-
-
Notifications
You must be signed in to change notification settings - Fork 668
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
ImageIOFactory read tiff image get error pixel type. #2959
Comments
Confirmed that ITK's IO detects 8-bit image. Loading it in Slicer results in a mostly black image. Slightly updated code which works on my computer: #include <itkImageFileReader.h>
int
main(int, char**)
{
std::string filename = "tiff_bug_2.tif";
itk::ImageIOBase::Pointer imageIO = itk::ImageIOFactory::CreateImageIO(
filename.c_str(), itk::CommonEnums::IOFileMode::ReadMode);
if (imageIO.IsNull()) {
std::cout << "fail create Image io." << std::endl;
return false;
}
imageIO->SetFileName(filename);
imageIO->ReadImageInformation();
const auto pixelType = imageIO->GetComponentType();
std::cout << "image pixel type: " << pixelType << std::endl;
std::cout << "image pixel type: "
<< imageIO->GetComponentTypeAsString(pixelType) << std::endl;
std::cout << "image dim: " << imageIO->GetNumberOfDimensions() << std::endl;
return 0;
} |
My suggestion would be to distinguish better pixel and component type #include <itkImageFileReader.h>
int
main(int, char**argv)
{
std::string filename = argv[1];
itk::ImageIOBase::Pointer imageIO = itk::ImageIOFactory::CreateImageIO(
filename.c_str(), itk::CommonEnums::IOFileMode::ReadMode);
if (imageIO.IsNull()) {
std::cout << "fail create Image io." << std::endl;
return false;
}
imageIO->SetFileName(filename);
imageIO->ReadImageInformation();
const auto componentType = imageIO->GetComponentType();
std::cout << "image component type: " << componentType << std::endl;
std::cout << "image component type: "
<< imageIO->GetComponentTypeAsString(componentType) << std::endl;
const auto pixelType = imageIO->GetPixelType();
std::cout << "image pixel type: " << pixelType << std::endl;
std::cout << "image pixel type: "
<< imageIO->GetPixelTypeAsString(pixelType) << std::endl;
std::cout << "image dim: " << imageIO->GetNumberOfDimensions() << std::endl;
return 0;
} Output:
RGBA is suspicious, not sure about it. Gimp said scalar, 16-bit. But the images are everywhere mostly black. Not sure. |
Test data 1 all pixel is black. test data 2 need adjust the display gray range to show more: |
I found this issue may cause by image orientation info.
When I use ITK/Modules/IO/TIFF/src/itkTIFFReaderInternal.cxx Lines 194 to 207 in c779d09
ITK/Modules/IO/TIFF/src/itkTIFFImageIO.cxx Lines 507 to 513 in c779d09
Seem ITK only support read ORIENTATION_TOPLEFT and ORIENTATION_BOTLEFT tiff image? I'm not sure. If so, when use it read other not support Orientation image, need throw exception. ITK/Modules/IO/TIFF/src/itkTIFFImageIO.cxx Lines 1373 to 1376 in c779d09
|
Yes if (m_InternalImage->m_Orientation == ORIENTATION_TOPLEFT)
{
image = out + inc * row * width;
}
else // bottom left
{
image = out + inc * width * (height - (row + 1));
} The real problem that the logic is buggy. Even if the @Hconk i don't have much experience with TIFF metadata, if you have examples (small files are preferred) of images with unsupported orientations, please share. Thanks. if (TIFFGetField (tif, TIFFTAG_ORIENTATION, &orientation))
{
gboolean flip_horizontal = FALSE;
gboolean flip_vertical = FALSE;
switch (orientation)
{
case ORIENTATION_TOPLEFT:
break;
case ORIENTATION_TOPRIGHT:
flip_horizontal = TRUE;
break;
case ORIENTATION_BOTRIGHT:
flip_horizontal = TRUE;
flip_vertical = TRUE;
break;
case ORIENTATION_BOTLEFT:
flip_vertical = TRUE;
break;
default:
g_warning ("Orientation %d not handled yet!", orientation);
break;
} |
There are a great number of options for TIFF file. ITK implemented a generic function to read types and options commonly used with ITK. If the TIFF features in the file are not supported by the ITK generic function that the TIFF library function TIFFReadRGBAImageOriented, which produces 8-bit RGBA images. This may not be ideal for all input especially with a narrow range of intensities used in the higher bit depth image. This was reasonable "fallback" behavior IMHO. There have been number changes over the year, to expand capabilities and may have introduced bugs. It's not clear to me ( sorry I could spend more time and dig deeper ), if there is a genuine bug with the RGBA fallback behavior, or if this is a request to read the TIFF file with the native file pixel type. |
I believe simple fix would be to throw in the Edit: with "simple" fix several tests failed. |
Looked at test files (failed tests): cthead_oriet_tl.tif
cthead_oriet_tr.tif
cthead_oriet_bl.tif
cthead_oriet_br.tif
|
Hello @issakomi, Thank you for your efforts on this issue. It sounds like the expectations for behavior are different than what is implemented and may be consider it a bug OR it could be considered a new feature request. The pixel information reported by TIFFImageIO is not the layout of the pixels is the file but the the layout of the itk::Image which is supported by the ImageIO to load the data. When the TIFF file has option unsupported by For the above br/tr oriented images there is no pixel value loss or reduction in pixel depth, which is a good thing. Unfortunately, there is a 4x increate in memory size, a bad thing. However, for this case it is preferred that ITK reads the image as oppose to throwing an exception after the TIFFImageIO indicated it could read the image. Another feature not supported by the There is a forth coming PR, which adds a warning to at least inform when the bit depth is decreased, like the OP example. The best solution is to add the new feature which implements adding support the these additional orientation to the ReadGenericImage method. |
I have found another tiff file that doesn't work well with the IO. Gimp and other can open the file better. I guess it is scalar image, but it has "extra samples". I didn't look closer at the image. |
Description
I use Photoshop/ImageJ open the test image , both get real pixel type of 16bit. But ITK TIFFIO get UCHAR( 8bit ).
tiffinfo :
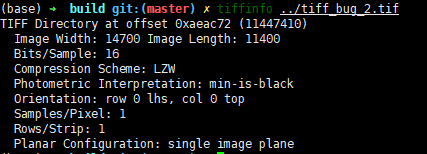
Fiji:
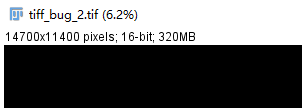
Steps to Reproduce
Expected behavior
Test image real pixel type is unsigned shot(16bit).
Actual behavior
Reproducibility
everytime.
Versions
ITK Version: 5.1.0/5.2.0/master latest
Environment
CMake: 3.21.1
Compiler: gcc4.8.5/vs2017
Additional Information
test data 1:
https://drive.google.com/file/d/1O3PCYt6nz1HKuvnZxtbI_U3fox582yAt/view?usp=sharing
sha256: 9201f1ac5888d6c3efbf3055fd6505c84e100383e4f5bb14d7237b1187f77186
test data 2:
https://drive.google.com/file/d/17I1ua6HOtIKV7jQId-10ioJCra6s6i9Z/view?usp=sharing
sha256: b693140a8fdd76765b5091732a0b7db902621b7873588819c783282d9598dd73
The text was updated successfully, but these errors were encountered: