You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
import eccrypto from 'eccrypto';
function removeLines(str: string): string {
return str.replace('\n', '');
}
function base64ToArrayBuffer(b64: string): Uint8Array {
const byteString = window.atob(b64);
const byteArray = new Uint8Array(byteString.length);
for (let i = 0; i < byteString.length; i++) {
byteArray[i] = byteString.charCodeAt(i);
}
return byteArray;
}
function pemToArrayBuffer(pem: string): Uint8Array {
const b64Lines = removeLines(pem);
const b64Prefix = b64Lines.replace('-----BEGIN PUBLIC KEY-----', '');
const b64Final = b64Prefix.replace('-----END PUBLIC KEY-----', '');
return base64ToArrayBuffer(b64Final);
}
describe('test', () => {
it('', async () => {
const pk = '-----BEGIN PUBLIC KEY-----\n' +
'MFYwEAYHKoZIzj0CAQYFK4EEAAoDQgAEW3yGReZI3dI37WZipbNcNunkHSX3gx5n\n' +
'NFC8V5HyWtHktU17mDH+0zWDVodNazTqqQFIUIuXz/e++o1JFEYh2A==\n' +
'-----END PUBLIC KEY-----';
const publicKeyAsArrayBuffer = pemToArrayBuffer(pk);
console.log('publicKeyAsArrayBuffer', publicKeyAsArrayBuffer);
try {
await eccrypto.encrypt(publicKeyAsArrayBuffer, Buffer.from('test', 'utf8'));
} catch (e) {
console.warn(e);
}
});
});
This code snippet is written as a unit test for sake of simplicity, the execution was also in the browser.
Basically, I am trying to transform pk pem to ArrayBuffer and encrypt a message, but getting Error: Bad public key
( btw public key in the example is legit)
Could someone suggest what needs to be done in order to be able to encrypt properly?
The text was updated successfully, but these errors were encountered:
@misko-frame I don't support this library but I was just reading in passing whilst working on another project. If I convert the base64 to bytes manually from the example you posted above I get a buffer of 88 bytes.
ecdsa public keys are 64 bytes long. I think the issue might actually be the initial input?
This is the code I am trying to run:
This code snippet is written as a unit test for sake of simplicity, the execution was also in the browser.
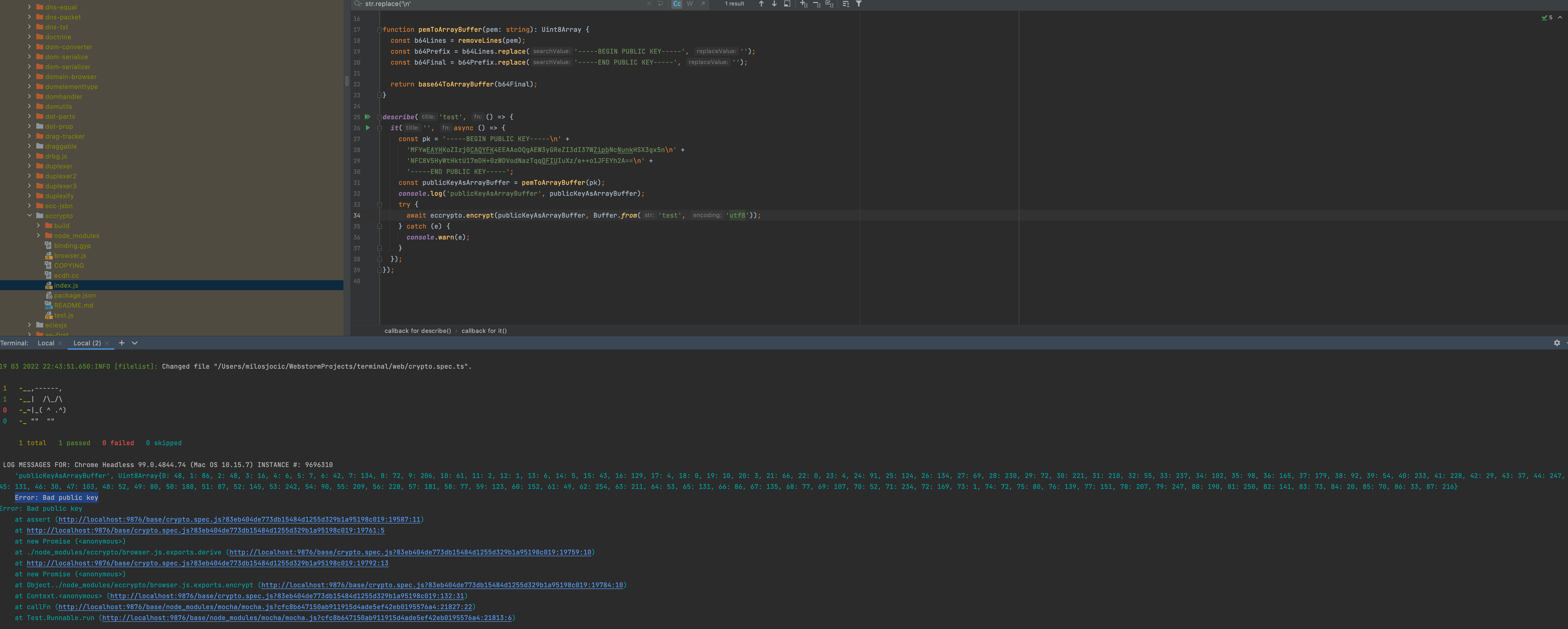
Basically, I am trying to transform pk pem to ArrayBuffer and encrypt a message, but getting
Error: Bad public key
( btw public key in the example is legit)
Could someone suggest what needs to be done in order to be able to encrypt properly?
The text was updated successfully, but these errors were encountered: