0UADqEIBtQC17/uWVVm57/ubD4V2MBgSiZZo9kjmv+LGe3nz3nPvlzo3z7n/c04xLZVGqgsldrwpNna38frVCA1OVnd8GPQ65tYOqS61cflulqlFNyajBrNeS04RuDq+jstqIJYuZGJ+B4fVQDyRZGRqly89VUVzqYp/+fVpTnXWMryURgDSqQR3FuIsbRxR5LSiKMf05kcGmnl9NEg+Haek0IpAjn1PgFsLBqwWI5eH5jjf34A3KhCKJJncyqEiTTSWIpnMUFtsJJtV4Q1nuDzho6T5F89GU1DI/v1xo/0j4C8FQfiwhk4x8NKDTv7lYfNTUF5RibOyHGtHK6HVNQoHTxKdXsB0ug/Z7UFnMSG3N+PQySRCYcL3Zil74mGCw3cQ4nGKBk8RmppHVVmK68xJwour2Ab7cbhc0N6KqJYQRTCmChBLXchGmVQ8SToQRKPXo6ksRV1gJzE8Svkj59DIMhZnAbruduLTc9R+8hnC96aRG2tJ+IPUXzhHXhQxdHZRqYCo1ZKNxZBkGXVrI5WiSEYAscCGWOSiLpUhGQ5h1+kwmQzoe9rwXR+h5unHiM4ukcvnsV88S3DoDlJNJa4TXcQ3tzFZ7TjPnSV4Yxjr2dMQDGOymFCMBkyPnidz6COZyxLe3sX54vOElpapLS9FnUhi6e0kMjKOwW4jtLZB8cApFK+flJKj6uwgUqEDubqC1JWbtH7xs6RWNpBlPUlRwFJeRq6qEv3ODnJtJfKRl+T+Ic7BfpIqEbOrEMPJbupVoEbA2N6C573rFHd1YOntxGAwkE2n0Z/oIJ/Nors3zbknH2f+9i1+5YXnMMfukc8mKS1Us7qfoKtGz05dMWV2iWA0TW25A41WgyQK1Ne7eLRDSyxm4rlTDm7OhZE1aooKbQw2qAmHzchaDQMtJjQiDGskNJrjO5nHOmXeuRejxJLhf32xmfG1JJ8atCNSgDeYpKVUxXtTcfrby8kk45QVmhlos+E0Ofj+dT2pLLRXySzvFdJRZ6WzSscrt/w0Vzs4Xafi3btRGqqLcBoyfOHJBrKKRFWxnh/dDvG5R8v4YDZBaXEBmVSMplI1NpNEJFpLXhFpqLCztJuksdTC7lGaF85Xs3GUw6xJ8eXnWlh2Z+lvK0dQqXi230Imp5DJ5mkoEbEaJI6CJdhNavrr1QhKBQgqHm7XMbPqYW0/Sj6vUFNqp7vOgFmbQ6+uIJwUebxPjdNuIhDN88JpK7FYEeSzPNlj5LXRHBUlGpb2M/yTX23n5nycxzplguFqDFoRlzlDV4OD8gKodOq5dCfDJwYN+GICkYya336+hqGF5MeSGw3+/tzZKIoyJghCE9DIcR60xQ8JYw+CX7rRfgYKLgwSGh5DCkXQOR1ommoZ/8p/Qut0EA2Gmf3G98ipJQSDzMHSCgfXbpJKZ1h65Q1yqTRSfQ37V4fQuVwYeztY+tb3iSZTqJJp1j8Y4nBqnoLSEia//j327kxgrCpHkERavvR5IgvLZDw+DJKKgqYGUlu7FPcfp/3XpjNorBbsDw0SuDVGamkNS08HyXCYg6HbaOprCWzvoC0vIREJE7o1iqGrFXtPB9GtbXbffh9tUx3mk32EtrcJhyNM/If/TF6vQ2U0kLOZyWUyqCSJfGEBq5fewtLUyM6NW8htjYhqEUNvJ0vf/HNMzQ2k9g5ILa0i11Ti2dkmsLlJyVOPErkzjsoXxN7WTEIjMffH38DQ3UY2l4W9Q3TlJWzevUfc78c1eILY+haRnT0MFaVoTSby4TCCWsTW00l0donovRm0hYWsvfkess1KXqPBd2uM/N4BtZ/+BIHxSdQ2K3F/kKPxSUxNxwG5vtvjCA4bSoGN5P4B3hu3qfzMJ7n0ne/QWqTjMy8+xZLPxOx2lvoSHRc6zHzl+7MMNMrEMwKoNPzGkxVEUyAJIr3Val69HUIQNbxyO0hdiYHOphJeHLTy1kSYRErhqRM2hhaSvDUR4WKHgUQqi16WkCQV9cUqPpj2U1Go57FOPV9/d49IPE0knub9mTifOm0ll8uiiDKfPlvIrfkI0WSOYqeVz55z8O/+YgmLHpa2o/xoxMe5NhNPnXQythLDapapKxJZ2kvRWWvmMJTn2r0AA03HrqRoIoNOyvNEXwFDiwkm10K0VmjwBwIsrbmx69P8+ZU9nFYd1YUSsXiaVe9x1oACfY5kKktzicgPPnDTVWPiMw85uT4b4+WhQ/rrZaIpeGsszNkWPfF4nNXdCE8PVGG3WcmrLXz5E9VMr0V5a/SI9kqZcDTBu+Neuqq01BQKvHHniMEWK/21En/wg2Wi0Rh7BwFKHHqsRonGYhXv3/XTWilj0qZ5/dYez58pZmkvx635IL11MkU2Dd94fZ5YNMxRIE4mnWJ///Bj0SHKA/79HUEj0AJ0A58VBOELDzrxl5bNT0EqnSJ1b5ZsLsfB4jK5nEI4FsVSXIStux3tkQ+1JGJpbyEdj5M48FDyyHkS/gDBzW3Qy0S3tokFAoTuTaE2mxFEFfrSIoxNDRQcHWFwFqDvbKHh0AM6DaHZebxr6xhNZvQGAyuX3kRUizhTKVZujVLc0UrC5wdBIJvNIOtkvNt7oBJQ35lAlVc4nJvDKIrsLiygMRlI+APEIlH0EyZyuTyqvEJgawt5TEckGCafV6h44mGy3w+htlo4HB5Flcni2djEMOVAY9Cj1ckc3Z0mForgvTVGLpclrxZJRqNEl1dJ+XzEI1E0kxYEtRqj0Uhud49sMolnZxeN2YQkSqgkkdCdu+xNz2FyOMhdz2G025G0WqL3ZhAQWH75DeofPkt4cYVAOERifQOL10fM4yEZiyNbLJQ1N2I9N0AulWJ/6DZHu/vo51bYGhmjsLcTHQpbQ7dpOnuGnNfP7tQ0pspSJLOB3Ws3yWVz6PUyK9NTLBbq2dk9xOKq5ebkJG+p1RwceTHqtQzPRxib2aGrsYjLUypEQcTtDbEfdGI3aXjzxgKPDrawcZRnecuLIIjYLAbem93iZqmd+TU3ep2akWWZ1S03VqOaH4WMGAwGPL4ot5YSaMQ8SxsenjhRyHwgTl7Jc3lKx+TSIWUuM29mLKglFb//zWn62iq5u2nAajJSYlfz/oQHlShiMhrQqAUu3Vjn0YEm9jwZljc8XHNaWd/2EI4l0crVLB/kmVnew6TX4gtZcR8FGJ0OM9hTR6XLQDyrRq9JMrt6iM1m5iCksLJ1SD6v8I5aRKvRsrq9Q7mrjvEFNxqNmul1BX8ozs5BEIPRwtjsFnqNxGvZJJDnm+/s8lh/CW/f2aet1sHrowpaWcfS3BYjRQ7MRi1Xb++CSoNareHa2A4qqYlwJIhGUtNda+Dbl7fR60T2/GY0ksS7I+s81N/A3r4fSRL50Yifla0j0pk8J9orcRgVLGY9z5wuZvMwRSASZ2t++ReuQ47jbH7hy/53gSAI/5zjgpgtHMfYPAkMAd96kPm/PGx+CrQaLdrOFlRXhyhtbMB27hRcG8L25MOEhsfIZbJYmhtI7h8QXN1AlGUyyRSJe7NUvfAJckc+dDodbZ/7DNn9AxSdlqoLD5G7n33WaLVAKkN4ew9TfQ2xjS3UZcWYs3nEihJUZhNFoTDkc5h6OmhQHfvm84qCOq/gOD+IIAgU5/OIOpmsUUbyB6nq7EB/opsGlQqNyQiCgNnpRNfbBYD3/etUdbSj7+lEHLuLvsiFZ3KG8icuklndouTCGY7eu0Fpfy/qQieh7V1kZwFSLkf52dOYS4rQFrnwXR2i9dc/R9p9iJTNIVss6FsbccQSpDIZ9PXVJP0BnKUlyJ2txD64SUFzA6buTopTSYwuF6lclqKOVmLTsxi62sjMLGArK0FbXUVeURCW13FWV6J2FmAtdkHumAGYczlIrW6gq6/BbjDg6ukkazJgdBagzuZwXDhDTlHIZDOY+row3BlHLLCRjkTJpDM4qysxnOxhoKaBpG+HLz5/gW986y/oaCrjfKuO63MFvHRex+JuillZw7MDTmStxKu3vPzKhQoMBpF9f5ze1nIe7dAxvxVFLYo83mPk7mqYR09W4JDT9LWUEo4m6a3RMrmkJptX8/yAg0wuTzZThV4jUF0o0dvkwm7W8fgpG5GEwMV2HelsBQatxCNdRvaP4sjaRhRUFBhyPH6yBLc/xYm2MoLRFOeaNSgozCzZaS5VM5lQ6GspoqtKJBwxkUgZ6K7WsLAd4/HTNWzsR3m028LIvEA4mkVUKXTVmrg6myYWU3jubDmFdvG4TELAjtmg5fFOmaHZAOUuM93VIge+cqqL9VQ6RYYW1NjMMk9060kki1FLIs+cMHNt0k+Jy0pLlZnbi0FOtrqoK9YwthSktNDMQIPE+EqS2goHz52yM7Yc4dGTFVS7YDYt88kzJtb2k3zxmWZ2/Tke7TSw74sRbi+nziUiqQqpsCvotCLxhIVcXqG7SsKghbUyM25/BlCoKLFS3vKLZ6MBf1dS0TwIPg10AvcURfmiIAgufs5Bnf9Dw3s/91bOZCC8uYXW6UClUpGURKLhENamOrLbexgkicKzp9j4wauYT/Uh26wQiSJlc+iKCkkGQ2TXt5GrK0jks3inZlEVFxKLxcisbaKrKsfQ3Y7nzj3KHnmI+NIavg+G0dRXo8gyc9/4LrlsjsOtbcRsFsfZfqJ3pwltbqMpK2ZjbBxicUz9PSQyaTxDtzG2NyPEExhNJlSlRSR39wlMzmJubSaTyxIcGsF65iQFnW1EllaRXYVkDTI7b17B1N2OtaONyNwimnQGc0sTqWgMZ08nma1dgnenMLU0oDYaWLtyDX1XK/ZzA0TGplDLMvaBfhKzS+h1MrrGWqKrG+htNpwnegjPzGGurCCdSJDyeNHYrWhbm0ksrSGEo5Q/9xTxqTmUXB57WQnu+aXjmKGOFsL7bnDYMJaVEt3dJ+bxItosLF0fJrKxRc0Lz6J1FeK5eRtDbRUql4PV779C5SeeQNk7ILvjpu6FT2DqasNz5QaSSqDpkad59a0hMv418orCm+MRHus2YtRrmNmI8NKFCm7MJTgMHKexaa+xMroUodypp6dGx+p+gk2vwGcvFjO7mcAbUXGhq4BvvLNKMBhEVFL8P9+d53yXkyf6C7h8L8q74wEudFjwRgWuzyZ59rSTa1Neemp0hKNxRpeCdFVpUXJJDgIp7qymeLjThEHK8MbIAW5PiCu3Nxhs0vJEt4nbywneGffz64+VcGcpgqjR81S/g/HVNHpZ5oVBO3/w/QXev3vA5l6AfD7Nv/nzWexmNT1tFZxt1vL6WJiN7QMkjczJZjvL7hw/Ggny/CkrBnUGtz9DOKPjxXMlfPvyHgPNJnZ8Od67F+XJbiOlNri77Ke2WKahWMWbo15MBplPn3GycaRwpqOEzYMYG4cZPBEVz512MroYIp2XaC7XcW8lSCov4TCr+MZbK1xsN2Iz61jei9FcriObThKOZ7mznOa5ASdff3OJcCTMsjvJH19aJJ9NUekQuDzu4Tvv7/HMqUJuzAQIJUUGmx449vDnCoW/V/VsEveD+bOCIJgBD1DzoJN/adn8FGysrZOMRomM3iOTSrFx5QOaLpwjePM2ke0ddCYT/g+G8e27ySTTlAgCokZD9O4UWr3M/Psf0HzxIZKTcySjEaK+AJJWTT6eZGdklNKeLvwbm6gUBZ3RQPDgiGgwhPvKdTyLy8gFVqRoDJQ8pY0NuB4+i2Z5lf0bI6TmlgkfHuG7eZuqzjY0eh2BzS1UyRTZVBoyGQS1hsO9PWxVFViqKzl67zpBzxFCJMLu5AwF5aWkJ2fJZrIkY3Git++hymY4Wt/AYDIeR3F7/cRjEYTlVXLpNAWTs+zOL4CiUJpM4xkeQWc2kT44Qtnex7OxST6XoziRZOX2KI7qSgzxOOuj4xS3tyKGw+zemaBhoJ9EMIRneR1joRO1IDB74ybl3d0oiyuEIlEC3/we5W0t6C1m9u9OUxKJsT07R4lWg39lHSWXY+3PvkX9yRPobWYko57D22NIeYXt8UnK4knkQgch9wHm6Vn8u260Jj16o4FcMsXR5jbLGj06vZ4rb7xPtTHC8oaHhmoHN+bT5PMK82v7WE3V7Oz7mF5WGOwo5vpChnsLuxRYm9hJ5BmZWuGFCzXs+3N87/Iyp7qquXTLh6vARH+LE6dFYmYzwtJWgA2dgXA0w86BFwSR6aU9RFFErRFJJDO8fTdMKBJnfM5HX0sZklrNV38wx8OnmhhaTOE+imPSS3TVmbg15+PlYS9Wi4nljQMqSmxsHKYxaeDewg6iqpKRyQ3KXGbeiMuUFdtprLD+t7ubxc0QSztRNt0h0ukSrCY9G7tHLG0coNeVcXNijaZqF3dWMxj1Gi7f81NsU3NtMsL6vp/pbScjk2u01RZzazmNyyTzytAuF3or8EYlbtzd4uFTzSSzKobubVFVqEU2mPgvr83SUFnIsr6At0ZWePpsExmMvDO8xMX+ekKpHOXFNu4sBAgmREKRJJdu+yGX4qs/XKLEaWFoQY2rwMzF7kKcFjUrW0GeHSgik82hlmJcuunhnQkjs8v7uOx6bsyr0Ln8v3AdoigKmXzuv/s6giD8C+A3gQ/TJPyfiqK8df+73+U4zUwO+B1FUd79Gy4zLgiCFfhTYILj4M7RB538y8Pmp6C6tgbr3gbmgRNEtnYxbG8jtzSg0mlRaTRIWi36rlay14aIHPkwnuxBMOpRl5cSGBrBVlaKtrqCdDSOXaxCbzZjPNWHOhgienSEra4GCZAEAbm3g8S1YYpaGjHU12AtLyMXDCIVu8glUsRUIulwBA68FNfXYTt3mvzQHRRRxHCyD9FmJeUPIHe1IMXizH/7B1hXK0kEQmRii8QDIY7WNynpaMU6eJJGWUciGkPb3kRqbQOL04murxP/B8MUtbei72wlHY7h0OkQsjnyuRw5mwVBlnFVVyNptZhO95G/MYLhsQtktt3Ina3YA0Hy+TyGkz3Uo5CKJyg4dxrv2jol5wcJLa3gqK1GrqslPbdI52//T/g/GMZ08SwVXh+GokI0VeUE3QdYy0sx9vei3dwiHY2grq+h5MCDVlGwnTlJ8vCIdDCE9VQv+tUC4v4Arv4e8irVMa08r5AIBOn5h18iMbtI9MiLo7UFXUsjgbG71D3zJEWZPCcvnEcf3se9MoHTbiadTnO+RU04liaXrUHWaSg02Rld9HOiTubadITfeKaRcALUgoryYhuFFgmLQcJi0vN4t5EbM0G+/HQ1794LU+4Q+Pxj1ax78pxp0vHyrQCN1UU8028llsig00g82W1geVPL2WYDEysgyxq66m1Uu9Qc+uNUu0Rqi7S8FjZSqNGwdZDgc49WMr6S4EKrlmzKwOKmh46yIiJpaK8vZLBRQzxeRIFF5myLzPBigkQyhSeoxhOI8amz5SRzCmq1mmQqwyMdOhLJIhQEzjRpSGfrCUXTnGmWOfDFeX3PhyTYeG6gkMNwnjK7itPtlahEibMteq6M7ZPJ5Ggvl1jZj9NZf1x11KKXmFrUojcYefaUHa1OJhpPMdAgMTFv5kyTlqtTQdrrCmkqE7m9JPOFhy1M7ygk8ykaql08fcJKMp1jYTPExW4HZXYRrbqIkaU4Z5q0PHrCxdhahnqXQDQrc6KthCKLyD/+bBs356I83ClxoLV/LHokl/+FRdH8B0VR/uDHOwRBaAE+A7QCJcAVQRAaFEV54BNQEIRBRVGGgf9NUZQU8DVBEN4BzIqiTD+wnP9BStP8N/T19Snj4+MPNPZH77zN1+7cxFheRmx+Edu504RvjZFR8lh7OsktrRFLpTDVVIJBxj88RjQWw1VVSdIfwPbQILuX3sJSWoK5v4f4kQ8CQVL7B1gfGuDw8jUSkQiCxUR0bRN7bxf5bJbQ/CLVn3yG6OIqWX+Q4qceQcnn2fzhJSoeu4h/eQViCRw97eQ0WjZefYOq555GMugJ37xNWiWgry5HHYmSj6dIx+NIVWWoFUhGo2TCMQxFTuTqCvxXbyJpdUh1VSR2D9DptOia6gkO30ElqJDqq1j5wY9w1tdha27Ac2ccW3UVeQGQ9RhkLfr6ajxXbiK3NoI/gKqkCP+tURxtzYhOB/6bI8glLtK+IFqjEUN3O96rN1HrtFgG+klFoiRn5tHKMvFgCOtDA0SGR0nlMuSAosF+JJ0Oz+UP0NtsKFXl7Lz+DhpZR81LzxMZn0TQyxhbGvG9fxMEAduZfua/9RfoLRaKmutZ+WAIU2kJRpMJdWkxsqRGW1eN7t4crcVFvHS+kc2tPf7sj77K0/0uYhk1a/sxnj1p5UfDh8RTeU42mvFG8giSllJLjv/69jqn20t5vNfO2xNRTNoMdSUGVg9zxFLwZI+B5e0g33t/m+cfqmFuM4IoqXn+lI1tT4zrUwE+da4UfzjJ5mGK4gItd1cjVLpMnGszcm0qiEEnotWoWN1PUeEQcFh0JJMprk8H+PLT5eRyCq/eDiBJIvuHARw2My+ecyAA70/HURCJJZKYtRl6GmzYjGpeuRVAko6py9+84uZMs5lim8QPh71YDGpqitTsHiVoKLdi0CrMbGeIJPI8e9LK1akQR4Eoz54u4vK9EFq1GpdFodCmZ2ojSWWBgtGgYX43w5PdRu4sR9k5SvH8aRsTKzGK7Rr2Ank6K9VcnQqTSSfobSxgxZ3jsS49r44EcFq1NBWr+NO3tqmvsPB0v5Ox1TS+UJKnek28ey+CLKXoqLURimW5PObhN54s48/e2sJg1PPFx8t4d/SAaFbNCwMFpLM5Lo0E6Tv7FJ/8/D/+SPpCEIQJRVH6Pqqe+RANne3Kf3zrtQca+3hZzd94rfuWTfSvOGx+F0BRlH9z//O7wL9QFGXkI8ieUBSlVxCEu4qi9PzsGX81PhbLRhCETSDCsVmXVRSlTxAEO/AXQBWwCfyKoigBQRAE4KvAU0Ac+HVFUe7el/NrwD+7L/b3FUX55s9zn6lUCl06y/TXv0PtmVPEZxYIHHqIeLwkU2l8C8vo7TaETAaNRsv+4jI6i5kUCr59N7rxSYKHhyQTSVR5BUmrYXH4NraSItR3JvDv7WMtK8HQ3ERsYwtbTQVKNkdsdZ18IEw+m+NwcxPNzRFUgkDwwEPx7j6JwyNiXh+yXo8oikQOvSSn58krefxHXmK+IwqzGY4WV9Db7SgCRCenqehsQyfLrNy+Q3V3F6pIjLAvQCwYolqjZePGTVrODpKdnmNjbIKK/l6keBJHZQVGl5O8RkMun+dgepaq7k4W3r9K84WHiEzPI1iMzH37+9Se6EWOxHDPziMbDOTXtjhYXcfoDxDY3aOiqxNheh7RbGTjzjj1RiMqRSGw7yaXzWEqK2b9ez+k8rmn0SkK7nev4J9fQghFiAZCrN2bouGJR6j9zAsEbtwieH2E3flFbB0tJK4OEfEckYjGSAlgNJlw9XVhqK3CtrSKta2ZfDDM5tWbNAyeJLq4zFEqjm1xiveFGBNj49jNBnYDKkamN9GoRd6b0rLhjmDUa5jfTTM+v8eTg40ksyoqix1E4gnemojh9oSIJZLUFmnZO4rhC0Z5W3FhNwjYLAYqC9W8OexDEEQmC3VEE1m8wRhzuzkyOTVXJ9Zpqy1kfduL1x9FLZWg0Wp5+co8p7qq0GnVvDm0QV9bBSgKR4EIV2bSaCSBhbVDmqtsqBBY3vby7qQeg1ZkY/eIyhI7J+s1vHozCEqeaFpNKJrm8CiATqNmfsVNmcvKwl4Sjy9MJKrBYi7mxuQGotaKViNxeWSRh/trGF1JYdDL3J7eZbTQgUoUGZ3ZpL3OwcsfbDLYXoRWa+LSzR1cdh1jqxKvXV/lwsk6xtezCKKOr706y2B3NXc31YzO7lFbbufrb6zQWGXj3UmBmZUD7GYNQtZCdamNvaMYE+tZ7szuIqkErqiPSTJXRvfRGQrIKxr2jkK8c9eEzWqmtULHG2NhjnxJApEgrwxl0Wh1iKLI6+9c+8iHzd8WivKRvKg8QgAAIABJREFUSgw4BEH48V/Cf6Ioyp98hOV++z4VeRz43+9XVy4Fbv/YmN37fR8FGUEQvg6UCYLwhz/5paIov/MgQj5ON9oFRVF+vCj4PwXeVxTl3wqC8E/vf/4nHNPr6u+3k8AfASfvH07/HOjj+B5uQhCE136e5au1Wi1KeQk15wZR5XIYOlpI+ALIBgOOM6eI7exRdrIPbXUl4YVlmj/xFDmfn/3ZRYrbWjD1tNMgisTCEQz93UTXNymsr8HWUI8ia6m2Wkl5jkivbVL3wrMoHi+JfA6d1YquxEVie4eai+eQLRbCqxs0/MrzEAijN5mxO5yYTp8gurVD4zOPo4TCWPt7ENc3yd8axTlwAq2oRm0xk1IU1LKM7ChA39ZEiyCSz2WQu1qxhSKY62oRC51UdrQhGPTI7U20KQpRXwB9RRmqQIhsLI5cUEBRQz1ZtYhU6MBZXobosGEoKyG/toGjvBRLTzsJjxdHUwNyezPxezO0/vpniN+dpaimmkQijtrlJLyyQnFPJ1KJC7XVgjUURm2zktaqiU5Nc/DBTXLpDEG3h6Lz56BChbS8jrO6ivihl7zDidluQ2ysQbO5jZQXsJw8gWZ6lnQyibqyDGtlBYo3gC8Yovj8GTL7B8RDEUo6WtHWV6MIEJhdRHQaeemTFzlaGyWbMvFQq8xhoIByp8zpRj2SWEY6q/BEl47tgzCNpSKH/jTnuwrY9ilcaJO5dDvD7kGWaEpgc8dLX0sRj3TLvHbbS0uFnmwOettrqLTniaQlIokETbXFnG3Wsr4f44mBGkSVCofdjFWbxWJS01iqwRuqodwusu9Pc6K1iM4aPfveGGUuK/21IhaDRC5fA4JIQYHCo/YcoaREX50OX0DHzLKbtS0JfzhBWaGTxnIjr97yU2R30Vejxhcsx2YQqHFIlDoq2T1K01SU57DBRVmBSEu5FiXfTCKdY6BJ5tZcgDKXlYttOm7Np2ivc9Lf4iQcz+Mq0BONJZB1ErUlJhymHB0NLlrKdVQ4NbwzEeSzjzeRzQucqNeQStcia8AbjHOi2UlDiYZstgyNWs1gu47r82nOtVnY8inUV9hJZwUe7jCg04hMLug5USPw/lSET52rRKORSGTU2Ex5Nn0ZEqksoihxrsOOw6zm5WEfj174mCp1Kg/sRvP+NMtGEIQrHFdO/kn8Xxzrxd/jWBf+HvAV4EscB1/+JD6qO+sZjhNwXuT4ruZvhP8/sdGeAz60TL4JPP9j/d9SjnEbsAqCUAw8DrynKIr//gHzHvDEz3tTmdUNHKf70DTWsfGXryG7nNjPDRCfmaeyo41kKERocgYxmUJTWUY8HKa0qYG8kiftPgSNGtOJTrZfexspnaXk8YfJug9JLa2ia6yFkkKyiTj6QgexAw+ZgyMqn36U5MIKRqMRe3sznrG75JQ8stNJzOdDo9GQlnWkfH5y27sYG+vI6bQk99zk9g+oevE5pv/kW+jbmyGVRorFqfjEE6QMeha/8wP8wSApSWL/+gja+mpsXW14p2ZQ2+0kEwnSR16QJKxnT7Hz6pvgsJMvdLJz6U10rY1Y21sJzS3hqK4kurSGoijkd93UffYFIhPTKG4PpY9cYOOV17H0dqI2GKC4kHAggP3sAMGVNaI7+zhP9pFZWcd/YwTTyR6UbAYOjuj6rf8ZUVAhqlQ0fv4lMpvbxO5OY+rvRkDB8fA54vtulkbu4Lk+Qu3nfgUdEL51B0NvJ7raatYuvY0i6wgHQ4iJFFpnAd7FFUxN9RTcZ8pJOh2PnjqFvbKer3/zB/SUZ0jEo9xdCdFXbyKUELg2FaCrUkRNnHfHPXzmYikLu1k2vAK1xTrC0SQvD/sYbDFxvrMAlSjR21FNOgfheBZZb+RCl4OF3QwGjUJThZFELMqeJ0yBPsfCZpiVgxznWo3E0qDVqOhvNrN1GOeH190MNhuZ24pRUWjgqX4HI0tRPFGRLzxSzPBikomlIPUugbWtA9yHhxhlgUQyyfVpP5VFNnpby6kodfDPPt/CqjvN0m6CQpuOJ/sKuDYTpbpIz85hjDtLMTorNZRYc7wx5ufFcy4WtiPMrAcpLwC7nGf7KE0oqaG9Ss+Vux6Meh2fPutibClMZUkBt2a9LO1l+F+erSGY0jC/p/CrD5cysRLj5WEfXTUGumsNeCMK793zc7Jey9XRTX79iUo2D1K4A1lkWUsqGWNoxkt9kYpSpwy5NIFQgk8N2HhjLML1aT/PnSnihzcPaK40U1Os57++tsieN0E0LfLcSSslBQb+4bOV3FmMcOWuj8ZyIwb5F89IU1BI53MP1H6mLEV5RFGUtr+iXVIU5VBRlNx9ttifcly+GY4tmfIfE1MG7P+k7J+xrhf4S+CriqJ88yfbg8r5uCwbBbgsCIIC/PF9U9GlKIobQFEU94/Vti4Fdn5s7odm4F/X/3PDxtoaiViM2L1ZcqJAYM+NwVlAPhjmcGsLY0EBoiSxMXaX6r5uVBNTJKMxPBubOKor2Ru/h9ZopDAW43BlDY1BTyYQZHt6BoPdhnJzBM/mNvl0GuvoJNlMhtDBIUUCJHx+Upk03LlL1O9HliQ8QyNko1E8Rz4quzvZfvs9rE0N7F+9gU5SM3flKtU9Pey99wGiWo1/cpq9iSkqe7qIT82i0mqRTebjfE3BMAdLy1iMRmIeLxGvl/DREeWNjay+8R4qtURxNEYum8EzcQ+T1Yp/d4/i+WWygFbWs353Gluxi3tf/RplvV3E787g3dlB1MlY5xaIh8JEVtfRabWI6QyHq2tYiouQC2wczi0Sn1tkZ34BndmMbm2DhSvXaTo3SOTWGAH3ATqTkcDEJAdTM5S1tRJfWiGbSBK9PYpOUaE1m8ikM8dyFhfRaLWkb46gKS1GNhlJBEN4d3ZQISAaDfj23RhW1tF4fYRCIdTXRqh79hN499y89+3vcFTvJBgM44/o+LVHTCxthYkKGhwWDRc67HztzR2eOqXj8t1Dkok4b4zBzLIbvaxm1mrApBUZm/XT22ChtcLGX9zw0lAqo1KpuD2zz6lWB5cnBeZXg5QUHgdMvnZzmdOdFbw3HscTSKNRS7wSkDAYjMxv7OAqLGBx/RCHzcj2UYp9T4BUKoOsrcTrCzK1GKOvrYJwLEWp00YwBhpJ4tKNFS6eauLdoQXOdNfw7lQSnaznT340w2BPDW+N5xif3yRYasMfThKLZ3hFzCGgYscd4OqcEwGFP39njWcGy8krCt94c4fqUguytoBb06ucPWHl+kIWlUrFxMwmkkYkl1Nzcz7B/PI+Go3IB3Na5lf30eu1mI16lt05NncO8IdTpHMSWrWKy+NHFBUW8P2r2zitOppL1Vy6dcCJhJpFt0I+oxCOxHn9tg8EiaEpN73tNaztBKgtKyCmyeG0G8mk4mweaTgMp5nfCqA1GDHoZd6+Mc+Z3hpcho+BjcYvplKnIAjFH+pP4JPA7P3314DvCoLw7zkmCNTzERhkH0JRlJwgCJ8A/v3fdI8f12EzqCjK/v0D5T1BEH5aoYm/zgx8YPNQEIQvA18GqKioeOBNVtfWot9ew9DdRsLnp+7hswiCiLm9iZRWjXdhCWdjPZ1f/AdkdvYx9nWSeO8GhtoaCi4MosoraE1GFLuVjs++QN7rw9DZSrVaQsnlULc1U2Y0klIUxOpKnN3t7P/hH+ObmDouOd3ShFJWQrXNihKKYDjRiffqEAUuF7r2ZhK3bmMIBnE9dJbI8gr28nLs5wcRrg1T+NKnyHm8NOpk8oC+s+047Xw0hqDVkPB4cTU2IDXVkVzdoPaRh0h4A6hKizF6PIQ9x5miawf6iE3NkQiEaPm1f0BqcQXLwAm8N25R0dKMuqmOwM4etp5ORFlHUSqJWq0hFInS/du/SeDGLeSHH8J3bYiKh84g2m1EVzew1lShrqqgNBZHkiRydhumQgfGE10IKhVVkkgmkcL60GnsNivJSBRNeSlr712j9vlnyGUyVJQWk45G0MgypU2Nx0G2A/34JyYpe+xhxFiMwqpKVGoJTXUlTekBoqEQmpoqxHSK8PwKKkGFSlD41KdfRBMYJ2BKc3XSx931OAtbQTLZPIUWDctbPjz+CO/f87G5d0RLTSHPnDAjihXUFiq4gzlqiyRev+klnUoRTRawtHGAL6Bn22PCH45h0jko1WdxmssIJVT4ghG++Ew9u34V/fUavvHONjptnt98upilnQjbTjN1ziwn2wqpcEi0lOt5AxWZTIYLrRoupwykc9BVAXZTBYfBLM0VBqZWg9SXWSm3pjjRUozTJjPQJPPORIB/9FI7O748Qj7DIz1l2E0SkayTUguEUyKhcISTrS5ayiT8IR1HgQKMBi2SKk9ViY3majvpRJSGygK6KlXYTWpeHo7S234chBuNHWe69gZsCKLE+VYtkVgp6Uz2+D2e4cBrprJEzRM9RsxyBZ5AioutGoSclX1vkkK7EbNRprvORLlDzau3ojTXl/NIu4a/HA7Q21ZFMpHg+bMVCJKINxjntz5Zz+hqmid7jAzPBXFaZQbq1WweJvjN55rZ9CoUOT8GNpryC2Oj/b+CIHQdr8gmx5U0URRlThCEHwDzQBb4rY/CRPsJ3BIE4T9yfLce+7Dzwzv0n4WPxY2mKMr+/acHeJVjk+/wvnuM+88PU7T+dWbgA5uHiqL8iaIofYqi9Dmdzo+0V6HATnLXTWxqDnNbM1ImQ3B+GZPRSFFlBZqcgs7lJB4O45+cxdhYh6qslMC9GQwlxaSzGZLr2xgqy0iEIxxeHcLQ3UpGr8Pz/nUMXW0U9HaSXlnHPzpBy5d+FSmdpaanG4vRSHp5Dbm+mngkQtzrR+tykkwmCU7O0vTZFzFVVRIenUCIxNAVOji6NYqxoxnZZkUIRRBkGcViJr3rxj86gaaxFvfkDJbu9uMqpOOTaDIZtGUl+FdWUXb2KH/uCYrqakgk4oSHR4m4D5FtNmS7FbG0mND4PfRWKwCxu9M0funzJGYW8N28jaGzjWQshlGrRa3TYj1zkrk//SYZrRpbayPJ9S30Wi2uwVNsvfoGht4uYtEI8ZlFKp97kvjUHAmfH8FmQagsJbS4TN6gR9faQH7PTeOZAdKrG+R33cgVpVhamvCubxIJhrCdPUVsfBI9AqbyEnL+IAajEdvASeJTsyAIOC6eJXR7nHwogjzQRz6XR4wc8elPPcF+pozVgxwXe4u4PLrLo31FPNRdRDSepKHayb/8Yju+iMLZnmr6Gwy8ORZC1og0lJkotuR5/baXC71lOGx6VndC/M4LTVQUO5C1Ev/HS81segWmd/J01+iYXz2grliLRS9h0qT52qU1SgoNnOtwMLmZZuVQwWnRMLwQ5en+QhZ346TSOaxGkVONOv7LWzs0VlgoL9RzZTpKb60OpzHLnjfNTkDFS+dLeGvUw9P9DrxeD7//7Vk2dv1s7oe4t+QhL6hpqdIzshDkTJOe2lIDnkCC/UCOp04W8fKNHe6uhnikx8nVSQ8Luxm+8Fgp24dxDsIqPnuxlKH5OEvbEZrK9CRSKbRqFefbdIytJjCbjXRUSExtppB1Gp7sNvHd9/cZX03x6UEbSi7D9SkPtS6Bhzv0DC8myKHFZDSwsJPgy89UM7UexRPKYDHqyefzvHo7xFN9VjLZPFaTTGetGbc3wtp+HK1awKrL8OqwG5tJxxceK2NuJ8NOQKS2WEsyGccXCH6k//2fBxQU8sqDtb/VOoryeUVR2hVF6VAU5dkfs3JQFOVfK4pSqyhKo6Iob/8tlhngmEL9rzi+E/oK8Ac/dcaP4Rdu2QiCYABUiqJE7r8/xvHmXwN+Dfi395+X7k95jWOWxfc5JgiE7rvZ3gX+b0EQbPfHPQb87s9zr+l0mpzHy869GUxFhXgXl3HfHkNnNFDW2sLGxBTO6grUE9PoNFrWhkZoPHUSDbBw4ybNZwZRpXPsb2xiun0XnVbH+vwUao2GZCaFd2cP6+27iLKWw+1tZL1MZv+Ag/VN8pkMmUQCZ2sTmVgMbW0l7vdvUPnCs4T9QVKbW2TDYYwmE3vLK9grykkFQxzuu8nE4ijA/tQMrp5OHF1tRCbnSIbDaPIK4UCA7L4bleJmc2ycxtOnOHj3GoE9N66qSqJTcwiFDlTZPIlMlq3JaSoUBVEFEgKLH9yk5kQvW5PTGJwO0teH8W9vI0kSWpeTcCpFanePMrXE0dYuiiBgrCgjNrvAztw8OrMZ1e4eyViMzMwCoqLiYG0FbW0luWyG2M0Ryp99ElkQmPvan1Hx1CPkI1G8kzPkkkmMVguRYBidxUJWBdl0hmQggO/mCDGPl3Q2Q4nJgNfrRS1JWI98pPUyEbcb8UYYtShyOLeA1WTmT//zH3GuqYw3L49QUlnPB1evUeIo42yHi0QyydB0gH1viJNtlRx4Rda2j3AfqdFKpaTTKda2D9FKJajIc29hH4exlANvDF8wxdC8nn1vlHAkhiBWMLvixiCreSWTZH3vCJdVIOvSUVkoYzQaKLJq8MVEplbcqMjR22Bmz6+gUgk81qnnKz9cpbbMyv6RxN5RhMm1INmcwMrWET8aUVNRANcmA9iM8PbdNJFoisW9DH2NTtwhkcFWO0VWgTdH9rEZRCYW0sQTaYaXM6Ao6PUGRmd3ubVgYu8gyJkL5aBkMck6DrxhXrutRtbpmJg7LtHg8YWZXAzxuUeqIB1FNKjQqnXMrh5SVqinrNDGm2NBQuEEVxJGlne8JDNZrklqTEYtV+5sEInnEdR6ro+ucaa3ClnMsO1J0VRhwGWV+MO/nKex0sHc2hG9rWXcWc0yfHedvtZyhhZVCEIetzfC8IyPSDLP6KwbnWxidTfErjdBV62R10eiKCoti8ubP0/18MD4O5Id4GdCUZQLf5v5H4cbzQW8esxoRgK+qyjKO4IgjAE/EAThN4Bt4MX749/imPa8yjH1+YsAiqL4BUH4PWDs/rh/pSjKz9Upq9FoKDg/QPB7rxAPBHC1NqLWaqh6+jFUJgN1ikLS70fT3kRkdBJnTTWaljrUej3a0XG0PR3Er92k8uIZRI2WvE6Dye3G3NWKsuvGHk2g62xBbdCjOTwkFo4gSxKlrc0oqTRBj4fEgYfk7gFJrxffzi7OmTlykTAhr4+yZx5H1OkoBzJ6LYGtbcpam7H3dSKazUi5LPlslnwgTDgUIpdJo83nqXhoENFhJxWNU36yD01lKYmlVaoevYBoNCCXlxC5M4FnaZWyR8/TWeIiurOPrrWR6O4+rZ98lnwkSnFtDabSEgzd7ag+GEIrSegqSslPz2FxFaJtb8IUT1D4yEPkDg7IiRLV586QyWXJHfkxFBeiqq9CdHuwxyLo9DqSfvBu7aC7NkQ6myWVSCBks8dR9moJZ30LmUgUvcWCrrOFfD6PEouRNOqx9feiGh5FlESM7S0Ed/eO/arxBLH9Q1KBALUXzxK4eYeSvh6kplpqRIme9nIev3CC7373FXoabOweBjnfYWdsPcPnH69mxZ3mwJ/imZN21BqZdDrDqUaZSyMxWurLOd0k88MhH196qoZ4SsBkNhOJxBlsNfD+dJ6GygLONmqQVOWEYmmePWHGVWDgIJChp9HG1mGch7pcBON5Bhq1HAbM/H/svWeQZFl23/d76b2tLO+ry/s21V1tp6e7x8/O7qzFLnahBREMiqQQoBgkQ4QkMhSSQpRAAEESBCSABBfLdbO7Y3ZM90x73+W9N1lZVem9f2lePn3oZRAfgMEgAI0WiPlF3MjnTmRERuS57/7PPecoFQqW3DECkRw1TiNyRUIUC7x+2sX7MxlODdRxdsDG9fkUF4830lKtR4nED26s8YXzTQTTSr7ybCsVhZp6l5beNgVbvjxalYqvP3eEbEHAbpex6Co0OqHFpeXGXJzOJjtVZonXzncgK5UkcmWuHK9hdifNi8etzGylqK2y8NJxKw9XQSFAMp1nYz9FbjuBXt1MOityEKywsKthzxtDp1by6gkzRkM3SAWO1CqoMmm4MSFw5Xg1C7s5vnCxA7tJTSBaJpLIkEobyItKvnSpm0C8yDm7BaVC5vKgFknqIpnJc6pTzXuTSn7lhTbiWSUjtgrVNi0qDRzts/NPf3+PSrnEr77YjF6rYl818tfpHj4RT2M2f0Nao/0FCILwP/9Z12VZ/l8+kf1nSZ1/Pm9fu8of3LyGdXSQzPYehrpqyocBysUixWIB6/gYgiCQfjSJSqtDf2KE7NQsFauZdDCMnMnRcH4ctc1C+PYD8mKBhsvnSU/MksuLVJ0/Q35xBUVTA0IwTDYURmMyYhk7SmRiBmWhgK6vm/K2m5IoYjt9gsSjSUp6HdaebjLT8yiqq0isrNF8/jQlXxDTyVEidx5i7DqCFI5QlCroa2oQd9xUJAm1VoPx5DGyEzPIkoT13EnST55KroYTo0Ru38N5bpz4vSeodFoqpSKOi2eRSiUyU/PIJQnLuVMEPriOuaONUqmIwWaj7A9SMhvwP56i5cXLZNe3oCThePYcCqUS/3sfYmtvQd/XQ+j2fUwN9eiOtJGbXaRSKFAoFal+5gyRmw+wnDlJZmKGSqWC5eRR0jMLKCoV7BfGERdWqZTKVFx2lAoVWbcHx6ljIID7p+/TdOk8+WyO7N4+jpYm5GSassOGKpMhHQhBWcJx8SyCUknyyTSvjB6jHDrg9FAzy3ffoKcW/vkfzvPFy32c6dGz688go6TBoeGjhRwum4b2GoFAAsJpKBbLqJUVRtqMaJQVfvcn6/zmL/ehVMCdFRGlQiCTl5CkIldGLKRyZd55FOQLZ2tBltkMCkSSRV47aeXWcpFEKstLx61sH2aZ2U4z3G5Cq9WyeZhBKuUZbjeTKekYaNZwfy2DUqHkfL+RHz+I0OoSMOm1vHlvn19/vQuzQcWbD6NcHjGz6S3S4lLy79/e4vPnW3nzrod/8IVOqu063noc5bkRCw83Cjw7YOB339zmH3+5kwdrRTLi07bKObHEzG6RjChztFWJN1Yhkq5wedjItdkMMgJHXGV+eOuQF07W4w4+3exwssdCKpunWNESzyl4pl/LB1Nx/JEMLx53MLeTpcph5mSXnjtLWXIFCY2iiFiQ6Gy2092g4fFqnGCiQo1VoNahwxuvcLRVzc2lHEaDlmcHDfz0YRQlJV4ac3FnKYlJLWE169n2FyhX4GSXEanmIq994zf+Uv7ir5rU2TrQJ//mT7/3iZ79uz1H/0rf9f81giD84z91quPplug1WZZ/9ZPYf1au5mPY398nFYqgOfChb2siOb2AzmLBf3BIKZlCo9GiVKkJH3opF4o4s1nEchH8AVQmIyHPPlXr1eSkMgqFksDKGmqLCbXZSDkcRalSIiaT5PY8ONvb2F9YpOf0ONnJGRQ5kYDHQ4tOTzoYIp1MotU/fat2P3xCezaPyWRk8+ZtmoaHSPgDGGtdpLd20dusLHzn+/ScO4PBaGDphz+hdWSITCxKIS/SrNXhWVzCXO1CtbjK/uoaCAqs+TwVQcH87/0hlvoGkj4vttpaVI+mUKlUTwti6nWU7j4k4HYj/jwAvzN5E5VWg72hgYpUIX1wSGRnD6lURlI+DQv6trbRGAxIpTKBlTWqDXoStw8ILq1ibWrEdXKU7TfexjnYT2Fji2QwRCGXw+CwE97ZpXlogLznkMj2LtWnT2BqrMf//nUkpYLCxjayWCAViSAFw+hkmd2VNay1NRTiSZJb2zjqaglsbKLWatFMzqFWqwnNzBOpbWB/d5977/6A8eEmlkoydquRSELk9oqCtZ0wJ3prOIxXWNr24TDrCcYsiEUJl1XL4sYhep2eYgkCoTBGvZYnG1kqgpYPH25y+WQ7u/tBSmVw2QyUZQVuX5JVXz0KAaaW95FluKpScH/WzWhPPRM7ZXLZEh5/FJfDwtzaLi6blitHnVybjvHN5xrZOMzx/r0tzhxt4+qMjF6r4Z17OxzrayKVFrk6E8dlN9PsUvP7P3NzYaSW20sFNGoVLovAQGcDE+sJBKUek17P//hHs1wc6+YHd4LE4lluLOb58MEaF48383hdRQWBaw+2GOyqZ2pHy/2ZHcYGmnh/uozHF6dUKmMz1HNmpAm7QeIjT4RSqYzZ3I1OpcLjj2A2GXnnUYp8SSCWyLAXreLu7CGXTvdwdTbLvelNzh7rRFAbWFjexWE383i9xI43iz+cxtDfzE9+ssLRvnqyBSvbB1F0aiUKZR12k4KJpTgqBVQUOm5Pujkx0EgmX+SVMSffvb5Pa+8ar33KPuRpi4G/HS/0siz/6z99LgjCb/E0zPGJ+Gyy+Riam5uxbLtQmYwkd/dIR6NEA0GMZjMauw3dcD8qvR5H9mmxTPuFM4QmZogc+LBXu6gd7EfVWIe+roa0ew+Lpwr7QC+plXUiB16ci6vEDg9RarQUKmVanz2P2m5D19ZM5MY92gb7MY6NULh5H0dTA8YTIygDQQzrm2gEAd2RVtrsFnQ2C7tvfYDe5aD+2fMU8iL9X3gFQaqg6+9G93gCy/hxhMdTGCsV9KN91KYSqJRKjEN9WENhysk0zqPDIAiIXh8NZ0+iW15Dr1RiOX3iaSuDYgmF2YSkVtPb0UbpwIt2ZBBXIonOYkLM52l96TKqQpFKewtKhQr7sWEqyJjqaskf+tD3daN4PIm1uRFDXTU6lZp8IoGQyZPyB3CODGDo78GZFykWi6iOtNEmV9AfGyK0vMLBxhZqswkpGifo9uBsb8U4MkDo8RSN4ydQVjvI7D+NPUlmE9vvfkDflz+PpaMVlUZDQQBDeytFsYDr+CgtnUew2cwEJB/P9CkoFCV2myyMtBtordFxayJLb4OaWKaM7UwbiRyc79Hw2z/eJJbQ0H+kkbJU4eXjJt6blvnW8yY+nMvy6gkNm54aOuq1pHMWdDod4906PMEcQx0uOqpl6uxaIokqFIKCF4+ZyRVaaKzScKpby1uPMwx21vHScRMlqR4kBy+QAAAgAElEQVS1Cma3UwSiaR4txxg9Yub0aCtmvYbLIybW91MUuqoZbDPhtPWQK0hcHNDhC0vEUzkKpTJatcxvfKmL+6s5XE4j53tsXJ2OIQhKqu0WzvRoub8m83debqdQETg+0IJYFhhuUaJUCmzuVXG804QvXqSnxcmVYTNmo5o3H1VQKhXI5Twus5JlL7x4spptX4HBJgUui5pIVGZxw8s/+3ofH0ynGO5rwaAq8u1XutgJlnjlhJXNPSsnOjTs+pI01VkZadUgFiUyogWnw0pfnUz5VAelMlwZ0lEoVOG0aTnTo2N2SwRkxvvsTGzmuDLWSEutmcnVCH/4vof/7gvthI29/7/4kb8tMZs/AwN/iarPn8loH8Pb167yk3KK2MQMGquFcixBfN9L7ekxVHU1xG7dx3npPPnZRSo1LsQDL1q1GrFcphxPUPfiZaJ3H2IaGUBcXkPV3kIpHEVIZ1C3NqEolikHwggKAAHL6eOEb9xDMJuwdLSQW9tGqHai0+vJew7R9HZS3NghJ0u4jg6TXttCWSrjW1vnyJc+9zTBUgCNUoVppJ/MkxlEtRpBqyZ9GKCmrxvZaKBy6IW8SNlhQ6/TUjgMoB0dJHn/CbJSgW38BJknM0iA9dgghbUtTMeHyS6tIZQkinkR69mThKdmia2s0/TK82TWt9AIAs7zp4jcvI+sUGA7e4rM4ynypRJVZ04iSxKpiRnUVU6KvgDOS+cQ55bQjvSz8/03MbY24WxqIOM+wHHh1FOp68EUOrOJvCyhVamQ8wXEXA59dwdyNE5ZENBpNJRiccynjpF6NI0MxHM5lJks9a9cIX7nEc5L58gtrDydmD66g0Klwn7hDFUrW/RYtXzlpVP88e/9NuV8jHODdmbcFUqFHEKlQG+LjendEp8bM/PuZIo2l4BCpWV5L0WDy0BrFXjjCvIluNCnY8eXZf2wQGuNnmhOSaEkUyrmOd9v4taSyGunrNxfzRGM5Xl1zEYoUSCQhFJZJhgXefmEjYfrBZrsZSSU+BOQF0XiySKDrXpUWgO+cJrRDguBWJ6irGUvmOO1MQtvPopRY9ejEkrUuYyE43mcVj0LuxnyhRIjrRom1mMEogVODLZwslPL5n4ah1XLm/cO+dbzLTjNKq7O5bEYlJzu1vLmwxihSIJvv9jMj+6FGe2qYrBZzf3VHFSKdNQZEUWR793c55njbZzr1XFtKsT5QQe3VkReHDHywWyGE0c03JxPcKrXwYavCAi8csKCJ5jl3lKCcwMO1nwSYl7k1ZMO7q7kiKULfHHcyvduBVCqVHzlrJNYusTMbgm9VgkKNY22EqG0klNdWv7PH21zut/JmT4LH87nAZmRVhWrhxKdR5//1GW05v5e+Z+88Yl6i/HrA2O/6DLaEv81vUQJuHgaK/93n8T+s5XNxxBPxPDevI6jqZHde4+oam8jtOfBVl9LZddDTi5TuXUPWavBGAxzMD1Lz6mTmLRaNt0edPcfo9ZoWP4P36X37GmU/jCbDx7Re/E8UjLD/uw8glpFIRrHWl+LemYRtVaLZ24RrdVMJp/DFJRRnjqG99Y9dIde2r78OUqbu4ixOMVsBq2gIB2LEZ2cA+BwdoG67iNkYzEKoojZaEI2uYhubGI/0oZCqyETjWE0GrB1dRC5cQ9JUJB+OIFcEImHQuhsNipOO+n1DaqtFoo1Lja++wa14yfYvH6V9mNHST2cJLbrRmu1kPXsk/T6KaZTaDQa8rkcuVQac6GIqNWSOTjEuL6FqiwR2NxB7TnAVFdL+NZ9BIUC8eEUpUIBMRBia2UdrdkMDyaRpDJxr49UMEL3517A0H2E7PQ8JbUSz4/eouv0SbRKFduPJtCZzShVanamZ7FUV5NJxFEpFNgm50GtZuM7P0LrtGNLZYh6/VSQUT/RcuA5pGRUIuVSiLp6vB4/L+rVxNNJLHotzwxb+Xdve/j65UaSmSL5bJK31tOMj3awux9l358geaSOqaVd6qosyNSgEDTcm90m1llHNl9kfMDFUKudf/u2m5M9dma301x9sMvR3jpmdkvIKHjn5goDnS7aajT83jt7/PKVJlxWA//Xj7a4OOoglpQJxTOk6i0sr3mxGI24fUn88RJrHj+CADcNWkLRDFueCH1H6llw+8nlJY60OPGHU2iUYNAZsZv1eMMFpAqk8hLuiEw4VyIQTnJ9JorVYmRu3U+VVU8iYyeXzxFJ5rk2HWd9N0h9jYPdxzEOQglEUSJbVCHLMql0nlwRFtxPPw06FU1WiX/xn5Y53t/Imk/H4qafGqcN90EIu1XPWw8LGI1GZla9uJw2spkUkqzi4WqCaw/3GO6u42cTcbYOYrTVW5jZySKjYHbdi9OiQ64omC4UOT7QzPvTaTLZHC5rHVPbIg/mdulrq2bDbyeayOK5/fAvPdn8Vfm0kjo/JV75U8dlICjLcvmTGn822XwMdpsDXW01slZL4/OXyPn89H7xNUpeP7ZzpzBkc6x/5wfYO1pRlSXqe7rQHR8mOTOPq7kB+7lxsr4ANs8BusEe4lNzNPb2YBjuQ5YkpEeTNJ46gXjgw2A2oT82RPrmfWraWhGqXERv3SOt09PgsKE3GCik0yTml0m79wnEYvR+86vEN3doPHUSS2M92sZ6jDYbYiyG49w4++9/RCC8R2NLAzqzCVmtIOv1EXd7SKrVCHo9QbcbY10dTc8/S+zuY1p6+9AP9ZGbWyR66MVy+yGCTkMmkaSiUtE0NIjaZMR8fASFQoFKo8Y4NoIuX6RQqkJ3dBiT203s5l2is3Nk9g5Q63UYertILa/R+fIVUus72C+Ms/PGW6g1atq//Bpakwm5VKRYW4uMjKW3E02NC9W9x+iNRvKJJMHvvkEqHKHzuYv0/crXqIQiSGYTLWMnkJApOx10nDuDXCrhKNRSlCT0owMYtRqCm9sYTCYsZ06CIKDR69GNDtKoN9Jl0fBrX3+OH/74PWYfZZjYzDGzsk9Ho4O3cwb8kRTXpiLUO9RIspKh7gYuD2opl5sRC0VeGDEg0Eq+UOBEm0BZqqC62Em2oGB22cPanppC0UIwkiaXNzDUbqah2sapXiuNVVqiSZH0eAcWnZL2GgXvPvTyaNVKMJZDFAsUSgIXB43s1+lxWQTuzRY48Cc53XuEBpeBEjqqrFpGmgWEShXprMgLowa+82GEKpuOV09YuTqrRKlQ0lKrZ80PR7vVnOxQMrOZZGsvQk+bk3/x7UFuLOZ58aiJityATqvk8pCBtx5JjPVrOd1rR6FQ4DAKXBly8m9+Euf0YA1n+oy89TjOv/z2EFO7JRrsAm/cCKJQKHF7E3Q0OXjhqJUtb46eVgenOpUsbYFUlnntlJ2VvQzHemo40a5kYl3gwYKPU691cfFkJ3mxxOdOWjCZTKTSOUbbjcxsZfjKpU7CyTL35jw01dgZ71QjVZRsuXU0VqlRxwp89XI33liZZ/o1fDitoKW181P3ITJ8Kv1sPiVUwKEsywVBEJ4BvigIwp/IsvyJEpg+k9E+hv8io2W8fgobOwTdHrp+5ZfI7HnQVEDd1kxudpGo20PtsWFEZMRwFLvTQa4gondVk1/bxHx6jPTsPFqjAWqrEZJpxEQSY2szxd19BCCXy2Ls60IOx8DlpBwIoSyXqRj0hJdXsXV3YWisIz0xg0KtxjDYh7i6QaUi47x4lvjtB8g1LvQOOwqjgeLaJsgVCnkRyajH0tFO+PZ9lEol9S9eIjU5jySVsR0borTjoajXojGZSW1uU/XMWWI37uC8dJ7Q9TtUKjKCVo1CqcI+doycL4AUjqBz2hEDoafFNLU6ZKOB1MIKVqeNaCSKnEpTf/kC0ak5dI31qGUBXWc7+WiUw2s3aX3mDPlEgrQ3SM3YKCm3ByGdo+riGaIT0+RjSewdbUgqJcmNLaq7O5FiCcpVdiSPl0QojKXahe3sKbJzSxSSKRzPnCF+9xF6qwX9cC+Z2SWocqAWBCSVkqLHi8qgp5LLo6h28Y2jYxTyWXbuvE2fPYZRXSCaVRBJP80riiWfSjrXZhL0tZjZCZaoNZXR69T4kwKDjfDD2z5eP1dPlUXJm4+TKAR47ZSN63NxKoIGUHCmR8NOoIw/UeHZAR1LHpFkXslzIwZ++jDO66dtvPMkwZluDbcW4rh9aZ493sBYt4V3n8SJp1JYTHrqqkz4wlmeP2rhh3fCWC16Xj/t4PZygVgyy+dPWSkUJeb3iuRLClqrZD6aDvOFc408WUtgMygZaDfjNCu5u5wjkc6j1WrRqJ4mV+54sxzEJCxGLb5Ijv5mHYm8ksEWLT+45eP8kIPFvTzPDluZ2ikRT+Y4cURNMKXkWIeOO4sJVtwJBKlIWVDz7ecaMeqVvD+dAYWC8z0avnP9kG9eaSYYF0nk1URSZU51qvhwOkxXaw3HOzS89fjpyvR4h5oNXxmzUc1Ak5Y3H4Y40mTnZJeeu6sFBLnCqW4N700mCEWSvDJWRTwHu6EKnxszM7WRxKhTEc0qaBm68qnLaI39PfI//P5//ETP/g8jZ37RZbR5nhY+bgU+5OnmgG5Zll/6JPa/SIU4f+GIRiJEbz9E8IbQWKwkQmHSjybJeQ6I7+4RuHmPVCRCqSCS2j8Er5/Dx5PEfX6MzY0EHzxG3VhHeGKa7fuPSQbD5EJhcp4DVIICtdNBLpOmolYi1Faz8t0fUZEkhECIzWs3oVRGKRbJBsMUI1FKoSiq2lp25xaQfAG0Fgv7S0ukH02RyqQJTUwhJVPI227Wbt+FikxZKhOemSe7uEIyHEUsiAQfPOFgdR2pWKIYilLIZpEjCbQNdSjra9n8zg8Q9Hpy0wvEAyFS4TCZaJx8PEH08RSqaIztu/cpR6JkxRzi7j4VSaKyu4d/aZlMLE7C7SGfzlDc3kPK5tj98BZyIkXy4QTxuSUy8TiRjW2KkTiBpRVK7kM0ZZmNh0+IPZ5GpdeTjUSIe734Hj0muuuhmEhRzIuojCbK5TKx/QNiB4dkJ2ZYvX4LpdmIuLRKMhLGu7lFZmOHRCRCbHqexM4e8mGAvakZwpvbBPf2aUpmifh9LE5OMz89gz8uMe+p8IMPNzEIadzeBGJB5P0nIRAU/PS2m9UtL3thiR/d3GV5y8uDjSIHwSTbwQqPt8ooFTI7+2E+ms+y602zsObFH4rxw9s+hlr1tDtl/vjDfQabNZSLWX5yL8izw2YEQWCkTcP/8/4e294MBr0ag0bBxHoGp03H1LIfqaKgxiyTzJa4vpgnFM9y4I9xfSHP9UfrhCNxfvY4wvX5FO/c2SSRFpnfK3DojzO1lSWezrO8FyOdyZHIFAnHc4SiGW5NbBFO5Li5XOQgqeb9Bzv4YmXKFQX/4b0trDqJeLqA25fEZVVzZcTCf7y6j1kt0uRS8Qfv7BBOy9xdKxKI5RELRfQaUCng5nyM9yaTrO8G8AVifDAdJxBJs35YIJ5XcXtqj3giybWZJDuHKcqSzMSmiEErs7B+gDui4MPHO7gDOa4vZphe9eKNiNyejzC/4afaVOKDyRhmo55iSWLFB9enA5w4omHzMEtJVvEn17ZI5SUCwfBf/Kf/a+Zpi4HKJxp/A6j8XDZ7HfhdWZb/EVD3SY0/k9E+BpvdjkqvR3dsiMjcIoPf/Col9z5VZ06RXd9k584DBn/tW5TXt5Draym5Pdjra7F1tFEORYh49jE1N2Ef6kchFjA6HCiqXKxdv4uzsR4xlSbi9qCNxjA01ONsbUVbX4va6aAjGqMiCJiH++ksS+TyeXQuJ7H1TXq/9mUIhijZLLQ9cx6NRkNt/xHWv/djVHXV5JbXqRnoRzcygA7wL69h6umiWa2hLAhY+rtRKdVUPXOGtNfP4o27NPX3oFlaJbm5RUWSsJ0eIzkxTfvnXqTi3icYClHJZql99iyVUpHmdApVQx2luQhhzz4d3R2UMllqe7uoOjeOwWAgn8ujG+hGkU5T09GOurcLvclA4f4jms6fxuRyobRaaTcYqaiUiMkUnSdPIOXymNqacbW2oGpqwF5VRSYaR93ezNZ3fkizXof9whkMFgu5TAb9SB/2zS2M3Z2oTEZssRhaowlNWwv52UXKxQINr72EQqWitVxGsFmJbm6TDoU5/vf+Lnm/h5f/+39IdPldOmpNbO9byBQU+IMx2hrtvDJeTalUQakU0Go0XBrUcxBIcGHYxZq3yLdeaAdkuhu1vPlYyWBPMxcHtNxVKChJFc72GvidN9Z4vFUGdBz4EzxYryaWLuM+iKDT6wCZYDiFyaChs62OzmoQJTUne7QkMgVeONNOo0vH6l6cUrHEqS47TVXNHEaKPDdiwBuqZrDdwfEjOgB2fGnOD5i4u5Th+GAjnztp42ePy8STeZJZiWyhiPsgQl9HFfV1DiSpwqUBDQehHF+61EWposSmA2/waefS6f0slYrEjbkIDU4jB/4o0UYt+bKa7tYqznWrMepVvJsxcaRJ8zQPTa3j1VN2FtxpWuvaSIkC8bTIP/ulXiZ3SlwZ1pHINmHRC8QyEq+fb0ZQKxlo1vDmQ5GR7mqOtauI545QbVGTTOf5598cYvmgzGCzmmuPDziMWXjlpJMPpxP8Ny+0cH02jj+cYmo9QX+zjoOwxFefbUWn12Cs+cuVqvrr4m9RzKYkCMIvAd8CXv35NfUnNf5sZfMxKJVKCuUSpVQaTS6Poa4Woaaa8r6XYjBCxysvUtz3EvUHkf0B5Lpq6s6dIrO5gyiKDH79S0jxBIn5ZeynjiMZdJBM0n3+DEaLBblUpOtrX6S2rRV9uUzb66+QnF+iXCyittuRrWYKO25QKnCcPUlyZhF1oYSpoRZJpSC9uIJ9qI90KEzyyRz1x46SWt3E2NiAobWZYipD8uEkQ3//18isrCEg4zx9guz8MjqdjuTm01po/RfPYWlqROGqwllfT9Pli3jf+whjYwNquw0xl8NuseA40kEpFCH2aIqai+cglcZZ5aTni69S2PZgrK7COT5GYm4JwWrGcfZpKX+doKT6uYskHk9SkSoY1FqqhgapeP3EJ6exjAySj0TRmwwItdWY+p5WKvCurFLxhzAO9uIcP0bBfcCR0ydRFYpk/H6osuO8ME5maoGm4UFyC8ukdtwYO9pIJZNEbt2j9dUXaPvia6QnZkmsbaJta0ZRLFFVV0dNRxuHOzuc6G/i1NgIu3ET6WyR8YEaUgUVJ4bb6G0xs7xf4r3pJM+NmEmlsmTzJUa6a7g2FeBcn5me5qc9V9Y8afpbjBTEDO88ifPskBGnoUwsVaCz2UajXUYuZfkHX+hFLJSxmPS8crqRI7Va6q0CQ51VOK16nAaBzkYTO74M+UKZ2wspnjtqJ5CEIno6m6xcm44x2q6jv1HJ2oH4tPpxXsQTLrHiTvHa6Tq+f/OAC4NGpHIJty9LY7WB3/hiO9vBCodRka89106pokSv1TDUrGZ2t8isu8xYp4FwXMQThb/3ahtrPpmywsy//PYgKI14Y2V+81dGcAfLDLTZ+eXLDUzulAjH8zQ4VFwaNnMQznFx0MBP7geQZB1DLWpiKZFqixKrSUO9tcLcbgGLXmBtN05/o4aBNhPBWJa3HvgZ6zJwacTO9FYWl0lFNFVBrdXhsmlJpPOsH2T4+1/sJ56RCccL+KMZHqwVeO6Yg8snO1CodMy4JT5/0spop421/RwV6dOPncjIVCqVTzT+BvBtYBz432RZdguC0Ab8509q/Nlk8zEURBFJpSS3uILSYCAbCJLz7LP81ruUCgU0yRRr735AJhJB19dNfu8Qtc1GWanEc+cBBMIYbRaCK6uU17dQZ3KsvHOVdCDIwfoGqXCEwso6mw8fEfb7CTyepFLjYvt7P6agUVECDmbm2ZtfJHH/CVqDHu/mJokHT4hv7hAPBvHdvkfEvUcsEEQlFgjML1IWRfQuJ8F7DzEO9pL3HKBz2NlbXCbzZJpcOs3KvfvkDv1o+7oRKjJFMc/yD94gl0qjSKYIbG6R2twhM7dEWhQRNGrsRwcJTc+i0upQqlXEtnbIFkTKe4fsz8+T8BwgLqywe/8RCpcTlUZDLpFAqdWiUCiwnBnD9941FNVVAGRSGdSCEnF9k9iBl8D2DhqxQGx2ETmVoVQs419dJ/14huLKJusf3USoq0bSakhOL6BvaSS+sU0k4Gd3dp6oz4/76nVK/hDhrR1i/iCp+WWktS2K2Sx7N+6QjSfxzS9iGOlnXcyweOMqY0f7ADh54RLfu+2nxlwml8uxsOYhLmp4594WmUyOBXeegRYN//d7uxwE0/gCceZ2c7w7mWRj18+95RSrnhTesEgglGBqK09fi5kf3T7kS+frWXDn2TpMM7WdY203xMZukFhBy49ubnNjNkgoWWb3IMaDRS9XZzOohTK3l7JYzXpUSgXZVJzdgyiZgoptT5hrczm2fTnWDvLoVCUuDNqY3UpzbznOskckFEmy5CkjSxJPNtLolBKPNkvIsszcRoh1r4SgUDCz7GbDJ/PBg12CkSQ3lopseiKs7fp5bzLK44U90ukc708l2fEE2PSEubucZs8XYzdUZm5XJJnOcXs5y2CLlmV3ingqz8weLGwGCSULXF8UeTi7Q6ogcGe1RDin4/sfruKP5NncD7ETEHm8KaLXaZjfCLHqlfhwNsnN6UMkqczdqS0S6SI3l0TUai13ZnzsRaDKYeR//c48RQkMeg23V/JsH8Z5srhPPJHh1kqBm8tFNGoF71y99ek7kZ9Xff4k4xcdWZZXZVn+dVmWf/Dzc7csy//Hf7kvCMJPP87+MxntY9DqdNi6Oln//htYq2uoslkw9XXTqtOhrXKg6zpCnWcfU88RIpOzhJZW0Go1VI2NkvP5EZrq0VutqJdW0I4OUpEkmhMJ9M2NdLpciOk0utFBmhJJNA4H2u52UrseKsiUQmGsPUcoWEyo7HZs504RufOAmvY2rOPHUUzMYKxyYDs2jNXpRCkoSMfjNAwPImtUBG/dJxuNkljbxDrUR94fxNnRgW6oDyGVJp/JYOzuwHvnPoVonM6vfwmD9ufNpeqqGfjCy5QCEQzD/QTe/oCDQx9odaQTaRK+AEVRJLi3T9/XvoixsY5enR5BKWA6NoR5c5vE8hplh51Kuczmoyf0aNVUEJ62zq6pppLJEN7dRaFW41J10PbCZcqhELLdSmZtjaqL52lVKlFU2dHo9ahbm9A/mSLtD0I8SWjPg2NpDX2tC2tDHZV0DsvYKNIHN1D3dtFcKlIqlLCdHkMqFFE8ekLNkXZUOg3ZdJrIo0nERBJ3Occff+99FAoFxVKJaEIkW1RhMerQanU806chk23CoFPRUa3gOx/5UAkCLjP8k1/qZ8Ur8fyogbfFDPvhPJ8fb+K9ySRGg5axTi3XZ0Ik0zk+nM0wt+ZhsMPF50/Z+JkARp1AX72CTLaeYqnCi6MGoBVRLHJpQItYUPE7P9mk3mUhkTKw7UvT3VaDJBV4cbyJhmo1DoOd33ljnUjcQkFSo1FDqSDy8rF6VDRiNSqYXUmBoORcn4HOejXvJ1WcGungYp+Gnz7K09ZQxeVhHWKxlhqHnhYn2IzNHIZzvDxmx2AwohAkLg0aQBAw6tUYFTk+f6YRk1mgwS4ws54jmsgyU2Ok3mXEatLjMhQ42lXN8XYtZr2CXK6JarPMcKsaTzBHb1sV/Y0CrTVdhNMy4106nqzFaa23cL5HzdKemoWtCv0tOsSzPaSzBS4N6rg+m2Wwu5ELfRoWdlL82ucHSeUFLvRreftxnoZaBy0Nzqe/6VETkaTIvRWZFy+d/9R9SAWZYvkT7w7+m87HJnh+trL5C8i5PfS8+hImlwNtdRXFtU0c4ydQZPNEH07ievY86lIZZbFE/9/5BqqKTGp3j/rLFyhsuYlMTNHy8hXy224SDydxnj9DcGIGTWcrtvHj+K7ewNh1hLJcIbm0hr4i0/ftX8as01M89FNzfBRdcz3JjW2MVQ7Mw/1sfPdHaHs7UTfWcXj1JqaRAYRKBaPRSPXZMfKbO7jammm/8gzOpkakaBxTYwN1z56lsLFLYW2bmrPjVGIJqmprqRsfw3vtBrr+HopKgdiTKYxH2hCcT2ue2ew2qjs70LY2UdfSRO3IIPbhfrounKF86CPlOYAqO8V0lpTnkObL51GXJIy9XahUanq/+VXEaJxipUzfV16nkMtTTqTo/sZXMRgMmBsbUVdXUU5lKO976f7W18iFwqTCYSy9XWSjcfbfvUbbq89jMplQCkp6vv5l5GIRQ001erUW01AvB+9fp/2rrz9NHNXpMR4bInj7Hvn5RaouX8B2bIjI5AzN58ax9fcy1N5B/8mzfPG1Z/jGl68Q8SzS2+agq0GL2WTgTK+Bxb08JoOOUCTFR/NpPn++ic62egoYqHFokaUCkVQJndHMK6eqmd4tYDZpSWXyACRFJf/Tt/oRBJlzx7poqDKyFZAwGAxcHrFxfzWDUa/l0pCB28t5DFo1Lx0382ijgFGv5PRoO+3N1VwZMTM+3IJCqKDRajnd72TRLXJtNsl/+3ovTpuJU51qikWZgQ4ndxfCjHQYOQzlaKl30t5cTX2VgXcmk7x4zEKlAtfnkpzps/LSCRuTWwUsZj25osDkdoETR3S8eNTKo/U8Oo1AOitycy7K0TYN2XyJQFrFyT4H2748apWC+ho7zxxtoN6hYdZdoKvJzG5I5ksX6lnzVfhoPs1Lx234Yk9lpVl3iVdPVnFjLsZoh54qs8ReqEQsr+FL52r5ow/2MRn1/MqVVjYOcjiNFUZb1Uxti8gKPZJUQpIqHMYFRjtMCHKJ958EGWk3MNKqJhhJMd6j5Y07fhb3JV4/bUev1376DkQGSap8ovG3gI8NTn022XwMHrebyMoamd09lEolC3/wn5A0GgrxBPlYApVaRSEYZvX6bQSTkVImj1xlJ7W6gaHGRVmvhWwec30d+f1D1DYLoSeTZJNJyhu7lFe3CG3vkKYFFdMAACAASURBVDk4RKPVsvfgEZRLFOeXOFhZJbq5Q9kXRFsosfP+NeRsjtTcElKlTGrXQ3xzh2wiSejmPfYXlolnMiTuTxBwewhsbKHK5PHOzLF/5wEloFwoUkgkKCOT29pm5+YdNEoVurxI8tCH7N7DrNaQ8AUI336ApBTIbGxjMBlxnjlJfmEFld6A4/hRdt58D7nKQUWvJTo5h765kYrTRnxuAX1zI/YLZ4jfn0BSKEjML1MURfyz8yiiUQKLS1SA+JNpol4f+YMDMk+m2XoyiVJQELs3gRhP4tvcJrGwgkqnIbCxTW59h/jaJuaWRgxVTiSDEffbHxBPpSlueRCzWYrL6+TSafZX1qjsHeJdXEJhMpBdXUcOhslEYpBIEZ+eY7R/gGe/8lU+uj3Db/2r3+alQYETRwz80Qf7jHVqaXAZOYiUCUfjbO1HCEdTbPklljb3iURi3J0Pk88X+Vf/eZl4Msu6H248cbPuDuILxfnNP5ynzq5m3lMhGE2zuetDVur4cOKAxbV9rs3lSGdFFtYOWDgQ2PUmmFhwM72ZJJnJ8dajCKe7daQzWT6YTZFM5zgI5Vne8PLWwxATi3tE41nWvGVaq9X87EmM+iotF4ftPFyNY9QpqDaVWdkJUWeD33pjmzaXknSuyJYnjNWkQauSSOdKzK772d0LcGdig2KxyOMNkcU9kcUNL1NLe0jlCpOrIQ4iJQrZJMhlDkI5lHKe//27KziMYNKreO/RAZlMHp1GwfZ+gLnNCHu+GNU2LWqVApuuyJ/c8HOhT0exVCGXLzK5GkEnlLgxE2J3P8T1hSyeQIK9cJlkQcmN6QANDoEld4oP7m+zsnVIm0vgDz/w0FVT4e1HITJFFZOrAdZ9JTYDAsFImt2ggqlVP5FEnjsrBXzByKfuQ2TkvzUy2l+Vz2S0j6GppYWqxkYcz5wmtb5F0/FhTI21JHd2OZxbwNXTgbaYp7qzA2PnETJ+P/mDQxLBEJG7jyikMoTce5hrazhYXaHxxAmqjo9SDIRRNdajqnbSrYCKWEDV2kTvqy9Q8AUxXz6PK5FE77BhGB0gteOmaewo6roahGIJZ1MT1UeHUN2boHrIhf7EMJ5/8wc0vnAJXbWLDrWGfKmAsrMNcyqFrboKhUlHeHKa6MYGjuYW6p57lkImj1BlR93UgO3gAMHleJoPMzeP69lz5AMhDqfnKR2UaVWq8K5voDUbqVMrKZWKZHb20FvMhPcPsU/MoJIk4l4/1XPLyILA/uIqroEeqk+PkXwwQfvQIJlcjo4rF9FbbUgGHa6CiLGzjXwySdPpMVR1tRhbGok8eMLgr34TIRyloBDoeO4SGo2G/avLqLQabGIeRbFEaGuH/l/+GlqHDeXmFtqhPiypNAaLFV1/N50FEQVgHuwj+GiS6t5ubGOjbH3vp0w/eEghm+XWWx9wxFnk4aYNUBFL5ZnckYAys6sHNNcYOXu0DUGh4Jk+LQcBM+VKhaEOCwCH0SLnhpw0VWkAAYtBRTabo7HWwYWhp03mgnEztQ4dp3u0BKJ2KhWZF0YNvDsp4bDoudCrJpbQYbfoaK8zUfAWmN718dhmI5oqkkpl+LWX27m7qsVirOFUlwqHzUQsled4u4pYqswPP9rjWF8D3nCWXLbA7HaWnkYzVnOaSrlMuVyhWJY5iJQ4DEQxqivk8zoMGgVKlYqBI04aGqopFkqc7nkqqW4eWqi2a9EpJeSWGkba9cxthEn6wrQ4XZQqKoa6ahlo0VOWKigVCkw6gVimTEdjNdV2Pf6pCMVCCaWiilCyxIY7wgOjkmQmT53LSkeDCbEok8rGGe+tIpEt8o++0st2CBodSrK5AkvuPM+OViGrjCBXKMsC8WSOolTNy2Nmrs7E6Wl18dywgemNJM31DqRiln/6jUHuLqU536fBq6r69J3Ip9ep8xeBP6t78n+9+VlS55/P29eu8ie+XXKrm2jNRszHhonevI8sg+PiaZKPplBqNJhOjJCemMM0dpTojbuU9FpcRwdJT86jPdIKmSySWKQQiaMd6ELI5snv7SOpVbhOjJJa2yThDdD83DNUikXE1S1UKiVSsYjlzBjxe4+xnx8nfOMeKo0a/cgg5QMfci5PJpFEqdU8leIOvAgNdQjpDIYjbfje+xBLYx2y3Up6fRur04n5xDCRe48pKpXYe7rIzC9DXQ16u/VpV9DBHuIr69hamimEIhirq0jsepAFBdb2ZjLbbkyjA1SCYeREmnwmi+PiGQIf3kKlVKHrbEVTrqDtbCM7s4hclhBzORzjx8kvrCAoVZjGRghdv0sRqD53msLcIhVJwnp2jOithxhOjFDa2ME4Okj60SRypYLl7CmCH1zH3NlBIRbD0txAcm0L29kxcksb5MolKoJAJZujqr+HikZD+NEE1ceGyWzu4Dh5jPzsEsbxY+y/+T7Vx0YREimOyDK/dGWU2zdu0Gf1MbedJJUt8twxJ7eXspzssfDDmx6+daWRRxsiV4b1TG2XqLZUyBRVeII5Xjvl4KP5LOOdanZCFULJClq1gCjmuThk4+FaipF2C+t+iaKYp6fJQF4scGM2wlcu1BNPF8iKEM8rONWl5e6ySEYsoVIqONauZTtYIZMrcLzTwE6wQr6kIJPJ8vwxKxqVgvemUuTEEi8fszC3VyKYEHnluIU7q0UqJZFEWsRkMvHaKRs/fhBDoMLnTtp5dzLBi8esrHiyVNn0bHiL2I0Kaq0ygZSCcCLPeK+Zu0sp9DoNUiFLg0ONSmvkMJzGpAWXw0pXvYqfTaa4PGRgwVOmVFFQLJUp5HMMtegIZpQcBPO8Nm7jvakUzw6ZntZVE5QY1EUGmg3shUScNiPuYJli+Wms5fpcjFhK5PljLq7Pp+hrUIFCTS4vMr+dotap58Kwk0yuiC+hoMpYplBWsBuqUCqXUKs1vHjUSCZX5MFagb4Tz/G5Tzmps7q7U/7y7//2J3r231/63C90UudfhCAIz8my/NGfd/8zGe1jiMVi5JbW8G9tE/H68F6/w+7cIslYlOi9J+zOzhPZcRO5+4h4KEzgxl0sx0epPnmc/Xc/RKh2ktrcZflnV5EKRWSrmb233kcsFBCLJUKrG5R29tBptUS2tkhNzpFfWifm9bE7O08qn8d38x7GgV7ESBS1xYx3e5eS55DDiWlEjZpsJoV3aZlyuYyYy5GaW0TpsJGZnse3vkn4wEdicwf/yjrFXJbsxBxGkxnf1Oz/296bR8lxXoe9v6/3daZ79n2AGQCzYRnsO0BQNJeYEm1aSijrSX6xchznSHl28nJerKessnPixDlPL0qkWDyyImszSclcIAoLARIAsQ9mMPu+72tPd0/vVd395Y9u0CMGAAeEBsuwfufUmapb31f13Zruul33u3Uvyswsxg3rmL18DWt+HqZNlYz+8gw5O7bR/fPjhKamic95UJaWmOsfIDmzgDAa6frhqyRiCgONzUSCQWIdPQSWlpifnETv8TPd3snIWyeI260MNjQR8iwye+0GU30DqHYL8129RIVgtrMb75UGhpqa8czMEmjrRDHo6PnBT1kKB5k8c56xzm6mB4eJNDQz1tFFzLOI1Wqj7dU3iITCKDPzxEIhdMEw9sIC5rt6iM/OkxyfZLqjC//wGO59Owk2t6MIWDh3mcD8AhGPh7AOWq9f4/L1Vtz55bx2LURL3wKFuS7+9K87kEJH60iMZFLSOqpi04V59fwMezaYsJr1nGsco390gVMNc9h0EX72/iwLi0EuNAwwNO7BaYVTTT4cViu5mQa6B+cZmfJzczBC34xkYsbH9X6Fca+R//l2N7OeAGdaQgxOLDK7sMT03CLffqMXjzdALKbwX37aSXWRnmhMwWG30D0a4Bc3/FxsGiKmxLg+lOTUlQFkQqVlKMLc7DwtfbMMTPgYnVrg+PVFFhaXGBid50p/nAK3iVfOTdE1FqJvzMOZy92Mz4fompKcvDzA+GyAlpF4Kt/a8AxJYeatS+NMeeMYzQ5OXptgzBPnRFOA+UUf//EnXXh9Pk6+38XE5BzRmMJ3fzFARS48vd3O6Zt+8twmbvT4mPEEae8dJxFP0DioshDSU5FvYikYxmLUca55AX84VeLhWn+MTIeVH54cYMoHCyEj4ZhKYbad631RGvoVdlaaWVfo4FTDDDs3mJj3+EnEAlztmONS5yJDY7OcOXflgd9DpHwwbjQhxOeEEJ1CiKQQYteH9n1NCDEghOgVQjyzTP5sWjYghPiTuxy7XQjRdqdlma53NDSgudHuisvlQlVVNvzOC8RHxpA5WWzeUEFwYITsI/uxOuzEojGctVUYZmYZOHkGs8lEMp7ANzNH3q4d5B7ejyEpMbkzseTnMHbhIgaTmew9O4gterFtrSU076G0fivmLDfW2irU8xfJKS5EWq30n38fk06HzHIhY1FKqzZiraog8u55oj4/TrsT+3oLhCNYHXbGm1sx5WSRvWs7NQikUU900cv6557C4c7EUlxIaHoWd0E+jqpKJi5cIRoMEbhyA51ez9LsPMrgKM7cHPIP7cWUk034vSCVRYXY99SjdvZgd7mw1VZRGY4SDYWwbq3BteDBbrfj2FOPLCmg60ev4t66mbKtmzGYjTh2baPjez8iODFF1rY6kj4/WWWlZO7YhslgSIVr11QTuN6E2W7DXVeTKlVstWByONBVrmMDSQwZGSg6HdXPPU1CUVH1epRAEM/0DHlmI+6yYszFBZhysykaGMKcmUG4f5i+9y9jstup+dI/IOvwXkKt3RiiCi/+8T+nPLnA7h3V+D1TzA0nOFRjYWymhLJcMzsrzFhNJRyuNvBXJ+eZml/i5E076/P0VFUUkogn2FdjY2ROYc4zy0vHijBaHISicapKzJx8rZPdm8u40iMYnVrgn32uiuwME29c8fLUrmJqyg3kZBg4dcmIxWLhmR1OJE5ah8KMz6tkuzJ4crsLh0VH/+QS7zTO09w3z+bKPDZscbN1vRGTvpLcTD1WY5yjO0opyLGzpcyENxDB4XRAMo7N7uS5nQ7evKajfoOLwhwdZTl2rndMUZRjZ39NLnN+2FjsYGOhAaOuHJNBz+FaE4FgBtmZZsKhEDUV+ezZYMSok8TCRViN8PS2TJr6dYQjCZ6oz2XKm2D/llyqis0MTARo6PETTRpo7Jxiy4Y8nt2dQzTuoyCnlJ1VFv7LTzuoLM/lnVYj43NB5jxLfO5oCefal3hmbzEleQZynIJgoIQcJ1QVmMh25FOUJfjBL9vYtqmYd9vNqPE484tLXO70MTYbJDvDTGWRA7vVSDBmoKz6YeRGe2DRaB2k3uz/7nKhEKIWeAmoA4qAs0KITend3wZ+A5gAbgghjkspu25z7FsJOL+S/vuj9N8vkKqevCI0N9pdePPUSV6N+Vk4d5FELIbV4cB9dD9KMMjc+StkbanBVl7K3DvnURMJsg7uI3C9ESSY6zah9wUhx01y0YvwLZHIzsRgspCY8xBZ8qMvyMOR5SLcPUDm0QP4WztQYzFchfksDY0iBZjLihEeP/btW4i0dYKqophM2EqKCLR1YcnNxri+DKV3EFFSSKB/CFdWFmGZwJabS3xgGGExY9+xlfD1JpwHdrF47hKqlJgL8tFHYyyFQtiKi0kMjaArLSA6u4Cropxgdz85Tx4mfLOdaDCAZVMlwuPFvKkC340WLDlZGIqLUHoHQFEJqQq5+3bhvXQd9+G9BBtaMJpMxMx6guNTFBw5gL+xBX1hPmadAVPlOhbPXcRWVIi+uADf1SYyigswbtqI7+JV9AY9riP7CV9vJhIJk3XkAMEbqaqiGft2Eb3ZRrKkCKV/EFVVEckkeU8dYeG9S7j370qVaFBUFCSOsiJiU7NEYjFsOj1Tg0M8s/8gv/PFL9Dwsx+xf9t6Gs/8GGMyitVqZM6fgIRKXpaVGW+ceX8UNRZFpxfsr8ulbTjI3ionLrueN6750MkEFkMcNS44uCULj19BlUY8gQSz3jDP7nTx9jUvm9fbKXJB/0ySvZusnOuIEg6HyLZLcrNstIwoHKnL4GzTHNurcqgpMfOzSx4ScZXP7MumYUAhFhck4lGqim30jAU4utXN1d4o8USS53Zm8PoVDy/sc3OmJUwyHiEQSVCabaQkz87YQoJDtXb+9rKHT+9x8X5XlHA0DkmVT23L4J2WIMmk5DN7MzjdEuZTWyw0DipE43qSySRPb7PyxlU/Fn2MI1vc9E5GWIoI9AYTtcU6uidiWMxGpr1xCp0qRbk22sbiWAwJNhXb6BwNU1Vqp2dSJRIOUZxjJMNuYXYxQlamnf7xJSxWCxvyIJbQUVdq5sTNMAk1zFP1Lk7eDGDVK+zckMH73VGSyQQ6nZ7nd2fwbqufLDsU5jgYmZcsBiL81j43b1/3cqDGTjjrwVfqzNlYKX/zv/75RzcEfvibf/++3WhCiPPAv5BSNqa3vwYgpfyP6e3TwL9LN/93UspnbtfuDse+LKU8+FGyO6E92dyFxYV5pq9fwWQ0Mt03gCUzE5PVitDrme7pTyXW9C5htNuYbu3AXVhAcGmJ4JyHPJ2OZFyFiQlyjx1h4fI1DGMhnMcOszAwhM3hwF5TxcCPX8WYnU38/SvYbFaGrjXgPHQQJRDAO7dAoV5P1B+g51vfYd2xoxCOkPD5Ub0+Jto6yCktwe71suQLwPAI5S9+Gt/NNhZ7elGLipi42UxB9SZiVjOJRJzQxatYivIZO36ajIJ8CjdUYgzHGHr7JOVb6ohMzTLf2UPenu24Du5m8u3TOKsqmRsYINLaTvWxoyjdAwxeaWD9jnrEzDyeiSmsThtFzzzJ+MmzFO3eTsTrY3Z6GjUQpLy2muneAbJKSrFmuuk6cZa6Y0dQ2roZaW6j0mrFGI8z2dGJtJqx9vYx1t5OXmUF5uZ2xnp70QmB7vI1Rm62UH3kEMH2Lqa7etD1DZC7tRZjOMpEcwvo9cQTcZr++8vkVlTgn5rGZLexbkM5ajBE3hMH8NxoIR6NcfPKFawGA0Ndvcz0XOWFnSbAxCvvL1BVZMKdqeMHJ/sIRhW+/n/UcbFbz2f2uflvbwwTiSqocTCaTARCCgsLPrZUlXCzawidyYpMJrjc0k91mZPf2JnHiRs+8rLtDM0o9E2m3mwH8Aej2G1W9tXZuNQT4Xf2u/ju26OMTnvJynIx5QWXw0xD2zSnjEaUeILiXCd1FTZ+dHacaDSOzmDhfEM/zx+toqk/QF2JgW+/OczvfqqULKeNb785zPO7S/izH3fz2cNFDE8l2LfJzPdPjeO2CzyBOIGwgtPpYHzGRzgS4z1zApcFXr3g4x8cLeTlX45RkW+moTuIiRhXO7yomHDYbbxzpZsn9m6ifULHiQsDHNtVRInLTENvAJtFT2v3DAiIqsVI9Lx1cZy4Gqe4MJNfXhplR20JUkLn8BQef4jsDBPzCzae3ZnJyEyASNDHnC/B0KSOytwkr743i9Tb+PTuDG4MKuzbaOZnlz04rUa2rLPxnbfHeao+C0Uf41+93EJFcSbnWyIUVs0+8HuI5J4CBHKEEMt/Cb8spXz5PodQDFxbtj2RlgGMf0i+9yOOZRdCHJJSXgIQQhwA7CsdiGZs7oIzw4UzMxPH7u2oiSS6RAJDbRUGq4UaVSUpJbb6OkJnLlC0qx5DWTEuvx9XTjaOvbsIt3cz3NBEpjuL0NQMQe8ituxsgh4Pi5PTFFvM+Ofm2fz8s5hdmSw2tpBdXoZlxxbMfj/lm/PIOLCLiNeHEotiMpsY6ewme+MGio4exGq1EQ2HcB3ch93ro+PHr5HT2UMiHicSDFFxZD92q4VEIonZ7SYRU+i/fI1NTz9FSf0WdPEElm21BK81UlpTjfvIAeIxhembrYQGRxHJBLNDw2CxYnG7cdrsGNaVY3DYqInFUi9NHtqLvNZEYHKC6MAw8wNDuIoLMebm4LDbcZaUEIpGqfrsbyGDISJKiA2feY64P4Bjx1ZqImFw2IkGgtT/4f9JuKMPx5ZaqqMxYqEw1u1bMI9PkJmTTdbBvYQ9i+jzcrAUFZBsbMa9sRLbhg34W9rIKS4h52jqR9bi4DDFzxwjt6OH0FKQyNgUkaUg3lfeoOyZYxhVFX1mJtXb63Hn53P6O3+KjOWj1+sZGJklGLCzuzqLLdVFIKFrUqVzYIbsTDMkYxzYVsyhWicAr19RcNnzOFxjZHDcyY5KG0VZRpQ4mA0SX1hgN0NTxzC5WS7mvUucc6ait/qG53HYjZiMxVxsHCUeL6WmxIbdaqK2xERZrpE3rgbZuWU9v7HNyjdf62N8epEMcxGbSjLwBlNRbfOLhWQ5jKzPE5y6PsWsJ8DAdAxlPMKsZ4meyRhWkxG9MVUmYcqjsOgLUl1WgNupIvU5PFFrJBzJRcoke6rNXOoMMTrtp2Ewj1hMZcyjo35DBmV5dloGA/zmbjfz/iideU6O1poZmYlQUpDJrk0u2odDjM14sezOo2p9HlJKjtRasVsNHFdiSKFnXXaSZCKfT+9JReydbQGn3Uy+E95vm2P7Bidmo56YKjAbDTjtZtqGlsiwm1GiYb75sylys6wY9VmMTi2SlWGhf9pAJBxhelFlX42LwVmV/VsLqCs1MmbIf+D3ECklicSKvUcLd3uyEUKcBQpus+vrUsq37tTtdsPi9vP1HzXQLwPfF0Jkprd9wO9/RJ+/G4jmRrszb546ycs3r2PJdKJOTGPbswPvuUtkPXmYSFsnEUVB8XnJ37cHoyuDpWtN6Ewm4k47RrOZ8MQUSZkkEZdkFeaRzHSgDk8ilRiqouI6sp+lhpsYM5yYN24g0tJGPMvFUlsH5Z9+Bk9LB66aTSw1tpL95CFmTpwls6Kc0Mwczh31JPqG0FdvJNrVg8zLIeHzYzYYiMzOYa/fQnJqBqHGiVlMWO12ggsekkmJ0+EgtuDBuX8XnrMXMNsd6NaXoI/EiIRCJBIJMnKzifmXsGRlEezpR+oE7sP78Zy9gHF9GWaTETWmEhqfIGtdGeq8h7jdgq2wgKXm9nR6m61EOvtAp8e+ZzsL5y+jFwL30QMs9Q1gNhiRgQChOQ+2wlwc2+qYv9KAs6aa+PAosiAX4QuiejwoySSu+i2ogyPE/EskM53YCwsIdvSQeXA3kdZOZEEexqQkMjmFyHUTmZwht64aS2E+s6feIy4lBrMJ7HacpcWY8/OwNrZRnumkvthMYvISFflGTjeHiCpxolGFFw+4ONXkJRxNUl1iAoOdwUk/Qhh4YZ+b0ze97NloYymkcLJhji8+XcY7zSFePODiQmeUSDTMno123msLYjUbUVQVs1Gwe5OdbIeed9siqAmoLYbFEAzNKBgMOn57fxbHry2yd5ONnqkEvqCKEk9wpC6DsdkwFquZ6cU4FbkJoqqeQAy8AZX8TIjELVTkJVkI6uibCGGQMbZV2gkoJmZ8SQ5XW3i7cYkXD7h488oiRoMevd5Atl2hOM/Joj/CjCeKMNnIsiVx2syMeZIc3KjnF41LJOMKT2zJZNKvY3w+xtE6K11jYcKqkf1VZrrGYkx4VJ7d4eRqbxSDQceRWitvXPXx4gE3Z9si5NgUGnp8VJfZqSlzEo6qzAf1RMJBpn2SZ7ZncK1fYWu5gZEFyaI3TE6mHqPJxnwgQYYpQX6WmcHZBJlmhVy3ldahAKVZApfTTv90mO0VDvpnkohkhByXDWvZg3ejZW2okE/9xZ+tqO3PXvzCI+1GW3aODFK2w38vY9Oi0e5CJBImGo0y39yONBjQ6XTYd2xj7sx5PHMLGGMqwel5wp29BK8303vpKkseD9acbOJjU6g+P/qYin9wgNDMLEr/MBPtHcxPTmHduI65cxcxFhUQm5tn8dIVworC4tVGooEg4eYO4qEw4yfOEk2oRBpbGWntIBEMI4Rg8tS7JAx6jDYLCYOBpeY2nJsqGW9sJhQIklgKMN7czqLPT8amDQT6h9AHQ+Tu3EYsEsE/N8fcxSsYLRZmh4YI9w8THZ/EGAiRu30rielZCIQxFeaTyM9lurefcGcP9rxcBt8+BQte5KKX6e4e4sEQwVAIQzCCUFTCgQBzw6PEegbpu9bA4sw0kRstqKEgM8PDBK81YlwKMfTeBaZ7+xhpbUP1LRFs7cRVv4VAcxtxsxlbfj6hqWn0JhNZB/YQbu0koROomU7GL15lobWdsBKl+VvfxR8Jk4jH8XX0YM/OImtjJbGFRYKLXqbPvo/BZGJhbAyHw8HEtRvIqVkibZ1Mk6C3pYmjh/fRu+jiQtsCMh5kZNJDIBTm1M0gM4tRJud8hFQj47N+IrEkh2qsHL82R77bgscXoWUoyNR8kHdveil1q3zvxBilWUlKs83857/pojRbhxINkGk38fd2uTjfHuTEDS/7NpooyIjzTpOXucVw+mlnhvc6olitFv7ipx0EI0kuNw9hNSbpm44TSZp57Uwfsws+woqO95rnUKJhwkqCE1cmiCUEzaNJXnmnlw0FBo7VZ/HmpTnqyixEwkG+8cMOCrNMXO1TcNot9A7PMLewwMC0SmOfj7FFwfWuOQx6EzNLgl9cGaO9b5yz7WFy3A6mF8L0TEvG52NkZ5jwhpL88soIUSXOhc4Ix98fwGI2cq0/wYWmESbnAlzpU3DbJd89PsL8wgKTXkEwopDjstM6qnJjIFWbZnBGYWbeT8uYYMEX5S/fHCCe0BOTBt5rmiGqxBkam2cpHEeJBunqG+Pdphlu9nqQySRvXhynZcCHWRfj9M0F1LhKeY6eiy2TNDW3P/B7SFKmAgRWsqwSx4GXhBDmdOLMjUADcAPYKIRYL4QwkQoiOH63A6WP8bvAV4E/EkL8GyHEv1npQB57Y7PS8L2Pg9liJaNiHaqqMtM3QKC9i/joOAPXbiCUGBmH9lJSU41j3y7imU7cZWW4dm7DNzBI29l3SSDJPnaQ/A2VZB/Zj6G4gLL9e8jdXE3M62PkRhNyaoaAx4N33mwPFQAAFRxJREFUeoasrXXkVa6npLoK5/5dZO3ZgWdiEvfWLUSSSYr370KUl+I+fAB0gpmeXoLtXfiGhljyLDJ3rREhBNbSYhIGA0aDHpmIE+7sJezzMzc2QaS1E//IKPMjo2SUlOA6sp/SzbVk7d3B/MQEc2PjRJta6Tp/CYPRQKi9i2BvP7nry3FsqSUWi5FfWYF15zbi4QiV2+sxVKxjoX+AiZ5eIuEw7qIi1m3ejHX3doqqNpFdvQnr7nqsGRmsq6sh8+BudJVl5FSsw11bTfXTxzBkOLDUVOFpamXw+g3U2Xmize34JyaZ7O0j0tjKZHc3noEhlnr6KN1SR/FvHMNVXYUrP4+8nfUY9HrGOjpYHJ9g6OfHUaJRbDnZ5D95iLiismH7dqI6He7SIoyba7BurQO9DpFdwHdev4LMLOUXF0fZWJLJP/2tddRV5PDczkwKczLYWVdOfbmJRCJJ98A0reNwvX2KqCoxmMzYrRaqK/J5fn8uNquJgbEFukcDDEyG2LqpiIpCC/2TQXpH5rnUq5BMJukZ8dA6IWgfjRCJRjlY58Zo0LO1qpRjtUZcZoXyYjdPbrXw6SObcNqtHKo2UeBUOFRfzI6NmeRlwOD4Au4MK3qho3ZjEZ/aYmFziY4MuwWJnhvDSYKRGCcbAwxPBTiyq4JDtQ4ObDLhDUTZvXU9iWSScDjM83tySMRVaivzOVpn5PldGVSWZKEoCbZXWHmyzsSWqmIOVFu4fHOQ/tEFGns9VK1LucPKswUlBZk8XW/jwCYDR3eto7zAyaFqC6XZBrqGZ8l02Hl+Tya1GwqxGeOcvthLJBKmIl+Ql+1kc1UJR2uNZNr17Kgr5XCNCZ2AXVvWs2eDhck5PxZDArPJhNNuY2d1Pi8eysftNLBpXR4vHsqlvsJFJBzl7fPdTHnjfGZ/PptrN330l34VeBBZn4UQvy2EmCCVlfmX6ScYpJSdwGtAF3AK+IqUMpGuS/NVUkXQuoHX0m3vxlvAC6RKQoeWLSsb4+PsRhNC6IE+loXvAZ+/Q/ge8DHcaM3XsdrsqLPzmLfV4r14jZjRgKO4EJ3HS1xRsVRVIqdmCRv12HOziXT0EAWsWW4swNLSEq5ttSg9Q2Ts20WwswvVFyAUDpG9rY5Y3wgkkyTdmTjyc/F295F7YDfe81egMBdzZgY6jxfrlhqCvYPEPT4cdVXEJ6aJJVQc2VkkPD4CnkVch/cT6+knuuglc89OlIFBVKMRs04P0QgxoxGr2UTC50cxGTFbbUj/EqrDjlmnIxEME4urCIcDs9GAEo1hdTqITc+QzHBitdvxD4+gt9lwbliPv6Mb68YKdPMeSCQIh8Jk7txGsKmNhEFHRl0NSncfMb3AWVGBv7Ud94FdBBqaydi7k/5XXqf6i38f/5UbqRdXz18hYTVjKyggNjSCs3oT6uwcSixlOHyTM9gdDkI+P+7d9YSa2qCoALPFTKi7D8uWGsTSEsmFRWLxONn7d+O9eA3XgV0oHb2oiTiW2iqUiRnUcARbTjblsTiHD+5npvEigakulryzHKjJoG8myaI3wHM7M7jUG2fnOni3LYJeDwadoKrYQDRuRI2r2MwmBqbCHKq1c6EzRCKusKnYhs5gYWBiifI8E2aTiXFPgt0Vgit9KkLo2FFhoGcySSCiUpQpycq00jrgY1+Vnb5ZHRZdmJ7xCC8dK+Z0c4BPbbFyvjPCs9vt3OgPM+FR2VKqZ8ITJ8vlJBoOUpZv58ZgjPp1eqIKtI5EETLOk9sy6J0WLPpDPLXdzYlGP0/vyOTGYJx4PE44EuVT2zK4OaRSV6JjclGysKSwqcTOwGyShBKhKNvC6EIcq16lPN/CuCdJKCapX6djzicY86g8WWemdSzBzGKET+9xcb5LYWsp3BhQiEUjbKtwMDAriURjFGcZcNpMzPoTRKMx9lQ5udQVpiJPgN5M30SAQpeB4lwbTf0Bspw6NhbZaRtTKXUn0RlM9E3G2L/JSN+MJBQMsL/GxYWuMDs2OBiaiTPni3C41kkk+4kH7kbLrFgnD/2Hlf34P/G7X36kX+oUQnRIKTd/3P6P+5PNHmBASjkkpVSAV0hZ3l8LoWCQ0PAYcYOeufFxJt54G9umShKRCDKRRGY6GW26yey5S4jcbNRAiNHjp7BUlmPMcCBlEtVqJjg6zvDP30ZfVEh4YoqRi9cwuDNwb6xk6PVfYiwtImrQ4e/qJa6oWIsK6fn+TzBXlGFzuRg7cRaRk4Uyu8DUlRvgsKGGI0zcbCEZU0gYDIzcbEGfm4Myv8DUzRZEphPF62WmvYvY7DxJh43prh7U+XmkzUpo0YclJxv/7CwDNxoJj02QsFmZ7etDDYUwZTiYa24lPDlF3KBnfmyc8Og4cYMe3/QMiteHGonim55j8eoNdLnZzI+MooTCKIs+vJ4Foh4v6lKAhelZIlOzqOEw4WSCwVfewLw+laHAYDETnZ4jnIjT+4O/Qe92YcvLY/gXp9C5XaiKwujVBtDrkSYT853diCwXlpIi+n70KobiwlTgxNunMRYVoFPjjL53EV1BLrbyUvp++Cr6LBeqx8fIzRZ0Odkkl4LMNN5EDYWI6wSj3V10nnqL3ds2EoqmfsGfb5njfEM/drNkyqPSPzLPaxc9bK+0MjHjQyYTGAwGrnYu0DMawqxPMDbj45ULs2wtt+DxhmjsD2A1xvF4A9zsX8KsTzAxPc9Pzs1TU2zEs7jIz9+foyxbEA0t0di3hEwkyLDCj9+bpiRL4LBbCEXjTHhi2PQK335rhA0FekZmFd5pmGR9ngGDwURDt4cMS5Jct5W/OjHMhgIDCann5+9Psj5PT12Zjb88PkpehqSqxMa3Xh8kP1PPnE/hcvMwhZmSmhIL3/zZADlOiT8iea95lpgSR1FV5jwh1heY6Bv3cq11GG9QJZlI0tA5jd2gEI7Be83TmEWMGW+c5t559CQZm48xNDrH65dSOvsCURJSkONMcLVtgvaRACa9pH9skXm/gjcQJ7Dk52KHH6shiUjGaB1aQlUTWPVxukcCRGIJ/EtLvN/uxaRLYtFF+OHZKQoyoTTPxn97a4QchyAajbPgj1K/zsrb1+foHxj9dd0a7ok1lBvtihBiy8ft/Lg/2XwWeFZK+Y/S218E9kopv/qhdn8A/AFAWVnZztHRlX3oYrEYgUAAgEQigU6nQwhBMplECIEQAkVRADCZTCSTSRKJBEaj8YM+er0eKSXRaBSrNZVvSlEUTCbT/7auquoHfZev36n9/awnk0l0utRvjXA4jM1mu+t47nZMo9GIEAJVVdHr9eh0OhLpQlV6vf5XrksymSQej3/QPx6PYzAYVny+e70ud7qmy897r9flbuO5dS2Wr8fjcXQ6HTqd7gN3ya31O12Xe9V5JZ+dO7W503VZvn7rswwQiUQ++Cyv9LwGgwEhxK8cR1EU9Hr9B58RKeX/9nmRUqKq6se+FsvPZ7FYcDgc3Av3+2STsb5c7v3G11fU9uyX/vGj/mTTBWwAhoEYqUg3KaXcupL+j3vo853C+n5VkIpVfxlSbrSVHtxsNmM2P4S05BoaGmuGNVI+AOC5++n8uBubCaB02XYJMPWQxqKhoaHxK9yKRlsLSClHAYQQeYDlXvs/7nM29xy+p6GhofHAWENloYUQnxFC9JNyo10ARoCTK+3/WD/ZSCnjQohb4Xt64PsrCN/T0NDQeCDcKp62RvhTYB9wVkq5XQhxDPj8Sjs/1sYGQEp5AjjxsMehoaGhcTvu9x2aRwhVSukRQuiEEDop5TkhxH9aaefH3tjcK01NTQtCiHuJgcwBHnw92YeLpvPa55OmL3w8ncvv54RSrilj4xNCOID3gZ8IIeZIveC5Ij5xxkZKmXsv7YUQjY9yOOJqoOm89vmk6QsPS2e5lozNC0AU+GekatlkAt9YaedPnLHR0NDQeFBIKYmvnWi05alp/vpe+2vGRkNDQ2MVedyfbIQQAW5ffuDWS50ZKzmOZmw+mvstXvQ4oum89vmk6QsPQWcpU+/aPM5IKZ2/juNoxuYj+DVUynvs0HRe+3zS9IWHpfOamrO5LzRjo6GhobGKaMYmhWZsNDQ0NFaJtRQgcL887ulqVo3VLMr2IBBCfF8IMSeE6FgmyxJCnBFC9Kf/utNyIYT4VlrXNiHEjmV9fi/dvl8I8XvL5DuFEO3pPt8SQtwuKeoDRQhRKoQ4J4ToFkJ0CiH+KC1fs3oLISxCiAYhRGta53+flq8XQlxPj//VdDqnW9UWX02P/7oQYt2yY30tLe8VQjyzTP7IfReEEHohRLMQ4u309iOp7633bFa7eNrjgGZsboNIFWX7Nqksp7XA54UQtQ93VPfMD4BnPyT7E+BdKeVG4N30NqT03Jhe/gD4H5C6SQP/FthLqnbQv711o063+YNl/T58rodBHPi/pZQ1pNJqfCX9f1vLeseAJ6WU24B64FkhxD7gPwHfTOvsBb6cbv9lwCul3AB8M92O9HV6CagjpdN30jf0R/W78EekKkze4hHVV66Z3Gj3i2Zsbs+qFmV7EEgp3wcWPyR+gb+Lj/9r4LeWyX8oU1wDXEKIQuAZ4IyUclFK6QXOkLqZFQIZUsqrMlUQ6YfLjvXQkFJOSylvptcDpG5GxaxhvdNjD6Y3jelFAk8CP0/LP6zzrWvxc+BT6aezF4BXpJQxKeUwMEDqe/DIfReEECXAbwLfS28LHlF9JdqTzS00Y3N7ioHxZdsTadnjTr6UchpSN2YgLy2/k753k0/cRv7IkHaXbAeus8b1Tv8ibwHmSBnGQcCXrjMPvzrOD3RL7/cD2dz7tXiY/P/A/wPcukNn86jq+4DcaEKIz6XdqEkhxK5l8nVCiIgQoiW9/OWyfQ/UJawZm9uzoqJsa4g76Xuv8kcCkcrf9LfAH0spl+7W9Dayx05vKWVCSllPqp7THqDmds3Sfx9rnYUQzwNzUsqm5eLbNH1E9JUP6smmA3iRVN6yDzMopaxPL3+4TP5AXcKasbk9a7Uo22zaFUT671xafid97yYvuY38oSOEMJIyND+RUr6eFq95vQGklD7gPKn5KpcQ4la06fJxfqBben8mKXfrvV6Lh8VB4DNCiBFSLq4nST3pPJL63opGW8lyP0gpu6WUvStt/zBcwpqxuT1rtSjbceBWZNXvAW8tk38pHZ21D/Cn3U2ngaeFEO70BPnTwOn0voAQYl/60ftLy4710EiP5a+Abinl/7ds15rVWwiRK4RwpdetwFOk5qrOAZ9NN/uwzreuxWeB99I3m+PAS+norfWkfuk28Ih9F6SUX5NSlkgp16XH8p6U8gs8ovo+InM269ORexeEEIfTsgfuEtbes7kNa6EomxDib4AngBwhxASp6Ko/B14TQnwZGAM+l25+Avh7pCZJw8A/BJBSLgoh/pTUFxDgG1LKW0EH/4RUxJuVVLW+FVfsW0UOAl8E2tNzGAD/L2tb70Lgr9NRVDrgNSnl20KILuAVIcSfAc2kjDDpvz8SQgyQ+oX/EoCUslMI8RrQRSqq7ytSygTAY/Jd+Jc8ivreW7qaHCFE47Ltl5dnPRBCnAUKbtPv61LKO/3omQbK0nVodgJvCiHqeAjuUSEf87w9GhoaGo8q+vxcafn876yobfi/frfpfksgCCHOA/9CStl4t/3AJHBOSlmdln8eeEJK+Y/v5/x3Q3OjaWhoaKwSD9uNlnaz6tPrFaTchUMPwyWsGRsNDQ2N1eLBhT7/dtpdvh/4pRDidHrXEaBNCNFK6j2jP/yQS/h7pNzIg6yyS1ibs9HQ0NBYJR5UbjQp5RvAG7eR/y2p6Mzb9WkENq/y0D5AMzYaGhoaq4ZEfgKyA6wEzdhoaGhorCZSMzagzdlofEIRQljT7x3o76HPV4UQ/3A1x6WxxkhFCKxsWeNoxkbjk8rvA6/ferdihXwf+L9WaTwaaxIJyRUuaxzN2GisKYQQu0WqNo1FCGFPJye83SToF0iHegohnkg/5bwmhOgTQvy5EOILIlUnpl0IUQkgpQwDI0KIPQ9QJY3HGQkk4itb1jjanI3GmkJKeUMIcRz4M1Jv+f9YStmxvE06DUmFlHJkmXgbqQSWi8AQ8D0p5R6RKsD2T4E/TrdrBA6TSm2iofERyE+Ei2wlaMZGYy3yDVKpZqLc3u2VA/g+JLtxqwyBEGIQeCctbweOLWs3B1T/WkersbbRAgQAzdhorE2yAAepQmIWIPSh/ZG0fDmxZevJZdtJfvV7Ykn319D4aOQnYz5mJWhzNhprkZeBfw38hHQZ4OWkq2/qhRAfNjgrYROp2iEaGitDi0YDNGOjscYQQnwJiEspf0oq2/NuIcSTt2n6DnDoY5ziIHD2Poao8UlDJle2rHG0rM8an0iEENuBfy6l/OJq9tH4ZCPcmVIc27+itvKN0/ed9flRRpuz0fhEIqVsFkKcE0Lo7+FdmxxS7jkNjRWj/aBPoRkbjU8sUsrv32P7M6s1Fo01itRCn2+hGRsNDQ2N1UQzNoBmbDQ0NDRWEfmJmPxfCZqx0dDQ0FgtbiXi1NCMjYaGhsbqISFxL7le1y6asdHQ0NBYLbQnmw/QjI2GhobGaqLN2QBaBgENDQ2NVUQ+kHQ1Qoi/EEL0pMtrvCGEcC3b9zUhxIAQolcI8cwy+bNp2YAQ4k/uawArQDM2GhoaGquF5EEVTzsDbJZSbgX6gK8BCCFqgZeAOuBZ4DtCCH26Qu23geeAWuDz6barhuZG09DQ0Fg15AMpjCalfGfZ5jXgs+n1F4BXpJQxYFgIMQDcKv43IKUcAhBCvJJu27VaY9SMjYaGhsZqEYyc5lJLzgpbW4QQjcu2X5ZSvvwxzvr7wKvp9WJSxucWE2kZwPiH5Hs/xrlWjGZsNDQ0NFYJKeWzv65jCSHOAgW32fV1KeWtEudfB+KkymsAiNsNi9tPoaxqEjfN2GhoaGg8Bkgpn7rbfiHE7wHPA5+Sf5f9cwIoXdasBJhKr99JvipoAQIaGhoajzlCiGeBfwl8RkoZXrbrOPCSEMIshFgPbAQaSJVN3yiEWC+EMJEKIji+mmPUnmw0NDQ0Hn/+O2AGzgghAK5JKf9QStkphHiN1MR/HPjKrZIaQoivAqcBPfB9KWXnag5QK56moaGhobHqaG40DQ0NDY1VRzM2GhoaGhqrjmZsNDQ0NDRWHc3YaGhoaGisOpqx0dDQ0NBYdTRjo6GhoaGx6mjGRkNDQ0Nj1flf/BuUl3Pm+HgAAAAASUVORK5CYII=\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "child.quick_plot(\n",
+ " \"land_surface__elevation\", edgecolors=\"k\", vmin=-200, vmax=200, cmap=\"BrBG_r\"\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 7,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAZsAAADxCAYAAAAdgBpwAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4xLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvDW2N/gAAIABJREFUeJztvXu4XWV17//5JiAXFRMIIrceIk9six5FRMBiFbDFQK0RKxbaRxB4ivUHivXCRW2xKj1IRY9WxUaJwCmCiFBSRCFQ0FOPCOEiFxGJgJiSA0WuR24m+f7+mO9KZrZrzf2uueZce+21x4dnPnvO9zrmXGGNNcc73jFkmyAIgiBok1lTLUAQBEEw/oSyCYIgCFonlE0QBEHQOqFsgiAIgtYJZRMEQRC0TiibIAiCoHVC2QRBEEwDJO0o6WpJd0i6XdJxqXxLScsk3ZX+zk3lkvR5SSsk3SJpt6mUP5RNEATB9GA18AHbvw/sBRwjaRfgROAq2wuAq9I1wAHAgnQcDZwxfJHXE8omCIJgGmB7le0b0/kTwB3A9sAi4OzU7GzgLel8EXCOC64F5kjadshir2OjqZo4CIJg3HnRFrP87Oq8to885duBp0tFi20v7tZW0k7AK4EfAdvYXgWFQpL0wtRse+CXpW4rU9mq/DtojlA2QRAELfHsanjD7+Z9zV5482+etr37ZO0kPQ/4FvA+249L6tm0S9mUxScLM1oQBEGLzFLekYOkjSkUzbm2L0rFD3TMY+nvg6l8JbBjqfsOwP1N3FMdQtkEQRC0hAQbz1bWMflYEnAmcIftz5SqlgKHp/PDgUtK5Yclr7S9gMc65rapIMxoQRAELZL71pLB3sA7gFsl3ZzKPgycClwg6SjgPuDgVHcZcCCwAngSOKIxSWoQyiYIgqAlRHPKxvZ/0H0dBuANXdobOKaZ2QcnlE0QBEFbCGbFYgUQyiYIgqA1mnyzme6EsgmCIGiR2b1dk2cUoWyCIAhaQoKNZk+1FKNBKJsgCIIWCTNaQSibIAiClhDhINAhlE0QBEFbKNZsOoSyCYIgaInwRltPKJsgCIKWEOEg0CGUTRAEQVv0EWRz3AllEwRB0BIi1mw6hLIJgiBokfBGKwhlEwRB0CJhRisIZRMEQdASijWbdYSyCYIgaAmRlxhtJhDKJgiCoEXizaYglE0QBEFLjJMZTdILKbKFbgc8BdwGLLe9Nqd/KJsgCIIWmTXNtY2kfYETgS2Bm4AHgU2BtwA7S7oQON3241XjhLIJgiBoCzWnbCQtAd4EPGj7ZansY8BfAf+Vmn3Y9mWp7iTgKGAN8F7bl9ec+kDgr2zf10WmjZJMfwx8q2qQUDZBEAQtIcRGzcWrOQv4AnDOhPLP2v70BvNKuwCHAC+lMHtdKeklttf0O6ntD0maJentti+YULca+NeccWK7URAEQUsUazbKOibD9veBhzOnXgScb/sZ2/cAK4A96t5HWpd5T93+EMomCIKgVWbNmpV1DMCxkm6RtETS3FS2PfDLUpuVqWwQrpD0QUk7Stqyc+R2DmUTBEHQIrNmKesA5klaXjqOzhj+DGBnYFdgFXB6Ku/2quQBb+VI4Bjg+8AN6Vie2znWbIIgCFpCUj8OAg/Z3r2f8W0/UJrrK8Cl6XIlsGOp6Q7A/f2M3WWu+YP0jzebIAiCFmnTjCZp29LlQRR7XwCWAodI2kTSfGABcN0g9yFpc0kflbQ4XS+Q9Kbc/vFmEwRB0BISbLRRM7/pJZ0H7ENhblsJnAzsI2lXChPZvcC7AGzfLukC4CfAauCYOp5oE/gahensD9L1SuCbrH+bqiSUTRAEQUsIDbr4vw7bh3YpPrOi/SnAKY1MXrCz7T+XdGga/ykpP1lPKJsgCIK2aHBT5wjwrKTNSI4GknYGnsntHMomCIKgRWZpbJbGPwZ8F9hR0rkUcdKOyO0cyiYIgqAlCjPaeLzZ2L5C0g3AXhSu1cfZfii3fyibIAiCthgjM5qkq2y/Afh2l7JJCWUTBEHQEoU3WmOx0aYESZsCm1N4wc1l/YbRLSjirmURyiYIgqA1xsKM9i7gfRSK5cZS+ePAF3MHaW3lKsXPuVrSHZJul3RcKt9S0jJJd6W/c1O5JH1e0ooU52e30liHp/Z3STq8VP4qSbemPp/vxw0vCIKgbcRQYqO1iu3PpegBH7Q9v3S8wvYXcsdp8w5XAx+w/fsUC0rHpLDXJwJX2V4AXJWuAQ6g2OW6ADiaIuYPKdDbycCeFFFLTy4Fmzsjte30W9ji/QRBEPSH+oqNNuosGSSCQGvKxvYq2zem8yeAOyiiji4Czk7NzqbI9kYqP8cF1wJzUiiGNwLLbD9s+xFgGbAw1W1h+4e2TZHjoTNWEATBlCPELM3KOqYBS4Bn2TCCwCdzOw9lzUbSTsArgR8B29heBYVCSnmtoXdI7KrylV3Ku81/NMUbEBttusmrttihaFboqK7tc2+tJ73Grhp/Yp9yu3JdlXxV7XLHaJqqZ1Gm1/1OVpczXq5ML1rzyMRRyr36qOspVY3xJt7HoMF7c2li3qp/Z8Mcr96zvePeRx+yvXWebN2nbSpczQgw2hEEJD2PIl3o+2w/XiFbr5DY/Zb/dqG9GFgMsNWCnX3AZ/8BgDVruv/jnD178C/iXmNXjT+xT7ldua5Kvqp2uWM0TdWzKNPrfieryxkvV6bjH71wg2vNWu9J5LVrsut6UWe8cnk/cw1KE/NOHGOqxqv7bHd757d+0a+MG8wLI70e0ycDRRBo9SlI2phC0Zxr+6JU/EAnUmn6+2Aq7xUSu6p8hy7lQRAEo4Hy1mumyZrNyWwYQeAq4Pjczm16o4kiSNwdtj9TqloKdDzKDgcuKZUflrzS9gIeS+a2y4H9Jc1NjgH7A5enuick7ZXmOqw0VhAEwUig2bOzjlHH9jLgrcA7gfOA3W1fk9u/TTPa3sA7gFsl3ZzKPgycClwg6SjgPuDgVHcZcCBFruwnSTF3bD8s6RPA9andx2138nC/GzgL2Az4TjoGJtcEVmXqaZOqeduWr5cprom5qp57E6a4Xu0+8KtvrjuvMqvUNStVmX5yxqsyt+VSdR91aNs8litvr7lyx2vi2VZRJE8bfUXSB9sDsyl0x+skUbJaVdKasrH9H/Rezfut8AbJo+yYHmMtofCEmFi+HHjZAGIGQRC0ynR4a8lB0hLg5cDtwNpUbGBqlU0QBMGMR7OYtfEmUy1FU+xle5e6nUPZJJo2geWac5o2D+VS19sr10Mu19NvUM+yif3rzHvanLetOz/h8Ys3aJdrVsk19ZTbNe1llmsSasKbrcrM1YSZatB2dT39mkZinMxoP5S0i+2f1OkcyiYIgqA1NDZmNIpN+D+U9H8pXJ5FsQLy8pzOoWyCIAjaQmr97WmILCE5fbF+zSabsdltFARBMIo05fosaYmkByXdVirrO7DxANxne6nte2z/onPkdp7RbzaD7uSfrN+g7epQV7460QUGXefpZ4w60R5yn3N5naafKAF1IgjU2eWeO0Zd9+HcPk3cY5k2f/Hnyj7NXJ/PAr5AEQeyQyew8amSTkzXJ7BhYOM9KYIW7zng/D+V9HXg3yhFDphy1+cgCIIZj8Ss5zTjjWb7+ynOZJlFwD7p/GzgGgplsy6wMXCtpDmStu3EpazJZhRKZv+yWITrcxAEwVTT15rNPEnLS9eLU1zHKvoNbFxb2dg+om5fCGWzjjqmrSYCXU5VcMy65qc6prMq6kQkKNfVlaFc9+m5b113Xo4mAPmmqKZ3ueeOndN/sro6pqOmXcJz+9U10U2d67OYle+N9pDt3ZuauktZLfu9pI8CXypFbplYvx+wue1Lq8YJZRMEQdAiLSu0BzrmsczAxnW4Ffg3SU9TpIX+L2BTivWgXYErgX+YbJDwRguCIGgLqe1AnP0GNu4b25fY3hv4a4pQNbOBx4F/Afaw/Te2/2uyceLNZgDqen71CpxZNUZVnzq5buoG86xDE2a5On1qeaNNqKvjcTaRQX/ZNpH3po7Zq4mIBE143+XIMHGMYUZTqEISszd+TlNjnUfhDDBP0kqKkP99BTYeBNt3AXfV7R/KJgiCoDWEZjXzNWv70B5VfQU2nipC2QRBELSFxifq86CEsgmCIGgLjVVstIEIZZNBnd3rOf0na5cbYbnOjv+queu4YNeN+tyrrm406DpRn3Ndn6toYof+oGM0sbZTJc+grtkTaVqmJtalmkaMT/I0SVsDfwXsREl32D4yp38omyAIgrYYLzPaJcD/pnB17ltLh7IJgiBoC4lZDXmjjQCb2z6hbucZrWwGdeutMntVldcxq7VpAquiaVNh02PkfgZV7cqms7o73qvaNREBoNd4uW7HTWwsrJOobLK2OWPUcZ+uk8yuDdRfuJpR51JJB9q+rE7nGa1sgiAI2kVIY6NsjgM+LOlZ4DepzLa3yOkcyiYIgqAt1P7b07Cw/fxB+s9oZTNoUMmJfQbdAd9Ebpaq8ZreyV+HuqbHuuPnjHfanLetOz/+0Qt7tmsiv0sTASxz2/XaUd90QNGqdnXn6tWurvdY215nvRmrNxskvRl4Xbq8ZrLgm2VmtLIJgiBoG80ajxCUkk4FXg2cm4qOk/Ra2yfm9A9lEwRB0BJFioGx8UY7ENjV9loASWcDN1FkB52U1lRuj3zZH5P0n5JuTseBpbqTUr7sOyW9sVS+MJWtSGlPO+XzJf0o5d7+hqSx+USDIBgXCjNazjFNmFM6f0E/Hdt8szmL386XDfBZ258uF0jaBTgEeCmwHXClpJek6i8Cf0yRn+F6SUtt/wT4VBrrfElfBo6iyLPdOLnrKk0nDKtDE+MNa/2m7rx1omZXUY76TMvrMnXWDoYZwbjpKM1Nt8tdN2s6WdwgjIsZDfgfwE2SrqZIzvY64KTczq09BdvfB7pmduvCIuB828/YvociLPYe6Vhh+27bzwLnA4skCdgP6Kzmng28pdEbCIIgGBSNz5uN7fOAvYCL0vEa2+fn9p8KlXuspFuSmW1uKuuVL7tX+VbAo7ZXTygPgiAYIVS8LeccI4qk30t/dwO2pfi+/SWwXSrLYtgOAmcAn6DIUfUJ4HTgSHrny+6mDF3RviuSjgaOBth863nrynPNWU0n6xrU3beJpG1Vbtu5Zqoq6gTEbMI8lpsErlxXFYhzmPQy7zQRfLJX/7r96gQAzR0vV4a6/YZpYiscBDZudMwp4P0U35+nd6kzhZVpUoaqbGw/0DmX9BWg46NdlS+7W/lDwBxJG6W3m8r82rYXA4sBtlqw8/AWIYIgmPFMBxNZFbaPTqcH2H66XCdp09xxhmpGk7Rt6fIgoOOpthQ4RNImkuYDC4DrgOuBBcnz7DkUTgRLUxa6q4HObrxy7u0gCIIRoYiNlnNMA/5PZllXWnuz6ZEvex9Ju1K8et0LvAvA9u2SLgB+AqwGjrG9Jo1zLHA5MBtYYvv2NMUJwPmSPknh633mIPLWCWaZaxKqI0PdMep6kg3qSVd3vF5j547XRJDTKm+0MrnmorpmmkE91ZrOU1N33jpz5T6zJmRv+jOYZLZG32wk3Qs8QRHif7Xt3SVtCXyDIs/MvcDbbT/S4JwvolgT30zSK1m/jLEFsHnuOK0pmx75snsqBNunAKd0Kb8M+K0oo7bvpvBWC4IgGEmkVlyf97X9UOn6ROAq26emvYgnUvwYb4o3Au+kWK74TKn8CeDDuYNEBIEgCILWGEpstEUUViQotoFcQ4PKxvbZwNmS/sz2t+qOE8omCIKgLfoLVzNP0vLS9eLk3FTGwBWSDPxzqt/G9ioA26skvXBgubtg+1uS/oRi8/2mpfKP5/QPZZMYNAJ0FXVdqXNlqBPpuO1kbIO6gee6ZleNlyvDp7Y4aN35Bus3NLMmMOgYTezCz11XqRMJoYl1jqpn1kRUgzr32Bj5ZrSHbO8+SZu9bd+fFMoyST8dTLh8UqSWzYF9ga9SOGhdl9t/bOIoBEEQjBpqODaa7fvT3weBiynWrR/oePqmvw+2dDt/YPsw4BHbfw+8hg23plQSyiYIgqAtUvK0JlyfJT1X0vM758D+FNtHllJs/4B2t4E8lf4+KWk7imyd83M7hxkt0YTpqNd4uXO1HTizaVPhoMni+hmvadnL7T74yEXrzuvuhi9Td5d71RhNylTXHbnKBTlnvH6oE2y0iWRszZvVGnUQ2Aa4uAgNyUbA121/V9L1wAWSjgLuAw5uasIJXCppDvCPwI0U60dfye0cyiYIgqA1mgtXk7Z7vKJL+a+ANzQySfX8n0in35J0KbCp7cdy+4cZLQiCoC0EaFbeMeJI+rGkD0vaOUXoz1Y0EG82jdLL1FPX86uOua2uuanNvDWDeqZNHKNOQNEqGcreaMc/emHPdk2YWKrMWYOOnxuwM9dUmGsqq7qPps2BVUyV5+AkMzBGv+nfDPw5hcluLUXUggts35fTeWyeQhAEwUgyJm82tn9h+zTbrwL+Ang5cE9u/3izCYIgaI2xerNB0k7A2ynecNYAx+f2DWUTBEHQJtPgrSUHST8CNga+CRycHBaymdHKJnf3ehOu0Dky5M5bN4JA1fpQm27Rbbp3NxHhoLxO03SisiradG+uom6isl5j9OOO3CsyQBMRq5u+r2YQaGy+Zg+3XTtiwXio3CAIgpFlVuYx8jwi6UxJ3wGQtEva25PFtLjDIAiC6YnGxkEAOIsit9h26fpnwPtyO4/N+12bDOqCnEuu629d6uyub9olum50gkEDoFaZFE+b87Z15xNdn5tw4811Qe7VJ3eutgOANj3vMF2k63wGzTEtFEkO82xfIOkkANurJWU/wFA2QRAEbdHZ1Dke/FrSVhRhapC0F5C9sXNSZSNpd+APKV6dnqII/Hal7YdriRsEQTBjGCvX5/dTBP3cWdIPgK0p0gxk0VPZSHon8F6KTTs3AHdSJMx5LXCCpNuAv83dPRoEQTATcfuZOoeC7RslvR74XQoteqft3+T2r3qzeS5Fop6nulVK2hVYQBFldKyosu/nrmHkrhfkytFEJOZcN+s6oXbqyjQouWs7Ve3KUZ9pIXxJXTfhfsfOnXcoCcO6zFs1d10X6aq5eo0/fNfn6f1mI+mtPapeIgnbF/Wo34Ceysb2F6s62r45Z4IgCIKZzfRWNsCfVtQZGEzZdJA0H3gPsFO5ve0350wQBEEwc5n+bza2j2hinBxvtH8FzgT+DVjbxKSjQtMJ08rUcTOeSK7LdW6kgTZpIop0nWjOdcaeSDnq8wmPX7xBXRNJwpre5V6m6SRmubLWMd/1M37uXLnt6kQkaI7prWw6SNoG+AdgO9sHSNoFeI3tM3P65yibp21/fhAhgyAIZiQaq3A1ZwFfAz6Srn9GkWYgS9nkqNzPSTpZ0msk7dY5aokaBEEw0xifCALzbF9AsnDZXk0R+TmLHJX734F3APux3ozmdN0TSUuANwEP2n5ZKtuSQhPuBNwLvN32IyqSan8OOBB4Enin7RtTn8OBj6ZhP2n77FT+KgpNuxlwGXCc7b5sN3U8l5rwMutF3SRjg5rb+pk7d7w67QbtUzVG1bOYaDor04QXV50d620G4mx7t355jCbMhnW8x+o+i1a80Ro0o0laSPFdORv4qu1TGxt8cgba1JnzFA4CXmz79bb3TUelokmcBSycUHYicJXtBcBV6RrgAAo36gXA0cAZsE45nQzsCewBnCxpbupzRmrb6TdxriAIgqmnoTcbSbOBL1J8X+4CHJrWTYbFxE2d51A4j2WRo2x+DMzpVyrb3wcmRhlYBJydzs8G3lIqP8cF1wJzJG0LvBFYZvth248Ay4CFqW4L2z9MbzPnlMYKgiAYETpvNo1Efd4DWGH7btvPAudTfHcOhWRtej3wB8C7gJfavqVTL+mPq/rnmNG2AX4q6XrgmdLEdVyft7G9KvVfJemFqXx74JelditTWVX5yi7lXZF0NMVbEJtvPa+G2EEQBDXJX4+ZJ2l56Xqx7cWl627fh3sOKF1fpHWa23tUf4rihaArOcrm5DpC9Uk3A71rlHclfWCLAbZasHPXdrlrMYNGH544Ru68dXb1V7Wru96Um6it6SjNufLUSTj36bnrN0hvEE2AZqI0j1qCryaiNFfRhOx17rHNtZ1B6GM59CHbu1fU9/W9NwVU/k9bFRtNyaz1vcna9CHMA5K2TW812wIPpvKVwI6ldjsA96fyfSaUX5PKd+jSPgiCYGQwsLY5fdDre3JUqLzRqve7qyW9R9LvlAslPUfSfpLOBg7vU5ilpT6HA5eUyg9TwV7AY8ncdjmwv6S5yTFgf+DyVPeEpL2SJ9thpbGCIAhGBjvvyOB6YIGk+ZKeAxxC8d05Lagyoy0EjgTOSyFrHqWI+jwbuAL4bFV8NEnnUbyVzJO0ksIcdypwQUoleh9wcGp+GYXb8woK1+cjAGw/LOkTFA8Z4OOl1AbvZr3r83fS0RejkBisTbNc3fur6z5dpukIAr3aNWFu+8Cvvrm+ouEEZFU0bb7K7de+u29v6gTVzE3oVme8qnZN0OSbTUpWdizFj/DZwBLbvdZPpoL/VlVZFYjzaeBLwJckbQzMA56y/WjOrLYP7VH1hi5tDRzTY5wlwJIu5cuBl+XIEgRBMFU0GePL9mUUP85HkcoMAFlxFFLOglWNiBMEQTBjMGv722s+nam80bEJ2jMoTZiOcsZuQqZcT7J+PM4GzWdTN5pAruw5MvQzRrnfaXPWJxs8/tELs+aFdnPZtxkAtMobrYl5m/Dgyxm7aoyJ7dr8rKqwYc3MUTaVhLIJgiBokQa90aY1k+42knRsKURMEARBkImBtXbWMQbcW1WZs7X1RcD1ki6QtDC5GgdBEAQZrM08Rh1Jm0v6W0lfSdcLJL2pU2+7V/poIMOMZvujkv6WYo/LEcAXJF0AnGn754OJPzrU2UHfhNttE5ELcl2fc5OTVdGrXRNu5HWiXteNZF3uV44aMLFHmzveq8ZoIslYr7WJJlyfh/lc6kSpbjuydS7G42RG+xpwA/CadL0S+CZwaU7nrKA9yTX5/6ZjNTAXuFDSaf1KGwRBMJNYY2cd04CdbZ8G/AbA9lNMEqKmzKRvNpLeS7Hb/yHgq8CHbP9G0izgLuD4OlIHQRCMO501mzHhWUmbsT6fzc6UgjNPRo432jzgrbZ/US60vbZsr5spNLHLfVBTVJV5LFe+XOomdOs1Rj+mvdzxB5XpU1sctO58YiK1JswxueaxNt2nm3ZBrurfRBDNOgnYmnbbborpsB6TycnAd4EdJZ0L7A28M7dzzprN31XU3ZE7URAEwYzD4/NmY3uZpBuBvSjMZ8fZfii3/7RIfB0EQTAdMYWTQM5/o46kg4DVtr9t+1JgtaTspJUzelNn05EBBg2CWTfHTNPUMW3lmvYm0svjrspbruloD+WoAVVPPHfn/USTTa5XWK+56kQMqKJuoNCpMgfWmatpD75BGCczmu11dmbbj0o6GfjXnM4zWtkEQRC0iTGrx8SMRndLWLYOCWUTBEHQIuOyZgMsl/QZ4IsURoD3UOy7ySLWbIIgCFpkXNZsKJTLs8A3KDZzPk2P1DDdiDebxDATqTU91yhGaW7zedYZu2pt5/StDl53Xo4mMBm91gXqrivk7oDvNUbuGkvT9OP6PGhkhNx1rty6thPJFftsGh1yyrD9a+DEuv1D2QRBELTIuISrkbQ1xSb+l1JkbQbA9n45/cOMFgRB0BJmrMLVnAv8FJgP/D1FlOfrczvHm02iCffcXn3qzjtoQrPc8fohV6ZeMuY+s7pBSeuMNzFqQJkmEnw1bZqp67qc066OCayf6ARNm/bqmMfqmPLqYpvfDCFZm6SPAX8F/Fcq+nBKIY2kk4CjgDXAe21fXnOarWyfKek4298Dvifpe7mdQ9kEQRC0yJq1Q9tp81nbny4XSNoFOITC9LUdcKWkl9iuowF/k/6ukvQnwP3ADrmdQ9kEQRC0hJlyE9ki4HzbzwD3SFoB7AH8sMZYn5T0AuADwD8BWwB/k9s5lE0X6prH6uSpaSL4ZB0TU9VcdUyAdQNs9upTlzrmwU/PXZ/z6QO/+mZ2vzbNY3VMW1Xj5c5bVd7LW67uc2gz103daA+Ne6OZfpTNPEnLS9eLbS/uY7pjJR0GLAc+YPsRYHvg2lKblaksG0mfsn0CsJntx4DHgH37GQPCQSAIgqBV1npt1gE8ZHv30rGBopF0paTbuhyLgDOAnYFdgVXA6Z1uXUTq9xfZgZI2Bk7qs98GxJtNEARBSxjzbEMOArb/KKddStvcyZ65EtixVL0DxVpLP3yXIp/ZcyU9Xp6qEMtb5AwyJW82ku6VdKukmzuvjZK2lLRM0l3p79xULkmfl7RC0i2SdiuNc3hqf5ekw6fiXoIgCHoxLNdnSduWLg8CbkvnS4FDJG0iaT6wALiur3uwP2T7BcC3bW9ROp6fq2hgat9s9p2QC+FE4Crbp0o6MV2fABxA8YAWAHtSvC7uKWlLimQ+u1N8pjdIWprslH3ThNvtoGsTVZGOc+epK0OdyABNrLHkyDBxrqbXlKrWaZpw1R10XaFqvCaiQ9dZp6i77jHoWk/uXG1GTOgLD80b7TRJuxYzci/wLgDbt0u6APgJsBo4po4nmqTZwHMHEXCUzGiLgH3S+dnANRTKZhFwjm0D10qak7T4PsAy2w8DSFoGLATOG67YQRAE3TEeSiBO2++oqDsFOGXA8ddIelLSC5KTQN9MlbIxcIUkA/+cFsK2sb0KwPYqSS9MbbcHflnq2/Gm6FUeBEEwMkyT6AA5PA3cmn7Y/7pTaPu9OZ2nStnsbfv+pFCWSfppRdte3hTZXhaSjgaOBth863ldJ2liJ3+vdk2btnLlm2z8nLo2IyFMVjcouabRciDOiSa1NnebN2H2asJFOpdBzW1VcjSR+K2JiAmNJ34D1nhs0qd9Ox21mBJlY/v+9PdBSRdTbDJ6QNK26a1mW+DB1LyXN8VK1pvdOuXX9JhvMbAYYKsFO4/Nz4wgCEYbuzlvtKnG9tmD9B+6N5qk50p6fucc2J/Cc2Ip0PEoOxy4JJ0vBQ5LXml7AY8lc9vlwP6S5ibPtf1TWRAEwUhQpBhw1jHqSLpH0t3rmeUqAAASSElEQVQTj9z+U/Fmsw1wsaTO/F+3/V1J1wMXSDoKuA/o2DYuAw4EVgBPAkcA2H5Y0idYH3X04x1ngTo0vZO/qn+uR1eb81bRprmtCZrOFZQbNaBuUMlB87HUNfUMamLL9Zara9pqwkQ5aGSAYXitjdGaze6l800pvqO3zO08dGVj+27gFV3KfwW8oUu56ZENzvYSYEnTMgZBEDSB7WEG4myV9B1d5n9K+g/g73L6j5LrcxAEwVjR2dQ5DpQ31FMswewOPD+3fyibIAiCljAMJZ/NkDi9dL6aYvPo23M7h7LJIHcnf5vrGU3srm/azTjXtbiXDP3U5cgwcYzcezxtztvWnU9MpNaEm2zumkhu/yZ23ueMN+j9dRt70OgHVYnp6qwPte36zNSnGGgM231Hei4TUZ+DIAhaokgxsDbrGHUkHSdpi+QZ/FVJN0raP7d/KJsgCIIWGUYgziFxpO3HKbaZvJDCM/jU3M5hRutCXdfaQV2Q65q2pip52jDHqxMMNVeG4x+9cP1FHyaqXPPToGaqXFNPlYkplzpmw6YjK1SN30SyuDJtuz4bs3ZMvNFYH7XlQOBrtn+stIclh1A2QRAEbTG8qM/D4AZJVwDzgZPS5vzsmwtlEwRB0BJrMc+uXj3VYjTFURSZQO+2/aSkrUib7AEkvdT27b06z2hlU8fLrI6JrW2zVBOBOOt4j1VR51k0EQw1d64yZW+0DUxqNLPzPqdPFU2Yeto0F/Vj2so1dQ0anaHOPK1gWLNmPN5sbK8Fbixd/woob/T8X8BuE/t1mNHKJgiCoE3M+EQQyKDyl2komyAIgrYYrzWbyag0JYSyCYIgaAkzo5RNJTNa2eSuA9Rxp2163jq79SdSZ60jN0pCFXWiVzfxGeTe48R1ml7UXfeoE1W5ql3T804VuTv+c8eoE/W5bYYViFPSwcDHgN8H9rC9vFR3EsXi/hrgvbYvT+ULgc8Bs4Gv2s7eM9ODZ6sqY1NnEARBSzh5o+UcA3Ib8Fbg++VCSbsAhwAvBRYCX5I0W9Js4IvAAcAuwKGpbU8kfXzC9WxJ5667V3uvqv6hbIIgCNoirdnkHANNY99h+84uVYuA820/Y/seirxge6Rjhe27bT8LnJ/aVvE76S0JSZsAFwN35co4o81oZdo09fQzRi+ZqkxbvfpUzZtL3cCZuUFJ67pW58yb+5xP3+rgdecffOSiDepyd9RXmWnaNOFUyTBokMqJNLFDv1cQzLbNXlNlUuxzzWaepOWl68Uppf0gbA9cW7pemcoAfjmhfM9JxjoCODcpnH2B79j+bK4goWyCIAhawnY/P/Iesr17r0pJVwIv6lL1EduX9OrWTSy6W7W6Cjohj83ngH8GfgB8T9Jutm/s1m8ioWyCIAhapCkHAdt/VKPbSmDH0vUOwP3pvFf5RE6fcP0IxTrP6RQKar8cQWa0shk0gGUTO/mb9sBqIlpBFXWeWR2Zmr7Hqj4f+NU311/UDMRZZ5d7E+SaonLbNSFfHdNU01EXqsYvj9d2IM61nvJwNUuBr0v6DLAdsAC4juKNZ4Gk+cB/UjgR/EW3AQbNY9MhHASCIAhaZO3atVnHIEg6SNJK4DXAtyVdDpBilV0A/AT4LnCM7TW2VwPHApcDdwAXVMU1S3P8g6Q5peu5kj6ZK+OMfrMJgiBok2Hts7F9MYV3WLe6U4BTupRfBlzWxzQH2P5wqf8jkg4EPprTOZRNEARBi4xRBIHZkjax/QyApM2ATXI7h7JJ5K4DNJG4q4lkZznkRiSooul2bSd3q/Nsy1GfT3h8wx+HTayJ1BmvTF234F4uzXVduHNpM0pzGzK1Ou+Q3myGxL8AV0n6GoVjwJHA2bmdQ9kEQRC0yBilGDhN0i1AxyvuE53QNzmEsgmCIGiJEfBGa5qbgI0p3mxu6qfjtFc2gwSTa3P3ehPzDGu8Jmhi7Nwx2vzc+jG31AkWOcx2wxq7CTmmyszVOmOUYkDS24F/BK6hcJ3+J0kfsp0VyXZaK5tSMLk/pti8dL2kpbZ/MrWSBUEQjF3ytI8Ar7b9IICkrYErgfFXNpSCyQFI6gSTC2UTBMFIMOgemhFiVkfRJH5FH3s1p7uy2Z6MYHKSjgaOTpfPnPunh9w2BNmqmAc8NMUywGjIMQoyQJLj3EmbtS/D1IoAjIYcoyADwO8O0tkeK2Xz3bRZ9Lx0/ef0sU9nuiubXkHmNiwoIqcuBpC0vCrY3TAYBRlGRY5RkGFU5BgFGUZFjlGQoSPHYCN4bJSN7Q9JeivwWorv3sVpM2kW013ZVAWZC4IgmFJss3oMvNHS+vjlKRjoRZO178Z0VzbXkxlMLgiCYCoYhzcb22skPSnpBbYfqzPGtFY2tldL6gSTmw0smSyYHMmcNsWMggwwGnKMggwwGnKMggwwGnKMggwwoBx2sddmTHgauFXSMuDXnULb783pLI/PgwiCIBgpNt1hO//Oe96V1fauEz92wyisU/VC0uHdym1nhayZ1m82QRAEo844mNEgX6n0IpRNEARBS4yDg4CkW+mRMhrA9stzxpkxydMkLZR0p6QVkk5sYfx7Jd0q6eaOu6SkLSUtk3RX+js3lUvS55Mst5RzfEs6PLW/q9dr64R5l0h6UNJtpbLG5pX0qnRfK1LfrrFiesjxMUn/mZ7JzSn3RafupDTmnZLeWCrv+jlJmi/pR0m+b0h6ThcZdpR0taQ7JN0u6bhhP48KGYb9LDaVdJ2kHyc5/r6qr6RN0vWKVL9TXfkyZDhL0j2lZ7FrW59Hqd1sSTdJunSYz6Gzz6bt5Gkt8ybgTymSr30X+Mt0XEZm9ACg0LzjflA4D/wceDHwHODHwC4Nz3EvMG9C2WnAien8ROBT6fxA4DsUvup7AT9K5VsCd6e/c9P53EnmfR2wG3BbG/NSpJB9TerzHYoESrlyfAz4YJe2u6TPYBNgfvpsZld9ThTZBg9J518G3t1l3G2B3dL584GfpbmG9jwqZBj2sxDwvHS+MfCjdI9d+wL/H/DldH4I8I268mXIcBbwti4yt/nv8/3A14FLq55h089h42238XZ/d3zWASwf5vdije+4H+SU9TpmypvNurA2tp8FOmFt2mYR6/M9nA28pVR+jguuBeZI2hZ4I7DM9sO2HwGWAQurJrD9feDhNuZNdVvY/qGLf1nnlMbKkaMXi4DzbT9j+x5gBcVn1PVzSr9W92P9r6jyPZVlWGX7xnT+BEW62+2H+TwqZBj2s7Dt/5cuN2Z9pN5efcvP6ELgDWmuvuTLlKHqWTT+71PSDsCfAF9N11XPsNHnYMbizabDcyW9tnMh6Q+A5+Z2ninKpltYm6ovgDoYuELSDSrC4wBsY3sVFF9CwAsnkacpOZuad/t0Pog8xyaTyBIl81UNObYCHnWRNz1LjmT+eCXFr+kpeR4TZIAhP4tkOroZeJDiC/rnFX3XzZfqH0tzDfRvdaIMtjvP4pT0LD4rqZPtsa3P438CxwOdb/SqZ9jscxiSGU3SwclUuVbS7qXynSQ9VTJZfrlUl2WCLHEU8EUVSwb3AF+iSKCWxUxRNllhbQZkb9u7AQcAx0h6XQ152paz33kHlecMYGdgV2AVcPow5JD0POBbwPtsP14hX2tydJFh6M/C9hrbu1JE1tgD+P2Kvq3IMVEGSS8DTgJ+D3g1hWnshLZkkPQm4EHbN5SLK/o1LIOH9WZzG/BW4Ptd6n5ue9d0/HWp/AyKmJEL0jGZFeUG268AXg50xrsxV8CZomxaD2tj+/7090HgYor/uR9Ir/qkv52Iqb3kaUrOpuZdmc5ryWP7gfRlsxb4CsUzqSPHQxQmlY0mlP8Wkjam+JI/13YnrMZQn0c3GabiWXSw/ShFDpK9Kvqumy/Vv4DCLNrIv9WSDAuTqdEuctl/jfrPIufz2Bt4s6R7KUxc+1G86QzlOdiFN1rOMQi277B9Z277fkzkpT7bSDqTYh3rMUm7SDoqd86ZomzWhbVJXieHAEubGlzScyU9v3MO7E/xS2Mp0PGcORy4JJ0vBQ5L3jd7AY8l887lwP6S5iYzy/6prF8amTfVPSFpr/SKfVhprEnpfMEnDqJ4Jh05DkmeP/MpflVdR4/PKf3PcDXwti73VJ5PwJnAHbY/MxXPo5cMU/AstpY0J51vRpHK946KvuVn9Dbg39NcfcmXIcNPS4pfFF9w5WfR6Odh+yTbO9jeKcn477b/cljPYUTWbOar8MT7nqQ/TGV1TORnUXwW26XrnwHvy5bCI+DlMIyDwtPlZxR26480PPaLKbxQfgzc3hmfwtZ7FXBX+rtlKhdF0refA7cCu5fGOpJi8XEFcETG3OdRmGV+k/7BHNXkvMDuFF8GPwe+QIo6kSnH/0rz3ELxP+C2pfYfSWPeScmDqNfnlJ7xdUm+bwKbdJHhtRT/f98C3JyOA4f5PCpkGPazeDlF2t5bkrx/V9UX2DRdr0j1L64rX4YM/56exW3Av7DeY621f5+p7T6s90YbynOY9cKt/by/eXfWQeHRurx0HD1B/ivTvU48FpXaXDPhuW0CbJXOX0WxvrQFhQnzylK7PwT+bZLvmuvT35tKZTfnfk9GuJogCIKWmL3N1t700D/Lavvk5/554HA1kq6hcLHvmhqhU08RuPhq27+Xyg8F9rHdM7ZO6vtnFI4eu6W3z0/Zfn2ObBFBIAiCoCU6ZrSpQkXq5oddRG1+MYX5727bD0t6IimMH1GYIP9pkuHeT/FWvrOkHwBbs94UOSmhbIIgCNrCw1E2kg6iUBZbA9+WdLPtN1Jstv64pNXAGuCvbXf2w72bYh1mM4oNsd+pmsP2jZJeT5G9VMCdtn+TK2MomyAIgpawhxMbzUXGzN/Kmmn7WxSekd36LAdeljuHpE0pIix01iX/t6Qv2346p38omyAIgtYwnh7RAXI4B3iC9ea2QykcXw7O6RzKJgiCoE08Nsrmd11s6uxwtaQf53aeKftsgmADJG2W9h3M7qPPsZKOaFOuYMwoPATyjtHnpuRQAICkPYEf5HaON5tgpnIkcJHtNX30WULxP9fX2hEpGD8Ma6f39hKtz2ezMcWm2/vS9X8DfpI7TiibYKyQ9GqKHfx7UIR/vw74c9u3TWj6l8BfpD77AH8PPEARu+wiik2Fx1F46rzF9s9tP6kiCOEetq8bxv0E0xwDa6Z38jSKfDYDE8omGCtsXy9pKfBJCkXxLxMVTQor8mLb95aKX0ERqPJhinwpX7W9h4rkZ+9hfViO5RS7rUPZBBl4upjIemL7F02ME8omGEc+ThGz6mngvV3q5wGPTii73ikNgaSfA1ek8luBfUvtHqSIWBwEeYyPg8BAhLIJxpEtgedR2Jg3BX49of6pVF7mmdL52tL1Wjb8/2TT1D8IJsfTf82mKcIbLRhHFgN/C5wLfGpipYtsj7PTJrV+eQnroxQHweSMjzfaQISyCcYKSYcBq21/HTgVeLWk/bo0vYJiJ3S/7E0RfTcI8vDavGPMiajPwYxE0iuB99t+R5t9gpmN5r7A2vc1WW198eUDR30eZWLNJpiR2L5J0tWSZvex12YehXkuCLKJH/QFoWyCGYvtJX22X9aWLMGY4unv+twUoWyCIAjaJJQNEMomCIKgRTwjFv9zCGUTBEHQFp1AnEEomyAIgvYwrOkn1uv4EsomCIKgLeLNZh2hbIIgCNok1myAiCAQBEHQIh5KuBpJ/yjpp5JukXSxpDmlupMkrZB0p6Q3lsoXprIVkk4cSIAMQtkEQRC0hSkCceYcg7EMeJntlwM/A04CkLQLcAjwUmAh8CVJs1OG2i8CBwC7AIemtq0RZrQgCILW8FCSp9m+onR5LfC2dL4ION/2M8A9klZQJBYEWGH7bgBJ56e22Zk3+yWUTRAEQVv8v6cu5z9unpfZelNJy0vXi20vrjHrkcA30vn2FMqnw8pUBvDLCeV71pgrm1A2QRAELWF7YVNjSboSeFGXqo/YviS1+QiwmiK9BoC6iUX3JZRWg7iFsgmCIJgG2P6jqnpJhwNvAt7g9dE/VwI7lprtANyfznuVt0I4CARBEExzJC0ETgDebPvJUtVS4BBJm0iaDywArqNIm75A0nxJz6FwIljapozxZhMEQTD9+QKwCbBMEsC1tv/a9u2SLqBY+F8NHNNJqSHpWOByYDawxPbtbQoYydOCIAiC1gkzWhAEQdA6oWyCIAiC1gllEwRBELROKJsgCIKgdULZBEEQBK0TyiYIgiBonVA2QRAEQev8/1wJB9GGxZm8AAAAAElFTkSuQmCC\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "sedflux.quick_plot(\"bedrock_surface__elevation\", vmin=-200, vmax=200, cmap=\"BrBG_r\")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 8,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "text/plain": [
+ "array([ 4.])"
+ ]
+ },
+ "execution_count": 8,
+ "metadata": {},
+ "output_type": "execute_result"
+ }
+ ],
+ "source": [
+ "sedflux.set_value(\"channel_exit_water_flow__speed\", 1.2)\n",
+ "sedflux.set_value(\"channel_exit_x-section__mean_of_width\", 400.)\n",
+ "sedflux.set_value(\"channel_exit_x-section__mean_of_depth\", 4.)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 9,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "now = child.time\n",
+ "times = np.arange(now, now + 1000, 1.0)\n",
+ "sedflux.update()\n",
+ "child.update()\n",
+ "for t in times:\n",
+ " child.update_until(t, units=\"years\")\n",
+ "\n",
+ " sedflux.set_value(\"channel_water_sediment~bedload__mass_flow_rate\", mapfrom=child)\n",
+ " sedflux.update_until(t, units=\"years\")\n",
+ "\n",
+ " z = child.get_value(\"land_surface__elevation\")\n",
+ " child.set_value(\n",
+ " \"land_surface__elevation\",\n",
+ " mapfrom=(\"land-or-seabed_sediment_surface__elevation\", sedflux),\n",
+ " nomap=np.where(z > 0.0),\n",
+ " )"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 14,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAZsAAADxCAYAAAAdgBpwAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4wLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvqOYd8AAAIABJREFUeJzs3Xd0XOd94P3vM7139N47CZAgwF5ESqREFcqWHMdNyZu8ThzlrPe8SXazjrPezZ60TdnEu4kdJ7ZlObEtxZZVSYu9V4AEiN57G8wAmN7nvn8AUpiNLFESIUX2/Zxzz1z85lb88fzmKfc+QpIkZDKZTCZbT4oP+wJkMplM9rNPTjYymUwmW3dyspHJZDLZupOTjUwmk8nWnZxsZDKZTLbu5GQjk8lksnUnJxuZTCb7CBBCFAghzggh+oQQPUKIL67FHUKIE0KIobVP+1pcCCG+KoQYFkLcFkJs+jCvX042MplM9tGQBH5LkqQaYCvwtBCiFvhd4JQkSRXAqbW/AR4EKtaWzwNf++Av+V/IyUYmk8k+AiRJmpMk6ebaegDoA/KAx4DvrG32HeDI2vpjwLPSqquATQiR8wFf9ptUH9aJZTKZ7GddtkUhxZN3t+1yROoBoneEviFJ0jfealshRDHQBFwDsiRJmoPVhCSEyFzbLA+YumO36bXY3N3fwb0jJxuZTCZbJ/Ek7K+6u2L2hx2JqCRJze+0nRDCBPwI+I+SJPmFED9107eIfWjvJ5Ob0WQymWwdKcTdLXdDCKFmNdH8kyRJL6yFF95oHlv7dK/Fp4GCO3bPB2bvxT29F3KykclksnUiBKiV4q6Wdz6WEMA3gT5Jkv7yjq9eBp5aW38KeOmO+OfWRqVtBXxvNLd9GORmNJlMJltHd1truQs7gM8CXUKIjrXYl4A/AZ4XQvwKMAk8ufbdUeAhYBgIA798z67kPZCTjUwmk60Twb1LNpIkXeSt+2EA9r/F9hLw9L05+/snJxuZTCZbLwIUcmcFICcbmUwmWzf3smbzUScnG5lMJltHyp8+NPnnipxsZDKZbJ0IASrlh30V/z7IyUYmk8nWkdyMtkpONjKZTLZOBPIAgTfIyUYmk8nWi5D7bN4gJxuZTCZbJ/JotH8hJxuZTCZbJwJ5gMAb5GQjk8lk6+VdvGTzZ52cbGQymWydCOQ+mzfIyUYmk8nWkTwabZWcbGQymWwdyc1oq+RkI5PJZOtEyH02b5KTjUwmk60Twd1NjPbzQE42MplMto7kms0qOdnIZDLZOpGb0f6FnGxkMplsHSnkbAOAPChPJpPJ1otYTTZ3s7zjoYT4lhDCLYToviP234QQM0KIjrXloTu++y9CiGEhxIAQ4uA63eFdk2s2MplMtk4EAtW9e1/NM8D/AZ79v+L/S5KkP/9X5xWiFvgkUAfkAieFEJWSJKXu1cW8W3LNRiaTydbJap+NuKvlnUiSdB5YustTPwb8QJKkmCRJY8Aw0PLe7+T9k5ONTCaTrSOFQnFXy/vwm0KI22vNbPa1WB4wdcc202uxD42cbGQymWwdvYs+G5cQou2O5fN3cfivAWVAIzAH/MVa/K2qStI9uaH3SO6zkclksnUixN11/q/xSJLU/G6OL0nSwh3n+nvg1bU/p4GCOzbNB2bfzbHvNblmI5PJZOtoPZvRhBA5d/z5OPDGSLWXgU8KIbRCiBKgArj+vm7kfZJrNjKZTLZOhACV6t78phdCfB/Yy2pz2zTwFWCvEKKR1SayceDXACRJ6hFCPA/0Akng6Q9zJBrIyUYmk8nWjUC8387/N0mS9ItvEf7m22z/h8Af3pOT3wNyspHJZLL1IuQ3CLxBTjYymUy2jhRC7hoHOdnIZDLZulltRpNrNiAnG5lMJls/cjPam+RkI5PJZOtkdTTaPXs32keanGxkMpls3cjNaG+Qk41MJpOtEwH3bOjzR52cbGQymWy9yH02b5KTjUwmk60TgfiZGPoshMhndX6cXazOjxNh9dU4rwHHJElKv9Mxfu6SjcvlkoqLiz/sy5DJZB8B7e3tHkmSMt7zAe7h62o+LEKIb7M6PcGrwJ8CbkAHVAKHgN8TQvzu2nw7P9XPXbIpLi6mra3tw74MmUz2ESCEmHhf+/Mz0WfzF5Ikdb9FvBt4QQihAQrf6SA/d8lGJpPJPjDvboqBf5d+SqK58/s4qzOBvq11S7lCiAIhxBkhRJ8QokcI8cW1uEMIcUIIMbT2aV+LCyHEV4UQw2uzzm2641hPrW0/JIR46o74ZiFE19o+XxXiLuZWlclksg+QUCrvavn3TgjxsBDilhBiSQjhF0IEhBD+u91/Pet3SeC3JEmqAbYCTwshaoHfBU5JklQBnFr7G+BBVudcqAA+z+oMdAghHKy+SruV1Tm0v3LH1KdfW9v2jf0OreP9yGQy2buyOnma8q6Wj4C/Ap4CnJIkWSRJMkuSZLnbndetGU2SpDlWpylFkqSAEKKP1U6mx1idkwHgO8BZ4D+vxZ+VJEkCrgohbGsTA+0FTkiStAQghDgBHBJCnAUskiRdWYs/CxwBjt2re2hpaeHGjRv36nAy2c+MOxsRftr6nX0Vyjt+uatU/1LsqNXqN9c1Gs2b6zqd7s1jGQyGN49lNpvfXHc4HG8eNzs7+831kpKSN9cbGhreXN+5c+e/OvcH5aNQa7lLU0D3Whn9rn0g/3khRDHQBFwDstYSEZIkzQkhMtc2y2P1Zt4wvRZ7u/j0W8Tf6vyfZ7UGRGHhO/ZjvWlhcfGut5XJfp78q/JGkkCsfigEq+tpkKQUCiFIpyXS6SRKhSCVkkgl4qhUgmRaIhkXqFUKEok0cZUCrVpJLJEiElRg1KuJxpMEfQosBi2hWALPvIJMhx5JgpmxOIXZViKxBAO3k9SVZoCAjsuv0VKXS1qSeOUHf8vhneUY9Grc45184pe++MH+o4QChVr7wZ5z/fwn4KgQ4hwQeyMoSdJf3s3O655shBAm4EfAf5Qkyf823Spv9YX0HuL/NihJ3wC+AdDc3HzXWfmvv/a3fLPvFupogqXZOXRWC5ayYmJDY6SsJvQ6HZ6xCcylRajCUeLLPhKJOK69O/BfukEikcC5bwcr56+irigF9yKGDbUsXLyGyWDAuKkBf3cf8QUP9i1NjLx8lLLHH2bl2i30tRUolv1EFtwYmupJjE2R0OtQqVWIpRUsLU34b3UTXfSQcWA34a5+QgtuTM0bCPQMYsrKJJFOobOY8fcM4NjZSrx7gEgkjLmuhsTQKEGfH/veHcR6+kkkEpjycvCPjKHQ6zEV5OEfGMaxeyueE+dIKwRGiwVz6yaCNzqJ+v0YNtaSnnMT8Xixt25m+Vo72pIiFMsrpCNR0hYzepOR2MwcSa0aU1EBS5296HKz0BuNeMYmcG3eiO/ydQwNtcTcHoTPj6l5I9GpWWJzC1jraxg/foaiQwdY7u3HUlNBrG+YtNNG1LuEs6SYwNISSknCaDITTSUpKSpkrr2D/D3bSQ+MMT83R9aurRSFEkyPj5OzpQnt8AQtdQ2M9l8lEY+xtDjJJx+s4vRNH16Ph50bTHQMhTFoJIiuMLuUIJlI8tiODE51+HAv+vjYNhunuqKUZkkMTkcJBkNsqbRyeyJGJBKhMs9AAi0Tsz7SKDmy1crrHSES8QQHN9t4/aYPKS1xeIuVU10xqnJh3qdkOZBgZ7WaU7fD7K3Xceqml/ryDPonA1Tna4iltCx4V9hUbuV0V5jHt1p55vgsh1oyaR8OcKjJykvXfBzZauPaUJzlYJzHWq1c7I8RDEepyjcyMhtBo1GzpVxD10SESXecj2+3cb4vweJSiCPbrJzpiRMIRnigycyVoSQ+f4THtlq5PJRCq4xj1OuYXUoSCEU4stXGme4YgjS7a7VcGYiSSCaoLTRyucuNUqXn0GYLlwfilGdJDM6lCYUCJCQ1j293caIzQjQa57FtNk7djpBhTKLTaRldiJOIxzi4ycqZ7ihVOeAJazArw/ROhWmpcdI+EmFvnZEbI3H2Nhh49vUJ9mx0Mu0OcHBzJed6wjxaqWVR/Z5+kL8vQvBRaSK7G38IBFkd9qx5h23/jXUdkyeEULOaaP5JkqQX1sILb8ybvfbpXotPAwV37J4PzL5DPP8t4veUtaKMpE7DysQkytwcJl4/QzAWwVxZTsi7TMIfQONyMd3VzcLkJMaGGoK3e1GYTeiqywkPj6M2GzHlZpHUawl292HMzECyW1m50YHSYMC2o5WhH78KShWBW13onDZIJBk4fZZwOETS7SESCRPsHcBaXkJKp8V9vR2lw4ZlazOeM5dIqpWQk0nn15/BWlNBYn4BFr3ocrNx7d/F0vkroBA4d2/D19lFPB7DtrOVgW//E+qiPBytmwhMzRD0LuFoaSIwMkbE72Px+Fmce7aj0WqJRMIsdvagzMvG3NJEz7PPoS7Mw7lnO4G2DrRaHeaifJYX3MwOj6JNJBk9c57FuXks9bX4Onox6PXYaqsIjE8hIjG0ZhPW7S30P/cCy4PD+CIRRn/4Cp6uPmybN4LRQNamDcQGhjEqlBhcTtRFebiv3iB351Yio+OoVvxkNG0gMD6JctGLtTCP6scPM3n6AtFohKzyUq7+9d+h1uvZvG8vU1duMNDVxexYOx97eCcajZptW1s4enaAnEwbT/3iQb7+g1uMTcyiUKrom44wMDpLeYmVU22z5DjVHNqRy/meAAatREWBjZoCHde6Z7k5FiUYTnCjZ4ah2Qg3e2aYXQyuFtgDUYw6NYebrVzoi2I0GHigycx3T85S6IKVENzomWFsyk33tIRVL/GNVyeoLjDSPxlEo9VSXWBm1hNmJaTAZdXyaIuZl675qC9zcnsigcVoQKdV8fHtdl665kOtkmgu03CxP4JSpKjLV/PcyRHa+2ZYWgnw4qV5Ggq17N9g4Ex3BKVC4qFmMyc6QigUCo5stXK03Y9AcLjZzE86gmiVKZrLTUzMeukbnuL+jSZOdITQqQWxaJiXLi/SUq7hwEYrV/sCROMpHmmx8hf/PIxJHSGakNAoYlzqnGVHpY7zPRHMesF9G418/ZUJqnIEZoOKVy5OMDnjpq5Ay7dfn6GuUE1pjpF0IsK57hUe3Z7DjaEwTrMWu0VLSYbgxxfd7G9y0TEewxtWke3Q8eQOGy9f9+HzB+518XAXxM/MAAHAIUnSxyRJ+ookSf/9jeVud163ms3ayLBvAn3/VzXrZVY7mf5k7fOlO+K/KYT4AauDAXxrzWyvA390x6CAB4D/IknS0tpoiK2sNs99Dvjf9/IeYrEYwe4e4rEoPreH/HgcdBqCM/Okzl9Co1CyODqOc3YOc24uUihMsGeAsattFDY2kHYvMt/VTcHObaS7elGnYfTKDYwOO1nFhUzc7MCUmYErFseamYlSqWDkWjsZ5SXYHTYK6+vQmE2os7OIz8zhmZzCcaUdrVrFwKlz1O7dDQse5voHsYRDZLZsonRTIxNHT6LR6ggFA2gMBkilUWrUjHV2kR+NIqXSTA72UWK3EvEHmL/ajlarQSUULAyM4LjajsFopO/MOer27iHcP4zfvYi2uJDpk2ep2bkNXziMLTOD+QtXsdlsBJdX8LkXyY3FIBYnv6oCfVM9Gd4lhFbD4pU2FOkUk339lOm06A0GJm93oTuvJ6ZUUtzQgNZhQ1tVRu+3v4dCpSTeP4x3fpFoOEgiFEJKS5QoFQitFr93Gc+ZS8T8PgLLPnR2Oyq9FvfwGLNnLpFIJAj7/HjdS3z2N75A+GY3zniK5Pg0dqGie2oSb1UJz790kY7OPkKRKl4+1ceBlJ3uIS+ZueXUVeVw8L6N7NwZ4ff/+BnG5iVOnhvl0QcacfvinLw2QU15NssRgcmgxOFw8fDuYnQaFUmhZkOFmUxbJn/yTAfnezNo752gKMuEVulgeMxNKJpAkooYnlpGq5J4ZFsWEzl2jHot26u0+IKCtn7BwHSIaDxF72gIu7EEi0lPW98IR9t1SJLExOwS03MgsdokFYk5SSWTTM0tM7sgkaov5ErnBDazFmtDBtVlOQgEeS4NL50dIC/bhUIo6B9fIBqJseTLYG7Rx7I/QjCYiVajpK17DLW6nJ7BecxGDfMePfF4Er1ew+lbHsYXoigVKeor8+kYHKE9045CxMh2aPj+9TksFhtKJEJRiXyXhFknqCvP4kJfkL6xJcoLXQQjJiamvXSN6qgtNFBelIHFqCYaizGzGODli2lK8p0oVWrGZpY51ZVJ/9gCt+MpVKpy0qjpGFgglsgkHk+y5AviMOtIpBVIaYnLV9v53BfuZQlxF4RA/OzUbE4KIR6QJOn4e9l5PZvRdgCfBbqEEB1rsS+xmmSeF0L8CjAJPLn23VHgIVbHa4eBXwZYSyr/A3ijp/4P3hgsAHwBeAbQszow4J4NDgDQarUY6qoIn75IxSMHURkNZObnExcCa3kxCrORaCCE0mTCtOIn7rBjzHRRlkiiz8nCUFdFMhAg7QtgbtnEys3bVBzYQ8ofRFtfQ0EiSTqRQDKbsJWX4p+do7y1GSHAWlRAyO0l7PNjMhvRSRJVTz6GIhwlFonQ8NjDSPEEKbORikP7SfkCRObdWDdtQDM9R1qlRExOg16HobaS4JmLFNXXYmppwnPyHFnl5QibldrHHoJYDE15CSsXr9H0H/5fYoMjJCxGcqsqse9qZaWrn2gwhCYRJ7OqHKHTolAKCg/fj7+tE8v2ZpRzC0TOXERdVoQrkSQ4MU3s5m3MDbVEBofJ2bcD99nLuPLzUDfU4j19juyNDZibNsDQKKaqMsJ9QwRudVH3qSeIDgxjbGkifPI89vwcIqEg6XgChd1GKBikYEcrppJiGBlDqNQY6quJnL+CPT+PrO3NaPR6HKcuUVhRTiQQZNee3Xg9izz+C7/AwsQkn/vcL1NeXs7R116msrqeX/iFI6jVGuKJBL/4xIO8evQ4bvciQgheeO0aNRXFHDnUgN2ZQTS0wiP7K4imNWiUKR7alc+Lp4Z5+qmdDIzPMD4X4qlHKvnJpRm6x8Ps3VLEtmodiWQeGpWSbTUW5pcTRGJJdtdqUCiq8AXCCIUSq1mHzx/E6xOc643y/z1ZzvWhGN5gim0ZZspzlEwvhCjOtrC1UsfJzgC//FAJ57v8pBNhUkLDgQ0G1CrBCZ2GZErCoU+wd3MRgUiaeCzOxhIjkUgUoYC9zSW4l8I8ttXKwpIFIdaa9ToFSqWCQ5ustA8FWMmw0FKmIBItQKkUbCyAwQV4slrPqdsRSlRRjDoFOdY0BVkW8hyCkkwNfRNJdm0qoiRDictSxPxyDIVCwWJQzS89kM/xzigtDRbC0TgHNhoIRcrIzdShVqcpzVYxOhdmb52dB7YZMavjRNNqil0wOGpmR6UKpaKQpiIll/qjHNpsIRavQKmAcCSGVqtmR40eSZJ46ZqaHTv23svi4a7dq1qLEOJbwMOAW5Kk+rWYA3gOKAbGgU9IkrS89mP/r1ktU8PAL0mSdPN9XsLTwH8SQsSABKtdGdLdjkhbt2Y0SZIuSpIkJEnaIElS49pyVJIkryRJ+yVJqlj7XFrbXpIk6WlJksokSWqQJKntjmN9S5Kk8rXl23fE2yRJql/b5zff6yiJt7kHFk+ex7lvB46aKmbOXURdWoSjuZFwzwDeKzfIPbiPufNXUJcW4WyoITk5jcHlILKwyFJnD9m7tmGqr6b9r75OPBJFW1ZMKhJl+cIVzFs2Qk4mQy+8QjocwWQ0Mjc+TspmJTo5A2oVpk0NjL/4GoayEkilGDh+Gk/fAGmNGvfICMmZOfRlxRCPowqE0WW6sDXVM3X+MimlwOv1snylDb3BCOk0nnNXsLZuRl9RgudaG8bKUkKz8/ivtuHavxuN0UBs2Ud6ZoGs6kpWOnvQ6TRYsrNg2Y/GbsMzNs7M1XYS3mVMLU0EO3uID46Ss28nwy8dJRYO4/OtsDI6jjbLRdygIzgzi95iRl2UT9fffRvH7u1k72wl0j+EKhZHUiiYGxrBOzWN0GkJJ5P4JqYw5ucS9wfQqTVY6qtZGhlj4cYtXDWVRMYm0BkMZNy3k4XTFzBlZ5J9YDczF64zc/kGm3bvprp5M9/9269RVFqKVqvjlR+9wL7du7DbHXzlK/+VA/ftQqvV8Pf/8F0eOriL0uJc/vjPv8Z9e5o4eKCVyzf6sZiNqNVKXjjWyWMPNFFWWsCNrjlcDhPReArvcgiT0URRnoOJhQh52VYCoRhXOsZZ9PqpKrLyzaMTHNxkoyRLyQ/OzLGxzMIndmVwtM2PSqngyFYbf/b9PhY9K+h1Wv7y+QEqc9Sk0xLdo0s0FGrYt9FO+0icaZ+K+7dk8z+/10NxphqjVrBvg4mRuRCPbXVwpjvKsXYf2yo0uBc8vHRxCpcphS8QYtanJM+hxGpS8e3X+ljyR9FqlPzeNzow6ZWYtfDnzw1SnmvgM/tzuDoUw5/Q8Zn9ebx2fYV8p5JYJMhrN5apy1fiWYniXfIy5/Zh0qv5hxf70KgVXO5Z4lr/Cv64ik/szuLVa7PolXEseiV/++IIZoOKvskQyVgApRIeabXy+q0gDpuB2aUk7aNxNhRp2Vtv4NztFay6JE3lJlaWl/nGy0N8Yk8O/3x+ho2FSlxWDZnGOP/9W7dBSnPuxihOs4pd9RZ6JmO8en2FAxvN97JouGv3eOjzM/zbxzve1aMk78faUGeFJEn6f1dDn38WjI6OEI/FCd+4TTQWITC/gLenD4DwwiKxFR9mownv3CwZQ2OkZ+YZbe/EVlSA1mxi5twlyps3EZLSOHKykBQCz+mLTPX2Y3U4UF1tZ3FqhoziIvQNNfinprF6nKQ9XvrOXsBRXIQrnmBhaAyV2YylpoK8ygp0DhtKu5XQio94MIRGo2X4ejvm7ExU128RDoUw2KxYbDb0BbmMHzuNzmwkML+IOdOFRqMm7F0isLiE99wVpnsHsORmkTx7EaVCyfzQEAabjVBPLwazmeyyUtxDI1hyssjfvQ2VTov/H76LsBjxtXUy39OHyekA9yLmzAz02Znk5mYz+IMf4z17Gb3BQPezz1GzawchtxuVVsPcuctYLRb6L16muHED0dEJXMVF6M1mlto7MKjU9D73YxoO7GN5bAKj0UBjVQUl9XUErA4US376rlynef8+LAtLzI2MYVKpiMWTTPT2Yk5KTKoNDF66gjqVZm54lNCKj/a2NhLBEIFImNrmZjqHJlkOROjt7eG1Y0YSyTjDI6O8+vo1dFo1//zDV3nsoV1MzHgJhSK8cLwHhULJq69dZ8/2Gpx2K//1r0/x5MMtnLkxTVv3NAfv20L/nMR9e7cgJcPERIo5b4jOqQQatY7bw8OYzQaG5zT4gzHGphZRiXzKizLIcpnZWKikf9SERZ/mJ20exme8DBe4mPTGGZv24PVFMGlyyHKayLYrOdHuRaUxsuQLc/J2mMnZFZb9IeJJCY1eT2NOBvFkkpFJDykglbBTmKEmL8vOrgY7WTY1K4FcijK1VOYYudAxw82BZYYsZi60jVBZksHlQTXtvVMYDRX0ji2RTKe5PaJEqxJEonFMJj1bqwx4g1XoVBKZFvinnwyxY1MxL14JsbISIiW50ChTVBRlsK3ayJwnxPC0n3hyBZWqiJVAhK7+aUwmHZKU5uqgFoVIcezyGK0bilgKKyjONjGxGOfSQJyRaR83xzIxatP4g2mK8h3sqTOy5MvmSrebytJsrtwaJNtl5tqIHnOO54MvRIRAobk3o9EkSTq/NrL3Tu/qUZI3RgK/G0KIYkmSxt/mewHkSZI0/dO2ATnZvK2ysnKss9MYWpqI3ewko6QYZ2kxmuxMvNfawWknnZtF7cMPklz0YGiqxzk9jdZqQWm3klFehq4oj+jMLPmH9hPrH8a8fxek0ujyc1BkOMm3WSEni8jACPHZOfQmE47d24j6/JgyM0hluaj/xBHCQ6OYc3JQznmIJBLohYL8igrUWg2GzRvICQRQazQYW5rQxuPoegYgEsOQm0NBXQ2YDBgtFjQGA8bmRpKXrlG4bwc6vYEai5nw8grOfTvw9Q1SWXKA0Nw8zsICSKdR1VZQYTaQVKtJTc+xPO8md882iCdxbNuCSqlAm5lBatGLbc82Fk9fgOwMspob0dtspIwGsmuqEVoNSslI5aeewHf5BvrGOqrUKlLBMI7NG4m23yYRjZGzZweeC1co2diAQq/j0ac+w8LIKPk1VaRuD1LZ2sJYxM/TTz9Nd28v+RvrKTBamJqbpeHIw/SgIMtq4/AnnuDUj1/k8EMPsbW1hZdefoXGxka2bt/OwOwUTdu3cfGlVzGYLFTX1fHwA5tYcHuxmo1YLBaWVvzUb2jk8OEHCSZOY7OYyMsys7W5BoVSQzwWpmFDMdPeJBqDg/07KrhwY5ydm/N56eQAT31sE//44xtkFZv41U9uo2fQwyf2uhiZKaQi38jicpTqEifzFj11+UrUSgvelSgXuiJ8an8uHeNp7ttoR6/TYdELWiv1eJZtFOQ6ybVLFO3IpXd6ta9naDqIprUEk15F3GWgqthJfZ5gwisRj8ewmMyUF2WQRsHhrXbG5oI82JpD32ya/oklDre4uDoYw+cL8quPlDHqFlh1KR7dW0kiCRpFhOJcGwc2GtCoCnAYUhiNWipz1KwkzNh0ca73r1CapWd8Psy8N84XHq+ga0riyFYrDpsJTzgFEhxqNtA5HiGdkjjQWszwtJ8dVWquokOtsJPn0NA3FWZbtZ5wNEFHUQaFWUZaKnS8dNVLQa4TizZFeWEmBxv1rATj3JowkSlFeK1tmYdb7dwYVFOcrSEQyEGtVrKvTsuMyvUhlCLvqs/GJYS488WN31gbSft23u2jJO862QB/JoRQsNq/3g4ssjoirRzYB+xn9cH7t002H/k3xK23SCJGaH4RrUJQ9NiDhCdn8A8MY3Y5EUYDgY5ujJWliEwX4z9+jYztLahSaXB7KTh8AN/EFOGZeTQ2K+FkipWxCQxF+SjDEaK3ezE01GDMcBKbn8dkNhGVJNyXb5C9s5VkIk6gqw9jcQG2nVtxnzhDymjA0bKZQEcPGr2OUDDI4umLOHdvQ1NeTLi7H+/Fa6t9TckE8yfPY2isZWV0AvuGWhTqyIgvAAAgAElEQVT5ObR/9eso9XpU8SRDx07icbvR1JSzcOo8icUl4vE4wxevEfT5cc/MMfyDF0jp9ZBIMNfbx+LwKPaqSvAs4bt0DceubYSmZtDkZgPg3LuDqeNnifr8eAeH8bXdQpvhxLfgZurKNWK3+0Ch4NbXvkUwECDhsOA9dwnJbCSmVTP12gk0LheLk1Okx6cxZWUQi8cZefUEew4/SFV9HZ0vvIpnZo58m50rf/8dpkdGKcrJ5eU/+Uv27tlNIpEk4PNjU2nZs2c3R48eY0NTExsbG/nfX/8amXl5rCwtEYjHSMVj/NqvPc0rx69z+kInDx3czXe+9yITU/M89dkn+eO//CYPPbCPHVub6ega4+nf/jN0GhWRSIy//dartGyqZSUY5ze/9E2KCnL40v98kZ3Nq89zHTm4ge+81EN1iZ3H7y/nay8OEo9GeOaVXqYWguQ7BJsr9Hz35DSbSrXsb7RwtdeDRq3EoE7yzxfc7N1gZcYT5nTHMttqzeTb4WznEoVZBpzGNOPuGH0zKfY32hmf9WPUqokn4WJ/lE2lOrbX2ugcj2M0GjiwwcC1wSg902kq83T4/GHCKR1Oi4Zca4re6SgGjZKLt8Y43+lGo0jTP7GIP6pgb6ODZ49PsbVKx6ZKKz3jIdoHlqnKVZFhUfLa5WlWwmAz67jQMc9SAHLMSb7+6jgLC25u9U0yNu1mzJ3k9I1pptwRcm0SezdYePbEDAoh0VJl5fj1WTKMEm0jUY62BfiVQ3kkE3FGFxKYTCYS8QTxlIpDzXZujsY51RVhX72J5RU/Y9Nejl334I/CV5/vwmpSUZ6l4EeXlojFEx94+SGEQKFU3tUCeCRJar5jeadE87anfovYe+pmkCTpSeD3gSrgb4ALrCaeXwUGgPskSTrxTseRazZvIxaLY9Ro6f3+c1Rv20r4egcGg57e42eo3NaCKpHEPTOL7tJ1AksrLI6MY3O5cI+OkUqnUapUeIbHUAjwnr2EyWik54cvUXNwPwNnL1LYtIFIZy8CSKVTTPb1YXE4mZ2ZwaTVYtIZGJjsxXS5jUQyycLoOLrFJbKWlgn7/cxPTKDV6YgGg5hv21CqVMz0DRANBNGoNaglWJycwjSSyeLoCPZMF0qbFXtWFrryErROOxnzbiSVivDUHJM3u8jcWIvRYcOS4SRrRysoBd3f/CfQazEW5JMC0p3dBNs6GTh/kbymjYR6+pju7qXaYiHsD5JSKbDlZmM0GMDlxNvTT+n+3ZBOE/X70daUYzKbWBwdI3trM/GVFQbOXcYVCGHJyWZ2eARzSQEZRQUUlpUz3naLxbFJslQaJi9fZ3JigkKHi9/47GfR6XT8XVqitLSMAwcOcO61n9B5+SoGk5n/9aUv85u//usMDQ1z8tQpUCmJJZPEBQxMTxJcWmZ4aBBWfBj0em51jRILB3jh6EVqamppaGjkWlsv7sUlunsHsNnMPHhoH/0DAxzY28x3n3uNkuJsqssy2dxQwMXL7RzcU8fC3AwjE26u3ZrCYDLi9oa42LlMNJpg3h3g0PYi5r0xDm/NYnIhyMBUhBV/hFeueVFISdKpNEMzUWJpJaPTXs72ZJBISlzqnCGtKCOZUjEx5+PGcBwJLX/zwx52NJXy6g0/4zMrzHoChCIJNla48PiiAKSiPhb8KeasRnpHAsQSaX58Kc6SP44/6MGoLyGV0jA9v0zPhIa60hyynRrKs5WcvBHGH4iTqsxl1u3jQq8TAI1GzXdf7+PhvXWUZKrZ1ljCjmo9Z2/7qCrLobbIyODUMoNjHr74ZDUagxWNWklLpZEbfSqW/BE6hiUisTRT88tU5mqJxaG2IoelcITrpweoL8/iylAKodDz7LFBCnIcTM4tk+kwoVRnc7F9jKqSTJ49PkPnkIdPHKhmR52VVEoiGisiy65Dr4mTSsQ4evwcT/7y73zg5cg6j0ZbeKN57C4fJXlPJEnqBX7vvV+mXLN5W1qthrhOQ37jBrQlBRhaGolIaZzlJRiaN5JKJimqr8O+sxWDUU/FkQfRFOXhyM8jt7IcUZRPweYmbLl5OPZsJ6HX4SwuQldaTElTI3qbBUNjHfrGOoQkKGrciM5oIK+mGnVVGaKyFJ3JhLa+mnQqTdUnnyCruBDzti1oTCZyS0twFeST17oFVU4mitJCrFmZZJQWY968gaSUpnhjA2m9Hmt2NuZtzUQWFin6+KMkRidJxpMYXU4sJiMak576Tz+JRavD3zdE6ZOPErrdQyqeoGjXNhRuLyqNCuVKAGdzE+rcbLJKS7Hl5WDeUEdWSTGqTCeGzQ3EgiEKHrgPg0pNyu/HlpmB2mAg0NZB6ZNHiE/P4rl8g7InHyM2OkkqEKL+Y49gdzpJadU4S0twlZdid7kIB8NsOniAuqoqCspKOfjAA2g1Gn7v977E2bPnALDZbMRjMZ57/nme/p3foraunl17dqNWqdGazNzu76N1zy42tLbQsms3jZs3oVWp2XrwfooKCqlsaODJJz5GTXU1BYUFPHHkEKWlZUxOzXDkscNUVpSTn59Ny+YNnDl7ierKYtpu9rKpJgejXofVYuS7z5+itjyLM5e6+Z3P7yYQhk8f2URNiYWtjQWU5urJsit46tE6fFElm+oKuDkWZ0O5E5VaTW1ZNo9vz0CodPzao2Vo1Aq8/gT/4eNV6NVpVErB/uZ8yrNVWLVJHmgtxKAFuzbO4Z2lZDu1PNBkpGVjIVsb8snNsjE2F2J0LszoXJiFpRgSEqFomnRKIsOu58g2B06bgaI8F7tqVPgCEeoqcmmptJHt1LIcApNBw6a6IkoKHGSbYlQVu6jMVXOoyUAkGmdXcxmBUAylUkk6neJKr48cpx6LUY1Wo6B/TvCfP13HwkqKLJuGbHOaycUENWU5VBTYKMlz4LLpKStwsm9TNkPzEg8228jOdLBnSyVqrZZdNVp21epoqi2kptjOvpZyNlc7sGihqTqX8iwVlcV2HtheTSChRgjBsbYlDm+xM+WJU5FvxWDQcWDvtg++EBHr/pzNG4+SwL99lORzay843sraoyTv72beHznZvA1JklCEI+Qe2IO/b4hEOII6kSJn70687R0Y8rKJRiN42zowb6jFkJlBzz+/RCweY3ZohIljJ9BWlqEqzGe5qx9VPEHJxx/B39aBymknhoL4vBvvlTbMTQ2MX2/DsqWRjH07WLl8g+W2W5Q88SiD3/shpopS1FYLSbOJld5BjDlZuGdmWJyaRiOlGT56gt7vfB9dcQHmTQ0sXbqGzmBAIYE0M0/u/fuIDIxgMOhRajSEfD68F66gr6siFAwivD60OVkkM134JqdR6/WkTUZmTp7DUlNJeGkZT0cXuvJi7FXlpGbmcJQWE4vFiU1M4ygtJuTx4rnVidHlRG2zEAyH0Qol9tZNTB09gbmqHKVahTKexKDVorPbSC6vkF72oSvKJ2Uzs9w7RPHDD7ByqweFVsuyRkH70eM0b2tl8/59/LevfIXDhx/C5XIxOzvD/Pw8ToeTeCJBOJmgqqaGxfl5Thw9xud/57c48fpPaGzdwi/80lO0XbvGlfPnaNy+jYmeXpY9HgoKC9n98GH+7M/+gs0NhXzuk4d45bVTaPU6trQ28/0f/JBHHrqP621deJeWyXIZWFlZYdE9x8bafMoK7Fy+3kO2U0u2y4TdrEShUOCyKRkc83D91hifOlxN/3iA2fkQ5bl6EtEwqVSKZV+UYDiOw6yhwKXgWq+XgkwDyRT84+vDuCxaBhcUXOmcYcHjB+Xqr/u2fg82k4Zr3XN0jIXZXW/FH4jy3Nl5tlfpGJ8N0Frr5MAmFwaTjdZaF/m5GUTjKbono/zKQwXYTWqu9S1TW2TkQKOJk7f8ZDv1ZJolfnhxnk0lGgyKCK9cmaehUMX9G8283ubhcGsG5zsX+IvnhmksViGQaCxWcbptiuOX+mgf9KAmQjoZ4QfnPTzaaifbaeBc5wJbKlab37omYqhVCnbVW7k9GmDKm8JuUnG9f5mqAhNWo5pocIloOMCBBiNnusJ0DvtoKNRyo8+NToQQqRgd/XOcuTaIUGnYVqlDq9NiUERxr8Qw6PXotUqqshX0T0cwGXSYTcYPvAwRQqBUa+5quYtjfR+4AlQJIabXHh/5E+B+IcQQcP/a37D6KMkoq4+S/D3wG+txf++G3Iz2NsbGxgh6vKiv30Kj0dD+1b+joLEBRVsHUx23qXpgH0tuD9HefoqjMVZCYcxOB5l7d2KcdzN1/AyRnn70yRS9Fy9Ru3snkbbbTN68jau0GIsrg74fvYJWp8MZiRIOhAgNjZBUqlDr9Uzduo0iniIZj+O+dRvL6Dg6nZ7e82co2bQRg8WCNTMDw8Y6XG4vOqsZhcWEf3yK2b5BTDYrkYAfa3kZqts9zN+6TU5DPXT1otRqmeq8jdFgxDsxRTqVRmU1o9PpiAUDLJy+iMGgZ2likoz2TlRKFePnLlGwoYF07wCTtzrJrK7EWVjA8MkzKFVqCmtr6Dl1gaodW0nf6kZtMjF9s4MCIfAMj5KRm0PAu8zkrU6sxQUobnYxfPMmuRUVaG90oFUpWZ6ewn32MjPdPdizs8koyKP70nUK9Sa0ei2DQ0OcOHWaZDxOMpnky1/+ff7wj/6IP/gff8CeBw9x4thRjh87RkFpKTevXefS9RvYCvIZGh1FaDS8/vLLpNQqnK4M/v7Lf8CjTzzOtZOn6e/vIT/XQd/QFNev38LmzEKjUvPyK8coL87CbNDx5f/6p2xpqmRsdJzJ8WHiER+RWIrjZ9t4+EAz5y91I6XjpKIhUmkFz/zgLPfvbuDYpXleP93Ojs2lnO30YbPo6eiZ4kBzJn/z40Ge3J2LJ6DmhQuT3Le1CpNDTU6GhX0bTAghWArm4LDo2FFrYH45jyybhrIcFTf7U4zOePnxJQkJBROzK1wccNIzModWq0CnTLEUWOH1K2HKijJRKpRsLDPy8lUv8ZTg7M1p7t+xgTm/xOkbY+zeXIrZaGZkapZj7TokCTr6PeiMDrQaBZ6lAAPTETaWGDjnj+JejtI9tIhZl8WRnXnoDSbsJjWSUDE07SccSXMr2wQoCIVjXOhPkEikmPcsMzoZI5kqZMYdIByJotNpuTXoYXdzOX2Ty0x746iUSS70apn3BrngXmZrYymzC0tQZaU0x0CWXY8/kqRrZImlgJVlX4SdNQa++doYh7ZkcPF2iJVgkjPts+zaUsac2/shlCICobg3xawkSb/4U77a/xbbSqw+F/Pvhpxs3kZpaSmmiRGMLU0s3bhF2X07MdkciCwXkeVlNHY7FpeTrPxcrDtaSJy6QN6nniTS00fYs0zFp54g1tOPakMNpt5+lBWloFRSrhBIyRSKkkLy00lUOj3K0kKqcjKRVvwYmpvA48U6O4dQKan/9f+H0PWbWLa3sNzRhaukEOeebUTauwgGg2jDEfROO9EVH06HA5VajVmthlSa2a5eNCoVzq1bUKUkDC4nhoZawqcvkN+yBaXdhquwEGWmC7VOiz8QwJyZSdZ9O/Hd7qXi4AFUQCIWI6umCmtDDRqXA2VaQmMyosjJJK+6mlQqhbq8mCohkU4ksTTVE+sdQGcyYtzcQHYwiHDYMBblo2jrRJ0GfWMdNckU6WQCS+smgnPz5NfXYSsuQKdWIUmgdDrZ9sQRVha8PP7op9CmIJVI8MnPfIaJ8XFOXrjAc8deZS4YZGhuhqbdO3FUlJJVVExufS2HTHqUQknrgftoP3eRQ0ceo6ymBu+Cm8qyUj79sSd59lv/wGc+/QkK8/OorCjFF4hhMuo5sG8LJ0++ztz8AhOTk1gtOh49uAmtOk2OS0MkHKI530Z3t569DVr8SzkUOEEoBSoR55MHynEHIuxrMhINVqAQaXZUKBmaCpGW4NytBRKJBP1TQQRKttRmk+9UUpGjoijHSu9UFJcZCjP0LKykePmKh8MtDo5dX8JcrScnN5s9TeCPqZl0h6grc7G1Us3olJMtNS4yrQpO3JhnOZCgucLCwKyeynwVo+4A+XYVSz47TaUqNErQKmuY90axaqKU5TvYWmNFr5aYXQzRXKambcjPZw6WoVQpGZxK01BqJ8ep48EdZUx7otjNWnKzbEiJMAadhqqiLLZU6GgfibOrVs9ooY3SDEFRpoEXo2a0WQ5yLUls9VmEYquJLDfDRE2eilhUQ0lWLtPeFA9uMnCyI01uholNxQoyLLUshyQyHQZeuLzMFz9WyqmuKKRjLC4F8Aa0JJMJJj0JttdaWVhKsLOpkPJcA5ZM5wdfiIifqbc+I4TIA4q4I3e803TQb5Cb0d5JloupY6cwupxktDQTnJnDe/k6xR97BN/QCOlYnJgEvvFJ9LlZqDQq4is+jAY9aoOBlMnExCuvU/rko0Q6e/FdvoGxsY65sTHmTpzBvrOFeDLB4uVrGMuKSem0xBYWCXf2oTIY0GY4UapU4LATGhlDk0yR+8B9BHsGSWs12FuaGHnuBQz1VZg3byA8MEyw/TaG+mpiPj85mzeitFm4/fVvocjNIuLz4WnvxFxZjqW+hvmhIeaGhgnMzDBxvR1f3yCOTRtZ6u5DmZaw1VfjGZ8ksuAm74F9+Du6CS96MeTn4J6dZfB7P0JtMqKzWeh65ntEEgmwmolNz6LwLlP+qY8z+I8/xLqhlujENMHpOYr37cC6tZnZoycJhAOkXXbC03OEugfI2beT7qMn8C0tE4nFWLh4FdJppEwHf/WHf8zC3Bw5OTmcP3uWV06fZP/jR5iZX+CJL/02GPUszc7jzM0hajHwk+8/R+v++1hYWODm5cuoFYKDjx/hwusnsOr03PfQQ3R2dJDpsnLgvj3cvNXNj188xoMH70OhUPKP3/sRX/zCL3HuwjUikSCPPLiHb/zjSZKJGLUVuQQjKb75/HW+8MkmLnZ6MWjVNFY4mJlf4ZWzQ1Tm6SjLFFzp9WPWSUSjUQLhBD3TKULhKNGEYO/mEvonQ9zoc1NfrGfWE2ZoNk5+hp5Jb5orAzEaS/W0dY0yMbuEQgG76k3cHIlg0ioozzNxvWeasWkvD2x28nevjPHpg8XcGPTTNRqgINfFb3yskis9bi61D9I2muDIdieLgSSff6SEmyMxjrev0FplorncwMuXpnh4q4uLPQGOt6/wa48Uc647iFKlZUOZjdkVgcFoYv8mJ8+8PkFdgZp99QbO94TQK5Ps22jnUl8AjVZLjkNHa7mSP/j2bVqrVpvPbo/6qc43seBZpn8mxuZSHYvLIQqz9DzasjoS7Ufnp6gt0JCOhxieDmA161EolVzsD9NYoiMQDPOTNg87qvUs+eNc75pgfC5ARa6JjpEAv/3JGjIdBkYW0kwtCx7fmcnwTJAV34fwbrT177P5wAgh/hS4BHwZ+J215bfvdn+5ZvM24vE4ihU/M13daDRqNNNzqJQKZsYm0Zy/wvLMPMlwiOKNG+h9/kWqD+3H3z9EXKfDMzJOjtGALpliZXqWvL4hPFNTJOJxDF19CI0GtUpF6HYfBrWK8eFR9Of1pHU6ApevE/EFiPhWiC4vk4/0/7P33kGSnceB5++VfeW96a723vtpMz1+MAMMMMAAoAONaERREuX2VrtSrO4iVntHKUSFTtJKWomiJHIFigJBwpDwwGAG40276Z723ndXu/LevvtjsBG6DR4F3dJIDP4iMioiX36vzB+Zld+XLxN1Ns/k629R1tFGdGSMg5k5zBVlWOJxEpEYidFJZGo1S7fuYCr2II7PsDg0Qu2RPjKJOPbKcmSpFGqzkZnL1yhtbSa7vIzociLFEngeOkl4fZONty4hrW2wNDhC47F+YmOT5OQywhtb6K7fQZDLmHz2OeqPHEYAyro60LU2kPAFMLndSEoFGe8ei29fpqCygtz1u8TCYUIT0+TTGfxvvENlzyHy3j3CBz7EpA6j0cz91y5S1tLI3uAIRqsZe1c7Se8OsnQaR0MtgiCwNr/IWuAAczzCt5/9BkfPniG1v8fswjzirTuAwKvPfwdBqaCwvJSNlWXuvvkONpuVt7/1HY6ePME7L7zE/P1xKj0e4qkUQ7dv8r/92hcYvjfG+Pgk8WQCpVqNTC5naGAQu1mNRpRRVuzE7TCg1yi5d3+WbDpKPptjec3LlWEH49PLaFUCqXQBk4t7WM16bo/vEc8quDGyypHOcpxmkT/+9gxumxGVWs3JDjcNJRpCURO+sI68oEShFHjp6ipWk4ZgJImoEbm9YKay3I1GpeTecopwQuLi9Wna6ou5JpMjihrKi3RMbuTwh5MMr4DVbuGN25N0NpWyfRBD1GgoKnISjOe5MZNkazfCyKzE/HKEZCbPlQkNG1v72Cx6RpfCyBBY2AowsGhlcmELm0lHLJFhbesAjajkrZwVhVzgu7d2KfU4uDSwTFWxDX9MQi7A8MQKalUVOgVUlNiZWI0SSwkMT/roaS1n359gYyeCxahlZHIVc18dd+ZTOE0Kcnktrw74Uas0PHtxhS+cr2ZtK4wkU/DqnV0EmZqBiU1SmUKsujwNVQXIhTyheJLZlX1Giu3IUPLCuxP0tpTy+lCeRCrHjTsjfPrHfHIhIPw0dX1+EqiVJCn1z1p+H36W2fwAVCoViWiM9i9+HlGrw3K0h2wuh7OyHHN/DwXlpRQePYyquBBPTTVKuQKxpIhMKITWoMPY1khaVFJ2/AiSxYTJ6cRa5EGsq8ZgMqI3GNC3NiCUFlHU3oKpyIO9vZl4MISjppLKQ104G+tQuhxoO5tpOHkMAXAe7iafl7BWV5IUBOy1laib6xBKPVg8hVg8HnRtjVR0tiM3GtErlJQ/+SgEQujbmmg8dRyVKGKqqUQVjmKurSK17yO/so69pgJ1Uz2OijIULge6tia0KhUFh9rRNdRg6OnCXlKMuqgQp8tNKpkgHYsTG5vE8+hD6IxGlDYLFUf6MDfWgqim5NhhTK1N6MpL0JnM6DuayYpKqh49g63AjaqhGkdlOaamOlQ6HYUnj5Hw7iDPSzgffYj7b19GLldgdTtR2a3Ud3Vi8hQgNlazGPCh8BTgc1nxl7gwlBVRWleDWqbgP//5n5LLZiiqrKCwoJDzTz6JRqnij/7wy2RTaX7pc5/DolexvjyNw5Cn0G2gq6OF8+dO4dvfob+/k0PtdRxqb0Cv05CXQK9V01RfyYceaSMvwacvtFFXLOKwmHDbTdR75Bw/VEZjuZHGCitNpRpO95RS51GilmWQywQ+ecrNoQY3a748ADK5lqONBhJpGf11Ig3VBTTXuKirLKCizM2pDhtOhw2HVaS6UM25LhN1lcV01dk50aSjtMiBRqun1Cnjof5aTAYluXSSztZKHunz8OSpCvQmK9XlHj5y0kMkkcduN1Fd4USQq6gvs3KmVYvVYsRj13KsxY4kyGmtL8WuTfP0iWrKSxycadWjUMgR1QpaS2V86HgZJr2GRo+MIx3l6HRqzraImHUSR1tdGEUJKZ/hQn8BVosZu1FJb2sZxxvU9LcX8/OPViDlc3jcNqqc0F+nQVIacdiMPNVnQ8pnqCl1EIommF8PsrET4tFuB/VFAoUOAydbTSCT012tJZeTkCsU/MpTVUTjaapd8NjhSmqLtBypVaHTKHjs4eM/fici/PSMheZBwYHyn7X6/+BnweYHIEkSGr0OtUGPZNQRGJtA63Zi7e1k7Xtvoq6uwFxbTWJpFWuxh4xcRnxqDovTiVKvI5fJQCiCo6uV/J4PtcmI9Xg/wTvDqFUqxIZq4ourJKZmcZ/oJ+4P4BscpeLRs0hALp/H0tlKaHKOhD+ApNNi6OnEd+02VSePklheQy1B4cnjxGcWiN0bp/jCOSSVgtXvvYmy1MPs2xeRFRcCoGltJD49Dwo5lqM9+Abv4dv2ohRF1t68iL6lEbGinM2L71H6xCPEdvdJe3fR6XTY2ltIzC4RGhim7OnzBOYW2N3aQq7XMvbXX0esq0Jt0LE3OoHGZMTa3U7Gu4tKqcRSX0NsbRNlLo+trRH/+BRqpQql005SrWL7nauUXniE5PI6slQatcPG+uAIivJi4gc+NnJpXv2T/0b1scPUnXuIy999haojvezPLpKVy3Ce7Gfnxl1237mCvbmeqYkJcpEHLflzEty5eIlf/Y+/ya2rV5Hn85SWlvKRj3yYz3/+85SUeLhxZ5Q/+JO/4/GHD2M1yPm7r3+Tsye7+fiHz/GN59+kyG2ho6WSv/ibFxAVadSKPP5gDIVcoqXOxVeeH0Qhl9jeD/P3b8zhsUB3g52xtTQzmxnOHbJzdewApVrDrz5ZyVdfX6G9XIVWnuLK6B61HgUlLh1L3gRvDAU41awnFM/jchpor9AwPBdCo5ZzutvD8GKSrf0oPc1OFr0p3hvz0VmtI5nOMriU5Vx/Mdt7YfJykY+fb+DOdJjvXtvibJ+bfD7PG3f2ONJZQEmBgf1Qiu4WDxlUrO3EKHep6G8w8g/vbtJTZwQk5nfy1BTKWV3z8nv/MMmTfXZMOjXXJ8PUFYnUeeR8+R/uk03HaS1V8O79KNm8ksYSPbMr+1we2kbKpgnHMijUWtLpHO+MhjlUJeJxaFDLkpDPML6WYdkbo8KlRC1LMrkSpMCm5ak+KyNLCZ4+VcXnHinljeEwk2spPneumOtTcWJpBaIij9UgZ3p5n3vLGXb2Q/z5S3P01uk5iMC792M81mn8f00R/bEhCMiUqg8k/waIA2OCIHxVEIQ//x/yQRf/bBvtB7C4tEQiGEI1Mo4oCNx/9z3qjvSRD0UIrK/jWCggOj2Hf/8AUimcpSUsDY1Q3NKI0mpm8bmXKTh+mPDENPM3b1Pc0kTq5l2CB34Sfj8us4HgvQkEtRrZzUFkksTO5DQKIBGNsL+8Rq1cjtZiYvQr/526k0eRQmEWB4cp7mgltrtHJpGkWlQz++4V6o70Eb07Qj6dYXdxCW2JB7PLTXB5lez6NqJWw/r0LJIERfEErm4NyxoAACAASURBVL5D5Hb2SSnkxIJBEjNzKLVa0okk+zNzSAKsXLpGLpPBEAoT9e6SjscRRZG8XIbVU4Ci0I21sJBkKExgYprAthfbppfk9i4Lt4coaW1EHomwMjBE88NnENMZpt67TsORw8QGR8ns7BILhUjcn2bp3ih6u4NoLEo6lSKwtgFyOfFohHjQz9vffB67ycz9y1cp7mjBv7qJsshNfnCU/bUNDFoRdU6ipqoKMZXjyovfRZDLWZ2Z5fUXX2R/7wCrwcDLL79MIBDEYLXy1NMfY3l5heuXv8vY0FUW1oIsbYZRKSRu3h1jYmoWIRuhp6MKt03HyPgCnkInv/fnr1NdauPmPQmzxcjnPtbFtaFNVjf87EZhcH6fWyOr1JVZeX1QYnXbT3GBFW9AQSyeYWAph1yp4/LwPElBz8IehBMZfL4QBoOBawMLnD/dgjcocXVoHlGtwKBKYtYJvHlnn8+dryAcjjC6EKS62Mj0/CZ6g57LI0Ei8TxbK+uIosjNoSXsNh33ZoNcH5ilqNBGRhIQBDlvX5/jqdN1mDRZXrnlo7vBwfimgo2dEPNeF1cGFuhqKuHtIT9mo5aSIgcHMRmiKDI2t82tWSMOvQyDQcORRiPTaxHevbNOQ4UNUdAgqjU0VBuIZxVMLayh06mR8nkiseSD0mSVjHAky0EwjkIRYHk9zYePF+K2afjHdxY53l1LJKVgfs2HXqPiwGlBK6q4dW8di9nMyOQ6drMGUaYnmZFRXeri/CETWwdKEqkc790PcmV4nTP9dVybyaAv+PFXown/snY1/9p59X35/8XPgs0PoKqykht7W4idLQRm5mn8yJNIgSCSw0bt+bPk9v04TvYjTEwTnJ4Du5XmZz5EYnkNpaeQ4KWrGLy7ODpaKd89QO1xo6+vQbh+BymTQmu34w1HqXz6BNoCN/4rN3DV1WA72sPBtTtUPHwShVaHzG5Bd2cQTUMNkaExyo4fwVBejHnPTV6nRVDIqTt6mHQ8ge3UUXw371LY1oJCp6Ogvxtp7wB9TwcApv0DfN4dUChQmE3kt3bIL69R98xTyFIZZDYL2YEhlCo12uIipEAIhVyO/WQ/B1duEdzdBbUad08H0YFRknPLuE/2IwvHyGg0lJ89iSiKiJVlNCqVxMMRrMf6MK2uoyx0gV6LZWEJeXkxie0dbHU1WCJR0mYjNQ8/RHR9E2NPJ9lcHqPJhKaiFP/V24i11Wi62tjf3KbwRD+STEYoNkNZZS+ashJqFApkSgVWlxNLIEp5TTVaCSpqalDEk7TWN/DS9Mu4rBaefvpppmdmKG6sZ2x8irB/i09+9DE2VmfR6vO0txTy6U9c4PkXX+dLv/N53rp0m/amUtY3d3BYtZztK2BkdJoLJ0oBCY22nrv394in5TRWuym0yOhuKsRsMhCNxOksl6OWFWM2qThUJTI2o6SvRsXwfJTKEieP9jpRKeW8dF3C47JytFFNMFKOXqOkt9HMXiBFKi9QXOzi5ugWu/4Ir93axOtPodIYELRGdFY7ToeZc8fL+fZb8zidFs4f8+D3hSguMNDfamd2yU19pZu+Jgsb3jBrG1ZaKs1888099gNRyhwFLHtjNJRbaS6REeuqwqCRc7rVwJvDYZKJBFVuHdPrITrr3DSVKLgxleCTpzxs+TJE0wp+85kmFnayqBVZWl06ppYDVBaItDUUEYtnMIo5doI6+mpUGLUKXhnQ8NvP1DC0lOPtW/Os70ZBkChyWzjVrEGtkiOX1bLri9NbpWA/mGFn30qNWyDbUYGUh2MtIpfuxymxp/H6UwwvZnHbdRxt1GPUV3MQSnG2V8Om6idQjcaDwP7TgCRJzwqCoAJq3lfNSZL0gXsA/Wwb7QcgCALJSJR8NovMF0BfUUoqlSI8PoWhthplRSnR8RnkgTCaokL8k9MoHFYEt4Oda7fp+PVfQh6OENveQSwpIu8PsffOFUwtDVR86HF8AyPUPf0YbO0Qnl9CV1mBqaOV6MIyGpMRS10NscUVfFdvY29rYvxvnkVsqEEuioRGJtG2NGKoqiCyvUsul8N0uIuV73wPmUpFwbE+du8Moi0rJpZKkYnF2b98HWNbI3KFglgwyMJzL3H/nfdQVZVh8BQiHfiJjIzT/MufJbu2SXRtA0tVOXmFnIPRCYy1lXgaG1BVlnLw3k0OvDtojAb0bhcp7y46hRJbYx3RTS+ZSBRJpUTb1sj+9TsUHe0jurDM5puXsXa0MP2tl5ByOcSaCnLpNNnVTTQ1FdhOHGb9u29ibWkkvrKOf3QcfUMNjp4OAkOjyEIRtLWVLF6/RcVjDyNt7RBe38JQWkTxiX6GXn6NorJSalqaGRu7zxsvvMQv/MLnmZ+fp6GhAZvNzubmJtdu3qSxvY21LS9Om5adfT9f/9Z10pk8vZ0V/PXfPUdthZPiIgfHDrfx+//1JY50FoGU49tvTPH7v/kQr17f5NLAJoeaC3nl4hjNVRaOdlcwvhLjtetrdNcZsZmUvHV3hyPNNiKxFF5/mu5GFzcmw6DS8qlzFQzMRrg+tktfo5VsJsn3bu3x+GEXO/shAuEUFpuZh/uLmV6JolaJNNcW47KbuPBwG0+dqcEXztPTVsHTp2v45mszWExa4ok4cysHNJYb2PXF+d57y3zoaCEBf5Bdf5K7UwHaqi2ML/hprHLx+1/s5sZMkpW9DB8+VsDIUgq7UYVelWdlJ41WLae/Xs9//toYBlWS3nojVyeiOEwi5YUGhueCFFi1FFqVrHvDRNMKqguU9DfoefbiGh1lSlqKYWhmj/PdFt4aDnJl9ID2chWZTJ73hpY4e6gAb1jFTkjBL54v5eJYlMXNMAVGice6TFwajzKwkObTZ4p4Y2CXCqcM8gleurFLb61IX6OVt4Z81JeKyKUM79wL0ler43SLjteGwvyQJ5B8QCfyoF3NB5F/7QiCcAJY4EF/tL8C5gVBOPZB1/8ss/kBpFNpsuk088+9jLOjmejYJLJ0loB3F8PtB81Z5+8M4m6sQ9CIpBIpvDcHETJZAtteHGNTaE0mJr/9Es3Hj7JwdxB7eRnKiRkEBHYXlzHb7eysrGIvKkJ5pAcpl2Pl2i0MJUVItwbZXVoGlRJ1Nou7qpJ8LEFobgEplydyZwhJgmw0ind9A3sqTSoRJxoIIE4vkEkmSY1OojcYGP3K1yiuq2P71gCiTofzRD9uhYLZr/8j6b19Qpte9tY3sRd7iC2sIFcpWb1yk5rebrRqkeXBe2gePgWZDOGFJWQC+FfXyaaSeBQK4tEoBxub2DNplA4bWxevIDMaUB748E5O4QhFsJcWs7K1ibO7HYPVSnhvn/zV2ywNDlPV3UVs4B7ZbJbAzg66kXHUopqNO8PU9HWT28izOjhCeUcbkZFxVCo1/vuTpJNJdD4/ua4Ott+7xe7qOtfffpf1hUWUopq54VFef/0NMpkMU1PTtLe385d/+VdIahXvvvYGAyPDKHN1PPbISXq6m9ColWxv7TE8fI9Ct4ltrx8E2N7Z5+LNFdI5OSsbu1wfteK0m7l0awKZaKWurprnXrtPa2M5y2v7eFwGZDLwOLW88PY+Bp0CrVrO3762SW2JidF5H0+cbmZhM8q6N4xcISedOSCZlbO8GWBk0YbVqOJ3v3qbR061cX8xwavvjnGit47N3TBza2kQrUhIvPLOPR59qJvBqQOWVr1sbO5RUerg4o0lPnq6lNmlKNOre7iM5VR7NLx2dZlEMkXWrGVofI2H+ht4eyRMIp1lazvA26N6xma3MBo0lBVaeOvuDGa9hg2vmupSO1VFJrZ8WYYm12mtLSSSgtk1HzaLkcXNEKtbfnZ9YeJJF2q1yM5+jDlvnkQsjcUgMr4YRA5cHdkhEC9Eq8hS5DSyF8yi1qi4P+uFfBYhn+Z7txIcbbIxvhJlbs2HRq3krXtK5lZ2qC61oRL1zE8vYjabyeVTzK/sUuaxYtLKSQRzjC0E2AzkSWUEXn/7Ck9+6t//mL3IT09mA/wxcFaSpDkAQRBqgG8BnR9k8c+CzQ9ApVZh7e/G+7V/IBNPYO5qI/HeTUqbGtF1P/in3fzJj5LZ2CYaDmEtKMB+tA/f1VtUPvkYskyWZCBI3ROPIsgE6k8cIR4IYT7Sg3/gHlUPn0ZlNKAJh1mfmMCRzwKQSiapPNSOxmGnWJCBKJJPJBDbmojdG0dfXIw6l8N4uJtsOk3u1iBlzU2kpSzVH75AanaReCqFo7kBweMmtbqBs6Ic59njiHfvYehpJzoygbGnneL2VvL5HIYjnQTeCLM1++BMQq5UUXbyKDKnk8jKGu6KMqI+Pzt3R6j+2AUEBGqsFoR0Bm17EzGfH2dpCdaOFrKJBDsHBzgKXJgb69CZjMjyEkJ5MZpRE6hVuLvayOwdoG6oodZgQIaErrOFVCRKg+7BoLekXIY55ETd0kB4aYWKk0fRajQoSgoxmoxIgkA8HCY0s4CmyE0mk+bcFz+PbN3LIx96mle//vcc7u+nq6uTW7fv0NbWxs99+tP87pf+L6zV5VhrKnnK9CkiG4uMT0zx8NFqRibWqa8poKW5DrvVxOFDdbx9eYCa6jIunG3hhddHqK2u4NzRchZWfUwuuPnIY4cYuL9OpspNaYFIOODn3uQKsnwCfzhJX2sJDWUGiqxKNvaTOGxGTvVZCEQzNFZYePHSIuVlhRw/1srlu+s0abSc6i3i9v09jve30lhbSlO1g1g8S5lThoSARqOiraGAdDpDJttJoUOLWpnnidONbO8nMIoZrt/a5I5ZorfWyNIaVDlgcy/E1vYejZVO2qt1xOMuqoo06JR57iFR6DBxokGFIC8hl5d4qNVANJ6h3KVm6yDBx096ePm2nyd6zJzsqUWS8pxu1pJMFGLUKjjaZcBs0pNJpWir1DKzHsXjNNBTpeCtETVuu8DRFhuvDwapq3ByocfKG8MhPnrczr3VPK3FsOHVcazFSiqdY3B2lhJ3CXkhQ1VxJdFEFq8vwX98phFvCBKJKKUeG2daNdyYCPDU8TJMBpheTjA656eqsIwn+4x8906Q04d/Ar3RAEH2U7OBpPwfgQZAkqR5QRA+cHXaT82v8KMiMjBK+2/8MrJwjK03L2Hs6SSVTBCankVrt6J02omHQ+g0WvStDWy9exVDZTmizcb8xSuEN7eR6fV4R8fJGw3oOlsIDIw8eKq/pYHI7Dz2kmJqnjqPraAAEYG2X/0FUrNLhJdXURS5ETIZBI2IQhQRnA6Ci0vkjXpS+z4C125jPtqLd2UFFEoUBj2xaBRVJoOr9xDLr72DTKGg6PwjxCZmUGg1KNRqskoF8Y0tciol6XCMfD6PxWLGYDaTWt7A3H8IW2sjudV1NHkJbGb2743hOX2U4NB94hMz6NuaiMXj7A+MYGqqw3XmOPGxSTL+ILXnzmLUaokOjWHobCEWjxG4O0L5hXNsvn0ZbVUZQoGDrYvvYexoIp3JkA6ECA/cQ9tcTz6RQBGOUHD2BImpWSTvHvbOVuKBEDuXb6IuL2Hx8vUH3Q4OtZOPp9AkUtjKStjy+3jzm89x/sIFfAf73L59h2eeeQZ3QQFf/r//iE9/8Yto1Bpmbt2h68hhRFcR7156l/2DACf6qnj2+SucONLJ0qqXfV+Ivb0gn/noKUanNzEZtTzUX83I9C5jCxE+9GgHX/nGe/S2l/LwiWZujmyjVqtorC5CKVfSUWXjQ8cKmdtKk8nmaKq0M7fiIxIK01utYmg2zOGeJspLncSTOURRjcWi5fWba1icLj7zdAf3prZ458Ycpw5XsBfMYbfqefJ0BZduL/DGtQWeOtvE8kaY6XkfbTVmQpEoG944/+kzbaQlDQfBJKfabIwsp1nbl/jMwxUkswKDcyE+da6S6yObvHJzi4e7LEhIvDoY4KEOE4UW+NNvT/PREy6iGQUGgwGZTOCJXjNvj0ZQqeTolRneHdnhUI0RoybPd67tUOEUONNh4vpUlFhGxUePu3np5j61xWqqCxTcnvJR4tbSV6/ntTu7VBXqMGjkRGIprk5E+eUnyhhcTDO/leRQnZ2huQhWk0iJTeLW2Cb9DUYK7FoCMRnBlJpKt5LtgyTxjJyeegtbAVBpLXz5lzuIpQX+9s11PHYVOo3mx+4/HowYUH0g+TfAsCAIXxME4cT78rc8mG/zgfhZZvMDWFtdIxGNkJqYQeOwsnLpKkaLlZ3ZBQT5CqXdHSTGp0GQsTE/T6lCjm9hEa1GTWZ7C7unAF2Rh1QiQWjnANXSCtZEguXbgxS3NCG7P8XG9AwFeQlj1MzK2ASixYxmep5wMkHqzhDOY4dZujuIqbKcuD+IKKqJHfhwhSNMPvsc5Z1tBG4PkAxHCPt8pC9dJ+n3cxCNYkomQSYQ2tlFicDC1VsUtjQgjE8hk8uYeP5lap46j7ykkLlvfBtHWSmRYJCcDGL3JpDSaVbu3UelUSMBJqcTjdnErncQcnnkKhVKQcbm2ARaQUZqZZ3IgY/g9BwVfYeYvXQVZ20VB9duI+Vy7K+toy9wEQ9HiQ+NkYzHCXn3CLxfibfy+ts46uvIpVKEYnFUchn4AuxNTiOTy1GPTaFRyNnc3EI1NolCrSSbSKDMZLn+lb/j6GOPMPPOZTY3NziIpx48dX7nLidPneQv/uarzMzMEggFUb70AvMzc1TX1nLp5e+yMDlFcWk5ruIGlrwBVjYOuHFnArPFyO/8n3/Nsd46LGY9b1wa5Tc+e5xEIs03Xh6goaaczR2R3YMgt4ZXiSdzbO0GiAb2ENUqgoEgfe2V7AYzyHMJ/vjbK/S0VtHZ6CIYzbJyILGzFyAY99PaXM2X/vIiZ473IMhFbowuYnRU8fqNHWQKNa9fHucgkOC9m+OcOtLGu0MhAqEkW9u7PPviLe6OLFNZbOWNG3J8ByFCoTCl9iJcJok37uzyxGEXN+6uYNCK6DRyCox5Lo/uoVRq8PljZCWBNweD3J9ew27RcXksyu5egFwuz0s39jjwR2mrsTK24Gd5J81+KMXy+h4VRQ6uDG6QzCnRaXUMTXtx2Y1s+lOsbx+QlwAKmFzao9BtQyHT8MbtWfrby9hRKhlbOMBhMzO1mkYkSlKhJZ7MEosEGFkN0dpYwq3hBRxWLcmUxL4/wsBcDJNBZGZpg9pSO7VFOr7yygq/8GgJkVga784BkWSOK5NqwjGIpySmV4II1v2fgBf5qdpG+yIP+q39Bg/m5VznwdnNB+JnweYHUFpWijh9H1VdFblcFtdWLUqPG1sshlyS0DTWkU2mkQ0OUdbSRM5ixOByYD9+GP/9SWy11YTvT2FpbULT34NMqSCr1dL41HmSG9voWhupT6ZIJ5IY+rrwZLMoVGr0rY1Eh0Y5mJnDHk+is1gwWyyYe7vI5/PUymUIShXO8jL01ZUIVitqiwV5Jov+UCvBGwMoRTXZAjfmkhKyO3uomuooj8WQq5ToWxrJ5/O45pYIzi0gZHOkUylMvZ2oTQZS/gDatkbCS6t4mutRmEzIE0nyVhOKTBZPbQ2Cw4JGLRJLpzHsH6DtakWQyUhcuk6x3Y6ypgrHyhqO2ho0NRUcXL9LYXUVOGzozCY0nS0kbw3R8e9/Gf97t7CdPoJ3fp7A1jbJeJzw1hZqUYOuphJkcqwlRRg6mtm9dI3SpgaySiW1zzxNZmUDQ3c7zr19kkoF7u52XKkUCqD73Fl29vao72ijuqGB1Le+hVKno6qpnpGBQc6ePEltXR0vfONrmHQK9HotfaUFrG+H8RRYOdzdxMqqF43exu1RL/u+KO/e2aSmqoiurh7qa4pw2HTcHFnlzLFGZDIZiXgMJVZCoShph47+WjWpdI7XdxTIFEo0opz2aj13FzI4TQKJ0gIqVHoOt7kQdSeRy+REogn+3RefIeAPcay3mtmFTQ58Yc4criAWT1Nc5ORUbyXfjqc42VdFPhNDIQhkUilONSp56UBBqauQUqeSd+7u4j0IMLWs5Hh7Kdu+JN01Wl6/G0WrUnC2Tc81ZSHReIIjjRruTebpbHDRUaXn5RspPn2mmNcGQ2TSCcbn9znX7aC53Mgrg2GsJjfH6pUsbznoqDLhMArIpAq0ah5Ui+WKCMWyPNymwR9y4jbkyOYy1JTb6a7RoZRn2dk1UmSTo5LluTQcJZ2LIMrNRBMC9ZVu6hxZnMdK2I9kaWvR8/ixKiRBSXe1gqlFJVPLPgosbsLROGNLEWQy2PWnqC6zcLJJZOsgR7nbw3YgR4Hb8RPxIz8t22jvdw74k/flX4zwE6nQ+AnS1dUlDQ8P//OGwPfefovvpIMcXLpO3mrCVFFGfGwKlahB3lBDYmKaXCKB/fQxondHyGQyqGsqIJkiv7OH8fAh0tEo2+9ex9HVRn7DSy6bwXysl8jyGgpACoTIOaykvbuIZhPR7R1shw8RvjOC+Ug3gesDiCYDktWEFIoSj8awtjbiuzWI6Ckk5d0hh4T92GECtwZQmI0YSosIT85BLo/5+GFC80sEJ6YpvfAwoclZDOUlRNe30BW6yaxskIzFyEoSyGXY+7pIxxOsvvIWpT1d6BpqWH3xVdyHuxEL3exfuo7WaETX3YbvzjDh7R1cJw8jeQ/IymUo1SKh9XWysQSuk0cIj04gqRToTGbCa+vIEDD195K8P45MpcLQ0UzCHyS9tIJMJkcA0okk+oZqwtPzmNqaSE3OkUkmUXpcqEUNB/OL6NRqLMf62Ll0HYXHjdZgIBuN4Bsc5eyvfQHRYCDw3h3MTjuyWIJ0JkPtyWPoTUaufOsFDGYTaZ+fTzzzcebv3eD0iT6+88JLxBMJPvz0eW7cHGBtc5fPfuojvPy910HK0dTYiP9gg5W1HT7y1BnevHib9c0dfv6T57h24zZ2sxoFKd68fJ/eejNGDXz1xfv0d5TzcLeTwel9Ch0mhmZ93L2/ytljTTx2tITvXd8hJ4h8/hOneefqfQbHVvjd3/oUF6/eo8xj4fbwAp/58GH+6C9f4nB7EbsHMXJ5qK8ppKbMzte/fRutIkuBIY1coWbblyYcS4OUxWNVMbXgZTeUpa3SgD+UZmwpyOFmN0eaLbxy109blRlfMMylIS+/9fEmpjaSuGx6NvdTnGrWMLcR5vLIAW6bjlMtBibXYhQ59UxtpMmmU5xsNXFtKkE6neKxLhOXp1LE42me6rMwux5hfCnAyTYH91bzZLI5nugx8cKNA8glebzXyWvDYUptMvQ6LZuBPHZtBr1WTTKZYHQhwBOH3WRzAn/92jKt5UbmvDHkyPmlx8uY20ywthOnslBNIifiNuUJxOVs7cU41mzi8niUCz0mLt87QOvp4Vd+6w/+Rf5CEIQRSZK6/uWe5gGtjaXSxed+5wPZutu++L/0Xj8qBEH4jiRJHxUEYYLvM+1TkqSWD3KfH1lmIwjC14HzwJ4kSU3v6/4L8AUezLAG+N8lSXrz/Wu/A3weyAG/IUnSO+/rHwH+DJADfydJ0pff15cDzwNW4B7wc5IkpX+Y3yGVThO+NUQyncZ7+RqF4ShZX4B4JEK5SsnK4DDumipiEzOEQkHMLif6QjeL//AdjPU1xC5dQ6fRsLu4gM3tYnFoGFOBk/jla2grK0guriCaTMRW11m9PUhdfy/pZJLxv/57rJXlCHdGyGYzeBcW8VRXsbeyyv7GFgZRjaBRs3t/HLvHw+bkJFqtlnQiwebkFAX+OvybW0jZPKJOi1ImEPRu47o/jUorEhgZR6lWE8hssn13EEtJEXq3k82RMXQaDTlBIBEIEfB6ie/ucbC6hrO8jIR3H5lczurkNGWCgKhQsby+gXF8ltDeHiqtFn15GRpRw9rcIpaJGbQqFVPXb1B/pJ9MPE5wZw+1RmTx7iCexnqUo5MgwOyVG5R3tLI1NYulvBR1MoW5uwPflZuIBS4O9vaITU7jrKsmGgoRzWTJzy+hqihh9Y13qerrJh2JEggEGHrrElq5gu3pGRw2B/lkEkdhIcblFWKRCLFEgvnpaQqKi/gPv/FrfPjxM7z1zlXGp5dwOmyolApu3R3jicfPMjA0xp3BCSQph81mZ2JykQN/kBdfvY5GIzI/v8Sl62PMzW0hZeNUlzuZnl9HJSQpL9Sj02rY8UV57a4CjShy99oa7a012LairG8H+d51BbeG5mlpaePNa4sc+BJEonEuXR8jlZb4i799lYICB9954x6zC9uYzUY8BU5eevU9snk5M0s+FEqRsfH7qNvK+O67oxztqmJkchWtWkGm2MjmfoT6Mgcd1Sa0agUL23FKHUpuTviYmd/BJEJfg5k7kz4Gp/dJ5EWujcxhM6qQ4SYaT7G9F6DIZeaF6zsUusw0iBKzi1vI5DKy2RTr3gipbJ4hi467Yyu47QbeHlWSz0uML/spKbSyuOollswhkEMrKrk9uoHZYkWlVHB5eJMjnRVMzW/gtBk41SxDp5AzseTHoDdgMuhQyQScNh2CLM9eSOLy6B6SoObW+CZmcx1rXj9eg44n+3S0lKp4bSiM1aBgeNZPMJ5nanTqh+kePiAC/Bsoa/5n+Hfvv57/X7nJjzK/+3vgke+j/1NJktrel/8RaBqAZ4DG99f8lSAIcuHBZudfAueABuDj79sC/OH796oGAjwIVD9U1CoV5qM9mGorsRQXY62pRG+14CwpRtvVQt2RPswFbjQN1Rwsr7IzM0/s/hSh/QNkCjnuU0eRlRVR1tON5LRT0dmOwWzBdawfpUJGPBbHu7iETKen9twZlC4H1vZmUrEYJrMJQ3c7rrMncDfUQUUJOouFotoqEuEw1s5WihrryeWylBzpR+0pxHH8KAa7HWt9Hdb6epzlpeh7O0gk4pQcP4KypBCxppKdxSWC/gCGyjLK2luxuFxYew4hGvToDrWRSiRo+NwnMNvtyC0map48j6RWoelsJpfP46mtRuxoIYmEvboKbWsj2UyOeCyOylNAKhKlsKIcTWcrCa1IUU83gt2KSqOlWTI5NwAAIABJREFUuL4OfW8X1b3daAx6xPYmkjIZlaeOoakso6SlEbPNRiISZffmHVbujZGMRNGoVJS1NuM5fRydTocoiqh1WhSCABKoy4ox9rTjamnCUlKE+6FjVLS1YvEUUHK0l0Q8jrPIQ/vRfkx6PQ11tXz8s5+hrr4JmVJL+6FeRI2BtY09bgzMsLSyRSSaorSsnIaGGpoa6mhprmd3L0h5aRGf/cRjSPksTQ3lPHKingKHCV8ghFIOv/1LpyhwOTgI5/nNT7ai1ep44riHh3udSHIlva2FdLXX4Skq4ENnKunrbqOqspinHu1BqRL53d/+DBIyzp9po6y0mAuP9BCLxfmvv/8FXE47h5o9PPP0Q6TTKR7tLyQZD1NZ6qKjTInDrONIrYr+9iraalz0NzlorXaRyMox6VSMLIT51NkSAik1XbVmPnymFplSi92k4khrAUqNgQs9ZhrK7VSWOOivkRNL5GmvLeSxLj2pdJbJeS8j8z50Wg0uu4nzvW7Ki2wUuS20linpbS3HYTFwrEFDZ6USl9XA4XoDLqeV8iI7Z9tNnGnX01jtwmWEfDZDW2MpR+rUiCo1uUyK9d0Yi1thbEYN3bVapFyCzz1WSSilJiNpsJoMnO9xUeFU8JFTlZh1MmKxOOPzW9yeTXJrLs3QxCpzqz6KnDqUSgXH+3/8ScODAgHlB5J/rfyTCZ+/IknS2j8V/gVD2X5kweb9GQf+D2h+AXhekqSUJEkrPJgu1/2+LEqStPx+1vI8cEF40OToFPDi++uf5UFH0h86wckZVDmJmk98CP/wffQlRYh11cQWlpHUapLhCPlsnqq+HqzlpahKiqg/eQz8IbLRGKn5FZxHexF8QeRaDYbeLmL3JtAUFmA0myisqkQZT2BpbSR/ECA5PU/bF38ehcFA4PYgsclZYtt7pKYXsJ06glKnx3bqKKGRceZv3kFXWY71/dLgvXtjeB45TXRtg+T6Jkqn48HDlmoNjvZm4vMrBK7epuGzH8fqsJNNpFAVuMmKapI7ezgbG4nem0Cn1aKxmIjs7kMkhrGqnPjuPqloHI3VTCKZIBmKoACKzpxg98YART2dOAsLyCXi6ApcyDwFJLe9CPs+nL1d+O9PoS/ykFGrSPuDyDQiOb2OwMQMyryEtaOF7dtDGNqawGrCoFajVqro+MXPotGImK0WMkjEdvewFnkwVJShlMtJbG7T/POfIDByn927I9gOtZPY2WN/YgZPayMFvZ1sTUxTWVtLPBxmc2GR2upqPv75z/MXv/sl6mtqWNvc5fd+/w/58NOP8uu/8jlisQQff+Zp1je8FBcV4LKb6e9pYnl1i872ejpbK7l6c5R0OsVnPnKSV94aRKdVUllexN5BkJICA/F0BpVCiahW8Hifk4sDe1wd3uaLn+jn+sgWCrkCs17Nq1fmOd5XT8B/wOC9WZrqSrFajOwfBPnqNy7y67/wKN955QanjzRgNGgJhxO8/t4kJ/pqaWuu4g++cpmaQgXHO5382QtzfO7RcsZWkxjUOc52Wrg2GSaby9NbIzK6nCKQVFFsFwlEMtyeSdBRqaGmQOCd4QM8FiWpZJJQLIPRIBKNxfnunQAPtxswa/Ns7EZprXHRVOUA5Jw95OZog4G3hgPYLSIfO2rjzaEgelHg6cMWXh8Mcns2SUe1kW9e2uJMm4Gn+8y8PhTinWEfF3psTK2GMRvUJBNJ5jajHG934XI66KixsRcT+e2P1zK5lkGuUOEwKchnoiDI6atVM76aZNUn0F1rZGUnTVN1IT93toxkJsv2fpT/9MkmKjxG5jdjVBQYsJqNPwoX8c8iCPIPJP8GOPN9dOc+6OKfRIHArwmC8GlgGPgPkiQFAA9w95/YbL6vA9j4n/Q9gA0ISpKU/T72PzTWN9bYHR6jqKGO0M1BtuZmsXgKyO3sMnNnkJqPXECu17DxypuUf/QCBrmcvYtXySuVSFo141/7JtbSYpRzi6wMjWBra0Tr8xFPxvB+8wXsrY1Mv/MeNcf6iY9NsTY5hUwux2Exgi+Eqa+LvSs32JyZo/n4MfzX77I5v0AylcRkMpHLpIlvexH8QdSiiqWrN7EY9KjlcvZWVjA77SyPjKEQVbhyOVZHxzG6HGhnloikkuSHRvE8/jAaQWDj5dcQy4pYvTWIvaQYFEr865vkUinUokg6lWLm2eeo+dhTqE0GFp9/kZK+btKTs+zPz2M06tnZ2kazu0fxk4+hEQQCV2+RzOfIX7vF3soK6VQSnd3G5sXL2DtbUSaSzL57hcaTx0iOzxD3B4hOzCCTyZkcfA97WTGaLS9zl69TcewwKqOBmW+9RMMnP4LFYSNwYwCNWk02myUjKtkaHHuwoZxOU5jNkSstZmdyhtXJaXoPdTP87ntEDnw01dczGAiRjkbp6elmdmYGv2+fyZlVgoEgIyMjlJeXYDTo+T/+y5f5wqeeIJXJ8J0XX0KtyBONlDK/uE4+k4JslLfevc3xw83cvz+DQi4Rj4RY3vSRy+aRKVVoVAK7u/ukJDWPnzNgMyrZ2o8iWnXcHt3B4jjAbNLz3/72JT70xGmWVrdZXdtgf++Af3whSzaTYej+GpPzXkLhMBtb+zz/yl30WiVe7x6RMpHJQIS9gzAD80kGJ9b4rWfqAHi828SXn5vjdLuNu3NBtGqJkUXY2fOTSOW4PadFhoKLA6vYzlTTVaXlW+95KbIpiSVgY8dPgU1LXbGO56966au3kMnLuT3mJY2WbDbD5buL9LUUsrUjMLfmZ8OrZN9nYXc/QjKThRIHK5s+hmwm5O93N74/v4tCqWVp/QBfUERUybmXEvnoUS3z6wH+6rU4h+ps3JlPc3VkheOHqnlzJEo6K2NyYZN81sHwzA6dDR7em1QyMrVKe50btcLA5YElSlxGLo8+OJy/PrzC2SP1xLUHP2wX8QH4t98bTRCEL/Igg6kQBGH8n1wy8GC+zQfixx1svgJ8iQeHTF/iwROpP8+DMrr/GYnvn3lJP8D++yIIwi8CvwhQUlLygT9sSXEplgI3Yks9clGkKpkCkwHzoTbsW9sEp2YfzJLf2cU+NQvA/tY2aq2G4nMPoQjH0HgKyMhl6J12ZMkUKoWScChCKh5HabdRUFWJ6LAhVpZRHI2RyWVR6vWENneIvH0ZY20VJSYj8kIntqJCkuEwOqMRSjzU2R5FCgTRdjaTuDWAvawUsbGO4PgUjZ/4GBzso9SoMRW4MfYfojiTQWYyIDebkSJh9lZWsE5MIZPJiez7kBsNuOtr0RuNqBtrKc5mkCxG5HIFBZ0t7P7ZVwktrxHb2QFAX1uJIBMoicVQm42UNtUy9d+fQ3nxMhZPIQtDwzQ++Tg5mUBDZRnx5TWM/T3sfPXv+X/Ye+8gS477zvNTz3vbr733fnraTI+3GAADDwKgAymKkkhJy71d7cWFVnuKO60UkihKK7cUZeghEiBBeDMY73t6ps10T3vv+3X3896/enV/zDCCcXEHjiSQ0ir4iah4ZTIrK19EZVb+8vv7pXJ+EUNJEbVPPIwkSohGPUa7Dcv+HmQKBVU5EZXZhKysmPyaKvQlRaSzWUx5diLOLQIT0/gWFlFotJRaTViaGgjMLJK/pxPX+ctEogkKZCoqerpQOd3UFJcwNzCEUanisy++yOryCroXX2RtbY1gMMDHnn0atUpFQ0MdGo0Wo0HBscN7+A//8be5fuM6u1urqS6zYtAreeFUE9942UVJUREGdY76KiuP7CtCyiZJpVJ8/GQlb191IuYknn24DoAbgwrO31rnxsgOvojI3LKHw4d6cYfkVFZVEQiEefzhg+SZZNRVlZAMb1FiL6C+0kj3rjqa64t5/fRt3G4fRp2cjz/ayMTsBlWlNtob8jl7a5tffbIOpULGyLSc165u0VRlJZeTiEbjXBkPsez0UmzX8VCHlXWPCa1Gw/56JX3TYU70VJFOZ5leDbHq9NJZW0lWkDjeVYxRJ+PKXQ+hSIKywlLadXJiMQdV+QrqijT4Q6X0NJiQy6CmzEYsBfvrlZy7qyOdEWkolIhGzHRXKzFqZbxxS2RPew3ZTJIvPl3L4naGUDjC8KyPkWU9294YmUyOXVU6Frfi7GkuoKVUSXm+gavjQUoKbHzsYD5rrjhH20yE4xk+ebKeda9Id62WZLYWkHG0Vcv18QBfer6ZDb9EUUHeA7/7Hx0frfRZEIRVIMK9ue2sJEndgiDYgFeBSmAV+Pj9j/iPileAM8CXgd/5ifMRSZIe1Hr1s1WjCYJQCbz/Y4HA/9+1++IAJEn68v1r54D/fj/pf5ck6ZH7538s6/gT7okMCiVJygqCsO8n030Y/yw12vlr2E8cJDE9D6kMSbmAqaKU8NgUIKBtb4YdNzhsSL4gMY8PdWkhKkGGa3AEbX4+tv09BPuHQC5gqatB5rATH51ErlSSiMdIxmIU9HahtJjxXLyOKIroDHoymSz2o/sJXLlJTqPC2FBLaGqWbDRG4aMn2Dx7GVkuh2NvD6lkAjGRApcX494uvFf60Br05PKsqFJpMsEQhr09+AeH8c4uUvn8U8SH72I+0EtsdIJsLI6gUJDOpMnlcuQf7EWh1eDtu00ymcbcUIMQipDxBTAf6MF7uQ9RpcCxt5vw5CzBTSdlDx8nPDFDYG2dvOYGZEoVosuD9fBeols7hKYWsNWUE1xcJh1PUPbko/j6BshEY+QdO0hqZp5UNoOpvob45BzZVAr7iUMEr98b+JoP9RIbGMW0r4tg/xCSTofWZiE0v4SyqozUlovutlYiXh/dB/Yzf+Eqn/m1X+X2lasIyRRPP/MM3/rGNwkFgxw5dpSXvv0tvvbVv0KlUvHa628QCoZ48dMv8OZbbyNIKU4d38Ub71zm6P4GVlfWyGUzXO2f4Dc+1cO1ETfxaIRTBwq5PRm4Vx+HnPHFIO2NRcQTCcxmE0a9nJG5GDkxy0MHm3n32hpGg45gOMZ/+NVn+Kuvv00oGOJTz53gzPlbODdX+c1PdmDUq/ndv7xOb3cTOrWCQ92lvHdllp7WIoJRkbmFDR7ba+WHH8zRVmmipVzN195a5vG9+cxtJqgv0XJrNsbDu42cHXLTWe9gfivFqd06bs6lyGWTbPtS7G+1U5mv5Op0Co0sRbFDz9J2FkEGR5vVvD8YIBLPcrjVwMIOxONRjnXYOTsSwaLJ0lZlYGE7izsk8nSviYsTKeLxBA91mPFHUrzfv80vPVLBxfEY8USGR7tMfPfcBqd6S2koUdI3myaayFJgzGI26ZnbylBqEVEqVSy70jzebeLSeIKqvBz+uJwCU5Yb434OtOWx4s4Rimd5qsfI3aUww/MhPn28lIvjUZ7sMXH+boxHO4281e+luethPvFr//WB3v0f8y9Vo+1uq5Euv/UnD5TWVvfxn1rW/c6mW5Ik70+c+1PAL0nSnwiC8DuAVZKkf1pF/wkIgpAPaH58LEnS+oPk+7mObARBKPqJyaZngcn7++8CrwiC8BdAMVAHDHJvBFN3X3nm5J6I4NOSJEmCIFwBnufePM7ngHc+6udNpVL4rveTzIlMffN7lBw5QMbtRS4IeMMRsqEIkUCAYpWKncUlMsk01ppKZEisv32Gut5u/FvbOBx23LcHibk9JAIBtGoN8h03O4tL5ESR4toa1mbnsRYVklp3Ek/EiXi8KBVKlBoNmYvXEaMxfPMuahRKdCoNy+tzmMamiQYCxN1eTHY78sI8Nq72UXz0ALGxqXvBN1dWKG9pwbmySjIeo1KpIrC4jEwhJ7OwjJgVGf3q31Pz+KOIOi2x2QWUJgO+5RWsNisZQK/RsTE6jtVuY2NsAqXJQOzqTQRRxD25iMViRSuTs7S4Qn7JPEqtmpBzC6vDgce5jX1XC9uXbqDXaNi6exc5IrFEkkwozPaVGygkCffaGroRI1vzCyiUajRqNZszs6j1OnQjE0T9fkJeH6lsBlEmI/DeWfIP7iXi3Gb97EWUSiVlJhOhhWXS5RWoVSpe/oMvs3//ARZmZ7ly9hzdnV28+oMf4nG7yaTTaFVqBODc+YskE0nOXbhEvsPOzZu32dpxYdFJrKw6kXI5/uAr3+XhY12sb+7g9Xg4c2OZ67en+eRjzYhijis3Z9nXnsedmQzDUxs48sy01pi5NRUklhD5pWc6cHsj/Lc/e4fnnjqBUqnk9vA0754dJBoKgZghFfPS3VbC8NAgl/rmSKQlXK4d4rFS8q15qJRyLCYDjTWFvPLuCM6tHW5PCswsbGE363H6Ymy5A0w77QiCir9+bYrDPbWMb8LCeoDOOgvVDpFvn9ug0KZFrtAwtrBNgcPK3UUv8VQOm0VPBVn6RpYoK7RwIWtnceOec+f4hh63L8zqpp9kSiSazDK/HKWrzsjAtIdPHy8hFE0zs+ikqdKOTAalDh2eUJor4yE2tiPIhRy3prJ4/DHuLoVIZQyML3h5cl8+DpOKr3+wTjYrIlYU0H93ls7mYm7NylhYdTO7ItDd6GDdr2B+PYjJZOLW2CplBWbO3lWgVunYdG0wvJQmX5/lW2c2+OTRYrZ8CaxGOeeu9P2TO5t/OT8Xp86ngaP3918CrgIfeUUFQXiSez42xYAbqABmuCfs+qn8LKXPP+DeH5AnCMIm8HvAUUEQOrhn8loFfh1AkqQpQRB+BEwDWeBLkiSJ9+/zH4Fz3JM+f1uSpB/rF/8r8ENBEP4QGAW+9VHXQa1W4zh2kNi2CzGRIjizQCIURG+3U/bIcYI3B7EU5GM60EM2lyPu9VFy4hChyVnslVG0u9tp1KgRRRHT3i7cl65jzXeg7e5AkMmwBENorRYSwRA1jz+MTJChrionEwyTX1ZGMBQkuLxKxbOP4RmbxCIXUDXXI9dqkE1Mom6sxREMYnrmCUK3BlELDqJ+P6lYgrxdLaQCAQr0Vej27KZ8dzvrb76LvrOVAjFLWi5DWVlGJidiKy5BbtDivtpPKhKhuKUBcyqNvKIEVZ4d3+0RCmqqUVSVoV5aRi5XUHz0AAmfn1Q4jLajhcCdMVpeeAbR40OWZ0dvt2E51MvKX/8DqlUjZadOEFpcovnjzyBuu+85uhkM5B3ei/9aP4V1NZj2dpFUKkhsbmHoaKU0EiWTzaJsqEEdDJFvMGA/vJ/4hpOZN9/FVF6KrqCAgpoqdHY7qUCQmo42mh46wub4FM+++GmkYJgCnYFcOs0nPvkJAoEAVrMJr9fL3bujfOUrX+bq1Wu88MLHCAYDCAI0N9dz4eIlsKoxGk0k0xnamqp5+ng9L70ZoLO9jif22VmY0zI5vcn6mptIJEwoZOS5I2X4I2ny7SZGZ71cubVMY20Fr55dQqmU09LSyFOPHeH1927Q0d7A3q4GQqEQve0lrG0HCQcD/N5/OsmZG6s8fbyUpVUXJzuNqFVZ/uh/voNKrcbr2WFhaZNSh4GAz09jdT4nWtWcu+PnaEcR9SVy5LIc1pMNBBMSLYVZpm164ok0K640a9tBXjhczKYrwmN7S7CZBI622PmzH87hC0XI01qoryygqkhHoSmHWVdCLCXneJua04NZslmRpw4UEo6l+fp7cfom/ex4QvRPaakuNpDNZplbD5BKJ0mmJdzeIAp5EV96upJrU0kKTRkaKhpYconkGQXWt7yseey4ghl0SgX5RTae6DGSk8pIpiU6qtSMTKfRanV01ejI5nL03VHxcIeeeKoUuUzg5C4d4ViarR0LlfkyFjZybHvCXBjVUGaXseWVOHn02EfdRPx07oereUDyBEH4SbPL1yVJ+vr/K40EnBcEQQL+4f71gh9/xEuStH1/5PGz4A+BvcBFSZJ2C4JwDPjUg2b+hVPnh/D22TN8e24M/GH0ne34rvShUqtAkpCVl6DM5Yh4vCCXY7JZyZmMpFfXELKg291KfHwapVxGNB7HuquF9OwiQlUZoieAqFSgESC2uonaYcPQ3ozv0g1yMgFrbxfhgRHUdivqxlr8V/vJIFH00BFCNwbIFeejUqvBG0CWzaLfs5tkOMrGe2exd3WAx4d5/x4SI+PIqsoQ3T5iO27EdAqVWoV1Xw8KjZr5f3wVR1sz8USSxPomhb1dCFoNK++do/HzL+K/fANDdxu59S12FhZRKVWUPvUoSY+X7KqTdCxGWhSx7Goht+XC0NGCf2iUiHMbdVEBWa+fwmMHSey4Sa1ukIhEKThxiPDCMpkdN45jB1h96zTlJ4+SU6nJbmwh+gPkjHp0RfmIzh00Ha34Lt1Aazahaq4jtbBCIhbD1NxIfHIG2+F9RAdHifj85DfUEQ+FOXL8CMGhMZ777GdYX1pmrn+A4sJC5Ao5WxubfPGLX2B0dJTBgQF+4ze+wGuvv0koGOSxR0/gDwb44INznHrkCAO3BlDKsxzaU8vKmpMCs8To5AaBgB9yGU7uKeTcrS0e6XYwMB9Fq8yhVasJJGWEoimyaPjcJ47z7vlRDu9t5lLfLGqtjkN7W1ndcHPicAdf+9Y72Cxqnn14F++cu8Pi8hpf+FgTi2te/ual6/zvn2qlfyZMVlTwUI+DG5MRQqEozx4s5PXL65Q5NMhzCfJMCtZ9cKRFy6XJJJFokqd7zVybCLG2E+HTx0t4fziCnBy7q9WseAVCwRAnux2cHwlxfJeJwcUssWQGMZvlkU4T16ZTpFJZnt5r4sxoHDGbobvehFGdY3Ijy44/waNdZr7y8iz/+fla+mfitFTomNvOoZGlqC7SsbQdQy3PkRbBG5WRE3OoVEqe6jVzadRHLieSb1YTS8spsuSIpBRolVnCKQWuQJaT7VreHgijUgqYNALNFQaG5wN01WiZ2Uhj0KtpKVVy+k4UQcrw9P58rkwmSCQSZLMip3psvDcY5MkeMx79EZ5+8bf+Se3Fv9iM1l4nXXv/rx8orbni8QcxoxVLkrR1v0O5APxvwLuSJFl+Ik1AkiTrP/eZP6Ts4ftzRGPAbkmScoIgDEqStOdB8v8iXM2HsL66yk7/MPlVlcQGR8kmkng2N9GazUSnpsmrrUGjUbM2egf9vj1Ibh+LN/opqKshNpBClkwhd9jJ29+D68xlTA016PLsBGeXyKSSKAoLWRoZoWH/PuJ3xkknkwTcXgxmMyt3x6nu2k16cBS5UsnG2ASCUoHSbsPXP0T9Zz6Of3EVjcWM+9YwWkkisOlEYzCQkyBy5jz5u3ehzbPhn5pDq1HjCvoJObfQ6rQk4gmCLhf6mkrS0RhhtwedcwvEHMlIhNTENCqthqnvvEJdTw9xX5CcQU90aha5KBHa2SYaCKHLd7Dy7hnKejqJj05CLEHI5cYsisQjEezzy8hkApF4nFQwiHdskmwigX9lFW1pEf6NTRxzyyhLCxH9AWRGPeaOVlZffZuMXIY9EiMVjeJ1blGhULA1M4elwIHSaEBVWcbyG++hzbPjnJxGp9ORkgmc/+o/cOqxx7h+5izJRJJLly+yv2cPoyOjdHV389I/fo9wJMzdu3cpPVOBWqdn5OJZFEKSbCbFyPAABq2CuYVlMokg2XSCshIL//jmIE8fr+HscpiNDQ+ZdAqDVsYff2eIjsZ8EBRsurfJSjIkVGiNZs5dn8NkMvK33z5NUUkxNqWK987d4vC+Nj64MEAykWBwfoZkLEouJ7K4sMTFPiUKWY5MKs3loW2GJp08fqSV0Wkvl67O0NNaxPCUi7VNFxpFASaDmm+dnuPhLgcXh8PcnvahVit4FwmFQsnqVohrM/kEwwm23QGQ7Og1crYDaTQqOU/ssfA376yjVUE4mUOBRJ9eR//oCjXldi6Mq7g5skxpgZlMJkNnrQ53KEc6K3FpLE4uJ3JtIojZZOaDgR2isQTVxSaG57zUllp5stdK32yaJ3tU/N/fncCoUSITJORyNTdHFulsKsYbjOE0GXj+oB5Qc3ksRCwhIuY0lNjg8rCTzz9Wx83ZKDq1DrNByen+efa2l+AKadFqlYxMOMnPszC5sEk6LVFVbOL3vj1Gd0sJF8ciOKp//rHRhI/YjCZJ0tb9X7cgCG9xzz3E9eMpCkEQirhn4vpZEBQEwcC9mGgvC4Lg5p4l6oH4RWfzIZSUlVHQWI+h8140hvSVG5Q3NJDNt5MOhbE21qMqLkS3uo6qphKlzUKTXE48GMS8u5WNc5fxrK5SK0k4Z+dQaNTIIzGWh0bRO2xU9XbSfOQwmVQSXVc7kUs3KG9uRCywYypwYOzehUKnJby0gnHTSf6+HhJrm0TcHiK3hwlsOpFcbiofO0lsbYPWTz5HNhJFV1fF8F/+PdbCInJuH5uzcyhUKnQOG5bqSlTVlShUSurtNuRZkbhOi1qnw15dSdi5ja2qHN2uZgITMziqqzB0tlGjVpErdCALhdF3tJDwB1AbjKSNOhTBIIa2JmQyGfGL16lqbyWVSKI1m1G1NCBXKrCGwogGPfYDvbiu3cJRVYGupor6hx9CSCbJSjB7a4CSxnpSwRDRYIiCxjpsR/YhXrqBIS8PXWcbercH/4aTzPVbRDadRHw+mvZ202I0kAyEKDu8j6W5JVRmM+37esnlcqhyEna9nqaWVjwuF5/+7Gf53ksv8eLnfwW1TKCyqoqB6+f5/KdOcvbSLf7wd3+NmZUwOo0CvUaid3cFmYzIN9e3cfmKKbAZsRj1fOrRWmKJNBNLIcpL8uhtK+D8wDbusIRBp0Gpy+P5p4+jVqtw+RJ4fQG2d5YQsykeP9ZE64FalhdnqKvM54mDDl5+d4KmmkKO7Hbw6rkFHuouwmFWIpfyMWrBpsvx6VONLDrj9DYYiCdLKbHkmHGmEYDKQgNWowpvVMJqMfBIh4EPhkLUlOXxUJuad+JaHBY1exv0rLgzrDk3ODfmwOMNk0qn2d9WyoY7hk5n4FizEqWsGn84ycEGJelUOfFUlse6zay5U0zMO3GY1SjtFv7bi02cH09wpFlFLG4gJ5k51WXk5YvbLGz4eE+QMT63jZQrY1+jDX9cziOdJjY9cVKPSxEcAAAgAElEQVTJAhorzKxviVwd3aQ4T4tMJscfzbKw6uG8PIsoSlSX5bHti7LhDCGRw6IvoqLIyu46GzWFKk4P+qmpcHCoSc2Wx4pCJvBop5F4MkNjuZX2CiXrin+F2Gj3F0/7SG4lCHpAJklS5P7+w8AfcG/O+3PcE079TOav7/M0kAD+C/AiYL5f/gPxCzPah/D22TP87YUzWPZ0EpqcwVRTSeDuJHIErEcPEBqbRIwnUBcVIm5soutsJ7O9g7q8hK0zl8irqUbUqlEhIIajZJMJcmo1hvISgpOzqOurkWeyiLkcarmcrNdPKp4AScJ6ZB/RobsYejoI9w0ilBejkMtJLKxg6unAdfkGtspyMqEIpoN7CN8axnJgD7E748S8XnTtzeTCMbKhCPriAlJrG4hiDuvhvfj6BgjtuKl4+hTe67dIZzOUPHQE35WbqDQasg4baoOe7MoGpv3dRAdHUWg06DpaCM0tkHJ5MRQWoKkuZ/LrL1HQ2IB9bxfB0UnMzfWExqfQ6PRodjURuzOBsq4SyRsgrZCh1mgRndvkivJx3R6i6olHiMwvEXd5yW9ture8tkGPpaaSZCiMQhSRIjESchmCQoFOpSK0vgkyAWtDHfKCPJxnL1G6twddYT7b565QWVmBWqmmvb4e1/omTxw/ztf+x1/wR3/yJ9y6eROb3c7o3VGe/eQn+eFL/0gqHqGzpYJCh47bg2N85oVjfON7F8mzGXj28b38/bff4eFDTUxOThOLJwiGk7TWmojGUux4I3S1FHJtaItHevK4syJxYl8V/+efn+GrX/kt3r84TigS49knjrC6vsVb712hpNDG5z9xmFfeuMaRThszSy7WnX5aq63suAI4LBo2t0LsqdfxZ69M8p+eq+XKRIRAVOQzJ4rwhZJ8+4MVHBYdXfVWGooVDC7EcYdy99VgSfSKNDv+BLvqHLg8QQxaGaJMQ3u5ijf7g+QEAUFMcbjdyvBShse7Tbw/ECArSqjlIpFEhid6HWhUAu8MhtFp1BxuVHJ5MkFTiZxAQo7Ln8RuUtNbr2FmLUIgLiHIlWx74xRaFZTk6bi7HL8nDy/RcGcphUwGJ9sN3JhNk8yIPLXHzNu3/OQQONVp5NxolKYSJa4wRJMiNfkCsYyC9Z0oT/VaeW84BoBemaW7wczV8TAVeXLUGh2ZdIyRxQjPHixmdSfGwJSXzz1SxuRqHKVKjbX2+M/djNa5q0G6cebvHyitoeT4h5YlCEI18Nb9QwXwiiRJfyQIgh34EVAOrAMv/FMkyQ+KIAj/BXhNkqTNf07+X4xsPoREMoWoVREaHEFt1JNMJIiGQ8Q9fjQ6HVqVkpmhOziqq8iKIttvvEv5gV5S4zPszC8i12nR6/XM3p3AVlJMIhhGkgvIMxmQCUy//CNKOzuQy2SszC1gLCwgm8uRjcRQNdaQSqWJnr+K4/Be5BoNU//wXWzlZaQmZ3Etr957SKUS32vv4NjTRWRsitnL16g9uA8pFMF5a4iKQ/tQlxYR9XjJ+QN4RsdRShD3+0iMTqAtK8J3/RahhWXcK6uI2SzFe7vZvDVMzXNPEHO5WZ+aQa3TUiKKyDIZ5i5dpaC2hqJUEkEhRyEIbJy5hLm0iKTbw8bYBPkNdeQWV4mlU6Su3qTyuSfRCwLrb54mf89uNKXFbF28jn9gBLVCgWtuHmtRATq7jcVbA+hkMuSCwOroGCqTicLKCmav3aShtwfXwhIKpQJLXh7pbTeuuUUc+QXkNncQRZHViSlaujo5f+YMskAY0RfA4/Xw5ptvEg4GiUajlFVXsb66hqOwgPdfv8re3bW8+c5ljh5o5Tsvf8Ds3DIyAZKJOIlkht//8+/zO188waXbfgxaJfWVNr76j7fRahTsarCTb5Xxle+O0t5azXtXVwhFknxwdZ6LVwYpKy3g8vURBEFgdWWNfKuWb3zvIiZtirPXPcjkSu6MLmLStZBn1vH6+Sl+6/k6ZDIBmVzBtbEg3kiOuaUtbjqUCAKsO31Ul9rwRCSWBnZorbJQYBa4ORNFIYkEYjCx5MNssWHWaHm7b5XWWgeeiJHJxW0K7UY0GhV/++YC+zpruDSRJJmRWNlwsaupnOWFVUYKbAiChFwQGRpfIZUsxBtKMDoTorO5nGvDqxzrreXCeAq5TM2F/hlOHmgilsxybcTNke5q4okUs8sB1NoqZDKBqcUtbKYaNl0hwpE4V/RqVCo5k/PbXNMoCYSTvL7g5EhXJc5tH1LOxuPdajzeIK/3eXm818H4oo9Lox50ej0Ws563bsyxf3cNkUiGuRUPN6wG5DI5274YN+dFFHINc1MuNNu3efrFn3crInxkoWgkSVoGdv1/nPcBJz6SQj4cE3BOEAQ/91TAr0uS5HrQzL/obD4ErUaNraGOie++QmFTAxaHHVNVJVqVBuP+LnK5HLXpNJJBj6RW4z99FkmnJZtJ0/ap55CicTAbKcpk0VWXY9rYRiYImA/sIZvOEtlxk9feiirfQSYYxbK7jfTqOnG5gmwwTE6QcC0uYSoqIJFIIkoSRadOEBybou0zLxCfnke7q4Xxb34PU3MDtuZ67PMLaCtKkZkMJKMR/Isr5BIJcv4Ai4N3qD64D/PBXjJGPYrSIiIuD/FoDK3RSGlTI9l0GkNJMcsXrxObW0Rut5FXXITGZETX1U5wdpHiXW2YqisRsiJ5rU0Y2lrYeOkHZNNpyh5/mOLmJnR5dtSV5fi2tvCub2C43IdKo8azto6lqAD3nTESsSj2/Xvw9Q9SurcHRVEh4Zk5HNVVaDvbycbjlAogE2Ro2hoxr6yibqihOJVElWdDnmdHrtdRHvCjLspHV1NJ7sYAZQ0NNJ08RjYcoaF9N9FgiN/80peQq1Q0t7Tw51/5U25ev47FYiURT7C7p5d4MsPo+BQOm4aPP7kfkJMVRZ5/vJtYLMFXtlZx+eO4PWFGd3xYLCbSOSVqScDpirG8EcVqt/DLHz/I6StzfOkLL2C2FnH82AHSqRjPPd5DMBQlFlhHIRd4/8IQx/bW8MyJGt48N0dzbSEH6hVcHt7E5Q3RNxlAoVDg9UXY81gFVyeifOm5elbdUGbL8dnHGklk5BxsUvO376bZCXppqS3i3WvT7G4sQKXR0lZXxPF2LdMrQWQygSf3OlAqZKjkdYSjcRyGLEpFPqc6DQTCKfrnFHS3VxMMx6ityGdfvRK5TODi3SQ1FQ6e2mvj9FAAi1HPY10GEslylHKBE+1qrk0EqSu3cqhBwXthFT1tlexvUOMPyOloLudQk5a3bydorLDTXqGArBpPWMuxZhXv3I7Q3ljGqU4Db98WqSiu5UCTiv7xDDs+J2ZDFVuBHMFwjLFVM0s7KSpL7Bxo1DK5EqS+zEpvrZrBmSi/+UwDC64ch5o0TC6qONCgRKtW4PEbqG1t/Pk3IgIg/LtZYuD3gd8XBKEd+ARwTRCETUmSHnqQ/P8+/oWfIdHpBVo/8ymUMjmawgKEYAhjSwOJlXV8/UPo2prwTkyhzom0f/GXkAXDyINR9JXlZP0BMqsbWPfsZrtvAGNnO5LDRnJzG/+NW1S+8BTppVWCE9M4eruIrqyjLymi7NnHyKw7IZmm5tRJ5FotUiJB1SMnSK5uII8nUTvyUFSVE1tcpaRnNwadDt/oJCUPHSOxuEJOzFF78jhanQ5lQT6CIKPmwF40FSX4rtxELeZw9w2gDEcpb28huuFE19qAprmexI6bhkePk/YHiW+7sXa0ktPrSLk8CB4fRUf2o9HrID8PwlHW3jlDy6+8SH73LtLzSxhMRnLRKAqNCqOgoPULv4zabEKyWchvayZr1GMuLKTh4x8jvbqB3mCgYE8n6aVVNGoNjiP7SMwuEh4axdi1i1Q0hmdwlIqnTxGbmEGr0WLb1UpoZp7g7WHKTj1EZHUd7+U+Kh46TFbMMn32EidPnSISiVBdXUV3dzeTd8eQyWRUVlSwu7OLguJiLGYzn//ir3Otb5D/67d/FYPRyLXbs1itFh450UP/8Dw/fKePysoSFHIZe7ob+c9feJQ1p58j+5qw2my0N5WS53Dw9PEGvv/WEDV1DRzZv4sz52+wu72OspIiFpadvP7OFZ460UI4muLEwXbUag0L62HUMgk5aZKpLOGkit//td0EYhItZUoe3lfJxREf9SVaimw6IvEkl8bCdNbo8YZS5HI5WmqLKC6ws69exfF9dWRFgdYKI/saDcyuJ1h0Cfwfn2rk8niMoRk/dYUynthj5dygh5ZSNXcWY1yZjPFYt4lVp4+GMgMPdZgYWU7SNxmhutiIRikjlshgMmipLZAzsRKhulBFtUPi9FAQmVzNiQ4bHwzs0FapJxqLkxVzaPQGEokk18YC7K4x8syBAgYXUgQSSvRqiVA0g0FvJJFI8MFQgKOtBmLxJKFomiO7S/mVxyqIxNJYjWqqSvNoLpVTkm+jo1LP8k6KZbfAZx8uZ3QlTUbQUFlkoCJP4GvvrPD5R0qZXE/xxk0fJ3ebsVstP/2F/8gRuNfMPsj2vwxuYAfwAQ8ss/5fqoY/b9bX1vCurCLKBGxHDhC6OYROr8dUVU58eY3w2ibxsSl8Ticpj5fs7BLzF68Q9HqJD48ze/MWApAYmyYZieIbvEPM5WGnfxC5UU/SHyAQCrEzMkZWyuGankFMp0iOTaPSatmaX0Dm9rF28zauuUViK6tMv/EemZxIbHwaWTDM/IVLmMpLia5toEgkUVvNyMtLWH//HOqyEtRWC3e/8zIqvR5tSRGCy4uoUZKJRO7dXyYjFg6jApQWM7r8PNau9RNad6LRG9gcuoPodCGPJZh4+UekdRoUajWxzW2iswtojUbi4TDRkQlw+wiurbE2M0coEmHj3XMYezoQAL/LzfrVPux5eSxfuIJSpUTYdjFz+gw+5xaB6wPM9d0i5HKRGJ1i9vwlZBYzYiaLrLSQjNePSq+D0kJWJiYI9g2SiERxb2zivXYL/4aT0PYO/rFppm4NknZ5uHXpClcvXCSdShONRiktLeHrf/d3HDp2lHQ6zfe+9U2SqSRvvv46S4vLbG6FMZkdXO6bZPDOJCPjK9wansNi1LC+6eLlt4eIJ3KMzPi4OTjH1NwmOq2O3/7yadLZHGuuLENjayyuuHj1nZvMLSxy8/YokWiMqzdn0KthZtGF3WpkeGQKMZPlGy/3seP201Zt5lvvLdDboEOjUtBWoeIvX51FkCsYntrCHYabc2n0Oh0bOwGuTSWpsEt868wGu6uUVOXBNz9YQyMXmV5xs+ZOIMnkjC5FqC3WoNOocBhFzg/vsOzOcWE8hShJzDoTvHp+FoVczumhEFML26y7kgwt53i/bxl3KEF5npxKh5xXr7vZ36hlV52Fd25uIRckVrwKbo9vMLvqY9GjZGjWSzaV4HCLnu9f2KS2AHJilhtjW9yZDzAw4yOVSqBUyDjZZePsSJB8Y47ldQ+L6x5yUg61LMkHw0G6a1R4g0ncgQhzqx5sFj1feXkKm16ivkzPtDOFxXDPRBVPJFDIFYwtBlnYgU1XkIFFkdcuzBGLJbgyHmBz+2cl0vopCLIH2/6NIwjCbwqCcBW4BOQBX3jQtWzgF2a0D6W0rAytVktyfQMEGcloBNf6OuViDtfyMsbiYswH91CRSqIuyEfXUINtZQ3H7nYkjYbynm6UFWVEl1YorK3CcXgfYjrD9HdfISMIGDVaUCjQ6nWIYga5XIGuqR65Ukn4ej9Fu9pR1VdjS93zj7Hs60aWzqJz2NE11hEYGaf+xDFCiysIqTTeDSca3b31a0I7bnxDo1h2tZJfX4uyrJisJLHQd5u2X/sswaG7NL7wDAoxR2hgmHQoQiIeJ5PNkk6lsHXvJunyYCkrQdlSjzyVwr6zg5jJsH7+Cgmvj6bPfYqcmMWwvon1YC8A2cs3sFdW4Y9GcM+No7itBZ2GXCZNflUFuo4W7AuLKPLzUJUUUrC6hr2tGXlRIfVaNZlIDPOBHoxz86gK83HfGkSVyRH0uDFcv4VndY3CqiosB/eQu9qPvbAQy4Ee9Lc1aBRK6g72Epycoaa5ib0njpOLxHC5XYzcuUMoFGJsZJTy8nJmZmaw2O3se+Qk77/6I3r2HuDQ/g5kMoFwNIJcpuTpxw/x7gfXyGbTJNJKnn/mIY7sa2F6dp36mlJ2tjapqzCztFLKqSNNjE47eeLRfbS3NVNSlEcknKChpoiaqkL++E+vQy5OfVkHmVSI0kILD3UYySSrSSRTTK2EWN8OMrSQhyhG2PGGUKmU5Jtl/PJTrewEshxq1nPlroea8nyO7TJyZjDOji/GwEKGYDjNsjPIkwdKkGva2fGEWNkKc3d2E7PxXoyxHU8Qo1HHkXYTO/44BbZmgpEMCrWWcoeKjmotem0juZzEwSYliWQZq1tB3urbAZmSje0gtxYKyWYzBIIR9GqIJFL8xjONDC8mqLBlKM4zIcnkTG/B0laIPKsBUZLTVFPIk71WAuE0O9NB5te2USjKGZ7epMheT1mRDbVazd2FEJteEafLx+0FA2U2Dc1lMvRaDfWFOWaKLGiVEufveBia9lDoMCFSSFpUMLuySdPxKjzhGI/sKaW9So43kE9LtYOuWjXrsp+Vr+OH8eORzb8LKoDfkiTp7j8n8y/UaB/Cj2Oj+S73YWhvITo+jc5mJa1WodNpSMTiIGaRa3WkNpxIKgWWjjZS0/MkY3Hsxw4QH5tCSmXQ7GoiPj5DOp7A3L2LwO07WA8fID54B01bI+mlVcRcDtIZLPt7iN6+g2FvF77LfShNRlKhMObe3aTXnCTcHnJKJdbyUlTlJURu3yEZiSLLs2HMdxDZcJKRyzEXOAgvrZB3YA+pyVmSQFYU0dqsEIpg7GzDf7UfpVaDqNWiNegQ8u0kF1eRECASRd+zi8TCKskdF3kPHb4Xo0wQMO7tJnj9FkJ5EdGNLQpam4mtrmKqqyU5v0wmnULb0khmeQ3drlbidycgPw+lKJKNx0m6vEgGHZa6GmITM4hiFtvRAyS9PvAGyKXTIIGypoLInTHUGu09B9KWerzj02grStEIMkIra6iNeqp7ughtOolOLfDcl77IfP8A+UYzJWYrMkFGKhZlaWmJfYcOsbO1xYZrh3gsxonHH2N1bILOri6cc/1MTC/wS594lFffuhfM8xMvPMat/puAApfLzedeOMh3f3SVz71wiB++dY1wOMxnnu7ge2/fxWi28eLzR3nt9CipVIpPP/8o333lPT7/yRO88tpZZFKCXQ02+geXUEhRorE0B3cV4LCo+f65dfQ6JSa9ho5qDbdn46RSKUDgqV4LV8e81JfoGF3OoJLF2VVl4uJYGLVS4HCznoHFDI92WXi9z0OJQ8/BViM/vLyNSa/BZFTTXqnhzmKC+hIFwUiWeWeCjx108NYNF3azmkA4Q4ExQ3GejjuLUeLJLB874EAhF3ijP0AinqC7wYQ3Kmfbm2BPrYJAHPwxOSfadazuxHjvtpvffKKCS5MJ7LoscxtRju12sOoW2V2pZHA5y9FWPW/fCmAz65BLCawGBa4Q5JBRZskQycjZ8mWpdsjYCsKJdh1nRuPIZDKSyTRP95q4NJkizyCiUWvY8sSpKtIyshRFjsSjXRZuziY40qzh795b5TMnK1hxpVCq1FiqjvDUz1uN1tEs3bz4/QdKq3N0/ZtcqfMnEQThIFAnSdJ3BEFwAIb7y8L8VP7ddLk/CxKJBL5r/Wj1eka/+RLashLS0SiyaAwh307c72fuzAWSG06knMj25AyxsWmWh+8S9PuRRJGIcxshz0raF2R9bBzX4hK+8UkMne2Eh0eRmY0otBrcy6tsTc2S1qrxDo6QUihwXbrB+vgUoe1t5MX5rLz1AfI8G+l4gu3xSRLrTqK37rA4MEwiGkWIxJg7fY7A+ib2jjYym9vo9XrkKhXJcBR5IoWlpYG5d04jAvG7U3g3nThn5pCCIVb6Blh+430MrY1kPD4UDhtxr5/ZcxeQBEgMjbE2PkXI5SY+NEosEcd58RpFe7uJTEyjVKpJ+gPM9t1EodOR3dom4HIz8fXvkLYY0RU6iCyvEQuGyEo5tkfHyS6v493YxLu5ReruFMK2m7XbQ6yPTbI9O8/y6++isdtYHLxDIhgk6XShKS3GeaWPSDRCyOdjZ3yKwPgUOX+IqMfD6u07KBB446XvMT0xiWtnm9Pvvc/62hqzk5O89uqrzM3M4nZ7+O7//BsePXWKgoICxiYXqasq5fadaS5duU1OglAoyoUrQxw/tJt9e9r45ssX6OmoJpPJMjG9xNa2l/N9y2y7vMzOr3Hm0l2czg3kMiVqtYoXXzjFm6f7sRoVtDeV8Id/dZpoJIxarWN4apuhhSSXxuPs+GOsbkeYWw/yFz+YICPmuHV3hUAgyNRqhLYKPX1TYXRqiTKHni9/bxyyCWaWtvkfr85i0Km5OJ5gxxtmej3I6KaMlCRjftPP4MQmr13Z5HC7mYoiE3eXwtgMMkYXwnj8EW6OrKCVx7m7HGPdHScUF+8tCbCU5vZ8CqUgsez0s7CV5uzNBSKxOGtBNT+4sEgsmeHiRIq5bYlQJM6V6TTLGz76Jrzsa7byjfcW2V2lRKtRkEwmOTPooqtWy6Y7RFqUseOLcH1kmYVVN+tBFT88N4tKqWAjKMMXTvDH35/B5/OyteMn3yxjbDHA8MQaZ2458UUl5CodX3tjEp1Gjdmg5E9/ME2FLcu6O4E3mOD2tI8NV4QLA6tc7x/6V2hFBBAUD7b9G0cQhN/jXpiwHwdEVgIP1pPyCzPah6LVanEcO4j3+i1qHj5OxOtlY2CIlhNHSW1sYW1vRp7KoCkrIbnhpHhXK/rGevKSCbLROLHpeTbnFsjPZjE21eGorkKuUKIpLUHc2mHpRj9l7a1EfD7UGg22khIUWh2zF89SsX8P9oO96MwmxGQSMZ4k5g8QWd1Ao9NT1FCPYU8H6WiMWo0a0mmMvZ2IqRSpdIrk1CzrYxPoHXYESWJrbh6VXk+hzYxSrUbfXI9cpcQRjiAIApZDvZj2dXH3q9/Ad+M2y3dGqdNpSHq8FLe3YawoQ1tXTU0uh5QTMe3vwZDNEv7OK2y8f47A1jaO2hryyroorKlGZbeiqSonnRVJ+PwoZXJiy+t41jfQmIwUnzxKeMeNrmcXeYkE2UwGZWsjCrUKq9eP3m5la3waW201hl0tlPj86O02DG2NOPsHEeQyzCUl5LwBTKVllBw/xPKZS1Tv6aKqvRVrvgNVJodeLufQ8ePIczkCgSDPv/ACiXiCcCrBk599kd/9wm/wza9/A5PJxLUbA8hl+6ivq+LXv/jLrK1vsLGxQzgc5Y33b6BUKrhxewxJkhi8M4dSoaR7VzkdjQVksjkiSXjsoS48Xi/9t+9gt1vIijlOn71OZ0sJVr1AZXkBBzoK2PbEqKwo4ESPA41KRk6uwW5S4wlEsdtMPLq/kEw2SzaTI9+uZWYtxPDUFq11DrxRDYd66nl2v40fXt1Bo9PyUM/98PkqDXaLht5WO8G4QEOtnGwqytC4k/MjPlIZkfE5JzLJQWd9KasuE4UOCyX5Cq7cmeXx3nx2glE6GwtoKlYgkMMfUdLbXMChdjNarQatUqDCnqWp0kFHtZ7yPAVv9CfobKniZLuGXMZEIJohk82SEXO8eWMHvV6LUqliYHwd5DruTm9QerCS+jIDsYwGo17N4WY1qWQNZq1Ab72GgbkscvKoylfybv8WJp2MfU0WqkvtmE06DjaqyeVyjEyb2FunREDBtRGBREokEEliNWnorDNhM6o4N6LGVln2r9SS/Lv5pn8W2A2MwL1oBoIgGB808y/MaB/C22fP8I3h/nsy3tIi3JduIOi06LRa9B0teC5ew37sIOE74yhUSgwdrXguXkel1SCZjWhtVrKb28TDESz7ukhNzGLY23VvCQGVAkt1JRmXB3VTA6m5BWSZLPFoFEt3B4Hbd8ggUXB4P9HVDeQyGZv9AxQc3Icql0NZVkJ6Zp50MIjt2EE8NwYQk0ny9nUTGBwh78h+wgMjpOMJ7Id6iY9MkFEqScQiWGprybncJGMJLJ1tRNY20NmshBdXkLQaTGXFiGtOMokkSGA9ug//4Cja0kKy3gCpdBpjVQXR+UWMDbUERyfR6nQkwmHsxw+Rnpgh4g+gqq5AGYujbWkgOjTG/8Pem0fHeV5nnr+v9n3FvgPEDhAgAZAgCYqLJJLaRduSYyeW7aSTznTP4skfk0zP9LTn9Mx03J1JpuN04liybEmWLGslKW7iIpAgABIEARD7DlQBKABVqH3f65s/KM/45DgOnSi2k9PPOe9B1f3e78U9OAf31vfUvc9NRqOY9rYSnF0g6g+iKSshHYlgqapEUZRP6O59TD37iNwbJxWJoCjIJxWJoaupILlsJxWLkf/4Efx9d0gJUHCgk+j4DFmtmsraGnJrm+x+8gQb1/tRqpQc7TnMyMAgYecOf/CN/4Ht7W0Wl5fx+fzs7erixtAgVrOF4LYLg05DZbGehcUVkukMv/O1L/Kjd85iNmioKtVxY2CS3//6s5w9f5PN7R1+47kD9N+ZYNvlI5uD335xP4s2F6I8j5lFJ/W7KjEadchlErY2HczNL5JnkNBUrWdy1kEwmuP08XLOfGJDLoPHH9nN4Ng6MrmME4cqef/iBJlMhmO79dwc91NZICOOCqUUdgIZkvEoDUUS/AkFemWONAp2FSuYdytJJjO4vBG+9Mxurg7YSaSzkEvxaGch1+5u0rPbwuB9N6c69fSO+dAp0szaghxrtzC/mUUmFTjWqubccIhsVuRzB82kMll+3LvJ8T35zG7mSKbSPLdfz9mhACp5lj21FkYWw3TXKVl1g9MbY1+dmmBCwtJWkuf3G7g65kOvAotRx4ZPJB5Pkkxneb7byKXRMFp5lv2NRnonQ6jlUFWkp6FUxsB8gmxOwBOI8VibhvktyGVTVOrE97cAACAASURBVBVpmFwN0lWrY82dY92T5GC9CldIwL6T5NkuLRfHougVIjWlOoTCI78CGq1VHOx996H2aqwtv9Y02k900ARBGBNFseNTFYM7D1sk8M8m5f5jwOFw4JqYJuX3E52YYW18gnQkwrZtlbULV9HW7SIdj7N2b4ysRkVocgaJUoF9cpqYz09gchZRJiP/8SP4+u8SdHvY+aQflV7H9uQM6R0vEY8P/+AwsrIS5gbukIknSc+voNbpcC+ukJycBY+XmQ/Ooc7PY/nMBfy2NUJ3Rljo7SOdTJMYm0Kj1eBaWiY+OUcyk2XxzffQtDRgPryfras3ca5vQDSOY3iM7NoGtuEx4qEgEqUSS0sjafsGGoWCwq49eO+OERNz2MbG8bt3SIxNoRQEJt98h1Q0itJsYvtGP/FQmOT6Fhq9jtX7E6SlErw3BxElApqyElbOX0aIJ4mPTLA4OEgiHCa9ZEejUuNdtSELR9m6O4Z/c5NMIgFGHRtXe4mQwz4+hRhPoCwuwH9/CgRQtTZhO3MRQ0s9hT3dLL1zFnVDLda2FmbOXcJYUvJAXy4exTG3QN/lKzjsa2ysrfHuu+8xNnafc2fOMjM9Te+1a3xy/hILE5Nsbm9xe3CA9e0gk7M2Jidn+fDsx4givPfeRwyPzrC7qZxX3rgI5Ohq38W//sNv4/FFmF9ew2bf5MKNBUKRFKP3FygqzOfwob0MDE3w4blraDUK5HI1fXdm0KhlrG1HqCnT4QvG2dj2MLXkJRRNMjyxymMHKpBIJJSWmAkGI8ysBth0hTjbv057nYWPB1Y41pHHo/vLePOKjeYKFY1VRlYdQT667eJAeyHj81sc7KhCIZex6fRRXarncyca+f6ZKWpK9ViNSvQ6gR9ft1NXJCGSlLLq8GH3CozMbjC94uLOQpKR6TU8/jDXJ+MMLWVZXvcxtZbg7qQNh8vP9akUcrmckRkXm940yWSSwfkk3XVK9tWq+KB/m6YyNe2Vcu7bkghSFQebLcysx5AIsLLhYscb5O5yCoMyxfJWhBuTQSRSGYOTDuzuNB8N7XB3eosKS44CXZr/+NY8BmWSg00G7i3Hkci05JvV2JwxdpU80Ex79/oC2XSSwcUMNoeXuzNOxlfCrG86f0WR5J9N6fO7giB8FzAJgvB7wHXglYe9+b/SaD8H+Xn5WMvL0LU2IQgC5qlZLHvasMgkTH3vDURAqteRy2URBQn61ib8EzMYiwqxtLeyce0m3s0trIEgQZ8PURSpf+YknolpzKWlqJrrcH6wQDwURmI2sKtzD3KjAc2eVjxjk5irK1E016PVacmzrVPY0U7CtUNhZxuKPCtSASRyGerONrzzS+gLC9Dua0eRSOB85Q1iG5sIEhlBl4vC1mYsB/fRIJMg0WnJq6kiHY/h6r+NSiZnbfQ+BbW1SEYn2VpcorWxnrpDDyrMVB27yaQyVAVDKM0m0GnxOTYpfeQg2rYmPLfuUFBTRf7hbjxDI6zeG0Ofb6V07x5kDTWk43GanjhBLp5A09lOYH4R665qNM31aJeW0VeWk3BsI0tnWL93n12HD9LwlS+CP0A6myHk9bK9tEKDSonHtobOoEehVhN0ewjPLZLQaYmHIzjGJ5FnsvgcWxRq9Xzx61/lozd/RGl+AV/80pcQBIGJqSkO9/Rw9NgxFHI5MpkUmVSGS63i9OnTBENhrCYDbS0VZDMZZibH+MJT+9lwuFmYm2NJyPHEsRYadxXzSFcZOpVILJ7gUFseyVSal384zJNaPVeu3WJp2Y7RqKOkrJKphW1aWlsYuO9hZMpGfr4ZQaYiv7CUXaY8/HEV4UiSO+NuRMDpjmPfCvL0Y41sx3Yor1Lx8dAOqXSWy0NOErEoaq2a925uYtBrkEjl2NZ9XBzYxr7mZnEtxKojyujUKoUFRvzBBA6nn757It5gGVlBw8zyDjqVlEwWHttXRrlVYHddCVIhS32JFF97Nel0hp4GBaF4iu3aPGqK1SSTFpQqHSf3aPhg0MfpI5UkM2kml1yYDVpuzKjJ5qSEIgmuT8YACTfuzNNSX8K1KSnZHAyMLtFaX4ZGpeBwo4rhuSibriBfO1HC1GqQjvoC2qqU5OnV3JvdYWkzRlu1Eb0+iEQi5e5inAWbE4VEgkJRwfi8A41mF46tCI3VBZQXGdhfp8YbMCGKIif3GnGpin75QeSfV1Pn/y0IwgkgBDQA/04UxWsPe//fSaMJgtAFPMKDgTlxHgw8u/6Pob3zy8AvSqO94bSRXllHv6+d2NQcpNLEAeOuSkJj0+SkApZD+/H13cZy9BCx0Qk0XXuIT80iJpKkEkmsjx4mcGsIocCKWq8jumxH1d5MaGoOrUZDIhDEevww8alZooEg1gOdRO5NoO/ZR3RskpRUiq6shLRtHYlSQdTtxXSoi/T0IhmrCQXgt62R/8gB4jOLJP1+lI11SGMJcnoNkkCY+JYTZXMdQjROzL6BSqslJQH9rhqi6xuozSZSbi9xnx/BYiLudFN85AD+8Sms3Z34B+9heaSb2MgkKYmAvqGW2NQcugNdxCdnEMqKESIxYvZ1kpEIeW0tqGoqCd0ZIZNIYj52CM+NAfKOHyY4MIzpkW5CQ6OgVpGLxjAd2of7+i0AFGXFiB4f6UQSiV6Lxmwmq1Uj8QcRMlkiHh+SPDO6PAvh2SXM3XuxJtLEXW72PXoM3/1pDGYTBWYL0nSG4pISUuEIiWQSg8HA2toaqVSKQ4cOMTszw/TUFC995TcZGR0hFg3z+dPP8tfffRkpKY71tLC5scbE1DIvPtXGjburBAIhfuOpVv79n1/g3/w3R5FJJZz5xEa+RUNZWSm+qIzCfDPJrJK1jU3KiswoFTA9u0Qk4OHxnlou9s5QU26korKaJds2sXiG/DwzFl2Wppo8fnhmDKUih1Ypoamhmvsz6wRCKbQaGSf2WTh/c40vHC/jwoCDzqY87kz70ejN6DRy9u0u4frtFZ45VsPN23P4w1m+9FQ9VwfWIJemrMjAos2DY9PNbx4v5MZUjBN79Jy/G0CjlHGsRcH7t/0YdRoeb1NxfjhEThR5fr+ej+4GSKWzNJUpmV6L0rO7gIp8OR/e9iMVcuQZJGg1Gla3IqRSST7fk8/ocgSTTs3qTorOGgXDS4kHT3uCiCQXp7ZMy9B8hFMdZs4PBxByST5/pJhzd0MUGaGyUMO9pRjPdBm4Mx9FFKTEEkkaynT4Q3EkMiV3prZ45mAxs/Yw+xr03F+J0VqlZ3YzzeF6OVcn4+zef+qXT6Pt3S0O9p75uzcCGkvdrzWN9g/F35pyBUH4uiAIYzyoPFADCzzoHD0MXBME4XVBECp+OW7+apBMxIls75BUybG9ew5ZWQmxUBhFMoUoQlYmYWfVTmpuCWWelelXf0ggECA+t8jyrSHczh2UTfV4hkZRFuVjaqonvLCMTCEnt7HJ5tg4SCVodjfiuHgNId9K3pGDBIfHkZr0SCQS4sEwsmgMZb6VaCRCVgTro4fxDdxFlEsx7KoisuVCkhPJZUWWBu+SkkjQFhWSdu2QmltG3bALZWMtm1dvkspmiQeDbC4vo1Eomfvx+3hnF8g4d5jv7QNAKwrEPB6S61uYOttx3xpCW1qMIJPh3NomuLlFFhGxwIr9/XOodzehKyogMLuA3+lia2GZjNtHYnQS+/1JIsEQkbkl5LsqWX3vIzQt9cS9PjZm5/CuOwhFY6ydvYypow1BLsPYWEdaqWB5eAT/sg3CEaRuH1uT06xNz6A1m1i+2kt2ewf5p2MQcvE4aqOBH37z/yKZSBD0+Xj9O99lw76G1WplaWkJ2+oqu3fvJhwOk0mlGBgYwLGxweT4PT65doGzZ85y//44b/7oHXI5gcWlZUYnVnn1h+exmHX0j6xx8cptJGKaq/3zbLs89N7d5OOBDTa2XNwaXqGy1EwgGGFidpMD+9rwuP1c+PgmW9s+PL4o88ub3Jl0gkzOlf5lbA4/Wo2K0YllHj+yh4kFL2+du8/+tiI0ag23Rh3MrobZ3EmwYt9GpZJzY8SNyfBgKm9LjYG/fHuMUDRJJp0ilREozDeyp6mIP/4vVzm0O4/9TQbOXF/GqAGrUcK7lyaYnt/geEc+f312le46FQ53nI2tHWZXNhmYT6FTy5mYszMwn8EbjLFoc9I7k0alUjG9tI07pmR83snSdoqPxyJEY3FmlrfJoeDi4Arb7gCVhRreveUkngLblg/7hofvXVhhb7WSqUUXdUUSelrNTG9k0eu0qFUy9lTLuTe7zcV7gQfKA6ObJJIZ9lTKeP3aOjplmov98zi9CWzuHP6Ego9uLROLp7m/mgCpCrNOzoEGLa98tEg46Gd5O0EmHvoVVaOBKEgfav26QhCEsCAIoZ+xwoIghB72nJ9Ho2mBHlEU43+LA3t4ML75oeZP/1OETK5AW2Al4fbistkp2tzGv+EgnU5RoFEjZHOU1tWi2dNC3B9Au7CIsbYGff0uqkMR0qkUgtuDffAOLUePEB0eJ+TxEg2FKNrfSWFzI7KifEJLNrbm5rAWFZLYdLF6d4TKjnZk96eJuNz4M2mkMvmD1xsOKoFMOoNtdJxdggS5TMqmzYa6pAhzQR6CUoHrxgDOuQWMhfko742TTaeJ+IOUq9WkTUasVZWo97SgHBun4ukTpNMZWs1GIvYNVHtbqVLIkddVsXbhGs75BeqkUjLpFEI2QzaVIrhiI7Hjwbu5hfrWbSTA+vQMHf/t71K0ZCMZDqM91kNxKIRao0VRVYFzcAifw4F6dAKp1Yy5uBityUTSoMV++TqmfCvbs/NIBAHD7iZaUmky6QzqzjZymQz6bSfawnziyRTNLzyPGE+irK/BGg6jtJjJa64nHQyhMeg5/tyz7Gw4aGppZnZ6mouXLtFz8CAfvP8+ly5c4PTp0zx6/Bgff3yRr/3W81gtZoJ+D5b8Ul74wvO8+uprHNjXgdEgp7G+kucfbwLAbl9jV4WeugoDUqETfyjOi080EgpHMFkLOP/JJNf6pnj0+DFe/9F5JmfmOdjZyHNPHuKVN7zs39fOC0+28+o7d9jX1c6TJ48gk0k4e2WCgbEN1rfDBHxuGhpqyUhE9u3r4je/+Civ/biXirJ8Hu0u43/5j+/RUF3MR4MCbU0lHD3SQyQaYWxqhXyrjksKUEgfVN+dvTaLICi5O7lGY00ej3YWUV9uJCXKmVlL4PJFuTbmo7pQSr7VQrFVxaFGNRfuxqkuy+eRRimegIp8k4r9tXJSKQHHton6IhHhUA2Q49QeDYmUnO9djnG4SUUkWUEmm6OyQMpH/R40SgknOqzkCPI7T1XQO+7G5nDRP6fHahLoHV5md10x/bMCuXSanr1VFJrVVOdLiCcMZLNpJjdSzKy4ad9l5YmeejyBJMeaFWRyObLZShBhYn4Dk17NoEpALc2i0yrIMyoxagQyogTzr0qu5p84jSaK4kNXnP08/NdqtJ+Dsx9f5i2vg/DoBHExh760FKUoEt12om1vIbu1Q9jjxbS3lej9KazHeoiNTEJ5MYSjJMMRUok4MrkSlUaFrKKCxPQM2UQSQSJgOXqI0OAwuoNd+PuHUFktCAV55HY8iPEEirpqkks2SGdIRCKYOtoITM1iOdBFeGiUjEKGoaWR4PAYokSKRCrF3N1Bcn6ZRCqJrrSYtC+AZncT7qt9yMqL0FrMZBxOpIKEaC6NvraGtG2DRPABlZdNZwkM3EGh1ZIMR9DvqiLnCxKLRFCVlTx4yqgsJb1sJxUKo26uRwhFiG5soWltYHvwLuXHDpPLZMht75CLJ4ilklj37cU/OIy2Yze5jW10e1qI3BsnHgqTkUlRlxYRtW9gLisl4XRhOdZDbGyKXGEeQihCZG0D66cUnDLPjK69BW/vAGIuh/XRw0QHhjGXFbN7zx6CTheFEgU6uQL/lpOTT5xi/O4w6XSa50+f5p23f4TBYOC5557jnTe/x5deOMH3f3gGvU5DNpPj2OOnuN3fRywWJp0M88i+ajbWHdjWXRztLORy/ypWvUhbUymhSBLHTopwHBBkWC16KqsbmFnY4khPB0ur6ywtrfJ7L53iw496CfgD5MjxzKlDmAxqrvTNYjJoCUREKkr0LNtc+H1+vvZiD+euT1OYb6TQqmFpdZtwOEhXo5H7814SyRSfP9nED89N8ltfOMI754aQy2UoZSKlBQoqCjX84Me3eGJfIQ5PHINWxbQ9wovHirk0HKTILGAxaegbtrG3zsqyI8yRVj3j9ixWTRJRULC66aehTE0qp6KlQsGlkQipTIbTB8z85w9X+K3jJUytxWmv0XJnNkQmkybPKKem1Eg8kWJuI0prlR53IEUkJWPHH8eoU5FJhqksMbPoiHP6oJkL90IUmhUcatRwZijA6W4jNyYCODxxHms34Y1KiMYSqBUSbo67+L2nq0mlM9xbSROOpXh2v4lL93zkciAi4dn9BuzOKBkUeCMC+bosyZwCc82voKlzb5s4cPPiQ+3Vmip+7Wm0v9HUmQfoP7OmTkEQqgVB+DNBED4UBOGjn6x/qNP/FOD1erCfu4yyoozIjofVK5/g3domFIsz++a7ZMgh16iYfPkNVC31CIJALBwmNruAvKSAhNvD1ug4KomErYlpti59TEwU8bl2cMwtELSvk9aq2bh0HcPeNhJuL/HpebQtDcSCIWwfXkSCwHz/IIJEIOPYRlVUwNpHH5PRqlDodUy++iYqo5Goz4/Hvk56cZX5vkFygKKsBEkmi7d3AMuRbqwtTbgG7yEpKiCdTqHIiigtZuKBIIJKxfbtYfyDQ2QSSeb6B9E1N6CoLCMjlYBOw9Q7H5KOxhDsDjan5wjsuMmtOVi4cp1kOkXG4yW0vol/2UZg2cbm/DxbKzbU5SXMvvYjtM0NaKwWUokkm5/cQrGrEnVLAyH7OlJ/iI1794lvOwmnUniHRkmrFESXbEyfu4BCoyE1OcfK6CjEk8SGx4lHIrgdWyQm58iqVSz39uNeXCblD/Le93+A2+lix+/jze//gKeefppAIMB7777LyZMn8fn8XPn4Mh3t9Ww73aytrTM9M4tBr+KP/uh/pbK8kOWVNSqKjFSWFbG5EwVRxKhX0brLwuiMi3yLDgG41jfF4so6d0emGRix4fYGCPg9/PGfvkz9rnJe/Nwpvvmt7yOQxhOIMb+8zf0pG5FYErfHx8q6l8ePH+IvvvseFWX5PP/0Ef7kr87Q0V7P8Ue6+C/fO8fuplK6Oxv51neucuxALRqVnPPXpznQ1UQikUIUs4zen8EfjHCtf4k//strvPRsE/cWwoRjAg1lKg43abgzG0SnkbKvpYDzfXZaq3S8dWWOaDSORikhlshid+doKZdzuMXApbs7KKRZhhZibO74sW14uD6VYNsVYNIhkm9Scmchjlqr5ZHdRoZmvWx5U7gjMoYmt1n3SQmmlKw6/IzPrtNRLScrUbO7QsHTnTo+GvZTYlHgj2S5POrnYL2KS3fdRNJSHE4fC06BvjEHt8ZdOENS/KEY/fMPtNvm1zxsOv2MrCSpKpATCMd4skPHzZk481sCTWVKPMEkNneWIkOGQCjyK4giwj8nbbS/2dSp4DNu6jwLvAqcB3K/qIP/lCGXyJDLZQgGPdrCAoqqKjF0tRNYXiUbDGJoqsczOklJUwPRJTvC5g7ZZBLPlhNlXh4SUaSovg7t3hZUAT8h2xpFTfVIkynyy0uRSqSIqQz+tXUKlopw2+yIgoB4S8LO0jKG0hJ0+9pplUqIeLwPZG2icTwfXcbQVEcqFKayrRVDdwfZeAK5WoW8cRdVkTDh9S0kiSSr9+5T3tpEanqRSCKOc3kVXZ4F29g45S3NSO6OsTW/gL64iNITx1Dqdbg+uUXr8aPE3B4c12+iNBkp6O6k+fFjSJUKVO0tlESiyLUaVG1NKCdnKHikG0EqQ3VnBE2eGVVhAUI6QyoQICMI5LI5Io5Nch4vKqWC1aEZzGYzqVSSVDqNur2FBgSkuRxFuyoYf+1HVHV1oCgppu6Jx1HIlaiaaqnLpEkm4liOHkJ6I0xZQQHqPS1kV9eoqa/n8KmTONfWCM2v8NjJEwz1D/DJhQuc/+gcCrmMG729pJJJRFHk7bff4tFjhzEYzdRUFqORJ+lpVXLlmowV2zper4/rt/xsuUJc6b1LbUUeH1xNI0gk7HgCXO63U1agpKWxAo1GR6Y0RUowcfyRTsrLCllYWmfF5iCZSrPucHHq0f0EIwJlFTWcePxRzp7vJRhOsGpfRalQcPq5U5SVFvHtv36HucVlSkqKuNE/jDcQ4vbIMoX5ZjR6EzP2BEpdPlcu9hJLK1k1qYjHkzTWlvGV59qIJ1J849/9kAt96/TdXeSJI630TkSQSAQu3Zympb6UVCrHtsvDqllG664iVAqBi3e9DI476Ggu5fZiGtuGj2xWRCXL0VmrZidooKFKTVeNhLklI13VAvGkyNmbDorzdUiyerI5aK/RoJRLWXYUU2SE2iIVl+J6GqsL6Jv0suWOck2tJJvJMD67yU6BAREJ/lAEs7aS43vMfDwWoqu1hmMtSiYW4NGuMpTSNA2VFrpqFOQZFUTjRsx6NQfrFdy4H2BzJ8SoPZ+pRRdyucCVcSmjM2tUl5ioyLMiWH9VLM6vfyJ5SPzjNnUKgnBXFMXuf5CLv0b4RWm0H8c8eHsHSecyyJVK8o4cJHRnBGlFKTJBQnbTif5QF+5P+jF3d+AfGkMml5FVKdFVlpNIZZBn02Q2nUiry8m5fZDJkAiGyDveg/vqTXJKBfqmOsStHRL+AJYjB4iNTpKKxzE/coDI3CKauhrC98bJadT4V2wUdu1FlskS2dpGolGj31WDf2qGXCJJ4eNHiG1sIkmkSHr9ZFMpDAe6CA4OPaCnJmdJBUIorRZUzXXEp+ZIRmMYO9qQatRERiaQIpBWyVGp1MS2nKTFHNZD+4mNTiBvqiPncpPx+Eml0xi62h5otnm8WA50Erx9D1lxIUqlkoBrh7TXT8nJY4SGxjAfOYBn8B4Sow61Wk1kzUE6l0VUKslvbyGyZENXUUJ8YQVBKiWbTD2oZOsdQLSY0JcWkxMgY1tHyORIxOPkHe9BOT6LTITnvv4SY++dY29nBzq9ntm793j6qSe5c+cObW1tnDlzhlOnTlFeXs6f/cW3+dLpZ+i7eY2vvXiID871kk0neOKJI1zunQNASoovf+4Qr799nVQixJefaeX+jIMLveP8wdf2cXVwnf3tZSysepheDvD7X3+S3iEHkVia3/nqC7z5zgWa6ytRq1X0D46g02vJ5nJ8+fOnkMmkfO+Ns7Q2VhMIRVlbd6BRyzne08h7Z2/x+PEDLNu26NnfTP/QPMlknKJCMxWleYxNLBOJRHjuVCdrG27iYQ9qpUAknsPnCxDwbFFiVeH1xfCFs5zsNHB20EtnrZaR5ThtNWpEyYMJm0q5hEQiSU+jmg2fyI4/QX2plvmNBMlUktMHrXxw28fz3SauT8ZJJhIcalThDORw+jPUl2lY2s4Sj4fZU6NjJyxlV6HAhifLmjtDOpPhyQ4dI7YcvlACMQfPd+tZ34kTTCpY2YpRZc2wE8iQk2ppKBEIxuV4wjki0QRPdhm5Ph4gnhL54iN53JyO0VQi4PDlcIdEjrcquXI/goIUh5qN9M8n0allFOjTiIKCHX+SqmIdkqJfQVNnxx5xoO/hqoO1hoJfaxrtl9HU+eeCIHxTEISDgiB0/GT9w9z+p4FMJsPO0AhSlRLH1AzpVBr7hxdR11ZjqKogMDGDkGch7g+gqqlk4tU3Cfl8uGwb7MwukJVK0ZcWEZpbRJJnIbK0yuzFq2SiUTK5LFN//QPkxYWoTSbm3nqfoN9P1mJk+e0PkddUomyuw37hKoqSIpBIcG9ts31/ikwuS2huEaEwD6/Xh3NimuDkNEqplB37GomJWaS+IMuf3CDq9SGTShn/q++hslqIrqzh33SiKLAS8/tx3xhEs7vpQRIdvo/n9jCqxlqWhkfxzS2Si8dJJRNszy0QWVsnlsvhu30PTf0ufAEf8UgUpV6PEEug1ulIRaJEwhFsvbcQ/UH0MjnuVTs7/UMklHK8w2OoTQYsrU2EbWuoDHryDu0jtu5AYdBj6WwjNDGLVKnENjHJ9qqNrb7bCCWFBGcXkGo1ZDwPKMOtlVWEwny2z1+l+dhhjNXlfPzKD3j+85+jorKSc2+/w9NPPUlBQQEqlZq33nqLP/iDP2BkZIRXXn2VF7/6Vb71J/8PyZgf546PcCSBSmsmz2ygrsqIzx/AaDTy8usXOXmsmZ7uFvqG15hbdfMvXthH/+gGarWaQquWI/sq2XYF+OTWFIuL80zPLPHDdy6yvrHFK6+9z6p9G4PRyM2+IcqL8+kfmuC7PzjDFz/3GLFkitfe/AC73UZbYynFBWbqa0pYsTtJp3IUFVgQs2mS6RwnjnUxPm0HJHz9yye5NbTI+NQSe5qKaKgpZGbRidcX4omeas72rtBerSEYCvO/fXcMo1bJuhdUCoH3ri0TiKQxqnKs2F20VCj483fnWHNGECQK3r9hY2Pbi04p8t0Ldo606MnmRCJBH6KYRSIIfHx3k6YKNVUFclKJKIJURWWRji1PlKujPqoLFWxsu9ly+UikRRbsHnZXqumskTG5lmJiLUt7lZJHmlTcng1xoNmCRpHhu2fn8URE+oaXCMcS9M8l0ajVzC5tcn7IjTcQZ3Auzt5qJbFYjI+Ggpzao+WxvRa+d3mDA/VKIoksS84HVNrR3Qbm1kLMLz3UVwufPf6Z0Gj8Epo6dwMvAY/y/9No4qfv/1YIgvB94BlgRxTF1k9tFuAdoAqwA18URdEvCIIA/DnwFBADvi6K4tin93wN+LefHvt/iqL4+qf2TuA1HpRlXwK+IX7G1Q6iKJLftYfw1BytX/4CWZcX19wihmUbGbeXHfsaQa8Pc2sTmtIirEVF5HV3Zv5uywAAIABJREFUkFpdJ5fLEbKvE52aZWtxiYayEjQ1lTQa9AgKJbJ0GrXThaaqnPDkLEV1tRQ/coBEIIj9Si/WmQXUOi078wvkFxWSUirRaXWY6utwzM0TcnuQLK6QDoXJKysj//hhnIN3ya+tQV5XjdygpzqVRpBJ0O3bS5HPh9xsAosJ/41bJEIhShobWBgcQm82IZPJyKQzbM/OE9/xojIZKHr8OEqTEcHrp7C6GrlGg0QRwe7YRNl3m3QgTEYUiY5NMnP9JjX796Lx6VGVFmMBFC31+BaWKWpvxVBeRjabZf7MeWq7u4jdGGR7fgmpSklhOEwsGCR2fxpEkYg/gNe1g7WiHLlc/mCCqdONf9uJengMY1MDprw8lAY9kXCI4NoGm/enAJGAY4u7fbfI5nKE/H5u3uxDqVSwvLyC0+niypUrGAwGPv7kE/TFRTS376G1wYpjK8DAnfs01lfz+ttRovEEq6ubVJUXMjw2g9ViRAAuXhthV7mF8UUNH1ya4NTxLi4PbCKV5IhGozza00Q0IVJUrOLF06eYm1/m6icDPPfUUQZuj7C7tRaZXMl3XnmL7n3tXPz4Jl949ij21S6qijVk4j7+8JuXKcw3MTFj48SjPbx7to/VtXU2Nn18lJfPlet3KC/N491zGS5cuUVnSzkffDxNNpthZm4RhUQkl0kTT8S5NrKDVCqlo7GYnkYFaqWMOzNR6qsLOdSg5tJQCCQPpGMsRg37Gy1U5MvJZIpJp1M0V6joHdng9owSpUxgzRVFr0kzupAmFE5wfznEmsdANC1hZW0TpUKBXKlkZMaBVq2gqjQflVLGvcUwdoeHpSITuVyO/pElaivz+ejug5CytOZlYC6PXCbFwd2ltFdI2HYX0b7LyJ5qFX0THmrKLDzSrOfD2y68gTg3DWpWtvyQFbk2ISOTTrHtCXJnIZ+xmU3yrFpuzauQCTmisQSh5ENPMP4M8dmOGBAE4QkexEop8D1RFL/1mR3+d+CX0dQ5D7SJopj6RRwTBOEIEAHe+Klk858AnyiK3xIE4X8GzKIo/pEgCE8B/z0Pkk038OeiKHZ/mpxGgC4eJLhRoPPTBDUMfAMY4kGy+bYoipf/Lr9+URrt+3PjKBBQ1VWzc2MAtVZLMhRBt6+dzPoW6Uz6/9MVMx/uxv7heSoeO0oymUQSSxIPhwmurVH17BMEBoaxHO8hOjJBOp54IOfv8WIqLiJn1CN6/aSBbDyOVK4gveVEWmBFrdESXl3DcuwQyclZsggk02mMTXVEpmZR1tcgbu8gxpPoD3UR6L9LzmpCYzYRnF/CsGc3GdcOCecO5q69JKZmicfiZPVaDJVlRCfnMOxtIzAyjlytIpfJYjxykPjkLEJJEYLPh6S0hNz6BrFAEJkIqqY68PlJptNo8qwkbRskU0m0RQUknG5MRw4QGR4nk0xiOXoQz83bpAQBffmD5k9dWzORu2PEYzGwmIjY1yl79ChStZLA4D3kCgUgounaQ3BwGFlNBblwGEkiTcLjpfDkMaL3JogkE5haG5HNLlO5p5XkxBx/9I3/kR//+B2y2Qxf+9rXWFlZxW63s7Pj4qWXXuKVV1+l69AhtvxeUr4AUd8mpXlKLOoIa84Ej/U0886lCWRSGQa9GqsBUhk4frCBd84OEI2GefxABR9emUav1/K5x+v44MoS6VyOF5/tYcae4OTxLn58dohEMsnRng5cO17mFpb57S8/zvsX7hIJh0imkvzuV55i6N40Zh3MLa6zueXk6L4ybg7ZyGTSVFRU8vRjLbx9foqG2lKMlmJm55dQKaQo5TnW7A4UkjhPHixiZtlNJJxkx58mnYjg9kX4XE8Bw0sJDtUruTGT4ORePZfHoqRSSeRyGW01eiZXo4jZJI+2G7kxGWFfg4E5R5LDjUrO3wsRjYTpbCqgrkhO71ScHBJi0QhP77dyYdhPc5mcJZeITilSX6ZlyRFlZTNEsVXNvno9t+dj9DQquTUVpLzYRGeNkktjUSLRFM8dMNM3FUQuzVFoUuHwi5zco2VwPg6ClFAoxuFWPQNzCY42Kxm3xfGGcsilOfbV6xhZSaGRpykv0LLsCNJWpWXakSOeyiIVcuyu1lOeJ+ej4RB7DvwKmjo79ooD/Tcfaq9WZ/q5v0sQBCmwCJwAHMA94MuiKM7+ff37LCEIwh1RFA/+bdcfJuVOAL9wgbooireAv6ky8Dzw+qevXwdO/5T9DfEBhnjwmFYMnAKuiaLoE0XRD1wDnvj0mkEUxTufPs288VNnfWaIR6Ns37lHYG39QcATwT4xiSjmmHj5daJuN0I0xuKlqwSdTpITMwSdbtK2dTK2DZZ7b5Jyeyh9/ChrF66ia2lAEASCLjeRSAR5LM7W6ASZYBipICHj9iK6PKjUahYuXUVjNKJKZZg+ewFBISO2bGN1ZAxlSwOW7k5C96dQKJSo86xszS2yPr+I+8Ygro0NfJMzKIsKsB7ax/r5j5EXF2I9chD/7WFyKhWSqjI27wwTnV1EpdMx9vIPEKQSVkfvE3S7CS2vkovFSc4uoGqoQ6nXEdvxopLLSWbSRCZmUDfUYt7dTGR2EZlCgXH/Xtxzi6zNzBG+PcLWwgK+7W0iEzNo9Dpck9MPxEbdXtz3J5FVVWDq7sRx6zYVT50gubSC5+ZtDAc6iSYTiFIpUrkMoayY5TMXkSUzLPX1k5NIyCVTZNIpNAolaquF3J5mrr/yGvr8PP765Veoq6vlK1/5Cn/2Z3/G7OwMzzzzNE8++STf/vZfcOjYMYpLSnj/9R8yMT7BzMIa5y9eQaUQ8PlDvHl2hN996VlOHO/Cbl/n8P5mMuk0b7zXR2utmS893c4bZ+/j9QWJxmN88z9fQ6GQYtIp+eDKDCePdzEyNset/kG2t50U5luZW7Sj16kB6Lt1m+XlZQqsOv70r96l/84YtnU373xwDbkM7s/uEIklsNk2uTdyn796vZeSQiMLS2v8hz/5Dl6Pm3AozOLKNreHJ9nxhHB6Isws+dlbqyMYjiIIUp7ab+U751bprlUgk0lQy9JcGXZhUKaYnN9mcyeIVBDJpOLIFGrUShnV+fCf3rrPtjtM31ya+RUX2RxMrUa4Mupjf52SRCyCSqXm/mIAmUzGa5dXUCkVSOVq3ry6zOi8m7JCI0NTmyRTGUpNIh8O7PB0dz7xRJKLQ26q8qCyQMH//oMJfOE0wUiGM7fsHGtVI5FI2PImMKkyPN1toW86hlohYdmZ5kL/ClqNAoNOzV98OM/y2jbhSJyLt9eZXwux5Aizsu7CtuGmwpLl3ryX9/u22V2pIJVOf9Yh4iHwmY6F3g8si6K4+umH/x/zIHb+ukD18y4+DI1WCMwLgnAPSP7EKIric38PZwpFUdz+9P5tQRB+MjqvFNj4qX2OT20/z+74GfafCUEQ/iXwLwEqKh5e9MAXDGCuqSK/Zz+CIOC+3k9pXR2mIwfwrG9QcPwwYjZLuSBBEEW0+/dS4vMjGA2YG2tJxuOEPV6MK+v47GvkFxcRW1hhZ2WVwtZGlK3NqCdnUNbXEFhcxjk5gyCXYsxlqD1xHEEuR9PSQG0sjtxkQFpWQjqRxNN/G3NePmImg316mkqlHI3JiN5gwHh4P7LZeZzDE7g+6Uen0xLYdFI8vUBOqcC1akPlD1DY3YHGaCT/+GEiThctuieR5HLUdO2FHCh1OlZuDqIx6lGOTJDLZPGsbyCRSVGqVEQCAfSjZnK5LNlMBtvEFJUqBZqqCnKAtqsdi0xKYtuFrr0Fz9Ao1l1VpJUKxEyG9b5BGrr34wmHUOl0+AbusjE2iaWqHM3YFMTibKzYqFYpiTi2MBTkoe/YTTOQjsXx3RtnbWiEkpYGZHdGyQpQ09BIfXsb5159Da1aBQhsO50EwxGC4TCRcITxuVnyaqtRbxkwlJWwr+cg2xsOchErgZSBm7d7aW6q5bW3L2Ozb2CzrXHusgGFQkFv3z3CgWoGpCJ+vx+pXMuXnj+CcyfAgs3D3bEFVFoTF68OUb+rmH1dezEaDZy72MvKip1kIoRCmqGs2EhddQndu4tZXV7C4/Fz4MVO5mYqKc9XsK/ZytsX3XS2VXGk3cy//c4we1tK+dzJRuKxCJUlBo4frGN4fAW9oh6PL8r/8Zc3Ob6/jmv3fMwtbqFWSdEpi3F6wtxZTCKTychK1PRPbPDUwXIO7q0EQcKGK8jiRhCVPIhGUUyJUUZHUzmNZSqs+hTKjnIS6Rz2zQALqwEkcg2JjMDi2ga/eaKatl0y5lY0HG1WkExlWbDp6Kw3Yt/J0FRTjCuYw5dU4XA5+GQqgUKh4taEjWyukMc78lipL2PPLj0NZUpG511cGvah0Wq5N2GHXCWemJylDTdkM3ztZDn5Fj0dVVL0ainb3lIsegWHGlXcnvGwvpOhqVJPMJYjlpKg12lADDC+6CbPpEAje+hm988WD/99TJ4gCD9Nu7wsiuLLP/X+Z8XDX6firZ9Lkz3MX+GbPCh5+w/An/7U+iwh/Ayb+Pew/0yIoviyKIpdoih25efnP7RTpSWlCLkcmWiMRCiCutBK1qjHcb0Pa0cbccc23ltD6LraEc1Gktsu9KVFRJ0u4l4fusICrI31xMhR+9RJ4uEI+s526g/sR6UzsHntBtXPP0liaRVtWSlV3Z1U7dmDXirHuqeVZDJJZMWOLM9C3OcnODRC+3/3u8gFKcqWejRtzShUajStjRitFuKZNJlEAsEbpLCmgvzjj5BSa6jq6UZeUkgim6H+C89hLshHotUgUchJRWOk5pbRtzaSCUWQyeXIqsognqCytQljaQmK2irSEqh96nHK23djKiygrGsv0tIitPv3glRKXnkp2uZ6FJEY5U+fIjI1j0qQoC4uIBUMoQTKTh5H4gsgSCQU1deh3tOCQq7AVFKMtrme2uOH0eh0KNubkKvVlLQ2kTPoMeXnYS4qwn1nGE1TPViMmBp20XRwH6VVFTQ9dwqzRMbpb/wr+i99zB/9T3+IRCrj6NEjHD1+nJbdrXR1dyM3GnjpX/8+CpUau22Vni+9wOLqKla9gRd+4+sEQ2le/MLzVFdV8LsvPU1tTQU/+Mt/w9ZOhPoqM1994TBGg4YXTzXS3VHPo4fbOP/JNBqNGq1GS2fHHvbvbaKmqpC7Y0ucfvZxEokUbS31dLbXs6e9mb27K6ksK8TjDbKwskXH7kp2VZXwycAszxzbxYYzjMsbxaKVEo+nmFz08o2vdjO75GRk3M7R/TXYN9xkszkWl52cPtGCUqmiq60Gk1ZKT5Oc7rYyGiot1JcoePJwHYl0jsONCqS5OLXlFrzRHAfrVUQjUdqq9NRV5FGYZ+ZgvZJ4KsO+Oj3+mJThlQwHGx6IajZVaqkoNrJ/lwS5kKOpOh+VQkrvuI/ffKyUCVuUy6NhfvuJUuY3YtSWajl9yEowLuHRFgVlRUZKrXL2V8MzR2oxGg2EYynaarTY3Vl2/HGePFhGRZEepTTNv3qhDQTo3iWlsaqA5roygtEMT3cXMrWR4fy9IM/sM6CQZvCEcyRyOvJMKlQKKTKVDoNBx44/S2VpHv/+X7QRzygoyrc+9P/+Z4ms+HAL8PwkTn26Xv4bR/1Cce/XDT9PG00AEEWx72etn97zC8D1KQXGpz93PrU7gJ+ebFQGbP0d9rKfYf/MYT3cTWhknPDwfTTNjZgaavEtLmOoqcI7OoGhugKZUoGppYHAxCw5uQJZVTmT33uDbDaDUibD1ttHbseLRCFn6Z0P2JifR57LEnJskbZtEHHuEJ9ZQNfRRiwURFApcI1PkfT5mX73DNlAEKVSiWtlFU/fHdStDfgH7hKZnqf6hedYeftDFLXV5B3ax8q759DsbiQlleIZn0Ipl2Pp7mSt9xYSlRJ5ngVlcy0bF69R87mncd7oR7urEkEQyJj0xCNRDFXl2PsG2LavIwGmX/8RyUgEeWE+Ca8XRWE+5o42gnOL7NwextTaiHH/XnzDY0itZtJuD4t9fSSjUVKJJIvvnCEugfj0AgvXb5JKpzB1thGbnEWj05LTa9i8fgvBYkJRUsjCm+/hdWwiFwUWz11EqteRFHOokCLXa8lrayG6sIwxPw+pUc/8uctIs/8ve+8dJNd5nXn/buecJvTEnpwjZjDAAIMMEgRJMEmicqIke3dtr6t2HWrt9WdXfbZkeT9bsqT1mrIZRJGUmMQAEiBymMEAM4NJwOScu2e6ezrndL8/Bt7P9VWJgndlayX7qbp1u++9771v/3HP6XPe5zwnw8SZi/i3nExNTtLe0cFLL71Ebl4eObm5/Nk3vwkKGUtz81w6e4bhkREWBoa4MzXB6OQ4l2708Orbl5lfXCWVyvDiq2c4sr+eLZcXjVbDn337bURRRCGX8P3XhznUlkciJfLFj+3lcs8kr/7kGtF4klAkwfMvn2F8colbA2P03hriuR+8gdcfIBZN8hfffZOpmQV8gRBvnb5OSb6e8kI988sOsswaDrRZ+ZNvXyDfLBKNBLH7oLzISEOJjFff6Wbw7iLlBSr++P95E4Mmw6WbywSCYVbWXQzPOPnr16dpLJJwos3E+RE/BpVIgUFkci1OOCkjV59hxe5jdtVLUZbIt9+a5UiDipO7NFyfiLLkEsik43zQPY1WlkAURSLRKJ6wjM8fL+D6eBStXseje7PpnoggUWiRy5W8cWkOi1FNz1SSOzMO5tf9ROMp4vE45wecPLnfSjgc5aWLdvbVaIlEE1y+E6CuWI1Zk+Rsv4s9NUbevDzD4kYQly9Baa6C77+/hEJIIMtEuTUdpKpQw7XBFRxOH2dvb+P0xfnbd6aJRwM0FCv567fm0SuS3BxdoG/KxdK6h4tDW0RCPsam5v85TMRHQgQyiPe13Qd+mj38pcBHpdGuCoLwE+A9URT/p/6ZIAgKdsQ4vwRcZYcRdr84fW/cN+/t3/tHx39LEITX2AkL/ffSbOeBbwiCYL533QngD0RR9NwTgesE+oEvAt/7J8zjvhCLxohMTO2ssfh8SBUygrE4EqmMhGML59ISCo0agz+IKIo4l5fQx6JktzZhLshHZStGmWNBNzCIfnczco0GMZlCbTGjqKukTKtBtJiYO32GvIoy0td62ZyZRyKTUvGxU6haGslEYsgK8git28kpKyHnaBfR5TUEmYyt6Vk0Gg3erS1UNwdQajX4N52Ex6bQyuUsDg5TtWc30dk5EskUvvWdWhilXEksHCI5Pot7YYWcggIibi9aYH5xCe2wBY3ZhLm4CP3uVoricUSFgu2rvSzeHqamax/hgVHUeh3TvbdQC1KkMhnz3b1U7G4n6nJh270bXUkRsrwcNgZHUOZZSYYjlD94FJVahRgMM3fzFrnlFfg2t1CZDGRkMrTVFVg3XahyslHVVqCbnUVZYMXffZOQVIpOp0VAwLu6Ttrrp7C0hMDEDF/4/d9FpdEQsjvY07Wfvlt9vH/2LHv8fnYfOogxN4ea44eRyeUsOOwYgMLD+/B5PNTayjjxxOOkwwFys/TodBr+7u+eJ9tiJDfLwKeeOsb09CyN1bm8eWYN+6aXS30ORqYcyBR62tubWXXG+fdf/RwSiYS/f+k0er2eUycP4nT7iEZCfOWLH0cikfCX34myuzGPqbkNGusr6OmbIikqWVxc5exVFdl6qCrNZXTGiy+cxrMeAAFkYhS9Ssq+ej3eQIyN9TVKjlqx5StYXxOpryrkyQNWfu9b11hxJVjcSrG27sK9raC5toAzFyc51lnJqjuJUafCatGSSGcIR5KcH/SQbzVzd8aORCqhprCYzpZS4qkMvdMxFja8ZJIJoID1LR/RWBxBKCESizM+u4ZWYsWWZ6K2QEIyGedEpw2VSsWSK4NErubK7SUEpQGlQsHyhod3b+kx6HUsrNk5P6JHzAgs2f30TOXQ0VBEllFNZ62WVCrD1KqP+XU/5TYrcysbXJu0kG3WcnhXHo02FYlUmmRGisWsxBdOEI4mqC7S4ImWoZILHG9SE4qmGF8KcHd94+dtIu4LP0eO7G2gShCEMmAD+DTw2Z/b3f/38ZGtUH8qG00QBBXwFeBzQBngY2cBSApcAP5GFMXRn3pjQfgxcATIBrbYSce9C7wB2NgR8Hz6nuMQgP8OnGSH+vyMKIqD9+7zFeAP793266Iovnjv+G7+P+rzh8B/vB/q8z9dG22N6PgMQo4FeTxJyOlCu6uVyMQE6twcYptODA21pNIpxC03YjROJBHH3NZMdGwKSWkxaa8PSSpNMplCV5hHbH6ZWDyOVK9FrVYT2fYgyBXoayuIzywiKSsiubhKxqhHm20hZd/RGJPXV5G2b6Gtr8Z5/ipKg4FYOIJxbxvR0THMBzsJjIwTc7oQLWYSG5sUPfEQ20N30JeVEJuaxXhgL967k/jnlzDWVxJZ3yK7oRZlfi7u3gGiPj/5xw4SG5smmkyhr60kvbBMJplE3lSH7/YIKo0a077dOzVIWg0ajYbwhoNoOkVucyORsSnMR/bj7+knnk5hamsmNruAGE9g7NqD+3IPlqNd+Hr6kFssiIEgiXiMrKMHcF/rxbynnfjEDMFohIxUQv6uZhLjMyiK8ki7PFgP7CXWN0xOdhZxf5AnPv4JPvzx6yhkMr74lWe49uE59uzfT29/H/FYFJfHS93RQziWlwmHI8jzsggHgogiiA4XaiDfks3h9nqudfdAJsne9jp6btzii588xstvXMDn3uJTj9Tx5rlxMukkWo2K2iobmz4BrVpOXmEFPn+I3GwTkbjIxMQsSqWco8eOoFQoOHf+AjlZRmxFOVy8eJWm+mI6mkv44euXkQopBDFOWa6SbL2EmSUPq844MjGGVKmhobaQ8VknD+4rZHg2RFGODKfDQ1qmpqlcx+i0B6UsiShKSCdiBCIChxo0XL4bIRCK0VQswxOTkUyBN5RELknTWWtgYNrH3ho93RNh2io1DC/GQczQVa9naj1BKpmkqkhL35QftVrJ3koF/bNRkskkR5oMXB3zoVVKCITi7K0z0zcbQxAEntpn5vywlz1VeoxaKd96Y45fO1XK9fEARxv1DC2niccTbHnCfPHBQnrG/Ni3wzSU6BAlcoLhBDarluJsOZfvRsiIUJ+fpmciSE1pFr6wiC8Y5clOE+/c9PJoh5Gzgz6kQpq9NXqmHGmkiKTTSWoKNeSZ5bx9y0/7gUd48vP/6b7e/X/A/y4brbWtTbzSff2+rs3SG37ms+4xd/+aHTv8giiKX/9fndvPG4IgjIiiuOunnf+paTRRFGOiKP4PURS7gBLgONAmimKJKIq/9lGO5t74z4iimC+KolwUxSJRFJ8XRXFbFMXjoihW3dt77l0riqL4m6IoVoii2PQPjubeuRdEUay8t734j44PiqLYeG/Mb/28a2wA0uk0W1d6iUgEIisbbC8s41xaRZxbYOnWbdIuDxJBYPGD8yy+dw5JYR6hUBClVIZcqyGWTuIbuoOhppKU14cslUJhzcEXCeNeXEJfXIimsRa5TE7W/t1MvfYTnKurxGcXiQdDLF28RjwaJx2OIGjVqExGku7tHbWC/R0EHJvoS4t3IqbsLBw3biHNsWDY18HipauoqiuIzi2hSKRQZpkRc7OJrdmRBEMojAZweil84BChydmdwlSdlsKHjuK5NUhGr8XS2U50fApBISdMhu2eW+Qe6UJRbsPXextlOoPKYGD83TM77acbaln98BLS0iLsvf1sLi3hXlwmNb/M7NUe0qJIYGwSodDK/KtvoW9rRohGkahVqJvqWf3gAoaKMmRaNeFgEK1cTn7nblYvXsdQW4m5ooxgLMbN//YdLLYiPJtOivMKGBscRCqVMHl3jLOnT+N0uXj37bc5dOJBIpEoKORkWXMJOF24nTv1O7bGehwTU2j1OjIqJUHXJhqNhpnZBebnFtGq1Tx4/DCvv3cDqSCiUEh5+d1hOhqyCYcjjM1s4gnE2bSvcfbSMPW15cwvrtE/PINSISMaj9F3+y79AyN03xhg3e7m9JlLzC2sc3tkkm1vmA+vTrDpCjN4Z55UWmRsOcQ715ZpLtfSWqZk1RnjgTYL127OcHdmA1EE53aI22MudtdZWHcEOdu7wd46A7trczjTs0RLuZ5AJM4HfU7aKxSU5kj58ZVVavLluHwx1ColJ9rMDMwnSEvU6NRSFNIU33ptHIVcilmv4tuvTbBmd5GlTXN52I1EIuDe9vCdt2ZoLBJ4tMNE92QUpVJNjknNrbt2pjcliKLIxPwGZ/q2ONxgoHsyzIe3Pfz7x0q4ORPFrFcTiCS4OjCPczvA/jodH/RtYTRoONGezcvnF8g3S+lqMDEwE2Bw2ktVnpRgKETfXIKPH8xjcNzO4NgymWSQly+uU2CRcG3URTKZYnzBxchKhg+vz7Js97KnUsvt+TiTK0EabGr+6Vn/nw8y97ndD0RRPCuKYvU9u/d/jKO5h4+0wffVqVMUxSTg+LlM55cIDvs6GYlAVkMtCr0O9+AoErkM3d5dlGx7kOVmY6wuJ6NSknB78C+v4VpYRq5SIhUENFIFjrV1LENjzN4aoHLvbrau9BBYWgZBQnRhhdTGJvaZGaQyGYacXHJqq9A11RFYXsPo9SJGosze6qd8z24kg3dZn5hBodeiW1hma3kZg9WK6AuglEiY6b5FPVKSGg25FeVE5hdZmVsgv6kOxiaRAuPvn8O2q5nQ5hbboRASqYRwOMjiC69Sd/wIsfEZlgeHqD14kPjIGFvLK0hlMrKLi1i3OzAOjCACzsVF4tEYJUX5WHKzMe9uIbRmx7u5hTUWJ6ulASGRRGs2oaivJW/djrIwH3VxAevdvcTDEWLjs0z19GBr34U+lcY5O4fJbCbt9hAJhdhe36BEpyW2tYV8y40YDFPb2oTG7qJMb+L1vjcpMVpoP3qUrs59fPcv/oJdBw7w/Pe+Rzye4PWXX2FyfIJILIpMpeLa+2ep3beHhYvXETPyxNPeAAAgAElEQVRpgqsb2INR8grymZqZYWK6AYVSg14tYc3hxusNcHt4Cp0qg8vtB1Lsaiqjoa4CgzPGicO76OmfYmYlwKXrw7i3/bjcbvbtaaK1qZZEPMWTp44D8HfPb2BpquLRB1o4d/4CJw/VIYoiDvsGOZZyTh60MTSxxe2hKW5OmREECVtuPx/etGPWSojFFNyd2ECSyTA5v0a3UUkyEWNpzcUVjYJ4LIrH4+e9my4kEhkj01ukRBmBYIjm2kIG53x4A0kW19wkkwWMz20gk0pQy/Npr9SzsW2lyiqhLE/J7UkdDaVG9DoFLu824ZCAUq2mtjyPcFLKwHyCOzN2NCopuhoTbXV51OZl6AtAe20eTSUa7q5ESSWSTK+50Ol1XBtY5EBbCX6dgrqKfCw6CeOrCUamN0kjZ3s7RXmRhXN9mxiNBpRyOe/dWGRvcyn+UJJNl5v30ilMJj25uRYO1av55qsTNJYb2N9oYW4tQlrM5uQuLfF4OTqVyN3VJIFwhNcvb3GwowqtwvkRb/s/F0Qy/8qU9X8a/q0t9EegqLgE/fICQiqFIAgoE0nkVeVE1x3o83JJOl1QXY4ilSGtUqJQyKl+8AhpfxBNawPOi9ex1dcRV0ipffIU0kwatr3UfOJJopOzGLo6cF3uwVRVgdxWgFUqIe7xAZBZWaf8E48TuTtFZUc7glyGZnczRdEoKURk5SXUJbsIbXswt3WRSWWoiXQhCpD0eil96lFC/SOoGhVoLBaUFaX4bw6S19aCobEWpVwOudlIw1FyD+0n6gsiLytBlEipOtiFRK0kqVJi270LMZEk4vGSV16KtrON4Mo6RQYdkS0XuqICaKwntb4JvgBNz3yO1PIqSp0OnUqNDAFPbx95J46QmJghlEmTlZ2DRatH0VRLrn0DQ3kp0UCQxs9+kuD4FIauDjT+AEqFAgrzyC0tIau6ApOtiJX3L/KF3/tPOGfn+cTTn8S5uUm2NZfTb75FZX0dbpeLqpZmErEYB556nLRMikKrobCmmlOCQDAYorqlGcfiCse+9jVmxieIhSPs2bOXdCbN8aMHmZ6aprGuisvXbvLVzz9K780B9rTXk0klMei1zCwtUlKUR9/QHIG4hr/+89/h9IUhrNYc9uxuJh5PMTmzSHl5Po5NF47NLVobbIRCYc5fGeBIZzUj4ytMzq7w6OFyltc9LNuDzC062d1oo6NKQfcdD+XFWTx+sICzA14Ot2gRZDKSyTDlBRZy1FFCKhkNlYUcrpPzZk+A3/9sA6vbIouOKPuaCqgrVrHkUuAPpzjWms07t3zk55p4sFlNOJqDTCblcIOGDwa2+dShPG4vptAoY+ytzyGWEpiajfK1R8t589oGh1qsuLxhdBoF5flSQtEiBIkETzTJJw9bePumi+piE80lSk4PBHl8jx67O86u+mKqrWk0x6tY2opRkafEHRLIIEMpD1Fdks1DbUYuDPv40oMFnB0KcLJNx5nBAJW2bB7ebeDt3jT7WrNwesM8tc/EmjOKw5fhZFcN7lCGNo0CRzCGUgb+UJw8swynP8WxZjXBSBxFZS71hVJSubk/443/+UMUIf2vx9l8ZOj4SyHI84tE1r7d+AfvEPX5kZiMmKorSG04SEslpE0GNi5dR1pcQCqRxD4wjKwoH5Iptnv6Mbe3EIlGSTi3kWpUzF28RnDbQ9TpxrW9zd2/eYGkXoMi34q95xZpnRahwIpzYBhlUT4SmYyYz49SpwWjnvi6HYVWQ/bBTnz9QwgSCTkPHMJ7vRfn5avILGa8djvbGxvEJ2Zw2tfxbG7hXlrm7rM/QF1eQuHh/fhHxpFbc9GWlbLt9XLn71+i8GgXoaE7BAYG0bU0sNDXj2dqBlVNBclAEHWWBWVVOd67E4ibTjQNNWia61h89ywKWxFJrxe1xYQ6y0xSzBB3uUGlIJNIoNbrkKmULI/eJbnpRNtcTzqVwn9jANuTj5LedCILhFDn52Lcs4vtazdR6fUoq8pZ+ckHtHz24yz1D7E2OMqerv0YzCb6r3XT2NrCp7/8JU6//gaCAA0tLXz/O99h19HDiDIpH77yY5qPHKS2Yzcvf/0vSCWTWHOyOfODV5gZuI1MLiMWj6FTq7DZinjxhReZnpklmUrxX/74v9HX18/t0Wlu9I2x6fQTCkcZndwgEkkRiaX4wVu9yOQKLl8fYnJyEqlMSk1VGX0Do6SSSU49dIi+26OMjo6yp62GY4faeO/MdU4cbuTctRHUsiST807c/gT/4weXaanQ8uhBG8PzESQSOTUFCt7vWaO5REWNzciCI4E7KGFl3c37NzfYVSYnnQjx+rVNWkrkzG2leevyDBajCq1Oz7PvzGBWZ5CLEV69ssUju40I6TA/vr7FY3tMtJTIGV2Oo1Rp0KikBCNJeqdj7CpX88alWQREbs7E2doOEYwkaK/Sc2c5zpkBH7m6JB/emAFR5OZcitHpLWZXffTccWIzJXmrZ4tam5ZoLEHfbJzdlWoebtPxpy9P0FQsJRyJE0mp+PSRAm7NxDBoVShkEuoKZPzJC2PIJSkabSr+/JUpTBr4sGeSaCzGlWEXZp2MqdUIBnUGgzLF9FqYbL2MfXU6Xjq/wq4yJUXmDAPTPvRaFU8fsjIwG2LL6f6F2JCfIxvtFw5BEEoEQXjg3mf1/0/1+QsfNfZnRjaCIPwW8Oq9Cv5/VYjH40TujKHQaBj92xeoeeg4vvFpFvtvo7Nayc63stw/SKPFRN6xA+gG7+C50c/q6BiWogKUcjlb0zPocnORp0vJthWTVVuNrDgf6eoahrwcDLZiSKXxOLYQZTJy8/OZ7xugsqOduMNJ0LXNtsNBQVM982cvIdGoMGzvKEcvXL5GQyKBNicH7/gEuYV5GLdcyORyNK0NGKJhpLEkojULmUJBRi7HefUGK8OjVOu0pOMJcprria3bCW7Yd+qDgkHSUoGs/HwU1hy2um+yPjhKya5mdOk0s9d7qD90gOjwGIJEwLeySsHyGvMDw9R0dRK4OUgmEWf0hR9h69zNWt9tqvbtJTQ8BiL4Np1Ium8x13+bys4OouPTrE5NI5PLkWvUpBMJ5vsHKdm/B61RRzIRZ+7sJfR6PbPnLpN/XGRjZpbtzU1mxsaYGRvD6djE4/MQDocpLivj6vsfIM2ITA0NU5JfgNFiptBq5elTj2M0m8n4g2RZskh7ffi2nCxtrdHZXEh1RT7trfXU1VbyO78zyGc/cYLaqmIWFpY5uK+JnCwjA8Oz/Pjtq3z5y59nct5Hc1MjpbYCevvHmZ2Zo6m2jPLSAk6fOc977+t4492LdLbX8ca7V5HLJfh9Xn7w2jUmJ6apzq+mKl/H2LyPhooc1uweZtaiXOmdZFd1Nj6FhPF5D9lmLauuOGNTayRSaXQqKaXFFiaXvMyuBYhEkpTm5FKRq6Yk30RziQKlTGQwW49SlmFqJUAyBXeWDISjKaYXnAwUWEikZFzrn6E4z8DpqJ6pBScI8KovQJUtm+OtJix6OfMrakqypXx428P0qgetUkZzWSEP7q8jnU5yoEZB/5ie460W0uk0wwtBBiccZFtMLG1sgwhnhyRsOT2o5TKujgWZXfEglUImnU/34CJt9YWc7o9QaBHIzTZwcncO791yoNcqOdRsJC2tIRCM0VmvY9mZZHLegT9opKQgi3c+mOLkgVpmNyW4/RGuTMQRUHB9YJZjnbWcH40iAjf6Rncqu/8FIcKvTBrtnvjmrwMWoIId6vWz7KznI4ri+EeNv580Wh5wWxCEYeAF4Pw/x2L8/4lQKpVo2prYvtGPbXcb2oI8EqkUBfW1aC0WEoKIrbGe+LYPtcdHIhbHfGAP6WQSjdmMrqMVWywK6TSp5XXyThzG1z+M2VaIRaslU1SILJUmKYpUHO5ColYRd21TuLsFdVUpSmsuyYvXkSkU6JsbMCyuoistwtzahOvKDSp2tRKLRtFlZ5FdXERkaQVtfTUh+yYJtwcNEiKIJNbsGFsbkcXjGFobqbPmElm3k9Xegufmbco/+STxqVmEglzMYi7G/Z3EJmcQozHiciXNX/kCgZEx1LsascwtIi3OR2nNxTMwTMnB/QgWE7VdnaBSYtjdQiwQojAYxJCfh62xHrlRj66lgdJ0mkwmg6a9GVsigSonG01tFblOJzKFEk17M+7uW+z69S+SXLMTdbqo+9JnyYxPo6koweoPUtPcRElVFWM3+zl16jFSqRQ+p5vCwgIiyST1jQ3k1tcyP3KHpz79aVLxOG37OolvexkYGMDn8fDYqVNcunSJrpp9LM6MUlXcTDKR5GhXKzd7b+Lz+/jt3/gMF6/0YSvKYXdLJbdH5/nUEweYX93mj//gPzC7sEZ7WxO9t4axFeXR3NKC2WJAqVSyurZBa2MNJ460kE4n2dxyc2BPFeNTy1RXFLC7sYC6ChOrG36UChlb7ihfeqyWS7ddNBVCxJdPTWkWwVCIWFKgvUzOmitGc42VaDyDIGQwqwUKcoyEUmoi8SS7q02c7vfz66dKuXQ3QjgS4zefLKd7Ikp1eSEqWYoyqwKnV8JnT5QRz4BOFuOJw5XYPQke69hRdRYRkEngsT1G3uz18Ui7AaVMJJkWOLU3C6QKJIKEinwVdm8Us0bC1HqSzqZC+ucTNBVLEKQa/uALDUxvpCixGlCoNByslXNDsHC8zUI8LUcQZEgk0FqhQa6oJhyJc7BRT89EkCf2WekZ81CQa0GjDJFIihiUcKjWwLnhII/tMeJssSGRSDhSL2N0Ukdlbpq+2ThPHSghI5XSWCxjy5VDgVlCXZGS0/1RHjq+/xdiR36FmoD9JjuSOf0AoijO/SMVmJ+Jn5lGE0Xxj4AqdhqofRmYEwThG4IgVPwvTfeXCKIo4rrUjb6+mpz9HaxevMpW903kJUVsr65BIIRUpyPryH5C9k3CPh9r731IztGDYDERnZ5HpVQh5mRhn5zGfa8PTqB/CIlOi6m+mvDMPMn5JUytjbjvTqCzFZJ/cD+RmZ0CTtPeNiSFeSy8/g55xw+iTGXw9A9haqxFpdOQc+wgKbUK19Iy6wPDJNMZTA012C9fR15ahKVzNyH7FoaSYqKrG4RHx9E11JDzwCEcZy8hUyqQq1WkwhG0SjVCbharZ84hKchlpreP4KaTqGMTWXUFmxeukdPRSmxuGe/dSbQ52eR07CKyvIpMpyWRSJDwBwj2DVH46AniGw4MBXmkZFLiS6sglxOORtm81kt2117C6w6c129h3LPzG9fOXkRfakNpMSMkkqikMuR6LUlbAXMfXGDf5z9J95WrDF7v5tFPPMXYnbv88PkX+NIzz2AxmZApFDzwyCPM3x5CiMXZc/ggeouZ9197E5vNxsLcPHk5OWRlZWG32/mb7/4l7a21lBTn839/43sMDN0hEnTzwvMvsbpq5+OPH+OHb1xDqRAQMnG+8/dneebzT1JZbmNmbhmJREJ5aSHf/NZzPHTiKAf27eWD8zeQChkefrCDP/url3n0wQ6efvIwf/fSOW4PTfDUyd2cvjxOS00usUSSl9+f4kRHNhKJBI8/ytBMkIc6splYcDK+6EevkrBoDzO3KfJAs5ZKK2xthxAzSX5wdo4Cs8CRBg3DizHMRg0SiUA8GiIQijK3EaX/zjLhoJ/jLUZ6p0IIUhV1Nj3nbi1wdWSTLF2GSqvA6FKMLIOSTDzE5MIm797axqSM89zZVR7ak8e0PcP7A14eaNZSkiVyechFgUmksVTP3EaYjU0P4/PrfP/0Am3lckx6FQ5PArVGh0mV5LkPVzm5S0dJnp45R5ziXDVP7rNwbXiLu1NrCGKaH5xbYmnNzbpfweXBDaKRMMd3mXnl0hrlVikalZyybJH/+ncjlFjS+INhzgx4Odhs5m/eniXfLEevkREMhvnhhXWe2JfDvD1MMJJCq9Wi12r+5W3IfabQfknSaPF/LMgsCIKMf4KCwf2y0URBEDaBTSAFmIG3BEG4KIri7/8TJ/xLg9W1VbY37CTENIJGQyKWIKupHplKTSKRZGtxiayCAhZ/8j7W4mIMudksDd3BencSEgnWFpeJBYPkN9ajMRrJPdhJzOlmfnIaU54VCeDdchKPRJCplPg2t8h3e4m6vbgWl0gmU0gFgW2Hg4jPT3RsioXbQ6hNJqKxGKHVdSzRGBq9HrlOS47VSiwYxDc5w+bSCiZbMUqli0jAv9NG2R/A53YjV6mIxmIE/X5Cfj8KiQT3hoNkNIKlxIZv1U5utYei5gZ0uTmoairYvjvJxtQ05m0PEoWCwF03VXt3E/H4CG5v41xZo6imitG//yHlbS0Ebg0S9nlxLC1RVFPNYt8QSCXkltqwT89h0OmQyeWsTEwiEyCRTGIfm8JstZLedDHZ3UNJcyOyobtIZTKiXj/TZy6QUcp5/8dv8PRnPsV3/+qveOjkSX7y5ptMjI3h9nkxKJXcungZW3Exl15/C7VKRfeH50j7/YwMDRMPh1hdWWFqZob21kZQmKmsrWF/Zxs1JUr277Lxh3+5TmlxLguLK0zPLmO3Kyiz5TEzv8Y7H/SgViuJRqJcu36T9rZWpmfmefmV11CrFAQCPuIRkdPn+vC4XVy60otBpyLLKGN+2U3fyBqbW24+7FnGqFdzq2+cd6U75NeJGQdSCWgkJqx6kWV7mLIiM99/f4aHDzVyfSqJBAWLq24e62wgih6tKknPdJyewTlaa4u4LIosO4LEkhnMWjPVpVlseWJ0T8ZIptLcmd5ApaykNN9CUa6ecDTOrCPFzdE5DuyyUZijxhcTeKorF38owfU7U1y/G2BmxU2+RcPZRAxBkNI7usbjxxpZdEVZtvvIMiqotVmIJKVseEXGN+JEY0km5jdorspDIgicHvCh02q4NrTEA5219ExG0CihtMjCk/ssnO4XyTJqyNbFsOUbqCrU0DebYGHNw8iKFd1mFCEtUmQ1kRRliGKKiaUtokVGasqs7KvVsGgPsrkdYm7Fza35HPRaFV9/ZZyGyny0m78INtqvFEHguiAIfwio77Ua+A12OjjfF+5nzea32an2dwPPAb8nimJSEAQJMAf8yjqbElsJ2SU2jO3NyPU6TCo1EZ8fpclMXnkZMiAYi6FJJNC0NeEavktx1x4kudlI5VKyojFiRgPJRILSpx4lMjaFrNRGxcF9JNxe1M31ZPuDZNRKwokkuXU1SKw5yK3Z5EYiJBNJ9B2tcBtyi4oQs8xUHOwi6Q9g7OwgvulCY81BXVNBrpghGo2QVVKE175J+298jejULOF7VfuCUok6FEJTkIemooT4yDhVn3yC2MgEus529NEoUpkMQa2kcM8u5HotWr2OdDCMTKFAIUDTpz9BaHmVkM9LXmEjglaDurYSQzCEIUskqVFRsm8PurxcVBWlJC73oDAa0bY0khNPkEmlMHW249t0Ii8qIOx2k1VWgr65Hu/4NE1f/gwZpxtZRQkV8RgKtRpdRyve2yOU19dy7JOfYPb2MKLDTV5BAW3tbRzo6qKispJXXn4Zq9VKQWkJ2y4XJWVlnHj4YT54913+/M/+lL6+Pn7ta19lYmKSZ555BqlCiVYlp6Gujueff45f//IjvPyj96gqCXDqoT1Mz62TSiX5ja88yfXeO2REkZMPHGD/vr0UFFh5+70LCBIlpx4+QrZZw9LaFk8/dZKr13qIx2ME/F6OH2qhusyKQa/E7nCi18qprcghFipCrRQ40JrLrT4VR1pM3Bz38tVHKrg5FeBIq4kzAx5aKrPZVSonlbDhC0Q53KDh1nSErz1ehd2bQS6TUFloYGnLS3lxDgqFgo5yGalUPh0VMkaWk+j1BmqL1DsCqlIZvoCJB5o1XJ+AQDTFwXojC1s+fufTjdycjrAVgpO7s5hYjTOzHuF3Pl3P3EYcuVyKKEJHrQmrScLMio+9lXI2PXFM2kJ8YZFEKkVrIaQECbmaOEGdkvKiLNKpGAU5ek7tMXNjMsij+8tQKiXsr1VzdjBNPLGj76vT6sggZcaR4muPlHB9IsrRJi3hWA2BYJQj9WY+HIpRZDVQbpUzvhyloaoIBDjULOfuaoIcnQK9wcTXTpkQZBJkEpH2uiIqCo3k5P0C2Gj86qzZAP8F+CowBvw7dlq7PHe/g++HjZYNfEwUxYdEUXzzXs0Noihm2GmO9iuN7CP78fUNEfF4yei1aFoamH/9J6ib60nGYmikUvIffRBPzy2UqTS6ijLG33qP1bOXUJTZ0Lc2EnN7UGjURENhfAPDaBvrUNZWEp6aQ6rXIi+wstF3G6kg4B6+g+vqDXQdu9DtamT8+VfZdm4x038be28/olJJWqdh+gc/xlhfQ8gfYOXds2ia6sjat5e1Dy6AWoVzcJiFgUECDgfSZIqFy924NxxkolFGXvwRkWCQZCRKRBDZHpvEaCuCeAIFEqz7OvDPLiJIpSRUclyjYyi1OlJyCfFoFMedSZQZcNydYOR7zxFPpYhGIqx096EpLSKwvEbU7UGdbSHnSBeu7psos8xkP3AI55UespsbCC2vEl1epejkcYKj46jlCjTWHKJON/7e22Qd6CQtlxG3b5Gv0qAwGXBvbiL3Bjj55ONcPXOW//y7v8u1a9dYWFigqKiIB0+c4PrlKxQV23DYHQz091NkzcNoNHLhwgWKior4whc+z1tvvYVcIWfbH2B09A4NlbnotGpOHD/A3758g33tNbz7wWWu945wd2KevDwDDmeATzx5jMvX+/B4fEhlchrqq/n+Cz9m394mjuxv5rf+8x/RtbeBUCiKSiHw2IPt3Bxe5K0PBjh5sIonH2zihde7+diJerKMCt67ssSR9iK+/doY+SYpVrOck21GeqdjqNUaHmwzc27QS0W+hsc79LxycY3VzQAWvYoVZxzI8OaNbZrKdNRVFPCJLjPfe3uWlQ03U3aRVUeA6YUNAgkZp3sWGVvycWpPFq9cWKU6T8CkivOtNxeIR4P0TniIxuIsrDpZ8ch49dw0ckmKcCzF9VEHeyrVNJZoODewwZ+9NM5XH7ExvhrlznKSjkoV0WgUrUqORS/jpQ8muTzqxqxTYLPq6Z9w0lmr47WrDrKNGo40m1FJovz3d+ZpKZWTb8jw7g0HTcUy4sk0Rq0ciURCIBTlzryX6gI5D7fp+ZPnR0knY2y5ffzw4iZ7KhWk4gEm5zYYmPJzunuJt7sd7KmQU1Ws585ihEWnyCcPW9nyRrBvun4hNuTnWdT5C4aaHdWCp0VR/AQ7a/jq+x38MyMbURT/+CPOTd3vg34ZEYvHiQyPkkqlmHjxVaoO7MM7Ok4mkcDfO8Da3bsYrFay02lkCiVrU9NUWHMobW1GaTKASoFn8A4Bl4vQnQl863bigSDG0QmEVJqRcxewNTaQbTJiO3wAfUkRzoFhvEurpABBp0VrNpLf0oxSqcZUWYa6rBhZKETiRh/xWIy414NreQXT5AxShZzt5TWMNVXktbcgT2dQ5+ehra9GNjRK4eF9aArykMhkyFQqMh4/aqmM+Ws9VHd0sDYxhSHPSjoWR6FUsDw2TkljA9NXumk4doSML4i+phLd/CLG/buRLK0S776F9egBtq/dwNZQiySRQq3XM/y3L9J47BCx0Ql8Hg+ReBzFlhPvyiryTSclu1pYuj1E7sg49rlFFColyXicgMtF0OVGZzEjTSS5+9rbVFVXkFVi461vfoujRw6zOjvH5uYWb73zDiq9lv/6f/0Rjzz6KK+//ROGhoep9PswGYy8/NxzHDp4EI9nG6PRiMPhYHR0lNnZOVxeD9lWK9/97rd5+qkHef98H+l0BpcnzPX+OWpqaqmtLufg/hacTg8fXBjl5dcvsri0zF98a4bPfeoJtr1+JsYnOH1Gz8rKMlUl2Zz58CKXrw3SUFPMu+dF+m/fQaMUePf8zr/bUMDHhRvLCIhc6R2npdyCViFjZjWAJyIlEksyteCgNF/PmkvLyOQGCEVsBmX8uycqGVpMMDwfwuWLcGNwjlOHa1jZinFnegsxk4ctz4wt38j+ajmhkAJbXgH7a3U4tvNwe4MsOAVmVr0UFliZXQojCCIn9+ShU8sYnfdj0OagkcZ4qLMEtVqJPxjD7Qmy4TZSV2LgRHsO5wYl3JyOcnN0iUe6KumbDjG77ESjVmJQ5bG3uRSrWUFXrZJMJsOVQRUL6yEmFpzotEpmVpJolRJW7T5uTRkwGzTMrLlorzHTP7bCyd25TK8ksGWJvH5lma62CtwGGVUlVnZVGnivJ4hBm8IXjCOVCFSX5vLIXjPhuATIsOUXWXBGGRhbpautjDODfnyBJBvj0//yRkT8lYpsLgMPsNMUE3YczQXgvpgX/1bU+RFQKZVoOlpJ3J3A5PWiKC7CnExhbmlAqdVSTIZ0Iom2tZHA8grG/HyCbjdZTXX4xiYxVpah02jQdLSTlMsoam0ijojUZEIw6qiLduFZ3SC55cLcUk90ag6jXo+lyYJ+Xzuu7puUPvUIvpuDaI168PqR1VXhGxil9nNPk3Z5UKg0FP/2fyA8dAfdriZq0iJR+yZCXRU6kwkxGicwv0TpA0fIuLdxL65i6WzD0z+Mub4K35aTirZdCBUl1Jj0RJzbaBtr8IxNkl1URDQSofbpJ4ivbGBqqcdz9QZZLU34V9cR3B4KHzyMf3oOdXYWaZ0WiURCQi7FWlFKIhJBXV9NWWUZSccmqVAYa1M9gcUVUkY9to7doNVQ0lRPKp7AfKgT4fotcg90Irq9GLtaKYtGKasoJ7DuoLysjE9++jNMjI0xvzDPg6cexb6+gS8WIzsvj/2HDxGLRCnIyWFxcZEvf+nLqFRK5HI5zzzzDNvb23zsYx/D5XKj0mn5zFee4W++/sd07q7FYjJw8Uo/Tzy0C5lUoL2thZXVdTKiSP/QBLtaG/n85z7JO++dY2JyAqVCwO9zU1qcS+euUlSyBP5glKdOtiOmE8hkEnbVWBATZTjdfroadQRCMdGkIigAACAASURBVG4PymmvUHC2187vf7aRV84t8KWTpVwfD3GoTolEoiIRi7Jo91FXIMeg15BKQ02hEq1aRjKT4sEOK75uF48fa+JQvYq3brgpK7RwrFHNdUEgEE6SyWQQZCqkMjkfDno52qRnZUuKRJKmpjSb4iwJsUQ2zcUCE+tpOqvlrPukKJRynMEMJ9uMXBjyUlGl59ThGjyhJNkmNb0zforzTJTnSrC7LOyv06NUSNnYzkUiEWirUBOIgS8YJhCR0zcV4CsP23D4oKOpmLI8Pa3lKs4NevjNj1XjDkmwexIY9Dq8gShCRsQTypBlVLOxHaeiyEJhlpIcXQptYxYOP7TWFxKJpWit1rEZjhKPR0mmMpTk6zGp06RQoFfG+Nrjdcw6Ejzcpue9/jQHug7+i9sQkR2SwK8IVKIo/oOjQRTFkCAI9826+Leizp+B7Rv9CIKUvNZmxn/0BoqqMkw1laTtm6h1OqwPHcVza4DgzALmshIcQ3eIzswjl8oYffZFVAYDeoWChXOX8Dk2SQOByRn8t4Ywd3Viys9Du6sJb98Q05evo26uIxyPEtn2oDYakWs06Dta2ZieJSKIhDYcqAx6VFkW5i7s6JDJFDIyOjVr568gLy7AcrQLT3cfaYWcWDgMmy5UtkIi/iAqtRqZSkX2wU4Ct0dRqXei4NjYFOqaSrIPdeLtH0aaTBOMRNmcmSM6t0zI62foO88SF0WS4TArZy8hLS5Aplax3n0TSVE+MoOOtes3UcgVqPNyMR/aR3ByBnHTSWBheedZBj1Ki4nZd8+g1WmZ+vACSb0WSVE+gclZ5BYz+qICUlo1d559kYxCRv/5i2jlCo5/9lM8/9xzDA8N88jHnuLu8AgXz53n1KeeZnZujpmpacqrKrl69SqPPXaKw4cP4XA4OHfuPG1tbTgcmzz77LN8/GNP8ciJE7zy/Av89n/8LX70VjehUASHY52W+mJeeu08q2sOnnrsBGfO3UQiU5JnzWJ8YgaVWkNlZSVefwCLQUHX3gZef/syJw/XkYgnePmtbh4+XMP2tp8Pr93h2J48PvVwNae717nSv0FjmYk//dsbSMQYS/YAKrmUbKOCB1p03JiKYndHKMjVc6wtjw1vhmO7C9lTa2RsMcjIYoxYLM61O9vsrzeSTAucG/JysMGILUvKu70OqvIlFJjSvHLZTme1ihWHH5NOjjuQIJqS8aMLc9jyzbx8fholEQqz1SQTcZa24sglaa7cmiYaS/Ber4PSHIFrdwMopGlaS+T8/ZkVdpWrSSeTLDpFvvqwjb7ZGBdHvBxqNLCnUkn/bBSFJM3D7UZ6p+MIMhWF2Roc3jTleWoWHWGi8SQKhZyiHDXv31gi1yDwVFc2t+fCfPmRKoIJGQXZKqJpNV99pIRoNMSz782hkmUYndmiJl9CWY5I/4yfPPNOO4VnTy/RUqqgoVSP2xvi0oiLbX+Q7e1tvvHKJMcb/+WZaP+AX6E0WlgQhLZ/+CIIQjsQvd/B/xbZfATWVlfYml3AXF9FVnMTeZXlOPuGsGRnYZ+bR6JQkhuLEXJ7SSTjZOXlkFVRiqowH2VpMVlr6yjLipBazJhn5rDUVpORS3FMzhJyutAYjQDMv/4OhTXVlLY04x+6i0KpYPKHr1N7+ADB3tsEEzHSiSQqQcLEK29Qf/gAW1d7UOoNxO1bhEIRlOkMzrEpsrOySWXS2Gfn0FksxINBFBoN8oERNmfnkauVyFQKAFZH7mIuzGd7bQNjQR7qkXHEZAqv00kmlUZTWICmsJCsg3uRyuX4n30R66H9OK70oDXoiHi9iIkU8UiUwOIqgkJOIhrBPTsPgsDalW7Ucjm+uQXWxidQaTUkvF7kOi25pTa0rQ2YJqeIbbkxGfSMXbpKw7HDBG8NEvD7ScUT5HR2oJbKkFuMDI3fJUyGucHbpKQCs2Pj7Dl8mIHem2izTHzrG9/gxKOPsLSywuTkFNPTMwiChIWFBX7w4ov09NygsqqS693XUalUDPXdJFcvR6ZQ82u//XUOd7Uythjj0OFj6IwmBu/M0jd0l6L8fKoqSvjLbz/LZz/zMYaG7tLb42Pf3lZm55Yw6eX8v+y9Z5Rc13Xn+7uVc1dXV3Xurs650d1AdyM0ciIBkgCjRCpQlmTJM7afn+1Z4zQza8Zp7Pee/TTy2BZljUxREpWYQIAAARA5dETnnGNVh8o5130fGvTS0rIlyNaIzzP61zrr3jr3pPpQZ9+9z3/v/f0LA3T2jpFlVHFBkmBqZpZYNMp3k2GkEimrti1C/gAleRoaq/I41ZrFljfG6Nwm10ZzSKbSTMza6BpJcaC5kEBSzu2hRY7tqeJqnx1rXgazq2tMLjioLs1GJZdyp2+BXIuOZFqCVCplYNpJpslMKq1mbXOdzukoI1MrZKjyycvQUZAppbEih/JcKcsFWaw4onRNRUhL1XzlrQlOH6ygpiKPOquO0mwZq84Yi2srON1yhCoLc6sutBoV4/OblBZkcnUgyfDUOnqdAoNGSUoUuPFggRyjllA8m/6JVcxGLeFIlK7hNT53uoTaAgl/+OoIe5rKuT8dRwDisQg9k2GmFx3kWoyI4ja9+fi+Wi70eknHw+RnGxFIYd/y0DmpxZih4+q9cQ63V+EMCvgCUYaWU0iIEwzFiEYTlOfpSCYlOP0Jrva7yK/9+bPRRESS/+uY0X4TeEMQhA9z6OQBH3/Uzr8QNj8GRcVWLNYijDvq8c3Nk92xm+DIJPq9rag3t1Dq9agba7Go1YT9fgJDYxSdOo7rbjdCOEze/j34hycQrAXkHthNYGIO0/52zLk5mJvqkEplSKWgi2UhLcxBrVUTj0ZIuLwotWoUxQUozCZC93soOn4QuVqNwWxGs7ORyPA4lc8/he/BCOrqciJuLxXHDiJKZeiamykBpAoFiVAImUqNvL4aq1RKUgBZlglZfg4ViSQxjxdBrUKr1aJuacB1u4uyJ04SHJ8mnRbJPLof770eZGVWctqaCS+tkGHKJPfIAdy37pN1aC+ix4c+Q4+o06I/fpjUlotIKoV/ZY3sjz+Nd2qGquICZOEYmW1N+O/3ocky4ZtbJH//HtJONzExTcmBDqSWLNSlxcSu36X+c58gNDOPVqvFK0BFWSnSmipWFxbIa6xnaGGOB+MjtD91Gl84gDLbTPuZJxElUpKpJE88dpqvfvWrHDt2lNOnHker1RKJRHnu2WdJJpN8cPUqT55op7NnmGfPPk5NlRXEFKVVeYyMjbN/Xy1zi6uUl1pxuTzU1FQjl8lRKBTU1zTyiedP8LXX3uFTT+/i/RvDaFVy8rINHGnNwuvJQimDAy3ZKKQC19Mx5HIz4XCcx3cZuNjnIT8jyb/7ZAvd00FeOJSDTJLA4Y7QWKLi6mCQ9h2lpJNxrIUWDu/KI9uo5FuX54hGkzSVa5lasbCjPIOmEhVvd7qpLTWzt0LClYEgVaW5WLQJXj5VRVKUUZKn4Vy3j0+dKOTWWISCvCwSsRA1BXIy9TICwXLSopSqYhPTa1GqCzJYc8R57nApi44UBkWML56tY2Y9SXtDEYJEwpn2DBIpkUQyTVW+FKNWhsObj0kvp71SjiAWgyDhWKOK0bkt5u1B0mmRsgITLRVaDMoUGnkx/qiUx1rlWEx6PME0z+01EgrlQjrJqZ06zvemKM5XMG1P8LufbOTuRJiTTWq8/lK0Sik5hgTNVWaKssBq0fBuT4KnOrS4QgKBhJxff7qMe5NRCvJ+/mw0+F/nzEYUxT5BEGqAarbjoE19SBh7FPzCjPYTkHWkA9/9PmS+ACqLGUVNOQ/+8m9RWsyE/H7Gv/FdUgoZgkbD+vQs69fvkEgkmH77PVKxGIqactZv3EOdk4Nu1w4mv/VdArEYQizOwq17bA5PYCrIZ/Dr32Gt5wFaazGCTEb951/GPzFNYsuJVpCQVVNFbHmNvPadhEYmUcUSKIwZZB3twNvZR3xmYTsWm8+P/U73dhyzlTWUhflEAn58nb1om+vJ3LmDwNIKq+9fQ1VTgX5vG57lVfz+AP1f+lvSGhUSnZaU0UAyEUcik5HOzmLu3Utk1FSzdrcTTWMNUrkUfVsTk69+G0N9FTHbBrHpOdRlVjZWV/AsLlLwxAn83Q8QnB5MjXVElTJGXnkV7a5GkskkadsGqqICFh8MEnK4yOloI7SwTGDVht5ahEqvJ+n1IVcqKdjXyside4xfv4O5qIhv/O1XUBgzCEsEei5cYnVyij2f/xR3L71PTmEBi+vrXLx4kYMHD/L888/z1ltvU1ZWRllZKVNT07z22jf5kz/9Y1777nvk5WXzwjOPcb9njO6BBRob63j26Sf5nT/4Y04/doxAIEhaFPi93/1tfIEwUqmE48eO8nevXURMi/z99+7SUFtKS3MVn32uhXeuLxAOJ3j6cAm3Bpycu7nM8WY90VgSjVqOTCahplDB7WEXxbk6TreZ+Pp7iwTDSYLhONeGgzx/wEI6lQCFjhdPlHJnyEEgkiA/x8Rnnqjk/3p9jAy1yPRKkHNdLg426Dm920LfbAijQU1FrpRpW4ymcgObvjQ3Bz3sq9k2JQUjCVSyNI+3ZnFvKsLQvI/6YgVuj4fp+XVMmjivX7NhMaoozZYRCseZc25HDcjSpIjGktTmS/nBrXWay/S8eMjC7bEQb93bpL1STTAGl/r8HKjTEA6HmVsL8MS+EkyZRtLyDL74VCkj80Eu9TpotKrxByNceeCkuURJWbbAez0OOuqMtJfL+IsfzBAMhrBteMg3azDqZFTnSbg+4KbeqkavjHOh08bT+/OYtqXonPCyq0JNbqaCb1yYIBT04/CEScRj2O2bH8keIj7i518JqoE6oAV4SRCElx+14y80mx+DWDxGbHCMZCrFxtQMqZSIPxQkIy+HrJ07UDucyKUyjDvqiYfDhNc3yT12mIjbg3FxBbQaAovLBL0evP3DyIwGBCToCnMx1FSRtelEazahaaqjcnMLVAq8I+M45xfR6fVotFpmzl1EkEqxxGLMdvaSt6OeqNuNgEAsEUetUuNYtSFIJMi7+pGl09gmJtBJpaxOTCLXaYi4PYQCQTT9esRUGmk6jWtxBaVKTcjnI51OU/z4MZLf8yE3ZrB5vxdJIsnW4hJaixmFVoNSrcYxMELYF8Bxr5dEKoUglxINBAlMzxN1uQkFAiiGMpDI5Ki1OpIra8TDEZxraygzDMhkMqRSKd6ufmwjYxgsZlK30uhMJqRKJcHBUQQEZt9+j8pjh0hMzODyePGt2fG6XHhtdoRYEolOTW51Jeaj+0lGYyzduEN0Zg6lQc9CZy8nTj1OMBHn7Xfe4eyZMywsLHLz5i1qa2swm818+/XXSSYSXDj/HiNjs1gsFpbXHCgUaobGZvjmt77P+sY6GXo9ly5f487dbna3t/Kd772BUi5jbW2F2dkZLOYsvv/G2zz5+AEmZteZmJwnFQ+RaTRwv2uUgiwVI6OLaNRSOicVzC/ZMOqUvOPXo9Xr2fRE6JwKo5CJzK15OXOsmml7GMEf4epIlMFpB6XFAu92CiiVSv7zK120NVjpnRLI0OvIN8m53r+FRCpFr9OikAu8e2eBE/tqsG0lmFnc4qbFyMLKFv5QFKW6lJmNNKMzNvQaJS6fkXWHh94RPx07K7DmaAkn5WgUUcbmNsnMNLDhE5ld3iSdFrksl6JUKJlbWaUop4IHk+soFHJGFkTcvjCrG160ugz6xpbRKGScT0aBNK9dXuNkez7v99hpKDdzoVdEqVYxPb5MV64Zg07Jje41kCiQyxXc7FtFIqvBH/CikMlpKdfyrasraFRSbG4DCpmMK10LHGqvwmZ3I5NJOdflZnbZQTyRpq3RilknkmHQ8OTePJY2Y3gCYZYnZn7ue8i2n83Pfdr/KRAE4T+znRCzjm0fm1PAPeCbj9L/F8Lmx0CpUKJsqkNy4x4F1VVkHtwDN++ReeoY/s4+UokkhppKorZ1PLOLyDRqEtEYkeExSl84Q8rhQqlW0/TySyRsG6BSUnr8MOmHfH9dhgFiCfwrNvSVZYQWl5EX5mFMp5FaC5Bm6Mn1B0gnU+h37qBKsm2bd4si8rSI+XAHgiCQl04j16hJ6jTI3F7Kmnag291CjUyCTKMFQcCQnY22fTuJ3tYHtylrakCzqxl5Tz+avBy2hkYpevwoibll8o/sx3n9DgV7WpFnW/CtrKE1ZyFLpSg+uA99fi6qvBwcN+7S+PlPE7NvIk0kURsNaOqrsYQjRBNJ1JWlRNwesgoK0DTXE7p+F1NNFRmtzeTHomhzc4inUuQ31eMfHEXb3EBidBJTYT66ilLSiEjn58kuL0Wbk01GTjYKiZRgIICQl01gahZDbRV6jYacXc2EDDpEo4F1r5fjL76AWiYjFk/wwvNnuPz++1itxbg9buKxKDW1Nbz48edRqyQ4tpx87IXneP27b9G6axfPPP0U5869y69+8VMMDI3T3z/IZ19+Aa1Ww//4+jf51V/+OBqNhsXFZfa2N/Cxp9p5MDyHXApPHy2lZ3iVxzvKyNImaa3PJxCMsqtcxdCUgqRExQsnSkkkUqTFGrQqCWV5Sna3lJOVaeDMiTzcLh+P7bYgytVo1XKePFTCqt2DPkNPPBzCrBc5taeAta0QbY3FeINRDtYqEBEZnTZRWyBnKCLSWpdLc4kUf0BPJKalpVTB5EqIx/aWsWgPcqIlg65JAX8wiVQi0lyu58ZYnFBI5OyBIrJNUgqyZDg9JgxaJY81qbk35qEox0BLqZQNVxGleRqsFin3JuVkGtQ83qIhEs1DLpPyZJuBm0Nu8nOM1JUY6J7ysrs+h4o8BX3TXgqyDeyrkvFgNkp5sZmze0z0zQQ4sbuY0hwYi6t5Zr+eeXuUzz5Zy5o7xYkmLXZXCH9jERU5UmSSbIpNIiqllHAkg1RapKVEhlYJ84UG1t0JQKQ430hR3c+fjQb8awlF8yh4HmgCBkVR/KwgCDn8jJ06/7eG8+Z9TIf2kdJr8S8to7Rsx7GKSKX4fT6MNRXEl21o5TJyD+1l6Y13yNzbhibTCP4g8kQSVW42Ua+P+PwymtJiImIKx/AYkrxsQqEQifklVCVFaFsacfQOUnjiMOGpOZw37qGsKAWdmvFvfIdUMsXm8grSZBLzgXaCAyP4llZQFOYx3/sAMRjCsHsnoWSCjTvd6BprkYQj6PR6JPk5RFfteIbGyGioJZFM4b3TRebBPWQ1NRKYmUOdk01Sq2bt4jUMzTvIamogMD6FMpHE0FhLIhzGsquJxNIa7gfDGGqrkeu0zH5wA+3OBsyHOwj0DSNTqzHvbycyNo1GpUZVVUZgbhFVphFL2068I+MYSqzEIlGimw4UJiPqHXVEpueRBoIUP/MEgcFR0sk0WYWFLA2OQCpJdnsLzpVVJNlZZFiL8K6sEdzcQmrMYPLmHcIra7R97hOEM3XceuscDTtbMJVY+Y//6T/xm7/5G4yMjjI0NMy///e/zZOnT/GVr34NRJETxw/w7oUraLUaRDHNN7/1Oi99/AwGg56engH+7a98mvPvXcFms1NanE3brkau3+qhtDCDg3tqGJtaYWx8ll9+cS8DE1us2bwca8vn798dx+P1IogR/uzboxzdY+Wp/YVc6nZwqXudE3vy2PKJ3BoJ8MLpOq7cX2RvUx7+cJyukQ1278hHQGR9M8C9wS1OHyzBmKnh3M0F7Fs+rvUusr9WyamdGXTPRLn8wM0vncynZzqAVKHhdLuZB3NxNGo1z3WY+IvvTXJ9YIMlm4d0Os6fvT6GyaBiZ0MxB2qVXOjzs7iygUyhZnetiZn1FOe6vDy9x4hWnmDdncCfUPHCwXy+ddXGvlo9q64UHwwGOdWioyATBmbclOepqcqTcLHXiV6r5vn9FhYdIvt35LO0EWJxM8FWQMLZvRZ6p3zE0zJqi1QMznqJpWWYDRK+cWmWo406Mg0qZmwhaotUJONR/OEkPTNxzu6z8OrFafwBPzPrUb767hTpZAyrWeDqgy2+fd3Gk3uyuTPqwReV0lHzyL6HP1OIbIereZTyrwCRh878SUEQDMAWUPaonX+h2fwYLM4vEA0GCfQOkojFWLx2i5ojB/He7SawsopKr8d96z4u+zrJaJwCQYJEoSDQP4RSrWH8+k1qjx0iMjhG1B8g4PEgU8hJhiOs3u8hf2cznsUlBFFEpdPi3XAQ8vqwf3CLjYkZtOZM5OEQpNIUVFeTc+wAipk57He6iI3P4N904LrXTcmOBpQaFe7FZSSRGIlIDEkiiUSuYMtmI6OkmIxSK87rt/FuOrGUBFgZHCGruJBo/yjJZJJYKEyoZxBJMoljcRGtQU9UKtnOvxMMIszMkozHMQ2MsjoxgZiGoliM1XvrqPUGEnYHYsKOY2mJVDJFfjTGbHcPWVYr2nCYhd4H5DXWI/X7sfX0U71vN1G/j83ZefS9g8glEsZv36V4ZzPi5Cy+YICFV1/H2liHTKtl6n4PMZeXqQcDFCGyNTFDOpWi82++RlV7GxnmLLKysli73YWYSDDS3Y8mnqCguIjFxSUuvf8+CwtLGI0G7t/rIhQJMzc7j9u5iVqt4sJ7l2iorWR0YoaG+mrOvXuRtCgyPDyMOUvH4tIaDx484MSRPbxz4RadXZ1kaNqZjSe5daePTzzdxsq6n7//3l32Npfwxq0VsrN07K4zY8mQMbYcYnbVy+KGGl8wxtqGG4lSx+DoAnKVhsv37cQSAuduLhOMJHn7+gJ726TIZEr+6ys3OHmohes9dmx2LwatnJ1VJu6PbPLWXSdGo46ZxQ2K8owsbsbRK2BwchWpxErX0CKFOQbeC6spzDNRXWz8h7Ob6RUfM2t+Fu1e4vF8jHoNi2sOphc30KgKuds/T01pDj1zCXQaBVcH3eRlyrk5FGDB7mZkxULX0DwN5Xl0zsTJ0at5+94aR3YV4wzKuDOwzLE9tUSTEu4NLlOSrUSt1fM/zo9RZc1mRpPFpa5ZnjhQQwIdl+9Pc7S9El8sRVFeJj2THrwRKb5AlHe73ZCK8eU3p8m3ZHBvUk5OloGjLdlYMuTMLns5sy+XRDKFXBbi3btbXO7XMTZjJ8ek4c6EBFWO++e+h4iiSCKd+p8+jyAI/wX4AvBhmIQ/EEXx0sNnv892mJkU8BuiKF75Z07zQBAEI/A1oJ9t587eR+38C2HzY1BaXobRtohhXxuB5TW0Kyuo66qQqJRIFApkSiWa5nqSN+8RcrrQ79mJoFWjsBbivN2JsbAAVYmVWCiESVqC2qnHsK8NhddHcMuBqbocuQAyBNS7dhC91UlufQ26yjIMBYWIPi/y3BwSoShhqZS4PwAbTvIqK8g8uJf0vR5EmRTDvjbk05lEXW5UzXVYQmEmvvUDDNPFhDw+YqEpwh4fjrklCpvqMR3Yg0KlJBIMoWisITq/SIbFgqa9GeeNe+TtaEDbUk/cH8KiUiEkU6STSVImI1KNiuzSUhQqFYZ9rSRvdaI9cZikbQNNcz1ZHi/pdBr9np1UiGlioQhZB/finF8g/3AHvplZLBVlqKvKiY1PsfP/+ALOG/cxHD+A1elCn5eLqqwY38YmpuICDHvakM8vkAiEEEuLyFkth0SSrI52wpsOzE4nmfva0Kw7CLvcND52DEEmZYe1DDUSPJtbfOVv/4arV65gt9k5uH8/R44c5O133uXXf+3f0N/fx4ljBwn4vfj9fo4f2UcsnuD5s8fwev2QDKDR6CnMzeDazU6OdVTy9oW7/OYXTuNy+wkE4hTlGbAYRIwGAaNeyal2E7cGNvg3Zyu58sBNkVnCZ56oZskl5eguC9+/uUFDfRafOLODSEKCRinnhcfrmVvxc+ZUB7e7JzEYs9nXWkZNeQ6bTj+VVgM1pUa+e8FLdraJpY0QnzxZyoPZMEcaVCSiOqaWHTQV5xFKCOyoyqGjWkE4kkuWQc2BOjX3pyJEojG2vHK2PCGePVxKJJZGLpcTjSU4vkNFJJqLiMD+GgXxZBW+YIz9tWo2XGEu2FzIhEzO7stm05+m0CRhb6MViVTGgToN1/rsJBIpGotkzNrDNFVms6tUQoZGxvCUEo1Wx5k9JpQqNcFwjH1VMvonDOyvUXJj2EtjRTY1hVK6p9W8fCyDkVWRaDpGVWkOT7QZicZTTC75ONpiptAkRSnPpWs6zP4aJSfacuibT1CZIxBMqmlryCc3Q8pvv9TA3fEgx5pkbChNH8k+kkr/3LxoviSK4l/8cIUgCHXAi0A9kA9cEwShShTFR5aAgiB0iKJ4H/gtURRjwCuCIFwGDKIojjzyOP+bpKb5B7S2tooPHjx4pLbnLr/PKz130RUVEpqYIvPgXvydfSTENMadTaSm5wnFYujLrKBV4+l8QCgYJLvESsTtwXx0PyvnLmEsyCdj905CDheC20vEvo7pcAcbV24SDgSQZOjxzy5ibmshnUriGZ2k9JknCU7Nkfb6yH/iOGI6zfz3z1F88ijumVkIRTDvbCSlULJ07j3KnnkSmVaD53YXcUFAU1qEPBAkHY4RD4eRlRQiFyEWDJIIhNHkmFGXFuO+cReZUoWsooSYbQOVWoW6thLP3W4kEgmyylJmv38Oc1UFmbWVbHU/wFRWQgoBiVaDSqlAU1GK4/pdNI014PIgzc/Fdb8XU30NUosZ990u1Pk5xF1e1AY9up2NOK7fRa5SYuxoJ+oPEh2dQKVWE/L6MB/dj/duD/FkkhQi2R3tyFUqNq/cQpuViVBazNK5S8jVKipefIbAgyFy8vLIa21i4dxlMpQqnv/Up/jKH/0JJdk5tLW18cYbb1BVVUlGhoEdDXUgSOjYt4dvvPoN1CoZx4+0s7y6xltvXeKljz2BPxhlfGKKz3/yBF//1kX8gSAnDrZg23ATj3gpLcrgS69cpGOXlTPHKnnr6gxGLZTlqphc8BEIxTm9S8/MiofXP1ji2WPVjC8HUeky+fQzu1hYjmoGRgAAIABJREFUcXL5zhSfeXYvTk+A6YUtqmpqudM9SVV5MU+e3MW773ejUaRRyiWMTi5TkivHqEoTiUT4oHOJL54uIpUSebvLjVwqYNv0Ys4y8PFD2QjAB4MBkMgIhiIYlAl2VmWSqZPzdqcHuVzG0/uyePXyCgcaMsk3Kfj+rXUytDLKcuWsOSJUFZvQqURGlmIEImnO7DZyY9iHwxPkzN5crg76UMrl5GSIZGdqGF6MYs0S0WkVTKwlONWio2cmyKojxtN7M+mfDZFnUmDzpGmyyrkx7CcRj7CrOovZ9RQnmzW80+XBYlRSkyfha5dWqCzO4Il2C31zcVy+KKd36bkyGEAti7GjPBNfKMnVvi0+f6qQv7+0jFan4bOPFXKld4NgUs5z+7KIJ1O82+Wl9cBpnvn0b/9U+4UgCP2iKLb+M7YaAKqaGsW/vnT+kdo+Vlj2z57roWYT/EeEze8DiKL4Zw+/XwH+iyiKXT/F2P2iKO4SBGFAFMWdP7nHP46PRLMRBGEJCLCt1iVFUWwVBMEEfB8oAZaAj4mi6BEEQQC+DJwGwsAviaI48HCczwD/8eGwfyKK4ms/y3XGYjFU8SQjr36b8v17CI9O4tncIrDlJBqL45qcQWvKRJJIIlcqsU/NoDToiSLitq+j6h3Eu75BLBJBkhaRKhRM3+/CmJ+Loqsf16oNY1E++tpqAvNLZJQUISZTBGYXwB8AMcXmwiKK251IJBK8G1sU2NaJbTkJOJxotBqkUimBLSeR4XFSaRGP00nQ4SA7mcAxPYvWZCItQHBohOKmBlRqNTNd3ZS2NCMJhPC7PIR9PsqUShZu36X2YAfxwXEWevux7m5FHo5ithahz7GAUkEqncY+PEZpSxMTV69Te+QQgZEJMOgYe+27lLfuQuULYhubQKXVkl5cZmNuAZ3bg8dmw9rShDA0jlSvY6GnjyqdFokIbpuddCpFRlEBM99+g/JnnyCVhtWLH+Aan0bw+gm5PcwPDlJ7+jGqP/UCjhv3cN+8z9rkNMpD+/Cfv4x/w04sKdB54T3yzFmcOnWKtrZW+np7OXz4AHbbOt96/TucPnWK23fuoTMYGB3uR5CIDA2NYTEbmVtY5tadHhRyCd95U8LE1Ax6rZLuB2Pc7RrkmdN7iUSSlFoLCIajvPXBPPYND1M+HyWPVWDbCuBwuHkfAbNegdmUSaXVxMX7y8jVCXpH1wkEo3gDKYYXYiTi8MG9KTwRLYtLGzhcPhQKGQqFitfeuMj+9nq0Oj1vvddJa1MJIOJwB7g2GkepkDC5uEV9mQmZTMbcspPLA1q0KinLNifF+Sb21Kh5+5YXRJFgUkEgkmR9eROlSsHE3AZFeVlM2iI4fWECYSkZhjzuDC0h12ShkEv5oHuSo22l9M7G0GrUdI+s0ZttRiKV0ju6RGOFmbduLdHRmItSqefdu6vkmFT0zck4f3uOI7sreLCQRJCqeOWdMTpaShlYktM7ZqO8yMSr781SXZLJlSGB0dkNTAYFQjKD0oJMbI4Q/QtJesbWkEkErsm3STLXeu2otFmkRQU2h4/LA3oyjQbqi1W81+fH4YriCXh5+14ShVKFVCrlwuWbP7Ww+ZdCFH+qFANmQRB++E3470RR/LufYrpff0hFfgD8u4fZlQuA7h9qs/aw7qdBQhCEV4FCQRD+6kcfiqL4G48yyEdpRjsiiuIPJwX/PeC6KIp/LgjC7z38/rts0+sqH5bdwFeA3Q+F038GWtk+h+sXBOH8zzJ9tVKpRCzKp+xgB5JUCu2OOiIuD2qtFvP+PYRWbRTubUNdZsU7MUPdmVOknG7WJqbJbahDv7ORSomUcCCAbncL/vklsivLyaypRFQrKcnIIOZwEJ1fouK5M4hbTiLpFKqMDNR5OYSWVig5ehBtphH/3AI1Lz6D6PWjMejJtJjJ6GjHv7RK7ZOPkfb6Me3ZhXR+kdT9Xiz72lDLFcgy9MQBhUaN2pyFpqGGeqmUVCKBurmeTH+AzOoKpNlmSpoaETQaVI011ItpQm4PGmshgsdHIhhCnWUmr7qSpEyG1JKFpagQqTkTbWE+6flFsgoLMOxsJLLlxFJdiXZHLYH+Eep/6UXCA2Pkl5cRjoSR52Xjn5olv7UZeX4u8swMjF4fiiwjCYWC4MAwq9duk44n8W5skHfsAJQUoZhZILu8lNDmFqlsM/osE/LqMjRrNlQIVJw6TqhnGEkiQUNTE8rGHcwvLOBwbPHyZz7N5OQEDoeDA/v3s2/vHmQyKV/68l8hkcr52PNnScTjICZ55okD2Ox2yorMnDzcjJQ40VicF8+0Mb+wQnNdAbZ1N6eONDA9b+P0QSvffW+UcChIIJRkYXmLtoY8Hms3ce6unfrybefH3a11VBXrCCZk+ENJGuurOXNyF1Mzq2gMTyOVSMnOtpBt0WHQymlpKMFmW6O0QMfyqpO9raU0lRpY2/JTlG9ib42KDK2ctFgFQFYWnMwS8YYE2qo0uH0BxhedLNpl+CIJrEVmaksyePP2BrmmAtorlLh9JWRlyMnMk1GYU8bKepjafBFHbR6FWTLqrWpEsZ5wJM6+GjWd4x4Kc4wcbVDRORGjscJCe50FfzhNTpaGYCiCWiWjPF+PWZ9iR1UOdUUqii0KLvd7eemxGpJpgbZKBbF4OWoFOL1h2motVOUrSCYLUcjldDSquD0R52BDBssukcpiE/GkwLEdWlQKKUOTGtrKBK4PB3j2oBWFQkYkISdTn2bJlSASSyKVyji4w4TZIOet+y5OHPmIMnWKj2xGc/44zUYQhGtsZ07+UfwHtvfFP2Z7L/xj4C+Bz7HtfPmj+GnNWU+yHYDzKNtnNf8s/P+JjXYW+FAzeQ14+ofqvyluoxswCoKQBzwGfCCKovuhgPkAePxnvajE3CLmva0oqitYfOM86hwLpoP7CI9OYN3RQNTjxTM4iiwaQ1lSRMjvp6CqkrSYJr6+CQo5utambbNPPEHBqWMkbBtEJmZRVZdDXjaJUBhNtpnQxhaJDQfW0yeITM6i0+owNday2dtPShTRZFsIO1zIFQoSahUxl5vU8hq6mkpSahVR2zpp+yblH3+a0a99E82OWoRYHGkwRMmZUyT1Wqa+/QM8Xi8xuRz7nS401WVkNjfgHBxFlpVJLBoh7nCCTEbG/j0svfUegtkEORaW33kPdX0NmU31+ManMZdaCU7PI4oi6bV1Kl56jkD/COL6FvnHjzD35nkydjUh12ohLxu/x4P50D6803MEVm1k724lOrOA63YXGXt2kU4kETe2aPmNX0EmkSCRSKj9zIskFpYJDYxi3LMLgJwThwmu2Znu6sZ1t5vGz38KMZbAduU2e554jOLmRl79678hw2jE7fXgcrkosVrp6+njwP59vPTix7h2/QY6nY6a6mqsJaV8+ztvcuLIXiKRGHfuD3C4owm3J8z5y110tFWgVkp482IXv/KZkwyM2pic26K2Mhd/MMZr7wxxuDWXU4erkEiltLdUkEhJ8IUSqDR6TrQXMrYYRK+VU1dpwe/dxL7pIz/bwODoPKNzLs483oEvEEKjVnL8UBsLyy6+9s1LHO+oYGBslUKLgmeOV3F/3MO6K8Xnnijn7liIvik31UUK5pc3sG9sotdIiMTj3BpyUlqcS2tjGaWlBfzJ/3mIha0UUysBckxqnuzI48awn4oiAyubAbrGfbSUaSjMErjY6+Jjh/OZWvYzOu/GapZgMQisOOL4ogoaSzRcG9hCp1Hx/IEc+qb9WPOz6BxzMm1L8G/PlOGNKZiwiXzyWAH9syHeuu+iuUxLS7kWZ0Dkg0E3uyuV3Ohd4pcet7K0EWPdk0StVhKLhrg36qQyV0KBRQ2pOB5fhGf3ZfJeX4DbI27O7s/lzbsb1FoNlOVp+Pr5KWzOCMG4lLO7jeRnafnVM1Z6pgJcG3BRXaRDq/75M9JEROLp1COVnziWKB4XRbHhHynviqK4KYpi6iFb7Gtsp2+GbU2m6IeGKQTsPzr2T5jXCbwBfFkUxdd+tDzqOB+VsBGBq4Ig9AuC8MWHdTmiKK4DPLx+GFuiAFj9ob4fqoH/VP3PDIvz80RCIUKDY6TWN/DY1gltOYgOjrI5O4/X6SLh9TNz5QYRt4dw3zDRUIjFkVFiXh8z59/HNj5JbGyKzZl5PKs2PHd7WBoexbuxifduF5vdfXhtdsJ9Q6QSCXw2O4IAYacLl8NBsGeAgMtN2B9k/XYnIa8X28wsSkHC8sUPiKtV2K7fQfT5Gf3Om6STKdau3EIqk+MeGGHizj0SsTihwTEk0Rhag4G0CCmvj82RcVL2LYLD4/gcTtZHJ1CkROYvXmNlbILIwAipZIL1BwPE1+x41mzEx2eI9I+g0mpZHBwhGokw+OVXSEgEwgOjOFdXca5vEBifJOzzE5hbIDQygdTrZ31ujtDwBEpTJpFAkNDoJGsTk/idLkJzi0x+cAupUonvfi8u+ybxWAz3gyFm7twnlUoSnJwlHo2w1dmLMpEi02RCJpGQmp5naWIS//oGfecvoUylMWYYcTldzEzPMDQ0xOXLV1hcXqaru5dL71/B7/fz377836mqqiTTZOLO3W7eu3wHt8fLve4BaioKkUjA7QmQazFy5mQrE9M2CnJNzK9sYl/f5LsXBhgcX2RxaZ3+KS+RuMDdoS3yTTKe2p/Pe51bZGdIkUgkdA6tgpjk/O1VHozZsZiNLK45+H9fOU8oHOOt87fY2txifWODr712gWQ6zeDILCMzXsYmF1jbivLOzTXsjiAT83auD4dw+fxc7bUzuZokmJCQZdTjDQsoFXKu9KziCaa5dGeCNDLe7/WhM2bz1Xem2PInudQfYHBqnYlFD3OrPqaWHbx9b5MlZ5rVTR/XxxKIAnzz0izrmwGi0RjfuDSL0+0mlFLTObKJKyRwezKJRCKhf3SJVCpBKpXk7kSEiRk7KzYnt8ZjTMzZWVn3MLqS5M5ElKXVDXrHNrg/FUEpl3D1gQOJXMH3bqxg33CSl5Hmat86/XMhro9GCcVE/IEwF7pdIAjcG15nzadgftXDpieO3ZXAYtKRiIVZciS5OR5nYtnDtdEoWo2aD7qXWFwPs+H4CNho/Hyozw9fwD/EM8DYw/vzwIuCICgFQShl20L0yAyyf/gd24SCp/4la/yozGgdoijaBUHIBj4QBOHHJZr4p9TAR1YPHwq0LwIUFxc/8iJLy8vRrMyjbWkg4nJTcewAgiDF0FhDTCnHOTmNpbqSps9+gsSqHU1rE+FrPrTlZZiOdCBJiyj1OkSTkcaXniPtdKFtqqdUJkNMp1A21qE06ImJaaSlVnJ27cD+pVdw9Q+zMbeAuaEWwVpAaaYR0RdA29aE6+Y9LHm529GhO7vQer3kHjlAYHqWLGsRWUc64MY9sj/xHOlNJ7UqNSlA29JAOp2GQAhBISey5SSnthJFbQXBmQXKjx8i5vEizc9Du7GJf2s7UnT5vlZCw+NEvT7qP/tJYpMzGDvaCd66T3F9LcraSjxrNjJ3NiFVq8iNRZHLFfgCQVp+/Qt47nSiPnYI1817FB/cj9SUSXBuEVN5CYoyKwXhCDKZjLQ5E0OOGUN7C4JEQplURiwaJevwPiwmE7FgCEOZlaFrt6h94SzpRIKmuioi/gAqtYr6piYMUhmnXniOzisf8IVf+SJbW1vU1NSgVatob28jGArgcrnZs2cPnZ1ddHf3cPzYYSSCwOOnTlFqzcPt9vLuube43TnKwOgssWiEgoJcRifm2XQGOP/BCIsrGzRUF/KJs61IEKkp0bG46qK6xMAbFzeJRSOEYnnMrbrxhmKseRL4Q1EyM7SUGpQU5JrwR+V4fDF+5zdfZnrRzZGjB/h/vvQKSnma//BbLzI8tsDCXA47anLx+fdRZIbmGjM/eF9CLGLk8T3ZvN+VIiXT0tFeRG6ekTWbl7oSA0PTLqqsJsrzZezfXUdevoXje0p459oUf/g7H2d6ZhFBSPHE8WZMOhF/TEFxroqtTR9+v599O600lChx+fS4vNlotUpk0jQl+ZnUlmYSjwSpsmbRbJVg0st5636QXY0lpEWRYChKR40KpycTQSrjcL2SQKiAeCK5fR9OsOE0YM2X8/hOHQZ1MVueGEfrFQgpI3ZnlGyTDoNOTUuFniKznHc6g9RWFnG8UcEb9z3saighGonw9IFiBJkUpzfMrz1TSe9cnFM7ddwf92IxqtlXKWdpM8IXztay5BTJtXwEbDTx58ZG+78FQWjenpEltjNpIoriuCAIPwAmgCTwaz8NE+1H0CkIwl+zfbYe+rDywzP0n4SPRLMRRdH+8LoFvMO2yrf5oXR+eP0wROs/pQY+snooiuLfiaLYKopiq8Vi+anWKmSZiK6tExoex9BQiyyRwDsxg16nI9dajCIlosqxEPb7cQ+Poa+pRGYtxDM4ijY/j3gyQXRhBa21kIg/wOaNe2hb6kmoVWxeu4WupQFzazOx2QVc3f00/PKnkMeTlO9qwajTEpucQ11ZSjgQIOx0bzuIRqN4hsao/8QL6EuteHseQCCIMtvM5v1e9E11aB4KKDQqyDQQW7Pj7O1HWV2OfWiUjF07yD7QgbdvEGk8gbIwH+f0HKnlNYrOPk5uRRmRSBj//V4C65uoTZmoTUYkBXl4ewdRZxqRIBDoH6Hul18mMjqJ62432qYGoqEQOqVym222fzfjX3uNhFJOZn010YVlNColOfv3svDWBfStzYQCAYKjk5ScPU1oaJyI041gykBWUoh3cgbBoEO/o474io3GwweITM+Ttm2QWVFK/s4d2KfniAeC7P/Yc9w4dx55IkVlTQ1rK6tkGAx88pOf5L2Ll5AIEn7li7/MD37wBhsbm3z+858jlUrj9wd59pmzDA9PMTY2zrNnn+TNd2/y/NPHeOr0UULBEDsaavj6f/8DNpwBjh9q48ShFr53YQC1Skp9VR6lRSbevDLN8T2VZGfpmVvz8Qe/fpKSkkK0Wi1/+FtPMbsapH/Sy97mQobHFmhqrCUzQ4/ZIOWP/vS/UVKcx1NPnORuzxTD40vk5Zq5emecTzx7kKnlELF4AlOGkkPtRXzlrUkaG0ooKczkvVuL7NmRTUGuhjVHhBU3vHSilAv3bDx3qh63y8nv/Pm7LCw5WFja4MGoDVFQ01yXy50BO8d2F1BdasEdTLHqiHHmUBlvXl9mYMbDyb2F3BjYZHwpyssn8lnZDLPhl/DS0QLuTYSZXglQU6ghEouhlEs43KCiby6CwaBjR7GM4aUYapWCUy16vnPdzoO5GM93ZCKmEtwe3qI8R+DYDg33pyKkUKLXaZlcjfDFJ0sZXgiy5UuQodOQTqd5p9vH6VYjiWQao15NU7mBdWeAeXsYpVzAqErwzv11MvUqXj5ZyPhqglWPlPI8JdFoGJfH+1P9938WEBFJi49W/kXziOKnRVFsFEVxhyiKZz60Ej189qeiKJaLolgtiuL7/4Jp9rFNof4jts+E/hL4ix/b44fwc9dsBEHQAhJRFAMP70+yvfjzwGeAP394ffdhl/Nssyy+xzZBwCeK4vpDCt9/FQQh82G7k8Dv/yzXGo/HSW05WR0cRZ+bjXNqhvXuPlQ6LYX1dSz2D2MpLUbeP4JKoWThbhc1e3cjB2Zu36VufweSeAr74hL67gFUShULE8PIFQqiidg2G62rH5layebyKhqNmph9g42FJdLJBPFIBEtdDbpQCGW5lY0bdyh94Sx+t4fowhJJrx+tTsfG1CxZ1mLiXi8b9nUSwRCiAOuDo+S0NmFpbsT/0LE0nBTxuT3EV+1IRFjseUDV3j1sXLmJZ81OjrWY4PA4QrYZSTJNJJFkeWgEq7j9ZiJFYPLWHSraWlkcHEGXYyZ+6x7u5RWkUhnKHAv+WIzYmo1CuQzH8hqiREBXXEhobJLViQnUhgykazaiwRCxkUlkgsD67Dyq8hLEZILA3U6sZ0+DIDD2t39P5dOnSfoDbA4MIySTmLJMBJwuYgNjCDIZBqmciMNJ11vnsC+voJVIuXblKk6nA6VMzurqKjk5OUxOTvDtb7+OTCanp7cPnV7P7Vs3KS0t4eL7VzCZzdzrvI/VauXxx04Sicm5fK2P1bUVDnbsxGZ3MDe/wtqKgFopIZlIMzS1gEYpRSoReTBmI+dwHU5/CocnwPVeO+ubXvyhGDJlBmMzm+g0Ml6/MMrCkp3xqRlSqSIqy7PRdaspKshlfcNNT98Ighjn0L565hbWkUgkvPTsEf7gD79CRVkeNmecDW+S4RkvkZiMmYV1vn9ZQWm+musDLkw6gYs9CYLRNFMrSQ7ub8PuEjm8p5ziXAPfO99FVqaanoE5AsEw13vsgIhao2F+aZI7Q0bWNtzsb7SSjkfQqRVsOH2c75ahVqnoH19EIZey5fIzNOXjU8dLIB5EqpWglKsYm9ukMFtDYXYmF/u8+PwRrkV0zKw6iSaS3JTJ0euUXOtZJBBOI8g13O6dZ/+uEtTSBCtbMWqKteQYZfzVGxNUW82MzzvYVV9Iz1yS+wMLtNYXcW9KgiCkWXcGuD/qIhBN0zu2jkq9LezXnBGay3Vc6AoiSpRMzSz9LLeHR8a/kugAPxGiKB75l/T/KMxoOcA724xmZMB3RFG8LAhCH/ADQRA+D6wALzxsf4lt2vMc29TnzwKIougWBOGPgb6H7f5IFMWfqVFWoVCQdXgf3u++TdjjIae+GoVSSckTJ5HotVQJEHa5UTTWEOgdwlJehqy2ArlGg7L3Aeq2JkLX72A9uh+pQklapUC/vo6huR5xbZ3McBRNcz1yrQblxiZBfwCNXEphYx1iNIbH6SCyuUV0bYOo04lrZQ3z0BgJnx+/003xmceRqlRYBUioVbiXlimsq8XU2oTUYECaSEIyRcrrx+/zkUokUJCm9Mh+pGYTsWCYwt2tKKwFRKbnKHvsKFKNBnVRPoGefram5yg8cZim/ByCq3ZU9dUE1+w0PnuGVCBIXmUZhoJ89Dsbkdy8h0IiRVVcQHpknIycbJSNNRiiUXJPHiZhXycllVF+6ADJZIKkw402LwdZZQnSjU1M/gBKpZKoKOJcWkV14y6JZJJYNArxBP8fe+8ZHed13es/0ytmMIPeAaKSAAiAqCyg2MVOUaJkFUuiiiU7yd+xnWs7Ua6dxE65aU53laxeqMJOihQ7SJDoRO+9z2B67+/9wNzcfMhVlJVE/8RLz1pnrTmz3r3eT+8+5/z22XuLpFLitVrySksIOd1IBRElWzf/kzRIvIG9Rx7kk/c+QK1Q0rhzO8MjI4jEImw2G6Ojo5hMZp595hnefPNt9u3dzYaNG3E6HBTk57Jz5zY++PAEmzZtZGp6moN7t3H5ajPf+vqzdHd3MjO3zNOP7uQtsYDf52X7ptW88b6ZstICtjas4rXjd/nN5/bgctiIT0jF7fKwY2MBZ65PkJiYxP4da1HHGbBYrDxxsIKc7Bzmll1srF/L2MQ8B/bsYMVqY++ujcwvmpBIxLR1zzI3N8elW+mAQCAKzz62hffP9rF1YxW7Nq/m+IVu9u9uJDMhikQU49UP2ziyrYiVgIajD60BuZKcjCTKVq9icMyCWiHm6JEafAExOo0DvSqBvHQlqzJ1nL8+TlGOkRSDiENbChDEUuyeEDuqk+macLOnRk/nmIvURB17a/Q0D4JYBE63n5FZF75xBypZNm5vgDlTjJ5JOdMLNpQyCQdq49CoiyEapCBVTKJWzuVWETtrkumZ9HF4az4GrYxlawSLw4PLrcYfkHBkezHL9hCNBh0SscCOcgXRaBFOj5+GQhln2yQ8vTsPu1dCZXyM5HgFUjmsW2PgOz+5t2l7dk82KoWUWWnlf6R7+Ezci9n8N2mN9q8gEom+/y/9LwjCDz6T/RdJnf9vTl74mJ9euYC+qhzP+DTqtGRiiybCgSDBUBDjpnpEIhGOW63IlUrUdVW4WzqJ6uPwmi3EvD7SNzUgi9excu0W/kCQjB2bcbd24Q8ESN6yCV93P+KcTFg24zOvINdq0devw9zagdQfQllWTGh0krA/QPyGWhy32wirlBhLS3C1dyNNTsDWP0RW4wbCiya09VVYrjejKSogumIhHI2hSU/BNz5FLBpFJlcQt74a950OYpEo+sZ63C1diESgrV+H+XITxsYG7E0tyJQKouEQxq2biIbDeNq7EaJRDJvXs3j2E3QFqwiHQqgN8YQWlgnHqVm6007Onh14h8cQRaIk7tiMWCJh4dQFDPm5qMtKMF25SVxmGsrCVXg7e4j5g/jDIZK3bMR69RbxjQ24WzqIxmIY1tfi7eolXi5n7QP7WLrTgSwKSXnZhIQYi4PDbDl0AJFIxIWfvsLDT30Zj8vNVF8/JavX4DKvkJ+fz/z8PLMz0wSDIV544XlkMhnvvnuMxEQDy8vLVNdUYllZoa6miqNHX+DpJ4+w7/7NDI2MEw3ayc9N552PrpJsVFFalMb0nJn5ZRsBnxe5FNZXF6JUSPiDv/yQv/j+40gkYs5eHkAVl4jT7SUWDfPwoU3Y7W5++eZZnntqHwgCfaMWllY8PH/0UT44cRG73cETj+5ncGiUa01tbKivQC4O0tM/RjTkpaYsDVdASl1FLucu9yCVS9m7tYyfvn6B/HQpCiHCB1fG+J9f348+TslbZwZ5aF8tfSPLFOYm8sO/eJtH9q3l3dN3+cYT60hN1HDs0gwHt+Vx6dYUO6vi+fO3evj242to6vfhcvvYs06LLxCmczKEJyCwLlfCgi2GxR1jR4WGC10eBEQUJEV47+o8u+vTmTKFkMuk1JfocHn9hGIK7D4xW0oVnG+3s2TxsKfGyN0JL4nGOOqLVFzv8+ILRpGLQwSCUQqzDRRnyLkzaMfkiJGiF5FqVLJgj7EuV8aVPh8atYJt5Wo+arYiIczeuiSu9znRyqLo41SMLwWJxKC+SEM0ZSuHnvjGv8lf/HuTOnPL1gi/+9Hbn+ldqmb/AAAgAElEQVTZF0rW/bve9Z+NSCT6rX82VXLvSvSQIAjPfhb7L8rVfAqzs7O4zBbkc4uo8rJwdvSg0utYnp8n5Ly3ExdLZKzMLRANhUjw+ghEQrC0jEyrwTI9Q2JyEv5IFIlYgmlgCJlOiyxOQ9hiRSKVEHC68N1oxpifx3R3H6s3NOC+04nYF2BpZoYclRKPyYzL4UShUhEKhZlubkHs86NRaxi5dI2sirU4lpbRpCbhHptEFa+n5/V3KGnciEqjpuedD8mrWovHaiPg95OrVDDd209cUiLS3kFmB4cQiyXo/H5iEhHd//AL9BkZOBYWiE9NRXq7HalUytLgCFK1isi1ZpYnpwh4faTk5TB+8QoSuQxDRgaxaAz33DyWiWli0QjRaxIAlsbHkWvVRMNhFgeGSNUosV65gal3iPjsDBLrqpj44BSJa0sJDI/hXDYTC4dJnp7HMztHTm0NK6MTTA8Os+XQftILCzj/i1dRK5WM3mkj7POzsrjM1MgoQkyg7U4r2bl5LJtMdHV1kZubS0dnFxqNmmPvf4BCLufMubNs3b6NqfFJmptvsmNrI3eaPRiM8SybLHx48hJ9ff1s3lDG6MQSXT1DGA1xLJpseDxeUhLU3O0bR6OJIyrSYlqxE29M4eZdM6FwjNNXuji8bxvjEzMEQmGaWpKIRGBy3k5b9xwSiYjmlm5EEg1vvneGy1duUF9bxaWrLfh8XiYn50hNSaaltYNko5KH9tXy3snb/Obze+kZnOPDszfZtnkdx053oNMZeO/MTeoqV+H2hDlxaYSkRB256Rr++G9PsWd7NWcvD6BUKkg1ylm7Oos7vcsIIgVajZJv/vA0WxqKef3CJBari4vtDi40D7G1OpM7wxJiiLhwa4zyonTaJxTc7Jygrjybcx1RZpbshENh4tXpbKzMwqCO8smMhXA4QlxcMUqplJklC3FaDaduu/CHRdgcHqatidzommf7hhI+7vLS1DHKpupCRDI1Pf2TGA1x3BkOM7HgZWnFjbo0mw8/HGDdmnS8QT3jc1aUMgliSRoGrZjWPjtSMcTESq61TVFblonHH2J/XQJvXpold/UQhz5nH3KvxcCvxoZeEIS//OdzkUj0F9wLc3wmvlhsPoXs7Gx040lItRqck9O4rVZsJjNqrRZdTjyKtaVIVSqMXi8iIUbCtk2YWjpYmV0gISWZ1PJSZJlpaNJTcU1MoZtJxFC2GtfAMNa5BRK7B7DOzSFWKAhEIuRubURqiEeZl03wchO5ZaVo6ioJXLmJITMDTW0lkmUTquFRpIhQFOSSZ9ChjNcxeeI8qiQj6ds2E/QHKD28H1E0hrK0GOWdNuLX18LtNjSxeBQVa0h1OJBKJGjWrkFvXiHm8pBUUwkiEf75RbI2N6DsHUQpvld7TSQSkRYKI9VpiSjkrCk4QGh2AWVVOUkOJ4o4LQGfj9y9O5AGQ8RW5SCTSjHUVCIgoE1Lxb+wiLqsBMntNuIyM1GnJaOSygg4XEi8AZyLyyRVlqMpXY0iEiUcDqMrLaZULqd4xxbG2zoxD4+zmHYXhcWFe2aO1NJSDh06xKWTpzjwwEFS83KZGhmjeHUJaRnpvPHzn/Odb3+b6upq5HI5SqWCuro6PF4v7miUzMwsNPp4lqfHeejQTvz+ACMjY2zcUEtJcT7nzl+kpuIgphUHSUn7MZvNPLCnju/83s+w2R1UV68jJoh57qm9vPruZb79jWd5851TPPVwI8Nj86wtW43HH0Ol0nJg/35Gx6aZW3ZTVVVOblYqFkcMxBKefOwQPp+fvNwM7t++gZdf+4jq6nKeeuwAoXAEhSxGa+8SyxYP1zoW2Lx+Hbt2ulHLIzy4u4LewWm8rmIqShJJSjISDEs5tKOMuQULduddIlExKpWMH3z3MU593EJygo7tNQmcuj6NCAlJBg0bi+Q09ct5fl8BgahAzZosAhExlXlyJBIYnUmiplDLoj1ESW4Cuyr1xGlkfNQcQyIRI0T8JMVJ6F+APfXJjC8GKc8Sk6STYbEK9I4s8N3H13C+w0XFmhzU0hDP7C9iwhRmf62e0Wk9tflyJhedZKXpqcyVEwhF8QR0JBj1rEkTiDTkE47AzrVKgsFEEuIVbCxR0jUWAATWrzHQOupjZ10mOalxtA1a+MW5Gf6/w6tY0az+/8WP/KrEbP4F1Pwbqj5/IaN9CicvfMyHERe21k7keh0RmwPn3ALJ6+uQpqVgu3qThO2b8Xf1EktJIriwiFImJRCJErbZydi3k5Vrt9BVlePrHUSyKofwihWR24MsNwtpJEp42YwIEQKg21DDyuUmRHFadPk5+IbGESUnoFSp8M/MI19dSGhkAp8QJWldBe6hMSThCItDwxQcOXgvwVIEcokUbWUpnpZOAjIZIoUM75KJ5NXFiLRqwjPz4A8QMcajUioILSyjql6LvakFQSzGuKEWV8u9RFLdunKCQ2Noayrw9g0hjsbuSXqN9ZjburD2D5Nz8H7cQ6NIBREJmxuwXLkJYjHG++7VkguEQyRtakCIRnHc6USemEBgcYmEbY0Eu/tRVZUx9taHaPKyScrMwDe3SN7ubYilEpau3iItORmxTEpeXDwiXwC/z0fZ+nrss/PI5XKCIrAtLvHQo1/ivbffQSaREImEca1Y+OY3vsErr/ySF198gXPnzrF//35+8pOfEJFIePjo03z0xltoFHIOP3CY8yffA6Ls372VK02thEMhZJIYlWtSuXJrkOefPMgv3zrFmsJ0xFIFLW095K/KYU1xFmOTS3j9EQ7v28zA0ATt7Z2UFK9iacWDPyQiGo1xcG8jH5y4wvNHH+bU6fMsmqw88+QjLCwtMzOzSCAQZHHJxJOPHeDshSaKC7OJRiJMTi/i93lZsVpZX1eJSARzc0tsrC9lbnEZu2WRsfE5Ht1TxGvHO0lP0iKXCuTm5mKyuMjMKeR2+zBul50N67K41jzIzPQs9ZX5bFxrZHDSil4a4v2rUzx9fw4JcTLOd3rQqSVsKFZw/LYds9XJs3uyOXbdRGVRIuXZMm4N+SEWJj9djT8Q5O1L02ypyaNxtZIL7WY2lxu5OhBgT6WG810eagvkXOl20LDayMhiCBCxv1bHjMlLU5+DxjIjQ4tRAv4AB+qN3BjwYXMHeWi9nrevLiORSnlkUwI2d5jOyTAqhQTEMjLjw5jdEhqKFPzZsXE2lCawcY2Oi91+QKAyV8rgfJTCdfd/7jJadulq4dvvf6beYny9rO6/uozWx/9NL5EASdyLlf/9Z7H/4mTzKdgdNhauXMKYlclk020SV+WxPD2DLi2V2OQMPiFC7GoTgkKOxrTCXFsnJRsa0MjljE7PoGq6g1yuoPflNyjZuAHJ0gqjt26zeutmok4PM13diOVS/FYH+rQUZJ29yBQKZrt7Uejj8Ph9aE0CkoZqFq42oZxfIO/hg4RHJwnY7IS8HhQiMR6bHVv7XQDm7vaQVliA12YjGAgQp9EiaJOwDI1gzM9DHJHjsdpQa9TEF+VjudxETCLBc6uVmN+Pw7yCyqAnlmDANThCkl5HKCWJkTffJ3V9LWOnPya/thrnzVasU1PIdXG4JmdwzC0RdrtRyOX4/T78Tjf6YIiQUoFndg7N4CiSSAzT2CSKuXk0/9h8TSyVILR0oZCIkTicTIyMkWA0Yr3ZQjgSwTQ7y3JnN09/9UXWNtTRefYCEo2GV/7sL9m5+36kUinNN28SbzDw8YkTtN1oIisnB7vVgggR77//PhqNmpdeeomMjAxef/0Npqam8AkCJ957j4nJSYRAgFAgiNvlxO0w8/gjB7FYrMTrtRzav4OXfu/P+M2vPY7N7iQUDPHae5fZet8mRidXmJ63YXUEuXmrhazMdGIxERKJhE9u3GXFEcLjdrNj5w42NlTzu7/3p2zbupGm5nY+Ov0JGxrquHKjFQSBt9/5iOp1ZaxZXcD3fvi3fOPXnyY9LYlvffePeeDANoYtdpZNNiw2F513+9HFqRgb1zI1Z6br7gDRsIuzGiVWR5iJ2XnWrs6m6+MOAhEpaxwSFpYtyMUxdPpEUlOSmJiaIxwFlzfM2IwLjULEstnJ5U4rOp2Gu8OLJMarcQUSCIbDWN0hLna5GJ62kJGWxKlWO3MmJ4FABE8oHQQBlzuALwQ9U358IVArpWTpo/zea/3UlGYytKikd3SJlIR4pubMGPQqTjQH0Wg0dA4ukJQQj9fjIipIaR50cKF5moriNE632hmbs5GXrqNzwouAmK7hBRJ0SoSYmI5giJqybM51uPF4fSTp02gfD3Dr7iRr8pIZWTJgdXiZudb8b15s/r38n6TOXxH2/7PfEcAkCELksxp/sdh8CoZ4I8rUZASFgsz7t+NbXKLsyCGCc0vENzag9voYfuNdjPm5hCJR0lYXoa6twN7eTVJWBsbN6/EsLhM/M4uyvAR7+10yV5egrliDEI0SvdNO+oY6AnMLKDVaVNVr8Vy5ScqqXKQpyVivNuFWqsgwxqPWagg4XTi6+3FPzbJss7H6yS9hH50gc0Md+swMlFnpaOPj8VltGBvXM3vuE5ZXpsnMyUCp1RKRiAjMLmCbmsEhkyFSqTBNTaFJSyPr/m3Ym+6QU7oadUUp/q5ebPPz6K41I1LK8TicoJCRXVmOTKtBV1uJSCxGIpOhqatEGQwTCoVQ11YSnZjCfuU6lo67uKfnkSkVaEqLcfUNUXZoD7ahUVK3NzLy7keoVEpKvnQYd+tdvB4vOTk5xMkV5FZXkZiTRe+p8wRXrAQdTl7+wZ9gWVrk6NNP8+d/+r8YHR0lOTkZgzYOiUTMqlWrMGh1BAJ+XC43giBw8OBBNBoN3/rWt9BqtTz99FO8+sYbiFRK7juwD+9rbxAnV3D06FGOf/QhVwd7uXj1Fs23O1hdks/Lr59gftHCux/dICc3m6CgpKa6hi8dOUAoLOD3+/nyYw+BRIHP62Hntjoi4TBKhRyH08udti7u9g7jD0aYX1rB6/Gxvq6K7KwMdm7bSH5eFiazBZdzC/F6HeWr83jj7Q/4+MIVFpZN+P1+vL4Ihw/uYHR8hsyMZD7+5DqTU1727mokJy+HQDBCkkHOptoCVLppnHYHj+yv5G9fuYBBHcczj9/PWx9cQyREKFyVTkt7P+ur8tlYFk9L3xKj4/OU5Br5/WfXcrnXz77aeBDLUCnl3F9r4IMmMRtTDTQUa5EpFRh1Uu6vyeCv3nWycW0KG9doOXHbxh88V0nbeIAMg4j3L5sQiyVMLTjIzzKye52esQUfJblGGgol9I1BNCJwqMHAwLSH6pIUaldJaB0WcatnkYZDRWytL8QfCHOwXodWq8Xl9lG1SkPnmIdHthey4ozQdHeGrBQD6wtlRGMSxqaUZCbKkNmCfGlHMQu2CFtK5VzsEJOTW/i5+xABPpd+Np8TUmBeEISgSCTaAjwkEoneEAThMyUwfSGjfQr/R0bzLCwRHJnAND3D6mcexz05jTQKsrxsAt19WCanSVlXQQCBkNWK3mDEFwygSU3GNzCKflM9jva7KDRqSE1G5HQTdDjR5ucQmpxBJIDH50NTUojIaofkBCJLZsShMFG1Ckv/IIbVRagz03G2dCCVyVFXrMHXN4wgCCRtb8R65SakJaM2GBBp1AQHRhCEGEF/gKhGhaEoH9PlJsQSCel7tuNq6yYajRBfvZbwxAwhlQKlTodzZIzkbY2sfHKdhG2NmC9dJxYTEClkSGQyEuqr8S0uEzFbkBviCSybkaanoFSpiGnVOLsH0BsMWK0WcLnJ2LkFS2sXiuwMtBIp2pICfBYr0+c+ofrAHjxWO9aZWWrv38HC4Ahij48djz9C69kL2C0W7qtrQKFU0tfaxpaGBmZnZykqKqajo4OlpUUyMjI5evRpTp06zcqKmeeff55XXnkFo9HIgQMHOHHiJIWFBUSjMVQqJd3dPah1OpatFtLy89CoVPh8Pkbb2tnWuA6DIY6lpRUWl23IJAJms5WjTz3MO8dOsa5qLf2DE+Tl5aBRCEzOLLJxfTX/8JNXef6ZJ0hLS+ZnP38NiVTM8089wPvHPyEUlSIIcGDfDnp7+piZW+TIAztpaW3FYvPy6JF9/OTlt/nqMw/yyhun2L97Ex+dPMPo+CIH925h+33VvPruRex2L/F6LdkZBqZnl3nikT386CfvkWiM54WnD/H+8fNY7W5ePHoIvz/I1eu38IdllBbn8P6Jyzz35AEuXGkhXiOiLFdOklHNuStD2GwOJCIBmRh2VGgYX/CwYBcwGOJYMLspz4/DFVJRVRTPq8d72FqfTdeQje2VOlpHA9jsHmoLZJicYqoLVFzrsTEwaUcUDRERyXhmVyYalYRzHR4Qi9lcIuf1S/M8uTMbkz2Awy/D4orQUCjlYscKRbkp1OTLOXHHjlQspiZfxshihDiNjLIsBcebzRRkGagvUnFjMIhIiNFQLOdsmwOzxcn+ukTsPpg0xzhYF0f7iBONUorVKyZn7c7PXUbLLC0RfuOdX36mZ3+ncuN/dRmtm3uFj3OBi9y7HFAsCMLez2L/X6kQ5385rFYLtmvNiJfMKOP1OE0ruG614puZxzE9zfLVJlwrFkKBIK7ZeVhYYra5DfviEprsTExNLciz0jG3dDB+8w5O8wo+8wq+mTlkEjHyBCMep5uoVIokNZnBt44Ri0YQLZoZvnAZIlFkoTBBqw2514fWFyA5fxWzvf3IV2zokxJZ6B/E19KF2+tl+U47UacLJqaZuHkbrUIBCFg6ewj2DRNyuQhHw1jutLM4PEIsHCZkthL0ehGsDpSZacgy0xh69R1ESiW+jh7sJjOulRU8VjsBmx3L7TbEZiuj15qIWKx4Az6C03NEo1Fi49Ms9vbhtlmxT87gc3sIjU8T9fuYu3QNkcuN81Ybnt4hZN4AwclZpHY3lv5hmJonWapg8PpNZi7doDglDYnVgXV6hhunzzA1NMzysgmv14fBaCAYiXB3aIi7w0P8/I03eOfdd0hMTOLcufMsLi3R29vLrVu3MJtNnD59hq67d+ns7ubi5ct0dXYyPTrGQEcXpoVF+lrbGRwYYHrOQtOtbn76izcwxOsZHpkgEAzw5jsnEBDz6mvH6OnpZWhomJ+98g53u/s5+/ENpmYW6Okf5uNPbiCVqxgemeTtDy4xNDJDW0cniwsL/P2Pf8mmDdWUrSngz370c9bXlBKLBPnpy+9y5OA2RCIRmzdW8sM//TH9g1MopBFkgoOz585j1ES5fv0a4ZCHrIwE7A43bx07h3l5mcmZRd49fo2zF29hXrHz6lsfc+zENd45fgOb3cXNlj4mJqe4dLUVu91Ne1cfLo8Xm8PHijPA4rKNKy0jWBw+rvQHmXfKOdc8yZJDICaS8/PjQ8RrxNhcfiYXnaQYlOzdlM4vzkyiUwTJSVXwkxOjWDwCTUMhTPYQgVAElUKEVAxXum2cbXMyPLnM4rKN8x12li1uhueD2P1SrrVPY3c4udDpZGLeRSQq0DoaQK0Q6BmeY8oi5uKdCaaWfVzq9dAxuMCCJcC1bgvdI0ska8Ocb7MRp1ERCkcZWIRLHcvUFsgZnfcSFqS8cWEMlz/KsmnlX/3m/6O512Ig9pnGfwNi/yibPQj8tSAI3wTS/hWbf+ILGe1TiI83IFWrUddUsNLVS8XRRwlNzpLU2IB7aJSJazep+MrTGAZHiaWnEp6awZCeSnx+HhGzBfP0DNqcLIwVpYgCATQJCUhSkhm8fANjZjp+pxvr9Awemw11ZgaJubko0tOQJxopsFqJiUTEVZZSKpEQDIbQpiaz3DfIjq+/iHloFLHRQPXBPQgxiK8so/u1t0gsyMPdM8iaDXVkNDaQgcD1kTEyairQajRERKAsKUAulZG6vRHnwiK9b79PdmkJip5B7KOjxKIxEhrrsd9pp+DgXiJTM5hMZiJeL1lbNhELh8h2u5BmpBG+a8EyO0ZhcT5Bj4fUkiISG9ejUWvw+/0oy4qRej3k6eLI3FCLIk7L5CfXKHvgAMmZGeiTkihMT0ekkON3mtm2cyc+l5uyygoc5hXWrVtHRkoqKytmGhrq+c53fxu5MZ7DR58koFbidbuo372Lnu5uGjZtJN5oZHZhAWO8npqaGj65dAmvP8Bvfvc7yGQyQqEwmdlZdLV3YFlc4utf/RrvmVfY+eILqFRKystKGRoex+F0M79oprAwn6ee+jKhUOhehQSFjEceOsDk5CR79+6hs6ubb379a8SECFUVpfzs5beprq7moQfu58TpiwSDIfbv3cZL3/tfnL94A0EQmJyc4OSZT1g2rTA6MY9SHkMQBBYWzcRpFBTlr6I0Px5/UMS2DSXYHB5WdlWxKktN651b+L0eHt63jqLCPManV3js0YeYnVukbl0x2xqrABgYHOHQng0cP32FjfWlPPFANa8fu8acz49lxY7D7mZ8fI6SHB2ZqcVEojF2VqiZM/t4dG8VUakCo1bLnMlJKBSktcdMLBLhwp15MpPjmFu0YcuLwxeSsLogjc1rVGhUUk55NBTmyAgGAuilCg40xNMz5SY3LQ9XQITdHeC7j62mbSLMzgolDm8WOpUImyfKg5uzEckklGXLOd4coLI4mepVUuy+ApJ1MpxuPy89uZb+uQjl2TIu3Jlj3qZjf30CFzscHN2dw6UuO0srLtqHHZRmK5lbifKlbbkoVXI0Kf+2UlX/UfwKxWzCIpHoMeAp/m9RTtlnNf7iZPMpSCQSgpEwYZcbudeHOi0VcWoy4dkFQiYLBQf3EJxdwLJkQlhaRkhLJq2xAc/oBIFAgPLHjxCx2bF195GwvpaoRonI4aT4vo1o9XqEUJjix4+QlpeLOhxh1ZEDOO72EQmFkBuNCPFxBMamEEsk5OzYzGJzG7JAiMScLNQ6HfbuPjJrq/BbLNha2ilu3Iitf5hVpSWkFBcScLpYvHKLx/7w+zh7hxAhkL9rC567fSgVCuzDo0Sn5ijffh/67CykyYkkpaeTvXML82c+QZuVgTwhnqDPh0Gnw1iQT9hswXa7nZStjeByk5CYQPGDB/CPT6NOSiRhfR2Ou32I43UkNtbj7RtCJ5VTfHg/kxevEYtGSVJqKGvcwNLwKD0XL7Fp9y5si0skxuvJXV3Mhi33MTUyRntrG6ODg+zefT9Hjhyho6ODbXv34HY4mRwfx5CdwcaHH+STk6eo2rSB02fO0NXVxbqaGiwWKz97+WV+7Te/wXde+h0+OnaMltu3qWmox+fzkZmdTXlZGRPj46wtL6OurpbOzi6cTic7du7EYnOwaeMGatZVcedOO6+9/g6PfekBXE4Xbreb9fXr+ODD4xzYu5OKinKGh8fputtHzbq1hMJBXn71GEcO7yYjPZmVFQtrVhdSUJCNWCzwBy89jz8QQKuW8fDeKlbnJ5GXEUddZT7JiXoSDXJKizMZHJvD5w9y+mInh3eVMbvowOMNU5yr59iZNjZvqKBhXT4dXX2UFOXi8/kZGZ+j4+4gTzzUyN/8+F32bFmNEI0yMr5ARpKU7/36FoZnfcwuOnl872oiwr3r4BWrlHSOB+iaEdiwNgGT2cXkop9vPV1H37QPf0jMD16sQRDLmV3x8ftfXc/kkp+yVUae3ruKlrEAZrufzEQ5O6vimTP72LZWy4c3l4kKStbmyLC5AiTrJOi1ctL1Me5OBtGpRAxN2inNlFOWp8Vk83Li1hJ1RWq2VxroGPOSpJVidcWQKZQkxStwuP0Mz3n4tYdKsXsEVuxBlqwebg0F2VVtZEd9PmKpks6pKA/U66kqjGdo1kcs+vnHTgQEYrHYZxr/DXgGWA/8kSAIU/9YRfqtz2r8xcnmUwgGAsSkEjzdA0i1GjzLy3imZpnv6iFzdTFah5v+S1fQJiWRtmMzrjsdaOqqiEgkLF2/RemmDaj1OiY67qJXqpGLRPRdayKztISl8QniEhLQ9g0xcrvlXh5KcxuS1CRG3/yA5PX3erfMdtxFGo0S8HhQajTMDwyiOX8Z29IyAauN+U+u45pdQCQCo07PUv8godxsDOkptJ84R/2h/ZhGxkhJS6P3xk0SNXHIw1G6m1vJrl9Hcl014bYuwqEgQ+8dJ3ttKQnBMPPTMxjidYStDtyBIHq9jvh15cyduYDGmIBEJsU2NoFEr0M/t8RCdx+pa4rQOJxMtXZS9sxjSOVy/A4nyUlJiMVisndtofudj9i0ZQsAbqcTo0pN542bzE1OY1lYYq1MzlhvP1KFAqffy807LfgjEXRqNe8ee59vfu8lpicmOH3yJPe/8BxDnV0MTU4S6O1Br9fTfOsWW7dvp7O3B61KzcfnzqJUKnE6Xbz56mt8+ejTXL96le/94Ac032ji6qWLfPMb9xoNlpWV8td/8/c8/vgT9Pb0MDYygkRSx6Ur11iVm03z7XbWr6/lD//kr8jOSGZ2Zpqmm80EQxEmJiYZHR8nMz2DmdkFImE/l682U1dbye//8Ef8+R/9Dm+9d5IV0zymZSMDQzNEgg5U8mKaTt5CrdFSUbqKgf5BpqcUmM12lDI4fakHXZwSqVSCx21nfMrE6qJsRsbmePuDy0gl4HCHKcxLZvt9Nfzi9dPMzsyQmqhmYXGe1rtTRGMxLl5ro2ZNEtfalhGLZXQNToM4D6lCQdfAFOFIDm29k6SlGDnfamV4chmZTEYkGqW5bYy1hUmcuS0wMrUMUQGiUabnVpjMSsbpDeLyBLjaE+WhTYl0DNlwuH10Tgv0jprQarVc6o3R3DXBjg3FXB8MA0rO3einvjyH0VkzOelaTK4YKqWcps5ZNFodHo+X3jETO+uyudE+SUNlLlf6JMhkCq53ztBYW0SiUcMfvn6X0qIM1Co51wb8zC3YWTS7yErRc3VAiVwmRS4Tc+rjqxx+6rf+5Y/9P4vPr+rzfzqCIAwCX/9n8ynu1bIEQCQSfSQIwkP/L/svFptPQaFUEl9cyNBbx9CnpJAUr0NXWvR1f4wAACAASURBVMwqtRJFghF1cQHpM7NoivKxtHVh7htAoZCTWFeFb3EJUVY6Kr0eWf8gyupyYtEo2Q4H6pxMipKTCLjcqKrXkuVwoUgwoCjJxzU5Q4wYweUV9CUFqHRa4tQasndtZe7CVXKKCqnav5uhC1dJSkmhaNtmstJGiQEuq40NmxtJNSbSfPoiMauDyMgkW7Zv4+71G2yua6Bs305cNhuSYIjynTvovXwd/7KZuheP4khOwRsMosrN5IEXnmNufIKCPdu58fIb+KdnKcjOwiWWYBufQCkW45hfoGLrJnRZmai1WoLRCNqaCuJGx3H0DhFJMBANR+hvuoVMrUIQiVgaHMK7upSpW60sjIzh1mhQqdQcfOZJrBNTpGZn0XznNg8++hghMajTkklJSKZ83To+OH+OrrERHHOLdPT04Dt9CnVmKrFEPSKFlFV7djL80Rkqt2zGEwwQC4d59Mtfxu/3886bb1JaXo5KrcbjcnHi2PuYl5dZmJ/mtV++jFgiIRSOYDKv4HA6iI/Xo5Bn89DhvXg8brRaDeWlxfzlX/0DMkmU9FQDP/qTb3O7c4zHH32AX772HuOTszzzzFFee/1N4tRSdm2p5oMTF3E5bLz7/gnutHZQV72G5586xMuvH0clDVJRqMflyiIUFnhwRz7RsB+/38e+zVn4AxF++DcXyUgzYjGbGJlcZG1pMSDhSw9sIXdVPslJBr77/b/DtKjH7bQhEwXxe1Z4+PGtKORiDHoVrR0DxCIBdm8upqI0ng8+maBxQyJ7GjN4/UQPhQU57G24d8U5PUVPXpqSlOQKZhbtHLm/AI1SQdTvYUelllhMQKuWEycNcGhzLjqdlMxEKR2DXqw2Dx2TOjLSdOi0KhLVQaqKkqlZpSBOJcbnyyI5TqAiV8aMycfqvERKM0XkphSx4hZYX6SkZchObrqOzSUy+qZl9IzFKM1REthUgtsbZHu5kktdXsqLM7lvjZyeCRfPP1COyy/ivlIFJ+/4yUg1kpORQCgcY886LRZngKYBgT3bN3/uPiSGQCjymW8H/3fnUxM8v5DR/hV8kzOsPrQPbWICipREgkOjJKyvReL1s3KrlZTtm5GFI0hCYUqfewJpTMA1OU36jvsIjk1haW0ne+8OfGNT2G+2krRlI8stHSgK8zBsrGXh3CW0JQVEiOHoHUQZjVH23JPoVCpC80uk1FRBahLm/iGSMtLI2ljL2b/8e/LW15JSUkTbux9Rtu0+YpEoRr2exgcOMtraQVVpGU8+/RRFRcWsLCxSXlrGE88cZbSlg4k77Xzpy4/jWlqmMC+PvQ8dZuCD0+RtqEOt1WC+2UJuRTkp2Zk4pufITkujpraW1DXFFOQXsHXnDooaG2g8sI/wzCL2qRlkqUlE/QFcM3Pk7LgPaTSKdk0RMpmUgscfxmlaISrE2Pnic/g8HrxWK7/+B99HpdaQUVhAem4udquNlvZ2Hvnt32JwYoylhQUKaqvpnZ7kZ3/9tzzwwnP3rsBGwmz6jeeJBgLoM9IRYgLS4nxa336fbb/+FU6+/wFarZZtB/bzxi9/ydkTJ3nuhRfYs38f50+e5JGHH2b71i2kpRhZX1/Fgw/s4vFH9hEMeKhcW0JVZSV6nYq9e3Zwu6UdrVbL4uIS7x37kGee2M/qkkK8/jCZGalIhDBLy2YUChVffuIIV681Ea/X4nR5AFixrPDjv/g6IiLs2raR3OwMevonUCulPLi3jst3ZtGo5OzZmMbZ6yOoVDKO3F/C1ZY54jRyNqzLY1W2kb0b0tiyoQIxUVRaAzu2VNPc0sc7H1zi93/nRZKSk9jZuBqvx01VaRaf3BymoTqf6Tkz+XmZFBUXkZ2ewNtnBnn0UD0xQeDMlRG2b1jFw/uruD3oRq9V4QnEaB32srEqlcM7CrjWtoRCLsEXivJJh4maAgVeX5All4j1pUmMznmQScWkJxq4rzqTjEQFnaMeirPimDRFOXJfOkOLMT7pdrO3Jp5F2z1ZqWsqzIH6RC7ftVGVryIxLsq0OYzNL+dIYyovn59Fq1Hx9M5cRuZ8JGhiVOXKaB8PIIhV/9ioLca8XURVvhaREOZci4nKVWoqc2WYLC7Wlyh4//oSvbNRHtxgQKVSfP4ORIBoNPaZxq8Anxqc+mKx+RRmpqdY6R/EPTmNRCKm+8evEpHJCNgd+Kx2ZDIZAdMKQ5euIdJqCHv8CIn3kiHVKUlEVArw+olLT8M7M4c0Xo/pdhseh5PQyAShgVGWxyfwzM4jUyiYbrqNEIrg7+xltn8Q6+gEkUUT8kCI3o/OEHJ5mLrZgliAlZExlnoH8DucNL/3EYNt7QQCAVqPn2J5cpqp/gGCNgcdV69x+diHiMUS/F4fPqsVuUTK4J12+s5cIFmpRu32ETWtIBqZJlejJ2K20n38DFKZAnN3P8nxRnY/coTZW23E6+Np3LeXjjeOoctIJzElCVNLB7pVOchSk7B29qDOySLhvo1Yb7YQk0px9vYT8HmZb+vCt7hM581b+KIRTr13jMHREbq7ujj7znucPncedyjIubfeY3hqkubWNo4fP8GEx8W1lju03GjibnMLspx09CnJKBMMtL/+HhaXE//YJB6Xm6HrN5kxL9PV3kFfRyfNN5pITUrk9tWrzIyOsby0zNzcHKdPnyE3O43HHjnE5au3+Mkv3uK5px9h+9Z6/uiP/ogd2zaTl5vD2NgUK2YzA/0DmEyLdPWO0NnVx8qKldPnr+L1evjmt/8nVpuFzo4uTp04SV//AHPzSzz9lf9BTmYi128PsjC/yMDgEJGIwPvHL9He2cexM114AzE6e8doG7AxPDbNrdvd3O6cxuHy8NaJLjZXGnA53JxsWsDp9jA5Z+Fu3xivvnOZW7c7WFmx0tY1RNmaQl7/sImMZBV77ivh2p0pdFolGclaugcmyV+Vwfd/dI41xZk4XT5GxufR6VSo5CLcHj/do2Ymppe4dnuQcCTK9Y4lOvpX6B6Ypu3uGFGRmPaBZWbNIYJ+J0IswpzJi0Tw84NftGHUglYl5XTTNG63F4VCzPjsMndHLUwv2kiOVyCTiolXhnjj8hL3rVESCsfw+UO0DVpQisJc7jQzOWvmUo+XmWUH0ysRnEEJlzuWyTCK6Jtycf7mOANj8+QlifjF+RmKUmKcvG3GE5LSNrjM8GKY0WURJoubSZOY9sElLA4/1weCLJosn7sPERCIxmKfafyq84WM9ilkZeeQkJmJcfN6XMNjZFZXoMlIxT46wcLdHhKL81EEfCQV5qMrKcS9tIRvdh6H2Yzlxm2CLg/mqWniUlOYHxgks76GpNoqAstmZFnpyJITKQYIhpDlZFNyYDfBRRNxOzaT5HCiMsajrirDPTlN3vpaDLmZRKai6ApUVG3ZTOvx06TV19FwYC9/+41vs+W+LWTkZJOkiSMWibKpsRGPwwEZmaxKSuL6uXPM9A5QVlrK1772VaIeD/n5+ZSuXcvc5DR5+QWsWlPCQEsbz33lK8xOTrHY3MK8ycKwNg7n6Dgx3QoLcXqSpHIks0vkxOvpM60guTtAajjCgs1O3NgMEomE2bEpUivLKNq1hcWL19Hn5OLxeKl9cD8JScko9DqWXA4Syopx2mykra8hlJxAfEEe1otXWXP0MaQuD1GphOJ9uxDrtLQfO06K34PRZCYSCjHVN0Dp04+hNMbjmZ0jd/MGFP4QCkRs2rEdaSBENBpj7969fPDBhzQ01HP48GG+973vYbet4PGGOHX6YyorSjl7/goCYFlZ5tKVGyBAS2sLhXmZ3L99PYhEPHRwCzPTEwQDbtavywNgcmKSfTs3UZCfTSwawWCIx+vzkpMZz8Hd6wGYm50jIz2BXRuzmZ0ZJxrR8PjhDfzy7YvEKbPZ1ZCC1WInTmEgTePDZvFzd2gejUqG2ebFG4zy8J4KYihIzchj745akpJTWbFY2b65EovFzt/9uIO6iixmFix4AkFae81UlZfR1D6DEIVwTEQ4KmJq3sHswgpajZKIICFOrUSpMVJZEk++R0IgGGLHhnuKyMi0jSS9HJUsRig3haoCFV3DJhxuF1nxSQRDAuWFqZTlKAlHYkhEAlqlBLsrRn5mMskGFUvtFkLBMBJxImZnmJEpC7c0EpweP2lJevIztARCAi6vnfWrE3F4Q3zzkdWMmyHTKMHrC9I35WdbVSKCVANCjIggwu70EYoms68ujo877ZTkJrGrQk3HiJPsdCPRkJfvPFHOjT43m9fIWZAmfv5O5FcoZvMZ+Je6J/8TX5xsPgWxWIyqrBjrjTuIfX7StjUSnJpD7vFT/Vu/RrzRiFapJvvA/QRHxklcU4JKIiO1ci266rUoNWoKH9iLIJdStHUzEn+QgMtFck0lroERzM1tqEoKCMulLF2/hXpVLrq6KlwtXWh0cQg+PwAyk5X8XVuZ7hlAKYip3b+b8fYudIZ4PB4PLWfO8/Xf+5+M9fWzMDtLVk4ODzz6CG+9/jrpKSk0NNRz/vzHaDVafvqTH6PX6zh16hSPPvoo/Z1ddLa1s/fQQYY6OzAtLrGutoaRvgEmBod45KGHKV+zhog/wNee+wp52dlUrKviyCOPoNeoca9Y+YdXXiHscCEPR3nxa19Fl2gkf8tG7tu9C6NWy/ylJuoPHyQWjaLVaClsqGOy/S7915pY++B+loZGME3OsGpbI46xSQIeL4JMhjo5kYDVRsxkIaO6guW+IcoO7kWdaERuNOA2r1D5G88TnV3A2tlDZl01PafP09C4mY179/DmT39ORcVarFYrbrebYDDAs88+y09/+jOOfuUFytdVMzUzy0u/81sYDAYaakuJRkKUla5m6+Y6HDYzv/2NpzCZLWysL8Pp8hAIBCjMS2PXlnJ6B2f56Gwzv/s/nuB2aycWq43srDScLjdSMchlStxeH++fvMburWVEozFOnG9j+6YyGuuK+cMfvceujTnUV2bQPWQhQSfnyJYseqeCOF1B1uSnsSZDTGGOkaIcA15fkNRkAwF/gLc/vMaubXU8++QhPjx5g9PnbvDjP/0qWemJCCIZ//DHz+MPRGhuHyYzPYnZ+WX+/HcfYWLaxMDILH/3Jy+i1enZXL8aQSTm8N5qHB6B5AQtm9Zl09Qxz/sfD3Hk/nJ8IQGXJ4xGIWJgwsXGigzWFiXj8gusyY3nkc3JXOnxoJCJKS9Kw2A0kmDUIZfdk5B21OeSaNBRU6AGsZyXnipDqtSQkJRCdqoasViC2RXh8H15+AUVInkc6YkagsEg/7u9Nw+P4roSvn+3N7XU2vcNIQmQEEIsYt8N2IAXTOzYBpvYWTyvM/MlM5m833xJPJlMlsnMJO98bzyOx3Hi2I73BRuwWQ1ILBIgFgnt+77vLalb6r37vn9041H8YizsiEXU73nqUdWpe6vuKXXX6Tr31DnHy4z8YGcmg2PQ3G0hNVpDbLCHkvoh5s6IZFq0H/3DNuIjDKzICKa+08qgRUNwoB9jbj3RoTruXhTEseKxz/m2Tw4Sbqcnmx9ebadibK6C0WjEWl5NT0MDA51ddB47SVNxGSPGQQbzztF0qYSBxmYGTp3F2NtP97GThC5ZSOyKJbTtP4KIjsBU30TFvsO47Q5kSDDNew5ic9ixuZz0VNbgbGxB7+dHf1095gvF2MprGO7soulSCSarlZ4Tp4leupDR3n5UQYE019bSXllN4bFc/IKDGTWbKDt3HofLiXnUzOlDR4iLj2P/nj1cKi6mqamZCxcucv78OUwmE++++y4Gg4GDBw9SU1PLhrVr+XDXLqanpLB+/Qbef/GPrN+8iVefe47uxiZaGhsYMhopu1RMbW0tIYZA/u0nP8VisVCUdxppsVB54hRBDhfG9g4GWzoYKKum4NW3CYqIoLHgIirTGBVHj9NcXYMuIpSGS6V4dBpqCotoOnKcqnMX6O3upq+wBJdOw+nn/sCo1ULf8Xzaq6rpbmpm6MwFuiqqCXY4SYmJpe6D/VjHrDh6+rGOjqF3OAlNTKCvrJqOpkbqSku5WFBAaWkpjzzyMPv27Uer1fLyyy/T2dVFR0c7AYZAzl8o5GzBJQIMwfzn829w7kIZCYkJ/D9/+wOkx86ZcyVIt528glJCg/T89oW3uHNNJgY/Pw4cPklFRQ3vfJBDaCD87o/v0Nvbx8dHjlFbW09ERAjv7j5FgJ8gLjqE0vJGGpraOXmmlIvFVTS3tJJb0EJjxxjPv36Krt4hDpztprG1n+6+ITq7e3n2nWIGBkewjI7x02cOMn9OHBbLGIFBIRSX1vDKGx9xJDcfu8NBzpkG9uWWoVJpKChqoquznfMXKyirrKOhoZ7Xd52io72Vqup6Pj5ZybS4CP741ikulbdSXdvG4eOXaOk0cqmmn71Himht7+NsaSe9/SPU1LXjljr25rfSNehCpzNw+FwHbYMuDhWZ6Rsc4t/fqGB4eIRDeZW0d/Vhs9n5w/4GUqNg00IDRy6NEB2m42LNMD2Do5TXtuN2uSlsdDIwpiY1Rodp1IJeq+JE8QAjFhXVjf2cq7cTEujP64cb6BqGgTEtFruTuAgD5+tsXKh3sGiGH8lxgXx8oYdFM3X0D47gtpspqOjjdKWRprZejp04e93vIVJeHzeaEOJhIUSlEMIjhFj8qX1PCyEahBC1QojN4+RbfLIGIcSPrnLsciFE2Wct43Q9erUxKm60qxAaGorb5SLt4a9gb2pFRoQzd2Yqow0tRKxdgX+gAbvNTtCcdDQ9vTR+nINWp0O63Iz09hGzdBGh61ai9Ug0oSHoYiKxn8xDo9URuWwR1sEhAubNYax/kGkL5qOPDCcgMx1nbh7hCXFIf39aT58l1E+PJiYCl91GQnoagXPS6PlgD21dndg1KsKTk2ju7sKt11FeWkrM9ETW3n8fDimJCwyir7eXb3/724SHh5ORkUFjYyOlpaWsWrWSd999F6vZzL533kOoBQMdnTQUXiI5IZGdO7aTnJzMyy+/TEpSEtu3P8KpU6eIi47ivrvuwjZiwmQyce8999DT3UPqjJk88uijNDY28uN/+jEZ938Fccd6hFbL+m338ev/72mcHd1kr1tL08gYusy5zL5rIwmGEEZGR5m7aSOVx04iYuNZce/dSOnBcqkKq5+GuOwsMiJjGNJrUGm1PPCNx6nu7UITGU5LfSP9PX0EhAYTmTyNGWlpJCRPp6GknJiYWAoKCjhw4ABBwcH88pe/5GuPP8H+w4cYHBjkb//++2iEZMniRVhtdqyjw2zdvJqG2gpmpsSxYc189BrJ1o2z+fXze2ht7eDN9yE9JYxZyRF43B5WZcdT39xHT1c73/2rrWiEE/OYnXlzZvDWO3tYkT2TQ7ZRGhrq+cnfbiEqIpA3Pizm7nUZzJ8TQ0ykgb37NOi0Wu5eFIpcFEJR9QDt/U4iwkPYmB1JoF5NQ6eJPfsLKCzvYPGiBWSlx/KN7Xeg1/uREBtOUKCebffeRWJCNCsWZ2By5BIcasTjsqHByQMbp/POARsLZ4WQHAMzpgVyIq+fpKQE1q/MpKN7kDmz4sicFYla2tFqBHcuj2JooI+02AQsY6PMSY1m6SwdWrXEZonHXwub5odQVK/CYnWzNiuMjgEnq+ZFkp7oT0OHmQs1I9g8Ggoru8iaGc2WJZHYXMPERk5jUbqe///tCmZMj+JoqZb2vlH6Bk08vC6RE+UmNi9LIDFaQ2SQYNScSGQQpMfqiAiMIT5c8OrBMuanJZBb7ofT5aLfaOJM5TBtvaNEBPsxIz4Qg7+WUbuGpNk3IjfadYtGq8D7Zv8fxguFEHOAHUAmEA/kCCHSfLufB+4COoCLQoh9vvDmT3M5Aed3fH/f8P3dibd68oRQnmyugkqlInrzekYulmLu6sHd3IZ/ShIhK7LpOpyDKjqCiDXLGC4qwdbeybxvfxPpdILHQ/r2B1A7ndiGR/BPSkAOj+DoH2Du9q+iHjZhLqkgMnse1q4ebOXVxG5cg93uoO/cRQJTk7FarFiNRqbdvRGdwUBsVib+AQFIoOl8EcuefBz7iBm/6CiiNqxmsLOb6PSZJC7NRu8fQGH+GTIWzGPAOERERASrVq2ipKQEgOPHT5CcnEJhYSEREZHcd++9LFu8CMeYle//3fcwm8w88cQTHD58GLfbTUhICKOjY9TV1WGxWPjpT3/Krl3vk5iYwPbtj/Dhhx+i1+vxeNxYLBZOHj/On175Ew2VVRgCAoiNiuSDl/7EP/38pwRr/TB295KcOI2nvv/3VH2cS/L0ZB585GEuvreHOTHx/PCXv6DhxGnMF8u4f+ejCLuDltzTbNh6H6O9A7h6+8lYvgStw4lW70dkVBTTZqehsTlY963HOXr0KKMmM5nzsujo6mRoaJh//uefsGXzZnJzc9mzezeXLhbicbtYvGQJDQ2NNDQ0kJwYxaKFmZRX1jI7LRmHy0N5VTNareDV944TalAzPyORNUtn0NJuZNPKZB69bw6HjpfT3D7I8uwZ7D+Yz5qVWSxekEpnzyAP3rsGjxQszUogMy2e9p5RBowmEmOD2bRmFsXVA3xwqJwHN84iK1nPntN9DI16qG83kTE9iIdXR/Lx+V7eP9XNPzy+kJjocDbdsZDwEC1Op5t39p5i692r6RsYoaahg+0PbqSiugUpJVKqCQ4Ow+lwERmqo6VzhMgQHWsWxnL2YhNOl4cFWTPx1/txMLeMnffNpaahh/cOFLNpZQLDo3ZsdhfhAQKrEzzCj0fWxXK8zMypMiPrskII0Ng5UTrMmEPNzo0JFDdayE6PoK7LQWGNkSe2JCP8gggL0vGdr84hIjQAk02g8QvE6bDR0DXGE/dmMDPeQHyYmmlRgSQlRDNocpCeGMDyjDDKWp0cKx5my+JQmrotnCwbZHqEijPVVtZkpxIaHMCqNDVup417ViSwIDWIOxan4lbpiAjVk1NiYt3cQGKib0AGAXl93GhSymopZe0Vdm0D3pVS2n3vxTQAS31Lg5SySUrpAN71tb3SsVullK3AKinlD6SU5b7lR8DmK/W5EoqxuQrGgX46j5wAtaCnrpHupmYsF0vx1DXTXVvPWFMrlpJKtIYAzJ2dBPf0o3K6MHV24axvxtTagam4nICZqYzZ7bjau/BPSsA6YsLfYCB8Tjptx05icdixFxQR76dnqLgcv2Ez7rExjM2tiO5+ejs6Oflvz+DUqhm1jNHf0kr/pTIaiosx1tTTd+Y8lfV1nNq1l3n33EX9QA9njh7j/Kl8Dh06SGNjI2fPngUEb7/9NllZczl58gT7Dx1meNRM78AAzz77W3Q6LfX1dZw4cZzk5Ons3LmT3/zmGWJiYunp6eZXv/oVUsKxYzkcOnSQ6uoa9u3bR1FREd3d3Xz1wQf5/fP/xYoVK+jr6aG/s4vSoiKsQyPUl5VRe6mE9PhETr79HmrzKNU5J+m+VIYYGma4soah8mo0JjPdF4oYLKvEMWLi9IFDtFbXMNTWQc67u6jOO02gfwBlp/JpK6uidv8R/KMi8A8JprehmcI9+xlz2vnJd/+OusoqcnJzKSg4S2BgIMahIbZv344hMBDr2Bj5p/J4+403KS4pJefYx9yxZilLFy/gRP4FggP9mZmcwLN/2MVb7x3knnVp6HQ6vvHQcl5//xQXS2o4klfLB0cqGRjop7qmEZfLSf7ZCxw6ks/Fogp++7vXqW1oZPP6uew6XE5sZDDVjb0cymtkdbY3pdTQiBm18LB6YSJdQ7B9fRy7jzdSXtdDa5+DnDIrIQYdDa39fHSqnY7OXgyBeu5Ylswb73zIxaIKPtp/nH0HcwgJNnDyTAnLF8/mJ//6EhvXLuSxhzfR1T3A4qx4/vdLeRi0DhrbjazKCuK3r51hcHCAluYWSsprOXmxk+aWNqoq6zl8qp7IYBV/+qCYJWkBVNV3YrcYuVDVhw4zBRU95JaPMWzX8/HZJsbsHso7YH9eI/2DIySGuSmsG2HYbKe0upPzFT2UtdhxSTUf5bdTWduB3eXh4OlWatvHGBr1kF/SRWVTLy3t3RRUjRAd6KGlx4x1dJCO3lGaOk3MiHKTX9JLYbOLrUuCiY808JVlIewtMCKliqzkQHadbCdYayFQbeOfXiyhb2CYkyUDtHf2Xvd7yDXO2UQKIQrHLU/9BYaQALSP2+7wyT5LfjUMQojVlzeEECsBw0QHorjRrkJwcChBoaEEL1uIy+1B5fGgm5uOxl/PHJcLt9tDwIJM5KkC0lYvJzQtFcyjJMTHEX/nWjoLCik9mUdibSNiwEhvXz9hsbE4RkZwGYeIi4tFPWbhvkcfITginPoT+WRnLWD+A/dRsecg1unTiVi3grFBI4NaHTGh4VTkXyRxXgbLH3mQrshoTCYz6du2YOrr58Pf/BchJ/Oxjo1hNpu4/7EdhPsbUEtJdHQ0AwMDHDhwkJSUFFavXcuYzc5d993Lvt17WZidzTe/+U2sVis5OblcuHARp9NJdXUVYWGhxMXF4e/vz4oVywkLC8Nms2Gz2fj6159g9+7dVFVVcebMGcrLK0ibNYuUlBSCAw3MSEnG4XDy43/8R7q6uhgbG+P73/s7Ojo6+Mq2+zGNjBAeGsbw0BDP/OY3HD9+gk133cWQ0YjJZObJhx/B1NFJTEwsO3c+xnBvP4vnzCUtI4OavAKSFy0k+671FB87TsjMWWz52mMAvFLXxM7/8STnc3IxDY9QUVHJyPAQP//5z3nqqacYMZuJjY0hIT6B2Lg43n/zJV4SdtRqNTWVFZiHulm/ZgGL56fjck6jsKKD4rIawgJVSMcoa5eksGFZIgBv7h8lPMTAllWJ1NU3siwrmqT4MCymQXRa6O7sItBfcK6wkqiIEAaGrezXa3C5XFSU1xPgr0Yl3ZwqqMVuSSItzg+9JprMJD1JUVr2nuln8bwU7lkRw/96rYiO7iGCdTbSk4IwjqnYft8CujrbiA4PIXN2Ers+zKWjo4NLRcXYJzRfUQAAHZdJREFUHS46e7opqWzDT+0BlwPbGHT3OOhp7yA9LpXAGB0yNog7MjQM9QUhowLJnq4lr3yIpuZOCiIDsFrttPd7WJAaxLQof0oaTNy7JIz+ERuV0UGsm+NHS4+VhJhgFs0Kprx5jLZuI/rFUaSnRCOl/CR/2j6HHSnUJEd48Lhj2Lo0FICcEggy+BETBHllfSycGYSfVo3dKfDTaggy+FHWZCLY4IfDZuGZ97uICvdHqw6ntctIeLCe+m4NVouVbqOT5RmhNPY6WTEvlsxpWto0Mdf9HiKlxO2ecG60gatlfRZC5ACxV9j1YynlR5/V7UrD4soPGp830CeBV4QQIb7tYeBbn9PnvweilBj4bD78+DAvlV7APygIR0c3huXZGHPzibhzLZaSSqwOO26Tmfl334V/RBhtOXlEh4RiiIliBA8jLa0EqLQYrWPEJibgFxlOY1EpAQg8Lhczt27Cdb4UR4CeaYvmYz5XTEJqCocOHOD+bz9JYe5JbNMTGCssZePXdnDk5VdZvWQ5TU2NxK1ZhrOynlV3bmTPvo8Imj4NtWmUbssorp5+Nmy9l9HGFvQON1GRkYQEB9HV1YXb7SEw0EBTaysPPLqDl1/4PSEhIWQvW4rTl/fL5XIRHx9PX18/iYmJ5OXlodGo+cY3vsELL7zAkiVL0Gq1OJ1OysvLycjIoKmpmejoKGbNmsXBgwfx89OzdetWjh07hk6nZfv27bz66qu43W6efPJJCgoKUKlU9PT00tHRQWpqCnfffTfvv/8+69at49y588ydm0lHRwctLa2oVCruueduzpw5S2dfL5Hx8aTMnMGxY8fI3nYfdcdPkTong16zid7GJubPSqOxoZFNq1eTlpbG888/jxAqdH46oqKimTl7NjNmzuCVF19EI2BGUghxUf5kpk/nzV1HsdkdWCw2nnp8E+/sPsHw8CDz06Ox2ezUN3fj8cCOe9L46HgDq+ZHYxq1cSCvnf+xfQn7T7XzzUdWsj+nFLvVwtqlKew9XIyfBuxWCzqNZHlmJBHBGo4UDuF0SeYmaeg32mjqcaBWwwMrwtl33siK2UHUdLkZsXhwoWLD4jhaus0ER8TQ0WNmdnIQ+MczODjE4LCdGanJDAz0MXdWDN2DYxSX1qB2j7Jodii9PcN0D7m5Y34IH+Z18dXVEew9a0Sr1aLRaIgMESSEaRkattI95EAKNeEBHoIC/Wnrd7FyloZ9F4bxuOzckRVC54iK9n476zL9qWqzYHFqWZHuR1WbnY5BJ1uygyiotaHRqFg7x5+9BcM8uDKMnDIrkQEOLtQMMzvJQEZSEBabk/5RNVbLKN3Dks0LgzlX72DedA0tAxLjkIXIEDVaXQD9ZjfBOjcx4X409roJ8XMQFeZPaZOZaeGC0CAD9d0WFqYGUt/jQXisRIYG4J+0/rqXGAifmSrv/I9fTqjt+w/u/NIlBoQQJ4F/kFIW+rafBpBS/rtv+wjwM1/zn0kpN1+p3eecIxiv7Ri5lrEpbrSrYLVasVls9JWUg1aDSqUicPECeo6eYLC/H63DhWPASF9hKR3HT1N56gwjg0bC42IxNzQjh0z4O91YG1qQvYPYqhqw1DUy0tNL/Ly5VHx4iOnpaVi6e6nZfxSVhIIPD+BnddBy4gzCYqPho4No1Wpqj52kv7wGzaiFMJ2e8+/sxt8/gIDAQKaFR9GUf5Y5SxbRdr4QlcPJSP8geSdOMDY6ysqVKykoOEd3dw/33nsPFouV9vYO3nvzLQyGQKorqyg8d4GSsjK6urrYsmUL1dU19Pf3M3t2OllZcykuLuHo0aMkJSXx/PPP09jYSFNTE+fOncdoNGKxWHwlAMYYHh6htraGkydPkpOTQ2trG++9t4vBQSPV1TW8+eZbtLe38/rrb1BUVMTp06fp6enl8OGP2bJlC/v3HyAsLJTU1FTq6urw9/fnscce5eDBg+h0WmalpHBg9x5O5xxH55H81//8AS63C7fbTdXZAtKnTWfBkiUMtLfT19fHH//4R/z8/KiuriYoMIiP9u+jsqKcwwcPEhkdTUlxIWtWLiX/XDX7Pj6NkE6qqyoZNvbzxntHaG3vorW1k5FRF529ZiwWBxuWxrLrcDWxEQYGjGYulnfT2trNgdwqpscI/vfvPyY1wcD0hDB+9O+7mBalxmEfI8hfy71LI8kt6mf/mR5WZgQQHyo5XNBNn3EMp8tNfXMvJyrtBPgH8L/eLMNs9XD6Yi1+wkFF/SDmUSevvpNLV88Ao1Y3ew+ewemwMmTs5dXX3sI03M+JM2X84U/7SE/y5+470nhrfwVzkw1Yxkz85HcFxIbrOFttJVCvpaahnb6+fupbR7hQNUBjn4szJa2oVBq6R2D/6WZKa9rIKRslKiyQ7gELNd2S9n47EcE6hsY8HDzbgs3h4lSllX15Dej9tJyrd3OqqIXOPjNn6xyEGSR/2NdC/8AAnUOCUauDyFADpa1OLjZ4a9M09jjo6R+hpE0wMGzj9x824HKrsUsNx4t6sDlcNLX1Y7K4cNhGqaprI7eoh0u1g0iPhw/z2ylpGMZPZefIpQGcLifTI9Xkl3RSVFx+3e8hHukNEJjIMknsA3YIIfx8iTNnAReAi8AsIUSKEEKHN4hg39UO5DvGY8B3ge8JIf5ZCPHPEx3ILW9sJhq+90Xw0+sJmZmMw+Ggq7YeU3kVjuZ26gsugt1ByJplJGVmMH3zHeiiI0lOn8XmbVsx1zVRdvgY0QGB7PzWN5k3bz737XyUjHnz2PqVB1i3bh0RDhdt54ow1jXiGRyC/kEe3nw387OyWJSdzfbHHuOxhx5GDAzx0Nb7iTUEsm3rVlYsX87fPPlXzAgKpaO8kua8s/RV1cCImcbcPOL9A1m+IJv0yGgi/A04HA5yc3MZGByksbGBAwcOUFpaSm1NDbPSZ/Po159g0dIlbH3gKzQ0NVNf38D773/ARx99hE6n5fDhw+Tl5TF79my2bNmCzWZn7twsHnroIYaHR1izZjUrV67k0qVLFBdfwmKxkJKSwrJly9ixYzsLFsxn5coVbN/+CGFhoSxatIivfW0nq1atIisri7Vr17Jz506ioiLZuHEDBw4c5OjRo9TV1bF374c0NDRQUlLMrl27OH/+AsXFxeSdymPNihV872/+mjvXriMjOZmvb3uAZP9AWorL6Gxs4oVf/wdWi5WEhASefPJJbDY7a9euIcAQwIwZM9lw9xY23nM3aNTExCfw9u5jSKHjtTd2kxqn4h//Zj3zMhJ5aEsmUSEqFs9PZVFGMA6HnZKKJi6U9XP6XBVjw8O4rRZ0wkNacgT3Lg1HZTdRUVFJYVElJSWVzJ0RTUqUhprGPqobuzlVPorL6aSqvouieivF9UasVhur5oaj06jISk9kfaYfIX52kuJD2ThPz33r0gky6Fk710BcsIuVWXFkp/oTKoaorapG7xlCOszMmRHDluURZKWoCdA4sJmGOXm2GbNpjIMFAzS2GVmzKJlV6f4sn6nGODLG4swkHHYbZpOJexaH4XbamZMaw7o5Wu5dFERKfBgOh5uFMwxsyNKTlZ7Aytl6zlxqpL51gMLaQdKTve6w6RGCxNgQNi0IYGWahnWLk5keG8Tq2XqmRWioau4lJNDAfUtDmDMzjgCtiyP5tVitFlJjBNERQcxNT2TdHC0hBjXZmdNYk6FDJWBxVgpLZ+rp7BtBr3Hjp9MRZAhg0ewYHlwdQ1iQhrTkaB5cHcWC1FCsFhsHTlbTNeTi/hUxzJ2T9vlf+kngemR9FkI8IITowJuV+aDvCQYpZSWwC6gCPga+I6V0++rSfBdvEbRqYJev7dX4CG8QgQsYG7dMbIy3shtNCKEG6hgXvgc8+hnhe8AXdKMFBODsHUC/YA7GvALsGi2BiXGIASMaKUlatgh1Zx+qIAPZaemUHc/Dz9+fpPh4Ri0Whk0mMlavoOniJTY99CB5OTmM9g8g7E423nknJefO43a5SEpKIjklhfzTp7lj21Z2vf4GMzNmExgairmji02bN1Nw9izdHR1svPNOykpL8Xg8xMbGUt/aQndPD4888ThnT55ioKeHxx7Zzun8fIKCg5EeD8PD3gSTQqWmq7OTwNBQ9AYDPV2dxMbHoxMqhgYH8Hgk0dHeTM12u52QkBCqqqpJTEwgODiYkhJvhuVly5Zy7NgxVq1aRW1tLQ6HE4tljG3btrF374fo9X5s2LCBnJxcAgL8WbJkCR9//DEPP/ww77//ATt2bOdnP/s5//Zv/8qbb77lTWL56mtERIQzY8ZMLly4wJo1q6mrq8dqtZCYmEhTUzNBQUEMDg7wwAMPsHv3HubNy8LfP4ATJ45zzz330NnZSVNTEy6Xmx07tvP662+wY8d2jhw5isPlZOOdd1FaVYnJbCI6No72uiqWLMjkfP4RxoxtWG0ONt2RSWWTmd7ePratieb4pRFWL4xm78cVqIUHtQrSYgROVQBOpwO9Tkd9h5n12VHkFvbjdjuYMyMCj0fQ0D5CaqweFZL2ARdLUtWcqbUhhIrsVA01nR7MVhcJYRAW5Edp4wgrZgdR2y3RqyzUdth4dOM0jhSPsmlxCLnFJu5ZHEJRs4u23lHmJfvRPaoiRK/FZhkjLSOZ/IsdZM/QY3drKKzqQ7rs3LkwjKp2J8aRMe5cGMahwhE2ZYdwsdGFy+XCYrWxcX4wl5qcZCaq6DRKBkwO0hINNPR6cDusxEcF0NbnRK92MD1GT/ughzG7ZEGyir5hQdugkw2ZfpS2uekxWtm6NJSTVQ7mTYOLDQ7sNivzUwNp6JVYbXYSwjUEBejoHXFjs9lZmh7E6SoLqdEC1H7UdZiJC9WQEBVAUb2Z8CAVs+INlLU5mRbmQaXRUddpZ0WalroeydiomRUZoZyqspA9M5CmHhd9w1bWzAnCGnHHdXejhaQmy9X/OrEf/4cee/Jmr9RZIaWc+0X73+pPNhMO3/sijI2OMtbUhlujob+tnbbdBwhIm4HLZgW3GxkWTP35QloO5xKWlIhlcIi9v/sj85YsJjQsHI/LTUxkFE1V1Xzw3AukZmTQVF3D6X0HmZk0nexFi3jhmf9kwfz5BAYGkp+fj81mIzMjg3/50dPMy84mIiKSD17+EympqTQ1NXHgo4+IiopiZGSEnNxcLJYxAgL8yT95iumpM2hrbiYv9zjT4xPo7Ozk9OnTNDY0EB0dxYULF6hvbCIiIoLe/n6SkpLoam/n1IkT1FVUEhEezsWLhQwNDREeHs6RI0epqKhAr9dTX19HaWkZer2e5uYmuru7MJlMtLW18d577zFjxgyqq6sZHh6ho6OD3t5eOju76O/vp7Ozg9raOoaHh1GpVPzsZz9jyZIlNDU1YTAYqKurQ61W84Mf/ICkpGkkJyfz7LPPkpiYgM1mY/9+7wuZBoOB/Pw8pk9PYs6cTH74wx8yb14Wfn5+PPfcc2RmzmVsbIw33niTjIwMFixYwNNPP820adPo6OjgxMmTpKSk0tvXx9GDBxkcNKLVaSkrK+fAh++wIDOBUYuT+cka9h06x5Hc8xi0Dtp6xqitb+ePb58le0YA7d2DuJ12tFoNZ0q6qWzy/tJu7zbyxuFG5qUE0G8c5VxZHwath36jiXMVfejULtq7BnjzeA8ZCVoGjUY+yOsjKUJgGxvhYs0w0u0mWC95I6eDxHBBoEHPmN1Nx4Adf7WNZ3fVMStOS3OPlUP59aTE6NCotZy+2EqwP0SFB/Dca2dJi/fD5VHz9qFKkiNVzE028PzeBqKDJemJAfx2TyMxIWr6hh2cKW4mLkSSkajnmfcbiAySjFglx4t7sTtcOJxO+gbHSInVUdc6SEFpE0OjTjxuDxcquzFoHFjscLy4Gz9hp2fIRXFtP2o8tPXbaWrtY8/pfjIStAybbbilIDLITUFZB+UtZnRqSX2bkf4RB0NmF2bTCPkVI/hrPAiPndImE06nG3+1i+oWM1a7mxGTibzyIXQqD3qVlddzuogNgWnRATz3UQuRgQKbzcXAiI0Fyf4cON9HfUPrX+rWcE1MoQwCZ4UQWV+0863+ZPMQsEVK+Ve+7ceBZVLK736q3VPAUwBJSUmLWlsn9qGz2+2YzWYA3G43KpUKIQQejwchBEIIHA4HADqdDo/Hg9vtRqvVftJHrVYjpcRms+Hv7w+Aw+FAp9P9X+tOp/OTvuPXP6v9l1n3eDyoVN7fGhaLhYCAgKuO52rH1Gq1CCFwOp2o1WpUKhVuX6EqtVr9Z9fF4/Hgcrk+6e9yudBoNBM+37Vel8+6puPPe63X5WrjuXwtxq+7XC5UKhUqleoTd8nl9c+6Lteq80Q+O5/V5rOuy/j1y59l8M5lXv4sT/S8Go0GIcSfHcdb/VT9yWdESvl/fV6klDidzi98LcafT6/XExgYyLXwZZ9sglOmy2W/+PGE2uY88e2b/cmmCpgJNAN2vJFuUko5byL9b/XQ588K6/tzgZQvAi+C14020YP7+fnh53cD0pIrKChMGaZI+QCAu79M51vd2HQA08ZtJwJdN2gsCgoKCn/G5Wi0qYAviwBCiGhAf639b/U5m2sO31NQUFC4blyndDXXAyHE/UKIerxutFNAC3B4ov1v6ScbKaVLCHE5fE8NvDKB8D0FBQWF68Ll4mlThH8BlgM5UsqFQoj1wKMT7XxLGxsAKeUh4NCNHoeCgoLClfiy79DcRDillINCCJUQQiWlPCGE+PVEO9/yxuZaKSoqGhBCXEsMZCRw/evJ3lgUnac+t5u+8MV0nv5lTijllDI2w0KIQCAPeEsI0Yf3Bc8JcdsZGynlNeUZF0IU3szhiJOBovPU53bTF26UznIqGZttgA34Pt5aNiHALyba+bYzNgoKCgrXCyklrqkTjTY+Nc1r19pfMTYKCgoKk8it/mQjhDBz5fIDl1/qDJ7IcRRj8/m8eKMHcANQdJ763G76wg3QWUrvuza3MlLKoL/EcRRj8zn4sg/cVig6T31uN33hRuk8peZsvhSKsVFQUFCYRBRj40UxNgoKCgqTxFQKEPiy3OrpaiaNySzKdj0QQrwihOgTQlSMk4ULIY4JIep9f8N8ciGE+K1P1zIhRPa4Pl/3ta8XQnx9nHyREKLc1+e3QogrJUW9rgghpgkhTgghqoUQlUKI7/nkU1ZvIYReCHFBCFHq0/nnPnmKEOK8b/zv+dI5Xa62+J5v/OeFEMnjjvW0T14rhNg8Tn7TfReEEGohRLEQ4oBv+6bU9/J7NpNdPO1WQDE2V0B4i7I9jzfL6RzgUSHEnBs7qmvmVWDLp2Q/AnKllLOAXN82ePWc5VueAl4A700a+CmwDG/toJ9evlH72jw1rt+nz3UjcAH/r5QyA29aje/4/m9TWW87sEFKOR9YAGwRQiwHfg0849N5CHjS1/5JYEhKORN4xtcO33XaAWTi1el3vhv6zfpd+B7eCpOXuUn1lVMmN9qXRTE2V2ZSi7JdD6SUeYDxU+Jt/Hd8/GvAV8bJX5dezgGhQog4YDNwTEpplFIOAcfw3szigGApZYH0FkR6fdyxbhhSym4p5SXfuhnvzSiBKay3b+yjvk2tb5HABuADn/zTOl++Fh8AG31PZ9uAd6WUdillM9CA93tw030XhBCJwL3AS75twU2qr0R5srmMYmyuTALQPm67wye71YmRUnaD98YMRPvkn6Xv1eQdV5DfNPjcJQuB80xxvX2/yEuAPryGsREY9tWZhz8f5ye6+faPABFc+7W4kfwn8APg8h06gptV3+vkRhNCPOxzo3qEEIvHyZOFEFYhRIlv+f24fdfVJawYmyszoaJsU4jP0vda5TcFwpu/aTfw91JK09WaXkF2y+ktpXRLKRfgree0FMi4UjPf31taZyHEfUCflLJovPgKTW8SfeX1erKpAB7Em7fs0zRKKRf4lr8eJ7+uLmHF2FyZqVqUrdfnCsL3t88n/yx9ryZPvIL8hiOE0OI1NG9JKff4xFNebwAp5TBwEu98VagQ4nK06fhxfqKbb38IXnfrtV6LG8Uq4H4hRAteF9cGvE86N6W+l6PRJrJ8GaSU1VLK2om2vxEuYcXYXJmpWpRtH3A5surrwEfj5E/4orOWAyM+d9MRYJMQIsw3Qb4JOOLbZxZCLPc9ej8x7lg3DN9YXgaqpZS/GbdryuothIgSQoT61v2BO/HOVZ0AHvI1+7TOl6/FQ8Bx381mH7DDF72VgveX7gVusu+ClPJpKWWilDLZN5bjUsqd3KT63iRzNim+yL1TQog1Ptl1dwkr79lcgalQlE0I8Q5wBxAphOjAG131K2CXEOJJoA142Nf8EHAP3klSC/BNACmlUQjxL3i/gAC/kFJeDjr4G7wRb/54q/VNuGLfJLIKeBwo981hAPwjU1vvOOA1XxSVCtglpTwghKgC3hVC/BIoxmuE8f19QwjRgPcX/g4AKWWlEGIXUIU3qu87Uko3wC3yXfghN6O+15auJlIIUThu+8XxWQ+EEDlA7BX6/VhK+Vk/erqBJF8dmkXAh0KITG6Ae1TIWzxvj4KCgsLNijomSuof/eqE2lqe/UPRly2BIIQ4CfyDlLLwavuBTuCElHK2T/4ocIeU8ttf5vxXQ3GjKSgoKEwSN9qN5nOzqn3rqXjdhU03wiWsGBsFBQWFyeL6hT4/4HOXrwAOCiGO+HatBcqEEKV43zP660+5hF/C60ZuZJJdwsqcjYKCgsIkcb1yo0kp9wJ7ryDfjTc680p9CoG5kzy0T1CMjYKCgsKkIZG3QXaAiaAYGwUFBYXJRCrGBpQ5G4XbFCGEv++9A/U19PmuEOKbkzkuhSmGN0JgYssURzE2Crcr3wL2XH63YoK8AvzdJI1HYUoiwTPBZYqjGBuFKYUQYonw1qbRCyEMvuSEV5oE3Ykv1FMIcYfvKWeXEKJOCPErIcRO4a0TUy6EmAEgpbQALUKIpddRJYVbGQm4XRNbpjjKnI3ClEJKeVEIsQ/4Jd63/N+UUlaMb+NLQ5IqpWwZJ56PN4GlEWgCXpJSLhXeAmx/C/y9r10hsAZvahMFhc9B3hYusomgGBuFqcgv8KaasXFlt1ckMPwp2cXLZQiEEI3AUZ+8HFg/rl0fMPsvOlqFqY0SIAAoxkZhahIOBOItJKYHxj613+qTj8c+bt0zbtvDn39P9L7+Cgqfj7w95mMmgjJnozAVeRH4CfAWvjLA4/FV31QLIT5tcCZCGt7aIQoKE0OJRgMUY6MwxRBCPAG4pJRv4832vEQIseEKTY8Cq7/AKVYBOV9iiAq3G9IzsWWKo2R9VrgtEUIsBP6nlPLxyeyjcHsjwkKkWL9iQm3l3iNfOuvzzYwyZ6NwWyKlLBZCnBBCqK/hXZtIvO45BYUJo/yg96IYG4XbFinlK9fY/thkjUVhiiKV0OfLKMZGQUFBYTJRjA2gGBsFBQWFSUTeFpP/E0ExNgoKCgqTxeVEnAqKsVFQUFCYPCS4ryXX69RFMTYKCgoKk4XyZPMJirFRUFBQmEyUORtAySCgoKCgMInI65KuRgjxH0KIGl95jb1CiNBx+54WQjQIIWqFEJvHybf4ZA1CiB99qQFMAMXYKCgoKEwWkutVPO0YMFdKOQ+oA54GEELMAXYAmcAW4HdCCLWvQu3zwN3AHOBRX9tJQ3GjKSgoKEwa8roURpNSHh23eQ54yLe+DXhXSmkHmoUQDcDl4n8NUsomACHEu762VZM1RsXYKCgoKEwWo9YjnC6JnGBrvRCicNz2i1LKF7/AWb8FvOdbT8BrfC7T4ZMBtH9KvuwLnGvCKMZGQUFBYZKQUm75Sx1LCJEDxF5h14+llJdLnP8YcOEtrwEgrjQsrjyFMqlJ3BRjo6CgoHALIKW882r7hRBfB+4DNsr/zv7ZAUwb1ywR6PKtf5Z8UlACBBQUFBRucYQQW4AfAvdLKS3jdu0Ddggh/IQQKcAs4ALesumzhBApQggd3iCCfZM5RuXJRkFBQeHW578AP+CYEALgnJTyr6WUlUKIXXgn/l3Ady6X1BBCfBc4AqiBV6SUlZM5QKV4moKCgoLCpKO40RQUFBQUJh3F2CgoKCgoTDqKsVFQUFBQmHQUY6OgoKCgMOkoxkZBQUFBYdJRjI2CgoKCwqSjGBsFBQUFhUnn/wBJcLl1L7uFqQAAAABJRU5ErkJggg==\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "child.quick_plot(\n",
+ " \"land_surface__elevation\", edgecolors=\"k\", vmin=-200, vmax=200, cmap=\"BrBG_r\"\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": 10,
+ "metadata": {},
+ "outputs": [
+ {
+ "data": {
+ "image/png": "iVBORw0KGgoAAAANSUhEUgAAAZsAAADxCAYAAAAdgBpwAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4xLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvDW2N/gAAIABJREFUeJzs3XeUXNd94PnvrVyvcuhQnXNudDfQaGQCIEASzKREyhrZchjP2NbKZzxnJ3nGs8c7M8ez9njsGWvHtlaygmlZFJUoSswAASLHBjrnnLu6qqsr53r7RzdpeEyRIIkmTel9zrmnXt+6L/Uf91c3vHeFLMsoFAqFQrGdVB/3BSgUCoXiZ58SbBQKhUKx7ZRgo1AoFIptpwQbhUKhUGw7JdgoFAqFYtspwUahUCgU204JNgqFQvEJIIQoFUKcEUIMCyEGhRC/s5XvFEKcFEKMb306tvKFEOJLQogJIUSfEGLnx3n9SrBRKBSKT4YM8K9kWW4E9gJfFEI0Ab8LvCHLci3wxtbfAA8CtVvpN4C//Ogv+e8owUahUCg+AWRZXpZl+ebWdhgYBoqBx4G/3ir218ATW9uPA8/Im64AdiGE5yO+7LdpPq4TKxQKxc+6QqtKTmXurGwgLg8CiduyviLL8lfeqawQogLoAK4CBbIsL8NmQBJC5G8VKwbmb9ttYStv+c7v4O5Rgo1CoVBsk1QGjtXfWTX7/Z50QpblzvcqJ4QwAz8A/qUsyyEhxE8t+g55H9v7yZRuNIVCodhGKnFn6U4IIbRsBpq/lWX5h1vZq291j219erfyF4DS23YvAZbuxj19EEqwUSgUim0iBGjV4o7Sex9LCOBrwLAsy39621c/Bn5la/tXgBduy//lrVlpe4HgW91tHwelG02hUCi20Z22Wu7AAeDzQL8Qomcr7z8Afwh8Vwjx68Ac8PTWdy8DDwETQAz4tbt2JR+AEmwUCoVimwjuXrCRZfkC7zwOA3DsHcrLwBfvztk/PCXYKBQKxXYRoFIGKwAl2CgUCsW2uZstm086JdgoFArFNlL/9KnJP1eUYKNQKBTbRAjQqD/uq/jHQQk2CoVCsY2UbrRNSrBRKBSKbSJQJgi8RQk2CoVCsV2EMmbzFiXYKBQKxTZRZqP9HSXYKBQKxTYRKBME3qIEG4VCodgu7+Mlmz/rlGCjUCgU20SgjNm8RQk2CoVCsY2U2WiblGCjUCgU20jpRtukBBuFQqHYJkIZs3mbEmwUCoVimwjubGG0nwdKsFEoFIptpLRsNinBRqFQKLaJ0o32d5Rgo1AoFNtIpUQbAJRJeQqFQrFdxGawuZP0nocS4utCCK8QYuC2vP9bCLEohOjZSg/d9t2/F0JMCCFGhRAPbNMd3jGlZaNQKBTbRCDQ3L331XwT+F/AM/9b/v+QZfm//73zCtEEfBZoBoqAU0KIOlmWs3frYt4vpWWjUCgU22RzzEbcUXovsiyfA9bv8NSPA9+RZTkpy/I0MAF0ffA7+fCUYKNQKBTbSKVS3VH6EH5bCNG31c3m2MorBuZvK7OwlfexUYKNQqFQbKP3MWbjFkLcuC39xh0c/i+BaqAdWAb+ZCv/nZpK8l25oQ9IGbNRKBSKbSLEnQ3+b/HJstz5fo4vy/Lqbef6KvDi1p8LQOltRUuApfdz7LtNadkoFArFNtrObjQhhOe2P58E3pqp9mPgs0IIvRCiEqgFrn2oG/mQlJaNQqFQbBMhQKO5O7/phRDPAkfY7G5bAH4fOCKEaGezi2wG+E0AWZYHhRDfBYaADPDFj3MmGijBRqFQKLaNQHzYwf+3ybL8T94h+2vvUv4PgD+4Kye/C5Rgo1AoFNtFKG8QeIsSbBQKhWIbqYQyNA5KsFEoFIpts9mNprRsQAk2CoVCsX2UbrS3KcFGoVAotsnmbLS79m60TzQl2CgUCsW2UbrR3qIEG4VCodgmAu7a1OdPOiXYKBQKxXZRxmzepgQbhUKh2CYC8TMx9VkIUcLm+jiH2FwfJ87mq3FeAl6RZTn3Xsf4uQs2brdbrqio+LgvQ6FQfAJ0d3f7ZFnO+8AHuIuvq/m4CCG+webyBC8CfwR4AQNQB5wAfk8I8btb6+38VD93waaiooIbN2583JehUCg+AYQQsx9qf34mxmz+RJblgXfIHwB+KITQAWXvdZCfu2CjUCgUH5n3t8TAP0o/JdDc/n2KzZVA39W2hVwhRKkQ4owQYlgIMSiE+J2tfKcQ4qQQYnzr07GVL4QQXxJCTGytOrfztmP9ylb5cSHEr9yWv0sI0b+1z5eEuIO1VRUKheIjJNTqO0r/2AkhHhFC3BJCrAshQkKIsBAidKf7b2f7LgP8K1mWG4G9wBeFEE3A7wJvyLJcC7yx9TfAg2yuuVAL/AabK9AhhHCy+SrtPWyuof37ty19+pdbZd/a78Q23o9CoVC8L5uLp6nvKH0C/E/gVwCXLMtWWZYtsixb73TnbetGk2V5mc1lSpFlOSyEGGZzkOlxNtdkAPhr4E3g323lPyPLsgxcEULYtxYGOgKclGV5HUAIcRI4IYR4E7DKsnx5K/8Z4Anglbt1D11dXVy/fv1uHU6h+JlxeyfCT9u+faxCfdsvd43m76odrVb79rZOp3t722AwvH0sSZLePpbFYnl72+l0vn3cwsLCt7crKyvf3m5tbX17++DBg3/v3B+VT0Kr5Q7NAwNbdfT79pH854UQFUAHcBUo2ApEyLK8LITI3ypWzObNvGVhK+/d8hfeIf+dzv8bbLaAKCt7z3Gst62urd1xWYXi58nfq29kGcTmh0qwuZ0DWc6iEoJcTiaXy6BWCbJZmWw6hUYjyORkMimBVqMinc6R0qjQa9Uk01niERUmo5ZEKkMkqMIq6Ykm0/hWVOQ7jcgyLE6nKCu0EU+mGe3L0FyVBwJ6Lr1EV3MROVnmJ9/5Cx4+WINk1OKd6eUzv/o7H+0/SqhQafUf7Tm3z78FXhZCnAWSb2XKsvynd7LztgcbIYQZ+AHwL2VZDr3LsMo7fSF/gPx/mCnLXwG+AtDZ2XnHUfnP/vIv+KuBm2iSaQIrKxhsViwV5aSnZsjazBgNBnzTs1iqytHEEqQCQdLpFO4jBwhdvE46ncZ19AAb566gra0C7xrSjiZWL1zFLEmYdrYSGhgmterDsbuDyR+/TPWTj7Bx9RbGplpUgRDxFS+GtmayswukJQMarQaxvoG1q4PQrQESqz6cxw6RHBohurqGuXMH4cExzAX5pHNZDFYLocFRnAf3kBoYJR6PYWluJDk2RSwUwnHkAIn+YdKZDJYSD6HJaVRGI+bSYkKjEzjv2Yvv5FlyKoHJasWyZyeR670kQiGktiZyy17iPj+OPbsIXO1GX1mOKrBBLp4gZ7VgNJtILi6T1mkxlZawPjCEobAQg2RifW4WZ/sOQpevY2xtJOPzI4IhzJ1tJOaXSC6vYmtpZPr1M1ScOE5gaARTXQ3p0UkyDhvJQAB3RTlh/zpyTsZsMRPPpDHZbUQGR7Dv7yQ9MknE58fS1UF2dY34yhqW5gbqwgl27NjB8sws5nQY/+QVHt0tcWEsSzAU5WCrg97pOEZ1Bo2cYcGXJJvN8WiXldP9MfyBKE/uc3C6P06FM8OkVyYcjbK71kL/QpZ4LEpdsZmMMDC7HCQnq3hin53XeqKkU2ke2GXntZtB5JzMw7ttvNGfpL4IVoJqAuE0Bxu0vNEX40iLgTdu+mmpyWNkLkxDiY5kVs+qf4OdNTZO98d4cq+Nb76+xImufLonwpzosPHC1SBP7LVzdTxFIJLi8T02LowkicQS1JeYmFyKo9Np2V2jo382zpw3xaf32zk3nGZtPcoT+2ycGUwRjsS5v8PC5fEMwVCcx/fauDSeRa9OYTIaWFrPEI7GeWKvnTMDSQQ57mnSc3k0QTqTpqnMxKV+L2qNkRO7rFwaTVFTIDO2nCMaDZOWtTy5383J3jiJRIrH99l5oy9OnimDwaBnajVFOpXkgZ02zgwkqPeAL6bDoo4xNB+jq9FF92ScI80mrk+mONIq8cxrsxxuc7HgDfPArjrODsZ4rE7PmvYD/SD/UITgk9JFdif+AIiwOe1Z9x5l/4FtnZMnhNCyGWj+VpblH25lr761bvbWp3crfwEovW33EmDpPfJL3iH/rrLUVpMx6AjOzKEp8jB38k3CyTiWuhqi/gDpUBid281C/wCrc3OYWhuJ9A2hspgxNNQQm5hBazFhLiogY9QTGRjGlJ+H7LCxcb0HtSRhP7CH8edfBLWG8K1+DC47pDOMnn6TaDRKZs1HLBYjMjSKraaSrEGP91o3aqcd6/5O1s9eJKPVgCef3i9/E1tjLemVVVjzYygqxH3sEOvnLoNK4LpnH8HeflKpJNb9XYx8/W9Rlxdj391BeG6RiH8dZ1cH4clp4qEga6+/ievwfnR6PfF4jLXeQdTFhVi6Ohh85jm0ZcW4Du8nfKMHvd6ApbyEwKqXpYkp9OkMU2fOsba8gq21iXD/ECaDhK2xjsjcHCKWQG8xY923m7HvPk9gbIJgPM7U93+Cr38Y+642MEnkt7eSGJ3AqFIjuV2oy4rwXbuBZ/8e4pOzqIMh3O0tRGbnYdmLsaiAvPsOs37hGolEglx+Hn1f/WuyGjWm1gaC/YNMj4ziX17myKMPo9dpuee+xznV7SPfoeVTx6v56g8HmZ5dRqVWMzi7wdj0MhX5as70+vFY4ViblfPDUSRtlupiE7UFMtf7F+meihMKR7k+sMTYYpTuwXmWvGHuazdxeTSByaDl4U4b54cTmCSJ+zss/M2pJcrcsBGF64OLTM97GViQsRllvvLiLA2lJkbmIuj0ehpKLSz5YmxEVbhteh7rsvDC1SAt1S76ZtNYTRIGvYZP73fwwtUgWo1MZ7WOCyNx1CJLc4mW505N0j28yPpGmB9dXKG1TM+xHRJnBuKoVTIPdVo42RNFpVLxxF4bL3eHEAge7rTwak8EvTpLZ42Z2SU/wxPz3Ndm5mRPFINWkEzEeOHSGl01Oo632bgyHCaRyvJol40/+d4EZm2cRFpGp0pysXeJA3UGzg3GsRgF97aZ+PJPZqn3CCyShp9cmGVu0UtzqZ5vvLZIc5mWKo+JXDrO2YENHtvv4fp4DJdFj8OqpzJP8PwFL8c63PTMJPHHNBQ6DTx9wM6PrwUJhsJ3u3q4A+JnZoIA4JRl+VOyLP++LMv/6a10pztvW8tma2bY14Dh/62Z9WM2B5n+cOvzhdvyf1sI8R02JwMEt7rZXgP+622TAu4H/r0sy+tbsyH2stk998vA/3s37yGRSBIdGCKdSLCx5qMklUIYdUQXV1g+dxGdSs3a1AyupWUsRUXI0RiRwVGmr9ygrL2VnHeNlf4BSg/uI9c/hDYHU5evY3I6KKgoY/ZmD2a3G2c8iTUvD41GzeTVbvJqKnE47ZS1NKOzmNEWFRJaWmFtdh7HpRvotVpG3zhL05F7YNXHyug41niM/K6dVO1sZ/blU+j0BqKRMDpJgmwOtU7LdG8/JYkEcjbH/OgwFTYb8XCI1avdGPQ61ELFyugkzivdSCYTw2fO0nzkMLGRCULeNfQVZSycepPGg/sIxmLY8/NYOnsZh8NOJLBB0LtGUTIJyRQl9bUYO1rI868j9DrWLt9AlcsxNzxClUGPJEmM9g1gkCSSGhVlrS1ILgf6+mqGvvFtVBo1qZEJ/CtrxKIRMtEoci5HhUqF0OsI+QMEzl4iHgwSCmygs9vQGPQsjY4iXTOTTqeJbASJzc5R+dgJHAUFpKIxEqEwiUSC4fFJ8irK8D33PdZH+kjG6nn92gqHhIWx+UXcniIaKhwc6ipiZ1sZf/gXp5n25Th3ZYZHjjThjci8dmmKpqo81oIpjJo0ZouJ+9qtGLRqkmmZlkobeVYbf/zsCOeG3dwcnqe8wIxe7WRi2ks0kSKXK2diPoBeI/PovgJmPQ5MRj376/UEI4IbI4LRhSiJVJahqSgOUyVWs5Ebw5O83G1AlmVml9ZZWAaZzS6peNJFNpNhfjnA0qpMtqWMy72z2C16bK15NFR7EAiK3TpeeHOU4kI3KqFiZGaVRDzJejCP5bUggVCcSCQfvU7NjYFptNoaBsdWsJh0rPiMpFIZjEYdp2/5mFlNoFZlaakroWdsku58ByqRpNCp49lry1itdtTIRBMyJW4Zi0HQXFPA+eEIw9Pr1JS5icTNzC746Z8y0FQmUVOeh9WkJZFMsrgW5scXclSWuFBrtEwvBnijP5+R6VX6Ulk0mhpyaOkZXSWZzieVyrAejOC0GEjnVMg5mUtXuvnlL9zNGuIOCIH42WnZnBJC3C/L8usfZOft7EY7AHwe6BdC9Gzl/Qc2g8x3hRC/DswBT2999zLwEJvztWPArwFsBZX/Arw1Uv+f35osAHwB+CZgZHNiwF2bHABgMOgxNtYRP3OB6ofvR2OSyC8pISUEtpoKVBYTiXAUtdmMeSNEyunAlO+mOp3B6ClAaq4nEw6TC4axdO1k42YftccPkw1F0Lc0UprOkE2lkS1mHLXVhJaWqdnTiRBgKy8l6vUTC4YwW0zoZZnapx5DFUuQiMdpefwRSKXJWkzUnjhGNhgmvuLFtnMHuoVlcho1Ym4BjAakpjoiZy5Q3tKEuasD36mzFNbWIOxWGh97CBJJdNWVhC5fY+e/+OckxyZJW00U1dfhOLSHjf4REpEounSK/PoahEGPSi0oe/g+gjd6Me/tRKysEj97EW11Oe50hsjsAsmbfVham4iPTeA5egDvmUu4SorRNDeyfuYchTtaMLW3Ik9OYaqtJjE6QepWP82fe4rE6ASmrg6ip85hL/YQj0TIptOo7Dai0Sgl+/dgrCxHnpxBpdFgaGogduEKzuIS9DsakAwG5HNZXG1NqJNpPG2tyNE41v2d+E6dw3TPARadFmIzizTXNvALn3mSXHyRVDLBp+5v4vWLU6z5AgghePnsFHXVhZzYV4jFpCeVTPPATivhWDV6jeDYDolXbqzzaw9WMLGcZtYb4bOH8zk7nGJoLsHhnR72N+pJpz3otGr21ptY9ttJpLPc06hFpa4nFIoiVGpsFgPBUAR/UHB2KMH/+XQN18aT+CNZ9uVZqPGoWViNUlFoZW+dgVO9YX7toUrO9YfIpWNkhY7jOyS0GsFJg45MVsZpTHNkVznheI5UMkVbpYl4PIFQwZHOSrzrMR7fa2N13YoQW916vQK1WsWJnTa6x8Ns5FnpqlYRT5SiVgvaSmFsFZ5uMPJGX5xKTQKTQYXHlqO0wEqxU1CZr2N4NsOhneVU5qlxW8tZCSRRqVSsRbT86v0lvN6boKvVSiyR4nibRDReTVG+Aa02R1WhhqnlGEeaHdy/z4RFmyKR01LhhrEpCwfqNKhVZXSUq7k4kuDELivJVC1qFcTiSfR6LQcajciyzAtXtRw4cORuVg937G61WoQQXwceAbyyLLds5TmB54AKYAb4jCzLga0f+3/GZp0aA35VluWbH/ISvgj8WyFEEkizOZQh3+mMtG3rRpNl+YIsy0KW5R2yLLdvpZdlWfbLsnxMluXarc/1rfKyLMtflGW5WpblVlmWb9x2rK/Lslyzlb5xW/4NWZZbtvb57Q86S+Jd7gH/6fM4jhzA2VDP4tkLaKvKcXa2ExscxX/5OkUPHGX53GW0VeW4WhvJzC0guZ3EV9dY7x2k8NA+zC0NdP/PL5OKJ9BXV5CNJwicv4xldxuiKJ/JH71ILhbHbDKxPDND1m4jMbcIWg3mna3MPP8SxqoKyGQZP3kG/8gYsk6Ld3KSzOIyxuoKSKXQhGMY8t3YO1qYP3eJrFrg9/sJXL6BUTJBLofv7GVse3ZhrK3Ed60bqaaK2NIqkevduI/dg84kkQwEyS2uUtBQx0bvIAaDDmthAQRC6Bx2fNMzLF7pJu0PYOnqINY3RGZiGs/Rg0y88DLJWIxgcIONqRn0BW5SkoHI4hIGqwVtWTGDX/0Gjnv2kX9gD4mxCTTJFLJKxdL4JP75BYRBTyyTITg7j1RURCoYxqDVYWmqZ31qmqVr3bgaaklOz2KQjLiOHsR39gKS20X+vYeI3hrE392LsbEOW30tQ8+/iMhzkFILli9dw9xQg8FqYfn5l7jngfuIaLR8+ctf4b59ZZR5LPyPr53mnq5K7t1fx/UBL2ZJh0YFL15Y4r7dBZQUGumZiuI0q4klEqyHEkgGPSV5EvO+FB6XgUgszeWbk3jXw9SVWPnGq3Pc126mMk/wnbMrtFWbefqAk5e7I2jU8PgeK3/87DBrvg2MBj1/+t1R6jxacjmZgal1Wst0HG1z0D2ZYiGo4b7dhfy3bw9Ska/FpBcc3WFmcjnK43udnBlI8Ep3kH21OryrPl64MI/bnCUYjrIUVFPsVGMza/jGS8OshxLodWp+7ys9mI1qLHr478+NUVMk8UvHPFwZTxJKG/ilY8W8dG2DEpeaZDzCS9cDNJeo8W0k8K/7WfYGMRu1/NWPhtFpVVwaXOfqyAahlIbP3FPAi1eXMKpTWI1q/uJHk1gkDcNzUTLJMGo1PLrHxmu3IjjtEkvrGbqnUuwo13OkReJs3wY2Q4aOGjMbgQBf+fE4nzns4XvnFmkrU+O26cg3pfhPX+8DOcfZ61O4LBoOtVgZnEvy4rUNjrdZ7mbVcMfu8tTnb/IPH+94X4+SfBhbU51Vsiwb/1FNff5ZMDU5STqVInGzn3g8TnhlFf/gMACx1TWSG0EsJjP+5SXyxqfJLa4w1d2LvbwUvcXM4tmL1HTuJCrncHoKkFUC3+kLzA+NYHM60VzpZm1+kbyKcoytjYTmF7D5XOR8fobfPI+zohx3Ks3qxBRqqwVbYy3F9bUYnHbUDhvRjSCpSBSdTs/EtW4shflort4iEoki2W1Y7XaMpUXMvHIag8VEeGUNS74bnU5LzL9OxL9O4PwVFkdGsXjySZ++gEqtYnV8HJPdTnRwCMliobC6itXxSWyeAkru2YfGoCf0V3+DsJoI3uhleXAYi8sJ3jUs+XkYC/MpKipk7DvP43/zEkZJov+Z52g8dIDYmhetQc/quUvYrFZGL1ymvK2V5MwceRVl6Mxm1q7dwqTTMvSd52k+doSViXHUOj15NhNSnhs5kyE6Nc34+Qs0HjhAbmyKpYFBijJZVMkUyxOTJCMRqnMyoYEhcpkMkYVlNNEEK0NDqBJJcqkMhY2NrE7OEA9HGe/rxmpoJpPJMjXr5bULkxh0Wr7/wiUeOtzMwkqQcCTBazdMCKHmtTdHOLCjFLvZwP/zrQEeP1zL5ZE41wfmObqnlsG5OPs7KlCrNURiSRZXg9yaiqPXaugbXcUiSUysaAmF48zMr6KhlJpyNwVuK21lakamzFiNOV694WNm0c9EqZs5f4rpBR/+YByzzkOBy0yhQ83Jbj8anYn1YIxTfTHmljYIhKKkMjI6o5F2Tx6pTIbJOR9ZIJt2UJanpbjAwaFWBwV2LRvhIsrz9dR5TJzvWeTmaIBxq4XzNyapq8zj0piW7qF5TFItQ9PrZHI5+ibV6DWCeCKF2Wxkb72EP1KPQSOTb4W/fXWcAzsr+NHlKBsbUbKyG506S215HvsaTCz7okwshEhlNtBoytkIx+kfWcBsNiDLOa6M6VGJLK9cmmbPjnLWYyoqCs3MrqW4OJpiciHIzel8TPocoUiO8hInh5tNrAcLuTzgpa6qkMu3xih0W7g6acTi8X30lYgQqHR3ZzaaLMvntmb23u59PUry1kzg90MIUSHL8sy7fC+AYlmWF35aGVCCzbuqrqnBtryAqauD1M1e8iorcFVVoCvMx3+1G1wOckUFND3yIJk1H1JHC66FBfQ2K2qHjbyaagzlxSQWlyg5cYzkyASWY4cgm8NQ4kGV56LEbgNPAfHRSVJLyxjNZpz37CMRDGHOzyNb4KbpqSeIT0xh8XhQL/uIp9MYhYqS2lq0eh3Srh14wmHUWi2GznY0qRTSyBjEk0hFHkqbG8EsYbJa0UkSps52MhevUn70AAajRKPNQnR9A8fh/YRGxqivKie6vIKrrBRyOTRNtVSbJLJaLdmFZQIrXooO74NUBue+3aiFwFCYT3bNj/3wPtZOn4fCPAo62zHa7WRNEp7GBlR6HRrZRN3nnmLj4jUMbc3U67Skw1EcHTtI3OwjnUiQf2gf6xevUtraTEqnpu7EcVLLq+gqy0gMjSFVlSM0WloffYjY4gr6pnrqDh4gsbGBcWcLzlAQrcGAaXc7icvX8ezuwFZXTfTWAKUN9UgNtUjJNJbGWmJj89isZuw7dvHQvbV410LYbWYko4ZgKEJLYzX33dNIPKvFIqlwW9W019rIZdOkkykqC9V0NBQjVFp21+i50COxs0zFqQEVn95v5YXLfho9Bj7/YANjyyk+vd/G9IqHao8OXzBJfbmdVYuB5hKBTmPDv5HgfH+czx0romcmx71tDowGA1ajYE+dEV/ATmmRiyKHTPmBIoYWNsd6xhci6PZUYjZqSLkl6itctBQLZv3y5mQQs4Wa8jxyqHh4r4Pp5QgP7vEwvJRjZHadh7vcXBlLEgxG+GePVjPlFdgMWR47Ukc6AzpVnIoiO8fbJHSaUpxSFpNJT51Hy0bagt2Q4trIBlUFRmZWYqz4U3zhyVr652We2GvDaTfji2VBhhOdEr0zcXJZmeN7KphYCHGgXssVDGhVDoqdOobnY+xrMBJLpOkpz6OswERXrYEXrvgpLXJh1WepKcvngXYjG5EUt2bN5MtxXroR4JE9Dq6Paako1BEOe9Bq1Rxt1rOocX8Mtcj7GrNxCyFuf3HjV7Zm0r6b9/soyfsONsAfCyFUbI6vdwNrbM5IqwGOAsfYfPD+XYPNJ/4NcdstlkoQXVlDrxKUP/4gsblFQqMTWNwuhEki3DOAqa4Kke9m5vmXyNvfhSabA6+f0oePE5ydJ7a4gs5uI5bJsjE9i1RegjoWJ9E3hNTaiCnPRXJlBbPFTEKW8V66TuHBPWTSKUL9w0jlpdj278X7+hmyJgln1y7CPYPojAaikQhrpy/gumcfhtpKYoMjbFy6htRcTyyTZuXUOaT2JjamZnHsaEJV4qH7S19GbTSiSWUYf+UkPq8XfVMNa6fPk/UHSKZSTFz/j7S0AAAgAElEQVS4SiQYwru4zPizPyRnNEAmzdLgMGsTUzjq68C3zsaFq9gP7iM6v4iuqBAA15EDzL/+JolgCP/YBMEbt9DnuQiuepm/fJVk3zBCrab3y18nEgqTddlYP3eJnEkiodOy+PJJhMPG2twcgaExZLuVaCKO/+xlzB2tWCpKmX39DTZWvQidlvHvP49vbg7MJoa+/i1sDbUkUymS0Rg6jQZXSyO+7j7sNVXoy0uYe/UNhMNGMhRmyLuMIZ3kt77wW7x6boyz1yY4cbSd7zx/jtm5VT73ZBd/9s0LHNtTwe7WYoan1vmPf/YmWpEiFovyrdcmaal24N+I8Ptf76MoT+KPnh1md/Xmr9n7Oqw8e2aRmiIDD3RY+OrL8yQSCZ55dZy51RjF9hwdlVq+dWqZnVV6jrVbuTLkQ6dVI2kzfO+8lyM7bCz6YpzuCbCvyUKJA97sXaesQMJlyjHjTTK8mOVYu4OZpRAmvZZUBi6MJNhZZWB/k53emRQmk8TxHRJXxxIMLuSoKzYQDMWIZQ24rDqKbFmGFhJIOjUXbk1zrteLTpVjZHaNUELFkXYnz7w+z956AzvrbAzOROkeDVBfpCHPqualSwtsxMBuMXC+Z4X1MHgsGb784gyrq15uDc8xveBl2pvh9PUF5r1xiuwyR3ZYeebkIioh01Vv4/VrS+SZZG5MJnj5RphfP1FMJp1iajWN2WwmnUqTymo40eng5lSKN/rjHG0xE9gIMb3g55VrPkIJ+NJ3+7GZNdQUqPjBxXWSqfRHXn8IIVCp1XeUAJ8sy523pfcKNO966nfI+0DDDLIsPw38X0A98OfAeTYDzz8DRoF7ZVk++V7HUVo27yKZTGHS6Rl+9jka9u0ldq0HSTIy9PoZ6vZ1oUln8C4uYbh4jfD6BmuTM9jdbrxT02RzOdQaDb6JaVQC/G9exGwyMfj9F2h84Bijb16grGMH8d4hBJDNZZkbHsbqdLG0uIhZr8dkkFiYG8Ry5QbpTIbV6RkMvnUK1gPEQiFWZmfRGwwkIhEsfXbUGg2LQ6MkIhF0Oh1aGdbm5jFP5uOdmsTudiHsNuz5BeiqKtA5HbiWV0GjITy3xEJPP/k7mjDYbFjzXBQc2ANqwcDXvgVGPVJJCTlZRu4fJHKjl9GzFyjqaCM2NMLCwBANViuxUISsRoW9qBCTJIHbhX9whKpj90AuRyIUQt9Yg9liZm1qmrw9O0lvBBl58xJ5lSEshYUsTk5RXFKEq6QYo8vB+tAI4fklspkMpsERAsur6EwmPIf2otbpyGUzGAvysbY2Mnf5GhtDo+jNJga/9i1qH76f1IoXb18/brOZdCaNXqdHjkQJLy3jm5/n1uwCFslM36iXeHiNn7zRT2NjHa31RfQMreJbDzEy7cNq1nH8YD1jE0sc6CjihTMzVBQ7qfEYaK0w0TPm42CznUAwwcxymJtTcUyShHc9yrXJHIlUmpW1IA/sKcUbzPLwnjzmvVFGFpNshGP85KoflZwhl80xvpggmVMzteDnzcE80hmZi70L5FQ1ZLJqZpeDXJ9IIaPnz78/yIGOKl68HmJ6McDSWohoIkNbrRtfMAFANhFkNZRl2WZiaDJMMp3j+Ysp1kMpQhEfJmMl2ayOhZUAg7M6mqs8FLp01BSqOXU9RiicIltXxJI3yPkhFwA6nZa/eW2YR440U5mvZV97JQcajLzZF6S+2kNTuYmx+QBj0z5+5+kGdJINnVZNV52J68Ma1kNxeiZk4skc8ysB6or0JFPQVOthPRbn2ulRWmoKuDyeRaiMPPPKGKUeJ3PLAfKdZtTaQi50T1Nfmc8zry/SO+7jM8cbONBsI5uVSSTLKXAYMOpSZNNJXn79LE//2r/5yOuRbZ6NtvpW99gdPkrygciyPAT83ge/TKVl8670eh1Jg46S9h3oK0uRutqJyzlcNZVInW1kMxnKW5pxHNyDZDJS+8SD6MqLcZYUU1RXgygvoXRXB/aiYpyH95M2GnCVl2GoqqCyox2j3YrU3oyxvRkhC8rb2zCYJIobG1DVVEFNJUazBX1rI7mcTP1nn6KgogzLvt3ozGaKqipxl5ZQvGc3Gk8+qqoybIX55FVVYNm1g4yco6KtlZzRiK2wENPeThLeNSqeeozM9ByZVAazy4XFZMYoSbT+4tNY9XrCo+NUPf0Y0b5Bsqk05Yf2o/Kuo9Fp0IQiuDo70BYVUlBdhaPEg629mYLKCjT5LqRdrSQjUUrvvxdJoyUbDGHPy0MrSYRv9FD19BOkFpbwXbpO9dOPk56ZIxOO0vqph7G73GT0WtzVlTirKrG73WgyOTx7d5NfVkpeWQmW1iasRomapx8nMbL5olmz1Yo6J7N26Tp1Tz2GVFaMvrYarVaD1iSR9fqo3d2JvakO144m8pvqMBn0VB3aR0tNHVUtLTz91KdpamykrLSITz3YRVV5MYurIR65r5PaqhI8+VY6moq40D1LTWU+A+MBWiutSEYDFpOOF6/6qS2x0D2V5AuPlRPLSjyx10GVK8Pu5kLKXDJ55iyff6ieUErLzsZiemYztFY70el0NFV7eHJ/HkJj4Dcfq0anVeEPpfkXn67HqM2hUQnu3VVMdb7Aqstw/54yJD049CkePlhFoUvP/R0m9uwoY8+OYooKbEwvR5ha2kwr63FkZKKJHLmsTJ7DyBP7nLjsEuXFbg41agiGYzTXFtFVZ6fQpScQBbOkY2dzOZWlTgrNSeor3NQVaTnRIRFPpDjUWU04mkStVpPLZbk8FMTjMmI1adHrVIwsC/7dLzazupGlwK6j0JJjbi1NY7WH2lI7lcVO3HYj1aUuju4sZHxF5sFOO4X5Tg7vrkOr13OoUc+hJgMdTWU0Vjg42lXDrgYnVj10NBRRU6ChrsLB/fsbCKe1CCF45cY6D+92MO9LUVtiQ5IMHD+y76OvRMS2P2fz1qMk8A8fJfnlrRcc72XrUZIPdzMfjhJs3oUsy2hiCYqOHyY0PE46FkebzuI5chB/dw9ScSGJRBz/jR4sO5qQ8vMY/N4LJFNJlsYnmX3lJPq6ajRlJQT6R1AlUpQ9+SihGz1oXA6SqEitePFfvoGlo5WZazew7m4n7+gBglduEOi+ReVTjzH27e9jrq1Ca7OSsZjZGBrD5CnAu7jI2vwCOjnHxMsnGfrrZzFUlGLZ2cr6xasYJAmVDPLiCsX3HSU+OolkklDrdESDIdYvXEbfVE80EkZsBNF7CsgW5BGeW0RrNJIzm1g8dRZrYx3xjQD+ngEMNRU46mvILi7jrKogmUyRnF3AWVVB1OfHd6sXk9uF1m4lEouhE2qsuzuYf/kklvoa1FoN6lQGSa/H4LCTCYQgEERfVkLObiU4Ok7ZQ8eJD44g63SkJCPLF69hqKnA2NrExLM/xNzejMFmJepfJ7GxQU4yospm0QsVlrISVKEI8f4h6j/zJIFbfRS2NFHx4DECI+Os9Q7iaWvGPzZJdD1ATXUVux46wX/74z+mvdHJ53/hOC+duoVBr6Gro47v/uQKJ+5tpWdkjfWNKG6rjlA4SjAcp6HUQKlbxc0xPwUOPW6bFrtJjUqlwmnKML2SZGgxy1MHC5hYzbIWUlFVoCOViJHNZgmEkkRiaVzWzeNcHfJTmi+RycK3Xp3AbdUztqLicu8iK74gqI088+oE3aN+7GYdVweW6ZmOcU+LjVA4wXNvrrCvTsfMUpg9TW6O78zDaLLR1eiitKiARCrLwFyCX3+oFIdZy9XhAE3lJo63mzl1K0Shw0CeOcv3L6yws1KHpIrzk8srtJZpuK/Nwms3fDy8J49zvav8yXMTtFdoEMi0V2g4fWOe1y8O0z3mQ0ucXCbOd875eGyPg0KXxNneVXbXbna/9c8m0WpUHGqx0TcVZt6fxWHWcG0kQH2pGZtJSyKyTiIW5niriTP9MXongrSW6bk+7MUgoohskp6RZc5cHUNodOyrM6A36JFUCbwbSSSjEaNeTX2hipGFOGbJgMVs+sjrECEEaq3ujtIdHOtZ4DJQL4RY2Hp85A+B+4QQ48B9W3/D5qMkU2w+SvJV4P/Yjvt7P5RutHcxPT1NxO9He+0WOp2O7i/9f5S2t6K60cN8Tx/19x9l3esjMTRCRSLJRjSGxeUk/8hBTCte5l8/Q3xwBGMmy+D5izQdOkjyVj8z3X3kV1dgdecx/IOfoDcYcMUTxMJRouOTZNQa9JKRxVt9aNI5MqkU3lt9WKdmMBiMDJ07Q+XONiSrFVt+HlJbM26vH4PNgspqJjQzz9LwGGb75kObtppqNH2DrNzqw9PagugfQmPQM9fTh9lsIjC/QDabRWW1oNXrSUbDrJ6+gCQZWZ+dI6+7F61aw8yFi4gdreSGRpm71Ut+Qx2uslImTp1BrdFS1tTI4BvnqT+wl9ytAbRmM4vdPZQI8E1MkVfkIewPMHerF1tFKaqb/Ux2d1NYW4vuRi96jYbA/BK+c5dZ6h/GUpCHu6SYsSvXsOp0CK2W9flFCofHiWcypDNZhp/5Lo2//AtMfu9HOHe2Ebt+k8VLV8krKcUyu8Da2CRlVVWE/QGsZjMTFy5RYLejt1m5+edfp/zpp+k7c5aRkUFKCy2MTnq5en0Qh9WARt3AyyevUlZ4GItJzX/9X6/S0VzO/FKA5SU/2ZyaeEJw5vI4Dxxs5OrwOioh0Gj1ZLJGvvf8IPfuqePMYJrTV0bZ317F+eEkNoue/vEVju108+UfT/P0kSJ8YS0/PD/HvXvrMTu1FOZZONJiRAiBP1SAw6Jlb62GZX8hHpeRao+WmyMZphb9PH9RRkbFzOI6F8ecDE6uYNCpMWiyrIfTvH4lRnVFPmqVmrZqEz++4ieVFbzZPc/xAy0sb8Dpa1Mc2lWF1WRmcn6FV7oNyDL0jPgwmJzodSp862FGF+K0VUqcDSXwBhIMjK9hMRTwxMFijJIZh1mLLDSML4SIxXPcKjQDKqKxJOdH0qTTWVZ8AabmkmSyZSx6w8TiCQwGPbfGfNzTWcPwXIAFfwqNOsP5IT0r/gjnvQH2tlextLoO9TaqPBIFDiOheIb+yXXWwzYCwTgHGyW+9tI0J3bncaEvykYkw5nuJQ7trmbZ6/8YahGBUN2dalaW5X/yU7469g5lZTafi/lHQwk276Kqqgrz7CSmrg7Wr9+i+t6DmO1ORIGbeCCAzuHA6nZRUFKE7UAX6TfOU/y5p4kPDhPzBaj93FMkB0fQ7GjEMjSCpr4K1GrqVAI5k0VVWUZJLoPGYERdVUa9Jx95I4TU2QE+P7alZYRGTctv/VOi125i3d9FoKcfd2UZrsP7iHf3E4lE0MfiGF0OEhtBXE4nGq0Wi1YL2RxL/UObg+R7d6PJykhuF1JrE7HT5yndsxuNw467rAx1vhutXkcoFMaSn0/BvQcJ9g1R+8BxNEA6maSgsR5bayM6txN1TkZnNqHy5FPc0EA2m0VbU0G9kMmlM1g7WkgOjWKwmDB37qAwGkU47ZjKS1Dd6EWbA2N7Mw3pDJl0GqmzjejKKsXNjVjLStCpNeRyOXJ2KzX3HyPhW8dxaA+NqRTZbBb7gS7U3jWW+4fw9Q6QSWdQxxLYdzQh1tZxFxdjrCil3WYFAfm7O1i7NcADTz5BaX0tkTU/1lo/v/Tpp/jON77B53/pc5R7HNTVlhOOxDFosxzdV8upM3ms+mPMLgawWgzcv7cAg1ZFviVDIpmlrUzP2JSNexo1xJPFlDpAqEFNis/eV8daKMfRZi2ZbB1qleBQo56JhSR9CM73+0ln0ozMRxCo2d1USIlLTa1HQ0WRneH5JC4LlObpWd3I8OL1EA/utPJ6TxhLg4TH4+FwW45QWs/sSoTmKjddNWom5hzsqrOTZxWc6vbhN2jorLUyumSkrkTD5GoIj0Pgy7fRVibQqUGrqmc1kMSqS1Jd4mRvow2jVmZpLUpntZYb4yF+6YFq1Bo1Y/M5WqsceFwGHjxQzYIvgcOip6jAjpyOIRl01JcXsLvWQPdkikNNRqbK7FTlCcrzJX6UsKAvcFJkzWBvKSCa3AxkRXlmGos1JBM6KguKWPBneXCnxKmeHEV5ZnZWqMizNhGIyuQ7JX54KcDvfKqKN/oTkEuyth7GH9aTyaSZ86XZ32RjdT3NwY4yaookrPmuj74SET9Tb31GCFEMlHNb7Hiv5aDfonSjvZcCN/OvvIHJ7SKvq5PI4jL+S9eo+NSjBMcnySVTJGUIzsxhLCpAo9OQ2ghikoxoJYms2czsT16j6unHiPcOEbx0HVN7M8vT0yyfPIPjYBepTJq1S1cxVVeQNehJrq4R6x1GI0no81yoNRpwOohOTqPLZCm6/14ig2Pk9DocXR1MPvdDpJZ6LLt2EBudINLdh9TSQDIYwrOrDbXdSt+Xv46qqIB4MIivuxdLXQ3WlkZWxsdZHp8gvLjI3PWbhEbGce5sY31gGHVOxt7SgG9mjviql+L7jxLqGSC25kcq8eBdWmLs2z9AazZhsFvp/+a3iafTYLOQXFhC5Q9Q87lPM/at72Pb0URidoHIwjIVRw9g29vJ0sunCEUjyG47icVlEoNj5B/ez8jrbxAKBEimksydvYhARuVyMPHc8wS8a+SsJkKDIyRHJig9tI9cOMKOX/88BqsZsRHCXVSEbDMzc/IMZfs6ifkDrPYOUONwsveRB+k7c44yq5MjD5+gv6cHT56N4/ce5mbvKD/88UkePNaFVmvg28+f5zd+8SiXro8Rj0W5/1Adf/vKBJlUnNpSK+F4mmffmOefPlTO1fEkZqOOHVVmltfCvHpthTqPlsp8FVfHElgMMol4jHAszdCiTCyWJJkWHO4sZ3guwvXhVVoqjCz5YowvpSjJMzK3LnNpNEFbhZ7ugRlml9ZRqWQO1Ou5ORnHrIfqYhNXB+aZXljjeIedr7w4zeeOFnN9LMLAdJSyIidfeLSSywMrXLw+xrXxBI91WfGHM/zzh8q4NZ3hZG+YrlojO6t0/OTSIo/sdXNhMMzr3Rv85qMVnB2IoNbo2VFtZ2lDIJnMHNvp4puvzdJcquVoi8S5wShGdYajbQ4uDofR6fV4nAb21Kj5z9/oY0/9ZvdZ31SIhhIzq74AI4tJdlUZWAtEKSsw8ljX5ky0H5ybp6lURy4VZWIhjM1iRKVWc2EkRnulgXAkxqs3fBxoMLIeSnGtf5aZ5TC1RWZ6JsP86882ku+UmFzNMR8QPHkwn4nFCBvBj+HdaNs/ZvOREUL8EXAR+I/Av9lK//pO91daNu8ilUqh2gix2D+ATqdFt7CMRq1icXoO3bnLBBZXyMSiVLTtYOi7P6LhxDFCI+OkDAZ8kzN4TBKGTJaNhSWKh8fxzc+TTqWQ+ocROh1ajYZo3zCSVsPMxBTGc0ZyBgPhS9eIB8PEgxskAgFKkNFncgy8+AoVO9uJdP//7L1nkGTXdef5e2nee+l9VmZ5732X6WqLbqAb3pIgIVKOFEVS0ox2pR2NVqvY2NkYxUra0Uojs6LMyECkMBQJEAQBNLob3Wjf1V2my3tvs0xWpffm7YfGbGg1XBKaoUhRwV/EiYo479yX9b6cG/fec/9nFP/MHNbKcmzxOIlIjMTIJCpJYulOP5aSIuTxGRYHh6k70UcmEcdZVYEqlUKympm5eoOythayy8vIBW6UWIKix84QXt9k470rKGsbLA0M03TqOLHRSXJqFeGNLQw3+xHUKiZffY2GE8cQgPKuTgxtjSQOAlg8HhSthoxvj8WLV/FWVZK7eY9YOExoYpp8OsPhu5eo6u0m79sj7D9AMhiwWK2Mv/M+5S2NHAyOYrHZcBxpI7mzi7XQg76iDASIbm6TTSTQ5AVmL12h4XgfqmSK/e0d0jMLiAisfHALWSfjrSgjvbuPMDJDfUkZA5evUnn6FONvX8Q3NUugrJJYMsnrd2/zS//68ww9GGNsYoZELIioAbVG5P7gOCY5j6hRKPaYcNlEdCKMz22STcfJ5/KsbR5wZ9bN5NwWBp2GVLqA6bUgdquR/ukg8ayG2w/WOHGkHLdF4ve+PkeB04woSTzSUUBjiUw4YsYfNpBX1Gg0Kt64toLNoiMYSSJJGu7M6qgosqPTyYyspIkkVVy5O0VbfRE3EJC1Wsq9VibXsxwE4wws57FaZN69u0BHQxHrvgwq8hQWWAhGM9yeVbG9H2V4Ls/CaoxkWuG6Xsfm9gEOm5GRpTAqBBa2AtxftDO5sIXDYiCWyLC25Ucna3kvZ0ejFnjzzi5lRS6u3F+musTBYUxBLcDQxAqSWI1BA5WlTiZWo8RSAkOTB/S2VbB/mGBjJ4LNrGd4chVrXz398yncFg25vJ5v3T9EEnW8enmFn32mhrWtMIpKw7f6dxFUEvcnNkllCrEb8jRWe1ELeULxJLMr+wyXOFGh5evvT3C0tYx3BvMkUjlu9Q/zk9/nkwsB4V+S6vMLQJ2iKKnvGvlt+NHK5jsgiiKJaIyOn/sZZL0B28lesrkc7qoKrMd78VaUUXjyGGJJIUW1NWjVGuTSYjKhEHqTAXN7E2lZS/npEyg2Cxa3G3txEXJ9DSaLGaPJhLGtEaGsmOKOVizFRTg7WogHQ7hqq6jq7sLdVI+2wIX+SAuNZ04hAO5jPeTzCvaaKpKCgLOuCqmlHqGsCFtRIbaiIgztTVQe6UBtNmPUaKl44SkIhDC2N9N09jSiLGOprUIMR7HWVZPaPyC/so6zthKpuQFXZTmaAheG9mb0ooi3uwNDYy2m3i6cpSVIxYW4CzykkgnSsTix0UmKnnoMg9mM1mGj8kQf1qY6kCVKTx3D0taMoaIUg8WKsbOFrKyl+qlz2L0eNPVVFFRVYG6qQ2vQ4z1zkoRvD60iUPLcE8SGx1Gp1Q/Pp5wO9FVl2Au9aGsrSGXSuGurUVWWoqmvoqKxnrrWZmyynl//w/8I+RzuynLKiot54WMvYdMb+J3f/m3yqTQ//9nPYjcbWF9dwmWVKPRYONLZxDPne9n1bXG0q46OejcdTWUYdSIKWsxGI401JTx7shRFpeGVc1XUeFS47GYKnGbqvHCqo5iGEh2NFVaaSiUe7S2noURG1iqo1Sp+/KyX7kY36/6H1x4EjZ6TjUYSGTXH6kQaqz20VLuorXBTUeziVJMel9OO3SRQ7dFyvk2mttxDZ42Vk/UipUVOdHojZU6BR7pKMesUMok4rbVuHm238MzRAowmG1XlHl7osxOMpLCaDVQWmVEUFfXlNh5rkbDbLBQ5dJxqdaIIatoaynDq07z0SA0VpS7OtRnRaNTIkoa2MhUfO12OxaijqUjFic4KDAaJ860yVoPCybYCzLKCks/w/HEvdpsVp1nL0bZyTjdKHO8o4bNPVaLkcxR5HFS74Xi9DkVrxuUw82KfAyWfobbMRSiaYH49yMZOiKd6XDQUCxS6TJxps4BKTU+NnlxOQa3R8PMvVhONp6kpgKePVVFXrOdEnYhBp+Hpx09//5OI8C+nLTQPCw603zXq/4cfTTbfAUVR0BkNSCYjitlAYHQCvceN/egR1r55AammEmtdDYmlVewlRWTUKuJTc9jcbrRGA7lMBkIRXF1t5PcOkCxm7KePE+wfQhJF5MYa4ourJKZm8TxynPhhgIOBESqfOo8C5PJ5bEfaCE3OkTgMoBj0mHqPcHDjLtVnTpJYXkNSoPDMaeIzC8QejFPy/JMooobVb15AW1bE7MXLqEoKAdC1NRGfngeNGtvJXg4GHnCw7UMry6xduIyxtQm5soLNyx9Q9twTxHb3Sft2MRgMODpaScwuEbo/RPlLzxCYW2B3awu1Uc/on/wlcn01ksnA3sgEOosZe08HGd8uolaLraGW2Nom2lweR3sTh+NTSFoRrdtJWhbZuXyDkuceJ7W6gTaTQXQ62B56gLqsmIT/kIQsMv/aGxjbm3Ad7yFw/wHuI21k17YwGIy4HzlOoH+Y8LW7OFrqmZ+cRInGECURjUrN1LWb/Owv/4/cu34TbU6hrKyMl1/+OJ/9mc9RUlLCjTtD/OZ/+COePd+H3WLkz/76DU51F/Hxp47wtfcmKXBoaKq28Rd/dx+tkEIjpAmEk0gaNU2VFv7Tt2bQqHJs7Yb48uVVPOY8XbVmxtfTzPnyPNFl5/qoH62k4xdeqOJP31mhvVxEp05wbdRPnVdNaYGexe0E7w6FONNiIJRQKLDpaCvTMLKcRBYFHmm1M7ySYdufoKfeztJOhmvjQTrKRRKpLANLac512PEdxMgLWl48XsDAYpq37wd4pFlHPpfn4nCAow1mip1a9kMZups8ZHIa1nbjVBSIHGsw8eX3N+mtNwMK8zt5agvVrK75+I2/meSFPicWg8TNyTD1xTL1RWp+68tjZNNx2so0vD8WJZvX0lRqZHZln6uD2yjZNOFYBo2kJ53OcWkkTHe1TJFLh6RKQj7D+FqGZV+MygItkirJ5EoQr0PPi312hpcSvHS2ms88Uca7Q2Em11J85skSbk7FiaU1yJo8dpOa6eV9Hiw/vMv0B2/McbTeiD8C74/FePqI+f/TRfT7hiCg0oofyX4IiAOjgiD8qSAIf/Bf7KMO/tE22ndgcWmJRDCEODyOLAiMvf8B9Sf6yIciBNbXcc57iEzNEvAfQCqFu6yUpcFhSlqb0NqtLL72DbynjxGemGb+9l1KWptJ3b5H0H9I4vCQAquJ4IMJBElCdXsAlaKwOzmNBkhEI+wvr1GnVqO3WRj50l9Rf+YkSijM4sAQJZ1txHb3yCSS1MgSs+9fo/5EH9F7w+TTGXYXl9CXFmEt8BBcXiW7vo2s17E+PYuiQHE8QUFfN7mdfVIaNbFgkMTMHFq9nnQiyf7MHIoAK1dukMtkMIXCRH27pONxZFkmr1ZhL/KiKfRgLywkGQoTmJgmsO3Dsekjub3Lwt1BStuaUEcirNwfpOXxc8jpDFMf3KTxxDFiAyNkdnaJh0Okx2dYGRnD5HQSjcfIZjJEtwDXd2oAACAASURBVLZBrSaVTJDLZNm/2Y9Bp2fp/gDFrS0ENzaxVVWgGhwlsLmF1WDAoRFprm1An8ow8ObbaFUqdheWeP/1N9nz71FgsvCNb3yDQCCI3mbl8Zc+ydryCkM3LzAzPcn8ko+pmXnI1XNvdIPpuTWUdITORjcFdj0Ts9sUeWz87lfGqC61c28WLEY9rzxazJ2pIBt7SXYCSQbmo9yf3KahsoB3BxVWtw8p8drxBTREYykGlhXUopEP7s6RyMnM+XIEw0kC4Qhmk5FrA/M8daIeX0jNrQeriBoBg8qBSQvvDcX5yce8hMJhxldiVBfqmVnYRKeTuDGtJxLLsrWziyhW0D+6hsMqM74qc3NoiZICC+msgoCKKwOrPH+qEpOY4J17Mbob3UxsqdnYCTHvc/PB/Xm6m0t5d8CPxSRRWuRkPwKSJDE6t82dWTMuowqTSceJJjPTaxHe71+nsdKBLOiQJR2NNSbiWQ1TC2sYDBJKPk8klnxYmiyqCEey+INxNJoAy+tpPn66EI9Dx99eWuR0Tx2RlIb5tQOMOhG/24ZeFrnzYB2b1crw5DpOqw5ZZSSZUVFTVsAz3Ra2/FoSqRwfjAW5NrTOueP13JjJYPR+/6vRhH+cXM0/d771of038aPJ5jtQXVXFrb0t5COtBGbmaXr5BZRAEMXloO6Z82R2D3CcPo4wOUNodg6cdlpe+RiJ5TW0RYUEr1zH5NvF1dlGxa4fqciDsaEW4WY/SiaF3unEF45S9dIj6L0eAtdv42msw3GyF/+NfiofP4NGb0DltGHoH0DXWEtkcJTy0ycwVZRg2/egGA0IKhX1J4+RjidwnD3Jwe17FLa3ojEY8B7vQdnzY+ztBMCy7+fAtwMaDRqrhfzWDvnlNepfeRFVKoPKYSN7fxCtKKEvKUYJhNCo1TjPHMd/7Q7B3V2QJDy9nUTvj5CcW8Zz5jiqcIyMTkfF+TPIsoxcVU6TVks8HMF+qg/L6jrawgIw6rEtLKGuKCGxvYOjvhZbJEraZqHu8ceIrm9i7j1CLpfHbLGgqyzj8Ppd5Poa7D2dxDe3aXj8MVQqFYHVNczVFejLS6kXRTSiiMPtxuAPUVldg05RqKytRZtI0d7UyBtvzKKxOXj+xZeYmZnBXl/LxNg4iUiIVz79OfZW7yBJATrbGvnU8128/u4gv/rFx7jWv0RLlQ3fXgK33cDpRomRyTWePGJFQUFUFzK0mCCZlagtUeO1S3TUaLBZTSTSCp3lKiR1ETarSHe1zOiMSG+1huHFGGVFdh5r1SFq1byVzFLstdJXo+bgsAS9JNBTLbJ74CCZAY9Dz/3ZILv+MO/eV7N9kERQq0mm0kiyiNNm4lSDxFthkbaGcs616QhFCih06uiplVneclNTZqW7WmZrP8bGjpnGUh1f/WCf/cMY5S4VK9sxGivstBQLRDsrMcpqzrbouTiSIJmMU+2xMLMZ4Ui9h+ZSDbemEnz6bBFbBxmiaQ2//EozCztZJE2WtgIDU8sBqrwy7Y3FxOIZzHKOnaCBvloRs17DW/d1/NtXahlcynHxzjzru1EQFIo9Ns626JBENWpVHbsHcY5Wa9gPZtjZt1PrEch2VqLk4VSrzJWxOKXONL7DFEOLWTxOAyebjJiNNfhDKc4f1bEp/gCq0RAQhH8Zk42iKK8KgiACtR+65hRF+cgaQD/aRvsOCIJAMhIln82iOghgrCwjlUoRHp/CVFeDVF1OfGIGTSiMrriQw8lpNC47gsfFzo27dP7rL6AOR4ht7yCXFpM/DLF36RqW1kYqP/YsB/eHqX/padjaIbywhKmmAuuRNqILy+gsZmz1tcQWVzi4fhdnezPjf/YqcmMtalkm/GASY3sz5ppKYr5dcrkclmNdrHztm6hEEe+pPnb7B9CXlxBLpcjE4uxfvYm5vQm1RkMsGGThtTcYu/QBYnU5pqJCFP8hkeFxWr7402TXNomubWCrriCvUeMfmcBcV0VRUyNiVRn+D27j9+2gM5swegpI+XYxaLQ4muqJbvrIRKIoohZ9exP7N/spPtlHdGGZzQtXsXe2Mv2f30DJ5ZBrK8ml02RXN9HVVuJ45Bjrb17A3tpEfGWdw5FxjI21uHo7CQyOoApF0NdVsXjzDpVPP46ytUN4fQtLeQllZ09w9/VvUlpeTkN7K6OjY7zz9df53Od+hvn5eRoaG3E6nWxubvLB7dvUtbeysb2Fx+ViZ3ePP/mbCyRTcfq66vmL1z6g3CtT6DbS0+blP37lAT11JvLZNG/e3uN//ekWLj4Ic3P8kPYqI+/eWqS+SM3RRgfTW1kuDR9ypELEKme4OLjH8SY74WgC32GaniYPd6Yi5BQNnzpTyPBSmjvTIXrqDKRSMb7V7+epbiv7gTjBaAarWc+ZVgPzviySrKexyoPbpufJvhKe7nUTTGjoqHVxvt3AG3f82IwisXiMpe0IDaUG/OEM7w0FePaojUAozl4ow+BShpYKI5MrYZqqPfy7zzRxeyrByn6Oj5/yMrycxmESMYpZVvay6GUVxxsM/G9/OYFJTHG0wcz1iSgui0xFoYmhuSBeu55Cu5Z1X5hoWkONV8vxRiOvXl6js1xLawkMzuzxTI+N94aCXBvx01Ehksnk+WBwifPdXnxhkZ2Qhs8/U8bl0SiLm2G8ZoWnuyxcGY9yfyHNT54r5t37u1S6VZBP8MatXY7WyfQ12Xlv8ICGMhm1kuHSgyB9dQYebTXw9mCY73EHko+YRB7K1XwU++eOIAiPAAs81Ef7Y2BeEIRTH3X8j1Y234F0Kk02nWb+tW/g7mwhOjqJKp0l4NvFdPehOOt8/wCepnoEnUwqkcJ3ewAhkyWw7cM1OoXeYmHy796g5fRJFu4N4KwoRzsxg4DA7uIyVqeTnZVVXKUlaE/2ouRyLN64g6m0GOXOALtLyyBqkbJZPNVV5GMJQnMLKHmF0J1BFEUhE42xt76OM5UmlYgTDQSQpxfIJJOkRiYxmkyMfOkvKKmvZ/vOfWSDAfcjx/FoNMz+5d+S3tsntOljb30TZ0kRsYUV1KKWtWu3qevrQS/LLA88QPf4WchkCC8soRLgcHWdbCpJkUZDPBrFv7GJM5NG63Kwdfnaw0ui/gN8k1O4QhGcZSWsbG3i7unAZLcT3tsnf/0uSwNDVPd0Ebv/gGw2S2BnB8PwOJIssdE/RG1fD7mNPKsDw1R0thMZHkcUJQ7Hp0kn4hgPA+R6uti4epud1XWuv3eJtfkFRJ3MzOAw77zzLplMhsmpado6OvjSH/8xaa2G6+9c4M69flDyPPrkk/T19SLmw/h29xkem6PAaWT3YBNBAN9eiOvjQTJ5kfXtLe4u2HA6DFzrnwfRTG1lEa/f2KCltojl9T1KvQ5UKvDaRd68foBZL6KXVPz529vUlVp4MLPL06frWfIl2diPoVKpSaUPSabyrGweMlJgxm7S8huvTvDo0Wqmt/VcuDXNqa4qNv0R5laT9HVUAhreuT7BuWMNjG8orGwcsLUbpMxj4vpYkI+dcDO3FmN2I4THoqHao+G9gX1SqRR5q4Hh6VXO9tVzaSxBNJHGtx/g4oiBkZktzAaR8kIbF+/NYTXr2diWqClzUl1sYesgy+DkOm11hURSMLt2gMNmZnEzxOrWIbsHYeLJAiRJZmc/xpwvTyKWxmaSGV8MogauD+8QiBei12QpdpvZC2aRdCJjsz7IZxHyab55J8HJZgfjK1Hm1g7QSVree6BlbmWHmjIHomxkfnoRq9VKLp9ifmWX8iI7Fr2aRDDH6EKAzUCeVEbgnYvXeOHHf+n7nEX+5axsgP8LOK8oyhyAIAi1wH8GjnyUwT9a2XwHREnEfryH2IGfTDyBvrXx/9VDMx7tIqNW0/LpT2B1OFFnc9i9Xjwn+9CoVFS98DQqp52UAPXPPYVgM9PwyAkMZhPWE73ktBqqH38UsaQQncPO2tg4W9dus32zn1Qyibu7A/PxHkqaGilpacHpcOB5/Cz53X2MJSU4i4sw9XWj72pHrdVS3tyMqJOo+fjzOJxO4qkUrpZGhCIPqXQad2UF7vOncRZ4KHn2PNHhCQBKOtqQ1BosJ3rRWsxszS6Q1apQa0XKzpxEXV5KgjyeynKiB4cs9g9iKi3G2NJI7ZkTeCsr0Hc0I0oS7rJSnJ2tGJx2wn4/WoMea3c71cf7sHkKECpK0JktIIl4utox6w3omuupe/QMWpMRQ28ncmsjjefOYvYWoDjtWD1upNZGUiYDlWdOYvC4cXY0U9bXjb2iFHOxl8j+AcbSQtQWIy/8wheobqznuU+9Qioa4/jx43R1HUFvNNHa1saP/finyWu1qEoKSBU66fvY82j0euYmxnjh8V6sDjf11YW0NFZis0g81leKijTV5W6e6HWBkKWm0suZZh1VLhVul42nj3lpqHRwvKOUplIZu0lkdGaDWxMH3Js+5GiLl8YKO8dbHBS6TbgcZh7prSYQyeCyqJhZ3iEUjnKkxopBJ1Jb6aWvVkYlKBxtK6aqyMDZZokTnZWUOTUUu8201rip9SiU2dKc7SqjwAQO3UOdtJa6Ejx2NYtr+wwvBDnRZEUS1dQWSaTSaXb9IbwOPT01MvXldipcatpLBbxuE90tlTzWItNeX0RpoZOzLQZqyws42uxGrxf5mSeLGVtNUles40xvHTaLgUdb9BxtLsSs1/DCMScnj1TQVu2mp0aPSJwit4neag2JrITHaeRkqxOtRkN9pZvne+2kFYlPnPbidjs41ajDbjFwqtVOT6OLw1CcUo8Br13m6RNV9DQXkkim+DevNGHRgVaJUlbk4FybDoMmzYunyym0gj+UYGRuB7VGwwt9TkStmkd/ENpogKBSfST7IUD7XyYaAEVR5vlHVKf9UHzhD5LI/RE6fvGLqMIxti5cwdx7hFQyQWh6Fr3TjtbtJB4OYdDpMbY1svX+dUxVFcgOB/OXrxHe3EZlNOIbGSdvNmE40krg/vDDW/2tjURm53GWllD74jM4vF5kBNp/4XOkZpcIL6+iKfYgZDIIOhmNLCO4XQQXl8ibjaT2DwjcuIv15FF8Kyug0aIxGYlFo4iZDAVHu1l++xIqjYbiZ54gNjGDRq9DI0lktRriG1vkRC3pcIx8Po/NZsVktZJa3sB6vBtHWxOZlXXkrAIOK/sPRil69CTBwTHiEzMY25uJxePs3x/G0lxPwbnTxEcnyRwGqXvyPGa9nujgKKYjrcTiMQL3hql4/kk2L15FX12O4HWxdfkDzJ3NpDMZ0oEQ4fsP0Lc0kE8k0IQjeM8/8lAnzbeH80gb8UCInau3kSpKmb96A4PLjetIG7lECn0shbuijO3AIW99+TWefeF5/H4/t+7c5eVPfpICr4f/8z/8Di9+8XOYdHo2h0ao6O4kZpQZuHaZnX0/Tzzax1feuMvxI9Wsb0c4DCbwHyZ5+Yl6JpfDmA0yx5utjK/GmdrM8/SxQl59Z47OWhOnOz3cn4tgMJqoqyhAo5XorHPz4vEC5rZSZDI5WqqczK0cEAuHOFor8WAlS29rFaUeM4m0gCSKmKQsl4cOMBkNvHzKy+RqlBujfo41GPDHVdhNEk92WbkzFeX6eIjHj9hY82dY2hdoKhUJh6PshrT8yqeayCh6DkJJzrY7GFnNsn6g4qceryCdVzOwEOeVM0XcHtvlwuAh59qM5BWFt4fCnG3VU2jL8fvfWOTlUy6iKQ0moxGVSuC5HgsXH0QQRTVGbYb3h3forjVj1uX52o0dKt0C5zot3JyKEsuIfOK0hzdu71NXIlHj1XB36oBSj56+BiNv9+9SXWjApFMTiaW4PhHli8+VM7CYZn4rSXe9k8G5CHaLTKlD4c7oJscbzXidegIxFcGURJVHy7Y/STyjprfBxlYARL2N3/piJ7G0wJ9fWKfIKWLQ6b7v+eNhiwHxI9kPAUOCIPyFIAiPfGh/zsP+Nh+JH22jfQfWVtdIRCOkJmbQueysXLmO2WZnZ3YBQb1CWU8nifFpEFSsz81RrlZzsLCITpbIbG7hLPJgKC4mlUgQ2vEjLq1gTyRYvjtASWszqrEpNqZn8OYVzFErK6MTyDYruul5wskEqf5B3KeOsXRvAEtVBfHDILIsEfMfUBCOMPnqa1QcaSd0d4BkJEL44ID0lZskDw/xR6NYkylQqwjv7qEVBBZu3KGwpRFhfAqVWsXEV79B7YvPoC4tZO5v/g5XeRmRYJCcCmIPJlDSaVYejKGVJRDA4najs1rY9Q1ALo9aFNEKKjZHJ9ALKlIr60T8BwSn56js62b2ynXcddX4b9xFyeXYX1vH6C0gHo4SHxwlGY8T8u0R+LASb+Wdi7ga6smlUoRicUS1Cg4C7E1Oo1KrkUan0GnUbG5uIY1NopVE8skkUjbHtT/4U8488xQj711ibW2NrWiCLAq3+u9w6swj/MGf/SmLc3OEw2Gkt77F9PgEnqpy5i5dY2d+gfKiYixWL2OzOyxv+BkwSlgsJn7jDy/R21qA1eTlcv8qn3u2mmQqy1cvzVFb4cKn1+MPxhici5BMC2zvh4hHo+gkLfPxFGqxkr1QCiEb43e/tkpPaznt1RYOIymWd7PsHUTxH4Zpqivm9742yYmualTI9I8u85jBwtZYHIDLAxscxou4/WCdE50VXJ9ScxiMsBeI8eZdFQ9md6kodnJ1QuYgnCAWT7Du9uI0pHh34JDn+tzc7l/DZJAx6TV4LQpXhvbQijqCkRQZReDSSIyxuS2cVh3XJmV290JkcwrfuHvIQSBCR42D0YVDVvZy7IdSLG/sUVns4trABsmcFoPewOC0jwKnmc3DFOvbfvIKgJfJpT0KPQ40Kh3v3p3leEc5O1otowt+XA4rU6tpZKIkNXriySyxSIDh1RBtTaXcGVrAZdeTTCnsH0a4PxfDYpKZWdqgrsxJXbGBL721wueeKiUSS+Pb8RNJ5rg2KRGOQTylML0SRLDv/wCyyL+obbSf46He2i/ysF/OTR6e3XwkfjTZfAfKysuQp8cQ66vJ5bIUbNWhLfLgiMVQKwq6pnqyyTSqgUEqWppJW82Y3C7sJ/sIjk9iajhKeGwKW1szuuO9qLQasno9TS8+Q3JjG0NbEw3JFOlEElNfF0XZLBpRwtjWRHRwBP/MHM54EoPNhtVmw3q0i3w+T51ahaAVcVeUY6qrRuWwI9mtCOksxu42grfuI+pk8kUeLKUlZHf30LU2UBGNoRa1GFubyOfzFMwtEZxbQMjmSKdSWI4eQbKYSB0G0Lc3EV5apbC5AdFmQRVPkrNZ0GSyFNXVIrhs6CSZWDqNad+PvqsNQaUieeUWZpcLuaEG98o6rvpadDWV+G/eo7i2GlWBE6PNiu5IK8k7g3T+0hc5/OAOjkdP4JufJ7C1TTIeJ7y1hSTrMNRWgVqNvbQYU2cLe1duUt7SSE4Uafixj5FaXsfc24lzd5+oVkXh0SNYolGEvELzubMs+baobm+noqEe7RtvIptN1DY2Mnp/kOfOnaeyro6rf/d1nCYTRqORvvIS1te3KbBmOdpZxfpWAKNFw8hyFn8gzo3JMJWlVjo6G6guNmM3wMD0ISeabahUAslEAlHjIhZLk8mmOVajJZXJ8c6uBkElIIkCbZUGBpZ0uEwZ4gUmir1W2ktAI1ShUmuIxBL85FM1RFNwpErP8naeg0Mbp5qtJNICXruO3lqReNLBsSYbGq2EJBnII/Boi8SbET1VxQ7KXCreH/Kz4w8wvSpx+kg52wcJumt0vHs/hlEvc65Vx02xkFg8zrE6kZHpHEfqXLRX6njrnpWfeKyYd4diZNIpxhcPeLLHRWuVzFsDYewWD6catCxvueistuAyC6iUSvQSD6vFcsWEYlkeb9dxGHLjMeXI5jLUVjjpqTWgVWfZ2TVT7FAjqvJcGYqSzkWQ1VaiCYGGKg/1rizuU6XsR7K0txp59lQ1iqClp0bD1KKWqeUDvDYP4Wic0aUIKhXsHqaoKbdxpllmy5+jwlPEdiCH1+P6geSRH5Itsu/Kh8oBv/uh/aMRfiAVGj9Aurq6lKGhoe8eCHzz4nt8LR3Ef+UmebsFS2U58dEpRFmHurGWxMQ0uUQC56OniN4bJpPOINVVko8nUfb2sRzrJh2Nsv3+TVxd7eQ3fOSyGaynjhJZXkMDKIEQOZedtG8X2Wohur2D41g34f5hrCd6CNy8j2wxodgtKKEo8WgMe1sTB3cGkIsKSfl2yAvgfuQYh7fuobKYMZUVE5mcR8nnsJ46Rmh+idDkNKXPPU5ochZTRSnR9S0MhR4yKxskYzGyigJqFc6+LtLxBKtvvUdZbxeGxlrW3nibgr5u5EIP+1duojebMfS0c9A/RHh7h4Izx1B8frIaFbJOJri6QTYex3P2JMEH4+Q1Gkw2K6HVDdSA9WQfsQdjqEQRU2cLicMg6aUVVCo1ApBOJDE21hCensfS3kx6ap50Mom2sACdXo9/dgG9LOM43cf2+9cRizzozGaykSh794Y59oWfQjIZid8cQGU1YUorkM9z7PFzmCwWLnz5NewOO/s7Ph5/6SUCs/M8evYMX//qq8TjUT7+wnmuXbnAhu+Qz3zqCf7ujffIZxI015ewt+NjbSvAc4818P6dJba2D3j5iWoGRn3YdDm0KoVrw7v0NlgwiTlevbhGb2sJj7WbGZoP4rHrGFmKcX9inTPdFZzrsPLuYJhUNsdLxxzcmvQzuhDkF56von8mTLFLZnQ5zvO9Fv76fR/dtVb2ginSqQS1pVbK3SLfuBvEYtJRaE6j0sj4gjki8QyCkqfIJTG1uMteKEt7lYnDUJrRpQDHWrycaLHx5u192qqMHAaiXH2wyy9/oo6ZrQxuu4HtwwyPNErMbca4NnqI12nkbKuJybUYxW4jUxtpsukUZ9os3JhKkE6neLrLwtWpFPF4mhf7bMyuRxhfCnCm3cWD1TyZbI7nei18/ZYfckmePerm7aEwZQ4VRoOezUAepz6DUS+RTCYYWQjw3DEP2ZzAn7y9TFuFmTlfDDVqvvBsOXObCdZ24lQVSiRyMh5LnkBczdZejFMtFq6OR3m+18LVB370Rb38/K/85j8qXwiCMKwoStc/OtF8SFtTmXL5tV/7SLGe9p/77/qtfyoEQfiaoiifEARhgm/T7VNRlNaP8p5/spWNIAh/CTwD7CmK0vyh798BP8vDHtYA/4uiKBc+fPZrwM8AOeAXFUW59KH/CeD3ATXwnxRF+a0P/RXAVwE78AD4CUVR0t/Lb0il04TvDJJMp/FdvUFhOEr2IEAsHKFCq2FlYAhPbTWxiRlCoSDWAjfGQg/zX/4a1oZafFduYNDp2F1cwOEpYHFwCIvXTfzqDfRVlSQXV5AtFmKr66zeHaD++FHSySTjf/LX2KsqEPqHyWYz+BYWKaqpZm9llf2NLUyyhKCT2B0bx1lUxNrkJHqdjlQ8we7EFN7Deg43t1CyeWS9Hq1KILC9jXtsGlEvExgeRytJBDKbbN8bwFZajNHjZnN4FINOR04QSARCBHw+4rt77K+s4iovI+HbR6VWszY1Q4VKhV4UWV3fwDI+S3BvD9lgQFVVjk6nY212AdvYDHpRYur6LRpPHCMTj7O/s4ek17HYP0BRYz3akUkQYPbaLSo629iamsVWUYaUTGHt6eTg2m30RR4O9/aITkzhbqglGgwTyfjJzy8iVZWx8s5lao71kgpHCQYOmbh8DZ1aw87MHFa7jXwqTXVZORuLS8QiERKpJEO3blNUWspv/ptf4RMvvsB7Fy8yPr2I265H1Gq4NzzHc0+d4P7wDIOjy+QyCRw2E5Oz+xwEQ7xzYxlZlFlY2eLumIOF5X2EXJbKYiszyz4kdYbyAhFZ0uLbD3JhCHQ6Hfdvb9NU48FskFnfDnAhr6F/bJnaCi+3ZtPsB7PE4mnuz0fJIPHXF+bwOs1cGtEwu+jDKAl43TYu31sG0czKfhpBJTA+u4bcWs43P5jiVFcVw1MbGGQt2ayZrf0IDeUuOmss6CUNC9txylxabk8cMLu8i9Wg4liDlftzIYbnQyTzEjdHFnFaZdQqL9F4Ft9+kBKPja/f3KGwwEqjrDC7uIVKrSKbTbHui5DK5hm0Gbg3uoLHaeLiiJZ8XmF8+ZDSQjuLqz5iyRwCOfSylrsjG1htdkSthqtDm5w4UsnU/AZuh4mzLSoMGjUTS4eYjCYsJgOiSsDtMCCo8uyFFK6O7KEIEnfGN7Fa61nzHeIzGXihz0Brmcjbg2HsJg1Ds4cE43mmRqa+l+nhIyLAD0FZ83fhf/jw7zP/PS/5p9xG+2vgj4C/+Qf+31MU5Xf+vkMQhEbgFaAJKASufFhWBw9rus/xsM3poCAI3/qwRelvf/iurwqC8Cc8nKi+9L38AEkUsZ7sRVhYIhmOYK+tIja3hMFqRe5spT6XA40aubGGxdt3SR4GUWk0RPb92FsacJ09Scy3Q3muB8XtpPJIB+l4HPup4yR3dwnF4gT2/Vhrq6l78hxanYy9poLNkXEsVgum7g40kohhfBpKCjEEQ0h6HYlwGHtPJ0bzwwZiJSeOI1otONtaiIfC2BvqwWRGE41iPNrJ7o07lJ4+gdbjQmW3sXyrH3OhF2/rMeRkCjRqrMe68c8vYuhuZ//WPRo/8yly65uklTy1LzyDkkyhb64ncvUWxbXV6LpaCQyP4ayvwdDRzMGF94nFYtgLvUQGRyisrkTf3UZwdoGSY90IbgdSOEJJYx2mvi5qFYW8oiB3NBMYn6bq7Ckkh41StRq1KJGIRAnOzrPxYIxqkwFZK+Jqb8V+spf1i1fJJ9PIBgP5VAolD9rSYkS9TIlahaW4CEtdFVa9nnwmg+B2klpco6S0FLvLSXRnF3dDI6+88kn+KBZEpSTpbGnmwcB11ja2uXVvjJW1baKxFNXlRTTWlZHLpGhuKOXqnXEqi1188skmvnl5goaqAk63mggdiMwt+YJchgAAIABJREFUBagqlPnFl5uY387ij8b5Vy9W8O5QhCeOmFAJMD6bp71MSzpbiqIoPNlpJJ2pwG7VcabNxFt3UvzCx5xMrqY51SCxsmHnRIuNydUEv/7TrQwvZWgsVBBO13AYznC+y8Qbd+OUFzvoKFNz127kRL2ETq5GySZprzIia1WEEgIWg8i9mSA/fr6UNT901enwOMxsHWZxWEROtHnIKSLnm3UoChgNMsdr1XyjP09HXSFPdxn50rf8TM77EDFj0Oswm2SeOerkvUGRaDJLW7mWcKKCWDzFqUYdkUSapXUTxxpMbPpTKHmF8x0WBBWEogUUmGEpmqG9qYwT9RKTCxK5TIr1XRXJdBaHWUdPnZ7ZjQSfebqKkdUsGSWL3aLmmV4LU6tRPGerECUV07E4674gbptMToHBiVXKvDaeOVrAdijG6ePf/0XDwwKB/2Y5sX8W/L0Onz+vKMqv/v1nHypB/+p/Peq/5p9sM/HDHgeHHzH8eeCriqKkFEVZ4WF3uZ4PbVFRlOUPVy1fBZ4XHoocnQVe/3D8qzxUJP2eE5ycQcwp1H7qYxwOjWEoKUKqrSa+sIwiSyTDEfLZPNV9vdgryhBLi2k4cwoOQ2SjMVLzK7hPHkU4CKLW6zAd7SL2YAJdoRez1UJhdRXaeAJbWxN5f4Dk9DztP/dZNCYTgbsDxCZniW3vkZpewHH2BFqDEcfZk4SGx5m/3Y+hqgJ7ayPhpRX2HoxS9MSjRNc2SG5sovG6Hl62lHS4OlqIz68QuH6Xxp/+MexOJ9lkCqnIQ14vk9zZw93URPTBBAa9Hp3NQmR3HyIxzNUVxHf3SUXj6OxW4skEyVAYDVBy7hF2b96jpLeLgsJCcok4hkIPqiIPiS0f7B9QcLSbg9EpDKVFZGSR1EEAQZbJGQ0EJmbQ5hXsna1s3x3E1N4MdgsmSULSinR+/qeQ9XosDispJU9sdx97STHmyjK0ajXxzW1aPvspAsNj7N0fxtPXTW7Pz8HkLOUdrVSdOoZ/apai6mpi4RCr8/O019bxhS98nl//9V+ntqacjY0tfuP/+F1efv4RfvHzLxKLJ/nES4+xsbVPSbEbm0lDT0sBa1tB2hsraKmxcXdki0wmz8cfq+LyvW2MOpnyYgfBmEKRXSQaiz/UvxPVPNlp5NpEnFsTh/zE+RIGFlNo1Cp0mjQXB3borjUQiUSZWDykyithNcr4w0m+fnOXTz/m5b172/TWmzDpNEQTaa5PROmpMVDtVfN/f3OFGo+K4/U6/vCby/zU+RLG1lKYpDznj9i4MRkmm8tztFZmZDlFIClS4pQJRDLcmUnQUSlR6xW4PHRAoU1LKpkkFMtiNumIxuK82R/g8Q4TVn2ejd0obbUFNFe7ADXnuz2cbDTx3lAAp03mkycdXBgMYpQFXjpm452BIHdnk3TWmPnKlS3OtZt4qc/KO4MhLg0d8Hyvg6nVMFaTRDKRZG4zyumOAgrcLjprHezFZP7tj9UxuZZBrRFxWTTkM1EQ1PTVSYyvJlk9EOipM7Oyk6a5ppCfOF9OMpNlez/K//zpZiqLzMxvxqj0mrBbzf8UKeK7Igjqj2Q/BJz7Nr4nP+rgH0SBwL8SBOEngSHgf1IUJQAUAff+Xszmhz6AjX/g7wUcQFBRlOy3if+esb6+xu7gKEWN9QRvDbA1O4ut0EM+tcds/33qXn4BtVHHxlsXqPjE85jUavYuXyev1aLoJcb/4ivYy0rQzi2yMjiMo70J/cEB8WQM31e+jrOtielLH1B76jjx0SnWJqdQqdW4bGY4CGHp62Lv2i02Z+ZoOX2Kw5v32JxfIJlKYrFYyGXSxLd9CIdBJFlk6fptbCYjklrN3vIKNreTlYFR1LJIQS7H6sg45gIX+tklIqkkysADip97Ap0gsPb6t5DKilm9O4CztATVqMjhxia5ZApJlkmnUsy8+hq1n3wR0WJi/rXXKe/rJTkxw978PEazkb3NbXR7e5S++DR6QeDggzsk81ly1+6wu7xMJpXA4HSycekqjs42tIkks+9fo+nMKZLjM8QPA0QnZlCp1EwOfICzvATdlo/Z969TceoYotnE1N++TvNPvIzd5eTwZj+yKJHNZsnIWvwjE+glmUQshievkFXB/uQ0vtk5DH19XLt4ieSen6bGJvb39wmHw/R0tTM7t4h/b4PRsTHCkTQPxuYoLy3AbDLya//7H/OpZ5pIZ/K8/votNEKaaEUBi6t75DMplEwJV29Ncbyzkqk5H6IokIjHWN0Ok1dApS5DJ8Lu/iGJNJxuc2KR4uyEUtj0cG9qH6PFgcVk5G8uTPPU6RYuj8bw7Uc5OAzz3oCabC7P1EaWpZ0sgUCQ3UCSSw8kDDoNu4EokaiRQDjJ3kGEgaUMQ1Mb/Mor9QA822Pht16b49EOB/fmguglheFF2Nk7JJHM0j+vQxBUXB5Yx26u4ki1zFev71Ds0BJLCGzsHOJ16KkvMfDV6z76Gmxk8mrujvpIoyebzXD13iJ9rYVs7QjMrR2y4dOyf2Bjdz9CMpOFUhcrmwcMOiyoP1Q3HpvfRaPVs7Tu5yAoI4tqHqRkPnFSz/x6gD9+O053vYP++TTXh1c43V3DheEo6ayKyYVN8lkXQzM7HGks4oNJLcNTq3TUe5A0Jq7eX6K0wMzVkYeH8zeHVjh/ooG43v+9ThEfgR9+bTRBEH6Oh22lKwVBGP97j0w87G/zkfh+TzZfAv49Dw+Z/j0Pb6R+lodldP8QhW+/8lK+Q/y3RRCEzwOfBygtLf3I/2xpaRkWrwexuR6dLFOdSoHVjK22CtfWNsGp2Ye95Hd2cU7NArC/tY2k11Hy5GNowjF0RV4yahVGtxMSKdRqDelAhGQsjtbpwFtdhexyIFeVUxKNkcll0RqNhDZ3iFy8irmumlKLGXWhG0dxIclwGIPZDKVF1DueQgkE0R9pIXHnPs7yMuSmeoLjUzR96pPg30ejk7B4PZiPd1OSyaCymNHaLCjRCLtLK9hGJxFUasL7B9jMZjwN9ZgsZuTmOkrSaRSbGbVag/dIK7u//6eElteI7eygKKCrrURQCZREYkhWMyVNdUz91Wuo37uCraiQ+YFBWl56jpxaoLGyjMTyGtZTR/F96a/QzC9iLPJS/cx5lJzC/8PeewdJct13np8sl+Wruqqrvfdmpqft9HgHDICBB0iQBEmREkPUSqc7aRV3EbsXeyut9rQrs3fc0+qklShRooMhSBAeM4Pxfrqnp733vrq895mV90cPFYyNPXAkgZRWwU9ERmVlvlcvqyLqmd/7/n4/2WLC4nRgP9SHSqOhNi+js1lRVZbhqq/FVF5KVpKwFDoIb2wRGJvCv7iIRjRQUWDF3tqMetPDs5/+FDe+8zqy10+LaKXn8DHETR8dlTUsD95Hq9Hy+c+/zPLyMl/84hdZW98iHPTx4nOnUQspmutK0enUGHUKR3qr+c1/e43rt9LsqXdQVaLHLFp4an8Br4UjlBQ6MKiTNFTZOLnXiCBUk8tJPNtv4+x9GzlJ5rFOIwBaxcDlYR93ZxOEkjC/5uMzj9bRFiulxCkSjiY5ub8Wp1mhplCFkrNT4dRT6RJoqyqjvlTk/P0QkWQei1HHU30WZtfD1JRY2Vtv59JYnK88VY+oUzE6q+b7V7dprS0gn1eIx5NcGY+wvOWnzGnk0c4C1n1WDHo9h5p13JyO8sj+WnJSnpn1OKtbAbobapAEhVM9ZViMKq6M+ojEUlSWVNBhVJNIuKgt0tBYqicYqaCv2YpaBfWVDhIZONSk5fyokWxOprlEIR6z0VunxWJQ8eYdmf0d9Ui5NL/yXAOL7hyRaIyh2QDDyybc/gS5XJ59tUYWt5PsbyumvUJLVZGZq+NhyosdvHikiDVPkhN7rUSTOT53uol1v0xvg4G01ACoOLHHwPXxEL/+6TY2ggqlxYUP/d//5Phkpc+CIKwCMXb3tiVFUXoFQXAA3wNqgFXgMw8m8Z8UrwJngd8H/vWPXY8pivKw1qufrhpNEIQa4P0fCQT+/+49EAegKMrvP7h3Hvh3D4r+O0VRHn9w/Ueyjj9gV2RQoiiKJAjCwR8v93H8XdVor6VCBC5ew3HyCNm5BcjkSKsFrNUVRMemAAFDRxvseMHlQAmESfgCiBUl6AQVnsFhDEVFOA71Ebp1D0UtYGuoQ1XoJDM+hVqrJZVMkE4kKO7vQWu34bt4HVmWMZpN5HISzhOHCF25RV6vw9LcQGRqFimeoOSJR9g8dxlVPo/rQN9udORUBjx+LAd68F+5icFsIl9YgC6TJReOYD7QR3BwCP/sIjWffpbk0Ci2w/0kRyeQEkkEjYZMNkteyVN0uB+NQY//5l3S6Sy25nqESIxcIITtcB/+yzeRdRpcB3qJTs4S3tyi8rFTRCdmCK2tU9jWjEYUybm9FBw7QHx7h+jMAgW1VYQXl8kmU1Q+8wSBmwPk4gkKTx4hMzNPRsphbaonOTmHlMngfOQo4eu7C1/b0X4SAyNYD/YQvn0PxWjE4LAjrW3S3t9DdHWT/c1teHfctPcfYPDDs/zyV7/KlQsXyKXSvPDC83z9639JOBzm1KmTfOPrf8Kf/d//Cp1Oy+s/+IBIOMzLLxzhrQ/uIOUSHO8u4oOr8xzYY2drK4osZ7g95uYXH6vi7mKOVDLNqb0iw8sZcrJAhT3P5Gqc9lo7iWQai1mPWacwti4jZZMcarVzYSKFUacQS0m8/Fgt3z23QjiS4qnD5Vwf9RAIJfnFxyswG7T83ndm6Gwuwmwy0tcgcmk0yt5qHYmclhV3gse7TLx900dHo5O2Sh1//v4GTx0oZm4zRVO5gTuzCR7rsnDunpfuJhfz2xnOdBm5NZchL6VxBzIc2uOkpkjL1ekMelWGMpeJJbeEoIITbSLvD4aIJSWO7TGzsAPJZJyTnU7ODcew6yX21ppZcEt4IzLP9Vu5OJEhmUzxaOeuP9H7t9186fFqLo4nSKZyPNFj5ZvnNzjTX0FzuZabs1niKYlii4TNamJuO0eFXUar1bHsyfJUr5VL4ylqC/MEk2qKrRI3xoMc3lvIijdPJCnxbJ+F0aUoQ/MRPn+qgovjcZ7ps/LRaIInui28ddtPW89jfPaXH2p74W/5h6rRuvbWK5ff+oOHKuto/MxPbOvBYNOrKIr/x679ERBUFOUPBEH410DBf7u38kkiCEIRoP/Re0VR1h+m3s90ZSMIQumPbTa9AEw+OH8XeFUQhK+xKxBoBAbZXcE0PlCebbErIvi8oiiKIAhXgE+zu4/zZeCdT/p5M5kM4Rt3SOdlpr/xXSpPHibn9aMWBPzRGFIkRiwUokynY2dxiVw6i72+BjUK62+fpbG/l+C2G5fLiffuIAmfj1QwhFEUUe/42FlYJJ/PU9ZQz9rsPAWlJWTWt0imksR8frQaLVq9ntzF68jxBIF5D/UaLUadnuX1Oaxj08RDIZJeP1anE3VJIRtXb1J24jCJsand4JsrK1S1t7O1sko6maBGqyO0uIxKoya3sIwsyYz8yZ/T+MwZFLORyPQ8WouZwPIKBQUF5ACT3sjGyDgFTgcbYxNorWYSV28hyDLeyUXs9gIMKjVLiysUlc+jNYhEtrYpcLnwbbtxdrTjvnQDk17P1vAoqrxMIpUmF4nivnIDjaLgXVvDOGxhe34BjVZEL4pszswimowYhyeIB4NE/AEyUg5ZpSL03jmKjhwgtuVm/dxFygoKSGzu4B4eJ15YgknU8yf/5v/g0KHDzM7M8NHZc/R0d/Pqq6/h9XrJZrNotToEAc5dGiCdyXHhyiCFBSZuD8zg9voxqCXWt9TIUoavfeMWpw62srUTIhSMcmUizu3hJV44WkE+L3JzdJv9TVZGV1QMT+9QWGCmpdzAvSWJeDLNi4cc+MNq/uC1GZ44XIdGq2NkdoWr44VEYnFUghopk2JvtZ7vLXq4OREgIwn4Q1GkfBkmHWjVYLMaaSjX8+4dPzu+GEPLOmbXAxQVOdkKZdj2hJneKkQQdPzx96c41tfA+CYsrIfobrRT55L56/MblDgMqDV6xhbcFLsKGF30k8zkcdhNVCNxc3iJyhI7FyQnixshZDnP+IYJbyDK6maQdEYmnpaYX47T02hhYNrH50+VE4lnmVncorVmNzZchcuIL5LlyniEDXcMtZDnzpSEL5hgdClCJmdmfMHPMweLcFl1fP3DdSRJRq4u5vboLN1tZdyZVbGw6mV2RaC3xcV6UMP8ehir1cqdsVUqi22cG9Ug6oxsejYYWspSZJL4xtkNPneijO1AigKLmvNXbv6dB5t/OD8Tp87ngBMPzr8FXOUhN+3/LgiC8Ay7PjZlgBeoBmbYFXb9RH6a0ufX2P0BCgVB2AR+BzghCEInuyavVeBfACiKMiUIwhvANCABv64oivzgc/5n4Dy70ue/VhTlR/rFfwW8LgjC7wEjwDc+6e8giiKO44fR73jIp7OEZxZIRcKYnE4qHz9F+NYg9uIirIf7kPJ5Ev4ApSeOEJ2axVlThaGrgxa9iCzLWA/04L10nYIiF8a+LgSVCns0gqHATiocof6px1AJKsTaKnLhKEWVlYQjYcLLq1S/8CS+sUnsagFdWxNqgx7VxCRiSwOucBjr808TuTOIKLiIB4NkEikK97WTCYUoNtVi3N9FVVcH6z98F1P3HopliaxahbamklxexlFWjmDU47t6m0wsRllbM7ZMFnV1ObpCJ4G7wxTX16GprURcWkat1lB24jCpQJBMNIqhs53Q/THaX3oe2RdAVejE5HRgP9rPyh//BTqrhcozjxBZXKLtM88ju727jm5mM4XHDhC8dpuSxnqsB3pIazWkNrcxd+6hIhYnJ0lom+sRwxGKzGacxw6R3Nhi5ofvYq2qwFhcTHF9Le0tLcjBCAf7D/ClT3+awcFBCr/0Zfx+P1ZRTy6b5eWXP0coFMJms+L3+xkdHeIP/+Nvc/XqVT7z/EmCfi8oOVqayrhw/R5WI5gtZeQkgdaGUk53mXnzaoTO1ioe36dned3C4laCnWCaWCJFLGvjhUOFhOI5CkwaRhfDXLvvpqHKxTt3Q6hVOZrqSjnVV8VH9zy0NZbSUaMnHi+io0qDOywQjab53z7bwtWpJGf6rKzvxDi1V0TUqPnTt5fQajSEwmZWt8JUFNuIxdO01JZwao+O88MhTnSV0lSuRq3KU3C6mXBKob1EYtphIpnKsuLJsuYO89KxMjY9MZ48UI7DKnCi3cl/en2OQCRGocFOU00xtaVGSqx5bMZyEhk1p/aKfDAoIUkyzx4uIZrI8vX3ktycDLLji3B7ykBdmRlJkphbD5HJpklnFbz+MBp1Kb/+XA3XptKUWHM0Vzez5JEptAisb/tZ8znxhHMYtRqKSh083Wchr1SSzip01ooMT2cxGIz01BuR8nlu3tfxWKeJZKYCtUrg9D4j0USW7R07NUUqFjbyuH1RLozoqXSq2PYrnD5x8pPuIn4yD8LVPCSFgiD8uNnl64qifP2/KaMAHwmCoAB/8eB+8Y8m8YqiuB+sPH4a/B5wALioKEqXIAgngZcftvLPnTo/hrfPneWvZscQQlHM3R0ErtxEJ+pAUVBVlaPN54n5/KBWY3UUkLdaSC+vocqDqWsPyfFptGoV8WSSgn3tZGcXEWorkX0hZK0GvQCJ1U1ElwNzRxuBSzfIqwQK+nuIDgwjOgsQWxoIXr1NDoXSR48TuTFAvqwInSiCP4RKkjDt7yIdjbPx3jmcPZ3gC2A7tJ/U8Diq2kpkb4DEjhc5m0En6ig42IdGLzL/7e/h2ttGMpUmtb5JSX8PgkHPynvnafmlLxC8fANz717y69vsLCyi0+qoePYJ0j4/0uoW2USCrCxj39dOftuDubOd4L0RYltuxNJiJH+QkpNHSO14yaxukIrFKX7kKNGFZXI7XlwnD7P61gdUnT5BXicibWwjB0PkLSaMpUXIWzvoO/cQuHQDg82Krq2RzMIKqUQCa1sLyckZHMcOEh8cwZyTOX3kGJFggKcPH+PcubP84i/+IouLi1y5cpXi4mI0Gg0bGxv8yq98lZGREQbvXONXv/oy33/zfaJBD48ebSQcjvPhpfs8eqSFgZEF1EqW/S021rdDFJoUZlaThMMRVGoVJztsXB6L8minjfvLOUR1DoNBTygBoWiSrKTwwtFSLo4E6Wuxc3sqhE6no6vBhDuU50CrjVcvrOMwizyyz8il4QDrnjhferSUZXecb364xG+82MCd6SiyWs+J9l3zVzyR4Zn9Nt65G6S6xIw6n6TQpmcjpOJ4u4FLk2li8TTP9du4NhFhbSfG50+V8/5QDDV5uupEVvwCkXCE070uPhqOcGqflcFFiUQ6hyxJPN5t5dp0hkxG4rkDVs6OJJGlHL1NVixinskNiZ1giid6bPzhK7P85qcbuD2TpL3ayJw7j16Voa7UyJI7gajOk5XBH1eRl/PodFqe7bdxaSRAPi9TZBNJZNWU2vPEMhoMWoloRoMnJHG6w8DbA1F0WgGrXqCt2szQfIieegMzG1nMJpH2Ci0f3I8jKDmeO1TElckUqVQKSZI50+fgvcEwz/TZ8JmO89wX/uXfqb/4B5vROhqVa+//8UOVtVU/9TBmtDJFUbYfDCgXgP8FeFdRFPuPlQkpilLw933mj2l76MEe0RjQpShKXhCEQUVR9j9M/Z+Hq/kY1ldX8d4Zoqi2hsTgCFIqjW9zE4PNRnxqmsKGevR6kbWR+5gO7kfxBli6sTtLTw5kUKUzqF1OCg/14Tl7GWtzPcZCJ+HZJXKZNJqSEpaGh2k+dJDk/XGy6TQhrx+zzcbK6PiuX87gCGqtlo2xCQStBq3TQeD2PZq++BmCi6vo7Ta8d4YwKAqhzS30ZjN5BWJnP6Koax+GQgfBqTkMehFPOEhkaxuD0UAqmSLs8WCqryEbTxD1+jBubYOcJx2LkZmYRmfQM/U3r9LY10cyECZvNhGfmkUtK0R23MRDEYxFLlbePUtlXzfJkUlIpIh4vNhkmWQshnN+GZVKIJZMkgmH8Y9NIqVSBFdWMVSUEtzYxDW3jLaiBDkYQmUxYevcw+r33ianVuGMJcjE4/i3tqnWaNiemcNe7EJrMaOrqWT5zfcwFjoJTs2xVOhC1Bv43X//u5x54gnef/99kskkV65cpqenh3vDI3R1d/OX3/om0WiM8dFxiqobUJmKGPzoQ+RcGCmbZWhkCr0OllbcpOJhpGw1JQ41P7iywZmeApZWo2z70+RyOUwi/KfXpumot4NKw7bfTyYnIedBNOi5MWPHYjbx7Q/nKHJasJklro6k6G0u4PpYkGw6x8i6m6xUgZJXs7bp59a0FY1KQs7nuTkVY3DKzZPHWpnalLkxtERvWynja2k2dsIYDTqsRgN/fXaRx3qKuDgU5e50AFHU8C4KGo2W1e0I12aKCEdTuL0hUJyY9GrcoSx6nZqn99v5f99Zx6CDaDqPBoWbJiO3R1aor3JyYVzHreFlKopt5HI5uhuMeCN5spLCpbEk+bzMtYkwNquNDwd2iCdS1JVZGZrz01BRwDP9BdyczfJMn47f/uYEFr0WlaCgVovcGl6ku7UMfzjBltXMp4+YAJHLYxESKRk5r6fcAZeHtvilJxu5NRvHKBqxmbV8cHueAx3leCIGDAYtwxNbFBXamVzYJJtVqC2z8jt/PUZvezkXx2K46n72sdGET9iMpijK9oNXryAIb7HrHuL50RaFIAil7Jq4fhqEBUEwsxsT7RVBELzsWqIeip8PNh9DeWUlxS1NmLt3ozFkr9ygqrkZqchJNhKloKUJXVkJxtV1dPU1aB122tRqkuEwtq49bJy/jG91lQZFYWt2Do1eRB1LsHxvBJPLQW1/N23Hj5HLpDH2dBC9eIPKthbkYifWYheW3n1ojAaiSytYNrcoOthHam2TmNdH7O4Qoc0tFI+XmidPk1jbYM/nPoUUi2NsrOXef/6vFJSUkvcG2JydQ6PTYXQ5sNfVoKurQaPT0uR0oJZkkkYDotGIs66G6JYbR20Vxn1thCZmcNXVYu7eS72oI1/iQhWJYupsJxUMIZotZC1GNOEw5r2tqFQqkhevU9uxh0wqjcFmQ9fejFqroSASRTabcB7ux3PtDq7aaoz1tTQ99ihCOo2kwOydAcpbmsiEI8TDEYpbGnEcP4h86QbmwkKM3Xsxe30EN7bIXb9DcttNLhKl97kzOBqaSfgDHH/macZv3sLhcHDkyBHy+Ty5XA6DwUB5SzMBr5cnX/4cr3/7O/S+9CI2q4OqmhruXXuPzz3ZzqXbc/zb33iGpc0EBlGHqC6hq96EJMu8uuUn0Oqk0GnF7nDx6SOFJNM5pteSlBdb6Wm0cnk0jDecQa9VUBusPNZfhE6nwRdJEQjF8az4UBQ4vtdMS5sVtzdCY00Rj3Xoeet2gKbaQg616HnnTpJT3cW4bBrUqmIseigwyLz8WDNL7hR9DQbSuUrKbBKz7jwCAjUlZgosOvxxhQK7mcc7zXx4L0J9ZSGP7hV5J2nAZRc50GxixZtjbWuD82MufP4omWyWQ3sr2PAmMBrNnGzTolXVEYymOdKsJZupIpmReLLXxpo3w8T8Fi6biNZp53//Qisfjac43qYjkTSTV2yc6bHwykU3CxsB3hNUjM+5UfKVHGxxEEyqebzbyqYvSSZdTEu1jfVtmasjm5QVGlCp1ATjEgurPj5SS8iyQl1lIe5AnI2tCAp57KZSqksL6Gp0UF+i44PBIPXVLo62imz7CtCoBJ7otpBM52ipKqCjWsu65h8hNtqD5GmfyEcJgglQKYoSe3D+GPDv2d3z/jK7wqmfyv71A54DUsBvAV8AbA/afyh+bkb7GN4+d5Y/u3AW+/5uIpMzWOtrCI1Ookag4MRhImOTyMkUYmkJ8sYmxu4Ocu4dxKpyts9eorC+DtkgokNAjsaR0inyooi5qpzw5Cybhi0qAAAgAElEQVRiUx3q3O4MVlSryXmDZFJJBKDg+EHi90Yx93USvTmIUFWGRq0mtbCCta8Tz+UbOGqqyEViWI/sJ3pnCPvh/STujxPz+THsaUVIJJEiMUxlxWTWNpDlPAXHDhC4OUBkx0v1c2fwX79DVspR/uhxAlduodPrkVwORLMJaWUD66Fe4oMjaPR6jJ3tROYWyHj8mEuK0ddVMfn1b1Hc0ozzQA/hkUlsbU1ExqfQG03o97WSuD+BtrEGxR8iq1Eh6g3IW27ypUV47t6j9unHic0vkfT4KdrTSnh6Dswm7PU1u46jsowSS5BSqxA0Gkx6kfDqBgZRR3N/L6aKMka+9xbPP/88JTXVXP7Oq7RV1iBqNFRVVbK6usrRo0f5D3/0h/yv/+e/5/7duxQUFHD3/n3an3qMkTffwyLL9O6txSLPMzS1w2fO7OHbb49QYNHyxOFqvvXDYY512FhYDZHKyEQSMu1VOpJp8ASTdNRZuD0d49QePfdXJA616vn978zx21/t4dpUkmgiw+n+EjZ34py/tUxZkY0XjxTz/h0Ph5v1LGzGcQfTtNUU4PFFKbTr8Cc09NWLfO0Hi/zGi/VcnYgRjOZ4+UQhgUiWb3+0gctppqfJQXOZhsGFJN5I/oEaLI1Jk2UnmGJfowuPL4zZoEJW6emo2o2llhcEBDnDsY4ChpZyPNVr5f2BEJKsIKplYqkcT/e70OsE3hmMYtSLHGvRcnkyRWu5mlBKjSeYxmkV6W/SM7MWI5RUENRa3P4kJQUayguNjC4nMYt5msr13F/KoFLB6Q4zN2azpHMyz+638fadIHkEznRbOD8Sp7VciycK8bRMfZFAIqdhfSfOs/0FvDeUAMCklehttnF1PEp1oRpRbySXTTC8GOOFI2Ws7iQYmPLz5ccrmVxNotWJFDSc+pmb0br3NSs3zv75Q5U1l5/62LYEQagD3nrwVgO8qijKfxAEwQm8AVQB68BLfxdJ8sMiCMJvAd9XFGXz71P/5yubjyGVziAbdEQGhxEtJtKpFPFohKQvgGgwoNdpmR4YorihDkmWcb/5LlWH+8mMz7Azv4jaaMBkMjE7OoGjvIxUOIqiAlU2hyIITL/yBhXdnahVKlbmFjAXFyPLMlIiia6lnkwmS/yjq7iOHUCt1zP1F9/EUVVJZnIWz/Lq7kNqtQS+/w6u/T3ExqaYvXyNukMHUCIxtgeHqD56ELGilLjPTz4YwjcyjlaBZDBAamQCQ2Upget3iCws411ZRZYkyg70snlniPpPPU3C42V9agbRaKBcllHlcsxdukpxQz2lmTSCRo1GENg4ewlbRSlpr4+NsQmKmhvJL66SyGbIXL1FzaeewSQIrP/wA4r2d6GvKGP74nWCA8OIGg2euXkKSosxOh0s3hnAqFKhFgRWR8bQWa2U1FQzf/02bQf7Ca2uIVptmMNxku4xgvOLeCZniC6uolXg/tAQ+/v388G5c+y43Wy43ay5d3jzB2+SjcfIJpJoS4vwbW6hddoZOXuOQz0tvPfhDEd6a3nlnfssLu6gKFmyWZlsTuFr3x7kN15q5+ZkCIvJRH25hb94ZwGDVkV7lZ5CU46vfX+d9voiLo1BNJHmxpzEtcElKoqtDE4bAYFNd5BCi8gbVzawGQSujCXRikbGFwLYbTbsZpH37+7wmy/Wo1IJqNVqrk+E8UUV5pfdDJQaEARY2wlRV+3CF1NYGthhT62dYpvArZk4GkUmlICJJT82ewFWvYG3b66yp96FL2pmYsFNSaEFg17Hn/1wgYPd9VyaSJPOKaxseNjXWsXywirDxQ4EQUEtyNwbXyGTLtlNSjYTobutimtDq5zsb+DCeAa1SuTC7RlOH24lkZa4NuzleG8dyVSG2eUQoqEWlUpganEbh7WeTU+EaCzJFZOITqdmct7NNb2WUDTNDxa2ON5Tw5Y7gJJ38FSviM8f5gc3/TzV72J8McClER9Gkwm7zcRbN+Y41FVPLJZjbsXHjQIzapUadyDBrXkZjVrP3JQHvfsuz33hZ92LfHLhahRFWQb2/XeuB4BHPpFGPh4rcF4QhCC7KuAfKIriedjKPx9sPgaDXsTR3MjEN1+luKUZq9OJqboaUStiOtBDPp+nIZdDMJtQRJHgB+dQjAakXJa9L38KJZ4Em4XSnISxrgrL+jaCIGA52IchK+HyeCns2IOuyEUuHMfevZfM6jpJTZhsMIKs5NlZXMJaWkwqlUZWFErPPEJ4bIq9X3yJ5PQ8hn3tjP/Vd7C2NeNoa8I5v4CpthKV1Uz6cozg4gr5VIp8MMTi4H3qjhzEdqSfnMWEpqKUmMdHMp7AYLFQ0dqClM1iLi9j+eJ1EnOLqJ0OCstK0VstGHs6CM8uUrZvL9a6GgRJpnBPK+a97Wx86zWkbJbKpx6jrK0VY6ETsaaKwPY2gfUNzJdvotOL+NbWsZcW470/RioRx3loP4Hbg1Qc6ENTWkJ0Zg5XXS2G7g6kZJIKAVSCCv3eFpybmxR0tKEBaupqKauqxuSwI8ZTtLY0s7e7mx9+57s0VdfwzPPPs+3xUl5fhy8U5FNf/QqCTktVcxOv/Oc/YXNuhgo5jS6eoq23l3QyzsTUKsUOE58+08EP1Vpy2SxPH68ikSrG6/ESTKrwh1NMLHix21qRFDV5QcV2KMeqJ43NYuRTx8q4NBbiF57Zi82h5fiBFrLpDKe7bETjadLRajRqFRfuLHO0s5Sn9rs4OxSkvamCo21Gro7s4AsluT0bR63R4A/G6DtTxdXJJP/TC42s+QUqHQpferKVVE7NkVaRP3snw07IR1tDKe9em6GrpRidqGdvQwkn2kWmV8KoBHi634FWo0KrrieaSOMy5tBqijjTbSYUzXB7TkNvRx3haIKG6iIONmlRqwQujqapr3bx7AEHH9wLYbeYeLLHTCpdhVYt8EiHyLWJMI1VBRxt1vBeVEff3hoONYsEQ2o626o42mrg7bspWqqddFRrQBLxRQ2cbNPxzt0YHS2VnOk28/ZdmeqyBg636rg9nmMnsIXNXMt2KE84mmBs1cbSToaacieHWwxMroRpqiygv0FkcCbOrz3fzIInz9FWPZOLOg43azGIGnxBMw17Wn72nYgACP9sUgz8LvC7giB0AJ8FrgmCsKkoyqMPU/+fx6/wUyQ+vcCeL76MTqVGX1yEKhzF3NpMZnWD4N17GNpa8E9MIeZlOn7lS6jCUdThOKaaKqRgiNzqBgX7u9i+MYCps4O800Fmy0345h2qXnyGzNIq4YlpXP09xFfWMZaWUv7ck+Q2thEyORrOnEZtMKCkUtQ+/gjp1Q3UyTSiqxBNbRWJxVXK+7owG40ERiYpf/QkqcUV8nKehtOnMBiNaIuLEAQV9YcPoK8uJ3DlFqKcx3tzAG00TlVHO/GNLYx7mtG3NZHa8dL8xCmywTBJt5eCzj3kTUYyHh+CL0Dp8UPoTUYoKoRonLV3ztL+lS9Q1LuP7PwSZquFfDyORq/DqtLS8au/hN5mRXHYKdrbhmQxYSspofkzL5Jd3cBkNlO8v5vs0ip6UY/r+EFSs4tE741g6dlHJp4gcG+UtpeeJzgyjtVkounwAcbuDjD+4Uf8wr/4FabGJ3jjm9/ixZdeQpbzfP+NN+h79CSJWBxHRTnN3Z2sTU6jUqkwlxZR1d6KudDJ/uoGXvjKV7hy/Q6/9ZXjiBqBm/c3sFlNnDrUxL1JL+9cnKem0oVGrdDXWcevvdzL+k6Mwz21OOxW9jY6cTodPH24nLdubFNZ6mR/exFXBzdorTFRXKhldSfJ+QEPj3Y7dx0k+xrQaXWseiVMBhF1PkU6KxHLGfjtL7cRTkB7uZrTB2q4NBqkqVxPqcNALJHh0liU7noT/kiGfD5PW30ppcVODjZqOHWwEUneVW0daDIxu5lmySfwWy81cnkiyb3ZEI0lAk/3WvloyEd7hcj9xQRXJhM82WtldStAc6WZRzutDC+nuTkZo67Mgl6rIpHKYTUbaChWM7ESo65ER51L4YN7YVRqkUc6HXw4sMPeGhPxRBJJzqM3mUml0lwbC9FVb+H5w8UMLmQIpbSYRIVIPIfZZCGVSvHhvRAn9phJJNNE4lmOd1XwlSeriSWyFFhEaisKaatQU17koLPGxPJOhmWvwC88VsXISpacoKem1Ex1ocCfvrPCLz1eweR6hjdvBTjdZcNZYP/Jf/hPHIHdbvZhjv9h8AI7QAB4aJn1/1Df8GfN+toavpVVZJWA48RhYnfuYTIZsdZWkVxdI7a+SWZyisDWFhmfH2l2ifmLVwj7/SSHxpm9dQcBSI1Nk47HCQ8Nk/T68dy9h9piIh0MEwyH2RkeQ1Ly7EzPIGczZMdnEA0GthcWUHkDrN26i2dukcTKKlNvvkcuL5MYn0YVjjJ/4RLWqgriaxtoUmnEAhvqqnLW3z+PWFmOWGBn9G9eQWcyYSgvRfD4kUUt2UiM7fkFVCoViWgUHaC12zAWFbJ27TaR9S30JjOb9+4jb3lQJ1JMvPIGWaMejSiS2HQTn13AYLGQjEaJD0+AN0B4bY21mTkisRgb753H2t+JAAS9Xtav3sRZWMjyhStodVoEt4eZD84S2NomdH2AuZt3iHg8pEammP3oEiq7DTknoaooIR+MIJpNiNUVrE1MsnzuMkIixc7qOu++8jpryyusLC1z89p1rly9yszSItcuXuTc+fMk0mmS8TjOslJ++Fd/g7NrD5lcjvCFm+SyGS6//Q4Lc7N4QjlsditXb09yf3SR8Xk/QxNbWI1aNt1+3ri4TDID48sp7oysMrPkw2Ay8m/+9A6ZrMR6SMPwvJ91X45zQxGW1j3cn/YTT2YZnI1g0qtZ3EpQYDMwNrOGSmfgO2fn2PFHaa8x8c3z6+xvENHrNLRXqPjjN5dQqTTcm3TjjSjcnM1i0Iusbwe4NpWm2qnwjbMbdNVqqHUpfOPcJnqVzNTyDmueNIpKw+hynPoSEaNeQ6FZ4sJ9D8tehQvjGSRZZnYryfc+mkWjVvPBvQhTC27WPWnuLed5/+Yy3kiKqkI1NS4137vu5VCLgX2Ndt65tY1aUFjxa7g7vsHsaoBFn5Z7s36kTIpj7Sa+e2GThmLIyxI3xra5Px9iYCZAJpNCq1FxusfBueEwRZY8y+s+Ftd95JU8oirNh0Nheut1+MNpvKEYc6s+HHYTf/jKFA6TQlOliemtDHbzrokqmUqhUWsYWwyzsAObnjADizLfvzBHIpHiyniITfdPS6T1ExBUD3f8E0cQhF8TBOEqcAkoBL76sLls4OdmtI+lorISg0FPcnWdvEpNKhbHu75BRV7Bu7SMpbwM25F+qjMZxOIijM31OFbWcHV1oOj1VPX1oq2uJL60QmlDLa7jB5GzOaa++SqSIGAxGlFpteiNBrK5LGq1BnN7E2qtltj125Tu24uuqQ5HZtc/xn6wF1VWQu9wom9pIDwyTsOpE0QWVxAyWfwbW3+bvyay4yVwbwT7vj0UNTWgrSxDUhTmb9yl/StfJHp/lJaXnkcj54kMDJGNxEglk+QkiWw6g6O3i7THh72yHG17E+pMBufODnIux/pHV0j5A7R++WXysoR5fZOCI/0ASJdv4KypJRiP4ZsdR31zEPQGpHSGotpqjJ3tOBcW0RQVoisvoXh1DefeNtSlJTQZRHKxBLbDfVjm5tGVFOG9M4gulycVChK9fpfs5jY9ezt54fMvc+HV1zHV1vLiZz/Ld779bfIK7DlxlHcvXcBSV82e40eY3t5kdm0Fr5wlF40xcv8+XU4b7oUlumrrOXXmDOe+9zqHDh3gQGcRKgGSWTUqlcBTJ1v48MIg2bSJRFbFs493cqizgtllL7VVJ/D7wtSX61lcKuJ4p4OJ5TCnD9XR2uSgyKEnnkxQW2aiyqXlv7w2glZQqD9ehpLLUVnq5GS7SC5XSzqdYWYtwcZOhKGlQiQpjscXQasRcFngy0+2sBPJc6RFz9WxAHVVLo61iZwfiuH2xxhYlAhHcyxthniyr5AnjrTi8cdY3kozOrWB1VDPwlacHV8Ek0HLkRaRnVAGl6WRWAbUOiNVLh2ddQZMhhbyeYUjrVpS6UpWt8O8dXMHVFo23GHuLJQgSTlC4RgmEWKpDL/6fAtDiymqHTnKCq0oKjXT27C0HaGwwIysqGmtL+GZ/gJC0Sw702Hm19xoNFUMTW9S6myistSBKIqMLkTY9MtseQLcXTBT6dDTVqnCZNDTVJJnptSOQavw0X0f96Z9lLisyJSQlTXMrmzSeqoWXzTB4/sr6KhV4w8V0V7noqdBZF310/J1/Dh+tLL5Z0E18C8VRRn9+1T+uRrtY/jbTJ2XbmLa20ZicgaTo4CsqMNo1JNKJEGWUBuMZDa2UHQa7J17yUzPk04kcZ48THJsCiWTQ7+vleT4DJlECkt3B9HBYQqOHyY5eB/93hbS8ysoKJDLYX+QqdO0v5vQtVvorBYykSi2/i6ya1skPT7yGg226gp0FeUkB4dJx+OoCh1YilzENrbIqdXYil1El1YoPLyfzOQsaUCSZXR2O+pYHEv3XoJXb6M16JENBgxmI0KRk+T8KoJKgFgcU98+UgurpHc8FD56bDdGmSBgOdBL+PodhKpS4hvbFO9pI7G6irWxgfT8Mtl0BrG9GWl5DUPHHjITk1BUiFaWkZJJ0h4/itmIvbGexMQMsizhOHGYtD8A/hD5bBYU0NZXY5hfwWaxoFepOXnqFEPXb1K/pw2bomJ6YhKzzUrL/l7Wl5e5df0Gj/zyl5i+dReNqMMjZ1EEAW06i29tA8e+dvLBMIlgEKui4qUXX8Q7MkBPVxtT995hZinIFz51mB+eHSEWi/H8Y3sYGJoFlYgvGOOzTzTwvbPzfPZME+9cXCAaifLi0WLeuLyJyajnmSMlnLsXJpVO8dzJWt68sMwLx0r48JYbVT7FnmoTI8tptPkEibTEwbYCCm06vncjgEEnYLUY2VelYWAhQy4noVJrebrPwrXxEI1lBkZXc4gk6KizcGkiiagVONZm5M5Clsf3mfjhnTBlhSKHmg384FYYs1GH1aimvULFyIpMfXGeREbDwnaGZ/utvDsQpdBuIJzIUWyRKCs0cn8xTjIt8eJhFxq1wJu3Q6SSKXqbrfjjatz+FPsbNISSEEyoeaTDyOpOgvfuevm1p6u5NJnCaZSY24hzssvFqlemq0bL4LLEiT0m3r4TwmEzolZSFJg1eCKQR0WlPUcsp2Y7IFHnUrEdhkc6jJwdSaJSqUinszzXb+XSZIZCs4xe1LPtS1JbamB4KY4ahSd67NyaTXG8Tc9/fW+VL56uZsWTQasTsdce59mftRqts025dfG7D1XW6Or5J5mp88cRBOEI0Kgoyt8IguACzA/SwvxE/tkMuT8NUqkUgWu3MZpNjP31tzFWlpONx1HFE7udcjDI3NkLpDe2UPIy7skZEmPTLA+NEg4GUWSZ2JYbobCAbCDM+tg43sUlAhNTGDr3Eh0aQWWz7Aa7XFtje3qWrEHEPzhMRqPGf/kG6+NTRNxu1GVFrLz1IepCB7lUip3Jqd04aoPDLAwMkYzHIZpg7v3zhNY3cXbuJbfpxmQyodbpSEfjqFMZ7O3NLL73ITKQHJ3Cv7nF1swcSjjCys0Bln/wHqb2ZrK+ABqXg6Q/yOz5CygCpO6NsTY+RXjHS+LeCPFUgq2L1yg90EtsYhqtViQdDDFz4xZqo4H81g4hj5fpv/omWbsFY4mL2PIaiXAEScnjHhlHWl7Hv7GJf3ObzOgUgtvL2t17rI9N4p6dJ/jhRZxlpYzevIMUjrG2sEhdSzMfvf59YvE4Pq+Hq1evMnzzFgH3DnMrS1y/eIlAIs6733oF//wyuUCIkctX8Wxukl5ZZ/jD8+wsr7K0uc5r/+X/4cnHj1FS7GJqwUtjfTn3xta4cmMESZKIxjNcvbvIkZ5yeveU8N13RulqdZGTZCZmVtl2+7k2HsETiDG/vM210SDb2260Wh2iTsOzJ2u4MOjFps/TUiHytVfHSCQSiAYjw7M+7q/IXJ3O4faGWd0KMb8a5I/fnEOWZQYm1gmFw0ytxdlTbeD2TByjTqbcZeAPX50mn0sxu7zN//X6HGaDjstTWba9YSYXPNxbzBBPJJld3mFwaps3b/k51CJSVWRidDmO3QSjywn8wQi3RlfRC0lGlxOse5NEEtJuSoClLHfnM2gFheWtIAvbWc7dWiCWSLIWFnntwiKJdI6LExnm3AqRWJIr01mWNwLcnPBzsK2Av3xvka5aLQa9hnQ6zdlBDz0NBja9EbKyip1AjOvDyyyselkP63j9/Cw6rYaNsIpANMV//O4MgYCf7Z0gRTYVY4shhibWOHtni0BcQa0z8qdvTmLUi9jMWv7otWmqHRLr3hT+cIq70wE2PDEuDKxy/fa9f4ReRABB83DHP3EEQfgddsOE/SggshZ4uJGUn5vRPhaDwYDr5BH81+9Q/9gpYn4/GwP3aH/kBJmNbQo62lBncugry0lvbFG2bw+mliYK0ymkeJLE9DybcwsUSRKW1kZcdbWoNVr0VeUoHg9LN25T2bGHWCCAqNfjKC9HYzAye/Ec1Yf24zx2AL3dhpJOIyfTJIIhYqsb6I0mSpubsPR3kY0naNSL5NMZzPu7kbMZMukMyYkZ1kYnsBQ5ERSF7bl5dCYTJQ4bWlHE1NaEWqfFFY0hCAL2o/1YD/Yw+id/SejWACv3R2g06kn7/JR17MVSXYmhsY76fJ68JGM+0ItRkoh+61U23j9PaNuNq6GewsoeShvq0Lsc6GuryCkymVAIFSpii2v41tbR26yUnT5BdMeLsW8fhakUUi6Hdk8LGlFHgT+IyVmAe2KGio69VB/u3/ULqmug5/hRLr37PlqNhobGBrbW17AXFfH4S5/ijW99h84jhxAbajEVOmhLxFEJAo6uPTTJeVKRCOWnjiBlsiiyTPOzT3D3d/+Ar3/jFcxWK7fuzaPVmWiqdfHVL55hdXOHTXeASCzBB1eX0Go13BleRhE03J/cQKvV0NngoK1aQzpVQDyd53inA5/Px+DoIgV2I3IeLt2do7OhGJvRRFWZk/5mK55QispSB8fbDYhagWy2FJtRIBjNYjNrOdVhIZstQ1IEHIY8k8shBsa3aa+z4fbpONRVy/MH7LxxXYuo13G8TQRAkksoMKvpbhAJJQtoqhXJpqKMzAe5MOwnI8H43A5Co5PO/UWs+ayUFDspK1JzZXieJ/uc7ITydLcU01qmQSBPMKalv62Yox02DAY9Bq1AtVOitcZFZ52JqkINb95O0d1ey+kOPfmclVA8R06SyMl5fnhjB5PJgFarY2B8HdRGRqc3qDhSQ1OlmUROj8UkcqxNJJOux2YQ6G/SMzAnoaaQ2iIt797exmpUcbDVTl2FE5vVyJEWkXw+z/C0lQONWgQ0XBsWSGVkQrE0BVY93Y1WHBYd54dFHDWV/0g9yT+bOf0LQBcwDLvRDARBsDxs5Z+b0T6Gt8+d5S+Hbu/KeCtK8V66gWA0YDQYMHW247t4DefJI0Tvj6PRaTF37sF38To6gx7FZsHgKEDadJOMxrAf7CEzMYv5QM9uCgGdBntdDTmPD7G1mczcAqqcRDIex97bSejufXKKQuHRg8RXN9Bq1GzdHqD4yEF0+TzaynKyM/Nkw2EcJ4/gvTGAnErjOtRLcHAYx5GDxAeHyaVSOI8dIDk8QU6rJZWIYW9oIO/xkk6ksHfvJba2gdFRQHRxBcWgx1pZhry2RS6VBgUKThwkODiCoaIEyR8ik81gqq4hPr+IpameyNgkRpOJVDSK89RRshMzxIIhdHXVaBNJDO3NxAZHySaT2Dv3EJmZIxGKYKwoIxeP46ipRlfiIjowgv1wH/F7o2TjcXRFLpR0hu4DffgmZ9Fk/z/23jw4kvy68/tk3feBwg007htoNLqBbvQ1fcxBcjjkHKTElSjvStZq13bYjt1whI9wOCR7ww5L2o1dc+WwJYoUxeE1HM49PT3Tx/SNBhoNoHGjcBaOAuq+j6zKrMr0Hz0KMxQU2ZRGJKXYb8QvqvLl7/erF1VR72V+8x0yv/V7/5wPvvsD9Ho9v/6lL3H9w49wVXpwNzQw9mCcw88/x7U33qKggcq+HoKzCxRjcY7+5pfJRmJk/Ado8wUq+7rxzy/zbEcPCf8adpNApSmOLyhTlEr8sy+f4rV372O3QIPHyP1H+/zTl49wZWybYCjBS8+0M/loh0A4hSyX+PWnqvAdZCjp7GwECrQ2WLHbreg0JUJhka3dGBWmEp31RjaCJZJ5leePWvhwJosWmZM9Dma2RHRaLWd7zVydzaEoKme69YytFjnkhlwRjHqIZDQUC3kGms3E8jpsxhJS2UBrFSzvq0iqlnAsy4ujLu6tligUS6glkacGnNxdynKy28bkRpHnBo3cXSli1RVZ3svz9KCLlQMFvU7LhQEz706mKZdVXjnlRiqVee3GPheHqljeVyhKMi+esPPORBKTvsxQRwVTaxlGO41sRSAYy3O800yqoGH9oMhLJxxcnYljN0GF08ZeXEUUixTlMi+NOrk8ncGqL3Oix8mN+TRmPbTU2ulu0HHPW6CsCESTeZ4ZtOA9AKUs0VJrYX4rxUiHjZ2Iwm60yKkuE6G0wHa4yBdHrHwwk8NuUGlrsCHUnPsl0GgD6tiN159orsXT/ytNo/1VHTRBEGZUVT32SRWD8ScNEvhH43L/PuD3+wnNLSIlEuTmltiZnUPOZgn4tti5dBVrZzuyKLLzcIayxUR6fgmN0cD2/CL5eILk/DKqTkfVs+eI331AKhIl/PFdTHYbgfkl5HCMbDROYmwSXWM9K/fGKYlFZO8mZpuN8PompYUVtLE4y2++i7mqko23L5Hw7ZAan2L1xm3kokxhZgGr1UJ4YwNxfgWpVGbj+29g6uvGcfoEB1dvEtzdg5yIf3KG8s4evhtG1VcAACAASURBVMkZxHQKjdFIRX8P8vYeFoOBmpEhYg9myKsKvplZEpEwhZkFjILA/Hd+iJTLYXS7Cdy6QyGdQfIfYLbb2Ho0h6zVELs1hqoRsDTWs/n+hwhiEXFqjvX79ylkMsgb21hMZmJbPnSZHAcPZkjs71MqFMBpY+/qDbIobM8uoIoFNFUeZq/fQiMI1I4e4ztf+xOGzp7m4pde4j/84R9z8uwZzl+8yJuvfgdrXQ1SoYBRoyGyukFoYopCKELyIMjBzftkN3wsfnyL7dVVtsenWLh+g7lHj9gOJLl182P8EZHZuWUWFtd498osCDre/mCcmaU9ulqsfPe9eVBKHOmp4r//P94hliqy7ouwsxfm5nyWTEFgaStBVYWV44frebgQ4KPbW5gNChoB7s/vYzFo2A3laa7UkcxK7PrDLGxEyIklZrwhTveY0Wg0VNoUkpk8yzsZ/IEk747t0XfIxLWpIGd6TJwdcPLda7v01GvpbrSxHcpzaTLOsXYji+shhjvsjy9QwmkaKwQ+N1LB9z4+oK3OSoVDj80g88adIB01kJX1+PbjbCd0TC/vs7gZYny1yNTiDtFEhuvzIhPrZTZ24yzsFHgw78MfSnB9QUKv1zO1FGI/JlMsFhnzFhntNHK8w8SbdwP0Npo50qznka+IoDVxqq+Cpd08GgE290KEYykebEg4jBIbB1luzqfQaHWMzfvZjsi8NxHmweIBTRUK1TaZP/qeF4exyKleBw83RDQ6K1VuM75gnvb6xzXTXr++SlkuMrb2mA58sBRkdjPD7n7wl2RJ/tGEPr8uCMKfAS5BEP4FcB348ydd/J9otJ+CqsoqPIcasQ30IggC7oVlKoYGqdBpWPjGq6iA1m5DKZdRBQ32gV4Sc0s4a2uoODLA3rVbxPYP8CRTpOJxVFWl6wufITq3iLuhAVNfJ8E3VxHTGTRuB+3DQ+idDixDA0Rn5qlobcbQ34XVZqVye5fKoUHywRCVQ4cxVHrQCaDR6zAPDxLzrmOvrsJ6/AiGQoHgn79K8eAAQaMjFQpTM9BHxanjdOs0aGxWKttakMU8obv3Men07Ew/orqjA830PAdr6wz0dNF5+nGEmenYYUpSieZkCoPDiWK2EPcfcOjcKWxH+ojeGae6rYWqs6NEJ6bYejiDvcpDw9EhdN1tyKJI7+eeQxELWIaPkPSu4WlvxdLXhXV9A3vzIQr+ADq5xO7DR7SfPUX3f/YVSCSRyyUiBwfsra3RW5LZmp1DYzFjslhZ39nmyq1b2FxO9iNhpIfTiPnH4dAGk4nBL32RrQ+uUemp5PALn0EQBCIbW3SMHKNz9Dh6vQ6H3Y3VqMdOgufPtpLNyVS4bfR31VIqFZmfd/DC2Ub2QxnW17fY0mp5drSRrtYqTg1WYNGXEXMFRjpMFCWZ77y/yIUzGm6OS2xtB3A6LNTWVbC2X6S7o4nx1TTTizu47Z2UFR2eCheHLCYiaYl0VmR6qwAIhOMyvr0oTx9p5yClpabKydWZOEVZ5vpchnw2g8mo4407ARx2K1qdjq39BNfmHGztRdhs9rATg7mVXardPaQLRQ4iGe7MQSRTS1mwsOTbwWY1UiqrPHOsjiaPwOGuerRCma56LfEjrchyiTPdBtKiRKCjkrY6M8ViBUaTjc8MWXhzLM7L55oplmTm10O4HVZuLpkpK1rS2QLX5/OAhpvjXvq76rm2oKWswL3pdQa6GrGYDJztMTG5kmM/lOK3n6tnYSvFsa5qBluMVNrNPFwOs76fZ7DVid2eQqPR8mBNZNUXxKDRYDA0Mev1Y7G04z/I0tNazaFaByc6zcSSLlRV5TNHnYRMtb94I/KPK6nz3wmC8ByQBrqB31dV9dqTrv+ZNJogCCPAUzxumCPyuOHZ9b+P2ju/CPy8NNqrQR/y5i7240fIL6yAJCMCzvZm0jOLKBoB58njpO6NU3H+NPnpOSwjQ4gLy6iFIlKhiOfpsyTvTCBUezDbbeQ2tjEd6SO9sILVYqGQTOG5eBZxYZlcMoXn5DDZh3PYzxwnNzOPpNVia6ynuLWL1mAgG43hOjlMaWWdsseFAYhv7eA5M4rkXaeYSGDs6USbL6DYLWiSGcSDIMa+ToScSH57D5PViqQBe3sbud09zG4XUiSGGE8gVLgQgxHqzp0kMbuAZ3SY+NhDXKdPIM4sUNQI2DvbKSx7sZ8cQZxfQmisQ8jmyW/vUsxmqRzsx9TWTHp8ilKhiPvCaaI371F58Sype5O4nholPTENZhNKLo/r9HEi1+8AYGisQ43GkQtFNHYrFrebstWMKZtHkGSUTJbaliZcNTUk5pd49uUXmZ1fILp/QM9Tp5m5ew/BasHscGBRwVJVgZTNI0kSDoeD9EEQsVCg4nAPqa0d3AcBfverL3L32usUyzpefG6Qb/7gDpQlTgy4CYYTrGzE+OKZOh54cyQzIq+cb+CP/2KSf/2VbrRagUsTMSqsKtVOE3HJQKVTi2pwsR/OUe3SYNTAylYIMSfy1GE3Nx4laKrUUlflZHM/QS4vUeE0U1Nhpr3WwNv3Exg0JSxGgbZ6O4tbSTIFsJg0PH3YzrW5DC+ecHF1Js1gu5VJbwa9wYBJV+JIq5WJtQLPDFqY2igSz5Z4+aSLu8sF1HLhcSfO/QzBaI6vPl3LraUCzw5auDydw2LSc6HfwBv3EzhtFp4dNPH+ZBpFVXnphJ33HiSR5DK9jUYWd3KcOVxNU5Wet+4n0AoKlQ4NVouFrYMsklTkS2eqmN7I4rKZ2QpLDLcZmFwvYLUY0AsqGkWko9HKhDfLZ4+5eX8yiaAU+dK5Ot59kKbWCc01Fh6u5/nCiINxbw5V0JIvFOlutJFIi2h0RsYXDvjCqTqWtzMc77bzaDPPQIud5X2Zs116rs6LHD7x2V88jXb0sDp24+2fPRGwVHT+StNof1f8jS5XEITfEQRhhseRB2ZglceZo2eBa4IgfFsQhKZfjJq/HBQLItlAmKJJj+/1d9E11pNPZzAUJVQVyjoN4S0fsncdfUUFi9/8DslkEnFljY07E0SCYYy9XUQnpjHWVuHq7SKzuoHOoEfZ22d/Zha0GiyHe/B/cA2hykPluVOkJmfRuuxoNBrEVAZdLo+xykM+m6WsqlRcOENybBJ0WhztLWQPQmgUFbWssj42gaTRYK2tQQ6FkVY2MHe3Y+zpYP/qLaRyGTGVYn9jA4vByMprbxBbXqUUDOO9cQtVBasqkI9GKe4e4Bo+QuTOBJa6OgSdjuDBARn/PmVUFE8F22+8i/lwL7baapLLqySCIQ5WN5AjcfLT8/hm5smm0mRX1tG3N7P1o/ew9HchxuLsLa8Q2/WTzuXZeedDXMcGEfQ6nD2dyEYDG5NTJDZ8kMmijcTZmZnFt7CEajFz7Qc/YmNxiYgs8X/+D/8zYi6H3e3iG7//bygXJYRsnvHv/ZCo34/V7Sa5t09uP0BNVztSLkdRktiemiXqDzC7sML1O4+4fNvL7MIar707SalcYm3Tx8Jmhu+9M4XbaWHSm+XyrVk0aombD0MEwnHuLma5MZdlP5xibHafeo+WjFhmLaBwtLeSSDzJ9bF1gokiiVyZtZ0oM1sygkbDxzMB9mJlrGYji74kpw9XsbRb4J37MYZajZjMRsbmg6yFVAJJmS1/DINWZWy1gNv+uCtvd6OBP39vlUQqQ7ksowhGqlxGuus0/N9vb3K808Rwm4HLUwnsRpkKK7x9e4sVX4Rz/Xb+7NIOo+169qMiO/thljf3ueeVsJn1zK1sc89bIpbKs+YLcmNJxmQysbgeIJI3MusNsh6Q+GgmSy4vsrQRQMHAB2ObBCJJmmssvH4niCiB7yDO9l6Ub1za5GirkYW1EJ21Gs4MuFncK2O3WTGbdAy16nm4HOCDh8nHlQem9ykUSww16/j2tV1sRpkP7noJxgr4IgqJgoH37myQF2UebRVAa8Jt03Oy28qfv7dGJpVgI1CgJKZ/SdFooAraJxq/qhAEISMIQvonjIwgCOkn3een0WhW4IyqquLfoMAQj9s3P1H/6X+I0OkNWKs9FCIxQr5tavcDJPb8yLJEtcWMUFao7+zEfKQfIZHEuraOs6MNe1c7rekssiQhRKJsj43Tf/4cuclZ0tEYuXSa2hPD1PT1oKutIr3u42BlBU9tDYX9EFsPpmg+dgTdo0WyoQiJkoxWpycbjpD0+2nWCJTKJXwzs7RrNOh1WvxbPkx1Nbirq1D1BoI37hLyruGsqcL4cJayLJNNpDhkNiO7nHhamjEP9WOcmaXpheeQ5RIDbicZ3y76wX5aDHr0nS3sXLpGwLtKp0ZDSZKgVKYsS2S3txHDUWL7B5jv3EcD7C4ucey//j2qVrcopLO4L5ymJpnGarNibGkiODZB3O/HPD2H1uPGXVeH1eWi6LCy/eF1XFUeAsteNIKA43Av/ZJMSS5hHh5EKZWwBYJYa6pIiwXaXnwBqVxC39GCJRYnpFFxdrXRdeoEVoed7mcvkAwEqe/qJLa+xfT1G/SODOO9eoN7H16l88JZPEMDxKbmOPHlV+htbiERDWDXp3jx2T5effMBI0NdWA3y4xYAx1yAyu5eDa01Rtrr9AilDlL5Al88VUk6ZaC1tpHr0zHuzB5w/uwQb1zbxLseZKinmmdO1BKNZxkeaOWzww5evysz1NfM2eEatBqByxN+Hu3I7AZTZLN5mmobKZYFBvtaeP64i3ekEg01bk516fmj7y3S3VJDPJWlu97IyOEW8rkCs94A1RV27hp16ASBdK7IR1NhBMHA1NI+Xc0ezh+poLvJhazoWN6XCMezXJ9L0VKppbrSTX2lhdM9Zi49EGltrOKpHi3RpIkql4kTHXokScAfcNFVqyKcbgMUPjtkoSDp+caHec72msgWmyiVFZqrtbx3N4rFqOG5Yx4UUvzu55u4MRvB5w9xd8WOxyVwY3KDw5113F0WUGSZM0dbqHGbaa3SIBYclMsy83sSS5sRjrR7+NyZLqLJIhf6DJQUhXK5GVSY8+7hspsZMwmYtWVsVgOVTiNOi0BJ1eD+ZZWr+QdOo6mq+sQRZz8N/yka7afgnY8+5HsxP5npOURVwd7QgFFVyQWCWI/0Uz4Ik4lGsQ8OIM4u4D5/hsKjBYRDdZDJUcxkkQoiOr0Rk8WErqkJcX6JcrGIRitQcf406bFJbKdGSNydwOSpQKiuRAlHUcUChs5Wius+kEuImSyOo4dJL67gOTVCZmKakkGHo7+H1OQMikaLoNHgOTmMuLyOJEvYGuuQ40ksh3uJXL2N7lAt1go3JX8QraAhp8jYO9qQfXsUUo+pvLJcJnFnHKPdSjGTxd7eghJPkctkMDbUP77LaGqg7NtGSmcx93UhpLPk9g6wDHQTGHtA/bkzKKUSSiCMKhYQZYnKE0eJ35vEMjQAB0FsQ/1kH84ipjOUdFrMDbXktvdwNzZQCIaouHCG/MwCSk0lQjpLdmcPzycUnMHjxnK4j9Sd+whA9TNPEb05htFTQdNAL9lIGLuiRavTkA9FaXvqJDszc1BW6HzmHA/f/QBVr6f61AjC9CJPvfIi3ncuUalNIhdTnDnWxMSMj1xeRC7mOd5lIRgR2Q2kOdNv5+Z8FrdZpq/FQSZf4iAJ6WwJRVFxWFQONdWxGSwxOljD9kGWzZ0IX3nmEFfv7ZJIpQGBZ0YbsZt13FlIYjMq5IpQ7dThj5VJpfO8craKj2ezuC1lPDbYjZTJZjMcaTazElCRZJXPDFl5ezzOCyfcfDiVQq/TodfIHKqyUudWefPOAZ87Uc9BrIjDZmJxO8crp1x8NJun2irhcVoYW4hwtMvDxkGeC4NOZrfLeCxFVMHA1n6C7kYzkmKiv8nA5aksUqnEyyfd/F9vbfJbF+tZ2BE50mZlfDlNqSRT6dTT1uBELEis7OUYaLETSUpkJR3hhIjTZqJUzNBc72bNL/LyKTeXHqapcRs43WPh7YkkL486uTmXxB8VeeaIi1hOQy5fwGzQcGs2xL94oRVJLvFwUyaTl/jiCReXH8ZRFFDR8MUTDraDOUoYiGUFqmxliooBd9svIanz6KB679YHTzTX6mr6lafR/lpSZyVg/9SSOgVBaBUE4d8LgvCWIAjv/dX4uyr9DwGxWJTtdz/E2NRINhxl68rHxA4CpPMiy999nRIKeouZpW+8irGvC0EQyKXT5JdX0ddXU4hEOZiaxaTRcDC3yMEHH5FDIR4K419ZJbW9i2w1s3f5Oo6jgxQiMcRFL9b+bvKpNL63PkCDgPfuGAgCij+AsbqKnfc+omQ1YbDbmP/mdzE5neTjCeI7u8hrW6zdHUMRwNBYj6ZUJnbjHhXnRvH09xIae4imthpZljCUVYwVbsRkCsFkInB/ksTYBGWpyMrdMWx93RiaGylpNQh2C0s/epuymEezt8/+opdkOIKy42f1ynWKskQpGiO1u09i00dq00fAu0rAt42psY6lb30fc28XZk8FhUKB/Y/vYGhvxtzfTXp7F20izd7DR4j7QTKSRGxiGtlkILfuY+HdSxjMFqT5FTanpqFQpDA9Rz6dIby7R3Z2EZ3Nytbd+2S2tlGTWa5//zWykRixZIKJ19+i7dxpMukUs5evUnviKMVcjv3JGTqOHCYZjjC/scnysheH3cbv/9s3aKgy49sJUOfW01DjJJhU0GgNOKwGuuo0zK4n8DhNgMrNB5us+wJML+wwtZYglhKJx0L8x2/fobXexosXu/gPr06hyFkSeYUNf4Kl7SxisUw8mWM/rnD2+CFefX+Reo+eZ0/U8ufvrdPTZObkYC3f/XCTzjoNg20O/t93NxjtNGHUylyfjjDY5qQolVGkPPPeXVLpAjem9viTNzf4zacPMb2ZI1PU0lVv4EyviQdrIjajwHCXm8sTIfoOmfj+1TVEsYjFqCFfKLMdUeg/pOdsv4PLD8IYtGUmVvPshxP49qJcXygQCCWZ96tUuYyMr4qYrVaeOuxkYjnGQUwiktUxMR9gN64lJRnZ8ieYXd7lWKuessbM4SYDLwzbeG8yQX2FgUS2zIfTCU51mbj8IEJW1uIPxlkNCtye8XNnNkQwrSWRznPX+7h2m3cnyn4wwdRmkZZqPclMnueP2bi1JOI9EOhtNBJNFfFFytQ6SiTT2V+CFRH+MdVG++tJnQY+5aTOd4BvAu8Dys+r4D9k6DU69HodgsOOtaaa2pZmHCNHSG5sUU6lcPR2EZ2ep66vm9zmDpqDMOWiRCQQxFhZiUZVqe3uxDTYhyEeJ7uzS21fNzpJpvpQA1qNFlUqkdjZpXq9lohvG1UQUO9oCK9v4Giox3b8CANaDZlwFMNAD0JeJPr+R1i7Oigm0zQPDuAYPUZZLKA1NqLpbKMlmyGze4CmUGTr4SMaB3qRFtfIiCKB9U2sngq2Z2Y5NNCH5sEMB95V7HW1NDx3AaPdRujjOwxcPE8+EsV//RZGpxPP8WP0PH0evdmI+Ug/9dkceqsF02Avxvklqp8aRdDqMI4/xOKpwFRdhUYuISeTyAiUy2Xy/n2IxTHrDWzPT+JyuSkUChQlCcNAH10qaBSFqtYmFr7zfZqHh9HX19L53DPojEb03R20yzKSKFJx4TTpmxlqq6vR9feS3/JR3XKIiuNDpPYPqG5pxnN8iOzUI9Y/nsP18S0QBObujNGWzaIqKns377El6NBZLZzo6aZC6+HZs51cuz3NTiBJIpXn7qM9wmmFm/eXaWtw8/4DGY2gIRzN8PFMklqHQk9rDUaDHqmQoaR3cepIHfVVZnwHOfZCIsVSnkA4w/kTLeSVLIca6zg32sCVu5tkxTK+tW0MOoXPXxykpsLIt95ZZM0XpNrj5MFikHgqz8xaCo/DgEGvZ9kvodObuTm1S07WYjeWkco6ulrreOWUi4JU5n/71gIfTcUZe7THZ872cmupiEaj5fKdJfraaympWkLxFL6wnoH2KoxGHZcmotyf2+dYXwP312R8e3HKZRWTTmG4w0w45aC7xcxIm4aVdScjrQJiUeWdW37qqmxoynbKChxps2DUa9nw11HrhI5aE5dFOz2t1dyej3EQyXHNbKRcKjG7vE+42oGKhkQ6i9vazMUhNx/NpBkZaONCv5G5VXh6pBGjVqa7uYKRNgOVTgM50YnbbuZUl4Gbj5Lsh9NMb1exsBZCrxe4MqtlemmH1noXTZUeBM8vi8X51XckT4i/36ROQRAeqKo6+ndS8VcIPy+N9lo+SuzGGLJSQm80UnnuFOnxKbRNDegEDeX9IPbTI0Q+votj5Ciph4/Q63WUTUZszYcoSCU0soRyEERoPoQQT6BKMoVMmqqLZ4lcvYViNGDv7UQ9CFNIJKk4d5L89DySKOJ+6iTZlTUsnW2kHjxCtZhJ+LapOjaEUVHIHgTQWMzY29uIzy+hFArUPnee/N4+moJEMZagXCxiOzFCauwB7gunKSysIKdTGD0eTH2diAsrFHN5nMcG0VrMZB7OoRMEZJMek8lMfj+IpCi4Tx2nODuPvrcTJRShFE0gyTKOkUGknX3EaAzn8WOPv5+6GkxGA6lQGDmWoPa5C2QnH+E8O0p8/CGCw47ZZCK756dUVlCNBjyD/eQ2fdga6xHXthC0GsqShOvcaeK37qFUuLDX16EAyu4eglwmL4p4zp8hPzFFWZbp//IX8H90E3t3Gw67g+DsIlWjR0mvrGFta2HpysccOj6MrsLN+nsf8hu/+RscTD/iX//z3+Wt175OqRDj4qlOPrq5hKqWESjz8sUmfnR1HTmf4+UzlSxsJLg6scN/8UIztxdSHGmzsXmQZWk7y1c/28bElkJBUvmtl0d484qXziYHJpOB8RkfVqsRRZb44sU2tFoNr324RleTlVS2jN8fxmqxcKLbyofjQU4dqWUnkOFop53p9TyFfI5Ku5Y6j4nlnSz5fJFnhivZj4qo6DBqZPIlHcm0SD4v0lBpJSVpSGQVnhuy8f5EkuEuOw9XMww0G1AEA969PEaDFkmSOdNtZi8hEEkU6Dpkw7tXoCgVefmUhzfvx3lp1MX1eZFiocDpHhPBpEIwUaKr0cJ6oIwoZhhqsxHOaGmvEdiLltmJlJBLJZ4/ZmPKpxBPF1AVeGnUzm5YJFU0sHmQp8VTIpwsoWitdNcLpEQ90YxCNlfg+REn12eTiJLKV56q5NZint56AX9cIZJWuThg5MqjLAYkTvc5uestYjPrqLbLqIKBcKJIS50NTe0vIanz2JB67/aTRQdbHdW/0jTaLyKp82uCIPyBIAinBEE49lfj76b2PwyUSiXCE1NoTUb8C0vIksz2Wx9g7mjF0dJEcm4JobICMZHE1NbM4l9+j0w8Tsi3R3h5lbJWi72hlqx3HaGygtymj+UPriLn849ra/3pt9DX1WB2uVj53hukEgnKFU42fvAW+rZmjH2dbF+6iqG+FjQaYsEggbkF5FKZ3Oo6Qk0lsVic4NwiqflFTDotkZ1dCnPLaOMpNj6+SSYaQ6PTMf+n38TkcZPf2iF5EMBQVUk+kSBycwzL4d7HTnTyEdGxSQxd7aw/mCa+soYiikhSgcCKl+zuHrlSmfj9h1i62okn44jZHEa7HSFfwGixImdz5LMZdm7eQU2ksOsMRLZ3iN17gGjQEZ96hNnpwN3fQ3Z7F5PDQcWpEfJ7+xgcdtxHB8ksrKA1GdiZWyC4tU3g7n3UuhpSy6torBZKsQRh3w77m1toqqvYe+9DXEcH0NVVs/jDd2h5+izuhnrmL31EzcljWCvclLU6Vi5doffXXiK5vsnWRx9Tef4U3/van2ApKwSDIdL5MjqdlgqXlc7WShKpLA6bke+8O8eFkVqOH6lnfCnN+kGRrz7XwgNvEqPRQJVTz8leN+GEyNh8mK0tP97NAG9eXcV/EObVtybYDeZwOF3ce7BMXbWVh0tRvv+BlxeeaqQga/jhpUn8gQSdDQaqXAZaGxzsRSRKioYqlwmlVKSkaDl9pIbVgxJanZFXztUztVlkI/A4FLmt3saaXyQj6XjmWBUfTAY50mwilc7wB9+cx2E3sRsDs1nPGzd3SOXKOIwltnZD9NZp+dqbq+wGM6DV86MbW+wFYtiMKn92aZtz/XbKiko2FUdVy2gEgY8e7NPbZKalWo9UyCFoTTTX2jiI5rg6Hae1xsBeIMJBKE5BVlndjnK42cxwm475HYm5nTJHWow81Wvi/nKak30VWAwl/uwdL9Gsyu3JdTL5AndXiljMZpbX93l/IkIsKTK2InK01Ug+n+e9iRSfHbLyzNEKvvHhHie7jGQLZdaDj6m084cdrOyk8a4/0aOFTx//SGg0fgFJnYeBfwo8zf9Po6mfHP+NEAThL4AvAGFVVQc+kVUAPwRagG3gK6qqJgRBEICvAZ8H8sDvqKo688ma3wb+l0+2/d9VVf32J/Jh4C95HJZ9GfhX6qcc7aCqKlUjQ2QWVhj4zS9TDsUIrazh2PBRisQIb++QjMVw9fdirquloqaGiuPHkLd2UFFJb++SW1gmsL5O96F6bO3NdNltaE0mFFnGHA5jaTlEZn6Z2s4O6p46SSGZYvvKDTxLq5htVsLeVapqa5CMRmxWG/UdHex7vaTCUQTvBlIqQ1VjI1UXzxIce0BVRxv6zlb0DjutkgwaDZaRIWpicYyVbqhwsXP7LoVMhvqeblbHJrC7Xeh0OkpyiYNlL+5wDJPbQe2zFzG6nAixBDXtrRjMZrRGI7srXoy37yMnM5RUldzMPEvXb9J64hjWlANTYz0eQcB0uJu4d4PawQEcTY2Uy2VW3nqf9hMj5G6PEVhbR2swUJ3OkEulKMwuAQq5RJJ4OIyn5RB6nR77kcPIoQjJQAjL5Az2nm5clZWYnQ6yuQwx3zYNq5sYgJB/n/D0HOWyQjwSJfBwFkGrJbzrJxQIYZ+YBoOBfe8qgstO58AA/d2H8PsWuHf7Dl0dtbz2XplcocD2boimQ7U8KErdsQAAIABJREFUWtylstKDgMrVe2s01zpY2Tfy3l0fT5/q5dayhEYokxMLnDlSTXE+Q63OwkvP9ePdqOTm+CqfPdvKxIyP3vZqdDoDf/n6PY4ebuX6PR+fPd3A7nAHDW5QxAx//OoGHpeV5Y0QZ4+3c+Vhgv1wlr1gAk+FjVsPN2isdqAicP3+Kkd7Grg6m0dRFDZ3Qxj0WgRqKRYlbszG0ev0DPfWc6ZLh9moZWJZpqe1htM9Fj6czKMRNBSKMh6HhZFuN01VekrlWkqSTF+TmRtTe9xfMmLUCeyEctgtMtOrMulMgUcbaXaiDnKyhs2dfYwGA3qjkaklP1azgZaGKkxGHQ/XMmz7o6zXulAUhbtT63Q0V/Heg8cmZX0nxr2VSpSSxKnDDRxp0hCI1HKk3clQq4nbc1HaGit4qs/OW/dDxJIitxxmNg8SUFa5NqejJEsEoinGV6uYWdqn0mPljteETlDI5Quki0/cwfhTxKfbYkAQhM/x2FZqgW+oqvqHn9rmPwO/iKROLzCoqqr08ygmCMI5IAu8+mPO5o+BuKqqfygIwv8EuFVV/R8FQfg88N/y2NmMAl9TVXX0E+c0BYzw2MFNA8OfOKhJ4F8BEzx2Nv9RVdUPf5ZePy+N9hcrsxgQMHW2Er55D7PVSjGdxXb8CKXdA6SSjMnpJrvlw3n6BLvvvE/jxfNIUhFdQULMZEjt7NDyxc+RvDuJ8/xpCjPzyIUCQrWHQjSGq64WxWlHjSWQgbIootUbkA+CaKs9mC1WMls7VFw4TWFumbIgIMkytq4OcosrmHvaUANhVLGI/fQIybsPUDwuLG4XqZV1rIMDlEJh5HAE9/GjFBaWEfMiZbsVR3MjufkVHEcHSU49Qmc0oSoKznOnEOeXEeprEeJxNA31lLZ3ySXTGAUw9XZCPEFRlrFUeij69h7XTKutphCM4Dp3kuzkLHKhiOupk8TujiMLAtaGOjTZHNbDfeSnHiHm8iguJ+LeHnXnz6E1G8lMTKE3GAAVy8gQyXuTaFsPUU5n0BVlCrE4Vc+dozC9QLZYwN7XRX5mAUNbE8mFFQ7/2kvs3byHLMvUP/MU2UAIKRQlE09Qe/4U4Zv3MLa3oJNKnHRVos+maK1xUGEIshOSeOZMH99/5z4CZWwmqLAqSGUNZ45W884VL/lcnrP9di6PB7FZjDw/4uDSgySSLPH8qQa2UhaeGq7n8v0wkqRw6mgjoWia1Y0AX7pYz9XJKJlslmJB5tefaWJmJYzLWMYXKBKMZjnd52B8JYOiqNRUWrk46OC9Bwmaq7TYbDY2/VkMGhmTychBJIfNrOPpwxa8exkkxUQkrSBLOeJpiVdOV/JwU+Z0l5EbiyKfGbLy0ayIVCyi02oYaLWxsJ1DLcs8c8TBzYUcI512vPsSZ3sMXJrOkctmGO6tprNWz40FEQUN+VyWF054uDSZoK9Rz3pIxWZU6Wq0su7Psbmfps5j5niXnfvePGd6jNxZSHGozsVwm5HLMzmyOYkXT7q5vZBCr1WocZnwJx5H2Y15RRC0pNN5zg7YubdS4HyfkVmfSCytoNcqHO+yMbUpYdHLHKq2suFPMdhiZdGvIEpltILC4VY7hyr1vDeZZujkLyGp89hR9d7dW08012pz/dTPEgRBC6wBzwF+4CHwm6qqLv9t9fs0IQjCuKqqp/6m80/icueAnztAXVXVO8BfrzLwEvDtT95/G3j5x+Svqo8xwePbtDrgs8A1VVXjqqomgGvA5z4551BVdfyTu5lXf2yvTw1iLkdg/CHJnV1SY5PoVNiem0dVFea+/m1ykQiaXJ7NK1dJBoPI88ukQ1HKO3uUd/xsfHwLKRKl4dnzbF+6iuWTiLVUKEI2m0WfFzmYnqOUyqAVNJQiMdRQFJPZzOrlq1icTkxSicV3LiEYdOQ3fGxNzWDq76ZidJjc7AImkxFzpYeDlTV2vWtEbo4R2tsjPr+EsbYaz5nj+D+4gr6uBtfZkyTuT6KYTGhaGtkfnyS3vIbJZmPm698CjQbfzCypSIT0xhZKXqS4vIqpuxOj3YYYiWEx6CmWZLJzS5i7O3Af7iO7vIbOYMB54iiRlTV2llbI3J/iYHWVeCBAfn4Zq9VKcH4BrVxCjMaIPlpA39KE++QwB2PjNH3+OaT1LRJ3xnGcHCZXLKBqtWj1OjSH6th85zJ6qcT6nbuUNQJKUUKWJSwGA2ZPBbaTwyz96B10djubl65iqq2m6txJNt+6hLjjx350ANdQP773r+Ds68HoqWDto+usLy4x593k/ffexGiAeDzFt1+/wz/78mmePdvHrj/E6NEmyqUSr32wRHeDnpfO1vKjm3vEEilEMc8ffd+LTlvGYdZw5VGap4brmV0JMDYxTyAYodpjY2M3gdViAGDswTKbm/u4rQp/8e4q00sh/BGZ924uYjIaWN4vkxMldvYjLKwe8J0rO3gsJXwHWf6f16ZIZfLkZR2+gwxTCzsEwwlCiSLrQRhs1pPOZNHpTLxwvII/fX+H0Q4DOp0Gi67ElYdhHEaZ+dUg+5E0WkGhJBUwGMyYjTpaK+Hf/WCeQCzLHW8Z70aQsgILW1muTMc50WmkkM9iMpl5tJZEp9Pxlx9uYjIa0OrNfPfqBtPeCI01TiYW9ilKJRpcKm/dC/PCaBViocgHExFaKqG52sD/+q054hmZVLbE23e2uTDwuDbcQayAy1TihdEKbi/mMRs0bARlLt3dxGox4LCZ+ZO3vGzsBMhkRT64v4t3J826P8PmbgjfXoSmijIPvTHeuB3gcLMBSZY/bRPxBPhU20KfADZUVd365OL/NR7bzl8VmH7aySeh0WoAryAID4HiXwlVVX3xb6FMjaqqgU/WBwRB+KvWeQ3A3o/N838i+2ly/0+Q/0QIgvAvgX8J0NT05EUP4qkk7rYWqs6ceFxX6/pdGjo7cZ07SXR3j+qLZ1HLZQ4JGgRVxXbyKPXJBBqXg4qeDiRRJBON4dzcJe7boaq2FnF9k9DWFrUDPRgH+jDPL2HsaiO5tkFwfglBr8WplOh47iKCXo+lv5uOvIje5UDbWI9cLBK5fR93dSVlpcz24iLNRj0WlxO7w4Hz7Al0y16Ck3OEPr6LzWYluR+kdnkVjAaCWz7MiSQ1o8ewOJ1UXTxLNhii3/Y8GkWh1XgUVBWTzcbmrTEsTjvGqTmUUpnYnh+NTovRZCKbTGKfdqMoZcqlEr65BZpNBiwtTSiAdeQIFTothUAI+9F+ouPTeNpakQ161FIJ/70xLKMniGbSmG024vcesDszj6flEJaZBciL7G36aDYaSO8f4Kj2YDs2QDcqpZxI/OEMO5Mz1Pd0Ubr7gGxJpqq5GWtbE5uXrlCpKjgkiXg4SiadpZDPUxYLJHb9VDY3oaSMuBrqGT59irh/B2Jldv1BbtyZobe7je+9Ncb2XoCtLR8f3jBjMBi4O+4ll6zlgaCQSGXQaHV84XwrkViW9QORR94wJqvCzakgbQ12Ro4N4HBY+fDOOr7dILlkHJ3STJ3HSku9gyMtJvb8CVKpPMfOV7PdV09jpZ6hVhNvZwwc6m/mZKeWf/PqMv0t7Xz+RCWyoqPBY2S0y8z8pozTVE88I/PvX1vhwskubq9IeLdjWE06bKY6gtEM42tFdDodZa2Ze/O7fP5UE6eONqMCB5Es63sZzMYsFlMd9U4Dx/oO0dtowmOXMQ43UZAVtveTrG4l0egtFEoCazt7fPW5VgbbdaxsWjjfZ6AolVn12RjucrIdLtHbVkcopRAvmvCH/Hy8UMBgMHFnzkdZqeHZY5VsdjUy1G6nu9HItDfE5ck4FquVh3PboDQTzetZ34tAucRvf+YQVRV2jrVosZu1BGINVNgNnO4xcX8pym64RG+znVReIS9psNssoCaZXYtQ6TJg0T1xsvuniyd/HlMpCMKP0y5fV1X16z92/JPs4a9S8NZPpcmexNn8waekyE+D8BNk6t9C/hPxyQ/2dXhMoz2pUg31DQjeRUq5PGVFxVzjQSyV8V+/jefYIKI/QG59C/eZE2Q3fBQDIewNteSCIYSqCmw11RgqPeRR6HzhMxR296k4M0q30UQR2L92k9aXnqewvoW1sYGWURAUKBUKuIYGSMzMo2xuo6usQAxHKAfDDP03v0f05hj63i7MyRSGUATLQA9IMjlRxFooIMRS1LQ14TxzktSil9azo+jqaimEI3R/+UUyi140Vgsagx4pl0da2cB94TSpe5Po9XrU2hoQCzQP9CIpCoaOFjKrG7R99lnK0ShSMoWroxVtXS3mumqy1+7gaWzA2tdFeewhh174LNm5JUyCBqGuGimVxqCqNDx7gfziCoJGQ21XJ+ahfsSJaVz1dVj7uuh0OihEYhiP9FJ8MENtXw+Kw4a7WEnRbCZ6fwr3UD/ZrR0cVR5aZQW93Ypz9Bjy9TvU/dqL7Lz1Pn1feYXMohdHXxftxSL5fB5jUxOl3T06X3oeTTZPIRKl4TMXmF9dZaTWwcXn/gm3rr/NK188j1hQ+CdfHOHbr9/gv/udUf7yjYdcOGrlN57vIxgr8OygnTtLNiwWI9enopzsd2O1CAz2tWI16WiuszPrjfKFZ0e4NrZBf1cdeq2KmHPT22ykUKwkkcmxFVAZ6qllaz/D2EKU545WMObN0uCGSqeBbKHE0o7If/lSD9NbBcrlFKPdViZXs5QVI7txlReGPbw1nmSovxGXRcNwuw61fIhisUhXvY7nz3ZSkBU+N2Dg3lKKjkMeYjmFpwdNXJ9JcPaYg0BKgyQrnOoy4t3LcrzTjj+usBmWeOWkjevzBXqbrei1KifaNdyaU+htrcJk0HJjNs5Xn2lgzpfDF5T5zz/XwOu3wwy0uems1TK+WuDpfgPbficNHj2tlSp6TQeKIpDJSwy2WdmOlHFbRZ4/1YjJZCGTL/Jf/dogU2spRtu15MVqNBoNqVyJF0ZrWNgrEUtl+cJxB1PrWaIZhYJio9JVwGTQojPZcJg0hBNlmhsqeflMHVce5Wir8jzpX/9TRfnJnyRHfwZl93PZvV81/LTaaAKAqqq3f9L48Tk/B0KfUGB88hr+RO4HfryzUSNw8DPkjT9B/qnDc3aU9NQsmclHWPp6cHV3EF/bwNHWQmx6DkdrEzqjAVd/N8m5ZRS9AV3LIea/8SrlcgmjTofvxm2UcAyNQc/6D99kz+tFr5RJ+w+QfXtkg2HEpVVsxwbJp1MIJgOh2QWK8QSLr79NOZnCaDQS2twienscy0APqbFJcsurNH3pi2z+4C0MHa1Unj7O5uvvYjncg6TVEp1dwKjXUzE6zO6tO2gtRvSVFRj7Otj74Bptr7xA8OZdrO3NCIJAyWUnn81ha25k+9Y9Atu7aIDFb38fMZtFV1NJIRbDUFOF+9ggqZU1wvcncfb1YB8ZIj45g9bjRo5EWbt9m2Iuh1Qosvba2+Q1AsXlNdY+voVcknEND5KfX8Zis6LYLexfv4NQ4cJQX8Pqd39EbO8AoyCw/u5lNA4bRaWMUSOgt1lxD/Yhrm1idDkpm83sX7tFXiyQmZgiFYkh7wfRtTYRvjVGyWbF5HLhfeMdjAYj2kic7fFJoptblNd8zC3M/3/svXeQZNl13vl7L703VZVZWZWV5b133dW+p3scZgaDATgwAkiCAkCKEinGSozVyiyXsYZmg7EkJJAiIMFjBnYGmB7TY9vbqq4u773NrPTem7d/VFPL2AgMmiJICCC/iBeZ+d679938450b55zvfIelpW1u3t/kwjvzrG/uU8in+OZL1zjeW4k/mECtUvAnX7lDqSShEIt84+0djjarKZREnj9bxc1JLy+9O0e2UCJVEPn2hQmWNgLcm93nzvgcX//uFSLROOlcgS+9PMPK+i6xRJq3bm1SZRWpKRPZ9WexGlUcbVLzR9+axWEskE4l8URl1NrVtFWJvHJ9m5n1EE5zgc+/tIZefijpH09k2XOHuL94wH96eZ3Oanis38g7kzGMaokqo8TCbpZkXo7NUGLbHWFlJ4yzXOLzL69ztlPDk/1ars2n2fQLlIpZXr++hE6eQ5IkUuk0oaScXz5fxbW5NDqDnqePlnN9PoWo1KFQqPj+e6tYTRpuLOaZXvawthclnS2QzWZ5e8zHc8ftJJNpvvGum2OtOlLpHJemY7TXaLBo81wc9XOk1cQPLi2zsR/HH8lRZ1Pypdc2UQo55KU0d5biNFdruTq+jccX4eK9IL5Ilr/80RLZdIzOGhWff2kNgzLP7al17i762dwL8e59L6lEhNnFtb8LE/G+kIAS0kMdD4EfZw9/LvB+ns0VQRBeBi5IkvTf9M8EQVByKMb5aeAKh4ywh8WrD8b98YPPC3/t/G8LgvBdDt3C6IMw29vAHwqCYHlw3+PAv5MkKfRABG4EGAV+FfjC32AdD4VMOkNqfvEwxxKJIFPKiWeyiDI5OY8X3+YmSq0GYzSOJEn4tjYxZNKU93VjqXKgdtWgqrCiHxvHMNSDQqtFyhfQWC0o25uo12mRrGZWX32DysZ6ildvcbC8hiiX0fiRZ1D3dlFMpZFXVZLYc1NRX0vFIydIb+0iKuT4l1bQarSEvV5Ut8ZQ6bREPD7i0wtoFQo27k3QPDxEcnGVfC5PaGefpDeIWqUik0yQn1shsL5NRVUVqUAYHbCyuYluyorGYsLqqsEw1Iczm0VSKglfu836+ARtx4+RiEyi1utZunmHVkRkcjlr12/RODRI2u/HNTSEvtaJvLKC/fFJNFV2CskUDY89glqjRoonWb19B1tDA2GPF7XZREGUoWlswObxo7GVo25rRLe0isxeQfTaLSKCgFJ12JHSu7WNKFdS5qzCPTNP+8efQ1Ao0e3to2yuJ7W4yuadezj7u9G1t2AoL0c90IUol+OIJxBlMnTDvTiyWTo7uvil554l5lvFYpDQaxR85dvvYtIOU2aS8+z5VlZWt2lzqvnRhhe3L8KNJSvTy25ERTtdbQ72YyKf++XHEEWRF1+dxWDQ89T5foKRDIlYhE8+24UoinwhkqDDKWfdnaa1rpy70x5KCgNb+0Guzpkp00m01tuZ2c4QTRbx7R7aEnkphUGv5libmWiygD+0Q6PDjtOmIhDTUm7R89Sglt/76jw7wSKb/gy7+0ECISU9bVW88e4C50aa2AnkMenV2K06csUSyXSet8dDOOwWZpbdiDKR1uoaRnrryBZK3FrKsL4fppTPAVXseSOkM1kEoZZUJsvcyi460Y6r0kxblUg+n+XxERdqtZpNfwlRoeHyvU0ElRGVUsnWfohX7hgwGvSs77p5e9KAVBLYdEe5sVjBcKeTMpOGkTYdhUKJxZ0Ia3tRGlx2Vrf3ubpgpdyi40x/JV0uNblCkXxJhtWiIpLMkUznaHFqCaXrUSsEzndrSKQLzG3GmNnb/2mbiIfCT5Ejew9oFgShHtgHPgF88qc2+98e79sK9cey0QRBUAOfAT4F1AMRDhNAMuAd4C8kSZr6sRMLwneAs0A54OUwHPcK8H3AxaGA50cfbBwC8OfAkxxSn/+pJEnjD+b5DPDvH0z7B5Ikfe3B+SH+P+rzm8C/fBjq899cG22X9NwyQoUVRTZPwudH199Han4eja2CzIEPY2cbhWIByRtASmdJ5bJYBnpIzy4i1tVQDEcQC0Xy+QL66koya1tksllkBh0ajYZUMISgUGJoayS7vIFY7yS/sUPJqEdttSJ5/ZTSGRQdzRTdXnQdLfjevoLKaCSTTGE6OkB6chbjiaPEp+bI+gMUrWYK7gOqnnmC8MQM+vpaMosrGE8cITK7QGx9C3NnE6k9L+WdbagcNgK3xkhHotjOnCQ3v0ymUMDQ1kRxfYtSPo+iu53IvUlUajXGkSECd+8j6rVoNRpSbg8ZqYitp4vU7OJhWO7GKNliAfNAD5mVdaRsDtOJIwQu3cD6yAnC1+8it1iQ4glymQzWsycIXb+NaWiAwtIysXSaoihQ0dNJZnYJwV6OFIpgGO4jdvsecq2OVDSGabiP6K0xSgJUnDtFanoORWMd+c1dpHyeXCKJob+bnM9PIZPB4KgkE0+iVMhxZPI4FErqysx0OdNcH12lVMww2FXN7fF1PvpECz94c5ZoKMSHTth547aPUjGPRi2nvtpAIHUo61/T0EY0maPcrKYotzK/tIlSBo+c7EapkHHxnTuY9TLK1WluTuzR5tTS6VLx2mgEmVBARoEmpwmrtsSGr8ReqIhcSiOICpprjCxsRTjXY2J6u4DDVCIUz1NESXu1yOK+hFLIUZSKiDIFiayM051aLs2kiCUydNfICWXk5AsQTuRRiEVG2oyMLUU42mrg+nySgSYtExtZkEqc6DCwuJejkM/T7NRxdzGKRqPiaJOS0ZU0+Xyes91GrsxG0KlEYoksR9st3F3JIAgCHz5m4e2JMEeaDZh0Mv70+6v8+jN1XJuL8UiXgftbRbLZHN5Qkl99rJobs1HcwSSdtXokUUE8mcNl11FTruDSTIqSBB2OIjfm47TWlRFJSkTiaZ4bMfOj22GeHjZxcTyCTChytNXAoqeIDIliMU9rtZZKi4If3okyePIpnvvlf/VQ7/5f4W/LRusbGJAuX7/2UPeWGYw/8VkPmLuf59AOf1WSpD/4713bTxuCIExKktT/467/2DCaJEkZSZL+syRJJ4Ba4DwwIElSrSRJv/5+G82D8f9EkiSHJEkKSZKckiR9RZKkoCRJ5yVJan7wGXpwryRJ0m9JktQoSVL3X200D659VZKkpgfH1/7a+XFJkroejPntn3aNDUCxWMR7+RYpUSC1vU9wfQvf5g6llXU279yj6A8hCgIbr7/NxoW3EKsrSSTiqGRyFDotmWKeyP1pjK1NFMIR5IUCSnsFkVSSwMYmhppqtF1tKOQKyo4Psfjdl/Ht7JBd2SAbT7D53jVymSzFZApBp0FtNpEPBPFfuoHl+DAxzwGGuppDj6mijINbd5FVWDGMDLF16SqaliYy61soc3mUVjNSRRnZPTeyRBKF0QC+MNWPniaxsHJYmKrXUf3EI0RGx5GMOqwjg6TnFhGUCpKUCN64g+3sCVRNtUTv3ENVKqE2Glh47SL5TIayzjZ23nwPWZ0T961RDjY3CWxsUVjbYuXKDYolidjsAkK1nbUXX0LX142QziCqVWi62tl/410MjXXIdRoSsTg6uYLKo4O4L11D1ViLvt5FLJ3m/uf/EqHceti3x2ggsrJGUQDv2gaBsftkIzES49PoezvIpFPI1Sq0ZRbEWAJ5KoOq0oapuZ7Y6gYmk4mkQo5nex6NRsHa+jYb2160GhXnTrRx4fIGckFAIYOXrnroq1OQSKaY3wgSS4P/wMvViQNaGx1s7ASZXo6gUghkM3nG7i8xOrnJzbE1vME0b7wzxpY3y9TiLpEU3FjM4gnEmF7xUSiUmNmI8fpokO5aFd1OgR1flvN9Zu7OeljYCFCUJPyRNNNbGQabDXhCaS7NJBhqVjPQauade156G/TEEmlev+tjsFFJXYWM71zeodWhwB/JoFGreHzAwthajqKoQa+RoZQV+NPvzqFUyLAY1PzZd+fZdfsp0xW5NBFAFAUCwRD/8aVlupwCTw+bub6QRqXSUGHWcGfGzdKBiCRJzK/t88ZdL2c6jVxfSPLmvRC/+cFabi+nsRg0xFI5royt4QvGON6u5/W7XkxGLY8PlvOtt9dxWGSc6DQzthxjfClMc6WMeCLB3dUcv3SqkvE5N+OzW5Tycb717h5VVpGrU37y+QJz634mt0u8eW2FLXeYI0067q1lWdiO0+nS8DeP+v90UHrI42EgSdJFSZJaHti9/2E2mgf4WxMEkCQpD3h+Ksv5OYLHvUdJFCjrbENp0BMYn0KUy9EO9+EKhZDbyjG1NFBSq8gFQkS3dvGvb6FQq5AJAlqZEs/uHtb7s6zcGaPp6BDeyzeIbW6BIJJe36awf4B7eRmZXI6xwkZFWzP67nZiW7uYwmGETIblO6M0HBlCHJ9hb34ZpUGHfn0L79YWRrsdKRJDJYos3bxDOyI5nRZbQz2ptQ22Vtep7GqD2UXkSMy9/ja1/d0kvT5CiQSCKJJIxNn46ou0nz9LZm6Z7fsTtGlPkpmcxbu1jUwup7zGyZ7bg2lsEgkIbGySTaepdTmw2sqxDPWS2HUTPvBiz2Qp6+1EyOXRmszI2lux7bpRVFeirq4ifP02qUSK3MIKizdu4hroQ18o4ltbxWS1IAVCJONJgnv7uPRaInsebHsHxL0B9C4nurVN8sUi6+MT1B0ZxNTTgdjWQszrw9rVwcaFNygVishujhJc36KYz6PWaFi4eoP6wX6SY1MUi0UCO7usFEqUO+y4FxZoqtEilwvotCr2D0JE41km5zbQygr4wknkAnQ11dHWWIExDKeHqhmdlbEZjnB3PkIsJeHb2mLkSBs9nbUk4yGeOFYFwN7OBp3NVZzu1HLttorjLQokSSIQ1lNZbuRsl5qZrQxvL3sYXbchCEq8gShvj6uwGORk8jrWDwoolQrmV3a5bdWRy5fY8YS4aTaSzWYIx5K8eieIKFcwteSmIMmJxRP0tFUzvhohHMuzsRsgn69ibnUfuUxEo3Aw2GRgP2in2S5SX6ni3oKezjoTBr0SfzhIMiGg0mhoa6gkmZcxtpZjetmNVi1D32pmoL2StsoSd2Mw2FZJd62Wme00hVyepV0/eoOeq2MbnByoJapX0t7owKoXmdvJMbl0QBEFwWCBBqeVt+4eYDIZUSkUXLi5wdGeOqKJPAf+ABeKBcxmAzabldMdGv74xXm6Gowc77KyupuiKJXzZL+ObLYBvVpiZidPLJnie5e8nBpuRqf0ve/7/ncDidI/MGX9H4efC42EnxWcNbUYbBUIhQKCIKDK5dG1NJBxezBW2ij4/AAoCyVkahUqpYKWx87i6ulCf2yQVDyOq6OdrFJG23PPoLJXIFcqaX3+OVztbZhODJNMRS9QAAAgAElEQVSMRDE3N6JwVWGvryUfigBQ2t6j4flnkWWyNA0PIlPI0Q714Oxqx1pbg7yhlvZTJ8jEYyg6W5E1N9J2+gSSAIVQGNeHnsZstVLT1Y7RWoaysZaMP0DVUC/6rnbsLie1j5xEVKspP3kMQ0UFivpaxMYGGk+eAJWKnFaDa6ifqo42Cvk8lQ116EYGkBw2nIN9OJqb0DurqOjqoLB3AJEY3f/0UwjJFCq9Hr1agxyB8O272B87A8EIyd09yu0VNPb1oOhuo6KxHn1DPRm1kq5PfoxcJo1moBu92YjFbkN0OjA5HYhVDvTdHRQ3d2j82IdQyWR0njuLkC+gNBkJjk2gra4mHY7iaG+lpr0V6+ljODvaqB4eQFZTRfvZU4gyGXJnJXm5jPrHHsFe50IvV3DsxKMUiwVOHWnCoFXR1mAjm83yiWf6qKq08sSpdh451ojRYMQdKlBdoeH+oo+sooo/+rfPky+UsNsreOYDp8hmSyyu7FDntOANxJhe2KOlWoWzQsWNKQ8jnVYWdzO8cS/C+W4tTRUSu8ECO4E8A602ButFkokUTa5yPnjMjkxp4FSXEYNGjlVXpMFlw6bPYzWq6WpxcrpdQSxV4H/5ZBc1Ng35fJFj3VUMNqqpq7YiCgLn+soxGTR0NDt5rFdHvbOC+hobZzq13FlO8PHTleyEBLzhDEc7KsgUDlsvf+7pBhRKJad77dTblei1Sgab1BzpctLdUk0oreRjZxzcXozSVKXlA0NWJjbzHGnWIpMJ9HfU0GIv8vz5ZtK5Eo2VKqrKtSjVeix6kZbacp4YMBHPyfj0Y1Vo9TqeHNCTL+RpcpXzgSEjeq2aY30NlAQ5zx2z0F0jwxMp8eSJVgIJGQatEk9cRCWHaCJLpUVOKidwtEWD3aykq8lGR7WMaoftfd72vxtIEhQl6aGOXwC8r+v4j5vNT0DZsSGi49OkI1FEswlzSyPFfQ9FmUjRbGT/vWvIaqoo5PK4xyaQOx2QLxC8MYplsJdUOk3OF0SmVbP67lXiwRBpXwB/MMjMX3yVvEGL0mHHfeMORb0OocqOb2wCldOBKJeTiURR6XVgMpDdc6PUaSk/NUJk9D6CKFLx6GnC127hu3QFudVCxOMh5HaTW1jGu7dH2OMluLnF/H/9BtrGOqrPHCc2PYey0oauvo5wNMLcV75J1enjxO9PExsdR9fTwebYGJHlFdStjeRjcTRlVlTNDYRn5pEOfGg7W9H2tLPxykWULif5cBiN1YymzEJeKpH1B0CtRMrn0OgNyFUqdqZmKB740PV0UCwWiN2+R82zT1Py+lDEE2gcNkxH+glevY3aYEDV3MDa91+h9tmniC8uEZ5fwtDahMpoIDA1h8xVhfXscSK376FTKNA31LJ64XW0XW2kSkXc711D292OvqWJya++SDaXQ2sysvraO3hm5ymJIlsBP3a9ntr6Jr710nXWtgLkCnn+j8+/xuj9ZWaWfdyZ3sQXSZNIZZhZDZDKlsgURF66so9Cqeba2Aazs/PIZQKtTU7GJlbIplM8cbqD8TkPk7PrdDcaONbn4NI9N6d7bNyY9aNVFFn1ZImk5Xz94go9dWoeH65gcjOLXKmiuUrJ63c8dLkUtNYYWPcVCCXlbO8HuXj3gP46OcVckh/c8NNbq2TVW+SHV1axmtTo9Aa++KNlLJoSCinFi5e9PDVkQigm+c41Lx88Yqa3VsHUVhaVWotWLSOeynNrKUN/g4bvv7eCgMTt5SzeYIJ4Ksdgs4HprSxvjEWw6fO8eXMZJInbqwWmlrys7ES4Me3DZc7z0g0vbS4d6UyOuytZhpo0fGBAz//5rXm6a2QkU1lSBTWfOFvFneUMRp0apVykvUrO7391FoVYoMul5o9eWMSshTdvLJDOZLg84ceil7O4k8KoKWFUFVjaTVJukHOsXc833t6mv16F01JibCmCQafmo6ftjK0k8PoCPxMb8lNko/3MIQhCrSAIjz74rvn/qT7/yvuN/YlhNEEQfht48UEF/z8oZLNZUtOzKLVapv7yq7Q+cZ7I3BLrY+MY7HbKHXa2RsfpspqpPHcS/fj0YXHi1CxWZxUqhQLv0jJ6mw1FsY5yVw1lbS3IaxzIdnYxVlZgdNVAoUjI40WSy7E5HKzdHaNpeJCsx0fcHyTo8VDV3cHaxfcQtWqMwRAUCqxfukpnLoeuooLw3Dy26krMXj9yhQL9QBembApZJo9kL0OhUlJSKPBducnuxDQag55iNkdFTweZPTdJj4fkgZd0PE5RLlDmcKC0V+C9fpu98Slq+3vQF4usXLtBx+mTpCdmEUSByPYOVVu7rI1N0HpihNjtcUq5LFNf/TaukSF27t6j+egRUlNzCIJAxOtDvH6H1dF7NB4ZJruwxO7iEgqlAqVWQzGXY210nNrjR9CZ9BQKefzXbqM3Gli5eoPWo0MU9j1EfD4q3Qfk3QckAyHisRj6RIxyZzX+uxOoRYG9hSXM5eWUtGr0FhOW/m4Ueh2OWBylXkcmEibh87MYDHFmqJOm+mp62hw011r4vf9nn196spMml5mtHS9He6soMymZWgpw4fIqn/m159kM3mJoaJg6l537s19hYWGJ5ho9NZUqLrx2mwvqAq9fmqG3zcHbd0UUIiRiCV6+4WZty09bdTWt1WoWd9N0NZSz70+y7i1x7d4q/S02okqRmbUAFWYte4E8s0v7FIol9BoF9TVlLG7HWN2LkUrlqauw0WjTUOsw01OrRCWXGC83oJKXWNyOkS/A9KaRZLrA0rqPsSoruYKcq6PL1FQaeTVtYHHdBwK8GInR7CrnfJ8Zq0HB2raG2nIZb94LsbQTQqeS01NfzWPH2ykW85xsVTI6a+B8n5ViscjEepzxeQ/lVjOb+0GQ4OJ9Ea8vhEYh58psnJXtEDIZlIoOro9vMNBRzaujKaqtArZyI08OVXDhjgeDTsXpHhNFWSuxeIaRDj1bvjwLax6icRO1VWX86PVFnjzZxsqBSCCa4vJ8FgEl18ZWODfSxttTaSTg5t2pw8ruv0dI8AsTRnsgvvkbgBVo5JB6/UUO8/lIkjT3fuMfJmdTCdwTBGEC+Crw9t9FMv5/RKhUKrQD3QRvjuIaGkBXVUmuUKC6ow2d1UpOkHB1dZANRtCEIuQyWSwnj1DM59FaLOiH+3Bl0lAsUtjao/LxM0RGJ7C4qrHqdJSc1cgLRfKSROOZE4gaNVl/kOqhXjTNdajsNvLvXkOuVGLo6cS4sYO+zomlrxv/5Zs09veRSafRl5dRXuMktbmNrqOFhPuAXCCEFpEUErldN6a+LuTZLMa+LtrtNlJ7bsoGewndvkfDx54ju7iCUGXDItkwHR8hs7CMlM6QVajo+cyvEJucRdPfhXV1A1mNA5XdRmhsgtpTxxGsZlqOjyCoVRiHesnEElTH4xgdlbi6OlCYjGh7OqgtFpGkEtrBHly5HBp7Odq2Zux+P3KlCu1gD4Hrd+j/jV8lv+sm7fPT/Wu/THx8CpwOrA11KFzVKB125JMzqHo6KBWLaEJhjLYKioUCRasVY1M9sdVN6s+dBknA2NaMK5Ygvb5FOBbHMtBNem4ZbWMtbYIKq15LLp/nxGAtY9N7RKNxfvNXznHlzjLVNh19bQ6mVyI8e8bJXlDkP/xPH2dty8dQfxe3xuZwOSvo7e3AYtSgUmXY3vHS0VLN8S4j+Wwz/lCKwSYNK9th6qstdNdqaXW24o0JKJUyQkmRT56v5vpChg5riUymmhaXlUQ8QabexnCLjl1/hv72KtLZEoJQwqIRqKowkShoSGXzDLWYeXU0ym88U8d7MymSqQy/9VwD1+fTtDRUo5YXqLcr8YVFPvl4PdkS6OUZPnSmCXcoxweHD1WdJQTkInzwiIkf3Irw1KARlVwiXxR45mgZyJSIgkijQ407nMaiFVncyzPSXc3oWo7uGhFBpuXf/UonS/sFau1GlGotp9oU3BSsnB+wki0qEAQ5ogh9jVoUyhaSqSynugzcmI/zoWN2bsyGqLJZ0aoS5PISRhWcbjPy1kScDx4x4et1IYoiZzvkTC3oabIVubuS5cMnaynJZHTVyPH6K6iyiLQ7Vbw6muaJ88d/JnbkF6gJ2G9xKJkzCiBJ0upfU4H5ifiJYTRJkv5XoJnDBmq/BqwKgvCHgiA0/nct9+cIkiThf+86ho4WKo4Ps/PuFbzXb6OodRLc2YVYApleT9nZ4yTcByQjEXYvvEnFI6fAaia9tIZapUaqKMO9sETgQR+c2Oh9RL0Oc0cLyeU18mubmPu6CMzMo3dV4zh1nNTyYQGn+egAYnUl69/7EZXnT6EqlAiN3sfc1YZar6Xi3CkKGjX+zS32xibIF0uYO1txX7qGos6JdWSIhNuLsbaG9M4+yak59J2tVDx6Gs/F95CrlCg0agrJFDqVBsFWxs4bbyFW2Vi+dZf4gY+05wB5SyMH71ylYriPzOoW4ZkFdBXlVAz3k9raQa7Tks1kyUVjxO/ep/rpx8nuezBUVpKXi+S2dg9Zbek0B1dvUX7iKMk9D75rdzAdOfyPuxffxVDnQmW1IOTyqGVyFAYdypYG3JeuUfv0Y0TnlgjMzOE4foTk1i7BK7ewnjlOVilHUsipGO4nt7aJplCkvKeTokaF7/odRKuZlPsArcmIXK8j6PMhvztJ60Afhqoqfv9//7+ZXtgnk0nzwitj7Lr9PPtoJy+/u4FSVoJ8kq9d2OTTH3+EhjoHKxteBFGkodbBH3/+RZ44P8LJ44O8e2OJUj7BI0MO/vw7k5zps/LkiI0X31pletnPY8PlXJoM0lGjJpVK8dKNAI90axFFkVA0xcx2nicGrCys+5jfimNQi2y4k6weSDzao6PJDt5gAqmU5+sXV6myCJzt1DKxkcFi0iKKAtl0glgizep+mtHpLZLxKOd7TdxaTCDI1LS7DLx1Z50rkweU6Us02QWmNjOUGVWUsgkW1g945U4QsyrLly/u8MSRSpbcJV4bC/Noj47aMolL9/1UmSW66gys7ifZPwgxt7bHl15dZ6BBgdmgxhPKodHqMavzfPnNHZ7s11NbaWDVk6XGpuG5Y1auTniZWdxFkIp8/a1NNncD7EWVXBrfJ51Kcr7fwgvv7dJgl6FVHyoQ/If/MkmttUg0nuSNsTCneiz8xQ9XcFgUGLRy4vEk33xnjw8dq2DNnSSeKqDT6TDotH//NuQhQ2g/J2G07F8XZBYEQc7fQMHgYdlokiAIB8ABUAAswEuCILwrSdK/+Rsu+OcGO7s7BPfd5KQiglZLLpOjrLsDuVpDLpfHu7FJWVUVGy+/hr2mBqOtnM3709hnFiCXY3dji0w8jqOrA63JhO3UCBlfgLWFJcyVdkQg7PWRTR3ScyMHXhyBMOlAGP/GJvl8AZkgEPR4SEWipGcXWb93H43ZTDqTIbGzhzWdQWswoNDrqLDbycTjRBaWOdjcxuyqQaXyk4pFSY1NkY7GiPgDKFRqUpkM8WiURDSKUhQJ7HvIp1NYa11EdtzYWkI4ezrR2ypQtzYSnFlgf3EJSzCEqFQSmwnQfHSIVChCPBjEt71LdUszU//lmzQM9hK7M04yEsazsYmzrYXNsQkEmYitzoV7aRWjXo9coWB7fgG5ALl8HvfsIha7neKBn4XrN6jp6UI2Po0olxMPhoneHEOp07L63g2aHz3DwksXaDl+lOTYFKHVNbKJBGqlkvVbY+hs5UiAQqlk4eZdXL1d+FbXqMhlyUUipA8OqDtxijKVBmuNi8jJ4/Q16zk53MS/+r0vU+OwsLkbZnXTi8crw+UwsbkX5fXLK6iVIulUmms3xxjs72VxaYVvvPASapWccMhPOB/l3UyGcCjK3ekDdGoBi05g9yDJ7I4OfyjO9aUiJqOeiYVVLqpEkCSWNgKIMtDJzNhNJbYPktTXWPjSa8t84HQX1xbziChZ3wnwwZFO0hjQqfPcWMpyY3yVvjYnlySJLU+cTL6ERWehpa4MbyjD9YUM+UKR6aV91Kom6hxWnDYDyXSWFU+B21OrnOx3UV2hIZIR+PAJG9FEjmvTi1ybibG8HcBh1XIxl0EQZNya2uXZc11s+NNsuSOUmZS0uayk8jL2wxJz+1nSmTzza/v0NFciCgKvjkXQ67Rcvb/JoyNt3FhIoVVBndPKc8esvDoqUWbSUq7P4HIYaa7Wcnclx/puiMltO/qDNEJRwmk3k5fkSFKB+U0vaaeJ1no7x9q0bLjjHAQTrG4HuLNWgUGn5g9emKOzyYHu4GfBRuMXJfkPcE0QhH8PaB60GvgXHHZwfig8TM7mdzis9g8AXwb+Z0mS8oIgiMAq8Au72dS6aimvdWEa7EFh0GNUqclEY6jMFiob6pED8UwGbS6HdqAb/8QMNSeOINrKkSlklKUzZExG8rkcdR9+mtTsIvI6F42njpELhNH0dFAejVPSqEjm8tjaWxHtFSjs5dhSKfK5PIbhPrgHNqcTqcxC46kT5KMxTCPDZA/8aO0VaFobsUkl0ukUZbVOwu4DBv/F50gvrpB8ULUvqFRoEgm0VZWo6mrJTs/R/LEPkZmcRz8yiCGdRiaXI2hUVB/pR2HQoTPoKcaTyJVKlAJ0f+J5Els7JCJhKqu7EHRaNG1NGOMJjGUSBZ2a2uNH0FfaUDfWkbt0A6XJhK63C1s2R6lQwDwySOTAh8JZRTIQoKy+FkNPB+G5Jbp/7Z9Q8gWQN9bSkEmj0KjRDvXiH72Pvake86mjRJZWsVTZEc1G7K3N6FoaUdorcJRKFPVa5JU2KtuaMTgqMfZ3ERy9z9BnP0VkZZ3jH/sIwfUtGp95nDK9EZPFSlN7Gxe/+Q1+87Of5MUXXqC1PsrTjx9hfTdOPpfl088f5e7kDogij51r59TJU1Q5bPzw1csoNAGeeeIEZl2e7b0gH3nqKG/LU6RTZqLhCKcGnNRVqtCrRAIRA0aNgnq7QCFdjlpR4kiTiol5Nae7DNxdTvGZpxu5vRjnTJ+Ji/dC9Dap6a9TUMi5iMTSh8yxpRSfe6YRd7iEQi7SVG1k0xumoaYCpVLJcIOcQsHBcKOcya08BoORNqfmUEBVJicSM/Noj5Zr8xBLFzjVYWLdG+F3P9HF7aUU3gQ8OVTG/E6W5b0Uv/uJDlb3sygUMiQJhtvM2M0iy9sRjjYpOAhlMeuqiSQlcoUCfdVQEERs2ixxvYoGZxnFQoaqCgPPHLFwcyHO08frUalEjrdpuDheJJs71PfV6/SUkLHsKfC5p2q5Np/mkW4dyUwrsXiasx0W3ryfwWk30mBXMLeVprPZCQKc7lEws5OjQq/EYDTzuWfMCHIRuSgx2O6ksdpEReXPgI3GL07OBvi3wGeBWeCfcdja5csPO/hh2GjlwEckSXpCkqQfPKi5QZKkEofN0X6hUX72OJG790mFwhR1OtRdHax972U0PR3kMxm0MhmOpx8jdOMOqkIRfWM9cy9dYOfieyjrXRj6usgEQii1GtKJJJGxCXRd7ajamkguriIz6FBU2dm/ew+ZIBCYmMZ/5Sb64X70/V3MfeVFgj4vy6P3cN8aRVKpKOq1LH39O5g6WklEY2y/chFtdztlx46y+/o7oFHjG59gfWycmMeDLF9g/dJ1AvseSuk0M9/4NqlEnHwqTUqQCM4uYHI5IZtDiYj92DDRlQ0EmYycWoF/ahaVTk9BIZJNp/FML6AqgWdmnskvfJlsoUA6lWL7+l20dU5iW7ukAyE05VYqzp7Af/02qjIL5Y+exnf5BuU9nSS2dkhv7eB88jzxqTk0CiVaewVpX4DIzTEsJ45SUCrIebyoSxKCXk8qEEKMxik7OkTw3iR1zz1FdG6JhMeLzGrG2tdFbHYRna2CVCBIeGkFndUCGg3u+1OoKspofuo8+9dvo9Go2Qv4WJqZYbi9Eb1Oy6OPPc5/+vJFRvobePPSBDfvLbO4uk+FSYY/kuPDTw5z6doYoXAEmUJBZ0cjX/rq9zna38SpI838y3/zZwy1l5FK51Er5ZwfcjC1keHt8RCnu3Q8PlTGD67u89SIHZO6yJv3o5zptfGFl1eptsqxm+U82W/g9nIGtUbPYwMW3hoP0+jQ8uywgW+9u8u2J4rVqGLblwVK/OBmkO56Pe2NVTx/wsIXfrjC9n6ARbfEjifG0vo+sZycV29sMLsZ4ZkjZbzwzg4tlQJmdZY//cE62XScW/Mh0pks6zs+tkNyXnxrCYVYIJkpcG3Kw5EmDV21Wt4a2+f/+sYcn33KxdxOmumtPMNNatLpNDq1AqtBzjdeX+DSVACLXonLbmB03sdIm57vXvFQbtJytseCWkzz5z9ao7dOgcNY4pWbHrpr5GTzRUw6BaIoEkukmV4L01Kl4AMDBn7/K1MU8xm8gQjffPeAI01KCtkYC6v7jC1GefX6Jj+87uFIo4LmGgPTGyk2fBIfO2PHG07hPvD/TGzIT7Oo82cMDYeqBR+VJOl5DnP4mocd/BM9G0mS/rf3ubb4sA/6eUQmmyU1MUWhUGD+ay/SePwYwZk5Srkc0Vtj7M7MYLTbKS8WkStV7C4u0WivoK6vB5XZCGolofFpYn4/iel5IntusrE4pql5hEKRybfewdXVSbnZhOvMSQy1TnxjE4Q3dygAgl6HzmLC0duDSqXB3FSPpr4GeSJB7uZdspkM2XAI/9Y25oVlZEoFwa1dTK3NVA72oiiW0Dgq0XW0IL8/RfWZY2irKhHlcuRqNaVQFI1MztrVG7QMD7M7v4ix0k4xk0WpUrI1O0dtVydLl6/Tee4spUgcQ2sT+rUNTMeHEDd3yF6/g/2RkwSv3sTV2YaYK6AxGJj4y6/Rde40mal5IqEQqWwWpddHeHsHxYGP2v5eNu/dxzY5h3t1A6VaRT6bJeb3E/MF0FssKAp5Zr/7Q/SVdpS2Cha+8V3qB3tR+YIkQiEyU/PojAZmv/ldOs+cJDM5h2dpBWN1JXqjie23r9B6ZBB5sYS9rBxTKkdqb5n4nhtvPI6pvIKX//I/8/Hnn+X1Ny9RLBTxBmLcur9Ha3srDU4TI31O/KEYVybu8p1X77Oxsc0fL6zyyx97hmAkxszMDBf0eba2dqmv0vPujUWuj67T6rLw3iRMzO2gV8u4PKsGCdLZLDeXcwjIuD25Rk9TGTqNkpW9JOG0kmQ6z+KGm/oqI7t+HZML+0iSE09E4NefcjGxVWB8OYo/lOfm+CrPnGll25thesmLVKrEVWnB5TBxvEVBIqHEVVnF8TY9nmAlgXCcdZ/A8k6Y6io7K5tJBEHiySOV6DVyptaiGHUVaGUZnhipRaNREY1nCITi7AdMtNcaeXywgrfGRW4vpbk9tclTJ5q4u5RgZcuHVqPCqK7kaE8ddouSE20qSqUSl8fVrO8lmF/3odepWN7Oo1OJ7Lgj3Fk0YjFqWd71M9hqYXR2myeHbCxt53CVSXzv8hYnBhoJGOU019rpbzJy4UYco65AJJ5FJgq01Nl46qiFZFYESnijEuu+NGOzO5wYqOeN8SiRWJ79uaW/fyMi/UJ5NpeARzlsigmHG807wEMxLx4qZ/MPFWqVCu1wH7mZeczhMOpaJ9ZiAWtfFyqdjhpKFHN5dH1dxLa2MTkcxAMByrrbicwuYGqqR6/Voh0eJK+Q4+zrJouEzGxGMOlpT58gtLNP3uvH0ttBenEVk8GAtduK4dgg/uu3qfvwU0Ruj6MzGSAcRd7eTGRsirZPfZSiP4RSraXmd/45yfvT6Pu7aS1KpN0HCO3N6M1mpHSW2NomdY+epRQIEtjYwToyQGh0AktHMxGvj8aBfoTGWlrNBlK+ILquVkKzC5Q7naRTKdo++iGy2/uYezsIXblJWW830Z09hECI6sfOEF1aRVNeRlGvQxRFcgoZ9sY6cqkUmo4W6pvqyXsOKCSS2Ls7iG1sUzAZcA0PgU5LbXcHhWwOy+kRhGt3sJ0coeQPYhgewpVIobSaiO55sFRVYj42THRrB73Vira/i1QgSGP2KHKzCV1HC025HKJeB+EIJz72YVRqNQUBOj7+S8TDEbofP0chFkfI5nnqVz/Fq3/2eY4O92K1mHjvves8/+wZZKLAQHcTWztuJElict7LQG8Hn/6VT/Cj195jdmYKuRAn5Nui2q6lt0mNUrIQTeT5wIkqKBSQiRLtjgJibzXBSIYjDSLxVJ5pg5peZ4lLs2n+9cfb+O7lXT79hIvrCylOd6gQRTX5nIUNd4T2KgVGvZZisUizQ45OIydfKvLoQDkv347wwXNdnOnQ8NLNAPXVVs51abgmCMSSeUqlEoJcjUyu4M3xMI90G9j2yhDFIq115dSUiWRy5fTUCMzvFRlpUbAXkaFUKfDFSzw5YOKd+2Eamw08c6aVUCJPuVnDreUoNZVmGmwibr+V4+0GVEoZ+0Eboigw0KghloFIPEkspeDuYozPfMCFJwLD3TXUVxroa1Dz1niI3/pIC4GEiDuUw2jQE46lEUoSoUSJMpOG/WCWRqeV6jIVFfoCuq4yPFHo66gmlSnQ16LnIJkmm02TL5SodRgwa4oUUGJQZfjcs+2seHJ8YMDAhdEiJ0+c+nu3IRKHJIFfEKglSfqrjQZJkhKCIDw06+Ifizp/AoI3RxEEGZV9Pcx9+/som+sxtzZRdB+g0euxP/EIoTtjxJfXsdTX4rk/TXp5DYVMztQXv4baaMSgVLL+1ntEPAcUgdjCMtE797GcGMHsqETX30347n2WLl1D09NOMpsmFQyhMZlQaLUYhvvYX1ohJUgk9j2ojQbUZVZW3znUIZMr5ZT0GnbfvoyipgrrIycIXb9LUakgk0zCgR+1q5pUNI5ao0GuVlN+aoTYvSnUmkMvODO7iKa1ifLTI4RHJ5Dli8RTaQ6WV0mvbpEIR7n/H79IVpLIJ5NsX3wPWU0Vco2aveu3EZ0O5EY9u9duo1Qo0VTasJw+RnxhGenAR2x96/BZRgMqq7+zlMkAACAASURBVJmVV95Ap9ex+OY75A06RKeD2MIKCqsFg7OKkl7HzJe+TlEpY+P2KBLgOHcK/+WbpLd2sB0bJrmxTWp6AdupYyTcHqI7eygrbfim52h55DSuoX7ivgAHoxPUdXeRDISY/P4rPPNLH2Hk3CO89s0X+O3f+dd85/tvkEgkCXi3Gexr5Js/eJfdfT/PPTnCOzc3UGos2Mv1zC2solLpaG5qIhyOoxGTDHdVceHdBc4OVJDP5/jhe+uc6dYTiqa5OZ/iZLuGZ0fMvD0Z5/ZSmo5aI3/y3SVkpSzbnjhqlYJyk5Lz3VpuLqZxB1JU2QycG6hkP1zi3FAlQy1GZrdTTG/lSGdyXJuLcqxNR74Ab90Pc6rThKtMxiu3PDQ7RKrMRV645GakRc22J4pZryAQy5EuyPn2O6u4HBa+9fYSKlJUl2vI57JserMoxCKX7yyRzuS4cMtDXYXA1ZkYSlmRvloF//WNbfobNBTzeTZ8Ep/9gIu7KxnenQxzusvIkSYVoytplGKRDwyauLWURZCrqS7X4gkXaajUsOFJks7mUSoVOCs0vHZzE5tR4MMnyrm3muTXnmomnpNTVa4mXdTw2adqSacTfPHCKmp5iallL60OkfoKidHlKJUWkccHzHzx1U1665R01hkIhBO8N+knGI0TDAb5wxcWON/1989E+yv8AoXRkoIgDPzVD0EQBoH0ww7+R8/mfbC7s413ZR1LRzNlPd1UNjXgu3sfa3kZ7tU1RKUKWyZDIhAml89SVllBWWMd6moHqroaynb3UNU7kVktWJZXsba1UFLI8CyskPD50ZpMAKx970dUt7ZQ19tD9P4MSpWShW9+j7YzJ4nfukc8l6GYy6MWROZf+D4dZ07ivXIDlcFI1u0lkUihKpbwzS5SXlZOoVTEvbKK3molG4+j1GpRjE1ysLKGQqNCrj5sT7wzOYOl2kFwdx9TVSWayTmkfIGwz0epUERbXYW2upqyU0eRKRREv/g17KeP47l8A51RTyocRsoVyKbSxDZ2EJQKcukUgZU1EAR2L19Ho1AQWV1nd24etU5LLhxGoddhq3Oh6+vEvLBIxhvAbDQw+94VOs+dIX5nnFg0SjGXo3xkCAUCCpWKlOcAmUqOe24JlUKJd3Wd8t5OfLML5HQaNr/9Mt1nT1EMRykLxhEjSRrMVm6777Fw4U3WxsZpaGpi7tYd5Eoli6PjvGUpQ5Sr+Wf//Hd55PQQ92Y8nD7zGKYyAzPrCSbm93BW5Wiqr+JP/uzLfOrjH2J8aoErl/c40tfMytoORlWJC9fc3J3cwKpXcEWEjR0fuXyBV6Q8MpmMfU+YTC5LXaWO7pYqnhy24ov8v+y9d5hc6XXe+bt1K+fY1bk65wSgG6mR02ACJ4/JYaYo0ruSVtZ65bW0K60tS157dyVRkm2JEiWRQw7TDCdgZoAZYJDRAehGB3TOuatT5Zyv/2jQDx8+EjmkaM5K5tvPfe6tW1/6p79T53zvOW+S0dkF3i+0k83CxLyb3pEcR9uKCWfk3Bx0c/pQNVfur+HKNzG34mV60Ut1mR2NQsad+yvkO4xkcjJEUWRw2oPFaieb07C2tUHPdIKRqRVM6kIKTHqKLCLNVU4q80WWi2ys7CTonYqTEzX8xWsTPHasirqqAhpcesrz5Kx6kiyureDxKRBqHMytetFp1YzPb1FeZOHKYIYHUxsY9EqMWhVZSeD6/QWcZh3RVB4DE6vYzTpi8QS9D9b4pcfKqC+S8XtfHeFgayXd0ykEIJWMc28yxvTiDvkOM5K0S28+c7iet/sC5FIxCvPMCGRxb/vpmdRhNum50jXOif01eCICwXCC4eUsMlJEokkSiTSVBXoyGRmeUJorA14K63/+bDQJicw/nTDabwCvCoLwfQ2dAuCjH7TzL4zNj0BJqQuHqwRzSyPBuXnyOg8QGZnEcKgdzdY2KoMBTXM9Do2GWChEeHiMkkfP4L1zFyEWo+DIQUIPJhBcReQfPUB4Yg7rkf3Y853YWxsQRTmiCPqkDbHYiUanIZWIk/YGUOk0KEuLUNqtRLvvUXLmGAqNBqPdjnZvM/EH41Q//xGC90fQ1FYS9wWoOn0MSZSjb2ujLCchqlWko1Hkag2KxlpcokhGALnNirzQSVU6Q9IfQNCo0el0aPY04b3VS8Xj54iMT5PLSVhOHSHQdQ95hQtnRxuxpRVMVgv5J4/iu9mN7fghJH8Qg8mApNdhOHOC7LaXeDZLaGWNvI8+TWBqhprSIuSxJJaOVkLd/WhtVoJzixQeOUjO4yMp5Sg72onosKEpLyV57Q5Nv/QJEjOLaDRaUnIRc3ExuTIX3uU1tJVlaHZ2SGxskXfkADKPiLXQSf7xQ7hsNsjl2Hf6BNde+ibnzpzl0Pmz5JssJBJxnnn2OTKZDMM3b/ORR4/R09vHM089QV11MZCjvNrG6OgIRw5VMbu0l8ryUry+AHW1lSjkaeSyNK31pXzkeDHfCvp4+nAeNwe3UMsh327kcI2cYMCAWqXgcIMBpQjdChGlcvdM5lyrhveGohSa0vyLF+q5P5/g2U4rIjY8gQQNRTLefxBjf3MJ6USM4jwjh5ssOAx2vnNLSzIDzWUaZtbzaKky01qm5vUeH/Xldg5Vybg8GKGmPB+HLs2nH60hI8kpK9Dy5t0gnzxbzM2xOEUFNtLJKHVFCiwGOeFIJTlJpKbUyvRagtoiE2s7KZ47Uc7iThajMskXn2pgZiPD/qYSBJmMJ/ebSGcl0pkcNYUiZp2cnUAhVoOC/dUKBKkUBBmnm9WMzm0z746Qy0lUFFnZU6XDqMqiVZQSSog80q7AYTXgj+R47pCZaDQfchke3avnrb4spYVKpt1p/vUnmrkzEeNcq4ZAqBydSsRpTNNWY6fEBi6Hlgv30nykU4c3KhBOK/i1pyvomkx8KLXR4J/OmY0kSf2CINQBtezWQZv6PmHsg+AXYbQfA9vJToLd/ciDYdQOO8q6Su7/0Z+jctiJhkKMf+3bZJVyBK2WjelZNq7dJp1OM/36O2STSZR1lWxc70LjdKLf18LkN75NOJlESKZYuNnF1oMJrEWFDP3Nt1i7dx+dqxRBLqfx858mNDFNetuDTpBhq6shubxGwf69REcmUSfTKM0mbKc6CfT0k5pZ2K3FFgzhvn0XRXUl/pU1VMWFxMMhgj196NoasextIby0wuq7V1HXVWE41IF/eZVQKMzAl/6cnFaNTK8jazaSSaeQyeXk8mzMXbiEqa6WtTs9aJvrEBUiho5WJr/6MsbGGpLrmySn59BUuNhcXcG/uEjR42cJ3b2P4PFjbW4goZIz8uWvotvXTCaTIbe+ibqkiMX7Q0Q9Xpydu6Gx8Oo6BlcJaoOBXDCITCHH1t5KZHyK8OAI6rw8Zi9e3tX6USjY6eqDjS1qP/YsG739WArzWdtw03flGqeOn+Cp557l8utvUF5RTnl5BXPT07z+8jf5vT/493z95VcodNp54bnH6e4f5e7ADM3NDTzzzFP8q9/9Yx47d4JwJEYuC7/1v/8LAhEJGXDqSCPfvjSLhIzvXluhslBLa10xHz3u4NL9ELFUjsc6LHRNJrg0EOZUi454MoNWI0cul1FdIOPmiI/SPC3nWrV89fI64ViKcCzFtdEYzx4yk81mkEQNzx/No2ciTCSRpcBh5sVjdv6/705j0sL0SoQ3e70cazLw2AEH/bNRzEYNVfki0+tJWiuNbAVz3Bjyc7huN5QUiadRy3Ocb7fRNRVneD5IY6kSn9/P9PwGVm2Kb15dx2FWU54nJxpLMefZrRpg02ZJJDPUF4q8cnODtgoDHzvu4NZYlNe6tthfrSGShEv9IY42aInFYsythXn8cBlWi5mcwsQXP1LOyHyES307NLs0hCJxLt/30FamoiJP4J17O3Q2mNlfKecPX5khEomyvumn0K7FrJdTWyDj2qCPRpcGgyrF2z3rPH2kgOn1LD0TAfZVaci3KPna2xNEIyF2/DHSqSRu99aHsodIH/DvHwlqgQZgD/CiIAif/qAdf+HZ/AgkU0mSQ2Nkslk2p2bIZiVC0QimAie2vS1odjwoRDnmlkZSsRixjS3yT58g7vNjXlwBnZbw4jKRgJ/AwAPkZiMCMvTF+RjrarBtedDZrWhbG6je2ga1ksDIOJ75RfQGA1qdjpk3LyKIIo5kktmePgpaGkn4fAgIJNMpNGoNO6vrCDIZit4B5Lkc6+MT6EQZqxOTKPRa4j4/0XAE7YABKZtDzOXwLq6gUmuIBoPkcjlKz58m850gCrOJre4+ZOkM24tL6Bx2lDotKo2GncERYsEwO119pLNZBIVIIhwhPD1PwusjGg6jHDYhkyvQ6PRkVtZIxeJ41tZQmYzI5XJEUSTQO8D6yBhGh53szRx6q3U30XR4HJkgY/b1d6g+fZz0xAy+YIjE4jJmr5fQ5iapaBytyUhpQz22k51kkknWb/XiWV1H92CCxbv9lBpMCDmJe5cu45ApWFla5t6tO7Q0NGG123jl299GyGR59+23GBkZxWEzsbLmRqlUMzwyxddffoWNDTdmo4FLl29y+04vBzra+PZ3XkElF1hdWWFmzo7VouO1N/s4d7SZ+c0U0wubIOVhMenoH13kTqGVifkNtGoFvTMa5pY3MOsVvBnUo9Pp2PZG6JmOoxRzTC9uc74jjwl/jJyU48oDNcPTWxQ7jVxMm1DIZfzBSyO0N7kYXNJhNugptCq4NrCNTBQx6HUoFQIXbi9w9nAd69tpZha3ueEws7CyTSiaQKUpZ2Yzx+jMOgatCm/QzMaOn76REJ17q3A5dcQyCrTKBGNzW1gsRjaDErPLW+RyEu8pRFRKFXMrq5Q4q7g/uYFSqWBkQcIXjLG6GUCnN9E/toxWKeetTALI8dJ7a5zbX8i799w0Vdp5u09CpVEzPb5Mb74do17F9btrIFOiUCi50b+KTF5HKBxAKVewp1LHN66soFWLrPuMKOVyLvcucHx/DetuH3K5yJu9PmaXd0ilc3Q0u7DrJUxGLU8cKmBpK4k/HGN5Yubnvofs5tn83Kf97wJBEP4Nu4KYDezm2DwKdAFf/yD9f2FsfgRUShWq1gZk17soqq3Bcuwg3OjC8uhpQj39ZNMZjHXVJNY38M8uItdqSCeSxB+MUf7Ck2R3vKg0Glo//SLp9U1Qqyg/c4LcQ76/3mSEZJrQyjqG6gqii8soigsw53KIriJEk4H8UJhcJothbws1st3YvE+SUOQk7Cc6EQSBglwOhVZDRq9F7gtQ0daC4cBe6uQicq0OBAFjXh66/bsietvv36KitQntvjYU9wbQFjjZHh6l5Pwp0nPLFJ48gufabYoOtqPIcxBcWUNntyHPZik9dhhDYT7qAic71+/Q/PlPkXRvIaYzaMxGtI21OGJxEukMmupy4j4/tqIitG2NRK/dwVpXg6m9jcJkAl2+k1Q2S2FrI+GhUfR7mkiNTmArKUJfVU4OCXF+Hkd5KUq7DZPTiSBJSOk0Ur6D2MwCutpKzDodhe17kEwG7IX5KDM5zn3q4wxo3kLIZXnuI09z88pl6ivK8fn8COk0dXV1vPixj6JTptne9vLPnn2Eb37nAu379vDMM0/x5uuv8quff5aBkVkG7vfzSy+eRqfT8OW/eYXPf+w4SnmW5bVVOlrKOd9uYmwhgEIu41ybjsG5EGcPlGLXpGhvKCIUSbCvQsXwtIJMTsHTh+2kszky6TK0SoHyPDn76pxYjWoeOWghHBc41awmlSlFp5Jzpk2PeyeGRlWLhAybLssjBwrZ8CXpaComEElyrF6JhMTotJX6IgXDcYn2hnzaykRCYQPxpI495UomV6I8cqiCRXeEs3tM9E4IhCK77Lm2SgPXx1JEoxJPHS0hzypSZJPj8Vsx6lQ80qqha8xPidPInnKRTW8J5QVaXA6RrkkFFqOG83u0xBMFKOQiT3QYuTHso9BppqHMyN2pAAcanVQVKOmfDlCUtxtyvD+boLLUzlMHrfTPhDl7oJRyJ4ylNDxzxMC8O8HnnqhnzZflbKsOtzdKqLmEKqeIXJZHqVVCrRKJxU1kcxJ7yuToVDBfbGTDlwYkSgvNlDT8/NlowD+WUjQfBM8DrcCQJEmfEwTByc84qfN/aHge1t7KGnSElpZROezIZDLiokgoGMRcV0VqeR2dQk7+8UMsvfoGlkMdaC1mCEVQpDOo8/NIBIKk5pfRlpcSl7LsPBhDVpBHNBolPb+EuqwE3Z5mdvqGKD57gtjUHJ7rXaiqykGvYfxr39rNA1leQcxksB/dT2RwhODSCsriAub77iNFohgP7CWaSbN5+y765npksTh6gwFZoZPEqhv/8BimpnrSmSyB271Yjh3E1tpMeGYOjTOPjE7D2sWrGNtasLU2ER6fQpXOYGyuJx2L4djXSnppDd/9Bxjra1Hodcy+fx3d3ibsJzoJ9z9ArtFgP7J/t9ilWoO6poLw3CJqixlHx14CI+MYy1wk4wkSWzsorWbULQ1Ep2YRQxHKnn2C8NAouUwOW3Ex7vFpZDkJfWsDQfc6gt2KoaSIyNo60e0dRLOR6ds9ZN2b7P/8p4hY9Fx/7Q1a9+0lr7yM3/nd3+U3fuM3GBkZZXh4mH/1m7/JE48/zl98+S+BHOfOHOLCxWtoNSqkXIZvfP0lPv7CIxiNeu71DfIrn3+aC+/2sL6+RbFDyb6WMroGVyiyyumoNzOzGmHeneTjp4sYW4rjCcs42Wbja+/NEQgEEKUk/8+3JjjR5uD8fhtXhiJcvu/nZIsJT0Tg1liCJw85uPHAw94KNaFIjL7pAG1lKqRsgk1/kntzSU63GtDJ07zTu8nGdpCrdxfprFNxfo+BuzNx3rvv47PnCrk3HUZUanlsv537cym0Gg3PdVr5w+9Mcm1wk6V1P7lciv/wzTGsRgV7m0o5Wq/i7f4QiyubyJUaDtRbmdnI8mZvgKcPmtEp0mz40oTSal44Vsg3rqxzuN7AqjfL+0MRHt2jp8gCgzM+Kgs01BTIuNjnwaDT8PwRB4s7EkdaClnajLK4lWY7LOOpQw76poKkcnLqS9QMzQZI5uTYjTK+dmmWU816LEY1M+tR6kvUZFIJQrEM92ZSPHXYwVcvThMKh5jZSPCXF6bIZZK47AJX7m/z8rV1njiYx+1RP8GESGfdB849/JlC4p+Unk38YTJ/RhAEI7ANVHzQzr/wbH4EFucXSEQihPuGSCeTLF69Sd3JYwTu3CW8soraYMB3sxuve4NMIkWRIEOmVBIeGEal0TJ+7Qb1p48THxojEQoT9vuRKxVkYnFWu+9RuLcN3+ISMklCrdcR2Nwh4g+yduUm25Mz6OwWFLEoZHMU1dbiPH0U5cwc7tu9JMdnCG3t4O26S1lLEyqtGt/iMrJ4knQ8iSydQaZQsr2+jqmsFFO5C8+1WwS2PDjKwqwMjWArLSYxMEomkyEZjRG9N4Qsk2FncRGd0UBClO3q70QiCDOzZFIprIOjrE5MIOWgJJlktWsDjcFI2r2DlHazs7RENpOlMJFk9u49bC4XuliMhb77FDQ3IoZCrN8boPbwARKhIFuz8xj6hlDIZIzfukPpvj0IEzOEohEWvvpNXM0N6IxG1gceQDjC8sgExUol3pk5pGyW2b96iZoDHeQ5HLjyC/F395NOJunquYcmmaG4tJSlpSUuXXqXhYUFzGYT3d3dRGMx5uZm8W2r0KhVXHjrEg01RYxPLtPSXMcbF95DkiSGh+5j0eVYWdvi3r27nDhQw8Ub0/T1j2PobGA5Eaerb5bnTlaw7svw7SvTHGwr50KPF6fNwP4GBw6TnNGlMNPLfhbVOkKRNKubHhBERqbXEUU5CqWceCLDe4MRgpE498c9tDcUI1co+NNXxjl9sI6uqSQbOzEMWjltVQZ6xr281u3BbDIws7hJaaGFxa0UBiUMTa4iylz0Di9S7DTyTkxDcYGV2lLzfzu7mVoKMr0aYWkjSCpViNmgZXFth+nFTbTqYu4MzFNX7uTeXBq9VsmVIR8FFgU3hsMsuH2MrDjoHZ6nqbKAnpkUToOG17vWOLmvFE9Ezu3BZU4frCeRkdE1tExZngqNzsBfvzVGjSuPGa2NS72zPH60jjR63uue5tT+aoLJLCUFFu5N+gnERYLhBBfu+iCb5E+/N02hw0TXpAKnzcipPXk4TApmlwM8eTifdCaLQh7lwp1t3hvQMzbjxmnVcntChtrp+7nvIZIkkc5l/7vPIwjCvwW+AHy/TML/IUnSpYff/Ta7ZWaywK9LknT5p5zmviAIZuArwAC7yZ19H7TzL4zNj0B5ZQXm9UWMhzsIL6+hW1lB01CDTK1CplQiV6nQtjWSudFF1OPFcHAvgk6D0lWM51YP5uIi1GUuktEoVrEMjceA8XAHykCQ8PYOlqoKFIBcENDsayFxs4eCxjo0VeWYi4uRggEU+U7S0QQxUSQVCsOmh4LqKizHDpHruockFzEe7kAxbSHh9aFua8ARjTHxjVcwTpcS9QdJRqeI+YPszC1R3NqI9ehBlGoV8UgUZXMdiflFTA4H2v1teK53UdDShG5PI6lQFIdajZDJkstkyFrNiFo1eeXlKNVqjIfbydzsQXf2BJn1TbRtjdj8AXK5HIaDe6mSciSjcWzHDuGZX6DwRCfBmVkcVRVoaipJjk+x93/5Ap7r3RjPHKXU48WQ70RdUYpvYxNraRHGgx0o5hdIh6Ooayop3txGmQPrkYPEtnZI+gNYDrVj9wQI+nwcfuJRZDKR1tLd2nXerS3+/L/8F65cuYLbvc6xo0c5efIEr7/+Br/2q7/CYH8XZ08dIuB1EwyHOX2ig1Qmx/MfOUQgECYZdqNSCjgabNzo3mR/g5ZLt+f558+34Q9niAgipQUWnGYRk06B2ajlkT16bo8G+OLj5VweClFiF/jUuXIWtnMcqVPzWo+f2vJ8nthvJhpPo1YpeGyfgdllNcdbjPRPC2g1KtqqLZQ7FWz5YpQ7RSrzVbwV0pOnVLK8GeeTZ13cn41zslFFJqljammbluJ8wilors6js1ZJLJaPzaThaIOG7qk48USS7YCCbX+UZ4+WkMhKKBQKEsk0Z1rUxBP5SAgcqVOSylQTjKQ4Uq9h0xvj7XUvcsHCU4fz2ArlKLbKONTsQibKOdqg5Wq/m3Q6S3OJnFl3jNbqXdVRk1bOgykVWp2eJw9aUak1RGJJDtfIGZgwcqROxfUHAZqr8qgrFrk7reHTp02MrEokcklqyp083mEmkcoyuRTk1B47xVYRlSKf3ukYR+pUnO1w0j+fptopEMlo6GgqJN8k8i9fbOLOeITTrXI2VdYPZR/J5n5uWTRfkiTpD3/whSAIDcDHgEagELgqCEKNJEkf2AIKgtApSVI38L9KkpQEviwIwnuAUZKkkQ88zv8g0jT/De3t7dL9+/c/UNs333uXL9+7g76kmOjEFJZjhwj19JOWcpj3tpKdnieaTGKocIFOg7/nPtFIhLwyF3GfH/upI6y8eQlzUSGmA3uJ7ngRfAHi7g2sJzrZeO8G8XAYmdlAaHYRe8cectkM/tFJyp95gsjUHLlAkMLHzyDlcsx/901Kz53CNzML0Tj2vc1klSqW3nyHimeeQK7T4r/VS0oQ0JaXoAhHyMWSpGIx5GXFKCRIRiKkwzG0Tjua8lJ81+8gV6mRV5WRXN9ErVGjqa/Gf+cuMpkMeXU5s999E3tNFZb6arbv3sdaUUYWAZlOi1qlRFtVzs61O2ib68DrRyzMx9vdh7WxDtFhx3enF02hk5Q3gMZoQL+3mZ1rd1CoVZg795MIRUiMTqDWaIgGgthPHSFw5x6pTIYsEnmd+1Go1WxdvonOZkEoL2XpzUsoNGqqPvYMob4hyl2llHbsZfr1ixhVaj7xqU/yh//X71Gcl0dHRwevvvoqNTU1mEwmWpobQZDoPHyYr/3tX6NRZjlxsJTl1W3euNjLx58/QziWYWRkkhefaOLr3+shFApwtL2EzZ0QmUSKYpuMv/zeKIdaC3n0gJO3u3cwqlJUFemZcaeJJiUe3atjZiXAt6+t8PTxCsaXwohyBU8ftLCyHeXWAz/PHivCF0qwvJ2h0K5kcC5CqdPA8WY914d9aJUyVEoZc+4kpXYBu0lNIpHk1oifLz5eQjYr8cZdP3K5iHvLj91i5IVjdgTg2kgMCZFoPIFRlWZvjQWLXsHrPX7k8l3q8ktXNzhSb6TAIud73R5MOgUV+QrWduLUlJjRqSRGV9KE4zmePGDm+oMgO/4ITx7K58pQEJVCgdMkkWfR8mAxgcsmodcpmVhL8+gePfdmIqzuJHn6kIWB2SgFViXr/hytLgXXH4RIp+Lsq7Uxu5HlXJuWN3r9OMwq6gpkfOXSCtWlJh7f76B/LoU3mOCxfQYuD4XRyJO0VFoIRjNc6d/m848W87eXltHptXzukWIu920SySh47rCNVCbLhd4A7Ucf45lP/cufaL8QBGFAkqT2n2KrAaCmtVn6z5fe+kBtHymu+KnneujZRP4OY/PbAJIk/YeHny8D/1aSpN6fYOwBSZL2CYIwKEnS3h/f4+/Gh+LZCIKwBITZdesykiS1C4JgBb4LlAFLwD+TJMkvCIIA/CnwGBADPitJ0uDDcT4D/M7DYf9AkqSXfpbrTCaTqFMZRr76MpVHDhIbncS/tU1420MimcI7uVvoUZbOoFCpcE/NoDIaSCDhc2+g7hsisLFJMh5HlpMQlUqmu3sxF+aj7BnAu7aOpaQQQ30t4fklTGUlSJks4dkFCIVByrK1sIjyVg8ymYzA5jZF6xsktz2EdzxodVpEUSS87SH+YJxsTsLv8RDZ2SEvk2Znehad1UpOgMjwCKWtTag1GmZ671K+pw1ZOErI6ycWDFKhUrFw6w71xzpJDY2z0DeA60A7ilgCu6sEg9MBKiXZXA73gzHK97QyceUa9SePEx6ZAKOeC8WA6QAAIABJREFUsZe+TWX7PtTBCOtjE6h1OnKLy2zOLaD3+fGvr+Pa04owPI5o0LNwr58avQ6ZBL51N7lsFlNJETMvv0rls4+TzcHqxffxjk8jBEJEfX7mh4aof+wRaj/5AjvXu/Dd6GZtahqLyYD/jXcIuDeIZnJcvfAWeXY7jz76KB0d7fT393HixAnc7nW+8fI3eeyxR7l1+w56k4WR/ptI2RgPRqexmtRMTc9z5+4EopDhe+9mmJ5bQqcUGBoTuTs4x0fOtpERFZSXFRKJp3nnXgC3N8RsOLYrKuaJ4PGHeZd8bDoRi0mHK0/BxW4vgiAynKcmEs/gDcWYcEtksiquDyzQVFPI/JqPbW8IhbwIpVLNa9fGONhWjlql4GLXAu1NpSBJ7PjDXB1NoZQLTM5vUV9mQYbAzIqHy8NadCqRxbUdXIVWDlQreeNOAKQckZSCYCTF1o4ftVLBxOwGxU4zk+sJtr0hwhElJmMBt4cXEVVmVEo5V3qnOL2/gr7ZJDqthrsja/Tl2ZGJIn2jSzRX2Xnt5hKdzfmoVAYu3FnFaVXTPyfnrVtznDxQxf2FDIKo5stvjNG5p5zBJQV9Y+tUllj56juz1JZZuDwsMDq7idWoRMiYKC+ysL4TZWAhw72xNeQygauKXZLM1T43ap2NnKRkfSfIe4MGLGYjjaVq3ukPseNN4A8HeL0rg1KlRhRF3n7vxk9sbP6hkKSfSGLALgjCD/4S/itJkv7qJ5ju1x5Ske8D/9tDdeUi4O4PtFl7+O4nQVoQhK8CxYIg/NkPfylJ0q9/kEE+zDDaSUmSflAU/LeAa5Ik/UdBEH7r4ed/zS69rvrhdQD4C+DAQ+P0b4B2ds/hBgRBeOtnKV+tUqmQSgqpONaJLJtF19JA3OtHo9NhP3KQ6Oo6xYc60FS4CEzM0PDko2Q9PtYmpslvasCwt5lqmUgsHEZ/YA+h+SXyqisxVVchaVSUm00kd3ZIzC9R9dyTSNse4rksapMJTYGT6NIKZaeOobOYCc0tUPexZ5ACIbRGAxaHHVPnfkJLq9Q/8Qi5QAjrwX2I84tku/twHO5Ao1AiNxlIAUqtBo3dhrapjkZRJJtOo2lrxBIKY6mtQsyzU9bajKDVom6uo1HKEfX50bqKEfxB0pEoGpudgtpqMnI5osOGo6QY0W5BV1xIbn4RW3ERxr3NxLc9OGqr0bXUEx4YofGzHyM2OEZhZQWxeAxFQR6hqVkK29tQFOajsJgwB4IobWbSSiWRwQesXr1FLpUhsLlJwemjUFaCfGoeR2U5sa1tsnl2DDYritoK1CtryLNQ+9hZwveGIZWmpbWVlsYm5hcW2NnZ5tOf/gyTk5Ps7Oxw9OhRDh86jFwu8qUv/QmiQsGLz50hlYiRy2V54nQT6+4dXAVaTh4sR8hESCRSPHnEyeKqh6YKI+6dMGcPu5hbCnB2n4Xv3ciwnEgRTsLSqof2xgLO7NFxoXebhlItmSzsa67AZc0RTskJx+PUVxZxvEnH/HqEx443IMoEHDYzZm0Go1pBXbGSnUAFpTYFbm+KjqYCWiu0uD1Rip1m9leKmHRysrkKEERsNomz1izBhJz2KjVev5rRmQ3ml+X4QnGK8xzUluh5o8dHvtVJe4UCb6AEi06gwi6nyO5ibSdFXX6OrRonxTaRhhIVUq6eeCrL4ToNPeN+ip1mTjWp6ZlI0lzlYH+Dg1Ash9OmJRKNo1HLqSw0YDdkaalx0lCiptSh5L2BAC8+UkcmJ9BRrSSZqkSjBE8gRke9g5pCJZlMMUqFgs5mNbcmUhxrMrHslagutZLKCJxu0aFWigxPaumoELj2IMyzx1wolXLiaQUWQ44lb5p4MoMoyjnWYsVuVPBat5ezJz8kpU7pA4fRPD/KsxEE4Sq7ysk/jP+T3X3x99ndC38f+CPgl9hNvvxh/KThrCfYLcB5it2zmp8K/39ioz0FfN8zeQl4+gfef13axV3ALAhCAfAI8L4kSb6HBuZ94PzPelHpuUXsh9pR1lax+OpbaJwOrMcOExudwNXSRMIfwD80ijyRRFVWQjQUoqimmpyUI7WxBUoF+vbW3bBPKk3Ro6fJbGyRnJ5DXVsJBXmkozG0eXaim9ukN3dwPXaW+OQsep0ea3M9W30DZCUJbZ6D2I4XhVJJWqMm6fWRXV5DX1dNVqMmsb5Bzr1F5UefZvQrX0fbUo+QTCFGopQ9+SgZg46pl1/BHwiQVChw3+5FW1uBpa0Jz9AocpuFZCJOascDcjmmIwdZeu0dBLsVnA6W33gHTWMdltZGguPT2MtdRKbnkSSJ3NoGVS8+R3hgBGljm8IzJ5n73luY9rWi0OmgII+Q34/9+GEC03OEV9fJO9BOYmYB761eTAf3kUtnkDa32fPr/xy5TIZMJqP+Mx8jvbBMZGAE08G9gITz7Akia26me+6yfbuX+s+9SCaRYPm9Gxz5yGNU7G3ly3/2nzCZzfgCfrxeH2VlLvr7+zh69CgvvvgiV69dRa/XU1frwlVeyde/8y7HD1YQj8Xo6p+mc38V/nCGSzcm6GgwoZZnuHh7ns8+3cTIXIi51QS1ZWbC8RTfubrMkUYDp9vzEUU5e1vKSaUlQrEMGo2ek212JtfS6JQSdaV64tEI7p0Idn2W8aUAc1sSJ1rNRBMSaqXIoUY7KztJXr25Tme9nrHlMKX5eh4/kMfd6QjbEZFPnymgeyrBwHSAaqfA/PImG1tb6DUC8USCWyM+XPkW9jWWUFpk53c+1cDcRorptTh5FjWPttu4MRqhPF/L6laUe9NRWl1KCs1Z3un38cIxJ5MrYUYXApTYwKrJsbKTIphQ0lym5ergNnqtmuePOumfDuEqtNEz5mF6Pc3//GQFgaSSiXWJT5wuYmA2ymvdXtoqdOyp1OEJS7w/5ONAtYrrfUt89ryLpc0kG/4MGo2KZCJK16iH6nwZRQ4NZFP4g3GePWzhnf4wt0Z8PHUkn+/d2aTeZaSiQMvfvDXFuidOJCXy1AEzhTYdv/Kki3tTYa4Oeqkt0aPT/PwZaRISqVz2A10/dixJOiNJUtPfcV2QJGlLkqTsQ7bYV9iVb4ZdT6bkB4YpBtw/PPaPmdcDvAr8qSRJL/3w9UHH+bA8Gwm4IgiCBPzlQ1fRKUnSBoAkSRs/oG1dBKz+QN/vu4F/3/ufGRbn54lHo0SHxsiKAv71DXQOG7lAiK3lZfQ2G6JczmL/IBXte5D1PyARjbK1sIS93MX6/SFUej150ShbM/MotVqSvgArD8bQWc1wp5ftlRVyyRTW/mGy6TTBzS3yBYh5vCRSKbg3SNjrQyPK2bjVQyYSIeLx4trbxvLF9zHV1bB+bbcG2eiV61S072Xt8k1EuQLf4Airg8OU791DdGgMmUaFzmgkJ0E2EGRrehazwUBkc4fgjofgjofSulrmL15FkIsURKJkM2k27g9iMJvxr61TMD5DGgm1Tsfi0AjmfCdDf/plive1ERscxbO6iqjWYB6fJBYMEZ5bQK1SIabSbMzNYch3orJaiI9PER2dZG1iErXRiGpukcn3b1J3vJNgdx9e9xYagx7f/WE2hkcobmokOjVLJp5gu6cPrSBislgQcjmSk7MsjozgsuVx+ZXXKKmtxmgy4fV4mZmeQRQEHA47C4tL9PTeZWFhkVAowp986Y84eXw/a+tbvP3mTeKxMP5AkLv9fn7982cYn1zGF4jgsNg411nOH3+9j6fO6nnr9jLJeJzXrsHIxCoquYTNqMGgEbg34WNvlYHGJjPfve2lpkiNTKbi7qibg412rjwQmJgLUuQ0sxGQuHB7hsP7Krlyb40tTxKlUsb3bobQ6fRMLPpw5tmZXtjCYTGwshPHve0nkUyjUbnweAM8mIrS3lRKKJqkyGEhEAWlXM6F27OcOljH5a5Jjuyp4PKDBGqNlr96c5TOvRVcup/l/sQSgSILvlCCaCzN62IWARmrG36ujzsQkPjme/M80VlCTpL42sVVyotMaFQ2ekbmONph5tZkBplMxsDoEnKlSDar4M5EnIkZN0qlyM1xFRNzbrRaFUa9lpmNLEurm/hCSVJZOSqFjCv3d8jPs/Gd6ys4zGrqixRc6NmkI65gakMil5YIhWO8fdcLgpyuBxvsa65gftVPZbGNqDKLw6onnYyxtKNkK5RiYtmPSqdHp9Xw7u0JjuyrwKn7ENho/HyUOgVBKPj+/gk8A4w9fH4L+JYgCH/MLkGgmp+AQfZ9SJKUFQThI8Af/7Rr/LCMTackSe6HBuV9QRB+lNDE3+cGfmD3UBCELwJfBCgtLf3AiyyvrES7Mo9uTxNxr4+q00cRBBFjcx1JlQLP5DSO2mpaP/dx0qtutO2txK4G0VVWYD3ZiSwnoTLokaxmml98jpzHi661kXK5HCmXRdXcgMpoICnlEMtdOPe14P7Sl/EOPGBzbgF7Uz2Cq4hyixkpGEbX0Yr3Rhd2Zz6qxjqiPb3oAgHyTx4lPD2LzVWC7WQnXO8i7+PPkdvyUK/WkAV0e5rI5XIQjiIoFcS3PTjrq1HWVxGZWaDyzHGS/gBiYQG6zS1C27uVoisPtxN9ME4iEKTxc58gOTmDuXM/kZvdlDbWo6qvxr+2jmVvK6JGTX4ygUKhJBiOsOfXvoD/dg+a08fx3uii9NgRRKuFyNwi1soylBUuimJx5HI5ObsFo9OOcf8eBJmMClFOMpHAduIwdouVRDSCodzFyLVbNLzwNLl0moaqcuKhCAqVkuqWZgyigqNPP8ng1et84gu/TMzjpa6uDp1Gw/79+wmEw3g8Xlo6Ooj19tDV083xM+fJyaM89sSzlDrS+Hx23njnFrd7xxkanSOZTFKUb2V8Zh2vL8GVe5usrHtpqMrnudMuBClHVb7A6naSqkIVb17fIZlIEE3YmVnawOvXsrJtwB+KYtDmUayVcBiKCKXk+ENxvvB8G6s+6Gyx8JXvjaNMZvifni5jajnAUp6JaqdEsKWYEruCxlI9b0sC6VSak41KriR1pLLQVgpWQylbgQz1pToezAWoLjZTYk7S0VCAw6LhcJ2G9wb8/MZHm1n15hByac7sLcZqkBPOOCgyQSgpEgyFOdDopKFYji+oZsdvQ69TIZflKCu0UF9uJRWPUOOy0eaSYTUoeK07wr7mMnKSRCSaoLNOjcdvQRDlnGhUEY4WkUpndp9jaTY9RlyFCs7v1WPUlLLtT3KqUYmQNeP2JMiz6jHqNeypMlBiV/BGT4T66hLONCt5tdvPvqYyEvE4Tx8tRZCLeAIxfvWZavrmUjy6V0/3eACHWcPhagVLW3G+8FQ9Sx6JfMeHwEaTfm5stP9XEIS23RlZYldJE0mSxgVBeAWYADLAr/4kTLQfQo8gCP+Z3bP16Pdffv8M/cfhQwmjSZLkfnjfBt5g1+Xbehge4+H9+yVa/z438AO7h5Ik/ZUkSe2SJLU7HI6faK2CzUpibYPog3GMTfXI02kCEzMY9HryXaUosxJqp4NYKITvwRiGumrkrmL8Q6PoCgtIZdIkFlbQuYqJh8JsXe9Ct6eRtEbN1tWb6Pc0YW9vIzm7gPfuAE2//EkUqQyV+/Zg1utITs6hqS4nFg4T8/h2E0STCQIPxmj8+AsYyl0E7t2HcARVnp2t7j4MrQ1oHxootGqwGEmuufH0DaCqrcQ9PIppXwt5RzsJ9A8hptKoigvxTM+RXV6j5Knz5FdVEI/HCHX3Ed7YQmO1oLGakRUVEOgbQmMxI0MgPDBCwy9/mvjoJN47d9G1NpGIRtGrVLtssyMHGP/KS6RVCiyNtSQWltGqVTiPHGLhtbcxtLcRDYeJjE5S9tRjRIfHiXt8CFYT8rJiApMzYNCib6onveqm+fhR4tPzZFbcmCrLyN/TxM7cPIlQhLonz9Pz9kU0uRxVtTUsr6xgMhr5xCc+zjvvXEQQZHz2i1/g9VdeYWNjkyc+9Umy2SzRoI9nn32K0dkgY1MrPHX+AG9fGebJs62cP9FCOBqnsa6cP/m9j7HjS3LicBPHD1Tw+vVl1AoZdS4LJXkKLnRtcXJvEXazhrn1EL/+fAOlBQ40Kjm/+WITyzswspxmT4Wa8dktakuNmPUKDMoU/+mbAxTnGzh9uJzB+Sgz62nyzCq6x0M8cbiAmfU4yVQWi07kYK2av760Sm2piZI8LVdHIuyrVOPQZ1j3pFj1y/joiUIu9W3z+H47Hs82f/CNMRbXfCy5gwxNb5MTFDSUaemdDHCkTktlkY5tfxy3P8tjB/J57fYqg3NBzux1cH14m8m1NJ8+V8TKVozNkIwXTxXRNRFjeiVMXbGWeDKJSiHjRJOa/rk4RqOellI5D5aSaNRKHt1j4FvX3NyfS/J8pwUpm+bWg20qnQKnW7R0T8XJosKg1zG5GueLT5TzYCHCdjCNSa8ll8vxxt0gj7WbSWdymA0aWiuNbHjCzLtjqBQCZnWaN7o3sBjUfPpcMeOraVb9IpUFKhKJGF5/4Cf63/9ZQEIiJ32w6x80jyR9SpKkZkmSWiRJevIHvBwkSfr3kiRVSpJUK0nSu/+AaQ6zS6H+d+yeCf0R8Ic/sscP4Ofu2QiCoANkkiSFHz6fY3fxbwGfAf7jw/uFh13eYpdl8R12CQLBh2G2y8D/LQiC5WG7c8Bv/yzXmkqlyG57WB0axZCfh2dqho27/aj1OoobG1gceICjvBTFwAhqpYqFO73UHTqAApi5dYeGI53IUlnci0sY7g6iVqlZmHiAQqkkkU7iXV3H3DuAXKNia3kVrVZD0r3J5sIS2UyadDyOo6EOfTSKqtLF5vXblL/wFCGfn8TSMrlQGJ1ez+bULDZXKalAgE33BulIFEmAjaFRnO2tONqaCT1MLI1lJII+P6lVNzIJFu/dp+bQQTYv38C/5sbpKiXyYBwhz44skyOezrA8PIJL2v1lIiIwefM2VR3tLA6NoHfaSd3swre8gijKUTkdhJJJkmvrFCvk7CyvIckE9KXFRMcmWZ2YQGM0Ia6tk4hESY5MIhcENmbnUVeWIWXShO/04HrqMRAERv/8b6l68jzpSIStwWFkmSwmq4VoKIRtcARJlCETJCJeH6NvXsLrdmNTqLl15Spb29sYlCpWV1dxOvMYmZjgOy+/jKhQ0Nt3j0NaDRe7vkt9eSkX372KLa+Yrju3cJUUcP6RI+REBbfuTbO8ukrn/no2tnzMLbpZWwOlrIJUMsHUwhoqoRiZkGNwbBVbRxFb3iieQIIuvRq3N0w4HEem0DI2t4VeI/JGd5rldQ8zeRoyGT3l+ToG9QaK8k34onIGxtcgHaej1sKqJ4NMJnC+3cwffXeSimIT6ztKNjxhRhaCpDIyZpc3ebNXQakNbgz7sejh3cEU4UiSqfU07bUONoIinY1W8s0CF3vdWHQiA5MpYvEU3TNpkCS0Wh19Y2v0TBpY3wxw5GQJSBkMGjWbnhBv3VWgUasZGF9EqRDZ9oYYngryyTNlkIog6mSoFGrG5rYoztNSnGfhYn+AYCjO1biemVUPiXSGG3IFBr2Kq/cWCcdyCAott/rmObKvDI2YZmU7SV2pDqdZzp+9OkGty874/A77Gou5N5ehe3CB9sYSuqZkCEKODU+Y7lEv4USOvrEN1BoDc2tB1jxx2ir1vN0bQZKpmJpZ+lluDx8Y/0iqA/xYSJJ08h/S/8MIozmBN3YZzciBb0mS9J4gCP3AK4IgfB5YAV542P4Su7TnOXapz58DkCTJJwjC7wP9D9v9O0mSfqZBWaVSie3EYQLffp2Y34+zsRalSkXZ4+eQGXRUSRIJnw9lcx3hvmEclRXI66tQaLWo+u6j6Wgleu02rlNHEJUqcmolho0NjG2NSGsbWGIJtG2NKHRaVJtbREJhtAqR4uYGsvEkwZ0d4lvbJNY2SXg8eFfWsA+PkQ6GCHl8lD55HlGtxiVAWqPGt7RMcUM91vZWRKMRMZ2BTJZsILSrD5NOoyRH+ckjiHYryUiM4gPtKF1FxKfnqHjkFKJWi6akkPC9Aban5yg+e4LWQieRVTfqxloia26an32SbDhCQXUFxqJCDHubkd3oQikTUZcWkRsZx+TMQ9VchzGRIP/cCdLuDbKinMrjR8lk0mR2fOgKnMiryxA3t7CGwqhUKhKShGdpFfX1O6QzGZLxOKQzyOUiGrWaoroaUqEwZpuNws795HI5koEwGZ2B1o88wvCFdzFrtdSdOMLY1CQGQcDj9TE1PcPW1hYvfuYzfPfllzl3/jxVHe0k/it77xlkyXmdaT55vfflvffV1VXVVe19A2igGyAIECRoQIKkREmj4VBmpBW1WpmYUShmNSa0MpRIkQIIgiBcgwDaob2r6jJd3nt369b13pvcH63Z1Q8tBIU0WIqBJyLj3sybJ+6vPCe/c75z3nCYXbUVPHLqGG+88SYHD3SxtuHgzKlurt2b4d//4pMMj4yz5Qjy3ONtSASIx6Mc2p3P6xd9tNSXs7/VyE+u2/nq2TpiiSwGk4lQOMqBZh3XJgUaq4o40qJGoagiEErwVK+RogIjO/403c2FrDlCnDxYiz+U5khXPjveKBJEJjfc2HcCFOYbEHNZ4okkzxws4PxwhN72Ug61mvhgJMCJ7jLK8lVIyfLjq7M8fbgMZ1jKc8cryUnkFOcpaaqSsLgdRymT8flHaokmBcxmEYMqR6kVKvKUXB31U1dmxqbP8tThGkSplEAsw6nuAkaWw5zuNvJgMUShzcDj3UbuzYBEgGA4zvxGiNhSALW8nHA0waYzx/iKgjW7D5Vcytk9erSaBsgmqS2UYNMpuDogcKo7n/GVGE8fq8Gsk7PjzeAJRAiFNcQTUp490cCOP8UhswGpRORkm5Jstp5gJM7eOjnvD0r58mNV+KNSOkw58k1KZArobDbzW3+1Ri6T5quny1ErZWzIOv413cNH4mHN5t+INNo/gSAI/8c/dl0UxT/6SPafNHX+f/POpYt859oljLvbiCytoSnKJ7ftJJ1IkkwlMe7rQRAEwn2DKNQqND27Cd9/QNaoJ+rykIvGKD64F7nJgPvGXeKJJCUnDxMeGCGeSJB/9CCxsSkkFaWw4yLmcqPQ6TD2duIaGEYaT6FubSC1sEI6nsC0fw+BvkHSahWWlkZCQ2PI8q34pmYpO7Sf9LYTXe9uPDfvoa2vJev2kM7m0BYXEFtaJZfNIlco0e/rItw/TC6TxXiol/D9EQQBdL2duK7exnJoL/7b95GrlGTTKSzHDpJNp4kMjSFms5gP72P7/Q8w1FaTTqXQmE2k7Duk9Roc/UNUnD5JdG4RIZPFdvIwEqkU+08vYa6pRNPaiPPaHfSlRajqqok+GCcXTxJPp8g/egDv9buYDu0lfH+YbDaHcW8X0dFJFAiUP3KM6NgUuUwGZWkhCqkM19wiVccPgkTCzCtvsvfZp4iHI+RWt2lqaSLn9VNXU8vG1iZra+vEkgm+8LWvIpfLefXVV6ksLMa7Y6enux2PfZqejlq+9I0/4IXPPspjR9uYX9okEnBQUWLinSsLWPUC9WVqtjwpHDthEok4MiFLR7UWpVTkv702ye99pR2pRODaRBi5XEEkniGbSXN6bwGhSIK3rq/z7IlKxJzIolPAE87wudO1XOxzEgxF+dSJSuZWvdwfsdPZaEOSSzO/HiSTjLKrWkc0q6atQs3NyQAyqZQjrTp+cstFZR7o1Erevr3BNz9dj14j4+17Xk526Fmwp6jIk/KX7yzyqcOVvH1rnX/3dB35ZhXn+r080mHg3nyS460a/sfbS/zGZ+q4O5sikngoqxxLpHmwkiKSEOmslGL35fCEc5zcpeXSSAQRgdq8DK9d3+Kx3mJWnSkUchm9jQZC0TipnBJ/TMLRFiUXhvw4PBFOd1sYXY5is+jprVdzczJKLJlFIUmRSGapKzfTUKKgf8aPM5CjwChQaFFh9+forJRzbTKGVqPkeJuGt+55kZLm8Z48bk4G0cmzGPVqlhxJMjnordeSLTjGU1/41j/LX/xLmzorW5vF333rRx/p3l9s7PwX/df/agRB+I1/cKri4ZboWVEUv/pR7D8ZV/MhbGxsEHJ5UGxuo64qIzg8jtpoYGdri2QghEKhRCqT496yk0mmsEWiJLJpcOwg12nxrK1jy88jnskilUhxTs8iN+iQ67WkPV6kMimJYIjYrXtYaqpYG5ukaf9ewv0PkMQS7KyvU6FWEXG6CAWCKNVqUqk0a/fuI4nF0Wq0zF+5QdmudgKOHbSFeYQXV1CbjIy/9CqNhw6g1moYf/VNqna3E/H6SMTjVKqUrE1Moc+zIZuYYWNmFolEiiEeJycVGPuL72IsKSFgt2MqLETWN4RMJsMxM49MoyZz4x47K6skojEKqipYunwNqUKOuaSEXDZHeHMLz/IauWyG7A0pAI6lJRQ6Ddl0mu3pWQq1KrzXbuGcmMVYXkJez26W3/gptvYWErOL+B0ukok4WqsF99IydZ27Ca2s4ZhboOGRo5irKpj88TlEmQT36BTpaAzn9jaOxWWEnMjKwABV1ZX4nE7GR0Ypq6xgbGQElUbNhTffRq5QcPeDD1CfOM7K0ir3797g5PGD9I9uY7Pm4fQEOXd5lMnJOXrai1mxRxidXMSkU+BwmYknM1i1MD67gVqpJJ2T4PT40OsfDsXMouCDviUeOVDPyrqDdFbggVVPFinrzghTmyISCQyOryBRqHjnpopb96fpaq/mzpiPWCzG+raX/DwTw2PLWPVSHu3O48KAmy+frmRuM8r52wsc6qzi/HDu4Xy320t0tZQRiiS5+MBPnllPeZ6cv3p3lSMdhdyYTKKQy8gzCLTWlTAwF0CQqtGp1fzv3xvhWE8DP77pxOePcnUizuW7sxzrLqd/TkYOgUt3F2mrL2ZoWcmdB8v0tJZxfjjD+rafdDqDSVPMgY4yzJosH6x7SKcz6PUNqGQy1h0e9DotP+0LEU8L+AIR1rw2bo1scWLyi4xrAAAgAElEQVR/IxdHotweXuBgVx2CXMP41AoWs57+uTTL9igOdxhNSzlvvjlNZ3Mx0aSRpU0vKrkUibQIs07CwKQfmQRyEhU3BlfZ01pKJJ7iTI+VH17ZoLJplqc+Zh/yUGLg5+OFXhTF//oPzwVB+FMeljk+Ep8Emw+hvLwcw1IeMp2W4MoaYa8X744TrV6PodKEalcLMrUaSzQKYg7zkQO4Bh7g3bBjLcinsK0FeWkR2uJCQsurGNZtmFubCE3P4d20Yxubxru5iUSpJJHJUHnsEDKzCVVVOcmrt6lsbUHb00Hi2h3MpSVo93Qg3XGinltAhoCytpIqswGVycDKuQuo8ywUHz9MMp6g5ekzCNkcqpYGVP2DmPbtgb5BtDkTyl3NFAYevhVr25sxutzkQhHyujtAEIhvbVN2eC+qiRlUkoez1wRBoCiVRmbQkVEqaK49S2rDjmp3G3mBIEq9jkQsRuXjJ5ElU+SqK5DLZJi7OxAR0RUVErdvo2ltRNo3iL60FE1RPiqpnHgggBCJE9x2YNnVgqq5AWssTiqdQl5XRTUC+p7d7IxNsTYzh9ZkJL7jZnNpkfyaGix7Oli/eY+m44fRlxThX9uguqEBW1ERb3/vB/zGf/xNOru6UCmUKJVKOvfsIRaNIpdIqCi2kmfSsbKi4ZmnHiUeTzI/v8SBnkoaakt4/8INvvbpRtz+GOZHd+H2RjjZZeWPv9ePRyrQ3lxFJivy6WMlnLuj4BufKeGd66s8uU/H4kYJDVUWYqkcWr2BU4crWdkK4ArV01xvpjRPRzAhQZAIfPpUNfFUhrJCPYc7C3jt4jy7msv59IlKMtkcErKMrQVx+mP0z4bpbrJyuKcBrVLgVIeeufUgqcZ82qv1WE1NxBJpjrWq2HZn8YdiJNMZlHKRbz1bz52ZGHlWLYcbTVwc9iEIUvLNBg40KrkzK/K1J6pJ5gS6WytIZAR2VUiRSgUW1mx01+nY9qdorLByapcevVbO2305pFIJYiZOnl7KlB1O9+aztJ2krUxCnkGOxysyMW/ntz/fzIXhELuaK9DIUrx4pp5lZ5oze4wsrBnZU6NgZTtIWZGRjkoFiVSWSMKA1WKkuUgks7eGdAZOtatIJm1YTUoONKoYWUwAIvuazQwsxDjVU0pFoZ7BGQ/fPb/Ov3+6Gre26f8XP/LzUrP5R9Dwz5j6/Eka7UN459JF3syE8A08QGE0kPEF8G/YKdzfg6yoAN/1O1hPHCY+MkGuII/Eph21Uk4ikyXt81PyxCncN+5i2N1GbGIGaXUFabcXIRxBXlmGLJMlveNCQEAEDPu7cV+9jaDXYaipIDa7hJBvRaVWE1/fQtFUR2p+mZiYJa9zF+HZRaTpDNuzc9Q+++TDBksBFFIZuo4WIvcfkJDLEZRyog4n+U0NCDoN6fUtiCfIWEyoVUpS9h3UXe34b99HlEiw7N9D6P7DRlJDZxvJ2UV03buITs4iyeYepvQO9eIaHME7NUfFk48Snl1AJgpYD+/Fc+0OSCRYjjycJZdIp8g7uBcxmyXQ/wCFzUpi24H1+CESo5MoO1pYfvVttJVlWMtKiKxuYjmyF0EqJXRvCL3JSBLQq5QIiRSJZIKCjlaCG3ZyUikquYysP0jdYydYuXQdm1qLmM0gC0b5tW99i+9//2/5+i/+IhfPX+DMmTN856/+Crlczotf/xo//MH3UMgEPnX2Ed577wKQ5cxjx7ly5TKpVBQxFaKhQkPfqIPPPlbHa+dnqS2UI8gUjM05KCsyU1uiZs0RJ5EWONWTz+K6j9EZB7VlVtwRkSwKMtkkJ/YUc6nfyefPtnLx5hxOb5TnTjfi9EbY3ImRSufYcYd4+kQV1wd3qCqUkxMFNncSxOJRvP4YuxvzEHJgdwXprDfj8ESJxbOs78R4stfIW/e8FFpUSElTZNPg9sexGtWMr0SIJ9N0VCoYmPOx402yp62C3jolCxthLEYlb9/e4oVHK7DqZVwcjWPQSNnfoOTtez5cngAvni7nJ7fd7K630VYu585MDHIpaoq0JBIJfnRtg6PdVRxqUnFpyMXhNgvXpxOc7tByYSTCnloF18YC7G2yML+dAgTO7DGw7oxyezLAoVYLs9tZEvEEZ3st3JqO4QsneWafkR9d30Eqk/HcQSu+cJoHK2nUSilI5JSa0rjCUvbWK/kvP1lif4uVA80GLo/FAZGOShkzW1nqOh/92NNo5S1N4n98/SNpi/HN1p6f9TTaJP9ve4kUyONhrfzPP4r9JyubD8Ef8GG/dgVLWSkrt/uwVVfhWlvHVFxIbmWdmJghd/02olKB1ulma3iExv170SoULKyuo7rVj1yuYPx7L9N0YD9Sh5uFu300HTtMNhhhfWQMQSEj4QlgLC5A/mACuVLJxtgESqOeSDyGziki3duF/fptVFt2qj7zJOmFFRI+P6loBKUgIeLz4xsaBWBzdJyiulqiPh/JRAK9Voeoy8MzO4+lpgpJRkHE60Oj1WCqr8Fz9TY5qZTI3QFy8TgBlxu12UjOaiY0M0+e0UCqII/5H75O4b49LL57kZo9XQTvDOBdXUVh0BNaWSew6SAdDqNUKIjHY8SDYYzJFCmVksjGJtqZBaSZHI6FZRTrG+iLC3Ffv4MgkZD4e1mAxI6Lxek5VAY93Bskm8nit9tZ8fnofuZpbG1NePuHycll3P7O39FyaB9SmYzxwSEsNhvya3eY6r9Pc3UdIb8PvVTOm2+8jlaj5fe+/buUlpbww5dfZn19DUR47dVXWV5ZJxHxE4/FCEcebqT4/HM6vIEYWnmSMyc6+IM//Qlfe7aLQChGMhXjzetejhzsYsOTxe5xEk2Xc29gkeJ8I6JEhlQi4e6Eg1BKQTgU4uiBZrpbKvmTv77Nod467o9t8f71CXp219I34QYR3njvPruaSqirtPCn3x/g68+0k2/R8Ed/eZdH9pfjCYi4fFEC0Twm5zbRqhWs2H04PEmmln0IuQzXNCpc/hiLG25a64oZX3EQi2epq8zD4QmjkIhoVFrMejV2d5JsDkLxLKseEXcszY47yJUHXowGLaNzDmxGNYGImVg8hicY59Kwn7kVJ8UFFlb6fWy6AiQSWaIpGaIoEgrHiaVgfPXhp0Ylo8yY5ff/borullJmt1VMLDgosJpY3XRhNqo5dy+JVqvlwYydPKuJaCREVpRxbybApXtr7Goo4t0BP4ubPqqKDTxYjiIiYWTOjtWgQsxJGE6m6G4t5/xwmEg0Rp6xiKGlBHdHV2iuymfeYcYbiLJ+494/O9j8S/m4mjo/Js78g+8ZwCmKYuajGn8SbD4Es8mCqjAfUamk9NETxLYdND3zFGm7A9OhvWiiMeZe+jHmmkpkmSxFTfVo9uzCPzSGrawE08G9RLd3MK1vomprxD80SmlTI5pdzYjZLJm+QUr37iGxuY1Gr0Pd1U7k2h0KqiuRFeTjvX6bsEpNicWERqclEQwRGJsivLrBjs9H05c+i39hmdL9PRhLS1CVFaMzmYh5fVgO7WPj/AfsuNcorShBpdORkQokNuz4VtcJyOUIajXO1VW0RUWUPXoc/+1+Klqa0OxqIT4ygW9rC8ONewgqBZFAEJRyyjvakOu0GPZ0IEgkSOVytD0dqJJpUqkUmj0dZJdW8V27iWtohMjaJgq1Cm1LA8GJWRqeOEVofgnL0f0svvY2Mrmc6s88hVKnQ0ynSBc9HP1kbKpHUZiH4mYfMYMBr8fN8l/2E3S76XjyNL2//BXCG3Yyej1lPd2YdFqURYXs15+mUKYkF40jB5568km0Wi2//uu/jl6n4ytffoGXX34ZvV7P008/zXf/+s+RSfJ48Yuf4s1zl/hgdpHLN4bpG5ygrszIK+diODxRzt/bprK8CIW2mJ49lTz7RDfINMRicZ5/sh2ZSkck5OfgLguZTAaVeh/hWJbBkTAzy36yohKnJ0osmqCrp4jSIhtHeiqpKDbi9oQIHGjAqFNRV6zmjffdXL+/wY4n/DBwJ0Ue6S1irdxIoUXJ9f4oa1s+jnbvpqJMSlrQYNFK6axRIJEVEg7HON1l4AcX/dhMKp7sNXFhWIpEEKgoVDPrgM4GOb01Uh4sBFlc89BYZeX3X2zj6kSc0506cmIJKqWUk+0azvVl6WlRsr/JjEQiwaIVONVu5c/e9LO/rYADzVrO9fv5gxfbGVpJU2IWeP2qE4lEyqo9QE2Zhcc6jSzaYzRWWthbJ2VyEbIZkaf2mplei9DVWMCeaikDcwJ3x7fZ+1Q9x3rriCfSPNlrQKfTEQrH2F2t5cFihOdO1OEOZrg9uk5ZgZl9dXKyOSmLqypKbXLkviSfPdmA3ZfhaIuCy8MSKirrPnYfIsLHomfzMSEDtkRRTAqCcBR4RhCEl0VR/EgNTJ+k0T6E/5lGi9gdJOeXca6uU//l54msraPIgbyqnNjIBN7VdQq7dpFAJOX1YjRbiCUTqPPyic8uoN/fQ3hkDKVWA4X5CMEwiUAQbWU5qZUNpAJE4jG0jXUIXj/kW8k4XEhSabIaNZ6pGcxN9WhKiwneH0YmV6DZ1Uxscg5RFMk7cQjvtTtQlI/GbEbQakhOzyOKOZLxBFmtGnN9Dc6rt5FIpRSfPkFocIxsNoOpq5308joptRKVwUBwfpH844dwf3AT6/FDuK7cJJcTEZRypHI51t4uYts7ZFweFGYTiR0XsuICVGo1OZ2G4Ng0BvPDUTFiKEzxySP4hkZRlZWgREBVX03M62Xz/FUqjh4gHggQtjsp6NlNaHUdaSRO3vGDePuHiQWCmGsqycqkxBZWKGhuIO3xIRZYya3Z8TmdaG3Wh/LYs4sQidLzmadZePcydYXFPPrE49x+/wJ1dbVksznUahVjY+MYjQb8fj/1dXWopRFisSh9A2McPbwXs0nHtjOCw76CmPLj9oV48flHefXtW+xuqWBuNUBVZTGStJttb5aDvc389794ha985gCFeQb+5odXkJDj82dbee/mIqKgQRRFHjlQxdTCDluOEKcPlzM8tk4gLvDU8RpeenuMz50s441rGxzrtHL+xjyrjhin9tewr83GudvbBEIRDDoVRWY1dneUs0fLeendRUwGNc+dKOPyfSe+QJRnDheQTGUYng8SS0JVvpQPBrd5+nApfVMBTBoJrdV6rHopt6ZiBMJxlEolCtnD5sple5RNXxaDVsm2J0ZLuYpAXEpbhZIfX9/mcLuFibU4x3cZGVpO4w/G2FMrxxmS0lWj4uZEgOnVAEI2RUaQ8+IjpWjVUs4PR0Ai4XCjgpeubPGlU+U4/QkCcTmeUIa9dTIuD7upryygu0bBuX4/MomE7ho589sZ9Fo5rWVK3r7norbMTG+9mlszSQQxx94GBe8PBnB5gpzpseGPwYorx5M9eobmg2hVMrxRCRXtpz72NFppS6P4q69+/yPd+zsdB37W02hjPBx8XAlc5uHmgAZRFB//KPafrGw+BK/Hg3d0CJVWh8JgJOByE+4bJJ5OEU1nya2sQTZDOpkgtLGFQiph48EYpZ27sOxuw3HlFtZdrbgHhtm6P0Tp7nbkEgFhx43CZEJutRCYmEZjMSE15jPzyk9oOnUcybaLuctXaT58EHkqTcTlRm21IpUpkBUUsnz1Oo0WM0qDgfk7d1EolYTCEWJr65Qf2IvU4WTmxm1ajh4kk83ifTCOPJYg6PGhMRtw3hvAs7xKYWUFKZeXVDQKsTiq5gZi0QizP3iVvJpqYsPj+J0uBECqVqNUyPH0DaLTaFm4cZvGwweIJmKo1jaR19cgLq2xPT6JpL2FwOo6CoWC1NIamWiMlcvXqe/dg//OALF4jHDAj2dxGaVEys7kNLaCfFRZkcl7/QhKBRKtmsiiC5QKIlt2EqEIpqICMvEEap2WQDLJ9vIaxmgM6d0B1geGOfLUWZZv97OxtUHa7cVsMrK542B2dpaSkhI0GjXXrl2joqKcTCaD37tNbWUJc4srTE6MU15sJBJLc+3WEF9+/jSDQzto1Ap+9NYtpHI1L795F6XyodDY4NAgBfkFBIJxtra9zK1FmV2LoDUWMDU5yU9vbrKy5icQ3KKwwMz3XnPwza8cRKuW8Bev9PGNTzdzZWCbl94e5dHeAgRBoLvRwJ+98nDLt14jR56Lc2twA7NKyoVri5zY30ihWcfMWpr3b9txugOEInEuDmi4cm+GygID5+483Pl458EKvW2VjEZENrb9DC5YCEQTbLkSVORLkQpK3P4Y/mCUxc0NulpKuTYlQyqRc/7uAkf21JPJSfjb9xf56hN1+MNJVreDPHOogFMdcr57YYP9LWZ0eTK+89NlDnbXcWs2xY4vTiKZwqKBeBaujfmQSBXMr7kx6FRciCjZ8YSZ20qCRMbNoTVKC/RceiBjZStEdVk+AwsJNEqRoclNrMZaLvcv09VajsOfZnjGjlqt5kYsysRqhEc7LVwYjKDXatly+JjehumlHT53vJSFrShpUcbLlxY51F3LjtP9Tz3y/+o8lBj4+eizAXKiKGYEQfg08D9EUfy/BEEY/ajGnwSbD8FkNiNTq1F1teMZnaDtS58lvbqB7cBeonMLLN+8S9vXXyAzt4hYXEh6dR1zcSGmmioyLg+e9Q0MleXYdrciTSXRmC1IbHnMXrmFtbSYRCiMZ3UdpdeHpqQYa2Ul8qJC5FYLNR4vOUFA39FCQzZLNBZDlWfFN7dA4+c+A04XaZOBqqOHkSsUFDTXMv+jN5AX5ROdnKWwvRl1RysqEXamZ9A31VOukJMVBAytjShkMvKOHSRsdzBx7RblLY0ox2fwLyyQy+awHurF3z9E7ZOPk1ldx+l0kYlGKTt6kFw6RXk4hKykiPSoB8/GInUNNSQjEQqb6rEd2odGoyEei6NqbUASCZMvr0bZXI9WpyF1u4+Kw/vR5ecjNRmp1WgRZVLiwRC1vXvIxOIYq8rJq6hEUVGCyWoh7PVDRSkLP/gxFUo5piP7aNJpiYYjKNqbsG5sUb93DxqjAUkgRJk1j/auLu5dv0k6meR3fud/Qy6Xk06nKS8vZ2BwELt9m298/YtsOxz80lefQamA1sZyZhfWCERybLtj1NcW8JWvfIFUKoVMfgmlQspznzrGysoKTzx2iOHRWb71S58lm8vS0VrJ37x8ie7Ods4ca+D969OkUmlO7a/iD//7O1ztWwVgdc3BlXt6XN4Qy5tuFEIWRHC4AmgUArWVZdQXQCInY1+TmkAkwekDNZTlyxmf2SIRzbCv20RFUT2b7hRPHi3DvuOjtcZCb6MegMV1L0d3m7g5FqCnrYyn9pr56b00Pn+UYDRLNJliddNDc42N4iIL2WyOE60KNl0xnj1RTzonxaQCu9NAKpVieCNKLpfl6qiHEquWTYcXb6mSeEZOQ6WNQw1ytGoZ70V01JYpSKWSGOUqzu41M74aprKoilBCwB9O8NvPNzG4nObULhWBaBkGtYAvkuXTh8sR5FJayxW8fS9BR0M+XdUy/LFa8g1yguE43/5SO1ObGdrK5Vzq32TLZ+BMr5XLwwG+8lgFV0b8ONwhhuYCtJSr2HRn+ezxSlRqBdqCf96oqn8tfo5qNmlBEJ4HXgDO/v01+Uc1/lmSGPiZQyqVksykSYfCKGJxNEWFCAX5ZDbspJweas6cJrVhx+twIjp2EIvyKTq0l8jCMolEgrbPP0vG58c3Nol13x5yejVCMEjD4QNoDQbEdIr6zz1DYVUl6kyGmmfPEhqbIpNKITebyRl1JBZXESQSbIf2EhyZQJ5MoyspJCuTEJ6YxtzeTNjlJnh/lKKuToIzC+jKStBWlJMKhwn0DdLxq79AaGoWQQTbgR4io1OolCqC80tkN7ZoPXYYY3kZsnwbecXFlJ86ytZ7H6ArK0FhNZGMxTAbDFhqa0i7PPj6hig4dghCYaw2Kw2fPkt8aQ1Nng3rvh4Co5MIRj2Wgz3Ep+ZRC1KKHj2Gr2+AXDaHRq4gr6Od7JYDf/8Qxs42Ym4vaq0GWVE+uuaHkwq2pmdI251o2pqw7usitbJB9f4eJIkkkW0HotWM9cg+IkPjVHbvZvLqTTYmp9mzZw8ur4c3fvASv/nrv8bv/u63ee21n9DX10dvby/RaIzS8nKaW9pYXF6hvbmM3j0tjEysEQxFOXXiCB5vgIP799K9u52+gTH+7pW3ef7ZRwiGIoTDUfZ0VPP6W5d54mQXu9pqmFtyMDq5RGdLGalUih+eG+TJk80U2NS4fWHqawqoLFIhZmJ86/kuYsk0Oo2Ks/vLqClUUGTI0VZjxmJUY9WJ1JXpWNoKE09muDYa5NFuK46ASDKnoq5Ex4UBD92NJtqrlEwu+akusxGPp1hzJpha8vLkoVJeubTKkTYduWyGle0wJXlqvvVMNUvOHFveBJ97pJp0TopaqaC9XM7ISoqR1Qw9dRrc/gTrXvils1XMbotkJHr+4MU2kGqx+zL87pc7WHVmaK0y88WTJQwup3H745RYZJzYpWfTHeNYm4Y37+yQFVW0V8jxhRLkG6QYdQqKjTlGV5IY1AKzK35aShW0Vulw+qKcu+ugp17DiQ4zw4tR8nQyvKEccqWKPJOSQDjO3GaEX3mmBX9ExO1P4vBGuDub5JEuCyd7a5DIVDxYzfKpXiO760zMbsTIZT/+2omISC6X+0jHvwFeBPYB/1kUxVVBEKqAVz6q8SfB5kNIJhIP6wUT00g1GqI7TmLrG0yde490MokiGGL2vQtEPB5UzQ3E17aQm0xkpFLWb96FHTcaowHnxAzpmUUUoRhTP71IeMfJ5tw8IbeH5PQcC/f68Ozs4Lg3CEV5LP3oTZIKGWkRNoZHWRkbx3e7H5VWg31hgcDd+/gXlvE7nWzfuI1ndQ3fjhNZIoljbIJ0Mokm34bj5j307U3E1jZRW8ysjU8SujdELBRm6tYdIlvbqFsaEcQcyUSc8R/9hGgojCQQwjG/QGh+mfDIJKFEEkEhx9zZhmt4BJlShVQuw7e4TDSZILO2xcbIGIH1TRLj06zc6UOSZ0WmUBALBJCplEgkEkwHe9l69xKSfBsA0VAYmVRGfGYe7+YWjsVl5IkkvpEJxFCEdCqNY2aOcP8DUtMLTH9wFaEon4xCgX9wDHVFKf75JTw7DubuD7K6usrAa2+xtrjE9OQU9s1N3n//PB988AHBYIC//dvv4/F4uHrtKqfPnMFkK+SDy1fo6WwEoK2lmj/73nkqyoqIhkMMDQ+zvePklVffIhQMcPf+OPt62vmjP/krlpZWWFlZ4vrN+3z/lQvMzi3x/qUBxqY32LD72N52cnNghd2tFXz/9Xu88KkOhqfdTM1t0T/tZW7JwdySHXdEyo8vznB5YAuXL8nyhpe7Y3YuDIeRS1NcGwth1KuQSSVEwn6WN92EE1IW15y8f8/F/FqQyUUfanmWY10FDM/6uf7AyeRqDKc7wPhqipyYoX8mjEqapW8hjSiKjM67mLNnESQSHkytMr8tcuHuCk5PkKuTKRbWPcyuOHh/0Ev/+BrhcIzzQ0GW13dYWHdzayrM2raPFVeG0ZUEwXCMG1NR2iqUTK2G8IfiPFiD8QUnrmCSKxMJ7o0sE0oK3JxJ446pePXyDA5PnIUNF8s7CfoXEqhVCsbmXczYs1weCXJteItsNsOtoUUC4RTXJhPI5UpuPthmzQM2i5b/9NIYqSxo1ApuTMdZ2vJzf2IDfyDC9ekk16ZSKOQSfnrx+sfvRP5+6vNHOX7WEUVxRhTFb4qi+OO/P18VRfFP/ufvgiC89WH2n6TRPgSlSoWpvo65V1/HmF+AzWRA19xApUqF0mZBVV9L0cYmuoYaPIMjuCanUSoV2Hp2E9t2IJQVozYakU/NoOpqI5fNUu73oy4vpS4vj0Q4jGp3G2WBIAqLBWVDNaGVdURypF1ujI21JA065BYzlsP7cF2/S0F1FcZ93UgGHqC1WTB17cJotSIVJIT9forbWslKpTiv3ibq9eKbnsfQ1kzcvoOlpgZlezOGUBhbOIK+sZbN63dI+fzUf+FZNEoVAiCUFNDyqSfIuDxoO1pwvH2erS07gkZFJBQmsL1DKpHAubZB8+eeQVtaRJNaDRIBXVc7+oUlAlOzZCxmcpkM8333aVIqyAkC9rl51IX55MIRXMsrSBRy8mW1VJ8+RWrHiWg2EpmexXbsMJVSKRKbGYVajbyyDPX9IaJOF6I/gGttHfPkLOrCPIwlRShTGeoeOcbWux/QdfwomXQaSTrDl770ReLxOC+99DLt7e2o1RqCwRBvvPYaLqcLx+YGP3j1GhKphFQ6jcvtw+9zYDYoUSqLeebJY4RDPnQaKS01Rv7rn79OLunFqsvn9375ENMb8OzpVl55O8yaPcBXP3+Kv3vtKiqZiQNtRt67/gCfz8e5y3MMjs7TXpvHs0cKeUvMopFlaSmREIkUkUrneKxTi0gliUSSE21KEkk5/+31OYoLjAQCERY3AjRVF5IT0zyxv5zyMg0WnYH/8tIDnDYd8UQWqZAlGY9ypqsImViMUS3wYDoEosChZjV1xXLOB2Xs7ajhWLOCt/riVJXYOLlLRSJVSIFFTYUVTNpyttwxnugxo9FokQhZTrRpQBDQquVoJTE+daAUnV6gxCzwYC6GNxDlQYGW4jwtRp2aPE2Szvp8uquV6NUSYrEy8vUiuyrlrDtjNFXZaCkVqCyoxx0W2Vev4v6sn8piA4cb5UyuyRlfzNFSoSJxsJFwNMmJNhVXRqK0NZRypFnB+HKIr3+qjVBc4EiLknf645QUWqgosZJK5zjdqcMTTHB7WuT0icMfuw/JIZLKfOTdwf/W+dAGz09WNv8EsdV1Gs8+ji7PgjLfRmp2Acu+PUiicbx9gxSePIw8nUGaStPytS8gy4mEVtYoPnmE5OIqnoEhyh8/SWxxFf+dAayHD+AceICirhLTvm62L15FW19LRswRnJxFg0jr17+EQa0mteWgoHs3ykmBY4YAACAASURBVLJiAvNLaKxm9LtamP/hT1A21SEvLWLr4jV0Ha0IuRxarZb8gz3EF5bJqyqn+tRRLKWlZL1+dKUlFB0/SHJ+heTsEvkH95L1BsgvKqRofw+bF66iaW0iJZXguTeItrYKzCZS205MFjMF9bWoK8spKi+neHc75l0t1B85QGZrm9D6JkKehXQkSmh9i/KTh5Gns2ib6pHJ5DS/8DniXj+pTIa2zz5DKhojHQjT9MLn0Gq0GMpKUBTYyIQjZDbsNLzwOWIuNyG3G0NTPVGvn433LlF19lG0Oh0yiZTmLz2HmEqhKchHLVcibahh6ifvcPRXvsZ7r7+BXqfj+NkzfO97f8u5c+f4pV/6BmfOnOHcubf57HOf4cjRoxQVFrBv3yGeefpxvvDZp0jEwnS01tDZVovBqOWJk530DYyj1Sqxb7v50U8u8uwj1dTXlhJPyyjK15FJ+HG6Q6jUWp47283Nvln0GimhcBwAtzvIH359N7lMikPd9ZTk6ZjfSqBRqzjVZeXOdAitWsmJdjU3JmNoVAoe32Oiby6BVi1l/+4qqkttnNylY9+uCiCHQqVmf3seI/MB3u9z8M3n27Ga9exrVJNKZWmtsXJr3MXuGj1b7igVRRaqy/Mptmn46WCQ010Gcjm4MhrkQLORx/eYGFxMYtCriaUEBpeS7KlVcbrTSN9cHJVCIBxNcG3US2eVgmg8zU5YRm+zhaXtOHKZhOICM0c7Syi2KBhZTVJfpmfFJfLskWJmt3N8MBbm8W4T276HaaWR1TRne21cHfWxu0aNTZ9lzZXGF1fw7KFCvndhA51WzZdPVTK/GcOqzbG7Us7QUgJRoiabTZPN5tjyC+yu0SGIac7fd9JRraGjUo7TE2Jfo5LXbzqY2Mjy6f1m1Grlx+9ARMhmcx/p+DngQ4tTnwSbD2F9dRXP9CyRlTWkUinj3/k7sgoFSX+AuC+AQiEn4XQze+UGgk5LOhJHtD1shtQU5JFRKyEaR19cRHR9E6nRgOv+INFgkMz8CpmZRVxLy0Q2t1Aolazd7UNMZYg/mGBjagbvwjKZbSfKZJrF9y4iRmOERifJ5jKEVtbxLywTDQRxXbvNxvgUgUiE4J0BnKvrOBeWkMcSbI+MsXHzLmkgk0yRDATIIBJfXGbpyg2kghRlNE5gy052aRWtTE5gewf3jbtkpQKR+UU0Wi22g71Ex6aQa9VYezpZOfc+os1CTq3EOzSKpqKUnM2Mf3QcdXkp5iMH8N8ZQJRJ8Y9OkorH2X4wiujx4hifJCeIePuH8GzZia5vEuobYvH+EFKJBP+dAZKBII7FJQLj08hUCpzzS8Tnl/HPzKOrLENjs5LTa1l75wL+UJj00jrRUJi5W3dZde0wNDjE0OAg12/dwmixcvnKVWbm59nY3mZ5fYPz779PdWUVz3/+i1y50c9f/PUPePGzRzl2sIX//H/+gFNHO6mqKGZhaQ2X08nkxAT2rXUmF7yMT6/i8bi5cm+JSCTMb/+nV/B4PAyPrfDWu5eZnF5gy+HmV3/vNQrNMoYX4jicPuYWN8gJci7cWWZ0eoXzQyHC0STjsxuMbwosb/m5P77C8EKAYDjO2/fc7G9QEY5EuTASIhSJsemKMTm3yVu3trk/uozXH2ZqLUFNiYZ37jkptig53mHl7pQPrVpCvj7D9LKTIhP86etLVOVJCcdSLK67MeoUKGVZwrE0I3MOVtZ2uDkwTyqVon8+wcRagol5O0OTa2QzOQZnXGx60iSjQRAzbLpiSMU4f/zDaSxa0KllvN+3SSQSR6WQsLSxw+iCh7VtH/kmJXKZBJMqxctXHRxpVpFK54jFUwzOeFAJaa4+cLGy4eLKeJT1nQBr7gzBpJSrwzuUWAQmV0NcuLPE9OIWVXkC372wTn1Bjnf6XERSMgZndpjbTrOwI+D0hFlxShiaceAJxLk5nWTb6fnYfYiI+HOTRvuX8kka7UMoq6jAVlqK5eh+QnOLlHXvQldaSHB5ha3RcfIaa1AmYuTV1aCtqyXicBDf3CLgdOG51UcyFMG1uoa+sICt6RlK9nRj695NaseNrLQYWb6VBgnkEklklWU0nX2M5LYT/cnD5AWCqC0mNLtbCS2vUranE3lRAUIqjbWsjPzOdmR3BsjflYe6exfrf/Ydyp84iTo/jxqlkkQqibyuCn0whDHPikSnwj04jHd+HmtlJSWPHicVjSHYzMjLSrBsbSHkWVCVFiMfGSPv+CHiOy62hsfIZuxUyqTYZxdQ6DQUSaSkUikiy2uoDXo861uY+4eRZbL47Q7yR6cQBYGNiRkK2psoONCD/9Z9qtrbiMRiVJ06hs5iIqtRk5dIoKmtIh4MUrZvD/KSIgwVpbjv3GfX114g5/SQlAjUPnYSpULBxoUrSBUKTNEYQiqNa3GZ1i8/j8piIrSxSenBvUiicZRI2HvyOGI8SS6X4+Tp07z51lu0dndx9Mkz/PHv/wEun5edUICL715ld0sJ56+NAeB0u7l87aFyRX//XSqK9BzurQNEHjtQyOrqOolEivZqI4Iosrbp50hXAZUlRrLpOAa9ilgkQr6uiqO7HsotOVxhCswm9tVK2XZoyeQ0nO7U8u79NGa9kiNNcnwBJWaDkqoCLYlMiplpF/1GA95QilAkyi+cqeXmVASTQcv+ZhVWsx5fMM6eWhXeYJxX3l2ms7kEuztMLJrgwUKIxlLdQ/GxTIZMJkcqI7LpSbO140UrzxGPq9AoJEhlMlprrZSU5JNKptnfqAZgYctAvlmJSppFrCigo1rN6Lyb4LabCmse6ZyM9vpCWivUZLI5pBIJOpWAL5KhpjSffLMax5CHVDKNVGLDFUwzv+rhrlZKMBKnKM9ITYmOREokFPWzr8lGIJri155rYskFpRYp0ViSydU4x3fbEGVaEHNkRAF/MEYqm88TPXouPvDTWJnHI7s0DM8HKS+2kE1F+a0vtHFrMszhZgV2me3jdyIfn1LnzwL/mHry/8MnK5sPQSKRoG5twHurH0ksTtHxQyRXN1FE4nT/5q9gsljQqTSUn32U9OIy1uZG1FI5BR3tGLraUWk11H3qcUSFjPpjh5EmkiRDIWxduwjNzePuH0TdWEtWKcdx6x6a6koMPbsJ3R9Ba9Ajxh6mYrL2HQqPHCC8vIZKImDd30tsfgWVXkcsGsV/f5iWL36OzOY20R03gsWI7WAvrpv3UBr1yKrKCYxMYtIb6Pjlr6PR6nDeH6bgYC+RlTX880vk9XYRXVwl5vFirKsmtr5FYnObioP7sFaUEYpEqHvsBOaCAqTlxdQeP4xJqyMXjND5H75B1B8gEYtT9dgJ0GpQ7Wqm7kAPRq0W/91BzAd7yGWzaDVarO3NhOYWCYxMUHrqGOl1OxJvkMIDvcRX1knFYqi0WjT5NrLBIFKvD2t7M+GlVZrOPIrKYkZuMZHyB+n+5i+S3bDjeTBOfmc7k+9don3/XtpPHePVv/4urR3tOF0uIuEw4XiUZ778Ai//zd9w9PPPYWusZ31tnW//3u9jyqtkX1cj2UyKlvoyDnTk43Js8Cuf72HHFaS72Uo4miCRzFBVbOBQm4XFrQSXB1z8ymeaGZ5y4QtEKcpTE4kmkSCgUEiIxTNcGtjhcJuBnChwZdRHb7ORrho1f/3eKgeb1bRXyJlai2EzqTnbY2J6M0MolqW5tpimIqgtNdFQYSOeymDRyUhlcpy/72Ffk46nDxXwwQMftyeC/OEvdFOcrycrSvnDr7eTQs3YapwCixpvXMm3v9TMlh+Wd1L8/lfa0er19DTaEKRKTveWEIxLsGol7K5SMLAQ5/1BH4/3Wokmc8RyStTyLDOrIQ7sKqG9zkYoLtJcaeK5IwVcn4yhkEloqyvAbLFiNZtQyESy2RwneyuxmQ1012pAouDbL7QiU2mx5hVQXqhBIpHiCmV4+kgVcVGNoNBTbNOSTCa5PuHjt77QgjcKq44Y1fkyCg05xhb9tNbYKMtX4g4kKLZq2ddkYNEexxuTYdApiWZV5JsUnO7Sc2U0+k887f9rEPn52SDwEfjtD/vxk2DzIfh8PuKTs+wsLeGxb2O/cpOV0QmCPi/e2/93e28aJcd1HWh+L/e9srL2FUABqBX7vhOgaJKSSJGmFkutlt225sjukabdPeMzbY1mut1qz2m3PTOy3S3bYstsk7QkkpK4gCRAECCxEkuhgNr3fa/Kqqzc18jMNz8yQZfYIFggVViK8Z0TpyJuvBfxblRm3Iz7btx7maHrLcwPDjN/9iJe9xwzp87i2LGVwt07GHv9BKIwj0DfEB1Hj5OKJ8DpYOSVN4nF48QTCu6uXpTBEUxGI57+foKNzcTae/BNTjF0vYVANMrUO+ewbqgjNu9B77AzOTCEMjrBxJUm4kYD4XCQyfYOkskk0VAE3/VWdLlOgo3NTHb3MT8xhb9/iOnOHhKRMOErzVhsNqauXic+PYuxeg3ui5cxFxVirFvH6Jsnyd+2me5fHCU8NU3S7SHuD+DuHUCZmUMYDXQ99yKpeIKBpmaioRDxjh6CgQBzk5NoPX6m2zsZee0YSauZ/itNhDwLzF6+ylTfAIrVxFxXL1EE053dLFy4wtD1FhZmZwm2dRLXauj6+58SCIcYf/s0o53dTA+NEL7SzFh7J3HPAmazmfaXXiMejRCfdhMLhTFE4+SUlTLd2sHM0Aij7Z00XbxEW0srj335i7z++utIrYafPvPf6Rsdob2/l6lUgiuXr3DhvQuY7Xn85Y/f5FJjG2VlRfyr7z1NKhmnsW2adDJBY7sbm1HyzM+vsa/Ohtmo5+SFbnoGJnjj7AgWEeLZV9uYm/Ny8kwL/SMz5Nh1HG90YzMZKMjR0zU0x+ikn+b+CD3TacZnfFzpTzDu1fPf3+hm1hPkZEuYwYkFZucDTLsX+OErvXi8QWKxBH/+fDu1pVpi0Th2q4WuYS+vXnBztrGPeCLOxf4EJ94bRKaTtAxFcbvnaelzMzARYHRqnqNXFphfCDAwOsfF/iTFuQZeOD1F11iYvjEPJ9/rZnwuTNeU5Ph7A4zPBmkZSeL2hOgfniEtjLx2YZwpbxK90cbxyxOMeZIcuxZkbsHHf/pJF16fj+PnupiYdBOLJ/jR6wNUFcDDW62cuO6nMNfA1R4fM54Q7b3jpJIpmgYV5sNaqooMBEIRTHoNp5vn8Uc0dA/Ocbk/To7NzHPHB5jywXxYTySuUJJn5UpfjMb+BNvXGlldYuOtxhm2rzMw5/GTige51OHmQucCQ2OznDx98Y7fQ6S8M240IcSXhRCdQoi0EGLHB/Z9VwgxIIToFUI8skj+aFY2IIT441scu10I0fZhyyJd377VGFU32i1wOp0kFYXqLz9JfGgUmediw7oqQgMj5B3ai9lmJR6LY6+vQTczy8DxkxgNBtLJFL4ZN4U7tlFwcC+6tETnzMFQlE/izDkMpkwBs5jXi2VTPeE5D+VbNmHKd2FpqEF55xyushKk2Uz/mXMYNBqky4mMx6ioWY+5porou2eIeH3YLDYsa1ZnMgDYrIw1t6LPyyNvxxZqpUAYtEQXfKz73ENYnDmYykoIT8/iLCrCUl3FxNmLREMR/O81otVoCczOkRgcxV6QT9GB3Rjy84i8G6KqtATrri0onT1YnU4s9TWsjcSIhcOYN9XhnPdgtVqx7dqCLC+m6/kXyd20gcqNG9AZ9dh2bKbjx88TmpjCtbmBtM+Pq6IC544t6HU6lHgc24Y6wpebMNgsuDbUgZRYTSYMNhu69WtYTwp9Tg6KRkPD5x8mlVCQBh1KKMyCew69zYKxuBBdWTG55aUUXW/FkJfLuxcu8Mujr6GzWvjn3/0jtj35eU4dP47iD/LZb/0udfZctu3YQSTiJ+Yd4bOHN9DXN8jqMif7t5Zg0CT5zLYc/valdkbHZzlqEFQVaVlfWUAqmWR3rY3hmRjTkzN87UgppGuJxJLUrnLy2skudm4o50IXjE7O8W++Uk+ew8DLFz08tKOMulU68h063rqgx2Qy8cg2OxI7rUMRxucU8pwOHtzqxGbS0D8Z4O2mOZr75tlYXczaTS4e32nBoKumwKnDYpA8sGMVJXlmNq4y4g3GsNttkE5ittj47HYbr17WsGWdk5J8DZX5Vq50TFGab2VvXQFuP6wvs7G+RIdeswqDTsvBegPBkIO8HCORcJi6qiJ2rdOj10jikVLMenh4cw7X+jVEoikObylgypti78YCasqMDEwEaezxE0vraOqcYuO6Qh7dmU8s6aM4v4LtNSb+n592sHZVAW+36hl3h3B7Anz5gXJOtwd4ZHcZ5YU68u2CULCcfDvUFBvIsxVR6hL8w5ttbK4u4512I0oyydxCgPc6fYzNhshzGFlbasNq1hOK66isvRu50e5YNFoH8BTwo8VCIUQ98FWgASgFTgkhqrO7fwj8BjABXBVCHJVSdt3k2DcScH47+/f57N+vk6mevCTU3Gi34NW3jvNi3I/n9AWS8Rhmm43cB/aSCIVwn7mIa2MdllUVuN8+g5JK4dq/h+CVJpBgbKhG6wtBfi7pBS/CFyCVl4POYELOe4gGAuiKCzE7c4h0D5DzwD78rR2klDiOoqJMCLQAY2UZwuPHunUj0bZOUBQSBgP2VWX4mjsxFeShX1NJoncQUV5CsH8Ip8tFRKawFBSQGhxGmI3Yt28meOka9r3bWTh9AUVKjMVFaGNxAuEw9opyEgPDaCqKic3O46xaRai7n/wHDxK53k4sFMRUvRbh8WKsrsJ3tQVTvgtdWSmJ3gFIKISVBAV7duC9cIXcg7sJNbagNxiIG7WExqcoPrQPf1ML2pIijBodhrWr8Z6+gLWsBF15MZ5LTZkw5pr1LJy7hFanwfXAPsJXrhMNR8g7vJ/AlWtohIbc/TsJN7VCZSmx3sFM9U6NhqrHHmH67TPsffLzTDS14pACrU5H4doqRvv6iMUTaJF0dXSwvq6Ww196isHj77B7104WhhoxGzXoNXFGx6YQUiHfoWVkbJq5+SCxaBiSCfZtLqGl38eeWhtOq5ZXLvvRijRGbZqU0HFoawnzviiKNOCeCzLjCfHoDidHL3nYuNpGaa6gb0phd7WZ0x0xIpEweVZJgctCy0iCQw0OTl1zs7Umn7pyIz+/4CGVVPjCnjwaBxLEk4J0Kk5dpYPusQBHthZwsTuEoqT43M5cfnHezZN7c3m7OUw6GSUQTlKRp6O80MrYfIoD9VZ++Z6Hx3c5OdcVIxJLQlrhM5sdvN0SIp2WfGG3gxMtET6z0UTTYIJYUks6nebhzWZeueTHpI1zaGMuvZNRAlGBVmegvkxD90Qck1HPtDdJiV2htMBC21gSky5FdZmFztEINRVWeiYVopEwZfl6HFYTswtRXDlW+scDmMwm1hVCPKWhocLIsesRUkqEh7Y4OX49iFmbYPs6B+e6Y6TTKTQaLY/tdPBOqx+XFUrybYzMSRaCUZ7ck8sbV7zsq7MScd35Sp3569fKz//Vn310Q+C5z3/lE+dGE0KcAf5IStmU3f4ugJTyP2W3TwB/km3+J1LKR27W7kOO/Z6Ucv9HyT4M9cnmFizMzzF95SIGvZ7pvkFMOQ4MZjNCq2W6pz+TWNMbQG+1MN3aQW5JMaFAgJDbQ6FGQzqpwMQEBUcOMf/eZXRjYexHDjI/OITFZsNeX0Pvsy+gz8sjee4iFouZnouN1B/YTyIYxOuep0SrJeYP0vPXf8PqIw9AJIr0B/D6fIy3dVBQWY7V68XvC8LwCKueehzf9TYWenpRSkuZvN5MUW01CYuJVCpJ+PwlTKVFjL1+AkdRESXr1qKPxBl4/RiVGxuITs0y19lD4a6tOPfvZPKNE9hr1uIeGCDa2k7tkQdIdA8weLGRqu1bELNzzI9PYbZbKH3kQcaPn6J051aiXh+z09MowRCr6muZ6Rsgr6ICa66LjuOn2HDkEEpHD6PNrVRZzRiSCpPtnQizCUtPL2NtbRRVVWG+3sZYTx8aBOL8ZUauNVN/5BD+1k4mO7rR9fZTuLkBEYkx3tKO1GhRkgrPfu8/sKauDu/EJHa7g6c2NRDxBzjwW1+k8e1TxCJRui5fxarV09/Vg+Kd4H/+3c8C8Fd/+wL1VTkUuBz85X87hm/Bw598aw/vXlP4wsEi/uqX/UQiMRJKAXqDAX8ggmchwIbacppaRxBaPTItOX99iLoKO4/sKubNRi+FLitDM3H6JlM8scsBgD8Uw2oxs6fBwoWeKF/c6+RHb4wyOu3F5XIy5QWnzUhj2zRv6fUkkinKCuw0lJt5/tQw8UQaoTVx5kovjx+upakvwIZKA//15SG+/huVuOxW/svLAzy2s5w//cduvnSwlOGpFHuqjTzz1ji5VoEnmCQYSWC32xif8RGJxnnXmMJpghfP+vitB0p4+s0xqoqMNHaHMBDnUocXBQM2q4W3L3ZzeHc17RMajp0d4MiOUsqdRhp7g1hMWlq7Z0BATClDouW18+MklSRlJTm8eWGUbfXlSAmdw1N4/GHyHAbm5i08uj2HkZkg0ZAPty/F0KSGtQVpXnx3Fqm18PhOB1cHE+xZb+Tn73mwm/VsXG3hb94Y56EtLhLaOP/n0y1UleVwpiVKSc3sHb+H3JizWSL5QojFv4SfllI+/QmHUAZcXrQ9kZUBjH9AvvsjjmUVQhyQUl4AEELsA6xLHYhqbG6B3eHEnpODbedWlFQaTSqFrr4GndlEnaKQlhLLlgbCJ89SumMLusoynH4/zvw8bLt3EGnvZrjxGjm5LsJTM4S8C1jy8gjNe/BOTlNuNuF3z7HhsUcxOnNYaGohb1Ulpm0bMfr9rNpQiGPfDqJeH4l4DIPRwEhnN3nr11F6aD8mk4V4JIzr0F6sCz7an3uR/M4eUskk0VCYqkN7sZpNpFJpjA4nqUicgYuXqXnkISq2bEIkk5g21xO63ERFXS35h/eTjCeYvt5KeHAUkU4xOzSMsJgx57mwW20Y165GZ7NQF4+TUhRyD+6By034xyaIDQwzNzCEs6wEfUE+NqsVe3k5kVic2q88iQhECEfC1DzxOVL+APZtG6kJh9HYrMSCIbb8/r8g2tWHY1MDdbEEsVAY87ZNGMcnceTlkX9wN9GFBXRF+RjLSkhfbSandi226vV4r7eQW15KwZHMj6yFgWE2f/Ex5htbMxkT2tuZW1jguT//f9nzW0/REAyRU1RIw6oqCopLuPDz5/ixLolWo6WnbwTvvJGDu6rZ2rCGZKKQrokE7b2T5Fo1kIqzb1MpB+ozechevqjgtBVwqNbA0KiFbWvMlOYZiMfKMerAG0pjM8G19mEK8nKYWwhw2mYimUrTNzyHzarHoC/jfNMoyWQFdeUWrGYD9eUGKgv0vHIpxPaNa/iNzWZ+8FIf49MLOIylVJfb8YXhc9ttzHlKcNkNVBVpOH5pkpl5P30TERJJyex8gJ7JOGZDJnN3SmqZ8iRY8IWorSwm164gtfkcrtcTiRYgZZpdtUYudIYZnfbTOFhIPK4w5tGwZZ2DykIrLYNBPr8zlzl/jM5COw/UGxmZiVJenMOOaiftw2HGZryYdhZSs6YQKSWH6s1YzTqOJuJIoWV1Xpp0qojHdzkBONUCdquRIjuca3OzdZ0do15LXBEY9TrsViNtQwEcViOJWIQf/HyKApcZvdbF6NQCLoeJ/mkd0UiU6QWFPXVOBmcV9m4qpqFCz5iu6I7fQ6SUpFJL9h7N3+rJRghxCii+ya7vSSlf+7BuNxsWN5+v/6iBfhN4RgiRk932Ab/3EX3+aSCqG+3DefWt4zx9/QqmHDvKxDSWXdvwnr6A68GDRNs6iSYSJHxeivbsQu90ELh8DY3BQNJuRW80EpmYIi3TpJISV0kh6RwbyvAkMhFHURTyDu/De+kaeocd4/p1RFvaSLqcBNo6WPX4I3haOnDWVRNoaiXvwQPMHDtFTtUqwjNu7Nu2kOobQlu7nkR3DxQVkFjwYdTpiM66sW7ZSHpqBqEkiZsMmK1WQvMe0mlJjsNGbN5Dzr6duE+cxWi1ollTjiGRIBIKk04lseblEfcHMLlcRHoHkAJyDu5l4Z1zGKoqM/MsCYXoxCSu1ZUk3PMoFhOWkmICze3Z9DabiHb2IXRa7Lu3MXf6PTQC8g7vx987gEmnI+kPEpn3YC4qwLa5gbmLjTg31JEYHEWWFqDxBlE8CyTSaZxbN5IaHCHmD0KOHVtZMb62LvIO7iHa0om2rBhdOk1kahZXZSnKlJtte/dSuGYVZ5//GWlAq9dhynWyvq6OwtWVXH3xZex6A1vXV1DiVGioWcVzP3uTSDhEOBjgG0808MsTHQQW/NRVmkgk0gxN+pFoeWK3kxPNPnavtxGIJDh+ZYZ//hsVnGyN8KWDBZxuDxGPRthV4+Bksw+zQUdCUTDqBTurreTZtLzTFkVJQX0ZLIRhaCaBTqfhN/e6OHp5gd3VFnqmUvhCColkikMNDsZmI5jMRqYXklQVpFCkkUA0zUJQocSpJZTQUlWQZj6koW8ijE7G2bzWSjBhYMaX5mCtiTeaAjy1z8mrFxfQ67RotTryrAnKCjPh1DOeGMJgwWVJY7cYGfOk2b9ey+tNAdLJBIc35jDp1zA+F+eBBjNdYxEiip69NUa6xuJMeBQe3WbnUm8MnU7DoXozr1zy8dS+XE61Rcm3JGjs8VFbaaWu0k4kpjAX0hKNhJj2SR7Z6uByf4JNq3SMzEsWvBHyc7ToDRbmgikchhRFLiODsylyjAkKcs20DgWpcAmcdiv90xG2Vtnon0kj0lHynRbMlXfejeZaVyUf+os/XVLbnz/19XvajbboHA4ytsN/O2NTo9FuQTQaIRaLMdfcjtTp0Gg0WLdtxn3yDB73PPq4Qmh6jkhnL6ErzfReuETA48Gcn0dybArF50cbV/APDhCemSXRP8xEewdzk1OY161m5p3z6EuLibvnWLhwkUgizVfwRwAAGG1JREFUwcKlJmLBEJHmDpLhCOPHThFLKUSbWhlp60CGImiEYPKtd0jptOgtJhStDu/1VuzVaxlvaiYcDJEKBBlvbmfB58dRvY5g/xDaUJiC7ZuJhqMEZueYPfseBrOR2aEhIv3DREYn0QfDFGzbTGp6FoIRDCVFKIX5TPb2E+nswVKQT//R4zDvRXq8THd1owRDhMIRdKEoIqEQCQaZGxkj0TNI3+VGFqZmCF1uJh4MMTM4TOBiE3p/iP53zjLd28dwcxuKL0CotRPnlo34r7WRNhuxFRURnpxGazCQt38X4ZZOkhoNyRw7w+feY/Z6O5F4nKt/+bcEYjFSySSe1k6KSospbagj6p7HOzfPhRd+gd5oZHpgEFdOLt2nzzPXP0jnu+fQOR30t7VxcP9+LjQOcfT4BUQqRm//CH6/l1+81cXkrI/xqXlCcR1TC3Ei8RQHG6wcveymONeExxemZTDApDvIO9e9lOck+NHRQSpyJWX5Rv7s+TYqXIJELEiO1cDndjg50x7i2FUve9YbKHYkefuaF/dCJPu0M8O7HTHMZhN/8dMOQtE07zUPYdan6ZtOEk0beelkH7PzPiIJDSebZojHIkRiSV4/P0w8KWgeTfPC272sK9ZxZIuLVy+4aag0EY2E+P5zHZS4DFzqS2C3mugdnsE9P8/AtEJTn4+xBcGVLjc6rYGZgOD1i2O0941zqj1Cfq6N6fkIPdOS8bk4eQ4D3nCaNy+OEEskOdsZ5ei5AUxGPZf7U5y9NsKkO8jFvgS5VsmPjo4wNz/PpFcQiibId1ppHVW4OpCpTTM4k2Bmzk/LmGDeF+PvXh0gmdISlzrevTZDLJFkaGyOQCRJIhaiq2+Md67NcL3Xg0ynefX8OC0DPoyaOCeuz6MkFVblaznfMsm15vY7fg9Jy0yAwFKWZeIo8FUhhDGbOHM90AhcBdYLIdYIIQxkggiO3upA2WP8M+A7wB8KIf6dEOLfLXUg972xWWr43sfBaDLjqFqNoijM9A0QbO8iOTrOwOWriEQcx4HdlNfVYtuzg2SOndzKSpzbN+MbGKTt1DukkOQd2U/RurXkHdqLrqyYyr27KNhQS9zrY6SxCTk1Q9DjwTs9g2tTA4Vr11BeW4N97w5cu7bhmZgkd9NGouk0ZXt2oKmqJO/wftAKZnp6CbV34RsaIjC/gPtyE0IIzBVlpHQ69DotMpUk0tlLxOfHPTZBtLUT/8gos8Mj2MrKyTm4l4oN9bh2b8M9Ps7MyBjhxha6zlxAp9cRbu8i1NtPwZpV2DbWE4/HKVpbhXn7ZpKRKFVbt6Jfu4b5/n4menqJRiLklpayemMD1j3bKKutwVW7HvPOLVgddlY11OHYtwOqKslfs5rc+lpqHz6CzmHDVFeD51orA1caSUy7iV5vxzsxyWRPL9HGFia6upnvG8Db1UPlxgbKHj6Ms64GZ1Ehpbu2odXqGO/qYWFsgmv/8DMS0SiukiL2feUpNEqK3QcPYrfZWLt+PbsffogDn30Em8lMXnEJz77wGoow8+w/vsLacjPf+5eH2FhbypMPrqbQbmD7xtVsXWchnU7T1T9N62iaxvYpook0Wr0Bi8lIbVURj+0twGI2MDA6R9eIj/6xIBurS6gqMdE/GaJ3ZI4LvQnS6TQ9Ix5aJwTto1GisRj7G3LR67RsqqngSL0epzHBqrJcHtxk4vFD1ditZg7UGii2JziwpYxt63ModMDgmBunzYhGCOrXl/KZjSY2lGtwWE1ItFwdThOKxjneFGR4KsihHVUcqLexr9qANxhj56Y1pNJpIpEIj+3KJ5VUqF9bxAMNeh7b4WBtuYtEIsXWKjMPNhjYWFPGvloT710fpH90nqZeDzWrM+6wVXmC8uIcHt5iYV+1jgd2rGZVsZ0DtSYq8nR0Dc+SY7Py2K4c6teVYNEnOXG+l2g0QlWRoDDPzoaach6o15Nj1bKtoYKDdQY0AnZsXMOudSYm3X5MuhRGgwG71cL22iKeOlBErl1H9epCnjpQwJYqJ9FIjDfOdDPlTfKFvUVsqK/+yO/8cnAnsj4LIX5TCDFBJivzm9knGKSUncBLQBfwFvBtKWUqW875O2SKoHUDL2Xb3orXgCfIlIQOL1qWNsb72Y0mhNACfSwK3wO+9iHhe8DHcKM1X8FssaLMzmHcXI/3/GXieh22shI0Hi/JhIKpZi1yapaIXou1II9oRw8xwOzKxQQEAgGcm+tJ9Azh2LODUGcXii9IOBImb3MD8b4RSKdJ5+ZgKyrA291Hwb6deM9chJICjDkONB4v5o11RPqGSHi82OprSE5ME08p2PJcpDw+gp4FnAf3Eu/pJ7bgJWfXdhIDgyh6PUaNFmJR4no9ZqOBlM9PwqDHaLYg/QEUmxWTTksqGCamKAibDaNeRyIWx2y3EZ+eIe2wY7Za8Q+PoLVYsK9bQ6CjG0vNWpidh1SKSCRC7s4thJpaSWm15GysI9rRS1yrIWddFd6WNnL37iDY2Ixj93b6X3iZ2m98Bf/Fq+Qe2sv8mYukLCaspUXEBkZx1FWTmJnNjCPfRXB6BovNTtjvw7VzK6GmVkRpMXa7jVBnL65dW7BFEyTdHmQ6xZ7HP0/La8c48sUnaTtzHpFOs/3IYfq6uogEgxSUFBMYHmfX9m10XT5F2DNEJBLioQPraetx456d53Pb7ZzrjLCr2szxSzNoRBqdRlBTpiOW1KMkFSxGAwNTEQ7UWznbGSaVTFBdZkGjMzEwEWBVoQGjwcC4J8XOKsHFPgUhNGyr0tEzmSYYVSjNkbhyzLQO+NhTY6VvVoNJE6FnPMpXj5RxojnIZzaaOdMZ5dGtVq72R5jwKGys0DLhSeJy2olFQlQWWbk6GGfLai2xBLSOxBAyyYObHfROCxb8YR7amsuxJj8Pb8vh6mCSZDJJJBrjM5sdXB9SaCjXMLkgmQ8kqC63MjCbJpWIUppnYnQ+iVmrsKrIxLgnTTgu2bJag9snGPMoPNhgpHUsxcxClMd3OTnTlWBTBVwdSBCPRdlcZWNgVhKNxSlz6bBbDMz6U8RicXbV2LnQFaGqUIDWSN9EkBKnjrICC9f6g7jsGtaXWmkbU6jITaPRGeibjLO3Wk/fjCQcCrK3zsnZrgjb1tkYmkni9kU5WG8nmnf4jrvRcqpWywP/99J+/B/7Z9+81yt1dkgpN3zc/vf7k80uYEBKOSSlTAAvkLG8vxbCoRDh4TGSOi3u8XEmXnkDS/VaUtEoMpVG5tgZvXad2dMXEAV5KMEwo0ffwrR2FXqHDSnTKGYjodFxhn/xBtrSEiITU4ycv4wu10Hu+rUMvfwm+opSYjoN/q5ekgkFc2kJPc/8BGNVJRank7FjpxD5LhKz80xcbASrBSUSZeJ6C+l4gpROx8j1FrQF+STm5pm63oLIsZPweplp7yI+O0faZmG6qwdlbg5pMRNe8GHKz8M/O8vA1WvEJiaQFjMzfX0o4TAGhw13cyuRySmSOi1zY+NERsdJ6rT4pmdIeH0o0Ri+aTfzFxvRFOQxNzJKMhxB8fjwzHuIeRZQ/EE8s7PEpmdIRiLE0mkGX3gF45pK4u55dCYjsWk3kVSS3n/4Gbo8J9aiQoZeewutK4dkIsHoxUakVoM0GJjt6Ea4nJjLS+l59gV0ZSUYjEb6XzuObXUFIqbQ/sYJXGtWUVBdzct//gOKK8rxTE1z/fx7lKxehXdujisnThH0+tDo9fS0t3HuzRfZsXktoYjCtnUW3jjZwsnzrZi1cSbnYvQNz/L8sUG2rDEyMeNDplPodDoudc7TMxrGqE0xNuPjhbOzbFplwuMN09QfxKxP4vEGud4fwKhNMTE9x09Oz1FXpsezsMAvzrmpzBPEwgGa+gLIVAqHGf7x3WnKXQKb1UQ4lmTCE8eiTfDD10ZYV6xlZDbB242TrCnUodMZaOz24DClKcg18/fHhllXrCMltfzi3CRrCrU0VFr4u6OjFDokNeUW/vrlQYpytLh9Cd5rHqYkR1JXbuIHPx8g3y7xRyXvNs8STyQz2bA9YdYUG+gb93K5dRhvSCGdStPYOY1VlyASh3ebpzGKODPeJM29c2hJMzYXZ2jUzcsXMjr7gjFSUpBvT3GpbYL2kSAGraR/bIE5fwJvMEkw4Od8hx+zLo1Ix2kdCqAoKczaJN0jQaLxFP5AgHPtXgyaNCZNlOdOTVGcAxWFFv7LayPk2wSxWJJ5f4wtq828ccVN/8Dor+vWcFusoAwCF4UQGz9u5/v9yeZLwKNSyv8pu/0NYLeU8jsfaPct4FsAlZWV20dHl/ahi8fjBINBAFKpFBqNBiEE6XQaIQRCCBKJBAAGg4F0Ok0qlUKv17/fR6vVIqUkFothNmfyTSUSCQwGw/+wrijK+30Xr39Y+0+ynk6n0WgyvzUikQgWi+WW47nVMfV6PUIIFEVBq9Wi0WhIZQtVabXaX7ku6XSaZDL5fv9kMolOp1vy+W73unzYNV183tu9Lrcaz41rsXg9mUyi0WjQaDTvu0turH/YdbldnZfy2fmwNh92XRav3/gsA0Sj0fc/y0s9r06nQwjxK8dJJBJotdr3PyNSyv/h8yKlRFGUj30tFp/PZDJhs9m4HT7pk41jzSq5+/vfW1LbU7/9+/f6k00XsA4YBuJkIt2klHLTUvrf76HPHxbW96uCTKz605Bxoy314EajEaPxLqQlV1FRWTGskPIBAJ/9JJ3vd2MzAVQs2i4Hpu7SWFRUVFR+hRvRaCsBKeUogBCiEDDdbv/7fc7mtsP3VFRUVO4YK6gstBDiC0KIfjJutLPACHB8qf3v6ycbKWVSCHEjfE8LPLOE8D0VFRWVO8KN4mkrhP8I7AFOSSm3CiGOAF9bauf72tgASCmPAcfu9jhUVFRUbsYnfYfmHkKRUnqEEBohhEZKeVoI8Z+X2vm+Nza3y7Vr1+aFELcTA5kP3Pl6sncXVeeVz6dNX/h4Oq/6JCeUckUZG58QwgacA34ihHCTecFzSXzqjI2UsuB22gshmu7lcMTlQNV55fNp0xfuls5yJRmbJ4AY8G/I1LLJAb6/1M6fOmOjoqKicqeQUpJcOdFoi1PTPHu7/VVjo6KiorKM3O9PNkKIIDcvP3DjpU7HUo6jGpuP5pMWL7ofUXVe+Xza9IW7oLOUmXdt7meklPZfx3FUY/MR/Boq5d13qDqvfD5t+sLd0nlFzdl8IlRjo6KiorKMqMYmg2psVFRUVJaJlRQg8Em539PVLBvLWZTtTiCEeEYI4RZCdCySuYQQJ4UQ/dm/uVm5EEL8dVbXNiHEtkV9fifbvl8I8TuL5NuFEO3ZPn8thLhZUtQ7ihCiQghxWgjRLYToFEL8YVa+YvUWQpiEEI1CiNaszv8hK18jhLiSHf+L2XRON6otvpgd/xUhxOpFx/puVt4rhHhkkfye+y4IIbRCiGYhxBvZ7XtS3xvv2Sx38bT7AdXY3ASRKcr2QzJZTuuBrwkh6u/uqG6bfwAe/YDsj4F3pJTrgXey25DRc312+Rbwt5C5SQP/HthNpnbQv79xo862+daifh88190gCfxvUso6Mmk1vp39v61kvePAg1LKzcAW4FEhxB7gPwM/yOrsBb6Zbf9NwCulXAf8INuO7HX6KtBARqe/yd7Q79Xvwh+SqTB5g3tUX7licqN9UlRjc3OWtSjbnUBKeQ5Y+ID4Cf4pPv5Z4MlF8udkhsuAUwhRAjwCnJRSLkgpvcBJMjezEsAhpbwkMwWRnlt0rLuGlHJaSnk9ux4kczMqYwXrnR17KLupzy4SeBD4RVb+QZ1vXItfAJ/JPp09AbwgpYxLKYeBATLfg3vuuyCEKAc+D/w4uy24R/WVqE82N1CNzc0pA8YXbU9kZfc7RVLKacjcmIHCrPzD9L2VfOIm8nuGrLtkK3CFFa539hd5C+AmYxgHAV+2zjz86jjf1y273w/kcfvX4m7yl8D/Dty4Q+dxr+p7h9xoQogvZ92oaSHEjkXy1UKIqBCiJbv83aJ9d9QlrBqbm7OkomwriA/T93bl9wQik7/pl8C/llIGbtX0JrL7Tm8pZUpKuYVMPaddQN3NmmX/3tc6CyEeA9xSymuLxTdpeo/oK+/Uk00H8BSZvGUfZFBKuSW7/MEi+R11CavG5uas1KJss1lXENm/7qz8w/S9lbz8JvK7jhBCT8bQ/ERK+XJWvOL1BpBS+oAzZOarnEKIG9Gmi8f5vm7Z/Tlk3K23ey3uFvuBLwghRsi4uB4k86RzT+p7IxptKcsnQUrZLaXsXWr7u+ESVo3NzVmpRdmOAjciq34HeG2R/Lez0Vl7AH/W3XQCeFgIkZudIH8YOJHdFxRC7Mk+ev/2omPdNbJj+XugW0r5/y3atWL1FkIUCCGc2XUz8BCZuarTwJeyzT6o841r8SXg3ezN5ijw1Wz01hoyv3Qbuce+C1LK70opy6WUq7NjeVdK+XXuUX3vkTmbNdnIvbNCiINZ2R13Cavv2dyElVCUTQjxM+AwkC+EmCATXfVnwEtCiG8CY8CXs82PAZ8jM0kaAX4XQEq5IIT4j2S+gADfl1LeCDr4l2Qi3sxkqvUtuWLfMrIf+AbQnp3DAPg/WNl6lwDPZqOoNMBLUso3hBBdwAtCiD8FmskYYbJ/nxdCDJD5hf9VACllpxDiJaCLTFTft6WUKYD75Lvwb7kX9b29dDX5QoimRdtPL856IIQ4BRTfpN/3pJQf9qNnGqjM1qHZDrwqhGjgLrhHhbzP8/aoqKio3Ktoiwqk6WtfXFLbyF/96NonLYEghDgD/JGUsulW+4FJ4LSUsjYr/xpwWEr5+5/k/LdCdaOpqKioLBN3242WdbNqs+tVZNyFQ3fDJawaGxUVFZXl4s6FPv9m1l2+F3hTCHEiu+sQ0CaEaCXzntEffMAl/GMybuRBltklrM7ZqKioqCwTdyo3mpTyFeCVm8h/SSY682Z9moANyzy091GNjYqKisqyIZGfguwAS0E1NioqKirLiVSNDahzNiqfUoQQ5ux7B9rb6PMdIcTvLue4VFYYmQiBpS0rHNXYqHxa+T3g5RvvViyRZ4B/tUzjUVmRSEgvcVnhqMZGZUUhhNgpMrVpTEIIazY54c0mQb9ONtRTCHE4+5TzkhCiTwjxZ0KIr4tMnZh2IcRaACllBBgRQuy6gyqp3M9IIJVc2rLCUedsVFYUUsqrQoijwJ+Secv/H6WUHYvbZNOQVEkpRxaJN5NJYLkADAE/llLuEpkCbP8L8K+z7ZqAg2RSm6iofATyU+EiWwqqsVFZiXyfTKqZGDd3e+UDvg/Irt4oQyCEGATezsrbgSOL2rmB2l/raFVWNmqAAKAaG5WViQuwkSkkZgLCH9gfzcoXE1+0nl60neZXvyembH8VlY9GfjrmY5aCOmejshJ5Gvi/gJ+QLQO8mGz1Ta0Q4oMGZylUk6kdoqKyNNRoNEA1NiorDCHEbwNJKeVPyWR73imEePAmTd8GDnyMU+wHTn2CIap82pDppS0rHDXrs8qnEiHEVuB/lVJ+Yzn7qHy6Ebk5UhzZu6S28pUTnzjr872MOmej8qlEStkshDgthNDexrs2+WTccyoqS0b9QZ9BNTYqn1qklM/cZvuTyzUWlRWKVEOfb6AaGxUVFZXlRDU2gGpsVFRUVJYR+amY/F8KqrFRUVFRWS5uJOJUUY2NioqKyvIhIXU7uV5XLqqxUVFRUVku1Ceb91GNjYqKispyos7ZAGoGARUVFZVlRN6RdDVCiL8QQvRky2u8IoRwLtr3XSHEgBCiVwjxyCL5o1nZgBDijz/RAJaAamxUVFRUlgvJnSqedhLYIKXcBPQB3wUQQtQDXwUagEeBvxFCaLMVan8IfBaoB76WbbtsqG40FRUVlWVD3pHCaFLKtxdtXga+lF1/AnhBShkHhoUQA8CN4n8DUsohACHEC9m2Xcs1RtXYqKioqCwXoegJLrTkL7G1SQjRtGj7aSnl0x/jrL8HvJhdLyNjfG4wkZUBjH9AvvtjnGvJqMZGRUVFZZmQUj766zqWEOIUUHyTXd+TUt4ocf49IEmmvAaAuNmwuPkUyrImcVONjYqKisp9gJTyoVvtF0L8DvAY8Bn5T9k/J4CKRc3Kgans+ofJlwU1QEBFRUXlPkcI8Sjwb4EvSCkji3YdBb4qhDAKIdYA64FGMmXT1wsh1gghDGSCCI4u5xjVJxsVFRWV+5//ChiBk0IIgMtSyj+QUnYKIV4iM/GfBL59o6SGEOI7wAlACzwjpexczgGqxdNUVFRUVJYd1Y2moqKiorLsqMZGRUVFRWXZUY2NioqKisqyoxobFRUVFZVlRzU2KioqKirLjmpsVFRUVFSWHdXYqKioqKgsO/8/Cr7KC08srKkAAAAASUVORK5CYII=\n",
+ "text/plain": [
+ ""
+ ]
+ },
+ "metadata": {
+ "needs_background": "light"
+ },
+ "output_type": "display_data"
+ }
+ ],
+ "source": [
+ "child.quick_plot(\n",
+ " \"land_surface__elevation\", edgecolors=\"k\", vmin=-200, vmax=200, cmap=\"BrBG_r\"\n",
+ ")"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.6.5"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}
diff --git a/lessons/pymt/subside.ipynb b/lessons/pymt/subside.ipynb
new file mode 100644
index 0000000..2756e91
--- /dev/null
+++ b/lessons/pymt/subside.ipynb
@@ -0,0 +1,289 @@
+{
+ "cells": [
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "## Flexural Subsidence\n",
+ "\n",
+ "This example explores how to use a BMI implementation using sedflux's subsidence model as an example.\n",
+ "\n",
+ "### Links\n",
+ "* [sedflux source code](https://github.com/mcflugen/sedflux): Look at the files that have *deltas* in their name.\n",
+ "* [sedflux description on CSDMS](https://csdms.colorado.edu/wiki/Model_help:Sedflux): Detailed information on the CEM model."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "### Interacting with the Subside BMI using Python"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Some magic that allows us to view images within the notebook."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import matplotlib.pyplot as plt\n",
+ "import numpy as np"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Import the `Subside` class, and instantiate it. In Python, a model with a BMI will have no arguments for its constructor. Note that although the class has been instantiated, it's not yet ready to be run. We'll get to that later!"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "import pymt.models\n",
+ "subside = pymt.models.Subside()"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Even though we can't run our subsidence model yet, we can still get some information about it. *Just don't try to run it.* Some things we can do with our model are get the names of the input variables."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.output_var_names"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.input_var_names"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "We can also get information about specific variables. Here we'll look at some info about lithospheric deflections. This is the main input of the Subside model. Notice that BMI components always use [CSDMS standard names](http://csdms.colorado.edu/wiki/CSDMS_Standard_Names). With that name we can get information about that variable and the grid that it is on."
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "OK. We're finally ready to run the model. Well not quite. First we initialize the model with the BMI **initialize** method. Normally we would pass it a string that represents the name of an input file. For this example we'll pass **None**, which tells Cem to use some defaults."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "config_file, config_folder = subside.setup()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.initialize(config_file, dir=config_folder)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.var[\"earth_material_load__pressure\"]"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.grid[0].node_shape"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Before running the model, let's set an input parameter - the overlying load."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "load = np.zeros((500, 500))\n",
+ "load[250, 250] = 1e3"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "The main output variable for this model is *deflection*. In this case, the CSDMS Standard Name is:\n",
+ "\n",
+ " \"lithosphere__increment_of_elevation\"\n",
+ "\n",
+ "First we find out which of Subside's grids contains deflection. "
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.var['lithosphere__increment_of_elevation']"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "With the *grid_id*, we can now get information about the grid. For instance, the number of dimension and the type of grid (structured, unstructured, etc.). This grid happens to be *uniform rectilinear*. If you were to look at the \"grid\" types for wave height and period, you would see that they aren't on grids at all but instead are scalars."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.grid[0]"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Because this grid is uniform rectilinear, it is described by a set of BMI methods that are only available for grids of this type. These methods include:\n",
+ "* get_grid_shape\n",
+ "* get_grid_spacing\n",
+ "* get_grid_origin"
+ ]
+ },
+ {
+ "cell_type": "markdown",
+ "metadata": {},
+ "source": [
+ "Allocate memory for the water depth grid and get the current values from `cem`."
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.set_value(\"earth_material_load__pressure\", load)"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "subside.update()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "dz = subside.get_value('lithosphere__increment_of_elevation')"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "plt.imshow(dz.reshape((500, 500)))"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "load[125, 125] = 2e3\n",
+ "subside.set_value(\"earth_material_load__pressure\", load)\n",
+ "subside.update()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": [
+ "dz = subside.get_value('lithosphere__increment_of_elevation')\n",
+ "plt.imshow(dz.reshape((500, 500)))\n",
+ "plt.colorbar()"
+ ]
+ },
+ {
+ "cell_type": "code",
+ "execution_count": null,
+ "metadata": {},
+ "outputs": [],
+ "source": []
+ }
+ ],
+ "metadata": {
+ "kernelspec": {
+ "display_name": "Python 3",
+ "language": "python",
+ "name": "python3"
+ },
+ "language_info": {
+ "codemirror_mode": {
+ "name": "ipython",
+ "version": 3
+ },
+ "file_extension": ".py",
+ "mimetype": "text/x-python",
+ "name": "python",
+ "nbconvert_exporter": "python",
+ "pygments_lexer": "ipython3",
+ "version": "3.8.2"
+ }
+ },
+ "nbformat": 4,
+ "nbformat_minor": 2
+}